-
-
Notifications
You must be signed in to change notification settings - Fork 376
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Police to pigui #4790
Police to pigui #4790
Conversation
I don't think you need to rename it, I use it with than name with a lot of views. What I'm not sure is if using In any case it's just a local variable for storing widget sizes, for consistency, and so they don't need to get recalculated every frame, etc.
Maybe we could use a slightly lighter shade of grey for the criminal record, but otherwise looks good. |
0c53f2b
to
5902d7f
Compare
Thanks for review! I've:
Example: |
@impaktor looking good, I like what you've done with the previous criminal record! A couple of tips / suggestions for you:
local countPlusPadding = 40 -- or however much space you want for the count and padding
ui.sameLine(countPlusPadding - ui.calcTextWidth(offenseCountText))
ui.text(offenseName)
Otherwise, this looks pretty good! I'll try to go over the code tomorrow and see about merging. As an aside @vakhoir can you do something about the need to load all of the newUI screens to have proper tab ordering? From what I'm looking at, if I start deleting some but not all of the newUI station view screens it'll mess up the pigui-to-newUI hosting because we rely on tab indicies to properly display the correct newUI screen. |
thanks for review and pointers, @Web-eWorks!
|
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
@impaktor here's a suggestion of how to make sure your text and offense counts align. This particular suggestion should be able to be automatically merged with no issues, but you might want to move that 40
to its own file-local variable.
In general, I'd recommend you avoid the use of tab characters for spacing and handle the alignment via ui.sameLine()
in conjunction with ui.calcTextSize()
.
EDIT: hrmm, I didn't see that you'd already addressed this particular suggestion; in theory this should work perfectly fine although I'll leave it up to you to find what looks best. There's a function ui.setColumnWidth()
that you can use to adjust the alignment of individual columns if that's the route you decide to go down.
f7bb379
to
6928999
Compare
Thanks for the code; but the way I read the source: However, I've only tested this on the resolution I use (1600x900), so it sounds reasonable that I should make use of some Well, the code path for ui.rescaleUI = function(val, baseResolution)
local rescaleVector = Vector2(pigui.screen_width / baseResolution.x, pigui.screen_height / baseResolution.y)
local rescaleFactor = math.min(rescaleVector.x, rescaleVector.y)
return val * rescaleFactor
end In summary: At the moment I don't quite understand what/how it is used, although the name of the function sounds like it's something useful. (also I squashed the commits to one. (One is legion, we are unity) So what remains is:
Status: |
I might need to rename the parameters, but I can't think of anything better. Or maybe I'll just add a comment explaining how it works. The idea is that you pass the current resolution you're working with, and the size of the widget has at that resolution, tand the function with rescale it to whatever the player's resolution is. The
I think you can skip it, but I'd leave more room than 2 digits. 4 just in case? |
You are in fact correct; this one's my bad. For some reason, even after reading the source, I was thinking that It looks like you've left room for three digits of offenses; although you might want to add just a little more space, it's totally optional and we will adjust it later if it ever becomes necessary. |
99487c1
to
f9b4b3b
Compare
OK, pushed final(?) changes, basicaly settled to what we said above, just use I also couldn't remove adding a small fix as a second commit, ping @vakhoir (or should I remove it?) If anyone cares, you might want to check my indentation style, for breaking long lines. |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
for k,v in pairs(past_crimes) do | ||
ui.textColored(gray, v.count) | ||
-- start second column at this position: | ||
ui.sameLine(55) |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
I'd recommend to move this to widgetSizes as something like crimeRecordColumnWidth = 55
. Then you can use this here as
ui.sameLine(55) | |
ui.sameLine(widgetSizes.crimeRecordColumnWidth) |
Since the whole widgetSizes table is passed to rescaleUI
, this will be automatically rescaled to the screen resolution.
if #utils.build_array(pairs(crimes)) > 0 then | ||
|
||
-- headline | ||
ui.dummy(Vector2(0, 10)) |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Same here. I'd move it to widgetSizes
.
@Web-eWorks, I've been wondering about something here. Does calling Vector2() from LUA call a constructor on the C++ side? If so do you reckon it would improve performance to just set a vector once on import and refer to it during rendering, rather then constructing it every frame? Or are any gains to small for it to matter?
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Yes and yes. Ideally, call local v = Vector2()
as few times as possible, and when you're updating the vector call v(x, y)
instead of v = Vector2(x, y)
to set the values without allocating a new vector object.
Overall gains will be incredibly tiny, considering that the entire pigui call tree runs in 1-2ms or so, but it is "best practice" for whatever that's worth.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
and when you're updating the vector call
v(x, y)
instead ofv = Vector2(x, y)
Oh, I didn't realize that was an option, sweet!
for k,v in pairs(crimes) do | ||
ui.text(v.count) | ||
-- start second column at this position: | ||
ui.sameLine(55) |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Same here
-- 1. If outstanding fines, show list & offer to pay | ||
outstanding_fines() | ||
|
||
ui.dummy(Vector2(0,50)) |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
And same here
@vakhoir I've fixed in accordance to your suggestions I've pushed it as a separate commit, so you can check that it looks OK. I'll squash it and rebase when you give thumbs up, so this can be merged afterwards. Thanks for suggestions |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Looks good!
Features are 1:1 with old behavior plus notification of which faction the police belongs to.
2f9b55b
to
05c492c
Compare
Introduction
This is my first pigui contribution. I hope to get some eyes on this, there's at least one "breaking issue" that needs fixing before merge (see below), that I haven't managed to figure out.
Other than that, it has all the same functionality as the original, which wasn't very flashy to begin with, since that was also something I barely managed to hack together.
Description
So I'm sure the data here can be presented better, (e.g. remove gray color for crime record, use some collapsing header or similar instead), but the question is how much more work should be done before this is merged.
My plan was to do another PR after this where I add some sort of dialogue button as well, so the player can ask for missing persons (needed for #4746), but if this PR looks OK, I could attack the ShipRepair screen next, which should be trivially similar to this.
I've made a little Debug-module that allows for adding crime and money to the player's record that's useful for testing this PR, if anyone wants to play (it also allows playing with hull damage, swap ships, adjust reputation and killcount). See Appendix-section below.
Know issues
When trying to pay fines higher than what the player has, the modal popup window saying "not enough money" crashes the game when clicking "OK". I'm not sure what the correct way of implementing this is. Pointers are most welcome, ping @vakhoir
widgetSizes
table is a copy-paste from lobby, that I haven't put that much thought into. Ideally I should rename it (and think through what it's doing)I'm not sure how pioneer-style compliant I am with colors, etc. Please point out if it's too uncceptably deviant.
edit also, I added a as a separate commit the faction name and police faction name. Not sure we want this, if we want it I'll add it to translation system and squash the commit
Screenshots
Compare below with original shown in #3254
Appendix: Debug-window
Drop into
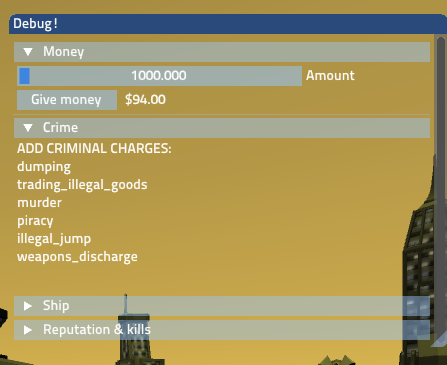
data/modules/
adds a window on worldview.