Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Fix VirtualizedList with maintainVisibleContentPosition (#35993)
Summary: `maintainVisibleContentPosition` is broken when using virtualization and the new content pushes visible content outside its "window". This can be reproduced in the example from this diff. When using a large page size it will always push visible content outside of the list "window" which will cause currently visible views to be unmounted so the implementation of `maintainVisibleContentPosition` can't adjust the content inset since the visible views no longer exist. The first illustration shows the working case, when the new content doesn't push visible content outside the window. The red box represents the window, all views outside the box are not mounted, which means the native implementation of `maintainVisibleContentPosition` has no way to know it exists. In that case the first visible view is #2, after new content is added #2 is still inside the window so there's not problem adjusting content offset to maintain position. As you can see Step 1 and 3 result in the same position for all initial views. The second illustation shows the broken case, when new content is added and pushes the first visible view outside the window. As you can see in step 2 the view #2 is no longer rendered so there's no way to maintain its position. #### Illustration 1 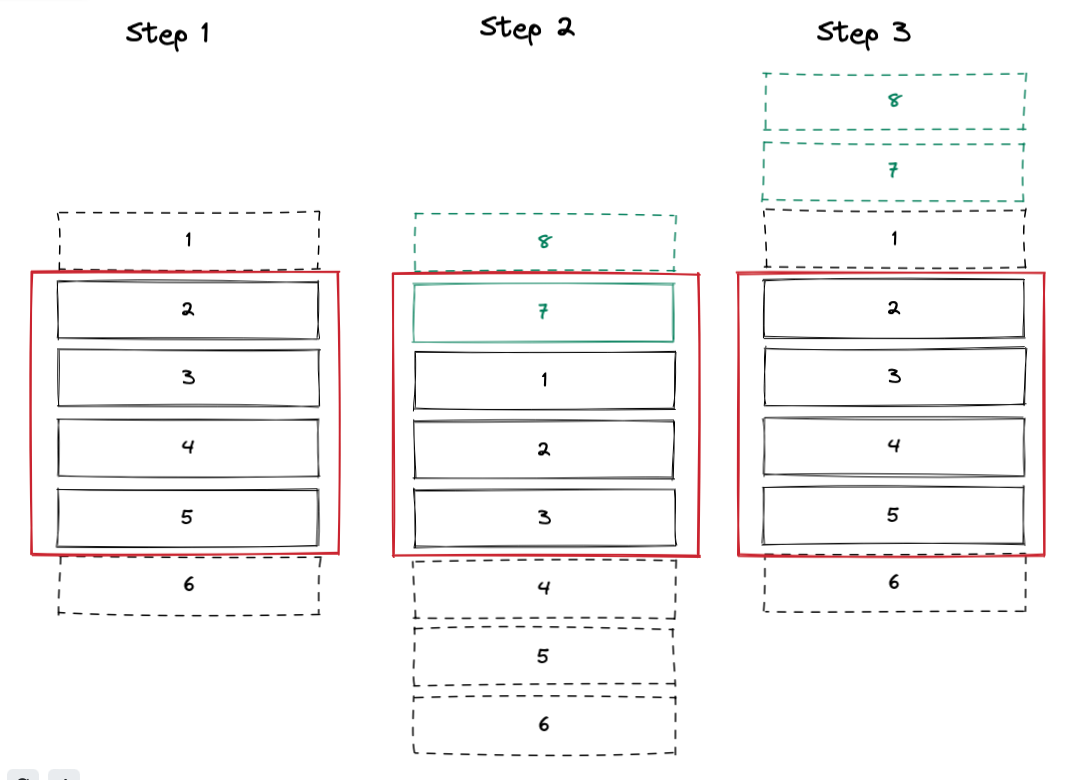 #### Illustration 2 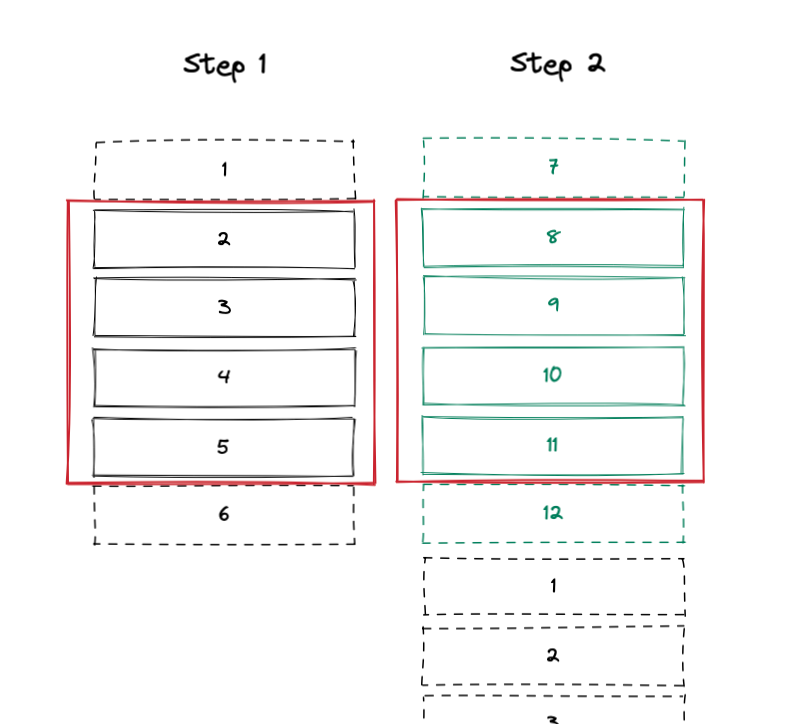 To fix `maintainVisibleContentPosition` when using `VirtualizedList` we need to make sure the visible items stay rendered when new items are added at the start of the list. In order to do that we need to do the following: - Detect new items that will cause content to be adjusted - Add cells to render mask so that previously visible cells stay rendered - Ignore certain updates while scroll metrics are invalid ### Detect new items that will cause content to be adjusted The goal here is to know that scroll position will be updated natively by the `maintainVisibleContentPosition` implementation. The problem is that the native code uses layout heuristics which are not easily available to JS to do so. In order to approximate the native heuristic we can assume that if new items are added at the start of the list, it will cause `maintainVisibleContentPosition` to be triggered. This simplifies JS logic a lot as we don't have to track visible items. In the worst case if for some reason our JS heuristic is wrong, it will cause extra cells to be rendered until the next scroll event, or content position will not be maintained (what happens all the time currently). I think this is a good compromise between complexity and accuracy. We need to find how many items have been added before the first one. To do that we save the key of the first item in state `firstItemKey`. When data changes we can find the index of `firstItemKey` in the new data and that will be the amount we need to adjust the window state by. Note that this means that keys need to be stable, and using index won't work. ### Add cells to render mask so that previously visible cells stay rendered Once we have the adjusted number we can save this in a new state value `maintainVisibleContentPositionAdjustment` and add the adjusted cells to the render mask. This state is then cleared when we receive updated scroll metrics, once the native implementation is done adding the new items and adjusting the content offset. This value is also only set when `maintainVisibleContentPosition` is set so this makes sure this maintains the currently behavior when that prop is not set. ### Ignore certain updates while scroll metrics are invalid While the `maintainVisibleContentPositionAdjustment` state is set we know that the current scroll metrics are invalid since they will be updated in the native `ScrollView` implementation. In that case we want to prevent certain code from running. One example is `onStartReached` that will be called incorrectly while we are waiting for updated scroll metrics. ## Changelog [General] [Fixed] - Fix VirtualizedList with maintainVisibleContentPosition Pull Request resolved: #35993 Test Plan: Added bidirectional paging to RN tester FlatList example. Note that for this to work RN tester need to be run using old architecture on iOS, to use new architecture it requires #35319 Using debug mode we can see that virtualization is still working properly, and content position is being maintained. https://user-images.githubusercontent.com/2677334/163294404-e2eeae5b-e079-4dba-8664-ad280c171ae6.mov Reviewed By: yungsters Differential Revision: D45294060 Pulled By: NickGerleman fbshipit-source-id: 8e5228318886aa75da6ae397f74d1801d40295e8
- Loading branch information