diff --git a/.gitignore b/.gitignore
index 54cf77d5..89f79069 100644
--- a/.gitignore
+++ b/.gitignore
@@ -1,4 +1,6 @@
downloads/
+downloading/
+uploadedTorrents/
storage.db.lock
storage.db
.torrent.bolt.db.lock
@@ -10,4 +12,5 @@ output.json
.idea/workspace.xml
.idea/vcs.xml
*.torrent
-boltbrowser.win64.exe
\ No newline at end of file
+boltbrowser.win64.exe
+logs/server.log
\ No newline at end of file
diff --git a/config.toml b/config.toml
index 013996f2..d5be0450 100644
--- a/config.toml
+++ b/config.toml
@@ -3,16 +3,16 @@
ServerPort = ":8000" #leave format as is it expects a string with colon
ServerAddr = "" #blank will bind to default IP address, usually fine to leave be
- LogLevel = "Info" # Options = Debug, Info, Warn, Error, Fatal, Panic
- LogOutput = "stdout" #Options = file, stdout #file will print it to logs/server.log
+ LogLevel = "Debug" # Options = Debug, Info, Warn, Error, Fatal, Panic
+ LogOutput = "file" #Options = file, stdout #file will print it to logs/server.log
SeedRatioStop = 1.50 #automatically stops the torrent after it reaches this seeding ratio
- DefaultMoveFolder = "downloads" #default path that a finished torrent is symlinked to after completion. Torrents added via RSS will default here
+ DefaultMoveFolder = "downloaded" #default path that a finished torrent is symlinked to after completion. Torrents added via RSS will default here
- [notifications]
+[notifications]
- PushBulletToken = "o.QW6G7F6FUOKXCUKmw948fBceCUn0msFi" #add your pushbullet api token here to notify of torrent completion to pushbullet
+ PushBulletToken = "o.QW6G7F6FUOKXCUKmw948fBceCUn0msFi" #add your pushbullet api token here to notify of torrent completion to pushbullet
[EncryptionPolicy]
@@ -23,9 +23,12 @@
[torrentClientConfig]
- DownloadDir = "downloads" #the full OR relative path where the torrent server stores in-progress torrents
+ DownloadDir = "downloading" #the full OR relative path where the torrent server stores in-progress torrents
Seed = true #boolean #seed after download
+
+ # Never send chunks to peers.
+ NoUpload = false #boolean
#The address to listen for new uTP and TCP bittorrent protocolconnections. DHT shares a UDP socket with uTP unless configured otherwise.
ListenAddr = "" #Leave Blank for default, syntax "HOST:PORT"
@@ -38,9 +41,6 @@
# Don't create a DHT.
NoDHT = false #boolean
- # Never send chunks to peers.
- NoUpload = false #boolean
-
# Events are data bytes sent in pieces. The burst must be large enough to fit a whole chunk.
UploadRateLimiter = "" #*rate.Limiter
diff --git a/engine/cronJobs.go b/engine/cronJobs.go
index 2c02c324..df87ebfb 100644
--- a/engine/cronJobs.go
+++ b/engine/cronJobs.go
@@ -22,6 +22,7 @@ func InitializeCronEngine() *cron.Cron {
//RefreshRSSCron refreshes all of the RSS feeds on an hourly basis
func RefreshRSSCron(c *cron.Cron, db *storm.DB, tclient *torrent.Client, torrentLocalStorage Storage.TorrentLocal, dataDir string) {
c.AddFunc("@hourly", func() {
+ torrentHashHistory := Storage.FetchHashHistory(db)
RSSFeedStore := Storage.FetchRSSFeeds(db)
singleRSSTorrent := Storage.SingleRSSTorrent{}
newFeedStore := Storage.RSSFeedStore{ID: RSSFeedStore.ID} //creating a new feed store just using old one to parse for new torrents
@@ -36,6 +37,12 @@ func RefreshRSSCron(c *cron.Cron, db *storm.DB, tclient *torrent.Client, torrent
singleRSSTorrent.Link = RSSTorrent.Link
singleRSSTorrent.Title = RSSTorrent.Title
singleRSSTorrent.PubDate = RSSTorrent.Published
+ for _, hash := range torrentHashHistory.HashList {
+ linkHash := singleRSSTorrent.Link[20:60] //cutting the infohash out of the link
+ if linkHash == hash {
+ Logger.WithFields(logrus.Fields{"Torrent": RSSTorrent.Title}).Warn("Torrent already added for this RSS item, skipping torrent")
+ }
+ }
clientTorrent, err := tclient.AddMagnet(RSSTorrent.Link)
if err != nil {
Logger.WithFields(logrus.Fields{"err": err, "Torrent": RSSTorrent.Title}).Warn("Unable to add torrent to torrent client!")
diff --git a/engine/doneTorrentActions.go b/engine/doneTorrentActions.go
index 9948567f..26cbddd4 100644
--- a/engine/doneTorrentActions.go
+++ b/engine/doneTorrentActions.go
@@ -1,6 +1,7 @@
package engine
import (
+ "fmt"
"io"
"os"
"path/filepath"
@@ -11,44 +12,77 @@ import (
Storage "github.com/deranjer/goTorrent/storage"
pushbullet "github.com/mitsuse/pushbullet-go"
"github.com/mitsuse/pushbullet-go/requests"
+ folderCopy "github.com/otiai10/copy"
"github.com/sirupsen/logrus"
)
//MoveAndLeaveSymlink takes the file from the default download dir and moves it to the user specified directory and then leaves a symlink behind.
func MoveAndLeaveSymlink(config FullClientSettings, singleTorrent *torrent.Torrent, db *storm.DB) {
+ Logger.WithFields(logrus.Fields{"Torrent Name": singleTorrent.Name()}).Error("Move and Create symlink started for torrent")
tStorage := Storage.FetchTorrentFromStorage(db, singleTorrent.InfoHash().String())
oldFilePath := filepath.Join(config.TorrentConfig.DataDir, singleTorrent.Name())
newFilePath := filepath.Join(tStorage.StoragePath, singleTorrent.Name())
+ _, err := os.Stat(tStorage.StoragePath)
+ if os.IsNotExist(err) {
+ err := os.MkdirAll(tStorage.StoragePath, 0644)
+ if err != nil {
+ Logger.WithFields(logrus.Fields{"New File Path": newFilePath, "error": err}).Error("Cannot create new directory")
+ }
+ }
+ oldFileInfo, err := os.Stat(oldFilePath)
+ if err != nil {
+ Logger.WithFields(logrus.Fields{"Old File info": oldFileInfo, "error": err}).Error("Cannot find the old file to copy/symlink!")
+ return
+ }
+
if oldFilePath != newFilePath {
if runtime.GOOS == "windows" { //TODO the windows symlink is broken on windows 10 creator edition, so doing a copy for now until Go 1.11
+ if oldFileInfo.IsDir() {
+ os.Mkdir(newFilePath, 0644)
+ folderCopy.Copy(oldFilePath, newFilePath) //copy the folder to the new location
+ notifyUser(tStorage, config, singleTorrent)
+ return
+ }
+
srcFile, err := os.Open(oldFilePath)
if err != nil {
Logger.WithFields(logrus.Fields{"Old File Path": oldFilePath, "error": err}).Error("Windows: Cannot open old file for copy")
return
}
- destFile, err := os.Create(newFilePath) // creating new file to copy old one to
+ defer srcFile.Close()
+ destFile, err := os.Create(newFilePath)
if err != nil {
Logger.WithFields(logrus.Fields{"New File Path": newFilePath, "error": err}).Error("Windows: Cannot open new file for copying into")
return
}
- _, err = io.Copy(srcFile, destFile)
+ defer destFile.Close()
+ bytesWritten, err := io.Copy(destFile, srcFile)
if err != nil {
Logger.WithFields(logrus.Fields{"Old File Path": oldFilePath, "New File Path": newFilePath, "error": err}).Error("Windows: Cannot copy old file into new")
return
}
- Logger.WithFields(logrus.Fields{"Old File Path": oldFilePath, "New File Path": newFilePath}).Info("Windows Torrent Copy Completed")
+ err = destFile.Sync()
+ if err != nil {
+ Logger.WithFields(logrus.Fields{"Old File Path": oldFilePath, "New File Path": newFilePath, "error": err}).Error("Windows: Error syncing new file to disk")
+ }
+ Logger.WithFields(logrus.Fields{"Old File Path": oldFilePath, "New File Path": newFilePath, "bytesWritten": bytesWritten}).Info("Windows Torrent Copy Completed")
+ notifyUser(tStorage, config, singleTorrent)
} else {
err := os.Symlink(oldFilePath, newFilePath) //For all other OS's create a symlink
if err != nil {
Logger.WithFields(logrus.Fields{"Old File Path": oldFilePath, "New File Path": newFilePath, "error": err}).Error("Error creating symlink")
return
}
+ notifyUser(tStorage, config, singleTorrent)
Logger.WithFields(logrus.Fields{"Old File Path": oldFilePath, "New File Path": newFilePath}).Info("Moving completed torrent")
}
}
tStorage.TorrentMoved = true
Storage.AddTorrentLocalStorage(db, tStorage) //Updating the fact that we moved the torrent
+}
+func notifyUser(tStorage Storage.TorrentLocal, config FullClientSettings, singleTorrent *torrent.Torrent) {
+ fmt.Println("Pushbullet token", config.PushBulletToken)
if config.PushBulletToken != "" {
pb := pushbullet.New(config.PushBulletToken)
n := requests.NewNote()
@@ -60,5 +94,4 @@ func MoveAndLeaveSymlink(config FullClientSettings, singleTorrent *torrent.Torre
}
Logger.WithFields(logrus.Fields{"Torrent": singleTorrent.Name(), "New File Path": tStorage.StoragePath}).Info("Pushbullet note sent")
}
-
}
diff --git a/engine/engine.go b/engine/engine.go
index 4dfdf471..1c20b474 100644
--- a/engine/engine.go
+++ b/engine/engine.go
@@ -80,7 +80,7 @@ func timeOutInfo(clientTorrent *torrent.Torrent, seconds time.Duration) (deleted
}
-func readTorrentFileFromDB(element *Storage.TorrentLocal, singleTorrent *torrent.Torrent, tclient *torrent.Client, db *storm.DB) {
+func readTorrentFileFromDB(element *Storage.TorrentLocal, tclient *torrent.Client, db *storm.DB) (singleTorrent *torrent.Torrent) {
tempFile, err := ioutil.TempFile("", "TorrentFileTemp")
if err != nil {
Logger.WithFields(logrus.Fields{"tempfile": tempFile, "err": err}).Error("Unable to create tempfile")
@@ -92,13 +92,16 @@ func readTorrentFileFromDB(element *Storage.TorrentLocal, singleTorrent *torrent
if err := tempFile.Close(); err != nil { //close the tempfile so that we can add it back into the torrent client
Logger.WithFields(logrus.Fields{"tempfile": tempFile, "err": err}).Error("Unable to close tempfile")
}
- singleTorrent, _ = tclient.AddTorrentFromFile(tempFile.Name())
- if _, err := os.Stat(element.TorrentFileName); err == nil { //if we CAN find the torrent, add it
- singleTorrent, _ = tclient.AddTorrentFromFile(element.TorrentFileName)
- } else { //if we cant find the torrent delete it
- Storage.DelTorrentLocalStorage(db, element.Hash)
- Logger.WithFields(logrus.Fields{"tempfile": tempFile, "err": err}).Error("Unable to find Torrent, deleting..")
+ _, err = os.Stat(element.TorrentFileName) //if we CAN find the torrent, add it
+ if err != nil {
+ Logger.WithFields(logrus.Fields{"tempfile": tempFile, "err": err}).Error("Unable to find file")
}
+ singleTorrent, err = tclient.AddTorrentFromFile(element.TorrentFileName)
+ if err != nil {
+ Logger.WithFields(logrus.Fields{"tempfile": element.TorrentFileName, "err": err}).Error("Unable to add Torrent from file!")
+
+ }
+ return singleTorrent
}
//StartTorrent creates the storage.db entry and starts A NEW TORRENT and adds to the running torrent array
@@ -147,7 +150,6 @@ func StartTorrent(clientTorrent *torrent.Torrent, torrentLocalStorage Storage.To
func CreateRunningTorrentArray(tclient *torrent.Client, TorrentLocalArray []*Storage.TorrentLocal, PreviousTorrentArray []ClientDB, config FullClientSettings, db *storm.DB) (RunningTorrentArray []ClientDB) {
for _, singleTorrentFromStorage := range TorrentLocalArray {
-
var singleTorrent *torrent.Torrent
var TempHash metainfo.Hash
@@ -155,15 +157,13 @@ func CreateRunningTorrentArray(tclient *torrent.Client, TorrentLocalArray []*Sto
//singleTorrentStorageInfo := Storage.FetchTorrentFromStorage(db, TempHash.String()) //pulling the single torrent info from storage ()
if singleTorrentFromStorage.TorrentType == "file" { //if it is a file pull it from the uploaded torrent folder
- readTorrentFileFromDB(singleTorrentFromStorage, singleTorrent, tclient, db)
+ singleTorrent = readTorrentFileFromDB(singleTorrentFromStorage, tclient, db)
fullClientDB.SourceType = "Torrent File"
- continue
} else {
singleTorrentFromStorageMagnet := "magnet:?xt=urn:btih:" + singleTorrentFromStorage.Hash //For magnet links just need to prepend the magnet part to the hash to readd
singleTorrent, _ = tclient.AddMagnet(singleTorrentFromStorageMagnet)
fullClientDB.SourceType = "Magnet Link"
}
-
if len(singleTorrentFromStorage.InfoBytes) == 0 { //TODO.. kind of a fringe scenario.. not sure if needed since the db should always have the infobytes
timeOut := timeOutInfo(singleTorrent, 45)
if timeOut == true { // if we did timeout then drop the torrent from the boltdb database
@@ -173,8 +173,12 @@ func CreateRunningTorrentArray(tclient *torrent.Client, TorrentLocalArray []*Sto
singleTorrentFromStorage.InfoBytes = singleTorrent.Metainfo().InfoBytes
}
+ err := singleTorrent.SetInfoBytes(singleTorrentFromStorage.InfoBytes) //setting the infobytes back into the torrent
+ if err != nil {
+ Logger.WithFields(logrus.Fields{"torrentFile": singleTorrent.Name(), "error": err}).Error("Unable to add infobytes to the torrent!")
+ }
+ //Logger.WithFields(logrus.Fields{"singleTorrent": singleTorrentFromStorage.TorrentName}).Info("Generating infohash")
TempHash = singleTorrent.InfoHash()
- singleTorrent.SetInfoBytes(singleTorrentFromStorage.InfoBytes) //setting the infobytes back into the torrent
if (singleTorrent.BytesCompleted() == singleTorrent.Length()) && (singleTorrentFromStorage.TorrentMoved == false) { //if we are done downloading and havent moved torrent yet
MoveAndLeaveSymlink(config, singleTorrent, db)
@@ -190,7 +194,7 @@ func CreateRunningTorrentArray(tclient *torrent.Client, TorrentLocalArray []*Sto
downloadedSizeHumanized := HumanizeBytes(float32(singleTorrent.BytesCompleted())) //convert size to GB if needed
totalSizeHumanized := HumanizeBytes(float32(singleTorrent.Length()))
-
+ //Logger.WithFields(logrus.Fields{"singleTorrent": singleTorrentFromStorage.TorrentName}).Info("Generated infohash")
//grabbed from torrent client
fullClientDB.DownloadedSize = downloadedSizeHumanized
fullClientDB.Size = totalSizeHumanized
@@ -206,7 +210,7 @@ func CreateRunningTorrentArray(tclient *torrent.Client, TorrentLocalArray []*Sto
fullClientDB.DateAdded = singleTorrentFromStorage.DateAdded
fullClientDB.BytesCompleted = singleTorrent.BytesCompleted()
fullClientDB.NumberofFiles = len(singleTorrent.Files())
- CalculateTorrentETA(singleTorrent, fullClientDB)
+
//ranging over the previous torrent array to calculate the speed for each torrent
if len(PreviousTorrentArray) > 0 { //if we actually have a previous array
for _, previousElement := range PreviousTorrentArray {
@@ -217,6 +221,7 @@ func CreateRunningTorrentArray(tclient *torrent.Client, TorrentLocalArray []*Sto
}
}
}
+ CalculateTorrentETA(singleTorrent, fullClientDB) //needs to be here since we need the speed calcuated before we can estimate the eta.
fullClientDB.TotalUploadedSize = HumanizeBytes(float32(fullClientDB.TotalUploadedBytes))
fullClientDB.UploadRatio = CalculateUploadRatio(singleTorrent, fullClientDB) //calculate the upload ratio
diff --git a/engine/settings.go b/engine/settings.go
index b18a37ab..36e74d7e 100644
--- a/engine/settings.go
+++ b/engine/settings.go
@@ -67,7 +67,7 @@ func FullClientSettingsNew() FullClientSettings {
httpAddrPort := viper.GetString("serverConfig.ServerPort")
seedRatioStop := viper.GetFloat64("serverConfig.SeedRatioStop")
httpAddr = httpAddrIP + httpAddrPort
- pushBulletToken := viper.GetString("serverConfig.notifications.PushBulletToken")
+ pushBulletToken := viper.GetString("notifications.PushBulletToken")
defaultMoveFolder := viper.GetString("serverConfig.DefaultMoveFolder")
dataDir := viper.GetString("torrentClientConfig.DownloadDir")
@@ -117,9 +117,6 @@ func FullClientSettingsNew() FullClientSettings {
downloadRateLimiter := new(rate.Limiter)
viper.UnmarshalKey("DownloadRateLimiter", &downloadRateLimiter)
- rreferNoEncryption := viper.GetBool("EncryptionPolicy.PreferNoEncryption")
- fmt.Println("Encryption", rreferNoEncryption)
-
encryptionPolicy := torrent.EncryptionPolicy{
DisableEncryption: viper.GetBool("EncryptionPolicy.DisableEncryption"),
ForceEncryption: viper.GetBool("EncryptionPolicy.ForceEncryption"),
diff --git a/goTorrentWebUI/node_modules/attr-accept/package.json b/goTorrentWebUI/node_modules/attr-accept/package.json
index fefb7662..bcbbeeab 100644
--- a/goTorrentWebUI/node_modules/attr-accept/package.json
+++ b/goTorrentWebUI/node_modules/attr-accept/package.json
@@ -22,7 +22,8 @@
"fetchSpec": "1.1.0"
},
"_requiredBy": [
- "/"
+ "/",
+ "/react-dropzone"
],
"_resolved": "https://registry.npmjs.org/attr-accept/-/attr-accept-1.1.0.tgz",
"_spec": "1.1.0",
diff --git a/goTorrentWebUI/node_modules/react-dropzone/.babelrc b/goTorrentWebUI/node_modules/react-dropzone/.babelrc
new file mode 100644
index 00000000..b387ab56
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/.babelrc
@@ -0,0 +1,19 @@
+{
+ "env": {
+ "development": {
+ "presets": ["env", "react", "stage-1"],
+ "plugins": ["add-module-exports"]
+ },
+ "test": {
+ "presets": ["env", "react", "stage-1"],
+ "plugins": ["add-module-exports"]
+ },
+ "production": {
+ "presets": ["env", "react", "stage-1"],
+ "plugins": ["add-module-exports"]
+ },
+ "es": {
+ "presets": [["env",{ "modules": false }], "react", "stage-1"]
+ }
+ }
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/.codeclimate.yml b/goTorrentWebUI/node_modules/react-dropzone/.codeclimate.yml
new file mode 100644
index 00000000..b16eb728
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/.codeclimate.yml
@@ -0,0 +1,15 @@
+engines:
+ duplication:
+ enabled: true
+ config:
+ languages:
+ - javascript
+ eslint:
+ enabled: true
+ channel: "eslint-3"
+ratings:
+ paths:
+ - "**.js"
+exclude_paths:
+ - "dist/"
+ - "src/*.spec.js"
diff --git a/goTorrentWebUI/node_modules/react-dropzone/.editorconfig b/goTorrentWebUI/node_modules/react-dropzone/.editorconfig
new file mode 100644
index 00000000..e717f5eb
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/.editorconfig
@@ -0,0 +1,13 @@
+# http://editorconfig.org
+root = true
+
+[*]
+indent_style = space
+indent_size = 2
+end_of_line = lf
+charset = utf-8
+trim_trailing_whitespace = true
+insert_final_newline = true
+
+[*.md]
+trim_trailing_whitespace = false
diff --git a/goTorrentWebUI/node_modules/react-dropzone/.eslintrc b/goTorrentWebUI/node_modules/react-dropzone/.eslintrc
new file mode 100644
index 00000000..1d7e74ac
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/.eslintrc
@@ -0,0 +1,34 @@
+{
+ "extends": [
+ "okonet",
+ "prettier"
+ ],
+ "plugins": [
+ "prettier"
+ ],
+ "rules": {
+ // React
+ "react/forbid-prop-types": [
+ 2,
+ {
+ "forbid": [
+ "any",
+ "array"
+ ]
+ }
+ ],
+ "react/require-default-props": 0,
+
+ // Import
+ "import/no-extraneous-dependencies": [
+ 2,
+ {
+ "devDependencies": [
+ "webpack*.js",
+ "**/*.spec.js",
+ "**/testSetup.js"
+ ]
+ }
+ ]
+ }
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/.travis.yml b/goTorrentWebUI/node_modules/react-dropzone/.travis.yml
new file mode 100644
index 00000000..0d3eb038
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/.travis.yml
@@ -0,0 +1,12 @@
+language: node_js
+cache: yarn
+notifications:
+ email: false
+node_js:
+ - '8'
+after_success:
+ - bash <(curl -s https://codecov.io/bash)
+ - npm run semantic-release
+branches:
+ except:
+ - /^v\d+\.\d+\.\d+$/
diff --git a/goTorrentWebUI/node_modules/react-dropzone/LICENSE b/goTorrentWebUI/node_modules/react-dropzone/LICENSE
new file mode 100644
index 00000000..863075c4
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/LICENSE
@@ -0,0 +1,22 @@
+The MIT License (MIT)
+
+Copyright (c) 2014 Param Aggarwal
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
+
diff --git a/goTorrentWebUI/node_modules/react-dropzone/README.md b/goTorrentWebUI/node_modules/react-dropzone/README.md
new file mode 100644
index 00000000..cd216c23
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/README.md
@@ -0,0 +1,165 @@
+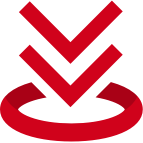
+
+# react-dropzone
+
+[](https://travis-ci.org/react-dropzone/react-dropzone) [](https://badge.fury.io/js/react-dropzone) [](https://codecov.io/gh/react-dropzone/react-dropzone) [](#backers)
+[](#sponsors)
+
+Simple HTML5-compliant drag'n'drop zone for files built with React.js.
+
+Documentation and examples: https://react-dropzone.js.org
+Source code: https://github.com/react-dropzone/react-dropzone/
+
+---
+
+**Looking for maintainers: https://github.com/react-dropzone/react-dropzone/issues/479**
+
+---
+
+## Installation
+
+Install it from npm and include it in your React build process (using [Webpack](http://webpack.github.io/), [Browserify](http://browserify.org/), etc).
+
+```bash
+npm install --save react-dropzone
+```
+or:
+```bash
+yarn add react-dropzone
+```
+
+## Usage
+
+Import `Dropzone` in your React component:
+
+```javascript static
+import Dropzone from 'react-dropzone'
+```
+
+ and specify the `onDrop` method that accepts two arguments. The first argument represents the accepted files and the second argument the rejected files.
+
+```javascript static
+function onDrop(acceptedFiles, rejectedFiles) {
+ // do stuff with files...
+}
+```
+
+Files accepted or rejected based on `accept` prop. This must be a valid [MIME type](http://www.iana.org/assignments/media-types/media-types.xhtml) according to [input element specification](https://www.w3.org/wiki/HTML/Elements/input/file).
+
+Please note that `onDrop` method will always be called regardless if dropped file was accepted or rejected. The `onDropAccepted` method will be called if all dropped files were accepted and the `onDropRejected` method will be called if any of the dropped files was rejected.
+
+Using `react-dropzone` is similar to using a file form field, but instead of getting the `files` property from the field, you listen to the `onDrop` callback to handle the files. Simple explanation here: http://abandon.ie/notebook/simple-file-uploads-using-jquery-ajax
+
+Specifying the `onDrop` method, provides you with an array of [Files](https://developer.mozilla.org/en-US/docs/Web/API/File) which you can then send to a server. For example, with [SuperAgent](https://github.com/visionmedia/superagent) as a http/ajax library:
+
+```javascript static
+onDrop: acceptedFiles => {
+ const req = request.post('/upload');
+ acceptedFiles.forEach(file => {
+ req.attach(file.name, file);
+ });
+ req.end(callback);
+}
+```
+
+**Warning**: On most recent browsers versions, the files given by `onDrop` won't have properties `path` or `fullPath`, see [this SO question](https://stackoverflow.com/a/23005925/2275818) and [this issue](https://github.com/react-dropzone/react-dropzone/issues/477).
+If you want to access file content you have to use the [FileReader API](https://developer.mozilla.org/en-US/docs/Web/API/FileReader).
+
+```javascript static
+onDrop: acceptedFiles => {
+ acceptedFiles.forEach(file => {
+ const reader = new FileReader();
+ reader.onload = () => {
+ const fileAsBinaryString = reader.result;
+ // do whatever you want with the file content
+ };
+ reader.onabort = () => console.log('file reading was aborted');
+ reader.onerror = () => console.log('file reading has failed');
+
+ reader.readAsBinaryString(file);
+ });
+}
+```
+
+## PropTypes
+
+See https://react-dropzone.netlify.com/#proptypes
+
+### Word of caution when working with previews
+
+*Important*: `react-dropzone` doesn't manage dropped files. You need to destroy the object URL yourself whenever you don't need the `preview` by calling `window.URL.revokeObjectURL(file.preview);` to avoid memory leaks.
+
+## Support
+
+### Backers
+Support us with a monthly donation and help us continue our activities. [[Become a backer](https://opencollective.com/react-dropzone#backer)]
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+### Sponsors
+Become a sponsor and get your logo on our README on Github with a link to your site. [[Become a sponsor](https://opencollective.com/react-dropzone#sponsor)]
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+## License
+
+MIT
diff --git a/goTorrentWebUI/node_modules/react-dropzone/commitlint.config.js b/goTorrentWebUI/node_modules/react-dropzone/commitlint.config.js
new file mode 100644
index 00000000..f78763c2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/commitlint.config.js
@@ -0,0 +1 @@
+module.exports = { extends: ['@commitlint/config-angular'] }
diff --git a/goTorrentWebUI/node_modules/react-dropzone/dist/es/index.js b/goTorrentWebUI/node_modules/react-dropzone/dist/es/index.js
new file mode 100644
index 00000000..01056bc8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/dist/es/index.js
@@ -0,0 +1,619 @@
+var _extends = Object.assign || function (target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i]; for (var key in source) { if (Object.prototype.hasOwnProperty.call(source, key)) { target[key] = source[key]; } } } return target; };
+
+var _createClass = function () { function defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } return function (Constructor, protoProps, staticProps) { if (protoProps) defineProperties(Constructor.prototype, protoProps); if (staticProps) defineProperties(Constructor, staticProps); return Constructor; }; }();
+
+function _objectWithoutProperties(obj, keys) { var target = {}; for (var i in obj) { if (keys.indexOf(i) >= 0) continue; if (!Object.prototype.hasOwnProperty.call(obj, i)) continue; target[i] = obj[i]; } return target; }
+
+function _toConsumableArray(arr) { if (Array.isArray(arr)) { for (var i = 0, arr2 = Array(arr.length); i < arr.length; i++) { arr2[i] = arr[i]; } return arr2; } else { return Array.from(arr); } }
+
+function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
+
+function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; }
+
+function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; }
+
+/* eslint prefer-template: 0 */
+
+import React from 'react';
+import PropTypes from 'prop-types';
+import { supportMultiple, fileAccepted, allFilesAccepted, fileMatchSize, onDocumentDragOver, getDataTransferItems } from './utils';
+import styles from './utils/styles';
+
+var Dropzone = function (_React$Component) {
+ _inherits(Dropzone, _React$Component);
+
+ function Dropzone(props, context) {
+ _classCallCheck(this, Dropzone);
+
+ var _this = _possibleConstructorReturn(this, (Dropzone.__proto__ || Object.getPrototypeOf(Dropzone)).call(this, props, context));
+
+ _this.renderChildren = function (children, isDragActive, isDragAccept, isDragReject) {
+ if (typeof children === 'function') {
+ return children(_extends({}, _this.state, {
+ isDragActive: isDragActive,
+ isDragAccept: isDragAccept,
+ isDragReject: isDragReject
+ }));
+ }
+ return children;
+ };
+
+ _this.composeHandlers = _this.composeHandlers.bind(_this);
+ _this.onClick = _this.onClick.bind(_this);
+ _this.onDocumentDrop = _this.onDocumentDrop.bind(_this);
+ _this.onDragEnter = _this.onDragEnter.bind(_this);
+ _this.onDragLeave = _this.onDragLeave.bind(_this);
+ _this.onDragOver = _this.onDragOver.bind(_this);
+ _this.onDragStart = _this.onDragStart.bind(_this);
+ _this.onDrop = _this.onDrop.bind(_this);
+ _this.onFileDialogCancel = _this.onFileDialogCancel.bind(_this);
+ _this.onInputElementClick = _this.onInputElementClick.bind(_this);
+
+ _this.setRef = _this.setRef.bind(_this);
+ _this.setRefs = _this.setRefs.bind(_this);
+
+ _this.isFileDialogActive = false;
+
+ _this.state = {
+ draggedFiles: [],
+ acceptedFiles: [],
+ rejectedFiles: []
+ };
+ return _this;
+ }
+
+ _createClass(Dropzone, [{
+ key: 'componentDidMount',
+ value: function componentDidMount() {
+ var preventDropOnDocument = this.props.preventDropOnDocument;
+
+ this.dragTargets = [];
+
+ if (preventDropOnDocument) {
+ document.addEventListener('dragover', onDocumentDragOver, false);
+ document.addEventListener('drop', this.onDocumentDrop, false);
+ }
+ this.fileInputEl.addEventListener('click', this.onInputElementClick, false);
+ // Tried implementing addEventListener, but didn't work out
+ document.body.onfocus = this.onFileDialogCancel;
+ }
+ }, {
+ key: 'componentWillUnmount',
+ value: function componentWillUnmount() {
+ var preventDropOnDocument = this.props.preventDropOnDocument;
+
+ if (preventDropOnDocument) {
+ document.removeEventListener('dragover', onDocumentDragOver);
+ document.removeEventListener('drop', this.onDocumentDrop);
+ }
+ if (this.fileInputEl != null) {
+ this.fileInputEl.removeEventListener('click', this.onInputElementClick, false);
+ }
+ // Can be replaced with removeEventListener, if addEventListener works
+ if (document != null) {
+ document.body.onfocus = null;
+ }
+ }
+ }, {
+ key: 'composeHandlers',
+ value: function composeHandlers(handler) {
+ if (this.props.disabled) {
+ return null;
+ }
+
+ return handler;
+ }
+ }, {
+ key: 'onDocumentDrop',
+ value: function onDocumentDrop(evt) {
+ if (this.node && this.node.contains(evt.target)) {
+ // if we intercepted an event for our instance, let it propagate down to the instance's onDrop handler
+ return;
+ }
+ evt.preventDefault();
+ this.dragTargets = [];
+ }
+ }, {
+ key: 'onDragStart',
+ value: function onDragStart(evt) {
+ if (this.props.onDragStart) {
+ this.props.onDragStart.call(this, evt);
+ }
+ }
+ }, {
+ key: 'onDragEnter',
+ value: function onDragEnter(evt) {
+ evt.preventDefault();
+
+ // Count the dropzone and any children that are entered.
+ if (this.dragTargets.indexOf(evt.target) === -1) {
+ this.dragTargets.push(evt.target);
+ }
+
+ this.setState({
+ isDragActive: true, // Do not rely on files for the drag state. It doesn't work in Safari.
+ draggedFiles: getDataTransferItems(evt)
+ });
+
+ if (this.props.onDragEnter) {
+ this.props.onDragEnter.call(this, evt);
+ }
+ }
+ }, {
+ key: 'onDragOver',
+ value: function onDragOver(evt) {
+ // eslint-disable-line class-methods-use-this
+ evt.preventDefault();
+ evt.stopPropagation();
+ try {
+ // The file dialog on Chrome allows users to drag files from the dialog onto
+ // the dropzone, causing the browser the crash when the file dialog is closed.
+ // A drop effect of 'none' prevents the file from being dropped
+ evt.dataTransfer.dropEffect = this.isFileDialogActive ? 'none' : 'copy'; // eslint-disable-line no-param-reassign
+ } catch (err) {
+ // continue regardless of error
+ }
+
+ if (this.props.onDragOver) {
+ this.props.onDragOver.call(this, evt);
+ }
+ return false;
+ }
+ }, {
+ key: 'onDragLeave',
+ value: function onDragLeave(evt) {
+ var _this2 = this;
+
+ evt.preventDefault();
+
+ // Only deactivate once the dropzone and all children have been left.
+ this.dragTargets = this.dragTargets.filter(function (el) {
+ return el !== evt.target && _this2.node.contains(el);
+ });
+ if (this.dragTargets.length > 0) {
+ return;
+ }
+
+ // Clear dragging files state
+ this.setState({
+ isDragActive: false,
+ draggedFiles: []
+ });
+
+ if (this.props.onDragLeave) {
+ this.props.onDragLeave.call(this, evt);
+ }
+ }
+ }, {
+ key: 'onDrop',
+ value: function onDrop(evt) {
+ var _this3 = this;
+
+ var _props = this.props,
+ onDrop = _props.onDrop,
+ onDropAccepted = _props.onDropAccepted,
+ onDropRejected = _props.onDropRejected,
+ multiple = _props.multiple,
+ disablePreview = _props.disablePreview,
+ accept = _props.accept;
+
+ var fileList = getDataTransferItems(evt);
+ var acceptedFiles = [];
+ var rejectedFiles = [];
+
+ // Stop default browser behavior
+ evt.preventDefault();
+
+ // Reset the counter along with the drag on a drop.
+ this.dragTargets = [];
+ this.isFileDialogActive = false;
+
+ fileList.forEach(function (file) {
+ if (!disablePreview) {
+ try {
+ file.preview = window.URL.createObjectURL(file); // eslint-disable-line no-param-reassign
+ } catch (err) {
+ if (process.env.NODE_ENV !== 'production') {
+ console.error('Failed to generate preview for file', file, err); // eslint-disable-line no-console
+ }
+ }
+ }
+
+ if (fileAccepted(file, accept) && fileMatchSize(file, _this3.props.maxSize, _this3.props.minSize)) {
+ acceptedFiles.push(file);
+ } else {
+ rejectedFiles.push(file);
+ }
+ });
+
+ if (!multiple) {
+ // if not in multi mode add any extra accepted files to rejected.
+ // This will allow end users to easily ignore a multi file drop in "single" mode.
+ rejectedFiles.push.apply(rejectedFiles, _toConsumableArray(acceptedFiles.splice(1)));
+ }
+
+ if (onDrop) {
+ onDrop.call(this, acceptedFiles, rejectedFiles, evt);
+ }
+
+ if (rejectedFiles.length > 0 && onDropRejected) {
+ onDropRejected.call(this, rejectedFiles, evt);
+ }
+
+ if (acceptedFiles.length > 0 && onDropAccepted) {
+ onDropAccepted.call(this, acceptedFiles, evt);
+ }
+
+ // Clear files value
+ this.draggedFiles = null;
+
+ // Reset drag state
+ this.setState({
+ isDragActive: false,
+ draggedFiles: [],
+ acceptedFiles: acceptedFiles,
+ rejectedFiles: rejectedFiles
+ });
+ }
+ }, {
+ key: 'onClick',
+ value: function onClick(evt) {
+ var _props2 = this.props,
+ onClick = _props2.onClick,
+ disableClick = _props2.disableClick;
+
+ if (!disableClick) {
+ evt.stopPropagation();
+
+ if (onClick) {
+ onClick.call(this, evt);
+ }
+
+ // in IE11/Edge the file-browser dialog is blocking, ensure this is behind setTimeout
+ // this is so react can handle state changes in the onClick prop above above
+ // see: https://github.com/react-dropzone/react-dropzone/issues/450
+ setTimeout(this.open.bind(this), 0);
+ }
+ }
+ }, {
+ key: 'onInputElementClick',
+ value: function onInputElementClick(evt) {
+ evt.stopPropagation();
+ if (this.props.inputProps && this.props.inputProps.onClick) {
+ this.props.inputProps.onClick();
+ }
+ }
+ }, {
+ key: 'onFileDialogCancel',
+ value: function onFileDialogCancel() {
+ // timeout will not recognize context of this method
+ var onFileDialogCancel = this.props.onFileDialogCancel;
+ var fileInputEl = this.fileInputEl;
+ var isFileDialogActive = this.isFileDialogActive;
+ // execute the timeout only if the onFileDialogCancel is defined and FileDialog
+ // is opened in the browser
+
+ if (onFileDialogCancel && isFileDialogActive) {
+ setTimeout(function () {
+ // Returns an object as FileList
+ var FileList = fileInputEl.files;
+ if (!FileList.length) {
+ isFileDialogActive = false;
+ onFileDialogCancel();
+ }
+ }, 300);
+ }
+ }
+ }, {
+ key: 'setRef',
+ value: function setRef(ref) {
+ this.node = ref;
+ }
+ }, {
+ key: 'setRefs',
+ value: function setRefs(ref) {
+ this.fileInputEl = ref;
+ }
+ /**
+ * Open system file upload dialog.
+ *
+ * @public
+ */
+
+ }, {
+ key: 'open',
+ value: function open() {
+ this.isFileDialogActive = true;
+ this.fileInputEl.value = null;
+ this.fileInputEl.click();
+ }
+ }, {
+ key: 'render',
+ value: function render() {
+ var _props3 = this.props,
+ accept = _props3.accept,
+ acceptClassName = _props3.acceptClassName,
+ activeClassName = _props3.activeClassName,
+ children = _props3.children,
+ disabled = _props3.disabled,
+ disabledClassName = _props3.disabledClassName,
+ inputProps = _props3.inputProps,
+ multiple = _props3.multiple,
+ name = _props3.name,
+ rejectClassName = _props3.rejectClassName,
+ rest = _objectWithoutProperties(_props3, ['accept', 'acceptClassName', 'activeClassName', 'children', 'disabled', 'disabledClassName', 'inputProps', 'multiple', 'name', 'rejectClassName']);
+
+ var acceptStyle = rest.acceptStyle,
+ activeStyle = rest.activeStyle,
+ _rest$className = rest.className,
+ className = _rest$className === undefined ? '' : _rest$className,
+ disabledStyle = rest.disabledStyle,
+ rejectStyle = rest.rejectStyle,
+ style = rest.style,
+ props = _objectWithoutProperties(rest, ['acceptStyle', 'activeStyle', 'className', 'disabledStyle', 'rejectStyle', 'style']);
+
+ var _state = this.state,
+ isDragActive = _state.isDragActive,
+ draggedFiles = _state.draggedFiles;
+
+ var filesCount = draggedFiles.length;
+ var isMultipleAllowed = multiple || filesCount <= 1;
+ var isDragAccept = filesCount > 0 && allFilesAccepted(draggedFiles, this.props.accept);
+ var isDragReject = filesCount > 0 && (!isDragAccept || !isMultipleAllowed);
+ var noStyles = !className && !style && !activeStyle && !acceptStyle && !rejectStyle && !disabledStyle;
+
+ if (isDragActive && activeClassName) {
+ className += ' ' + activeClassName;
+ }
+ if (isDragAccept && acceptClassName) {
+ className += ' ' + acceptClassName;
+ }
+ if (isDragReject && rejectClassName) {
+ className += ' ' + rejectClassName;
+ }
+ if (disabled && disabledClassName) {
+ className += ' ' + disabledClassName;
+ }
+
+ if (noStyles) {
+ style = styles.default;
+ activeStyle = styles.active;
+ acceptStyle = style.active;
+ rejectStyle = styles.rejected;
+ disabledStyle = styles.disabled;
+ }
+
+ var appliedStyle = _extends({}, style);
+ if (activeStyle && isDragActive) {
+ appliedStyle = _extends({}, style, activeStyle);
+ }
+ if (acceptStyle && isDragAccept) {
+ appliedStyle = _extends({}, appliedStyle, acceptStyle);
+ }
+ if (rejectStyle && isDragReject) {
+ appliedStyle = _extends({}, appliedStyle, rejectStyle);
+ }
+ if (disabledStyle && disabled) {
+ appliedStyle = _extends({}, style, disabledStyle);
+ }
+
+ var inputAttributes = {
+ accept: accept,
+ disabled: disabled,
+ type: 'file',
+ style: { display: 'none' },
+ multiple: supportMultiple && multiple,
+ ref: this.setRefs,
+ onChange: this.onDrop,
+ autoComplete: 'off'
+ };
+
+ if (name && name.length) {
+ inputAttributes.name = name;
+ }
+
+ // Destructure custom props away from props used for the div element
+
+ var acceptedFiles = props.acceptedFiles,
+ preventDropOnDocument = props.preventDropOnDocument,
+ disablePreview = props.disablePreview,
+ disableClick = props.disableClick,
+ onDropAccepted = props.onDropAccepted,
+ onDropRejected = props.onDropRejected,
+ onFileDialogCancel = props.onFileDialogCancel,
+ maxSize = props.maxSize,
+ minSize = props.minSize,
+ divProps = _objectWithoutProperties(props, ['acceptedFiles', 'preventDropOnDocument', 'disablePreview', 'disableClick', 'onDropAccepted', 'onDropRejected', 'onFileDialogCancel', 'maxSize', 'minSize']);
+
+ return React.createElement(
+ 'div',
+ _extends({
+ className: className,
+ style: appliedStyle
+ }, divProps /* expand user provided props first so event handlers are never overridden */, {
+ onClick: this.composeHandlers(this.onClick),
+ onDragStart: this.composeHandlers(this.onDragStart),
+ onDragEnter: this.composeHandlers(this.onDragEnter),
+ onDragOver: this.composeHandlers(this.onDragOver),
+ onDragLeave: this.composeHandlers(this.onDragLeave),
+ onDrop: this.composeHandlers(this.onDrop),
+ ref: this.setRef,
+ 'aria-disabled': disabled
+ }),
+ this.renderChildren(children, isDragActive, isDragAccept, isDragReject),
+ React.createElement('input', _extends({}, inputProps /* expand user provided inputProps first so inputAttributes override them */, inputAttributes))
+ );
+ }
+ }]);
+
+ return Dropzone;
+}(React.Component);
+
+export default Dropzone;
+
+Dropzone.propTypes = {
+ /**
+ * Allow specific types of files. See https://github.com/okonet/attr-accept for more information.
+ * Keep in mind that mime type determination is not reliable across platforms. CSV files,
+ * for example, are reported as text/plain under macOS but as application/vnd.ms-excel under
+ * Windows. In some cases there might not be a mime type set at all.
+ * See: https://github.com/react-dropzone/react-dropzone/issues/276
+ */
+ accept: PropTypes.string,
+
+ /**
+ * Contents of the dropzone
+ */
+ children: PropTypes.oneOfType([PropTypes.node, PropTypes.func]),
+
+ /**
+ * Disallow clicking on the dropzone container to open file dialog
+ */
+ disableClick: PropTypes.bool,
+
+ /**
+ * Enable/disable the dropzone entirely
+ */
+ disabled: PropTypes.bool,
+
+ /**
+ * Enable/disable preview generation
+ */
+ disablePreview: PropTypes.bool,
+
+ /**
+ * If false, allow dropped items to take over the current browser window
+ */
+ preventDropOnDocument: PropTypes.bool,
+
+ /**
+ * Pass additional attributes to the ` ` tag
+ */
+ inputProps: PropTypes.object,
+
+ /**
+ * Allow dropping multiple files
+ */
+ multiple: PropTypes.bool,
+
+ /**
+ * `name` attribute for the input tag
+ */
+ name: PropTypes.string,
+
+ /**
+ * Maximum file size
+ */
+ maxSize: PropTypes.number,
+
+ /**
+ * Minimum file size
+ */
+ minSize: PropTypes.number,
+
+ /**
+ * className
+ */
+ className: PropTypes.string,
+
+ /**
+ * className for active state
+ */
+ activeClassName: PropTypes.string,
+
+ /**
+ * className for accepted state
+ */
+ acceptClassName: PropTypes.string,
+
+ /**
+ * className for rejected state
+ */
+ rejectClassName: PropTypes.string,
+
+ /**
+ * className for disabled state
+ */
+ disabledClassName: PropTypes.string,
+
+ /**
+ * CSS styles to apply
+ */
+ style: PropTypes.object,
+
+ /**
+ * CSS styles to apply when drag is active
+ */
+ activeStyle: PropTypes.object,
+
+ /**
+ * CSS styles to apply when drop will be accepted
+ */
+ acceptStyle: PropTypes.object,
+
+ /**
+ * CSS styles to apply when drop will be rejected
+ */
+ rejectStyle: PropTypes.object,
+
+ /**
+ * CSS styles to apply when dropzone is disabled
+ */
+ disabledStyle: PropTypes.object,
+
+ /**
+ * onClick callback
+ * @param {Event} event
+ */
+ onClick: PropTypes.func,
+
+ /**
+ * onDrop callback
+ */
+ onDrop: PropTypes.func,
+
+ /**
+ * onDropAccepted callback
+ */
+ onDropAccepted: PropTypes.func,
+
+ /**
+ * onDropRejected callback
+ */
+ onDropRejected: PropTypes.func,
+
+ /**
+ * onDragStart callback
+ */
+ onDragStart: PropTypes.func,
+
+ /**
+ * onDragEnter callback
+ */
+ onDragEnter: PropTypes.func,
+
+ /**
+ * onDragOver callback
+ */
+ onDragOver: PropTypes.func,
+
+ /**
+ * onDragLeave callback
+ */
+ onDragLeave: PropTypes.func,
+
+ /**
+ * Provide a callback on clicking the cancel button of the file dialog
+ */
+ onFileDialogCancel: PropTypes.func
+};
+
+Dropzone.defaultProps = {
+ preventDropOnDocument: true,
+ disabled: false,
+ disablePreview: false,
+ disableClick: false,
+ multiple: true,
+ maxSize: Infinity,
+ minSize: 0
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/dist/es/utils/index.js b/goTorrentWebUI/node_modules/react-dropzone/dist/es/utils/index.js
new file mode 100644
index 00000000..f1d03af0
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/dist/es/utils/index.js
@@ -0,0 +1,42 @@
+import accepts from 'attr-accept';
+
+export var supportMultiple = typeof document !== 'undefined' && document && document.createElement ? 'multiple' in document.createElement('input') : true;
+
+export function getDataTransferItems(event) {
+ var dataTransferItemsList = [];
+ if (event.dataTransfer) {
+ var dt = event.dataTransfer;
+ if (dt.files && dt.files.length) {
+ dataTransferItemsList = dt.files;
+ } else if (dt.items && dt.items.length) {
+ // During the drag even the dataTransfer.files is null
+ // but Chrome implements some drag store, which is accesible via dataTransfer.items
+ dataTransferItemsList = dt.items;
+ }
+ } else if (event.target && event.target.files) {
+ dataTransferItemsList = event.target.files;
+ }
+ // Convert from DataTransferItemsList to the native Array
+ return Array.prototype.slice.call(dataTransferItemsList);
+}
+
+// Firefox versions prior to 53 return a bogus MIME type for every file drag, so dragovers with
+// that MIME type will always be accepted
+export function fileAccepted(file, accept) {
+ return file.type === 'application/x-moz-file' || accepts(file, accept);
+}
+
+export function fileMatchSize(file, maxSize, minSize) {
+ return file.size <= maxSize && file.size >= minSize;
+}
+
+export function allFilesAccepted(files, accept) {
+ return files.every(function (file) {
+ return fileAccepted(file, accept);
+ });
+}
+
+// allow the entire document to be a drag target
+export function onDocumentDragOver(evt) {
+ evt.preventDefault();
+}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/dist/es/utils/styles.js b/goTorrentWebUI/node_modules/react-dropzone/dist/es/utils/styles.js
new file mode 100644
index 00000000..f090a46c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/dist/es/utils/styles.js
@@ -0,0 +1,23 @@
+export default {
+ rejected: {
+ borderStyle: 'solid',
+ borderColor: '#c66',
+ backgroundColor: '#eee'
+ },
+ disabled: {
+ opacity: 0.5
+ },
+ active: {
+ borderStyle: 'solid',
+ borderColor: '#6c6',
+ backgroundColor: '#eee'
+ },
+ default: {
+ width: 200,
+ height: 200,
+ borderWidth: 2,
+ borderColor: '#666',
+ borderStyle: 'dashed',
+ borderRadius: 5
+ }
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/dist/index.js b/goTorrentWebUI/node_modules/react-dropzone/dist/index.js
new file mode 100644
index 00000000..1ca5b90c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/dist/index.js
@@ -0,0 +1,1029 @@
+(function webpackUniversalModuleDefinition(root, factory) {
+ if(typeof exports === 'object' && typeof module === 'object')
+ module.exports = factory(require("react"), require("prop-types"));
+ else if(typeof define === 'function' && define.amd)
+ define(["react", "prop-types"], factory);
+ else if(typeof exports === 'object')
+ exports["Dropzone"] = factory(require("react"), require("prop-types"));
+ else
+ root["Dropzone"] = factory(root["react"], root["prop-types"]);
+})(this, function(__WEBPACK_EXTERNAL_MODULE_2__, __WEBPACK_EXTERNAL_MODULE_3__) {
+return /******/ (function(modules) { // webpackBootstrap
+/******/ // The module cache
+/******/ var installedModules = {};
+/******/
+/******/ // The require function
+/******/ function __webpack_require__(moduleId) {
+/******/
+/******/ // Check if module is in cache
+/******/ if(installedModules[moduleId]) {
+/******/ return installedModules[moduleId].exports;
+/******/ }
+/******/ // Create a new module (and put it into the cache)
+/******/ var module = installedModules[moduleId] = {
+/******/ i: moduleId,
+/******/ l: false,
+/******/ exports: {}
+/******/ };
+/******/
+/******/ // Execute the module function
+/******/ modules[moduleId].call(module.exports, module, module.exports, __webpack_require__);
+/******/
+/******/ // Flag the module as loaded
+/******/ module.l = true;
+/******/
+/******/ // Return the exports of the module
+/******/ return module.exports;
+/******/ }
+/******/
+/******/
+/******/ // expose the modules object (__webpack_modules__)
+/******/ __webpack_require__.m = modules;
+/******/
+/******/ // expose the module cache
+/******/ __webpack_require__.c = installedModules;
+/******/
+/******/ // define getter function for harmony exports
+/******/ __webpack_require__.d = function(exports, name, getter) {
+/******/ if(!__webpack_require__.o(exports, name)) {
+/******/ Object.defineProperty(exports, name, {
+/******/ configurable: false,
+/******/ enumerable: true,
+/******/ get: getter
+/******/ });
+/******/ }
+/******/ };
+/******/
+/******/ // getDefaultExport function for compatibility with non-harmony modules
+/******/ __webpack_require__.n = function(module) {
+/******/ var getter = module && module.__esModule ?
+/******/ function getDefault() { return module['default']; } :
+/******/ function getModuleExports() { return module; };
+/******/ __webpack_require__.d(getter, 'a', getter);
+/******/ return getter;
+/******/ };
+/******/
+/******/ // Object.prototype.hasOwnProperty.call
+/******/ __webpack_require__.o = function(object, property) { return Object.prototype.hasOwnProperty.call(object, property); };
+/******/
+/******/ // __webpack_public_path__
+/******/ __webpack_require__.p = "";
+/******/
+/******/ // Load entry module and return exports
+/******/ return __webpack_require__(__webpack_require__.s = 0);
+/******/ })
+/************************************************************************/
+/******/ ([
+/* 0 */
+/***/ (function(module, exports, __webpack_require__) {
+
+"use strict";
+/* WEBPACK VAR INJECTION */(function(process) {
+
+Object.defineProperty(exports, "__esModule", {
+ value: true
+});
+
+var _extends = Object.assign || function (target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i]; for (var key in source) { if (Object.prototype.hasOwnProperty.call(source, key)) { target[key] = source[key]; } } } return target; };
+
+var _createClass = function () { function defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } return function (Constructor, protoProps, staticProps) { if (protoProps) defineProperties(Constructor.prototype, protoProps); if (staticProps) defineProperties(Constructor, staticProps); return Constructor; }; }();
+
+var _react = __webpack_require__(2);
+
+var _react2 = _interopRequireDefault(_react);
+
+var _propTypes = __webpack_require__(3);
+
+var _propTypes2 = _interopRequireDefault(_propTypes);
+
+var _utils = __webpack_require__(4);
+
+var _styles = __webpack_require__(6);
+
+var _styles2 = _interopRequireDefault(_styles);
+
+function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
+
+function _objectWithoutProperties(obj, keys) { var target = {}; for (var i in obj) { if (keys.indexOf(i) >= 0) continue; if (!Object.prototype.hasOwnProperty.call(obj, i)) continue; target[i] = obj[i]; } return target; }
+
+function _toConsumableArray(arr) { if (Array.isArray(arr)) { for (var i = 0, arr2 = Array(arr.length); i < arr.length; i++) { arr2[i] = arr[i]; } return arr2; } else { return Array.from(arr); } }
+
+function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
+
+function _possibleConstructorReturn(self, call) { if (!self) { throw new ReferenceError("this hasn't been initialised - super() hasn't been called"); } return call && (typeof call === "object" || typeof call === "function") ? call : self; }
+
+function _inherits(subClass, superClass) { if (typeof superClass !== "function" && superClass !== null) { throw new TypeError("Super expression must either be null or a function, not " + typeof superClass); } subClass.prototype = Object.create(superClass && superClass.prototype, { constructor: { value: subClass, enumerable: false, writable: true, configurable: true } }); if (superClass) Object.setPrototypeOf ? Object.setPrototypeOf(subClass, superClass) : subClass.__proto__ = superClass; } /* eslint prefer-template: 0 */
+
+var Dropzone = function (_React$Component) {
+ _inherits(Dropzone, _React$Component);
+
+ function Dropzone(props, context) {
+ _classCallCheck(this, Dropzone);
+
+ var _this = _possibleConstructorReturn(this, (Dropzone.__proto__ || Object.getPrototypeOf(Dropzone)).call(this, props, context));
+
+ _this.renderChildren = function (children, isDragActive, isDragAccept, isDragReject) {
+ if (typeof children === 'function') {
+ return children(_extends({}, _this.state, {
+ isDragActive: isDragActive,
+ isDragAccept: isDragAccept,
+ isDragReject: isDragReject
+ }));
+ }
+ return children;
+ };
+
+ _this.composeHandlers = _this.composeHandlers.bind(_this);
+ _this.onClick = _this.onClick.bind(_this);
+ _this.onDocumentDrop = _this.onDocumentDrop.bind(_this);
+ _this.onDragEnter = _this.onDragEnter.bind(_this);
+ _this.onDragLeave = _this.onDragLeave.bind(_this);
+ _this.onDragOver = _this.onDragOver.bind(_this);
+ _this.onDragStart = _this.onDragStart.bind(_this);
+ _this.onDrop = _this.onDrop.bind(_this);
+ _this.onFileDialogCancel = _this.onFileDialogCancel.bind(_this);
+ _this.onInputElementClick = _this.onInputElementClick.bind(_this);
+
+ _this.setRef = _this.setRef.bind(_this);
+ _this.setRefs = _this.setRefs.bind(_this);
+
+ _this.isFileDialogActive = false;
+
+ _this.state = {
+ draggedFiles: [],
+ acceptedFiles: [],
+ rejectedFiles: []
+ };
+ return _this;
+ }
+
+ _createClass(Dropzone, [{
+ key: 'componentDidMount',
+ value: function componentDidMount() {
+ var preventDropOnDocument = this.props.preventDropOnDocument;
+
+ this.dragTargets = [];
+
+ if (preventDropOnDocument) {
+ document.addEventListener('dragover', _utils.onDocumentDragOver, false);
+ document.addEventListener('drop', this.onDocumentDrop, false);
+ }
+ this.fileInputEl.addEventListener('click', this.onInputElementClick, false);
+ // Tried implementing addEventListener, but didn't work out
+ document.body.onfocus = this.onFileDialogCancel;
+ }
+ }, {
+ key: 'componentWillUnmount',
+ value: function componentWillUnmount() {
+ var preventDropOnDocument = this.props.preventDropOnDocument;
+
+ if (preventDropOnDocument) {
+ document.removeEventListener('dragover', _utils.onDocumentDragOver);
+ document.removeEventListener('drop', this.onDocumentDrop);
+ }
+ if (this.fileInputEl != null) {
+ this.fileInputEl.removeEventListener('click', this.onInputElementClick, false);
+ }
+ // Can be replaced with removeEventListener, if addEventListener works
+ if (document != null) {
+ document.body.onfocus = null;
+ }
+ }
+ }, {
+ key: 'composeHandlers',
+ value: function composeHandlers(handler) {
+ if (this.props.disabled) {
+ return null;
+ }
+
+ return handler;
+ }
+ }, {
+ key: 'onDocumentDrop',
+ value: function onDocumentDrop(evt) {
+ if (this.node && this.node.contains(evt.target)) {
+ // if we intercepted an event for our instance, let it propagate down to the instance's onDrop handler
+ return;
+ }
+ evt.preventDefault();
+ this.dragTargets = [];
+ }
+ }, {
+ key: 'onDragStart',
+ value: function onDragStart(evt) {
+ if (this.props.onDragStart) {
+ this.props.onDragStart.call(this, evt);
+ }
+ }
+ }, {
+ key: 'onDragEnter',
+ value: function onDragEnter(evt) {
+ evt.preventDefault();
+
+ // Count the dropzone and any children that are entered.
+ if (this.dragTargets.indexOf(evt.target) === -1) {
+ this.dragTargets.push(evt.target);
+ }
+
+ this.setState({
+ isDragActive: true, // Do not rely on files for the drag state. It doesn't work in Safari.
+ draggedFiles: (0, _utils.getDataTransferItems)(evt)
+ });
+
+ if (this.props.onDragEnter) {
+ this.props.onDragEnter.call(this, evt);
+ }
+ }
+ }, {
+ key: 'onDragOver',
+ value: function onDragOver(evt) {
+ // eslint-disable-line class-methods-use-this
+ evt.preventDefault();
+ evt.stopPropagation();
+ try {
+ // The file dialog on Chrome allows users to drag files from the dialog onto
+ // the dropzone, causing the browser the crash when the file dialog is closed.
+ // A drop effect of 'none' prevents the file from being dropped
+ evt.dataTransfer.dropEffect = this.isFileDialogActive ? 'none' : 'copy'; // eslint-disable-line no-param-reassign
+ } catch (err) {
+ // continue regardless of error
+ }
+
+ if (this.props.onDragOver) {
+ this.props.onDragOver.call(this, evt);
+ }
+ return false;
+ }
+ }, {
+ key: 'onDragLeave',
+ value: function onDragLeave(evt) {
+ var _this2 = this;
+
+ evt.preventDefault();
+
+ // Only deactivate once the dropzone and all children have been left.
+ this.dragTargets = this.dragTargets.filter(function (el) {
+ return el !== evt.target && _this2.node.contains(el);
+ });
+ if (this.dragTargets.length > 0) {
+ return;
+ }
+
+ // Clear dragging files state
+ this.setState({
+ isDragActive: false,
+ draggedFiles: []
+ });
+
+ if (this.props.onDragLeave) {
+ this.props.onDragLeave.call(this, evt);
+ }
+ }
+ }, {
+ key: 'onDrop',
+ value: function onDrop(evt) {
+ var _this3 = this;
+
+ var _props = this.props,
+ onDrop = _props.onDrop,
+ onDropAccepted = _props.onDropAccepted,
+ onDropRejected = _props.onDropRejected,
+ multiple = _props.multiple,
+ disablePreview = _props.disablePreview,
+ accept = _props.accept;
+
+ var fileList = (0, _utils.getDataTransferItems)(evt);
+ var acceptedFiles = [];
+ var rejectedFiles = [];
+
+ // Stop default browser behavior
+ evt.preventDefault();
+
+ // Reset the counter along with the drag on a drop.
+ this.dragTargets = [];
+ this.isFileDialogActive = false;
+
+ fileList.forEach(function (file) {
+ if (!disablePreview) {
+ try {
+ file.preview = window.URL.createObjectURL(file); // eslint-disable-line no-param-reassign
+ } catch (err) {
+ if (process.env.NODE_ENV !== 'production') {
+ console.error('Failed to generate preview for file', file, err); // eslint-disable-line no-console
+ }
+ }
+ }
+
+ if ((0, _utils.fileAccepted)(file, accept) && (0, _utils.fileMatchSize)(file, _this3.props.maxSize, _this3.props.minSize)) {
+ acceptedFiles.push(file);
+ } else {
+ rejectedFiles.push(file);
+ }
+ });
+
+ if (!multiple) {
+ // if not in multi mode add any extra accepted files to rejected.
+ // This will allow end users to easily ignore a multi file drop in "single" mode.
+ rejectedFiles.push.apply(rejectedFiles, _toConsumableArray(acceptedFiles.splice(1)));
+ }
+
+ if (onDrop) {
+ onDrop.call(this, acceptedFiles, rejectedFiles, evt);
+ }
+
+ if (rejectedFiles.length > 0 && onDropRejected) {
+ onDropRejected.call(this, rejectedFiles, evt);
+ }
+
+ if (acceptedFiles.length > 0 && onDropAccepted) {
+ onDropAccepted.call(this, acceptedFiles, evt);
+ }
+
+ // Clear files value
+ this.draggedFiles = null;
+
+ // Reset drag state
+ this.setState({
+ isDragActive: false,
+ draggedFiles: [],
+ acceptedFiles: acceptedFiles,
+ rejectedFiles: rejectedFiles
+ });
+ }
+ }, {
+ key: 'onClick',
+ value: function onClick(evt) {
+ var _props2 = this.props,
+ onClick = _props2.onClick,
+ disableClick = _props2.disableClick;
+
+ if (!disableClick) {
+ evt.stopPropagation();
+
+ if (onClick) {
+ onClick.call(this, evt);
+ }
+
+ // in IE11/Edge the file-browser dialog is blocking, ensure this is behind setTimeout
+ // this is so react can handle state changes in the onClick prop above above
+ // see: https://github.com/react-dropzone/react-dropzone/issues/450
+ setTimeout(this.open.bind(this), 0);
+ }
+ }
+ }, {
+ key: 'onInputElementClick',
+ value: function onInputElementClick(evt) {
+ evt.stopPropagation();
+ if (this.props.inputProps && this.props.inputProps.onClick) {
+ this.props.inputProps.onClick();
+ }
+ }
+ }, {
+ key: 'onFileDialogCancel',
+ value: function onFileDialogCancel() {
+ // timeout will not recognize context of this method
+ var onFileDialogCancel = this.props.onFileDialogCancel;
+ var fileInputEl = this.fileInputEl;
+ var isFileDialogActive = this.isFileDialogActive;
+ // execute the timeout only if the onFileDialogCancel is defined and FileDialog
+ // is opened in the browser
+
+ if (onFileDialogCancel && isFileDialogActive) {
+ setTimeout(function () {
+ // Returns an object as FileList
+ var FileList = fileInputEl.files;
+ if (!FileList.length) {
+ isFileDialogActive = false;
+ onFileDialogCancel();
+ }
+ }, 300);
+ }
+ }
+ }, {
+ key: 'setRef',
+ value: function setRef(ref) {
+ this.node = ref;
+ }
+ }, {
+ key: 'setRefs',
+ value: function setRefs(ref) {
+ this.fileInputEl = ref;
+ }
+ /**
+ * Open system file upload dialog.
+ *
+ * @public
+ */
+
+ }, {
+ key: 'open',
+ value: function open() {
+ this.isFileDialogActive = true;
+ this.fileInputEl.value = null;
+ this.fileInputEl.click();
+ }
+ }, {
+ key: 'render',
+ value: function render() {
+ var _props3 = this.props,
+ accept = _props3.accept,
+ acceptClassName = _props3.acceptClassName,
+ activeClassName = _props3.activeClassName,
+ children = _props3.children,
+ disabled = _props3.disabled,
+ disabledClassName = _props3.disabledClassName,
+ inputProps = _props3.inputProps,
+ multiple = _props3.multiple,
+ name = _props3.name,
+ rejectClassName = _props3.rejectClassName,
+ rest = _objectWithoutProperties(_props3, ['accept', 'acceptClassName', 'activeClassName', 'children', 'disabled', 'disabledClassName', 'inputProps', 'multiple', 'name', 'rejectClassName']);
+
+ var acceptStyle = rest.acceptStyle,
+ activeStyle = rest.activeStyle,
+ _rest$className = rest.className,
+ className = _rest$className === undefined ? '' : _rest$className,
+ disabledStyle = rest.disabledStyle,
+ rejectStyle = rest.rejectStyle,
+ style = rest.style,
+ props = _objectWithoutProperties(rest, ['acceptStyle', 'activeStyle', 'className', 'disabledStyle', 'rejectStyle', 'style']);
+
+ var _state = this.state,
+ isDragActive = _state.isDragActive,
+ draggedFiles = _state.draggedFiles;
+
+ var filesCount = draggedFiles.length;
+ var isMultipleAllowed = multiple || filesCount <= 1;
+ var isDragAccept = filesCount > 0 && (0, _utils.allFilesAccepted)(draggedFiles, this.props.accept);
+ var isDragReject = filesCount > 0 && (!isDragAccept || !isMultipleAllowed);
+ var noStyles = !className && !style && !activeStyle && !acceptStyle && !rejectStyle && !disabledStyle;
+
+ if (isDragActive && activeClassName) {
+ className += ' ' + activeClassName;
+ }
+ if (isDragAccept && acceptClassName) {
+ className += ' ' + acceptClassName;
+ }
+ if (isDragReject && rejectClassName) {
+ className += ' ' + rejectClassName;
+ }
+ if (disabled && disabledClassName) {
+ className += ' ' + disabledClassName;
+ }
+
+ if (noStyles) {
+ style = _styles2.default.default;
+ activeStyle = _styles2.default.active;
+ acceptStyle = style.active;
+ rejectStyle = _styles2.default.rejected;
+ disabledStyle = _styles2.default.disabled;
+ }
+
+ var appliedStyle = _extends({}, style);
+ if (activeStyle && isDragActive) {
+ appliedStyle = _extends({}, style, activeStyle);
+ }
+ if (acceptStyle && isDragAccept) {
+ appliedStyle = _extends({}, appliedStyle, acceptStyle);
+ }
+ if (rejectStyle && isDragReject) {
+ appliedStyle = _extends({}, appliedStyle, rejectStyle);
+ }
+ if (disabledStyle && disabled) {
+ appliedStyle = _extends({}, style, disabledStyle);
+ }
+
+ var inputAttributes = {
+ accept: accept,
+ disabled: disabled,
+ type: 'file',
+ style: { display: 'none' },
+ multiple: _utils.supportMultiple && multiple,
+ ref: this.setRefs,
+ onChange: this.onDrop,
+ autoComplete: 'off'
+ };
+
+ if (name && name.length) {
+ inputAttributes.name = name;
+ }
+
+ // Destructure custom props away from props used for the div element
+
+ var acceptedFiles = props.acceptedFiles,
+ preventDropOnDocument = props.preventDropOnDocument,
+ disablePreview = props.disablePreview,
+ disableClick = props.disableClick,
+ onDropAccepted = props.onDropAccepted,
+ onDropRejected = props.onDropRejected,
+ onFileDialogCancel = props.onFileDialogCancel,
+ maxSize = props.maxSize,
+ minSize = props.minSize,
+ divProps = _objectWithoutProperties(props, ['acceptedFiles', 'preventDropOnDocument', 'disablePreview', 'disableClick', 'onDropAccepted', 'onDropRejected', 'onFileDialogCancel', 'maxSize', 'minSize']);
+
+ return _react2.default.createElement(
+ 'div',
+ _extends({
+ className: className,
+ style: appliedStyle
+ }, divProps /* expand user provided props first so event handlers are never overridden */, {
+ onClick: this.composeHandlers(this.onClick),
+ onDragStart: this.composeHandlers(this.onDragStart),
+ onDragEnter: this.composeHandlers(this.onDragEnter),
+ onDragOver: this.composeHandlers(this.onDragOver),
+ onDragLeave: this.composeHandlers(this.onDragLeave),
+ onDrop: this.composeHandlers(this.onDrop),
+ ref: this.setRef,
+ 'aria-disabled': disabled
+ }),
+ this.renderChildren(children, isDragActive, isDragAccept, isDragReject),
+ _react2.default.createElement('input', _extends({}, inputProps /* expand user provided inputProps first so inputAttributes override them */, inputAttributes))
+ );
+ }
+ }]);
+
+ return Dropzone;
+}(_react2.default.Component);
+
+exports.default = Dropzone;
+
+
+Dropzone.propTypes = {
+ /**
+ * Allow specific types of files. See https://github.com/okonet/attr-accept for more information.
+ * Keep in mind that mime type determination is not reliable across platforms. CSV files,
+ * for example, are reported as text/plain under macOS but as application/vnd.ms-excel under
+ * Windows. In some cases there might not be a mime type set at all.
+ * See: https://github.com/react-dropzone/react-dropzone/issues/276
+ */
+ accept: _propTypes2.default.string,
+
+ /**
+ * Contents of the dropzone
+ */
+ children: _propTypes2.default.oneOfType([_propTypes2.default.node, _propTypes2.default.func]),
+
+ /**
+ * Disallow clicking on the dropzone container to open file dialog
+ */
+ disableClick: _propTypes2.default.bool,
+
+ /**
+ * Enable/disable the dropzone entirely
+ */
+ disabled: _propTypes2.default.bool,
+
+ /**
+ * Enable/disable preview generation
+ */
+ disablePreview: _propTypes2.default.bool,
+
+ /**
+ * If false, allow dropped items to take over the current browser window
+ */
+ preventDropOnDocument: _propTypes2.default.bool,
+
+ /**
+ * Pass additional attributes to the ` ` tag
+ */
+ inputProps: _propTypes2.default.object,
+
+ /**
+ * Allow dropping multiple files
+ */
+ multiple: _propTypes2.default.bool,
+
+ /**
+ * `name` attribute for the input tag
+ */
+ name: _propTypes2.default.string,
+
+ /**
+ * Maximum file size
+ */
+ maxSize: _propTypes2.default.number,
+
+ /**
+ * Minimum file size
+ */
+ minSize: _propTypes2.default.number,
+
+ /**
+ * className
+ */
+ className: _propTypes2.default.string,
+
+ /**
+ * className for active state
+ */
+ activeClassName: _propTypes2.default.string,
+
+ /**
+ * className for accepted state
+ */
+ acceptClassName: _propTypes2.default.string,
+
+ /**
+ * className for rejected state
+ */
+ rejectClassName: _propTypes2.default.string,
+
+ /**
+ * className for disabled state
+ */
+ disabledClassName: _propTypes2.default.string,
+
+ /**
+ * CSS styles to apply
+ */
+ style: _propTypes2.default.object,
+
+ /**
+ * CSS styles to apply when drag is active
+ */
+ activeStyle: _propTypes2.default.object,
+
+ /**
+ * CSS styles to apply when drop will be accepted
+ */
+ acceptStyle: _propTypes2.default.object,
+
+ /**
+ * CSS styles to apply when drop will be rejected
+ */
+ rejectStyle: _propTypes2.default.object,
+
+ /**
+ * CSS styles to apply when dropzone is disabled
+ */
+ disabledStyle: _propTypes2.default.object,
+
+ /**
+ * onClick callback
+ * @param {Event} event
+ */
+ onClick: _propTypes2.default.func,
+
+ /**
+ * onDrop callback
+ */
+ onDrop: _propTypes2.default.func,
+
+ /**
+ * onDropAccepted callback
+ */
+ onDropAccepted: _propTypes2.default.func,
+
+ /**
+ * onDropRejected callback
+ */
+ onDropRejected: _propTypes2.default.func,
+
+ /**
+ * onDragStart callback
+ */
+ onDragStart: _propTypes2.default.func,
+
+ /**
+ * onDragEnter callback
+ */
+ onDragEnter: _propTypes2.default.func,
+
+ /**
+ * onDragOver callback
+ */
+ onDragOver: _propTypes2.default.func,
+
+ /**
+ * onDragLeave callback
+ */
+ onDragLeave: _propTypes2.default.func,
+
+ /**
+ * Provide a callback on clicking the cancel button of the file dialog
+ */
+ onFileDialogCancel: _propTypes2.default.func
+};
+
+Dropzone.defaultProps = {
+ preventDropOnDocument: true,
+ disabled: false,
+ disablePreview: false,
+ disableClick: false,
+ multiple: true,
+ maxSize: Infinity,
+ minSize: 0
+};
+module.exports = exports['default'];
+/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(1)))
+
+/***/ }),
+/* 1 */
+/***/ (function(module, exports) {
+
+// shim for using process in browser
+var process = module.exports = {};
+
+// cached from whatever global is present so that test runners that stub it
+// don't break things. But we need to wrap it in a try catch in case it is
+// wrapped in strict mode code which doesn't define any globals. It's inside a
+// function because try/catches deoptimize in certain engines.
+
+var cachedSetTimeout;
+var cachedClearTimeout;
+
+function defaultSetTimout() {
+ throw new Error('setTimeout has not been defined');
+}
+function defaultClearTimeout () {
+ throw new Error('clearTimeout has not been defined');
+}
+(function () {
+ try {
+ if (typeof setTimeout === 'function') {
+ cachedSetTimeout = setTimeout;
+ } else {
+ cachedSetTimeout = defaultSetTimout;
+ }
+ } catch (e) {
+ cachedSetTimeout = defaultSetTimout;
+ }
+ try {
+ if (typeof clearTimeout === 'function') {
+ cachedClearTimeout = clearTimeout;
+ } else {
+ cachedClearTimeout = defaultClearTimeout;
+ }
+ } catch (e) {
+ cachedClearTimeout = defaultClearTimeout;
+ }
+} ())
+function runTimeout(fun) {
+ if (cachedSetTimeout === setTimeout) {
+ //normal enviroments in sane situations
+ return setTimeout(fun, 0);
+ }
+ // if setTimeout wasn't available but was latter defined
+ if ((cachedSetTimeout === defaultSetTimout || !cachedSetTimeout) && setTimeout) {
+ cachedSetTimeout = setTimeout;
+ return setTimeout(fun, 0);
+ }
+ try {
+ // when when somebody has screwed with setTimeout but no I.E. maddness
+ return cachedSetTimeout(fun, 0);
+ } catch(e){
+ try {
+ // When we are in I.E. but the script has been evaled so I.E. doesn't trust the global object when called normally
+ return cachedSetTimeout.call(null, fun, 0);
+ } catch(e){
+ // same as above but when it's a version of I.E. that must have the global object for 'this', hopfully our context correct otherwise it will throw a global error
+ return cachedSetTimeout.call(this, fun, 0);
+ }
+ }
+
+
+}
+function runClearTimeout(marker) {
+ if (cachedClearTimeout === clearTimeout) {
+ //normal enviroments in sane situations
+ return clearTimeout(marker);
+ }
+ // if clearTimeout wasn't available but was latter defined
+ if ((cachedClearTimeout === defaultClearTimeout || !cachedClearTimeout) && clearTimeout) {
+ cachedClearTimeout = clearTimeout;
+ return clearTimeout(marker);
+ }
+ try {
+ // when when somebody has screwed with setTimeout but no I.E. maddness
+ return cachedClearTimeout(marker);
+ } catch (e){
+ try {
+ // When we are in I.E. but the script has been evaled so I.E. doesn't trust the global object when called normally
+ return cachedClearTimeout.call(null, marker);
+ } catch (e){
+ // same as above but when it's a version of I.E. that must have the global object for 'this', hopfully our context correct otherwise it will throw a global error.
+ // Some versions of I.E. have different rules for clearTimeout vs setTimeout
+ return cachedClearTimeout.call(this, marker);
+ }
+ }
+
+
+
+}
+var queue = [];
+var draining = false;
+var currentQueue;
+var queueIndex = -1;
+
+function cleanUpNextTick() {
+ if (!draining || !currentQueue) {
+ return;
+ }
+ draining = false;
+ if (currentQueue.length) {
+ queue = currentQueue.concat(queue);
+ } else {
+ queueIndex = -1;
+ }
+ if (queue.length) {
+ drainQueue();
+ }
+}
+
+function drainQueue() {
+ if (draining) {
+ return;
+ }
+ var timeout = runTimeout(cleanUpNextTick);
+ draining = true;
+
+ var len = queue.length;
+ while(len) {
+ currentQueue = queue;
+ queue = [];
+ while (++queueIndex < len) {
+ if (currentQueue) {
+ currentQueue[queueIndex].run();
+ }
+ }
+ queueIndex = -1;
+ len = queue.length;
+ }
+ currentQueue = null;
+ draining = false;
+ runClearTimeout(timeout);
+}
+
+process.nextTick = function (fun) {
+ var args = new Array(arguments.length - 1);
+ if (arguments.length > 1) {
+ for (var i = 1; i < arguments.length; i++) {
+ args[i - 1] = arguments[i];
+ }
+ }
+ queue.push(new Item(fun, args));
+ if (queue.length === 1 && !draining) {
+ runTimeout(drainQueue);
+ }
+};
+
+// v8 likes predictible objects
+function Item(fun, array) {
+ this.fun = fun;
+ this.array = array;
+}
+Item.prototype.run = function () {
+ this.fun.apply(null, this.array);
+};
+process.title = 'browser';
+process.browser = true;
+process.env = {};
+process.argv = [];
+process.version = ''; // empty string to avoid regexp issues
+process.versions = {};
+
+function noop() {}
+
+process.on = noop;
+process.addListener = noop;
+process.once = noop;
+process.off = noop;
+process.removeListener = noop;
+process.removeAllListeners = noop;
+process.emit = noop;
+process.prependListener = noop;
+process.prependOnceListener = noop;
+
+process.listeners = function (name) { return [] }
+
+process.binding = function (name) {
+ throw new Error('process.binding is not supported');
+};
+
+process.cwd = function () { return '/' };
+process.chdir = function (dir) {
+ throw new Error('process.chdir is not supported');
+};
+process.umask = function() { return 0; };
+
+
+/***/ }),
+/* 2 */
+/***/ (function(module, exports) {
+
+module.exports = __WEBPACK_EXTERNAL_MODULE_2__;
+
+/***/ }),
+/* 3 */
+/***/ (function(module, exports) {
+
+module.exports = __WEBPACK_EXTERNAL_MODULE_3__;
+
+/***/ }),
+/* 4 */
+/***/ (function(module, exports, __webpack_require__) {
+
+"use strict";
+
+
+Object.defineProperty(exports, "__esModule", {
+ value: true
+});
+exports.supportMultiple = undefined;
+exports.getDataTransferItems = getDataTransferItems;
+exports.fileAccepted = fileAccepted;
+exports.fileMatchSize = fileMatchSize;
+exports.allFilesAccepted = allFilesAccepted;
+exports.onDocumentDragOver = onDocumentDragOver;
+
+var _attrAccept = __webpack_require__(5);
+
+var _attrAccept2 = _interopRequireDefault(_attrAccept);
+
+function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
+
+var supportMultiple = exports.supportMultiple = typeof document !== 'undefined' && document && document.createElement ? 'multiple' in document.createElement('input') : true;
+
+function getDataTransferItems(event) {
+ var dataTransferItemsList = [];
+ if (event.dataTransfer) {
+ var dt = event.dataTransfer;
+ if (dt.files && dt.files.length) {
+ dataTransferItemsList = dt.files;
+ } else if (dt.items && dt.items.length) {
+ // During the drag even the dataTransfer.files is null
+ // but Chrome implements some drag store, which is accesible via dataTransfer.items
+ dataTransferItemsList = dt.items;
+ }
+ } else if (event.target && event.target.files) {
+ dataTransferItemsList = event.target.files;
+ }
+ // Convert from DataTransferItemsList to the native Array
+ return Array.prototype.slice.call(dataTransferItemsList);
+}
+
+// Firefox versions prior to 53 return a bogus MIME type for every file drag, so dragovers with
+// that MIME type will always be accepted
+function fileAccepted(file, accept) {
+ return file.type === 'application/x-moz-file' || (0, _attrAccept2.default)(file, accept);
+}
+
+function fileMatchSize(file, maxSize, minSize) {
+ return file.size <= maxSize && file.size >= minSize;
+}
+
+function allFilesAccepted(files, accept) {
+ return files.every(function (file) {
+ return fileAccepted(file, accept);
+ });
+}
+
+// allow the entire document to be a drag target
+function onDocumentDragOver(evt) {
+ evt.preventDefault();
+}
+
+/***/ }),
+/* 5 */
+/***/ (function(module, exports) {
+
+module.exports=function(t){function n(e){if(r[e])return r[e].exports;var o=r[e]={exports:{},id:e,loaded:!1};return t[e].call(o.exports,o,o.exports,n),o.loaded=!0,o.exports}var r={};return n.m=t,n.c=r,n.p="",n(0)}([function(t,n,r){"use strict";n.__esModule=!0,r(8),r(9),n["default"]=function(t,n){if(t&&n){var r=function(){var r=Array.isArray(n)?n:n.split(","),e=t.name||"",o=t.type||"",i=o.replace(/\/.*$/,"");return{v:r.some(function(t){var n=t.trim();return"."===n.charAt(0)?e.toLowerCase().endsWith(n.toLowerCase()):/\/\*$/.test(n)?i===n.replace(/\/.*$/,""):o===n})}}();if("object"==typeof r)return r.v}return!0},t.exports=n["default"]},function(t,n){var r=t.exports={version:"1.2.2"};"number"==typeof __e&&(__e=r)},function(t,n){var r=t.exports="undefined"!=typeof window&&window.Math==Math?window:"undefined"!=typeof self&&self.Math==Math?self:Function("return this")();"number"==typeof __g&&(__g=r)},function(t,n,r){var e=r(2),o=r(1),i=r(4),u=r(19),c="prototype",f=function(t,n){return function(){return t.apply(n,arguments)}},s=function(t,n,r){var a,p,l,y,d=t&s.G,h=t&s.P,v=d?e:t&s.S?e[n]||(e[n]={}):(e[n]||{})[c],x=d?o:o[n]||(o[n]={});d&&(r=n);for(a in r)p=!(t&s.F)&&v&&a in v,l=(p?v:r)[a],y=t&s.B&&p?f(l,e):h&&"function"==typeof l?f(Function.call,l):l,v&&!p&&u(v,a,l),x[a]!=l&&i(x,a,y),h&&((x[c]||(x[c]={}))[a]=l)};e.core=o,s.F=1,s.G=2,s.S=4,s.P=8,s.B=16,s.W=32,t.exports=s},function(t,n,r){var e=r(5),o=r(18);t.exports=r(22)?function(t,n,r){return e.setDesc(t,n,o(1,r))}:function(t,n,r){return t[n]=r,t}},function(t,n){var r=Object;t.exports={create:r.create,getProto:r.getPrototypeOf,isEnum:{}.propertyIsEnumerable,getDesc:r.getOwnPropertyDescriptor,setDesc:r.defineProperty,setDescs:r.defineProperties,getKeys:r.keys,getNames:r.getOwnPropertyNames,getSymbols:r.getOwnPropertySymbols,each:[].forEach}},function(t,n){var r=0,e=Math.random();t.exports=function(t){return"Symbol(".concat(void 0===t?"":t,")_",(++r+e).toString(36))}},function(t,n,r){var e=r(20)("wks"),o=r(2).Symbol;t.exports=function(t){return e[t]||(e[t]=o&&o[t]||(o||r(6))("Symbol."+t))}},function(t,n,r){r(26),t.exports=r(1).Array.some},function(t,n,r){r(25),t.exports=r(1).String.endsWith},function(t,n){t.exports=function(t){if("function"!=typeof t)throw TypeError(t+" is not a function!");return t}},function(t,n){var r={}.toString;t.exports=function(t){return r.call(t).slice(8,-1)}},function(t,n,r){var e=r(10);t.exports=function(t,n,r){if(e(t),void 0===n)return t;switch(r){case 1:return function(r){return t.call(n,r)};case 2:return function(r,e){return t.call(n,r,e)};case 3:return function(r,e,o){return t.call(n,r,e,o)}}return function(){return t.apply(n,arguments)}}},function(t,n){t.exports=function(t){if(void 0==t)throw TypeError("Can't call method on "+t);return t}},function(t,n,r){t.exports=function(t){var n=/./;try{"/./"[t](n)}catch(e){try{return n[r(7)("match")]=!1,!"/./"[t](n)}catch(o){}}return!0}},function(t,n){t.exports=function(t){try{return!!t()}catch(n){return!0}}},function(t,n){t.exports=function(t){return"object"==typeof t?null!==t:"function"==typeof t}},function(t,n,r){var e=r(16),o=r(11),i=r(7)("match");t.exports=function(t){var n;return e(t)&&(void 0!==(n=t[i])?!!n:"RegExp"==o(t))}},function(t,n){t.exports=function(t,n){return{enumerable:!(1&t),configurable:!(2&t),writable:!(4&t),value:n}}},function(t,n,r){var e=r(2),o=r(4),i=r(6)("src"),u="toString",c=Function[u],f=(""+c).split(u);r(1).inspectSource=function(t){return c.call(t)},(t.exports=function(t,n,r,u){"function"==typeof r&&(o(r,i,t[n]?""+t[n]:f.join(String(n))),"name"in r||(r.name=n)),t===e?t[n]=r:(u||delete t[n],o(t,n,r))})(Function.prototype,u,function(){return"function"==typeof this&&this[i]||c.call(this)})},function(t,n,r){var e=r(2),o="__core-js_shared__",i=e[o]||(e[o]={});t.exports=function(t){return i[t]||(i[t]={})}},function(t,n,r){var e=r(17),o=r(13);t.exports=function(t,n,r){if(e(n))throw TypeError("String#"+r+" doesn't accept regex!");return String(o(t))}},function(t,n,r){t.exports=!r(15)(function(){return 7!=Object.defineProperty({},"a",{get:function(){return 7}}).a})},function(t,n){var r=Math.ceil,e=Math.floor;t.exports=function(t){return isNaN(t=+t)?0:(t>0?e:r)(t)}},function(t,n,r){var e=r(23),o=Math.min;t.exports=function(t){return t>0?o(e(t),9007199254740991):0}},function(t,n,r){"use strict";var e=r(3),o=r(24),i=r(21),u="endsWith",c=""[u];e(e.P+e.F*r(14)(u),"String",{endsWith:function(t){var n=i(this,t,u),r=arguments,e=r.length>1?r[1]:void 0,f=o(n.length),s=void 0===e?f:Math.min(o(e),f),a=String(t);return c?c.call(n,a,s):n.slice(s-a.length,s)===a}})},function(t,n,r){var e=r(5),o=r(3),i=r(1).Array||Array,u={},c=function(t,n){e.each.call(t.split(","),function(t){void 0==n&&t in i?u[t]=i[t]:t in[]&&(u[t]=r(12)(Function.call,[][t],n))})};c("pop,reverse,shift,keys,values,entries",1),c("indexOf,every,some,forEach,map,filter,find,findIndex,includes",3),c("join,slice,concat,push,splice,unshift,sort,lastIndexOf,reduce,reduceRight,copyWithin,fill"),o(o.S,"Array",u)}]);
+
+/***/ }),
+/* 6 */
+/***/ (function(module, exports, __webpack_require__) {
+
+"use strict";
+
+
+Object.defineProperty(exports, "__esModule", {
+ value: true
+});
+exports.default = {
+ rejected: {
+ borderStyle: 'solid',
+ borderColor: '#c66',
+ backgroundColor: '#eee'
+ },
+ disabled: {
+ opacity: 0.5
+ },
+ active: {
+ borderStyle: 'solid',
+ borderColor: '#6c6',
+ backgroundColor: '#eee'
+ },
+ default: {
+ width: 200,
+ height: 200,
+ borderWidth: 2,
+ borderColor: '#666',
+ borderStyle: 'dashed',
+ borderRadius: 5
+ }
+};
+module.exports = exports['default'];
+
+/***/ })
+/******/ ]);
+});
+//# sourceMappingURL=index.js.map
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/dist/index.js.map b/goTorrentWebUI/node_modules/react-dropzone/dist/index.js.map
new file mode 100644
index 00000000..74367389
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/dist/index.js.map
@@ -0,0 +1 @@
+{"version":3,"sources":["webpack:///webpack/universalModuleDefinition","webpack:///webpack/bootstrap 5c1f85df70828faca129","webpack:///./src/index.js","webpack:///./node_modules/process/browser.js","webpack:///external \"react\"","webpack:///external \"prop-types\"","webpack:///./src/utils/index.js","webpack:///./node_modules/attr-accept/dist/index.js","webpack:///./src/utils/styles.js"],"names":["Dropzone","props","context","renderChildren","children","isDragActive","isDragAccept","isDragReject","state","composeHandlers","bind","onClick","onDocumentDrop","onDragEnter","onDragLeave","onDragOver","onDragStart","onDrop","onFileDialogCancel","onInputElementClick","setRef","setRefs","isFileDialogActive","draggedFiles","acceptedFiles","rejectedFiles","preventDropOnDocument","dragTargets","document","addEventListener","fileInputEl","body","onfocus","removeEventListener","handler","disabled","evt","node","contains","target","preventDefault","call","indexOf","push","setState","stopPropagation","dataTransfer","dropEffect","err","filter","el","length","onDropAccepted","onDropRejected","multiple","disablePreview","accept","fileList","forEach","file","preview","window","URL","createObjectURL","process","env","NODE_ENV","console","error","maxSize","minSize","splice","disableClick","setTimeout","open","inputProps","FileList","files","ref","value","click","acceptClassName","activeClassName","disabledClassName","name","rejectClassName","rest","acceptStyle","activeStyle","className","disabledStyle","rejectStyle","style","filesCount","isMultipleAllowed","noStyles","default","active","rejected","appliedStyle","inputAttributes","type","display","onChange","autoComplete","divProps","Component","propTypes","string","oneOfType","func","bool","object","number","defaultProps","Infinity","getDataTransferItems","fileAccepted","fileMatchSize","allFilesAccepted","onDocumentDragOver","supportMultiple","createElement","event","dataTransferItemsList","dt","items","Array","prototype","slice","size","every","borderStyle","borderColor","backgroundColor","opacity","width","height","borderWidth","borderRadius"],"mappings":"AAAA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA,CAAC;AACD,O;ACVA;AACA;;AAEA;AACA;;AAEA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;;AAEA;AACA;;AAEA;AACA;;AAEA;AACA;AACA;;;AAGA;AACA;;AAEA;AACA;;AAEA;AACA;AACA;AACA;AACA;AACA;AACA;AACA,aAAK;AACL;AACA;;AAEA;AACA;AACA;AACA,mCAA2B,0BAA0B,EAAE;AACvD,yCAAiC,eAAe;AAChD;AACA;AACA;;AAEA;AACA,8DAAsD,+DAA+D;;AAErH;AACA;;AAEA;AACA;;;;;;;;;;;;;;;;;;AC3DA;;;;AACA;;;;AACA;;AAQA;;;;;;;;;;;;;;+eAZA;;IAcMA,Q;;;AACJ,oBAAYC,KAAZ,EAAmBC,OAAnB,EAA4B;AAAA;;AAAA,oHACpBD,KADoB,EACbC,OADa;;AAAA,UAiQ5BC,cAjQ4B,GAiQX,UAACC,QAAD,EAAWC,YAAX,EAAyBC,YAAzB,EAAuCC,YAAvC,EAAwD;AACvE,UAAI,OAAOH,QAAP,KAAoB,UAAxB,EAAoC;AAClC,eAAOA,sBACF,MAAKI,KADH;AAELH,oCAFK;AAGLC,oCAHK;AAILC;AAJK,WAAP;AAMD;AACD,aAAOH,QAAP;AACD,KA3Q2B;;AAE1B,UAAKK,eAAL,GAAuB,MAAKA,eAAL,CAAqBC,IAArB,OAAvB;AACA,UAAKC,OAAL,GAAe,MAAKA,OAAL,CAAaD,IAAb,OAAf;AACA,UAAKE,cAAL,GAAsB,MAAKA,cAAL,CAAoBF,IAApB,OAAtB;AACA,UAAKG,WAAL,GAAmB,MAAKA,WAAL,CAAiBH,IAAjB,OAAnB;AACA,UAAKI,WAAL,GAAmB,MAAKA,WAAL,CAAiBJ,IAAjB,OAAnB;AACA,UAAKK,UAAL,GAAkB,MAAKA,UAAL,CAAgBL,IAAhB,OAAlB;AACA,UAAKM,WAAL,GAAmB,MAAKA,WAAL,CAAiBN,IAAjB,OAAnB;AACA,UAAKO,MAAL,GAAc,MAAKA,MAAL,CAAYP,IAAZ,OAAd;AACA,UAAKQ,kBAAL,GAA0B,MAAKA,kBAAL,CAAwBR,IAAxB,OAA1B;AACA,UAAKS,mBAAL,GAA2B,MAAKA,mBAAL,CAAyBT,IAAzB,OAA3B;;AAEA,UAAKU,MAAL,GAAc,MAAKA,MAAL,CAAYV,IAAZ,OAAd;AACA,UAAKW,OAAL,GAAe,MAAKA,OAAL,CAAaX,IAAb,OAAf;;AAEA,UAAKY,kBAAL,GAA0B,KAA1B;;AAEA,UAAKd,KAAL,GAAa;AACXe,oBAAc,EADH;AAEXC,qBAAe,EAFJ;AAGXC,qBAAe;AAHJ,KAAb;AAlB0B;AAuB3B;;;;wCAEmB;AAAA,UACVC,qBADU,GACgB,KAAKzB,KADrB,CACVyB,qBADU;;AAElB,WAAKC,WAAL,GAAmB,EAAnB;;AAEA,UAAID,qBAAJ,EAA2B;AACzBE,iBAASC,gBAAT,CAA0B,UAA1B,6BAA0D,KAA1D;AACAD,iBAASC,gBAAT,CAA0B,MAA1B,EAAkC,KAAKjB,cAAvC,EAAuD,KAAvD;AACD;AACD,WAAKkB,WAAL,CAAiBD,gBAAjB,CAAkC,OAAlC,EAA2C,KAAKV,mBAAhD,EAAqE,KAArE;AACA;AACAS,eAASG,IAAT,CAAcC,OAAd,GAAwB,KAAKd,kBAA7B;AACD;;;2CAEsB;AAAA,UACbQ,qBADa,GACa,KAAKzB,KADlB,CACbyB,qBADa;;AAErB,UAAIA,qBAAJ,EAA2B;AACzBE,iBAASK,mBAAT,CAA6B,UAA7B;AACAL,iBAASK,mBAAT,CAA6B,MAA7B,EAAqC,KAAKrB,cAA1C;AACD;AACD,UAAI,KAAKkB,WAAL,IAAoB,IAAxB,EAA8B;AAC5B,aAAKA,WAAL,CAAiBG,mBAAjB,CAAqC,OAArC,EAA8C,KAAKd,mBAAnD,EAAwE,KAAxE;AACD;AACD;AACA,UAAIS,YAAY,IAAhB,EAAsB;AACpBA,iBAASG,IAAT,CAAcC,OAAd,GAAwB,IAAxB;AACD;AACF;;;oCAEeE,O,EAAS;AACvB,UAAI,KAAKjC,KAAL,CAAWkC,QAAf,EAAyB;AACvB,eAAO,IAAP;AACD;;AAED,aAAOD,OAAP;AACD;;;mCAEcE,G,EAAK;AAClB,UAAI,KAAKC,IAAL,IAAa,KAAKA,IAAL,CAAUC,QAAV,CAAmBF,IAAIG,MAAvB,CAAjB,EAAiD;AAC/C;AACA;AACD;AACDH,UAAII,cAAJ;AACA,WAAKb,WAAL,GAAmB,EAAnB;AACD;;;gCAEWS,G,EAAK;AACf,UAAI,KAAKnC,KAAL,CAAWe,WAAf,EAA4B;AAC1B,aAAKf,KAAL,CAAWe,WAAX,CAAuByB,IAAvB,CAA4B,IAA5B,EAAkCL,GAAlC;AACD;AACF;;;gCAEWA,G,EAAK;AACfA,UAAII,cAAJ;;AAEA;AACA,UAAI,KAAKb,WAAL,CAAiBe,OAAjB,CAAyBN,IAAIG,MAA7B,MAAyC,CAAC,CAA9C,EAAiD;AAC/C,aAAKZ,WAAL,CAAiBgB,IAAjB,CAAsBP,IAAIG,MAA1B;AACD;;AAED,WAAKK,QAAL,CAAc;AACZvC,sBAAc,IADF,EACQ;AACpBkB,sBAAc,iCAAqBa,GAArB;AAFF,OAAd;;AAKA,UAAI,KAAKnC,KAAL,CAAWY,WAAf,EAA4B;AAC1B,aAAKZ,KAAL,CAAWY,WAAX,CAAuB4B,IAAvB,CAA4B,IAA5B,EAAkCL,GAAlC;AACD;AACF;;;+BAEUA,G,EAAK;AACd;AACAA,UAAII,cAAJ;AACAJ,UAAIS,eAAJ;AACA,UAAI;AACF;AACA;AACA;AACAT,YAAIU,YAAJ,CAAiBC,UAAjB,GAA8B,KAAKzB,kBAAL,GAA0B,MAA1B,GAAmC,MAAjE,CAJE,CAIsE;AACzE,OALD,CAKE,OAAO0B,GAAP,EAAY;AACZ;AACD;;AAED,UAAI,KAAK/C,KAAL,CAAWc,UAAf,EAA2B;AACzB,aAAKd,KAAL,CAAWc,UAAX,CAAsB0B,IAAtB,CAA2B,IAA3B,EAAiCL,GAAjC;AACD;AACD,aAAO,KAAP;AACD;;;gCAEWA,G,EAAK;AAAA;;AACfA,UAAII,cAAJ;;AAEA;AACA,WAAKb,WAAL,GAAmB,KAAKA,WAAL,CAAiBsB,MAAjB,CAAwB;AAAA,eAAMC,OAAOd,IAAIG,MAAX,IAAqB,OAAKF,IAAL,CAAUC,QAAV,CAAmBY,EAAnB,CAA3B;AAAA,OAAxB,CAAnB;AACA,UAAI,KAAKvB,WAAL,CAAiBwB,MAAjB,GAA0B,CAA9B,EAAiC;AAC/B;AACD;;AAED;AACA,WAAKP,QAAL,CAAc;AACZvC,sBAAc,KADF;AAEZkB,sBAAc;AAFF,OAAd;;AAKA,UAAI,KAAKtB,KAAL,CAAWa,WAAf,EAA4B;AAC1B,aAAKb,KAAL,CAAWa,WAAX,CAAuB2B,IAAvB,CAA4B,IAA5B,EAAkCL,GAAlC;AACD;AACF;;;2BAEMA,G,EAAK;AAAA;;AAAA,mBAC2E,KAAKnC,KADhF;AAAA,UACFgB,MADE,UACFA,MADE;AAAA,UACMmC,cADN,UACMA,cADN;AAAA,UACsBC,cADtB,UACsBA,cADtB;AAAA,UACsCC,QADtC,UACsCA,QADtC;AAAA,UACgDC,cADhD,UACgDA,cADhD;AAAA,UACgEC,MADhE,UACgEA,MADhE;;AAEV,UAAMC,WAAW,iCAAqBrB,GAArB,CAAjB;AACA,UAAMZ,gBAAgB,EAAtB;AACA,UAAMC,gBAAgB,EAAtB;;AAEA;AACAW,UAAII,cAAJ;;AAEA;AACA,WAAKb,WAAL,GAAmB,EAAnB;AACA,WAAKL,kBAAL,GAA0B,KAA1B;;AAEAmC,eAASC,OAAT,CAAiB,gBAAQ;AACvB,YAAI,CAACH,cAAL,EAAqB;AACnB,cAAI;AACFI,iBAAKC,OAAL,GAAeC,OAAOC,GAAP,CAAWC,eAAX,CAA2BJ,IAA3B,CAAf,CADE,CAC8C;AACjD,WAFD,CAEE,OAAOX,GAAP,EAAY;AACZ,gBAAIgB,QAAQC,GAAR,CAAYC,QAAZ,KAAyB,YAA7B,EAA2C;AACzCC,sBAAQC,KAAR,CAAc,qCAAd,EAAqDT,IAArD,EAA2DX,GAA3D,EADyC,CACuB;AACjE;AACF;AACF;;AAED,YACE,yBAAaW,IAAb,EAAmBH,MAAnB,KACA,0BAAcG,IAAd,EAAoB,OAAK1D,KAAL,CAAWoE,OAA/B,EAAwC,OAAKpE,KAAL,CAAWqE,OAAnD,CAFF,EAGE;AACA9C,wBAAcmB,IAAd,CAAmBgB,IAAnB;AACD,SALD,MAKO;AACLlC,wBAAckB,IAAd,CAAmBgB,IAAnB;AACD;AACF,OAnBD;;AAqBA,UAAI,CAACL,QAAL,EAAe;AACb;AACA;AACA7B,sBAAckB,IAAd,yCAAsBnB,cAAc+C,MAAd,CAAqB,CAArB,CAAtB;AACD;;AAED,UAAItD,MAAJ,EAAY;AACVA,eAAOwB,IAAP,CAAY,IAAZ,EAAkBjB,aAAlB,EAAiCC,aAAjC,EAAgDW,GAAhD;AACD;;AAED,UAAIX,cAAc0B,MAAd,GAAuB,CAAvB,IAA4BE,cAAhC,EAAgD;AAC9CA,uBAAeZ,IAAf,CAAoB,IAApB,EAA0BhB,aAA1B,EAAyCW,GAAzC;AACD;;AAED,UAAIZ,cAAc2B,MAAd,GAAuB,CAAvB,IAA4BC,cAAhC,EAAgD;AAC9CA,uBAAeX,IAAf,CAAoB,IAApB,EAA0BjB,aAA1B,EAAyCY,GAAzC;AACD;;AAED;AACA,WAAKb,YAAL,GAAoB,IAApB;;AAEA;AACA,WAAKqB,QAAL,CAAc;AACZvC,sBAAc,KADF;AAEZkB,sBAAc,EAFF;AAGZC,oCAHY;AAIZC;AAJY,OAAd;AAMD;;;4BAEOW,G,EAAK;AAAA,oBACuB,KAAKnC,KAD5B;AAAA,UACHU,OADG,WACHA,OADG;AAAA,UACM6D,YADN,WACMA,YADN;;AAEX,UAAI,CAACA,YAAL,EAAmB;AACjBpC,YAAIS,eAAJ;;AAEA,YAAIlC,OAAJ,EAAa;AACXA,kBAAQ8B,IAAR,CAAa,IAAb,EAAmBL,GAAnB;AACD;;AAED;AACA;AACA;AACAqC,mBAAW,KAAKC,IAAL,CAAUhE,IAAV,CAAe,IAAf,CAAX,EAAiC,CAAjC;AACD;AACF;;;wCAEmB0B,G,EAAK;AACvBA,UAAIS,eAAJ;AACA,UAAI,KAAK5C,KAAL,CAAW0E,UAAX,IAAyB,KAAK1E,KAAL,CAAW0E,UAAX,CAAsBhE,OAAnD,EAA4D;AAC1D,aAAKV,KAAL,CAAW0E,UAAX,CAAsBhE,OAAtB;AACD;AACF;;;yCAEoB;AACnB;AADmB,UAEXO,kBAFW,GAEY,KAAKjB,KAFjB,CAEXiB,kBAFW;AAAA,UAGXY,WAHW,GAGK,IAHL,CAGXA,WAHW;AAAA,UAIbR,kBAJa,GAIU,IAJV,CAIbA,kBAJa;AAKnB;AACA;;AACA,UAAIJ,sBAAsBI,kBAA1B,EAA8C;AAC5CmD,mBAAW,YAAM;AACf;AACA,cAAMG,WAAW9C,YAAY+C,KAA7B;AACA,cAAI,CAACD,SAASzB,MAAd,EAAsB;AACpB7B,iCAAqB,KAArB;AACAJ;AACD;AACF,SAPD,EAOG,GAPH;AAQD;AACF;;;2BAEM4D,G,EAAK;AACV,WAAKzC,IAAL,GAAYyC,GAAZ;AACD;;;4BAEOA,G,EAAK;AACX,WAAKhD,WAAL,GAAmBgD,GAAnB;AACD;AACD;;;;;;;;2BAKO;AACL,WAAKxD,kBAAL,GAA0B,IAA1B;AACA,WAAKQ,WAAL,CAAiBiD,KAAjB,GAAyB,IAAzB;AACA,WAAKjD,WAAL,CAAiBkD,KAAjB;AACD;;;6BAcQ;AAAA,oBAaH,KAAK/E,KAbF;AAAA,UAELuD,MAFK,WAELA,MAFK;AAAA,UAGLyB,eAHK,WAGLA,eAHK;AAAA,UAILC,eAJK,WAILA,eAJK;AAAA,UAKL9E,QALK,WAKLA,QALK;AAAA,UAML+B,QANK,WAMLA,QANK;AAAA,UAOLgD,iBAPK,WAOLA,iBAPK;AAAA,UAQLR,UARK,WAQLA,UARK;AAAA,UASLrB,QATK,WASLA,QATK;AAAA,UAUL8B,IAVK,WAULA,IAVK;AAAA,UAWLC,eAXK,WAWLA,eAXK;AAAA,UAYFC,IAZE;;AAAA,UAgBLC,WAhBK,GAuBHD,IAvBG,CAgBLC,WAhBK;AAAA,UAiBLC,WAjBK,GAuBHF,IAvBG,CAiBLE,WAjBK;AAAA,4BAuBHF,IAvBG,CAkBLG,SAlBK;AAAA,UAkBLA,SAlBK,mCAkBO,EAlBP;AAAA,UAmBLC,aAnBK,GAuBHJ,IAvBG,CAmBLI,aAnBK;AAAA,UAoBLC,WApBK,GAuBHL,IAvBG,CAoBLK,WApBK;AAAA,UAqBLC,KArBK,GAuBHN,IAvBG,CAqBLM,KArBK;AAAA,UAsBF3F,KAtBE,4BAuBHqF,IAvBG;;AAAA,mBAyBgC,KAAK9E,KAzBrC;AAAA,UAyBCH,YAzBD,UAyBCA,YAzBD;AAAA,UAyBekB,YAzBf,UAyBeA,YAzBf;;AA0BP,UAAMsE,aAAatE,aAAa4B,MAAhC;AACA,UAAM2C,oBAAoBxC,YAAYuC,cAAc,CAApD;AACA,UAAMvF,eAAeuF,aAAa,CAAb,IAAkB,6BAAiBtE,YAAjB,EAA+B,KAAKtB,KAAL,CAAWuD,MAA1C,CAAvC;AACA,UAAMjD,eAAesF,aAAa,CAAb,KAAmB,CAACvF,YAAD,IAAiB,CAACwF,iBAArC,CAArB;AACA,UAAMC,WACJ,CAACN,SAAD,IAAc,CAACG,KAAf,IAAwB,CAACJ,WAAzB,IAAwC,CAACD,WAAzC,IAAwD,CAACI,WAAzD,IAAwE,CAACD,aAD3E;;AAGA,UAAIrF,gBAAgB6E,eAApB,EAAqC;AACnCO,qBAAa,MAAMP,eAAnB;AACD;AACD,UAAI5E,gBAAgB2E,eAApB,EAAqC;AACnCQ,qBAAa,MAAMR,eAAnB;AACD;AACD,UAAI1E,gBAAgB8E,eAApB,EAAqC;AACnCI,qBAAa,MAAMJ,eAAnB;AACD;AACD,UAAIlD,YAAYgD,iBAAhB,EAAmC;AACjCM,qBAAa,MAAMN,iBAAnB;AACD;;AAED,UAAIY,QAAJ,EAAc;AACZH,gBAAQ,iBAAOI,OAAf;AACAR,sBAAc,iBAAOS,MAArB;AACAV,sBAAcK,MAAMK,MAApB;AACAN,sBAAc,iBAAOO,QAArB;AACAR,wBAAgB,iBAAOvD,QAAvB;AACD;;AAED,UAAIgE,4BAAoBP,KAApB,CAAJ;AACA,UAAIJ,eAAenF,YAAnB,EAAiC;AAC/B8F,oCACKP,KADL,EAEKJ,WAFL;AAID;AACD,UAAID,eAAejF,YAAnB,EAAiC;AAC/B6F,oCACKA,YADL,EAEKZ,WAFL;AAID;AACD,UAAII,eAAepF,YAAnB,EAAiC;AAC/B4F,oCACKA,YADL,EAEKR,WAFL;AAID;AACD,UAAID,iBAAiBvD,QAArB,EAA+B;AAC7BgE,oCACKP,KADL,EAEKF,aAFL;AAID;;AAED,UAAMU,kBAAkB;AACtB5C,sBADsB;AAEtBrB,0BAFsB;AAGtBkE,cAAM,MAHgB;AAItBT,eAAO,EAAEU,SAAS,MAAX,EAJe;AAKtBhD,kBAAU,0BAAmBA,QALP;AAMtBwB,aAAK,KAAKzD,OANY;AAOtBkF,kBAAU,KAAKtF,MAPO;AAQtBuF,sBAAc;AARQ,OAAxB;;AAWA,UAAIpB,QAAQA,KAAKjC,MAAjB,EAAyB;AACvBiD,wBAAgBhB,IAAhB,GAAuBA,IAAvB;AACD;;AAED;;AA/FO,UAiGL5D,aAjGK,GA2GHvB,KA3GG,CAiGLuB,aAjGK;AAAA,UAkGLE,qBAlGK,GA2GHzB,KA3GG,CAkGLyB,qBAlGK;AAAA,UAmGL6B,cAnGK,GA2GHtD,KA3GG,CAmGLsD,cAnGK;AAAA,UAoGLiB,YApGK,GA2GHvE,KA3GG,CAoGLuE,YApGK;AAAA,UAqGLpB,cArGK,GA2GHnD,KA3GG,CAqGLmD,cArGK;AAAA,UAsGLC,cAtGK,GA2GHpD,KA3GG,CAsGLoD,cAtGK;AAAA,UAuGLnC,kBAvGK,GA2GHjB,KA3GG,CAuGLiB,kBAvGK;AAAA,UAwGLmD,OAxGK,GA2GHpE,KA3GG,CAwGLoE,OAxGK;AAAA,UAyGLC,OAzGK,GA2GHrE,KA3GG,CAyGLqE,OAzGK;AAAA,UA0GFmC,QA1GE,4BA2GHxG,KA3GG;;AA6GP,aACE;AAAA;AAAA;AACE,qBAAWwF,SADb;AAEE,iBAAOU;AAFT,WAGMM,QAHN,CAGe,6EAHf;AAIE,mBAAS,KAAKhG,eAAL,CAAqB,KAAKE,OAA1B,CAJX;AAKE,uBAAa,KAAKF,eAAL,CAAqB,KAAKO,WAA1B,CALf;AAME,uBAAa,KAAKP,eAAL,CAAqB,KAAKI,WAA1B,CANf;AAOE,sBAAY,KAAKJ,eAAL,CAAqB,KAAKM,UAA1B,CAPd;AAQE,uBAAa,KAAKN,eAAL,CAAqB,KAAKK,WAA1B,CARf;AASE,kBAAQ,KAAKL,eAAL,CAAqB,KAAKQ,MAA1B,CATV;AAUE,eAAK,KAAKG,MAVZ;AAWE,2BAAee;AAXjB;AAaG,aAAKhC,cAAL,CAAoBC,QAApB,EAA8BC,YAA9B,EAA4CC,YAA5C,EAA0DC,YAA1D,CAbH;AAcE,4DACMoE,UADN,CACiB,4EADjB,EAEMyB,eAFN;AAdF,OADF;AAqBD;;;;EAhZoB,gBAAMM,S;;kBAmZd1G,Q;;;AAEfA,SAAS2G,SAAT,GAAqB;AACnB;;;;;;;AAOAnD,UAAQ,oBAAUoD,MARC;;AAUnB;;;AAGAxG,YAAU,oBAAUyG,SAAV,CAAoB,CAAC,oBAAUxE,IAAX,EAAiB,oBAAUyE,IAA3B,CAApB,CAbS;;AAenB;;;AAGAtC,gBAAc,oBAAUuC,IAlBL;;AAoBnB;;;AAGA5E,YAAU,oBAAU4E,IAvBD;;AAyBnB;;;AAGAxD,kBAAgB,oBAAUwD,IA5BP;;AA8BnB;;;AAGArF,yBAAuB,oBAAUqF,IAjCd;;AAmCnB;;;AAGApC,cAAY,oBAAUqC,MAtCH;;AAwCnB;;;AAGA1D,YAAU,oBAAUyD,IA3CD;;AA6CnB;;;AAGA3B,QAAM,oBAAUwB,MAhDG;;AAkDnB;;;AAGAvC,WAAS,oBAAU4C,MArDA;;AAuDnB;;;AAGA3C,WAAS,oBAAU2C,MA1DA;;AA4DnB;;;AAGAxB,aAAW,oBAAUmB,MA/DF;;AAiEnB;;;AAGA1B,mBAAiB,oBAAU0B,MApER;;AAsEnB;;;AAGA3B,mBAAiB,oBAAU2B,MAzER;;AA2EnB;;;AAGAvB,mBAAiB,oBAAUuB,MA9ER;;AAgFnB;;;AAGAzB,qBAAmB,oBAAUyB,MAnFV;;AAqFnB;;;AAGAhB,SAAO,oBAAUoB,MAxFE;;AA0FnB;;;AAGAxB,eAAa,oBAAUwB,MA7FJ;;AA+FnB;;;AAGAzB,eAAa,oBAAUyB,MAlGJ;;AAoGnB;;;AAGArB,eAAa,oBAAUqB,MAvGJ;;AAyGnB;;;AAGAtB,iBAAe,oBAAUsB,MA5GN;;AA8GnB;;;;AAIArG,WAAS,oBAAUmG,IAlHA;;AAoHnB;;;AAGA7F,UAAQ,oBAAU6F,IAvHC;;AAyHnB;;;AAGA1D,kBAAgB,oBAAU0D,IA5HP;;AA8HnB;;;AAGAzD,kBAAgB,oBAAUyD,IAjIP;;AAmInB;;;AAGA9F,eAAa,oBAAU8F,IAtIJ;;AAwInB;;;AAGAjG,eAAa,oBAAUiG,IA3IJ;;AA6InB;;;AAGA/F,cAAY,oBAAU+F,IAhJH;;AAkJnB;;;AAGAhG,eAAa,oBAAUgG,IArJJ;;AAuJnB;;;AAGA5F,sBAAoB,oBAAU4F;AA1JX,CAArB;;AA6JA9G,SAASkH,YAAT,GAAwB;AACtBxF,yBAAuB,IADD;AAEtBS,YAAU,KAFY;AAGtBoB,kBAAgB,KAHM;AAItBiB,gBAAc,KAJQ;AAKtBlB,YAAU,IALY;AAMtBe,WAAS8C,QANa;AAOtB7C,WAAS;AAPa,CAAxB;;;;;;;;AChkBA;AACA;;AAEA;AACA;AACA;AACA;;AAEA;AACA;;AAEA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA,SAAS;AACT;AACA;AACA,KAAK;AACL;AACA;AACA;AACA;AACA;AACA,SAAS;AACT;AACA;AACA,KAAK;AACL;AACA;AACA,CAAC;AACD;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA,KAAK;AACL;AACA;AACA;AACA,SAAS;AACT;AACA;AACA;AACA;;;AAGA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA,KAAK;AACL;AACA;AACA;AACA,SAAS;AACT;AACA;AACA;AACA;AACA;;;;AAIA;AACA;AACA;AACA;AACA;;AAEA;AACA;AACA;AACA;AACA;AACA;AACA;AACA,KAAK;AACL;AACA;AACA;AACA;AACA;AACA;;AAEA;AACA;AACA;AACA;AACA;AACA;;AAEA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;;AAEA;AACA;AACA;AACA,uBAAuB,sBAAsB;AAC7C;AACA;AACA;AACA;AACA;AACA;AACA;AACA;;AAEA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA,qBAAqB;AACrB;;AAEA;;AAEA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;;AAEA,qCAAqC;;AAErC;AACA;AACA;;AAEA,2BAA2B;AAC3B;AACA;AACA;AACA,4BAA4B,UAAU;;;;;;;ACvLtC,+C;;;;;;ACAA,+C;;;;;;;;;;;;;QCOgB8C,oB,GAAAA,oB;QAoBAC,Y,GAAAA,Y;QAIAC,a,GAAAA,a;QAIAC,gB,GAAAA,gB;QAKAC,kB,GAAAA,kB;;AAxChB;;;;;;AAEO,IAAMC,4CACX,OAAO7F,QAAP,KAAoB,WAApB,IAAmCA,QAAnC,IAA+CA,SAAS8F,aAAxD,GACI,cAAc9F,SAAS8F,aAAT,CAAuB,OAAvB,CADlB,GAEI,IAHC;;AAKA,SAASN,oBAAT,CAA8BO,KAA9B,EAAqC;AAC1C,MAAIC,wBAAwB,EAA5B;AACA,MAAID,MAAM7E,YAAV,EAAwB;AACtB,QAAM+E,KAAKF,MAAM7E,YAAjB;AACA,QAAI+E,GAAGhD,KAAH,IAAYgD,GAAGhD,KAAH,CAAS1B,MAAzB,EAAiC;AAC/ByE,8BAAwBC,GAAGhD,KAA3B;AACD,KAFD,MAEO,IAAIgD,GAAGC,KAAH,IAAYD,GAAGC,KAAH,CAAS3E,MAAzB,EAAiC;AACtC;AACA;AACAyE,8BAAwBC,GAAGC,KAA3B;AACD;AACF,GATD,MASO,IAAIH,MAAMpF,MAAN,IAAgBoF,MAAMpF,MAAN,CAAasC,KAAjC,EAAwC;AAC7C+C,4BAAwBD,MAAMpF,MAAN,CAAasC,KAArC;AACD;AACD;AACA,SAAOkD,MAAMC,SAAN,CAAgBC,KAAhB,CAAsBxF,IAAtB,CAA2BmF,qBAA3B,CAAP;AACD;;AAED;AACA;AACO,SAASP,YAAT,CAAsB1D,IAAtB,EAA4BH,MAA5B,EAAoC;AACzC,SAAOG,KAAK0C,IAAL,KAAc,wBAAd,IAA0C,0BAAQ1C,IAAR,EAAcH,MAAd,CAAjD;AACD;;AAEM,SAAS8D,aAAT,CAAuB3D,IAAvB,EAA6BU,OAA7B,EAAsCC,OAAtC,EAA+C;AACpD,SAAOX,KAAKuE,IAAL,IAAa7D,OAAb,IAAwBV,KAAKuE,IAAL,IAAa5D,OAA5C;AACD;;AAEM,SAASiD,gBAAT,CAA0B1C,KAA1B,EAAiCrB,MAAjC,EAAyC;AAC9C,SAAOqB,MAAMsD,KAAN,CAAY;AAAA,WAAQd,aAAa1D,IAAb,EAAmBH,MAAnB,CAAR;AAAA,GAAZ,CAAP;AACD;;AAED;AACO,SAASgE,kBAAT,CAA4BpF,GAA5B,EAAiC;AACtCA,MAAII,cAAJ;AACD,C;;;;;;AC1CD,2BAA2B,cAAc,4BAA4B,YAAY,UAAU,iBAAiB,gEAAgE,SAAS,+BAA+B,kBAAkB,aAAa,qDAAqD,SAAS,iBAAiB,wFAAwF,OAAO,qBAAqB,eAAe,kHAAkH,GAAG,GAAG,iCAAiC,SAAS,wBAAwB,eAAe,iBAAiB,iBAAiB,8BAA8B,eAAe,8IAA8I,8BAA8B,iBAAiB,+DAA+D,kBAAkB,6BAA6B,mBAAmB,sDAAsD,WAAW,yBAAyB,EAAE,SAAS,kKAAkK,UAAU,2DAA2D,iBAAiB,mBAAmB,gCAAgC,6BAA6B,iBAAiB,iBAAiB,eAAe,aAAa,WAAW,mDAAmD,gNAAgN,eAAe,wBAAwB,sBAAsB,mEAAmE,iBAAiB,iCAAiC,sBAAsB,qDAAqD,iBAAiB,gCAAgC,iBAAiB,qCAAqC,eAAe,sBAAsB,iEAAiE,UAAU,eAAe,QAAQ,UAAU,sBAAsB,8BAA8B,iBAAiB,YAAY,0BAA0B,4BAA4B,UAAU,0BAA0B,oBAAoB,4BAA4B,sBAAsB,8BAA8B,wBAAwB,kBAAkB,8BAA8B,eAAe,sBAAsB,yDAAyD,UAAU,iBAAiB,sBAAsB,UAAU,IAAI,YAAY,SAAS,IAAI,wCAAwC,WAAW,UAAU,eAAe,sBAAsB,IAAI,YAAY,SAAS,WAAW,eAAe,sBAAsB,wDAAwD,iBAAiB,oCAAoC,sBAAsB,MAAM,qDAAqD,eAAe,wBAAwB,OAAO,gEAAgE,iBAAiB,6EAA6E,+BAA+B,iBAAiB,8BAA8B,4HAA4H,kCAAkC,qDAAqD,EAAE,iBAAiB,kDAAkD,EAAE,sBAAsB,qBAAqB,GAAG,iBAAiB,oBAAoB,0BAA0B,8DAA8D,qBAAqB,iBAAiB,4BAA4B,kCAAkC,MAAM,eAAe,UAAU,IAAI,EAAE,eAAe,6BAA6B,sBAAsB,mCAAmC,iBAAiB,uBAAuB,sBAAsB,uCAAuC,iBAAiB,aAAa,gDAAgD,6BAA6B,qBAAqB,iHAAiH,kDAAkD,EAAE,iBAAiB,0CAA0C,iBAAiB,qCAAqC,wEAAwE,GAAG,kOAAkO,G;;;;;;;;;;;;kBCAr2J;AACb0D,YAAU;AACRkC,iBAAa,OADL;AAERC,iBAAa,MAFL;AAGRC,qBAAiB;AAHT,GADG;AAMbnG,YAAU;AACRoG,aAAS;AADD,GANG;AASbtC,UAAQ;AACNmC,iBAAa,OADP;AAENC,iBAAa,MAFP;AAGNC,qBAAiB;AAHX,GATK;AAcbtC,WAAS;AACPwC,WAAO,GADA;AAEPC,YAAQ,GAFD;AAGPC,iBAAa,CAHN;AAIPL,iBAAa,MAJN;AAKPD,iBAAa,QALN;AAMPO,kBAAc;AANP;AAdI,C","file":"index.js","sourcesContent":["(function webpackUniversalModuleDefinition(root, factory) {\n\tif(typeof exports === 'object' && typeof module === 'object')\n\t\tmodule.exports = factory(require(\"react\"), require(\"prop-types\"));\n\telse if(typeof define === 'function' && define.amd)\n\t\tdefine([\"react\", \"prop-types\"], factory);\n\telse if(typeof exports === 'object')\n\t\texports[\"Dropzone\"] = factory(require(\"react\"), require(\"prop-types\"));\n\telse\n\t\troot[\"Dropzone\"] = factory(root[\"react\"], root[\"prop-types\"]);\n})(this, function(__WEBPACK_EXTERNAL_MODULE_2__, __WEBPACK_EXTERNAL_MODULE_3__) {\nreturn \n\n\n// WEBPACK FOOTER //\n// webpack/universalModuleDefinition"," \t// The module cache\n \tvar installedModules = {};\n\n \t// The require function\n \tfunction __webpack_require__(moduleId) {\n\n \t\t// Check if module is in cache\n \t\tif(installedModules[moduleId]) {\n \t\t\treturn installedModules[moduleId].exports;\n \t\t}\n \t\t// Create a new module (and put it into the cache)\n \t\tvar module = installedModules[moduleId] = {\n \t\t\ti: moduleId,\n \t\t\tl: false,\n \t\t\texports: {}\n \t\t};\n\n \t\t// Execute the module function\n \t\tmodules[moduleId].call(module.exports, module, module.exports, __webpack_require__);\n\n \t\t// Flag the module as loaded\n \t\tmodule.l = true;\n\n \t\t// Return the exports of the module\n \t\treturn module.exports;\n \t}\n\n\n \t// expose the modules object (__webpack_modules__)\n \t__webpack_require__.m = modules;\n\n \t// expose the module cache\n \t__webpack_require__.c = installedModules;\n\n \t// define getter function for harmony exports\n \t__webpack_require__.d = function(exports, name, getter) {\n \t\tif(!__webpack_require__.o(exports, name)) {\n \t\t\tObject.defineProperty(exports, name, {\n \t\t\t\tconfigurable: false,\n \t\t\t\tenumerable: true,\n \t\t\t\tget: getter\n \t\t\t});\n \t\t}\n \t};\n\n \t// getDefaultExport function for compatibility with non-harmony modules\n \t__webpack_require__.n = function(module) {\n \t\tvar getter = module && module.__esModule ?\n \t\t\tfunction getDefault() { return module['default']; } :\n \t\t\tfunction getModuleExports() { return module; };\n \t\t__webpack_require__.d(getter, 'a', getter);\n \t\treturn getter;\n \t};\n\n \t// Object.prototype.hasOwnProperty.call\n \t__webpack_require__.o = function(object, property) { return Object.prototype.hasOwnProperty.call(object, property); };\n\n \t// __webpack_public_path__\n \t__webpack_require__.p = \"\";\n\n \t// Load entry module and return exports\n \treturn __webpack_require__(__webpack_require__.s = 0);\n\n\n\n// WEBPACK FOOTER //\n// webpack/bootstrap 5c1f85df70828faca129","/* eslint prefer-template: 0 */\n\nimport React from 'react'\nimport PropTypes from 'prop-types'\nimport {\n supportMultiple,\n fileAccepted,\n allFilesAccepted,\n fileMatchSize,\n onDocumentDragOver,\n getDataTransferItems\n} from './utils'\nimport styles from './utils/styles'\n\nclass Dropzone extends React.Component {\n constructor(props, context) {\n super(props, context)\n this.composeHandlers = this.composeHandlers.bind(this)\n this.onClick = this.onClick.bind(this)\n this.onDocumentDrop = this.onDocumentDrop.bind(this)\n this.onDragEnter = this.onDragEnter.bind(this)\n this.onDragLeave = this.onDragLeave.bind(this)\n this.onDragOver = this.onDragOver.bind(this)\n this.onDragStart = this.onDragStart.bind(this)\n this.onDrop = this.onDrop.bind(this)\n this.onFileDialogCancel = this.onFileDialogCancel.bind(this)\n this.onInputElementClick = this.onInputElementClick.bind(this)\n\n this.setRef = this.setRef.bind(this)\n this.setRefs = this.setRefs.bind(this)\n\n this.isFileDialogActive = false\n\n this.state = {\n draggedFiles: [],\n acceptedFiles: [],\n rejectedFiles: []\n }\n }\n\n componentDidMount() {\n const { preventDropOnDocument } = this.props\n this.dragTargets = []\n\n if (preventDropOnDocument) {\n document.addEventListener('dragover', onDocumentDragOver, false)\n document.addEventListener('drop', this.onDocumentDrop, false)\n }\n this.fileInputEl.addEventListener('click', this.onInputElementClick, false)\n // Tried implementing addEventListener, but didn't work out\n document.body.onfocus = this.onFileDialogCancel\n }\n\n componentWillUnmount() {\n const { preventDropOnDocument } = this.props\n if (preventDropOnDocument) {\n document.removeEventListener('dragover', onDocumentDragOver)\n document.removeEventListener('drop', this.onDocumentDrop)\n }\n if (this.fileInputEl != null) {\n this.fileInputEl.removeEventListener('click', this.onInputElementClick, false)\n }\n // Can be replaced with removeEventListener, if addEventListener works\n if (document != null) {\n document.body.onfocus = null\n }\n }\n\n composeHandlers(handler) {\n if (this.props.disabled) {\n return null\n }\n\n return handler\n }\n\n onDocumentDrop(evt) {\n if (this.node && this.node.contains(evt.target)) {\n // if we intercepted an event for our instance, let it propagate down to the instance's onDrop handler\n return\n }\n evt.preventDefault()\n this.dragTargets = []\n }\n\n onDragStart(evt) {\n if (this.props.onDragStart) {\n this.props.onDragStart.call(this, evt)\n }\n }\n\n onDragEnter(evt) {\n evt.preventDefault()\n\n // Count the dropzone and any children that are entered.\n if (this.dragTargets.indexOf(evt.target) === -1) {\n this.dragTargets.push(evt.target)\n }\n\n this.setState({\n isDragActive: true, // Do not rely on files for the drag state. It doesn't work in Safari.\n draggedFiles: getDataTransferItems(evt)\n })\n\n if (this.props.onDragEnter) {\n this.props.onDragEnter.call(this, evt)\n }\n }\n\n onDragOver(evt) {\n // eslint-disable-line class-methods-use-this\n evt.preventDefault()\n evt.stopPropagation()\n try {\n // The file dialog on Chrome allows users to drag files from the dialog onto\n // the dropzone, causing the browser the crash when the file dialog is closed.\n // A drop effect of 'none' prevents the file from being dropped\n evt.dataTransfer.dropEffect = this.isFileDialogActive ? 'none' : 'copy' // eslint-disable-line no-param-reassign\n } catch (err) {\n // continue regardless of error\n }\n\n if (this.props.onDragOver) {\n this.props.onDragOver.call(this, evt)\n }\n return false\n }\n\n onDragLeave(evt) {\n evt.preventDefault()\n\n // Only deactivate once the dropzone and all children have been left.\n this.dragTargets = this.dragTargets.filter(el => el !== evt.target && this.node.contains(el))\n if (this.dragTargets.length > 0) {\n return\n }\n\n // Clear dragging files state\n this.setState({\n isDragActive: false,\n draggedFiles: []\n })\n\n if (this.props.onDragLeave) {\n this.props.onDragLeave.call(this, evt)\n }\n }\n\n onDrop(evt) {\n const { onDrop, onDropAccepted, onDropRejected, multiple, disablePreview, accept } = this.props\n const fileList = getDataTransferItems(evt)\n const acceptedFiles = []\n const rejectedFiles = []\n\n // Stop default browser behavior\n evt.preventDefault()\n\n // Reset the counter along with the drag on a drop.\n this.dragTargets = []\n this.isFileDialogActive = false\n\n fileList.forEach(file => {\n if (!disablePreview) {\n try {\n file.preview = window.URL.createObjectURL(file) // eslint-disable-line no-param-reassign\n } catch (err) {\n if (process.env.NODE_ENV !== 'production') {\n console.error('Failed to generate preview for file', file, err) // eslint-disable-line no-console\n }\n }\n }\n\n if (\n fileAccepted(file, accept) &&\n fileMatchSize(file, this.props.maxSize, this.props.minSize)\n ) {\n acceptedFiles.push(file)\n } else {\n rejectedFiles.push(file)\n }\n })\n\n if (!multiple) {\n // if not in multi mode add any extra accepted files to rejected.\n // This will allow end users to easily ignore a multi file drop in \"single\" mode.\n rejectedFiles.push(...acceptedFiles.splice(1))\n }\n\n if (onDrop) {\n onDrop.call(this, acceptedFiles, rejectedFiles, evt)\n }\n\n if (rejectedFiles.length > 0 && onDropRejected) {\n onDropRejected.call(this, rejectedFiles, evt)\n }\n\n if (acceptedFiles.length > 0 && onDropAccepted) {\n onDropAccepted.call(this, acceptedFiles, evt)\n }\n\n // Clear files value\n this.draggedFiles = null\n\n // Reset drag state\n this.setState({\n isDragActive: false,\n draggedFiles: [],\n acceptedFiles,\n rejectedFiles\n })\n }\n\n onClick(evt) {\n const { onClick, disableClick } = this.props\n if (!disableClick) {\n evt.stopPropagation()\n\n if (onClick) {\n onClick.call(this, evt)\n }\n\n // in IE11/Edge the file-browser dialog is blocking, ensure this is behind setTimeout\n // this is so react can handle state changes in the onClick prop above above\n // see: https://github.com/react-dropzone/react-dropzone/issues/450\n setTimeout(this.open.bind(this), 0)\n }\n }\n\n onInputElementClick(evt) {\n evt.stopPropagation()\n if (this.props.inputProps && this.props.inputProps.onClick) {\n this.props.inputProps.onClick()\n }\n }\n\n onFileDialogCancel() {\n // timeout will not recognize context of this method\n const { onFileDialogCancel } = this.props\n const { fileInputEl } = this\n let { isFileDialogActive } = this\n // execute the timeout only if the onFileDialogCancel is defined and FileDialog\n // is opened in the browser\n if (onFileDialogCancel && isFileDialogActive) {\n setTimeout(() => {\n // Returns an object as FileList\n const FileList = fileInputEl.files\n if (!FileList.length) {\n isFileDialogActive = false\n onFileDialogCancel()\n }\n }, 300)\n }\n }\n\n setRef(ref) {\n this.node = ref\n }\n\n setRefs(ref) {\n this.fileInputEl = ref\n }\n /**\n * Open system file upload dialog.\n *\n * @public\n */\n open() {\n this.isFileDialogActive = true\n this.fileInputEl.value = null\n this.fileInputEl.click()\n }\n\n renderChildren = (children, isDragActive, isDragAccept, isDragReject) => {\n if (typeof children === 'function') {\n return children({\n ...this.state,\n isDragActive,\n isDragAccept,\n isDragReject\n })\n }\n return children\n }\n\n render() {\n const {\n accept,\n acceptClassName,\n activeClassName,\n children,\n disabled,\n disabledClassName,\n inputProps,\n multiple,\n name,\n rejectClassName,\n ...rest\n } = this.props\n\n let {\n acceptStyle,\n activeStyle,\n className = '',\n disabledStyle,\n rejectStyle,\n style,\n ...props // eslint-disable-line prefer-const\n } = rest\n\n const { isDragActive, draggedFiles } = this.state\n const filesCount = draggedFiles.length\n const isMultipleAllowed = multiple || filesCount <= 1\n const isDragAccept = filesCount > 0 && allFilesAccepted(draggedFiles, this.props.accept)\n const isDragReject = filesCount > 0 && (!isDragAccept || !isMultipleAllowed)\n const noStyles =\n !className && !style && !activeStyle && !acceptStyle && !rejectStyle && !disabledStyle\n\n if (isDragActive && activeClassName) {\n className += ' ' + activeClassName\n }\n if (isDragAccept && acceptClassName) {\n className += ' ' + acceptClassName\n }\n if (isDragReject && rejectClassName) {\n className += ' ' + rejectClassName\n }\n if (disabled && disabledClassName) {\n className += ' ' + disabledClassName\n }\n\n if (noStyles) {\n style = styles.default\n activeStyle = styles.active\n acceptStyle = style.active\n rejectStyle = styles.rejected\n disabledStyle = styles.disabled\n }\n\n let appliedStyle = { ...style }\n if (activeStyle && isDragActive) {\n appliedStyle = {\n ...style,\n ...activeStyle\n }\n }\n if (acceptStyle && isDragAccept) {\n appliedStyle = {\n ...appliedStyle,\n ...acceptStyle\n }\n }\n if (rejectStyle && isDragReject) {\n appliedStyle = {\n ...appliedStyle,\n ...rejectStyle\n }\n }\n if (disabledStyle && disabled) {\n appliedStyle = {\n ...style,\n ...disabledStyle\n }\n }\n\n const inputAttributes = {\n accept,\n disabled,\n type: 'file',\n style: { display: 'none' },\n multiple: supportMultiple && multiple,\n ref: this.setRefs,\n onChange: this.onDrop,\n autoComplete: 'off'\n }\n\n if (name && name.length) {\n inputAttributes.name = name\n }\n\n // Destructure custom props away from props used for the div element\n const {\n acceptedFiles,\n preventDropOnDocument,\n disablePreview,\n disableClick,\n onDropAccepted,\n onDropRejected,\n onFileDialogCancel,\n maxSize,\n minSize,\n ...divProps\n } = props\n\n return (\n
\n {this.renderChildren(children, isDragActive, isDragAccept, isDragReject)}\n \n
\n )\n }\n}\n\nexport default Dropzone\n\nDropzone.propTypes = {\n /**\n * Allow specific types of files. See https://github.com/okonet/attr-accept for more information.\n * Keep in mind that mime type determination is not reliable across platforms. CSV files,\n * for example, are reported as text/plain under macOS but as application/vnd.ms-excel under\n * Windows. In some cases there might not be a mime type set at all.\n * See: https://github.com/react-dropzone/react-dropzone/issues/276\n */\n accept: PropTypes.string,\n\n /**\n * Contents of the dropzone\n */\n children: PropTypes.oneOfType([PropTypes.node, PropTypes.func]),\n\n /**\n * Disallow clicking on the dropzone container to open file dialog\n */\n disableClick: PropTypes.bool,\n\n /**\n * Enable/disable the dropzone entirely\n */\n disabled: PropTypes.bool,\n\n /**\n * Enable/disable preview generation\n */\n disablePreview: PropTypes.bool,\n\n /**\n * If false, allow dropped items to take over the current browser window\n */\n preventDropOnDocument: PropTypes.bool,\n\n /**\n * Pass additional attributes to the ` ` tag\n */\n inputProps: PropTypes.object,\n\n /**\n * Allow dropping multiple files\n */\n multiple: PropTypes.bool,\n\n /**\n * `name` attribute for the input tag\n */\n name: PropTypes.string,\n\n /**\n * Maximum file size\n */\n maxSize: PropTypes.number,\n\n /**\n * Minimum file size\n */\n minSize: PropTypes.number,\n\n /**\n * className\n */\n className: PropTypes.string,\n\n /**\n * className for active state\n */\n activeClassName: PropTypes.string,\n\n /**\n * className for accepted state\n */\n acceptClassName: PropTypes.string,\n\n /**\n * className for rejected state\n */\n rejectClassName: PropTypes.string,\n\n /**\n * className for disabled state\n */\n disabledClassName: PropTypes.string,\n\n /**\n * CSS styles to apply\n */\n style: PropTypes.object,\n\n /**\n * CSS styles to apply when drag is active\n */\n activeStyle: PropTypes.object,\n\n /**\n * CSS styles to apply when drop will be accepted\n */\n acceptStyle: PropTypes.object,\n\n /**\n * CSS styles to apply when drop will be rejected\n */\n rejectStyle: PropTypes.object,\n\n /**\n * CSS styles to apply when dropzone is disabled\n */\n disabledStyle: PropTypes.object,\n\n /**\n * onClick callback\n * @param {Event} event\n */\n onClick: PropTypes.func,\n\n /**\n * onDrop callback\n */\n onDrop: PropTypes.func,\n\n /**\n * onDropAccepted callback\n */\n onDropAccepted: PropTypes.func,\n\n /**\n * onDropRejected callback\n */\n onDropRejected: PropTypes.func,\n\n /**\n * onDragStart callback\n */\n onDragStart: PropTypes.func,\n\n /**\n * onDragEnter callback\n */\n onDragEnter: PropTypes.func,\n\n /**\n * onDragOver callback\n */\n onDragOver: PropTypes.func,\n\n /**\n * onDragLeave callback\n */\n onDragLeave: PropTypes.func,\n\n /**\n * Provide a callback on clicking the cancel button of the file dialog\n */\n onFileDialogCancel: PropTypes.func\n}\n\nDropzone.defaultProps = {\n preventDropOnDocument: true,\n disabled: false,\n disablePreview: false,\n disableClick: false,\n multiple: true,\n maxSize: Infinity,\n minSize: 0\n}\n\n\n\n// WEBPACK FOOTER //\n// ./src/index.js","// shim for using process in browser\nvar process = module.exports = {};\n\n// cached from whatever global is present so that test runners that stub it\n// don't break things. But we need to wrap it in a try catch in case it is\n// wrapped in strict mode code which doesn't define any globals. It's inside a\n// function because try/catches deoptimize in certain engines.\n\nvar cachedSetTimeout;\nvar cachedClearTimeout;\n\nfunction defaultSetTimout() {\n throw new Error('setTimeout has not been defined');\n}\nfunction defaultClearTimeout () {\n throw new Error('clearTimeout has not been defined');\n}\n(function () {\n try {\n if (typeof setTimeout === 'function') {\n cachedSetTimeout = setTimeout;\n } else {\n cachedSetTimeout = defaultSetTimout;\n }\n } catch (e) {\n cachedSetTimeout = defaultSetTimout;\n }\n try {\n if (typeof clearTimeout === 'function') {\n cachedClearTimeout = clearTimeout;\n } else {\n cachedClearTimeout = defaultClearTimeout;\n }\n } catch (e) {\n cachedClearTimeout = defaultClearTimeout;\n }\n} ())\nfunction runTimeout(fun) {\n if (cachedSetTimeout === setTimeout) {\n //normal enviroments in sane situations\n return setTimeout(fun, 0);\n }\n // if setTimeout wasn't available but was latter defined\n if ((cachedSetTimeout === defaultSetTimout || !cachedSetTimeout) && setTimeout) {\n cachedSetTimeout = setTimeout;\n return setTimeout(fun, 0);\n }\n try {\n // when when somebody has screwed with setTimeout but no I.E. maddness\n return cachedSetTimeout(fun, 0);\n } catch(e){\n try {\n // When we are in I.E. but the script has been evaled so I.E. doesn't trust the global object when called normally\n return cachedSetTimeout.call(null, fun, 0);\n } catch(e){\n // same as above but when it's a version of I.E. that must have the global object for 'this', hopfully our context correct otherwise it will throw a global error\n return cachedSetTimeout.call(this, fun, 0);\n }\n }\n\n\n}\nfunction runClearTimeout(marker) {\n if (cachedClearTimeout === clearTimeout) {\n //normal enviroments in sane situations\n return clearTimeout(marker);\n }\n // if clearTimeout wasn't available but was latter defined\n if ((cachedClearTimeout === defaultClearTimeout || !cachedClearTimeout) && clearTimeout) {\n cachedClearTimeout = clearTimeout;\n return clearTimeout(marker);\n }\n try {\n // when when somebody has screwed with setTimeout but no I.E. maddness\n return cachedClearTimeout(marker);\n } catch (e){\n try {\n // When we are in I.E. but the script has been evaled so I.E. doesn't trust the global object when called normally\n return cachedClearTimeout.call(null, marker);\n } catch (e){\n // same as above but when it's a version of I.E. that must have the global object for 'this', hopfully our context correct otherwise it will throw a global error.\n // Some versions of I.E. have different rules for clearTimeout vs setTimeout\n return cachedClearTimeout.call(this, marker);\n }\n }\n\n\n\n}\nvar queue = [];\nvar draining = false;\nvar currentQueue;\nvar queueIndex = -1;\n\nfunction cleanUpNextTick() {\n if (!draining || !currentQueue) {\n return;\n }\n draining = false;\n if (currentQueue.length) {\n queue = currentQueue.concat(queue);\n } else {\n queueIndex = -1;\n }\n if (queue.length) {\n drainQueue();\n }\n}\n\nfunction drainQueue() {\n if (draining) {\n return;\n }\n var timeout = runTimeout(cleanUpNextTick);\n draining = true;\n\n var len = queue.length;\n while(len) {\n currentQueue = queue;\n queue = [];\n while (++queueIndex < len) {\n if (currentQueue) {\n currentQueue[queueIndex].run();\n }\n }\n queueIndex = -1;\n len = queue.length;\n }\n currentQueue = null;\n draining = false;\n runClearTimeout(timeout);\n}\n\nprocess.nextTick = function (fun) {\n var args = new Array(arguments.length - 1);\n if (arguments.length > 1) {\n for (var i = 1; i < arguments.length; i++) {\n args[i - 1] = arguments[i];\n }\n }\n queue.push(new Item(fun, args));\n if (queue.length === 1 && !draining) {\n runTimeout(drainQueue);\n }\n};\n\n// v8 likes predictible objects\nfunction Item(fun, array) {\n this.fun = fun;\n this.array = array;\n}\nItem.prototype.run = function () {\n this.fun.apply(null, this.array);\n};\nprocess.title = 'browser';\nprocess.browser = true;\nprocess.env = {};\nprocess.argv = [];\nprocess.version = ''; // empty string to avoid regexp issues\nprocess.versions = {};\n\nfunction noop() {}\n\nprocess.on = noop;\nprocess.addListener = noop;\nprocess.once = noop;\nprocess.off = noop;\nprocess.removeListener = noop;\nprocess.removeAllListeners = noop;\nprocess.emit = noop;\nprocess.prependListener = noop;\nprocess.prependOnceListener = noop;\n\nprocess.listeners = function (name) { return [] }\n\nprocess.binding = function (name) {\n throw new Error('process.binding is not supported');\n};\n\nprocess.cwd = function () { return '/' };\nprocess.chdir = function (dir) {\n throw new Error('process.chdir is not supported');\n};\nprocess.umask = function() { return 0; };\n\n\n\n//////////////////\n// WEBPACK FOOTER\n// ./node_modules/process/browser.js\n// module id = 1\n// module chunks = 0","module.exports = __WEBPACK_EXTERNAL_MODULE_2__;\n\n\n//////////////////\n// WEBPACK FOOTER\n// external \"react\"\n// module id = 2\n// module chunks = 0","module.exports = __WEBPACK_EXTERNAL_MODULE_3__;\n\n\n//////////////////\n// WEBPACK FOOTER\n// external \"prop-types\"\n// module id = 3\n// module chunks = 0","import accepts from 'attr-accept'\n\nexport const supportMultiple =\n typeof document !== 'undefined' && document && document.createElement\n ? 'multiple' in document.createElement('input')\n : true\n\nexport function getDataTransferItems(event) {\n let dataTransferItemsList = []\n if (event.dataTransfer) {\n const dt = event.dataTransfer\n if (dt.files && dt.files.length) {\n dataTransferItemsList = dt.files\n } else if (dt.items && dt.items.length) {\n // During the drag even the dataTransfer.files is null\n // but Chrome implements some drag store, which is accesible via dataTransfer.items\n dataTransferItemsList = dt.items\n }\n } else if (event.target && event.target.files) {\n dataTransferItemsList = event.target.files\n }\n // Convert from DataTransferItemsList to the native Array\n return Array.prototype.slice.call(dataTransferItemsList)\n}\n\n// Firefox versions prior to 53 return a bogus MIME type for every file drag, so dragovers with\n// that MIME type will always be accepted\nexport function fileAccepted(file, accept) {\n return file.type === 'application/x-moz-file' || accepts(file, accept)\n}\n\nexport function fileMatchSize(file, maxSize, minSize) {\n return file.size <= maxSize && file.size >= minSize\n}\n\nexport function allFilesAccepted(files, accept) {\n return files.every(file => fileAccepted(file, accept))\n}\n\n// allow the entire document to be a drag target\nexport function onDocumentDragOver(evt) {\n evt.preventDefault()\n}\n\n\n\n// WEBPACK FOOTER //\n// ./src/utils/index.js","module.exports=function(t){function n(e){if(r[e])return r[e].exports;var o=r[e]={exports:{},id:e,loaded:!1};return t[e].call(o.exports,o,o.exports,n),o.loaded=!0,o.exports}var r={};return n.m=t,n.c=r,n.p=\"\",n(0)}([function(t,n,r){\"use strict\";n.__esModule=!0,r(8),r(9),n[\"default\"]=function(t,n){if(t&&n){var r=function(){var r=Array.isArray(n)?n:n.split(\",\"),e=t.name||\"\",o=t.type||\"\",i=o.replace(/\\/.*$/,\"\");return{v:r.some(function(t){var n=t.trim();return\".\"===n.charAt(0)?e.toLowerCase().endsWith(n.toLowerCase()):/\\/\\*$/.test(n)?i===n.replace(/\\/.*$/,\"\"):o===n})}}();if(\"object\"==typeof r)return r.v}return!0},t.exports=n[\"default\"]},function(t,n){var r=t.exports={version:\"1.2.2\"};\"number\"==typeof __e&&(__e=r)},function(t,n){var r=t.exports=\"undefined\"!=typeof window&&window.Math==Math?window:\"undefined\"!=typeof self&&self.Math==Math?self:Function(\"return this\")();\"number\"==typeof __g&&(__g=r)},function(t,n,r){var e=r(2),o=r(1),i=r(4),u=r(19),c=\"prototype\",f=function(t,n){return function(){return t.apply(n,arguments)}},s=function(t,n,r){var a,p,l,y,d=t&s.G,h=t&s.P,v=d?e:t&s.S?e[n]||(e[n]={}):(e[n]||{})[c],x=d?o:o[n]||(o[n]={});d&&(r=n);for(a in r)p=!(t&s.F)&&v&&a in v,l=(p?v:r)[a],y=t&s.B&&p?f(l,e):h&&\"function\"==typeof l?f(Function.call,l):l,v&&!p&&u(v,a,l),x[a]!=l&&i(x,a,y),h&&((x[c]||(x[c]={}))[a]=l)};e.core=o,s.F=1,s.G=2,s.S=4,s.P=8,s.B=16,s.W=32,t.exports=s},function(t,n,r){var e=r(5),o=r(18);t.exports=r(22)?function(t,n,r){return e.setDesc(t,n,o(1,r))}:function(t,n,r){return t[n]=r,t}},function(t,n){var r=Object;t.exports={create:r.create,getProto:r.getPrototypeOf,isEnum:{}.propertyIsEnumerable,getDesc:r.getOwnPropertyDescriptor,setDesc:r.defineProperty,setDescs:r.defineProperties,getKeys:r.keys,getNames:r.getOwnPropertyNames,getSymbols:r.getOwnPropertySymbols,each:[].forEach}},function(t,n){var r=0,e=Math.random();t.exports=function(t){return\"Symbol(\".concat(void 0===t?\"\":t,\")_\",(++r+e).toString(36))}},function(t,n,r){var e=r(20)(\"wks\"),o=r(2).Symbol;t.exports=function(t){return e[t]||(e[t]=o&&o[t]||(o||r(6))(\"Symbol.\"+t))}},function(t,n,r){r(26),t.exports=r(1).Array.some},function(t,n,r){r(25),t.exports=r(1).String.endsWith},function(t,n){t.exports=function(t){if(\"function\"!=typeof t)throw TypeError(t+\" is not a function!\");return t}},function(t,n){var r={}.toString;t.exports=function(t){return r.call(t).slice(8,-1)}},function(t,n,r){var e=r(10);t.exports=function(t,n,r){if(e(t),void 0===n)return t;switch(r){case 1:return function(r){return t.call(n,r)};case 2:return function(r,e){return t.call(n,r,e)};case 3:return function(r,e,o){return t.call(n,r,e,o)}}return function(){return t.apply(n,arguments)}}},function(t,n){t.exports=function(t){if(void 0==t)throw TypeError(\"Can't call method on \"+t);return t}},function(t,n,r){t.exports=function(t){var n=/./;try{\"/./\"[t](n)}catch(e){try{return n[r(7)(\"match\")]=!1,!\"/./\"[t](n)}catch(o){}}return!0}},function(t,n){t.exports=function(t){try{return!!t()}catch(n){return!0}}},function(t,n){t.exports=function(t){return\"object\"==typeof t?null!==t:\"function\"==typeof t}},function(t,n,r){var e=r(16),o=r(11),i=r(7)(\"match\");t.exports=function(t){var n;return e(t)&&(void 0!==(n=t[i])?!!n:\"RegExp\"==o(t))}},function(t,n){t.exports=function(t,n){return{enumerable:!(1&t),configurable:!(2&t),writable:!(4&t),value:n}}},function(t,n,r){var e=r(2),o=r(4),i=r(6)(\"src\"),u=\"toString\",c=Function[u],f=(\"\"+c).split(u);r(1).inspectSource=function(t){return c.call(t)},(t.exports=function(t,n,r,u){\"function\"==typeof r&&(o(r,i,t[n]?\"\"+t[n]:f.join(String(n))),\"name\"in r||(r.name=n)),t===e?t[n]=r:(u||delete t[n],o(t,n,r))})(Function.prototype,u,function(){return\"function\"==typeof this&&this[i]||c.call(this)})},function(t,n,r){var e=r(2),o=\"__core-js_shared__\",i=e[o]||(e[o]={});t.exports=function(t){return i[t]||(i[t]={})}},function(t,n,r){var e=r(17),o=r(13);t.exports=function(t,n,r){if(e(n))throw TypeError(\"String#\"+r+\" doesn't accept regex!\");return String(o(t))}},function(t,n,r){t.exports=!r(15)(function(){return 7!=Object.defineProperty({},\"a\",{get:function(){return 7}}).a})},function(t,n){var r=Math.ceil,e=Math.floor;t.exports=function(t){return isNaN(t=+t)?0:(t>0?e:r)(t)}},function(t,n,r){var e=r(23),o=Math.min;t.exports=function(t){return t>0?o(e(t),9007199254740991):0}},function(t,n,r){\"use strict\";var e=r(3),o=r(24),i=r(21),u=\"endsWith\",c=\"\"[u];e(e.P+e.F*r(14)(u),\"String\",{endsWith:function(t){var n=i(this,t,u),r=arguments,e=r.length>1?r[1]:void 0,f=o(n.length),s=void 0===e?f:Math.min(o(e),f),a=String(t);return c?c.call(n,a,s):n.slice(s-a.length,s)===a}})},function(t,n,r){var e=r(5),o=r(3),i=r(1).Array||Array,u={},c=function(t,n){e.each.call(t.split(\",\"),function(t){void 0==n&&t in i?u[t]=i[t]:t in[]&&(u[t]=r(12)(Function.call,[][t],n))})};c(\"pop,reverse,shift,keys,values,entries\",1),c(\"indexOf,every,some,forEach,map,filter,find,findIndex,includes\",3),c(\"join,slice,concat,push,splice,unshift,sort,lastIndexOf,reduce,reduceRight,copyWithin,fill\"),o(o.S,\"Array\",u)}]);\n\n\n//////////////////\n// WEBPACK FOOTER\n// ./node_modules/attr-accept/dist/index.js\n// module id = 5\n// module chunks = 0","export default {\n rejected: {\n borderStyle: 'solid',\n borderColor: '#c66',\n backgroundColor: '#eee'\n },\n disabled: {\n opacity: 0.5\n },\n active: {\n borderStyle: 'solid',\n borderColor: '#6c6',\n backgroundColor: '#eee'\n },\n default: {\n width: 200,\n height: 200,\n borderWidth: 2,\n borderColor: '#666',\n borderStyle: 'dashed',\n borderRadius: 5\n }\n}\n\n\n\n// WEBPACK FOOTER //\n// ./src/utils/styles.js"],"sourceRoot":""}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/examples/.eslintrc b/goTorrentWebUI/node_modules/react-dropzone/examples/.eslintrc
new file mode 100644
index 00000000..99cb1fb7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/examples/.eslintrc
@@ -0,0 +1,8 @@
+{
+ "globals": {
+ "Dropzone": true
+ },
+ "rules": {
+ "react/jsx-no-bind": "off"
+ }
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/examples/Accept/Readme.md b/goTorrentWebUI/node_modules/react-dropzone/examples/Accept/Readme.md
new file mode 100644
index 00000000..d8c22297
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/examples/Accept/Readme.md
@@ -0,0 +1,99 @@
+By providing `accept` prop you can make Dropzone to accept specific file types and reject the others.
+
+The value must be a comma-separated list of unique content type specifiers:
+* A file extension starting with the STOP character (U+002E). (e.g. .jpg, .png, .doc).
+* A valid MIME type with no extensions.
+* audio/* representing sound files.
+* video/* representing video files.
+* image/* representing image files.
+
+For more information see https://developer.mozilla.org/en-US/docs/Web/HTML/Element/Input
+
+```
+class Accept extends React.Component {
+ constructor() {
+ super()
+ this.state = {
+ accepted: [],
+ rejected: []
+ }
+ }
+
+ render() {
+ return (
+
+
+
{ this.setState({ accepted, rejected }); }}
+ >
+ Try dropping some files here, or click to select files to upload.
+ Only *.jpeg and *.png images will be accepted
+
+
+
+ Accepted files
+
+ {
+ this.state.accepted.map(f => {f.name} - {f.size} bytes )
+ }
+
+ Rejected files
+
+ {
+ this.state.rejected.map(f => {f.name} - {f.size} bytes )
+ }
+
+
+
+ );
+ }
+}
+
+
+```
+
+### Browser limitations
+
+Because of HTML5 File API differences across different browsers during the drag, Dropzone will only display `rejected` styles in Chrome (and Chromium based browser). It isn't working in Safari nor IE.
+
+Also, at this moment it's not possible to read file names (and thus, file extensions) during the drag operation. For that reason, if you want to react on different file types _during_ the drag operation, _you have to use_ mime types and not extensions! For example, the following example won't work even in Chrome:
+
+```
+
+ {({ isDragActive, isDragReject }) => {
+ if (isDragActive) {
+ return "All files will be accepted";
+ }
+ if (isDragReject) {
+ return "Some files will be rejected";
+ }
+ return "Dropping some files here...";
+ }}
+
+```
+
+but this one will:
+
+```
+
+ {({ isDragActive, isDragReject }) => {
+ if (isDragActive) {
+ return "All files will be accepted";
+ }
+ if (isDragReject) {
+ return "Some files will be rejected";
+ }
+ return "Dropping some files here...";
+ }}
+
+```
+
+### Notes
+
+Mime type determination is not reliable accross platforms. CSV files, for example, are reported as text/plain under macOS but as application/vnd.ms-excel under Windows. In some cases there might not be a mime type set at all.
+
diff --git a/goTorrentWebUI/node_modules/react-dropzone/examples/Basic/Readme.md b/goTorrentWebUI/node_modules/react-dropzone/examples/Basic/Readme.md
new file mode 100644
index 00000000..b6eff57c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/examples/Basic/Readme.md
@@ -0,0 +1,80 @@
+Dropzone with default properties and displays list of the dropped files.
+
+```
+class Basic extends React.Component {
+ constructor() {
+ super()
+ this.state = { files: [] }
+ }
+
+ onDrop(files) {
+ this.setState({
+ files
+ });
+ }
+
+ render() {
+ return (
+
+
+
+ Try dropping some files here, or click to select files to upload.
+
+
+
+ Dropped files
+
+ {
+ this.state.files.map(f => {f.name} - {f.size} bytes )
+ }
+
+
+
+ );
+ }
+}
+
+
+```
+
+Dropzone with `disabled` property:
+
+```
+class Basic extends React.Component {
+ constructor() {
+ super()
+ this.state = { disabled: true, files: [] }
+ }
+
+ onDrop(files) {
+ this.setState({
+ files
+ });
+ }
+
+ render() {
+ return (
+
+
+ this.setState({ disabled: !this.state.disabled })}>Toggle disabled
+
+
+
+ Try dropping some files here, or click to select files to upload.
+
+
+
+ Dropped files
+
+ {
+ this.state.files.map(f => {f.name} - {f.size} bytes )
+ }
+
+
+
+ );
+ }
+}
+
+
+```
diff --git a/goTorrentWebUI/node_modules/react-dropzone/examples/File Dialog/Readme.md b/goTorrentWebUI/node_modules/react-dropzone/examples/File Dialog/Readme.md
new file mode 100644
index 00000000..48cd2fab
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/examples/File Dialog/Readme.md
@@ -0,0 +1,16 @@
+You can programmatically invoke default OS file prompt. In order to do that you'll have to set the ref on your `Dropzone` instance and call the instance `open` method.
+
+```
+let dropzoneRef;
+
+
+
{ dropzoneRef = node; }} onDrop={(accepted, rejected) => { alert(accepted) }}>
+ Drop files here.
+
+
{ dropzoneRef.open() }}>
+ Open File Dialog
+
+
+```
+
+The completion handler for the `open` function is also the `onDrop` function.
diff --git a/goTorrentWebUI/node_modules/react-dropzone/examples/Fullscreen/Readme.md b/goTorrentWebUI/node_modules/react-dropzone/examples/Fullscreen/Readme.md
new file mode 100644
index 00000000..52cc657f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/examples/Fullscreen/Readme.md
@@ -0,0 +1,85 @@
+You can wrap the whole app into the dropzone. This will make the whole app a Dropzone target.
+
+```
+class FullScreen extends React.Component {
+ constructor() {
+ super()
+ this.state = {
+ accept: '',
+ files: [],
+ dropzoneActive: false
+ }
+ }
+
+ onDragEnter() {
+ this.setState({
+ dropzoneActive: true
+ });
+ }
+
+ onDragLeave() {
+ this.setState({
+ dropzoneActive: false
+ });
+ }
+
+ onDrop(files) {
+ this.setState({
+ files,
+ dropzoneActive: false
+ });
+ }
+
+ applyMimeTypes(event) {
+ this.setState({
+ accept: event.target.value
+ });
+ }
+
+ render() {
+ const { accept, files, dropzoneActive } = this.state;
+ const overlayStyle = {
+ position: 'absolute',
+ top: 0,
+ right: 0,
+ bottom: 0,
+ left: 0,
+ padding: '2.5em 0',
+ background: 'rgba(0,0,0,0.5)',
+ textAlign: 'center',
+ color: '#fff'
+ };
+ return (
+
+ { dropzoneActive && Drop files...
}
+
+
My awesome app
+
Enter mime types you want to accept:
+
+
+
Dropped files
+
+ {
+ files.map(f => {f.name} - {f.size} bytes )
+ }
+
+
+
+
+ );
+ }
+}
+
+
+```
diff --git a/goTorrentWebUI/node_modules/react-dropzone/examples/Styling/Readme.md b/goTorrentWebUI/node_modules/react-dropzone/examples/Styling/Readme.md
new file mode 100644
index 00000000..8bca8c09
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/examples/Styling/Readme.md
@@ -0,0 +1,23 @@
+By default, the Dropzone component picks up some default styling to get you started. You can customize `` by specifying a `style`, `activeStyle` or `rejectStyle` which is applied when a file is dragged over the zone. You can also specify `className`, `activeClassName` or `rejectClassName` if you would rather style using CSS.
+
+## Updating styles and contents based on user input
+
+By providing a function that returns the component's children you can not only style Dropzone appropriately but also render appropriate content.
+
+```
+
+ {({ isDragActive, isDragReject, acceptedFiles, rejectedFiles }) => {
+ if (isDragActive) {
+ return "This file is authorized";
+ }
+ if (isDragReject) {
+ return "This file is not authorized";
+ }
+ return acceptedFiles.length || rejectedFiles.length
+ ? `Accepted ${acceptedFiles.length}, rejected ${rejectedFiles.length} files`
+ : "Try dropping some files.";
+ }}
+
+```
diff --git a/goTorrentWebUI/node_modules/react-dropzone/examples/theme.css b/goTorrentWebUI/node_modules/react-dropzone/examples/theme.css
new file mode 100644
index 00000000..9b960d4e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/examples/theme.css
@@ -0,0 +1,12 @@
+section {
+ display: flex;
+}
+
+.dropzone {
+ flex: 1 auto;
+ width: 50%;
+}
+
+aside {
+ width: 50%;
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/.bin/loose-envify b/goTorrentWebUI/node_modules/react-dropzone/node_modules/.bin/loose-envify
new file mode 100644
index 00000000..0939216f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/.bin/loose-envify
@@ -0,0 +1,15 @@
+#!/bin/sh
+basedir=$(dirname "$(echo "$0" | sed -e 's,\\,/,g')")
+
+case `uname` in
+ *CYGWIN*) basedir=`cygpath -w "$basedir"`;;
+esac
+
+if [ -x "$basedir/node" ]; then
+ "$basedir/node" "$basedir/../loose-envify/cli.js" "$@"
+ ret=$?
+else
+ node "$basedir/../loose-envify/cli.js" "$@"
+ ret=$?
+fi
+exit $ret
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/.bin/loose-envify.cmd b/goTorrentWebUI/node_modules/react-dropzone/node_modules/.bin/loose-envify.cmd
new file mode 100644
index 00000000..6238bbb2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/.bin/loose-envify.cmd
@@ -0,0 +1,7 @@
+@IF EXIST "%~dp0\node.exe" (
+ "%~dp0\node.exe" "%~dp0\..\loose-envify\cli.js" %*
+) ELSE (
+ @SETLOCAL
+ @SET PATHEXT=%PATHEXT:;.JS;=;%
+ node "%~dp0\..\loose-envify\cli.js" %*
+)
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/CHANGES.md b/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/CHANGES.md
new file mode 100644
index 00000000..f105b919
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/CHANGES.md
@@ -0,0 +1,70 @@
+
+## 2.0.6
+
+Version 2.0.4 adds support for React Native by clarifying in package.json that
+the browser environment does not support Node.js domains.
+Why this is necessary, we leave as an exercise for the user.
+
+## 2.0.3
+
+Version 2.0.3 fixes a bug when adjusting the capacity of the task queue.
+
+## 2.0.1-2.02
+
+Version 2.0.1 fixes a bug in the way redirects were expressed that affected the
+function of Browserify, but which Mr would tolerate.
+
+## 2.0.0
+
+Version 2 of ASAP is a full rewrite with a few salient changes.
+First, the ASAP source is CommonJS only and designed with [Browserify][] and
+[Browserify-compatible][Mr] module loaders in mind.
+
+[Browserify]: https://github.com/substack/node-browserify
+[Mr]: https://github.com/montagejs/mr
+
+The new version has been refactored in two dimensions.
+Support for Node.js and browsers have been separated, using Browserify
+redirects and ASAP has been divided into two modules.
+The "raw" layer depends on the tasks to catch thrown exceptions and unravel
+Node.js domains.
+
+The full implementation of ASAP is loadable as `require("asap")` in both Node.js
+and browsers.
+
+The raw layer that lacks exception handling overhead is loadable as
+`require("asap/raw")`.
+The interface is the same for both layers.
+
+Tasks are no longer required to be functions, but can rather be any object that
+implements `task.call()`.
+With this feature you can recycle task objects to avoid garbage collector churn
+and avoid closures in general.
+
+The implementation has been rigorously documented so that our successors can
+understand the scope of the problem that this module solves and all of its
+nuances, ensuring that the next generation of implementations know what details
+are essential.
+
+- [asap.js](https://github.com/kriskowal/asap/blob/master/asap.js)
+- [raw.js](https://github.com/kriskowal/asap/blob/master/raw.js)
+- [browser-asap.js](https://github.com/kriskowal/asap/blob/master/browser-asap.js)
+- [browser-raw.js](https://github.com/kriskowal/asap/blob/master/browser-raw.js)
+
+The new version has also been rigorously tested across a broad spectrum of
+browsers, in both the window and worker context.
+The following charts capture the browser test results for the most recent
+release.
+The first chart shows test results for ASAP running in the main window context.
+The second chart shows test results for ASAP running in a web worker context.
+Test results are inconclusive (grey) on browsers that do not support web
+workers.
+These data are captured automatically by [Continuous
+Integration][].
+
+
+
+
+
+[Continuous Integration]: https://github.com/kriskowal/asap/blob/master/CONTRIBUTING.md
+
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/LICENSE.md b/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/LICENSE.md
new file mode 100644
index 00000000..ba18c613
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/LICENSE.md
@@ -0,0 +1,21 @@
+
+Copyright 2009–2014 Contributors. All rights reserved.
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to
+deal in the Software without restriction, including without limitation the
+rights to use, copy, modify, merge, publish, distribute, sublicense, and/or
+sell copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
+FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS
+IN THE SOFTWARE.
+
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/README.md b/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/README.md
new file mode 100644
index 00000000..452fd8c2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/README.md
@@ -0,0 +1,237 @@
+# ASAP
+
+[](https://travis-ci.org/kriskowal/asap)
+
+Promise and asynchronous observer libraries, as well as hand-rolled callback
+programs and libraries, often need a mechanism to postpone the execution of a
+callback until the next available event.
+(See [Designing API’s for Asynchrony][Zalgo].)
+The `asap` function executes a task **as soon as possible** but not before it
+returns, waiting only for the completion of the current event and previously
+scheduled tasks.
+
+```javascript
+asap(function () {
+ // ...
+});
+```
+
+[Zalgo]: http://blog.izs.me/post/59142742143/designing-apis-for-asynchrony
+
+This CommonJS package provides an `asap` module that exports a function that
+executes a task function *as soon as possible*.
+
+ASAP strives to schedule events to occur before yielding for IO, reflow,
+or redrawing.
+Each event receives an independent stack, with only platform code in parent
+frames and the events run in the order they are scheduled.
+
+ASAP provides a fast event queue that will execute tasks until it is
+empty before yielding to the JavaScript engine's underlying event-loop.
+When a task gets added to a previously empty event queue, ASAP schedules a flush
+event, preferring for that event to occur before the JavaScript engine has an
+opportunity to perform IO tasks or rendering, thus making the first task and
+subsequent tasks semantically indistinguishable.
+ASAP uses a variety of techniques to preserve this invariant on different
+versions of browsers and Node.js.
+
+By design, ASAP prevents input events from being handled until the task
+queue is empty.
+If the process is busy enough, this may cause incoming connection requests to be
+dropped, and may cause existing connections to inform the sender to reduce the
+transmission rate or stall.
+ASAP allows this on the theory that, if there is enough work to do, there is no
+sense in looking for trouble.
+As a consequence, ASAP can interfere with smooth animation.
+If your task should be tied to the rendering loop, consider using
+`requestAnimationFrame` instead.
+A long sequence of tasks can also effect the long running script dialog.
+If this is a problem, you may be able to use ASAP’s cousin `setImmediate` to
+break long processes into shorter intervals and periodically allow the browser
+to breathe.
+`setImmediate` will yield for IO, reflow, and repaint events.
+It also returns a handler and can be canceled.
+For a `setImmediate` shim, consider [YuzuJS setImmediate][setImmediate].
+
+[setImmediate]: https://github.com/YuzuJS/setImmediate
+
+Take care.
+ASAP can sustain infinite recursive calls without warning.
+It will not halt from a stack overflow, and it will not consume unbounded
+memory.
+This is behaviorally equivalent to an infinite loop.
+Just as with infinite loops, you can monitor a Node.js process for this behavior
+with a heart-beat signal.
+As with infinite loops, a very small amount of caution goes a long way to
+avoiding problems.
+
+```javascript
+function loop() {
+ asap(loop);
+}
+loop();
+```
+
+In browsers, if a task throws an exception, it will not interrupt the flushing
+of high-priority tasks.
+The exception will be postponed to a later, low-priority event to avoid
+slow-downs.
+In Node.js, if a task throws an exception, ASAP will resume flushing only if—and
+only after—the error is handled by `domain.on("error")` or
+`process.on("uncaughtException")`.
+
+## Raw ASAP
+
+Checking for exceptions comes at a cost.
+The package also provides an `asap/raw` module that exports the underlying
+implementation which is faster but stalls if a task throws an exception.
+This internal version of the ASAP function does not check for errors.
+If a task does throw an error, it will stall the event queue unless you manually
+call `rawAsap.requestFlush()` before throwing the error, or any time after.
+
+In Node.js, `asap/raw` also runs all tasks outside any domain.
+If you need a task to be bound to your domain, you will have to do it manually.
+
+```js
+if (process.domain) {
+ task = process.domain.bind(task);
+}
+rawAsap(task);
+```
+
+## Tasks
+
+A task may be any object that implements `call()`.
+A function will suffice, but closures tend not to be reusable and can cause
+garbage collector churn.
+Both `asap` and `rawAsap` accept task objects to give you the option of
+recycling task objects or using higher callable object abstractions.
+See the `asap` source for an illustration.
+
+
+## Compatibility
+
+ASAP is tested on Node.js v0.10 and in a broad spectrum of web browsers.
+The following charts capture the browser test results for the most recent
+release.
+The first chart shows test results for ASAP running in the main window context.
+The second chart shows test results for ASAP running in a web worker context.
+Test results are inconclusive (grey) on browsers that do not support web
+workers.
+These data are captured automatically by [Continuous
+Integration][].
+
+[Continuous Integration]: https://github.com/kriskowal/asap/blob/master/CONTRIBUTING.md
+
+
+
+
+
+## Caveats
+
+When a task is added to an empty event queue, it is not always possible to
+guarantee that the task queue will begin flushing immediately after the current
+event.
+However, once the task queue begins flushing, it will not yield until the queue
+is empty, even if the queue grows while executing tasks.
+
+The following browsers allow the use of [DOM mutation observers][] to access
+the HTML [microtask queue][], and thus begin flushing ASAP's task queue
+immediately at the end of the current event loop turn, before any rendering or
+IO:
+
+[microtask queue]: http://www.whatwg.org/specs/web-apps/current-work/multipage/webappapis.html#microtask-queue
+[DOM mutation observers]: http://dom.spec.whatwg.org/#mutation-observers
+
+- Android 4–4.3
+- Chrome 26–34
+- Firefox 14–29
+- Internet Explorer 11
+- iPad Safari 6–7.1
+- iPhone Safari 7–7.1
+- Safari 6–7
+
+In the absense of mutation observers, there are a few browsers, and situations
+like web workers in some of the above browsers, where [message channels][]
+would be a useful way to avoid falling back to timers.
+Message channels give direct access to the HTML [task queue][], so the ASAP
+task queue would flush after any already queued rendering and IO tasks, but
+without having the minimum delay imposed by timers.
+However, among these browsers, Internet Explorer 10 and Safari do not reliably
+dispatch messages, so they are not worth the trouble to implement.
+
+[message channels]: http://www.whatwg.org/specs/web-apps/current-work/multipage/web-messaging.html#message-channels
+[task queue]: http://www.whatwg.org/specs/web-apps/current-work/multipage/webappapis.html#concept-task
+
+- Internet Explorer 10
+- Safair 5.0-1
+- Opera 11-12
+
+In the absense of mutation observers, these browsers and the following browsers
+all fall back to using `setTimeout` and `setInterval` to ensure that a `flush`
+occurs.
+The implementation uses both and cancels whatever handler loses the race, since
+`setTimeout` tends to occasionally skip tasks in unisolated circumstances.
+Timers generally delay the flushing of ASAP's task queue for four milliseconds.
+
+- Firefox 3–13
+- Internet Explorer 6–10
+- iPad Safari 4.3
+- Lynx 2.8.7
+
+
+## Heritage
+
+ASAP has been factored out of the [Q][] asynchronous promise library.
+It originally had a naïve implementation in terms of `setTimeout`, but
+[Malte Ubl][NonBlocking] provided an insight that `postMessage` might be
+useful for creating a high-priority, no-delay event dispatch hack.
+Since then, Internet Explorer proposed and implemented `setImmediate`.
+Robert Katić began contributing to Q by measuring the performance of
+the internal implementation of `asap`, paying particular attention to
+error recovery.
+Domenic, Robert, and Kris Kowal collectively settled on the current strategy of
+unrolling the high-priority event queue internally regardless of what strategy
+we used to dispatch the potentially lower-priority flush event.
+Domenic went on to make ASAP cooperate with Node.js domains.
+
+[Q]: https://github.com/kriskowal/q
+[NonBlocking]: http://www.nonblocking.io/2011/06/windownexttick.html
+
+For further reading, Nicholas Zakas provided a thorough article on [The
+Case for setImmediate][NCZ].
+
+[NCZ]: http://www.nczonline.net/blog/2013/07/09/the-case-for-setimmediate/
+
+Ember’s RSVP promise implementation later [adopted][RSVP ASAP] the name ASAP but
+further developed the implentation.
+Particularly, The `MessagePort` implementation was abandoned due to interaction
+[problems with Mobile Internet Explorer][IE Problems] in favor of an
+implementation backed on the newer and more reliable DOM `MutationObserver`
+interface.
+These changes were back-ported into this library.
+
+[IE Problems]: https://github.com/cujojs/when/issues/197
+[RSVP ASAP]: https://github.com/tildeio/rsvp.js/blob/cddf7232546a9cf858524b75cde6f9edf72620a7/lib/rsvp/asap.js
+
+In addition, ASAP factored into `asap` and `asap/raw`, such that `asap` remained
+exception-safe, but `asap/raw` provided a tight kernel that could be used for
+tasks that guaranteed that they would not throw exceptions.
+This core is useful for promise implementations that capture thrown errors in
+rejected promises and do not need a second safety net.
+At the same time, the exception handling in `asap` was factored into separate
+implementations for Node.js and browsers, using the the [Browserify][Browser
+Config] `browser` property in `package.json` to instruct browser module loaders
+and bundlers, including [Browserify][], [Mr][], and [Mop][], to use the
+browser-only implementation.
+
+[Browser Config]: https://gist.github.com/defunctzombie/4339901
+[Browserify]: https://github.com/substack/node-browserify
+[Mr]: https://github.com/montagejs/mr
+[Mop]: https://github.com/montagejs/mop
+
+## License
+
+Copyright 2009-2014 by Contributors
+MIT License (enclosed)
+
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/asap.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/asap.js
new file mode 100644
index 00000000..f04fcd58
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/asap.js
@@ -0,0 +1,65 @@
+"use strict";
+
+var rawAsap = require("./raw");
+var freeTasks = [];
+
+/**
+ * Calls a task as soon as possible after returning, in its own event, with
+ * priority over IO events. An exception thrown in a task can be handled by
+ * `process.on("uncaughtException") or `domain.on("error")`, but will otherwise
+ * crash the process. If the error is handled, all subsequent tasks will
+ * resume.
+ *
+ * @param {{call}} task A callable object, typically a function that takes no
+ * arguments.
+ */
+module.exports = asap;
+function asap(task) {
+ var rawTask;
+ if (freeTasks.length) {
+ rawTask = freeTasks.pop();
+ } else {
+ rawTask = new RawTask();
+ }
+ rawTask.task = task;
+ rawTask.domain = process.domain;
+ rawAsap(rawTask);
+}
+
+function RawTask() {
+ this.task = null;
+ this.domain = null;
+}
+
+RawTask.prototype.call = function () {
+ if (this.domain) {
+ this.domain.enter();
+ }
+ var threw = true;
+ try {
+ this.task.call();
+ threw = false;
+ // If the task throws an exception (presumably) Node.js restores the
+ // domain stack for the next event.
+ if (this.domain) {
+ this.domain.exit();
+ }
+ } finally {
+ // We use try/finally and a threw flag to avoid messing up stack traces
+ // when we catch and release errors.
+ if (threw) {
+ // In Node.js, uncaught exceptions are considered fatal errors.
+ // Re-throw them to interrupt flushing!
+ // Ensure that flushing continues if an uncaught exception is
+ // suppressed listening process.on("uncaughtException") or
+ // domain.on("error").
+ rawAsap.requestFlush();
+ }
+ // If the task threw an error, we do not want to exit the domain here.
+ // Exiting the domain would prevent the domain from catching the error.
+ this.task = null;
+ this.domain = null;
+ freeTasks.push(this);
+ }
+};
+
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/browser-asap.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/browser-asap.js
new file mode 100644
index 00000000..805c9824
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/browser-asap.js
@@ -0,0 +1,66 @@
+"use strict";
+
+// rawAsap provides everything we need except exception management.
+var rawAsap = require("./raw");
+// RawTasks are recycled to reduce GC churn.
+var freeTasks = [];
+// We queue errors to ensure they are thrown in right order (FIFO).
+// Array-as-queue is good enough here, since we are just dealing with exceptions.
+var pendingErrors = [];
+var requestErrorThrow = rawAsap.makeRequestCallFromTimer(throwFirstError);
+
+function throwFirstError() {
+ if (pendingErrors.length) {
+ throw pendingErrors.shift();
+ }
+}
+
+/**
+ * Calls a task as soon as possible after returning, in its own event, with priority
+ * over other events like animation, reflow, and repaint. An error thrown from an
+ * event will not interrupt, nor even substantially slow down the processing of
+ * other events, but will be rather postponed to a lower priority event.
+ * @param {{call}} task A callable object, typically a function that takes no
+ * arguments.
+ */
+module.exports = asap;
+function asap(task) {
+ var rawTask;
+ if (freeTasks.length) {
+ rawTask = freeTasks.pop();
+ } else {
+ rawTask = new RawTask();
+ }
+ rawTask.task = task;
+ rawAsap(rawTask);
+}
+
+// We wrap tasks with recyclable task objects. A task object implements
+// `call`, just like a function.
+function RawTask() {
+ this.task = null;
+}
+
+// The sole purpose of wrapping the task is to catch the exception and recycle
+// the task object after its single use.
+RawTask.prototype.call = function () {
+ try {
+ this.task.call();
+ } catch (error) {
+ if (asap.onerror) {
+ // This hook exists purely for testing purposes.
+ // Its name will be periodically randomized to break any code that
+ // depends on its existence.
+ asap.onerror(error);
+ } else {
+ // In a web browser, exceptions are not fatal. However, to avoid
+ // slowing down the queue of pending tasks, we rethrow the error in a
+ // lower priority turn.
+ pendingErrors.push(error);
+ requestErrorThrow();
+ }
+ } finally {
+ this.task = null;
+ freeTasks[freeTasks.length] = this;
+ }
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/browser-raw.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/browser-raw.js
new file mode 100644
index 00000000..9cee7e32
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/browser-raw.js
@@ -0,0 +1,223 @@
+"use strict";
+
+// Use the fastest means possible to execute a task in its own turn, with
+// priority over other events including IO, animation, reflow, and redraw
+// events in browsers.
+//
+// An exception thrown by a task will permanently interrupt the processing of
+// subsequent tasks. The higher level `asap` function ensures that if an
+// exception is thrown by a task, that the task queue will continue flushing as
+// soon as possible, but if you use `rawAsap` directly, you are responsible to
+// either ensure that no exceptions are thrown from your task, or to manually
+// call `rawAsap.requestFlush` if an exception is thrown.
+module.exports = rawAsap;
+function rawAsap(task) {
+ if (!queue.length) {
+ requestFlush();
+ flushing = true;
+ }
+ // Equivalent to push, but avoids a function call.
+ queue[queue.length] = task;
+}
+
+var queue = [];
+// Once a flush has been requested, no further calls to `requestFlush` are
+// necessary until the next `flush` completes.
+var flushing = false;
+// `requestFlush` is an implementation-specific method that attempts to kick
+// off a `flush` event as quickly as possible. `flush` will attempt to exhaust
+// the event queue before yielding to the browser's own event loop.
+var requestFlush;
+// The position of the next task to execute in the task queue. This is
+// preserved between calls to `flush` so that it can be resumed if
+// a task throws an exception.
+var index = 0;
+// If a task schedules additional tasks recursively, the task queue can grow
+// unbounded. To prevent memory exhaustion, the task queue will periodically
+// truncate already-completed tasks.
+var capacity = 1024;
+
+// The flush function processes all tasks that have been scheduled with
+// `rawAsap` unless and until one of those tasks throws an exception.
+// If a task throws an exception, `flush` ensures that its state will remain
+// consistent and will resume where it left off when called again.
+// However, `flush` does not make any arrangements to be called again if an
+// exception is thrown.
+function flush() {
+ while (index < queue.length) {
+ var currentIndex = index;
+ // Advance the index before calling the task. This ensures that we will
+ // begin flushing on the next task the task throws an error.
+ index = index + 1;
+ queue[currentIndex].call();
+ // Prevent leaking memory for long chains of recursive calls to `asap`.
+ // If we call `asap` within tasks scheduled by `asap`, the queue will
+ // grow, but to avoid an O(n) walk for every task we execute, we don't
+ // shift tasks off the queue after they have been executed.
+ // Instead, we periodically shift 1024 tasks off the queue.
+ if (index > capacity) {
+ // Manually shift all values starting at the index back to the
+ // beginning of the queue.
+ for (var scan = 0, newLength = queue.length - index; scan < newLength; scan++) {
+ queue[scan] = queue[scan + index];
+ }
+ queue.length -= index;
+ index = 0;
+ }
+ }
+ queue.length = 0;
+ index = 0;
+ flushing = false;
+}
+
+// `requestFlush` is implemented using a strategy based on data collected from
+// every available SauceLabs Selenium web driver worker at time of writing.
+// https://docs.google.com/spreadsheets/d/1mG-5UYGup5qxGdEMWkhP6BWCz053NUb2E1QoUTU16uA/edit#gid=783724593
+
+// Safari 6 and 6.1 for desktop, iPad, and iPhone are the only browsers that
+// have WebKitMutationObserver but not un-prefixed MutationObserver.
+// Must use `global` or `self` instead of `window` to work in both frames and web
+// workers. `global` is a provision of Browserify, Mr, Mrs, or Mop.
+
+/* globals self */
+var scope = typeof global !== "undefined" ? global : self;
+var BrowserMutationObserver = scope.MutationObserver || scope.WebKitMutationObserver;
+
+// MutationObservers are desirable because they have high priority and work
+// reliably everywhere they are implemented.
+// They are implemented in all modern browsers.
+//
+// - Android 4-4.3
+// - Chrome 26-34
+// - Firefox 14-29
+// - Internet Explorer 11
+// - iPad Safari 6-7.1
+// - iPhone Safari 7-7.1
+// - Safari 6-7
+if (typeof BrowserMutationObserver === "function") {
+ requestFlush = makeRequestCallFromMutationObserver(flush);
+
+// MessageChannels are desirable because they give direct access to the HTML
+// task queue, are implemented in Internet Explorer 10, Safari 5.0-1, and Opera
+// 11-12, and in web workers in many engines.
+// Although message channels yield to any queued rendering and IO tasks, they
+// would be better than imposing the 4ms delay of timers.
+// However, they do not work reliably in Internet Explorer or Safari.
+
+// Internet Explorer 10 is the only browser that has setImmediate but does
+// not have MutationObservers.
+// Although setImmediate yields to the browser's renderer, it would be
+// preferrable to falling back to setTimeout since it does not have
+// the minimum 4ms penalty.
+// Unfortunately there appears to be a bug in Internet Explorer 10 Mobile (and
+// Desktop to a lesser extent) that renders both setImmediate and
+// MessageChannel useless for the purposes of ASAP.
+// https://github.com/kriskowal/q/issues/396
+
+// Timers are implemented universally.
+// We fall back to timers in workers in most engines, and in foreground
+// contexts in the following browsers.
+// However, note that even this simple case requires nuances to operate in a
+// broad spectrum of browsers.
+//
+// - Firefox 3-13
+// - Internet Explorer 6-9
+// - iPad Safari 4.3
+// - Lynx 2.8.7
+} else {
+ requestFlush = makeRequestCallFromTimer(flush);
+}
+
+// `requestFlush` requests that the high priority event queue be flushed as
+// soon as possible.
+// This is useful to prevent an error thrown in a task from stalling the event
+// queue if the exception handled by Node.js’s
+// `process.on("uncaughtException")` or by a domain.
+rawAsap.requestFlush = requestFlush;
+
+// To request a high priority event, we induce a mutation observer by toggling
+// the text of a text node between "1" and "-1".
+function makeRequestCallFromMutationObserver(callback) {
+ var toggle = 1;
+ var observer = new BrowserMutationObserver(callback);
+ var node = document.createTextNode("");
+ observer.observe(node, {characterData: true});
+ return function requestCall() {
+ toggle = -toggle;
+ node.data = toggle;
+ };
+}
+
+// The message channel technique was discovered by Malte Ubl and was the
+// original foundation for this library.
+// http://www.nonblocking.io/2011/06/windownexttick.html
+
+// Safari 6.0.5 (at least) intermittently fails to create message ports on a
+// page's first load. Thankfully, this version of Safari supports
+// MutationObservers, so we don't need to fall back in that case.
+
+// function makeRequestCallFromMessageChannel(callback) {
+// var channel = new MessageChannel();
+// channel.port1.onmessage = callback;
+// return function requestCall() {
+// channel.port2.postMessage(0);
+// };
+// }
+
+// For reasons explained above, we are also unable to use `setImmediate`
+// under any circumstances.
+// Even if we were, there is another bug in Internet Explorer 10.
+// It is not sufficient to assign `setImmediate` to `requestFlush` because
+// `setImmediate` must be called *by name* and therefore must be wrapped in a
+// closure.
+// Never forget.
+
+// function makeRequestCallFromSetImmediate(callback) {
+// return function requestCall() {
+// setImmediate(callback);
+// };
+// }
+
+// Safari 6.0 has a problem where timers will get lost while the user is
+// scrolling. This problem does not impact ASAP because Safari 6.0 supports
+// mutation observers, so that implementation is used instead.
+// However, if we ever elect to use timers in Safari, the prevalent work-around
+// is to add a scroll event listener that calls for a flush.
+
+// `setTimeout` does not call the passed callback if the delay is less than
+// approximately 7 in web workers in Firefox 8 through 18, and sometimes not
+// even then.
+
+function makeRequestCallFromTimer(callback) {
+ return function requestCall() {
+ // We dispatch a timeout with a specified delay of 0 for engines that
+ // can reliably accommodate that request. This will usually be snapped
+ // to a 4 milisecond delay, but once we're flushing, there's no delay
+ // between events.
+ var timeoutHandle = setTimeout(handleTimer, 0);
+ // However, since this timer gets frequently dropped in Firefox
+ // workers, we enlist an interval handle that will try to fire
+ // an event 20 times per second until it succeeds.
+ var intervalHandle = setInterval(handleTimer, 50);
+
+ function handleTimer() {
+ // Whichever timer succeeds will cancel both timers and
+ // execute the callback.
+ clearTimeout(timeoutHandle);
+ clearInterval(intervalHandle);
+ callback();
+ }
+ };
+}
+
+// This is for `asap.js` only.
+// Its name will be periodically randomized to break any code that depends on
+// its existence.
+rawAsap.makeRequestCallFromTimer = makeRequestCallFromTimer;
+
+// ASAP was originally a nextTick shim included in Q. This was factored out
+// into this ASAP package. It was later adapted to RSVP which made further
+// amendments. These decisions, particularly to marginalize MessageChannel and
+// to capture the MutationObserver implementation in a closure, were integrated
+// back into ASAP proper.
+// https://github.com/tildeio/rsvp.js/blob/cddf7232546a9cf858524b75cde6f9edf72620a7/lib/rsvp/asap.js
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/package.json b/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/package.json
new file mode 100644
index 00000000..3b88c321
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/package.json
@@ -0,0 +1,87 @@
+{
+ "_from": "asap@~2.0.3",
+ "_id": "asap@2.0.6",
+ "_inBundle": false,
+ "_integrity": "sha1-5QNHYR1+aQlDIIu9r+vLwvuGbUY=",
+ "_location": "/react-dropzone/asap",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "asap@~2.0.3",
+ "name": "asap",
+ "escapedName": "asap",
+ "rawSpec": "~2.0.3",
+ "saveSpec": null,
+ "fetchSpec": "~2.0.3"
+ },
+ "_requiredBy": [
+ "/react-dropzone/promise"
+ ],
+ "_resolved": "https://registry.npmjs.org/asap/-/asap-2.0.6.tgz",
+ "_shasum": "e50347611d7e690943208bbdafebcbc2fb866d46",
+ "_spec": "asap@~2.0.3",
+ "_where": "C:\\Users\\deranjer\\go\\src\\github.com\\deranjer\\goTorrent\\goTorrentWebUI\\node_modules\\react-dropzone\\node_modules\\promise",
+ "browser": {
+ "./asap": "./browser-asap.js",
+ "./asap.js": "./browser-asap.js",
+ "./raw": "./browser-raw.js",
+ "./raw.js": "./browser-raw.js",
+ "./test/domain.js": "./test/browser-domain.js"
+ },
+ "bugs": {
+ "url": "https://github.com/kriskowal/asap/issues"
+ },
+ "bundleDependencies": false,
+ "deprecated": false,
+ "description": "High-priority task queue for Node.js and browsers",
+ "devDependencies": {
+ "benchmark": "^1.0.0",
+ "events": "^1.0.1",
+ "jshint": "^2.5.1",
+ "knox": "^0.8.10",
+ "mr": "^2.0.5",
+ "opener": "^1.3.0",
+ "q": "^2.0.3",
+ "q-io": "^2.0.3",
+ "saucelabs": "^0.1.1",
+ "wd": "^0.2.21",
+ "weak-map": "^1.0.5"
+ },
+ "files": [
+ "raw.js",
+ "asap.js",
+ "browser-raw.js",
+ "browser-asap.js"
+ ],
+ "homepage": "https://github.com/kriskowal/asap#readme",
+ "keywords": [
+ "event",
+ "task",
+ "queue"
+ ],
+ "license": "MIT",
+ "main": "./asap.js",
+ "name": "asap",
+ "react-native": {
+ "domain": false
+ },
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/kriskowal/asap.git"
+ },
+ "scripts": {
+ "benchmarks": "node benchmarks",
+ "lint": "jshint raw.js asap.js browser-raw.js browser-asap.js $(find scripts -name '*.js' | grep -v gauntlet)",
+ "test": "npm run lint && npm run test-node",
+ "test-browser": "node scripts/publish-bundle.js test/asap-test.js | xargs opener",
+ "test-node": "node test/asap-test.js",
+ "test-publish": "node scripts/publish-bundle.js test/asap-test.js | pbcopy",
+ "test-saucelabs": "node scripts/saucelabs.js test/asap-test.js scripts/saucelabs-spot-configurations.json",
+ "test-saucelabs-all": "node scripts/saucelabs.js test/asap-test.js scripts/saucelabs-all-configurations.json",
+ "test-saucelabs-worker": "node scripts/saucelabs-worker-test.js scripts/saucelabs-spot-configurations.json",
+ "test-saucelabs-worker-all": "node scripts/saucelabs-worker-test.js scripts/saucelabs-all-configurations.json",
+ "test-travis": "npm run lint && npm run test-node && npm run test-saucelabs && npm run test-saucelabs-worker"
+ },
+ "version": "2.0.6"
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/raw.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/raw.js
new file mode 100644
index 00000000..ae3b8923
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/asap/raw.js
@@ -0,0 +1,101 @@
+"use strict";
+
+var domain; // The domain module is executed on demand
+var hasSetImmediate = typeof setImmediate === "function";
+
+// Use the fastest means possible to execute a task in its own turn, with
+// priority over other events including network IO events in Node.js.
+//
+// An exception thrown by a task will permanently interrupt the processing of
+// subsequent tasks. The higher level `asap` function ensures that if an
+// exception is thrown by a task, that the task queue will continue flushing as
+// soon as possible, but if you use `rawAsap` directly, you are responsible to
+// either ensure that no exceptions are thrown from your task, or to manually
+// call `rawAsap.requestFlush` if an exception is thrown.
+module.exports = rawAsap;
+function rawAsap(task) {
+ if (!queue.length) {
+ requestFlush();
+ flushing = true;
+ }
+ // Avoids a function call
+ queue[queue.length] = task;
+}
+
+var queue = [];
+// Once a flush has been requested, no further calls to `requestFlush` are
+// necessary until the next `flush` completes.
+var flushing = false;
+// The position of the next task to execute in the task queue. This is
+// preserved between calls to `flush` so that it can be resumed if
+// a task throws an exception.
+var index = 0;
+// If a task schedules additional tasks recursively, the task queue can grow
+// unbounded. To prevent memory excaustion, the task queue will periodically
+// truncate already-completed tasks.
+var capacity = 1024;
+
+// The flush function processes all tasks that have been scheduled with
+// `rawAsap` unless and until one of those tasks throws an exception.
+// If a task throws an exception, `flush` ensures that its state will remain
+// consistent and will resume where it left off when called again.
+// However, `flush` does not make any arrangements to be called again if an
+// exception is thrown.
+function flush() {
+ while (index < queue.length) {
+ var currentIndex = index;
+ // Advance the index before calling the task. This ensures that we will
+ // begin flushing on the next task the task throws an error.
+ index = index + 1;
+ queue[currentIndex].call();
+ // Prevent leaking memory for long chains of recursive calls to `asap`.
+ // If we call `asap` within tasks scheduled by `asap`, the queue will
+ // grow, but to avoid an O(n) walk for every task we execute, we don't
+ // shift tasks off the queue after they have been executed.
+ // Instead, we periodically shift 1024 tasks off the queue.
+ if (index > capacity) {
+ // Manually shift all values starting at the index back to the
+ // beginning of the queue.
+ for (var scan = 0, newLength = queue.length - index; scan < newLength; scan++) {
+ queue[scan] = queue[scan + index];
+ }
+ queue.length -= index;
+ index = 0;
+ }
+ }
+ queue.length = 0;
+ index = 0;
+ flushing = false;
+}
+
+rawAsap.requestFlush = requestFlush;
+function requestFlush() {
+ // Ensure flushing is not bound to any domain.
+ // It is not sufficient to exit the domain, because domains exist on a stack.
+ // To execute code outside of any domain, the following dance is necessary.
+ var parentDomain = process.domain;
+ if (parentDomain) {
+ if (!domain) {
+ // Lazy execute the domain module.
+ // Only employed if the user elects to use domains.
+ domain = require("domain");
+ }
+ domain.active = process.domain = null;
+ }
+
+ // `setImmediate` is slower that `process.nextTick`, but `process.nextTick`
+ // cannot handle recursion.
+ // `requestFlush` will only be called recursively from `asap.js`, to resume
+ // flushing after an error is thrown into a domain.
+ // Conveniently, `setImmediate` was introduced in the same version
+ // `process.nextTick` started throwing recursion errors.
+ if (flushing && hasSetImmediate) {
+ setImmediate(flush);
+ } else {
+ process.nextTick(flush);
+ }
+
+ if (parentDomain) {
+ domain.active = process.domain = parentDomain;
+ }
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/CHANGELOG.md b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/CHANGELOG.md
new file mode 100644
index 00000000..6fbcbb41
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/CHANGELOG.md
@@ -0,0 +1,409 @@
+## Changelog
+##### 1.2.7 [LEGACY] - 2016.07.18
+* some fixes for issues like #159, #186, #194, #207
+
+##### 1.2.6 - 2015.11.09
+* reject with `TypeError` on attempt resolve promise itself
+* correct behavior with broken `Promise` subclass constructors / methods
+* added `Promise`-based fallback for microtask
+* fixed V8 and FF `Array#{values, @@iterator}.name`
+* fixed IE7- `[1, 2].join(undefined) -> '1,2'`
+* some other fixes / improvements / optimizations
+
+##### 1.2.5 - 2015.11.02
+* some more `Number` constructor fixes:
+ * fixed V8 ~ Node 0.8 bug: `Number('+0x1')` should be `NaN`
+ * fixed `Number(' 0b1\n')` case, should be `1`
+ * fixed `Number()` case, should be `0`
+
+##### 1.2.4 - 2015.11.01
+* fixed `Number('0b12') -> NaN` case in the shim
+* fixed V8 ~ Chromium 40- bug - `Weak(Map|Set)#{delete, get, has}` should not throw errors [#124](https://github.com/zloirock/core-js/issues/124)
+* some other fixes and optimizations
+
+##### 1.2.3 - 2015.10.23
+* fixed some problems related old V8 bug `Object('a').propertyIsEnumerable(0) // => false`, for example, `Object.assign({}, 'qwe')` from the last release
+* fixed `.name` property and `Function#toString` conversion some polyfilled methods
+* fixed `Math.imul` arity in Safari 8-
+
+##### 1.2.2 - 2015.10.18
+* improved optimisations for V8
+* fixed build process from external packages, [#120](https://github.com/zloirock/core-js/pull/120)
+* one more `Object.{assign, values, entries}` fix for [**very** specific case](https://github.com/ljharb/proposal-object-values-entries/issues/5)
+
+##### 1.2.1 - 2015.10.02
+* replaced fix `JSON.stringify` + `Symbol` behavior from `.toJSON` method to wrapping `JSON.stringify` - little more correct, [compat-table/642](https://github.com/kangax/compat-table/pull/642)
+* fixed typo which broke tasks scheduler in WebWorkers in old FF, [#114](https://github.com/zloirock/core-js/pull/114)
+
+##### 1.2.0 - 2015.09.27
+* added browser [`Promise` rejection hook](#unhandled-rejection-tracking), [#106](https://github.com/zloirock/core-js/issues/106)
+* added correct [`IsRegExp`](http://www.ecma-international.org/ecma-262/6.0/#sec-isregexp) logic to [`String#{includes, startsWith, endsWith}`](https://github.com/zloirock/core-js/#ecmascript-6-string) and [`RegExp` constructor](https://github.com/zloirock/core-js/#ecmascript-6-regexp), `@@match` case, [example](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Symbol/match#Disabling_the_isRegExp_check)
+* updated [`String#leftPad`](https://github.com/zloirock/core-js/#ecmascript-7) [with proposal](https://github.com/ljharb/proposal-string-pad-left-right/issues/6): string filler truncated from the right side
+* replaced V8 [`Object.assign`](https://github.com/zloirock/core-js/#ecmascript-6-object) - its properties order not only [incorrect](https://github.com/sindresorhus/object-assign/issues/22), it is non-deterministic and it causes some problems
+* fixed behavior with deleted in getters properties for `Object.{`[`assign`](https://github.com/zloirock/core-js/#ecmascript-6-object)`, `[`entries, values`](https://github.com/zloirock/core-js/#ecmascript-7)`}`, [example](http://goo.gl/iQE01c)
+* fixed [`Math.sinh`](https://github.com/zloirock/core-js/#ecmascript-6-math) with very small numbers in V8 near Chromium 38
+* some other fixes and optimizations
+
+##### 1.1.4 - 2015.09.05
+* fixed support symbols in FF34-35 [`Object.assign`](https://github.com/zloirock/core-js/#ecmascript-6-object)
+* fixed [collections iterators](https://github.com/zloirock/core-js/#ecmascript-6-iterators) in FF25-26
+* fixed non-generic WebKit [`Array.of`](https://github.com/zloirock/core-js/#ecmascript-6-array)
+* some other fixes and optimizations
+
+##### 1.1.3 - 2015.08.29
+* fixed support Node.js domains in [`Promise`](https://github.com/zloirock/core-js/#ecmascript-6-promise), [#103](https://github.com/zloirock/core-js/issues/103)
+
+##### 1.1.2 - 2015.08.28
+* added `toJSON` method to [`Symbol`](https://github.com/zloirock/core-js/#ecmascript-6-symbol) polyfill and to MS Edge implementation for expected `JSON.stringify` result w/o patching this method
+* replaced [`Reflect.construct`](https://github.com/zloirock/core-js/#ecmascript-6-reflect) implementations w/o correct support third argument
+* fixed `global` detection with changed `document.domain` in ~IE8, [#100](https://github.com/zloirock/core-js/issues/100)
+
+##### 1.1.1 - 2015.08.20
+* added more correct microtask implementation for [`Promise`](#ecmascript-6-promise)
+
+##### 1.1.0 - 2015.08.17
+* updated [string padding](https://github.com/zloirock/core-js/#ecmascript-7) to [actual proposal](https://github.com/ljharb/proposal-string-pad-left-right) - renamed, minor internal changes:
+ * `String#lpad` -> `String#padLeft`
+ * `String#rpad` -> `String#padRight`
+* added [string trim functions](#ecmascript-7) - [proposal](https://github.com/sebmarkbage/ecmascript-string-left-right-trim), defacto standard - required only for IE11- and fixed for some old engines:
+ * `String#trimLeft`
+ * `String#trimRight`
+* [`String#trim`](https://github.com/zloirock/core-js/#ecmascript-6-string) fixed for some engines by es6 spec and moved from `es5` to single `es6` module
+* splitted [`es6.object.statics-accept-primitives`](https://github.com/zloirock/core-js/#ecmascript-6-object)
+* caps for `freeze`-family `Object` methods moved from `es5` to `es6` namespace and joined with [es6 wrappers](https://github.com/zloirock/core-js/#ecmascript-6-object)
+* `es5` [namespace](https://github.com/zloirock/core-js/#commonjs) also includes modules, moved to `es6` namespace - you can use it as before
+* increased `MessageChannel` priority in `$.task`, [#95](https://github.com/zloirock/core-js/issues/95)
+* does not get `global.Symbol` on each getting iterator, if you wanna use alternative `Symbol` shim - add it before `core-js`
+* [`Reflect.construct`](https://github.com/zloirock/core-js/#ecmascript-6-reflect) optimized and fixed for some cases
+* simplified [`Reflect.enumerate`](https://github.com/zloirock/core-js/#ecmascript-6-reflect), see [this question](https://esdiscuss.org/topic/question-about-enumerate-and-property-decision-timing)
+* some corrections in [`Math.acosh`](https://github.com/zloirock/core-js/#ecmascript-6-math)
+* fixed [`Math.imul`](https://github.com/zloirock/core-js/#ecmascript-6-math) for old WebKit
+* some fixes in string / RegExp [well-known symbols](https://github.com/zloirock/core-js/#ecmascript-6-regexp) logic
+* some other fixes and optimizations
+
+##### 1.0.1 - 2015.07.31
+* some fixes for final MS Edge, replaced broken native `Reflect.defineProperty`
+* some minor fixes and optimizations
+* changed compression `client/*.min.js` options for safe `Function#name` and `Function#length`, should be fixed [#92](https://github.com/zloirock/core-js/issues/92)
+
+##### 1.0.0 - 2015.07.22
+* added logic for [well-known symbols](https://github.com/zloirock/core-js/#ecmascript-6-regexp):
+ * `Symbol.match`
+ * `Symbol.replace`
+ * `Symbol.split`
+ * `Symbol.search`
+* actualized and optimized work with iterables:
+ * optimized [`Map`, `Set`, `WeakMap`, `WeakSet` constructors](https://github.com/zloirock/core-js/#ecmascript-6-collections), [`Promise.all`, `Promise.race`](https://github.com/zloirock/core-js/#ecmascript-6-promise) for default `Array Iterator`
+ * optimized [`Array.from`](https://github.com/zloirock/core-js/#ecmascript-6-array) for default `Array Iterator`
+ * added [`core.getIteratorMethod`](https://github.com/zloirock/core-js/#ecmascript-6-iterators) helper
+* uses enumerable properties in shimmed instances - collections, iterators, etc for optimize performance
+* added support native constructors to [`Reflect.construct`](https://github.com/zloirock/core-js/#ecmascript-6-reflect) with 2 arguments
+* added support native constructors to [`Function#bind`](https://github.com/zloirock/core-js/#ecmascript-5) shim with `new`
+* removed obsolete `.clear` methods native [`Weak`-collections](https://github.com/zloirock/core-js/#ecmascript-6-collections)
+* maximum modularity, reduced minimal custom build size, separated into submodules:
+ * [`es6.reflect`](https://github.com/zloirock/core-js/#ecmascript-6-reflect)
+ * [`es6.regexp`](https://github.com/zloirock/core-js/#ecmascript-6-regexp)
+ * [`es6.math`](https://github.com/zloirock/core-js/#ecmascript-6-math)
+ * [`es6.number`](https://github.com/zloirock/core-js/#ecmascript-6-number)
+ * [`es7.object.to-array`](https://github.com/zloirock/core-js/#ecmascript-7)
+ * [`core.object`](https://github.com/zloirock/core-js/#object)
+ * [`core.string`](https://github.com/zloirock/core-js/#escaping-html)
+ * [`core.iter-helpers`](https://github.com/zloirock/core-js/#ecmascript-6-iterators)
+ * internal modules (`$`, `$.iter`, etc)
+* many other optimizations
+* final cleaning non-standard features
+ * moved `$for` to [separate library](https://github.com/zloirock/forof). This work for syntax - `for-of` loop and comprehensions
+ * moved `Date#{format, formatUTC}` to [separate library](https://github.com/zloirock/dtf). Standard way for this - `ECMA-402`
+ * removed `Math` methods from `Number.prototype`. Slight sugar for simple `Math` methods calling
+ * removed `{Array#, Array, Dict}.turn`
+ * removed `core.global`
+* uses `ToNumber` instead of `ToLength` in [`Number Iterator`](https://github.com/zloirock/core-js/#number-iterator), `Array.from(2.5)` will be `[0, 1, 2]` instead of `[0, 1]`
+* fixed [#85](https://github.com/zloirock/core-js/issues/85) - invalid `Promise` unhandled rejection message in nested `setTimeout`
+* fixed [#86](https://github.com/zloirock/core-js/issues/86) - support FF extensions
+* fixed [#89](https://github.com/zloirock/core-js/issues/89) - behavior `Number` constructor in strange case
+
+##### 0.9.18 - 2015.06.17
+* removed `/` from [`RegExp.escape`](https://github.com/zloirock/core-js/#ecmascript-7) escaped characters
+
+##### 0.9.17 - 2015.06.14
+* updated [`RegExp.escape`](https://github.com/zloirock/core-js/#ecmascript-7) to the [latest proposal](https://github.com/benjamingr/RexExp.escape)
+* fixed conflict with webpack dev server + IE buggy behavior
+
+##### 0.9.16 - 2015.06.11
+* more correct order resolving thenable in [`Promise`](https://github.com/zloirock/core-js/#ecmascript-6-promise) polyfill
+* uses polyfill instead of [buggy V8 `Promise`](https://github.com/zloirock/core-js/issues/78)
+
+##### 0.9.15 - 2015.06.09
+* [collections](https://github.com/zloirock/core-js/#ecmascript-6-collections) from `library` version return wrapped native instances
+* fixed collections prototype methods in `library` version
+* optimized [`Math.hypot`](https://github.com/zloirock/core-js/#ecmascript-6-math)
+
+##### 0.9.14 - 2015.06.04
+* updated [`Promise.resolve` behavior](https://esdiscuss.org/topic/fixing-promise-resolve)
+* added fallback for IE11 buggy `Object.getOwnPropertyNames` + iframe
+* some other fixes
+
+##### 0.9.13 - 2015.05.25
+* added fallback for [`Symbol` polyfill](https://github.com/zloirock/core-js/#ecmascript-6-symbol) for old Android
+* some other fixes
+
+##### 0.9.12 - 2015.05.24
+* different instances `core-js` should use / recognize the same symbols
+* some fixes
+
+##### 0.9.11 - 2015.05.18
+* simplified [custom build](https://github.com/zloirock/core-js/#custom-build)
+ * add custom build js api
+ * added `grunt-cli` to `devDependencies` for `npm run grunt`
+* some fixes
+
+##### 0.9.10 - 2015.05.16
+* wrapped `Function#toString` for correct work wrapped methods / constructors with methods similar to the [`lodash` `isNative`](https://github.com/lodash/lodash/issues/1197)
+* added proto versions of methods to export object in `default` version for consistency with `library` version
+
+##### 0.9.9 - 2015.05.14
+* wrapped `Object#propertyIsEnumerable` for [`Symbol` polyfill](https://github.com/zloirock/core-js/#ecmascript-6-symbol)
+* [added proto versions of methods to `library` for ES7 bind syntax](https://github.com/zloirock/core-js/issues/65)
+* some other fixes
+
+##### 0.9.8 - 2015.05.12
+* fixed [`Math.hypot`](https://github.com/zloirock/core-js/#ecmascript-6-math) with negative arguments
+* added `Object#toString.toString` as fallback for [`lodash` `isNative`](https://github.com/lodash/lodash/issues/1197)
+
+##### 0.9.7 - 2015.05.07
+* added [support DOM collections](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/slice#Streamlining_cross-browser_behavior) to IE8- `Array#slice`
+
+##### 0.9.6 - 2015.05.01
+* added [`String#lpad`, `String#rpad`](https://github.com/zloirock/core-js/#ecmascript-7)
+
+##### 0.9.5 - 2015.04.30
+* added cap for `Function#@@hasInstance`
+* some fixes and optimizations
+
+##### 0.9.4 - 2015.04.27
+* fixed `RegExp` constructor
+
+##### 0.9.3 - 2015.04.26
+* some fixes and optimizations
+
+##### 0.9.2 - 2015.04.25
+* more correct [`Promise`](https://github.com/zloirock/core-js/#ecmascript-6-promise) unhandled rejection tracking and resolving / rejection priority
+
+##### 0.9.1 - 2015.04.25
+* fixed `__proto__`-based [`Promise`](https://github.com/zloirock/core-js/#ecmascript-6-promise) subclassing in some environments
+
+##### 0.9.0 - 2015.04.24
+* added correct [symbols](https://github.com/zloirock/core-js/#ecmascript-6-symbol) descriptors
+ * fixed behavior `Object.{assign, create, defineProperty, defineProperties, getOwnPropertyDescriptor, getOwnPropertyDescriptors}` with symbols
+ * added [single entry points](https://github.com/zloirock/core-js/#commonjs) for `Object.{create, defineProperty, defineProperties}`
+* added [`Map#toJSON`](https://github.com/zloirock/core-js/#ecmascript-7)
+* removed non-standard methods `Object#[_]` and `Function#only` - they solves syntax problems, but now in compilers available arrows and ~~in near future will be available~~ [available](http://babeljs.io/blog/2015/05/14/function-bind/) [bind syntax](https://github.com/zenparsing/es-function-bind)
+* removed non-standard undocumented methods `Symbol.{pure, set}`
+* some fixes and internal changes
+
+##### 0.8.4 - 2015.04.18
+* uses `webpack` instead of `browserify` for browser builds - more compression-friendly result
+
+##### 0.8.3 - 2015.04.14
+* fixed `Array` statics with single entry points
+
+##### 0.8.2 - 2015.04.13
+* [`Math.fround`](https://github.com/zloirock/core-js/#ecmascript-6-math) now also works in IE9-
+* added [`Set#toJSON`](https://github.com/zloirock/core-js/#ecmascript-7)
+* some optimizations and fixes
+
+##### 0.8.1 - 2015.04.03
+* fixed `Symbol.keyFor`
+
+##### 0.8.0 - 2015.04.02
+* changed [CommonJS API](https://github.com/zloirock/core-js/#commonjs)
+* splitted and renamed some modules
+* added support ES3 environment (ES5 polyfill) to **all** default versions - size increases slightly (+ ~4kb w/o gzip), many issues disappear, if you don't need it - [simply include only required namespaces / features / modules](https://github.com/zloirock/core-js/#commonjs)
+* removed [abstract references](https://github.com/zenparsing/es-abstract-refs) support - proposal has been superseded =\
+* [`$for.isIterable` -> `core.isIterable`, `$for.getIterator` -> `core.getIterator`](https://github.com/zloirock/core-js/#ecmascript-6-iterators), temporary available in old namespace
+* fixed iterators support in v8 `Promise.all` and `Promise.race`
+* many other fixes
+
+##### 0.7.2 - 2015.03.09
+* some fixes
+
+##### 0.7.1 - 2015.03.07
+* some fixes
+
+##### 0.7.0 - 2015.03.06
+* rewritten and splitted into [CommonJS modules](https://github.com/zloirock/core-js/#commonjs)
+
+##### 0.6.1 - 2015.02.24
+* fixed support [`Object.defineProperty`](https://github.com/zloirock/core-js/#ecmascript-5) with accessors on DOM elements on IE8
+
+##### 0.6.0 - 2015.02.23
+* added support safe closing iteration - calling `iterator.return` on abort iteration, if it exists
+* added basic support [`Promise`](https://github.com/zloirock/core-js/#ecmascript-6-promise) unhandled rejection tracking in shim
+* added [`Object.getOwnPropertyDescriptors`](https://github.com/zloirock/core-js/#ecmascript-7)
+* removed `console` cap - creates too many problems - you can use [`core.log`](https://github.com/zloirock/core-js/#console) module as that
+* restructuring [namespaces](https://github.com/zloirock/core-js/#custom-build)
+* some fixes
+
+##### 0.5.4 - 2015.02.15
+* some fixes
+
+##### 0.5.3 - 2015.02.14
+* added [support binary and octal literals](https://github.com/zloirock/core-js/#ecmascript-6-number) to `Number` constructor
+* added [`Date#toISOString`](https://github.com/zloirock/core-js/#ecmascript-5)
+
+##### 0.5.2 - 2015.02.10
+* some fixes
+
+##### 0.5.1 - 2015.02.09
+* some fixes
+
+##### 0.5.0 - 2015.02.08
+* systematization of modules
+* splitted [`es6` module](https://github.com/zloirock/core-js/#ecmascript-6)
+* splitted [`console` module](https://github.com/zloirock/core-js/#console): `web.console` - only cap for missing methods, `core.log` - bound methods & additional features
+* added [`delay` method](https://github.com/zloirock/core-js/#delay)
+* some fixes
+
+##### 0.4.10 - 2015.01.28
+* [`Object.getOwnPropertySymbols`](https://github.com/zloirock/core-js/#ecmascript-6-symbol) polyfill returns array of wrapped keys
+
+##### 0.4.9 - 2015.01.27
+* FF20-24 fix
+
+##### 0.4.8 - 2015.01.25
+* some [collections](https://github.com/zloirock/core-js/#ecmascript-6-collections) fixes
+
+##### 0.4.7 - 2015.01.25
+* added support frozen objects as [collections](https://github.com/zloirock/core-js/#ecmascript-6-collections) keys
+
+##### 0.4.6 - 2015.01.21
+* added [`Object.getOwnPropertySymbols`](https://github.com/zloirock/core-js/#ecmascript-6-symbol)
+* added [`NodeList.prototype[@@iterator]`](https://github.com/zloirock/core-js/#ecmascript-6-iterators)
+* added basic `@@species` logic - getter in native constructors
+* removed `Function#by`
+* some fixes
+
+##### 0.4.5 - 2015.01.16
+* some fixes
+
+##### 0.4.4 - 2015.01.11
+* enabled CSP support
+
+##### 0.4.3 - 2015.01.10
+* added `Function` instances `name` property for IE9+
+
+##### 0.4.2 - 2015.01.10
+* `Object` static methods accept primitives
+* `RegExp` constructor can alter flags (IE9+)
+* added `Array.prototype[Symbol.unscopables]`
+
+##### 0.4.1 - 2015.01.05
+* some fixes
+
+##### 0.4.0 - 2015.01.03
+* added [`es6.reflect`](https://github.com/zloirock/core-js/#ecmascript-6-reflect) module:
+ * added `Reflect.apply`
+ * added `Reflect.construct`
+ * added `Reflect.defineProperty`
+ * added `Reflect.deleteProperty`
+ * added `Reflect.enumerate`
+ * added `Reflect.get`
+ * added `Reflect.getOwnPropertyDescriptor`
+ * added `Reflect.getPrototypeOf`
+ * added `Reflect.has`
+ * added `Reflect.isExtensible`
+ * added `Reflect.preventExtensions`
+ * added `Reflect.set`
+ * added `Reflect.setPrototypeOf`
+* `core-js` methods now can use external `Symbol.iterator` polyfill
+* some fixes
+
+##### 0.3.3 - 2014.12.28
+* [console cap](https://github.com/zloirock/core-js/#console) excluded from node.js default builds
+
+##### 0.3.2 - 2014.12.25
+* added cap for [ES5](https://github.com/zloirock/core-js/#ecmascript-5) freeze-family methods
+* fixed `console` bug
+
+##### 0.3.1 - 2014.12.23
+* some fixes
+
+##### 0.3.0 - 2014.12.23
+* Optimize [`Map` & `Set`](https://github.com/zloirock/core-js/#ecmascript-6-collections):
+ * use entries chain on hash table
+ * fast & correct iteration
+ * iterators moved to [`es6`](https://github.com/zloirock/core-js/#ecmascript-6) and [`es6.collections`](https://github.com/zloirock/core-js/#ecmascript-6-collections) modules
+
+##### 0.2.5 - 2014.12.20
+* `console` no longer shortcut for `console.log` (compatibility problems)
+* some fixes
+
+##### 0.2.4 - 2014.12.17
+* better compliance of ES6
+* added [`Math.fround`](https://github.com/zloirock/core-js/#ecmascript-6-math) (IE10+)
+* some fixes
+
+##### 0.2.3 - 2014.12.15
+* [Symbols](https://github.com/zloirock/core-js/#ecmascript-6-symbol):
+ * added option to disable addition setter to `Object.prototype` for Symbol polyfill:
+ * added `Symbol.useSimple`
+ * added `Symbol.useSetter`
+ * added cap for well-known Symbols:
+ * added `Symbol.hasInstance`
+ * added `Symbol.isConcatSpreadable`
+ * added `Symbol.match`
+ * added `Symbol.replace`
+ * added `Symbol.search`
+ * added `Symbol.species`
+ * added `Symbol.split`
+ * added `Symbol.toPrimitive`
+ * added `Symbol.unscopables`
+
+##### 0.2.2 - 2014.12.13
+* added [`RegExp#flags`](https://github.com/zloirock/core-js/#ecmascript-6-regexp) ([December 2014 Draft Rev 29](http://wiki.ecmascript.org/doku.php?id=harmony:specification_drafts#december_6_2014_draft_rev_29))
+* added [`String.raw`](https://github.com/zloirock/core-js/#ecmascript-6-string)
+
+##### 0.2.1 - 2014.12.12
+* repair converting -0 to +0 in [native collections](https://github.com/zloirock/core-js/#ecmascript-6-collections)
+
+##### 0.2.0 - 2014.12.06
+* added [`es7.proposals`](https://github.com/zloirock/core-js/#ecmascript-7) and [`es7.abstract-refs`](https://github.com/zenparsing/es-abstract-refs) modules
+* added [`String#at`](https://github.com/zloirock/core-js/#ecmascript-7)
+* added real [`String Iterator`](https://github.com/zloirock/core-js/#ecmascript-6-iterators), older versions used Array Iterator
+* added abstract references support:
+ * added `Symbol.referenceGet`
+ * added `Symbol.referenceSet`
+ * added `Symbol.referenceDelete`
+ * added `Function#@@referenceGet`
+ * added `Map#@@referenceGet`
+ * added `Map#@@referenceSet`
+ * added `Map#@@referenceDelete`
+ * added `WeakMap#@@referenceGet`
+ * added `WeakMap#@@referenceSet`
+ * added `WeakMap#@@referenceDelete`
+ * added `Dict.{...methods}[@@referenceGet]`
+* removed deprecated `.contains` methods
+* some fixes
+
+##### 0.1.5 - 2014.12.01
+* added [`Array#copyWithin`](https://github.com/zloirock/core-js/#ecmascript-6-array)
+* added [`String#codePointAt`](https://github.com/zloirock/core-js/#ecmascript-6-string)
+* added [`String.fromCodePoint`](https://github.com/zloirock/core-js/#ecmascript-6-string)
+
+##### 0.1.4 - 2014.11.27
+* added [`Dict.mapPairs`](https://github.com/zloirock/core-js/#dict)
+
+##### 0.1.3 - 2014.11.20
+* [TC39 November meeting](https://github.com/rwaldron/tc39-notes/tree/master/es6/2014-11):
+ * [`.contains` -> `.includes`](https://github.com/rwaldron/tc39-notes/blob/master/es6/2014-11/nov-18.md#51--44-arrayprototypecontains-and-stringprototypecontains)
+ * `String#contains` -> [`String#includes`](https://github.com/zloirock/core-js/#ecmascript-6-string)
+ * `Array#contains` -> [`Array#includes`](https://github.com/zloirock/core-js/#ecmascript-7)
+ * `Dict.contains` -> [`Dict.includes`](https://github.com/zloirock/core-js/#dict)
+ * [removed `WeakMap#clear`](https://github.com/rwaldron/tc39-notes/blob/master/es6/2014-11/nov-19.md#412-should-weakmapweakset-have-a-clear-method-markm)
+ * [removed `WeakSet#clear`](https://github.com/rwaldron/tc39-notes/blob/master/es6/2014-11/nov-19.md#412-should-weakmapweakset-have-a-clear-method-markm)
+
+##### 0.1.2 - 2014.11.19
+* `Map` & `Set` bug fix
+
+##### 0.1.1 - 2014.11.18
+* public release
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/Gruntfile.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/Gruntfile.js
new file mode 100644
index 00000000..afbcd948
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/Gruntfile.js
@@ -0,0 +1,2 @@
+require('LiveScript');
+module.exports = require('./build/Gruntfile');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/LICENSE b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/LICENSE
new file mode 100644
index 00000000..669bcc98
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/LICENSE
@@ -0,0 +1,19 @@
+Copyright (c) 2015 Denis Pushkarev
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in
+all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
+THE SOFTWARE.
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/bower.json b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/bower.json
new file mode 100644
index 00000000..05688197
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/bower.json
@@ -0,0 +1,34 @@
+{
+ "name": "core.js",
+ "main": "client/core.js",
+ "version": "1.2.7",
+ "description": "Standard Library",
+ "keywords": [
+ "ES6",
+ "ECMAScript 6",
+ "ES7",
+ "ECMAScript 7",
+ "Map",
+ "Set",
+ "WeakMap",
+ "WeakSet",
+ "Dict",
+ "Promise",
+ "Symbol",
+ "console"
+ ],
+ "authors": [
+ "Denis Pushkarev (http://zloirock.ru/)"
+ ],
+ "license": "MIT",
+ "homepage": "https://github.com/zloirock/core-js",
+ "repository": {
+ "type": "git",
+ "url": "https://github.com/zloirock/core-js.git"
+ },
+ "ignore": [
+ "build",
+ "node_modules",
+ "tests"
+ ]
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/build/Gruntfile.ls b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/build/Gruntfile.ls
new file mode 100644
index 00000000..61518424
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/build/Gruntfile.ls
@@ -0,0 +1,84 @@
+require! <[./build fs ./config]>
+library-tests = <[client/library.js tests/helpers.js tests/library.js]>map -> src: it
+module.exports = (grunt)->
+ grunt.loadNpmTasks \grunt-contrib-clean
+ grunt.loadNpmTasks \grunt-contrib-copy
+ grunt.loadNpmTasks \grunt-contrib-uglify
+ grunt.loadNpmTasks \grunt-contrib-watch
+ grunt.loadNpmTasks \grunt-livescript
+ grunt.loadNpmTasks \grunt-karma
+ grunt.initConfig do
+ pkg: grunt.file.readJSON './package.json'
+ uglify: build:
+ files: '<%=grunt.option("path")%>.min.js': '<%=grunt.option("path")%>.js'
+ options:
+ mangle: {+sort, +keep_fnames}
+ compress: {+pure_getters, +keep_fargs, +keep_fnames}
+ sourceMap: on
+ banner: config.banner
+ livescript: src: files:
+ './tests/helpers.js': './tests/helpers/*'
+ './tests/tests.js': './tests/tests/*'
+ './tests/library.js': './tests/library/*'
+ './tests/es.js': './tests/tests/es*'
+ './tests/experimental.js': './tests/experimental/*'
+ './build/index.js': './build/build.ls*'
+ clean: <[./library]>
+ copy: lib: files:
+ * expand: on
+ cwd: './'
+ src: <[es5/** es6/** es7/** js/** web/** core/** fn/** index.js shim.js]>
+ dest: './library/'
+ * expand: on
+ cwd: './'
+ src: <[modules/*]>
+ dest: './library/'
+ filter: \isFile
+ * expand: on
+ cwd: './modules/library/'
+ src: '*'
+ dest: './library/modules/'
+ watch:
+ core:
+ files: './modules/*'
+ tasks: \default
+ tests:
+ files: './tests/tests/*'
+ tasks: \livescript
+ karma:
+ 'options':
+ configFile: './tests/karma.conf.js'
+ browsers: <[PhantomJS]>
+ singleRun: on
+ 'continuous': {}
+ 'continuous-library':
+ files: library-tests
+ grunt.registerTask \build (options)->
+ done = @async!
+ err, it <- build {
+ modules: (options || 'es5,es6,es7,js,web,core')split \,
+ blacklist: (grunt.option(\blacklist) || '')split \,
+ library: !!grunt.option \library
+ }
+ if err
+ console.error err
+ process.exit 1
+ grunt.option(\path) || grunt.option(\path, './custom')
+ fs.writeFile grunt.option(\path) + '.js', it, done
+ grunt.registerTask \client ->
+ grunt.option \library ''
+ grunt.option \path './client/core'
+ grunt.task.run <[build:es5,es6,es7,js,web,core uglify]>
+ grunt.registerTask \library ->
+ grunt.option \library 'true'
+ grunt.option \path './client/library'
+ grunt.task.run <[build:es5,es6,es7,js,web,core uglify]>
+ grunt.registerTask \shim ->
+ grunt.option \library ''
+ grunt.option \path './client/shim'
+ grunt.task.run <[build:es5,es6,es7,js,web uglify]>
+ grunt.registerTask \e ->
+ grunt.option \library ''>
+ grunt.option \path './client/core'
+ grunt.task.run <[build:es5,es6,es7,js,web,core,exp uglify]>
+ grunt.registerTask \default <[clean copy client library shim]>
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/build/build.ls b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/build/build.ls
new file mode 100644
index 00000000..274ffc42
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/build/build.ls
@@ -0,0 +1,218 @@
+require! {'./config': {banner}, fs: {readFile, writeFile, unlink}, path, webpack}
+
+list = <[
+ es5
+ es6.symbol
+ es6.object.assign
+ es6.object.is
+ es6.object.set-prototype-of
+ es6.object.to-string
+ es6.object.freeze
+ es6.object.seal
+ es6.object.prevent-extensions
+ es6.object.is-frozen
+ es6.object.is-sealed
+ es6.object.is-extensible
+ es6.object.get-own-property-descriptor
+ es6.object.get-prototype-of
+ es6.object.keys
+ es6.object.get-own-property-names
+ es6.function.name
+ es6.function.has-instance
+ es6.number.constructor
+ es6.number.epsilon
+ es6.number.is-finite
+ es6.number.is-integer
+ es6.number.is-nan
+ es6.number.is-safe-integer
+ es6.number.max-safe-integer
+ es6.number.min-safe-integer
+ es6.number.parse-float
+ es6.number.parse-int
+ es6.math.acosh
+ es6.math.asinh
+ es6.math.atanh
+ es6.math.cbrt
+ es6.math.clz32
+ es6.math.cosh
+ es6.math.expm1
+ es6.math.fround
+ es6.math.hypot
+ es6.math.imul
+ es6.math.log10
+ es6.math.log1p
+ es6.math.log2
+ es6.math.sign
+ es6.math.sinh
+ es6.math.tanh
+ es6.math.trunc
+ es6.string.from-code-point
+ es6.string.raw
+ es6.string.trim
+ es6.string.code-point-at
+ es6.string.ends-with
+ es6.string.includes
+ es6.string.repeat
+ es6.string.starts-with
+ es6.string.iterator
+ es6.array.from
+ es6.array.of
+ es6.array.iterator
+ es6.array.species
+ es6.array.copy-within
+ es6.array.fill
+ es6.array.find
+ es6.array.find-index
+ es6.regexp.constructor
+ es6.regexp.flags
+ es6.regexp.match
+ es6.regexp.replace
+ es6.regexp.search
+ es6.regexp.split
+ es6.promise
+ es6.map
+ es6.set
+ es6.weak-map
+ es6.weak-set
+ es6.reflect.apply
+ es6.reflect.construct
+ es6.reflect.define-property
+ es6.reflect.delete-property
+ es6.reflect.enumerate
+ es6.reflect.get
+ es6.reflect.get-own-property-descriptor
+ es6.reflect.get-prototype-of
+ es6.reflect.has
+ es6.reflect.is-extensible
+ es6.reflect.own-keys
+ es6.reflect.prevent-extensions
+ es6.reflect.set
+ es6.reflect.set-prototype-of
+ es6.date.to-string
+ es6.typed.array-buffer
+ es6.typed.data-view
+ es6.typed.int8-array
+ es6.typed.uint8-array
+ es6.typed.uint8-clamped-array
+ es6.typed.int16-array
+ es6.typed.uint16-array
+ es6.typed.int32-array
+ es6.typed.uint32-array
+ es6.typed.float32-array
+ es6.typed.float64-array
+ es7.array.includes
+ es7.string.at
+ es7.string.pad-left
+ es7.string.pad-right
+ es7.string.trim-left
+ es7.string.trim-right
+ es7.regexp.escape
+ es7.object.get-own-property-descriptors
+ es7.object.values
+ es7.object.entries
+ es7.map.to-json
+ es7.set.to-json
+ web.immediate
+ web.dom.iterable
+ web.timers
+ core.dict
+ core.get-iterator-method
+ core.get-iterator
+ core.is-iterable
+ core.delay
+ core.function.part
+ core.object.is-object
+ core.object.classof
+ core.object.define
+ core.object.make
+ core.number.iterator
+ core.string.escape-html
+ core.string.unescape-html
+ core.log
+ js.array.statics
+]>
+
+experimental = <[
+ es6.date.to-string
+ es6.typed.array-buffer
+ es6.typed.data-view
+ es6.typed.int8-array
+ es6.typed.uint8-array
+ es6.typed.uint8-clamped-array
+ es6.typed.int16-array
+ es6.typed.uint16-array
+ es6.typed.int32-array
+ es6.typed.uint32-array
+ es6.typed.float32-array
+ es6.typed.float64-array
+]>
+
+libraryBlacklist = <[
+ es6.object.to-string
+ es6.function.name
+ es6.regexp.constructor
+ es6.regexp.flags
+ es6.regexp.match
+ es6.regexp.replace
+ es6.regexp.search
+ es6.regexp.split
+ es6.number.constructor
+]>
+
+es5SpecialCase = <[
+ es6.object.freeze
+ es6.object.seal
+ es6.object.prevent-extensions
+ es6.object.is-frozen
+ es6.object.is-sealed
+ es6.object.is-extensible
+ es6.string.trim
+]>
+
+module.exports = ({modules = [], blacklist = [], library = no}, next)!->
+ let @ = modules.reduce ((memo, it)-> memo[it] = on; memo), {}
+ check = (err)->
+ if err
+ next err, ''
+ on
+
+ if @exp => for experimental => @[..] = on
+ if @es5 => for es5SpecialCase => @[..] = on
+ for ns of @
+ if @[ns]
+ for name in list
+ if name.indexOf("#ns.") is 0 and name not in experimental
+ @[name] = on
+
+ if library => blacklist ++= libraryBlacklist
+ for ns in blacklist
+ for name in list
+ if name is ns or name.indexOf("#ns.") is 0
+ @[name] = no
+
+ TARGET = "./__tmp#{ Math.random! }__.js"
+ err, info <~! webpack do
+ entry: list.filter(~> @[it]).map ~>
+ path.join(__dirname, '../', "#{ if library => '/library' else '' }/modules/#it")
+ output:
+ path: ''
+ filename: TARGET
+ if check err => return
+ err, script <~! readFile TARGET
+ if check err => return
+ err <~! unlink TARGET
+ if check err => return
+
+ next null """
+ #banner
+ !function(__e, __g, undefined){
+ 'use strict';
+ #script
+ // CommonJS export
+ if(typeof module != 'undefined' && module.exports)module.exports = __e;
+ // RequireJS export
+ else if(typeof define == 'function' && define.amd)define(function(){return __e});
+ // Export to global object
+ else __g.core = __e;
+ }(1, 1);
+ """
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/build/config.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/build/config.js
new file mode 100644
index 00000000..8df3dc6e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/build/config.js
@@ -0,0 +1,8 @@
+module.exports = {
+ banner: '/**\n' +
+ ' * core-js ' + require('../package').version + '\n' +
+ ' * https://github.com/zloirock/core-js\n' +
+ ' * License: http://rock.mit-license.org\n' +
+ ' * © ' + new Date().getFullYear() + ' Denis Pushkarev\n' +
+ ' */'
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/build/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/build/index.js
new file mode 100644
index 00000000..d9cf11f3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/build/index.js
@@ -0,0 +1,98 @@
+// Generated by LiveScript 1.3.1
+(function(){
+ var banner, ref$, readFile, writeFile, unlink, path, webpack, list, experimental, libraryBlacklist, es5SpecialCase;
+ banner = require('./config').banner;
+ ref$ = require('fs'), readFile = ref$.readFile, writeFile = ref$.writeFile, unlink = ref$.unlink;
+ path = require('path');
+ webpack = require('webpack');
+ list = ['es5', 'es6.symbol', 'es6.object.assign', 'es6.object.is', 'es6.object.set-prototype-of', 'es6.object.to-string', 'es6.object.freeze', 'es6.object.seal', 'es6.object.prevent-extensions', 'es6.object.is-frozen', 'es6.object.is-sealed', 'es6.object.is-extensible', 'es6.object.get-own-property-descriptor', 'es6.object.get-prototype-of', 'es6.object.keys', 'es6.object.get-own-property-names', 'es6.function.name', 'es6.function.has-instance', 'es6.number.constructor', 'es6.number.epsilon', 'es6.number.is-finite', 'es6.number.is-integer', 'es6.number.is-nan', 'es6.number.is-safe-integer', 'es6.number.max-safe-integer', 'es6.number.min-safe-integer', 'es6.number.parse-float', 'es6.number.parse-int', 'es6.math.acosh', 'es6.math.asinh', 'es6.math.atanh', 'es6.math.cbrt', 'es6.math.clz32', 'es6.math.cosh', 'es6.math.expm1', 'es6.math.fround', 'es6.math.hypot', 'es6.math.imul', 'es6.math.log10', 'es6.math.log1p', 'es6.math.log2', 'es6.math.sign', 'es6.math.sinh', 'es6.math.tanh', 'es6.math.trunc', 'es6.string.from-code-point', 'es6.string.raw', 'es6.string.trim', 'es6.string.code-point-at', 'es6.string.ends-with', 'es6.string.includes', 'es6.string.repeat', 'es6.string.starts-with', 'es6.string.iterator', 'es6.array.from', 'es6.array.of', 'es6.array.iterator', 'es6.array.species', 'es6.array.copy-within', 'es6.array.fill', 'es6.array.find', 'es6.array.find-index', 'es6.regexp.constructor', 'es6.regexp.flags', 'es6.regexp.match', 'es6.regexp.replace', 'es6.regexp.search', 'es6.regexp.split', 'es6.promise', 'es6.map', 'es6.set', 'es6.weak-map', 'es6.weak-set', 'es6.reflect.apply', 'es6.reflect.construct', 'es6.reflect.define-property', 'es6.reflect.delete-property', 'es6.reflect.enumerate', 'es6.reflect.get', 'es6.reflect.get-own-property-descriptor', 'es6.reflect.get-prototype-of', 'es6.reflect.has', 'es6.reflect.is-extensible', 'es6.reflect.own-keys', 'es6.reflect.prevent-extensions', 'es6.reflect.set', 'es6.reflect.set-prototype-of', 'es6.date.to-string', 'es6.typed.array-buffer', 'es6.typed.data-view', 'es6.typed.int8-array', 'es6.typed.uint8-array', 'es6.typed.uint8-clamped-array', 'es6.typed.int16-array', 'es6.typed.uint16-array', 'es6.typed.int32-array', 'es6.typed.uint32-array', 'es6.typed.float32-array', 'es6.typed.float64-array', 'es7.array.includes', 'es7.string.at', 'es7.string.pad-left', 'es7.string.pad-right', 'es7.string.trim-left', 'es7.string.trim-right', 'es7.regexp.escape', 'es7.object.get-own-property-descriptors', 'es7.object.values', 'es7.object.entries', 'es7.map.to-json', 'es7.set.to-json', 'web.immediate', 'web.dom.iterable', 'web.timers', 'core.dict', 'core.get-iterator-method', 'core.get-iterator', 'core.is-iterable', 'core.delay', 'core.function.part', 'core.object.is-object', 'core.object.classof', 'core.object.define', 'core.object.make', 'core.number.iterator', 'core.string.escape-html', 'core.string.unescape-html', 'core.log', 'js.array.statics'];
+ experimental = ['es6.date.to-string', 'es6.typed.array-buffer', 'es6.typed.data-view', 'es6.typed.int8-array', 'es6.typed.uint8-array', 'es6.typed.uint8-clamped-array', 'es6.typed.int16-array', 'es6.typed.uint16-array', 'es6.typed.int32-array', 'es6.typed.uint32-array', 'es6.typed.float32-array', 'es6.typed.float64-array'];
+ libraryBlacklist = ['es6.object.to-string', 'es6.function.name', 'es6.regexp.constructor', 'es6.regexp.flags', 'es6.regexp.match', 'es6.regexp.replace', 'es6.regexp.search', 'es6.regexp.split', 'es6.number.constructor'];
+ es5SpecialCase = ['es6.object.freeze', 'es6.object.seal', 'es6.object.prevent-extensions', 'es6.object.is-frozen', 'es6.object.is-sealed', 'es6.object.is-extensible', 'es6.string.trim'];
+ module.exports = function(arg$, next){
+ var modules, ref$, blacklist, library;
+ modules = (ref$ = arg$.modules) != null
+ ? ref$
+ : [], blacklist = (ref$ = arg$.blacklist) != null
+ ? ref$
+ : [], library = (ref$ = arg$.library) != null ? ref$ : false;
+ (function(){
+ var check, i$, x$, ref$, len$, y$, ns, name, j$, len1$, TARGET, this$ = this;
+ check = function(err){
+ if (err) {
+ next(err, '');
+ return true;
+ }
+ };
+ if (this.exp) {
+ for (i$ = 0, len$ = (ref$ = experimental).length; i$ < len$; ++i$) {
+ x$ = ref$[i$];
+ this[x$] = true;
+ }
+ }
+ if (this.es5) {
+ for (i$ = 0, len$ = (ref$ = es5SpecialCase).length; i$ < len$; ++i$) {
+ y$ = ref$[i$];
+ this[y$] = true;
+ }
+ }
+ for (ns in this) {
+ if (this[ns]) {
+ for (i$ = 0, len$ = (ref$ = list).length; i$ < len$; ++i$) {
+ name = ref$[i$];
+ if (name.indexOf(ns + ".") === 0 && !in$(name, experimental)) {
+ this[name] = true;
+ }
+ }
+ }
+ }
+ if (library) {
+ blacklist = blacklist.concat(libraryBlacklist);
+ }
+ for (i$ = 0, len$ = blacklist.length; i$ < len$; ++i$) {
+ ns = blacklist[i$];
+ for (j$ = 0, len1$ = (ref$ = list).length; j$ < len1$; ++j$) {
+ name = ref$[j$];
+ if (name === ns || name.indexOf(ns + ".") === 0) {
+ this[name] = false;
+ }
+ }
+ }
+ TARGET = "./__tmp" + Math.random() + "__.js";
+ webpack({
+ entry: list.filter(function(it){
+ return this$[it];
+ }).map(function(it){
+ return path.join(__dirname, '../', (library ? '/library' : '') + "/modules/" + it);
+ }),
+ output: {
+ path: '',
+ filename: TARGET
+ }
+ }, function(err, info){
+ if (check(err)) {
+ return;
+ }
+ readFile(TARGET, function(err, script){
+ if (check(err)) {
+ return;
+ }
+ unlink(TARGET, function(err){
+ if (check(err)) {
+ return;
+ }
+ next(null, "" + banner + "\n!function(__e, __g, undefined){\n'use strict';\n" + script + "\n// CommonJS export\nif(typeof module != 'undefined' && module.exports)module.exports = __e;\n// RequireJS export\nelse if(typeof define == 'function' && define.amd)define(function(){return __e});\n// Export to global object\nelse __g.core = __e;\n}(1, 1);");
+ });
+ });
+ });
+ }.call(modules.reduce(function(memo, it){
+ memo[it] = true;
+ return memo;
+ }, {})));
+ };
+ function in$(x, xs){
+ var i = -1, l = xs.length >>> 0;
+ while (++i < l) if (x === xs[i]) return true;
+ return false;
+ }
+}).call(this);
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/core.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/core.js
new file mode 100644
index 00000000..b9bac6c5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/core.js
@@ -0,0 +1,4910 @@
+/**
+ * core-js 1.2.7
+ * https://github.com/zloirock/core-js
+ * License: http://rock.mit-license.org
+ * © 2016 Denis Pushkarev
+ */
+!function(__e, __g, undefined){
+'use strict';
+/******/ (function(modules) { // webpackBootstrap
+/******/ // The module cache
+/******/ var installedModules = {};
+
+/******/ // The require function
+/******/ function __webpack_require__(moduleId) {
+
+/******/ // Check if module is in cache
+/******/ if(installedModules[moduleId])
+/******/ return installedModules[moduleId].exports;
+
+/******/ // Create a new module (and put it into the cache)
+/******/ var module = installedModules[moduleId] = {
+/******/ exports: {},
+/******/ id: moduleId,
+/******/ loaded: false
+/******/ };
+
+/******/ // Execute the module function
+/******/ modules[moduleId].call(module.exports, module, module.exports, __webpack_require__);
+
+/******/ // Flag the module as loaded
+/******/ module.loaded = true;
+
+/******/ // Return the exports of the module
+/******/ return module.exports;
+/******/ }
+
+
+/******/ // expose the modules object (__webpack_modules__)
+/******/ __webpack_require__.m = modules;
+
+/******/ // expose the module cache
+/******/ __webpack_require__.c = installedModules;
+
+/******/ // __webpack_public_path__
+/******/ __webpack_require__.p = "";
+
+/******/ // Load entry module and return exports
+/******/ return __webpack_require__(0);
+/******/ })
+/************************************************************************/
+/******/ ([
+/* 0 */
+/***/ function(module, exports, __webpack_require__) {
+
+ __webpack_require__(1);
+ __webpack_require__(34);
+ __webpack_require__(40);
+ __webpack_require__(42);
+ __webpack_require__(44);
+ __webpack_require__(46);
+ __webpack_require__(48);
+ __webpack_require__(50);
+ __webpack_require__(51);
+ __webpack_require__(52);
+ __webpack_require__(53);
+ __webpack_require__(54);
+ __webpack_require__(55);
+ __webpack_require__(56);
+ __webpack_require__(57);
+ __webpack_require__(58);
+ __webpack_require__(59);
+ __webpack_require__(60);
+ __webpack_require__(61);
+ __webpack_require__(64);
+ __webpack_require__(65);
+ __webpack_require__(66);
+ __webpack_require__(68);
+ __webpack_require__(69);
+ __webpack_require__(70);
+ __webpack_require__(71);
+ __webpack_require__(72);
+ __webpack_require__(73);
+ __webpack_require__(74);
+ __webpack_require__(76);
+ __webpack_require__(77);
+ __webpack_require__(78);
+ __webpack_require__(80);
+ __webpack_require__(81);
+ __webpack_require__(82);
+ __webpack_require__(84);
+ __webpack_require__(85);
+ __webpack_require__(86);
+ __webpack_require__(87);
+ __webpack_require__(88);
+ __webpack_require__(89);
+ __webpack_require__(90);
+ __webpack_require__(91);
+ __webpack_require__(92);
+ __webpack_require__(93);
+ __webpack_require__(94);
+ __webpack_require__(95);
+ __webpack_require__(96);
+ __webpack_require__(97);
+ __webpack_require__(99);
+ __webpack_require__(103);
+ __webpack_require__(104);
+ __webpack_require__(106);
+ __webpack_require__(107);
+ __webpack_require__(111);
+ __webpack_require__(116);
+ __webpack_require__(117);
+ __webpack_require__(120);
+ __webpack_require__(122);
+ __webpack_require__(124);
+ __webpack_require__(126);
+ __webpack_require__(127);
+ __webpack_require__(128);
+ __webpack_require__(130);
+ __webpack_require__(131);
+ __webpack_require__(133);
+ __webpack_require__(134);
+ __webpack_require__(135);
+ __webpack_require__(136);
+ __webpack_require__(143);
+ __webpack_require__(146);
+ __webpack_require__(147);
+ __webpack_require__(149);
+ __webpack_require__(150);
+ __webpack_require__(151);
+ __webpack_require__(152);
+ __webpack_require__(153);
+ __webpack_require__(154);
+ __webpack_require__(155);
+ __webpack_require__(156);
+ __webpack_require__(157);
+ __webpack_require__(158);
+ __webpack_require__(159);
+ __webpack_require__(160);
+ __webpack_require__(162);
+ __webpack_require__(163);
+ __webpack_require__(164);
+ __webpack_require__(165);
+ __webpack_require__(166);
+ __webpack_require__(167);
+ __webpack_require__(169);
+ __webpack_require__(170);
+ __webpack_require__(171);
+ __webpack_require__(172);
+ __webpack_require__(174);
+ __webpack_require__(175);
+ __webpack_require__(177);
+ __webpack_require__(178);
+ __webpack_require__(180);
+ __webpack_require__(181);
+ __webpack_require__(182);
+ __webpack_require__(183);
+ __webpack_require__(186);
+ __webpack_require__(114);
+ __webpack_require__(188);
+ __webpack_require__(187);
+ __webpack_require__(189);
+ __webpack_require__(190);
+ __webpack_require__(191);
+ __webpack_require__(192);
+ __webpack_require__(193);
+ __webpack_require__(195);
+ __webpack_require__(196);
+ __webpack_require__(197);
+ __webpack_require__(198);
+ __webpack_require__(199);
+ module.exports = __webpack_require__(200);
+
+
+/***/ },
+/* 1 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , $export = __webpack_require__(3)
+ , DESCRIPTORS = __webpack_require__(8)
+ , createDesc = __webpack_require__(7)
+ , html = __webpack_require__(14)
+ , cel = __webpack_require__(15)
+ , has = __webpack_require__(17)
+ , cof = __webpack_require__(18)
+ , invoke = __webpack_require__(19)
+ , fails = __webpack_require__(9)
+ , anObject = __webpack_require__(20)
+ , aFunction = __webpack_require__(13)
+ , isObject = __webpack_require__(16)
+ , toObject = __webpack_require__(21)
+ , toIObject = __webpack_require__(23)
+ , toInteger = __webpack_require__(25)
+ , toIndex = __webpack_require__(26)
+ , toLength = __webpack_require__(27)
+ , IObject = __webpack_require__(24)
+ , IE_PROTO = __webpack_require__(11)('__proto__')
+ , createArrayMethod = __webpack_require__(28)
+ , arrayIndexOf = __webpack_require__(33)(false)
+ , ObjectProto = Object.prototype
+ , ArrayProto = Array.prototype
+ , arraySlice = ArrayProto.slice
+ , arrayJoin = ArrayProto.join
+ , defineProperty = $.setDesc
+ , getOwnDescriptor = $.getDesc
+ , defineProperties = $.setDescs
+ , factories = {}
+ , IE8_DOM_DEFINE;
+
+ if(!DESCRIPTORS){
+ IE8_DOM_DEFINE = !fails(function(){
+ return defineProperty(cel('div'), 'a', {get: function(){ return 7; }}).a != 7;
+ });
+ $.setDesc = function(O, P, Attributes){
+ if(IE8_DOM_DEFINE)try {
+ return defineProperty(O, P, Attributes);
+ } catch(e){ /* empty */ }
+ if('get' in Attributes || 'set' in Attributes)throw TypeError('Accessors not supported!');
+ if('value' in Attributes)anObject(O)[P] = Attributes.value;
+ return O;
+ };
+ $.getDesc = function(O, P){
+ if(IE8_DOM_DEFINE)try {
+ return getOwnDescriptor(O, P);
+ } catch(e){ /* empty */ }
+ if(has(O, P))return createDesc(!ObjectProto.propertyIsEnumerable.call(O, P), O[P]);
+ };
+ $.setDescs = defineProperties = function(O, Properties){
+ anObject(O);
+ var keys = $.getKeys(Properties)
+ , length = keys.length
+ , i = 0
+ , P;
+ while(length > i)$.setDesc(O, P = keys[i++], Properties[P]);
+ return O;
+ };
+ }
+ $export($export.S + $export.F * !DESCRIPTORS, 'Object', {
+ // 19.1.2.6 / 15.2.3.3 Object.getOwnPropertyDescriptor(O, P)
+ getOwnPropertyDescriptor: $.getDesc,
+ // 19.1.2.4 / 15.2.3.6 Object.defineProperty(O, P, Attributes)
+ defineProperty: $.setDesc,
+ // 19.1.2.3 / 15.2.3.7 Object.defineProperties(O, Properties)
+ defineProperties: defineProperties
+ });
+
+ // IE 8- don't enum bug keys
+ var keys1 = ('constructor,hasOwnProperty,isPrototypeOf,propertyIsEnumerable,' +
+ 'toLocaleString,toString,valueOf').split(',')
+ // Additional keys for getOwnPropertyNames
+ , keys2 = keys1.concat('length', 'prototype')
+ , keysLen1 = keys1.length;
+
+ // Create object with `null` prototype: use iframe Object with cleared prototype
+ var createDict = function(){
+ // Thrash, waste and sodomy: IE GC bug
+ var iframe = cel('iframe')
+ , i = keysLen1
+ , gt = '>'
+ , iframeDocument;
+ iframe.style.display = 'none';
+ html.appendChild(iframe);
+ iframe.src = 'javascript:'; // eslint-disable-line no-script-url
+ // createDict = iframe.contentWindow.Object;
+ // html.removeChild(iframe);
+ iframeDocument = iframe.contentWindow.document;
+ iframeDocument.open();
+ iframeDocument.write(' i)if(has(O, key = names[i++])){
+ ~arrayIndexOf(result, key) || result.push(key);
+ }
+ return result;
+ };
+ };
+ var Empty = function(){};
+ $export($export.S, 'Object', {
+ // 19.1.2.9 / 15.2.3.2 Object.getPrototypeOf(O)
+ getPrototypeOf: $.getProto = $.getProto || function(O){
+ O = toObject(O);
+ if(has(O, IE_PROTO))return O[IE_PROTO];
+ if(typeof O.constructor == 'function' && O instanceof O.constructor){
+ return O.constructor.prototype;
+ } return O instanceof Object ? ObjectProto : null;
+ },
+ // 19.1.2.7 / 15.2.3.4 Object.getOwnPropertyNames(O)
+ getOwnPropertyNames: $.getNames = $.getNames || createGetKeys(keys2, keys2.length, true),
+ // 19.1.2.2 / 15.2.3.5 Object.create(O [, Properties])
+ create: $.create = $.create || function(O, /*?*/Properties){
+ var result;
+ if(O !== null){
+ Empty.prototype = anObject(O);
+ result = new Empty();
+ Empty.prototype = null;
+ // add "__proto__" for Object.getPrototypeOf shim
+ result[IE_PROTO] = O;
+ } else result = createDict();
+ return Properties === undefined ? result : defineProperties(result, Properties);
+ },
+ // 19.1.2.14 / 15.2.3.14 Object.keys(O)
+ keys: $.getKeys = $.getKeys || createGetKeys(keys1, keysLen1, false)
+ });
+
+ var construct = function(F, len, args){
+ if(!(len in factories)){
+ for(var n = [], i = 0; i < len; i++)n[i] = 'a[' + i + ']';
+ factories[len] = Function('F,a', 'return new F(' + n.join(',') + ')');
+ }
+ return factories[len](F, args);
+ };
+
+ // 19.2.3.2 / 15.3.4.5 Function.prototype.bind(thisArg, args...)
+ $export($export.P, 'Function', {
+ bind: function bind(that /*, args... */){
+ var fn = aFunction(this)
+ , partArgs = arraySlice.call(arguments, 1);
+ var bound = function(/* args... */){
+ var args = partArgs.concat(arraySlice.call(arguments));
+ return this instanceof bound ? construct(fn, args.length, args) : invoke(fn, args, that);
+ };
+ if(isObject(fn.prototype))bound.prototype = fn.prototype;
+ return bound;
+ }
+ });
+
+ // fallback for not array-like ES3 strings and DOM objects
+ $export($export.P + $export.F * fails(function(){
+ if(html)arraySlice.call(html);
+ }), 'Array', {
+ slice: function(begin, end){
+ var len = toLength(this.length)
+ , klass = cof(this);
+ end = end === undefined ? len : end;
+ if(klass == 'Array')return arraySlice.call(this, begin, end);
+ var start = toIndex(begin, len)
+ , upTo = toIndex(end, len)
+ , size = toLength(upTo - start)
+ , cloned = Array(size)
+ , i = 0;
+ for(; i < size; i++)cloned[i] = klass == 'String'
+ ? this.charAt(start + i)
+ : this[start + i];
+ return cloned;
+ }
+ });
+ $export($export.P + $export.F * (IObject != Object), 'Array', {
+ join: function join(separator){
+ return arrayJoin.call(IObject(this), separator === undefined ? ',' : separator);
+ }
+ });
+
+ // 22.1.2.2 / 15.4.3.2 Array.isArray(arg)
+ $export($export.S, 'Array', {isArray: __webpack_require__(30)});
+
+ var createArrayReduce = function(isRight){
+ return function(callbackfn, memo){
+ aFunction(callbackfn);
+ var O = IObject(this)
+ , length = toLength(O.length)
+ , index = isRight ? length - 1 : 0
+ , i = isRight ? -1 : 1;
+ if(arguments.length < 2)for(;;){
+ if(index in O){
+ memo = O[index];
+ index += i;
+ break;
+ }
+ index += i;
+ if(isRight ? index < 0 : length <= index){
+ throw TypeError('Reduce of empty array with no initial value');
+ }
+ }
+ for(;isRight ? index >= 0 : length > index; index += i)if(index in O){
+ memo = callbackfn(memo, O[index], index, this);
+ }
+ return memo;
+ };
+ };
+
+ var methodize = function($fn){
+ return function(arg1/*, arg2 = undefined */){
+ return $fn(this, arg1, arguments[1]);
+ };
+ };
+
+ $export($export.P, 'Array', {
+ // 22.1.3.10 / 15.4.4.18 Array.prototype.forEach(callbackfn [, thisArg])
+ forEach: $.each = $.each || methodize(createArrayMethod(0)),
+ // 22.1.3.15 / 15.4.4.19 Array.prototype.map(callbackfn [, thisArg])
+ map: methodize(createArrayMethod(1)),
+ // 22.1.3.7 / 15.4.4.20 Array.prototype.filter(callbackfn [, thisArg])
+ filter: methodize(createArrayMethod(2)),
+ // 22.1.3.23 / 15.4.4.17 Array.prototype.some(callbackfn [, thisArg])
+ some: methodize(createArrayMethod(3)),
+ // 22.1.3.5 / 15.4.4.16 Array.prototype.every(callbackfn [, thisArg])
+ every: methodize(createArrayMethod(4)),
+ // 22.1.3.18 / 15.4.4.21 Array.prototype.reduce(callbackfn [, initialValue])
+ reduce: createArrayReduce(false),
+ // 22.1.3.19 / 15.4.4.22 Array.prototype.reduceRight(callbackfn [, initialValue])
+ reduceRight: createArrayReduce(true),
+ // 22.1.3.11 / 15.4.4.14 Array.prototype.indexOf(searchElement [, fromIndex])
+ indexOf: methodize(arrayIndexOf),
+ // 22.1.3.14 / 15.4.4.15 Array.prototype.lastIndexOf(searchElement [, fromIndex])
+ lastIndexOf: function(el, fromIndex /* = @[*-1] */){
+ var O = toIObject(this)
+ , length = toLength(O.length)
+ , index = length - 1;
+ if(arguments.length > 1)index = Math.min(index, toInteger(fromIndex));
+ if(index < 0)index = toLength(length + index);
+ for(;index >= 0; index--)if(index in O)if(O[index] === el)return index;
+ return -1;
+ }
+ });
+
+ // 20.3.3.1 / 15.9.4.4 Date.now()
+ $export($export.S, 'Date', {now: function(){ return +new Date; }});
+
+ var lz = function(num){
+ return num > 9 ? num : '0' + num;
+ };
+
+ // 20.3.4.36 / 15.9.5.43 Date.prototype.toISOString()
+ // PhantomJS / old WebKit has a broken implementations
+ $export($export.P + $export.F * (fails(function(){
+ return new Date(-5e13 - 1).toISOString() != '0385-07-25T07:06:39.999Z';
+ }) || !fails(function(){
+ new Date(NaN).toISOString();
+ })), 'Date', {
+ toISOString: function toISOString(){
+ if(!isFinite(this))throw RangeError('Invalid time value');
+ var d = this
+ , y = d.getUTCFullYear()
+ , m = d.getUTCMilliseconds()
+ , s = y < 0 ? '-' : y > 9999 ? '+' : '';
+ return s + ('00000' + Math.abs(y)).slice(s ? -6 : -4) +
+ '-' + lz(d.getUTCMonth() + 1) + '-' + lz(d.getUTCDate()) +
+ 'T' + lz(d.getUTCHours()) + ':' + lz(d.getUTCMinutes()) +
+ ':' + lz(d.getUTCSeconds()) + '.' + (m > 99 ? m : '0' + lz(m)) + 'Z';
+ }
+ });
+
+/***/ },
+/* 2 */
+/***/ function(module, exports) {
+
+ var $Object = Object;
+ module.exports = {
+ create: $Object.create,
+ getProto: $Object.getPrototypeOf,
+ isEnum: {}.propertyIsEnumerable,
+ getDesc: $Object.getOwnPropertyDescriptor,
+ setDesc: $Object.defineProperty,
+ setDescs: $Object.defineProperties,
+ getKeys: $Object.keys,
+ getNames: $Object.getOwnPropertyNames,
+ getSymbols: $Object.getOwnPropertySymbols,
+ each: [].forEach
+ };
+
+/***/ },
+/* 3 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var global = __webpack_require__(4)
+ , core = __webpack_require__(5)
+ , hide = __webpack_require__(6)
+ , redefine = __webpack_require__(10)
+ , ctx = __webpack_require__(12)
+ , PROTOTYPE = 'prototype';
+
+ var $export = function(type, name, source){
+ var IS_FORCED = type & $export.F
+ , IS_GLOBAL = type & $export.G
+ , IS_STATIC = type & $export.S
+ , IS_PROTO = type & $export.P
+ , IS_BIND = type & $export.B
+ , target = IS_GLOBAL ? global : IS_STATIC ? global[name] || (global[name] = {}) : (global[name] || {})[PROTOTYPE]
+ , exports = IS_GLOBAL ? core : core[name] || (core[name] = {})
+ , expProto = exports[PROTOTYPE] || (exports[PROTOTYPE] = {})
+ , key, own, out, exp;
+ if(IS_GLOBAL)source = name;
+ for(key in source){
+ // contains in native
+ own = !IS_FORCED && target && key in target;
+ // export native or passed
+ out = (own ? target : source)[key];
+ // bind timers to global for call from export context
+ exp = IS_BIND && own ? ctx(out, global) : IS_PROTO && typeof out == 'function' ? ctx(Function.call, out) : out;
+ // extend global
+ if(target && !own)redefine(target, key, out);
+ // export
+ if(exports[key] != out)hide(exports, key, exp);
+ if(IS_PROTO && expProto[key] != out)expProto[key] = out;
+ }
+ };
+ global.core = core;
+ // type bitmap
+ $export.F = 1; // forced
+ $export.G = 2; // global
+ $export.S = 4; // static
+ $export.P = 8; // proto
+ $export.B = 16; // bind
+ $export.W = 32; // wrap
+ module.exports = $export;
+
+/***/ },
+/* 4 */
+/***/ function(module, exports) {
+
+ // https://github.com/zloirock/core-js/issues/86#issuecomment-115759028
+ var global = module.exports = typeof window != 'undefined' && window.Math == Math
+ ? window : typeof self != 'undefined' && self.Math == Math ? self : Function('return this')();
+ if(typeof __g == 'number')__g = global; // eslint-disable-line no-undef
+
+/***/ },
+/* 5 */
+/***/ function(module, exports) {
+
+ var core = module.exports = {version: '1.2.6'};
+ if(typeof __e == 'number')__e = core; // eslint-disable-line no-undef
+
+/***/ },
+/* 6 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $ = __webpack_require__(2)
+ , createDesc = __webpack_require__(7);
+ module.exports = __webpack_require__(8) ? function(object, key, value){
+ return $.setDesc(object, key, createDesc(1, value));
+ } : function(object, key, value){
+ object[key] = value;
+ return object;
+ };
+
+/***/ },
+/* 7 */
+/***/ function(module, exports) {
+
+ module.exports = function(bitmap, value){
+ return {
+ enumerable : !(bitmap & 1),
+ configurable: !(bitmap & 2),
+ writable : !(bitmap & 4),
+ value : value
+ };
+ };
+
+/***/ },
+/* 8 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // Thank's IE8 for his funny defineProperty
+ module.exports = !__webpack_require__(9)(function(){
+ return Object.defineProperty({}, 'a', {get: function(){ return 7; }}).a != 7;
+ });
+
+/***/ },
+/* 9 */
+/***/ function(module, exports) {
+
+ module.exports = function(exec){
+ try {
+ return !!exec();
+ } catch(e){
+ return true;
+ }
+ };
+
+/***/ },
+/* 10 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // add fake Function#toString
+ // for correct work wrapped methods / constructors with methods like LoDash isNative
+ var global = __webpack_require__(4)
+ , hide = __webpack_require__(6)
+ , SRC = __webpack_require__(11)('src')
+ , TO_STRING = 'toString'
+ , $toString = Function[TO_STRING]
+ , TPL = ('' + $toString).split(TO_STRING);
+
+ __webpack_require__(5).inspectSource = function(it){
+ return $toString.call(it);
+ };
+
+ (module.exports = function(O, key, val, safe){
+ if(typeof val == 'function'){
+ val.hasOwnProperty(SRC) || hide(val, SRC, O[key] ? '' + O[key] : TPL.join(String(key)));
+ val.hasOwnProperty('name') || hide(val, 'name', key);
+ }
+ if(O === global){
+ O[key] = val;
+ } else {
+ if(!safe)delete O[key];
+ hide(O, key, val);
+ }
+ })(Function.prototype, TO_STRING, function toString(){
+ return typeof this == 'function' && this[SRC] || $toString.call(this);
+ });
+
+/***/ },
+/* 11 */
+/***/ function(module, exports) {
+
+ var id = 0
+ , px = Math.random();
+ module.exports = function(key){
+ return 'Symbol('.concat(key === undefined ? '' : key, ')_', (++id + px).toString(36));
+ };
+
+/***/ },
+/* 12 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // optional / simple context binding
+ var aFunction = __webpack_require__(13);
+ module.exports = function(fn, that, length){
+ aFunction(fn);
+ if(that === undefined)return fn;
+ switch(length){
+ case 1: return function(a){
+ return fn.call(that, a);
+ };
+ case 2: return function(a, b){
+ return fn.call(that, a, b);
+ };
+ case 3: return function(a, b, c){
+ return fn.call(that, a, b, c);
+ };
+ }
+ return function(/* ...args */){
+ return fn.apply(that, arguments);
+ };
+ };
+
+/***/ },
+/* 13 */
+/***/ function(module, exports) {
+
+ module.exports = function(it){
+ if(typeof it != 'function')throw TypeError(it + ' is not a function!');
+ return it;
+ };
+
+/***/ },
+/* 14 */
+/***/ function(module, exports, __webpack_require__) {
+
+ module.exports = __webpack_require__(4).document && document.documentElement;
+
+/***/ },
+/* 15 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var isObject = __webpack_require__(16)
+ , document = __webpack_require__(4).document
+ // in old IE typeof document.createElement is 'object'
+ , is = isObject(document) && isObject(document.createElement);
+ module.exports = function(it){
+ return is ? document.createElement(it) : {};
+ };
+
+/***/ },
+/* 16 */
+/***/ function(module, exports) {
+
+ module.exports = function(it){
+ return typeof it === 'object' ? it !== null : typeof it === 'function';
+ };
+
+/***/ },
+/* 17 */
+/***/ function(module, exports) {
+
+ var hasOwnProperty = {}.hasOwnProperty;
+ module.exports = function(it, key){
+ return hasOwnProperty.call(it, key);
+ };
+
+/***/ },
+/* 18 */
+/***/ function(module, exports) {
+
+ var toString = {}.toString;
+
+ module.exports = function(it){
+ return toString.call(it).slice(8, -1);
+ };
+
+/***/ },
+/* 19 */
+/***/ function(module, exports) {
+
+ // fast apply, http://jsperf.lnkit.com/fast-apply/5
+ module.exports = function(fn, args, that){
+ var un = that === undefined;
+ switch(args.length){
+ case 0: return un ? fn()
+ : fn.call(that);
+ case 1: return un ? fn(args[0])
+ : fn.call(that, args[0]);
+ case 2: return un ? fn(args[0], args[1])
+ : fn.call(that, args[0], args[1]);
+ case 3: return un ? fn(args[0], args[1], args[2])
+ : fn.call(that, args[0], args[1], args[2]);
+ case 4: return un ? fn(args[0], args[1], args[2], args[3])
+ : fn.call(that, args[0], args[1], args[2], args[3]);
+ } return fn.apply(that, args);
+ };
+
+/***/ },
+/* 20 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var isObject = __webpack_require__(16);
+ module.exports = function(it){
+ if(!isObject(it))throw TypeError(it + ' is not an object!');
+ return it;
+ };
+
+/***/ },
+/* 21 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 7.1.13 ToObject(argument)
+ var defined = __webpack_require__(22);
+ module.exports = function(it){
+ return Object(defined(it));
+ };
+
+/***/ },
+/* 22 */
+/***/ function(module, exports) {
+
+ // 7.2.1 RequireObjectCoercible(argument)
+ module.exports = function(it){
+ if(it == undefined)throw TypeError("Can't call method on " + it);
+ return it;
+ };
+
+/***/ },
+/* 23 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // to indexed object, toObject with fallback for non-array-like ES3 strings
+ var IObject = __webpack_require__(24)
+ , defined = __webpack_require__(22);
+ module.exports = function(it){
+ return IObject(defined(it));
+ };
+
+/***/ },
+/* 24 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // fallback for non-array-like ES3 and non-enumerable old V8 strings
+ var cof = __webpack_require__(18);
+ module.exports = Object('z').propertyIsEnumerable(0) ? Object : function(it){
+ return cof(it) == 'String' ? it.split('') : Object(it);
+ };
+
+/***/ },
+/* 25 */
+/***/ function(module, exports) {
+
+ // 7.1.4 ToInteger
+ var ceil = Math.ceil
+ , floor = Math.floor;
+ module.exports = function(it){
+ return isNaN(it = +it) ? 0 : (it > 0 ? floor : ceil)(it);
+ };
+
+/***/ },
+/* 26 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var toInteger = __webpack_require__(25)
+ , max = Math.max
+ , min = Math.min;
+ module.exports = function(index, length){
+ index = toInteger(index);
+ return index < 0 ? max(index + length, 0) : min(index, length);
+ };
+
+/***/ },
+/* 27 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 7.1.15 ToLength
+ var toInteger = __webpack_require__(25)
+ , min = Math.min;
+ module.exports = function(it){
+ return it > 0 ? min(toInteger(it), 0x1fffffffffffff) : 0; // pow(2, 53) - 1 == 9007199254740991
+ };
+
+/***/ },
+/* 28 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 0 -> Array#forEach
+ // 1 -> Array#map
+ // 2 -> Array#filter
+ // 3 -> Array#some
+ // 4 -> Array#every
+ // 5 -> Array#find
+ // 6 -> Array#findIndex
+ var ctx = __webpack_require__(12)
+ , IObject = __webpack_require__(24)
+ , toObject = __webpack_require__(21)
+ , toLength = __webpack_require__(27)
+ , asc = __webpack_require__(29);
+ module.exports = function(TYPE){
+ var IS_MAP = TYPE == 1
+ , IS_FILTER = TYPE == 2
+ , IS_SOME = TYPE == 3
+ , IS_EVERY = TYPE == 4
+ , IS_FIND_INDEX = TYPE == 6
+ , NO_HOLES = TYPE == 5 || IS_FIND_INDEX;
+ return function($this, callbackfn, that){
+ var O = toObject($this)
+ , self = IObject(O)
+ , f = ctx(callbackfn, that, 3)
+ , length = toLength(self.length)
+ , index = 0
+ , result = IS_MAP ? asc($this, length) : IS_FILTER ? asc($this, 0) : undefined
+ , val, res;
+ for(;length > index; index++)if(NO_HOLES || index in self){
+ val = self[index];
+ res = f(val, index, O);
+ if(TYPE){
+ if(IS_MAP)result[index] = res; // map
+ else if(res)switch(TYPE){
+ case 3: return true; // some
+ case 5: return val; // find
+ case 6: return index; // findIndex
+ case 2: result.push(val); // filter
+ } else if(IS_EVERY)return false; // every
+ }
+ }
+ return IS_FIND_INDEX ? -1 : IS_SOME || IS_EVERY ? IS_EVERY : result;
+ };
+ };
+
+/***/ },
+/* 29 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 9.4.2.3 ArraySpeciesCreate(originalArray, length)
+ var isObject = __webpack_require__(16)
+ , isArray = __webpack_require__(30)
+ , SPECIES = __webpack_require__(31)('species');
+ module.exports = function(original, length){
+ var C;
+ if(isArray(original)){
+ C = original.constructor;
+ // cross-realm fallback
+ if(typeof C == 'function' && (C === Array || isArray(C.prototype)))C = undefined;
+ if(isObject(C)){
+ C = C[SPECIES];
+ if(C === null)C = undefined;
+ }
+ } return new (C === undefined ? Array : C)(length);
+ };
+
+/***/ },
+/* 30 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 7.2.2 IsArray(argument)
+ var cof = __webpack_require__(18);
+ module.exports = Array.isArray || function(arg){
+ return cof(arg) == 'Array';
+ };
+
+/***/ },
+/* 31 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var store = __webpack_require__(32)('wks')
+ , uid = __webpack_require__(11)
+ , Symbol = __webpack_require__(4).Symbol;
+ module.exports = function(name){
+ return store[name] || (store[name] =
+ Symbol && Symbol[name] || (Symbol || uid)('Symbol.' + name));
+ };
+
+/***/ },
+/* 32 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var global = __webpack_require__(4)
+ , SHARED = '__core-js_shared__'
+ , store = global[SHARED] || (global[SHARED] = {});
+ module.exports = function(key){
+ return store[key] || (store[key] = {});
+ };
+
+/***/ },
+/* 33 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // false -> Array#indexOf
+ // true -> Array#includes
+ var toIObject = __webpack_require__(23)
+ , toLength = __webpack_require__(27)
+ , toIndex = __webpack_require__(26);
+ module.exports = function(IS_INCLUDES){
+ return function($this, el, fromIndex){
+ var O = toIObject($this)
+ , length = toLength(O.length)
+ , index = toIndex(fromIndex, length)
+ , value;
+ // Array#includes uses SameValueZero equality algorithm
+ if(IS_INCLUDES && el != el)while(length > index){
+ value = O[index++];
+ if(value != value)return true;
+ // Array#toIndex ignores holes, Array#includes - not
+ } else for(;length > index; index++)if(IS_INCLUDES || index in O){
+ if(O[index] === el)return IS_INCLUDES || index;
+ } return !IS_INCLUDES && -1;
+ };
+ };
+
+/***/ },
+/* 34 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // ECMAScript 6 symbols shim
+ var $ = __webpack_require__(2)
+ , global = __webpack_require__(4)
+ , has = __webpack_require__(17)
+ , DESCRIPTORS = __webpack_require__(8)
+ , $export = __webpack_require__(3)
+ , redefine = __webpack_require__(10)
+ , $fails = __webpack_require__(9)
+ , shared = __webpack_require__(32)
+ , setToStringTag = __webpack_require__(35)
+ , uid = __webpack_require__(11)
+ , wks = __webpack_require__(31)
+ , keyOf = __webpack_require__(36)
+ , $names = __webpack_require__(37)
+ , enumKeys = __webpack_require__(38)
+ , isArray = __webpack_require__(30)
+ , anObject = __webpack_require__(20)
+ , toIObject = __webpack_require__(23)
+ , createDesc = __webpack_require__(7)
+ , getDesc = $.getDesc
+ , setDesc = $.setDesc
+ , _create = $.create
+ , getNames = $names.get
+ , $Symbol = global.Symbol
+ , $JSON = global.JSON
+ , _stringify = $JSON && $JSON.stringify
+ , setter = false
+ , HIDDEN = wks('_hidden')
+ , isEnum = $.isEnum
+ , SymbolRegistry = shared('symbol-registry')
+ , AllSymbols = shared('symbols')
+ , useNative = typeof $Symbol == 'function'
+ , ObjectProto = Object.prototype;
+
+ // fallback for old Android, https://code.google.com/p/v8/issues/detail?id=687
+ var setSymbolDesc = DESCRIPTORS && $fails(function(){
+ return _create(setDesc({}, 'a', {
+ get: function(){ return setDesc(this, 'a', {value: 7}).a; }
+ })).a != 7;
+ }) ? function(it, key, D){
+ var protoDesc = getDesc(ObjectProto, key);
+ if(protoDesc)delete ObjectProto[key];
+ setDesc(it, key, D);
+ if(protoDesc && it !== ObjectProto)setDesc(ObjectProto, key, protoDesc);
+ } : setDesc;
+
+ var wrap = function(tag){
+ var sym = AllSymbols[tag] = _create($Symbol.prototype);
+ sym._k = tag;
+ DESCRIPTORS && setter && setSymbolDesc(ObjectProto, tag, {
+ configurable: true,
+ set: function(value){
+ if(has(this, HIDDEN) && has(this[HIDDEN], tag))this[HIDDEN][tag] = false;
+ setSymbolDesc(this, tag, createDesc(1, value));
+ }
+ });
+ return sym;
+ };
+
+ var isSymbol = function(it){
+ return typeof it == 'symbol';
+ };
+
+ var $defineProperty = function defineProperty(it, key, D){
+ if(D && has(AllSymbols, key)){
+ if(!D.enumerable){
+ if(!has(it, HIDDEN))setDesc(it, HIDDEN, createDesc(1, {}));
+ it[HIDDEN][key] = true;
+ } else {
+ if(has(it, HIDDEN) && it[HIDDEN][key])it[HIDDEN][key] = false;
+ D = _create(D, {enumerable: createDesc(0, false)});
+ } return setSymbolDesc(it, key, D);
+ } return setDesc(it, key, D);
+ };
+ var $defineProperties = function defineProperties(it, P){
+ anObject(it);
+ var keys = enumKeys(P = toIObject(P))
+ , i = 0
+ , l = keys.length
+ , key;
+ while(l > i)$defineProperty(it, key = keys[i++], P[key]);
+ return it;
+ };
+ var $create = function create(it, P){
+ return P === undefined ? _create(it) : $defineProperties(_create(it), P);
+ };
+ var $propertyIsEnumerable = function propertyIsEnumerable(key){
+ var E = isEnum.call(this, key);
+ return E || !has(this, key) || !has(AllSymbols, key) || has(this, HIDDEN) && this[HIDDEN][key]
+ ? E : true;
+ };
+ var $getOwnPropertyDescriptor = function getOwnPropertyDescriptor(it, key){
+ var D = getDesc(it = toIObject(it), key);
+ if(D && has(AllSymbols, key) && !(has(it, HIDDEN) && it[HIDDEN][key]))D.enumerable = true;
+ return D;
+ };
+ var $getOwnPropertyNames = function getOwnPropertyNames(it){
+ var names = getNames(toIObject(it))
+ , result = []
+ , i = 0
+ , key;
+ while(names.length > i)if(!has(AllSymbols, key = names[i++]) && key != HIDDEN)result.push(key);
+ return result;
+ };
+ var $getOwnPropertySymbols = function getOwnPropertySymbols(it){
+ var names = getNames(toIObject(it))
+ , result = []
+ , i = 0
+ , key;
+ while(names.length > i)if(has(AllSymbols, key = names[i++]))result.push(AllSymbols[key]);
+ return result;
+ };
+ var $stringify = function stringify(it){
+ if(it === undefined || isSymbol(it))return; // IE8 returns string on undefined
+ var args = [it]
+ , i = 1
+ , $$ = arguments
+ , replacer, $replacer;
+ while($$.length > i)args.push($$[i++]);
+ replacer = args[1];
+ if(typeof replacer == 'function')$replacer = replacer;
+ if($replacer || !isArray(replacer))replacer = function(key, value){
+ if($replacer)value = $replacer.call(this, key, value);
+ if(!isSymbol(value))return value;
+ };
+ args[1] = replacer;
+ return _stringify.apply($JSON, args);
+ };
+ var buggyJSON = $fails(function(){
+ var S = $Symbol();
+ // MS Edge converts symbol values to JSON as {}
+ // WebKit converts symbol values to JSON as null
+ // V8 throws on boxed symbols
+ return _stringify([S]) != '[null]' || _stringify({a: S}) != '{}' || _stringify(Object(S)) != '{}';
+ });
+
+ // 19.4.1.1 Symbol([description])
+ if(!useNative){
+ $Symbol = function Symbol(){
+ if(isSymbol(this))throw TypeError('Symbol is not a constructor');
+ return wrap(uid(arguments.length > 0 ? arguments[0] : undefined));
+ };
+ redefine($Symbol.prototype, 'toString', function toString(){
+ return this._k;
+ });
+
+ isSymbol = function(it){
+ return it instanceof $Symbol;
+ };
+
+ $.create = $create;
+ $.isEnum = $propertyIsEnumerable;
+ $.getDesc = $getOwnPropertyDescriptor;
+ $.setDesc = $defineProperty;
+ $.setDescs = $defineProperties;
+ $.getNames = $names.get = $getOwnPropertyNames;
+ $.getSymbols = $getOwnPropertySymbols;
+
+ if(DESCRIPTORS && !__webpack_require__(39)){
+ redefine(ObjectProto, 'propertyIsEnumerable', $propertyIsEnumerable, true);
+ }
+ }
+
+ var symbolStatics = {
+ // 19.4.2.1 Symbol.for(key)
+ 'for': function(key){
+ return has(SymbolRegistry, key += '')
+ ? SymbolRegistry[key]
+ : SymbolRegistry[key] = $Symbol(key);
+ },
+ // 19.4.2.5 Symbol.keyFor(sym)
+ keyFor: function keyFor(key){
+ return keyOf(SymbolRegistry, key);
+ },
+ useSetter: function(){ setter = true; },
+ useSimple: function(){ setter = false; }
+ };
+ // 19.4.2.2 Symbol.hasInstance
+ // 19.4.2.3 Symbol.isConcatSpreadable
+ // 19.4.2.4 Symbol.iterator
+ // 19.4.2.6 Symbol.match
+ // 19.4.2.8 Symbol.replace
+ // 19.4.2.9 Symbol.search
+ // 19.4.2.10 Symbol.species
+ // 19.4.2.11 Symbol.split
+ // 19.4.2.12 Symbol.toPrimitive
+ // 19.4.2.13 Symbol.toStringTag
+ // 19.4.2.14 Symbol.unscopables
+ $.each.call((
+ 'hasInstance,isConcatSpreadable,iterator,match,replace,search,' +
+ 'species,split,toPrimitive,toStringTag,unscopables'
+ ).split(','), function(it){
+ var sym = wks(it);
+ symbolStatics[it] = useNative ? sym : wrap(sym);
+ });
+
+ setter = true;
+
+ $export($export.G + $export.W, {Symbol: $Symbol});
+
+ $export($export.S, 'Symbol', symbolStatics);
+
+ $export($export.S + $export.F * !useNative, 'Object', {
+ // 19.1.2.2 Object.create(O [, Properties])
+ create: $create,
+ // 19.1.2.4 Object.defineProperty(O, P, Attributes)
+ defineProperty: $defineProperty,
+ // 19.1.2.3 Object.defineProperties(O, Properties)
+ defineProperties: $defineProperties,
+ // 19.1.2.6 Object.getOwnPropertyDescriptor(O, P)
+ getOwnPropertyDescriptor: $getOwnPropertyDescriptor,
+ // 19.1.2.7 Object.getOwnPropertyNames(O)
+ getOwnPropertyNames: $getOwnPropertyNames,
+ // 19.1.2.8 Object.getOwnPropertySymbols(O)
+ getOwnPropertySymbols: $getOwnPropertySymbols
+ });
+
+ // 24.3.2 JSON.stringify(value [, replacer [, space]])
+ $JSON && $export($export.S + $export.F * (!useNative || buggyJSON), 'JSON', {stringify: $stringify});
+
+ // 19.4.3.5 Symbol.prototype[@@toStringTag]
+ setToStringTag($Symbol, 'Symbol');
+ // 20.2.1.9 Math[@@toStringTag]
+ setToStringTag(Math, 'Math', true);
+ // 24.3.3 JSON[@@toStringTag]
+ setToStringTag(global.JSON, 'JSON', true);
+
+/***/ },
+/* 35 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var def = __webpack_require__(2).setDesc
+ , has = __webpack_require__(17)
+ , TAG = __webpack_require__(31)('toStringTag');
+
+ module.exports = function(it, tag, stat){
+ if(it && !has(it = stat ? it : it.prototype, TAG))def(it, TAG, {configurable: true, value: tag});
+ };
+
+/***/ },
+/* 36 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $ = __webpack_require__(2)
+ , toIObject = __webpack_require__(23);
+ module.exports = function(object, el){
+ var O = toIObject(object)
+ , keys = $.getKeys(O)
+ , length = keys.length
+ , index = 0
+ , key;
+ while(length > index)if(O[key = keys[index++]] === el)return key;
+ };
+
+/***/ },
+/* 37 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // fallback for IE11 buggy Object.getOwnPropertyNames with iframe and window
+ var toIObject = __webpack_require__(23)
+ , getNames = __webpack_require__(2).getNames
+ , toString = {}.toString;
+
+ var windowNames = typeof window == 'object' && Object.getOwnPropertyNames
+ ? Object.getOwnPropertyNames(window) : [];
+
+ var getWindowNames = function(it){
+ try {
+ return getNames(it);
+ } catch(e){
+ return windowNames.slice();
+ }
+ };
+
+ module.exports.get = function getOwnPropertyNames(it){
+ if(windowNames && toString.call(it) == '[object Window]')return getWindowNames(it);
+ return getNames(toIObject(it));
+ };
+
+/***/ },
+/* 38 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // all enumerable object keys, includes symbols
+ var $ = __webpack_require__(2);
+ module.exports = function(it){
+ var keys = $.getKeys(it)
+ , getSymbols = $.getSymbols;
+ if(getSymbols){
+ var symbols = getSymbols(it)
+ , isEnum = $.isEnum
+ , i = 0
+ , key;
+ while(symbols.length > i)if(isEnum.call(it, key = symbols[i++]))keys.push(key);
+ }
+ return keys;
+ };
+
+/***/ },
+/* 39 */
+/***/ function(module, exports) {
+
+ module.exports = false;
+
+/***/ },
+/* 40 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.3.1 Object.assign(target, source)
+ var $export = __webpack_require__(3);
+
+ $export($export.S + $export.F, 'Object', {assign: __webpack_require__(41)});
+
+/***/ },
+/* 41 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.1 Object.assign(target, source, ...)
+ var $ = __webpack_require__(2)
+ , toObject = __webpack_require__(21)
+ , IObject = __webpack_require__(24);
+
+ // should work with symbols and should have deterministic property order (V8 bug)
+ module.exports = __webpack_require__(9)(function(){
+ var a = Object.assign
+ , A = {}
+ , B = {}
+ , S = Symbol()
+ , K = 'abcdefghijklmnopqrst';
+ A[S] = 7;
+ K.split('').forEach(function(k){ B[k] = k; });
+ return a({}, A)[S] != 7 || Object.keys(a({}, B)).join('') != K;
+ }) ? function assign(target, source){ // eslint-disable-line no-unused-vars
+ var T = toObject(target)
+ , $$ = arguments
+ , $$len = $$.length
+ , index = 1
+ , getKeys = $.getKeys
+ , getSymbols = $.getSymbols
+ , isEnum = $.isEnum;
+ while($$len > index){
+ var S = IObject($$[index++])
+ , keys = getSymbols ? getKeys(S).concat(getSymbols(S)) : getKeys(S)
+ , length = keys.length
+ , j = 0
+ , key;
+ while(length > j)if(isEnum.call(S, key = keys[j++]))T[key] = S[key];
+ }
+ return T;
+ } : Object.assign;
+
+/***/ },
+/* 42 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.3.10 Object.is(value1, value2)
+ var $export = __webpack_require__(3);
+ $export($export.S, 'Object', {is: __webpack_require__(43)});
+
+/***/ },
+/* 43 */
+/***/ function(module, exports) {
+
+ // 7.2.9 SameValue(x, y)
+ module.exports = Object.is || function is(x, y){
+ return x === y ? x !== 0 || 1 / x === 1 / y : x != x && y != y;
+ };
+
+/***/ },
+/* 44 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.3.19 Object.setPrototypeOf(O, proto)
+ var $export = __webpack_require__(3);
+ $export($export.S, 'Object', {setPrototypeOf: __webpack_require__(45).set});
+
+/***/ },
+/* 45 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // Works with __proto__ only. Old v8 can't work with null proto objects.
+ /* eslint-disable no-proto */
+ var getDesc = __webpack_require__(2).getDesc
+ , isObject = __webpack_require__(16)
+ , anObject = __webpack_require__(20);
+ var check = function(O, proto){
+ anObject(O);
+ if(!isObject(proto) && proto !== null)throw TypeError(proto + ": can't set as prototype!");
+ };
+ module.exports = {
+ set: Object.setPrototypeOf || ('__proto__' in {} ? // eslint-disable-line
+ function(test, buggy, set){
+ try {
+ set = __webpack_require__(12)(Function.call, getDesc(Object.prototype, '__proto__').set, 2);
+ set(test, []);
+ buggy = !(test instanceof Array);
+ } catch(e){ buggy = true; }
+ return function setPrototypeOf(O, proto){
+ check(O, proto);
+ if(buggy)O.__proto__ = proto;
+ else set(O, proto);
+ return O;
+ };
+ }({}, false) : undefined),
+ check: check
+ };
+
+/***/ },
+/* 46 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // 19.1.3.6 Object.prototype.toString()
+ var classof = __webpack_require__(47)
+ , test = {};
+ test[__webpack_require__(31)('toStringTag')] = 'z';
+ if(test + '' != '[object z]'){
+ __webpack_require__(10)(Object.prototype, 'toString', function toString(){
+ return '[object ' + classof(this) + ']';
+ }, true);
+ }
+
+/***/ },
+/* 47 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // getting tag from 19.1.3.6 Object.prototype.toString()
+ var cof = __webpack_require__(18)
+ , TAG = __webpack_require__(31)('toStringTag')
+ // ES3 wrong here
+ , ARG = cof(function(){ return arguments; }()) == 'Arguments';
+
+ module.exports = function(it){
+ var O, T, B;
+ return it === undefined ? 'Undefined' : it === null ? 'Null'
+ // @@toStringTag case
+ : typeof (T = (O = Object(it))[TAG]) == 'string' ? T
+ // builtinTag case
+ : ARG ? cof(O)
+ // ES3 arguments fallback
+ : (B = cof(O)) == 'Object' && typeof O.callee == 'function' ? 'Arguments' : B;
+ };
+
+/***/ },
+/* 48 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.5 Object.freeze(O)
+ var isObject = __webpack_require__(16);
+
+ __webpack_require__(49)('freeze', function($freeze){
+ return function freeze(it){
+ return $freeze && isObject(it) ? $freeze(it) : it;
+ };
+ });
+
+/***/ },
+/* 49 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // most Object methods by ES6 should accept primitives
+ var $export = __webpack_require__(3)
+ , core = __webpack_require__(5)
+ , fails = __webpack_require__(9);
+ module.exports = function(KEY, exec){
+ var fn = (core.Object || {})[KEY] || Object[KEY]
+ , exp = {};
+ exp[KEY] = exec(fn);
+ $export($export.S + $export.F * fails(function(){ fn(1); }), 'Object', exp);
+ };
+
+/***/ },
+/* 50 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.17 Object.seal(O)
+ var isObject = __webpack_require__(16);
+
+ __webpack_require__(49)('seal', function($seal){
+ return function seal(it){
+ return $seal && isObject(it) ? $seal(it) : it;
+ };
+ });
+
+/***/ },
+/* 51 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.15 Object.preventExtensions(O)
+ var isObject = __webpack_require__(16);
+
+ __webpack_require__(49)('preventExtensions', function($preventExtensions){
+ return function preventExtensions(it){
+ return $preventExtensions && isObject(it) ? $preventExtensions(it) : it;
+ };
+ });
+
+/***/ },
+/* 52 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.12 Object.isFrozen(O)
+ var isObject = __webpack_require__(16);
+
+ __webpack_require__(49)('isFrozen', function($isFrozen){
+ return function isFrozen(it){
+ return isObject(it) ? $isFrozen ? $isFrozen(it) : false : true;
+ };
+ });
+
+/***/ },
+/* 53 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.13 Object.isSealed(O)
+ var isObject = __webpack_require__(16);
+
+ __webpack_require__(49)('isSealed', function($isSealed){
+ return function isSealed(it){
+ return isObject(it) ? $isSealed ? $isSealed(it) : false : true;
+ };
+ });
+
+/***/ },
+/* 54 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.11 Object.isExtensible(O)
+ var isObject = __webpack_require__(16);
+
+ __webpack_require__(49)('isExtensible', function($isExtensible){
+ return function isExtensible(it){
+ return isObject(it) ? $isExtensible ? $isExtensible(it) : true : false;
+ };
+ });
+
+/***/ },
+/* 55 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.6 Object.getOwnPropertyDescriptor(O, P)
+ var toIObject = __webpack_require__(23);
+
+ __webpack_require__(49)('getOwnPropertyDescriptor', function($getOwnPropertyDescriptor){
+ return function getOwnPropertyDescriptor(it, key){
+ return $getOwnPropertyDescriptor(toIObject(it), key);
+ };
+ });
+
+/***/ },
+/* 56 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.9 Object.getPrototypeOf(O)
+ var toObject = __webpack_require__(21);
+
+ __webpack_require__(49)('getPrototypeOf', function($getPrototypeOf){
+ return function getPrototypeOf(it){
+ return $getPrototypeOf(toObject(it));
+ };
+ });
+
+/***/ },
+/* 57 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.14 Object.keys(O)
+ var toObject = __webpack_require__(21);
+
+ __webpack_require__(49)('keys', function($keys){
+ return function keys(it){
+ return $keys(toObject(it));
+ };
+ });
+
+/***/ },
+/* 58 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.7 Object.getOwnPropertyNames(O)
+ __webpack_require__(49)('getOwnPropertyNames', function(){
+ return __webpack_require__(37).get;
+ });
+
+/***/ },
+/* 59 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var setDesc = __webpack_require__(2).setDesc
+ , createDesc = __webpack_require__(7)
+ , has = __webpack_require__(17)
+ , FProto = Function.prototype
+ , nameRE = /^\s*function ([^ (]*)/
+ , NAME = 'name';
+ // 19.2.4.2 name
+ NAME in FProto || __webpack_require__(8) && setDesc(FProto, NAME, {
+ configurable: true,
+ get: function(){
+ var match = ('' + this).match(nameRE)
+ , name = match ? match[1] : '';
+ has(this, NAME) || setDesc(this, NAME, createDesc(5, name));
+ return name;
+ }
+ });
+
+/***/ },
+/* 60 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , isObject = __webpack_require__(16)
+ , HAS_INSTANCE = __webpack_require__(31)('hasInstance')
+ , FunctionProto = Function.prototype;
+ // 19.2.3.6 Function.prototype[@@hasInstance](V)
+ if(!(HAS_INSTANCE in FunctionProto))$.setDesc(FunctionProto, HAS_INSTANCE, {value: function(O){
+ if(typeof this != 'function' || !isObject(O))return false;
+ if(!isObject(this.prototype))return O instanceof this;
+ // for environment w/o native `@@hasInstance` logic enough `instanceof`, but add this:
+ while(O = $.getProto(O))if(this.prototype === O)return true;
+ return false;
+ }});
+
+/***/ },
+/* 61 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , global = __webpack_require__(4)
+ , has = __webpack_require__(17)
+ , cof = __webpack_require__(18)
+ , toPrimitive = __webpack_require__(62)
+ , fails = __webpack_require__(9)
+ , $trim = __webpack_require__(63).trim
+ , NUMBER = 'Number'
+ , $Number = global[NUMBER]
+ , Base = $Number
+ , proto = $Number.prototype
+ // Opera ~12 has broken Object#toString
+ , BROKEN_COF = cof($.create(proto)) == NUMBER
+ , TRIM = 'trim' in String.prototype;
+
+ // 7.1.3 ToNumber(argument)
+ var toNumber = function(argument){
+ var it = toPrimitive(argument, false);
+ if(typeof it == 'string' && it.length > 2){
+ it = TRIM ? it.trim() : $trim(it, 3);
+ var first = it.charCodeAt(0)
+ , third, radix, maxCode;
+ if(first === 43 || first === 45){
+ third = it.charCodeAt(2);
+ if(third === 88 || third === 120)return NaN; // Number('+0x1') should be NaN, old V8 fix
+ } else if(first === 48){
+ switch(it.charCodeAt(1)){
+ case 66 : case 98 : radix = 2; maxCode = 49; break; // fast equal /^0b[01]+$/i
+ case 79 : case 111 : radix = 8; maxCode = 55; break; // fast equal /^0o[0-7]+$/i
+ default : return +it;
+ }
+ for(var digits = it.slice(2), i = 0, l = digits.length, code; i < l; i++){
+ code = digits.charCodeAt(i);
+ // parseInt parses a string to a first unavailable symbol
+ // but ToNumber should return NaN if a string contains unavailable symbols
+ if(code < 48 || code > maxCode)return NaN;
+ } return parseInt(digits, radix);
+ }
+ } return +it;
+ };
+
+ if(!$Number(' 0o1') || !$Number('0b1') || $Number('+0x1')){
+ $Number = function Number(value){
+ var it = arguments.length < 1 ? 0 : value
+ , that = this;
+ return that instanceof $Number
+ // check on 1..constructor(foo) case
+ && (BROKEN_COF ? fails(function(){ proto.valueOf.call(that); }) : cof(that) != NUMBER)
+ ? new Base(toNumber(it)) : toNumber(it);
+ };
+ $.each.call(__webpack_require__(8) ? $.getNames(Base) : (
+ // ES3:
+ 'MAX_VALUE,MIN_VALUE,NaN,NEGATIVE_INFINITY,POSITIVE_INFINITY,' +
+ // ES6 (in case, if modules with ES6 Number statics required before):
+ 'EPSILON,isFinite,isInteger,isNaN,isSafeInteger,MAX_SAFE_INTEGER,' +
+ 'MIN_SAFE_INTEGER,parseFloat,parseInt,isInteger'
+ ).split(','), function(key){
+ if(has(Base, key) && !has($Number, key)){
+ $.setDesc($Number, key, $.getDesc(Base, key));
+ }
+ });
+ $Number.prototype = proto;
+ proto.constructor = $Number;
+ __webpack_require__(10)(global, NUMBER, $Number);
+ }
+
+/***/ },
+/* 62 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 7.1.1 ToPrimitive(input [, PreferredType])
+ var isObject = __webpack_require__(16);
+ // instead of the ES6 spec version, we didn't implement @@toPrimitive case
+ // and the second argument - flag - preferred type is a string
+ module.exports = function(it, S){
+ if(!isObject(it))return it;
+ var fn, val;
+ if(S && typeof (fn = it.toString) == 'function' && !isObject(val = fn.call(it)))return val;
+ if(typeof (fn = it.valueOf) == 'function' && !isObject(val = fn.call(it)))return val;
+ if(!S && typeof (fn = it.toString) == 'function' && !isObject(val = fn.call(it)))return val;
+ throw TypeError("Can't convert object to primitive value");
+ };
+
+/***/ },
+/* 63 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3)
+ , defined = __webpack_require__(22)
+ , fails = __webpack_require__(9)
+ , spaces = '\x09\x0A\x0B\x0C\x0D\x20\xA0\u1680\u180E\u2000\u2001\u2002\u2003' +
+ '\u2004\u2005\u2006\u2007\u2008\u2009\u200A\u202F\u205F\u3000\u2028\u2029\uFEFF'
+ , space = '[' + spaces + ']'
+ , non = '\u200b\u0085'
+ , ltrim = RegExp('^' + space + space + '*')
+ , rtrim = RegExp(space + space + '*$');
+
+ var exporter = function(KEY, exec){
+ var exp = {};
+ exp[KEY] = exec(trim);
+ $export($export.P + $export.F * fails(function(){
+ return !!spaces[KEY]() || non[KEY]() != non;
+ }), 'String', exp);
+ };
+
+ // 1 -> String#trimLeft
+ // 2 -> String#trimRight
+ // 3 -> String#trim
+ var trim = exporter.trim = function(string, TYPE){
+ string = String(defined(string));
+ if(TYPE & 1)string = string.replace(ltrim, '');
+ if(TYPE & 2)string = string.replace(rtrim, '');
+ return string;
+ };
+
+ module.exports = exporter;
+
+/***/ },
+/* 64 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.1 Number.EPSILON
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {EPSILON: Math.pow(2, -52)});
+
+/***/ },
+/* 65 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.2 Number.isFinite(number)
+ var $export = __webpack_require__(3)
+ , _isFinite = __webpack_require__(4).isFinite;
+
+ $export($export.S, 'Number', {
+ isFinite: function isFinite(it){
+ return typeof it == 'number' && _isFinite(it);
+ }
+ });
+
+/***/ },
+/* 66 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.3 Number.isInteger(number)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {isInteger: __webpack_require__(67)});
+
+/***/ },
+/* 67 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.3 Number.isInteger(number)
+ var isObject = __webpack_require__(16)
+ , floor = Math.floor;
+ module.exports = function isInteger(it){
+ return !isObject(it) && isFinite(it) && floor(it) === it;
+ };
+
+/***/ },
+/* 68 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.4 Number.isNaN(number)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {
+ isNaN: function isNaN(number){
+ return number != number;
+ }
+ });
+
+/***/ },
+/* 69 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.5 Number.isSafeInteger(number)
+ var $export = __webpack_require__(3)
+ , isInteger = __webpack_require__(67)
+ , abs = Math.abs;
+
+ $export($export.S, 'Number', {
+ isSafeInteger: function isSafeInteger(number){
+ return isInteger(number) && abs(number) <= 0x1fffffffffffff;
+ }
+ });
+
+/***/ },
+/* 70 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.6 Number.MAX_SAFE_INTEGER
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {MAX_SAFE_INTEGER: 0x1fffffffffffff});
+
+/***/ },
+/* 71 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.10 Number.MIN_SAFE_INTEGER
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {MIN_SAFE_INTEGER: -0x1fffffffffffff});
+
+/***/ },
+/* 72 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.12 Number.parseFloat(string)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {parseFloat: parseFloat});
+
+/***/ },
+/* 73 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.13 Number.parseInt(string, radix)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {parseInt: parseInt});
+
+/***/ },
+/* 74 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.3 Math.acosh(x)
+ var $export = __webpack_require__(3)
+ , log1p = __webpack_require__(75)
+ , sqrt = Math.sqrt
+ , $acosh = Math.acosh;
+
+ // V8 bug https://code.google.com/p/v8/issues/detail?id=3509
+ $export($export.S + $export.F * !($acosh && Math.floor($acosh(Number.MAX_VALUE)) == 710), 'Math', {
+ acosh: function acosh(x){
+ return (x = +x) < 1 ? NaN : x > 94906265.62425156
+ ? Math.log(x) + Math.LN2
+ : log1p(x - 1 + sqrt(x - 1) * sqrt(x + 1));
+ }
+ });
+
+/***/ },
+/* 75 */
+/***/ function(module, exports) {
+
+ // 20.2.2.20 Math.log1p(x)
+ module.exports = Math.log1p || function log1p(x){
+ return (x = +x) > -1e-8 && x < 1e-8 ? x - x * x / 2 : Math.log(1 + x);
+ };
+
+/***/ },
+/* 76 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.5 Math.asinh(x)
+ var $export = __webpack_require__(3);
+
+ function asinh(x){
+ return !isFinite(x = +x) || x == 0 ? x : x < 0 ? -asinh(-x) : Math.log(x + Math.sqrt(x * x + 1));
+ }
+
+ $export($export.S, 'Math', {asinh: asinh});
+
+/***/ },
+/* 77 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.7 Math.atanh(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {
+ atanh: function atanh(x){
+ return (x = +x) == 0 ? x : Math.log((1 + x) / (1 - x)) / 2;
+ }
+ });
+
+/***/ },
+/* 78 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.9 Math.cbrt(x)
+ var $export = __webpack_require__(3)
+ , sign = __webpack_require__(79);
+
+ $export($export.S, 'Math', {
+ cbrt: function cbrt(x){
+ return sign(x = +x) * Math.pow(Math.abs(x), 1 / 3);
+ }
+ });
+
+/***/ },
+/* 79 */
+/***/ function(module, exports) {
+
+ // 20.2.2.28 Math.sign(x)
+ module.exports = Math.sign || function sign(x){
+ return (x = +x) == 0 || x != x ? x : x < 0 ? -1 : 1;
+ };
+
+/***/ },
+/* 80 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.11 Math.clz32(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {
+ clz32: function clz32(x){
+ return (x >>>= 0) ? 31 - Math.floor(Math.log(x + 0.5) * Math.LOG2E) : 32;
+ }
+ });
+
+/***/ },
+/* 81 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.12 Math.cosh(x)
+ var $export = __webpack_require__(3)
+ , exp = Math.exp;
+
+ $export($export.S, 'Math', {
+ cosh: function cosh(x){
+ return (exp(x = +x) + exp(-x)) / 2;
+ }
+ });
+
+/***/ },
+/* 82 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.14 Math.expm1(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {expm1: __webpack_require__(83)});
+
+/***/ },
+/* 83 */
+/***/ function(module, exports) {
+
+ // 20.2.2.14 Math.expm1(x)
+ module.exports = Math.expm1 || function expm1(x){
+ return (x = +x) == 0 ? x : x > -1e-6 && x < 1e-6 ? x + x * x / 2 : Math.exp(x) - 1;
+ };
+
+/***/ },
+/* 84 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.16 Math.fround(x)
+ var $export = __webpack_require__(3)
+ , sign = __webpack_require__(79)
+ , pow = Math.pow
+ , EPSILON = pow(2, -52)
+ , EPSILON32 = pow(2, -23)
+ , MAX32 = pow(2, 127) * (2 - EPSILON32)
+ , MIN32 = pow(2, -126);
+
+ var roundTiesToEven = function(n){
+ return n + 1 / EPSILON - 1 / EPSILON;
+ };
+
+
+ $export($export.S, 'Math', {
+ fround: function fround(x){
+ var $abs = Math.abs(x)
+ , $sign = sign(x)
+ , a, result;
+ if($abs < MIN32)return $sign * roundTiesToEven($abs / MIN32 / EPSILON32) * MIN32 * EPSILON32;
+ a = (1 + EPSILON32 / EPSILON) * $abs;
+ result = a - (a - $abs);
+ if(result > MAX32 || result != result)return $sign * Infinity;
+ return $sign * result;
+ }
+ });
+
+/***/ },
+/* 85 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.17 Math.hypot([value1[, value2[, … ]]])
+ var $export = __webpack_require__(3)
+ , abs = Math.abs;
+
+ $export($export.S, 'Math', {
+ hypot: function hypot(value1, value2){ // eslint-disable-line no-unused-vars
+ var sum = 0
+ , i = 0
+ , $$ = arguments
+ , $$len = $$.length
+ , larg = 0
+ , arg, div;
+ while(i < $$len){
+ arg = abs($$[i++]);
+ if(larg < arg){
+ div = larg / arg;
+ sum = sum * div * div + 1;
+ larg = arg;
+ } else if(arg > 0){
+ div = arg / larg;
+ sum += div * div;
+ } else sum += arg;
+ }
+ return larg === Infinity ? Infinity : larg * Math.sqrt(sum);
+ }
+ });
+
+/***/ },
+/* 86 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.18 Math.imul(x, y)
+ var $export = __webpack_require__(3)
+ , $imul = Math.imul;
+
+ // some WebKit versions fails with big numbers, some has wrong arity
+ $export($export.S + $export.F * __webpack_require__(9)(function(){
+ return $imul(0xffffffff, 5) != -5 || $imul.length != 2;
+ }), 'Math', {
+ imul: function imul(x, y){
+ var UINT16 = 0xffff
+ , xn = +x
+ , yn = +y
+ , xl = UINT16 & xn
+ , yl = UINT16 & yn;
+ return 0 | xl * yl + ((UINT16 & xn >>> 16) * yl + xl * (UINT16 & yn >>> 16) << 16 >>> 0);
+ }
+ });
+
+/***/ },
+/* 87 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.21 Math.log10(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {
+ log10: function log10(x){
+ return Math.log(x) / Math.LN10;
+ }
+ });
+
+/***/ },
+/* 88 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.20 Math.log1p(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {log1p: __webpack_require__(75)});
+
+/***/ },
+/* 89 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.22 Math.log2(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {
+ log2: function log2(x){
+ return Math.log(x) / Math.LN2;
+ }
+ });
+
+/***/ },
+/* 90 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.28 Math.sign(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {sign: __webpack_require__(79)});
+
+/***/ },
+/* 91 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.30 Math.sinh(x)
+ var $export = __webpack_require__(3)
+ , expm1 = __webpack_require__(83)
+ , exp = Math.exp;
+
+ // V8 near Chromium 38 has a problem with very small numbers
+ $export($export.S + $export.F * __webpack_require__(9)(function(){
+ return !Math.sinh(-2e-17) != -2e-17;
+ }), 'Math', {
+ sinh: function sinh(x){
+ return Math.abs(x = +x) < 1
+ ? (expm1(x) - expm1(-x)) / 2
+ : (exp(x - 1) - exp(-x - 1)) * (Math.E / 2);
+ }
+ });
+
+/***/ },
+/* 92 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.33 Math.tanh(x)
+ var $export = __webpack_require__(3)
+ , expm1 = __webpack_require__(83)
+ , exp = Math.exp;
+
+ $export($export.S, 'Math', {
+ tanh: function tanh(x){
+ var a = expm1(x = +x)
+ , b = expm1(-x);
+ return a == Infinity ? 1 : b == Infinity ? -1 : (a - b) / (exp(x) + exp(-x));
+ }
+ });
+
+/***/ },
+/* 93 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.34 Math.trunc(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {
+ trunc: function trunc(it){
+ return (it > 0 ? Math.floor : Math.ceil)(it);
+ }
+ });
+
+/***/ },
+/* 94 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3)
+ , toIndex = __webpack_require__(26)
+ , fromCharCode = String.fromCharCode
+ , $fromCodePoint = String.fromCodePoint;
+
+ // length should be 1, old FF problem
+ $export($export.S + $export.F * (!!$fromCodePoint && $fromCodePoint.length != 1), 'String', {
+ // 21.1.2.2 String.fromCodePoint(...codePoints)
+ fromCodePoint: function fromCodePoint(x){ // eslint-disable-line no-unused-vars
+ var res = []
+ , $$ = arguments
+ , $$len = $$.length
+ , i = 0
+ , code;
+ while($$len > i){
+ code = +$$[i++];
+ if(toIndex(code, 0x10ffff) !== code)throw RangeError(code + ' is not a valid code point');
+ res.push(code < 0x10000
+ ? fromCharCode(code)
+ : fromCharCode(((code -= 0x10000) >> 10) + 0xd800, code % 0x400 + 0xdc00)
+ );
+ } return res.join('');
+ }
+ });
+
+/***/ },
+/* 95 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3)
+ , toIObject = __webpack_require__(23)
+ , toLength = __webpack_require__(27);
+
+ $export($export.S, 'String', {
+ // 21.1.2.4 String.raw(callSite, ...substitutions)
+ raw: function raw(callSite){
+ var tpl = toIObject(callSite.raw)
+ , len = toLength(tpl.length)
+ , $$ = arguments
+ , $$len = $$.length
+ , res = []
+ , i = 0;
+ while(len > i){
+ res.push(String(tpl[i++]));
+ if(i < $$len)res.push(String($$[i]));
+ } return res.join('');
+ }
+ });
+
+/***/ },
+/* 96 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // 21.1.3.25 String.prototype.trim()
+ __webpack_require__(63)('trim', function($trim){
+ return function trim(){
+ return $trim(this, 3);
+ };
+ });
+
+/***/ },
+/* 97 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , $at = __webpack_require__(98)(false);
+ $export($export.P, 'String', {
+ // 21.1.3.3 String.prototype.codePointAt(pos)
+ codePointAt: function codePointAt(pos){
+ return $at(this, pos);
+ }
+ });
+
+/***/ },
+/* 98 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var toInteger = __webpack_require__(25)
+ , defined = __webpack_require__(22);
+ // true -> String#at
+ // false -> String#codePointAt
+ module.exports = function(TO_STRING){
+ return function(that, pos){
+ var s = String(defined(that))
+ , i = toInteger(pos)
+ , l = s.length
+ , a, b;
+ if(i < 0 || i >= l)return TO_STRING ? '' : undefined;
+ a = s.charCodeAt(i);
+ return a < 0xd800 || a > 0xdbff || i + 1 === l || (b = s.charCodeAt(i + 1)) < 0xdc00 || b > 0xdfff
+ ? TO_STRING ? s.charAt(i) : a
+ : TO_STRING ? s.slice(i, i + 2) : (a - 0xd800 << 10) + (b - 0xdc00) + 0x10000;
+ };
+ };
+
+/***/ },
+/* 99 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 21.1.3.6 String.prototype.endsWith(searchString [, endPosition])
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , toLength = __webpack_require__(27)
+ , context = __webpack_require__(100)
+ , ENDS_WITH = 'endsWith'
+ , $endsWith = ''[ENDS_WITH];
+
+ $export($export.P + $export.F * __webpack_require__(102)(ENDS_WITH), 'String', {
+ endsWith: function endsWith(searchString /*, endPosition = @length */){
+ var that = context(this, searchString, ENDS_WITH)
+ , $$ = arguments
+ , endPosition = $$.length > 1 ? $$[1] : undefined
+ , len = toLength(that.length)
+ , end = endPosition === undefined ? len : Math.min(toLength(endPosition), len)
+ , search = String(searchString);
+ return $endsWith
+ ? $endsWith.call(that, search, end)
+ : that.slice(end - search.length, end) === search;
+ }
+ });
+
+/***/ },
+/* 100 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // helper for String#{startsWith, endsWith, includes}
+ var isRegExp = __webpack_require__(101)
+ , defined = __webpack_require__(22);
+
+ module.exports = function(that, searchString, NAME){
+ if(isRegExp(searchString))throw TypeError('String#' + NAME + " doesn't accept regex!");
+ return String(defined(that));
+ };
+
+/***/ },
+/* 101 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 7.2.8 IsRegExp(argument)
+ var isObject = __webpack_require__(16)
+ , cof = __webpack_require__(18)
+ , MATCH = __webpack_require__(31)('match');
+ module.exports = function(it){
+ var isRegExp;
+ return isObject(it) && ((isRegExp = it[MATCH]) !== undefined ? !!isRegExp : cof(it) == 'RegExp');
+ };
+
+/***/ },
+/* 102 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var MATCH = __webpack_require__(31)('match');
+ module.exports = function(KEY){
+ var re = /./;
+ try {
+ '/./'[KEY](re);
+ } catch(e){
+ try {
+ re[MATCH] = false;
+ return !'/./'[KEY](re);
+ } catch(f){ /* empty */ }
+ } return true;
+ };
+
+/***/ },
+/* 103 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 21.1.3.7 String.prototype.includes(searchString, position = 0)
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , context = __webpack_require__(100)
+ , INCLUDES = 'includes';
+
+ $export($export.P + $export.F * __webpack_require__(102)(INCLUDES), 'String', {
+ includes: function includes(searchString /*, position = 0 */){
+ return !!~context(this, searchString, INCLUDES)
+ .indexOf(searchString, arguments.length > 1 ? arguments[1] : undefined);
+ }
+ });
+
+/***/ },
+/* 104 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3);
+
+ $export($export.P, 'String', {
+ // 21.1.3.13 String.prototype.repeat(count)
+ repeat: __webpack_require__(105)
+ });
+
+/***/ },
+/* 105 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var toInteger = __webpack_require__(25)
+ , defined = __webpack_require__(22);
+
+ module.exports = function repeat(count){
+ var str = String(defined(this))
+ , res = ''
+ , n = toInteger(count);
+ if(n < 0 || n == Infinity)throw RangeError("Count can't be negative");
+ for(;n > 0; (n >>>= 1) && (str += str))if(n & 1)res += str;
+ return res;
+ };
+
+/***/ },
+/* 106 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 21.1.3.18 String.prototype.startsWith(searchString [, position ])
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , toLength = __webpack_require__(27)
+ , context = __webpack_require__(100)
+ , STARTS_WITH = 'startsWith'
+ , $startsWith = ''[STARTS_WITH];
+
+ $export($export.P + $export.F * __webpack_require__(102)(STARTS_WITH), 'String', {
+ startsWith: function startsWith(searchString /*, position = 0 */){
+ var that = context(this, searchString, STARTS_WITH)
+ , $$ = arguments
+ , index = toLength(Math.min($$.length > 1 ? $$[1] : undefined, that.length))
+ , search = String(searchString);
+ return $startsWith
+ ? $startsWith.call(that, search, index)
+ : that.slice(index, index + search.length) === search;
+ }
+ });
+
+/***/ },
+/* 107 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $at = __webpack_require__(98)(true);
+
+ // 21.1.3.27 String.prototype[@@iterator]()
+ __webpack_require__(108)(String, 'String', function(iterated){
+ this._t = String(iterated); // target
+ this._i = 0; // next index
+ // 21.1.5.2.1 %StringIteratorPrototype%.next()
+ }, function(){
+ var O = this._t
+ , index = this._i
+ , point;
+ if(index >= O.length)return {value: undefined, done: true};
+ point = $at(O, index);
+ this._i += point.length;
+ return {value: point, done: false};
+ });
+
+/***/ },
+/* 108 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var LIBRARY = __webpack_require__(39)
+ , $export = __webpack_require__(3)
+ , redefine = __webpack_require__(10)
+ , hide = __webpack_require__(6)
+ , has = __webpack_require__(17)
+ , Iterators = __webpack_require__(109)
+ , $iterCreate = __webpack_require__(110)
+ , setToStringTag = __webpack_require__(35)
+ , getProto = __webpack_require__(2).getProto
+ , ITERATOR = __webpack_require__(31)('iterator')
+ , BUGGY = !([].keys && 'next' in [].keys()) // Safari has buggy iterators w/o `next`
+ , FF_ITERATOR = '@@iterator'
+ , KEYS = 'keys'
+ , VALUES = 'values';
+
+ var returnThis = function(){ return this; };
+
+ module.exports = function(Base, NAME, Constructor, next, DEFAULT, IS_SET, FORCED){
+ $iterCreate(Constructor, NAME, next);
+ var getMethod = function(kind){
+ if(!BUGGY && kind in proto)return proto[kind];
+ switch(kind){
+ case KEYS: return function keys(){ return new Constructor(this, kind); };
+ case VALUES: return function values(){ return new Constructor(this, kind); };
+ } return function entries(){ return new Constructor(this, kind); };
+ };
+ var TAG = NAME + ' Iterator'
+ , DEF_VALUES = DEFAULT == VALUES
+ , VALUES_BUG = false
+ , proto = Base.prototype
+ , $native = proto[ITERATOR] || proto[FF_ITERATOR] || DEFAULT && proto[DEFAULT]
+ , $default = $native || getMethod(DEFAULT)
+ , methods, key;
+ // Fix native
+ if($native){
+ var IteratorPrototype = getProto($default.call(new Base));
+ // Set @@toStringTag to native iterators
+ setToStringTag(IteratorPrototype, TAG, true);
+ // FF fix
+ if(!LIBRARY && has(proto, FF_ITERATOR))hide(IteratorPrototype, ITERATOR, returnThis);
+ // fix Array#{values, @@iterator}.name in V8 / FF
+ if(DEF_VALUES && $native.name !== VALUES){
+ VALUES_BUG = true;
+ $default = function values(){ return $native.call(this); };
+ }
+ }
+ // Define iterator
+ if((!LIBRARY || FORCED) && (BUGGY || VALUES_BUG || !proto[ITERATOR])){
+ hide(proto, ITERATOR, $default);
+ }
+ // Plug for library
+ Iterators[NAME] = $default;
+ Iterators[TAG] = returnThis;
+ if(DEFAULT){
+ methods = {
+ values: DEF_VALUES ? $default : getMethod(VALUES),
+ keys: IS_SET ? $default : getMethod(KEYS),
+ entries: !DEF_VALUES ? $default : getMethod('entries')
+ };
+ if(FORCED)for(key in methods){
+ if(!(key in proto))redefine(proto, key, methods[key]);
+ } else $export($export.P + $export.F * (BUGGY || VALUES_BUG), NAME, methods);
+ }
+ return methods;
+ };
+
+/***/ },
+/* 109 */
+/***/ function(module, exports) {
+
+ module.exports = {};
+
+/***/ },
+/* 110 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , descriptor = __webpack_require__(7)
+ , setToStringTag = __webpack_require__(35)
+ , IteratorPrototype = {};
+
+ // 25.1.2.1.1 %IteratorPrototype%[@@iterator]()
+ __webpack_require__(6)(IteratorPrototype, __webpack_require__(31)('iterator'), function(){ return this; });
+
+ module.exports = function(Constructor, NAME, next){
+ Constructor.prototype = $.create(IteratorPrototype, {next: descriptor(1, next)});
+ setToStringTag(Constructor, NAME + ' Iterator');
+ };
+
+/***/ },
+/* 111 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var ctx = __webpack_require__(12)
+ , $export = __webpack_require__(3)
+ , toObject = __webpack_require__(21)
+ , call = __webpack_require__(112)
+ , isArrayIter = __webpack_require__(113)
+ , toLength = __webpack_require__(27)
+ , getIterFn = __webpack_require__(114);
+ $export($export.S + $export.F * !__webpack_require__(115)(function(iter){ Array.from(iter); }), 'Array', {
+ // 22.1.2.1 Array.from(arrayLike, mapfn = undefined, thisArg = undefined)
+ from: function from(arrayLike/*, mapfn = undefined, thisArg = undefined*/){
+ var O = toObject(arrayLike)
+ , C = typeof this == 'function' ? this : Array
+ , $$ = arguments
+ , $$len = $$.length
+ , mapfn = $$len > 1 ? $$[1] : undefined
+ , mapping = mapfn !== undefined
+ , index = 0
+ , iterFn = getIterFn(O)
+ , length, result, step, iterator;
+ if(mapping)mapfn = ctx(mapfn, $$len > 2 ? $$[2] : undefined, 2);
+ // if object isn't iterable or it's array with default iterator - use simple case
+ if(iterFn != undefined && !(C == Array && isArrayIter(iterFn))){
+ for(iterator = iterFn.call(O), result = new C; !(step = iterator.next()).done; index++){
+ result[index] = mapping ? call(iterator, mapfn, [step.value, index], true) : step.value;
+ }
+ } else {
+ length = toLength(O.length);
+ for(result = new C(length); length > index; index++){
+ result[index] = mapping ? mapfn(O[index], index) : O[index];
+ }
+ }
+ result.length = index;
+ return result;
+ }
+ });
+
+
+/***/ },
+/* 112 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // call something on iterator step with safe closing on error
+ var anObject = __webpack_require__(20);
+ module.exports = function(iterator, fn, value, entries){
+ try {
+ return entries ? fn(anObject(value)[0], value[1]) : fn(value);
+ // 7.4.6 IteratorClose(iterator, completion)
+ } catch(e){
+ var ret = iterator['return'];
+ if(ret !== undefined)anObject(ret.call(iterator));
+ throw e;
+ }
+ };
+
+/***/ },
+/* 113 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // check on default Array iterator
+ var Iterators = __webpack_require__(109)
+ , ITERATOR = __webpack_require__(31)('iterator')
+ , ArrayProto = Array.prototype;
+
+ module.exports = function(it){
+ return it !== undefined && (Iterators.Array === it || ArrayProto[ITERATOR] === it);
+ };
+
+/***/ },
+/* 114 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var classof = __webpack_require__(47)
+ , ITERATOR = __webpack_require__(31)('iterator')
+ , Iterators = __webpack_require__(109);
+ module.exports = __webpack_require__(5).getIteratorMethod = function(it){
+ if(it != undefined)return it[ITERATOR]
+ || it['@@iterator']
+ || Iterators[classof(it)];
+ };
+
+/***/ },
+/* 115 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var ITERATOR = __webpack_require__(31)('iterator')
+ , SAFE_CLOSING = false;
+
+ try {
+ var riter = [7][ITERATOR]();
+ riter['return'] = function(){ SAFE_CLOSING = true; };
+ Array.from(riter, function(){ throw 2; });
+ } catch(e){ /* empty */ }
+
+ module.exports = function(exec, skipClosing){
+ if(!skipClosing && !SAFE_CLOSING)return false;
+ var safe = false;
+ try {
+ var arr = [7]
+ , iter = arr[ITERATOR]();
+ iter.next = function(){ return {done: safe = true}; };
+ arr[ITERATOR] = function(){ return iter; };
+ exec(arr);
+ } catch(e){ /* empty */ }
+ return safe;
+ };
+
+/***/ },
+/* 116 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $export = __webpack_require__(3);
+
+ // WebKit Array.of isn't generic
+ $export($export.S + $export.F * __webpack_require__(9)(function(){
+ function F(){}
+ return !(Array.of.call(F) instanceof F);
+ }), 'Array', {
+ // 22.1.2.3 Array.of( ...items)
+ of: function of(/* ...args */){
+ var index = 0
+ , $$ = arguments
+ , $$len = $$.length
+ , result = new (typeof this == 'function' ? this : Array)($$len);
+ while($$len > index)result[index] = $$[index++];
+ result.length = $$len;
+ return result;
+ }
+ });
+
+/***/ },
+/* 117 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var addToUnscopables = __webpack_require__(118)
+ , step = __webpack_require__(119)
+ , Iterators = __webpack_require__(109)
+ , toIObject = __webpack_require__(23);
+
+ // 22.1.3.4 Array.prototype.entries()
+ // 22.1.3.13 Array.prototype.keys()
+ // 22.1.3.29 Array.prototype.values()
+ // 22.1.3.30 Array.prototype[@@iterator]()
+ module.exports = __webpack_require__(108)(Array, 'Array', function(iterated, kind){
+ this._t = toIObject(iterated); // target
+ this._i = 0; // next index
+ this._k = kind; // kind
+ // 22.1.5.2.1 %ArrayIteratorPrototype%.next()
+ }, function(){
+ var O = this._t
+ , kind = this._k
+ , index = this._i++;
+ if(!O || index >= O.length){
+ this._t = undefined;
+ return step(1);
+ }
+ if(kind == 'keys' )return step(0, index);
+ if(kind == 'values')return step(0, O[index]);
+ return step(0, [index, O[index]]);
+ }, 'values');
+
+ // argumentsList[@@iterator] is %ArrayProto_values% (9.4.4.6, 9.4.4.7)
+ Iterators.Arguments = Iterators.Array;
+
+ addToUnscopables('keys');
+ addToUnscopables('values');
+ addToUnscopables('entries');
+
+/***/ },
+/* 118 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 22.1.3.31 Array.prototype[@@unscopables]
+ var UNSCOPABLES = __webpack_require__(31)('unscopables')
+ , ArrayProto = Array.prototype;
+ if(ArrayProto[UNSCOPABLES] == undefined)__webpack_require__(6)(ArrayProto, UNSCOPABLES, {});
+ module.exports = function(key){
+ ArrayProto[UNSCOPABLES][key] = true;
+ };
+
+/***/ },
+/* 119 */
+/***/ function(module, exports) {
+
+ module.exports = function(done, value){
+ return {value: value, done: !!done};
+ };
+
+/***/ },
+/* 120 */
+/***/ function(module, exports, __webpack_require__) {
+
+ __webpack_require__(121)('Array');
+
+/***/ },
+/* 121 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var global = __webpack_require__(4)
+ , $ = __webpack_require__(2)
+ , DESCRIPTORS = __webpack_require__(8)
+ , SPECIES = __webpack_require__(31)('species');
+
+ module.exports = function(KEY){
+ var C = global[KEY];
+ if(DESCRIPTORS && C && !C[SPECIES])$.setDesc(C, SPECIES, {
+ configurable: true,
+ get: function(){ return this; }
+ });
+ };
+
+/***/ },
+/* 122 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 22.1.3.3 Array.prototype.copyWithin(target, start, end = this.length)
+ var $export = __webpack_require__(3);
+
+ $export($export.P, 'Array', {copyWithin: __webpack_require__(123)});
+
+ __webpack_require__(118)('copyWithin');
+
+/***/ },
+/* 123 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 22.1.3.3 Array.prototype.copyWithin(target, start, end = this.length)
+ 'use strict';
+ var toObject = __webpack_require__(21)
+ , toIndex = __webpack_require__(26)
+ , toLength = __webpack_require__(27);
+
+ module.exports = [].copyWithin || function copyWithin(target/*= 0*/, start/*= 0, end = @length*/){
+ var O = toObject(this)
+ , len = toLength(O.length)
+ , to = toIndex(target, len)
+ , from = toIndex(start, len)
+ , $$ = arguments
+ , end = $$.length > 2 ? $$[2] : undefined
+ , count = Math.min((end === undefined ? len : toIndex(end, len)) - from, len - to)
+ , inc = 1;
+ if(from < to && to < from + count){
+ inc = -1;
+ from += count - 1;
+ to += count - 1;
+ }
+ while(count-- > 0){
+ if(from in O)O[to] = O[from];
+ else delete O[to];
+ to += inc;
+ from += inc;
+ } return O;
+ };
+
+/***/ },
+/* 124 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 22.1.3.6 Array.prototype.fill(value, start = 0, end = this.length)
+ var $export = __webpack_require__(3);
+
+ $export($export.P, 'Array', {fill: __webpack_require__(125)});
+
+ __webpack_require__(118)('fill');
+
+/***/ },
+/* 125 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 22.1.3.6 Array.prototype.fill(value, start = 0, end = this.length)
+ 'use strict';
+ var toObject = __webpack_require__(21)
+ , toIndex = __webpack_require__(26)
+ , toLength = __webpack_require__(27);
+ module.exports = [].fill || function fill(value /*, start = 0, end = @length */){
+ var O = toObject(this)
+ , length = toLength(O.length)
+ , $$ = arguments
+ , $$len = $$.length
+ , index = toIndex($$len > 1 ? $$[1] : undefined, length)
+ , end = $$len > 2 ? $$[2] : undefined
+ , endPos = end === undefined ? length : toIndex(end, length);
+ while(endPos > index)O[index++] = value;
+ return O;
+ };
+
+/***/ },
+/* 126 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // 22.1.3.8 Array.prototype.find(predicate, thisArg = undefined)
+ var $export = __webpack_require__(3)
+ , $find = __webpack_require__(28)(5)
+ , KEY = 'find'
+ , forced = true;
+ // Shouldn't skip holes
+ if(KEY in [])Array(1)[KEY](function(){ forced = false; });
+ $export($export.P + $export.F * forced, 'Array', {
+ find: function find(callbackfn/*, that = undefined */){
+ return $find(this, callbackfn, arguments.length > 1 ? arguments[1] : undefined);
+ }
+ });
+ __webpack_require__(118)(KEY);
+
+/***/ },
+/* 127 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // 22.1.3.9 Array.prototype.findIndex(predicate, thisArg = undefined)
+ var $export = __webpack_require__(3)
+ , $find = __webpack_require__(28)(6)
+ , KEY = 'findIndex'
+ , forced = true;
+ // Shouldn't skip holes
+ if(KEY in [])Array(1)[KEY](function(){ forced = false; });
+ $export($export.P + $export.F * forced, 'Array', {
+ findIndex: function findIndex(callbackfn/*, that = undefined */){
+ return $find(this, callbackfn, arguments.length > 1 ? arguments[1] : undefined);
+ }
+ });
+ __webpack_require__(118)(KEY);
+
+/***/ },
+/* 128 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $ = __webpack_require__(2)
+ , global = __webpack_require__(4)
+ , isRegExp = __webpack_require__(101)
+ , $flags = __webpack_require__(129)
+ , $RegExp = global.RegExp
+ , Base = $RegExp
+ , proto = $RegExp.prototype
+ , re1 = /a/g
+ , re2 = /a/g
+ // "new" creates a new object, old webkit buggy here
+ , CORRECT_NEW = new $RegExp(re1) !== re1;
+
+ if(__webpack_require__(8) && (!CORRECT_NEW || __webpack_require__(9)(function(){
+ re2[__webpack_require__(31)('match')] = false;
+ // RegExp constructor can alter flags and IsRegExp works correct with @@match
+ return $RegExp(re1) != re1 || $RegExp(re2) == re2 || $RegExp(re1, 'i') != '/a/i';
+ }))){
+ $RegExp = function RegExp(p, f){
+ var piRE = isRegExp(p)
+ , fiU = f === undefined;
+ return !(this instanceof $RegExp) && piRE && p.constructor === $RegExp && fiU ? p
+ : CORRECT_NEW
+ ? new Base(piRE && !fiU ? p.source : p, f)
+ : Base((piRE = p instanceof $RegExp) ? p.source : p, piRE && fiU ? $flags.call(p) : f);
+ };
+ $.each.call($.getNames(Base), function(key){
+ key in $RegExp || $.setDesc($RegExp, key, {
+ configurable: true,
+ get: function(){ return Base[key]; },
+ set: function(it){ Base[key] = it; }
+ });
+ });
+ proto.constructor = $RegExp;
+ $RegExp.prototype = proto;
+ __webpack_require__(10)(global, 'RegExp', $RegExp);
+ }
+
+ __webpack_require__(121)('RegExp');
+
+/***/ },
+/* 129 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // 21.2.5.3 get RegExp.prototype.flags
+ var anObject = __webpack_require__(20);
+ module.exports = function(){
+ var that = anObject(this)
+ , result = '';
+ if(that.global) result += 'g';
+ if(that.ignoreCase) result += 'i';
+ if(that.multiline) result += 'm';
+ if(that.unicode) result += 'u';
+ if(that.sticky) result += 'y';
+ return result;
+ };
+
+/***/ },
+/* 130 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 21.2.5.3 get RegExp.prototype.flags()
+ var $ = __webpack_require__(2);
+ if(__webpack_require__(8) && /./g.flags != 'g')$.setDesc(RegExp.prototype, 'flags', {
+ configurable: true,
+ get: __webpack_require__(129)
+ });
+
+/***/ },
+/* 131 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // @@match logic
+ __webpack_require__(132)('match', 1, function(defined, MATCH){
+ // 21.1.3.11 String.prototype.match(regexp)
+ return function match(regexp){
+ 'use strict';
+ var O = defined(this)
+ , fn = regexp == undefined ? undefined : regexp[MATCH];
+ return fn !== undefined ? fn.call(regexp, O) : new RegExp(regexp)[MATCH](String(O));
+ };
+ });
+
+/***/ },
+/* 132 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var hide = __webpack_require__(6)
+ , redefine = __webpack_require__(10)
+ , fails = __webpack_require__(9)
+ , defined = __webpack_require__(22)
+ , wks = __webpack_require__(31);
+
+ module.exports = function(KEY, length, exec){
+ var SYMBOL = wks(KEY)
+ , original = ''[KEY];
+ if(fails(function(){
+ var O = {};
+ O[SYMBOL] = function(){ return 7; };
+ return ''[KEY](O) != 7;
+ })){
+ redefine(String.prototype, KEY, exec(defined, SYMBOL, original));
+ hide(RegExp.prototype, SYMBOL, length == 2
+ // 21.2.5.8 RegExp.prototype[@@replace](string, replaceValue)
+ // 21.2.5.11 RegExp.prototype[@@split](string, limit)
+ ? function(string, arg){ return original.call(string, this, arg); }
+ // 21.2.5.6 RegExp.prototype[@@match](string)
+ // 21.2.5.9 RegExp.prototype[@@search](string)
+ : function(string){ return original.call(string, this); }
+ );
+ }
+ };
+
+/***/ },
+/* 133 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // @@replace logic
+ __webpack_require__(132)('replace', 2, function(defined, REPLACE, $replace){
+ // 21.1.3.14 String.prototype.replace(searchValue, replaceValue)
+ return function replace(searchValue, replaceValue){
+ 'use strict';
+ var O = defined(this)
+ , fn = searchValue == undefined ? undefined : searchValue[REPLACE];
+ return fn !== undefined
+ ? fn.call(searchValue, O, replaceValue)
+ : $replace.call(String(O), searchValue, replaceValue);
+ };
+ });
+
+/***/ },
+/* 134 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // @@search logic
+ __webpack_require__(132)('search', 1, function(defined, SEARCH){
+ // 21.1.3.15 String.prototype.search(regexp)
+ return function search(regexp){
+ 'use strict';
+ var O = defined(this)
+ , fn = regexp == undefined ? undefined : regexp[SEARCH];
+ return fn !== undefined ? fn.call(regexp, O) : new RegExp(regexp)[SEARCH](String(O));
+ };
+ });
+
+/***/ },
+/* 135 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // @@split logic
+ __webpack_require__(132)('split', 2, function(defined, SPLIT, $split){
+ // 21.1.3.17 String.prototype.split(separator, limit)
+ return function split(separator, limit){
+ 'use strict';
+ var O = defined(this)
+ , fn = separator == undefined ? undefined : separator[SPLIT];
+ return fn !== undefined
+ ? fn.call(separator, O, limit)
+ : $split.call(String(O), separator, limit);
+ };
+ });
+
+/***/ },
+/* 136 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , LIBRARY = __webpack_require__(39)
+ , global = __webpack_require__(4)
+ , ctx = __webpack_require__(12)
+ , classof = __webpack_require__(47)
+ , $export = __webpack_require__(3)
+ , isObject = __webpack_require__(16)
+ , anObject = __webpack_require__(20)
+ , aFunction = __webpack_require__(13)
+ , strictNew = __webpack_require__(137)
+ , forOf = __webpack_require__(138)
+ , setProto = __webpack_require__(45).set
+ , same = __webpack_require__(43)
+ , SPECIES = __webpack_require__(31)('species')
+ , speciesConstructor = __webpack_require__(139)
+ , asap = __webpack_require__(140)
+ , PROMISE = 'Promise'
+ , process = global.process
+ , isNode = classof(process) == 'process'
+ , P = global[PROMISE]
+ , empty = function(){ /* empty */ }
+ , Wrapper;
+
+ var testResolve = function(sub){
+ var test = new P(empty), promise;
+ if(sub)test.constructor = function(exec){
+ exec(empty, empty);
+ };
+ (promise = P.resolve(test))['catch'](empty);
+ return promise === test;
+ };
+
+ var USE_NATIVE = function(){
+ var works = false;
+ function P2(x){
+ var self = new P(x);
+ setProto(self, P2.prototype);
+ return self;
+ }
+ try {
+ works = P && P.resolve && testResolve();
+ setProto(P2, P);
+ P2.prototype = $.create(P.prototype, {constructor: {value: P2}});
+ // actual Firefox has broken subclass support, test that
+ if(!(P2.resolve(5).then(function(){}) instanceof P2)){
+ works = false;
+ }
+ // actual V8 bug, https://code.google.com/p/v8/issues/detail?id=4162
+ if(works && __webpack_require__(8)){
+ var thenableThenGotten = false;
+ P.resolve($.setDesc({}, 'then', {
+ get: function(){ thenableThenGotten = true; }
+ }));
+ works = thenableThenGotten;
+ }
+ } catch(e){ works = false; }
+ return works;
+ }();
+
+ // helpers
+ var sameConstructor = function(a, b){
+ // library wrapper special case
+ if(LIBRARY && a === P && b === Wrapper)return true;
+ return same(a, b);
+ };
+ var getConstructor = function(C){
+ var S = anObject(C)[SPECIES];
+ return S != undefined ? S : C;
+ };
+ var isThenable = function(it){
+ var then;
+ return isObject(it) && typeof (then = it.then) == 'function' ? then : false;
+ };
+ var PromiseCapability = function(C){
+ var resolve, reject;
+ this.promise = new C(function($$resolve, $$reject){
+ if(resolve !== undefined || reject !== undefined)throw TypeError('Bad Promise constructor');
+ resolve = $$resolve;
+ reject = $$reject;
+ });
+ this.resolve = aFunction(resolve),
+ this.reject = aFunction(reject)
+ };
+ var perform = function(exec){
+ try {
+ exec();
+ } catch(e){
+ return {error: e};
+ }
+ };
+ var notify = function(record, isReject){
+ if(record.n)return;
+ record.n = true;
+ var chain = record.c;
+ asap(function(){
+ var value = record.v
+ , ok = record.s == 1
+ , i = 0;
+ var run = function(reaction){
+ var handler = ok ? reaction.ok : reaction.fail
+ , resolve = reaction.resolve
+ , reject = reaction.reject
+ , result, then;
+ try {
+ if(handler){
+ if(!ok)record.h = true;
+ result = handler === true ? value : handler(value);
+ if(result === reaction.promise){
+ reject(TypeError('Promise-chain cycle'));
+ } else if(then = isThenable(result)){
+ then.call(result, resolve, reject);
+ } else resolve(result);
+ } else reject(value);
+ } catch(e){
+ reject(e);
+ }
+ };
+ while(chain.length > i)run(chain[i++]); // variable length - can't use forEach
+ chain.length = 0;
+ record.n = false;
+ if(isReject)setTimeout(function(){
+ var promise = record.p
+ , handler, console;
+ if(isUnhandled(promise)){
+ if(isNode){
+ process.emit('unhandledRejection', value, promise);
+ } else if(handler = global.onunhandledrejection){
+ handler({promise: promise, reason: value});
+ } else if((console = global.console) && console.error){
+ console.error('Unhandled promise rejection', value);
+ }
+ } record.a = undefined;
+ }, 1);
+ });
+ };
+ var isUnhandled = function(promise){
+ var record = promise._d
+ , chain = record.a || record.c
+ , i = 0
+ , reaction;
+ if(record.h)return false;
+ while(chain.length > i){
+ reaction = chain[i++];
+ if(reaction.fail || !isUnhandled(reaction.promise))return false;
+ } return true;
+ };
+ var $reject = function(value){
+ var record = this;
+ if(record.d)return;
+ record.d = true;
+ record = record.r || record; // unwrap
+ record.v = value;
+ record.s = 2;
+ record.a = record.c.slice();
+ notify(record, true);
+ };
+ var $resolve = function(value){
+ var record = this
+ , then;
+ if(record.d)return;
+ record.d = true;
+ record = record.r || record; // unwrap
+ try {
+ if(record.p === value)throw TypeError("Promise can't be resolved itself");
+ if(then = isThenable(value)){
+ asap(function(){
+ var wrapper = {r: record, d: false}; // wrap
+ try {
+ then.call(value, ctx($resolve, wrapper, 1), ctx($reject, wrapper, 1));
+ } catch(e){
+ $reject.call(wrapper, e);
+ }
+ });
+ } else {
+ record.v = value;
+ record.s = 1;
+ notify(record, false);
+ }
+ } catch(e){
+ $reject.call({r: record, d: false}, e); // wrap
+ }
+ };
+
+ // constructor polyfill
+ if(!USE_NATIVE){
+ // 25.4.3.1 Promise(executor)
+ P = function Promise(executor){
+ aFunction(executor);
+ var record = this._d = {
+ p: strictNew(this, P, PROMISE), // <- promise
+ c: [], // <- awaiting reactions
+ a: undefined, // <- checked in isUnhandled reactions
+ s: 0, // <- state
+ d: false, // <- done
+ v: undefined, // <- value
+ h: false, // <- handled rejection
+ n: false // <- notify
+ };
+ try {
+ executor(ctx($resolve, record, 1), ctx($reject, record, 1));
+ } catch(err){
+ $reject.call(record, err);
+ }
+ };
+ __webpack_require__(142)(P.prototype, {
+ // 25.4.5.3 Promise.prototype.then(onFulfilled, onRejected)
+ then: function then(onFulfilled, onRejected){
+ var reaction = new PromiseCapability(speciesConstructor(this, P))
+ , promise = reaction.promise
+ , record = this._d;
+ reaction.ok = typeof onFulfilled == 'function' ? onFulfilled : true;
+ reaction.fail = typeof onRejected == 'function' && onRejected;
+ record.c.push(reaction);
+ if(record.a)record.a.push(reaction);
+ if(record.s)notify(record, false);
+ return promise;
+ },
+ // 25.4.5.1 Promise.prototype.catch(onRejected)
+ 'catch': function(onRejected){
+ return this.then(undefined, onRejected);
+ }
+ });
+ }
+
+ $export($export.G + $export.W + $export.F * !USE_NATIVE, {Promise: P});
+ __webpack_require__(35)(P, PROMISE);
+ __webpack_require__(121)(PROMISE);
+ Wrapper = __webpack_require__(5)[PROMISE];
+
+ // statics
+ $export($export.S + $export.F * !USE_NATIVE, PROMISE, {
+ // 25.4.4.5 Promise.reject(r)
+ reject: function reject(r){
+ var capability = new PromiseCapability(this)
+ , $$reject = capability.reject;
+ $$reject(r);
+ return capability.promise;
+ }
+ });
+ $export($export.S + $export.F * (!USE_NATIVE || testResolve(true)), PROMISE, {
+ // 25.4.4.6 Promise.resolve(x)
+ resolve: function resolve(x){
+ // instanceof instead of internal slot check because we should fix it without replacement native Promise core
+ if(x instanceof P && sameConstructor(x.constructor, this))return x;
+ var capability = new PromiseCapability(this)
+ , $$resolve = capability.resolve;
+ $$resolve(x);
+ return capability.promise;
+ }
+ });
+ $export($export.S + $export.F * !(USE_NATIVE && __webpack_require__(115)(function(iter){
+ P.all(iter)['catch'](function(){});
+ })), PROMISE, {
+ // 25.4.4.1 Promise.all(iterable)
+ all: function all(iterable){
+ var C = getConstructor(this)
+ , capability = new PromiseCapability(C)
+ , resolve = capability.resolve
+ , reject = capability.reject
+ , values = [];
+ var abrupt = perform(function(){
+ forOf(iterable, false, values.push, values);
+ var remaining = values.length
+ , results = Array(remaining);
+ if(remaining)$.each.call(values, function(promise, index){
+ var alreadyCalled = false;
+ C.resolve(promise).then(function(value){
+ if(alreadyCalled)return;
+ alreadyCalled = true;
+ results[index] = value;
+ --remaining || resolve(results);
+ }, reject);
+ });
+ else resolve(results);
+ });
+ if(abrupt)reject(abrupt.error);
+ return capability.promise;
+ },
+ // 25.4.4.4 Promise.race(iterable)
+ race: function race(iterable){
+ var C = getConstructor(this)
+ , capability = new PromiseCapability(C)
+ , reject = capability.reject;
+ var abrupt = perform(function(){
+ forOf(iterable, false, function(promise){
+ C.resolve(promise).then(capability.resolve, reject);
+ });
+ });
+ if(abrupt)reject(abrupt.error);
+ return capability.promise;
+ }
+ });
+
+/***/ },
+/* 137 */
+/***/ function(module, exports) {
+
+ module.exports = function(it, Constructor, name){
+ if(!(it instanceof Constructor))throw TypeError(name + ": use the 'new' operator!");
+ return it;
+ };
+
+/***/ },
+/* 138 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var ctx = __webpack_require__(12)
+ , call = __webpack_require__(112)
+ , isArrayIter = __webpack_require__(113)
+ , anObject = __webpack_require__(20)
+ , toLength = __webpack_require__(27)
+ , getIterFn = __webpack_require__(114);
+ module.exports = function(iterable, entries, fn, that){
+ var iterFn = getIterFn(iterable)
+ , f = ctx(fn, that, entries ? 2 : 1)
+ , index = 0
+ , length, step, iterator;
+ if(typeof iterFn != 'function')throw TypeError(iterable + ' is not iterable!');
+ // fast case for arrays with default iterator
+ if(isArrayIter(iterFn))for(length = toLength(iterable.length); length > index; index++){
+ entries ? f(anObject(step = iterable[index])[0], step[1]) : f(iterable[index]);
+ } else for(iterator = iterFn.call(iterable); !(step = iterator.next()).done; ){
+ call(iterator, f, step.value, entries);
+ }
+ };
+
+/***/ },
+/* 139 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 7.3.20 SpeciesConstructor(O, defaultConstructor)
+ var anObject = __webpack_require__(20)
+ , aFunction = __webpack_require__(13)
+ , SPECIES = __webpack_require__(31)('species');
+ module.exports = function(O, D){
+ var C = anObject(O).constructor, S;
+ return C === undefined || (S = anObject(C)[SPECIES]) == undefined ? D : aFunction(S);
+ };
+
+/***/ },
+/* 140 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var global = __webpack_require__(4)
+ , macrotask = __webpack_require__(141).set
+ , Observer = global.MutationObserver || global.WebKitMutationObserver
+ , process = global.process
+ , Promise = global.Promise
+ , isNode = __webpack_require__(18)(process) == 'process'
+ , head, last, notify;
+
+ var flush = function(){
+ var parent, domain, fn;
+ if(isNode && (parent = process.domain)){
+ process.domain = null;
+ parent.exit();
+ }
+ while(head){
+ domain = head.domain;
+ fn = head.fn;
+ if(domain)domain.enter();
+ fn(); // <- currently we use it only for Promise - try / catch not required
+ if(domain)domain.exit();
+ head = head.next;
+ } last = undefined;
+ if(parent)parent.enter();
+ };
+
+ // Node.js
+ if(isNode){
+ notify = function(){
+ process.nextTick(flush);
+ };
+ // browsers with MutationObserver
+ } else if(Observer){
+ var toggle = 1
+ , node = document.createTextNode('');
+ new Observer(flush).observe(node, {characterData: true}); // eslint-disable-line no-new
+ notify = function(){
+ node.data = toggle = -toggle;
+ };
+ // environments with maybe non-completely correct, but existent Promise
+ } else if(Promise && Promise.resolve){
+ notify = function(){
+ Promise.resolve().then(flush);
+ };
+ // for other environments - macrotask based on:
+ // - setImmediate
+ // - MessageChannel
+ // - window.postMessag
+ // - onreadystatechange
+ // - setTimeout
+ } else {
+ notify = function(){
+ // strange IE + webpack dev server bug - use .call(global)
+ macrotask.call(global, flush);
+ };
+ }
+
+ module.exports = function asap(fn){
+ var task = {fn: fn, next: undefined, domain: isNode && process.domain};
+ if(last)last.next = task;
+ if(!head){
+ head = task;
+ notify();
+ } last = task;
+ };
+
+/***/ },
+/* 141 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var ctx = __webpack_require__(12)
+ , invoke = __webpack_require__(19)
+ , html = __webpack_require__(14)
+ , cel = __webpack_require__(15)
+ , global = __webpack_require__(4)
+ , process = global.process
+ , setTask = global.setImmediate
+ , clearTask = global.clearImmediate
+ , MessageChannel = global.MessageChannel
+ , counter = 0
+ , queue = {}
+ , ONREADYSTATECHANGE = 'onreadystatechange'
+ , defer, channel, port;
+ var run = function(){
+ var id = +this;
+ if(queue.hasOwnProperty(id)){
+ var fn = queue[id];
+ delete queue[id];
+ fn();
+ }
+ };
+ var listner = function(event){
+ run.call(event.data);
+ };
+ // Node.js 0.9+ & IE10+ has setImmediate, otherwise:
+ if(!setTask || !clearTask){
+ setTask = function setImmediate(fn){
+ var args = [], i = 1;
+ while(arguments.length > i)args.push(arguments[i++]);
+ queue[++counter] = function(){
+ invoke(typeof fn == 'function' ? fn : Function(fn), args);
+ };
+ defer(counter);
+ return counter;
+ };
+ clearTask = function clearImmediate(id){
+ delete queue[id];
+ };
+ // Node.js 0.8-
+ if(__webpack_require__(18)(process) == 'process'){
+ defer = function(id){
+ process.nextTick(ctx(run, id, 1));
+ };
+ // Browsers with MessageChannel, includes WebWorkers
+ } else if(MessageChannel){
+ channel = new MessageChannel;
+ port = channel.port2;
+ channel.port1.onmessage = listner;
+ defer = ctx(port.postMessage, port, 1);
+ // Browsers with postMessage, skip WebWorkers
+ // IE8 has postMessage, but it's sync & typeof its postMessage is 'object'
+ } else if(global.addEventListener && typeof postMessage == 'function' && !global.importScripts){
+ defer = function(id){
+ global.postMessage(id + '', '*');
+ };
+ global.addEventListener('message', listner, false);
+ // IE8-
+ } else if(ONREADYSTATECHANGE in cel('script')){
+ defer = function(id){
+ html.appendChild(cel('script'))[ONREADYSTATECHANGE] = function(){
+ html.removeChild(this);
+ run.call(id);
+ };
+ };
+ // Rest old browsers
+ } else {
+ defer = function(id){
+ setTimeout(ctx(run, id, 1), 0);
+ };
+ }
+ }
+ module.exports = {
+ set: setTask,
+ clear: clearTask
+ };
+
+/***/ },
+/* 142 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var redefine = __webpack_require__(10);
+ module.exports = function(target, src){
+ for(var key in src)redefine(target, key, src[key]);
+ return target;
+ };
+
+/***/ },
+/* 143 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var strong = __webpack_require__(144);
+
+ // 23.1 Map Objects
+ __webpack_require__(145)('Map', function(get){
+ return function Map(){ return get(this, arguments.length > 0 ? arguments[0] : undefined); };
+ }, {
+ // 23.1.3.6 Map.prototype.get(key)
+ get: function get(key){
+ var entry = strong.getEntry(this, key);
+ return entry && entry.v;
+ },
+ // 23.1.3.9 Map.prototype.set(key, value)
+ set: function set(key, value){
+ return strong.def(this, key === 0 ? 0 : key, value);
+ }
+ }, strong, true);
+
+/***/ },
+/* 144 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , hide = __webpack_require__(6)
+ , redefineAll = __webpack_require__(142)
+ , ctx = __webpack_require__(12)
+ , strictNew = __webpack_require__(137)
+ , defined = __webpack_require__(22)
+ , forOf = __webpack_require__(138)
+ , $iterDefine = __webpack_require__(108)
+ , step = __webpack_require__(119)
+ , ID = __webpack_require__(11)('id')
+ , $has = __webpack_require__(17)
+ , isObject = __webpack_require__(16)
+ , setSpecies = __webpack_require__(121)
+ , DESCRIPTORS = __webpack_require__(8)
+ , isExtensible = Object.isExtensible || isObject
+ , SIZE = DESCRIPTORS ? '_s' : 'size'
+ , id = 0;
+
+ var fastKey = function(it, create){
+ // return primitive with prefix
+ if(!isObject(it))return typeof it == 'symbol' ? it : (typeof it == 'string' ? 'S' : 'P') + it;
+ if(!$has(it, ID)){
+ // can't set id to frozen object
+ if(!isExtensible(it))return 'F';
+ // not necessary to add id
+ if(!create)return 'E';
+ // add missing object id
+ hide(it, ID, ++id);
+ // return object id with prefix
+ } return 'O' + it[ID];
+ };
+
+ var getEntry = function(that, key){
+ // fast case
+ var index = fastKey(key), entry;
+ if(index !== 'F')return that._i[index];
+ // frozen object case
+ for(entry = that._f; entry; entry = entry.n){
+ if(entry.k == key)return entry;
+ }
+ };
+
+ module.exports = {
+ getConstructor: function(wrapper, NAME, IS_MAP, ADDER){
+ var C = wrapper(function(that, iterable){
+ strictNew(that, C, NAME);
+ that._i = $.create(null); // index
+ that._f = undefined; // first entry
+ that._l = undefined; // last entry
+ that[SIZE] = 0; // size
+ if(iterable != undefined)forOf(iterable, IS_MAP, that[ADDER], that);
+ });
+ redefineAll(C.prototype, {
+ // 23.1.3.1 Map.prototype.clear()
+ // 23.2.3.2 Set.prototype.clear()
+ clear: function clear(){
+ for(var that = this, data = that._i, entry = that._f; entry; entry = entry.n){
+ entry.r = true;
+ if(entry.p)entry.p = entry.p.n = undefined;
+ delete data[entry.i];
+ }
+ that._f = that._l = undefined;
+ that[SIZE] = 0;
+ },
+ // 23.1.3.3 Map.prototype.delete(key)
+ // 23.2.3.4 Set.prototype.delete(value)
+ 'delete': function(key){
+ var that = this
+ , entry = getEntry(that, key);
+ if(entry){
+ var next = entry.n
+ , prev = entry.p;
+ delete that._i[entry.i];
+ entry.r = true;
+ if(prev)prev.n = next;
+ if(next)next.p = prev;
+ if(that._f == entry)that._f = next;
+ if(that._l == entry)that._l = prev;
+ that[SIZE]--;
+ } return !!entry;
+ },
+ // 23.2.3.6 Set.prototype.forEach(callbackfn, thisArg = undefined)
+ // 23.1.3.5 Map.prototype.forEach(callbackfn, thisArg = undefined)
+ forEach: function forEach(callbackfn /*, that = undefined */){
+ var f = ctx(callbackfn, arguments.length > 1 ? arguments[1] : undefined, 3)
+ , entry;
+ while(entry = entry ? entry.n : this._f){
+ f(entry.v, entry.k, this);
+ // revert to the last existing entry
+ while(entry && entry.r)entry = entry.p;
+ }
+ },
+ // 23.1.3.7 Map.prototype.has(key)
+ // 23.2.3.7 Set.prototype.has(value)
+ has: function has(key){
+ return !!getEntry(this, key);
+ }
+ });
+ if(DESCRIPTORS)$.setDesc(C.prototype, 'size', {
+ get: function(){
+ return defined(this[SIZE]);
+ }
+ });
+ return C;
+ },
+ def: function(that, key, value){
+ var entry = getEntry(that, key)
+ , prev, index;
+ // change existing entry
+ if(entry){
+ entry.v = value;
+ // create new entry
+ } else {
+ that._l = entry = {
+ i: index = fastKey(key, true), // <- index
+ k: key, // <- key
+ v: value, // <- value
+ p: prev = that._l, // <- previous entry
+ n: undefined, // <- next entry
+ r: false // <- removed
+ };
+ if(!that._f)that._f = entry;
+ if(prev)prev.n = entry;
+ that[SIZE]++;
+ // add to index
+ if(index !== 'F')that._i[index] = entry;
+ } return that;
+ },
+ getEntry: getEntry,
+ setStrong: function(C, NAME, IS_MAP){
+ // add .keys, .values, .entries, [@@iterator]
+ // 23.1.3.4, 23.1.3.8, 23.1.3.11, 23.1.3.12, 23.2.3.5, 23.2.3.8, 23.2.3.10, 23.2.3.11
+ $iterDefine(C, NAME, function(iterated, kind){
+ this._t = iterated; // target
+ this._k = kind; // kind
+ this._l = undefined; // previous
+ }, function(){
+ var that = this
+ , kind = that._k
+ , entry = that._l;
+ // revert to the last existing entry
+ while(entry && entry.r)entry = entry.p;
+ // get next entry
+ if(!that._t || !(that._l = entry = entry ? entry.n : that._t._f)){
+ // or finish the iteration
+ that._t = undefined;
+ return step(1);
+ }
+ // return step by kind
+ if(kind == 'keys' )return step(0, entry.k);
+ if(kind == 'values')return step(0, entry.v);
+ return step(0, [entry.k, entry.v]);
+ }, IS_MAP ? 'entries' : 'values' , !IS_MAP, true);
+
+ // add [@@species], 23.1.2.2, 23.2.2.2
+ setSpecies(NAME);
+ }
+ };
+
+/***/ },
+/* 145 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var global = __webpack_require__(4)
+ , $export = __webpack_require__(3)
+ , redefine = __webpack_require__(10)
+ , redefineAll = __webpack_require__(142)
+ , forOf = __webpack_require__(138)
+ , strictNew = __webpack_require__(137)
+ , isObject = __webpack_require__(16)
+ , fails = __webpack_require__(9)
+ , $iterDetect = __webpack_require__(115)
+ , setToStringTag = __webpack_require__(35);
+
+ module.exports = function(NAME, wrapper, methods, common, IS_MAP, IS_WEAK){
+ var Base = global[NAME]
+ , C = Base
+ , ADDER = IS_MAP ? 'set' : 'add'
+ , proto = C && C.prototype
+ , O = {};
+ var fixMethod = function(KEY){
+ var fn = proto[KEY];
+ redefine(proto, KEY,
+ KEY == 'delete' ? function(a){
+ return IS_WEAK && !isObject(a) ? false : fn.call(this, a === 0 ? 0 : a);
+ } : KEY == 'has' ? function has(a){
+ return IS_WEAK && !isObject(a) ? false : fn.call(this, a === 0 ? 0 : a);
+ } : KEY == 'get' ? function get(a){
+ return IS_WEAK && !isObject(a) ? undefined : fn.call(this, a === 0 ? 0 : a);
+ } : KEY == 'add' ? function add(a){ fn.call(this, a === 0 ? 0 : a); return this; }
+ : function set(a, b){ fn.call(this, a === 0 ? 0 : a, b); return this; }
+ );
+ };
+ if(typeof C != 'function' || !(IS_WEAK || proto.forEach && !fails(function(){
+ new C().entries().next();
+ }))){
+ // create collection constructor
+ C = common.getConstructor(wrapper, NAME, IS_MAP, ADDER);
+ redefineAll(C.prototype, methods);
+ } else {
+ var instance = new C
+ // early implementations not supports chaining
+ , HASNT_CHAINING = instance[ADDER](IS_WEAK ? {} : -0, 1) != instance
+ // V8 ~ Chromium 40- weak-collections throws on primitives, but should return false
+ , THROWS_ON_PRIMITIVES = fails(function(){ instance.has(1); })
+ // most early implementations doesn't supports iterables, most modern - not close it correctly
+ , ACCEPT_ITERABLES = $iterDetect(function(iter){ new C(iter); }) // eslint-disable-line no-new
+ // for early implementations -0 and +0 not the same
+ , BUGGY_ZERO;
+ if(!ACCEPT_ITERABLES){
+ C = wrapper(function(target, iterable){
+ strictNew(target, C, NAME);
+ var that = new Base;
+ if(iterable != undefined)forOf(iterable, IS_MAP, that[ADDER], that);
+ return that;
+ });
+ C.prototype = proto;
+ proto.constructor = C;
+ }
+ IS_WEAK || instance.forEach(function(val, key){
+ BUGGY_ZERO = 1 / key === -Infinity;
+ });
+ if(THROWS_ON_PRIMITIVES || BUGGY_ZERO){
+ fixMethod('delete');
+ fixMethod('has');
+ IS_MAP && fixMethod('get');
+ }
+ if(BUGGY_ZERO || HASNT_CHAINING)fixMethod(ADDER);
+ // weak collections should not contains .clear method
+ if(IS_WEAK && proto.clear)delete proto.clear;
+ }
+
+ setToStringTag(C, NAME);
+
+ O[NAME] = C;
+ $export($export.G + $export.W + $export.F * (C != Base), O);
+
+ if(!IS_WEAK)common.setStrong(C, NAME, IS_MAP);
+
+ return C;
+ };
+
+/***/ },
+/* 146 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var strong = __webpack_require__(144);
+
+ // 23.2 Set Objects
+ __webpack_require__(145)('Set', function(get){
+ return function Set(){ return get(this, arguments.length > 0 ? arguments[0] : undefined); };
+ }, {
+ // 23.2.3.1 Set.prototype.add(value)
+ add: function add(value){
+ return strong.def(this, value = value === 0 ? 0 : value, value);
+ }
+ }, strong);
+
+/***/ },
+/* 147 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , redefine = __webpack_require__(10)
+ , weak = __webpack_require__(148)
+ , isObject = __webpack_require__(16)
+ , has = __webpack_require__(17)
+ , frozenStore = weak.frozenStore
+ , WEAK = weak.WEAK
+ , isExtensible = Object.isExtensible || isObject
+ , tmp = {};
+
+ // 23.3 WeakMap Objects
+ var $WeakMap = __webpack_require__(145)('WeakMap', function(get){
+ return function WeakMap(){ return get(this, arguments.length > 0 ? arguments[0] : undefined); };
+ }, {
+ // 23.3.3.3 WeakMap.prototype.get(key)
+ get: function get(key){
+ if(isObject(key)){
+ if(!isExtensible(key))return frozenStore(this).get(key);
+ if(has(key, WEAK))return key[WEAK][this._i];
+ }
+ },
+ // 23.3.3.5 WeakMap.prototype.set(key, value)
+ set: function set(key, value){
+ return weak.def(this, key, value);
+ }
+ }, weak, true, true);
+
+ // IE11 WeakMap frozen keys fix
+ if(new $WeakMap().set((Object.freeze || Object)(tmp), 7).get(tmp) != 7){
+ $.each.call(['delete', 'has', 'get', 'set'], function(key){
+ var proto = $WeakMap.prototype
+ , method = proto[key];
+ redefine(proto, key, function(a, b){
+ // store frozen objects on leaky map
+ if(isObject(a) && !isExtensible(a)){
+ var result = frozenStore(this)[key](a, b);
+ return key == 'set' ? this : result;
+ // store all the rest on native weakmap
+ } return method.call(this, a, b);
+ });
+ });
+ }
+
+/***/ },
+/* 148 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var hide = __webpack_require__(6)
+ , redefineAll = __webpack_require__(142)
+ , anObject = __webpack_require__(20)
+ , isObject = __webpack_require__(16)
+ , strictNew = __webpack_require__(137)
+ , forOf = __webpack_require__(138)
+ , createArrayMethod = __webpack_require__(28)
+ , $has = __webpack_require__(17)
+ , WEAK = __webpack_require__(11)('weak')
+ , isExtensible = Object.isExtensible || isObject
+ , arrayFind = createArrayMethod(5)
+ , arrayFindIndex = createArrayMethod(6)
+ , id = 0;
+
+ // fallback for frozen keys
+ var frozenStore = function(that){
+ return that._l || (that._l = new FrozenStore);
+ };
+ var FrozenStore = function(){
+ this.a = [];
+ };
+ var findFrozen = function(store, key){
+ return arrayFind(store.a, function(it){
+ return it[0] === key;
+ });
+ };
+ FrozenStore.prototype = {
+ get: function(key){
+ var entry = findFrozen(this, key);
+ if(entry)return entry[1];
+ },
+ has: function(key){
+ return !!findFrozen(this, key);
+ },
+ set: function(key, value){
+ var entry = findFrozen(this, key);
+ if(entry)entry[1] = value;
+ else this.a.push([key, value]);
+ },
+ 'delete': function(key){
+ var index = arrayFindIndex(this.a, function(it){
+ return it[0] === key;
+ });
+ if(~index)this.a.splice(index, 1);
+ return !!~index;
+ }
+ };
+
+ module.exports = {
+ getConstructor: function(wrapper, NAME, IS_MAP, ADDER){
+ var C = wrapper(function(that, iterable){
+ strictNew(that, C, NAME);
+ that._i = id++; // collection id
+ that._l = undefined; // leak store for frozen objects
+ if(iterable != undefined)forOf(iterable, IS_MAP, that[ADDER], that);
+ });
+ redefineAll(C.prototype, {
+ // 23.3.3.2 WeakMap.prototype.delete(key)
+ // 23.4.3.3 WeakSet.prototype.delete(value)
+ 'delete': function(key){
+ if(!isObject(key))return false;
+ if(!isExtensible(key))return frozenStore(this)['delete'](key);
+ return $has(key, WEAK) && $has(key[WEAK], this._i) && delete key[WEAK][this._i];
+ },
+ // 23.3.3.4 WeakMap.prototype.has(key)
+ // 23.4.3.4 WeakSet.prototype.has(value)
+ has: function has(key){
+ if(!isObject(key))return false;
+ if(!isExtensible(key))return frozenStore(this).has(key);
+ return $has(key, WEAK) && $has(key[WEAK], this._i);
+ }
+ });
+ return C;
+ },
+ def: function(that, key, value){
+ if(!isExtensible(anObject(key))){
+ frozenStore(that).set(key, value);
+ } else {
+ $has(key, WEAK) || hide(key, WEAK, {});
+ key[WEAK][that._i] = value;
+ } return that;
+ },
+ frozenStore: frozenStore,
+ WEAK: WEAK
+ };
+
+/***/ },
+/* 149 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var weak = __webpack_require__(148);
+
+ // 23.4 WeakSet Objects
+ __webpack_require__(145)('WeakSet', function(get){
+ return function WeakSet(){ return get(this, arguments.length > 0 ? arguments[0] : undefined); };
+ }, {
+ // 23.4.3.1 WeakSet.prototype.add(value)
+ add: function add(value){
+ return weak.def(this, value, true);
+ }
+ }, weak, false, true);
+
+/***/ },
+/* 150 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.1 Reflect.apply(target, thisArgument, argumentsList)
+ var $export = __webpack_require__(3)
+ , _apply = Function.apply
+ , anObject = __webpack_require__(20);
+
+ $export($export.S, 'Reflect', {
+ apply: function apply(target, thisArgument, argumentsList){
+ return _apply.call(target, thisArgument, anObject(argumentsList));
+ }
+ });
+
+/***/ },
+/* 151 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.2 Reflect.construct(target, argumentsList [, newTarget])
+ var $ = __webpack_require__(2)
+ , $export = __webpack_require__(3)
+ , aFunction = __webpack_require__(13)
+ , anObject = __webpack_require__(20)
+ , isObject = __webpack_require__(16)
+ , bind = Function.bind || __webpack_require__(5).Function.prototype.bind;
+
+ // MS Edge supports only 2 arguments
+ // FF Nightly sets third argument as `new.target`, but does not create `this` from it
+ $export($export.S + $export.F * __webpack_require__(9)(function(){
+ function F(){}
+ return !(Reflect.construct(function(){}, [], F) instanceof F);
+ }), 'Reflect', {
+ construct: function construct(Target, args /*, newTarget*/){
+ aFunction(Target);
+ anObject(args);
+ var newTarget = arguments.length < 3 ? Target : aFunction(arguments[2]);
+ if(Target == newTarget){
+ // w/o altered newTarget, optimization for 0-4 arguments
+ switch(args.length){
+ case 0: return new Target;
+ case 1: return new Target(args[0]);
+ case 2: return new Target(args[0], args[1]);
+ case 3: return new Target(args[0], args[1], args[2]);
+ case 4: return new Target(args[0], args[1], args[2], args[3]);
+ }
+ // w/o altered newTarget, lot of arguments case
+ var $args = [null];
+ $args.push.apply($args, args);
+ return new (bind.apply(Target, $args));
+ }
+ // with altered newTarget, not support built-in constructors
+ var proto = newTarget.prototype
+ , instance = $.create(isObject(proto) ? proto : Object.prototype)
+ , result = Function.apply.call(Target, instance, args);
+ return isObject(result) ? result : instance;
+ }
+ });
+
+/***/ },
+/* 152 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.3 Reflect.defineProperty(target, propertyKey, attributes)
+ var $ = __webpack_require__(2)
+ , $export = __webpack_require__(3)
+ , anObject = __webpack_require__(20);
+
+ // MS Edge has broken Reflect.defineProperty - throwing instead of returning false
+ $export($export.S + $export.F * __webpack_require__(9)(function(){
+ Reflect.defineProperty($.setDesc({}, 1, {value: 1}), 1, {value: 2});
+ }), 'Reflect', {
+ defineProperty: function defineProperty(target, propertyKey, attributes){
+ anObject(target);
+ try {
+ $.setDesc(target, propertyKey, attributes);
+ return true;
+ } catch(e){
+ return false;
+ }
+ }
+ });
+
+/***/ },
+/* 153 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.4 Reflect.deleteProperty(target, propertyKey)
+ var $export = __webpack_require__(3)
+ , getDesc = __webpack_require__(2).getDesc
+ , anObject = __webpack_require__(20);
+
+ $export($export.S, 'Reflect', {
+ deleteProperty: function deleteProperty(target, propertyKey){
+ var desc = getDesc(anObject(target), propertyKey);
+ return desc && !desc.configurable ? false : delete target[propertyKey];
+ }
+ });
+
+/***/ },
+/* 154 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // 26.1.5 Reflect.enumerate(target)
+ var $export = __webpack_require__(3)
+ , anObject = __webpack_require__(20);
+ var Enumerate = function(iterated){
+ this._t = anObject(iterated); // target
+ this._i = 0; // next index
+ var keys = this._k = [] // keys
+ , key;
+ for(key in iterated)keys.push(key);
+ };
+ __webpack_require__(110)(Enumerate, 'Object', function(){
+ var that = this
+ , keys = that._k
+ , key;
+ do {
+ if(that._i >= keys.length)return {value: undefined, done: true};
+ } while(!((key = keys[that._i++]) in that._t));
+ return {value: key, done: false};
+ });
+
+ $export($export.S, 'Reflect', {
+ enumerate: function enumerate(target){
+ return new Enumerate(target);
+ }
+ });
+
+/***/ },
+/* 155 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.6 Reflect.get(target, propertyKey [, receiver])
+ var $ = __webpack_require__(2)
+ , has = __webpack_require__(17)
+ , $export = __webpack_require__(3)
+ , isObject = __webpack_require__(16)
+ , anObject = __webpack_require__(20);
+
+ function get(target, propertyKey/*, receiver*/){
+ var receiver = arguments.length < 3 ? target : arguments[2]
+ , desc, proto;
+ if(anObject(target) === receiver)return target[propertyKey];
+ if(desc = $.getDesc(target, propertyKey))return has(desc, 'value')
+ ? desc.value
+ : desc.get !== undefined
+ ? desc.get.call(receiver)
+ : undefined;
+ if(isObject(proto = $.getProto(target)))return get(proto, propertyKey, receiver);
+ }
+
+ $export($export.S, 'Reflect', {get: get});
+
+/***/ },
+/* 156 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.7 Reflect.getOwnPropertyDescriptor(target, propertyKey)
+ var $ = __webpack_require__(2)
+ , $export = __webpack_require__(3)
+ , anObject = __webpack_require__(20);
+
+ $export($export.S, 'Reflect', {
+ getOwnPropertyDescriptor: function getOwnPropertyDescriptor(target, propertyKey){
+ return $.getDesc(anObject(target), propertyKey);
+ }
+ });
+
+/***/ },
+/* 157 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.8 Reflect.getPrototypeOf(target)
+ var $export = __webpack_require__(3)
+ , getProto = __webpack_require__(2).getProto
+ , anObject = __webpack_require__(20);
+
+ $export($export.S, 'Reflect', {
+ getPrototypeOf: function getPrototypeOf(target){
+ return getProto(anObject(target));
+ }
+ });
+
+/***/ },
+/* 158 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.9 Reflect.has(target, propertyKey)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Reflect', {
+ has: function has(target, propertyKey){
+ return propertyKey in target;
+ }
+ });
+
+/***/ },
+/* 159 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.10 Reflect.isExtensible(target)
+ var $export = __webpack_require__(3)
+ , anObject = __webpack_require__(20)
+ , $isExtensible = Object.isExtensible;
+
+ $export($export.S, 'Reflect', {
+ isExtensible: function isExtensible(target){
+ anObject(target);
+ return $isExtensible ? $isExtensible(target) : true;
+ }
+ });
+
+/***/ },
+/* 160 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.11 Reflect.ownKeys(target)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Reflect', {ownKeys: __webpack_require__(161)});
+
+/***/ },
+/* 161 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // all object keys, includes non-enumerable and symbols
+ var $ = __webpack_require__(2)
+ , anObject = __webpack_require__(20)
+ , Reflect = __webpack_require__(4).Reflect;
+ module.exports = Reflect && Reflect.ownKeys || function ownKeys(it){
+ var keys = $.getNames(anObject(it))
+ , getSymbols = $.getSymbols;
+ return getSymbols ? keys.concat(getSymbols(it)) : keys;
+ };
+
+/***/ },
+/* 162 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.12 Reflect.preventExtensions(target)
+ var $export = __webpack_require__(3)
+ , anObject = __webpack_require__(20)
+ , $preventExtensions = Object.preventExtensions;
+
+ $export($export.S, 'Reflect', {
+ preventExtensions: function preventExtensions(target){
+ anObject(target);
+ try {
+ if($preventExtensions)$preventExtensions(target);
+ return true;
+ } catch(e){
+ return false;
+ }
+ }
+ });
+
+/***/ },
+/* 163 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.13 Reflect.set(target, propertyKey, V [, receiver])
+ var $ = __webpack_require__(2)
+ , has = __webpack_require__(17)
+ , $export = __webpack_require__(3)
+ , createDesc = __webpack_require__(7)
+ , anObject = __webpack_require__(20)
+ , isObject = __webpack_require__(16);
+
+ function set(target, propertyKey, V/*, receiver*/){
+ var receiver = arguments.length < 4 ? target : arguments[3]
+ , ownDesc = $.getDesc(anObject(target), propertyKey)
+ , existingDescriptor, proto;
+ if(!ownDesc){
+ if(isObject(proto = $.getProto(target))){
+ return set(proto, propertyKey, V, receiver);
+ }
+ ownDesc = createDesc(0);
+ }
+ if(has(ownDesc, 'value')){
+ if(ownDesc.writable === false || !isObject(receiver))return false;
+ existingDescriptor = $.getDesc(receiver, propertyKey) || createDesc(0);
+ existingDescriptor.value = V;
+ $.setDesc(receiver, propertyKey, existingDescriptor);
+ return true;
+ }
+ return ownDesc.set === undefined ? false : (ownDesc.set.call(receiver, V), true);
+ }
+
+ $export($export.S, 'Reflect', {set: set});
+
+/***/ },
+/* 164 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.14 Reflect.setPrototypeOf(target, proto)
+ var $export = __webpack_require__(3)
+ , setProto = __webpack_require__(45);
+
+ if(setProto)$export($export.S, 'Reflect', {
+ setPrototypeOf: function setPrototypeOf(target, proto){
+ setProto.check(target, proto);
+ try {
+ setProto.set(target, proto);
+ return true;
+ } catch(e){
+ return false;
+ }
+ }
+ });
+
+/***/ },
+/* 165 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , $includes = __webpack_require__(33)(true);
+
+ $export($export.P, 'Array', {
+ // https://github.com/domenic/Array.prototype.includes
+ includes: function includes(el /*, fromIndex = 0 */){
+ return $includes(this, el, arguments.length > 1 ? arguments[1] : undefined);
+ }
+ });
+
+ __webpack_require__(118)('includes');
+
+/***/ },
+/* 166 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // https://github.com/mathiasbynens/String.prototype.at
+ var $export = __webpack_require__(3)
+ , $at = __webpack_require__(98)(true);
+
+ $export($export.P, 'String', {
+ at: function at(pos){
+ return $at(this, pos);
+ }
+ });
+
+/***/ },
+/* 167 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , $pad = __webpack_require__(168);
+
+ $export($export.P, 'String', {
+ padLeft: function padLeft(maxLength /*, fillString = ' ' */){
+ return $pad(this, maxLength, arguments.length > 1 ? arguments[1] : undefined, true);
+ }
+ });
+
+/***/ },
+/* 168 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // https://github.com/ljharb/proposal-string-pad-left-right
+ var toLength = __webpack_require__(27)
+ , repeat = __webpack_require__(105)
+ , defined = __webpack_require__(22);
+
+ module.exports = function(that, maxLength, fillString, left){
+ var S = String(defined(that))
+ , stringLength = S.length
+ , fillStr = fillString === undefined ? ' ' : String(fillString)
+ , intMaxLength = toLength(maxLength);
+ if(intMaxLength <= stringLength)return S;
+ if(fillStr == '')fillStr = ' ';
+ var fillLen = intMaxLength - stringLength
+ , stringFiller = repeat.call(fillStr, Math.ceil(fillLen / fillStr.length));
+ if(stringFiller.length > fillLen)stringFiller = stringFiller.slice(0, fillLen);
+ return left ? stringFiller + S : S + stringFiller;
+ };
+
+/***/ },
+/* 169 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , $pad = __webpack_require__(168);
+
+ $export($export.P, 'String', {
+ padRight: function padRight(maxLength /*, fillString = ' ' */){
+ return $pad(this, maxLength, arguments.length > 1 ? arguments[1] : undefined, false);
+ }
+ });
+
+/***/ },
+/* 170 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // https://github.com/sebmarkbage/ecmascript-string-left-right-trim
+ __webpack_require__(63)('trimLeft', function($trim){
+ return function trimLeft(){
+ return $trim(this, 1);
+ };
+ });
+
+/***/ },
+/* 171 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // https://github.com/sebmarkbage/ecmascript-string-left-right-trim
+ __webpack_require__(63)('trimRight', function($trim){
+ return function trimRight(){
+ return $trim(this, 2);
+ };
+ });
+
+/***/ },
+/* 172 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // https://github.com/benjamingr/RexExp.escape
+ var $export = __webpack_require__(3)
+ , $re = __webpack_require__(173)(/[\\^$*+?.()|[\]{}]/g, '\\$&');
+
+ $export($export.S, 'RegExp', {escape: function escape(it){ return $re(it); }});
+
+
+/***/ },
+/* 173 */
+/***/ function(module, exports) {
+
+ module.exports = function(regExp, replace){
+ var replacer = replace === Object(replace) ? function(part){
+ return replace[part];
+ } : replace;
+ return function(it){
+ return String(it).replace(regExp, replacer);
+ };
+ };
+
+/***/ },
+/* 174 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // https://gist.github.com/WebReflection/9353781
+ var $ = __webpack_require__(2)
+ , $export = __webpack_require__(3)
+ , ownKeys = __webpack_require__(161)
+ , toIObject = __webpack_require__(23)
+ , createDesc = __webpack_require__(7);
+
+ $export($export.S, 'Object', {
+ getOwnPropertyDescriptors: function getOwnPropertyDescriptors(object){
+ var O = toIObject(object)
+ , setDesc = $.setDesc
+ , getDesc = $.getDesc
+ , keys = ownKeys(O)
+ , result = {}
+ , i = 0
+ , key, D;
+ while(keys.length > i){
+ D = getDesc(O, key = keys[i++]);
+ if(key in result)setDesc(result, key, createDesc(0, D));
+ else result[key] = D;
+ } return result;
+ }
+ });
+
+/***/ },
+/* 175 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // http://goo.gl/XkBrjD
+ var $export = __webpack_require__(3)
+ , $values = __webpack_require__(176)(false);
+
+ $export($export.S, 'Object', {
+ values: function values(it){
+ return $values(it);
+ }
+ });
+
+/***/ },
+/* 176 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $ = __webpack_require__(2)
+ , toIObject = __webpack_require__(23)
+ , isEnum = $.isEnum;
+ module.exports = function(isEntries){
+ return function(it){
+ var O = toIObject(it)
+ , keys = $.getKeys(O)
+ , length = keys.length
+ , i = 0
+ , result = []
+ , key;
+ while(length > i)if(isEnum.call(O, key = keys[i++])){
+ result.push(isEntries ? [key, O[key]] : O[key]);
+ } return result;
+ };
+ };
+
+/***/ },
+/* 177 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // http://goo.gl/XkBrjD
+ var $export = __webpack_require__(3)
+ , $entries = __webpack_require__(176)(true);
+
+ $export($export.S, 'Object', {
+ entries: function entries(it){
+ return $entries(it);
+ }
+ });
+
+/***/ },
+/* 178 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // https://github.com/DavidBruant/Map-Set.prototype.toJSON
+ var $export = __webpack_require__(3);
+
+ $export($export.P, 'Map', {toJSON: __webpack_require__(179)('Map')});
+
+/***/ },
+/* 179 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // https://github.com/DavidBruant/Map-Set.prototype.toJSON
+ var forOf = __webpack_require__(138)
+ , classof = __webpack_require__(47);
+ module.exports = function(NAME){
+ return function toJSON(){
+ if(classof(this) != NAME)throw TypeError(NAME + "#toJSON isn't generic");
+ var arr = [];
+ forOf(this, false, arr.push, arr);
+ return arr;
+ };
+ };
+
+/***/ },
+/* 180 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // https://github.com/DavidBruant/Map-Set.prototype.toJSON
+ var $export = __webpack_require__(3);
+
+ $export($export.P, 'Set', {toJSON: __webpack_require__(179)('Set')});
+
+/***/ },
+/* 181 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3)
+ , $task = __webpack_require__(141);
+ $export($export.G + $export.B, {
+ setImmediate: $task.set,
+ clearImmediate: $task.clear
+ });
+
+/***/ },
+/* 182 */
+/***/ function(module, exports, __webpack_require__) {
+
+ __webpack_require__(117);
+ var global = __webpack_require__(4)
+ , hide = __webpack_require__(6)
+ , Iterators = __webpack_require__(109)
+ , ITERATOR = __webpack_require__(31)('iterator')
+ , NL = global.NodeList
+ , HTC = global.HTMLCollection
+ , NLProto = NL && NL.prototype
+ , HTCProto = HTC && HTC.prototype
+ , ArrayValues = Iterators.NodeList = Iterators.HTMLCollection = Iterators.Array;
+ if(NLProto && !NLProto[ITERATOR])hide(NLProto, ITERATOR, ArrayValues);
+ if(HTCProto && !HTCProto[ITERATOR])hide(HTCProto, ITERATOR, ArrayValues);
+
+/***/ },
+/* 183 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // ie9- setTimeout & setInterval additional parameters fix
+ var global = __webpack_require__(4)
+ , $export = __webpack_require__(3)
+ , invoke = __webpack_require__(19)
+ , partial = __webpack_require__(184)
+ , navigator = global.navigator
+ , MSIE = !!navigator && /MSIE .\./.test(navigator.userAgent); // <- dirty ie9- check
+ var wrap = function(set){
+ return MSIE ? function(fn, time /*, ...args */){
+ return set(invoke(
+ partial,
+ [].slice.call(arguments, 2),
+ typeof fn == 'function' ? fn : Function(fn)
+ ), time);
+ } : set;
+ };
+ $export($export.G + $export.B + $export.F * MSIE, {
+ setTimeout: wrap(global.setTimeout),
+ setInterval: wrap(global.setInterval)
+ });
+
+/***/ },
+/* 184 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var path = __webpack_require__(185)
+ , invoke = __webpack_require__(19)
+ , aFunction = __webpack_require__(13);
+ module.exports = function(/* ...pargs */){
+ var fn = aFunction(this)
+ , length = arguments.length
+ , pargs = Array(length)
+ , i = 0
+ , _ = path._
+ , holder = false;
+ while(length > i)if((pargs[i] = arguments[i++]) === _)holder = true;
+ return function(/* ...args */){
+ var that = this
+ , $$ = arguments
+ , $$len = $$.length
+ , j = 0, k = 0, args;
+ if(!holder && !$$len)return invoke(fn, pargs, that);
+ args = pargs.slice();
+ if(holder)for(;length > j; j++)if(args[j] === _)args[j] = $$[k++];
+ while($$len > k)args.push($$[k++]);
+ return invoke(fn, args, that);
+ };
+ };
+
+/***/ },
+/* 185 */
+/***/ function(module, exports, __webpack_require__) {
+
+ module.exports = __webpack_require__(4);
+
+/***/ },
+/* 186 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , ctx = __webpack_require__(12)
+ , $export = __webpack_require__(3)
+ , createDesc = __webpack_require__(7)
+ , assign = __webpack_require__(41)
+ , keyOf = __webpack_require__(36)
+ , aFunction = __webpack_require__(13)
+ , forOf = __webpack_require__(138)
+ , isIterable = __webpack_require__(187)
+ , $iterCreate = __webpack_require__(110)
+ , step = __webpack_require__(119)
+ , isObject = __webpack_require__(16)
+ , toIObject = __webpack_require__(23)
+ , DESCRIPTORS = __webpack_require__(8)
+ , has = __webpack_require__(17)
+ , getKeys = $.getKeys;
+
+ // 0 -> Dict.forEach
+ // 1 -> Dict.map
+ // 2 -> Dict.filter
+ // 3 -> Dict.some
+ // 4 -> Dict.every
+ // 5 -> Dict.find
+ // 6 -> Dict.findKey
+ // 7 -> Dict.mapPairs
+ var createDictMethod = function(TYPE){
+ var IS_MAP = TYPE == 1
+ , IS_EVERY = TYPE == 4;
+ return function(object, callbackfn, that /* = undefined */){
+ var f = ctx(callbackfn, that, 3)
+ , O = toIObject(object)
+ , result = IS_MAP || TYPE == 7 || TYPE == 2
+ ? new (typeof this == 'function' ? this : Dict) : undefined
+ , key, val, res;
+ for(key in O)if(has(O, key)){
+ val = O[key];
+ res = f(val, key, object);
+ if(TYPE){
+ if(IS_MAP)result[key] = res; // map
+ else if(res)switch(TYPE){
+ case 2: result[key] = val; break; // filter
+ case 3: return true; // some
+ case 5: return val; // find
+ case 6: return key; // findKey
+ case 7: result[res[0]] = res[1]; // mapPairs
+ } else if(IS_EVERY)return false; // every
+ }
+ }
+ return TYPE == 3 || IS_EVERY ? IS_EVERY : result;
+ };
+ };
+ var findKey = createDictMethod(6);
+
+ var createDictIter = function(kind){
+ return function(it){
+ return new DictIterator(it, kind);
+ };
+ };
+ var DictIterator = function(iterated, kind){
+ this._t = toIObject(iterated); // target
+ this._a = getKeys(iterated); // keys
+ this._i = 0; // next index
+ this._k = kind; // kind
+ };
+ $iterCreate(DictIterator, 'Dict', function(){
+ var that = this
+ , O = that._t
+ , keys = that._a
+ , kind = that._k
+ , key;
+ do {
+ if(that._i >= keys.length){
+ that._t = undefined;
+ return step(1);
+ }
+ } while(!has(O, key = keys[that._i++]));
+ if(kind == 'keys' )return step(0, key);
+ if(kind == 'values')return step(0, O[key]);
+ return step(0, [key, O[key]]);
+ });
+
+ function Dict(iterable){
+ var dict = $.create(null);
+ if(iterable != undefined){
+ if(isIterable(iterable)){
+ forOf(iterable, true, function(key, value){
+ dict[key] = value;
+ });
+ } else assign(dict, iterable);
+ }
+ return dict;
+ }
+ Dict.prototype = null;
+
+ function reduce(object, mapfn, init){
+ aFunction(mapfn);
+ var O = toIObject(object)
+ , keys = getKeys(O)
+ , length = keys.length
+ , i = 0
+ , memo, key;
+ if(arguments.length < 3){
+ if(!length)throw TypeError('Reduce of empty object with no initial value');
+ memo = O[keys[i++]];
+ } else memo = Object(init);
+ while(length > i)if(has(O, key = keys[i++])){
+ memo = mapfn(memo, O[key], key, object);
+ }
+ return memo;
+ }
+
+ function includes(object, el){
+ return (el == el ? keyOf(object, el) : findKey(object, function(it){
+ return it != it;
+ })) !== undefined;
+ }
+
+ function get(object, key){
+ if(has(object, key))return object[key];
+ }
+ function set(object, key, value){
+ if(DESCRIPTORS && key in Object)$.setDesc(object, key, createDesc(0, value));
+ else object[key] = value;
+ return object;
+ }
+
+ function isDict(it){
+ return isObject(it) && $.getProto(it) === Dict.prototype;
+ }
+
+ $export($export.G + $export.F, {Dict: Dict});
+
+ $export($export.S, 'Dict', {
+ keys: createDictIter('keys'),
+ values: createDictIter('values'),
+ entries: createDictIter('entries'),
+ forEach: createDictMethod(0),
+ map: createDictMethod(1),
+ filter: createDictMethod(2),
+ some: createDictMethod(3),
+ every: createDictMethod(4),
+ find: createDictMethod(5),
+ findKey: findKey,
+ mapPairs: createDictMethod(7),
+ reduce: reduce,
+ keyOf: keyOf,
+ includes: includes,
+ has: has,
+ get: get,
+ set: set,
+ isDict: isDict
+ });
+
+/***/ },
+/* 187 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var classof = __webpack_require__(47)
+ , ITERATOR = __webpack_require__(31)('iterator')
+ , Iterators = __webpack_require__(109);
+ module.exports = __webpack_require__(5).isIterable = function(it){
+ var O = Object(it);
+ return O[ITERATOR] !== undefined
+ || '@@iterator' in O
+ || Iterators.hasOwnProperty(classof(O));
+ };
+
+/***/ },
+/* 188 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var anObject = __webpack_require__(20)
+ , get = __webpack_require__(114);
+ module.exports = __webpack_require__(5).getIterator = function(it){
+ var iterFn = get(it);
+ if(typeof iterFn != 'function')throw TypeError(it + ' is not iterable!');
+ return anObject(iterFn.call(it));
+ };
+
+/***/ },
+/* 189 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var global = __webpack_require__(4)
+ , core = __webpack_require__(5)
+ , $export = __webpack_require__(3)
+ , partial = __webpack_require__(184);
+ // https://esdiscuss.org/topic/promise-returning-delay-function
+ $export($export.G + $export.F, {
+ delay: function delay(time){
+ return new (core.Promise || global.Promise)(function(resolve){
+ setTimeout(partial.call(resolve, true), time);
+ });
+ }
+ });
+
+/***/ },
+/* 190 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var path = __webpack_require__(185)
+ , $export = __webpack_require__(3);
+
+ // Placeholder
+ __webpack_require__(5)._ = path._ = path._ || {};
+
+ $export($export.P + $export.F, 'Function', {part: __webpack_require__(184)});
+
+/***/ },
+/* 191 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3);
+
+ $export($export.S + $export.F, 'Object', {isObject: __webpack_require__(16)});
+
+/***/ },
+/* 192 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3);
+
+ $export($export.S + $export.F, 'Object', {classof: __webpack_require__(47)});
+
+/***/ },
+/* 193 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3)
+ , define = __webpack_require__(194);
+
+ $export($export.S + $export.F, 'Object', {define: define});
+
+/***/ },
+/* 194 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $ = __webpack_require__(2)
+ , ownKeys = __webpack_require__(161)
+ , toIObject = __webpack_require__(23);
+
+ module.exports = function define(target, mixin){
+ var keys = ownKeys(toIObject(mixin))
+ , length = keys.length
+ , i = 0, key;
+ while(length > i)$.setDesc(target, key = keys[i++], $.getDesc(mixin, key));
+ return target;
+ };
+
+/***/ },
+/* 195 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3)
+ , define = __webpack_require__(194)
+ , create = __webpack_require__(2).create;
+
+ $export($export.S + $export.F, 'Object', {
+ make: function(proto, mixin){
+ return define(create(proto), mixin);
+ }
+ });
+
+/***/ },
+/* 196 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ __webpack_require__(108)(Number, 'Number', function(iterated){
+ this._l = +iterated;
+ this._i = 0;
+ }, function(){
+ var i = this._i++
+ , done = !(i < this._l);
+ return {done: done, value: done ? undefined : i};
+ });
+
+/***/ },
+/* 197 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $export = __webpack_require__(3);
+ var $re = __webpack_require__(173)(/[&<>"']/g, {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ "'": '''
+ });
+
+ $export($export.P + $export.F, 'String', {escapeHTML: function escapeHTML(){ return $re(this); }});
+
+/***/ },
+/* 198 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $export = __webpack_require__(3);
+ var $re = __webpack_require__(173)(/&(?:amp|lt|gt|quot|apos);/g, {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ ''': "'"
+ });
+
+ $export($export.P + $export.F, 'String', {unescapeHTML: function unescapeHTML(){ return $re(this); }});
+
+/***/ },
+/* 199 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $ = __webpack_require__(2)
+ , global = __webpack_require__(4)
+ , $export = __webpack_require__(3)
+ , log = {}
+ , enabled = true;
+ // Methods from https://github.com/DeveloperToolsWG/console-object/blob/master/api.md
+ $.each.call((
+ 'assert,clear,count,debug,dir,dirxml,error,exception,group,groupCollapsed,groupEnd,' +
+ 'info,isIndependentlyComposed,log,markTimeline,profile,profileEnd,table,' +
+ 'time,timeEnd,timeline,timelineEnd,timeStamp,trace,warn'
+ ).split(','), function(key){
+ log[key] = function(){
+ var $console = global.console;
+ if(enabled && $console && $console[key]){
+ return Function.apply.call($console[key], $console, arguments);
+ }
+ };
+ });
+ $export($export.G + $export.F, {log: __webpack_require__(41)(log.log, log, {
+ enable: function(){
+ enabled = true;
+ },
+ disable: function(){
+ enabled = false;
+ }
+ })});
+
+/***/ },
+/* 200 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // JavaScript 1.6 / Strawman array statics shim
+ var $ = __webpack_require__(2)
+ , $export = __webpack_require__(3)
+ , $ctx = __webpack_require__(12)
+ , $Array = __webpack_require__(5).Array || Array
+ , statics = {};
+ var setStatics = function(keys, length){
+ $.each.call(keys.split(','), function(key){
+ if(length == undefined && key in $Array)statics[key] = $Array[key];
+ else if(key in [])statics[key] = $ctx(Function.call, [][key], length);
+ });
+ };
+ setStatics('pop,reverse,shift,keys,values,entries', 1);
+ setStatics('indexOf,every,some,forEach,map,filter,find,findIndex,includes', 3);
+ setStatics('join,slice,concat,push,splice,unshift,sort,lastIndexOf,' +
+ 'reduce,reduceRight,copyWithin,fill');
+ $export($export.S, 'Array', statics);
+
+/***/ }
+/******/ ]);
+// CommonJS export
+if(typeof module != 'undefined' && module.exports)module.exports = __e;
+// RequireJS export
+else if(typeof define == 'function' && define.amd)define(function(){return __e});
+// Export to global object
+else __g.core = __e;
+}(1, 1);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/core.min.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/core.min.js
new file mode 100644
index 00000000..7a080f0b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/core.min.js
@@ -0,0 +1,9 @@
+/**
+ * core-js 1.2.7
+ * https://github.com/zloirock/core-js
+ * License: http://rock.mit-license.org
+ * © 2016 Denis Pushkarev
+ */
+!function(b,c,a){"use strict";!function(b){function __webpack_require__(c){if(a[c])return a[c].exports;var d=a[c]={exports:{},id:c,loaded:!1};return b[c].call(d.exports,d,d.exports,__webpack_require__),d.loaded=!0,d.exports}var a={};return __webpack_require__.m=b,__webpack_require__.c=a,__webpack_require__.p="",__webpack_require__(0)}([function(b,c,a){a(1),a(34),a(40),a(42),a(44),a(46),a(48),a(50),a(51),a(52),a(53),a(54),a(55),a(56),a(57),a(58),a(59),a(60),a(61),a(64),a(65),a(66),a(68),a(69),a(70),a(71),a(72),a(73),a(74),a(76),a(77),a(78),a(80),a(81),a(82),a(84),a(85),a(86),a(87),a(88),a(89),a(90),a(91),a(92),a(93),a(94),a(95),a(96),a(97),a(99),a(103),a(104),a(106),a(107),a(111),a(116),a(117),a(120),a(122),a(124),a(126),a(127),a(128),a(130),a(131),a(133),a(134),a(135),a(136),a(143),a(146),a(147),a(149),a(150),a(151),a(152),a(153),a(154),a(155),a(156),a(157),a(158),a(159),a(160),a(162),a(163),a(164),a(165),a(166),a(167),a(169),a(170),a(171),a(172),a(174),a(175),a(177),a(178),a(180),a(181),a(182),a(183),a(186),a(114),a(188),a(187),a(189),a(190),a(191),a(192),a(193),a(195),a(196),a(197),a(198),a(199),b.exports=a(200)},function(S,R,b){var r,d=b(2),c=b(3),x=b(8),O=b(7),o=b(14),E=b(15),n=b(17),N=b(18),J=b(19),j=b(9),p=b(20),v=b(13),I=b(16),Q=b(21),y=b(23),K=b(25),w=b(26),h=b(27),s=b(24),m=b(11)("__proto__"),g=b(28),A=b(33)(!1),B=Object.prototype,C=Array.prototype,k=C.slice,M=C.join,F=d.setDesc,L=d.getDesc,q=d.setDescs,u={};x||(r=!j(function(){return 7!=F(E("div"),"a",{get:function(){return 7}}).a}),d.setDesc=function(b,c,a){if(r)try{return F(b,c,a)}catch(d){}if("get"in a||"set"in a)throw TypeError("Accessors not supported!");return"value"in a&&(p(b)[c]=a.value),b},d.getDesc=function(a,b){if(r)try{return L(a,b)}catch(c){}return n(a,b)?O(!B.propertyIsEnumerable.call(a,b),a[b]):void 0},d.setDescs=q=function(a,b){p(a);for(var c,e=d.getKeys(b),g=e.length,f=0;g>f;)d.setDesc(a,c=e[f++],b[c]);return a}),c(c.S+c.F*!x,"Object",{getOwnPropertyDescriptor:d.getDesc,defineProperty:d.setDesc,defineProperties:q});var i="constructor,hasOwnProperty,isPrototypeOf,propertyIsEnumerable,toLocaleString,toString,valueOf".split(","),H=i.concat("length","prototype"),G=i.length,l=function(){var a,b=E("iframe"),c=G,d=">";for(b.style.display="none",o.appendChild(b),b.src="javascript:",a=b.contentWindow.document,a.open(),a.write("f;)n(e,c=a[f++])&&(~A(d,c)||d.push(c));return d}},t=function(){};c(c.S,"Object",{getPrototypeOf:d.getProto=d.getProto||function(a){return a=Q(a),n(a,m)?a[m]:"function"==typeof a.constructor&&a instanceof a.constructor?a.constructor.prototype:a instanceof Object?B:null},getOwnPropertyNames:d.getNames=d.getNames||D(H,H.length,!0),create:d.create=d.create||function(c,d){var b;return null!==c?(t.prototype=p(c),b=new t,t.prototype=null,b[m]=c):b=l(),d===a?b:q(b,d)},keys:d.getKeys=d.getKeys||D(i,G,!1)});var P=function(d,a,e){if(!(a in u)){for(var c=[],b=0;a>b;b++)c[b]="a["+b+"]";u[a]=Function("F,a","return new F("+c.join(",")+")")}return u[a](d,e)};c(c.P,"Function",{bind:function bind(c){var a=v(this),d=k.call(arguments,1),b=function(){var e=d.concat(k.call(arguments));return this instanceof b?P(a,e.length,e):J(a,e,c)};return I(a.prototype)&&(b.prototype=a.prototype),b}}),c(c.P+c.F*j(function(){o&&k.call(o)}),"Array",{slice:function(f,b){var d=h(this.length),g=N(this);if(b=b===a?d:b,"Array"==g)return k.call(this,f,b);for(var e=w(f,d),l=w(b,d),i=h(l-e),j=Array(i),c=0;i>c;c++)j[c]="String"==g?this.charAt(e+c):this[e+c];return j}}),c(c.P+c.F*(s!=Object),"Array",{join:function join(b){return M.call(s(this),b===a?",":b)}}),c(c.S,"Array",{isArray:b(30)});var z=function(a){return function(g,d){v(g);var c=s(this),e=h(c.length),b=a?e-1:0,f=a?-1:1;if(arguments.length<2)for(;;){if(b in c){d=c[b],b+=f;break}if(b+=f,a?0>b:b>=e)throw TypeError("Reduce of empty array with no initial value")}for(;a?b>=0:e>b;b+=f)b in c&&(d=g(d,c[b],b,this));return d}},f=function(a){return function(b){return a(this,b,arguments[1])}};c(c.P,"Array",{forEach:d.each=d.each||f(g(0)),map:f(g(1)),filter:f(g(2)),some:f(g(3)),every:f(g(4)),reduce:z(!1),reduceRight:z(!0),indexOf:f(A),lastIndexOf:function(d,e){var b=y(this),c=h(b.length),a=c-1;for(arguments.length>1&&(a=Math.min(a,K(e))),0>a&&(a=h(c+a));a>=0;a--)if(a in b&&b[a]===d)return a;return-1}}),c(c.S,"Date",{now:function(){return+new Date}});var e=function(a){return a>9?a:"0"+a};c(c.P+c.F*(j(function(){return"0385-07-25T07:06:39.999Z"!=new Date(-5e13-1).toISOString()})||!j(function(){new Date(NaN).toISOString()})),"Date",{toISOString:function toISOString(){if(!isFinite(this))throw RangeError("Invalid time value");var a=this,b=a.getUTCFullYear(),c=a.getUTCMilliseconds(),d=0>b?"-":b>9999?"+":"";return d+("00000"+Math.abs(b)).slice(d?-6:-4)+"-"+e(a.getUTCMonth()+1)+"-"+e(a.getUTCDate())+"T"+e(a.getUTCHours())+":"+e(a.getUTCMinutes())+":"+e(a.getUTCSeconds())+"."+(c>99?c:"0"+e(c))+"Z"}})},function(b,c){var a=Object;b.exports={create:a.create,getProto:a.getPrototypeOf,isEnum:{}.propertyIsEnumerable,getDesc:a.getOwnPropertyDescriptor,setDesc:a.defineProperty,setDescs:a.defineProperties,getKeys:a.keys,getNames:a.getOwnPropertyNames,getSymbols:a.getOwnPropertySymbols,each:[].forEach}},function(g,j,c){var b=c(4),d=c(5),h=c(6),i=c(10),f=c(12),e="prototype",a=function(k,j,o){var g,m,c,s,v=k&a.F,p=k&a.G,u=k&a.S,r=k&a.P,t=k&a.B,l=p?b:u?b[j]||(b[j]={}):(b[j]||{})[e],n=p?d:d[j]||(d[j]={}),q=n[e]||(n[e]={});p&&(o=j);for(g in o)m=!v&&l&&g in l,c=(m?l:o)[g],s=t&&m?f(c,b):r&&"function"==typeof c?f(Function.call,c):c,l&&!m&&i(l,g,c),n[g]!=c&&h(n,g,s),r&&q[g]!=c&&(q[g]=c)};b.core=d,a.F=1,a.G=2,a.S=4,a.P=8,a.B=16,a.W=32,g.exports=a},function(a,d){var b=a.exports="undefined"!=typeof window&&window.Math==Math?window:"undefined"!=typeof self&&self.Math==Math?self:Function("return this")();"number"==typeof c&&(c=b)},function(a,d){var c=a.exports={version:"1.2.6"};"number"==typeof b&&(b=c)},function(b,e,a){var c=a(2),d=a(7);b.exports=a(8)?function(a,b,e){return c.setDesc(a,b,d(1,e))}:function(a,b,c){return a[b]=c,a}},function(a,b){a.exports=function(a,b){return{enumerable:!(1&a),configurable:!(2&a),writable:!(4&a),value:b}}},function(a,c,b){a.exports=!b(9)(function(){return 7!=Object.defineProperty({},"a",{get:function(){return 7}}).a})},function(a,b){a.exports=function(a){try{return!!a()}catch(b){return!0}}},function(f,i,a){var g=a(4),b=a(6),c=a(11)("src"),d="toString",e=Function[d],h=(""+e).split(d);a(5).inspectSource=function(a){return e.call(a)},(f.exports=function(e,a,d,f){"function"==typeof d&&(d.hasOwnProperty(c)||b(d,c,e[a]?""+e[a]:h.join(String(a))),d.hasOwnProperty("name")||b(d,"name",a)),e===g?e[a]=d:(f||delete e[a],b(e,a,d))})(Function.prototype,d,function toString(){return"function"==typeof this&&this[c]||e.call(this)})},function(b,e){var c=0,d=Math.random();b.exports=function(b){return"Symbol(".concat(b===a?"":b,")_",(++c+d).toString(36))}},function(b,e,c){var d=c(13);b.exports=function(b,c,e){if(d(b),c===a)return b;switch(e){case 1:return function(a){return b.call(c,a)};case 2:return function(a,d){return b.call(c,a,d)};case 3:return function(a,d,e){return b.call(c,a,d,e)}}return function(){return b.apply(c,arguments)}}},function(a,b){a.exports=function(a){if("function"!=typeof a)throw TypeError(a+" is not a function!");return a}},function(a,c,b){a.exports=b(4).document&&document.documentElement},function(d,f,b){var c=b(16),a=b(4).document,e=c(a)&&c(a.createElement);d.exports=function(b){return e?a.createElement(b):{}}},function(a,b){a.exports=function(a){return"object"==typeof a?null!==a:"function"==typeof a}},function(a,c){var b={}.hasOwnProperty;a.exports=function(a,c){return b.call(a,c)}},function(a,c){var b={}.toString;a.exports=function(a){return b.call(a).slice(8,-1)}},function(b,c){b.exports=function(c,b,d){var e=d===a;switch(b.length){case 0:return e?c():c.call(d);case 1:return e?c(b[0]):c.call(d,b[0]);case 2:return e?c(b[0],b[1]):c.call(d,b[0],b[1]);case 3:return e?c(b[0],b[1],b[2]):c.call(d,b[0],b[1],b[2]);case 4:return e?c(b[0],b[1],b[2],b[3]):c.call(d,b[0],b[1],b[2],b[3])}return c.apply(d,b)}},function(a,d,b){var c=b(16);a.exports=function(a){if(!c(a))throw TypeError(a+" is not an object!");return a}},function(a,d,b){var c=b(22);a.exports=function(a){return Object(c(a))}},function(b,c){b.exports=function(b){if(b==a)throw TypeError("Can't call method on "+b);return b}},function(b,e,a){var c=a(24),d=a(22);b.exports=function(a){return c(d(a))}},function(a,d,b){var c=b(18);a.exports=Object("z").propertyIsEnumerable(0)?Object:function(a){return"String"==c(a)?a.split(""):Object(a)}},function(a,d){var b=Math.ceil,c=Math.floor;a.exports=function(a){return isNaN(a=+a)?0:(a>0?c:b)(a)}},function(a,f,b){var c=b(25),d=Math.max,e=Math.min;a.exports=function(a,b){return a=c(a),0>a?d(a+b,0):e(a,b)}},function(a,e,b){var c=b(25),d=Math.min;a.exports=function(a){return a>0?d(c(a),9007199254740991):0}},function(d,i,b){var e=b(12),f=b(24),g=b(21),h=b(27),c=b(29);d.exports=function(b){var i=1==b,k=2==b,l=3==b,d=4==b,j=6==b,m=5==b||j;return function(p,v,x){for(var o,r,u=g(p),s=f(u),w=e(v,x,3),t=h(s.length),n=0,q=i?c(p,t):k?c(p,0):a;t>n;n++)if((m||n in s)&&(o=s[n],r=w(o,n,u),b))if(i)q[n]=r;else if(r)switch(b){case 3:return!0;case 5:return o;case 6:return n;case 2:q.push(o)}else if(d)return!1;return j?-1:l||d?d:q}}},function(d,g,b){var e=b(16),c=b(30),f=b(31)("species");d.exports=function(d,g){var b;return c(d)&&(b=d.constructor,"function"!=typeof b||b!==Array&&!c(b.prototype)||(b=a),e(b)&&(b=b[f],null===b&&(b=a))),new(b===a?Array:b)(g)}},function(a,d,b){var c=b(18);a.exports=Array.isArray||function(a){return"Array"==c(a)}},function(d,f,a){var c=a(32)("wks"),e=a(11),b=a(4).Symbol;d.exports=function(a){return c[a]||(c[a]=b&&b[a]||(b||e)("Symbol."+a))}},function(d,f,e){var a=e(4),b="__core-js_shared__",c=a[b]||(a[b]={});d.exports=function(a){return c[a]||(c[a]={})}},function(b,f,a){var c=a(23),d=a(27),e=a(26);b.exports=function(a){return function(j,g,k){var h,f=c(j),i=d(f.length),b=e(k,i);if(a&&g!=g){for(;i>b;)if(h=f[b++],h!=h)return!0}else for(;i>b;b++)if((a||b in f)&&f[b]===g)return a||b;return!a&&-1}}},function(W,V,b){var e=b(2),x=b(4),d=b(17),w=b(8),f=b(3),G=b(10),H=b(9),J=b(32),s=b(35),S=b(11),A=b(31),R=b(36),C=b(37),Q=b(38),P=b(30),O=b(20),p=b(23),v=b(7),I=e.getDesc,i=e.setDesc,k=e.create,z=C.get,g=x.Symbol,l=x.JSON,m=l&&l.stringify,n=!1,c=A("_hidden"),N=e.isEnum,o=J("symbol-registry"),h=J("symbols"),q="function"==typeof g,j=Object.prototype,y=w&&H(function(){return 7!=k(i({},"a",{get:function(){return i(this,"a",{value:7}).a}})).a})?function(c,a,d){var b=I(j,a);b&&delete j[a],i(c,a,d),b&&c!==j&&i(j,a,b)}:i,L=function(a){var b=h[a]=k(g.prototype);return b._k=a,w&&n&&y(j,a,{configurable:!0,set:function(b){d(this,c)&&d(this[c],a)&&(this[c][a]=!1),y(this,a,v(1,b))}}),b},r=function(a){return"symbol"==typeof a},t=function defineProperty(a,b,e){return e&&d(h,b)?(e.enumerable?(d(a,c)&&a[c][b]&&(a[c][b]=!1),e=k(e,{enumerable:v(0,!1)})):(d(a,c)||i(a,c,v(1,{})),a[c][b]=!0),y(a,b,e)):i(a,b,e)},u=function defineProperties(a,b){O(a);for(var c,d=Q(b=p(b)),e=0,f=d.length;f>e;)t(a,c=d[e++],b[c]);return a},F=function create(b,c){return c===a?k(b):u(k(b),c)},E=function propertyIsEnumerable(a){var b=N.call(this,a);return b||!d(this,a)||!d(h,a)||d(this,c)&&this[c][a]?b:!0},D=function getOwnPropertyDescriptor(a,b){var e=I(a=p(a),b);return!e||!d(h,b)||d(a,c)&&a[c][b]||(e.enumerable=!0),e},B=function getOwnPropertyNames(g){for(var a,b=z(p(g)),e=[],f=0;b.length>f;)d(h,a=b[f++])||a==c||e.push(a);return e},M=function getOwnPropertySymbols(f){for(var a,b=z(p(f)),c=[],e=0;b.length>e;)d(h,a=b[e++])&&c.push(h[a]);return c},T=function stringify(e){if(e!==a&&!r(e)){for(var b,c,d=[e],f=1,g=arguments;g.length>f;)d.push(g[f++]);return b=d[1],"function"==typeof b&&(c=b),(c||!P(b))&&(b=function(b,a){return c&&(a=c.call(this,b,a)),r(a)?void 0:a}),d[1]=b,m.apply(l,d)}},U=H(function(){var a=g();return"[null]"!=m([a])||"{}"!=m({a:a})||"{}"!=m(Object(a))});q||(g=function Symbol(){if(r(this))throw TypeError("Symbol is not a constructor");return L(S(arguments.length>0?arguments[0]:a))},G(g.prototype,"toString",function toString(){return this._k}),r=function(a){return a instanceof g},e.create=F,e.isEnum=E,e.getDesc=D,e.setDesc=t,e.setDescs=u,e.getNames=C.get=B,e.getSymbols=M,w&&!b(39)&&G(j,"propertyIsEnumerable",E,!0));var K={"for":function(a){return d(o,a+="")?o[a]:o[a]=g(a)},keyFor:function keyFor(a){return R(o,a)},useSetter:function(){n=!0},useSimple:function(){n=!1}};e.each.call("hasInstance,isConcatSpreadable,iterator,match,replace,search,species,split,toPrimitive,toStringTag,unscopables".split(","),function(a){var b=A(a);K[a]=q?b:L(b)}),n=!0,f(f.G+f.W,{Symbol:g}),f(f.S,"Symbol",K),f(f.S+f.F*!q,"Object",{create:F,defineProperty:t,defineProperties:u,getOwnPropertyDescriptor:D,getOwnPropertyNames:B,getOwnPropertySymbols:M}),l&&f(f.S+f.F*(!q||U),"JSON",{stringify:T}),s(g,"Symbol"),s(Math,"Math",!0),s(x.JSON,"JSON",!0)},function(c,f,a){var d=a(2).setDesc,e=a(17),b=a(31)("toStringTag");c.exports=function(a,c,f){a&&!e(a=f?a:a.prototype,b)&&d(a,b,{configurable:!0,value:c})}},function(b,e,a){var c=a(2),d=a(23);b.exports=function(g,h){for(var a,b=d(g),e=c.getKeys(b),i=e.length,f=0;i>f;)if(b[a=e[f++]]===h)return a}},function(d,h,a){var e=a(23),b=a(2).getNames,f={}.toString,c="object"==typeof window&&Object.getOwnPropertyNames?Object.getOwnPropertyNames(window):[],g=function(a){try{return b(a)}catch(d){return c.slice()}};d.exports.get=function getOwnPropertyNames(a){return c&&"[object Window]"==f.call(a)?g(a):b(e(a))}},function(b,d,c){var a=c(2);b.exports=function(b){var c=a.getKeys(b),d=a.getSymbols;if(d)for(var e,f=d(b),h=a.isEnum,g=0;f.length>g;)h.call(b,e=f[g++])&&c.push(e);return c}},function(a,b){a.exports=!1},function(c,d,b){var a=b(3);a(a.S+a.F,"Object",{assign:b(41)})},function(c,f,a){var b=a(2),d=a(21),e=a(24);c.exports=a(9)(function(){var a=Object.assign,b={},c={},d=Symbol(),e="abcdefghijklmnopqrst";return b[d]=7,e.split("").forEach(function(a){c[a]=a}),7!=a({},b)[d]||Object.keys(a({},c)).join("")!=e})?function assign(n,q){for(var g=d(n),h=arguments,o=h.length,j=1,f=b.getKeys,l=b.getSymbols,m=b.isEnum;o>j;)for(var c,a=e(h[j++]),k=l?f(a).concat(l(a)):f(a),p=k.length,i=0;p>i;)m.call(a,c=k[i++])&&(g[c]=a[c]);return g}:Object.assign},function(c,d,a){var b=a(3);b(b.S,"Object",{is:a(43)})},function(a,b){a.exports=Object.is||function is(a,b){return a===b?0!==a||1/a===1/b:a!=a&&b!=b}},function(c,d,a){var b=a(3);b(b.S,"Object",{setPrototypeOf:a(45).set})},function(d,h,b){var e=b(2).getDesc,f=b(16),g=b(20),c=function(b,a){if(g(b),!f(a)&&null!==a)throw TypeError(a+": can't set as prototype!")};d.exports={set:Object.setPrototypeOf||("__proto__"in{}?function(f,a,d){try{d=b(12)(Function.call,e(Object.prototype,"__proto__").set,2),d(f,[]),a=!(f instanceof Array)}catch(g){a=!0}return function setPrototypeOf(b,e){return c(b,e),a?b.__proto__=e:d(b,e),b}}({},!1):a),check:c}},function(d,e,a){var c=a(47),b={};b[a(31)("toStringTag")]="z",b+""!="[object z]"&&a(10)(Object.prototype,"toString",function toString(){return"[object "+c(this)+"]"},!0)},function(d,g,c){var b=c(18),e=c(31)("toStringTag"),f="Arguments"==b(function(){return arguments}());d.exports=function(d){var c,g,h;return d===a?"Undefined":null===d?"Null":"string"==typeof(g=(c=Object(d))[e])?g:f?b(c):"Object"==(h=b(c))&&"function"==typeof c.callee?"Arguments":h}},function(c,d,a){var b=a(16);a(49)("freeze",function(a){return function freeze(c){return a&&b(c)?a(c):c}})},function(c,f,a){var b=a(3),d=a(5),e=a(9);c.exports=function(a,g){var c=(d.Object||{})[a]||Object[a],f={};f[a]=g(c),b(b.S+b.F*e(function(){c(1)}),"Object",f)}},function(c,d,a){var b=a(16);a(49)("seal",function(a){return function seal(c){return a&&b(c)?a(c):c}})},function(c,d,a){var b=a(16);a(49)("preventExtensions",function(a){return function preventExtensions(c){return a&&b(c)?a(c):c}})},function(c,d,a){var b=a(16);a(49)("isFrozen",function(a){return function isFrozen(c){return b(c)?a?a(c):!1:!0}})},function(c,d,a){var b=a(16);a(49)("isSealed",function(a){return function isSealed(c){return b(c)?a?a(c):!1:!0}})},function(c,d,a){var b=a(16);a(49)("isExtensible",function(a){return function isExtensible(c){return b(c)?a?a(c):!0:!1}})},function(c,d,a){var b=a(23);a(49)("getOwnPropertyDescriptor",function(a){return function getOwnPropertyDescriptor(c,d){return a(b(c),d)}})},function(c,d,a){var b=a(21);a(49)("getPrototypeOf",function(a){return function getPrototypeOf(c){return a(b(c))}})},function(c,d,a){var b=a(21);a(49)("keys",function(a){return function keys(c){return a(b(c))}})},function(b,c,a){a(49)("getOwnPropertyNames",function(){return a(37).get})},function(h,i,a){var c=a(2).setDesc,e=a(7),f=a(17),d=Function.prototype,g=/^\s*function ([^ (]*)/,b="name";b in d||a(8)&&c(d,b,{configurable:!0,get:function(){var a=(""+this).match(g),d=a?a[1]:"";return f(this,b)||c(this,b,e(5,d)),d}})},function(f,g,a){var b=a(2),c=a(16),d=a(31)("hasInstance"),e=Function.prototype;d in e||b.setDesc(e,d,{value:function(a){if("function"!=typeof this||!c(a))return!1;if(!c(this.prototype))return a instanceof this;for(;a=b.getProto(a);)if(this.prototype===a)return!0;return!1}})},function(q,p,b){var c=b(2),h=b(4),i=b(17),j=b(18),l=b(62),k=b(9),n=b(63).trim,d="Number",a=h[d],e=a,f=a.prototype,o=j(c.create(f))==d,m="trim"in String.prototype,g=function(i){var a=l(i,!1);if("string"==typeof a&&a.length>2){a=m?a.trim():n(a,3);var b,c,d,e=a.charCodeAt(0);if(43===e||45===e){if(b=a.charCodeAt(2),88===b||120===b)return NaN}else if(48===e){switch(a.charCodeAt(1)){case 66:case 98:c=2,d=49;break;case 79:case 111:c=8,d=55;break;default:return+a}for(var f,g=a.slice(2),h=0,j=g.length;j>h;h++)if(f=g.charCodeAt(h),48>f||f>d)return NaN;return parseInt(g,c)}}return+a};a(" 0o1")&&a("0b1")&&!a("+0x1")||(a=function Number(h){var c=arguments.length<1?0:h,b=this;return b instanceof a&&(o?k(function(){f.valueOf.call(b)}):j(b)!=d)?new e(g(c)):g(c)},c.each.call(b(8)?c.getNames(e):"MAX_VALUE,MIN_VALUE,NaN,NEGATIVE_INFINITY,POSITIVE_INFINITY,EPSILON,isFinite,isInteger,isNaN,isSafeInteger,MAX_SAFE_INTEGER,MIN_SAFE_INTEGER,parseFloat,parseInt,isInteger".split(","),function(b){i(e,b)&&!i(a,b)&&c.setDesc(a,b,c.getDesc(e,b))}),a.prototype=f,f.constructor=a,b(10)(h,d,a))},function(b,d,c){var a=c(16);b.exports=function(b,e){if(!a(b))return b;var c,d;if(e&&"function"==typeof(c=b.toString)&&!a(d=c.call(b)))return d;if("function"==typeof(c=b.valueOf)&&!a(d=c.call(b)))return d;if(!e&&"function"==typeof(c=b.toString)&&!a(d=c.call(b)))return d;throw TypeError("Can't convert object to primitive value")}},function(g,m,b){var c=b(3),h=b(22),i=b(9),d=" \n\f\r \u2028\u2029\ufeff",a="["+d+"]",f="
",j=RegExp("^"+a+a+"*"),k=RegExp(a+a+"*$"),e=function(a,e){var b={};b[a]=e(l),c(c.P+c.F*i(function(){return!!d[a]()||f[a]()!=f}),"String",b)},l=e.trim=function(a,b){return a=String(h(a)),1&b&&(a=a.replace(j,"")),2&b&&(a=a.replace(k,"")),a};g.exports=e},function(c,d,b){var a=b(3);a(a.S,"Number",{EPSILON:Math.pow(2,-52)})},function(d,e,a){var b=a(3),c=a(4).isFinite;b(b.S,"Number",{isFinite:function isFinite(a){return"number"==typeof a&&c(a)}})},function(c,d,a){var b=a(3);b(b.S,"Number",{isInteger:a(67)})},function(a,e,b){var c=b(16),d=Math.floor;a.exports=function isInteger(a){return!c(a)&&isFinite(a)&&d(a)===a}},function(c,d,b){var a=b(3);a(a.S,"Number",{isNaN:function isNaN(a){return a!=a}})},function(e,f,a){var b=a(3),c=a(67),d=Math.abs;b(b.S,"Number",{isSafeInteger:function isSafeInteger(a){return c(a)&&d(a)<=9007199254740991}})},function(c,d,b){var a=b(3);a(a.S,"Number",{MAX_SAFE_INTEGER:9007199254740991})},function(c,d,b){var a=b(3);a(a.S,"Number",{MIN_SAFE_INTEGER:-9007199254740991})},function(c,d,b){var a=b(3);a(a.S,"Number",{parseFloat:parseFloat})},function(c,d,b){var a=b(3);a(a.S,"Number",{parseInt:parseInt})},function(f,g,b){var a=b(3),e=b(75),c=Math.sqrt,d=Math.acosh;a(a.S+a.F*!(d&&710==Math.floor(d(Number.MAX_VALUE))),"Math",{acosh:function acosh(a){return(a=+a)<1?NaN:a>94906265.62425156?Math.log(a)+Math.LN2:e(a-1+c(a-1)*c(a+1))}})},function(a,b){a.exports=Math.log1p||function log1p(a){return(a=+a)>-1e-8&&1e-8>a?a-a*a/2:Math.log(1+a)}},function(c,d,b){function asinh(a){return isFinite(a=+a)&&0!=a?0>a?-asinh(-a):Math.log(a+Math.sqrt(a*a+1)):a}var a=b(3);a(a.S,"Math",{asinh:asinh})},function(c,d,b){var a=b(3);a(a.S,"Math",{atanh:function atanh(a){return 0==(a=+a)?a:Math.log((1+a)/(1-a))/2}})},function(d,e,a){var b=a(3),c=a(79);b(b.S,"Math",{cbrt:function cbrt(a){return c(a=+a)*Math.pow(Math.abs(a),1/3)}})},function(a,b){a.exports=Math.sign||function sign(a){return 0==(a=+a)||a!=a?a:0>a?-1:1}},function(c,d,b){var a=b(3);a(a.S,"Math",{clz32:function clz32(a){return(a>>>=0)?31-Math.floor(Math.log(a+.5)*Math.LOG2E):32}})},function(d,e,c){var a=c(3),b=Math.exp;a(a.S,"Math",{cosh:function cosh(a){return(b(a=+a)+b(-a))/2}})},function(c,d,a){var b=a(3);b(b.S,"Math",{expm1:a(83)})},function(a,b){a.exports=Math.expm1||function expm1(a){return 0==(a=+a)?a:a>-1e-6&&1e-6>a?a+a*a/2:Math.exp(a)-1}},function(k,j,e){var f=e(3),g=e(79),a=Math.pow,d=a(2,-52),b=a(2,-23),i=a(2,127)*(2-b),c=a(2,-126),h=function(a){return a+1/d-1/d};f(f.S,"Math",{fround:function fround(k){var f,a,e=Math.abs(k),j=g(k);return c>e?j*h(e/c/b)*c*b:(f=(1+b/d)*e,a=f-(f-e),a>i||a!=a?j*(1/0):j*a)}})},function(d,e,b){var a=b(3),c=Math.abs;a(a.S,"Math",{hypot:function hypot(i,j){for(var a,b,e=0,f=0,g=arguments,h=g.length,d=0;h>f;)a=c(g[f++]),a>d?(b=d/a,e=e*b*b+1,d=a):a>0?(b=a/d,e+=b*b):e+=a;return d===1/0?1/0:d*Math.sqrt(e)}})},function(d,e,b){var a=b(3),c=Math.imul;a(a.S+a.F*b(9)(function(){return-5!=c(4294967295,5)||2!=c.length}),"Math",{imul:function imul(f,g){var a=65535,b=+f,c=+g,d=a&b,e=a&c;return 0|d*e+((a&b>>>16)*e+d*(a&c>>>16)<<16>>>0)}})},function(c,d,b){var a=b(3);a(a.S,"Math",{log10:function log10(a){return Math.log(a)/Math.LN10}})},function(c,d,a){var b=a(3);b(b.S,"Math",{log1p:a(75)})},function(c,d,b){var a=b(3);a(a.S,"Math",{log2:function log2(a){return Math.log(a)/Math.LN2}})},function(c,d,a){var b=a(3);b(b.S,"Math",{sign:a(79)})},function(e,f,a){var b=a(3),c=a(83),d=Math.exp;b(b.S+b.F*a(9)(function(){return-2e-17!=!Math.sinh(-2e-17)}),"Math",{sinh:function sinh(a){return Math.abs(a=+a)<1?(c(a)-c(-a))/2:(d(a-1)-d(-a-1))*(Math.E/2)}})},function(e,f,a){var b=a(3),c=a(83),d=Math.exp;b(b.S,"Math",{tanh:function tanh(a){var b=c(a=+a),e=c(-a);return b==1/0?1:e==1/0?-1:(b-e)/(d(a)+d(-a))}})},function(c,d,b){var a=b(3);a(a.S,"Math",{trunc:function trunc(a){return(a>0?Math.floor:Math.ceil)(a)}})},function(f,g,b){var a=b(3),e=b(26),c=String.fromCharCode,d=String.fromCodePoint;a(a.S+a.F*(!!d&&1!=d.length),"String",{fromCodePoint:function fromCodePoint(h){for(var a,b=[],d=arguments,g=d.length,f=0;g>f;){if(a=+d[f++],e(a,1114111)!==a)throw RangeError(a+" is not a valid code point");b.push(65536>a?c(a):c(((a-=65536)>>10)+55296,a%1024+56320))}return b.join("")}})},function(e,f,a){var b=a(3),c=a(23),d=a(27);b(b.S,"String",{raw:function raw(g){for(var e=c(g.raw),h=d(e.length),f=arguments,i=f.length,b=[],a=0;h>a;)b.push(String(e[a++])),i>a&&b.push(String(f[a]));return b.join("")}})},function(b,c,a){a(63)("trim",function(a){return function trim(){return a(this,3)}})},function(d,e,a){var b=a(3),c=a(98)(!1);b(b.P,"String",{codePointAt:function codePointAt(a){return c(this,a)}})},function(c,f,b){var d=b(25),e=b(22);c.exports=function(b){return function(j,k){var f,h,g=String(e(j)),c=d(k),i=g.length;return 0>c||c>=i?b?"":a:(f=g.charCodeAt(c),55296>f||f>56319||c+1===i||(h=g.charCodeAt(c+1))<56320||h>57343?b?g.charAt(c):f:b?g.slice(c,c+2):(f-55296<<10)+(h-56320)+65536)}}},function(h,i,b){var c=b(3),e=b(27),g=b(100),d="endsWith",f=""[d];c(c.P+c.F*b(102)(d),"String",{endsWith:function endsWith(i){var b=g(this,i,d),j=arguments,k=j.length>1?j[1]:a,l=e(b.length),c=k===a?l:Math.min(e(k),l),h=String(i);return f?f.call(b,h,c):b.slice(c-h.length,c)===h}})},function(b,e,a){var c=a(101),d=a(22);b.exports=function(a,b,e){if(c(b))throw TypeError("String#"+e+" doesn't accept regex!");return String(d(a))}},function(c,g,b){var d=b(16),e=b(18),f=b(31)("match");c.exports=function(b){var c;return d(b)&&((c=b[f])!==a?!!c:"RegExp"==e(b))}},function(a,d,b){var c=b(31)("match");a.exports=function(b){var a=/./;try{"/./"[b](a)}catch(d){try{return a[c]=!1,!"/./"[b](a)}catch(e){}}return!0}},function(f,g,b){var c=b(3),e=b(100),d="includes";c(c.P+c.F*b(102)(d),"String",{includes:function includes(b){return!!~e(this,b,d).indexOf(b,arguments.length>1?arguments[1]:a)}})},function(c,d,a){var b=a(3);b(b.P,"String",{repeat:a(105)})},function(b,e,a){var c=a(25),d=a(22);b.exports=function repeat(f){var b=String(d(this)),e="",a=c(f);if(0>a||a==1/0)throw RangeError("Count can't be negative");for(;a>0;(a>>>=1)&&(b+=b))1&a&&(e+=b);return e}},function(h,i,b){var c=b(3),f=b(27),g=b(100),d="startsWith",e=""[d];c(c.P+c.F*b(102)(d),"String",{startsWith:function startsWith(i){var b=g(this,i,d),j=arguments,c=f(Math.min(j.length>1?j[1]:a,b.length)),h=String(i);return e?e.call(b,h,c):b.slice(c,c+h.length)===h}})},function(d,e,b){var c=b(98)(!0);b(108)(String,"String",function(a){this._t=String(a),this._i=0},function(){var b,d=this._t,e=this._i;return e>=d.length?{value:a,done:!0}:(b=c(d,e),this._i+=b.length,{value:b,done:!1})})},function(o,r,a){var i=a(39),d=a(3),n=a(10),h=a(6),m=a(17),f=a(109),q=a(110),p=a(35),l=a(2).getProto,c=a(31)("iterator"),e=!([].keys&&"next"in[].keys()),j="@@iterator",k="keys",b="values",g=function(){return this};o.exports=function(B,v,u,F,s,E,A){q(u,v,F);var r,x,w=function(c){if(!e&&c in a)return a[c];switch(c){case k:return function keys(){return new u(this,c)};case b:return function values(){return new u(this,c)}}return function entries(){return new u(this,c)}},C=v+" Iterator",y=s==b,z=!1,a=B.prototype,t=a[c]||a[j]||s&&a[s],o=t||w(s);if(t){var D=l(o.call(new B));p(D,C,!0),!i&&m(a,j)&&h(D,c,g),y&&t.name!==b&&(z=!0,o=function values(){return t.call(this)})}if(i&&!A||!e&&!z&&a[c]||h(a,c,o),f[v]=o,f[C]=g,s)if(r={values:y?o:w(b),keys:E?o:w(k),entries:y?w("entries"):o},A)for(x in r)x in a||n(a,x,r[x]);else d(d.P+d.F*(e||z),v,r);return r}},function(a,b){a.exports={}},function(c,g,a){var d=a(2),e=a(7),f=a(35),b={};a(6)(b,a(31)("iterator"),function(){return this}),c.exports=function(a,c,g){a.prototype=d.create(b,{next:e(1,g)}),f(a,c+" Iterator")}},function(j,k,b){var d=b(12),c=b(3),e=b(21),f=b(112),g=b(113),h=b(27),i=b(114);c(c.S+c.F*!b(115)(function(a){Array.from(a)}),"Array",{from:function from(t){var n,c,r,m,j=e(t),l="function"==typeof this?this:Array,p=arguments,s=p.length,k=s>1?p[1]:a,q=k!==a,b=0,o=i(j);if(q&&(k=d(k,s>2?p[2]:a,2)),o==a||l==Array&&g(o))for(n=h(j.length),c=new l(n);n>b;b++)c[b]=q?k(j[b],b):j[b];else for(m=o.call(j),c=new l;!(r=m.next()).done;b++)c[b]=q?f(m,k,[r.value,b],!0):r.value;return c.length=b,c}})},function(c,e,d){var b=d(20);c.exports=function(d,e,c,g){try{return g?e(b(c)[0],c[1]):e(c)}catch(h){var f=d["return"];throw f!==a&&b(f.call(d)),h}}},function(c,g,b){var d=b(109),e=b(31)("iterator"),f=Array.prototype;c.exports=function(b){return b!==a&&(d.Array===b||f[e]===b)}},function(c,g,b){var d=b(47),e=b(31)("iterator"),f=b(109);c.exports=b(5).getIteratorMethod=function(b){return b!=a?b[e]||b["@@iterator"]||f[d(b)]:void 0}},function(d,f,e){var a=e(31)("iterator"),b=!1;try{var c=[7][a]();c["return"]=function(){b=!0},Array.from(c,function(){throw 2})}catch(g){}d.exports=function(f,g){if(!g&&!b)return!1;var d=!1;try{var c=[7],e=c[a]();e.next=function(){return{done:d=!0}},c[a]=function(){return e},f(c)}catch(h){}return d}},function(c,d,b){var a=b(3);a(a.S+a.F*b(9)(function(){function F(){}return!(Array.of.call(F)instanceof F)}),"Array",{of:function of(){for(var a=0,d=arguments,b=d.length,c=new("function"==typeof this?this:Array)(b);b>a;)c[a]=d[a++];return c.length=b,c}})},function(f,h,b){var d=b(118),c=b(119),e=b(109),g=b(23);f.exports=b(108)(Array,"Array",function(a,b){this._t=g(a),this._i=0,this._k=b},function(){var d=this._t,e=this._k,b=this._i++;return!d||b>=d.length?(this._t=a,c(1)):"keys"==e?c(0,b):"values"==e?c(0,d[b]):c(0,[b,d[b]])},"values"),e.Arguments=e.Array,d("keys"),d("values"),d("entries")},function(e,f,d){var b=d(31)("unscopables"),c=Array.prototype;c[b]==a&&d(6)(c,b,{}),e.exports=function(a){c[b][a]=!0}},function(a,b){a.exports=function(a,b){return{value:b,done:!!a}}},function(b,c,a){a(121)("Array")},function(c,g,a){var d=a(4),e=a(2),f=a(8),b=a(31)("species");c.exports=function(c){var a=d[c];f&&a&&!a[b]&&e.setDesc(a,b,{configurable:!0,get:function(){return this}})}},function(c,d,a){var b=a(3);b(b.P,"Array",{copyWithin:a(123)}),a(118)("copyWithin")},function(d,g,b){var e=b(21),c=b(26),f=b(27);d.exports=[].copyWithin||function copyWithin(m,n){var g=e(this),h=f(g.length),b=c(m,h),d=c(n,h),k=arguments,l=k.length>2?k[2]:a,i=Math.min((l===a?h:c(l,h))-d,h-b),j=1;for(b>d&&d+i>b&&(j=-1,d+=i-1,b+=i-1);i-->0;)d in g?g[b]=g[d]:delete g[b],b+=j,d+=j;return g}},function(c,d,a){var b=a(3);b(b.P,"Array",{fill:a(125)}),a(118)("fill")},function(d,g,b){var e=b(21),c=b(26),f=b(27);d.exports=[].fill||function fill(k){for(var b=e(this),d=f(b.length),g=arguments,h=g.length,i=c(h>1?g[1]:a,d),j=h>2?g[2]:a,l=j===a?d:c(j,d);l>i;)b[i++]=k;return b}},function(g,h,b){var c=b(3),f=b(28)(5),d="find",e=!0;d in[]&&Array(1)[d](function(){e=!1}),c(c.P+c.F*e,"Array",{find:function find(b){return f(this,b,arguments.length>1?arguments[1]:a)}}),b(118)(d)},function(g,h,b){var c=b(3),f=b(28)(6),d="findIndex",e=!0;d in[]&&Array(1)[d](function(){e=!1}),c(c.P+c.F*e,"Array",{findIndex:function findIndex(b){return f(this,b,arguments.length>1?arguments[1]:a)}}),b(118)(d)},function(n,m,c){var f=c(2),i=c(4),k=c(101),l=c(129),b=i.RegExp,d=b,j=b.prototype,e=/a/g,g=/a/g,h=new b(e)!==e;!c(8)||h&&!c(9)(function(){return g[c(31)("match")]=!1,b(e)!=e||b(g)==g||"/a/i"!=b(e,"i")})||(b=function RegExp(c,f){var e=k(c),g=f===a;return this instanceof b||!e||c.constructor!==b||!g?h?new d(e&&!g?c.source:c,f):d((e=c instanceof b)?c.source:c,e&&g?l.call(c):f):c},f.each.call(f.getNames(d),function(a){a in b||f.setDesc(b,a,{configurable:!0,get:function(){return d[a]},set:function(b){d[a]=b}})}),j.constructor=b,b.prototype=j,c(10)(i,"RegExp",b)),c(121)("RegExp")},function(a,d,b){var c=b(20);a.exports=function(){var b=c(this),a="";return b.global&&(a+="g"),b.ignoreCase&&(a+="i"),b.multiline&&(a+="m"),b.unicode&&(a+="u"),b.sticky&&(a+="y"),a}},function(c,d,a){var b=a(2);a(8)&&"g"!=/./g.flags&&b.setDesc(RegExp.prototype,"flags",{configurable:!0,get:a(129)})},function(c,d,b){b(132)("match",1,function(c,b){return function match(d){var e=c(this),f=d==a?a:d[b];return f!==a?f.call(d,e):new RegExp(d)[b](String(e))}})},function(b,h,a){var c=a(6),d=a(10),e=a(9),f=a(22),g=a(31);b.exports=function(a,i,j){var b=g(a),h=""[a];e(function(){var c={};return c[b]=function(){return 7},7!=""[a](c)})&&(d(String.prototype,a,j(f,b,h)),c(RegExp.prototype,b,2==i?function(a,b){return h.call(a,this,b)}:function(a){return h.call(a,this)}))}},function(c,d,b){b(132)("replace",2,function(b,c,d){return function replace(e,f){var g=b(this),h=e==a?a:e[c];return h!==a?h.call(e,g,f):d.call(String(g),e,f)}})},function(c,d,b){b(132)("search",1,function(c,b){return function search(d){var e=c(this),f=d==a?a:d[b];return f!==a?f.call(d,e):new RegExp(d)[b](String(e))}})},function(c,d,b){b(132)("split",2,function(b,c,d){return function split(e,f){var g=b(this),h=e==a?a:e[c];return h!==a?h.call(e,g,f):d.call(String(g),e,f)}})},function(K,J,b){var s,l=b(2),F=b(39),k=b(4),j=b(12),I=b(47),d=b(3),D=b(16),E=b(20),m=b(13),G=b(137),p=b(138),q=b(45).set,A=b(43),B=b(31)("species"),z=b(139),v=b(140),e="Promise",o=k.process,H="process"==I(o),c=k[e],i=function(){},r=function(d){var b,a=new c(i);return d&&(a.constructor=function(a){a(i,i)}),(b=c.resolve(a))["catch"](i),b===a},h=function(){function P2(b){var a=new c(b);return q(a,P2.prototype),a}var a=!1;try{if(a=c&&c.resolve&&r(),q(P2,c),
+P2.prototype=l.create(c.prototype,{constructor:{value:P2}}),P2.resolve(5).then(function(){})instanceof P2||(a=!1),a&&b(8)){var d=!1;c.resolve(l.setDesc({},"then",{get:function(){d=!0}})),a=d}}catch(e){a=!1}return a}(),C=function(a,b){return F&&a===c&&b===s?!0:A(a,b)},t=function(b){var c=E(b)[B];return c!=a?c:b},u=function(a){var b;return D(a)&&"function"==typeof(b=a.then)?b:!1},g=function(d){var b,c;this.promise=new d(function(d,e){if(b!==a||c!==a)throw TypeError("Bad Promise constructor");b=d,c=e}),this.resolve=m(b),this.reject=m(c)},w=function(a){try{a()}catch(b){return{error:b}}},n=function(b,d){if(!b.n){b.n=!0;var c=b.c;v(function(){for(var e=b.v,f=1==b.s,g=0,h=function(a){var c,h,g=f?a.ok:a.fail,i=a.resolve,d=a.reject;try{g?(f||(b.h=!0),c=g===!0?e:g(e),c===a.promise?d(TypeError("Promise-chain cycle")):(h=u(c))?h.call(c,i,d):i(c)):d(e)}catch(j){d(j)}};c.length>g;)h(c[g++]);c.length=0,b.n=!1,d&&setTimeout(function(){var f,c,d=b.p;y(d)&&(H?o.emit("unhandledRejection",e,d):(f=k.onunhandledrejection)?f({promise:d,reason:e}):(c=k.console)&&c.error&&c.error("Unhandled promise rejection",e)),b.a=a},1)})}},y=function(e){var a,b=e._d,c=b.a||b.c,d=0;if(b.h)return!1;for(;c.length>d;)if(a=c[d++],a.fail||!y(a.promise))return!1;return!0},f=function(b){var a=this;a.d||(a.d=!0,a=a.r||a,a.v=b,a.s=2,a.a=a.c.slice(),n(a,!0))},x=function(b){var c,a=this;if(!a.d){a.d=!0,a=a.r||a;try{if(a.p===b)throw TypeError("Promise can't be resolved itself");(c=u(b))?v(function(){var d={r:a,d:!1};try{c.call(b,j(x,d,1),j(f,d,1))}catch(e){f.call(d,e)}}):(a.v=b,a.s=1,n(a,!1))}catch(d){f.call({r:a,d:!1},d)}}};h||(c=function Promise(d){m(d);var b=this._d={p:G(this,c,e),c:[],a:a,s:0,d:!1,v:a,h:!1,n:!1};try{d(j(x,b,1),j(f,b,1))}catch(g){f.call(b,g)}},b(142)(c.prototype,{then:function then(d,e){var a=new g(z(this,c)),f=a.promise,b=this._d;return a.ok="function"==typeof d?d:!0,a.fail="function"==typeof e&&e,b.c.push(a),b.a&&b.a.push(a),b.s&&n(b,!1),f},"catch":function(b){return this.then(a,b)}})),d(d.G+d.W+d.F*!h,{Promise:c}),b(35)(c,e),b(121)(e),s=b(5)[e],d(d.S+d.F*!h,e,{reject:function reject(b){var a=new g(this),c=a.reject;return c(b),a.promise}}),d(d.S+d.F*(!h||r(!0)),e,{resolve:function resolve(a){if(a instanceof c&&C(a.constructor,this))return a;var b=new g(this),d=b.resolve;return d(a),b.promise}}),d(d.S+d.F*!(h&&b(115)(function(a){c.all(a)["catch"](function(){})})),e,{all:function all(h){var c=t(this),b=new g(c),d=b.resolve,e=b.reject,a=[],f=w(function(){p(h,!1,a.push,a);var b=a.length,f=Array(b);b?l.each.call(a,function(g,h){var a=!1;c.resolve(g).then(function(c){a||(a=!0,f[h]=c,--b||d(f))},e)}):d(f)});return f&&e(f.error),b.promise},race:function race(e){var b=t(this),a=new g(b),c=a.reject,d=w(function(){p(e,!1,function(d){b.resolve(d).then(a.resolve,c)})});return d&&c(d.error),a.promise}})},function(a,b){a.exports=function(a,b,c){if(!(a instanceof b))throw TypeError(c+": use the 'new' operator!");return a}},function(b,i,a){var c=a(12),d=a(112),e=a(113),f=a(20),g=a(27),h=a(114);b.exports=function(a,j,o,p){var n,b,k,l=h(a),m=c(o,p,j?2:1),i=0;if("function"!=typeof l)throw TypeError(a+" is not iterable!");if(e(l))for(n=g(a.length);n>i;i++)j?m(f(b=a[i])[0],b[1]):m(a[i]);else for(k=l.call(a);!(b=k.next()).done;)d(k,m,b.value,j)}},function(d,g,b){var c=b(20),e=b(13),f=b(31)("species");d.exports=function(g,h){var b,d=c(g).constructor;return d===a||(b=c(d)[f])==a?h:e(b)}},function(n,p,h){var b,f,g,c=h(4),o=h(141).set,k=c.MutationObserver||c.WebKitMutationObserver,d=c.process,i=c.Promise,j="process"==h(18)(d),e=function(){var e,c,g;for(j&&(e=d.domain)&&(d.domain=null,e.exit());b;)c=b.domain,g=b.fn,c&&c.enter(),g(),c&&c.exit(),b=b.next;f=a,e&&e.enter()};if(j)g=function(){d.nextTick(e)};else if(k){var m=1,l=document.createTextNode("");new k(e).observe(l,{characterData:!0}),g=function(){l.data=m=-m}}else g=i&&i.resolve?function(){i.resolve().then(e)}:function(){o.call(c,e)};n.exports=function asap(e){var c={fn:e,next:a,domain:j&&d.domain};f&&(f.next=c),b||(b=c,g()),f=c}},function(s,t,b){var c,g,f,k=b(12),r=b(19),n=b(14),p=b(15),a=b(4),l=a.process,h=a.setImmediate,i=a.clearImmediate,o=a.MessageChannel,j=0,d={},q="onreadystatechange",e=function(){var a=+this;if(d.hasOwnProperty(a)){var b=d[a];delete d[a],b()}},m=function(a){e.call(a.data)};h&&i||(h=function setImmediate(a){for(var b=[],e=1;arguments.length>e;)b.push(arguments[e++]);return d[++j]=function(){r("function"==typeof a?a:Function(a),b)},c(j),j},i=function clearImmediate(a){delete d[a]},"process"==b(18)(l)?c=function(a){l.nextTick(k(e,a,1))}:o?(g=new o,f=g.port2,g.port1.onmessage=m,c=k(f.postMessage,f,1)):a.addEventListener&&"function"==typeof postMessage&&!a.importScripts?(c=function(b){a.postMessage(b+"","*")},a.addEventListener("message",m,!1)):c=q in p("script")?function(a){n.appendChild(p("script"))[q]=function(){n.removeChild(this),e.call(a)}}:function(a){setTimeout(k(e,a,1),0)}),s.exports={set:h,clear:i}},function(a,d,b){var c=b(10);a.exports=function(a,b){for(var d in b)c(a,d,b[d]);return a}},function(d,e,c){var b=c(144);c(145)("Map",function(b){return function Map(){return b(this,arguments.length>0?arguments[0]:a)}},{get:function get(c){var a=b.getEntry(this,c);return a&&a.v},set:function set(a,c){return b.def(this,0===a?0:a,c)}},b,!0)},function(v,w,b){var j=b(2),m=b(6),o=b(142),n=b(12),p=b(137),r=b(22),t=b(138),l=b(108),d=b(119),f=b(11)("id"),k=b(17),h=b(16),q=b(121),i=b(8),s=Object.isExtensible||h,c=i?"_s":"size",u=0,g=function(a,b){if(!h(a))return"symbol"==typeof a?a:("string"==typeof a?"S":"P")+a;if(!k(a,f)){if(!s(a))return"F";if(!b)return"E";m(a,f,++u)}return"O"+a[f]},e=function(b,c){var a,d=g(c);if("F"!==d)return b._i[d];for(a=b._f;a;a=a.n)if(a.k==c)return a};v.exports={getConstructor:function(d,f,g,h){var b=d(function(d,e){p(d,b,f),d._i=j.create(null),d._f=a,d._l=a,d[c]=0,e!=a&&t(e,g,d[h],d)});return o(b.prototype,{clear:function clear(){for(var d=this,e=d._i,b=d._f;b;b=b.n)b.r=!0,b.p&&(b.p=b.p.n=a),delete e[b.i];d._f=d._l=a,d[c]=0},"delete":function(g){var b=this,a=e(b,g);if(a){var d=a.n,f=a.p;delete b._i[a.i],a.r=!0,f&&(f.n=d),d&&(d.p=f),b._f==a&&(b._f=d),b._l==a&&(b._l=f),b[c]--}return!!a},forEach:function forEach(c){for(var b,d=n(c,arguments.length>1?arguments[1]:a,3);b=b?b.n:this._f;)for(d(b.v,b.k,this);b&&b.r;)b=b.p},has:function has(a){return!!e(this,a)}}),i&&j.setDesc(b.prototype,"size",{get:function(){return r(this[c])}}),b},def:function(b,f,j){var h,i,d=e(b,f);return d?d.v=j:(b._l=d={i:i=g(f,!0),k:f,v:j,p:h=b._l,n:a,r:!1},b._f||(b._f=d),h&&(h.n=d),b[c]++,"F"!==i&&(b._i[i]=d)),b},getEntry:e,setStrong:function(e,b,c){l(e,b,function(b,c){this._t=b,this._k=c,this._l=a},function(){for(var c=this,e=c._k,b=c._l;b&&b.r;)b=b.p;return c._t&&(c._l=b=b?b.n:c._t._f)?"keys"==e?d(0,b.k):"values"==e?d(0,b.v):d(0,[b.k,b.v]):(c._t=a,d(1))},c?"entries":"values",!c,!0),q(b)}}},function(l,n,b){var k=b(4),c=b(3),g=b(10),f=b(142),i=b(138),j=b(137),d=b(16),e=b(9),h=b(115),m=b(35);l.exports=function(o,v,y,x,p,l){var t=k[o],b=t,s=p?"set":"add",n=b&&b.prototype,w={},r=function(b){var c=n[b];g(n,b,"delete"==b?function(a){return l&&!d(a)?!1:c.call(this,0===a?0:a)}:"has"==b?function has(a){return l&&!d(a)?!1:c.call(this,0===a?0:a)}:"get"==b?function get(b){return l&&!d(b)?a:c.call(this,0===b?0:b)}:"add"==b?function add(a){return c.call(this,0===a?0:a),this}:function set(a,b){return c.call(this,0===a?0:a,b),this})};if("function"==typeof b&&(l||n.forEach&&!e(function(){(new b).entries().next()}))){var u,q=new b,z=q[s](l?{}:-0,1)!=q,A=e(function(){q.has(1)}),B=h(function(a){new b(a)});B||(b=v(function(e,d){j(e,b,o);var c=new t;return d!=a&&i(d,p,c[s],c),c}),b.prototype=n,n.constructor=b),l||q.forEach(function(b,a){u=1/a===-(1/0)}),(A||u)&&(r("delete"),r("has"),p&&r("get")),(u||z)&&r(s),l&&n.clear&&delete n.clear}else b=x.getConstructor(v,o,p,s),f(b.prototype,y);return m(b,o),w[o]=b,c(c.G+c.W+c.F*(b!=t),w),l||x.setStrong(b,o,p),b}},function(d,e,b){var c=b(144);b(145)("Set",function(b){return function Set(){return b(this,arguments.length>0?arguments[0]:a)}},{add:function add(a){return c.def(this,a=0===a?0:a,a)}},c)},function(n,m,b){var l=b(2),k=b(10),c=b(148),d=b(16),j=b(17),i=c.frozenStore,h=c.WEAK,f=Object.isExtensible||d,e={},g=b(145)("WeakMap",function(b){return function WeakMap(){return b(this,arguments.length>0?arguments[0]:a)}},{get:function get(a){if(d(a)){if(!f(a))return i(this).get(a);if(j(a,h))return a[h][this._i]}},set:function set(a,b){return c.def(this,a,b)}},c,!0,!0);7!=(new g).set((Object.freeze||Object)(e),7).get(e)&&l.each.call(["delete","has","get","set"],function(a){var b=g.prototype,c=b[a];k(b,a,function(b,e){if(d(b)&&!f(b)){var g=i(this)[a](b,e);return"set"==a?this:g}return c.call(this,b,e)})})},function(s,t,b){var r=b(6),q=b(142),m=b(20),h=b(16),l=b(137),k=b(138),j=b(28),d=b(17),c=b(11)("weak"),g=Object.isExtensible||h,n=j(5),o=j(6),p=0,e=function(a){return a._l||(a._l=new i)},i=function(){this.a=[]},f=function(a,b){return n(a.a,function(a){return a[0]===b})};i.prototype={get:function(b){var a=f(this,b);return a?a[1]:void 0},has:function(a){return!!f(this,a)},set:function(a,b){var c=f(this,a);c?c[1]=b:this.a.push([a,b])},"delete":function(b){var a=o(this.a,function(a){return a[0]===b});return~a&&this.a.splice(a,1),!!~a}},s.exports={getConstructor:function(f,i,j,m){var b=f(function(c,d){l(c,b,i),c._i=p++,c._l=a,d!=a&&k(d,j,c[m],c)});return q(b.prototype,{"delete":function(a){return h(a)?g(a)?d(a,c)&&d(a[c],this._i)&&delete a[c][this._i]:e(this)["delete"](a):!1},has:function has(a){return h(a)?g(a)?d(a,c)&&d(a[c],this._i):e(this).has(a):!1}}),b},def:function(b,a,f){return g(m(a))?(d(a,c)||r(a,c,{}),a[c][b._i]=f):e(b).set(a,f),b},frozenStore:e,WEAK:c}},function(d,e,b){var c=b(148);b(145)("WeakSet",function(b){return function WeakSet(){return b(this,arguments.length>0?arguments[0]:a)}},{add:function add(a){return c.def(this,a,!0)}},c,!1,!0)},function(e,f,a){var b=a(3),c=Function.apply,d=a(20);b(b.S,"Reflect",{apply:function apply(a,b,e){return c.call(a,b,d(e))}})},function(h,i,a){var e=a(2),b=a(3),c=a(13),f=a(20),d=a(16),g=Function.bind||a(5).Function.prototype.bind;b(b.S+b.F*a(9)(function(){function F(){}return!(Reflect.construct(function(){},[],F)instanceof F)}),"Reflect",{construct:function construct(b,a){c(b),f(a);var i=arguments.length<3?b:c(arguments[2]);if(b==i){switch(a.length){case 0:return new b;case 1:return new b(a[0]);case 2:return new b(a[0],a[1]);case 3:return new b(a[0],a[1],a[2]);case 4:return new b(a[0],a[1],a[2],a[3])}var h=[null];return h.push.apply(h,a),new(g.apply(b,h))}var j=i.prototype,k=e.create(d(j)?j:Object.prototype),l=Function.apply.call(b,k,a);return d(l)?l:k}})},function(e,f,a){var c=a(2),b=a(3),d=a(20);b(b.S+b.F*a(9)(function(){Reflect.defineProperty(c.setDesc({},1,{value:1}),1,{value:2})}),"Reflect",{defineProperty:function defineProperty(a,b,e){d(a);try{return c.setDesc(a,b,e),!0}catch(f){return!1}}})},function(e,f,a){var b=a(3),c=a(2).getDesc,d=a(20);b(b.S,"Reflect",{deleteProperty:function deleteProperty(a,b){var e=c(d(a),b);return e&&!e.configurable?!1:delete a[b]}})},function(f,g,b){var c=b(3),e=b(20),d=function(a){this._t=e(a),this._i=0;var b,c=this._k=[];for(b in a)c.push(b)};b(110)(d,"Object",function(){var c,b=this,d=b._k;do if(b._i>=d.length)return{value:a,done:!0};while(!((c=d[b._i++])in b._t));return{value:c,done:!1}}),c(c.S,"Reflect",{enumerate:function enumerate(a){return new d(a)}})},function(h,i,b){function get(b,h){var d,j,i=arguments.length<3?b:arguments[2];return g(b)===i?b[h]:(d=c.getDesc(b,h))?e(d,"value")?d.value:d.get!==a?d.get.call(i):a:f(j=c.getProto(b))?get(j,h,i):void 0}var c=b(2),e=b(17),d=b(3),f=b(16),g=b(20);d(d.S,"Reflect",{get:get})},function(e,f,a){var c=a(2),b=a(3),d=a(20);b(b.S,"Reflect",{getOwnPropertyDescriptor:function getOwnPropertyDescriptor(a,b){return c.getDesc(d(a),b)}})},function(e,f,a){var b=a(3),c=a(2).getProto,d=a(20);b(b.S,"Reflect",{getPrototypeOf:function getPrototypeOf(a){return c(d(a))}})},function(c,d,b){var a=b(3);a(a.S,"Reflect",{has:function has(a,b){return b in a}})},function(e,f,a){var b=a(3),d=a(20),c=Object.isExtensible;b(b.S,"Reflect",{isExtensible:function isExtensible(a){return d(a),c?c(a):!0}})},function(c,d,a){var b=a(3);b(b.S,"Reflect",{ownKeys:a(161)})},function(d,f,a){var b=a(2),e=a(20),c=a(4).Reflect;d.exports=c&&c.ownKeys||function ownKeys(a){var c=b.getNames(e(a)),d=b.getSymbols;return d?c.concat(d(a)):c}},function(e,f,a){var b=a(3),d=a(20),c=Object.preventExtensions;b(b.S,"Reflect",{preventExtensions:function preventExtensions(a){d(a);try{return c&&c(a),!0}catch(b){return!1}}})},function(i,j,b){function set(j,i,k){var l,m,d=arguments.length<4?j:arguments[3],b=c.getDesc(h(j),i);if(!b){if(f(m=c.getProto(j)))return set(m,i,k,d);b=e(0)}return g(b,"value")?b.writable!==!1&&f(d)?(l=c.getDesc(d,i)||e(0),l.value=k,c.setDesc(d,i,l),!0):!1:b.set===a?!1:(b.set.call(d,k),!0)}var c=b(2),g=b(17),d=b(3),e=b(7),h=b(20),f=b(16);d(d.S,"Reflect",{set:set})},function(d,e,b){var c=b(3),a=b(45);a&&c(c.S,"Reflect",{setPrototypeOf:function setPrototypeOf(b,c){a.check(b,c);try{return a.set(b,c),!0}catch(d){return!1}}})},function(e,f,b){var c=b(3),d=b(33)(!0);c(c.P,"Array",{includes:function includes(b){return d(this,b,arguments.length>1?arguments[1]:a)}}),b(118)("includes")},function(d,e,a){var b=a(3),c=a(98)(!0);b(b.P,"String",{at:function at(a){return c(this,a)}})},function(e,f,b){var c=b(3),d=b(168);c(c.P,"String",{padLeft:function padLeft(b){return d(this,b,arguments.length>1?arguments[1]:a,!0)}})},function(c,g,b){var d=b(27),e=b(105),f=b(22);c.exports=function(l,m,i,n){var c=String(f(l)),j=c.length,g=i===a?" ":String(i),k=d(m);if(j>=k)return c;""==g&&(g=" ");var h=k-j,b=e.call(g,Math.ceil(h/g.length));return b.length>h&&(b=b.slice(0,h)),n?b+c:c+b}},function(e,f,b){var c=b(3),d=b(168);c(c.P,"String",{padRight:function padRight(b){return d(this,b,arguments.length>1?arguments[1]:a,!1)}})},function(b,c,a){a(63)("trimLeft",function(a){return function trimLeft(){return a(this,1)}})},function(b,c,a){a(63)("trimRight",function(a){return function trimRight(){return a(this,2)}})},function(d,e,a){var b=a(3),c=a(173)(/[\\^$*+?.()|[\]{}]/g,"\\$&");b(b.S,"RegExp",{escape:function escape(a){return c(a)}})},function(a,b){a.exports=function(b,a){var c=a===Object(a)?function(b){return a[b]}:a;return function(a){return String(a).replace(b,c)}}},function(g,h,a){var b=a(2),c=a(3),d=a(161),e=a(23),f=a(7);c(c.S,"Object",{getOwnPropertyDescriptors:function getOwnPropertyDescriptors(k){for(var a,g,h=e(k),l=b.setDesc,m=b.getDesc,i=d(h),c={},j=0;i.length>j;)g=m(h,a=i[j++]),a in c?l(c,a,f(0,g)):c[a]=g;return c}})},function(d,e,a){var b=a(3),c=a(176)(!1);b(b.S,"Object",{values:function values(a){return c(a)}})},function(c,f,a){var b=a(2),d=a(23),e=b.isEnum;c.exports=function(a){return function(j){for(var c,f=d(j),g=b.getKeys(f),k=g.length,h=0,i=[];k>h;)e.call(f,c=g[h++])&&i.push(a?[c,f[c]]:f[c]);return i}}},function(d,e,a){var b=a(3),c=a(176)(!0);b(b.S,"Object",{entries:function entries(a){return c(a)}})},function(c,d,a){var b=a(3);b(b.P,"Map",{toJSON:a(179)("Map")})},function(b,e,a){var c=a(138),d=a(47);b.exports=function(a){return function toJSON(){if(d(this)!=a)throw TypeError(a+"#toJSON isn't generic");var b=[];return c(this,!1,b.push,b),b}}},function(c,d,a){var b=a(3);b(b.P,"Set",{toJSON:a(179)("Set")})},function(d,e,b){var a=b(3),c=b(141);a(a.G+a.B,{setImmediate:c.set,clearImmediate:c.clear})},function(l,k,a){a(117);var g=a(4),j=a(6),c=a(109),b=a(31)("iterator"),h=g.NodeList,i=g.HTMLCollection,e=h&&h.prototype,d=i&&i.prototype,f=c.NodeList=c.HTMLCollection=c.Array;e&&!e[b]&&j(e,b,f),d&&!d[b]&&j(d,b,f)},function(i,j,a){var c=a(4),b=a(3),g=a(19),h=a(184),d=c.navigator,e=!!d&&/MSIE .\./.test(d.userAgent),f=function(a){return e?function(b,c){return a(g(h,[].slice.call(arguments,2),"function"==typeof b?b:Function(b)),c)}:a};b(b.G+b.B+b.F*e,{setTimeout:f(c.setTimeout),setInterval:f(c.setInterval)})},function(c,f,a){var d=a(185),b=a(19),e=a(13);c.exports=function(){for(var h=e(this),a=arguments.length,c=Array(a),f=0,i=d._,g=!1;a>f;)(c[f]=arguments[f++])===i&&(g=!0);return function(){var d,k=this,f=arguments,l=f.length,e=0,j=0;if(!g&&!l)return b(h,c,k);if(d=c.slice(),g)for(;a>e;e++)d[e]===i&&(d[e]=f[j++]);for(;l>j;)d.push(f[j++]);return b(h,d,k)}}},function(a,c,b){a.exports=b(4)},function(x,w,b){function Dict(b){var c=f.create(null);return b!=a&&(r(b)?q(b,!0,function(a,b){c[a]=b}):o(c,b)),c}function reduce(g,h,l){p(h);var a,c,b=i(g),e=k(b),j=e.length,f=0;if(arguments.length<3){if(!j)throw TypeError("Reduce of empty object with no initial value");a=b[e[f++]]}else a=Object(l);for(;j>f;)d(b,c=e[f++])&&(a=h(a,b[c],c,g));return a}function includes(c,b){return(b==b?j(c,b):l(c,function(a){return a!=a}))!==a}function get(a,b){return d(a,b)?a[b]:void 0}function set(a,b,c){return v&&b in Object?f.setDesc(a,b,t(0,c)):a[b]=c,a}function isDict(a){return u(a)&&f.getProto(a)===Dict.prototype}var f=b(2),n=b(12),e=b(3),t=b(7),o=b(41),j=b(36),p=b(13),q=b(138),r=b(187),s=b(110),g=b(119),u=b(16),i=b(23),v=b(8),d=b(17),k=f.getKeys,c=function(b){var e=1==b,c=4==b;return function(l,m,o){var f,h,g,p=n(m,o,3),k=i(l),j=e||7==b||2==b?new("function"==typeof this?this:Dict):a;for(f in k)if(d(k,f)&&(h=k[f],g=p(h,f,l),b))if(e)j[f]=g;else if(g)switch(b){case 2:j[f]=h;break;case 3:return!0;case 5:return h;case 6:return f;case 7:j[g[0]]=g[1]}else if(c)return!1;return 3==b||c?c:j}},l=c(6),h=function(a){return function(b){return new m(b,a)}},m=function(a,b){this._t=i(a),this._a=k(a),this._i=0,this._k=b};s(m,"Dict",function(){var c,b=this,e=b._t,f=b._a,h=b._k;do if(b._i>=f.length)return b._t=a,g(1);while(!d(e,c=f[b._i++]));return"keys"==h?g(0,c):"values"==h?g(0,e[c]):g(0,[c,e[c]])}),Dict.prototype=null,e(e.G+e.F,{Dict:Dict}),e(e.S,"Dict",{keys:h("keys"),values:h("values"),entries:h("entries"),forEach:c(0),map:c(1),filter:c(2),some:c(3),every:c(4),find:c(5),findKey:l,mapPairs:c(7),reduce:reduce,keyOf:j,includes:includes,has:d,get:get,set:set,isDict:isDict})},function(c,g,b){var d=b(47),e=b(31)("iterator"),f=b(109);c.exports=b(5).isIterable=function(c){var b=Object(c);return b[e]!==a||"@@iterator"in b||f.hasOwnProperty(d(b))}},function(b,e,a){var c=a(20),d=a(114);b.exports=a(5).getIterator=function(a){var b=d(a);if("function"!=typeof b)throw TypeError(a+" is not iterable!");return c(b.call(a))}},function(f,g,a){var c=a(4),d=a(5),b=a(3),e=a(184);b(b.G+b.F,{delay:function delay(a){return new(d.Promise||c.Promise)(function(b){setTimeout(e.call(b,!0),a)})}})},function(d,e,a){var c=a(185),b=a(3);a(5)._=c._=c._||{},b(b.P+b.F,"Function",{part:a(184)})},function(c,d,b){var a=b(3);a(a.S+a.F,"Object",{isObject:b(16)})},function(c,d,b){var a=b(3);a(a.S+a.F,"Object",{classof:b(47)})},function(d,e,b){var a=b(3),c=b(194);a(a.S+a.F,"Object",{define:c})},function(c,f,a){var b=a(2),d=a(161),e=a(23);c.exports=function define(a,c){for(var f,g=d(e(c)),i=g.length,h=0;i>h;)b.setDesc(a,f=g[h++],b.getDesc(c,f));return a}},function(e,f,a){var b=a(3),c=a(194),d=a(2).create;b(b.S+b.F,"Object",{make:function(a,b){return c(d(a),b)}})},function(c,d,b){b(108)(Number,"Number",function(a){this._l=+a,this._i=0},function(){var b=this._i++,c=!(this._l>b);return{done:c,value:c?a:b}})},function(d,e,b){var a=b(3),c=b(173)(/[&<>"']/g,{"&":"&","<":"<",">":">",'"':""","'":"'"});a(a.P+a.F,"String",{escapeHTML:function escapeHTML(){return c(this)}})},function(d,e,b){var a=b(3),c=b(173)(/&(?:amp|lt|gt|quot|apos);/g,{"&":"&","<":"<",">":">",""":'"',"'":"'"});a(a.P+a.F,"String",{unescapeHTML:function unescapeHTML(){return c(this)}})},function(g,h,a){var e=a(2),f=a(4),b=a(3),c={},d=!0;e.each.call("assert,clear,count,debug,dir,dirxml,error,exception,group,groupCollapsed,groupEnd,info,isIndependentlyComposed,log,markTimeline,profile,profileEnd,table,time,timeEnd,timeline,timelineEnd,timeStamp,trace,warn".split(","),function(a){c[a]=function(){var b=f.console;return d&&b&&b[a]?Function.apply.call(b[a],b,arguments):void 0}}),b(b.G+b.F,{log:a(41)(c.log,c,{enable:function(){d=!0},disable:function(){d=!1}})})},function(i,j,b){var g=b(2),e=b(3),h=b(12),f=b(5).Array||Array,c={},d=function(d,b){g.each.call(d.split(","),function(d){b==a&&d in f?c[d]=f[d]:d in[]&&(c[d]=h(Function.call,[][d],b))})};d("pop,reverse,shift,keys,values,entries",1),d("indexOf,every,some,forEach,map,filter,find,findIndex,includes",3),d("join,slice,concat,push,splice,unshift,sort,lastIndexOf,reduce,reduceRight,copyWithin,fill"),e(e.S,"Array",c)}]),"undefined"!=typeof module&&module.exports?module.exports=b:"function"==typeof define&&define.amd?define(function(){return b}):c.core=b}(1,1);
+//# sourceMappingURL=core.min.js.map
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/core.min.js.map b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/core.min.js.map
new file mode 100644
index 00000000..fab30694
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/core.min.js.map
@@ -0,0 +1 @@
+{"version":3,"sources":["core.js"],"names":["__e","__g","undefined","modules","__webpack_require__","moduleId","installedModules","exports","module","id","loaded","call","m","c","p","IE8_DOM_DEFINE","$","$export","DESCRIPTORS","createDesc","html","cel","has","cof","invoke","fails","anObject","aFunction","isObject","toObject","toIObject","toInteger","toIndex","toLength","IObject","IE_PROTO","createArrayMethod","arrayIndexOf","ObjectProto","Object","prototype","ArrayProto","Array","arraySlice","slice","arrayJoin","join","defineProperty","setDesc","getOwnDescriptor","getDesc","defineProperties","setDescs","factories","get","a","O","P","Attributes","e","TypeError","value","propertyIsEnumerable","Properties","keys","getKeys","length","i","S","F","getOwnPropertyDescriptor","keys1","split","keys2","concat","keysLen1","createDict","iframeDocument","iframe","gt","style","display","appendChild","src","contentWindow","document","open","write","close","createGetKeys","names","object","key","result","push","Empty","getPrototypeOf","getProto","constructor","getOwnPropertyNames","getNames","create","construct","len","args","n","Function","bind","that","fn","this","partArgs","arguments","bound","begin","end","klass","start","upTo","size","cloned","charAt","separator","isArray","createArrayReduce","isRight","callbackfn","memo","index","methodize","$fn","arg1","forEach","each","map","filter","some","every","reduce","reduceRight","indexOf","lastIndexOf","el","fromIndex","Math","min","now","Date","lz","num","toISOString","NaN","isFinite","RangeError","d","y","getUTCFullYear","getUTCMilliseconds","s","abs","getUTCMonth","getUTCDate","getUTCHours","getUTCMinutes","getUTCSeconds","$Object","isEnum","getSymbols","getOwnPropertySymbols","global","core","hide","redefine","ctx","PROTOTYPE","type","name","source","own","out","exp","IS_FORCED","IS_GLOBAL","G","IS_STATIC","IS_PROTO","IS_BIND","B","target","expProto","W","window","self","version","bitmap","enumerable","configurable","writable","exec","SRC","TO_STRING","$toString","TPL","inspectSource","it","val","safe","hasOwnProperty","String","toString","px","random","b","apply","documentElement","is","createElement","un","defined","ceil","floor","isNaN","max","asc","TYPE","IS_MAP","IS_FILTER","IS_SOME","IS_EVERY","IS_FIND_INDEX","NO_HOLES","$this","res","f","SPECIES","original","C","arg","store","uid","Symbol","SHARED","IS_INCLUDES","$fails","shared","setToStringTag","wks","keyOf","$names","enumKeys","_create","$Symbol","$JSON","JSON","_stringify","stringify","setter","HIDDEN","SymbolRegistry","AllSymbols","useNative","setSymbolDesc","D","protoDesc","wrap","tag","sym","_k","set","isSymbol","$defineProperty","$defineProperties","l","$create","$propertyIsEnumerable","E","$getOwnPropertyDescriptor","$getOwnPropertyNames","$getOwnPropertySymbols","$stringify","replacer","$replacer","$$","buggyJSON","symbolStatics","for","keyFor","useSetter","useSimple","def","TAG","stat","windowNames","getWindowNames","symbols","assign","A","K","k","T","$$len","j","x","setPrototypeOf","check","proto","test","buggy","__proto__","classof","ARG","callee","$freeze","freeze","KEY","$seal","seal","$preventExtensions","preventExtensions","$isFrozen","isFrozen","$isSealed","isSealed","$isExtensible","isExtensible","$getPrototypeOf","$keys","FProto","nameRE","NAME","match","HAS_INSTANCE","FunctionProto","toPrimitive","$trim","trim","NUMBER","$Number","Base","BROKEN_COF","TRIM","toNumber","argument","third","radix","maxCode","first","charCodeAt","code","digits","parseInt","Number","valueOf","spaces","space","non","ltrim","RegExp","rtrim","exporter","string","replace","EPSILON","pow","_isFinite","isInteger","number","isSafeInteger","MAX_SAFE_INTEGER","MIN_SAFE_INTEGER","parseFloat","log1p","sqrt","$acosh","acosh","MAX_VALUE","log","LN2","asinh","atanh","sign","cbrt","clz32","LOG2E","cosh","expm1","EPSILON32","MAX32","MIN32","roundTiesToEven","fround","$abs","$sign","Infinity","hypot","value1","value2","div","sum","larg","$imul","imul","UINT16","xn","yn","xl","yl","log10","LN10","log2","sinh","tanh","trunc","fromCharCode","$fromCodePoint","fromCodePoint","raw","callSite","tpl","$at","codePointAt","pos","context","ENDS_WITH","$endsWith","endsWith","searchString","endPosition","search","isRegExp","MATCH","re","INCLUDES","includes","repeat","count","str","STARTS_WITH","$startsWith","startsWith","iterated","_t","_i","point","done","LIBRARY","Iterators","$iterCreate","ITERATOR","BUGGY","FF_ITERATOR","KEYS","VALUES","returnThis","Constructor","next","DEFAULT","IS_SET","FORCED","methods","getMethod","kind","values","entries","DEF_VALUES","VALUES_BUG","$native","$default","IteratorPrototype","descriptor","isArrayIter","getIterFn","iter","from","arrayLike","step","iterator","mapfn","mapping","iterFn","ret","getIteratorMethod","SAFE_CLOSING","riter","skipClosing","arr","of","addToUnscopables","Arguments","UNSCOPABLES","copyWithin","to","inc","fill","endPos","$find","forced","find","findIndex","$flags","$RegExp","re1","re2","CORRECT_NEW","piRE","fiU","ignoreCase","multiline","unicode","sticky","flags","regexp","SYMBOL","REPLACE","$replace","searchValue","replaceValue","SEARCH","SPLIT","$split","limit","Wrapper","strictNew","forOf","setProto","same","speciesConstructor","asap","PROMISE","process","isNode","empty","testResolve","sub","promise","resolve","USE_NATIVE","P2","works","then","thenableThenGotten","sameConstructor","getConstructor","isThenable","PromiseCapability","reject","$$resolve","$$reject","perform","error","notify","record","isReject","chain","v","ok","run","reaction","handler","fail","h","setTimeout","console","isUnhandled","emit","onunhandledrejection","reason","_d","$reject","r","$resolve","wrapper","Promise","executor","err","onFulfilled","onRejected","catch","capability","all","iterable","abrupt","remaining","results","alreadyCalled","race","head","last","macrotask","Observer","MutationObserver","WebKitMutationObserver","flush","parent","domain","exit","enter","nextTick","toggle","node","createTextNode","observe","characterData","data","task","defer","channel","port","setTask","setImmediate","clearTask","clearImmediate","MessageChannel","counter","queue","ONREADYSTATECHANGE","listner","event","port2","port1","onmessage","postMessage","addEventListener","importScripts","removeChild","clear","strong","Map","entry","getEntry","redefineAll","$iterDefine","ID","$has","setSpecies","SIZE","fastKey","_f","ADDER","_l","delete","prev","setStrong","$iterDetect","common","IS_WEAK","fixMethod","add","BUGGY_ZERO","instance","HASNT_CHAINING","THROWS_ON_PRIMITIVES","ACCEPT_ITERABLES","Set","weak","frozenStore","WEAK","tmp","$WeakMap","WeakMap","method","arrayFind","arrayFindIndex","FrozenStore","findFrozen","splice","WeakSet","_apply","thisArgument","argumentsList","Reflect","Target","newTarget","$args","propertyKey","attributes","deleteProperty","desc","Enumerate","enumerate","receiver","ownKeys","V","existingDescriptor","ownDesc","$includes","at","$pad","padLeft","maxLength","fillString","left","stringLength","fillStr","intMaxLength","fillLen","stringFiller","padRight","trimLeft","trimRight","$re","escape","regExp","part","getOwnPropertyDescriptors","$values","isEntries","$entries","toJSON","$task","NL","NodeList","HTC","HTMLCollection","NLProto","HTCProto","ArrayValues","partial","navigator","MSIE","userAgent","time","setInterval","path","pargs","_","holder","Dict","dict","isIterable","init","findKey","isDict","createDictMethod","createDictIter","DictIterator","_a","mapPairs","getIterator","delay","define","mixin","make","&","<",">","\"","'","escapeHTML","&","<",">",""","'","unescapeHTML","enabled","$console","enable","disable","$ctx","$Array","statics","setStatics","amd"],"mappings":";;;;;;CAMC,SAASA,EAAKC,EAAKC,GACpB,cACS,SAAUC,GAKT,QAASC,qBAAoBC,GAG5B,GAAGC,EAAiBD,GACnB,MAAOC,GAAiBD,GAAUE,OAGnC,IAAIC,GAASF,EAAiBD,IAC7BE,WACAE,GAAIJ,EACJK,QAAQ,EAUT,OANAP,GAAQE,GAAUM,KAAKH,EAAOD,QAASC,EAAQA,EAAOD,QAASH,qBAG/DI,EAAOE,QAAS,EAGTF,EAAOD,QAvBf,GAAID,KAqCJ,OATAF,qBAAoBQ,EAAIT,EAGxBC,oBAAoBS,EAAIP,EAGxBF,oBAAoBU,EAAI,GAGjBV,oBAAoB,KAK/B,SAASI,EAAQD,EAASH,GAE/BA,EAAoB,GACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBI,EAAOD,QAAUH,EAAoB,MAKhC,SAASI,EAAQD,EAASH,GAG/B,GA8BIW,GA9BAC,EAAoBZ,EAAoB,GACxCa,EAAoBb,EAAoB,GACxCc,EAAoBd,EAAoB,GACxCe,EAAoBf,EAAoB,GACxCgB,EAAoBhB,EAAoB,IACxCiB,EAAoBjB,EAAoB,IACxCkB,EAAoBlB,EAAoB,IACxCmB,EAAoBnB,EAAoB,IACxCoB,EAAoBpB,EAAoB,IACxCqB,EAAoBrB,EAAoB,GACxCsB,EAAoBtB,EAAoB,IACxCuB,EAAoBvB,EAAoB,IACxCwB,EAAoBxB,EAAoB,IACxCyB,EAAoBzB,EAAoB,IACxC0B,EAAoB1B,EAAoB,IACxC2B,EAAoB3B,EAAoB,IACxC4B,EAAoB5B,EAAoB,IACxC6B,EAAoB7B,EAAoB,IACxC8B,EAAoB9B,EAAoB,IACxC+B,EAAoB/B,EAAoB,IAAI,aAC5CgC,EAAoBhC,EAAoB,IACxCiC,EAAoBjC,EAAoB,KAAI,GAC5CkC,EAAoBC,OAAOC,UAC3BC,EAAoBC,MAAMF,UAC1BG,EAAoBF,EAAWG,MAC/BC,EAAoBJ,EAAWK,KAC/BC,EAAoB/B,EAAEgC,QACtBC,EAAoBjC,EAAEkC,QACtBC,EAAoBnC,EAAEoC,SACtBC,IAGAnC,KACFH,GAAkBU,EAAM,WACtB,MAA4E,IAArEsB,EAAe1B,EAAI,OAAQ,KAAMiC,IAAK,WAAY,MAAO,MAAOC,IAEzEvC,EAAEgC,QAAU,SAASQ,EAAGC,EAAGC,GACzB,GAAG3C,EAAe,IAChB,MAAOgC,GAAeS,EAAGC,EAAGC,GAC5B,MAAMC,IACR,GAAG,OAASD,IAAc,OAASA,GAAW,KAAME,WAAU,2BAE9D,OADG,SAAWF,KAAWhC,EAAS8B,GAAGC,GAAKC,EAAWG,OAC9CL,GAETxC,EAAEkC,QAAU,SAASM,EAAGC,GACtB,GAAG1C,EAAe,IAChB,MAAOkC,GAAiBO,EAAGC,GAC3B,MAAME,IACR,MAAGrC,GAAIkC,EAAGC,GAAUtC,GAAYmB,EAAYwB,qBAAqBnD,KAAK6C,EAAGC,GAAID,EAAEC,IAA/E,QAEFzC,EAAEoC,SAAWD,EAAmB,SAASK,EAAGO,GAC1CrC,EAAS8B,EAKT,KAJA,GAGIC,GAHAO,EAAShD,EAAEiD,QAAQF,GACnBG,EAASF,EAAKE,OACdC,EAAI,EAEFD,EAASC,GAAEnD,EAAEgC,QAAQQ,EAAGC,EAAIO,EAAKG,KAAMJ,EAAWN,GACxD,OAAOD,KAGXvC,EAAQA,EAAQmD,EAAInD,EAAQoD,GAAKnD,EAAa,UAE5CoD,yBAA0BtD,EAAEkC,QAE5BH,eAAgB/B,EAAEgC,QAElBG,iBAAkBA,GAIpB,IAAIoB,GAAQ,gGACmCC,MAAM,KAEjDC,EAAQF,EAAMG,OAAO,SAAU,aAC/BC,EAAWJ,EAAML,OAGjBU,EAAa,WAEf,GAGIC,GAHAC,EAASzD,EAAI,UACb8C,EAASQ,EACTI,EAAS,GAYb,KAVAD,EAAOE,MAAMC,QAAU,OACvB7D,EAAK8D,YAAYJ,GACjBA,EAAOK,IAAM,cAGbN,EAAiBC,EAAOM,cAAcC,SACtCR,EAAeS,OACfT,EAAeU,MAAM,oCAAsCR,GAC3DF,EAAeW,QACfZ,EAAaC,EAAeR,EACtBF,WAAWS,GAAWpC,UAAU+B,EAAMJ,GAC5C,OAAOS,MAELa,EAAgB,SAASC,EAAOxB,GAClC,MAAO,UAASyB,GACd,GAGIC,GAHApC,EAAS1B,EAAU6D,GACnBxB,EAAS,EACT0B,IAEJ,KAAID,IAAOpC,GAAKoC,GAAOzD,GAASb,EAAIkC,EAAGoC,IAAQC,EAAOC,KAAKF,EAE3D,MAAM1B,EAASC,GAAK7C,EAAIkC,EAAGoC,EAAMF,EAAMvB,SACpC9B,EAAawD,EAAQD,IAAQC,EAAOC,KAAKF,GAE5C,OAAOC,KAGPE,EAAQ,YACZ9E,GAAQA,EAAQmD,EAAG,UAEjB4B,eAAgBhF,EAAEiF,SAAWjF,EAAEiF,UAAY,SAASzC,GAElD,MADAA,GAAI3B,EAAS2B,GACVlC,EAAIkC,EAAGrB,GAAiBqB,EAAErB,GACF,kBAAjBqB,GAAE0C,aAA6B1C,YAAaA,GAAE0C,YAC/C1C,EAAE0C,YAAY1D,UACdgB,YAAajB,QAASD,EAAc,MAG/C6D,oBAAqBnF,EAAEoF,SAAWpF,EAAEoF,UAAYX,EAAchB,EAAOA,EAAMP,QAAQ,GAEnFmC,OAAQrF,EAAEqF,OAASrF,EAAEqF,QAAU,SAAS7C,EAAQO,GAC9C,GAAI8B,EAQJ,OAPS,QAANrC,GACDuC,EAAMvD,UAAYd,EAAS8B,GAC3BqC,EAAS,GAAIE,GACbA,EAAMvD,UAAY,KAElBqD,EAAO1D,GAAYqB,GACdqC,EAASjB,IACTb,IAAe7D,EAAY2F,EAAS1C,EAAiB0C,EAAQ9B,IAGtEC,KAAMhD,EAAEiD,QAAUjD,EAAEiD,SAAWwB,EAAclB,EAAOI,GAAU,IAGhE,IAAI2B,GAAY,SAASjC,EAAGkC,EAAKC,GAC/B,KAAKD,IAAOlD,IAAW,CACrB,IAAI,GAAIoD,MAAQtC,EAAI,EAAOoC,EAAJpC,EAASA,IAAIsC,EAAEtC,GAAK,KAAOA,EAAI,GACtDd,GAAUkD,GAAOG,SAAS,MAAO,gBAAkBD,EAAE3D,KAAK,KAAO,KAEnE,MAAOO,GAAUkD,GAAKlC,EAAGmC,GAI3BvF,GAAQA,EAAQwC,EAAG,YACjBkD,KAAM,QAASA,MAAKC,GAClB,GAAIC,GAAWlF,EAAUmF,MACrBC,EAAWpE,EAAWhC,KAAKqG,UAAW,GACtCC,EAAQ,WACV,GAAIT,GAAOO,EAASrC,OAAO/B,EAAWhC,KAAKqG,WAC3C,OAAOF,gBAAgBG,GAAQX,EAAUO,EAAIL,EAAKtC,OAAQsC,GAAQhF,EAAOqF,EAAIL,EAAMI,GAGrF,OADGhF,GAASiF,EAAGrE,aAAWyE,EAAMzE,UAAYqE,EAAGrE,WACxCyE,KAKXhG,EAAQA,EAAQwC,EAAIxC,EAAQoD,EAAI5C,EAAM,WACjCL,GAAKuB,EAAWhC,KAAKS,KACtB,SACFwB,MAAO,SAASsE,EAAOC,GACrB,GAAIZ,GAAQtE,EAAS6E,KAAK5C,QACtBkD,EAAQ7F,EAAIuF,KAEhB,IADAK,EAAMA,IAAQjH,EAAYqG,EAAMY,EACpB,SAATC,EAAiB,MAAOzE,GAAWhC,KAAKmG,KAAMI,EAAOC,EAMxD,KALA,GAAIE,GAASrF,EAAQkF,EAAOX,GACxBe,EAAStF,EAAQmF,EAAKZ,GACtBgB,EAAStF,EAASqF,EAAOD,GACzBG,EAAS9E,MAAM6E,GACfpD,EAAS,EACHoD,EAAJpD,EAAUA,IAAIqD,EAAOrD,GAAc,UAATiD,EAC5BN,KAAKW,OAAOJ,EAAQlD,GACpB2C,KAAKO,EAAQlD,EACjB,OAAOqD,MAGXvG,EAAQA,EAAQwC,EAAIxC,EAAQoD,GAAKnC,GAAWK,QAAS,SACnDO,KAAM,QAASA,MAAK4E,GAClB,MAAO7E,GAAUlC,KAAKuB,EAAQ4E,MAAOY,IAAcxH,EAAY,IAAMwH,MAKzEzG,EAAQA,EAAQmD,EAAG,SAAUuD,QAASvH,EAAoB,KAE1D,IAAIwH,GAAoB,SAASC,GAC/B,MAAO,UAASC,EAAYC,GAC1BpG,EAAUmG,EACV,IAAItE,GAAStB,EAAQ4E,MACjB5C,EAASjC,EAASuB,EAAEU,QACpB8D,EAASH,EAAU3D,EAAS,EAAI,EAChCC,EAAS0D,EAAU,GAAK,CAC5B,IAAGb,UAAU9C,OAAS,EAAE,OAAO,CAC7B,GAAG8D,IAASxE,GAAE,CACZuE,EAAOvE,EAAEwE,GACTA,GAAS7D,CACT,OAGF,GADA6D,GAAS7D,EACN0D,EAAkB,EAARG,EAAsBA,GAAV9D,EACvB,KAAMN,WAAU,+CAGpB,KAAKiE,EAAUG,GAAS,EAAI9D,EAAS8D,EAAOA,GAAS7D,EAAK6D,IAASxE,KACjEuE,EAAOD,EAAWC,EAAMvE,EAAEwE,GAAQA,EAAOlB,MAE3C,OAAOiB,KAIPE,EAAY,SAASC,GACvB,MAAO,UAASC,GACd,MAAOD,GAAIpB,KAAMqB,EAAMnB,UAAU,KAIrC/F,GAAQA,EAAQwC,EAAG,SAEjB2E,QAASpH,EAAEqH,KAAOrH,EAAEqH,MAAQJ,EAAU7F,EAAkB,IAExDkG,IAAKL,EAAU7F,EAAkB,IAEjCmG,OAAQN,EAAU7F,EAAkB,IAEpCoG,KAAMP,EAAU7F,EAAkB,IAElCqG,MAAOR,EAAU7F,EAAkB,IAEnCsG,OAAQd,GAAkB,GAE1Be,YAAaf,GAAkB,GAE/BgB,QAASX,EAAU5F,GAEnBwG,YAAa,SAASC,EAAIC,GACxB,GAAIvF,GAAS1B,EAAUgF,MACnB5C,EAASjC,EAASuB,EAAEU,QACpB8D,EAAS9D,EAAS,CAGtB,KAFG8C,UAAU9C,OAAS,IAAE8D,EAAQgB,KAAKC,IAAIjB,EAAOjG,EAAUgH,KAC/C,EAARf,IAAUA,EAAQ/F,EAASiC,EAAS8D,IAClCA,GAAS,EAAGA,IAAQ,GAAGA,IAASxE,IAAKA,EAAEwE,KAAWc,EAAG,MAAOd,EACjE,OAAO,MAKX/G,EAAQA,EAAQmD,EAAG,QAAS8E,IAAK,WAAY,OAAQ,GAAIC,QAEzD,IAAIC,GAAK,SAASC,GAChB,MAAOA,GAAM,EAAIA,EAAM,IAAMA,EAK/BpI,GAAQA,EAAQwC,EAAIxC,EAAQoD,GAAK5C,EAAM,WACrC,MAA4C,4BAArC,GAAI0H,MAAK,MAAQ,GAAGG,kBACtB7H,EAAM,WACX,GAAI0H,MAAKI,KAAKD,iBACX,QACHA,YAAa,QAASA,eACpB,IAAIE,SAAS1C,MAAM,KAAM2C,YAAW,qBACpC,IAAIC,GAAI5C,KACJ6C,EAAID,EAAEE,iBACNhJ,EAAI8I,EAAEG,qBACNC,EAAQ,EAAJH,EAAQ,IAAMA,EAAI,KAAO,IAAM,EACvC,OAAOG,IAAK,QAAUd,KAAKe,IAAIJ,IAAI/G,MAAMkH,EAAI,GAAK,IAChD,IAAMV,EAAGM,EAAEM,cAAgB,GAAK,IAAMZ,EAAGM,EAAEO,cAC3C,IAAMb,EAAGM,EAAEQ,eAAiB,IAAMd,EAAGM,EAAES,iBACvC,IAAMf,EAAGM,EAAEU,iBAAmB,KAAOxJ,EAAI,GAAKA,EAAI,IAAMwI,EAAGxI,IAAM,QAMlE,SAASJ,EAAQD,GAEtB,GAAI8J,GAAU9H,MACd/B,GAAOD,SACL8F,OAAYgE,EAAQhE,OACpBJ,SAAYoE,EAAQrE,eACpBsE,UAAexG,qBACfZ,QAAYmH,EAAQ/F,yBACpBtB,QAAYqH,EAAQtH,eACpBK,SAAYiH,EAAQlH,iBACpBc,QAAYoG,EAAQrG,KACpBoC,SAAYiE,EAAQlE,oBACpBoE,WAAYF,EAAQG,sBACpBnC,QAAeD,UAKZ,SAAS5H,EAAQD,EAASH,GAE/B,GAAIqK,GAAYrK,EAAoB,GAChCsK,EAAYtK,EAAoB,GAChCuK,EAAYvK,EAAoB,GAChCwK,EAAYxK,EAAoB,IAChCyK,EAAYzK,EAAoB,IAChC0K,EAAY,YAEZ7J,EAAU,SAAS8J,EAAMC,EAAMC,GACjC,GAQIrF,GAAKsF,EAAKC,EAAKC,EARfC,EAAYN,EAAO9J,EAAQoD,EAC3BiH,EAAYP,EAAO9J,EAAQsK,EAC3BC,EAAYT,EAAO9J,EAAQmD,EAC3BqH,EAAYV,EAAO9J,EAAQwC,EAC3BiI,EAAYX,EAAO9J,EAAQ0K,EAC3BC,EAAYN,EAAYb,EAASe,EAAYf,EAAOO,KAAUP,EAAOO,QAAeP,EAAOO,QAAaF,GACxGvK,EAAY+K,EAAYZ,EAAOA,EAAKM,KAAUN,EAAKM,OACnDa,EAAYtL,EAAQuK,KAAevK,EAAQuK,MAE5CQ,KAAUL,EAASD,EACtB,KAAIpF,IAAOqF,GAETC,GAAOG,GAAaO,GAAUhG,IAAOgG,GAErCT,GAAOD,EAAMU,EAASX,GAAQrF,GAE9BwF,EAAMM,GAAWR,EAAML,EAAIM,EAAKV,GAAUgB,GAA0B,kBAAPN,GAAoBN,EAAInE,SAAS/F,KAAMwK,GAAOA,EAExGS,IAAWV,GAAIN,EAASgB,EAAQhG,EAAKuF,GAErC5K,EAAQqF,IAAQuF,GAAIR,EAAKpK,EAASqF,EAAKwF,GACvCK,GAAYI,EAASjG,IAAQuF,IAAIU,EAASjG,GAAOuF,GAGxDV,GAAOC,KAAOA,EAEdzJ,EAAQoD,EAAI,EACZpD,EAAQsK,EAAI,EACZtK,EAAQmD,EAAI,EACZnD,EAAQwC,EAAI,EACZxC,EAAQ0K,EAAI,GACZ1K,EAAQ6K,EAAI,GACZtL,EAAOD,QAAUU,GAIZ,SAAST,EAAQD,GAGtB,GAAIkK,GAASjK,EAAOD,QAA2B,mBAAVwL,SAAyBA,OAAO/C,MAAQA,KACzE+C,OAAwB,mBAARC,OAAuBA,KAAKhD,MAAQA,KAAOgD,KAAOtF,SAAS,gBAC9D,iBAAPzG,KAAgBA,EAAMwK,IAI3B,SAASjK,EAAQD,GAEtB,GAAImK,GAAOlK,EAAOD,SAAW0L,QAAS,QACrB,iBAAPjM,KAAgBA,EAAM0K,IAI3B,SAASlK,EAAQD,EAASH,GAE/B,GAAIY,GAAaZ,EAAoB,GACjCe,EAAaf,EAAoB,EACrCI,GAAOD,QAAUH,EAAoB,GAAK,SAASuF,EAAQC,EAAK/B,GAC9D,MAAO7C,GAAEgC,QAAQ2C,EAAQC,EAAKzE,EAAW,EAAG0C,KAC1C,SAAS8B,EAAQC,EAAK/B,GAExB,MADA8B,GAAOC,GAAO/B,EACP8B,IAKJ,SAASnF,EAAQD,GAEtBC,EAAOD,QAAU,SAAS2L,EAAQrI,GAChC,OACEsI,aAAyB,EAATD,GAChBE,eAAyB,EAATF,GAChBG,WAAyB,EAATH,GAChBrI,MAAcA,KAMb,SAASrD,EAAQD,EAASH,GAG/BI,EAAOD,SAAWH,EAAoB,GAAG,WACvC,MAA2E,IAApEmC,OAAOQ,kBAAmB,KAAMO,IAAK,WAAY,MAAO,MAAOC,KAKnE,SAAS/C,EAAQD,GAEtBC,EAAOD,QAAU,SAAS+L,GACxB,IACE,QAASA,IACT,MAAM3I,GACN,OAAO,KAMN,SAASnD,EAAQD,EAASH,GAI/B,GAAIqK,GAAYrK,EAAoB,GAChCuK,EAAYvK,EAAoB,GAChCmM,EAAYnM,EAAoB,IAAI,OACpCoM,EAAY,WACZC,EAAY/F,SAAS8F,GACrBE,GAAa,GAAKD,GAAWjI,MAAMgI,EAEvCpM,GAAoB,GAAGuM,cAAgB,SAASC,GAC9C,MAAOH,GAAU9L,KAAKiM,KAGvBpM,EAAOD,QAAU,SAASiD,EAAGoC,EAAKiH,EAAKC,GACrB,kBAAPD,KACRA,EAAIE,eAAeR,IAAQ5B,EAAKkC,EAAKN,EAAK/I,EAAEoC,GAAO,GAAKpC,EAAEoC,GAAO8G,EAAI5J,KAAKkK,OAAOpH,KACjFiH,EAAIE,eAAe,SAAWpC,EAAKkC,EAAK,OAAQjH,IAE/CpC,IAAMiH,EACPjH,EAAEoC,GAAOiH,GAELC,SAAYtJ,GAAEoC,GAClB+E,EAAKnH,EAAGoC,EAAKiH,MAEdnG,SAASlE,UAAWgK,EAAW,QAASS,YACzC,MAAsB,kBAARnG,OAAsBA,KAAKyF,IAAQE,EAAU9L,KAAKmG,SAK7D,SAAStG,EAAQD,GAEtB,GAAIE,GAAK,EACLyM,EAAKlE,KAAKmE,QACd3M,GAAOD,QAAU,SAASqF,GACxB,MAAO,UAAUlB,OAAOkB,IAAQ1F,EAAY,GAAK0F,EAAK,QAASnF,EAAKyM,GAAID,SAAS,OAK9E,SAASzM,EAAQD,EAASH,GAG/B,GAAIuB,GAAYvB,EAAoB,GACpCI,GAAOD,QAAU,SAASsG,EAAID,EAAM1C,GAElC,GADAvC,EAAUkF,GACPD,IAAS1G,EAAU,MAAO2G,EAC7B,QAAO3C,GACL,IAAK,GAAG,MAAO,UAASX,GACtB,MAAOsD,GAAGlG,KAAKiG,EAAMrD,GAEvB,KAAK,GAAG,MAAO,UAASA,EAAG6J,GACzB,MAAOvG,GAAGlG,KAAKiG,EAAMrD,EAAG6J,GAE1B,KAAK,GAAG,MAAO,UAAS7J,EAAG6J,EAAGvM,GAC5B,MAAOgG,GAAGlG,KAAKiG,EAAMrD,EAAG6J,EAAGvM,IAG/B,MAAO,YACL,MAAOgG,GAAGwG,MAAMzG,EAAMI,cAMrB,SAASxG,EAAQD,GAEtBC,EAAOD,QAAU,SAASqM,GACxB,GAAgB,kBAANA,GAAiB,KAAMhJ,WAAUgJ,EAAK,sBAChD,OAAOA,KAKJ,SAASpM,EAAQD,EAASH,GAE/BI,EAAOD,QAAUH,EAAoB,GAAGiF,UAAYA,SAASiI,iBAIxD,SAAS9M,EAAQD,EAASH,GAE/B,GAAIwB,GAAWxB,EAAoB,IAC/BiF,EAAWjF,EAAoB,GAAGiF,SAElCkI,EAAK3L,EAASyD,IAAazD,EAASyD,EAASmI,cACjDhN,GAAOD,QAAU,SAASqM,GACxB,MAAOW,GAAKlI,EAASmI,cAAcZ,QAKhC,SAASpM,EAAQD,GAEtBC,EAAOD,QAAU,SAASqM,GACxB,MAAqB,gBAAPA,GAAyB,OAAPA,EAA4B,kBAAPA,KAKlD,SAASpM,EAAQD,GAEtB,GAAIwM,MAAoBA,cACxBvM,GAAOD,QAAU,SAASqM,EAAIhH,GAC5B,MAAOmH,GAAepM,KAAKiM,EAAIhH,KAK5B,SAASpF,EAAQD,GAEtB,GAAI0M,MAAcA,QAElBzM,GAAOD,QAAU,SAASqM,GACxB,MAAOK,GAAStM,KAAKiM,GAAIhK,MAAM,EAAG,MAK/B,SAASpC,EAAQD,GAGtBC,EAAOD,QAAU,SAASsG,EAAIL,EAAMI,GAClC,GAAI6G,GAAK7G,IAAS1G,CAClB,QAAOsG,EAAKtC,QACV,IAAK,GAAG,MAAOuJ,GAAK5G,IACAA,EAAGlG,KAAKiG,EAC5B,KAAK,GAAG,MAAO6G,GAAK5G,EAAGL,EAAK,IACRK,EAAGlG,KAAKiG,EAAMJ,EAAK,GACvC,KAAK,GAAG,MAAOiH,GAAK5G,EAAGL,EAAK,GAAIA,EAAK,IACjBK,EAAGlG,KAAKiG,EAAMJ,EAAK,GAAIA,EAAK,GAChD,KAAK,GAAG,MAAOiH,GAAK5G,EAAGL,EAAK,GAAIA,EAAK,GAAIA,EAAK,IAC1BK,EAAGlG,KAAKiG,EAAMJ,EAAK,GAAIA,EAAK,GAAIA,EAAK,GACzD,KAAK,GAAG,MAAOiH,GAAK5G,EAAGL,EAAK,GAAIA,EAAK,GAAIA,EAAK,GAAIA,EAAK,IACnCK,EAAGlG,KAAKiG,EAAMJ,EAAK,GAAIA,EAAK,GAAIA,EAAK,GAAIA,EAAK,IAClE,MAAoBK,GAAGwG,MAAMzG,EAAMJ,KAKlC,SAAShG,EAAQD,EAASH,GAE/B,GAAIwB,GAAWxB,EAAoB,GACnCI,GAAOD,QAAU,SAASqM,GACxB,IAAIhL,EAASgL,GAAI,KAAMhJ,WAAUgJ,EAAK,qBACtC,OAAOA,KAKJ,SAASpM,EAAQD,EAASH,GAG/B,GAAIsN,GAAUtN,EAAoB,GAClCI,GAAOD,QAAU,SAASqM,GACxB,MAAOrK,QAAOmL,EAAQd,MAKnB,SAASpM,EAAQD,GAGtBC,EAAOD,QAAU,SAASqM,GACxB,GAAGA,GAAM1M,EAAU,KAAM0D,WAAU,yBAA2BgJ,EAC9D,OAAOA,KAKJ,SAASpM,EAAQD,EAASH,GAG/B,GAAI8B,GAAU9B,EAAoB,IAC9BsN,EAAUtN,EAAoB,GAClCI,GAAOD,QAAU,SAASqM,GACxB,MAAO1K,GAAQwL,EAAQd,MAKpB,SAASpM,EAAQD,EAASH,GAG/B,GAAImB,GAAMnB,EAAoB,GAC9BI,GAAOD,QAAUgC,OAAO,KAAKuB,qBAAqB,GAAKvB,OAAS,SAASqK,GACvE,MAAkB,UAAXrL,EAAIqL,GAAkBA,EAAGpI,MAAM,IAAMjC,OAAOqK,KAKhD,SAASpM,EAAQD,GAGtB,GAAIoN,GAAQ3E,KAAK2E,KACbC,EAAQ5E,KAAK4E,KACjBpN,GAAOD,QAAU,SAASqM,GACxB,MAAOiB,OAAMjB,GAAMA,GAAM,GAAKA,EAAK,EAAIgB,EAAQD,GAAMf,KAKlD,SAASpM,EAAQD,EAASH,GAE/B,GAAI2B,GAAY3B,EAAoB,IAChC0N,EAAY9E,KAAK8E,IACjB7E,EAAYD,KAAKC,GACrBzI,GAAOD,QAAU,SAASyH,EAAO9D,GAE/B,MADA8D,GAAQjG,EAAUiG,GACH,EAARA,EAAY8F,EAAI9F,EAAQ9D,EAAQ,GAAK+E,EAAIjB,EAAO9D,KAKpD,SAAS1D,EAAQD,EAASH,GAG/B,GAAI2B,GAAY3B,EAAoB,IAChC6I,EAAYD,KAAKC,GACrBzI,GAAOD,QAAU,SAASqM,GACxB,MAAOA,GAAK,EAAI3D,EAAIlH,EAAU6K,GAAK,kBAAoB,IAKpD,SAASpM,EAAQD,EAASH,GAS/B,GAAIyK,GAAWzK,EAAoB,IAC/B8B,EAAW9B,EAAoB,IAC/ByB,EAAWzB,EAAoB,IAC/B6B,EAAW7B,EAAoB,IAC/B2N,EAAW3N,EAAoB,GACnCI,GAAOD,QAAU,SAASyN,GACxB,GAAIC,GAAwB,GAARD,EAChBE,EAAwB,GAARF,EAChBG,EAAwB,GAARH,EAChBI,EAAwB,GAARJ,EAChBK,EAAwB,GAARL,EAChBM,EAAwB,GAARN,GAAaK,CACjC,OAAO,UAASE,EAAOzG,EAAYlB,GAQjC,IAPA,GAMIiG,GAAK2B,EANLhL,EAAS3B,EAAS0M,GAClBvC,EAAS9J,EAAQsB,GACjBiL,EAAS5D,EAAI/C,EAAYlB,EAAM,GAC/B1C,EAASjC,EAAS+J,EAAK9H,QACvB8D,EAAS,EACTnC,EAASoI,EAASF,EAAIQ,EAAOrK,GAAUgK,EAAYH,EAAIQ,EAAO,GAAKrO,EAElEgE,EAAS8D,EAAOA,IAAQ,IAAGsG,GAAYtG,IAASgE,MACnDa,EAAMb,EAAKhE,GACXwG,EAAMC,EAAE5B,EAAK7E,EAAOxE,GACjBwK,GACD,GAAGC,EAAOpI,EAAOmC,GAASwG,MACrB,IAAGA,EAAI,OAAOR,GACjB,IAAK,GAAG,OAAO,CACf,KAAK,GAAG,MAAOnB,EACf,KAAK,GAAG,MAAO7E,EACf,KAAK,GAAGnC,EAAOC,KAAK+G,OACf,IAAGuB,EAAS,OAAO,CAG9B,OAAOC,GAAgB,GAAKF,GAAWC,EAAWA,EAAWvI,KAM5D,SAASrF,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,IAC/BuH,EAAWvH,EAAoB,IAC/BsO,EAAWtO,EAAoB,IAAI,UACvCI,GAAOD,QAAU,SAASoO,EAAUzK,GAClC,GAAI0K,EASF,OARCjH,GAAQgH,KACTC,EAAID,EAASzI,YAEE,kBAAL0I,IAAoBA,IAAMlM,QAASiF,EAAQiH,EAAEpM,aAAYoM,EAAI1O,GACpE0B,EAASgN,KACVA,EAAIA,EAAEF,GACG,OAANE,IAAWA,EAAI1O,KAEb,IAAK0O,IAAM1O,EAAYwC,MAAQkM,GAAG1K,KAKxC,SAAS1D,EAAQD,EAASH,GAG/B,GAAImB,GAAMnB,EAAoB,GAC9BI,GAAOD,QAAUmC,MAAMiF,SAAW,SAASkH,GACzC,MAAmB,SAAZtN,EAAIsN,KAKR,SAASrO,EAAQD,EAASH,GAE/B,GAAI0O,GAAS1O,EAAoB,IAAI,OACjC2O,EAAS3O,EAAoB,IAC7B4O,EAAS5O,EAAoB,GAAG4O,MACpCxO,GAAOD,QAAU,SAASyK,GACxB,MAAO8D,GAAM9D,KAAU8D,EAAM9D,GAC3BgE,GAAUA,EAAOhE,KAAUgE,GAAUD,GAAK,UAAY/D,MAKrD,SAASxK,EAAQD,EAASH,GAE/B,GAAIqK,GAASrK,EAAoB,GAC7B6O,EAAS,qBACTH,EAASrE,EAAOwE,KAAYxE,EAAOwE,MACvCzO,GAAOD,QAAU,SAASqF,GACxB,MAAOkJ,GAAMlJ,KAASkJ,EAAMlJ,SAKzB,SAASpF,EAAQD,EAASH,GAI/B,GAAI0B,GAAY1B,EAAoB,IAChC6B,EAAY7B,EAAoB,IAChC4B,EAAY5B,EAAoB,GACpCI,GAAOD,QAAU,SAAS2O,GACxB,MAAO,UAASX,EAAOzF,EAAIC,GACzB,GAGIlF,GAHAL,EAAS1B,EAAUyM,GACnBrK,EAASjC,EAASuB,EAAEU,QACpB8D,EAAShG,EAAQ+G,EAAW7E,EAGhC,IAAGgL,GAAepG,GAAMA,GAAG,KAAM5E,EAAS8D,GAExC,GADAnE,EAAQL,EAAEwE,KACPnE,GAASA,EAAM,OAAO,MAEpB,MAAKK,EAAS8D,EAAOA,IAAQ,IAAGkH,GAAelH,IAASxE,KAC1DA,EAAEwE,KAAWc,EAAG,MAAOoG,IAAelH,CACzC,QAAQkH,GAAe,MAMxB,SAAS1O,EAAQD,EAASH,GAI/B,GAAIY,GAAiBZ,EAAoB,GACrCqK,EAAiBrK,EAAoB,GACrCkB,EAAiBlB,EAAoB,IACrCc,EAAiBd,EAAoB,GACrCa,EAAiBb,EAAoB,GACrCwK,EAAiBxK,EAAoB,IACrC+O,EAAiB/O,EAAoB,GACrCgP,EAAiBhP,EAAoB,IACrCiP,EAAiBjP,EAAoB,IACrC2O,EAAiB3O,EAAoB,IACrCkP,EAAiBlP,EAAoB,IACrCmP,EAAiBnP,EAAoB,IACrCoP,EAAiBpP,EAAoB,IACrCqP,EAAiBrP,EAAoB,IACrCuH,EAAiBvH,EAAoB,IACrCsB,EAAiBtB,EAAoB,IACrC0B,EAAiB1B,EAAoB,IACrCe,EAAiBf,EAAoB,GACrC8C,EAAiBlC,EAAEkC,QACnBF,EAAiBhC,EAAEgC,QACnB0M,EAAiB1O,EAAEqF,OACnBD,EAAiBoJ,EAAOlM,IACxBqM,EAAiBlF,EAAOuE,OACxBY,EAAiBnF,EAAOoF,KACxBC,EAAiBF,GAASA,EAAMG,UAChCC,GAAiB,EACjBC,EAAiBX,EAAI,WACrBhF,EAAiBtJ,EAAEsJ,OACnB4F,EAAiBd,EAAO,mBACxBe,EAAiBf,EAAO,WACxBgB,EAAmC,kBAAXT,GACxBrN,EAAiBC,OAAOC,UAGxB6N,EAAgBnP,GAAeiO,EAAO,WACxC,MAES,IAFFO,EAAQ1M,KAAY,KACzBM,IAAK,WAAY,MAAON,GAAQ8D,KAAM,KAAMjD,MAAO,IAAIN,MACrDA,IACD,SAASqJ,EAAIhH,EAAK0K,GACrB,GAAIC,GAAYrN,EAAQZ,EAAasD,EAClC2K,UAAiBjO,GAAYsD,GAChC5C,EAAQ4J,EAAIhH,EAAK0K,GACdC,GAAa3D,IAAOtK,GAAYU,EAAQV,EAAasD,EAAK2K,IAC3DvN,EAEAwN,EAAO,SAASC,GAClB,GAAIC,GAAMP,EAAWM,GAAOf,EAAQC,EAAQnN,UAS5C,OARAkO,GAAIC,GAAKF,EACTvP,GAAe8O,GAAUK,EAAc/N,EAAamO,GAClDrE,cAAc,EACdwE,IAAK,SAAS/M,GACTvC,EAAIwF,KAAMmJ,IAAW3O,EAAIwF,KAAKmJ,GAASQ,KAAK3J,KAAKmJ,GAAQQ,IAAO,GACnEJ,EAAcvJ,KAAM2J,EAAKtP,EAAW,EAAG0C,OAGpC6M,GAGLG,EAAW,SAASjE,GACtB,MAAoB,gBAANA,IAGZkE,EAAkB,QAAS/N,gBAAe6J,EAAIhH,EAAK0K,GACrD,MAAGA,IAAKhP,EAAI6O,EAAYvK,IAClB0K,EAAEnE,YAID7K,EAAIsL,EAAIqD,IAAWrD,EAAGqD,GAAQrK,KAAKgH,EAAGqD,GAAQrK,IAAO,GACxD0K,EAAIZ,EAAQY,GAAInE,WAAYhL,EAAW,GAAG,OAJtCG,EAAIsL,EAAIqD,IAAQjN,EAAQ4J,EAAIqD,EAAQ9O,EAAW,OACnDyL,EAAGqD,GAAQrK,IAAO,GAIXyK,EAAczD,EAAIhH,EAAK0K,IACzBtN,EAAQ4J,EAAIhH,EAAK0K,IAExBS,EAAoB,QAAS5N,kBAAiByJ,EAAInJ,GACpD/B,EAASkL,EAKT,KAJA,GAGIhH,GAHA5B,EAAOyL,EAAShM,EAAI3B,EAAU2B,IAC9BU,EAAO,EACP6M,EAAIhN,EAAKE,OAEP8M,EAAI7M,GAAE2M,EAAgBlE,EAAIhH,EAAM5B,EAAKG,KAAMV,EAAEmC,GACnD,OAAOgH,IAELqE,EAAU,QAAS5K,QAAOuG,EAAInJ,GAChC,MAAOA,KAAMvD,EAAYwP,EAAQ9C,GAAMmE,EAAkBrB,EAAQ9C,GAAKnJ,IAEpEyN,EAAwB,QAASpN,sBAAqB8B,GACxD,GAAIuL,GAAI7G,EAAO3J,KAAKmG,KAAMlB,EAC1B,OAAOuL,KAAM7P,EAAIwF,KAAMlB,KAAStE,EAAI6O,EAAYvK,IAAQtE,EAAIwF,KAAMmJ,IAAWnJ,KAAKmJ,GAAQrK,GACtFuL,GAAI,GAENC,EAA4B,QAAS9M,0BAAyBsI,EAAIhH,GACpE,GAAI0K,GAAIpN,EAAQ0J,EAAK9K,EAAU8K,GAAKhH,EAEpC,QADG0K,IAAKhP,EAAI6O,EAAYvK,IAAUtE,EAAIsL,EAAIqD,IAAWrD,EAAGqD,GAAQrK,KAAM0K,EAAEnE,YAAa,GAC9EmE,GAELe,EAAuB,QAASlL,qBAAoByG,GAKtD,IAJA,GAGIhH,GAHAF,EAASU,EAAStE,EAAU8K,IAC5B/G,KACA1B,EAAS,EAEPuB,EAAMxB,OAASC,GAAM7C,EAAI6O,EAAYvK,EAAMF,EAAMvB,OAASyB,GAAOqK,GAAOpK,EAAOC,KAAKF,EAC1F,OAAOC,IAELyL,EAAyB,QAAS9G,uBAAsBoC,GAK1D,IAJA,GAGIhH,GAHAF,EAASU,EAAStE,EAAU8K,IAC5B/G,KACA1B,EAAS,EAEPuB,EAAMxB,OAASC,GAAK7C,EAAI6O,EAAYvK,EAAMF,EAAMvB,OAAM0B,EAAOC,KAAKqK,EAAWvK,GACnF,OAAOC,IAEL0L,EAAa,QAASxB,WAAUnD,GAClC,GAAGA,IAAO1M,IAAa2Q,EAASjE,GAAhC,CAKA,IAJA,GAGI4E,GAAUC,EAHVjL,GAAQoG,GACRzI,EAAO,EACPuN,EAAO1K,UAEL0K,EAAGxN,OAASC,GAAEqC,EAAKV,KAAK4L,EAAGvN,KAQjC,OAPAqN,GAAWhL,EAAK,GACM,kBAAZgL,KAAuBC,EAAYD,IAC1CC,IAAc9J,EAAQ6J,MAAUA,EAAW,SAAS5L,EAAK/B,GAE1D,MADG4N,KAAU5N,EAAQ4N,EAAU9Q,KAAKmG,KAAMlB,EAAK/B,IAC3CgN,EAAShN,GAAb,OAA2BA,IAE7B2C,EAAK,GAAKgL,EACH1B,EAAWzC,MAAMuC,EAAOpJ,KAE7BmL,EAAYxC,EAAO,WACrB,GAAI/K,GAAIuL,GAIR,OAA0B,UAAnBG,GAAY1L,KAAyC,MAAtB0L,GAAYvM,EAAGa,KAAwC,MAAzB0L,EAAWvN,OAAO6B,KAIpFgM,KACFT,EAAU,QAASX,UACjB,GAAG6B,EAAS/J,MAAM,KAAMlD,WAAU,8BAClC,OAAO4M,GAAKzB,EAAI/H,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,KAExD0K,EAAS+E,EAAQnN,UAAW,WAAY,QAASyK,YAC/C,MAAOnG,MAAK6J,KAGdE,EAAW,SAASjE,GAClB,MAAOA,aAAc+C,IAGvB3O,EAAEqF,OAAa4K,EACfjQ,EAAEsJ,OAAa4G,EACflQ,EAAEkC,QAAakO,EACfpQ,EAAEgC,QAAa8N,EACf9P,EAAEoC,SAAa2N,EACf/P,EAAEoF,SAAaoJ,EAAOlM,IAAM+N,EAC5BrQ,EAAEuJ,WAAa+G,EAEZpQ,IAAgBd,EAAoB,KACrCwK,EAAStI,EAAa,uBAAwB4O,GAAuB,GAIzE,IAAIU,IAEFC,MAAO,SAASjM,GACd,MAAOtE,GAAI4O,EAAgBtK,GAAO,IAC9BsK,EAAetK,GACfsK,EAAetK,GAAO+J,EAAQ/J,IAGpCkM,OAAQ,QAASA,QAAOlM,GACtB,MAAO2J,GAAMW,EAAgBtK,IAE/BmM,UAAW,WAAY/B,GAAS,GAChCgC,UAAW,WAAYhC,GAAS,GAalChP,GAAEqH,KAAK1H,KAAK,iHAGV6D,MAAM,KAAM,SAASoI,GACrB,GAAI8D,GAAMpB,EAAI1C,EACdgF,GAAchF,GAAMwD,EAAYM,EAAMF,EAAKE,KAG7CV,GAAS,EAET/O,EAAQA,EAAQsK,EAAItK,EAAQ6K,GAAIkD,OAAQW,IAExC1O,EAAQA,EAAQmD,EAAG,SAAUwN,GAE7B3Q,EAAQA,EAAQmD,EAAInD,EAAQoD,GAAK+L,EAAW,UAE1C/J,OAAQ4K,EAERlO,eAAgB+N,EAEhB3N,iBAAkB4N,EAElBzM,yBAA0B8M,EAE1BjL,oBAAqBkL,EAErB7G,sBAAuB8G,IAIzB1B,GAAS3O,EAAQA,EAAQmD,EAAInD,EAAQoD,IAAM+L,GAAauB,GAAY,QAAS5B,UAAWwB,IAGxFlC,EAAeM,EAAS,UAExBN,EAAerG,KAAM,QAAQ,GAE7BqG,EAAe5E,EAAOoF,KAAM,QAAQ,IAI/B,SAASrP,EAAQD,EAASH,GAE/B,GAAI6R,GAAM7R,EAAoB,GAAG4C,QAC7B1B,EAAMlB,EAAoB,IAC1B8R,EAAM9R,EAAoB,IAAI,cAElCI,GAAOD,QAAU,SAASqM,EAAI6D,EAAK0B,GAC9BvF,IAAOtL,EAAIsL,EAAKuF,EAAOvF,EAAKA,EAAGpK,UAAW0P,IAAKD,EAAIrF,EAAIsF,GAAM9F,cAAc,EAAMvI,MAAO4M,MAKxF,SAASjQ,EAAQD,EAASH,GAE/B,GAAIY,GAAYZ,EAAoB,GAChC0B,EAAY1B,EAAoB,GACpCI,GAAOD,QAAU,SAASoF,EAAQmD,GAMhC,IALA,GAIIlD,GAJApC,EAAS1B,EAAU6D,GACnB3B,EAAShD,EAAEiD,QAAQT,GACnBU,EAASF,EAAKE,OACd8D,EAAS,EAEP9D,EAAS8D,GAAM,GAAGxE,EAAEoC,EAAM5B,EAAKgE,QAAcc,EAAG,MAAOlD,KAK1D,SAASpF,EAAQD,EAASH,GAG/B,GAAI0B,GAAY1B,EAAoB,IAChCgG,EAAYhG,EAAoB,GAAGgG,SACnC6G,KAAeA,SAEfmF,EAA+B,gBAAVrG,SAAsBxJ,OAAO4D,oBAClD5D,OAAO4D,oBAAoB4F,WAE3BsG,EAAiB,SAASzF,GAC5B,IACE,MAAOxG,GAASwG,GAChB,MAAMjJ,GACN,MAAOyO,GAAYxP,SAIvBpC,GAAOD,QAAQ+C,IAAM,QAAS6C,qBAAoByG,GAChD,MAAGwF,IAAoC,mBAArBnF,EAAStM,KAAKiM,GAAgCyF,EAAezF,GACxExG,EAAStE,EAAU8K,MAKvB,SAASpM,EAAQD,EAASH,GAG/B,GAAIY,GAAIZ,EAAoB,EAC5BI,GAAOD,QAAU,SAASqM,GACxB,GAAI5I,GAAahD,EAAEiD,QAAQ2I,GACvBrC,EAAavJ,EAAEuJ,UACnB,IAAGA,EAKD,IAJA,GAGI3E,GAHA0M,EAAU/H,EAAWqC,GACrBtC,EAAUtJ,EAAEsJ,OACZnG,EAAU,EAERmO,EAAQpO,OAASC,GAAKmG,EAAO3J,KAAKiM,EAAIhH,EAAM0M,EAAQnO,OAAMH,EAAK8B,KAAKF,EAE5E,OAAO5B,KAKJ,SAASxD,EAAQD,GAEtBC,EAAOD,SAAU,GAIZ,SAASC,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAG,UAAWkO,OAAQnS,EAAoB,OAIjE,SAASI,EAAQD,EAASH,GAG/B,GAAIY,GAAWZ,EAAoB,GAC/ByB,EAAWzB,EAAoB,IAC/B8B,EAAW9B,EAAoB,GAGnCI,GAAOD,QAAUH,EAAoB,GAAG,WACtC,GAAImD,GAAIhB,OAAOgQ,OACXC,KACA7G,KACAvH,EAAI4K,SACJyD,EAAI,sBAGR,OAFAD,GAAEpO,GAAK,EACPqO,EAAEjO,MAAM,IAAI4D,QAAQ,SAASsK,GAAI/G,EAAE+G,GAAKA,IAClB,GAAfnP,KAAMiP,GAAGpO,IAAW7B,OAAOyB,KAAKT,KAAMoI,IAAI7I,KAAK,KAAO2P,IAC1D,QAASF,QAAO3G,EAAQX,GAQ3B,IAPA,GAAI0H,GAAQ9Q,EAAS+J,GACjB8F,EAAQ1K,UACR4L,EAAQlB,EAAGxN,OACX8D,EAAQ,EACR/D,EAAajD,EAAEiD,QACfsG,EAAavJ,EAAEuJ,WACfD,EAAatJ,EAAEsJ,OACbsI,EAAQ5K,GAMZ,IALA,GAIIpC,GAJAxB,EAASlC,EAAQwP,EAAG1J,MACpBhE,EAASuG,EAAatG,EAAQG,GAAGM,OAAO6F,EAAWnG,IAAMH,EAAQG,GACjEF,EAASF,EAAKE,OACd2O,EAAS,EAEP3O,EAAS2O,GAAKvI,EAAO3J,KAAKyD,EAAGwB,EAAM5B,EAAK6O,QAAMF,EAAE/M,GAAOxB,EAAEwB,GAEjE,OAAO+M,IACLpQ,OAAOgQ,QAIN,SAAS/R,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAClCa,GAAQA,EAAQmD,EAAG,UAAWmJ,GAAInN,EAAoB,OAIjD,SAASI,EAAQD,GAGtBC,EAAOD,QAAUgC,OAAOgL,IAAM,QAASA,IAAGuF,EAAGnJ,GAC3C,MAAOmJ,KAAMnJ,EAAU,IAANmJ,GAAW,EAAIA,IAAM,EAAInJ,EAAImJ,GAAKA,GAAKnJ,GAAKA,IAK1D,SAASnJ,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAClCa,GAAQA,EAAQmD,EAAG,UAAW2O,eAAgB3S,EAAoB,IAAIwQ,OAIjE,SAASpQ,EAAQD,EAASH,GAI/B,GAAI8C,GAAW9C,EAAoB,GAAG8C,QAClCtB,EAAWxB,EAAoB,IAC/BsB,EAAWtB,EAAoB,IAC/B4S,EAAQ,SAASxP,EAAGyP,GAEtB,GADAvR,EAAS8B,IACL5B,EAASqR,IAAoB,OAAVA,EAAe,KAAMrP,WAAUqP,EAAQ,6BAEhEzS,GAAOD,SACLqQ,IAAKrO,OAAOwQ,iBAAmB,gBAC7B,SAASG,EAAMC,EAAOvC,GACpB,IACEA,EAAMxQ,EAAoB,IAAIsG,SAAS/F,KAAMuC,EAAQX,OAAOC,UAAW,aAAaoO,IAAK,GACzFA,EAAIsC,MACJC,IAAUD,YAAgBxQ,QAC1B,MAAMiB,GAAIwP,GAAQ,EACpB,MAAO,SAASJ,gBAAevP,EAAGyP,GAIhC,MAHAD,GAAMxP,EAAGyP,GACNE,EAAM3P,EAAE4P,UAAYH,EAClBrC,EAAIpN,EAAGyP,GACLzP,QAEL,GAAStD,GACjB8S,MAAOA,IAKJ,SAASxS,EAAQD,EAASH,GAI/B,GAAIiT,GAAUjT,EAAoB,IAC9B8S,IACJA,GAAK9S,EAAoB,IAAI,gBAAkB,IAC5C8S,EAAO,IAAM,cACd9S,EAAoB,IAAImC,OAAOC,UAAW,WAAY,QAASyK,YAC7D,MAAO,WAAaoG,EAAQvM,MAAQ,MACnC,IAKA,SAAStG,EAAQD,EAASH,GAG/B,GAAImB,GAAMnB,EAAoB,IAC1B8R,EAAM9R,EAAoB,IAAI,eAE9BkT,EAAgD,aAA1C/R,EAAI,WAAY,MAAOyF,cAEjCxG,GAAOD,QAAU,SAASqM,GACxB,GAAIpJ,GAAGmP,EAAGhH,CACV,OAAOiB,KAAO1M,EAAY,YAAqB,OAAP0M,EAAc,OAEZ,iBAA9B+F,GAAKnP,EAAIjB,OAAOqK,IAAKsF,IAAoBS,EAEjDW,EAAM/R,EAAIiC,GAEM,WAAfmI,EAAIpK,EAAIiC,KAAsC,kBAAZA,GAAE+P,OAAuB,YAAc5H,IAK3E,SAASnL,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,GAEnCA,GAAoB,IAAI,SAAU,SAASoT,GACzC,MAAO,SAASC,QAAO7G,GACrB,MAAO4G,IAAW5R,EAASgL,GAAM4G,EAAQ5G,GAAMA,MAM9C,SAASpM,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9BsK,EAAUtK,EAAoB,GAC9BqB,EAAUrB,EAAoB,EAClCI,GAAOD,QAAU,SAASmT,EAAKpH,GAC7B,GAAIzF,IAAO6D,EAAKnI,YAAcmR,IAAQnR,OAAOmR,GACzCtI,IACJA,GAAIsI,GAAOpH,EAAKzF,GAChB5F,EAAQA,EAAQmD,EAAInD,EAAQoD,EAAI5C,EAAM,WAAYoF,EAAG,KAAQ,SAAUuE,KAKpE,SAAS5K,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,GAEnCA,GAAoB,IAAI,OAAQ,SAASuT,GACvC,MAAO,SAASC,MAAKhH,GACnB,MAAO+G,IAAS/R,EAASgL,GAAM+G,EAAM/G,GAAMA,MAM1C,SAASpM,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,GAEnCA,GAAoB,IAAI,oBAAqB,SAASyT,GACpD,MAAO,SAASC,mBAAkBlH,GAChC,MAAOiH,IAAsBjS,EAASgL,GAAMiH,EAAmBjH,GAAMA,MAMpE,SAASpM,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,GAEnCA,GAAoB,IAAI,WAAY,SAAS2T,GAC3C,MAAO,SAASC,UAASpH,GACvB,MAAOhL,GAASgL,GAAMmH,EAAYA,EAAUnH,IAAM,GAAQ,MAMzD,SAASpM,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,GAEnCA,GAAoB,IAAI,WAAY,SAAS6T,GAC3C,MAAO,SAASC,UAAStH,GACvB,MAAOhL,GAASgL,GAAMqH,EAAYA,EAAUrH,IAAM,GAAQ,MAMzD,SAASpM,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,GAEnCA,GAAoB,IAAI,eAAgB,SAAS+T,GAC/C,MAAO,SAASC,cAAaxH,GAC3B,MAAOhL,GAASgL,GAAMuH,EAAgBA,EAAcvH,IAAM,GAAO,MAMhE,SAASpM,EAAQD,EAASH,GAG/B,GAAI0B,GAAY1B,EAAoB,GAEpCA,GAAoB,IAAI,2BAA4B,SAASgR,GAC3D,MAAO,SAAS9M,0BAAyBsI,EAAIhH,GAC3C,MAAOwL,GAA0BtP,EAAU8K,GAAKhH,OAM/C,SAASpF,EAAQD,EAASH,GAG/B,GAAIyB,GAAWzB,EAAoB,GAEnCA,GAAoB,IAAI,iBAAkB,SAASiU,GACjD,MAAO,SAASrO,gBAAe4G,GAC7B,MAAOyH,GAAgBxS,EAAS+K,QAM/B,SAASpM,EAAQD,EAASH,GAG/B,GAAIyB,GAAWzB,EAAoB,GAEnCA,GAAoB,IAAI,OAAQ,SAASkU,GACvC,MAAO,SAAStQ,MAAK4I,GACnB,MAAO0H,GAAMzS,EAAS+K,QAMrB,SAASpM,EAAQD,EAASH,GAG/BA,EAAoB,IAAI,sBAAuB,WAC7C,MAAOA,GAAoB,IAAIkD,OAK5B,SAAS9C,EAAQD,EAASH,GAE/B,GAAI4C,GAAa5C,EAAoB,GAAG4C,QACpC7B,EAAaf,EAAoB,GACjCkB,EAAalB,EAAoB,IACjCmU,EAAa7N,SAASlE,UACtBgS,EAAa,wBACbC,EAAa,MAEjBA,KAAQF,IAAUnU,EAAoB,IAAM4C,EAAQuR,EAAQE,GAC1DrI,cAAc,EACd9I,IAAK,WACH,GAAIoR,IAAS,GAAK5N,MAAM4N,MAAMF,GAC1BxJ,EAAQ0J,EAAQA,EAAM,GAAK,EAE/B,OADApT,GAAIwF,KAAM2N,IAASzR,EAAQ8D,KAAM2N,EAAMtT,EAAW,EAAG6J,IAC9CA,MAMN,SAASxK,EAAQD,EAASH,GAG/B,GAAIY,GAAgBZ,EAAoB,GACpCwB,EAAgBxB,EAAoB,IACpCuU,EAAgBvU,EAAoB,IAAI,eACxCwU,EAAgBlO,SAASlE,SAExBmS,KAAgBC,IAAe5T,EAAEgC,QAAQ4R,EAAeD,GAAe9Q,MAAO,SAASL,GAC1F,GAAkB,kBAARsD,QAAuBlF,EAAS4B,GAAG,OAAO,CACpD,KAAI5B,EAASkF,KAAKtE,WAAW,MAAOgB,aAAasD,KAEjD,MAAMtD,EAAIxC,EAAEiF,SAASzC,IAAG,GAAGsD,KAAKtE,YAAcgB,EAAE,OAAO,CACvD,QAAO,MAKJ,SAAShD,EAAQD,EAASH,GAG/B,GAAIY,GAAcZ,EAAoB,GAClCqK,EAAcrK,EAAoB,GAClCkB,EAAclB,EAAoB,IAClCmB,EAAcnB,EAAoB,IAClCyU,EAAczU,EAAoB,IAClCqB,EAAcrB,EAAoB,GAClC0U,EAAc1U,EAAoB,IAAI2U,KACtCC,EAAc,SACdC,EAAcxK,EAAOuK,GACrBE,EAAcD,EACdhC,EAAcgC,EAAQzS,UAEtB2S,EAAc5T,EAAIP,EAAEqF,OAAO4M,KAAW+B,EACtCI,EAAc,QAAUpI,QAAOxK,UAG/B6S,EAAW,SAASC,GACtB,GAAI1I,GAAKiI,EAAYS,GAAU,EAC/B,IAAgB,gBAAN1I,IAAkBA,EAAG1I,OAAS,EAAE,CACxC0I,EAAKwI,EAAOxI,EAAGmI,OAASD,EAAMlI,EAAI,EAClC,IACI2I,GAAOC,EAAOC,EADdC,EAAQ9I,EAAG+I,WAAW,EAE1B,IAAa,KAAVD,GAA0B,KAAVA,GAEjB,GADAH,EAAQ3I,EAAG+I,WAAW,GACT,KAAVJ,GAA0B,MAAVA,EAAc,MAAOhM,SACnC,IAAa,KAAVmM,EAAa,CACrB,OAAO9I,EAAG+I,WAAW,IACnB,IAAK,IAAK,IAAK,IAAMH,EAAQ,EAAGC,EAAU,EAAI,MAC9C,KAAK,IAAK,IAAK,KAAMD,EAAQ,EAAGC,EAAU,EAAI,MAC9C,SAAU,OAAQ7I,EAEpB,IAAI,GAAoDgJ,GAAhDC,EAASjJ,EAAGhK,MAAM,GAAIuB,EAAI,EAAG6M,EAAI6E,EAAO3R,OAAkB8M,EAAJ7M,EAAOA,IAInE,GAHAyR,EAAOC,EAAOF,WAAWxR,GAGf,GAAPyR,GAAaA,EAAOH,EAAQ,MAAOlM,IACtC,OAAOuM,UAASD,EAAQL,IAE5B,OAAQ5I,EAGRqI,GAAQ,SAAYA,EAAQ,SAAUA,EAAQ,UAChDA,EAAU,QAASc,QAAOlS,GACxB,GAAI+I,GAAK5F,UAAU9C,OAAS,EAAI,EAAIL,EAChC+C,EAAOE,IACX,OAAOF,aAAgBqO,KAEjBE,EAAa1T,EAAM,WAAYwR,EAAM+C,QAAQrV,KAAKiG,KAAYrF,EAAIqF,IAASoO,GAC3E,GAAIE,GAAKG,EAASzI,IAAOyI,EAASzI,IAE1C5L,EAAEqH,KAAK1H,KAAKP,EAAoB,GAAKY,EAAEoF,SAAS8O,GAAQ,6KAMtD1Q,MAAM,KAAM,SAASoB,GAClBtE,EAAI4T,EAAMtP,KAAStE,EAAI2T,EAASrP,IACjC5E,EAAEgC,QAAQiS,EAASrP,EAAK5E,EAAEkC,QAAQgS,EAAMtP,MAG5CqP,EAAQzS,UAAYyQ,EACpBA,EAAM/M,YAAc+O,EACpB7U,EAAoB,IAAIqK,EAAQuK,EAAQC,KAKrC,SAASzU,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,GAGnCI,GAAOD,QAAU,SAASqM,EAAIxI,GAC5B,IAAIxC,EAASgL,GAAI,MAAOA,EACxB,IAAI/F,GAAIgG,CACR,IAAGzI,GAAkC,mBAArByC,EAAK+F,EAAGK,YAA4BrL,EAASiL,EAAMhG,EAAGlG,KAAKiM,IAAK,MAAOC,EACvF,IAA+B,mBAApBhG,EAAK+F,EAAGoJ,WAA2BpU,EAASiL,EAAMhG,EAAGlG,KAAKiM,IAAK,MAAOC,EACjF,KAAIzI,GAAkC,mBAArByC,EAAK+F,EAAGK,YAA4BrL,EAASiL,EAAMhG,EAAGlG,KAAKiM,IAAK,MAAOC,EACxF,MAAMjJ,WAAU,6CAKb,SAASpD,EAAQD,EAASH,GAE/B,GAAIa,GAAUb,EAAoB,GAC9BsN,EAAUtN,EAAoB,IAC9BqB,EAAUrB,EAAoB,GAC9B6V,EAAU,+CAEVC,EAAU,IAAMD,EAAS,IACzBE,EAAU,KACVC,EAAUC,OAAO,IAAMH,EAAQA,EAAQ,KACvCI,EAAUD,OAAOH,EAAQA,EAAQ,MAEjCK,EAAW,SAAS7C,EAAKpH,GAC3B,GAAIlB,KACJA,GAAIsI,GAAOpH,EAAKyI,GAChB9T,EAAQA,EAAQwC,EAAIxC,EAAQoD,EAAI5C,EAAM,WACpC,QAASwU,EAAOvC,MAAUyC,EAAIzC,MAAUyC,IACtC,SAAU/K,IAMZ2J,EAAOwB,EAASxB,KAAO,SAASyB,EAAQxI,GAI1C,MAHAwI,GAASxJ,OAAOU,EAAQ8I,IACd,EAAPxI,IAASwI,EAASA,EAAOC,QAAQL,EAAO,KACjC,EAAPpI,IAASwI,EAASA,EAAOC,QAAQH,EAAO,KACpCE,EAGThW,GAAOD,QAAUgW,GAIZ,SAAS/V,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UAAWsS,QAAS1N,KAAK2N,IAAI,EAAG,QAI9C,SAASnW,EAAQD,EAASH,GAG/B,GAAIa,GAAYb,EAAoB,GAChCwW,EAAYxW,EAAoB,GAAGoJ,QAEvCvI,GAAQA,EAAQmD,EAAG,UACjBoF,SAAU,QAASA,UAASoD,GAC1B,MAAoB,gBAANA,IAAkBgK,EAAUhK,OAMzC,SAASpM,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UAAWyS,UAAWzW,EAAoB,OAIxD,SAASI,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,IAC/BwN,EAAW5E,KAAK4E,KACpBpN,GAAOD,QAAU,QAASsW,WAAUjK,GAClC,OAAQhL,EAASgL,IAAOpD,SAASoD,IAAOgB,EAAMhB,KAAQA,IAKnD,SAASpM,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UACjByJ,MAAO,QAASA,OAAMiJ,GACpB,MAAOA,IAAUA,MAMhB,SAAStW,EAAQD,EAASH,GAG/B,GAAIa,GAAYb,EAAoB,GAChCyW,EAAYzW,EAAoB,IAChC2J,EAAYf,KAAKe,GAErB9I,GAAQA,EAAQmD,EAAG,UACjB2S,cAAe,QAASA,eAAcD,GACpC,MAAOD,GAAUC,IAAW/M,EAAI+M,IAAW,qBAM1C,SAAStW,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UAAW4S,iBAAkB,oBAI3C,SAASxW,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UAAW6S,iBAAkB,qBAI3C,SAASzW,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UAAW8S,WAAYA,cAIrC,SAAS1W,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UAAW0R,SAAUA,YAInC,SAAStV,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B+W,EAAU/W,EAAoB,IAC9BgX,EAAUpO,KAAKoO,KACfC,EAAUrO,KAAKsO,KAGnBrW,GAAQA,EAAQmD,EAAInD,EAAQoD,IAAMgT,GAAkD,KAAxCrO,KAAK4E,MAAMyJ,EAAOtB,OAAOwB,aAAqB,QACxFD,MAAO,QAASA,OAAMxE,GACpB,OAAQA,GAAKA,GAAK,EAAIvJ,IAAMuJ,EAAI,kBAC5B9J,KAAKwO,IAAI1E,GAAK9J,KAAKyO,IACnBN,EAAMrE,EAAI,EAAIsE,EAAKtE,EAAI,GAAKsE,EAAKtE,EAAI,QAMxC,SAAStS,EAAQD,GAGtBC,EAAOD,QAAUyI,KAAKmO,OAAS,QAASA,OAAMrE,GAC5C,OAAQA,GAAKA,GAAK,OAAa,KAAJA,EAAWA,EAAIA,EAAIA,EAAI,EAAI9J,KAAKwO,IAAI,EAAI1E,KAKhE,SAAStS,EAAQD,EAASH,GAK/B,QAASsX,OAAM5E,GACb,MAAQtJ,UAASsJ,GAAKA,IAAW,GAALA,EAAiB,EAAJA,GAAS4E,OAAO5E,GAAK9J,KAAKwO,IAAI1E,EAAI9J,KAAKoO,KAAKtE,EAAIA,EAAI,IAAxDA,EAHvC,GAAI7R,GAAUb,EAAoB,EAMlCa,GAAQA,EAAQmD,EAAG,QAASsT,MAAOA,SAI9B,SAASlX,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QACjBuT,MAAO,QAASA,OAAM7E,GACpB,MAAmB,KAAXA,GAAKA,GAAUA,EAAI9J,KAAKwO,KAAK,EAAI1E,IAAM,EAAIA,IAAM,MAMxD,SAAStS,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9BwX,EAAUxX,EAAoB,GAElCa,GAAQA,EAAQmD,EAAG,QACjByT,KAAM,QAASA,MAAK/E,GAClB,MAAO8E,GAAK9E,GAAKA,GAAK9J,KAAK2N,IAAI3N,KAAKe,IAAI+I,GAAI,EAAI,OAM/C,SAAStS,EAAQD,GAGtBC,EAAOD,QAAUyI,KAAK4O,MAAQ,QAASA,MAAK9E,GAC1C,MAAmB,KAAXA,GAAKA,IAAWA,GAAKA,EAAIA,EAAQ,EAAJA,EAAQ,GAAK,IAK/C,SAAStS,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QACjB0T,MAAO,QAASA,OAAMhF,GACpB,OAAQA,KAAO,GAAK,GAAK9J,KAAK4E,MAAM5E,KAAKwO,IAAI1E,EAAI,IAAO9J,KAAK+O,OAAS,OAMrE,SAASvX,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9BgL,EAAUpC,KAAKoC,GAEnBnK,GAAQA,EAAQmD,EAAG,QACjB4T,KAAM,QAASA,MAAKlF,GAClB,OAAQ1H,EAAI0H,GAAKA,GAAK1H,GAAK0H,IAAM,MAMhC,SAAStS,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QAAS6T,MAAO7X,EAAoB,OAIlD,SAASI,EAAQD,GAGtBC,EAAOD,QAAUyI,KAAKiP,OAAS,QAASA,OAAMnF,GAC5C,MAAmB,KAAXA,GAAKA,GAAUA,EAAIA,GAAK,MAAY,KAAJA,EAAWA,EAAIA,EAAIA,EAAI,EAAI9J,KAAKoC,IAAI0H,GAAK,IAK9E,SAAStS,EAAQD,EAASH,GAG/B,GAAIa,GAAYb,EAAoB,GAChCwX,EAAYxX,EAAoB,IAChCuW,EAAY3N,KAAK2N,IACjBD,EAAYC,EAAI,EAAG,KACnBuB,EAAYvB,EAAI,EAAG,KACnBwB,EAAYxB,EAAI,EAAG,MAAQ,EAAIuB,GAC/BE,EAAYzB,EAAI,EAAG,MAEnB0B,EAAkB,SAAS5R,GAC7B,MAAOA,GAAI,EAAIiQ,EAAU,EAAIA,EAI/BzV,GAAQA,EAAQmD,EAAG,QACjBkU,OAAQ,QAASA,QAAOxF,GACtB,GAEIvP,GAAGsC,EAFH0S,EAAQvP,KAAKe,IAAI+I,GACjB0F,EAAQZ,EAAK9E,EAEjB,OAAUsF,GAAPG,EAAoBC,EAAQH,EAAgBE,EAAOH,EAAQF,GAAaE,EAAQF,GACnF3U,GAAK,EAAI2U,EAAYxB,GAAW6B,EAChC1S,EAAStC,GAAKA,EAAIgV,GACf1S,EAASsS,GAAStS,GAAUA,EAAc2S,GAAQC,EAAAA,GAC9CD,EAAQ3S,OAMd,SAASrF,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B2J,EAAUf,KAAKe,GAEnB9I,GAAQA,EAAQmD,EAAG,QACjBsU,MAAO,QAASA,OAAMC,EAAQC,GAO5B,IANA,GAKI/J,GAAKgK,EALLC,EAAQ,EACR3U,EAAQ,EACRuN,EAAQ1K,UACR4L,EAAQlB,EAAGxN,OACX6U,EAAQ,EAEFnG,EAAJzO,GACJ0K,EAAM9E,EAAI2H,EAAGvN,MACH0K,EAAPkK,GACDF,EAAOE,EAAOlK,EACdiK,EAAOA,EAAMD,EAAMA,EAAM,EACzBE,EAAOlK,GACCA,EAAM,GACdgK,EAAOhK,EAAMkK,EACbD,GAAOD,EAAMA,GACRC,GAAOjK,CAEhB,OAAOkK,KAASN,EAAAA,EAAWA,EAAAA,EAAWM,EAAO/P,KAAKoO,KAAK0B,OAMtD,SAAStY,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B4Y,EAAUhQ,KAAKiQ,IAGnBhY,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAIjE,EAAoB,GAAG,WACrD,MAA+B,IAAxB4Y,EAAM,WAAY,IAA4B,GAAhBA,EAAM9U,SACzC,QACF+U,KAAM,QAASA,MAAKnG,EAAGnJ,GACrB,GAAIuP,GAAS,MACTC,GAAMrG,EACNsG,GAAMzP,EACN0P,EAAKH,EAASC,EACdG,EAAKJ,EAASE,CAClB,OAAO,GAAIC,EAAKC,IAAOJ,EAASC,IAAO,IAAMG,EAAKD,GAAMH,EAASE,IAAO,KAAO,KAAO,OAMrF,SAAS5Y,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QACjBmV,MAAO,QAASA,OAAMzG,GACpB,MAAO9J,MAAKwO,IAAI1E,GAAK9J,KAAKwQ,SAMzB,SAAShZ,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QAAS+S,MAAO/W,EAAoB,OAIlD,SAASI,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QACjBqV,KAAM,QAASA,MAAK3G,GAClB,MAAO9J,MAAKwO,IAAI1E,GAAK9J,KAAKyO,QAMzB,SAASjX,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QAASwT,KAAMxX,EAAoB,OAIjD,SAASI,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B6X,EAAU7X,EAAoB,IAC9BgL,EAAUpC,KAAKoC,GAGnBnK,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAIjE,EAAoB,GAAG,WACrD,MAA6B,SAArB4I,KAAK0Q,KAAK,UAChB,QACFA,KAAM,QAASA,MAAK5G,GAClB,MAAO9J,MAAKe,IAAI+I,GAAKA,GAAK,GACrBmF,EAAMnF,GAAKmF,GAAOnF,IAAM,GACxB1H,EAAI0H,EAAI,GAAK1H,GAAK0H,EAAI,KAAO9J,KAAKmI,EAAI,OAM1C,SAAS3Q,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B6X,EAAU7X,EAAoB,IAC9BgL,EAAUpC,KAAKoC,GAEnBnK,GAAQA,EAAQmD,EAAG,QACjBuV,KAAM,QAASA,MAAK7G,GAClB,GAAIvP,GAAI0U,EAAMnF,GAAKA,GACf1F,EAAI6K,GAAOnF,EACf,OAAOvP,IAAKkV,EAAAA,EAAW,EAAIrL,GAAKqL,EAAAA,EAAW,IAAMlV,EAAI6J,IAAMhC,EAAI0H,GAAK1H,GAAK0H,QAMxE,SAAStS,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QACjBwV,MAAO,QAASA,OAAMhN,GACpB,OAAQA,EAAK,EAAI5D,KAAK4E,MAAQ5E,KAAK2E,MAAMf,OAMxC,SAASpM,EAAQD,EAASH,GAE/B,GAAIa,GAAiBb,EAAoB,GACrC4B,EAAiB5B,EAAoB,IACrCyZ,EAAiB7M,OAAO6M,aACxBC,EAAiB9M,OAAO+M,aAG5B9Y,GAAQA,EAAQmD,EAAInD,EAAQoD,KAAOyV,GAA2C,GAAzBA,EAAe5V,QAAc,UAEhF6V,cAAe,QAASA,eAAcjH,GAMpC,IALA,GAII8C,GAJApH,KACAkD,EAAQ1K,UACR4L,EAAQlB,EAAGxN,OACXC,EAAQ,EAENyO,EAAQzO,GAAE,CAEd,GADAyR,GAAQlE,EAAGvN,KACRnC,EAAQ4T,EAAM,WAAcA,EAAK,KAAMnM,YAAWmM,EAAO,6BAC5DpH,GAAI1I,KAAY,MAAP8P,EACLiE,EAAajE,GACbiE,IAAejE,GAAQ,QAAY,IAAM,MAAQA,EAAO,KAAQ,QAEpE,MAAOpH,GAAI1L,KAAK,QAMjB,SAAStC,EAAQD,EAASH,GAE/B,GAAIa,GAAYb,EAAoB,GAChC0B,EAAY1B,EAAoB,IAChC6B,EAAY7B,EAAoB,GAEpCa,GAAQA,EAAQmD,EAAG,UAEjB4V,IAAK,QAASA,KAAIC,GAOhB,IANA,GAAIC,GAAQpY,EAAUmY,EAASD,KAC3BzT,EAAQtE,EAASiY,EAAIhW,QACrBwN,EAAQ1K,UACR4L,EAAQlB,EAAGxN,OACXsK,KACArK,EAAQ,EACNoC,EAAMpC,GACVqK,EAAI1I,KAAKkH,OAAOkN,EAAI/V,OACbyO,EAAJzO,GAAUqK,EAAI1I,KAAKkH,OAAO0E,EAAGvN,IAChC,OAAOqK,GAAI1L,KAAK,QAMjB,SAAStC,EAAQD,EAASH,GAI/BA,EAAoB,IAAI,OAAQ,SAAS0U,GACvC,MAAO,SAASC,QACd,MAAOD,GAAMhO,KAAM,OAMlB,SAAStG,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B+Z,EAAU/Z,EAAoB,KAAI,EACtCa,GAAQA,EAAQwC,EAAG,UAEjB2W,YAAa,QAASA,aAAYC,GAChC,MAAOF,GAAIrT,KAAMuT,OAMhB,SAAS7Z,EAAQD,EAASH,GAE/B,GAAI2B,GAAY3B,EAAoB,IAChCsN,EAAYtN,EAAoB,GAGpCI,GAAOD,QAAU,SAASiM,GACxB,MAAO,UAAS5F,EAAMyT,GACpB,GAGI9W,GAAG6J,EAHHtD,EAAIkD,OAAOU,EAAQ9G,IACnBzC,EAAIpC,EAAUsY,GACdrJ,EAAIlH,EAAE5F,MAEV,OAAO,GAAJC,GAASA,GAAK6M,EAASxE,EAAY,GAAKtM,GAC3CqD,EAAIuG,EAAE6L,WAAWxR,GACN,MAAJZ,GAAcA,EAAI,OAAUY,EAAI,IAAM6M,IAAM5D,EAAItD,EAAE6L,WAAWxR,EAAI,IAAM,OAAUiJ,EAAI,MACxFZ,EAAY1C,EAAErC,OAAOtD,GAAKZ,EAC1BiJ,EAAY1C,EAAElH,MAAMuB,EAAGA,EAAI,IAAMZ,EAAI,OAAU,KAAO6J,EAAI,OAAU,UAMvE,SAAS5M,EAAQD,EAASH,GAI/B,GAAIa,GAAYb,EAAoB,GAChC6B,EAAY7B,EAAoB,IAChCka,EAAYla,EAAoB,KAChCma,EAAY,WACZC,EAAY,GAAGD,EAEnBtZ,GAAQA,EAAQwC,EAAIxC,EAAQoD,EAAIjE,EAAoB,KAAKma,GAAY,UACnEE,SAAU,QAASA,UAASC,GAC1B,GAAI9T,GAAO0T,EAAQxT,KAAM4T,EAAcH,GACnC7I,EAAO1K,UACP2T,EAAcjJ,EAAGxN,OAAS,EAAIwN,EAAG,GAAKxR,EACtCqG,EAAStE,EAAS2E,EAAK1C,QACvBiD,EAASwT,IAAgBza,EAAYqG,EAAMyC,KAAKC,IAAIhH,EAAS0Y,GAAcpU,GAC3EqU,EAAS5N,OAAO0N,EACpB,OAAOF,GACHA,EAAU7Z,KAAKiG,EAAMgU,EAAQzT,GAC7BP,EAAKhE,MAAMuE,EAAMyT,EAAO1W,OAAQiD,KAASyT,MAM5C,SAASpa,EAAQD,EAASH,GAG/B,GAAIya,GAAWza,EAAoB,KAC/BsN,EAAWtN,EAAoB,GAEnCI,GAAOD,QAAU,SAASqG,EAAM8T,EAAcjG,GAC5C,GAAGoG,EAASH,GAAc,KAAM9W,WAAU,UAAY6Q,EAAO,yBAC7D,OAAOzH,QAAOU,EAAQ9G,MAKnB,SAASpG,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,IAC/BmB,EAAWnB,EAAoB,IAC/B0a,EAAW1a,EAAoB,IAAI,QACvCI,GAAOD,QAAU,SAASqM,GACxB,GAAIiO,EACJ,OAAOjZ,GAASgL,MAASiO,EAAWjO,EAAGkO,MAAY5a,IAAc2a,EAAsB,UAAXtZ,EAAIqL,MAK7E,SAASpM,EAAQD,EAASH,GAE/B,GAAI0a,GAAQ1a,EAAoB,IAAI,QACpCI,GAAOD,QAAU,SAASmT,GACxB,GAAIqH,GAAK,GACT,KACE,MAAMrH,GAAKqH,GACX,MAAMpX,GACN,IAEE,MADAoX,GAAGD,IAAS,GACJ,MAAMpH,GAAKqH,GACnB,MAAMtM,KACR,OAAO,IAKN,SAASjO,EAAQD,EAASH,GAI/B,GAAIa,GAAWb,EAAoB,GAC/Bka,EAAWla,EAAoB,KAC/B4a,EAAW,UAEf/Z,GAAQA,EAAQwC,EAAIxC,EAAQoD,EAAIjE,EAAoB,KAAK4a,GAAW,UAClEC,SAAU,QAASA,UAASP,GAC1B,SAAUJ,EAAQxT,KAAM4T,EAAcM,GACnCpS,QAAQ8R,EAAc1T,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,OAM9D,SAASM,EAAQD,EAASH,GAE/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQwC,EAAG,UAEjByX,OAAQ9a,EAAoB,QAKzB,SAASI,EAAQD,EAASH,GAG/B,GAAI2B,GAAY3B,EAAoB,IAChCsN,EAAYtN,EAAoB,GAEpCI,GAAOD,QAAU,QAAS2a,QAAOC,GAC/B,GAAIC,GAAMpO,OAAOU,EAAQ5G,OACrB0H,EAAM,GACN/H,EAAM1E,EAAUoZ,EACpB,IAAO,EAAJ1U,GAASA,GAAKgS,EAAAA,EAAS,KAAMhP,YAAW,0BAC3C,MAAKhD,EAAI,GAAIA,KAAO,KAAO2U,GAAOA,GAAY,EAAJ3U,IAAM+H,GAAO4M,EACvD,OAAO5M,KAKJ,SAAShO,EAAQD,EAASH,GAI/B,GAAIa,GAAcb,EAAoB,GAClC6B,EAAc7B,EAAoB,IAClCka,EAAcla,EAAoB,KAClCib,EAAc,aACdC,EAAc,GAAGD,EAErBpa,GAAQA,EAAQwC,EAAIxC,EAAQoD,EAAIjE,EAAoB,KAAKib,GAAc,UACrEE,WAAY,QAASA,YAAWb,GAC9B,GAAI9T,GAAS0T,EAAQxT,KAAM4T,EAAcW,GACrC3J,EAAS1K,UACTgB,EAAS/F,EAAS+G,KAAKC,IAAIyI,EAAGxN,OAAS,EAAIwN,EAAG,GAAKxR,EAAW0G,EAAK1C,SACnE0W,EAAS5N,OAAO0N,EACpB,OAAOY,GACHA,EAAY3a,KAAKiG,EAAMgU,EAAQ5S,GAC/BpB,EAAKhE,MAAMoF,EAAOA,EAAQ4S,EAAO1W,UAAY0W,MAMhD,SAASpa,EAAQD,EAASH,GAG/B,GAAI+Z,GAAO/Z,EAAoB,KAAI,EAGnCA,GAAoB,KAAK4M,OAAQ,SAAU,SAASwO,GAClD1U,KAAK2U,GAAKzO,OAAOwO,GACjB1U,KAAK4U,GAAK,GAET,WACD,GAEIC,GAFAnY,EAAQsD,KAAK2U,GACbzT,EAAQlB,KAAK4U,EAEjB,OAAG1T,IAASxE,EAAEU,QAAeL,MAAO3D,EAAW0b,MAAM,IACrDD,EAAQxB,EAAI3W,EAAGwE,GACflB,KAAK4U,IAAMC,EAAMzX,QACTL,MAAO8X,EAAOC,MAAM,OAKzB,SAASpb,EAAQD,EAASH,GAG/B,GAAIyb,GAAiBzb,EAAoB,IACrCa,EAAiBb,EAAoB,GACrCwK,EAAiBxK,EAAoB,IACrCuK,EAAiBvK,EAAoB,GACrCkB,EAAiBlB,EAAoB,IACrC0b,EAAiB1b,EAAoB,KACrC2b,EAAiB3b,EAAoB,KACrCiP,EAAiBjP,EAAoB,IACrC6F,EAAiB7F,EAAoB,GAAG6F,SACxC+V,EAAiB5b,EAAoB,IAAI,YACzC6b,OAAsBjY,MAAQ,WAAaA,QAC3CkY,EAAiB,aACjBC,EAAiB,OACjBC,EAAiB,SAEjBC,EAAa,WAAY,MAAOvV,MAEpCtG,GAAOD,QAAU,SAAS2U,EAAMT,EAAM6H,EAAaC,EAAMC,EAASC,EAAQC,GACxEX,EAAYO,EAAa7H,EAAM8H,EAC/B,IAaII,GAAS/W,EAbTgX,EAAY,SAASC,GACvB,IAAIZ,GAASY,IAAQ5J,GAAM,MAAOA,GAAM4J,EACxC,QAAOA,GACL,IAAKV,GAAM,MAAO,SAASnY,QAAQ,MAAO,IAAIsY,GAAYxV,KAAM+V,GAChE,KAAKT,GAAQ,MAAO,SAASU,UAAU,MAAO,IAAIR,GAAYxV,KAAM+V,IACpE,MAAO,SAASE,WAAW,MAAO,IAAIT,GAAYxV,KAAM+V,KAExD3K,EAAauC,EAAO,YACpBuI,EAAaR,GAAWJ,EACxBa,GAAa,EACbhK,EAAaiC,EAAK1S,UAClB0a,EAAajK,EAAM+I,IAAa/I,EAAMiJ,IAAgBM,GAAWvJ,EAAMuJ,GACvEW,EAAaD,GAAWN,EAAUJ,EAGtC,IAAGU,EAAQ,CACT,GAAIE,GAAoBnX,EAASkX,EAASxc,KAAK,GAAIuU,IAEnD7F,GAAe+N,EAAmBlL,GAAK,IAEnC2J,GAAWva,EAAI2R,EAAOiJ,IAAavR,EAAKyS,EAAmBpB,EAAUK,GAEtEW,GAAcE,EAAQlS,OAASoR,IAChCa,GAAa,EACbE,EAAW,QAASL,UAAU,MAAOI,GAAQvc,KAAKmG,QAUtD,GANK+U,IAAWa,IAAYT,IAASgB,GAAehK,EAAM+I,IACxDrR,EAAKsI,EAAO+I,EAAUmB,GAGxBrB,EAAUrH,GAAQ0I,EAClBrB,EAAU5J,GAAQmK,EACfG,EAMD,GALAG,GACEG,OAASE,EAAcG,EAAWP,EAAUR,GAC5CpY,KAASyY,EAAcU,EAAWP,EAAUT,GAC5CY,QAAUC,EAAwBJ,EAAU,WAArBO,GAEtBT,EAAO,IAAI9W,IAAO+W,GACd/W,IAAOqN,IAAOrI,EAASqI,EAAOrN,EAAK+W,EAAQ/W,QAC3C3E,GAAQA,EAAQwC,EAAIxC,EAAQoD,GAAK4X,GAASgB,GAAaxI,EAAMkI,EAEtE,OAAOA,KAKJ,SAASnc,EAAQD,GAEtBC,EAAOD,YAIF,SAASC,EAAQD,EAASH,GAG/B,GAAIY,GAAiBZ,EAAoB,GACrCid,EAAiBjd,EAAoB,GACrCiP,EAAiBjP,EAAoB,IACrCgd,IAGJhd,GAAoB,GAAGgd,EAAmBhd,EAAoB,IAAI,YAAa,WAAY,MAAO0G,QAElGtG,EAAOD,QAAU,SAAS+b,EAAa7H,EAAM8H,GAC3CD,EAAY9Z,UAAYxB,EAAEqF,OAAO+W,GAAoBb,KAAMc,EAAW,EAAGd,KACzElN,EAAeiN,EAAa7H,EAAO,eAKhC,SAASjU,EAAQD,EAASH,GAG/B,GAAIyK,GAAczK,EAAoB,IAClCa,EAAcb,EAAoB,GAClCyB,EAAczB,EAAoB,IAClCO,EAAcP,EAAoB,KAClCkd,EAAcld,EAAoB,KAClC6B,EAAc7B,EAAoB,IAClCmd,EAAcnd,EAAoB,IACtCa,GAAQA,EAAQmD,EAAInD,EAAQoD,GAAKjE,EAAoB,KAAK,SAASod,GAAO9a,MAAM+a,KAAKD,KAAW,SAE9FC,KAAM,QAASA,MAAKC,GAClB,GAQIxZ,GAAQ2B,EAAQ8X,EAAMC,EARtBpa,EAAU3B,EAAS6b,GACnB9O,EAAyB,kBAAR9H,MAAqBA,KAAOpE,MAC7CgP,EAAU1K,UACV4L,EAAUlB,EAAGxN,OACb2Z,EAAUjL,EAAQ,EAAIlB,EAAG,GAAKxR,EAC9B4d,EAAUD,IAAU3d,EACpB8H,EAAU,EACV+V,EAAUR,EAAU/Z,EAIxB,IAFGsa,IAAQD,EAAQhT,EAAIgT,EAAOjL,EAAQ,EAAIlB,EAAG,GAAKxR,EAAW,IAE1D6d,GAAU7d,GAAe0O,GAAKlM,OAAS4a,EAAYS,GAMpD,IADA7Z,EAASjC,EAASuB,EAAEU,QAChB2B,EAAS,GAAI+I,GAAE1K,GAASA,EAAS8D,EAAOA,IAC1CnC,EAAOmC,GAAS8V,EAAUD,EAAMra,EAAEwE,GAAQA,GAASxE,EAAEwE,OANvD,KAAI4V,EAAWG,EAAOpd,KAAK6C,GAAIqC,EAAS,GAAI+I,KAAK+O,EAAOC,EAASrB,QAAQX,KAAM5T,IAC7EnC,EAAOmC,GAAS8V,EAAUnd,EAAKid,EAAUC,GAAQF,EAAK9Z,MAAOmE,IAAQ,GAAQ2V,EAAK9Z,KAStF,OADAgC,GAAO3B,OAAS8D,EACTnC,MAON,SAASrF,EAAQD,EAASH,GAG/B,GAAIsB,GAAWtB,EAAoB,GACnCI,GAAOD,QAAU,SAASqd,EAAU/W,EAAIhD,EAAOkZ,GAC7C,IACE,MAAOA,GAAUlW,EAAGnF,EAASmC,GAAO,GAAIA,EAAM,IAAMgD,EAAGhD,GAEvD,MAAMF,GACN,GAAIqa,GAAMJ,EAAS,SAEnB,MADGI,KAAQ9d,GAAUwB,EAASsc,EAAIrd,KAAKid,IACjCja,KAML,SAASnD,EAAQD,EAASH,GAG/B,GAAI0b,GAAa1b,EAAoB,KACjC4b,EAAa5b,EAAoB,IAAI,YACrCqC,EAAaC,MAAMF,SAEvBhC,GAAOD,QAAU,SAASqM,GACxB,MAAOA,KAAO1M,IAAc4b,EAAUpZ,QAAUkK,GAAMnK,EAAWuZ,KAAcpP,KAK5E,SAASpM,EAAQD,EAASH,GAE/B,GAAIiT,GAAYjT,EAAoB,IAChC4b,EAAY5b,EAAoB,IAAI,YACpC0b,EAAY1b,EAAoB,IACpCI,GAAOD,QAAUH,EAAoB,GAAG6d,kBAAoB,SAASrR,GACnE,MAAGA,IAAM1M,EAAiB0M,EAAGoP,IACxBpP,EAAG,eACHkP,EAAUzI,EAAQzG,IAFvB,SAOG,SAASpM,EAAQD,EAASH,GAE/B,GAAI4b,GAAe5b,EAAoB,IAAI,YACvC8d,GAAe,CAEnB,KACE,GAAIC,IAAS,GAAGnC,IAChBmC,GAAM,UAAY,WAAYD,GAAe,GAC7Cxb,MAAM+a,KAAKU,EAAO,WAAY,KAAM,KACpC,MAAMxa,IAERnD,EAAOD,QAAU,SAAS+L,EAAM8R,GAC9B,IAAIA,IAAgBF,EAAa,OAAO,CACxC,IAAIpR,IAAO,CACX,KACE,GAAIuR,IAAQ,GACRb,EAAOa,EAAIrC,IACfwB,GAAKjB,KAAO,WAAY,OAAQX,KAAM9O,GAAO,IAC7CuR,EAAIrC,GAAY,WAAY,MAAOwB,IACnClR,EAAK+R,GACL,MAAM1a,IACR,MAAOmJ,KAKJ,SAAStM,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAGlCa,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAIjE,EAAoB,GAAG,WACrD,QAASiE,MACT,QAAS3B,MAAM4b,GAAG3d,KAAK0D,YAAcA,MACnC,SAEFia,GAAI,QAASA,MAKX,IAJA,GAAItW,GAAS,EACT0J,EAAS1K,UACT4L,EAASlB,EAAGxN,OACZ2B,EAAS,IAAoB,kBAARiB,MAAqBA,KAAOpE,OAAOkQ,GACtDA,EAAQ5K,GAAMnC,EAAOmC,GAAS0J,EAAG1J,IAEvC,OADAnC,GAAO3B,OAAS0O,EACT/M,MAMN,SAASrF,EAAQD,EAASH,GAG/B,GAAIme,GAAmBne,EAAoB,KACvCud,EAAmBvd,EAAoB,KACvC0b,EAAmB1b,EAAoB,KACvC0B,EAAmB1B,EAAoB,GAM3CI,GAAOD,QAAUH,EAAoB,KAAKsC,MAAO,QAAS,SAAS8Y,EAAUqB,GAC3E/V,KAAK2U,GAAK3Z,EAAU0Z,GACpB1U,KAAK4U,GAAK,EACV5U,KAAK6J,GAAKkM,GAET,WACD,GAAIrZ,GAAQsD,KAAK2U,GACboB,EAAQ/V,KAAK6J,GACb3I,EAAQlB,KAAK4U,IACjB,QAAIlY,GAAKwE,GAASxE,EAAEU,QAClB4C,KAAK2U,GAAKvb,EACHyd,EAAK,IAEH,QAARd,EAAwBc,EAAK,EAAG3V,GACxB,UAAR6U,EAAwBc,EAAK,EAAGna,EAAEwE,IAC9B2V,EAAK,GAAI3V,EAAOxE,EAAEwE,MACxB,UAGH8T,EAAU0C,UAAY1C,EAAUpZ,MAEhC6b,EAAiB,QACjBA,EAAiB,UACjBA,EAAiB,YAIZ,SAAS/d,EAAQD,EAASH,GAG/B,GAAIqe,GAAcre,EAAoB,IAAI,eACtCqC,EAAcC,MAAMF,SACrBC,GAAWgc,IAAgBve,GAAUE,EAAoB,GAAGqC,EAAYgc,MAC3Eje,EAAOD,QAAU,SAASqF,GACxBnD,EAAWgc,GAAa7Y,IAAO,IAK5B,SAASpF,EAAQD,GAEtBC,EAAOD,QAAU,SAASqb,EAAM/X,GAC9B,OAAQA,MAAOA,EAAO+X,OAAQA,KAK3B,SAASpb,EAAQD,EAASH,GAE/BA,EAAoB,KAAK,UAIpB,SAASI,EAAQD,EAASH,GAG/B,GAAIqK,GAAcrK,EAAoB,GAClCY,EAAcZ,EAAoB,GAClCc,EAAcd,EAAoB,GAClCsO,EAActO,EAAoB,IAAI,UAE1CI,GAAOD,QAAU,SAASmT,GACxB,GAAI9E,GAAInE,EAAOiJ,EACZxS,IAAe0N,IAAMA,EAAEF,IAAS1N,EAAEgC,QAAQ4L,EAAGF,GAC9CtC,cAAc,EACd9I,IAAK,WAAY,MAAOwD,WAMvB,SAAStG,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQwC,EAAG,SAAUib,WAAYte,EAAoB,OAE7DA,EAAoB,KAAK,eAIpB,SAASI,EAAQD,EAASH,GAI/B,GAAIyB,GAAWzB,EAAoB,IAC/B4B,EAAW5B,EAAoB,IAC/B6B,EAAW7B,EAAoB,GAEnCI,GAAOD,WAAame,YAAc,QAASA,YAAW9S,EAAevE,GACnE,GAAI7D,GAAQ3B,EAASiF,MACjBP,EAAQtE,EAASuB,EAAEU,QACnBya,EAAQ3c,EAAQ4J,EAAQrF,GACxBkX,EAAQzb,EAAQqF,EAAOd,GACvBmL,EAAQ1K,UACRG,EAAQuK,EAAGxN,OAAS,EAAIwN,EAAG,GAAKxR,EAChCib,EAAQnS,KAAKC,KAAK9B,IAAQjH,EAAYqG,EAAMvE,EAAQmF,EAAKZ,IAAQkX,EAAMlX,EAAMoY,GAC7EC,EAAQ,CAMZ,KALUD,EAAPlB,GAAkBA,EAAOtC,EAAZwD,IACdC,EAAO,GACPnB,GAAQtC,EAAQ,EAChBwD,GAAQxD,EAAQ,GAEZA,IAAU,GACXsC,IAAQja,GAAEA,EAAEmb,GAAMnb,EAAEia,SACXja,GAAEmb,GACdA,GAAQC,EACRnB,GAAQmB,CACR,OAAOpb,KAKN,SAAShD,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQwC,EAAG,SAAUob,KAAMze,EAAoB,OAEvDA,EAAoB,KAAK,SAIpB,SAASI,EAAQD,EAASH,GAI/B,GAAIyB,GAAWzB,EAAoB,IAC/B4B,EAAW5B,EAAoB,IAC/B6B,EAAW7B,EAAoB,GACnCI,GAAOD,WAAase,MAAQ,QAASA,MAAKhb,GAQxC,IAPA,GAAIL,GAAS3B,EAASiF,MAClB5C,EAASjC,EAASuB,EAAEU,QACpBwN,EAAS1K,UACT4L,EAASlB,EAAGxN,OACZ8D,EAAShG,EAAQ4Q,EAAQ,EAAIlB,EAAG,GAAKxR,EAAWgE,GAChDiD,EAASyL,EAAQ,EAAIlB,EAAG,GAAKxR,EAC7B4e,EAAS3X,IAAQjH,EAAYgE,EAASlC,EAAQmF,EAAKjD,GACjD4a,EAAS9W,GAAMxE,EAAEwE,KAAWnE,CAClC,OAAOL,KAKJ,SAAShD,EAAQD,EAASH,GAI/B,GAAIa,GAAUb,EAAoB,GAC9B2e,EAAU3e,EAAoB,IAAI,GAClCsT,EAAU,OACVsL,GAAU,CAEXtL,SAAUhR,MAAM,GAAGgR,GAAK,WAAYsL,GAAS,IAChD/d,EAAQA,EAAQwC,EAAIxC,EAAQoD,EAAI2a,EAAQ,SACtCC,KAAM,QAASA,MAAKnX,GAClB,MAAOiX,GAAMjY,KAAMgB,EAAYd,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAGzEE,EAAoB,KAAKsT,IAIpB,SAASlT,EAAQD,EAASH,GAI/B,GAAIa,GAAUb,EAAoB,GAC9B2e,EAAU3e,EAAoB,IAAI,GAClCsT,EAAU,YACVsL,GAAU,CAEXtL,SAAUhR,MAAM,GAAGgR,GAAK,WAAYsL,GAAS,IAChD/d,EAAQA,EAAQwC,EAAIxC,EAAQoD,EAAI2a,EAAQ,SACtCE,UAAW,QAASA,WAAUpX,GAC5B,MAAOiX,GAAMjY,KAAMgB,EAAYd,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAGzEE,EAAoB,KAAKsT,IAIpB,SAASlT,EAAQD,EAASH,GAE/B,GAAIY,GAAWZ,EAAoB,GAC/BqK,EAAWrK,EAAoB,GAC/Bya,EAAWza,EAAoB,KAC/B+e,EAAW/e,EAAoB,KAC/Bgf,EAAW3U,EAAO4L,OAClBnB,EAAWkK,EACXnM,EAAWmM,EAAQ5c,UACnB6c,EAAW,KACXC,EAAW,KAEXC,EAAc,GAAIH,GAAQC,KAASA,GAEpCjf,EAAoB,IAAQmf,IAAenf,EAAoB,GAAG,WAGnE,MAFAkf,GAAIlf,EAAoB,IAAI,WAAY,EAEjCgf,EAAQC,IAAQA,GAAOD,EAAQE,IAAQA,GAA4B,QAArBF,EAAQC,EAAK,SAElED,EAAU,QAAS/I,QAAOvV,EAAG2N,GAC3B,GAAI+Q,GAAO3E,EAAS/Z,GAChB2e,EAAOhR,IAAMvO,CACjB,OAAS4G,gBAAgBsY,KAAYI,GAAQ1e,EAAEoF,cAAgBkZ,IAAWK,EACtEF,EACE,GAAIrK,GAAKsK,IAASC,EAAM3e,EAAEmK,OAASnK,EAAG2N,GACtCyG,GAAMsK,EAAO1e,YAAase,IAAWte,EAAEmK,OAASnK,EAAG0e,GAAQC,EAAMN,EAAOxe,KAAKG,GAAK2N,GAHR3N,GAKlFE,EAAEqH,KAAK1H,KAAKK,EAAEoF,SAAS8O,GAAO,SAAStP,GACrCA,IAAOwZ,IAAWpe,EAAEgC,QAAQoc,EAASxZ,GACnCwG,cAAc,EACd9I,IAAK,WAAY,MAAO4R,GAAKtP,IAC7BgL,IAAK,SAAShE,GAAKsI,EAAKtP,GAAOgH,OAGnCqG,EAAM/M,YAAckZ,EACpBA,EAAQ5c,UAAYyQ,EACpB7S,EAAoB,IAAIqK,EAAQ,SAAU2U,IAG5Chf,EAAoB,KAAK,WAIpB,SAASI,EAAQD,EAASH,GAI/B,GAAIsB,GAAWtB,EAAoB,GACnCI,GAAOD,QAAU,WACf,GAAIqG,GAASlF,EAASoF,MAClBjB,EAAS,EAMb,OALGe,GAAK6D,SAAY5E,GAAU,KAC3Be,EAAK8Y,aAAY7Z,GAAU,KAC3Be,EAAK+Y,YAAY9Z,GAAU,KAC3Be,EAAKgZ,UAAY/Z,GAAU,KAC3Be,EAAKiZ,SAAYha,GAAU,KACvBA,IAKJ,SAASrF,EAAQD,EAASH,GAG/B,GAAIY,GAAIZ,EAAoB,EACzBA,GAAoB,IAAoB,KAAd,KAAK0f,OAAa9e,EAAEgC,QAAQqT,OAAO7T,UAAW,SACzE4J,cAAc,EACd9I,IAAKlD,EAAoB,QAKtB,SAASI,EAAQD,EAASH,GAG/BA,EAAoB,KAAK,QAAS,EAAG,SAASsN,EAASoN,GAErD,MAAO,SAASpG,OAAMqL,GAEpB,GAAIvc,GAAKkK,EAAQ5G,MACbD,EAAKkZ,GAAU7f,EAAYA,EAAY6f,EAAOjF,EAClD,OAAOjU,KAAO3G,EAAY2G,EAAGlG,KAAKof,EAAQvc,GAAK,GAAI6S,QAAO0J,GAAQjF,GAAO9N,OAAOxJ,QAM/E,SAAShD,EAAQD,EAASH,GAG/B,GAAIuK,GAAWvK,EAAoB,GAC/BwK,EAAWxK,EAAoB,IAC/BqB,EAAWrB,EAAoB,GAC/BsN,EAAWtN,EAAoB,IAC/BkP,EAAWlP,EAAoB,GAEnCI,GAAOD,QAAU,SAASmT,EAAKxP,EAAQoI,GACrC,GAAI0T,GAAW1Q,EAAIoE,GACf/E,EAAW,GAAG+E,EACfjS,GAAM,WACP,GAAI+B,KAEJ,OADAA,GAAEwc,GAAU,WAAY,MAAO,IACV,GAAd,GAAGtM,GAAKlQ,OAEfoH,EAASoC,OAAOxK,UAAWkR,EAAKpH,EAAKoB,EAASsS,EAAQrR,IACtDhE,EAAK0L,OAAO7T,UAAWwd,EAAkB,GAAV9b,EAG3B,SAASsS,EAAQ3H,GAAM,MAAOF,GAAShO,KAAK6V,EAAQ1P,KAAM+H,IAG1D,SAAS2H,GAAS,MAAO7H,GAAShO,KAAK6V,EAAQ1P,WAOlD,SAAStG,EAAQD,EAASH,GAG/BA,EAAoB,KAAK,UAAW,EAAG,SAASsN,EAASuS,EAASC,GAEhE,MAAO,SAASzJ,SAAQ0J,EAAaC,GAEnC,GAAI5c,GAAKkK,EAAQ5G,MACbD,EAAKsZ,GAAejgB,EAAYA,EAAYigB,EAAYF,EAC5D,OAAOpZ,KAAO3G,EACV2G,EAAGlG,KAAKwf,EAAa3c,EAAG4c,GACxBF,EAASvf,KAAKqM,OAAOxJ,GAAI2c,EAAaC,OAMzC,SAAS5f,EAAQD,EAASH,GAG/BA,EAAoB,KAAK,SAAU,EAAG,SAASsN,EAAS2S,GAEtD,MAAO,SAASzF,QAAOmF,GAErB,GAAIvc,GAAKkK,EAAQ5G,MACbD,EAAKkZ,GAAU7f,EAAYA,EAAY6f,EAAOM,EAClD,OAAOxZ,KAAO3G,EAAY2G,EAAGlG,KAAKof,EAAQvc,GAAK,GAAI6S,QAAO0J,GAAQM,GAAQrT,OAAOxJ,QAMhF,SAAShD,EAAQD,EAASH,GAG/BA,EAAoB,KAAK,QAAS,EAAG,SAASsN,EAAS4S,EAAOC,GAE5D,MAAO,SAAS/b,OAAMkD,EAAW8Y,GAE/B,GAAIhd,GAAKkK,EAAQ5G,MACbD,EAAKa,GAAaxH,EAAYA,EAAYwH,EAAU4Y,EACxD,OAAOzZ,KAAO3G,EACV2G,EAAGlG,KAAK+G,EAAWlE,EAAGgd,GACtBD,EAAO5f,KAAKqM,OAAOxJ,GAAIkE,EAAW8Y,OAMrC,SAAShgB,EAAQD,EAASH,GAG/B,GAqBIqgB,GArBAzf,EAAaZ,EAAoB,GACjCyb,EAAazb,EAAoB,IACjCqK,EAAarK,EAAoB,GACjCyK,EAAazK,EAAoB,IACjCiT,EAAajT,EAAoB,IACjCa,EAAab,EAAoB,GACjCwB,EAAaxB,EAAoB,IACjCsB,EAAatB,EAAoB,IACjCuB,EAAavB,EAAoB,IACjCsgB,EAAatgB,EAAoB,KACjCugB,EAAavgB,EAAoB,KACjCwgB,EAAaxgB,EAAoB,IAAIwQ,IACrCiQ,EAAazgB,EAAoB,IACjCsO,EAAatO,EAAoB,IAAI,WACrC0gB,EAAqB1gB,EAAoB,KACzC2gB,EAAa3gB,EAAoB,KACjC4gB,EAAa,UACbC,EAAaxW,EAAOwW,QACpBC,EAAiC,WAApB7N,EAAQ4N,GACrBxd,EAAagH,EAAOuW,GACpBG,EAAa,aAGbC,EAAc,SAASC,GACzB,GAAyBC,GAArBpO,EAAO,GAAIzP,GAAE0d,EAKjB,OAJGE,KAAInO,EAAKhN,YAAc,SAASoG,GACjCA,EAAK6U,EAAOA,MAEbG,EAAU7d,EAAE8d,QAAQrO,IAAO,SAASiO,GAC9BG,IAAYpO,GAGjBsO,EAAa,WAEf,QAASC,IAAG3O,GACV,GAAI9G,GAAO,GAAIvI,GAAEqP,EAEjB,OADA8N,GAAS5U,EAAMyV,GAAGjf,WACXwJ,EAJT,GAAI0V,IAAQ,CAMZ,KASE,GARAA,EAAQje,GAAKA,EAAE8d,SAAWH,IAC1BR,EAASa,GAAIhe;AACbge,GAAGjf,UAAYxB,EAAEqF,OAAO5C,EAAEjB,WAAY0D,aAAcrC,MAAO4d,MAEtDA,GAAGF,QAAQ,GAAGI,KAAK,uBAAyBF,MAC/CC,GAAQ,GAGPA,GAASthB,EAAoB,GAAG,CACjC,GAAIwhB,IAAqB,CACzBne,GAAE8d,QAAQvgB,EAAEgC,WAAY,QACtBM,IAAK,WAAYse,GAAqB,MAExCF,EAAQE,GAEV,MAAMje,GAAI+d,GAAQ,EACpB,MAAOA,MAILG,EAAkB,SAASte,EAAG6J,GAEhC,MAAGyO,IAAWtY,IAAME,GAAK2J,IAAMqT,GAAe,EACvCI,EAAKtd,EAAG6J,IAEb0U,EAAiB,SAASlT,GAC5B,GAAIxK,GAAI1C,EAASkN,GAAGF,EACpB,OAAOtK,IAAKlE,EAAYkE,EAAIwK,GAE1BmT,EAAa,SAASnV,GACxB,GAAI+U,EACJ,OAAO/f,GAASgL,IAAkC,mBAAnB+U,EAAO/U,EAAG+U,MAAsBA,GAAO,GAEpEK,EAAoB,SAASpT,GAC/B,GAAI2S,GAASU,CACbnb,MAAKwa,QAAU,GAAI1S,GAAE,SAASsT,EAAWC,GACvC,GAAGZ,IAAYrhB,GAAa+hB,IAAW/hB,EAAU,KAAM0D,WAAU,0BACjE2d,GAAUW,EACVD,EAAUE,IAEZrb,KAAKya,QAAU5f,EAAU4f,GACzBza,KAAKmb,OAAUtgB,EAAUsgB,IAEvBG,EAAU,SAAS9V,GACrB,IACEA,IACA,MAAM3I,GACN,OAAQ0e,MAAO1e,KAGf2e,EAAS,SAASC,EAAQC,GAC5B,IAAGD,EAAO9b,EAAV,CACA8b,EAAO9b,GAAI,CACX,IAAIgc,GAAQF,EAAO1hB,CACnBkgB,GAAK,WAuBH,IAtBA,GAAIld,GAAQ0e,EAAOG,EACfC,EAAoB,GAAZJ,EAAOzY,EACf3F,EAAQ,EACRye,EAAM,SAASC,GACjB,GAGIhd,GAAQ8b,EAHRmB,EAAUH,EAAKE,EAASF,GAAKE,EAASE,KACtCxB,EAAUsB,EAAStB,QACnBU,EAAUY,EAASZ,MAEvB,KACKa,GACGH,IAAGJ,EAAOS,GAAI,GAClBnd,EAASid,KAAY,EAAOjf,EAAQif,EAAQjf,GACzCgC,IAAWgd,EAASvB,QACrBW,EAAOre,UAAU,yBACT+d,EAAOI,EAAWlc,IAC1B8b,EAAKhhB,KAAKkF,EAAQ0b,EAASU,GACtBV,EAAQ1b,IACVoc,EAAOpe,GACd,MAAMF,GACNse,EAAOte,KAGL8e,EAAMve,OAASC,GAAEye,EAAIH,EAAMte,KACjCse,GAAMve,OAAS,EACfqe,EAAO9b,GAAI,EACR+b,GAASS,WAAW,WACrB,GACIH,GAASI,EADT5B,EAAUiB,EAAOzhB,CAElBqiB,GAAY7B,KACVJ,EACDD,EAAQmC,KAAK,qBAAsBvf,EAAOyd,IAClCwB,EAAUrY,EAAO4Y,sBACzBP,GAASxB,QAASA,EAASgC,OAAQzf,KAC1Bqf,EAAUzY,EAAOyY,UAAYA,EAAQb,OAC9Ca,EAAQb,MAAM,8BAA+Bxe,IAE/C0e,EAAOhf,EAAIrD,GACZ,OAGHijB,EAAc,SAAS7B,GACzB,GAGIuB,GAHAN,EAASjB,EAAQiC,GACjBd,EAASF,EAAOhf,GAAKgf,EAAO1hB,EAC5BsD,EAAS,CAEb,IAAGoe,EAAOS,EAAE,OAAO,CACnB,MAAMP,EAAMve,OAASC,GAEnB,GADA0e,EAAWJ,EAAMte,KACd0e,EAASE,OAASI,EAAYN,EAASvB,SAAS,OAAO,CAC1D,QAAO,GAEPkC,EAAU,SAAS3f,GACrB,GAAI0e,GAASzb,IACVyb,GAAO7Y,IACV6Y,EAAO7Y,GAAI,EACX6Y,EAASA,EAAOkB,GAAKlB,EACrBA,EAAOG,EAAI7e,EACX0e,EAAOzY,EAAI,EACXyY,EAAOhf,EAAIgf,EAAO1hB,EAAE+B,QACpB0f,EAAOC,GAAQ,KAEbmB,EAAW,SAAS7f,GACtB,GACI8d,GADAY,EAASzb,IAEb,KAAGyb,EAAO7Y,EAAV,CACA6Y,EAAO7Y,GAAI,EACX6Y,EAASA,EAAOkB,GAAKlB,CACrB,KACE,GAAGA,EAAOzhB,IAAM+C,EAAM,KAAMD,WAAU,qCACnC+d,EAAOI,EAAWle,IACnBkd,EAAK,WACH,GAAI4C,IAAWF,EAAGlB,EAAQ7Y,GAAG,EAC7B,KACEiY,EAAKhhB,KAAKkD,EAAOgH,EAAI6Y,EAAUC,EAAS,GAAI9Y,EAAI2Y,EAASG,EAAS,IAClE,MAAMhgB,GACN6f,EAAQ7iB,KAAKgjB,EAAShgB,OAI1B4e,EAAOG,EAAI7e,EACX0e,EAAOzY,EAAI,EACXwY,EAAOC,GAAQ,IAEjB,MAAM5e,GACN6f,EAAQ7iB,MAAM8iB,EAAGlB,EAAQ7Y,GAAG,GAAQ/F,KAKpC6d,KAEF/d,EAAI,QAASmgB,SAAQC,GACnBliB,EAAUkiB,EACV,IAAItB,GAASzb,KAAKyc,IAChBziB,EAAG4f,EAAU5Z,KAAMrD,EAAGud,GACtBngB,KACA0C,EAAGrD,EACH4J,EAAG,EACHJ,GAAG,EACHgZ,EAAGxiB,EACH8iB,GAAG,EACHvc,GAAG,EAEL,KACEod,EAAShZ,EAAI6Y,EAAUnB,EAAQ,GAAI1X,EAAI2Y,EAASjB,EAAQ,IACxD,MAAMuB,GACNN,EAAQ7iB,KAAK4hB,EAAQuB,KAGzB1jB,EAAoB,KAAKqD,EAAEjB,WAEzBmf,KAAM,QAASA,MAAKoC,EAAaC,GAC/B,GAAInB,GAAW,GAAIb,GAAkBlB,EAAmBha,KAAMrD,IAC1D6d,EAAWuB,EAASvB,QACpBiB,EAAWzb,KAAKyc,EAMpB,OALAV,GAASF,GAA6B,kBAAfoB,GAA4BA,GAAc,EACjElB,EAASE,KAA4B,kBAAdiB,IAA4BA,EACnDzB,EAAO1hB,EAAEiF,KAAK+c,GACXN,EAAOhf,GAAEgf,EAAOhf,EAAEuC,KAAK+c,GACvBN,EAAOzY,GAAEwY,EAAOC,GAAQ,GACpBjB,GAGT2C,QAAS,SAASD,GAChB,MAAOld,MAAK6a,KAAKzhB,EAAW8jB,OAKlC/iB,EAAQA,EAAQsK,EAAItK,EAAQ6K,EAAI7K,EAAQoD,GAAKmd,GAAaoC,QAASngB,IACnErD,EAAoB,IAAIqD,EAAGud,GAC3B5gB,EAAoB,KAAK4gB,GACzBP,EAAUrgB,EAAoB,GAAG4gB,GAGjC/f,EAAQA,EAAQmD,EAAInD,EAAQoD,GAAKmd,EAAYR,GAE3CiB,OAAQ,QAASA,QAAOwB,GACtB,GAAIS,GAAa,GAAIlC,GAAkBlb,MACnCqb,EAAa+B,EAAWjC,MAE5B,OADAE,GAASsB,GACFS,EAAW5C,WAGtBrgB,EAAQA,EAAQmD,EAAInD,EAAQoD,IAAMmd,GAAcJ,GAAY,IAAQJ,GAElEO,QAAS,QAASA,SAAQzO,GAExB,GAAGA,YAAarP,IAAKoe,EAAgB/O,EAAE5M,YAAaY,MAAM,MAAOgM,EACjE,IAAIoR,GAAa,GAAIlC,GAAkBlb,MACnCob,EAAagC,EAAW3C,OAE5B,OADAW,GAAUpP,GACHoR,EAAW5C,WAGtBrgB,EAAQA,EAAQmD,EAAInD,EAAQoD,IAAMmd,GAAcphB,EAAoB,KAAK,SAASod,GAChF/Z,EAAE0gB,IAAI3G,GAAM,SAAS,iBAClBwD,GAEHmD,IAAK,QAASA,KAAIC,GAChB,GAAIxV,GAAakT,EAAehb,MAC5Bod,EAAa,GAAIlC,GAAkBpT,GACnC2S,EAAa2C,EAAW3C,QACxBU,EAAaiC,EAAWjC,OACxBnF,KACAuH,EAASjC,EAAQ,WACnBzB,EAAMyD,GAAU,EAAOtH,EAAOhX,KAAMgX,EACpC,IAAIwH,GAAYxH,EAAO5Y,OACnBqgB,EAAY7hB,MAAM4hB,EACnBA,GAAUtjB,EAAEqH,KAAK1H,KAAKmc,EAAQ,SAASwE,EAAStZ,GACjD,GAAIwc,IAAgB,CACpB5V,GAAE2S,QAAQD,GAASK,KAAK,SAAS9d,GAC5B2gB,IACHA,GAAgB,EAChBD,EAAQvc,GAASnE,IACfygB,GAAa/C,EAAQgD,KACtBtC,KAEAV,EAAQgD,IAGf,OADGF,IAAOpC,EAAOoC,EAAOhC,OACjB6B,EAAW5C,SAGpBmD,KAAM,QAASA,MAAKL,GAClB,GAAIxV,GAAakT,EAAehb,MAC5Bod,EAAa,GAAIlC,GAAkBpT,GACnCqT,EAAaiC,EAAWjC,OACxBoC,EAASjC,EAAQ,WACnBzB,EAAMyD,GAAU,EAAO,SAAS9C,GAC9B1S,EAAE2S,QAAQD,GAASK,KAAKuC,EAAW3C,QAASU,MAIhD,OADGoC,IAAOpC,EAAOoC,EAAOhC,OACjB6B,EAAW5C,YAMjB,SAAS9gB,EAAQD,GAEtBC,EAAOD,QAAU,SAASqM,EAAI0P,EAAatR,GACzC,KAAK4B,YAAc0P,IAAa,KAAM1Y,WAAUoH,EAAO,4BACvD,OAAO4B,KAKJ,SAASpM,EAAQD,EAASH,GAE/B,GAAIyK,GAAczK,EAAoB,IAClCO,EAAcP,EAAoB,KAClCkd,EAAcld,EAAoB,KAClCsB,EAActB,EAAoB,IAClC6B,EAAc7B,EAAoB,IAClCmd,EAAcnd,EAAoB,IACtCI,GAAOD,QAAU,SAAS6jB,EAAUrH,EAASlW,EAAID,GAC/C,GAGI1C,GAAQyZ,EAAMC,EAHdG,EAASR,EAAU6G,GACnB3V,EAAS5D,EAAIhE,EAAID,EAAMmW,EAAU,EAAI,GACrC/U,EAAS,CAEb,IAAoB,kBAAV+V,GAAqB,KAAMna,WAAUwgB,EAAW,oBAE1D,IAAG9G,EAAYS,GAAQ,IAAI7Z,EAASjC,EAASmiB,EAASlgB,QAASA,EAAS8D,EAAOA,IAC7E+U,EAAUtO,EAAE/M,EAASic,EAAOyG,EAASpc,IAAQ,GAAI2V,EAAK,IAAMlP,EAAE2V,EAASpc,QAClE,KAAI4V,EAAWG,EAAOpd,KAAKyjB,KAAazG,EAAOC,EAASrB,QAAQX,MACrEjb,EAAKid,EAAUnP,EAAGkP,EAAK9Z,MAAOkZ,KAM7B,SAASvc,EAAQD,EAASH,GAG/B,GAAIsB,GAAYtB,EAAoB,IAChCuB,EAAYvB,EAAoB,IAChCsO,EAAYtO,EAAoB,IAAI,UACxCI,GAAOD,QAAU,SAASiD,EAAG8M,GAC3B,GAAiClM,GAA7BwK,EAAIlN,EAAS8B,GAAG0C,WACpB,OAAO0I,KAAM1O,IAAckE,EAAI1C,EAASkN,GAAGF,KAAaxO,EAAYoQ,EAAI3O,EAAUyC,KAK/E,SAAS5D,EAAQD,EAASH,GAE/B,GAMIskB,GAAMC,EAAMrC,EANZ7X,EAAYrK,EAAoB,GAChCwkB,EAAYxkB,EAAoB,KAAKwQ,IACrCiU,EAAYpa,EAAOqa,kBAAoBra,EAAOsa,uBAC9C9D,EAAYxW,EAAOwW,QACnB2C,EAAYnZ,EAAOmZ,QACnB1C,EAAgD,WAApC9gB,EAAoB,IAAI6gB,GAGpC+D,EAAQ,WACV,GAAIC,GAAQC,EAAQre,CAKpB,KAJGqa,IAAW+D,EAAShE,EAAQiE,UAC7BjE,EAAQiE,OAAS,KACjBD,EAAOE,QAEHT,GACJQ,EAASR,EAAKQ,OACdre,EAAS6d,EAAK7d,GACXqe,GAAOA,EAAOE,QACjBve,IACGqe,GAAOA,EAAOC,OACjBT,EAAOA,EAAKnI,IACZoI,GAAOzkB,EACN+kB,GAAOA,EAAOG,QAInB,IAAGlE,EACDoB,EAAS,WACPrB,EAAQoE,SAASL,QAGd,IAAGH,EAAS,CACjB,GAAIS,GAAS,EACTC,EAASlgB,SAASmgB,eAAe,GACrC,IAAIX,GAASG,GAAOS,QAAQF,GAAOG,eAAe,IAClDpD,EAAS,WACPiD,EAAKI,KAAOL,GAAUA,OAIxBhD,GADQsB,GAAWA,EAAQrC,QAClB,WACPqC,EAAQrC,UAAUI,KAAKqD,IAShB,WAEPJ,EAAUjkB,KAAK8J,EAAQua,GAI3BxkB,GAAOD,QAAU,QAASwgB,MAAKla,GAC7B,GAAI+e,IAAQ/e,GAAIA,EAAI0V,KAAMrc,EAAWglB,OAAQhE,GAAUD,EAAQiE,OAC5DP,KAAKA,EAAKpI,KAAOqJ,GAChBlB,IACFA,EAAOkB,EACPtD,KACAqC,EAAOiB,IAKN,SAASplB,EAAQD,EAASH,GAE/B,GAYIylB,GAAOC,EAASC,EAZhBlb,EAAqBzK,EAAoB,IACzCoB,EAAqBpB,EAAoB,IACzCgB,EAAqBhB,EAAoB,IACzCiB,EAAqBjB,EAAoB,IACzCqK,EAAqBrK,EAAoB,GACzC6gB,EAAqBxW,EAAOwW,QAC5B+E,EAAqBvb,EAAOwb,aAC5BC,EAAqBzb,EAAO0b,eAC5BC,EAAqB3b,EAAO2b,eAC5BC,EAAqB,EACrBC,KACAC,EAAqB,qBAErB3D,EAAM,WACR,GAAIniB,IAAMqG,IACV,IAAGwf,EAAMvZ,eAAetM,GAAI,CAC1B,GAAIoG,GAAKyf,EAAM7lB,SACR6lB,GAAM7lB,GACboG,MAGA2f,EAAU,SAASC,GACrB7D,EAAIjiB,KAAK8lB,EAAMd,MAGbK,IAAYE,IACdF,EAAU,QAASC,cAAapf,GAE9B,IADA,GAAIL,MAAWrC,EAAI,EACb6C,UAAU9C,OAASC,GAAEqC,EAAKV,KAAKkB,UAAU7C,KAK/C,OAJAmiB,KAAQD,GAAW,WACjB7kB,EAAoB,kBAANqF,GAAmBA,EAAKH,SAASG,GAAKL,IAEtDqf,EAAMQ,GACCA,GAETH,EAAY,QAASC,gBAAe1lB,SAC3B6lB,GAAM7lB,IAGwB,WAApCL,EAAoB,IAAI6gB,GACzB4E,EAAQ,SAASplB,GACfwgB,EAAQoE,SAASxa,EAAI+X,EAAKniB,EAAI,KAGxB2lB,GACRN,EAAU,GAAIM,GACdL,EAAUD,EAAQY,MAClBZ,EAAQa,MAAMC,UAAYJ,EAC1BX,EAAQhb,EAAIkb,EAAKc,YAAad,EAAM,IAG5Btb,EAAOqc,kBAA0C,kBAAfD,eAA8Bpc,EAAOsc,eAC/ElB,EAAQ,SAASplB,GACfgK,EAAOoc,YAAYpmB,EAAK,GAAI,MAE9BgK,EAAOqc,iBAAiB,UAAWN,GAAS,IAG5CX,EADQU,IAAsBllB,GAAI,UAC1B,SAASZ,GACfW,EAAK8D,YAAY7D,EAAI,WAAWklB,GAAsB,WACpDnlB,EAAK4lB,YAAYlgB,MACjB8b,EAAIjiB,KAAKF,KAKL,SAASA,GACfwiB,WAAWpY,EAAI+X,EAAKniB,EAAI,GAAI,KAIlCD,EAAOD,SACLqQ,IAAOoV,EACPiB,MAAOf,IAKJ,SAAS1lB,EAAQD,EAASH,GAE/B,GAAIwK,GAAWxK,EAAoB,GACnCI,GAAOD,QAAU,SAASqL,EAAQzG,GAChC,IAAI,GAAIS,KAAOT,GAAIyF,EAASgB,EAAQhG,EAAKT,EAAIS,GAC7C,OAAOgG,KAKJ,SAASpL,EAAQD,EAASH,GAG/B,GAAI8mB,GAAS9mB,EAAoB,IAGjCA,GAAoB,KAAK,MAAO,SAASkD,GACvC,MAAO,SAAS6jB,OAAO,MAAO7jB,GAAIwD,KAAME,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAG9EoD,IAAK,QAASA,KAAIsC,GAChB,GAAIwhB,GAAQF,EAAOG,SAASvgB,KAAMlB,EAClC,OAAOwhB,IAASA,EAAM1E,GAGxB9R,IAAK,QAASA,KAAIhL,EAAK/B,GACrB,MAAOqjB,GAAOjV,IAAInL,KAAc,IAARlB,EAAY,EAAIA,EAAK/B,KAE9CqjB,GAAQ,IAIN,SAAS1mB,EAAQD,EAASH,GAG/B,GAAIY,GAAeZ,EAAoB,GACnCuK,EAAevK,EAAoB,GACnCknB,EAAelnB,EAAoB,KACnCyK,EAAezK,EAAoB,IACnCsgB,EAAetgB,EAAoB,KACnCsN,EAAetN,EAAoB,IACnCugB,EAAevgB,EAAoB,KACnCmnB,EAAennB,EAAoB,KACnCud,EAAevd,EAAoB,KACnConB,EAAepnB,EAAoB,IAAI,MACvCqnB,EAAernB,EAAoB,IACnCwB,EAAexB,EAAoB,IACnCsnB,EAAetnB,EAAoB,KACnCc,EAAed,EAAoB,GACnCgU,EAAe7R,OAAO6R,cAAgBxS,EACtC+lB,EAAezmB,EAAc,KAAO,OACpCT,EAAe,EAEfmnB,EAAU,SAAShb,EAAIvG,GAEzB,IAAIzE,EAASgL,GAAI,MAAoB,gBAANA,GAAiBA,GAAmB,gBAANA,GAAiB,IAAM,KAAOA,CAC3F,KAAI6a,EAAK7a,EAAI4a,GAAI,CAEf,IAAIpT,EAAaxH,GAAI,MAAO,GAE5B,KAAIvG,EAAO,MAAO,GAElBsE,GAAKiC,EAAI4a,IAAM/mB,GAEf,MAAO,IAAMmM,EAAG4a,IAGhBH,EAAW,SAASzgB,EAAMhB,GAE5B,GAA0BwhB,GAAtBpf,EAAQ4f,EAAQhiB,EACpB,IAAa,MAAVoC,EAAc,MAAOpB,GAAK8U,GAAG1T,EAEhC,KAAIof,EAAQxgB,EAAKihB,GAAIT,EAAOA,EAAQA,EAAM3gB,EACxC,GAAG2gB,EAAM1U,GAAK9M,EAAI,MAAOwhB,GAI7B5mB,GAAOD,SACLuhB,eAAgB,SAAS6B,EAASlP,EAAMxG,EAAQ6Z,GAC9C,GAAIlZ,GAAI+U,EAAQ,SAAS/c,EAAMwd,GAC7B1D,EAAU9Z,EAAMgI,EAAG6F,GACnB7N,EAAK8U,GAAK1a,EAAEqF,OAAO,MACnBO,EAAKihB,GAAK3nB,EACV0G,EAAKmhB,GAAK7nB,EACV0G,EAAK+gB,GAAQ,EACVvD,GAAYlkB,GAAUygB,EAAMyD,EAAUnW,EAAQrH,EAAKkhB,GAAQlhB,IAqDhE,OAnDA0gB,GAAY1Y,EAAEpM,WAGZykB,MAAO,QAASA,SACd,IAAI,GAAIrgB,GAAOE,KAAM6e,EAAO/e,EAAK8U,GAAI0L,EAAQxgB,EAAKihB,GAAIT,EAAOA,EAAQA,EAAM3gB,EACzE2gB,EAAM3D,GAAI,EACP2D,EAAMtmB,IAAEsmB,EAAMtmB,EAAIsmB,EAAMtmB,EAAE2F,EAAIvG,SAC1BylB,GAAKyB,EAAMjjB,EAEpByC,GAAKihB,GAAKjhB,EAAKmhB,GAAK7nB,EACpB0G,EAAK+gB,GAAQ,GAIfK,SAAU,SAASpiB,GACjB,GAAIgB,GAAQE,KACRsgB,EAAQC,EAASzgB,EAAMhB,EAC3B,IAAGwhB,EAAM,CACP,GAAI7K,GAAO6K,EAAM3gB,EACbwhB,EAAOb,EAAMtmB,QACV8F,GAAK8U,GAAG0L,EAAMjjB,GACrBijB,EAAM3D,GAAI,EACPwE,IAAKA,EAAKxhB,EAAI8V,GACdA,IAAKA,EAAKzb,EAAImnB,GACdrhB,EAAKihB,IAAMT,IAAMxgB,EAAKihB,GAAKtL,GAC3B3V,EAAKmhB,IAAMX,IAAMxgB,EAAKmhB,GAAKE,GAC9BrhB,EAAK+gB,KACL,QAASP,GAIbhf,QAAS,QAASA,SAAQN,GAGxB,IAFA,GACIsf,GADA3Y,EAAI5D,EAAI/C,EAAYd,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,EAAW,GAEnEknB,EAAQA,EAAQA,EAAM3gB,EAAIK,KAAK+gB,IAGnC,IAFApZ,EAAE2Y,EAAM1E,EAAG0E,EAAM1U,EAAG5L,MAEdsgB,GAASA,EAAM3D,GAAE2D,EAAQA,EAAMtmB,GAKzCQ,IAAK,QAASA,KAAIsE,GAChB,QAASyhB,EAASvgB,KAAMlB,MAGzB1E,GAAYF,EAAEgC,QAAQ4L,EAAEpM,UAAW,QACpCc,IAAK,WACH,MAAOoK,GAAQ5G,KAAK6gB,OAGjB/Y,GAETqD,IAAK,SAASrL,EAAMhB,EAAK/B,GACvB,GACIokB,GAAMjgB,EADNof,EAAQC,EAASzgB,EAAMhB,EAoBzB,OAjBCwhB,GACDA,EAAM1E,EAAI7e,GAGV+C,EAAKmhB,GAAKX,GACRjjB,EAAG6D,EAAQ4f,EAAQhiB,GAAK,GACxB8M,EAAG9M,EACH8c,EAAG7e,EACH/C,EAAGmnB,EAAOrhB,EAAKmhB,GACfthB,EAAGvG,EACHujB,GAAG,GAED7c,EAAKihB,KAAGjhB,EAAKihB,GAAKT,GACnBa,IAAKA,EAAKxhB,EAAI2gB,GACjBxgB,EAAK+gB,KAEQ,MAAV3f,IAAcpB,EAAK8U,GAAG1T,GAASof,IAC3BxgB,GAEXygB,SAAUA,EACVa,UAAW,SAAStZ,EAAG6F,EAAMxG,GAG3BsZ,EAAY3Y,EAAG6F,EAAM,SAAS+G,EAAUqB,GACtC/V,KAAK2U,GAAKD,EACV1U,KAAK6J,GAAKkM,EACV/V,KAAKihB,GAAK7nB,GACT,WAKD,IAJA,GAAI0G,GAAQE,KACR+V,EAAQjW,EAAK+J,GACbyW,EAAQxgB,EAAKmhB,GAEXX,GAASA,EAAM3D,GAAE2D,EAAQA,EAAMtmB,CAErC,OAAI8F,GAAK6U,KAAQ7U,EAAKmhB,GAAKX,EAAQA,EAAQA,EAAM3gB,EAAIG,EAAK6U,GAAGoM,IAMlD,QAARhL,EAAwBc,EAAK,EAAGyJ,EAAM1U,GAC9B,UAARmK,EAAwBc,EAAK,EAAGyJ,EAAM1E,GAClC/E,EAAK,GAAIyJ,EAAM1U,EAAG0U,EAAM1E,KAN7B9b,EAAK6U,GAAKvb,EACHyd,EAAK,KAMb1P,EAAS,UAAY,UAAYA,GAAQ,GAG5CyZ,EAAWjT,MAMV,SAASjU,EAAQD,EAASH,GAG/B,GAAIqK,GAAiBrK,EAAoB,GACrCa,EAAiBb,EAAoB,GACrCwK,EAAiBxK,EAAoB,IACrCknB,EAAiBlnB,EAAoB,KACrCugB,EAAiBvgB,EAAoB,KACrCsgB,EAAiBtgB,EAAoB,KACrCwB,EAAiBxB,EAAoB,IACrCqB,EAAiBrB,EAAoB,GACrC+nB,EAAiB/nB,EAAoB,KACrCiP,EAAiBjP,EAAoB,GAEzCI,GAAOD,QAAU,SAASkU,EAAMkP,EAAShH,EAASyL,EAAQna,EAAQoa,GAChE,GAAInT,GAAQzK,EAAOgK,GACf7F,EAAQsG,EACR4S,EAAQ7Z,EAAS,MAAQ,MACzBgF,EAAQrE,GAAKA,EAAEpM,UACfgB,KACA8kB,EAAY,SAAS5U,GACvB,GAAI7M,GAAKoM,EAAMS,EACf9I,GAASqI,EAAOS,EACP,UAAPA,EAAkB,SAASnQ,GACzB,MAAO8kB,KAAYzmB,EAAS2B,IAAK,EAAQsD,EAAGlG,KAAKmG,KAAY,IAANvD,EAAU,EAAIA,IAC5D,OAAPmQ,EAAe,QAASpS,KAAIiC,GAC9B,MAAO8kB,KAAYzmB,EAAS2B,IAAK,EAAQsD,EAAGlG,KAAKmG,KAAY,IAANvD,EAAU,EAAIA,IAC5D,OAAPmQ,EAAe,QAASpQ,KAAIC,GAC9B,MAAO8kB,KAAYzmB,EAAS2B,GAAKrD,EAAY2G,EAAGlG,KAAKmG,KAAY,IAANvD,EAAU,EAAIA,IAChE,OAAPmQ,EAAe,QAAS6U,KAAIhlB,GAAoC,MAAhCsD,GAAGlG,KAAKmG,KAAY,IAANvD,EAAU,EAAIA,GAAWuD,MACvE,QAAS8J,KAAIrN,EAAG6J,GAAuC,MAAnCvG,GAAGlG,KAAKmG,KAAY,IAANvD,EAAU,EAAIA,EAAG6J,GAAWtG,OAGtE,IAAe,kBAAL8H,KAAqByZ,GAAWpV,EAAM7K,UAAY3G,EAAM,YAChE,GAAImN,IAAImO,UAAUR,UAKb,CACL,GAQIiM,GARAC,EAAuB,GAAI7Z,GAE3B8Z,EAAuBD,EAASX,GAAOO,MAAgB,EAAG,IAAMI,EAEhEE,EAAuBlnB,EAAM,WAAYgnB,EAASnnB,IAAI,KAEtDsnB,EAAuBT,EAAY,SAAS3K,GAAO,GAAI5O,GAAE4O,IAGzDoL,KACFha,EAAI+U,EAAQ,SAAS/X,EAAQwY,GAC3B1D,EAAU9U,EAAQgD,EAAG6F,EACrB,IAAI7N,GAAO,GAAIsO,EAEf,OADGkP,IAAYlkB,GAAUygB,EAAMyD,EAAUnW,EAAQrH,EAAKkhB,GAAQlhB,GACvDA,IAETgI,EAAEpM,UAAYyQ,EACdA,EAAM/M,YAAc0I,GAEtByZ,GAAWI,EAASrgB,QAAQ,SAASyE,EAAKjH,GACxC4iB,EAAa,EAAI5iB,MAAS6S,EAAAA,MAEzBkQ,GAAwBH,KACzBF,EAAU,UACVA,EAAU,OACVra,GAAUqa,EAAU,SAEnBE,GAAcE,IAAeJ,EAAUR,GAEvCO,GAAWpV,EAAMgU,aAAahU,GAAMgU,UAhCvCrY,GAAIwZ,EAAOtG,eAAe6B,EAASlP,EAAMxG,EAAQ6Z,GACjDR,EAAY1Y,EAAEpM,UAAWma,EAyC3B,OAPAtN,GAAeT,EAAG6F,GAElBjR,EAAEiR,GAAQ7F,EACV3N,EAAQA,EAAQsK,EAAItK,EAAQ6K,EAAI7K,EAAQoD,GAAKuK,GAAKsG,GAAO1R,GAErD6kB,GAAQD,EAAOF,UAAUtZ,EAAG6F,EAAMxG,GAE/BW,IAKJ,SAASpO,EAAQD,EAASH,GAG/B,GAAI8mB,GAAS9mB,EAAoB,IAGjCA,GAAoB,KAAK,MAAO,SAASkD,GACvC,MAAO,SAASulB,OAAO,MAAOvlB,GAAIwD,KAAME,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAG9EqoB,IAAK,QAASA,KAAI1kB,GAChB,MAAOqjB,GAAOjV,IAAInL,KAAMjD,EAAkB,IAAVA,EAAc,EAAIA,EAAOA,KAE1DqjB,IAIE,SAAS1mB,EAAQD,EAASH,GAG/B,GAAIY,GAAeZ,EAAoB,GACnCwK,EAAexK,EAAoB,IACnC0oB,EAAe1oB,EAAoB,KACnCwB,EAAexB,EAAoB,IACnCkB,EAAelB,EAAoB,IACnC2oB,EAAeD,EAAKC,YACpBC,EAAeF,EAAKE,KACpB5U,EAAe7R,OAAO6R,cAAgBxS,EACtCqnB,KAGAC,EAAW9oB,EAAoB,KAAK,UAAW,SAASkD,GAC1D,MAAO,SAAS6lB,WAAW,MAAO7lB,GAAIwD,KAAME,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAGlFoD,IAAK,QAASA,KAAIsC,GAChB,GAAGhE,EAASgE,GAAK,CACf,IAAIwO,EAAaxO,GAAK,MAAOmjB,GAAYjiB,MAAMxD,IAAIsC,EACnD,IAAGtE,EAAIsE,EAAKojB,GAAM,MAAOpjB,GAAIojB,GAAMliB,KAAK4U,MAI5C9K,IAAK,QAASA,KAAIhL,EAAK/B,GACrB,MAAOilB,GAAK7W,IAAInL,KAAMlB,EAAK/B,KAE5BilB,GAAM,GAAM,EAGsD,KAAlE,GAAII,IAAWtY,KAAKrO,OAAOkR,QAAUlR,QAAQ0mB,GAAM,GAAG3lB,IAAI2lB,IAC3DjoB,EAAEqH,KAAK1H,MAAM,SAAU,MAAO,MAAO,OAAQ,SAASiF,GACpD,GAAIqN,GAASiW,EAAS1mB,UAClB4mB,EAASnW,EAAMrN,EACnBgF,GAASqI,EAAOrN,EAAK,SAASrC,EAAG6J,GAE/B,GAAGxL,EAAS2B,KAAO6Q,EAAa7Q,GAAG,CACjC,GAAIsC,GAASkjB,EAAYjiB,MAAMlB,GAAKrC,EAAG6J,EACvC,OAAc,OAAPxH,EAAekB,KAAOjB,EAE7B,MAAOujB,GAAOzoB,KAAKmG,KAAMvD,EAAG6J,QAO/B,SAAS5M,EAAQD,EAASH,GAG/B,GAAIuK,GAAoBvK,EAAoB,GACxCknB,EAAoBlnB,EAAoB,KACxCsB,EAAoBtB,EAAoB,IACxCwB,EAAoBxB,EAAoB,IACxCsgB,EAAoBtgB,EAAoB,KACxCugB,EAAoBvgB,EAAoB,KACxCgC,EAAoBhC,EAAoB,IACxCqnB,EAAoBrnB,EAAoB,IACxC4oB,EAAoB5oB,EAAoB,IAAI,QAC5CgU,EAAoB7R,OAAO6R,cAAgBxS,EAC3CynB,EAAoBjnB,EAAkB,GACtCknB,EAAoBlnB,EAAkB,GACtC3B,EAAoB,EAGpBsoB,EAAc,SAASniB,GACzB,MAAOA,GAAKmhB,KAAOnhB,EAAKmhB,GAAK,GAAIwB,KAE/BA,EAAc,WAChBziB,KAAKvD,MAEHimB,EAAa,SAAS1a,EAAOlJ,GAC/B,MAAOyjB,GAAUva,EAAMvL,EAAG,SAASqJ,GACjC,MAAOA,GAAG,KAAOhH,IAGrB2jB,GAAY/mB,WACVc,IAAK,SAASsC,GACZ,GAAIwhB,GAAQoC,EAAW1iB,KAAMlB,EAC7B,OAAGwhB,GAAaA,EAAM,GAAtB,QAEF9lB,IAAK,SAASsE,GACZ,QAAS4jB,EAAW1iB,KAAMlB,IAE5BgL,IAAK,SAAShL,EAAK/B,GACjB,GAAIujB,GAAQoC,EAAW1iB,KAAMlB,EAC1BwhB,GAAMA,EAAM,GAAKvjB,EACfiD,KAAKvD,EAAEuC,MAAMF,EAAK/B,KAEzBmkB,SAAU,SAASpiB,GACjB,GAAIoC,GAAQshB,EAAexiB,KAAKvD,EAAG,SAASqJ,GAC1C,MAAOA,GAAG,KAAOhH,GAGnB,QADIoC,GAAMlB,KAAKvD,EAAEkmB,OAAOzhB,EAAO,MACrBA,IAIdxH,EAAOD,SACLuhB,eAAgB,SAAS6B,EAASlP,EAAMxG,EAAQ6Z,GAC9C,GAAIlZ,GAAI+U,EAAQ,SAAS/c,EAAMwd,GAC7B1D,EAAU9Z,EAAMgI,EAAG6F,GACnB7N,EAAK8U,GAAKjb,IACVmG,EAAKmhB,GAAK7nB,EACPkkB,GAAYlkB,GAAUygB,EAAMyD,EAAUnW,EAAQrH,EAAKkhB,GAAQlhB,IAkBhE,OAhBA0gB,GAAY1Y,EAAEpM,WAGZwlB,SAAU,SAASpiB,GACjB,MAAIhE,GAASgE,GACTwO,EAAaxO,GACV6hB,EAAK7hB,EAAKojB,IAASvB,EAAK7hB,EAAIojB,GAAOliB,KAAK4U,WAAc9V,GAAIojB,GAAMliB,KAAK4U,IAD/CqN,EAAYjiB,MAAM,UAAUlB,IADhC,GAM3BtE,IAAK,QAASA,KAAIsE,GAChB,MAAIhE,GAASgE,GACTwO,EAAaxO,GACV6hB,EAAK7hB,EAAKojB,IAASvB,EAAK7hB,EAAIojB,GAAOliB,KAAK4U,IADlBqN,EAAYjiB,MAAMxF,IAAIsE,IAD1B,KAKtBgJ,GAETqD,IAAK,SAASrL,EAAMhB,EAAK/B,GAMrB,MALEuQ,GAAa1S,EAASkE,KAGxB6hB,EAAK7hB,EAAKojB,IAASre,EAAK/E,EAAKojB,MAC7BpjB,EAAIojB,GAAMpiB,EAAK8U,IAAM7X,GAHrBklB,EAAYniB,GAAMgK,IAAIhL,EAAK/B,GAIpB+C,GAEXmiB,YAAaA,EACbC,KAAMA,IAKH,SAASxoB,EAAQD,EAASH,GAG/B,GAAI0oB,GAAO1oB,EAAoB,IAG/BA,GAAoB,KAAK,UAAW,SAASkD,GAC3C,MAAO,SAASomB,WAAW,MAAOpmB,GAAIwD,KAAME,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAGlFqoB,IAAK,QAASA,KAAI1kB,GAChB,MAAOilB,GAAK7W,IAAInL,KAAMjD,GAAO,KAE9BilB,GAAM,GAAO,IAIX,SAAStoB,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,GAC/BupB,EAAWjjB,SAAS2G,MACpB3L,EAAWtB,EAAoB,GAEnCa,GAAQA,EAAQmD,EAAG,WACjBiJ,MAAO,QAASA,OAAMzB,EAAQge,EAAcC,GAC1C,MAAOF,GAAOhpB,KAAKiL,EAAQge,EAAcloB,EAASmoB,QAMjD,SAASrpB,EAAQD,EAASH,GAG/B,GAAIY,GAAYZ,EAAoB,GAChCa,EAAYb,EAAoB,GAChCuB,EAAYvB,EAAoB,IAChCsB,EAAYtB,EAAoB,IAChCwB,EAAYxB,EAAoB,IAChCuG,EAAYD,SAASC,MAAQvG,EAAoB,GAAGsG,SAASlE,UAAUmE,IAI3E1F,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAIjE,EAAoB,GAAG,WACrD,QAASiE,MACT,QAASylB,QAAQxjB,UAAU,gBAAkBjC,YAAcA,MACzD,WACFiC,UAAW,QAASA,WAAUyjB,EAAQvjB,GACpC7E,EAAUooB,GACVroB,EAAS8E,EACT,IAAIwjB,GAAYhjB,UAAU9C,OAAS,EAAI6lB,EAASpoB,EAAUqF,UAAU,GACpE,IAAG+iB,GAAUC,EAAU,CAErB,OAAOxjB,EAAKtC,QACV,IAAK,GAAG,MAAO,IAAI6lB,EACnB,KAAK,GAAG,MAAO,IAAIA,GAAOvjB,EAAK,GAC/B,KAAK,GAAG,MAAO,IAAIujB,GAAOvjB,EAAK,GAAIA,EAAK,GACxC,KAAK,GAAG,MAAO,IAAIujB,GAAOvjB,EAAK,GAAIA,EAAK,GAAIA,EAAK,GACjD,KAAK,GAAG,MAAO,IAAIujB,GAAOvjB,EAAK,GAAIA,EAAK,GAAIA,EAAK,GAAIA,EAAK,IAG5D,GAAIyjB,IAAS,KAEb,OADAA,GAAMnkB,KAAKuH,MAAM4c,EAAOzjB,GACjB,IAAKG,EAAK0G,MAAM0c,EAAQE,IAGjC,GAAIhX,GAAW+W,EAAUxnB,UACrBimB,EAAWznB,EAAEqF,OAAOzE,EAASqR,GAASA,EAAQ1Q,OAAOC,WACrDqD,EAAWa,SAAS2G,MAAM1M,KAAKopB,EAAQtB,EAAUjiB,EACrD,OAAO5E,GAASiE,GAAUA,EAAS4iB,MAMlC,SAASjoB,EAAQD,EAASH,GAG/B,GAAIY,GAAWZ,EAAoB,GAC/Ba,EAAWb,EAAoB,GAC/BsB,EAAWtB,EAAoB,GAGnCa,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAIjE,EAAoB,GAAG,WACrD0pB,QAAQ/mB,eAAe/B,EAAEgC,WAAY,GAAIa,MAAO,IAAK,GAAIA,MAAO,MAC9D,WACFd,eAAgB,QAASA,gBAAe6I,EAAQse,EAAaC,GAC3DzoB,EAASkK,EACT,KAEE,MADA5K,GAAEgC,QAAQ4I,EAAQse,EAAaC,IACxB,EACP,MAAMxmB,GACN,OAAO,OAOR,SAASnD,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,GAC/B8C,EAAW9C,EAAoB,GAAG8C,QAClCxB,EAAWtB,EAAoB,GAEnCa,GAAQA,EAAQmD,EAAG,WACjBgmB,eAAgB,QAASA,gBAAexe,EAAQse,GAC9C,GAAIG,GAAOnnB,EAAQxB,EAASkK,GAASse,EACrC,OAAOG,KAASA,EAAKje,cAAe,QAAeR,GAAOse,OAMzD,SAAS1pB,EAAQD,EAASH,GAI/B,GAAIa,GAAWb,EAAoB,GAC/BsB,EAAWtB,EAAoB,IAC/BkqB,EAAY,SAAS9O,GACvB1U,KAAK2U,GAAK/Z,EAAS8Z,GACnB1U,KAAK4U,GAAK,CACV,IACI9V,GADA5B,EAAO8C,KAAK6J,KAEhB,KAAI/K,IAAO4V,GAASxX,EAAK8B,KAAKF,GAEhCxF,GAAoB,KAAKkqB,EAAW,SAAU,WAC5C,GAEI1kB,GAFAgB,EAAOE,KACP9C,EAAO4C,EAAK+J,EAEhB,GACE,IAAG/J,EAAK8U,IAAM1X,EAAKE,OAAO,OAAQL,MAAO3D,EAAW0b,MAAM,YACjDhW,EAAM5B,EAAK4C,EAAK8U,QAAU9U,GAAK6U,IAC1C,QAAQ5X,MAAO+B,EAAKgW,MAAM,KAG5B3a,EAAQA,EAAQmD,EAAG,WACjBmmB,UAAW,QAASA,WAAU3e,GAC5B,MAAO,IAAI0e,GAAU1e,OAMpB,SAASpL,EAAQD,EAASH,GAS/B,QAASkD,KAAIsI,EAAQse,GACnB,GACIG,GAAMpX,EADNuX,EAAWxjB,UAAU9C,OAAS,EAAI0H,EAAS5E,UAAU,EAEzD,OAAGtF,GAASkK,KAAY4e,EAAgB5e,EAAOse,IAC5CG,EAAOrpB,EAAEkC,QAAQ0I,EAAQse,IAAoB5oB,EAAI+oB,EAAM,SACtDA,EAAKxmB,MACLwmB,EAAK/mB,MAAQpD,EACXmqB,EAAK/mB,IAAI3C,KAAK6pB,GACdtqB,EACH0B,EAASqR,EAAQjS,EAAEiF,SAAS2F,IAAgBtI,IAAI2P,EAAOiX,EAAaM,GAAvE,OAfF,GAAIxpB,GAAWZ,EAAoB,GAC/BkB,EAAWlB,EAAoB,IAC/Ba,EAAWb,EAAoB,GAC/BwB,EAAWxB,EAAoB,IAC/BsB,EAAWtB,EAAoB,GAcnCa,GAAQA,EAAQmD,EAAG,WAAYd,IAAKA,OAI/B,SAAS9C,EAAQD,EAASH,GAG/B,GAAIY,GAAWZ,EAAoB,GAC/Ba,EAAWb,EAAoB,GAC/BsB,EAAWtB,EAAoB,GAEnCa,GAAQA,EAAQmD,EAAG,WACjBE,yBAA0B,QAASA,0BAAyBsH,EAAQse,GAClE,MAAOlpB,GAAEkC,QAAQxB,EAASkK,GAASse,OAMlC,SAAS1pB,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,GAC/B6F,EAAW7F,EAAoB,GAAG6F,SAClCvE,EAAWtB,EAAoB,GAEnCa,GAAQA,EAAQmD,EAAG,WACjB4B,eAAgB,QAASA,gBAAe4F,GACtC,MAAO3F,GAASvE,EAASkK,QAMxB,SAASpL,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,WACjB9C,IAAK,QAASA,KAAIsK,EAAQse,GACxB,MAAOA,KAAete,OAMrB,SAASpL,EAAQD,EAASH,GAG/B,GAAIa,GAAgBb,EAAoB,GACpCsB,EAAgBtB,EAAoB,IACpC+T,EAAgB5R,OAAO6R,YAE3BnT,GAAQA,EAAQmD,EAAG,WACjBgQ,aAAc,QAASA,cAAaxI,GAElC,MADAlK,GAASkK,GACFuI,EAAgBA,EAAcvI,IAAU,MAM9C,SAASpL,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,WAAYqmB,QAASrqB,EAAoB,QAIvD,SAASI,EAAQD,EAASH,GAG/B,GAAIY,GAAWZ,EAAoB,GAC/BsB,EAAWtB,EAAoB,IAC/B0pB,EAAW1pB,EAAoB,GAAG0pB,OACtCtpB,GAAOD,QAAUupB,GAAWA,EAAQW,SAAW,QAASA,SAAQ7d,GAC9D,GAAI5I,GAAahD,EAAEoF,SAAS1E,EAASkL,IACjCrC,EAAavJ,EAAEuJ,UACnB,OAAOA,GAAavG,EAAKU,OAAO6F,EAAWqC,IAAO5I,IAK/C,SAASxD,EAAQD,EAASH,GAG/B,GAAIa,GAAqBb,EAAoB,GACzCsB,EAAqBtB,EAAoB,IACzCyT,EAAqBtR,OAAOuR,iBAEhC7S,GAAQA,EAAQmD,EAAG,WACjB0P,kBAAmB,QAASA,mBAAkBlI,GAC5ClK,EAASkK,EACT,KAEE,MADGiI,IAAmBA,EAAmBjI,IAClC,EACP,MAAMjI,GACN,OAAO,OAOR,SAASnD,EAAQD,EAASH,GAU/B,QAASwQ,KAAIhF,EAAQse,EAAaQ,GAChC,GAEIC,GAAoB1X,EAFpBuX,EAAWxjB,UAAU9C,OAAS,EAAI0H,EAAS5E,UAAU,GACrD4jB,EAAW5pB,EAAEkC,QAAQxB,EAASkK,GAASse,EAE3C,KAAIU,EAAQ,CACV,GAAGhpB,EAASqR,EAAQjS,EAAEiF,SAAS2F,IAC7B,MAAOgF,KAAIqC,EAAOiX,EAAaQ,EAAGF,EAEpCI,GAAUzpB,EAAW,GAEvB,MAAGG,GAAIspB,EAAS,SACXA,EAAQve,YAAa,GAAUzK,EAAS4oB,IAC3CG,EAAqB3pB,EAAEkC,QAAQsnB,EAAUN,IAAgB/oB,EAAW,GACpEwpB,EAAmB9mB,MAAQ6mB,EAC3B1pB,EAAEgC,QAAQwnB,EAAUN,EAAaS,IAC1B,IAJqD,EAMvDC,EAAQha,MAAQ1Q,GAAY,GAAS0qB,EAAQha,IAAIjQ,KAAK6pB,EAAUE,IAAI,GAxB7E,GAAI1pB,GAAaZ,EAAoB,GACjCkB,EAAalB,EAAoB,IACjCa,EAAab,EAAoB,GACjCe,EAAaf,EAAoB,GACjCsB,EAAatB,EAAoB,IACjCwB,EAAaxB,EAAoB,GAsBrCa,GAAQA,EAAQmD,EAAG,WAAYwM,IAAKA,OAI/B,SAASpQ,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,GAC/BwgB,EAAWxgB,EAAoB,GAEhCwgB,IAAS3f,EAAQA,EAAQmD,EAAG,WAC7B2O,eAAgB,QAASA,gBAAenH,EAAQqH,GAC9C2N,EAAS5N,MAAMpH,EAAQqH,EACvB,KAEE,MADA2N,GAAShQ,IAAIhF,EAAQqH,IACd,EACP,MAAMtP,GACN,OAAO,OAOR,SAASnD,EAAQD,EAASH,GAG/B,GAAIa,GAAYb,EAAoB,GAChCyqB,EAAYzqB,EAAoB,KAAI,EAExCa,GAAQA,EAAQwC,EAAG,SAEjBwX,SAAU,QAASA,UAASnS,GAC1B,MAAO+hB,GAAU/jB,KAAMgC,EAAI9B,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAIrEE,EAAoB,KAAK,aAIpB,SAASI,EAAQD,EAASH,GAI/B,GAAIa,GAAUb,EAAoB,GAC9B+Z,EAAU/Z,EAAoB,KAAI,EAEtCa,GAAQA,EAAQwC,EAAG,UACjBqnB,GAAI,QAASA,IAAGzQ,GACd,MAAOF,GAAIrT,KAAMuT,OAMhB,SAAS7Z,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B2qB,EAAU3qB,EAAoB,IAElCa,GAAQA,EAAQwC,EAAG,UACjBunB,QAAS,QAASA,SAAQC,GACxB,MAAOF,GAAKjkB,KAAMmkB,EAAWjkB,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,GAAW,OAM7E,SAASM,EAAQD,EAASH,GAG/B,GAAI6B,GAAW7B,EAAoB,IAC/B8a,EAAW9a,EAAoB,KAC/BsN,EAAWtN,EAAoB,GAEnCI,GAAOD,QAAU,SAASqG,EAAMqkB,EAAWC,EAAYC,GACrD,GAAI/mB,GAAe4I,OAAOU,EAAQ9G,IAC9BwkB,EAAehnB,EAAEF,OACjBmnB,EAAeH,IAAehrB,EAAY,IAAM8M,OAAOke,GACvDI,EAAerpB,EAASgpB,EAC5B,IAAmBG,GAAhBE,EAA6B,MAAOlnB,EACzB,KAAXinB,IAAcA,EAAU,IAC3B,IAAIE,GAAUD,EAAeF,EACzBI,EAAetQ,EAAOva,KAAK0qB,EAASriB,KAAK2E,KAAK4d,EAAUF,EAAQnnB,QAEpE,OADGsnB,GAAatnB,OAASqnB,IAAQC,EAAeA,EAAa5oB,MAAM,EAAG2oB,IAC/DJ,EAAOK,EAAepnB,EAAIA,EAAIonB,IAKlC,SAAShrB,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B2qB,EAAU3qB,EAAoB,IAElCa,GAAQA,EAAQwC,EAAG,UACjBgoB,SAAU,QAASA,UAASR,GAC1B,MAAOF,GAAKjkB,KAAMmkB,EAAWjkB,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,GAAW,OAM7E,SAASM,EAAQD,EAASH,GAI/BA,EAAoB,IAAI,WAAY,SAAS0U,GAC3C,MAAO,SAAS4W,YACd,MAAO5W,GAAMhO,KAAM,OAMlB,SAAStG,EAAQD,EAASH,GAI/BA,EAAoB,IAAI,YAAa,SAAS0U,GAC5C,MAAO,SAAS6W,aACd,MAAO7W,GAAMhO,KAAM,OAMlB,SAAStG,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9BwrB,EAAUxrB,EAAoB,KAAK,sBAAuB,OAE9Da,GAAQA,EAAQmD,EAAG,UAAWynB,OAAQ,QAASA,QAAOjf,GAAK,MAAOgf,GAAIhf,OAKjE,SAASpM,EAAQD,GAEtBC,EAAOD,QAAU,SAASurB,EAAQrV,GAChC,GAAIjF,GAAWiF,IAAYlU,OAAOkU,GAAW,SAASsV,GACpD,MAAOtV,GAAQsV,IACbtV,CACJ,OAAO,UAAS7J,GACd,MAAOI,QAAOJ,GAAI6J,QAAQqV,EAAQta,MAMjC,SAAShR,EAAQD,EAASH,GAG/B,GAAIY,GAAaZ,EAAoB,GACjCa,EAAab,EAAoB,GACjCqqB,EAAarqB,EAAoB,KACjC0B,EAAa1B,EAAoB,IACjCe,EAAaf,EAAoB,EAErCa,GAAQA,EAAQmD,EAAG,UACjB4nB,0BAA2B,QAASA,2BAA0BrmB,GAQ5D,IAPA,GAMIC,GAAK0K,EANL9M,EAAU1B,EAAU6D,GACpB3C,EAAUhC,EAAEgC,QACZE,EAAUlC,EAAEkC,QACZc,EAAUymB,EAAQjnB,GAClBqC,KACA1B,EAAU,EAERH,EAAKE,OAASC,GAClBmM,EAAIpN,EAAQM,EAAGoC,EAAM5B,EAAKG,MACvByB,IAAOC,GAAO7C,EAAQ6C,EAAQD,EAAKzE,EAAW,EAAGmP,IAC/CzK,EAAOD,GAAO0K,CACnB,OAAOzK,OAMR,SAASrF,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B6rB,EAAU7rB,EAAoB,MAAK,EAEvCa,GAAQA,EAAQmD,EAAG,UACjB0Y,OAAQ,QAASA,QAAOlQ,GACtB,MAAOqf,GAAQrf,OAMd,SAASpM,EAAQD,EAASH,GAE/B,GAAIY,GAAYZ,EAAoB,GAChC0B,EAAY1B,EAAoB,IAChCkK,EAAYtJ,EAAEsJ,MAClB9J,GAAOD,QAAU,SAAS2rB,GACxB,MAAO,UAAStf,GAOd,IANA,GAKIhH,GALApC,EAAS1B,EAAU8K,GACnB5I,EAAShD,EAAEiD,QAAQT,GACnBU,EAASF,EAAKE,OACdC,EAAS,EACT0B,KAEE3B,EAASC,GAAKmG,EAAO3J,KAAK6C,EAAGoC,EAAM5B,EAAKG,OAC5C0B,EAAOC,KAAKomB,GAAatmB,EAAKpC,EAAEoC,IAAQpC,EAAEoC,GAC1C,OAAOC,MAMR,SAASrF,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,GAC/B+rB,EAAW/rB,EAAoB,MAAK,EAExCa,GAAQA,EAAQmD,EAAG,UACjB2Y,QAAS,QAASA,SAAQnQ,GACxB,MAAOuf,GAASvf,OAMf,SAASpM,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,EAEnCa,GAAQA,EAAQwC,EAAG,OAAQ2oB,OAAQhsB,EAAoB,KAAK,UAIvD,SAASI,EAAQD,EAASH,GAG/B,GAAIugB,GAAUvgB,EAAoB,KAC9BiT,EAAUjT,EAAoB,GAClCI,GAAOD,QAAU,SAASkU,GACxB,MAAO,SAAS2X,UACd,GAAG/Y,EAAQvM,OAAS2N,EAAK,KAAM7Q,WAAU6Q,EAAO,wBAChD,IAAI4J,KAEJ,OADAsC,GAAM7Z,MAAM,EAAOuX,EAAIvY,KAAMuY,GACtBA,KAMN,SAAS7d,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,EAEnCa,GAAQA,EAAQwC,EAAG,OAAQ2oB,OAAQhsB,EAAoB,KAAK,UAIvD,SAASI,EAAQD,EAASH,GAE/B,GAAIa,GAAUb,EAAoB,GAC9BisB,EAAUjsB,EAAoB,IAClCa,GAAQA,EAAQsK,EAAItK,EAAQ0K,GAC1Bsa,aAAgBoG,EAAMzb,IACtBuV,eAAgBkG,EAAMpF,SAKnB,SAASzmB,EAAQD,EAASH,GAE/BA,EAAoB,IACpB,IAAIqK,GAAcrK,EAAoB,GAClCuK,EAAcvK,EAAoB,GAClC0b,EAAc1b,EAAoB,KAClC4b,EAAc5b,EAAoB,IAAI,YACtCksB,EAAc7hB,EAAO8hB,SACrBC,EAAc/hB,EAAOgiB,eACrBC,EAAcJ,GAAMA,EAAG9pB,UACvBmqB,EAAcH,GAAOA,EAAIhqB,UACzBoqB,EAAc9Q,EAAUyQ,SAAWzQ,EAAU2Q,eAAiB3Q,EAAUpZ,KACzEgqB,KAAYA,EAAQ1Q,IAAUrR,EAAK+hB,EAAS1Q,EAAU4Q,GACtDD,IAAaA,EAAS3Q,IAAUrR,EAAKgiB,EAAU3Q,EAAU4Q,IAIvD,SAASpsB,EAAQD,EAASH,GAG/B,GAAIqK,GAAarK,EAAoB,GACjCa,EAAab,EAAoB,GACjCoB,EAAapB,EAAoB,IACjCysB,EAAazsB,EAAoB,KACjC0sB,EAAariB,EAAOqiB,UACpBC,IAAeD,GAAa,WAAW5Z,KAAK4Z,EAAUE,WACtDxc,EAAO,SAASI,GAClB,MAAOmc,GAAO,SAASlmB,EAAIomB,GACzB,MAAOrc,GAAIpP,EACTqrB,KACGjqB,MAAMjC,KAAKqG,UAAW,GACZ,kBAANH,GAAmBA,EAAKH,SAASG,IACvComB,IACDrc,EAEN3P,GAAQA,EAAQsK,EAAItK,EAAQ0K,EAAI1K,EAAQoD,EAAI0oB,GAC1C9J,WAAazS,EAAK/F,EAAOwY,YACzBiK,YAAa1c,EAAK/F,EAAOyiB,gBAKtB,SAAS1sB,EAAQD,EAASH,GAG/B,GAAI+sB,GAAY/sB,EAAoB,KAChCoB,EAAYpB,EAAoB,IAChCuB,EAAYvB,EAAoB,GACpCI,GAAOD,QAAU,WAOf,IANA,GAAIsG,GAASlF,EAAUmF,MACnB5C,EAAS8C,UAAU9C,OACnBkpB,EAAS1qB,MAAMwB,GACfC,EAAS,EACTkpB,EAASF,EAAKE,EACdC,GAAS,EACPppB,EAASC,IAAMipB,EAAMjpB,GAAK6C,UAAU7C,QAAUkpB,IAAEC,GAAS,EAC/D,OAAO,YACL,GAGkB9mB,GAHdI,EAAQE,KACR4K,EAAQ1K,UACR4L,EAAQlB,EAAGxN,OACX2O,EAAI,EAAGH,EAAI,CACf,KAAI4a,IAAW1a,EAAM,MAAOpR,GAAOqF,EAAIumB,EAAOxmB,EAE9C,IADAJ,EAAO4mB,EAAMxqB,QACV0qB,EAAO,KAAKppB,EAAS2O,EAAGA,IAAOrM,EAAKqM,KAAOwa,IAAE7mB,EAAKqM,GAAKnB,EAAGgB,KAC7D,MAAME,EAAQF,GAAElM,EAAKV,KAAK4L,EAAGgB,KAC7B,OAAOlR,GAAOqF,EAAIL,EAAMI,MAMvB,SAASpG,EAAQD,EAASH,GAE/BI,EAAOD,QAAUH,EAAoB,IAIhC,SAASI,EAAQD,EAASH,GAoF/B,QAASmtB,MAAKnJ,GACZ,GAAIoJ,GAAOxsB,EAAEqF,OAAO,KAQpB,OAPG+d,IAAYlkB,IACVutB,EAAWrJ,GACZzD,EAAMyD,GAAU,EAAM,SAASxe,EAAK/B,GAClC2pB,EAAK5nB,GAAO/B,IAET0O,EAAOib,EAAMpJ,IAEfoJ,EAIT,QAAS9kB,QAAO/C,EAAQkY,EAAO6P,GAC7B/rB,EAAUkc,EACV,IAII9V,GAAMnC,EAJNpC,EAAS1B,EAAU6D,GACnB3B,EAASC,EAAQT,GACjBU,EAASF,EAAKE,OACdC,EAAS,CAEb,IAAG6C,UAAU9C,OAAS,EAAE,CACtB,IAAIA,EAAO,KAAMN,WAAU,+CAC3BmE,GAAOvE,EAAEQ,EAAKG,UACT4D,GAAOxF,OAAOmrB,EACrB,MAAMxpB,EAASC,GAAK7C,EAAIkC,EAAGoC,EAAM5B,EAAKG,QACpC4D,EAAO8V,EAAM9V,EAAMvE,EAAEoC,GAAMA,EAAKD,GAElC,OAAOoC,GAGT,QAASkT,UAAStV,EAAQmD,GACxB,OAAQA,GAAMA,EAAKyG,EAAM5J,EAAQmD,GAAM6kB,EAAQhoB,EAAQ,SAASiH,GAC9D,MAAOA,IAAMA,OACP1M,EAGV,QAASoD,KAAIqC,EAAQC,GACnB,MAAGtE,GAAIqE,EAAQC,GAAYD,EAAOC,GAAlC,OAEF,QAASgL,KAAIjL,EAAQC,EAAK/B,GAGxB,MAFG3C,IAAe0E,IAAOrD,QAAOvB,EAAEgC,QAAQ2C,EAAQC,EAAKzE,EAAW,EAAG0C,IAChE8B,EAAOC,GAAO/B,EACZ8B,EAGT,QAASioB,QAAOhhB,GACd,MAAOhL,GAASgL,IAAO5L,EAAEiF,SAAS2G,KAAQ2gB,KAAK/qB,UA/HjD,GAAIxB,GAAcZ,EAAoB,GAClCyK,EAAczK,EAAoB,IAClCa,EAAcb,EAAoB,GAClCe,EAAcf,EAAoB,GAClCmS,EAAcnS,EAAoB,IAClCmP,EAAcnP,EAAoB,IAClCuB,EAAcvB,EAAoB,IAClCugB,EAAcvgB,EAAoB,KAClCqtB,EAAcrtB,EAAoB,KAClC2b,EAAc3b,EAAoB,KAClCud,EAAcvd,EAAoB,KAClCwB,EAAcxB,EAAoB,IAClC0B,EAAc1B,EAAoB,IAClCc,EAAcd,EAAoB,GAClCkB,EAAclB,EAAoB,IAClC6D,EAAcjD,EAAEiD,QAUhB4pB,EAAmB,SAAS7f,GAC9B,GAAIC,GAAmB,GAARD,EACXI,EAAmB,GAARJ,CACf,OAAO,UAASrI,EAAQmC,EAAYlB,GAClC,GAIIhB,GAAKiH,EAAK2B,EAJVC,EAAS5D,EAAI/C,EAAYlB,EAAM,GAC/BpD,EAAS1B,EAAU6D,GACnBE,EAASoI,GAAkB,GAARD,GAAqB,GAARA,EAC5B,IAAoB,kBAARlH,MAAqBA,KAAOymB,MAAQrtB,CAExD,KAAI0F,IAAOpC,GAAE,GAAGlC,EAAIkC,EAAGoC,KACrBiH,EAAMrJ,EAAEoC,GACR4I,EAAMC,EAAE5B,EAAKjH,EAAKD,GACfqI,GACD,GAAGC,EAAOpI,EAAOD,GAAO4I,MACnB,IAAGA,EAAI,OAAOR,GACjB,IAAK,GAAGnI,EAAOD,GAAOiH,CAAK,MAC3B,KAAK,GAAG,OAAO,CACf,KAAK,GAAG,MAAOA,EACf,KAAK,GAAG,MAAOjH,EACf,KAAK,GAAGC,EAAO2I,EAAI,IAAMA,EAAI,OACxB,IAAGJ,EAAS,OAAO,CAG9B,OAAe,IAARJ,GAAaI,EAAWA,EAAWvI,IAG1C8nB,EAAUE,EAAiB,GAE3BC,EAAiB,SAASjR,GAC5B,MAAO,UAASjQ,GACd,MAAO,IAAImhB,GAAanhB,EAAIiQ,KAG5BkR,EAAe,SAASvS,EAAUqB,GACpC/V,KAAK2U,GAAK3Z,EAAU0Z,GACpB1U,KAAKknB,GAAK/pB,EAAQuX,GAClB1U,KAAK4U,GAAK,EACV5U,KAAK6J,GAAKkM,EAEZd,GAAYgS,EAAc,OAAQ,WAChC,GAIInoB,GAJAgB,EAAOE,KACPtD,EAAOoD,EAAK6U,GACZzX,EAAO4C,EAAKonB,GACZnR,EAAOjW,EAAK+J,EAEhB,GACE,IAAG/J,EAAK8U,IAAM1X,EAAKE,OAEjB,MADA0C,GAAK6U,GAAKvb,EACHyd,EAAK,UAEPrc,EAAIkC,EAAGoC,EAAM5B,EAAK4C,EAAK8U,OAChC,OAAW,QAARmB,EAAwBc,EAAK,EAAG/X,GACxB,UAARiX,EAAwBc,EAAK,EAAGna,EAAEoC,IAC9B+X,EAAK,GAAI/X,EAAKpC,EAAEoC,OAczB2nB,KAAK/qB,UAAY,KAsCjBvB,EAAQA,EAAQsK,EAAItK,EAAQoD,GAAIkpB,KAAMA,OAEtCtsB,EAAQA,EAAQmD,EAAG,QACjBJ,KAAU8pB,EAAe,QACzBhR,OAAUgR,EAAe,UACzB/Q,QAAU+Q,EAAe,WACzB1lB,QAAUylB,EAAiB,GAC3BvlB,IAAUulB,EAAiB,GAC3BtlB,OAAUslB,EAAiB,GAC3BrlB,KAAUqlB,EAAiB,GAC3BplB,MAAUolB,EAAiB,GAC3B5O,KAAU4O,EAAiB,GAC3BF,QAAUA,EACVM,SAAUJ,EAAiB,GAC3BnlB,OAAUA,OACV6G,MAAUA,EACV0L,SAAUA,SACV3Z,IAAUA,EACVgC,IAAUA,IACVsN,IAAUA,IACVgd,OAAUA,UAKP,SAASptB,EAAQD,EAASH,GAE/B,GAAIiT,GAAYjT,EAAoB,IAChC4b,EAAY5b,EAAoB,IAAI,YACpC0b,EAAY1b,EAAoB,IACpCI,GAAOD,QAAUH,EAAoB,GAAGqtB,WAAa,SAAS7gB,GAC5D,GAAIpJ,GAAIjB,OAAOqK,EACf,OAAOpJ,GAAEwY,KAAc9b,GAClB,cAAgBsD,IAChBsY,EAAU/O,eAAesG,EAAQ7P,MAKnC,SAAShD,EAAQD,EAASH,GAE/B,GAAIsB,GAAWtB,EAAoB,IAC/BkD,EAAWlD,EAAoB,IACnCI,GAAOD,QAAUH,EAAoB,GAAG8tB,YAAc,SAASthB,GAC7D,GAAImR,GAASza,EAAIsJ,EACjB,IAAoB,kBAAVmR,GAAqB,KAAMna,WAAUgJ,EAAK,oBACpD,OAAOlL,GAASqc,EAAOpd,KAAKiM,MAKzB,SAASpM,EAAQD,EAASH,GAE/B,GAAIqK,GAAUrK,EAAoB,GAC9BsK,EAAUtK,EAAoB,GAC9Ba,EAAUb,EAAoB,GAC9BysB,EAAUzsB,EAAoB,IAElCa,GAAQA,EAAQsK,EAAItK,EAAQoD,GAC1B8pB,MAAO,QAASA,OAAMlB,GACpB,MAAO,KAAKviB,EAAKkZ,SAAWnZ,EAAOmZ,SAAS,SAASrC,GACnD0B,WAAW4J,EAAQlsB,KAAK4gB,GAAS,GAAO0L,SAOzC,SAASzsB,EAAQD,EAASH,GAE/B,GAAI+sB,GAAU/sB,EAAoB,KAC9Ba,EAAUb,EAAoB,EAGlCA,GAAoB,GAAGitB,EAAIF,EAAKE,EAAIF,EAAKE,MAEzCpsB,EAAQA,EAAQwC,EAAIxC,EAAQoD,EAAG,YAAa0nB,KAAM3rB,EAAoB,QAIjE,SAASI,EAAQD,EAASH,GAE/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAG,UAAWzC,SAAUxB,EAAoB,OAInE,SAASI,EAAQD,EAASH,GAE/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAG,UAAWgP,QAASjT,EAAoB,OAIlE,SAASI,EAAQD,EAASH,GAE/B,GAAIa,GAAUb,EAAoB,GAC9BguB,EAAUhuB,EAAoB,IAElCa,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAG,UAAW+pB,OAAQA,KAI7C,SAAS5tB,EAAQD,EAASH,GAE/B,GAAIY,GAAYZ,EAAoB,GAChCqqB,EAAYrqB,EAAoB,KAChC0B,EAAY1B,EAAoB,GAEpCI,GAAOD,QAAU,QAAS6tB,QAAOxiB,EAAQyiB,GAIvC,IAHA,GAEWzoB,GAFP5B,EAASymB,EAAQ3oB,EAAUusB,IAC3BnqB,EAASF,EAAKE,OACdC,EAAI,EACFD,EAASC,GAAEnD,EAAEgC,QAAQ4I,EAAQhG,EAAM5B,EAAKG,KAAMnD,EAAEkC,QAAQmrB,EAAOzoB,GACrE,OAAOgG,KAKJ,SAASpL,EAAQD,EAASH,GAE/B,GAAIa,GAAUb,EAAoB,GAC9BguB,EAAUhuB,EAAoB,KAC9BiG,EAAUjG,EAAoB,GAAGiG,MAErCpF,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAG,UAC7BiqB,KAAM,SAASrb,EAAOob,GACpB,MAAOD,GAAO/nB,EAAO4M,GAAQob,OAM5B,SAAS7tB,EAAQD,EAASH,GAG/BA,EAAoB,KAAK2V,OAAQ,SAAU,SAASyF,GAClD1U,KAAKihB,IAAMvM,EACX1U,KAAK4U,GAAK,GACT,WACD,GAAIvX,GAAO2C,KAAK4U,KACZE,IAAa9U,KAAKihB,GAAT5jB,EACb,QAAQyX,KAAMA,EAAM/X,MAAO+X,EAAO1b,EAAYiE,MAK3C,SAAS3D,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9BwrB,EAAMxrB,EAAoB,KAAK,YACjCmuB,IAAK,QACLC,IAAK,OACLC,IAAK,OACLC,IAAK,SACLC,IAAK,UAGP1tB,GAAQA,EAAQwC,EAAIxC,EAAQoD,EAAG,UAAWuqB,WAAY,QAASA,cAAc,MAAOhD,GAAI9kB,UAInF,SAAStG,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9BwrB,EAAMxrB,EAAoB,KAAK,8BACjCyuB,QAAU,IACVC,OAAU,IACVC,OAAU,IACVC,SAAU,IACVC,SAAU,KAGZhuB,GAAQA,EAAQwC,EAAIxC,EAAQoD,EAAG,UAAW6qB,aAAe,QAASA,gBAAgB,MAAOtD,GAAI9kB,UAIxF,SAAStG,EAAQD,EAASH,GAE/B,GAAIY,GAAUZ,EAAoB,GAC9BqK,EAAUrK,EAAoB,GAC9Ba,EAAUb,EAAoB,GAC9BoX,KACA2X,GAAU,CAEdnuB,GAAEqH,KAAK1H,KAAK,kNAIV6D,MAAM,KAAM,SAASoB,GACrB4R,EAAI5R,GAAO,WACT,GAAIwpB,GAAW3kB,EAAOyY,OACtB,OAAGiM,IAAWC,GAAYA,EAASxpB,GAC1Bc,SAAS2G,MAAM1M,KAAKyuB,EAASxpB,GAAMwpB,EAAUpoB,WADtD,UAKJ/F,EAAQA,EAAQsK,EAAItK,EAAQoD,GAAImT,IAAKpX,EAAoB,IAAIoX,EAAIA,IAAKA,GACpE6X,OAAQ,WACNF,GAAU,GAEZG,QAAS,WACPH,GAAU,QAMT,SAAS3uB,EAAQD,EAASH,GAG/B,GAAIY,GAAUZ,EAAoB,GAC9Ba,EAAUb,EAAoB,GAC9BmvB,EAAUnvB,EAAoB,IAC9BovB,EAAUpvB,EAAoB,GAAGsC,OAASA,MAC1C+sB,KACAC,EAAa,SAAS1rB,EAAME,GAC9BlD,EAAEqH,KAAK1H,KAAKqD,EAAKQ,MAAM,KAAM,SAASoB,GACjC1B,GAAUhE,GAAa0F,IAAO4pB,GAAOC,EAAQ7pB,GAAO4pB,EAAO5pB,GACtDA,SAAU6pB,EAAQ7pB,GAAO2pB,EAAK7oB,SAAS/F,QAASiF,GAAM1B,MAGlEwrB,GAAW,wCAAyC,GACpDA,EAAW,gEAAiE,GAC5EA,EAAW,6FAEXzuB,EAAQA,EAAQmD,EAAG,QAASqrB,MAKT,mBAAVjvB,SAAyBA,OAAOD,QAAQC,OAAOD,QAAUP,EAE1C,kBAAVouB,SAAwBA,OAAOuB,IAAIvB,OAAO,WAAW,MAAOpuB,KAEtEC,EAAIyK,KAAO1K,GACd,EAAG","file":"core.min.js"}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/library.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/library.js
new file mode 100644
index 00000000..65385ef0
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/library.js
@@ -0,0 +1,4550 @@
+/**
+ * core-js 1.2.7
+ * https://github.com/zloirock/core-js
+ * License: http://rock.mit-license.org
+ * © 2016 Denis Pushkarev
+ */
+!function(__e, __g, undefined){
+'use strict';
+/******/ (function(modules) { // webpackBootstrap
+/******/ // The module cache
+/******/ var installedModules = {};
+
+/******/ // The require function
+/******/ function __webpack_require__(moduleId) {
+
+/******/ // Check if module is in cache
+/******/ if(installedModules[moduleId])
+/******/ return installedModules[moduleId].exports;
+
+/******/ // Create a new module (and put it into the cache)
+/******/ var module = installedModules[moduleId] = {
+/******/ exports: {},
+/******/ id: moduleId,
+/******/ loaded: false
+/******/ };
+
+/******/ // Execute the module function
+/******/ modules[moduleId].call(module.exports, module, module.exports, __webpack_require__);
+
+/******/ // Flag the module as loaded
+/******/ module.loaded = true;
+
+/******/ // Return the exports of the module
+/******/ return module.exports;
+/******/ }
+
+
+/******/ // expose the modules object (__webpack_modules__)
+/******/ __webpack_require__.m = modules;
+
+/******/ // expose the module cache
+/******/ __webpack_require__.c = installedModules;
+
+/******/ // __webpack_public_path__
+/******/ __webpack_require__.p = "";
+
+/******/ // Load entry module and return exports
+/******/ return __webpack_require__(0);
+/******/ })
+/************************************************************************/
+/******/ ([
+/* 0 */
+/***/ function(module, exports, __webpack_require__) {
+
+ __webpack_require__(1);
+ __webpack_require__(32);
+ __webpack_require__(40);
+ __webpack_require__(42);
+ __webpack_require__(44);
+ __webpack_require__(46);
+ __webpack_require__(48);
+ __webpack_require__(49);
+ __webpack_require__(50);
+ __webpack_require__(51);
+ __webpack_require__(52);
+ __webpack_require__(53);
+ __webpack_require__(54);
+ __webpack_require__(55);
+ __webpack_require__(56);
+ __webpack_require__(57);
+ __webpack_require__(58);
+ __webpack_require__(59);
+ __webpack_require__(60);
+ __webpack_require__(62);
+ __webpack_require__(63);
+ __webpack_require__(64);
+ __webpack_require__(65);
+ __webpack_require__(66);
+ __webpack_require__(67);
+ __webpack_require__(68);
+ __webpack_require__(70);
+ __webpack_require__(71);
+ __webpack_require__(72);
+ __webpack_require__(74);
+ __webpack_require__(75);
+ __webpack_require__(76);
+ __webpack_require__(78);
+ __webpack_require__(79);
+ __webpack_require__(80);
+ __webpack_require__(81);
+ __webpack_require__(82);
+ __webpack_require__(83);
+ __webpack_require__(84);
+ __webpack_require__(85);
+ __webpack_require__(86);
+ __webpack_require__(87);
+ __webpack_require__(88);
+ __webpack_require__(89);
+ __webpack_require__(90);
+ __webpack_require__(92);
+ __webpack_require__(94);
+ __webpack_require__(98);
+ __webpack_require__(99);
+ __webpack_require__(101);
+ __webpack_require__(102);
+ __webpack_require__(106);
+ __webpack_require__(112);
+ __webpack_require__(113);
+ __webpack_require__(116);
+ __webpack_require__(118);
+ __webpack_require__(120);
+ __webpack_require__(122);
+ __webpack_require__(123);
+ __webpack_require__(124);
+ __webpack_require__(131);
+ __webpack_require__(134);
+ __webpack_require__(135);
+ __webpack_require__(137);
+ __webpack_require__(138);
+ __webpack_require__(139);
+ __webpack_require__(140);
+ __webpack_require__(141);
+ __webpack_require__(142);
+ __webpack_require__(143);
+ __webpack_require__(144);
+ __webpack_require__(145);
+ __webpack_require__(146);
+ __webpack_require__(147);
+ __webpack_require__(148);
+ __webpack_require__(150);
+ __webpack_require__(151);
+ __webpack_require__(152);
+ __webpack_require__(153);
+ __webpack_require__(154);
+ __webpack_require__(155);
+ __webpack_require__(157);
+ __webpack_require__(158);
+ __webpack_require__(159);
+ __webpack_require__(160);
+ __webpack_require__(162);
+ __webpack_require__(163);
+ __webpack_require__(165);
+ __webpack_require__(166);
+ __webpack_require__(168);
+ __webpack_require__(169);
+ __webpack_require__(170);
+ __webpack_require__(171);
+ __webpack_require__(174);
+ __webpack_require__(109);
+ __webpack_require__(176);
+ __webpack_require__(175);
+ __webpack_require__(177);
+ __webpack_require__(178);
+ __webpack_require__(179);
+ __webpack_require__(180);
+ __webpack_require__(181);
+ __webpack_require__(183);
+ __webpack_require__(184);
+ __webpack_require__(185);
+ __webpack_require__(186);
+ __webpack_require__(187);
+ module.exports = __webpack_require__(188);
+
+
+/***/ },
+/* 1 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , $export = __webpack_require__(3)
+ , DESCRIPTORS = __webpack_require__(8)
+ , createDesc = __webpack_require__(10)
+ , html = __webpack_require__(11)
+ , cel = __webpack_require__(12)
+ , has = __webpack_require__(14)
+ , cof = __webpack_require__(15)
+ , invoke = __webpack_require__(16)
+ , fails = __webpack_require__(9)
+ , anObject = __webpack_require__(17)
+ , aFunction = __webpack_require__(7)
+ , isObject = __webpack_require__(13)
+ , toObject = __webpack_require__(18)
+ , toIObject = __webpack_require__(20)
+ , toInteger = __webpack_require__(22)
+ , toIndex = __webpack_require__(23)
+ , toLength = __webpack_require__(24)
+ , IObject = __webpack_require__(21)
+ , IE_PROTO = __webpack_require__(25)('__proto__')
+ , createArrayMethod = __webpack_require__(26)
+ , arrayIndexOf = __webpack_require__(31)(false)
+ , ObjectProto = Object.prototype
+ , ArrayProto = Array.prototype
+ , arraySlice = ArrayProto.slice
+ , arrayJoin = ArrayProto.join
+ , defineProperty = $.setDesc
+ , getOwnDescriptor = $.getDesc
+ , defineProperties = $.setDescs
+ , factories = {}
+ , IE8_DOM_DEFINE;
+
+ if(!DESCRIPTORS){
+ IE8_DOM_DEFINE = !fails(function(){
+ return defineProperty(cel('div'), 'a', {get: function(){ return 7; }}).a != 7;
+ });
+ $.setDesc = function(O, P, Attributes){
+ if(IE8_DOM_DEFINE)try {
+ return defineProperty(O, P, Attributes);
+ } catch(e){ /* empty */ }
+ if('get' in Attributes || 'set' in Attributes)throw TypeError('Accessors not supported!');
+ if('value' in Attributes)anObject(O)[P] = Attributes.value;
+ return O;
+ };
+ $.getDesc = function(O, P){
+ if(IE8_DOM_DEFINE)try {
+ return getOwnDescriptor(O, P);
+ } catch(e){ /* empty */ }
+ if(has(O, P))return createDesc(!ObjectProto.propertyIsEnumerable.call(O, P), O[P]);
+ };
+ $.setDescs = defineProperties = function(O, Properties){
+ anObject(O);
+ var keys = $.getKeys(Properties)
+ , length = keys.length
+ , i = 0
+ , P;
+ while(length > i)$.setDesc(O, P = keys[i++], Properties[P]);
+ return O;
+ };
+ }
+ $export($export.S + $export.F * !DESCRIPTORS, 'Object', {
+ // 19.1.2.6 / 15.2.3.3 Object.getOwnPropertyDescriptor(O, P)
+ getOwnPropertyDescriptor: $.getDesc,
+ // 19.1.2.4 / 15.2.3.6 Object.defineProperty(O, P, Attributes)
+ defineProperty: $.setDesc,
+ // 19.1.2.3 / 15.2.3.7 Object.defineProperties(O, Properties)
+ defineProperties: defineProperties
+ });
+
+ // IE 8- don't enum bug keys
+ var keys1 = ('constructor,hasOwnProperty,isPrototypeOf,propertyIsEnumerable,' +
+ 'toLocaleString,toString,valueOf').split(',')
+ // Additional keys for getOwnPropertyNames
+ , keys2 = keys1.concat('length', 'prototype')
+ , keysLen1 = keys1.length;
+
+ // Create object with `null` prototype: use iframe Object with cleared prototype
+ var createDict = function(){
+ // Thrash, waste and sodomy: IE GC bug
+ var iframe = cel('iframe')
+ , i = keysLen1
+ , gt = '>'
+ , iframeDocument;
+ iframe.style.display = 'none';
+ html.appendChild(iframe);
+ iframe.src = 'javascript:'; // eslint-disable-line no-script-url
+ // createDict = iframe.contentWindow.Object;
+ // html.removeChild(iframe);
+ iframeDocument = iframe.contentWindow.document;
+ iframeDocument.open();
+ iframeDocument.write(' i)if(has(O, key = names[i++])){
+ ~arrayIndexOf(result, key) || result.push(key);
+ }
+ return result;
+ };
+ };
+ var Empty = function(){};
+ $export($export.S, 'Object', {
+ // 19.1.2.9 / 15.2.3.2 Object.getPrototypeOf(O)
+ getPrototypeOf: $.getProto = $.getProto || function(O){
+ O = toObject(O);
+ if(has(O, IE_PROTO))return O[IE_PROTO];
+ if(typeof O.constructor == 'function' && O instanceof O.constructor){
+ return O.constructor.prototype;
+ } return O instanceof Object ? ObjectProto : null;
+ },
+ // 19.1.2.7 / 15.2.3.4 Object.getOwnPropertyNames(O)
+ getOwnPropertyNames: $.getNames = $.getNames || createGetKeys(keys2, keys2.length, true),
+ // 19.1.2.2 / 15.2.3.5 Object.create(O [, Properties])
+ create: $.create = $.create || function(O, /*?*/Properties){
+ var result;
+ if(O !== null){
+ Empty.prototype = anObject(O);
+ result = new Empty();
+ Empty.prototype = null;
+ // add "__proto__" for Object.getPrototypeOf shim
+ result[IE_PROTO] = O;
+ } else result = createDict();
+ return Properties === undefined ? result : defineProperties(result, Properties);
+ },
+ // 19.1.2.14 / 15.2.3.14 Object.keys(O)
+ keys: $.getKeys = $.getKeys || createGetKeys(keys1, keysLen1, false)
+ });
+
+ var construct = function(F, len, args){
+ if(!(len in factories)){
+ for(var n = [], i = 0; i < len; i++)n[i] = 'a[' + i + ']';
+ factories[len] = Function('F,a', 'return new F(' + n.join(',') + ')');
+ }
+ return factories[len](F, args);
+ };
+
+ // 19.2.3.2 / 15.3.4.5 Function.prototype.bind(thisArg, args...)
+ $export($export.P, 'Function', {
+ bind: function bind(that /*, args... */){
+ var fn = aFunction(this)
+ , partArgs = arraySlice.call(arguments, 1);
+ var bound = function(/* args... */){
+ var args = partArgs.concat(arraySlice.call(arguments));
+ return this instanceof bound ? construct(fn, args.length, args) : invoke(fn, args, that);
+ };
+ if(isObject(fn.prototype))bound.prototype = fn.prototype;
+ return bound;
+ }
+ });
+
+ // fallback for not array-like ES3 strings and DOM objects
+ $export($export.P + $export.F * fails(function(){
+ if(html)arraySlice.call(html);
+ }), 'Array', {
+ slice: function(begin, end){
+ var len = toLength(this.length)
+ , klass = cof(this);
+ end = end === undefined ? len : end;
+ if(klass == 'Array')return arraySlice.call(this, begin, end);
+ var start = toIndex(begin, len)
+ , upTo = toIndex(end, len)
+ , size = toLength(upTo - start)
+ , cloned = Array(size)
+ , i = 0;
+ for(; i < size; i++)cloned[i] = klass == 'String'
+ ? this.charAt(start + i)
+ : this[start + i];
+ return cloned;
+ }
+ });
+ $export($export.P + $export.F * (IObject != Object), 'Array', {
+ join: function join(separator){
+ return arrayJoin.call(IObject(this), separator === undefined ? ',' : separator);
+ }
+ });
+
+ // 22.1.2.2 / 15.4.3.2 Array.isArray(arg)
+ $export($export.S, 'Array', {isArray: __webpack_require__(28)});
+
+ var createArrayReduce = function(isRight){
+ return function(callbackfn, memo){
+ aFunction(callbackfn);
+ var O = IObject(this)
+ , length = toLength(O.length)
+ , index = isRight ? length - 1 : 0
+ , i = isRight ? -1 : 1;
+ if(arguments.length < 2)for(;;){
+ if(index in O){
+ memo = O[index];
+ index += i;
+ break;
+ }
+ index += i;
+ if(isRight ? index < 0 : length <= index){
+ throw TypeError('Reduce of empty array with no initial value');
+ }
+ }
+ for(;isRight ? index >= 0 : length > index; index += i)if(index in O){
+ memo = callbackfn(memo, O[index], index, this);
+ }
+ return memo;
+ };
+ };
+
+ var methodize = function($fn){
+ return function(arg1/*, arg2 = undefined */){
+ return $fn(this, arg1, arguments[1]);
+ };
+ };
+
+ $export($export.P, 'Array', {
+ // 22.1.3.10 / 15.4.4.18 Array.prototype.forEach(callbackfn [, thisArg])
+ forEach: $.each = $.each || methodize(createArrayMethod(0)),
+ // 22.1.3.15 / 15.4.4.19 Array.prototype.map(callbackfn [, thisArg])
+ map: methodize(createArrayMethod(1)),
+ // 22.1.3.7 / 15.4.4.20 Array.prototype.filter(callbackfn [, thisArg])
+ filter: methodize(createArrayMethod(2)),
+ // 22.1.3.23 / 15.4.4.17 Array.prototype.some(callbackfn [, thisArg])
+ some: methodize(createArrayMethod(3)),
+ // 22.1.3.5 / 15.4.4.16 Array.prototype.every(callbackfn [, thisArg])
+ every: methodize(createArrayMethod(4)),
+ // 22.1.3.18 / 15.4.4.21 Array.prototype.reduce(callbackfn [, initialValue])
+ reduce: createArrayReduce(false),
+ // 22.1.3.19 / 15.4.4.22 Array.prototype.reduceRight(callbackfn [, initialValue])
+ reduceRight: createArrayReduce(true),
+ // 22.1.3.11 / 15.4.4.14 Array.prototype.indexOf(searchElement [, fromIndex])
+ indexOf: methodize(arrayIndexOf),
+ // 22.1.3.14 / 15.4.4.15 Array.prototype.lastIndexOf(searchElement [, fromIndex])
+ lastIndexOf: function(el, fromIndex /* = @[*-1] */){
+ var O = toIObject(this)
+ , length = toLength(O.length)
+ , index = length - 1;
+ if(arguments.length > 1)index = Math.min(index, toInteger(fromIndex));
+ if(index < 0)index = toLength(length + index);
+ for(;index >= 0; index--)if(index in O)if(O[index] === el)return index;
+ return -1;
+ }
+ });
+
+ // 20.3.3.1 / 15.9.4.4 Date.now()
+ $export($export.S, 'Date', {now: function(){ return +new Date; }});
+
+ var lz = function(num){
+ return num > 9 ? num : '0' + num;
+ };
+
+ // 20.3.4.36 / 15.9.5.43 Date.prototype.toISOString()
+ // PhantomJS / old WebKit has a broken implementations
+ $export($export.P + $export.F * (fails(function(){
+ return new Date(-5e13 - 1).toISOString() != '0385-07-25T07:06:39.999Z';
+ }) || !fails(function(){
+ new Date(NaN).toISOString();
+ })), 'Date', {
+ toISOString: function toISOString(){
+ if(!isFinite(this))throw RangeError('Invalid time value');
+ var d = this
+ , y = d.getUTCFullYear()
+ , m = d.getUTCMilliseconds()
+ , s = y < 0 ? '-' : y > 9999 ? '+' : '';
+ return s + ('00000' + Math.abs(y)).slice(s ? -6 : -4) +
+ '-' + lz(d.getUTCMonth() + 1) + '-' + lz(d.getUTCDate()) +
+ 'T' + lz(d.getUTCHours()) + ':' + lz(d.getUTCMinutes()) +
+ ':' + lz(d.getUTCSeconds()) + '.' + (m > 99 ? m : '0' + lz(m)) + 'Z';
+ }
+ });
+
+/***/ },
+/* 2 */
+/***/ function(module, exports) {
+
+ var $Object = Object;
+ module.exports = {
+ create: $Object.create,
+ getProto: $Object.getPrototypeOf,
+ isEnum: {}.propertyIsEnumerable,
+ getDesc: $Object.getOwnPropertyDescriptor,
+ setDesc: $Object.defineProperty,
+ setDescs: $Object.defineProperties,
+ getKeys: $Object.keys,
+ getNames: $Object.getOwnPropertyNames,
+ getSymbols: $Object.getOwnPropertySymbols,
+ each: [].forEach
+ };
+
+/***/ },
+/* 3 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var global = __webpack_require__(4)
+ , core = __webpack_require__(5)
+ , ctx = __webpack_require__(6)
+ , PROTOTYPE = 'prototype';
+
+ var $export = function(type, name, source){
+ var IS_FORCED = type & $export.F
+ , IS_GLOBAL = type & $export.G
+ , IS_STATIC = type & $export.S
+ , IS_PROTO = type & $export.P
+ , IS_BIND = type & $export.B
+ , IS_WRAP = type & $export.W
+ , exports = IS_GLOBAL ? core : core[name] || (core[name] = {})
+ , target = IS_GLOBAL ? global : IS_STATIC ? global[name] : (global[name] || {})[PROTOTYPE]
+ , key, own, out;
+ if(IS_GLOBAL)source = name;
+ for(key in source){
+ // contains in native
+ own = !IS_FORCED && target && key in target;
+ if(own && key in exports)continue;
+ // export native or passed
+ out = own ? target[key] : source[key];
+ // prevent global pollution for namespaces
+ exports[key] = IS_GLOBAL && typeof target[key] != 'function' ? source[key]
+ // bind timers to global for call from export context
+ : IS_BIND && own ? ctx(out, global)
+ // wrap global constructors for prevent change them in library
+ : IS_WRAP && target[key] == out ? (function(C){
+ var F = function(param){
+ return this instanceof C ? new C(param) : C(param);
+ };
+ F[PROTOTYPE] = C[PROTOTYPE];
+ return F;
+ // make static versions for prototype methods
+ })(out) : IS_PROTO && typeof out == 'function' ? ctx(Function.call, out) : out;
+ if(IS_PROTO)(exports[PROTOTYPE] || (exports[PROTOTYPE] = {}))[key] = out;
+ }
+ };
+ // type bitmap
+ $export.F = 1; // forced
+ $export.G = 2; // global
+ $export.S = 4; // static
+ $export.P = 8; // proto
+ $export.B = 16; // bind
+ $export.W = 32; // wrap
+ module.exports = $export;
+
+/***/ },
+/* 4 */
+/***/ function(module, exports) {
+
+ // https://github.com/zloirock/core-js/issues/86#issuecomment-115759028
+ var global = module.exports = typeof window != 'undefined' && window.Math == Math
+ ? window : typeof self != 'undefined' && self.Math == Math ? self : Function('return this')();
+ if(typeof __g == 'number')__g = global; // eslint-disable-line no-undef
+
+/***/ },
+/* 5 */
+/***/ function(module, exports) {
+
+ var core = module.exports = {version: '1.2.6'};
+ if(typeof __e == 'number')__e = core; // eslint-disable-line no-undef
+
+/***/ },
+/* 6 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // optional / simple context binding
+ var aFunction = __webpack_require__(7);
+ module.exports = function(fn, that, length){
+ aFunction(fn);
+ if(that === undefined)return fn;
+ switch(length){
+ case 1: return function(a){
+ return fn.call(that, a);
+ };
+ case 2: return function(a, b){
+ return fn.call(that, a, b);
+ };
+ case 3: return function(a, b, c){
+ return fn.call(that, a, b, c);
+ };
+ }
+ return function(/* ...args */){
+ return fn.apply(that, arguments);
+ };
+ };
+
+/***/ },
+/* 7 */
+/***/ function(module, exports) {
+
+ module.exports = function(it){
+ if(typeof it != 'function')throw TypeError(it + ' is not a function!');
+ return it;
+ };
+
+/***/ },
+/* 8 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // Thank's IE8 for his funny defineProperty
+ module.exports = !__webpack_require__(9)(function(){
+ return Object.defineProperty({}, 'a', {get: function(){ return 7; }}).a != 7;
+ });
+
+/***/ },
+/* 9 */
+/***/ function(module, exports) {
+
+ module.exports = function(exec){
+ try {
+ return !!exec();
+ } catch(e){
+ return true;
+ }
+ };
+
+/***/ },
+/* 10 */
+/***/ function(module, exports) {
+
+ module.exports = function(bitmap, value){
+ return {
+ enumerable : !(bitmap & 1),
+ configurable: !(bitmap & 2),
+ writable : !(bitmap & 4),
+ value : value
+ };
+ };
+
+/***/ },
+/* 11 */
+/***/ function(module, exports, __webpack_require__) {
+
+ module.exports = __webpack_require__(4).document && document.documentElement;
+
+/***/ },
+/* 12 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var isObject = __webpack_require__(13)
+ , document = __webpack_require__(4).document
+ // in old IE typeof document.createElement is 'object'
+ , is = isObject(document) && isObject(document.createElement);
+ module.exports = function(it){
+ return is ? document.createElement(it) : {};
+ };
+
+/***/ },
+/* 13 */
+/***/ function(module, exports) {
+
+ module.exports = function(it){
+ return typeof it === 'object' ? it !== null : typeof it === 'function';
+ };
+
+/***/ },
+/* 14 */
+/***/ function(module, exports) {
+
+ var hasOwnProperty = {}.hasOwnProperty;
+ module.exports = function(it, key){
+ return hasOwnProperty.call(it, key);
+ };
+
+/***/ },
+/* 15 */
+/***/ function(module, exports) {
+
+ var toString = {}.toString;
+
+ module.exports = function(it){
+ return toString.call(it).slice(8, -1);
+ };
+
+/***/ },
+/* 16 */
+/***/ function(module, exports) {
+
+ // fast apply, http://jsperf.lnkit.com/fast-apply/5
+ module.exports = function(fn, args, that){
+ var un = that === undefined;
+ switch(args.length){
+ case 0: return un ? fn()
+ : fn.call(that);
+ case 1: return un ? fn(args[0])
+ : fn.call(that, args[0]);
+ case 2: return un ? fn(args[0], args[1])
+ : fn.call(that, args[0], args[1]);
+ case 3: return un ? fn(args[0], args[1], args[2])
+ : fn.call(that, args[0], args[1], args[2]);
+ case 4: return un ? fn(args[0], args[1], args[2], args[3])
+ : fn.call(that, args[0], args[1], args[2], args[3]);
+ } return fn.apply(that, args);
+ };
+
+/***/ },
+/* 17 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var isObject = __webpack_require__(13);
+ module.exports = function(it){
+ if(!isObject(it))throw TypeError(it + ' is not an object!');
+ return it;
+ };
+
+/***/ },
+/* 18 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 7.1.13 ToObject(argument)
+ var defined = __webpack_require__(19);
+ module.exports = function(it){
+ return Object(defined(it));
+ };
+
+/***/ },
+/* 19 */
+/***/ function(module, exports) {
+
+ // 7.2.1 RequireObjectCoercible(argument)
+ module.exports = function(it){
+ if(it == undefined)throw TypeError("Can't call method on " + it);
+ return it;
+ };
+
+/***/ },
+/* 20 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // to indexed object, toObject with fallback for non-array-like ES3 strings
+ var IObject = __webpack_require__(21)
+ , defined = __webpack_require__(19);
+ module.exports = function(it){
+ return IObject(defined(it));
+ };
+
+/***/ },
+/* 21 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // fallback for non-array-like ES3 and non-enumerable old V8 strings
+ var cof = __webpack_require__(15);
+ module.exports = Object('z').propertyIsEnumerable(0) ? Object : function(it){
+ return cof(it) == 'String' ? it.split('') : Object(it);
+ };
+
+/***/ },
+/* 22 */
+/***/ function(module, exports) {
+
+ // 7.1.4 ToInteger
+ var ceil = Math.ceil
+ , floor = Math.floor;
+ module.exports = function(it){
+ return isNaN(it = +it) ? 0 : (it > 0 ? floor : ceil)(it);
+ };
+
+/***/ },
+/* 23 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var toInteger = __webpack_require__(22)
+ , max = Math.max
+ , min = Math.min;
+ module.exports = function(index, length){
+ index = toInteger(index);
+ return index < 0 ? max(index + length, 0) : min(index, length);
+ };
+
+/***/ },
+/* 24 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 7.1.15 ToLength
+ var toInteger = __webpack_require__(22)
+ , min = Math.min;
+ module.exports = function(it){
+ return it > 0 ? min(toInteger(it), 0x1fffffffffffff) : 0; // pow(2, 53) - 1 == 9007199254740991
+ };
+
+/***/ },
+/* 25 */
+/***/ function(module, exports) {
+
+ var id = 0
+ , px = Math.random();
+ module.exports = function(key){
+ return 'Symbol('.concat(key === undefined ? '' : key, ')_', (++id + px).toString(36));
+ };
+
+/***/ },
+/* 26 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 0 -> Array#forEach
+ // 1 -> Array#map
+ // 2 -> Array#filter
+ // 3 -> Array#some
+ // 4 -> Array#every
+ // 5 -> Array#find
+ // 6 -> Array#findIndex
+ var ctx = __webpack_require__(6)
+ , IObject = __webpack_require__(21)
+ , toObject = __webpack_require__(18)
+ , toLength = __webpack_require__(24)
+ , asc = __webpack_require__(27);
+ module.exports = function(TYPE){
+ var IS_MAP = TYPE == 1
+ , IS_FILTER = TYPE == 2
+ , IS_SOME = TYPE == 3
+ , IS_EVERY = TYPE == 4
+ , IS_FIND_INDEX = TYPE == 6
+ , NO_HOLES = TYPE == 5 || IS_FIND_INDEX;
+ return function($this, callbackfn, that){
+ var O = toObject($this)
+ , self = IObject(O)
+ , f = ctx(callbackfn, that, 3)
+ , length = toLength(self.length)
+ , index = 0
+ , result = IS_MAP ? asc($this, length) : IS_FILTER ? asc($this, 0) : undefined
+ , val, res;
+ for(;length > index; index++)if(NO_HOLES || index in self){
+ val = self[index];
+ res = f(val, index, O);
+ if(TYPE){
+ if(IS_MAP)result[index] = res; // map
+ else if(res)switch(TYPE){
+ case 3: return true; // some
+ case 5: return val; // find
+ case 6: return index; // findIndex
+ case 2: result.push(val); // filter
+ } else if(IS_EVERY)return false; // every
+ }
+ }
+ return IS_FIND_INDEX ? -1 : IS_SOME || IS_EVERY ? IS_EVERY : result;
+ };
+ };
+
+/***/ },
+/* 27 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 9.4.2.3 ArraySpeciesCreate(originalArray, length)
+ var isObject = __webpack_require__(13)
+ , isArray = __webpack_require__(28)
+ , SPECIES = __webpack_require__(29)('species');
+ module.exports = function(original, length){
+ var C;
+ if(isArray(original)){
+ C = original.constructor;
+ // cross-realm fallback
+ if(typeof C == 'function' && (C === Array || isArray(C.prototype)))C = undefined;
+ if(isObject(C)){
+ C = C[SPECIES];
+ if(C === null)C = undefined;
+ }
+ } return new (C === undefined ? Array : C)(length);
+ };
+
+/***/ },
+/* 28 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 7.2.2 IsArray(argument)
+ var cof = __webpack_require__(15);
+ module.exports = Array.isArray || function(arg){
+ return cof(arg) == 'Array';
+ };
+
+/***/ },
+/* 29 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var store = __webpack_require__(30)('wks')
+ , uid = __webpack_require__(25)
+ , Symbol = __webpack_require__(4).Symbol;
+ module.exports = function(name){
+ return store[name] || (store[name] =
+ Symbol && Symbol[name] || (Symbol || uid)('Symbol.' + name));
+ };
+
+/***/ },
+/* 30 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var global = __webpack_require__(4)
+ , SHARED = '__core-js_shared__'
+ , store = global[SHARED] || (global[SHARED] = {});
+ module.exports = function(key){
+ return store[key] || (store[key] = {});
+ };
+
+/***/ },
+/* 31 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // false -> Array#indexOf
+ // true -> Array#includes
+ var toIObject = __webpack_require__(20)
+ , toLength = __webpack_require__(24)
+ , toIndex = __webpack_require__(23);
+ module.exports = function(IS_INCLUDES){
+ return function($this, el, fromIndex){
+ var O = toIObject($this)
+ , length = toLength(O.length)
+ , index = toIndex(fromIndex, length)
+ , value;
+ // Array#includes uses SameValueZero equality algorithm
+ if(IS_INCLUDES && el != el)while(length > index){
+ value = O[index++];
+ if(value != value)return true;
+ // Array#toIndex ignores holes, Array#includes - not
+ } else for(;length > index; index++)if(IS_INCLUDES || index in O){
+ if(O[index] === el)return IS_INCLUDES || index;
+ } return !IS_INCLUDES && -1;
+ };
+ };
+
+/***/ },
+/* 32 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // ECMAScript 6 symbols shim
+ var $ = __webpack_require__(2)
+ , global = __webpack_require__(4)
+ , has = __webpack_require__(14)
+ , DESCRIPTORS = __webpack_require__(8)
+ , $export = __webpack_require__(3)
+ , redefine = __webpack_require__(33)
+ , $fails = __webpack_require__(9)
+ , shared = __webpack_require__(30)
+ , setToStringTag = __webpack_require__(35)
+ , uid = __webpack_require__(25)
+ , wks = __webpack_require__(29)
+ , keyOf = __webpack_require__(36)
+ , $names = __webpack_require__(37)
+ , enumKeys = __webpack_require__(38)
+ , isArray = __webpack_require__(28)
+ , anObject = __webpack_require__(17)
+ , toIObject = __webpack_require__(20)
+ , createDesc = __webpack_require__(10)
+ , getDesc = $.getDesc
+ , setDesc = $.setDesc
+ , _create = $.create
+ , getNames = $names.get
+ , $Symbol = global.Symbol
+ , $JSON = global.JSON
+ , _stringify = $JSON && $JSON.stringify
+ , setter = false
+ , HIDDEN = wks('_hidden')
+ , isEnum = $.isEnum
+ , SymbolRegistry = shared('symbol-registry')
+ , AllSymbols = shared('symbols')
+ , useNative = typeof $Symbol == 'function'
+ , ObjectProto = Object.prototype;
+
+ // fallback for old Android, https://code.google.com/p/v8/issues/detail?id=687
+ var setSymbolDesc = DESCRIPTORS && $fails(function(){
+ return _create(setDesc({}, 'a', {
+ get: function(){ return setDesc(this, 'a', {value: 7}).a; }
+ })).a != 7;
+ }) ? function(it, key, D){
+ var protoDesc = getDesc(ObjectProto, key);
+ if(protoDesc)delete ObjectProto[key];
+ setDesc(it, key, D);
+ if(protoDesc && it !== ObjectProto)setDesc(ObjectProto, key, protoDesc);
+ } : setDesc;
+
+ var wrap = function(tag){
+ var sym = AllSymbols[tag] = _create($Symbol.prototype);
+ sym._k = tag;
+ DESCRIPTORS && setter && setSymbolDesc(ObjectProto, tag, {
+ configurable: true,
+ set: function(value){
+ if(has(this, HIDDEN) && has(this[HIDDEN], tag))this[HIDDEN][tag] = false;
+ setSymbolDesc(this, tag, createDesc(1, value));
+ }
+ });
+ return sym;
+ };
+
+ var isSymbol = function(it){
+ return typeof it == 'symbol';
+ };
+
+ var $defineProperty = function defineProperty(it, key, D){
+ if(D && has(AllSymbols, key)){
+ if(!D.enumerable){
+ if(!has(it, HIDDEN))setDesc(it, HIDDEN, createDesc(1, {}));
+ it[HIDDEN][key] = true;
+ } else {
+ if(has(it, HIDDEN) && it[HIDDEN][key])it[HIDDEN][key] = false;
+ D = _create(D, {enumerable: createDesc(0, false)});
+ } return setSymbolDesc(it, key, D);
+ } return setDesc(it, key, D);
+ };
+ var $defineProperties = function defineProperties(it, P){
+ anObject(it);
+ var keys = enumKeys(P = toIObject(P))
+ , i = 0
+ , l = keys.length
+ , key;
+ while(l > i)$defineProperty(it, key = keys[i++], P[key]);
+ return it;
+ };
+ var $create = function create(it, P){
+ return P === undefined ? _create(it) : $defineProperties(_create(it), P);
+ };
+ var $propertyIsEnumerable = function propertyIsEnumerable(key){
+ var E = isEnum.call(this, key);
+ return E || !has(this, key) || !has(AllSymbols, key) || has(this, HIDDEN) && this[HIDDEN][key]
+ ? E : true;
+ };
+ var $getOwnPropertyDescriptor = function getOwnPropertyDescriptor(it, key){
+ var D = getDesc(it = toIObject(it), key);
+ if(D && has(AllSymbols, key) && !(has(it, HIDDEN) && it[HIDDEN][key]))D.enumerable = true;
+ return D;
+ };
+ var $getOwnPropertyNames = function getOwnPropertyNames(it){
+ var names = getNames(toIObject(it))
+ , result = []
+ , i = 0
+ , key;
+ while(names.length > i)if(!has(AllSymbols, key = names[i++]) && key != HIDDEN)result.push(key);
+ return result;
+ };
+ var $getOwnPropertySymbols = function getOwnPropertySymbols(it){
+ var names = getNames(toIObject(it))
+ , result = []
+ , i = 0
+ , key;
+ while(names.length > i)if(has(AllSymbols, key = names[i++]))result.push(AllSymbols[key]);
+ return result;
+ };
+ var $stringify = function stringify(it){
+ if(it === undefined || isSymbol(it))return; // IE8 returns string on undefined
+ var args = [it]
+ , i = 1
+ , $$ = arguments
+ , replacer, $replacer;
+ while($$.length > i)args.push($$[i++]);
+ replacer = args[1];
+ if(typeof replacer == 'function')$replacer = replacer;
+ if($replacer || !isArray(replacer))replacer = function(key, value){
+ if($replacer)value = $replacer.call(this, key, value);
+ if(!isSymbol(value))return value;
+ };
+ args[1] = replacer;
+ return _stringify.apply($JSON, args);
+ };
+ var buggyJSON = $fails(function(){
+ var S = $Symbol();
+ // MS Edge converts symbol values to JSON as {}
+ // WebKit converts symbol values to JSON as null
+ // V8 throws on boxed symbols
+ return _stringify([S]) != '[null]' || _stringify({a: S}) != '{}' || _stringify(Object(S)) != '{}';
+ });
+
+ // 19.4.1.1 Symbol([description])
+ if(!useNative){
+ $Symbol = function Symbol(){
+ if(isSymbol(this))throw TypeError('Symbol is not a constructor');
+ return wrap(uid(arguments.length > 0 ? arguments[0] : undefined));
+ };
+ redefine($Symbol.prototype, 'toString', function toString(){
+ return this._k;
+ });
+
+ isSymbol = function(it){
+ return it instanceof $Symbol;
+ };
+
+ $.create = $create;
+ $.isEnum = $propertyIsEnumerable;
+ $.getDesc = $getOwnPropertyDescriptor;
+ $.setDesc = $defineProperty;
+ $.setDescs = $defineProperties;
+ $.getNames = $names.get = $getOwnPropertyNames;
+ $.getSymbols = $getOwnPropertySymbols;
+
+ if(DESCRIPTORS && !__webpack_require__(39)){
+ redefine(ObjectProto, 'propertyIsEnumerable', $propertyIsEnumerable, true);
+ }
+ }
+
+ var symbolStatics = {
+ // 19.4.2.1 Symbol.for(key)
+ 'for': function(key){
+ return has(SymbolRegistry, key += '')
+ ? SymbolRegistry[key]
+ : SymbolRegistry[key] = $Symbol(key);
+ },
+ // 19.4.2.5 Symbol.keyFor(sym)
+ keyFor: function keyFor(key){
+ return keyOf(SymbolRegistry, key);
+ },
+ useSetter: function(){ setter = true; },
+ useSimple: function(){ setter = false; }
+ };
+ // 19.4.2.2 Symbol.hasInstance
+ // 19.4.2.3 Symbol.isConcatSpreadable
+ // 19.4.2.4 Symbol.iterator
+ // 19.4.2.6 Symbol.match
+ // 19.4.2.8 Symbol.replace
+ // 19.4.2.9 Symbol.search
+ // 19.4.2.10 Symbol.species
+ // 19.4.2.11 Symbol.split
+ // 19.4.2.12 Symbol.toPrimitive
+ // 19.4.2.13 Symbol.toStringTag
+ // 19.4.2.14 Symbol.unscopables
+ $.each.call((
+ 'hasInstance,isConcatSpreadable,iterator,match,replace,search,' +
+ 'species,split,toPrimitive,toStringTag,unscopables'
+ ).split(','), function(it){
+ var sym = wks(it);
+ symbolStatics[it] = useNative ? sym : wrap(sym);
+ });
+
+ setter = true;
+
+ $export($export.G + $export.W, {Symbol: $Symbol});
+
+ $export($export.S, 'Symbol', symbolStatics);
+
+ $export($export.S + $export.F * !useNative, 'Object', {
+ // 19.1.2.2 Object.create(O [, Properties])
+ create: $create,
+ // 19.1.2.4 Object.defineProperty(O, P, Attributes)
+ defineProperty: $defineProperty,
+ // 19.1.2.3 Object.defineProperties(O, Properties)
+ defineProperties: $defineProperties,
+ // 19.1.2.6 Object.getOwnPropertyDescriptor(O, P)
+ getOwnPropertyDescriptor: $getOwnPropertyDescriptor,
+ // 19.1.2.7 Object.getOwnPropertyNames(O)
+ getOwnPropertyNames: $getOwnPropertyNames,
+ // 19.1.2.8 Object.getOwnPropertySymbols(O)
+ getOwnPropertySymbols: $getOwnPropertySymbols
+ });
+
+ // 24.3.2 JSON.stringify(value [, replacer [, space]])
+ $JSON && $export($export.S + $export.F * (!useNative || buggyJSON), 'JSON', {stringify: $stringify});
+
+ // 19.4.3.5 Symbol.prototype[@@toStringTag]
+ setToStringTag($Symbol, 'Symbol');
+ // 20.2.1.9 Math[@@toStringTag]
+ setToStringTag(Math, 'Math', true);
+ // 24.3.3 JSON[@@toStringTag]
+ setToStringTag(global.JSON, 'JSON', true);
+
+/***/ },
+/* 33 */
+/***/ function(module, exports, __webpack_require__) {
+
+ module.exports = __webpack_require__(34);
+
+/***/ },
+/* 34 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $ = __webpack_require__(2)
+ , createDesc = __webpack_require__(10);
+ module.exports = __webpack_require__(8) ? function(object, key, value){
+ return $.setDesc(object, key, createDesc(1, value));
+ } : function(object, key, value){
+ object[key] = value;
+ return object;
+ };
+
+/***/ },
+/* 35 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var def = __webpack_require__(2).setDesc
+ , has = __webpack_require__(14)
+ , TAG = __webpack_require__(29)('toStringTag');
+
+ module.exports = function(it, tag, stat){
+ if(it && !has(it = stat ? it : it.prototype, TAG))def(it, TAG, {configurable: true, value: tag});
+ };
+
+/***/ },
+/* 36 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $ = __webpack_require__(2)
+ , toIObject = __webpack_require__(20);
+ module.exports = function(object, el){
+ var O = toIObject(object)
+ , keys = $.getKeys(O)
+ , length = keys.length
+ , index = 0
+ , key;
+ while(length > index)if(O[key = keys[index++]] === el)return key;
+ };
+
+/***/ },
+/* 37 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // fallback for IE11 buggy Object.getOwnPropertyNames with iframe and window
+ var toIObject = __webpack_require__(20)
+ , getNames = __webpack_require__(2).getNames
+ , toString = {}.toString;
+
+ var windowNames = typeof window == 'object' && Object.getOwnPropertyNames
+ ? Object.getOwnPropertyNames(window) : [];
+
+ var getWindowNames = function(it){
+ try {
+ return getNames(it);
+ } catch(e){
+ return windowNames.slice();
+ }
+ };
+
+ module.exports.get = function getOwnPropertyNames(it){
+ if(windowNames && toString.call(it) == '[object Window]')return getWindowNames(it);
+ return getNames(toIObject(it));
+ };
+
+/***/ },
+/* 38 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // all enumerable object keys, includes symbols
+ var $ = __webpack_require__(2);
+ module.exports = function(it){
+ var keys = $.getKeys(it)
+ , getSymbols = $.getSymbols;
+ if(getSymbols){
+ var symbols = getSymbols(it)
+ , isEnum = $.isEnum
+ , i = 0
+ , key;
+ while(symbols.length > i)if(isEnum.call(it, key = symbols[i++]))keys.push(key);
+ }
+ return keys;
+ };
+
+/***/ },
+/* 39 */
+/***/ function(module, exports) {
+
+ module.exports = true;
+
+/***/ },
+/* 40 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.3.1 Object.assign(target, source)
+ var $export = __webpack_require__(3);
+
+ $export($export.S + $export.F, 'Object', {assign: __webpack_require__(41)});
+
+/***/ },
+/* 41 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.1 Object.assign(target, source, ...)
+ var $ = __webpack_require__(2)
+ , toObject = __webpack_require__(18)
+ , IObject = __webpack_require__(21);
+
+ // should work with symbols and should have deterministic property order (V8 bug)
+ module.exports = __webpack_require__(9)(function(){
+ var a = Object.assign
+ , A = {}
+ , B = {}
+ , S = Symbol()
+ , K = 'abcdefghijklmnopqrst';
+ A[S] = 7;
+ K.split('').forEach(function(k){ B[k] = k; });
+ return a({}, A)[S] != 7 || Object.keys(a({}, B)).join('') != K;
+ }) ? function assign(target, source){ // eslint-disable-line no-unused-vars
+ var T = toObject(target)
+ , $$ = arguments
+ , $$len = $$.length
+ , index = 1
+ , getKeys = $.getKeys
+ , getSymbols = $.getSymbols
+ , isEnum = $.isEnum;
+ while($$len > index){
+ var S = IObject($$[index++])
+ , keys = getSymbols ? getKeys(S).concat(getSymbols(S)) : getKeys(S)
+ , length = keys.length
+ , j = 0
+ , key;
+ while(length > j)if(isEnum.call(S, key = keys[j++]))T[key] = S[key];
+ }
+ return T;
+ } : Object.assign;
+
+/***/ },
+/* 42 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.3.10 Object.is(value1, value2)
+ var $export = __webpack_require__(3);
+ $export($export.S, 'Object', {is: __webpack_require__(43)});
+
+/***/ },
+/* 43 */
+/***/ function(module, exports) {
+
+ // 7.2.9 SameValue(x, y)
+ module.exports = Object.is || function is(x, y){
+ return x === y ? x !== 0 || 1 / x === 1 / y : x != x && y != y;
+ };
+
+/***/ },
+/* 44 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.3.19 Object.setPrototypeOf(O, proto)
+ var $export = __webpack_require__(3);
+ $export($export.S, 'Object', {setPrototypeOf: __webpack_require__(45).set});
+
+/***/ },
+/* 45 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // Works with __proto__ only. Old v8 can't work with null proto objects.
+ /* eslint-disable no-proto */
+ var getDesc = __webpack_require__(2).getDesc
+ , isObject = __webpack_require__(13)
+ , anObject = __webpack_require__(17);
+ var check = function(O, proto){
+ anObject(O);
+ if(!isObject(proto) && proto !== null)throw TypeError(proto + ": can't set as prototype!");
+ };
+ module.exports = {
+ set: Object.setPrototypeOf || ('__proto__' in {} ? // eslint-disable-line
+ function(test, buggy, set){
+ try {
+ set = __webpack_require__(6)(Function.call, getDesc(Object.prototype, '__proto__').set, 2);
+ set(test, []);
+ buggy = !(test instanceof Array);
+ } catch(e){ buggy = true; }
+ return function setPrototypeOf(O, proto){
+ check(O, proto);
+ if(buggy)O.__proto__ = proto;
+ else set(O, proto);
+ return O;
+ };
+ }({}, false) : undefined),
+ check: check
+ };
+
+/***/ },
+/* 46 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.5 Object.freeze(O)
+ var isObject = __webpack_require__(13);
+
+ __webpack_require__(47)('freeze', function($freeze){
+ return function freeze(it){
+ return $freeze && isObject(it) ? $freeze(it) : it;
+ };
+ });
+
+/***/ },
+/* 47 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // most Object methods by ES6 should accept primitives
+ var $export = __webpack_require__(3)
+ , core = __webpack_require__(5)
+ , fails = __webpack_require__(9);
+ module.exports = function(KEY, exec){
+ var fn = (core.Object || {})[KEY] || Object[KEY]
+ , exp = {};
+ exp[KEY] = exec(fn);
+ $export($export.S + $export.F * fails(function(){ fn(1); }), 'Object', exp);
+ };
+
+/***/ },
+/* 48 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.17 Object.seal(O)
+ var isObject = __webpack_require__(13);
+
+ __webpack_require__(47)('seal', function($seal){
+ return function seal(it){
+ return $seal && isObject(it) ? $seal(it) : it;
+ };
+ });
+
+/***/ },
+/* 49 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.15 Object.preventExtensions(O)
+ var isObject = __webpack_require__(13);
+
+ __webpack_require__(47)('preventExtensions', function($preventExtensions){
+ return function preventExtensions(it){
+ return $preventExtensions && isObject(it) ? $preventExtensions(it) : it;
+ };
+ });
+
+/***/ },
+/* 50 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.12 Object.isFrozen(O)
+ var isObject = __webpack_require__(13);
+
+ __webpack_require__(47)('isFrozen', function($isFrozen){
+ return function isFrozen(it){
+ return isObject(it) ? $isFrozen ? $isFrozen(it) : false : true;
+ };
+ });
+
+/***/ },
+/* 51 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.13 Object.isSealed(O)
+ var isObject = __webpack_require__(13);
+
+ __webpack_require__(47)('isSealed', function($isSealed){
+ return function isSealed(it){
+ return isObject(it) ? $isSealed ? $isSealed(it) : false : true;
+ };
+ });
+
+/***/ },
+/* 52 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.11 Object.isExtensible(O)
+ var isObject = __webpack_require__(13);
+
+ __webpack_require__(47)('isExtensible', function($isExtensible){
+ return function isExtensible(it){
+ return isObject(it) ? $isExtensible ? $isExtensible(it) : true : false;
+ };
+ });
+
+/***/ },
+/* 53 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.6 Object.getOwnPropertyDescriptor(O, P)
+ var toIObject = __webpack_require__(20);
+
+ __webpack_require__(47)('getOwnPropertyDescriptor', function($getOwnPropertyDescriptor){
+ return function getOwnPropertyDescriptor(it, key){
+ return $getOwnPropertyDescriptor(toIObject(it), key);
+ };
+ });
+
+/***/ },
+/* 54 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.9 Object.getPrototypeOf(O)
+ var toObject = __webpack_require__(18);
+
+ __webpack_require__(47)('getPrototypeOf', function($getPrototypeOf){
+ return function getPrototypeOf(it){
+ return $getPrototypeOf(toObject(it));
+ };
+ });
+
+/***/ },
+/* 55 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.14 Object.keys(O)
+ var toObject = __webpack_require__(18);
+
+ __webpack_require__(47)('keys', function($keys){
+ return function keys(it){
+ return $keys(toObject(it));
+ };
+ });
+
+/***/ },
+/* 56 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.7 Object.getOwnPropertyNames(O)
+ __webpack_require__(47)('getOwnPropertyNames', function(){
+ return __webpack_require__(37).get;
+ });
+
+/***/ },
+/* 57 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , isObject = __webpack_require__(13)
+ , HAS_INSTANCE = __webpack_require__(29)('hasInstance')
+ , FunctionProto = Function.prototype;
+ // 19.2.3.6 Function.prototype[@@hasInstance](V)
+ if(!(HAS_INSTANCE in FunctionProto))$.setDesc(FunctionProto, HAS_INSTANCE, {value: function(O){
+ if(typeof this != 'function' || !isObject(O))return false;
+ if(!isObject(this.prototype))return O instanceof this;
+ // for environment w/o native `@@hasInstance` logic enough `instanceof`, but add this:
+ while(O = $.getProto(O))if(this.prototype === O)return true;
+ return false;
+ }});
+
+/***/ },
+/* 58 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.1 Number.EPSILON
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {EPSILON: Math.pow(2, -52)});
+
+/***/ },
+/* 59 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.2 Number.isFinite(number)
+ var $export = __webpack_require__(3)
+ , _isFinite = __webpack_require__(4).isFinite;
+
+ $export($export.S, 'Number', {
+ isFinite: function isFinite(it){
+ return typeof it == 'number' && _isFinite(it);
+ }
+ });
+
+/***/ },
+/* 60 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.3 Number.isInteger(number)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {isInteger: __webpack_require__(61)});
+
+/***/ },
+/* 61 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.3 Number.isInteger(number)
+ var isObject = __webpack_require__(13)
+ , floor = Math.floor;
+ module.exports = function isInteger(it){
+ return !isObject(it) && isFinite(it) && floor(it) === it;
+ };
+
+/***/ },
+/* 62 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.4 Number.isNaN(number)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {
+ isNaN: function isNaN(number){
+ return number != number;
+ }
+ });
+
+/***/ },
+/* 63 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.5 Number.isSafeInteger(number)
+ var $export = __webpack_require__(3)
+ , isInteger = __webpack_require__(61)
+ , abs = Math.abs;
+
+ $export($export.S, 'Number', {
+ isSafeInteger: function isSafeInteger(number){
+ return isInteger(number) && abs(number) <= 0x1fffffffffffff;
+ }
+ });
+
+/***/ },
+/* 64 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.6 Number.MAX_SAFE_INTEGER
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {MAX_SAFE_INTEGER: 0x1fffffffffffff});
+
+/***/ },
+/* 65 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.10 Number.MIN_SAFE_INTEGER
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {MIN_SAFE_INTEGER: -0x1fffffffffffff});
+
+/***/ },
+/* 66 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.12 Number.parseFloat(string)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {parseFloat: parseFloat});
+
+/***/ },
+/* 67 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.13 Number.parseInt(string, radix)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {parseInt: parseInt});
+
+/***/ },
+/* 68 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.3 Math.acosh(x)
+ var $export = __webpack_require__(3)
+ , log1p = __webpack_require__(69)
+ , sqrt = Math.sqrt
+ , $acosh = Math.acosh;
+
+ // V8 bug https://code.google.com/p/v8/issues/detail?id=3509
+ $export($export.S + $export.F * !($acosh && Math.floor($acosh(Number.MAX_VALUE)) == 710), 'Math', {
+ acosh: function acosh(x){
+ return (x = +x) < 1 ? NaN : x > 94906265.62425156
+ ? Math.log(x) + Math.LN2
+ : log1p(x - 1 + sqrt(x - 1) * sqrt(x + 1));
+ }
+ });
+
+/***/ },
+/* 69 */
+/***/ function(module, exports) {
+
+ // 20.2.2.20 Math.log1p(x)
+ module.exports = Math.log1p || function log1p(x){
+ return (x = +x) > -1e-8 && x < 1e-8 ? x - x * x / 2 : Math.log(1 + x);
+ };
+
+/***/ },
+/* 70 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.5 Math.asinh(x)
+ var $export = __webpack_require__(3);
+
+ function asinh(x){
+ return !isFinite(x = +x) || x == 0 ? x : x < 0 ? -asinh(-x) : Math.log(x + Math.sqrt(x * x + 1));
+ }
+
+ $export($export.S, 'Math', {asinh: asinh});
+
+/***/ },
+/* 71 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.7 Math.atanh(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {
+ atanh: function atanh(x){
+ return (x = +x) == 0 ? x : Math.log((1 + x) / (1 - x)) / 2;
+ }
+ });
+
+/***/ },
+/* 72 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.9 Math.cbrt(x)
+ var $export = __webpack_require__(3)
+ , sign = __webpack_require__(73);
+
+ $export($export.S, 'Math', {
+ cbrt: function cbrt(x){
+ return sign(x = +x) * Math.pow(Math.abs(x), 1 / 3);
+ }
+ });
+
+/***/ },
+/* 73 */
+/***/ function(module, exports) {
+
+ // 20.2.2.28 Math.sign(x)
+ module.exports = Math.sign || function sign(x){
+ return (x = +x) == 0 || x != x ? x : x < 0 ? -1 : 1;
+ };
+
+/***/ },
+/* 74 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.11 Math.clz32(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {
+ clz32: function clz32(x){
+ return (x >>>= 0) ? 31 - Math.floor(Math.log(x + 0.5) * Math.LOG2E) : 32;
+ }
+ });
+
+/***/ },
+/* 75 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.12 Math.cosh(x)
+ var $export = __webpack_require__(3)
+ , exp = Math.exp;
+
+ $export($export.S, 'Math', {
+ cosh: function cosh(x){
+ return (exp(x = +x) + exp(-x)) / 2;
+ }
+ });
+
+/***/ },
+/* 76 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.14 Math.expm1(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {expm1: __webpack_require__(77)});
+
+/***/ },
+/* 77 */
+/***/ function(module, exports) {
+
+ // 20.2.2.14 Math.expm1(x)
+ module.exports = Math.expm1 || function expm1(x){
+ return (x = +x) == 0 ? x : x > -1e-6 && x < 1e-6 ? x + x * x / 2 : Math.exp(x) - 1;
+ };
+
+/***/ },
+/* 78 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.16 Math.fround(x)
+ var $export = __webpack_require__(3)
+ , sign = __webpack_require__(73)
+ , pow = Math.pow
+ , EPSILON = pow(2, -52)
+ , EPSILON32 = pow(2, -23)
+ , MAX32 = pow(2, 127) * (2 - EPSILON32)
+ , MIN32 = pow(2, -126);
+
+ var roundTiesToEven = function(n){
+ return n + 1 / EPSILON - 1 / EPSILON;
+ };
+
+
+ $export($export.S, 'Math', {
+ fround: function fround(x){
+ var $abs = Math.abs(x)
+ , $sign = sign(x)
+ , a, result;
+ if($abs < MIN32)return $sign * roundTiesToEven($abs / MIN32 / EPSILON32) * MIN32 * EPSILON32;
+ a = (1 + EPSILON32 / EPSILON) * $abs;
+ result = a - (a - $abs);
+ if(result > MAX32 || result != result)return $sign * Infinity;
+ return $sign * result;
+ }
+ });
+
+/***/ },
+/* 79 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.17 Math.hypot([value1[, value2[, … ]]])
+ var $export = __webpack_require__(3)
+ , abs = Math.abs;
+
+ $export($export.S, 'Math', {
+ hypot: function hypot(value1, value2){ // eslint-disable-line no-unused-vars
+ var sum = 0
+ , i = 0
+ , $$ = arguments
+ , $$len = $$.length
+ , larg = 0
+ , arg, div;
+ while(i < $$len){
+ arg = abs($$[i++]);
+ if(larg < arg){
+ div = larg / arg;
+ sum = sum * div * div + 1;
+ larg = arg;
+ } else if(arg > 0){
+ div = arg / larg;
+ sum += div * div;
+ } else sum += arg;
+ }
+ return larg === Infinity ? Infinity : larg * Math.sqrt(sum);
+ }
+ });
+
+/***/ },
+/* 80 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.18 Math.imul(x, y)
+ var $export = __webpack_require__(3)
+ , $imul = Math.imul;
+
+ // some WebKit versions fails with big numbers, some has wrong arity
+ $export($export.S + $export.F * __webpack_require__(9)(function(){
+ return $imul(0xffffffff, 5) != -5 || $imul.length != 2;
+ }), 'Math', {
+ imul: function imul(x, y){
+ var UINT16 = 0xffff
+ , xn = +x
+ , yn = +y
+ , xl = UINT16 & xn
+ , yl = UINT16 & yn;
+ return 0 | xl * yl + ((UINT16 & xn >>> 16) * yl + xl * (UINT16 & yn >>> 16) << 16 >>> 0);
+ }
+ });
+
+/***/ },
+/* 81 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.21 Math.log10(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {
+ log10: function log10(x){
+ return Math.log(x) / Math.LN10;
+ }
+ });
+
+/***/ },
+/* 82 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.20 Math.log1p(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {log1p: __webpack_require__(69)});
+
+/***/ },
+/* 83 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.22 Math.log2(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {
+ log2: function log2(x){
+ return Math.log(x) / Math.LN2;
+ }
+ });
+
+/***/ },
+/* 84 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.28 Math.sign(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {sign: __webpack_require__(73)});
+
+/***/ },
+/* 85 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.30 Math.sinh(x)
+ var $export = __webpack_require__(3)
+ , expm1 = __webpack_require__(77)
+ , exp = Math.exp;
+
+ // V8 near Chromium 38 has a problem with very small numbers
+ $export($export.S + $export.F * __webpack_require__(9)(function(){
+ return !Math.sinh(-2e-17) != -2e-17;
+ }), 'Math', {
+ sinh: function sinh(x){
+ return Math.abs(x = +x) < 1
+ ? (expm1(x) - expm1(-x)) / 2
+ : (exp(x - 1) - exp(-x - 1)) * (Math.E / 2);
+ }
+ });
+
+/***/ },
+/* 86 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.33 Math.tanh(x)
+ var $export = __webpack_require__(3)
+ , expm1 = __webpack_require__(77)
+ , exp = Math.exp;
+
+ $export($export.S, 'Math', {
+ tanh: function tanh(x){
+ var a = expm1(x = +x)
+ , b = expm1(-x);
+ return a == Infinity ? 1 : b == Infinity ? -1 : (a - b) / (exp(x) + exp(-x));
+ }
+ });
+
+/***/ },
+/* 87 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.34 Math.trunc(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {
+ trunc: function trunc(it){
+ return (it > 0 ? Math.floor : Math.ceil)(it);
+ }
+ });
+
+/***/ },
+/* 88 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3)
+ , toIndex = __webpack_require__(23)
+ , fromCharCode = String.fromCharCode
+ , $fromCodePoint = String.fromCodePoint;
+
+ // length should be 1, old FF problem
+ $export($export.S + $export.F * (!!$fromCodePoint && $fromCodePoint.length != 1), 'String', {
+ // 21.1.2.2 String.fromCodePoint(...codePoints)
+ fromCodePoint: function fromCodePoint(x){ // eslint-disable-line no-unused-vars
+ var res = []
+ , $$ = arguments
+ , $$len = $$.length
+ , i = 0
+ , code;
+ while($$len > i){
+ code = +$$[i++];
+ if(toIndex(code, 0x10ffff) !== code)throw RangeError(code + ' is not a valid code point');
+ res.push(code < 0x10000
+ ? fromCharCode(code)
+ : fromCharCode(((code -= 0x10000) >> 10) + 0xd800, code % 0x400 + 0xdc00)
+ );
+ } return res.join('');
+ }
+ });
+
+/***/ },
+/* 89 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3)
+ , toIObject = __webpack_require__(20)
+ , toLength = __webpack_require__(24);
+
+ $export($export.S, 'String', {
+ // 21.1.2.4 String.raw(callSite, ...substitutions)
+ raw: function raw(callSite){
+ var tpl = toIObject(callSite.raw)
+ , len = toLength(tpl.length)
+ , $$ = arguments
+ , $$len = $$.length
+ , res = []
+ , i = 0;
+ while(len > i){
+ res.push(String(tpl[i++]));
+ if(i < $$len)res.push(String($$[i]));
+ } return res.join('');
+ }
+ });
+
+/***/ },
+/* 90 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // 21.1.3.25 String.prototype.trim()
+ __webpack_require__(91)('trim', function($trim){
+ return function trim(){
+ return $trim(this, 3);
+ };
+ });
+
+/***/ },
+/* 91 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3)
+ , defined = __webpack_require__(19)
+ , fails = __webpack_require__(9)
+ , spaces = '\x09\x0A\x0B\x0C\x0D\x20\xA0\u1680\u180E\u2000\u2001\u2002\u2003' +
+ '\u2004\u2005\u2006\u2007\u2008\u2009\u200A\u202F\u205F\u3000\u2028\u2029\uFEFF'
+ , space = '[' + spaces + ']'
+ , non = '\u200b\u0085'
+ , ltrim = RegExp('^' + space + space + '*')
+ , rtrim = RegExp(space + space + '*$');
+
+ var exporter = function(KEY, exec){
+ var exp = {};
+ exp[KEY] = exec(trim);
+ $export($export.P + $export.F * fails(function(){
+ return !!spaces[KEY]() || non[KEY]() != non;
+ }), 'String', exp);
+ };
+
+ // 1 -> String#trimLeft
+ // 2 -> String#trimRight
+ // 3 -> String#trim
+ var trim = exporter.trim = function(string, TYPE){
+ string = String(defined(string));
+ if(TYPE & 1)string = string.replace(ltrim, '');
+ if(TYPE & 2)string = string.replace(rtrim, '');
+ return string;
+ };
+
+ module.exports = exporter;
+
+/***/ },
+/* 92 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , $at = __webpack_require__(93)(false);
+ $export($export.P, 'String', {
+ // 21.1.3.3 String.prototype.codePointAt(pos)
+ codePointAt: function codePointAt(pos){
+ return $at(this, pos);
+ }
+ });
+
+/***/ },
+/* 93 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var toInteger = __webpack_require__(22)
+ , defined = __webpack_require__(19);
+ // true -> String#at
+ // false -> String#codePointAt
+ module.exports = function(TO_STRING){
+ return function(that, pos){
+ var s = String(defined(that))
+ , i = toInteger(pos)
+ , l = s.length
+ , a, b;
+ if(i < 0 || i >= l)return TO_STRING ? '' : undefined;
+ a = s.charCodeAt(i);
+ return a < 0xd800 || a > 0xdbff || i + 1 === l || (b = s.charCodeAt(i + 1)) < 0xdc00 || b > 0xdfff
+ ? TO_STRING ? s.charAt(i) : a
+ : TO_STRING ? s.slice(i, i + 2) : (a - 0xd800 << 10) + (b - 0xdc00) + 0x10000;
+ };
+ };
+
+/***/ },
+/* 94 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 21.1.3.6 String.prototype.endsWith(searchString [, endPosition])
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , toLength = __webpack_require__(24)
+ , context = __webpack_require__(95)
+ , ENDS_WITH = 'endsWith'
+ , $endsWith = ''[ENDS_WITH];
+
+ $export($export.P + $export.F * __webpack_require__(97)(ENDS_WITH), 'String', {
+ endsWith: function endsWith(searchString /*, endPosition = @length */){
+ var that = context(this, searchString, ENDS_WITH)
+ , $$ = arguments
+ , endPosition = $$.length > 1 ? $$[1] : undefined
+ , len = toLength(that.length)
+ , end = endPosition === undefined ? len : Math.min(toLength(endPosition), len)
+ , search = String(searchString);
+ return $endsWith
+ ? $endsWith.call(that, search, end)
+ : that.slice(end - search.length, end) === search;
+ }
+ });
+
+/***/ },
+/* 95 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // helper for String#{startsWith, endsWith, includes}
+ var isRegExp = __webpack_require__(96)
+ , defined = __webpack_require__(19);
+
+ module.exports = function(that, searchString, NAME){
+ if(isRegExp(searchString))throw TypeError('String#' + NAME + " doesn't accept regex!");
+ return String(defined(that));
+ };
+
+/***/ },
+/* 96 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 7.2.8 IsRegExp(argument)
+ var isObject = __webpack_require__(13)
+ , cof = __webpack_require__(15)
+ , MATCH = __webpack_require__(29)('match');
+ module.exports = function(it){
+ var isRegExp;
+ return isObject(it) && ((isRegExp = it[MATCH]) !== undefined ? !!isRegExp : cof(it) == 'RegExp');
+ };
+
+/***/ },
+/* 97 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var MATCH = __webpack_require__(29)('match');
+ module.exports = function(KEY){
+ var re = /./;
+ try {
+ '/./'[KEY](re);
+ } catch(e){
+ try {
+ re[MATCH] = false;
+ return !'/./'[KEY](re);
+ } catch(f){ /* empty */ }
+ } return true;
+ };
+
+/***/ },
+/* 98 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 21.1.3.7 String.prototype.includes(searchString, position = 0)
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , context = __webpack_require__(95)
+ , INCLUDES = 'includes';
+
+ $export($export.P + $export.F * __webpack_require__(97)(INCLUDES), 'String', {
+ includes: function includes(searchString /*, position = 0 */){
+ return !!~context(this, searchString, INCLUDES)
+ .indexOf(searchString, arguments.length > 1 ? arguments[1] : undefined);
+ }
+ });
+
+/***/ },
+/* 99 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3);
+
+ $export($export.P, 'String', {
+ // 21.1.3.13 String.prototype.repeat(count)
+ repeat: __webpack_require__(100)
+ });
+
+/***/ },
+/* 100 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var toInteger = __webpack_require__(22)
+ , defined = __webpack_require__(19);
+
+ module.exports = function repeat(count){
+ var str = String(defined(this))
+ , res = ''
+ , n = toInteger(count);
+ if(n < 0 || n == Infinity)throw RangeError("Count can't be negative");
+ for(;n > 0; (n >>>= 1) && (str += str))if(n & 1)res += str;
+ return res;
+ };
+
+/***/ },
+/* 101 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 21.1.3.18 String.prototype.startsWith(searchString [, position ])
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , toLength = __webpack_require__(24)
+ , context = __webpack_require__(95)
+ , STARTS_WITH = 'startsWith'
+ , $startsWith = ''[STARTS_WITH];
+
+ $export($export.P + $export.F * __webpack_require__(97)(STARTS_WITH), 'String', {
+ startsWith: function startsWith(searchString /*, position = 0 */){
+ var that = context(this, searchString, STARTS_WITH)
+ , $$ = arguments
+ , index = toLength(Math.min($$.length > 1 ? $$[1] : undefined, that.length))
+ , search = String(searchString);
+ return $startsWith
+ ? $startsWith.call(that, search, index)
+ : that.slice(index, index + search.length) === search;
+ }
+ });
+
+/***/ },
+/* 102 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $at = __webpack_require__(93)(true);
+
+ // 21.1.3.27 String.prototype[@@iterator]()
+ __webpack_require__(103)(String, 'String', function(iterated){
+ this._t = String(iterated); // target
+ this._i = 0; // next index
+ // 21.1.5.2.1 %StringIteratorPrototype%.next()
+ }, function(){
+ var O = this._t
+ , index = this._i
+ , point;
+ if(index >= O.length)return {value: undefined, done: true};
+ point = $at(O, index);
+ this._i += point.length;
+ return {value: point, done: false};
+ });
+
+/***/ },
+/* 103 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var LIBRARY = __webpack_require__(39)
+ , $export = __webpack_require__(3)
+ , redefine = __webpack_require__(33)
+ , hide = __webpack_require__(34)
+ , has = __webpack_require__(14)
+ , Iterators = __webpack_require__(104)
+ , $iterCreate = __webpack_require__(105)
+ , setToStringTag = __webpack_require__(35)
+ , getProto = __webpack_require__(2).getProto
+ , ITERATOR = __webpack_require__(29)('iterator')
+ , BUGGY = !([].keys && 'next' in [].keys()) // Safari has buggy iterators w/o `next`
+ , FF_ITERATOR = '@@iterator'
+ , KEYS = 'keys'
+ , VALUES = 'values';
+
+ var returnThis = function(){ return this; };
+
+ module.exports = function(Base, NAME, Constructor, next, DEFAULT, IS_SET, FORCED){
+ $iterCreate(Constructor, NAME, next);
+ var getMethod = function(kind){
+ if(!BUGGY && kind in proto)return proto[kind];
+ switch(kind){
+ case KEYS: return function keys(){ return new Constructor(this, kind); };
+ case VALUES: return function values(){ return new Constructor(this, kind); };
+ } return function entries(){ return new Constructor(this, kind); };
+ };
+ var TAG = NAME + ' Iterator'
+ , DEF_VALUES = DEFAULT == VALUES
+ , VALUES_BUG = false
+ , proto = Base.prototype
+ , $native = proto[ITERATOR] || proto[FF_ITERATOR] || DEFAULT && proto[DEFAULT]
+ , $default = $native || getMethod(DEFAULT)
+ , methods, key;
+ // Fix native
+ if($native){
+ var IteratorPrototype = getProto($default.call(new Base));
+ // Set @@toStringTag to native iterators
+ setToStringTag(IteratorPrototype, TAG, true);
+ // FF fix
+ if(!LIBRARY && has(proto, FF_ITERATOR))hide(IteratorPrototype, ITERATOR, returnThis);
+ // fix Array#{values, @@iterator}.name in V8 / FF
+ if(DEF_VALUES && $native.name !== VALUES){
+ VALUES_BUG = true;
+ $default = function values(){ return $native.call(this); };
+ }
+ }
+ // Define iterator
+ if((!LIBRARY || FORCED) && (BUGGY || VALUES_BUG || !proto[ITERATOR])){
+ hide(proto, ITERATOR, $default);
+ }
+ // Plug for library
+ Iterators[NAME] = $default;
+ Iterators[TAG] = returnThis;
+ if(DEFAULT){
+ methods = {
+ values: DEF_VALUES ? $default : getMethod(VALUES),
+ keys: IS_SET ? $default : getMethod(KEYS),
+ entries: !DEF_VALUES ? $default : getMethod('entries')
+ };
+ if(FORCED)for(key in methods){
+ if(!(key in proto))redefine(proto, key, methods[key]);
+ } else $export($export.P + $export.F * (BUGGY || VALUES_BUG), NAME, methods);
+ }
+ return methods;
+ };
+
+/***/ },
+/* 104 */
+/***/ function(module, exports) {
+
+ module.exports = {};
+
+/***/ },
+/* 105 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , descriptor = __webpack_require__(10)
+ , setToStringTag = __webpack_require__(35)
+ , IteratorPrototype = {};
+
+ // 25.1.2.1.1 %IteratorPrototype%[@@iterator]()
+ __webpack_require__(34)(IteratorPrototype, __webpack_require__(29)('iterator'), function(){ return this; });
+
+ module.exports = function(Constructor, NAME, next){
+ Constructor.prototype = $.create(IteratorPrototype, {next: descriptor(1, next)});
+ setToStringTag(Constructor, NAME + ' Iterator');
+ };
+
+/***/ },
+/* 106 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var ctx = __webpack_require__(6)
+ , $export = __webpack_require__(3)
+ , toObject = __webpack_require__(18)
+ , call = __webpack_require__(107)
+ , isArrayIter = __webpack_require__(108)
+ , toLength = __webpack_require__(24)
+ , getIterFn = __webpack_require__(109);
+ $export($export.S + $export.F * !__webpack_require__(111)(function(iter){ Array.from(iter); }), 'Array', {
+ // 22.1.2.1 Array.from(arrayLike, mapfn = undefined, thisArg = undefined)
+ from: function from(arrayLike/*, mapfn = undefined, thisArg = undefined*/){
+ var O = toObject(arrayLike)
+ , C = typeof this == 'function' ? this : Array
+ , $$ = arguments
+ , $$len = $$.length
+ , mapfn = $$len > 1 ? $$[1] : undefined
+ , mapping = mapfn !== undefined
+ , index = 0
+ , iterFn = getIterFn(O)
+ , length, result, step, iterator;
+ if(mapping)mapfn = ctx(mapfn, $$len > 2 ? $$[2] : undefined, 2);
+ // if object isn't iterable or it's array with default iterator - use simple case
+ if(iterFn != undefined && !(C == Array && isArrayIter(iterFn))){
+ for(iterator = iterFn.call(O), result = new C; !(step = iterator.next()).done; index++){
+ result[index] = mapping ? call(iterator, mapfn, [step.value, index], true) : step.value;
+ }
+ } else {
+ length = toLength(O.length);
+ for(result = new C(length); length > index; index++){
+ result[index] = mapping ? mapfn(O[index], index) : O[index];
+ }
+ }
+ result.length = index;
+ return result;
+ }
+ });
+
+
+/***/ },
+/* 107 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // call something on iterator step with safe closing on error
+ var anObject = __webpack_require__(17);
+ module.exports = function(iterator, fn, value, entries){
+ try {
+ return entries ? fn(anObject(value)[0], value[1]) : fn(value);
+ // 7.4.6 IteratorClose(iterator, completion)
+ } catch(e){
+ var ret = iterator['return'];
+ if(ret !== undefined)anObject(ret.call(iterator));
+ throw e;
+ }
+ };
+
+/***/ },
+/* 108 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // check on default Array iterator
+ var Iterators = __webpack_require__(104)
+ , ITERATOR = __webpack_require__(29)('iterator')
+ , ArrayProto = Array.prototype;
+
+ module.exports = function(it){
+ return it !== undefined && (Iterators.Array === it || ArrayProto[ITERATOR] === it);
+ };
+
+/***/ },
+/* 109 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var classof = __webpack_require__(110)
+ , ITERATOR = __webpack_require__(29)('iterator')
+ , Iterators = __webpack_require__(104);
+ module.exports = __webpack_require__(5).getIteratorMethod = function(it){
+ if(it != undefined)return it[ITERATOR]
+ || it['@@iterator']
+ || Iterators[classof(it)];
+ };
+
+/***/ },
+/* 110 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // getting tag from 19.1.3.6 Object.prototype.toString()
+ var cof = __webpack_require__(15)
+ , TAG = __webpack_require__(29)('toStringTag')
+ // ES3 wrong here
+ , ARG = cof(function(){ return arguments; }()) == 'Arguments';
+
+ module.exports = function(it){
+ var O, T, B;
+ return it === undefined ? 'Undefined' : it === null ? 'Null'
+ // @@toStringTag case
+ : typeof (T = (O = Object(it))[TAG]) == 'string' ? T
+ // builtinTag case
+ : ARG ? cof(O)
+ // ES3 arguments fallback
+ : (B = cof(O)) == 'Object' && typeof O.callee == 'function' ? 'Arguments' : B;
+ };
+
+/***/ },
+/* 111 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var ITERATOR = __webpack_require__(29)('iterator')
+ , SAFE_CLOSING = false;
+
+ try {
+ var riter = [7][ITERATOR]();
+ riter['return'] = function(){ SAFE_CLOSING = true; };
+ Array.from(riter, function(){ throw 2; });
+ } catch(e){ /* empty */ }
+
+ module.exports = function(exec, skipClosing){
+ if(!skipClosing && !SAFE_CLOSING)return false;
+ var safe = false;
+ try {
+ var arr = [7]
+ , iter = arr[ITERATOR]();
+ iter.next = function(){ return {done: safe = true}; };
+ arr[ITERATOR] = function(){ return iter; };
+ exec(arr);
+ } catch(e){ /* empty */ }
+ return safe;
+ };
+
+/***/ },
+/* 112 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $export = __webpack_require__(3);
+
+ // WebKit Array.of isn't generic
+ $export($export.S + $export.F * __webpack_require__(9)(function(){
+ function F(){}
+ return !(Array.of.call(F) instanceof F);
+ }), 'Array', {
+ // 22.1.2.3 Array.of( ...items)
+ of: function of(/* ...args */){
+ var index = 0
+ , $$ = arguments
+ , $$len = $$.length
+ , result = new (typeof this == 'function' ? this : Array)($$len);
+ while($$len > index)result[index] = $$[index++];
+ result.length = $$len;
+ return result;
+ }
+ });
+
+/***/ },
+/* 113 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var addToUnscopables = __webpack_require__(114)
+ , step = __webpack_require__(115)
+ , Iterators = __webpack_require__(104)
+ , toIObject = __webpack_require__(20);
+
+ // 22.1.3.4 Array.prototype.entries()
+ // 22.1.3.13 Array.prototype.keys()
+ // 22.1.3.29 Array.prototype.values()
+ // 22.1.3.30 Array.prototype[@@iterator]()
+ module.exports = __webpack_require__(103)(Array, 'Array', function(iterated, kind){
+ this._t = toIObject(iterated); // target
+ this._i = 0; // next index
+ this._k = kind; // kind
+ // 22.1.5.2.1 %ArrayIteratorPrototype%.next()
+ }, function(){
+ var O = this._t
+ , kind = this._k
+ , index = this._i++;
+ if(!O || index >= O.length){
+ this._t = undefined;
+ return step(1);
+ }
+ if(kind == 'keys' )return step(0, index);
+ if(kind == 'values')return step(0, O[index]);
+ return step(0, [index, O[index]]);
+ }, 'values');
+
+ // argumentsList[@@iterator] is %ArrayProto_values% (9.4.4.6, 9.4.4.7)
+ Iterators.Arguments = Iterators.Array;
+
+ addToUnscopables('keys');
+ addToUnscopables('values');
+ addToUnscopables('entries');
+
+/***/ },
+/* 114 */
+/***/ function(module, exports) {
+
+ module.exports = function(){ /* empty */ };
+
+/***/ },
+/* 115 */
+/***/ function(module, exports) {
+
+ module.exports = function(done, value){
+ return {value: value, done: !!done};
+ };
+
+/***/ },
+/* 116 */
+/***/ function(module, exports, __webpack_require__) {
+
+ __webpack_require__(117)('Array');
+
+/***/ },
+/* 117 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var core = __webpack_require__(5)
+ , $ = __webpack_require__(2)
+ , DESCRIPTORS = __webpack_require__(8)
+ , SPECIES = __webpack_require__(29)('species');
+
+ module.exports = function(KEY){
+ var C = core[KEY];
+ if(DESCRIPTORS && C && !C[SPECIES])$.setDesc(C, SPECIES, {
+ configurable: true,
+ get: function(){ return this; }
+ });
+ };
+
+/***/ },
+/* 118 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 22.1.3.3 Array.prototype.copyWithin(target, start, end = this.length)
+ var $export = __webpack_require__(3);
+
+ $export($export.P, 'Array', {copyWithin: __webpack_require__(119)});
+
+ __webpack_require__(114)('copyWithin');
+
+/***/ },
+/* 119 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 22.1.3.3 Array.prototype.copyWithin(target, start, end = this.length)
+ 'use strict';
+ var toObject = __webpack_require__(18)
+ , toIndex = __webpack_require__(23)
+ , toLength = __webpack_require__(24);
+
+ module.exports = [].copyWithin || function copyWithin(target/*= 0*/, start/*= 0, end = @length*/){
+ var O = toObject(this)
+ , len = toLength(O.length)
+ , to = toIndex(target, len)
+ , from = toIndex(start, len)
+ , $$ = arguments
+ , end = $$.length > 2 ? $$[2] : undefined
+ , count = Math.min((end === undefined ? len : toIndex(end, len)) - from, len - to)
+ , inc = 1;
+ if(from < to && to < from + count){
+ inc = -1;
+ from += count - 1;
+ to += count - 1;
+ }
+ while(count-- > 0){
+ if(from in O)O[to] = O[from];
+ else delete O[to];
+ to += inc;
+ from += inc;
+ } return O;
+ };
+
+/***/ },
+/* 120 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 22.1.3.6 Array.prototype.fill(value, start = 0, end = this.length)
+ var $export = __webpack_require__(3);
+
+ $export($export.P, 'Array', {fill: __webpack_require__(121)});
+
+ __webpack_require__(114)('fill');
+
+/***/ },
+/* 121 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 22.1.3.6 Array.prototype.fill(value, start = 0, end = this.length)
+ 'use strict';
+ var toObject = __webpack_require__(18)
+ , toIndex = __webpack_require__(23)
+ , toLength = __webpack_require__(24);
+ module.exports = [].fill || function fill(value /*, start = 0, end = @length */){
+ var O = toObject(this)
+ , length = toLength(O.length)
+ , $$ = arguments
+ , $$len = $$.length
+ , index = toIndex($$len > 1 ? $$[1] : undefined, length)
+ , end = $$len > 2 ? $$[2] : undefined
+ , endPos = end === undefined ? length : toIndex(end, length);
+ while(endPos > index)O[index++] = value;
+ return O;
+ };
+
+/***/ },
+/* 122 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // 22.1.3.8 Array.prototype.find(predicate, thisArg = undefined)
+ var $export = __webpack_require__(3)
+ , $find = __webpack_require__(26)(5)
+ , KEY = 'find'
+ , forced = true;
+ // Shouldn't skip holes
+ if(KEY in [])Array(1)[KEY](function(){ forced = false; });
+ $export($export.P + $export.F * forced, 'Array', {
+ find: function find(callbackfn/*, that = undefined */){
+ return $find(this, callbackfn, arguments.length > 1 ? arguments[1] : undefined);
+ }
+ });
+ __webpack_require__(114)(KEY);
+
+/***/ },
+/* 123 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // 22.1.3.9 Array.prototype.findIndex(predicate, thisArg = undefined)
+ var $export = __webpack_require__(3)
+ , $find = __webpack_require__(26)(6)
+ , KEY = 'findIndex'
+ , forced = true;
+ // Shouldn't skip holes
+ if(KEY in [])Array(1)[KEY](function(){ forced = false; });
+ $export($export.P + $export.F * forced, 'Array', {
+ findIndex: function findIndex(callbackfn/*, that = undefined */){
+ return $find(this, callbackfn, arguments.length > 1 ? arguments[1] : undefined);
+ }
+ });
+ __webpack_require__(114)(KEY);
+
+/***/ },
+/* 124 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , LIBRARY = __webpack_require__(39)
+ , global = __webpack_require__(4)
+ , ctx = __webpack_require__(6)
+ , classof = __webpack_require__(110)
+ , $export = __webpack_require__(3)
+ , isObject = __webpack_require__(13)
+ , anObject = __webpack_require__(17)
+ , aFunction = __webpack_require__(7)
+ , strictNew = __webpack_require__(125)
+ , forOf = __webpack_require__(126)
+ , setProto = __webpack_require__(45).set
+ , same = __webpack_require__(43)
+ , SPECIES = __webpack_require__(29)('species')
+ , speciesConstructor = __webpack_require__(127)
+ , asap = __webpack_require__(128)
+ , PROMISE = 'Promise'
+ , process = global.process
+ , isNode = classof(process) == 'process'
+ , P = global[PROMISE]
+ , empty = function(){ /* empty */ }
+ , Wrapper;
+
+ var testResolve = function(sub){
+ var test = new P(empty), promise;
+ if(sub)test.constructor = function(exec){
+ exec(empty, empty);
+ };
+ (promise = P.resolve(test))['catch'](empty);
+ return promise === test;
+ };
+
+ var USE_NATIVE = function(){
+ var works = false;
+ function P2(x){
+ var self = new P(x);
+ setProto(self, P2.prototype);
+ return self;
+ }
+ try {
+ works = P && P.resolve && testResolve();
+ setProto(P2, P);
+ P2.prototype = $.create(P.prototype, {constructor: {value: P2}});
+ // actual Firefox has broken subclass support, test that
+ if(!(P2.resolve(5).then(function(){}) instanceof P2)){
+ works = false;
+ }
+ // actual V8 bug, https://code.google.com/p/v8/issues/detail?id=4162
+ if(works && __webpack_require__(8)){
+ var thenableThenGotten = false;
+ P.resolve($.setDesc({}, 'then', {
+ get: function(){ thenableThenGotten = true; }
+ }));
+ works = thenableThenGotten;
+ }
+ } catch(e){ works = false; }
+ return works;
+ }();
+
+ // helpers
+ var sameConstructor = function(a, b){
+ // library wrapper special case
+ if(LIBRARY && a === P && b === Wrapper)return true;
+ return same(a, b);
+ };
+ var getConstructor = function(C){
+ var S = anObject(C)[SPECIES];
+ return S != undefined ? S : C;
+ };
+ var isThenable = function(it){
+ var then;
+ return isObject(it) && typeof (then = it.then) == 'function' ? then : false;
+ };
+ var PromiseCapability = function(C){
+ var resolve, reject;
+ this.promise = new C(function($$resolve, $$reject){
+ if(resolve !== undefined || reject !== undefined)throw TypeError('Bad Promise constructor');
+ resolve = $$resolve;
+ reject = $$reject;
+ });
+ this.resolve = aFunction(resolve),
+ this.reject = aFunction(reject)
+ };
+ var perform = function(exec){
+ try {
+ exec();
+ } catch(e){
+ return {error: e};
+ }
+ };
+ var notify = function(record, isReject){
+ if(record.n)return;
+ record.n = true;
+ var chain = record.c;
+ asap(function(){
+ var value = record.v
+ , ok = record.s == 1
+ , i = 0;
+ var run = function(reaction){
+ var handler = ok ? reaction.ok : reaction.fail
+ , resolve = reaction.resolve
+ , reject = reaction.reject
+ , result, then;
+ try {
+ if(handler){
+ if(!ok)record.h = true;
+ result = handler === true ? value : handler(value);
+ if(result === reaction.promise){
+ reject(TypeError('Promise-chain cycle'));
+ } else if(then = isThenable(result)){
+ then.call(result, resolve, reject);
+ } else resolve(result);
+ } else reject(value);
+ } catch(e){
+ reject(e);
+ }
+ };
+ while(chain.length > i)run(chain[i++]); // variable length - can't use forEach
+ chain.length = 0;
+ record.n = false;
+ if(isReject)setTimeout(function(){
+ var promise = record.p
+ , handler, console;
+ if(isUnhandled(promise)){
+ if(isNode){
+ process.emit('unhandledRejection', value, promise);
+ } else if(handler = global.onunhandledrejection){
+ handler({promise: promise, reason: value});
+ } else if((console = global.console) && console.error){
+ console.error('Unhandled promise rejection', value);
+ }
+ } record.a = undefined;
+ }, 1);
+ });
+ };
+ var isUnhandled = function(promise){
+ var record = promise._d
+ , chain = record.a || record.c
+ , i = 0
+ , reaction;
+ if(record.h)return false;
+ while(chain.length > i){
+ reaction = chain[i++];
+ if(reaction.fail || !isUnhandled(reaction.promise))return false;
+ } return true;
+ };
+ var $reject = function(value){
+ var record = this;
+ if(record.d)return;
+ record.d = true;
+ record = record.r || record; // unwrap
+ record.v = value;
+ record.s = 2;
+ record.a = record.c.slice();
+ notify(record, true);
+ };
+ var $resolve = function(value){
+ var record = this
+ , then;
+ if(record.d)return;
+ record.d = true;
+ record = record.r || record; // unwrap
+ try {
+ if(record.p === value)throw TypeError("Promise can't be resolved itself");
+ if(then = isThenable(value)){
+ asap(function(){
+ var wrapper = {r: record, d: false}; // wrap
+ try {
+ then.call(value, ctx($resolve, wrapper, 1), ctx($reject, wrapper, 1));
+ } catch(e){
+ $reject.call(wrapper, e);
+ }
+ });
+ } else {
+ record.v = value;
+ record.s = 1;
+ notify(record, false);
+ }
+ } catch(e){
+ $reject.call({r: record, d: false}, e); // wrap
+ }
+ };
+
+ // constructor polyfill
+ if(!USE_NATIVE){
+ // 25.4.3.1 Promise(executor)
+ P = function Promise(executor){
+ aFunction(executor);
+ var record = this._d = {
+ p: strictNew(this, P, PROMISE), // <- promise
+ c: [], // <- awaiting reactions
+ a: undefined, // <- checked in isUnhandled reactions
+ s: 0, // <- state
+ d: false, // <- done
+ v: undefined, // <- value
+ h: false, // <- handled rejection
+ n: false // <- notify
+ };
+ try {
+ executor(ctx($resolve, record, 1), ctx($reject, record, 1));
+ } catch(err){
+ $reject.call(record, err);
+ }
+ };
+ __webpack_require__(130)(P.prototype, {
+ // 25.4.5.3 Promise.prototype.then(onFulfilled, onRejected)
+ then: function then(onFulfilled, onRejected){
+ var reaction = new PromiseCapability(speciesConstructor(this, P))
+ , promise = reaction.promise
+ , record = this._d;
+ reaction.ok = typeof onFulfilled == 'function' ? onFulfilled : true;
+ reaction.fail = typeof onRejected == 'function' && onRejected;
+ record.c.push(reaction);
+ if(record.a)record.a.push(reaction);
+ if(record.s)notify(record, false);
+ return promise;
+ },
+ // 25.4.5.1 Promise.prototype.catch(onRejected)
+ 'catch': function(onRejected){
+ return this.then(undefined, onRejected);
+ }
+ });
+ }
+
+ $export($export.G + $export.W + $export.F * !USE_NATIVE, {Promise: P});
+ __webpack_require__(35)(P, PROMISE);
+ __webpack_require__(117)(PROMISE);
+ Wrapper = __webpack_require__(5)[PROMISE];
+
+ // statics
+ $export($export.S + $export.F * !USE_NATIVE, PROMISE, {
+ // 25.4.4.5 Promise.reject(r)
+ reject: function reject(r){
+ var capability = new PromiseCapability(this)
+ , $$reject = capability.reject;
+ $$reject(r);
+ return capability.promise;
+ }
+ });
+ $export($export.S + $export.F * (!USE_NATIVE || testResolve(true)), PROMISE, {
+ // 25.4.4.6 Promise.resolve(x)
+ resolve: function resolve(x){
+ // instanceof instead of internal slot check because we should fix it without replacement native Promise core
+ if(x instanceof P && sameConstructor(x.constructor, this))return x;
+ var capability = new PromiseCapability(this)
+ , $$resolve = capability.resolve;
+ $$resolve(x);
+ return capability.promise;
+ }
+ });
+ $export($export.S + $export.F * !(USE_NATIVE && __webpack_require__(111)(function(iter){
+ P.all(iter)['catch'](function(){});
+ })), PROMISE, {
+ // 25.4.4.1 Promise.all(iterable)
+ all: function all(iterable){
+ var C = getConstructor(this)
+ , capability = new PromiseCapability(C)
+ , resolve = capability.resolve
+ , reject = capability.reject
+ , values = [];
+ var abrupt = perform(function(){
+ forOf(iterable, false, values.push, values);
+ var remaining = values.length
+ , results = Array(remaining);
+ if(remaining)$.each.call(values, function(promise, index){
+ var alreadyCalled = false;
+ C.resolve(promise).then(function(value){
+ if(alreadyCalled)return;
+ alreadyCalled = true;
+ results[index] = value;
+ --remaining || resolve(results);
+ }, reject);
+ });
+ else resolve(results);
+ });
+ if(abrupt)reject(abrupt.error);
+ return capability.promise;
+ },
+ // 25.4.4.4 Promise.race(iterable)
+ race: function race(iterable){
+ var C = getConstructor(this)
+ , capability = new PromiseCapability(C)
+ , reject = capability.reject;
+ var abrupt = perform(function(){
+ forOf(iterable, false, function(promise){
+ C.resolve(promise).then(capability.resolve, reject);
+ });
+ });
+ if(abrupt)reject(abrupt.error);
+ return capability.promise;
+ }
+ });
+
+/***/ },
+/* 125 */
+/***/ function(module, exports) {
+
+ module.exports = function(it, Constructor, name){
+ if(!(it instanceof Constructor))throw TypeError(name + ": use the 'new' operator!");
+ return it;
+ };
+
+/***/ },
+/* 126 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var ctx = __webpack_require__(6)
+ , call = __webpack_require__(107)
+ , isArrayIter = __webpack_require__(108)
+ , anObject = __webpack_require__(17)
+ , toLength = __webpack_require__(24)
+ , getIterFn = __webpack_require__(109);
+ module.exports = function(iterable, entries, fn, that){
+ var iterFn = getIterFn(iterable)
+ , f = ctx(fn, that, entries ? 2 : 1)
+ , index = 0
+ , length, step, iterator;
+ if(typeof iterFn != 'function')throw TypeError(iterable + ' is not iterable!');
+ // fast case for arrays with default iterator
+ if(isArrayIter(iterFn))for(length = toLength(iterable.length); length > index; index++){
+ entries ? f(anObject(step = iterable[index])[0], step[1]) : f(iterable[index]);
+ } else for(iterator = iterFn.call(iterable); !(step = iterator.next()).done; ){
+ call(iterator, f, step.value, entries);
+ }
+ };
+
+/***/ },
+/* 127 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 7.3.20 SpeciesConstructor(O, defaultConstructor)
+ var anObject = __webpack_require__(17)
+ , aFunction = __webpack_require__(7)
+ , SPECIES = __webpack_require__(29)('species');
+ module.exports = function(O, D){
+ var C = anObject(O).constructor, S;
+ return C === undefined || (S = anObject(C)[SPECIES]) == undefined ? D : aFunction(S);
+ };
+
+/***/ },
+/* 128 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var global = __webpack_require__(4)
+ , macrotask = __webpack_require__(129).set
+ , Observer = global.MutationObserver || global.WebKitMutationObserver
+ , process = global.process
+ , Promise = global.Promise
+ , isNode = __webpack_require__(15)(process) == 'process'
+ , head, last, notify;
+
+ var flush = function(){
+ var parent, domain, fn;
+ if(isNode && (parent = process.domain)){
+ process.domain = null;
+ parent.exit();
+ }
+ while(head){
+ domain = head.domain;
+ fn = head.fn;
+ if(domain)domain.enter();
+ fn(); // <- currently we use it only for Promise - try / catch not required
+ if(domain)domain.exit();
+ head = head.next;
+ } last = undefined;
+ if(parent)parent.enter();
+ };
+
+ // Node.js
+ if(isNode){
+ notify = function(){
+ process.nextTick(flush);
+ };
+ // browsers with MutationObserver
+ } else if(Observer){
+ var toggle = 1
+ , node = document.createTextNode('');
+ new Observer(flush).observe(node, {characterData: true}); // eslint-disable-line no-new
+ notify = function(){
+ node.data = toggle = -toggle;
+ };
+ // environments with maybe non-completely correct, but existent Promise
+ } else if(Promise && Promise.resolve){
+ notify = function(){
+ Promise.resolve().then(flush);
+ };
+ // for other environments - macrotask based on:
+ // - setImmediate
+ // - MessageChannel
+ // - window.postMessag
+ // - onreadystatechange
+ // - setTimeout
+ } else {
+ notify = function(){
+ // strange IE + webpack dev server bug - use .call(global)
+ macrotask.call(global, flush);
+ };
+ }
+
+ module.exports = function asap(fn){
+ var task = {fn: fn, next: undefined, domain: isNode && process.domain};
+ if(last)last.next = task;
+ if(!head){
+ head = task;
+ notify();
+ } last = task;
+ };
+
+/***/ },
+/* 129 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var ctx = __webpack_require__(6)
+ , invoke = __webpack_require__(16)
+ , html = __webpack_require__(11)
+ , cel = __webpack_require__(12)
+ , global = __webpack_require__(4)
+ , process = global.process
+ , setTask = global.setImmediate
+ , clearTask = global.clearImmediate
+ , MessageChannel = global.MessageChannel
+ , counter = 0
+ , queue = {}
+ , ONREADYSTATECHANGE = 'onreadystatechange'
+ , defer, channel, port;
+ var run = function(){
+ var id = +this;
+ if(queue.hasOwnProperty(id)){
+ var fn = queue[id];
+ delete queue[id];
+ fn();
+ }
+ };
+ var listner = function(event){
+ run.call(event.data);
+ };
+ // Node.js 0.9+ & IE10+ has setImmediate, otherwise:
+ if(!setTask || !clearTask){
+ setTask = function setImmediate(fn){
+ var args = [], i = 1;
+ while(arguments.length > i)args.push(arguments[i++]);
+ queue[++counter] = function(){
+ invoke(typeof fn == 'function' ? fn : Function(fn), args);
+ };
+ defer(counter);
+ return counter;
+ };
+ clearTask = function clearImmediate(id){
+ delete queue[id];
+ };
+ // Node.js 0.8-
+ if(__webpack_require__(15)(process) == 'process'){
+ defer = function(id){
+ process.nextTick(ctx(run, id, 1));
+ };
+ // Browsers with MessageChannel, includes WebWorkers
+ } else if(MessageChannel){
+ channel = new MessageChannel;
+ port = channel.port2;
+ channel.port1.onmessage = listner;
+ defer = ctx(port.postMessage, port, 1);
+ // Browsers with postMessage, skip WebWorkers
+ // IE8 has postMessage, but it's sync & typeof its postMessage is 'object'
+ } else if(global.addEventListener && typeof postMessage == 'function' && !global.importScripts){
+ defer = function(id){
+ global.postMessage(id + '', '*');
+ };
+ global.addEventListener('message', listner, false);
+ // IE8-
+ } else if(ONREADYSTATECHANGE in cel('script')){
+ defer = function(id){
+ html.appendChild(cel('script'))[ONREADYSTATECHANGE] = function(){
+ html.removeChild(this);
+ run.call(id);
+ };
+ };
+ // Rest old browsers
+ } else {
+ defer = function(id){
+ setTimeout(ctx(run, id, 1), 0);
+ };
+ }
+ }
+ module.exports = {
+ set: setTask,
+ clear: clearTask
+ };
+
+/***/ },
+/* 130 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var redefine = __webpack_require__(33);
+ module.exports = function(target, src){
+ for(var key in src)redefine(target, key, src[key]);
+ return target;
+ };
+
+/***/ },
+/* 131 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var strong = __webpack_require__(132);
+
+ // 23.1 Map Objects
+ __webpack_require__(133)('Map', function(get){
+ return function Map(){ return get(this, arguments.length > 0 ? arguments[0] : undefined); };
+ }, {
+ // 23.1.3.6 Map.prototype.get(key)
+ get: function get(key){
+ var entry = strong.getEntry(this, key);
+ return entry && entry.v;
+ },
+ // 23.1.3.9 Map.prototype.set(key, value)
+ set: function set(key, value){
+ return strong.def(this, key === 0 ? 0 : key, value);
+ }
+ }, strong, true);
+
+/***/ },
+/* 132 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , hide = __webpack_require__(34)
+ , redefineAll = __webpack_require__(130)
+ , ctx = __webpack_require__(6)
+ , strictNew = __webpack_require__(125)
+ , defined = __webpack_require__(19)
+ , forOf = __webpack_require__(126)
+ , $iterDefine = __webpack_require__(103)
+ , step = __webpack_require__(115)
+ , ID = __webpack_require__(25)('id')
+ , $has = __webpack_require__(14)
+ , isObject = __webpack_require__(13)
+ , setSpecies = __webpack_require__(117)
+ , DESCRIPTORS = __webpack_require__(8)
+ , isExtensible = Object.isExtensible || isObject
+ , SIZE = DESCRIPTORS ? '_s' : 'size'
+ , id = 0;
+
+ var fastKey = function(it, create){
+ // return primitive with prefix
+ if(!isObject(it))return typeof it == 'symbol' ? it : (typeof it == 'string' ? 'S' : 'P') + it;
+ if(!$has(it, ID)){
+ // can't set id to frozen object
+ if(!isExtensible(it))return 'F';
+ // not necessary to add id
+ if(!create)return 'E';
+ // add missing object id
+ hide(it, ID, ++id);
+ // return object id with prefix
+ } return 'O' + it[ID];
+ };
+
+ var getEntry = function(that, key){
+ // fast case
+ var index = fastKey(key), entry;
+ if(index !== 'F')return that._i[index];
+ // frozen object case
+ for(entry = that._f; entry; entry = entry.n){
+ if(entry.k == key)return entry;
+ }
+ };
+
+ module.exports = {
+ getConstructor: function(wrapper, NAME, IS_MAP, ADDER){
+ var C = wrapper(function(that, iterable){
+ strictNew(that, C, NAME);
+ that._i = $.create(null); // index
+ that._f = undefined; // first entry
+ that._l = undefined; // last entry
+ that[SIZE] = 0; // size
+ if(iterable != undefined)forOf(iterable, IS_MAP, that[ADDER], that);
+ });
+ redefineAll(C.prototype, {
+ // 23.1.3.1 Map.prototype.clear()
+ // 23.2.3.2 Set.prototype.clear()
+ clear: function clear(){
+ for(var that = this, data = that._i, entry = that._f; entry; entry = entry.n){
+ entry.r = true;
+ if(entry.p)entry.p = entry.p.n = undefined;
+ delete data[entry.i];
+ }
+ that._f = that._l = undefined;
+ that[SIZE] = 0;
+ },
+ // 23.1.3.3 Map.prototype.delete(key)
+ // 23.2.3.4 Set.prototype.delete(value)
+ 'delete': function(key){
+ var that = this
+ , entry = getEntry(that, key);
+ if(entry){
+ var next = entry.n
+ , prev = entry.p;
+ delete that._i[entry.i];
+ entry.r = true;
+ if(prev)prev.n = next;
+ if(next)next.p = prev;
+ if(that._f == entry)that._f = next;
+ if(that._l == entry)that._l = prev;
+ that[SIZE]--;
+ } return !!entry;
+ },
+ // 23.2.3.6 Set.prototype.forEach(callbackfn, thisArg = undefined)
+ // 23.1.3.5 Map.prototype.forEach(callbackfn, thisArg = undefined)
+ forEach: function forEach(callbackfn /*, that = undefined */){
+ var f = ctx(callbackfn, arguments.length > 1 ? arguments[1] : undefined, 3)
+ , entry;
+ while(entry = entry ? entry.n : this._f){
+ f(entry.v, entry.k, this);
+ // revert to the last existing entry
+ while(entry && entry.r)entry = entry.p;
+ }
+ },
+ // 23.1.3.7 Map.prototype.has(key)
+ // 23.2.3.7 Set.prototype.has(value)
+ has: function has(key){
+ return !!getEntry(this, key);
+ }
+ });
+ if(DESCRIPTORS)$.setDesc(C.prototype, 'size', {
+ get: function(){
+ return defined(this[SIZE]);
+ }
+ });
+ return C;
+ },
+ def: function(that, key, value){
+ var entry = getEntry(that, key)
+ , prev, index;
+ // change existing entry
+ if(entry){
+ entry.v = value;
+ // create new entry
+ } else {
+ that._l = entry = {
+ i: index = fastKey(key, true), // <- index
+ k: key, // <- key
+ v: value, // <- value
+ p: prev = that._l, // <- previous entry
+ n: undefined, // <- next entry
+ r: false // <- removed
+ };
+ if(!that._f)that._f = entry;
+ if(prev)prev.n = entry;
+ that[SIZE]++;
+ // add to index
+ if(index !== 'F')that._i[index] = entry;
+ } return that;
+ },
+ getEntry: getEntry,
+ setStrong: function(C, NAME, IS_MAP){
+ // add .keys, .values, .entries, [@@iterator]
+ // 23.1.3.4, 23.1.3.8, 23.1.3.11, 23.1.3.12, 23.2.3.5, 23.2.3.8, 23.2.3.10, 23.2.3.11
+ $iterDefine(C, NAME, function(iterated, kind){
+ this._t = iterated; // target
+ this._k = kind; // kind
+ this._l = undefined; // previous
+ }, function(){
+ var that = this
+ , kind = that._k
+ , entry = that._l;
+ // revert to the last existing entry
+ while(entry && entry.r)entry = entry.p;
+ // get next entry
+ if(!that._t || !(that._l = entry = entry ? entry.n : that._t._f)){
+ // or finish the iteration
+ that._t = undefined;
+ return step(1);
+ }
+ // return step by kind
+ if(kind == 'keys' )return step(0, entry.k);
+ if(kind == 'values')return step(0, entry.v);
+ return step(0, [entry.k, entry.v]);
+ }, IS_MAP ? 'entries' : 'values' , !IS_MAP, true);
+
+ // add [@@species], 23.1.2.2, 23.2.2.2
+ setSpecies(NAME);
+ }
+ };
+
+/***/ },
+/* 133 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , global = __webpack_require__(4)
+ , $export = __webpack_require__(3)
+ , fails = __webpack_require__(9)
+ , hide = __webpack_require__(34)
+ , redefineAll = __webpack_require__(130)
+ , forOf = __webpack_require__(126)
+ , strictNew = __webpack_require__(125)
+ , isObject = __webpack_require__(13)
+ , setToStringTag = __webpack_require__(35)
+ , DESCRIPTORS = __webpack_require__(8);
+
+ module.exports = function(NAME, wrapper, methods, common, IS_MAP, IS_WEAK){
+ var Base = global[NAME]
+ , C = Base
+ , ADDER = IS_MAP ? 'set' : 'add'
+ , proto = C && C.prototype
+ , O = {};
+ if(!DESCRIPTORS || typeof C != 'function' || !(IS_WEAK || proto.forEach && !fails(function(){
+ new C().entries().next();
+ }))){
+ // create collection constructor
+ C = common.getConstructor(wrapper, NAME, IS_MAP, ADDER);
+ redefineAll(C.prototype, methods);
+ } else {
+ C = wrapper(function(target, iterable){
+ strictNew(target, C, NAME);
+ target._c = new Base;
+ if(iterable != undefined)forOf(iterable, IS_MAP, target[ADDER], target);
+ });
+ $.each.call('add,clear,delete,forEach,get,has,set,keys,values,entries'.split(','),function(KEY){
+ var IS_ADDER = KEY == 'add' || KEY == 'set';
+ if(KEY in proto && !(IS_WEAK && KEY == 'clear'))hide(C.prototype, KEY, function(a, b){
+ if(!IS_ADDER && IS_WEAK && !isObject(a))return KEY == 'get' ? undefined : false;
+ var result = this._c[KEY](a === 0 ? 0 : a, b);
+ return IS_ADDER ? this : result;
+ });
+ });
+ if('size' in proto)$.setDesc(C.prototype, 'size', {
+ get: function(){
+ return this._c.size;
+ }
+ });
+ }
+
+ setToStringTag(C, NAME);
+
+ O[NAME] = C;
+ $export($export.G + $export.W + $export.F, O);
+
+ if(!IS_WEAK)common.setStrong(C, NAME, IS_MAP);
+
+ return C;
+ };
+
+/***/ },
+/* 134 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var strong = __webpack_require__(132);
+
+ // 23.2 Set Objects
+ __webpack_require__(133)('Set', function(get){
+ return function Set(){ return get(this, arguments.length > 0 ? arguments[0] : undefined); };
+ }, {
+ // 23.2.3.1 Set.prototype.add(value)
+ add: function add(value){
+ return strong.def(this, value = value === 0 ? 0 : value, value);
+ }
+ }, strong);
+
+/***/ },
+/* 135 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , redefine = __webpack_require__(33)
+ , weak = __webpack_require__(136)
+ , isObject = __webpack_require__(13)
+ , has = __webpack_require__(14)
+ , frozenStore = weak.frozenStore
+ , WEAK = weak.WEAK
+ , isExtensible = Object.isExtensible || isObject
+ , tmp = {};
+
+ // 23.3 WeakMap Objects
+ var $WeakMap = __webpack_require__(133)('WeakMap', function(get){
+ return function WeakMap(){ return get(this, arguments.length > 0 ? arguments[0] : undefined); };
+ }, {
+ // 23.3.3.3 WeakMap.prototype.get(key)
+ get: function get(key){
+ if(isObject(key)){
+ if(!isExtensible(key))return frozenStore(this).get(key);
+ if(has(key, WEAK))return key[WEAK][this._i];
+ }
+ },
+ // 23.3.3.5 WeakMap.prototype.set(key, value)
+ set: function set(key, value){
+ return weak.def(this, key, value);
+ }
+ }, weak, true, true);
+
+ // IE11 WeakMap frozen keys fix
+ if(new $WeakMap().set((Object.freeze || Object)(tmp), 7).get(tmp) != 7){
+ $.each.call(['delete', 'has', 'get', 'set'], function(key){
+ var proto = $WeakMap.prototype
+ , method = proto[key];
+ redefine(proto, key, function(a, b){
+ // store frozen objects on leaky map
+ if(isObject(a) && !isExtensible(a)){
+ var result = frozenStore(this)[key](a, b);
+ return key == 'set' ? this : result;
+ // store all the rest on native weakmap
+ } return method.call(this, a, b);
+ });
+ });
+ }
+
+/***/ },
+/* 136 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var hide = __webpack_require__(34)
+ , redefineAll = __webpack_require__(130)
+ , anObject = __webpack_require__(17)
+ , isObject = __webpack_require__(13)
+ , strictNew = __webpack_require__(125)
+ , forOf = __webpack_require__(126)
+ , createArrayMethod = __webpack_require__(26)
+ , $has = __webpack_require__(14)
+ , WEAK = __webpack_require__(25)('weak')
+ , isExtensible = Object.isExtensible || isObject
+ , arrayFind = createArrayMethod(5)
+ , arrayFindIndex = createArrayMethod(6)
+ , id = 0;
+
+ // fallback for frozen keys
+ var frozenStore = function(that){
+ return that._l || (that._l = new FrozenStore);
+ };
+ var FrozenStore = function(){
+ this.a = [];
+ };
+ var findFrozen = function(store, key){
+ return arrayFind(store.a, function(it){
+ return it[0] === key;
+ });
+ };
+ FrozenStore.prototype = {
+ get: function(key){
+ var entry = findFrozen(this, key);
+ if(entry)return entry[1];
+ },
+ has: function(key){
+ return !!findFrozen(this, key);
+ },
+ set: function(key, value){
+ var entry = findFrozen(this, key);
+ if(entry)entry[1] = value;
+ else this.a.push([key, value]);
+ },
+ 'delete': function(key){
+ var index = arrayFindIndex(this.a, function(it){
+ return it[0] === key;
+ });
+ if(~index)this.a.splice(index, 1);
+ return !!~index;
+ }
+ };
+
+ module.exports = {
+ getConstructor: function(wrapper, NAME, IS_MAP, ADDER){
+ var C = wrapper(function(that, iterable){
+ strictNew(that, C, NAME);
+ that._i = id++; // collection id
+ that._l = undefined; // leak store for frozen objects
+ if(iterable != undefined)forOf(iterable, IS_MAP, that[ADDER], that);
+ });
+ redefineAll(C.prototype, {
+ // 23.3.3.2 WeakMap.prototype.delete(key)
+ // 23.4.3.3 WeakSet.prototype.delete(value)
+ 'delete': function(key){
+ if(!isObject(key))return false;
+ if(!isExtensible(key))return frozenStore(this)['delete'](key);
+ return $has(key, WEAK) && $has(key[WEAK], this._i) && delete key[WEAK][this._i];
+ },
+ // 23.3.3.4 WeakMap.prototype.has(key)
+ // 23.4.3.4 WeakSet.prototype.has(value)
+ has: function has(key){
+ if(!isObject(key))return false;
+ if(!isExtensible(key))return frozenStore(this).has(key);
+ return $has(key, WEAK) && $has(key[WEAK], this._i);
+ }
+ });
+ return C;
+ },
+ def: function(that, key, value){
+ if(!isExtensible(anObject(key))){
+ frozenStore(that).set(key, value);
+ } else {
+ $has(key, WEAK) || hide(key, WEAK, {});
+ key[WEAK][that._i] = value;
+ } return that;
+ },
+ frozenStore: frozenStore,
+ WEAK: WEAK
+ };
+
+/***/ },
+/* 137 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var weak = __webpack_require__(136);
+
+ // 23.4 WeakSet Objects
+ __webpack_require__(133)('WeakSet', function(get){
+ return function WeakSet(){ return get(this, arguments.length > 0 ? arguments[0] : undefined); };
+ }, {
+ // 23.4.3.1 WeakSet.prototype.add(value)
+ add: function add(value){
+ return weak.def(this, value, true);
+ }
+ }, weak, false, true);
+
+/***/ },
+/* 138 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.1 Reflect.apply(target, thisArgument, argumentsList)
+ var $export = __webpack_require__(3)
+ , _apply = Function.apply
+ , anObject = __webpack_require__(17);
+
+ $export($export.S, 'Reflect', {
+ apply: function apply(target, thisArgument, argumentsList){
+ return _apply.call(target, thisArgument, anObject(argumentsList));
+ }
+ });
+
+/***/ },
+/* 139 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.2 Reflect.construct(target, argumentsList [, newTarget])
+ var $ = __webpack_require__(2)
+ , $export = __webpack_require__(3)
+ , aFunction = __webpack_require__(7)
+ , anObject = __webpack_require__(17)
+ , isObject = __webpack_require__(13)
+ , bind = Function.bind || __webpack_require__(5).Function.prototype.bind;
+
+ // MS Edge supports only 2 arguments
+ // FF Nightly sets third argument as `new.target`, but does not create `this` from it
+ $export($export.S + $export.F * __webpack_require__(9)(function(){
+ function F(){}
+ return !(Reflect.construct(function(){}, [], F) instanceof F);
+ }), 'Reflect', {
+ construct: function construct(Target, args /*, newTarget*/){
+ aFunction(Target);
+ anObject(args);
+ var newTarget = arguments.length < 3 ? Target : aFunction(arguments[2]);
+ if(Target == newTarget){
+ // w/o altered newTarget, optimization for 0-4 arguments
+ switch(args.length){
+ case 0: return new Target;
+ case 1: return new Target(args[0]);
+ case 2: return new Target(args[0], args[1]);
+ case 3: return new Target(args[0], args[1], args[2]);
+ case 4: return new Target(args[0], args[1], args[2], args[3]);
+ }
+ // w/o altered newTarget, lot of arguments case
+ var $args = [null];
+ $args.push.apply($args, args);
+ return new (bind.apply(Target, $args));
+ }
+ // with altered newTarget, not support built-in constructors
+ var proto = newTarget.prototype
+ , instance = $.create(isObject(proto) ? proto : Object.prototype)
+ , result = Function.apply.call(Target, instance, args);
+ return isObject(result) ? result : instance;
+ }
+ });
+
+/***/ },
+/* 140 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.3 Reflect.defineProperty(target, propertyKey, attributes)
+ var $ = __webpack_require__(2)
+ , $export = __webpack_require__(3)
+ , anObject = __webpack_require__(17);
+
+ // MS Edge has broken Reflect.defineProperty - throwing instead of returning false
+ $export($export.S + $export.F * __webpack_require__(9)(function(){
+ Reflect.defineProperty($.setDesc({}, 1, {value: 1}), 1, {value: 2});
+ }), 'Reflect', {
+ defineProperty: function defineProperty(target, propertyKey, attributes){
+ anObject(target);
+ try {
+ $.setDesc(target, propertyKey, attributes);
+ return true;
+ } catch(e){
+ return false;
+ }
+ }
+ });
+
+/***/ },
+/* 141 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.4 Reflect.deleteProperty(target, propertyKey)
+ var $export = __webpack_require__(3)
+ , getDesc = __webpack_require__(2).getDesc
+ , anObject = __webpack_require__(17);
+
+ $export($export.S, 'Reflect', {
+ deleteProperty: function deleteProperty(target, propertyKey){
+ var desc = getDesc(anObject(target), propertyKey);
+ return desc && !desc.configurable ? false : delete target[propertyKey];
+ }
+ });
+
+/***/ },
+/* 142 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // 26.1.5 Reflect.enumerate(target)
+ var $export = __webpack_require__(3)
+ , anObject = __webpack_require__(17);
+ var Enumerate = function(iterated){
+ this._t = anObject(iterated); // target
+ this._i = 0; // next index
+ var keys = this._k = [] // keys
+ , key;
+ for(key in iterated)keys.push(key);
+ };
+ __webpack_require__(105)(Enumerate, 'Object', function(){
+ var that = this
+ , keys = that._k
+ , key;
+ do {
+ if(that._i >= keys.length)return {value: undefined, done: true};
+ } while(!((key = keys[that._i++]) in that._t));
+ return {value: key, done: false};
+ });
+
+ $export($export.S, 'Reflect', {
+ enumerate: function enumerate(target){
+ return new Enumerate(target);
+ }
+ });
+
+/***/ },
+/* 143 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.6 Reflect.get(target, propertyKey [, receiver])
+ var $ = __webpack_require__(2)
+ , has = __webpack_require__(14)
+ , $export = __webpack_require__(3)
+ , isObject = __webpack_require__(13)
+ , anObject = __webpack_require__(17);
+
+ function get(target, propertyKey/*, receiver*/){
+ var receiver = arguments.length < 3 ? target : arguments[2]
+ , desc, proto;
+ if(anObject(target) === receiver)return target[propertyKey];
+ if(desc = $.getDesc(target, propertyKey))return has(desc, 'value')
+ ? desc.value
+ : desc.get !== undefined
+ ? desc.get.call(receiver)
+ : undefined;
+ if(isObject(proto = $.getProto(target)))return get(proto, propertyKey, receiver);
+ }
+
+ $export($export.S, 'Reflect', {get: get});
+
+/***/ },
+/* 144 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.7 Reflect.getOwnPropertyDescriptor(target, propertyKey)
+ var $ = __webpack_require__(2)
+ , $export = __webpack_require__(3)
+ , anObject = __webpack_require__(17);
+
+ $export($export.S, 'Reflect', {
+ getOwnPropertyDescriptor: function getOwnPropertyDescriptor(target, propertyKey){
+ return $.getDesc(anObject(target), propertyKey);
+ }
+ });
+
+/***/ },
+/* 145 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.8 Reflect.getPrototypeOf(target)
+ var $export = __webpack_require__(3)
+ , getProto = __webpack_require__(2).getProto
+ , anObject = __webpack_require__(17);
+
+ $export($export.S, 'Reflect', {
+ getPrototypeOf: function getPrototypeOf(target){
+ return getProto(anObject(target));
+ }
+ });
+
+/***/ },
+/* 146 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.9 Reflect.has(target, propertyKey)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Reflect', {
+ has: function has(target, propertyKey){
+ return propertyKey in target;
+ }
+ });
+
+/***/ },
+/* 147 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.10 Reflect.isExtensible(target)
+ var $export = __webpack_require__(3)
+ , anObject = __webpack_require__(17)
+ , $isExtensible = Object.isExtensible;
+
+ $export($export.S, 'Reflect', {
+ isExtensible: function isExtensible(target){
+ anObject(target);
+ return $isExtensible ? $isExtensible(target) : true;
+ }
+ });
+
+/***/ },
+/* 148 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.11 Reflect.ownKeys(target)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Reflect', {ownKeys: __webpack_require__(149)});
+
+/***/ },
+/* 149 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // all object keys, includes non-enumerable and symbols
+ var $ = __webpack_require__(2)
+ , anObject = __webpack_require__(17)
+ , Reflect = __webpack_require__(4).Reflect;
+ module.exports = Reflect && Reflect.ownKeys || function ownKeys(it){
+ var keys = $.getNames(anObject(it))
+ , getSymbols = $.getSymbols;
+ return getSymbols ? keys.concat(getSymbols(it)) : keys;
+ };
+
+/***/ },
+/* 150 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.12 Reflect.preventExtensions(target)
+ var $export = __webpack_require__(3)
+ , anObject = __webpack_require__(17)
+ , $preventExtensions = Object.preventExtensions;
+
+ $export($export.S, 'Reflect', {
+ preventExtensions: function preventExtensions(target){
+ anObject(target);
+ try {
+ if($preventExtensions)$preventExtensions(target);
+ return true;
+ } catch(e){
+ return false;
+ }
+ }
+ });
+
+/***/ },
+/* 151 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.13 Reflect.set(target, propertyKey, V [, receiver])
+ var $ = __webpack_require__(2)
+ , has = __webpack_require__(14)
+ , $export = __webpack_require__(3)
+ , createDesc = __webpack_require__(10)
+ , anObject = __webpack_require__(17)
+ , isObject = __webpack_require__(13);
+
+ function set(target, propertyKey, V/*, receiver*/){
+ var receiver = arguments.length < 4 ? target : arguments[3]
+ , ownDesc = $.getDesc(anObject(target), propertyKey)
+ , existingDescriptor, proto;
+ if(!ownDesc){
+ if(isObject(proto = $.getProto(target))){
+ return set(proto, propertyKey, V, receiver);
+ }
+ ownDesc = createDesc(0);
+ }
+ if(has(ownDesc, 'value')){
+ if(ownDesc.writable === false || !isObject(receiver))return false;
+ existingDescriptor = $.getDesc(receiver, propertyKey) || createDesc(0);
+ existingDescriptor.value = V;
+ $.setDesc(receiver, propertyKey, existingDescriptor);
+ return true;
+ }
+ return ownDesc.set === undefined ? false : (ownDesc.set.call(receiver, V), true);
+ }
+
+ $export($export.S, 'Reflect', {set: set});
+
+/***/ },
+/* 152 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.14 Reflect.setPrototypeOf(target, proto)
+ var $export = __webpack_require__(3)
+ , setProto = __webpack_require__(45);
+
+ if(setProto)$export($export.S, 'Reflect', {
+ setPrototypeOf: function setPrototypeOf(target, proto){
+ setProto.check(target, proto);
+ try {
+ setProto.set(target, proto);
+ return true;
+ } catch(e){
+ return false;
+ }
+ }
+ });
+
+/***/ },
+/* 153 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , $includes = __webpack_require__(31)(true);
+
+ $export($export.P, 'Array', {
+ // https://github.com/domenic/Array.prototype.includes
+ includes: function includes(el /*, fromIndex = 0 */){
+ return $includes(this, el, arguments.length > 1 ? arguments[1] : undefined);
+ }
+ });
+
+ __webpack_require__(114)('includes');
+
+/***/ },
+/* 154 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // https://github.com/mathiasbynens/String.prototype.at
+ var $export = __webpack_require__(3)
+ , $at = __webpack_require__(93)(true);
+
+ $export($export.P, 'String', {
+ at: function at(pos){
+ return $at(this, pos);
+ }
+ });
+
+/***/ },
+/* 155 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , $pad = __webpack_require__(156);
+
+ $export($export.P, 'String', {
+ padLeft: function padLeft(maxLength /*, fillString = ' ' */){
+ return $pad(this, maxLength, arguments.length > 1 ? arguments[1] : undefined, true);
+ }
+ });
+
+/***/ },
+/* 156 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // https://github.com/ljharb/proposal-string-pad-left-right
+ var toLength = __webpack_require__(24)
+ , repeat = __webpack_require__(100)
+ , defined = __webpack_require__(19);
+
+ module.exports = function(that, maxLength, fillString, left){
+ var S = String(defined(that))
+ , stringLength = S.length
+ , fillStr = fillString === undefined ? ' ' : String(fillString)
+ , intMaxLength = toLength(maxLength);
+ if(intMaxLength <= stringLength)return S;
+ if(fillStr == '')fillStr = ' ';
+ var fillLen = intMaxLength - stringLength
+ , stringFiller = repeat.call(fillStr, Math.ceil(fillLen / fillStr.length));
+ if(stringFiller.length > fillLen)stringFiller = stringFiller.slice(0, fillLen);
+ return left ? stringFiller + S : S + stringFiller;
+ };
+
+/***/ },
+/* 157 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , $pad = __webpack_require__(156);
+
+ $export($export.P, 'String', {
+ padRight: function padRight(maxLength /*, fillString = ' ' */){
+ return $pad(this, maxLength, arguments.length > 1 ? arguments[1] : undefined, false);
+ }
+ });
+
+/***/ },
+/* 158 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // https://github.com/sebmarkbage/ecmascript-string-left-right-trim
+ __webpack_require__(91)('trimLeft', function($trim){
+ return function trimLeft(){
+ return $trim(this, 1);
+ };
+ });
+
+/***/ },
+/* 159 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // https://github.com/sebmarkbage/ecmascript-string-left-right-trim
+ __webpack_require__(91)('trimRight', function($trim){
+ return function trimRight(){
+ return $trim(this, 2);
+ };
+ });
+
+/***/ },
+/* 160 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // https://github.com/benjamingr/RexExp.escape
+ var $export = __webpack_require__(3)
+ , $re = __webpack_require__(161)(/[\\^$*+?.()|[\]{}]/g, '\\$&');
+
+ $export($export.S, 'RegExp', {escape: function escape(it){ return $re(it); }});
+
+
+/***/ },
+/* 161 */
+/***/ function(module, exports) {
+
+ module.exports = function(regExp, replace){
+ var replacer = replace === Object(replace) ? function(part){
+ return replace[part];
+ } : replace;
+ return function(it){
+ return String(it).replace(regExp, replacer);
+ };
+ };
+
+/***/ },
+/* 162 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // https://gist.github.com/WebReflection/9353781
+ var $ = __webpack_require__(2)
+ , $export = __webpack_require__(3)
+ , ownKeys = __webpack_require__(149)
+ , toIObject = __webpack_require__(20)
+ , createDesc = __webpack_require__(10);
+
+ $export($export.S, 'Object', {
+ getOwnPropertyDescriptors: function getOwnPropertyDescriptors(object){
+ var O = toIObject(object)
+ , setDesc = $.setDesc
+ , getDesc = $.getDesc
+ , keys = ownKeys(O)
+ , result = {}
+ , i = 0
+ , key, D;
+ while(keys.length > i){
+ D = getDesc(O, key = keys[i++]);
+ if(key in result)setDesc(result, key, createDesc(0, D));
+ else result[key] = D;
+ } return result;
+ }
+ });
+
+/***/ },
+/* 163 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // http://goo.gl/XkBrjD
+ var $export = __webpack_require__(3)
+ , $values = __webpack_require__(164)(false);
+
+ $export($export.S, 'Object', {
+ values: function values(it){
+ return $values(it);
+ }
+ });
+
+/***/ },
+/* 164 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $ = __webpack_require__(2)
+ , toIObject = __webpack_require__(20)
+ , isEnum = $.isEnum;
+ module.exports = function(isEntries){
+ return function(it){
+ var O = toIObject(it)
+ , keys = $.getKeys(O)
+ , length = keys.length
+ , i = 0
+ , result = []
+ , key;
+ while(length > i)if(isEnum.call(O, key = keys[i++])){
+ result.push(isEntries ? [key, O[key]] : O[key]);
+ } return result;
+ };
+ };
+
+/***/ },
+/* 165 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // http://goo.gl/XkBrjD
+ var $export = __webpack_require__(3)
+ , $entries = __webpack_require__(164)(true);
+
+ $export($export.S, 'Object', {
+ entries: function entries(it){
+ return $entries(it);
+ }
+ });
+
+/***/ },
+/* 166 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // https://github.com/DavidBruant/Map-Set.prototype.toJSON
+ var $export = __webpack_require__(3);
+
+ $export($export.P, 'Map', {toJSON: __webpack_require__(167)('Map')});
+
+/***/ },
+/* 167 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // https://github.com/DavidBruant/Map-Set.prototype.toJSON
+ var forOf = __webpack_require__(126)
+ , classof = __webpack_require__(110);
+ module.exports = function(NAME){
+ return function toJSON(){
+ if(classof(this) != NAME)throw TypeError(NAME + "#toJSON isn't generic");
+ var arr = [];
+ forOf(this, false, arr.push, arr);
+ return arr;
+ };
+ };
+
+/***/ },
+/* 168 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // https://github.com/DavidBruant/Map-Set.prototype.toJSON
+ var $export = __webpack_require__(3);
+
+ $export($export.P, 'Set', {toJSON: __webpack_require__(167)('Set')});
+
+/***/ },
+/* 169 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3)
+ , $task = __webpack_require__(129);
+ $export($export.G + $export.B, {
+ setImmediate: $task.set,
+ clearImmediate: $task.clear
+ });
+
+/***/ },
+/* 170 */
+/***/ function(module, exports, __webpack_require__) {
+
+ __webpack_require__(113);
+ var Iterators = __webpack_require__(104);
+ Iterators.NodeList = Iterators.HTMLCollection = Iterators.Array;
+
+/***/ },
+/* 171 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // ie9- setTimeout & setInterval additional parameters fix
+ var global = __webpack_require__(4)
+ , $export = __webpack_require__(3)
+ , invoke = __webpack_require__(16)
+ , partial = __webpack_require__(172)
+ , navigator = global.navigator
+ , MSIE = !!navigator && /MSIE .\./.test(navigator.userAgent); // <- dirty ie9- check
+ var wrap = function(set){
+ return MSIE ? function(fn, time /*, ...args */){
+ return set(invoke(
+ partial,
+ [].slice.call(arguments, 2),
+ typeof fn == 'function' ? fn : Function(fn)
+ ), time);
+ } : set;
+ };
+ $export($export.G + $export.B + $export.F * MSIE, {
+ setTimeout: wrap(global.setTimeout),
+ setInterval: wrap(global.setInterval)
+ });
+
+/***/ },
+/* 172 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var path = __webpack_require__(173)
+ , invoke = __webpack_require__(16)
+ , aFunction = __webpack_require__(7);
+ module.exports = function(/* ...pargs */){
+ var fn = aFunction(this)
+ , length = arguments.length
+ , pargs = Array(length)
+ , i = 0
+ , _ = path._
+ , holder = false;
+ while(length > i)if((pargs[i] = arguments[i++]) === _)holder = true;
+ return function(/* ...args */){
+ var that = this
+ , $$ = arguments
+ , $$len = $$.length
+ , j = 0, k = 0, args;
+ if(!holder && !$$len)return invoke(fn, pargs, that);
+ args = pargs.slice();
+ if(holder)for(;length > j; j++)if(args[j] === _)args[j] = $$[k++];
+ while($$len > k)args.push($$[k++]);
+ return invoke(fn, args, that);
+ };
+ };
+
+/***/ },
+/* 173 */
+/***/ function(module, exports, __webpack_require__) {
+
+ module.exports = __webpack_require__(5);
+
+/***/ },
+/* 174 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , ctx = __webpack_require__(6)
+ , $export = __webpack_require__(3)
+ , createDesc = __webpack_require__(10)
+ , assign = __webpack_require__(41)
+ , keyOf = __webpack_require__(36)
+ , aFunction = __webpack_require__(7)
+ , forOf = __webpack_require__(126)
+ , isIterable = __webpack_require__(175)
+ , $iterCreate = __webpack_require__(105)
+ , step = __webpack_require__(115)
+ , isObject = __webpack_require__(13)
+ , toIObject = __webpack_require__(20)
+ , DESCRIPTORS = __webpack_require__(8)
+ , has = __webpack_require__(14)
+ , getKeys = $.getKeys;
+
+ // 0 -> Dict.forEach
+ // 1 -> Dict.map
+ // 2 -> Dict.filter
+ // 3 -> Dict.some
+ // 4 -> Dict.every
+ // 5 -> Dict.find
+ // 6 -> Dict.findKey
+ // 7 -> Dict.mapPairs
+ var createDictMethod = function(TYPE){
+ var IS_MAP = TYPE == 1
+ , IS_EVERY = TYPE == 4;
+ return function(object, callbackfn, that /* = undefined */){
+ var f = ctx(callbackfn, that, 3)
+ , O = toIObject(object)
+ , result = IS_MAP || TYPE == 7 || TYPE == 2
+ ? new (typeof this == 'function' ? this : Dict) : undefined
+ , key, val, res;
+ for(key in O)if(has(O, key)){
+ val = O[key];
+ res = f(val, key, object);
+ if(TYPE){
+ if(IS_MAP)result[key] = res; // map
+ else if(res)switch(TYPE){
+ case 2: result[key] = val; break; // filter
+ case 3: return true; // some
+ case 5: return val; // find
+ case 6: return key; // findKey
+ case 7: result[res[0]] = res[1]; // mapPairs
+ } else if(IS_EVERY)return false; // every
+ }
+ }
+ return TYPE == 3 || IS_EVERY ? IS_EVERY : result;
+ };
+ };
+ var findKey = createDictMethod(6);
+
+ var createDictIter = function(kind){
+ return function(it){
+ return new DictIterator(it, kind);
+ };
+ };
+ var DictIterator = function(iterated, kind){
+ this._t = toIObject(iterated); // target
+ this._a = getKeys(iterated); // keys
+ this._i = 0; // next index
+ this._k = kind; // kind
+ };
+ $iterCreate(DictIterator, 'Dict', function(){
+ var that = this
+ , O = that._t
+ , keys = that._a
+ , kind = that._k
+ , key;
+ do {
+ if(that._i >= keys.length){
+ that._t = undefined;
+ return step(1);
+ }
+ } while(!has(O, key = keys[that._i++]));
+ if(kind == 'keys' )return step(0, key);
+ if(kind == 'values')return step(0, O[key]);
+ return step(0, [key, O[key]]);
+ });
+
+ function Dict(iterable){
+ var dict = $.create(null);
+ if(iterable != undefined){
+ if(isIterable(iterable)){
+ forOf(iterable, true, function(key, value){
+ dict[key] = value;
+ });
+ } else assign(dict, iterable);
+ }
+ return dict;
+ }
+ Dict.prototype = null;
+
+ function reduce(object, mapfn, init){
+ aFunction(mapfn);
+ var O = toIObject(object)
+ , keys = getKeys(O)
+ , length = keys.length
+ , i = 0
+ , memo, key;
+ if(arguments.length < 3){
+ if(!length)throw TypeError('Reduce of empty object with no initial value');
+ memo = O[keys[i++]];
+ } else memo = Object(init);
+ while(length > i)if(has(O, key = keys[i++])){
+ memo = mapfn(memo, O[key], key, object);
+ }
+ return memo;
+ }
+
+ function includes(object, el){
+ return (el == el ? keyOf(object, el) : findKey(object, function(it){
+ return it != it;
+ })) !== undefined;
+ }
+
+ function get(object, key){
+ if(has(object, key))return object[key];
+ }
+ function set(object, key, value){
+ if(DESCRIPTORS && key in Object)$.setDesc(object, key, createDesc(0, value));
+ else object[key] = value;
+ return object;
+ }
+
+ function isDict(it){
+ return isObject(it) && $.getProto(it) === Dict.prototype;
+ }
+
+ $export($export.G + $export.F, {Dict: Dict});
+
+ $export($export.S, 'Dict', {
+ keys: createDictIter('keys'),
+ values: createDictIter('values'),
+ entries: createDictIter('entries'),
+ forEach: createDictMethod(0),
+ map: createDictMethod(1),
+ filter: createDictMethod(2),
+ some: createDictMethod(3),
+ every: createDictMethod(4),
+ find: createDictMethod(5),
+ findKey: findKey,
+ mapPairs: createDictMethod(7),
+ reduce: reduce,
+ keyOf: keyOf,
+ includes: includes,
+ has: has,
+ get: get,
+ set: set,
+ isDict: isDict
+ });
+
+/***/ },
+/* 175 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var classof = __webpack_require__(110)
+ , ITERATOR = __webpack_require__(29)('iterator')
+ , Iterators = __webpack_require__(104);
+ module.exports = __webpack_require__(5).isIterable = function(it){
+ var O = Object(it);
+ return O[ITERATOR] !== undefined
+ || '@@iterator' in O
+ || Iterators.hasOwnProperty(classof(O));
+ };
+
+/***/ },
+/* 176 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var anObject = __webpack_require__(17)
+ , get = __webpack_require__(109);
+ module.exports = __webpack_require__(5).getIterator = function(it){
+ var iterFn = get(it);
+ if(typeof iterFn != 'function')throw TypeError(it + ' is not iterable!');
+ return anObject(iterFn.call(it));
+ };
+
+/***/ },
+/* 177 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var global = __webpack_require__(4)
+ , core = __webpack_require__(5)
+ , $export = __webpack_require__(3)
+ , partial = __webpack_require__(172);
+ // https://esdiscuss.org/topic/promise-returning-delay-function
+ $export($export.G + $export.F, {
+ delay: function delay(time){
+ return new (core.Promise || global.Promise)(function(resolve){
+ setTimeout(partial.call(resolve, true), time);
+ });
+ }
+ });
+
+/***/ },
+/* 178 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var path = __webpack_require__(173)
+ , $export = __webpack_require__(3);
+
+ // Placeholder
+ __webpack_require__(5)._ = path._ = path._ || {};
+
+ $export($export.P + $export.F, 'Function', {part: __webpack_require__(172)});
+
+/***/ },
+/* 179 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3);
+
+ $export($export.S + $export.F, 'Object', {isObject: __webpack_require__(13)});
+
+/***/ },
+/* 180 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3);
+
+ $export($export.S + $export.F, 'Object', {classof: __webpack_require__(110)});
+
+/***/ },
+/* 181 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3)
+ , define = __webpack_require__(182);
+
+ $export($export.S + $export.F, 'Object', {define: define});
+
+/***/ },
+/* 182 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $ = __webpack_require__(2)
+ , ownKeys = __webpack_require__(149)
+ , toIObject = __webpack_require__(20);
+
+ module.exports = function define(target, mixin){
+ var keys = ownKeys(toIObject(mixin))
+ , length = keys.length
+ , i = 0, key;
+ while(length > i)$.setDesc(target, key = keys[i++], $.getDesc(mixin, key));
+ return target;
+ };
+
+/***/ },
+/* 183 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3)
+ , define = __webpack_require__(182)
+ , create = __webpack_require__(2).create;
+
+ $export($export.S + $export.F, 'Object', {
+ make: function(proto, mixin){
+ return define(create(proto), mixin);
+ }
+ });
+
+/***/ },
+/* 184 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ __webpack_require__(103)(Number, 'Number', function(iterated){
+ this._l = +iterated;
+ this._i = 0;
+ }, function(){
+ var i = this._i++
+ , done = !(i < this._l);
+ return {done: done, value: done ? undefined : i};
+ });
+
+/***/ },
+/* 185 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $export = __webpack_require__(3);
+ var $re = __webpack_require__(161)(/[&<>"']/g, {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ "'": '''
+ });
+
+ $export($export.P + $export.F, 'String', {escapeHTML: function escapeHTML(){ return $re(this); }});
+
+/***/ },
+/* 186 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $export = __webpack_require__(3);
+ var $re = __webpack_require__(161)(/&(?:amp|lt|gt|quot|apos);/g, {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ ''': "'"
+ });
+
+ $export($export.P + $export.F, 'String', {unescapeHTML: function unescapeHTML(){ return $re(this); }});
+
+/***/ },
+/* 187 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $ = __webpack_require__(2)
+ , global = __webpack_require__(4)
+ , $export = __webpack_require__(3)
+ , log = {}
+ , enabled = true;
+ // Methods from https://github.com/DeveloperToolsWG/console-object/blob/master/api.md
+ $.each.call((
+ 'assert,clear,count,debug,dir,dirxml,error,exception,group,groupCollapsed,groupEnd,' +
+ 'info,isIndependentlyComposed,log,markTimeline,profile,profileEnd,table,' +
+ 'time,timeEnd,timeline,timelineEnd,timeStamp,trace,warn'
+ ).split(','), function(key){
+ log[key] = function(){
+ var $console = global.console;
+ if(enabled && $console && $console[key]){
+ return Function.apply.call($console[key], $console, arguments);
+ }
+ };
+ });
+ $export($export.G + $export.F, {log: __webpack_require__(41)(log.log, log, {
+ enable: function(){
+ enabled = true;
+ },
+ disable: function(){
+ enabled = false;
+ }
+ })});
+
+/***/ },
+/* 188 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // JavaScript 1.6 / Strawman array statics shim
+ var $ = __webpack_require__(2)
+ , $export = __webpack_require__(3)
+ , $ctx = __webpack_require__(6)
+ , $Array = __webpack_require__(5).Array || Array
+ , statics = {};
+ var setStatics = function(keys, length){
+ $.each.call(keys.split(','), function(key){
+ if(length == undefined && key in $Array)statics[key] = $Array[key];
+ else if(key in [])statics[key] = $ctx(Function.call, [][key], length);
+ });
+ };
+ setStatics('pop,reverse,shift,keys,values,entries', 1);
+ setStatics('indexOf,every,some,forEach,map,filter,find,findIndex,includes', 3);
+ setStatics('join,slice,concat,push,splice,unshift,sort,lastIndexOf,' +
+ 'reduce,reduceRight,copyWithin,fill');
+ $export($export.S, 'Array', statics);
+
+/***/ }
+/******/ ]);
+// CommonJS export
+if(typeof module != 'undefined' && module.exports)module.exports = __e;
+// RequireJS export
+else if(typeof define == 'function' && define.amd)define(function(){return __e});
+// Export to global object
+else __g.core = __e;
+}(1, 1);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/library.min.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/library.min.js
new file mode 100644
index 00000000..f665bedf
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/library.min.js
@@ -0,0 +1,9 @@
+/**
+ * core-js 1.2.7
+ * https://github.com/zloirock/core-js
+ * License: http://rock.mit-license.org
+ * © 2016 Denis Pushkarev
+ */
+!function(b,c,a){"use strict";!function(b){function __webpack_require__(c){if(a[c])return a[c].exports;var d=a[c]={exports:{},id:c,loaded:!1};return b[c].call(d.exports,d,d.exports,__webpack_require__),d.loaded=!0,d.exports}var a={};return __webpack_require__.m=b,__webpack_require__.c=a,__webpack_require__.p="",__webpack_require__(0)}([function(b,c,a){a(1),a(32),a(40),a(42),a(44),a(46),a(48),a(49),a(50),a(51),a(52),a(53),a(54),a(55),a(56),a(57),a(58),a(59),a(60),a(62),a(63),a(64),a(65),a(66),a(67),a(68),a(70),a(71),a(72),a(74),a(75),a(76),a(78),a(79),a(80),a(81),a(82),a(83),a(84),a(85),a(86),a(87),a(88),a(89),a(90),a(92),a(94),a(98),a(99),a(101),a(102),a(106),a(112),a(113),a(116),a(118),a(120),a(122),a(123),a(124),a(131),a(134),a(135),a(137),a(138),a(139),a(140),a(141),a(142),a(143),a(144),a(145),a(146),a(147),a(148),a(150),a(151),a(152),a(153),a(154),a(155),a(157),a(158),a(159),a(160),a(162),a(163),a(165),a(166),a(168),a(169),a(170),a(171),a(174),a(109),a(176),a(175),a(177),a(178),a(179),a(180),a(181),a(183),a(184),a(185),a(186),a(187),b.exports=a(188)},function(S,R,b){var r,d=b(2),c=b(3),x=b(8),O=b(10),o=b(11),E=b(12),n=b(14),N=b(15),J=b(16),j=b(9),p=b(17),v=b(7),I=b(13),Q=b(18),y=b(20),K=b(22),w=b(23),h=b(24),s=b(21),m=b(25)("__proto__"),g=b(26),A=b(31)(!1),B=Object.prototype,C=Array.prototype,k=C.slice,M=C.join,F=d.setDesc,L=d.getDesc,q=d.setDescs,u={};x||(r=!j(function(){return 7!=F(E("div"),"a",{get:function(){return 7}}).a}),d.setDesc=function(b,c,a){if(r)try{return F(b,c,a)}catch(d){}if("get"in a||"set"in a)throw TypeError("Accessors not supported!");return"value"in a&&(p(b)[c]=a.value),b},d.getDesc=function(a,b){if(r)try{return L(a,b)}catch(c){}return n(a,b)?O(!B.propertyIsEnumerable.call(a,b),a[b]):void 0},d.setDescs=q=function(a,b){p(a);for(var c,e=d.getKeys(b),g=e.length,f=0;g>f;)d.setDesc(a,c=e[f++],b[c]);return a}),c(c.S+c.F*!x,"Object",{getOwnPropertyDescriptor:d.getDesc,defineProperty:d.setDesc,defineProperties:q});var i="constructor,hasOwnProperty,isPrototypeOf,propertyIsEnumerable,toLocaleString,toString,valueOf".split(","),H=i.concat("length","prototype"),G=i.length,l=function(){var a,b=E("iframe"),c=G,d=">";for(b.style.display="none",o.appendChild(b),b.src="javascript:",a=b.contentWindow.document,a.open(),a.write("f;)n(e,c=a[f++])&&(~A(d,c)||d.push(c));return d}},t=function(){};c(c.S,"Object",{getPrototypeOf:d.getProto=d.getProto||function(a){return a=Q(a),n(a,m)?a[m]:"function"==typeof a.constructor&&a instanceof a.constructor?a.constructor.prototype:a instanceof Object?B:null},getOwnPropertyNames:d.getNames=d.getNames||D(H,H.length,!0),create:d.create=d.create||function(c,d){var b;return null!==c?(t.prototype=p(c),b=new t,t.prototype=null,b[m]=c):b=l(),d===a?b:q(b,d)},keys:d.getKeys=d.getKeys||D(i,G,!1)});var P=function(d,a,e){if(!(a in u)){for(var c=[],b=0;a>b;b++)c[b]="a["+b+"]";u[a]=Function("F,a","return new F("+c.join(",")+")")}return u[a](d,e)};c(c.P,"Function",{bind:function bind(c){var a=v(this),d=k.call(arguments,1),b=function(){var e=d.concat(k.call(arguments));return this instanceof b?P(a,e.length,e):J(a,e,c)};return I(a.prototype)&&(b.prototype=a.prototype),b}}),c(c.P+c.F*j(function(){o&&k.call(o)}),"Array",{slice:function(f,b){var d=h(this.length),g=N(this);if(b=b===a?d:b,"Array"==g)return k.call(this,f,b);for(var e=w(f,d),l=w(b,d),i=h(l-e),j=Array(i),c=0;i>c;c++)j[c]="String"==g?this.charAt(e+c):this[e+c];return j}}),c(c.P+c.F*(s!=Object),"Array",{join:function join(b){return M.call(s(this),b===a?",":b)}}),c(c.S,"Array",{isArray:b(28)});var z=function(a){return function(g,d){v(g);var c=s(this),e=h(c.length),b=a?e-1:0,f=a?-1:1;if(arguments.length<2)for(;;){if(b in c){d=c[b],b+=f;break}if(b+=f,a?0>b:b>=e)throw TypeError("Reduce of empty array with no initial value")}for(;a?b>=0:e>b;b+=f)b in c&&(d=g(d,c[b],b,this));return d}},f=function(a){return function(b){return a(this,b,arguments[1])}};c(c.P,"Array",{forEach:d.each=d.each||f(g(0)),map:f(g(1)),filter:f(g(2)),some:f(g(3)),every:f(g(4)),reduce:z(!1),reduceRight:z(!0),indexOf:f(A),lastIndexOf:function(d,e){var b=y(this),c=h(b.length),a=c-1;for(arguments.length>1&&(a=Math.min(a,K(e))),0>a&&(a=h(c+a));a>=0;a--)if(a in b&&b[a]===d)return a;return-1}}),c(c.S,"Date",{now:function(){return+new Date}});var e=function(a){return a>9?a:"0"+a};c(c.P+c.F*(j(function(){return"0385-07-25T07:06:39.999Z"!=new Date(-5e13-1).toISOString()})||!j(function(){new Date(NaN).toISOString()})),"Date",{toISOString:function toISOString(){if(!isFinite(this))throw RangeError("Invalid time value");var a=this,b=a.getUTCFullYear(),c=a.getUTCMilliseconds(),d=0>b?"-":b>9999?"+":"";return d+("00000"+Math.abs(b)).slice(d?-6:-4)+"-"+e(a.getUTCMonth()+1)+"-"+e(a.getUTCDate())+"T"+e(a.getUTCHours())+":"+e(a.getUTCMinutes())+":"+e(a.getUTCSeconds())+"."+(c>99?c:"0"+e(c))+"Z"}})},function(b,c){var a=Object;b.exports={create:a.create,getProto:a.getPrototypeOf,isEnum:{}.propertyIsEnumerable,getDesc:a.getOwnPropertyDescriptor,setDesc:a.defineProperty,setDescs:a.defineProperties,getKeys:a.keys,getNames:a.getOwnPropertyNames,getSymbols:a.getOwnPropertySymbols,each:[].forEach}},function(g,h,d){var c=d(4),e=d(5),f=d(6),b="prototype",a=function(h,j,l){var d,k,g,p=h&a.F,n=h&a.G,q=h&a.S,o=h&a.P,r=h&a.B,s=h&a.W,m=n?e:e[j]||(e[j]={}),i=n?c:q?c[j]:(c[j]||{})[b];n&&(l=j);for(d in l)k=!p&&i&&d in i,k&&d in m||(g=k?i[d]:l[d],m[d]=n&&"function"!=typeof i[d]?l[d]:r&&k?f(g,c):s&&i[d]==g?function(a){var c=function(b){return this instanceof a?new a(b):a(b)};return c[b]=a[b],c}(g):o&&"function"==typeof g?f(Function.call,g):g,o&&((m[b]||(m[b]={}))[d]=g))};a.F=1,a.G=2,a.S=4,a.P=8,a.B=16,a.W=32,g.exports=a},function(a,d){var b=a.exports="undefined"!=typeof window&&window.Math==Math?window:"undefined"!=typeof self&&self.Math==Math?self:Function("return this")();"number"==typeof c&&(c=b)},function(a,d){var c=a.exports={version:"1.2.6"};"number"==typeof b&&(b=c)},function(b,e,c){var d=c(7);b.exports=function(b,c,e){if(d(b),c===a)return b;switch(e){case 1:return function(a){return b.call(c,a)};case 2:return function(a,d){return b.call(c,a,d)};case 3:return function(a,d,e){return b.call(c,a,d,e)}}return function(){return b.apply(c,arguments)}}},function(a,b){a.exports=function(a){if("function"!=typeof a)throw TypeError(a+" is not a function!");return a}},function(a,c,b){a.exports=!b(9)(function(){return 7!=Object.defineProperty({},"a",{get:function(){return 7}}).a})},function(a,b){a.exports=function(a){try{return!!a()}catch(b){return!0}}},function(a,b){a.exports=function(a,b){return{enumerable:!(1&a),configurable:!(2&a),writable:!(4&a),value:b}}},function(a,c,b){a.exports=b(4).document&&document.documentElement},function(d,f,b){var c=b(13),a=b(4).document,e=c(a)&&c(a.createElement);d.exports=function(b){return e?a.createElement(b):{}}},function(a,b){a.exports=function(a){return"object"==typeof a?null!==a:"function"==typeof a}},function(a,c){var b={}.hasOwnProperty;a.exports=function(a,c){return b.call(a,c)}},function(a,c){var b={}.toString;a.exports=function(a){return b.call(a).slice(8,-1)}},function(b,c){b.exports=function(c,b,d){var e=d===a;switch(b.length){case 0:return e?c():c.call(d);case 1:return e?c(b[0]):c.call(d,b[0]);case 2:return e?c(b[0],b[1]):c.call(d,b[0],b[1]);case 3:return e?c(b[0],b[1],b[2]):c.call(d,b[0],b[1],b[2]);case 4:return e?c(b[0],b[1],b[2],b[3]):c.call(d,b[0],b[1],b[2],b[3])}return c.apply(d,b)}},function(a,d,b){var c=b(13);a.exports=function(a){if(!c(a))throw TypeError(a+" is not an object!");return a}},function(a,d,b){var c=b(19);a.exports=function(a){return Object(c(a))}},function(b,c){b.exports=function(b){if(b==a)throw TypeError("Can't call method on "+b);return b}},function(b,e,a){var c=a(21),d=a(19);b.exports=function(a){return c(d(a))}},function(a,d,b){var c=b(15);a.exports=Object("z").propertyIsEnumerable(0)?Object:function(a){return"String"==c(a)?a.split(""):Object(a)}},function(a,d){var b=Math.ceil,c=Math.floor;a.exports=function(a){return isNaN(a=+a)?0:(a>0?c:b)(a)}},function(a,f,b){var c=b(22),d=Math.max,e=Math.min;a.exports=function(a,b){return a=c(a),0>a?d(a+b,0):e(a,b)}},function(a,e,b){var c=b(22),d=Math.min;a.exports=function(a){return a>0?d(c(a),9007199254740991):0}},function(b,e){var c=0,d=Math.random();b.exports=function(b){return"Symbol(".concat(b===a?"":b,")_",(++c+d).toString(36))}},function(d,i,b){var e=b(6),f=b(21),g=b(18),h=b(24),c=b(27);d.exports=function(b){var i=1==b,k=2==b,l=3==b,d=4==b,j=6==b,m=5==b||j;return function(p,v,x){for(var o,r,u=g(p),s=f(u),w=e(v,x,3),t=h(s.length),n=0,q=i?c(p,t):k?c(p,0):a;t>n;n++)if((m||n in s)&&(o=s[n],r=w(o,n,u),b))if(i)q[n]=r;else if(r)switch(b){case 3:return!0;case 5:return o;case 6:return n;case 2:q.push(o)}else if(d)return!1;return j?-1:l||d?d:q}}},function(d,g,b){var e=b(13),c=b(28),f=b(29)("species");d.exports=function(d,g){var b;return c(d)&&(b=d.constructor,"function"!=typeof b||b!==Array&&!c(b.prototype)||(b=a),e(b)&&(b=b[f],null===b&&(b=a))),new(b===a?Array:b)(g)}},function(a,d,b){var c=b(15);a.exports=Array.isArray||function(a){return"Array"==c(a)}},function(d,f,a){var c=a(30)("wks"),e=a(25),b=a(4).Symbol;d.exports=function(a){return c[a]||(c[a]=b&&b[a]||(b||e)("Symbol."+a))}},function(d,f,e){var a=e(4),b="__core-js_shared__",c=a[b]||(a[b]={});d.exports=function(a){return c[a]||(c[a]={})}},function(b,f,a){var c=a(20),d=a(24),e=a(23);b.exports=function(a){return function(j,g,k){var h,f=c(j),i=d(f.length),b=e(k,i);if(a&&g!=g){for(;i>b;)if(h=f[b++],h!=h)return!0}else for(;i>b;b++)if((a||b in f)&&f[b]===g)return a||b;return!a&&-1}}},function(W,V,b){var e=b(2),x=b(4),d=b(14),w=b(8),f=b(3),G=b(33),H=b(9),J=b(30),s=b(35),S=b(25),A=b(29),R=b(36),C=b(37),Q=b(38),P=b(28),O=b(17),p=b(20),v=b(10),I=e.getDesc,i=e.setDesc,k=e.create,z=C.get,g=x.Symbol,l=x.JSON,m=l&&l.stringify,n=!1,c=A("_hidden"),N=e.isEnum,o=J("symbol-registry"),h=J("symbols"),q="function"==typeof g,j=Object.prototype,y=w&&H(function(){return 7!=k(i({},"a",{get:function(){return i(this,"a",{value:7}).a}})).a})?function(c,a,d){var b=I(j,a);b&&delete j[a],i(c,a,d),b&&c!==j&&i(j,a,b)}:i,L=function(a){var b=h[a]=k(g.prototype);return b._k=a,w&&n&&y(j,a,{configurable:!0,set:function(b){d(this,c)&&d(this[c],a)&&(this[c][a]=!1),y(this,a,v(1,b))}}),b},r=function(a){return"symbol"==typeof a},t=function defineProperty(a,b,e){return e&&d(h,b)?(e.enumerable?(d(a,c)&&a[c][b]&&(a[c][b]=!1),e=k(e,{enumerable:v(0,!1)})):(d(a,c)||i(a,c,v(1,{})),a[c][b]=!0),y(a,b,e)):i(a,b,e)},u=function defineProperties(a,b){O(a);for(var c,d=Q(b=p(b)),e=0,f=d.length;f>e;)t(a,c=d[e++],b[c]);return a},F=function create(b,c){return c===a?k(b):u(k(b),c)},E=function propertyIsEnumerable(a){var b=N.call(this,a);return b||!d(this,a)||!d(h,a)||d(this,c)&&this[c][a]?b:!0},D=function getOwnPropertyDescriptor(a,b){var e=I(a=p(a),b);return!e||!d(h,b)||d(a,c)&&a[c][b]||(e.enumerable=!0),e},B=function getOwnPropertyNames(g){for(var a,b=z(p(g)),e=[],f=0;b.length>f;)d(h,a=b[f++])||a==c||e.push(a);return e},M=function getOwnPropertySymbols(f){for(var a,b=z(p(f)),c=[],e=0;b.length>e;)d(h,a=b[e++])&&c.push(h[a]);return c},T=function stringify(e){if(e!==a&&!r(e)){for(var b,c,d=[e],f=1,g=arguments;g.length>f;)d.push(g[f++]);return b=d[1],"function"==typeof b&&(c=b),(c||!P(b))&&(b=function(b,a){return c&&(a=c.call(this,b,a)),r(a)?void 0:a}),d[1]=b,m.apply(l,d)}},U=H(function(){var a=g();return"[null]"!=m([a])||"{}"!=m({a:a})||"{}"!=m(Object(a))});q||(g=function Symbol(){if(r(this))throw TypeError("Symbol is not a constructor");return L(S(arguments.length>0?arguments[0]:a))},G(g.prototype,"toString",function toString(){return this._k}),r=function(a){return a instanceof g},e.create=F,e.isEnum=E,e.getDesc=D,e.setDesc=t,e.setDescs=u,e.getNames=C.get=B,e.getSymbols=M,w&&!b(39)&&G(j,"propertyIsEnumerable",E,!0));var K={"for":function(a){return d(o,a+="")?o[a]:o[a]=g(a)},keyFor:function keyFor(a){return R(o,a)},useSetter:function(){n=!0},useSimple:function(){n=!1}};e.each.call("hasInstance,isConcatSpreadable,iterator,match,replace,search,species,split,toPrimitive,toStringTag,unscopables".split(","),function(a){var b=A(a);K[a]=q?b:L(b)}),n=!0,f(f.G+f.W,{Symbol:g}),f(f.S,"Symbol",K),f(f.S+f.F*!q,"Object",{create:F,defineProperty:t,defineProperties:u,getOwnPropertyDescriptor:D,getOwnPropertyNames:B,getOwnPropertySymbols:M}),l&&f(f.S+f.F*(!q||U),"JSON",{stringify:T}),s(g,"Symbol"),s(Math,"Math",!0),s(x.JSON,"JSON",!0)},function(a,c,b){a.exports=b(34)},function(b,e,a){var c=a(2),d=a(10);b.exports=a(8)?function(a,b,e){return c.setDesc(a,b,d(1,e))}:function(a,b,c){return a[b]=c,a}},function(c,f,a){var d=a(2).setDesc,e=a(14),b=a(29)("toStringTag");c.exports=function(a,c,f){a&&!e(a=f?a:a.prototype,b)&&d(a,b,{configurable:!0,value:c})}},function(b,e,a){var c=a(2),d=a(20);b.exports=function(g,h){for(var a,b=d(g),e=c.getKeys(b),i=e.length,f=0;i>f;)if(b[a=e[f++]]===h)return a}},function(d,h,a){var e=a(20),b=a(2).getNames,f={}.toString,c="object"==typeof window&&Object.getOwnPropertyNames?Object.getOwnPropertyNames(window):[],g=function(a){try{return b(a)}catch(d){return c.slice()}};d.exports.get=function getOwnPropertyNames(a){return c&&"[object Window]"==f.call(a)?g(a):b(e(a))}},function(b,d,c){var a=c(2);b.exports=function(b){var c=a.getKeys(b),d=a.getSymbols;if(d)for(var e,f=d(b),h=a.isEnum,g=0;f.length>g;)h.call(b,e=f[g++])&&c.push(e);return c}},function(a,b){a.exports=!0},function(c,d,b){var a=b(3);a(a.S+a.F,"Object",{assign:b(41)})},function(c,f,a){var b=a(2),d=a(18),e=a(21);c.exports=a(9)(function(){var a=Object.assign,b={},c={},d=Symbol(),e="abcdefghijklmnopqrst";return b[d]=7,e.split("").forEach(function(a){c[a]=a}),7!=a({},b)[d]||Object.keys(a({},c)).join("")!=e})?function assign(n,q){for(var g=d(n),h=arguments,o=h.length,j=1,f=b.getKeys,l=b.getSymbols,m=b.isEnum;o>j;)for(var c,a=e(h[j++]),k=l?f(a).concat(l(a)):f(a),p=k.length,i=0;p>i;)m.call(a,c=k[i++])&&(g[c]=a[c]);return g}:Object.assign},function(c,d,a){var b=a(3);b(b.S,"Object",{is:a(43)})},function(a,b){a.exports=Object.is||function is(a,b){return a===b?0!==a||1/a===1/b:a!=a&&b!=b}},function(c,d,a){var b=a(3);b(b.S,"Object",{setPrototypeOf:a(45).set})},function(d,h,b){var e=b(2).getDesc,f=b(13),g=b(17),c=function(b,a){if(g(b),!f(a)&&null!==a)throw TypeError(a+": can't set as prototype!")};d.exports={set:Object.setPrototypeOf||("__proto__"in{}?function(f,a,d){try{d=b(6)(Function.call,e(Object.prototype,"__proto__").set,2),d(f,[]),a=!(f instanceof Array)}catch(g){a=!0}return function setPrototypeOf(b,e){return c(b,e),a?b.__proto__=e:d(b,e),b}}({},!1):a),check:c}},function(c,d,a){var b=a(13);a(47)("freeze",function(a){return function freeze(c){return a&&b(c)?a(c):c}})},function(c,f,a){var b=a(3),d=a(5),e=a(9);c.exports=function(a,g){var c=(d.Object||{})[a]||Object[a],f={};f[a]=g(c),b(b.S+b.F*e(function(){c(1)}),"Object",f)}},function(c,d,a){var b=a(13);a(47)("seal",function(a){return function seal(c){return a&&b(c)?a(c):c}})},function(c,d,a){var b=a(13);a(47)("preventExtensions",function(a){return function preventExtensions(c){return a&&b(c)?a(c):c}})},function(c,d,a){var b=a(13);a(47)("isFrozen",function(a){return function isFrozen(c){return b(c)?a?a(c):!1:!0}})},function(c,d,a){var b=a(13);a(47)("isSealed",function(a){return function isSealed(c){return b(c)?a?a(c):!1:!0}})},function(c,d,a){var b=a(13);a(47)("isExtensible",function(a){return function isExtensible(c){return b(c)?a?a(c):!0:!1}})},function(c,d,a){var b=a(20);a(47)("getOwnPropertyDescriptor",function(a){return function getOwnPropertyDescriptor(c,d){return a(b(c),d)}})},function(c,d,a){var b=a(18);a(47)("getPrototypeOf",function(a){return function getPrototypeOf(c){return a(b(c))}})},function(c,d,a){var b=a(18);a(47)("keys",function(a){return function keys(c){return a(b(c))}})},function(b,c,a){a(47)("getOwnPropertyNames",function(){return a(37).get})},function(f,g,a){var b=a(2),c=a(13),d=a(29)("hasInstance"),e=Function.prototype;d in e||b.setDesc(e,d,{value:function(a){if("function"!=typeof this||!c(a))return!1;if(!c(this.prototype))return a instanceof this;for(;a=b.getProto(a);)if(this.prototype===a)return!0;return!1}})},function(c,d,b){var a=b(3);a(a.S,"Number",{EPSILON:Math.pow(2,-52)})},function(d,e,a){var b=a(3),c=a(4).isFinite;b(b.S,"Number",{isFinite:function isFinite(a){return"number"==typeof a&&c(a)}})},function(c,d,a){var b=a(3);b(b.S,"Number",{isInteger:a(61)})},function(a,e,b){var c=b(13),d=Math.floor;a.exports=function isInteger(a){return!c(a)&&isFinite(a)&&d(a)===a}},function(c,d,b){var a=b(3);a(a.S,"Number",{isNaN:function isNaN(a){return a!=a}})},function(e,f,a){var b=a(3),c=a(61),d=Math.abs;b(b.S,"Number",{isSafeInteger:function isSafeInteger(a){return c(a)&&d(a)<=9007199254740991}})},function(c,d,b){var a=b(3);a(a.S,"Number",{MAX_SAFE_INTEGER:9007199254740991})},function(c,d,b){var a=b(3);a(a.S,"Number",{MIN_SAFE_INTEGER:-9007199254740991})},function(c,d,b){var a=b(3);a(a.S,"Number",{parseFloat:parseFloat})},function(c,d,b){var a=b(3);a(a.S,"Number",{parseInt:parseInt})},function(f,g,b){var a=b(3),e=b(69),c=Math.sqrt,d=Math.acosh;a(a.S+a.F*!(d&&710==Math.floor(d(Number.MAX_VALUE))),"Math",{acosh:function acosh(a){return(a=+a)<1?NaN:a>94906265.62425156?Math.log(a)+Math.LN2:e(a-1+c(a-1)*c(a+1))}})},function(a,b){a.exports=Math.log1p||function log1p(a){return(a=+a)>-1e-8&&1e-8>a?a-a*a/2:Math.log(1+a)}},function(c,d,b){function asinh(a){return isFinite(a=+a)&&0!=a?0>a?-asinh(-a):Math.log(a+Math.sqrt(a*a+1)):a}var a=b(3);a(a.S,"Math",{asinh:asinh})},function(c,d,b){var a=b(3);a(a.S,"Math",{atanh:function atanh(a){return 0==(a=+a)?a:Math.log((1+a)/(1-a))/2}})},function(d,e,a){var b=a(3),c=a(73);b(b.S,"Math",{cbrt:function cbrt(a){return c(a=+a)*Math.pow(Math.abs(a),1/3)}})},function(a,b){a.exports=Math.sign||function sign(a){return 0==(a=+a)||a!=a?a:0>a?-1:1}},function(c,d,b){var a=b(3);a(a.S,"Math",{clz32:function clz32(a){return(a>>>=0)?31-Math.floor(Math.log(a+.5)*Math.LOG2E):32}})},function(d,e,c){var a=c(3),b=Math.exp;a(a.S,"Math",{cosh:function cosh(a){return(b(a=+a)+b(-a))/2}})},function(c,d,a){var b=a(3);b(b.S,"Math",{expm1:a(77)})},function(a,b){a.exports=Math.expm1||function expm1(a){return 0==(a=+a)?a:a>-1e-6&&1e-6>a?a+a*a/2:Math.exp(a)-1}},function(k,j,e){var f=e(3),g=e(73),a=Math.pow,d=a(2,-52),b=a(2,-23),i=a(2,127)*(2-b),c=a(2,-126),h=function(a){return a+1/d-1/d};f(f.S,"Math",{fround:function fround(k){var f,a,e=Math.abs(k),j=g(k);return c>e?j*h(e/c/b)*c*b:(f=(1+b/d)*e,a=f-(f-e),a>i||a!=a?j*(1/0):j*a)}})},function(d,e,b){var a=b(3),c=Math.abs;a(a.S,"Math",{hypot:function hypot(i,j){for(var a,b,e=0,f=0,g=arguments,h=g.length,d=0;h>f;)a=c(g[f++]),a>d?(b=d/a,e=e*b*b+1,d=a):a>0?(b=a/d,e+=b*b):e+=a;return d===1/0?1/0:d*Math.sqrt(e)}})},function(d,e,b){var a=b(3),c=Math.imul;a(a.S+a.F*b(9)(function(){return-5!=c(4294967295,5)||2!=c.length}),"Math",{imul:function imul(f,g){var a=65535,b=+f,c=+g,d=a&b,e=a&c;return 0|d*e+((a&b>>>16)*e+d*(a&c>>>16)<<16>>>0)}})},function(c,d,b){var a=b(3);a(a.S,"Math",{log10:function log10(a){return Math.log(a)/Math.LN10}})},function(c,d,a){var b=a(3);b(b.S,"Math",{log1p:a(69)})},function(c,d,b){var a=b(3);a(a.S,"Math",{log2:function log2(a){return Math.log(a)/Math.LN2}})},function(c,d,a){var b=a(3);b(b.S,"Math",{sign:a(73)})},function(e,f,a){var b=a(3),c=a(77),d=Math.exp;b(b.S+b.F*a(9)(function(){return-2e-17!=!Math.sinh(-2e-17)}),"Math",{sinh:function sinh(a){return Math.abs(a=+a)<1?(c(a)-c(-a))/2:(d(a-1)-d(-a-1))*(Math.E/2)}})},function(e,f,a){var b=a(3),c=a(77),d=Math.exp;b(b.S,"Math",{tanh:function tanh(a){var b=c(a=+a),e=c(-a);return b==1/0?1:e==1/0?-1:(b-e)/(d(a)+d(-a))}})},function(c,d,b){var a=b(3);a(a.S,"Math",{trunc:function trunc(a){return(a>0?Math.floor:Math.ceil)(a)}})},function(f,g,b){var a=b(3),e=b(23),c=String.fromCharCode,d=String.fromCodePoint;a(a.S+a.F*(!!d&&1!=d.length),"String",{fromCodePoint:function fromCodePoint(h){for(var a,b=[],d=arguments,g=d.length,f=0;g>f;){if(a=+d[f++],e(a,1114111)!==a)throw RangeError(a+" is not a valid code point");b.push(65536>a?c(a):c(((a-=65536)>>10)+55296,a%1024+56320))}return b.join("")}})},function(e,f,a){var b=a(3),c=a(20),d=a(24);b(b.S,"String",{raw:function raw(g){for(var e=c(g.raw),h=d(e.length),f=arguments,i=f.length,b=[],a=0;h>a;)b.push(String(e[a++])),i>a&&b.push(String(f[a]));return b.join("")}})},function(b,c,a){a(91)("trim",function(a){return function trim(){return a(this,3)}})},function(g,m,b){var c=b(3),h=b(19),i=b(9),d=" \n\f\r \u2028\u2029\ufeff",a="["+d+"]",f="
",j=RegExp("^"+a+a+"*"),k=RegExp(a+a+"*$"),e=function(a,e){var b={};b[a]=e(l),c(c.P+c.F*i(function(){return!!d[a]()||f[a]()!=f}),"String",b)},l=e.trim=function(a,b){return a=String(h(a)),1&b&&(a=a.replace(j,"")),2&b&&(a=a.replace(k,"")),a};g.exports=e},function(d,e,a){var b=a(3),c=a(93)(!1);b(b.P,"String",{codePointAt:function codePointAt(a){return c(this,a)}})},function(c,f,b){var d=b(22),e=b(19);c.exports=function(b){return function(j,k){var f,h,g=String(e(j)),c=d(k),i=g.length;return 0>c||c>=i?b?"":a:(f=g.charCodeAt(c),55296>f||f>56319||c+1===i||(h=g.charCodeAt(c+1))<56320||h>57343?b?g.charAt(c):f:b?g.slice(c,c+2):(f-55296<<10)+(h-56320)+65536)}}},function(h,i,b){var c=b(3),e=b(24),g=b(95),d="endsWith",f=""[d];c(c.P+c.F*b(97)(d),"String",{endsWith:function endsWith(i){var b=g(this,i,d),j=arguments,k=j.length>1?j[1]:a,l=e(b.length),c=k===a?l:Math.min(e(k),l),h=String(i);return f?f.call(b,h,c):b.slice(c-h.length,c)===h}})},function(b,e,a){var c=a(96),d=a(19);b.exports=function(a,b,e){if(c(b))throw TypeError("String#"+e+" doesn't accept regex!");return String(d(a))}},function(c,g,b){var d=b(13),e=b(15),f=b(29)("match");c.exports=function(b){var c;return d(b)&&((c=b[f])!==a?!!c:"RegExp"==e(b))}},function(a,d,b){var c=b(29)("match");a.exports=function(b){var a=/./;try{"/./"[b](a)}catch(d){try{return a[c]=!1,!"/./"[b](a)}catch(e){}}return!0}},function(f,g,b){var c=b(3),e=b(95),d="includes";c(c.P+c.F*b(97)(d),"String",{includes:function includes(b){return!!~e(this,b,d).indexOf(b,arguments.length>1?arguments[1]:a)}})},function(c,d,a){var b=a(3);b(b.P,"String",{repeat:a(100)})},function(b,e,a){var c=a(22),d=a(19);b.exports=function repeat(f){var b=String(d(this)),e="",a=c(f);if(0>a||a==1/0)throw RangeError("Count can't be negative");for(;a>0;(a>>>=1)&&(b+=b))1&a&&(e+=b);return e}},function(h,i,b){var c=b(3),f=b(24),g=b(95),d="startsWith",e=""[d];c(c.P+c.F*b(97)(d),"String",{startsWith:function startsWith(i){var b=g(this,i,d),j=arguments,c=f(Math.min(j.length>1?j[1]:a,b.length)),h=String(i);return e?e.call(b,h,c):b.slice(c,c+h.length)===h}})},function(d,e,b){var c=b(93)(!0);b(103)(String,"String",function(a){this._t=String(a),this._i=0},function(){var b,d=this._t,e=this._i;return e>=d.length?{value:a,done:!0}:(b=c(d,e),this._i+=b.length,{value:b,done:!1})})},function(o,r,a){var i=a(39),d=a(3),n=a(33),h=a(34),m=a(14),f=a(104),q=a(105),p=a(35),l=a(2).getProto,c=a(29)("iterator"),e=!([].keys&&"next"in[].keys()),j="@@iterator",k="keys",b="values",g=function(){return this};o.exports=function(B,v,u,F,s,E,A){q(u,v,F);var r,x,w=function(c){if(!e&&c in a)return a[c];switch(c){case k:return function keys(){return new u(this,c)};case b:return function values(){return new u(this,c)}}return function entries(){return new u(this,c)}},C=v+" Iterator",y=s==b,z=!1,a=B.prototype,t=a[c]||a[j]||s&&a[s],o=t||w(s);if(t){var D=l(o.call(new B));p(D,C,!0),!i&&m(a,j)&&h(D,c,g),y&&t.name!==b&&(z=!0,o=function values(){return t.call(this)})}if(i&&!A||!e&&!z&&a[c]||h(a,c,o),f[v]=o,f[C]=g,s)if(r={values:y?o:w(b),keys:E?o:w(k),entries:y?w("entries"):o},A)for(x in r)x in a||n(a,x,r[x]);else d(d.P+d.F*(e||z),v,r);return r}},function(a,b){a.exports={}},function(c,g,a){var d=a(2),e=a(10),f=a(35),b={};a(34)(b,a(29)("iterator"),function(){return this}),c.exports=function(a,c,g){a.prototype=d.create(b,{next:e(1,g)}),f(a,c+" Iterator")}},function(j,k,b){var d=b(6),c=b(3),e=b(18),f=b(107),g=b(108),h=b(24),i=b(109);c(c.S+c.F*!b(111)(function(a){Array.from(a)}),"Array",{from:function from(t){var n,c,r,m,j=e(t),l="function"==typeof this?this:Array,p=arguments,s=p.length,k=s>1?p[1]:a,q=k!==a,b=0,o=i(j);if(q&&(k=d(k,s>2?p[2]:a,2)),o==a||l==Array&&g(o))for(n=h(j.length),c=new l(n);n>b;b++)c[b]=q?k(j[b],b):j[b];else for(m=o.call(j),c=new l;!(r=m.next()).done;b++)c[b]=q?f(m,k,[r.value,b],!0):r.value;return c.length=b,c}})},function(c,e,d){var b=d(17);c.exports=function(d,e,c,g){try{return g?e(b(c)[0],c[1]):e(c)}catch(h){var f=d["return"];throw f!==a&&b(f.call(d)),h}}},function(c,g,b){var d=b(104),e=b(29)("iterator"),f=Array.prototype;c.exports=function(b){return b!==a&&(d.Array===b||f[e]===b)}},function(c,g,b){var d=b(110),e=b(29)("iterator"),f=b(104);c.exports=b(5).getIteratorMethod=function(b){return b!=a?b[e]||b["@@iterator"]||f[d(b)]:void 0}},function(d,g,c){var b=c(15),e=c(29)("toStringTag"),f="Arguments"==b(function(){return arguments}());d.exports=function(d){var c,g,h;return d===a?"Undefined":null===d?"Null":"string"==typeof(g=(c=Object(d))[e])?g:f?b(c):"Object"==(h=b(c))&&"function"==typeof c.callee?"Arguments":h}},function(d,f,e){var a=e(29)("iterator"),b=!1;try{var c=[7][a]();c["return"]=function(){b=!0},Array.from(c,function(){throw 2})}catch(g){}d.exports=function(f,g){if(!g&&!b)return!1;var d=!1;try{var c=[7],e=c[a]();e.next=function(){return{done:d=!0}},c[a]=function(){return e},f(c)}catch(h){}return d}},function(c,d,b){var a=b(3);a(a.S+a.F*b(9)(function(){function F(){}return!(Array.of.call(F)instanceof F)}),"Array",{of:function of(){for(var a=0,d=arguments,b=d.length,c=new("function"==typeof this?this:Array)(b);b>a;)c[a]=d[a++];return c.length=b,c}})},function(f,h,b){var d=b(114),c=b(115),e=b(104),g=b(20);f.exports=b(103)(Array,"Array",function(a,b){this._t=g(a),this._i=0,this._k=b},function(){var d=this._t,e=this._k,b=this._i++;return!d||b>=d.length?(this._t=a,c(1)):"keys"==e?c(0,b):"values"==e?c(0,d[b]):c(0,[b,d[b]])},"values"),e.Arguments=e.Array,d("keys"),d("values"),d("entries")},function(a,b){a.exports=function(){}},function(a,b){a.exports=function(a,b){return{value:b,done:!!a}}},function(b,c,a){a(117)("Array")},function(c,g,a){var d=a(5),e=a(2),f=a(8),b=a(29)("species");c.exports=function(c){var a=d[c];f&&a&&!a[b]&&e.setDesc(a,b,{configurable:!0,get:function(){return this}})}},function(c,d,a){var b=a(3);b(b.P,"Array",{copyWithin:a(119)}),a(114)("copyWithin")},function(d,g,b){var e=b(18),c=b(23),f=b(24);d.exports=[].copyWithin||function copyWithin(m,n){var g=e(this),h=f(g.length),b=c(m,h),d=c(n,h),k=arguments,l=k.length>2?k[2]:a,i=Math.min((l===a?h:c(l,h))-d,h-b),j=1;for(b>d&&d+i>b&&(j=-1,d+=i-1,b+=i-1);i-->0;)d in g?g[b]=g[d]:delete g[b],b+=j,d+=j;return g}},function(c,d,a){var b=a(3);b(b.P,"Array",{fill:a(121)}),a(114)("fill")},function(d,g,b){var e=b(18),c=b(23),f=b(24);d.exports=[].fill||function fill(k){for(var b=e(this),d=f(b.length),g=arguments,h=g.length,i=c(h>1?g[1]:a,d),j=h>2?g[2]:a,l=j===a?d:c(j,d);l>i;)b[i++]=k;return b}},function(g,h,b){var c=b(3),f=b(26)(5),d="find",e=!0;d in[]&&Array(1)[d](function(){e=!1}),c(c.P+c.F*e,"Array",{find:function find(b){return f(this,b,arguments.length>1?arguments[1]:a)}}),b(114)(d)},function(g,h,b){var c=b(3),f=b(26)(6),d="findIndex",e=!0;d in[]&&Array(1)[d](function(){e=!1}),c(c.P+c.F*e,"Array",{findIndex:function findIndex(b){return f(this,b,arguments.length>1?arguments[1]:a)}}),b(114)(d)},function(K,J,b){var s,l=b(2),F=b(39),k=b(4),j=b(6),I=b(110),d=b(3),D=b(13),E=b(17),m=b(7),G=b(125),p=b(126),q=b(45).set,A=b(43),B=b(29)("species"),z=b(127),v=b(128),e="Promise",o=k.process,H="process"==I(o),c=k[e],i=function(){},r=function(d){var b,a=new c(i);return d&&(a.constructor=function(a){a(i,i)}),(b=c.resolve(a))["catch"](i),b===a},h=function(){function P2(b){var a=new c(b);return q(a,P2.prototype),a}var a=!1;try{if(a=c&&c.resolve&&r(),q(P2,c),P2.prototype=l.create(c.prototype,{constructor:{value:P2}}),P2.resolve(5).then(function(){})instanceof P2||(a=!1),a&&b(8)){var d=!1;c.resolve(l.setDesc({},"then",{get:function(){d=!0}})),a=d}}catch(e){a=!1}return a}(),C=function(a,b){return F&&a===c&&b===s?!0:A(a,b)},t=function(b){var c=E(b)[B];return c!=a?c:b},u=function(a){var b;return D(a)&&"function"==typeof(b=a.then)?b:!1},g=function(d){var b,c;this.promise=new d(function(d,e){if(b!==a||c!==a)throw TypeError("Bad Promise constructor");b=d,c=e}),this.resolve=m(b),this.reject=m(c)},w=function(a){try{a()}catch(b){return{error:b}}},n=function(b,d){if(!b.n){b.n=!0;var c=b.c;v(function(){for(var e=b.v,f=1==b.s,g=0,h=function(a){var c,h,g=f?a.ok:a.fail,i=a.resolve,d=a.reject;try{g?(f||(b.h=!0),c=g===!0?e:g(e),c===a.promise?d(TypeError("Promise-chain cycle")):(h=u(c))?h.call(c,i,d):i(c)):d(e)}catch(j){d(j)}};c.length>g;)h(c[g++]);c.length=0,b.n=!1,d&&setTimeout(function(){var f,c,d=b.p;y(d)&&(H?o.emit("unhandledRejection",e,d):(f=k.onunhandledrejection)?f({promise:d,reason:e}):(c=k.console)&&c.error&&c.error("Unhandled promise rejection",e)),b.a=a},1)})}},y=function(e){var a,b=e._d,c=b.a||b.c,d=0;if(b.h)return!1;for(;c.length>d;)if(a=c[d++],a.fail||!y(a.promise))return!1;return!0},f=function(b){var a=this;a.d||(a.d=!0,a=a.r||a,a.v=b,a.s=2,a.a=a.c.slice(),n(a,!0))},x=function(b){var c,a=this;if(!a.d){a.d=!0,a=a.r||a;try{if(a.p===b)throw TypeError("Promise can't be resolved itself");(c=u(b))?v(function(){var d={r:a,d:!1};try{c.call(b,j(x,d,1),j(f,d,1))}catch(e){f.call(d,e)}}):(a.v=b,a.s=1,n(a,!1))}catch(d){f.call({r:a,d:!1},d)}}};h||(c=function Promise(d){m(d);var b=this._d={p:G(this,c,e),c:[],a:a,s:0,d:!1,v:a,h:!1,n:!1};try{d(j(x,b,1),j(f,b,1))}catch(g){f.call(b,g)}},b(130)(c.prototype,{then:function then(d,e){var a=new g(z(this,c)),f=a.promise,b=this._d;return a.ok="function"==typeof d?d:!0,a.fail="function"==typeof e&&e,b.c.push(a),b.a&&b.a.push(a),b.s&&n(b,!1),f},"catch":function(b){return this.then(a,b)}})),d(d.G+d.W+d.F*!h,{Promise:c}),b(35)(c,e),b(117)(e),s=b(5)[e],d(d.S+d.F*!h,e,{reject:function reject(b){var a=new g(this),c=a.reject;return c(b),a.promise}}),d(d.S+d.F*(!h||r(!0)),e,{resolve:function resolve(a){if(a instanceof c&&C(a.constructor,this))return a;var b=new g(this),d=b.resolve;return d(a),b.promise}}),d(d.S+d.F*!(h&&b(111)(function(a){c.all(a)["catch"](function(){})})),e,{all:function all(h){var c=t(this),b=new g(c),d=b.resolve,e=b.reject,a=[],f=w(function(){p(h,!1,a.push,a);var b=a.length,f=Array(b);b?l.each.call(a,function(g,h){var a=!1;c.resolve(g).then(function(c){a||(a=!0,f[h]=c,--b||d(f))},e)}):d(f)});return f&&e(f.error),b.promise},race:function race(e){var b=t(this),a=new g(b),c=a.reject,d=w(function(){p(e,!1,function(d){b.resolve(d).then(a.resolve,c)})});return d&&c(d.error),a.promise}})},function(a,b){a.exports=function(a,b,c){if(!(a instanceof b))throw TypeError(c+": use the 'new' operator!");return a}},function(b,i,a){var c=a(6),d=a(107),e=a(108),f=a(17),g=a(24),h=a(109);b.exports=function(a,j,o,p){var n,b,k,l=h(a),m=c(o,p,j?2:1),i=0;if("function"!=typeof l)throw TypeError(a+" is not iterable!");if(e(l))for(n=g(a.length);n>i;i++)j?m(f(b=a[i])[0],b[1]):m(a[i]);else for(k=l.call(a);!(b=k.next()).done;)d(k,m,b.value,j)}},function(d,g,b){var c=b(17),e=b(7),f=b(29)("species");d.exports=function(g,h){var b,d=c(g).constructor;return d===a||(b=c(d)[f])==a?h:e(b)}},function(n,p,h){var b,f,g,c=h(4),o=h(129).set,k=c.MutationObserver||c.WebKitMutationObserver,d=c.process,i=c.Promise,j="process"==h(15)(d),e=function(){var e,c,g;for(j&&(e=d.domain)&&(d.domain=null,e.exit());b;)c=b.domain,g=b.fn,c&&c.enter(),g(),c&&c.exit(),b=b.next;f=a,e&&e.enter()};if(j)g=function(){d.nextTick(e)};else if(k){var m=1,l=document.createTextNode("");new k(e).observe(l,{characterData:!0}),g=function(){l.data=m=-m}}else g=i&&i.resolve?function(){i.resolve().then(e)}:function(){o.call(c,e)};n.exports=function asap(e){var c={fn:e,next:a,domain:j&&d.domain};f&&(f.next=c),b||(b=c,g()),f=c}},function(s,t,b){var c,g,f,k=b(6),r=b(16),n=b(11),p=b(12),a=b(4),l=a.process,h=a.setImmediate,i=a.clearImmediate,o=a.MessageChannel,j=0,d={},q="onreadystatechange",e=function(){
+var a=+this;if(d.hasOwnProperty(a)){var b=d[a];delete d[a],b()}},m=function(a){e.call(a.data)};h&&i||(h=function setImmediate(a){for(var b=[],e=1;arguments.length>e;)b.push(arguments[e++]);return d[++j]=function(){r("function"==typeof a?a:Function(a),b)},c(j),j},i=function clearImmediate(a){delete d[a]},"process"==b(15)(l)?c=function(a){l.nextTick(k(e,a,1))}:o?(g=new o,f=g.port2,g.port1.onmessage=m,c=k(f.postMessage,f,1)):a.addEventListener&&"function"==typeof postMessage&&!a.importScripts?(c=function(b){a.postMessage(b+"","*")},a.addEventListener("message",m,!1)):c=q in p("script")?function(a){n.appendChild(p("script"))[q]=function(){n.removeChild(this),e.call(a)}}:function(a){setTimeout(k(e,a,1),0)}),s.exports={set:h,clear:i}},function(a,d,b){var c=b(33);a.exports=function(a,b){for(var d in b)c(a,d,b[d]);return a}},function(d,e,c){var b=c(132);c(133)("Map",function(b){return function Map(){return b(this,arguments.length>0?arguments[0]:a)}},{get:function get(c){var a=b.getEntry(this,c);return a&&a.v},set:function set(a,c){return b.def(this,0===a?0:a,c)}},b,!0)},function(v,w,b){var j=b(2),m=b(34),o=b(130),n=b(6),p=b(125),r=b(19),t=b(126),l=b(103),d=b(115),f=b(25)("id"),k=b(14),h=b(13),q=b(117),i=b(8),s=Object.isExtensible||h,c=i?"_s":"size",u=0,g=function(a,b){if(!h(a))return"symbol"==typeof a?a:("string"==typeof a?"S":"P")+a;if(!k(a,f)){if(!s(a))return"F";if(!b)return"E";m(a,f,++u)}return"O"+a[f]},e=function(b,c){var a,d=g(c);if("F"!==d)return b._i[d];for(a=b._f;a;a=a.n)if(a.k==c)return a};v.exports={getConstructor:function(d,f,g,h){var b=d(function(d,e){p(d,b,f),d._i=j.create(null),d._f=a,d._l=a,d[c]=0,e!=a&&t(e,g,d[h],d)});return o(b.prototype,{clear:function clear(){for(var d=this,e=d._i,b=d._f;b;b=b.n)b.r=!0,b.p&&(b.p=b.p.n=a),delete e[b.i];d._f=d._l=a,d[c]=0},"delete":function(g){var b=this,a=e(b,g);if(a){var d=a.n,f=a.p;delete b._i[a.i],a.r=!0,f&&(f.n=d),d&&(d.p=f),b._f==a&&(b._f=d),b._l==a&&(b._l=f),b[c]--}return!!a},forEach:function forEach(c){for(var b,d=n(c,arguments.length>1?arguments[1]:a,3);b=b?b.n:this._f;)for(d(b.v,b.k,this);b&&b.r;)b=b.p},has:function has(a){return!!e(this,a)}}),i&&j.setDesc(b.prototype,"size",{get:function(){return r(this[c])}}),b},def:function(b,f,j){var h,i,d=e(b,f);return d?d.v=j:(b._l=d={i:i=g(f,!0),k:f,v:j,p:h=b._l,n:a,r:!1},b._f||(b._f=d),h&&(h.n=d),b[c]++,"F"!==i&&(b._i[i]=d)),b},getEntry:e,setStrong:function(e,b,c){l(e,b,function(b,c){this._t=b,this._k=c,this._l=a},function(){for(var c=this,e=c._k,b=c._l;b&&b.r;)b=b.p;return c._t&&(c._l=b=b?b.n:c._t._f)?"keys"==e?d(0,b.k):"values"==e?d(0,b.v):d(0,[b.k,b.v]):(c._t=a,d(1))},c?"entries":"values",!c,!0),q(b)}}},function(g,o,b){var d=b(2),f=b(4),c=b(3),h=b(9),e=b(34),j=b(130),k=b(126),l=b(125),m=b(13),i=b(35),n=b(8);g.exports=function(g,s,w,r,p,o){var t=f[g],b=t,u=p?"set":"add",q=b&&b.prototype,v={};return n&&"function"==typeof b&&(o||q.forEach&&!h(function(){(new b).entries().next()}))?(b=s(function(c,d){l(c,b,g),c._c=new t,d!=a&&k(d,p,c[u],c)}),d.each.call("add,clear,delete,forEach,get,has,set,keys,values,entries".split(","),function(c){var d="add"==c||"set"==c;c in q&&(!o||"clear"!=c)&&e(b.prototype,c,function(b,e){if(!d&&o&&!m(b))return"get"==c?a:!1;var f=this._c[c](0===b?0:b,e);return d?this:f})}),"size"in q&&d.setDesc(b.prototype,"size",{get:function(){return this._c.size}})):(b=r.getConstructor(s,g,p,u),j(b.prototype,w)),i(b,g),v[g]=b,c(c.G+c.W+c.F,v),o||r.setStrong(b,g,p),b}},function(d,e,b){var c=b(132);b(133)("Set",function(b){return function Set(){return b(this,arguments.length>0?arguments[0]:a)}},{add:function add(a){return c.def(this,a=0===a?0:a,a)}},c)},function(n,m,b){var l=b(2),k=b(33),c=b(136),d=b(13),j=b(14),i=c.frozenStore,h=c.WEAK,f=Object.isExtensible||d,e={},g=b(133)("WeakMap",function(b){return function WeakMap(){return b(this,arguments.length>0?arguments[0]:a)}},{get:function get(a){if(d(a)){if(!f(a))return i(this).get(a);if(j(a,h))return a[h][this._i]}},set:function set(a,b){return c.def(this,a,b)}},c,!0,!0);7!=(new g).set((Object.freeze||Object)(e),7).get(e)&&l.each.call(["delete","has","get","set"],function(a){var b=g.prototype,c=b[a];k(b,a,function(b,e){if(d(b)&&!f(b)){var g=i(this)[a](b,e);return"set"==a?this:g}return c.call(this,b,e)})})},function(s,t,b){var r=b(34),q=b(130),m=b(17),h=b(13),l=b(125),k=b(126),j=b(26),d=b(14),c=b(25)("weak"),g=Object.isExtensible||h,n=j(5),o=j(6),p=0,e=function(a){return a._l||(a._l=new i)},i=function(){this.a=[]},f=function(a,b){return n(a.a,function(a){return a[0]===b})};i.prototype={get:function(b){var a=f(this,b);return a?a[1]:void 0},has:function(a){return!!f(this,a)},set:function(a,b){var c=f(this,a);c?c[1]=b:this.a.push([a,b])},"delete":function(b){var a=o(this.a,function(a){return a[0]===b});return~a&&this.a.splice(a,1),!!~a}},s.exports={getConstructor:function(f,i,j,m){var b=f(function(c,d){l(c,b,i),c._i=p++,c._l=a,d!=a&&k(d,j,c[m],c)});return q(b.prototype,{"delete":function(a){return h(a)?g(a)?d(a,c)&&d(a[c],this._i)&&delete a[c][this._i]:e(this)["delete"](a):!1},has:function has(a){return h(a)?g(a)?d(a,c)&&d(a[c],this._i):e(this).has(a):!1}}),b},def:function(b,a,f){return g(m(a))?(d(a,c)||r(a,c,{}),a[c][b._i]=f):e(b).set(a,f),b},frozenStore:e,WEAK:c}},function(d,e,b){var c=b(136);b(133)("WeakSet",function(b){return function WeakSet(){return b(this,arguments.length>0?arguments[0]:a)}},{add:function add(a){return c.def(this,a,!0)}},c,!1,!0)},function(e,f,a){var b=a(3),c=Function.apply,d=a(17);b(b.S,"Reflect",{apply:function apply(a,b,e){return c.call(a,b,d(e))}})},function(h,i,a){var e=a(2),b=a(3),c=a(7),f=a(17),d=a(13),g=Function.bind||a(5).Function.prototype.bind;b(b.S+b.F*a(9)(function(){function F(){}return!(Reflect.construct(function(){},[],F)instanceof F)}),"Reflect",{construct:function construct(b,a){c(b),f(a);var i=arguments.length<3?b:c(arguments[2]);if(b==i){switch(a.length){case 0:return new b;case 1:return new b(a[0]);case 2:return new b(a[0],a[1]);case 3:return new b(a[0],a[1],a[2]);case 4:return new b(a[0],a[1],a[2],a[3])}var h=[null];return h.push.apply(h,a),new(g.apply(b,h))}var j=i.prototype,k=e.create(d(j)?j:Object.prototype),l=Function.apply.call(b,k,a);return d(l)?l:k}})},function(e,f,a){var c=a(2),b=a(3),d=a(17);b(b.S+b.F*a(9)(function(){Reflect.defineProperty(c.setDesc({},1,{value:1}),1,{value:2})}),"Reflect",{defineProperty:function defineProperty(a,b,e){d(a);try{return c.setDesc(a,b,e),!0}catch(f){return!1}}})},function(e,f,a){var b=a(3),c=a(2).getDesc,d=a(17);b(b.S,"Reflect",{deleteProperty:function deleteProperty(a,b){var e=c(d(a),b);return e&&!e.configurable?!1:delete a[b]}})},function(f,g,b){var c=b(3),e=b(17),d=function(a){this._t=e(a),this._i=0;var b,c=this._k=[];for(b in a)c.push(b)};b(105)(d,"Object",function(){var c,b=this,d=b._k;do if(b._i>=d.length)return{value:a,done:!0};while(!((c=d[b._i++])in b._t));return{value:c,done:!1}}),c(c.S,"Reflect",{enumerate:function enumerate(a){return new d(a)}})},function(h,i,b){function get(b,h){var d,j,i=arguments.length<3?b:arguments[2];return g(b)===i?b[h]:(d=c.getDesc(b,h))?e(d,"value")?d.value:d.get!==a?d.get.call(i):a:f(j=c.getProto(b))?get(j,h,i):void 0}var c=b(2),e=b(14),d=b(3),f=b(13),g=b(17);d(d.S,"Reflect",{get:get})},function(e,f,a){var c=a(2),b=a(3),d=a(17);b(b.S,"Reflect",{getOwnPropertyDescriptor:function getOwnPropertyDescriptor(a,b){return c.getDesc(d(a),b)}})},function(e,f,a){var b=a(3),c=a(2).getProto,d=a(17);b(b.S,"Reflect",{getPrototypeOf:function getPrototypeOf(a){return c(d(a))}})},function(c,d,b){var a=b(3);a(a.S,"Reflect",{has:function has(a,b){return b in a}})},function(e,f,a){var b=a(3),d=a(17),c=Object.isExtensible;b(b.S,"Reflect",{isExtensible:function isExtensible(a){return d(a),c?c(a):!0}})},function(c,d,a){var b=a(3);b(b.S,"Reflect",{ownKeys:a(149)})},function(d,f,a){var b=a(2),e=a(17),c=a(4).Reflect;d.exports=c&&c.ownKeys||function ownKeys(a){var c=b.getNames(e(a)),d=b.getSymbols;return d?c.concat(d(a)):c}},function(e,f,a){var b=a(3),d=a(17),c=Object.preventExtensions;b(b.S,"Reflect",{preventExtensions:function preventExtensions(a){d(a);try{return c&&c(a),!0}catch(b){return!1}}})},function(i,j,b){function set(j,i,k){var l,m,d=arguments.length<4?j:arguments[3],b=c.getDesc(h(j),i);if(!b){if(f(m=c.getProto(j)))return set(m,i,k,d);b=e(0)}return g(b,"value")?b.writable!==!1&&f(d)?(l=c.getDesc(d,i)||e(0),l.value=k,c.setDesc(d,i,l),!0):!1:b.set===a?!1:(b.set.call(d,k),!0)}var c=b(2),g=b(14),d=b(3),e=b(10),h=b(17),f=b(13);d(d.S,"Reflect",{set:set})},function(d,e,b){var c=b(3),a=b(45);a&&c(c.S,"Reflect",{setPrototypeOf:function setPrototypeOf(b,c){a.check(b,c);try{return a.set(b,c),!0}catch(d){return!1}}})},function(e,f,b){var c=b(3),d=b(31)(!0);c(c.P,"Array",{includes:function includes(b){return d(this,b,arguments.length>1?arguments[1]:a)}}),b(114)("includes")},function(d,e,a){var b=a(3),c=a(93)(!0);b(b.P,"String",{at:function at(a){return c(this,a)}})},function(e,f,b){var c=b(3),d=b(156);c(c.P,"String",{padLeft:function padLeft(b){return d(this,b,arguments.length>1?arguments[1]:a,!0)}})},function(c,g,b){var d=b(24),e=b(100),f=b(19);c.exports=function(l,m,i,n){var c=String(f(l)),j=c.length,g=i===a?" ":String(i),k=d(m);if(j>=k)return c;""==g&&(g=" ");var h=k-j,b=e.call(g,Math.ceil(h/g.length));return b.length>h&&(b=b.slice(0,h)),n?b+c:c+b}},function(e,f,b){var c=b(3),d=b(156);c(c.P,"String",{padRight:function padRight(b){return d(this,b,arguments.length>1?arguments[1]:a,!1)}})},function(b,c,a){a(91)("trimLeft",function(a){return function trimLeft(){return a(this,1)}})},function(b,c,a){a(91)("trimRight",function(a){return function trimRight(){return a(this,2)}})},function(d,e,a){var b=a(3),c=a(161)(/[\\^$*+?.()|[\]{}]/g,"\\$&");b(b.S,"RegExp",{escape:function escape(a){return c(a)}})},function(a,b){a.exports=function(b,a){var c=a===Object(a)?function(b){return a[b]}:a;return function(a){return String(a).replace(b,c)}}},function(g,h,a){var b=a(2),c=a(3),d=a(149),e=a(20),f=a(10);c(c.S,"Object",{getOwnPropertyDescriptors:function getOwnPropertyDescriptors(k){for(var a,g,h=e(k),l=b.setDesc,m=b.getDesc,i=d(h),c={},j=0;i.length>j;)g=m(h,a=i[j++]),a in c?l(c,a,f(0,g)):c[a]=g;return c}})},function(d,e,a){var b=a(3),c=a(164)(!1);b(b.S,"Object",{values:function values(a){return c(a)}})},function(c,f,a){var b=a(2),d=a(20),e=b.isEnum;c.exports=function(a){return function(j){for(var c,f=d(j),g=b.getKeys(f),k=g.length,h=0,i=[];k>h;)e.call(f,c=g[h++])&&i.push(a?[c,f[c]]:f[c]);return i}}},function(d,e,a){var b=a(3),c=a(164)(!0);b(b.S,"Object",{entries:function entries(a){return c(a)}})},function(c,d,a){var b=a(3);b(b.P,"Map",{toJSON:a(167)("Map")})},function(b,e,a){var c=a(126),d=a(110);b.exports=function(a){return function toJSON(){if(d(this)!=a)throw TypeError(a+"#toJSON isn't generic");var b=[];return c(this,!1,b.push,b),b}}},function(c,d,a){var b=a(3);b(b.P,"Set",{toJSON:a(167)("Set")})},function(d,e,b){var a=b(3),c=b(129);a(a.G+a.B,{setImmediate:c.set,clearImmediate:c.clear})},function(c,d,b){b(113);var a=b(104);a.NodeList=a.HTMLCollection=a.Array},function(i,j,a){var c=a(4),b=a(3),g=a(16),h=a(172),d=c.navigator,e=!!d&&/MSIE .\./.test(d.userAgent),f=function(a){return e?function(b,c){return a(g(h,[].slice.call(arguments,2),"function"==typeof b?b:Function(b)),c)}:a};b(b.G+b.B+b.F*e,{setTimeout:f(c.setTimeout),setInterval:f(c.setInterval)})},function(c,f,a){var d=a(173),b=a(16),e=a(7);c.exports=function(){for(var h=e(this),a=arguments.length,c=Array(a),f=0,i=d._,g=!1;a>f;)(c[f]=arguments[f++])===i&&(g=!0);return function(){var d,k=this,f=arguments,l=f.length,e=0,j=0;if(!g&&!l)return b(h,c,k);if(d=c.slice(),g)for(;a>e;e++)d[e]===i&&(d[e]=f[j++]);for(;l>j;)d.push(f[j++]);return b(h,d,k)}}},function(a,c,b){a.exports=b(5)},function(x,w,b){function Dict(b){var c=f.create(null);return b!=a&&(r(b)?q(b,!0,function(a,b){c[a]=b}):o(c,b)),c}function reduce(g,h,l){p(h);var a,c,b=i(g),e=k(b),j=e.length,f=0;if(arguments.length<3){if(!j)throw TypeError("Reduce of empty object with no initial value");a=b[e[f++]]}else a=Object(l);for(;j>f;)d(b,c=e[f++])&&(a=h(a,b[c],c,g));return a}function includes(c,b){return(b==b?j(c,b):l(c,function(a){return a!=a}))!==a}function get(a,b){return d(a,b)?a[b]:void 0}function set(a,b,c){return v&&b in Object?f.setDesc(a,b,t(0,c)):a[b]=c,a}function isDict(a){return u(a)&&f.getProto(a)===Dict.prototype}var f=b(2),n=b(6),e=b(3),t=b(10),o=b(41),j=b(36),p=b(7),q=b(126),r=b(175),s=b(105),g=b(115),u=b(13),i=b(20),v=b(8),d=b(14),k=f.getKeys,c=function(b){var e=1==b,c=4==b;return function(l,m,o){var f,h,g,p=n(m,o,3),k=i(l),j=e||7==b||2==b?new("function"==typeof this?this:Dict):a;for(f in k)if(d(k,f)&&(h=k[f],g=p(h,f,l),b))if(e)j[f]=g;else if(g)switch(b){case 2:j[f]=h;break;case 3:return!0;case 5:return h;case 6:return f;case 7:j[g[0]]=g[1]}else if(c)return!1;return 3==b||c?c:j}},l=c(6),h=function(a){return function(b){return new m(b,a)}},m=function(a,b){this._t=i(a),this._a=k(a),this._i=0,this._k=b};s(m,"Dict",function(){var c,b=this,e=b._t,f=b._a,h=b._k;do if(b._i>=f.length)return b._t=a,g(1);while(!d(e,c=f[b._i++]));return"keys"==h?g(0,c):"values"==h?g(0,e[c]):g(0,[c,e[c]])}),Dict.prototype=null,e(e.G+e.F,{Dict:Dict}),e(e.S,"Dict",{keys:h("keys"),values:h("values"),entries:h("entries"),forEach:c(0),map:c(1),filter:c(2),some:c(3),every:c(4),find:c(5),findKey:l,mapPairs:c(7),reduce:reduce,keyOf:j,includes:includes,has:d,get:get,set:set,isDict:isDict})},function(c,g,b){var d=b(110),e=b(29)("iterator"),f=b(104);c.exports=b(5).isIterable=function(c){var b=Object(c);return b[e]!==a||"@@iterator"in b||f.hasOwnProperty(d(b))}},function(b,e,a){var c=a(17),d=a(109);b.exports=a(5).getIterator=function(a){var b=d(a);if("function"!=typeof b)throw TypeError(a+" is not iterable!");return c(b.call(a))}},function(f,g,a){var c=a(4),d=a(5),b=a(3),e=a(172);b(b.G+b.F,{delay:function delay(a){return new(d.Promise||c.Promise)(function(b){setTimeout(e.call(b,!0),a)})}})},function(d,e,a){var c=a(173),b=a(3);a(5)._=c._=c._||{},b(b.P+b.F,"Function",{part:a(172)})},function(c,d,b){var a=b(3);a(a.S+a.F,"Object",{isObject:b(13)})},function(c,d,b){var a=b(3);a(a.S+a.F,"Object",{classof:b(110)})},function(d,e,b){var a=b(3),c=b(182);a(a.S+a.F,"Object",{define:c})},function(c,f,a){var b=a(2),d=a(149),e=a(20);c.exports=function define(a,c){for(var f,g=d(e(c)),i=g.length,h=0;i>h;)b.setDesc(a,f=g[h++],b.getDesc(c,f));return a}},function(e,f,a){var b=a(3),c=a(182),d=a(2).create;b(b.S+b.F,"Object",{make:function(a,b){return c(d(a),b)}})},function(c,d,b){b(103)(Number,"Number",function(a){this._l=+a,this._i=0},function(){var b=this._i++,c=!(this._l>b);return{done:c,value:c?a:b}})},function(d,e,b){var a=b(3),c=b(161)(/[&<>"']/g,{"&":"&","<":"<",">":">",'"':""","'":"'"});a(a.P+a.F,"String",{escapeHTML:function escapeHTML(){return c(this)}})},function(d,e,b){var a=b(3),c=b(161)(/&(?:amp|lt|gt|quot|apos);/g,{"&":"&","<":"<",">":">",""":'"',"'":"'"});a(a.P+a.F,"String",{unescapeHTML:function unescapeHTML(){return c(this)}})},function(g,h,a){var e=a(2),f=a(4),b=a(3),c={},d=!0;e.each.call("assert,clear,count,debug,dir,dirxml,error,exception,group,groupCollapsed,groupEnd,info,isIndependentlyComposed,log,markTimeline,profile,profileEnd,table,time,timeEnd,timeline,timelineEnd,timeStamp,trace,warn".split(","),function(a){c[a]=function(){var b=f.console;return d&&b&&b[a]?Function.apply.call(b[a],b,arguments):void 0}}),b(b.G+b.F,{log:a(41)(c.log,c,{enable:function(){d=!0},disable:function(){d=!1}})})},function(i,j,b){var g=b(2),e=b(3),h=b(6),f=b(5).Array||Array,c={},d=function(d,b){g.each.call(d.split(","),function(d){b==a&&d in f?c[d]=f[d]:d in[]&&(c[d]=h(Function.call,[][d],b))})};d("pop,reverse,shift,keys,values,entries",1),d("indexOf,every,some,forEach,map,filter,find,findIndex,includes",3),d("join,slice,concat,push,splice,unshift,sort,lastIndexOf,reduce,reduceRight,copyWithin,fill"),e(e.S,"Array",c)}]),"undefined"!=typeof module&&module.exports?module.exports=b:"function"==typeof define&&define.amd?define(function(){return b}):c.core=b}(1,1);
+//# sourceMappingURL=library.min.js.map
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/library.min.js.map b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/library.min.js.map
new file mode 100644
index 00000000..56305ad6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/library.min.js.map
@@ -0,0 +1 @@
+{"version":3,"sources":["library.js"],"names":["__e","__g","undefined","modules","__webpack_require__","moduleId","installedModules","exports","module","id","loaded","call","m","c","p","IE8_DOM_DEFINE","$","$export","DESCRIPTORS","createDesc","html","cel","has","cof","invoke","fails","anObject","aFunction","isObject","toObject","toIObject","toInteger","toIndex","toLength","IObject","IE_PROTO","createArrayMethod","arrayIndexOf","ObjectProto","Object","prototype","ArrayProto","Array","arraySlice","slice","arrayJoin","join","defineProperty","setDesc","getOwnDescriptor","getDesc","defineProperties","setDescs","factories","get","a","O","P","Attributes","e","TypeError","value","propertyIsEnumerable","Properties","keys","getKeys","length","i","S","F","getOwnPropertyDescriptor","keys1","split","keys2","concat","keysLen1","createDict","iframeDocument","iframe","gt","style","display","appendChild","src","contentWindow","document","open","write","close","createGetKeys","names","object","key","result","push","Empty","getPrototypeOf","getProto","constructor","getOwnPropertyNames","getNames","create","construct","len","args","n","Function","bind","that","fn","this","partArgs","arguments","bound","begin","end","klass","start","upTo","size","cloned","charAt","separator","isArray","createArrayReduce","isRight","callbackfn","memo","index","methodize","$fn","arg1","forEach","each","map","filter","some","every","reduce","reduceRight","indexOf","lastIndexOf","el","fromIndex","Math","min","now","Date","lz","num","toISOString","NaN","isFinite","RangeError","d","y","getUTCFullYear","getUTCMilliseconds","s","abs","getUTCMonth","getUTCDate","getUTCHours","getUTCMinutes","getUTCSeconds","$Object","isEnum","getSymbols","getOwnPropertySymbols","global","core","ctx","PROTOTYPE","type","name","source","own","out","IS_FORCED","IS_GLOBAL","G","IS_STATIC","IS_PROTO","IS_BIND","B","IS_WRAP","W","target","C","param","window","self","version","b","apply","it","exec","bitmap","enumerable","configurable","writable","documentElement","is","createElement","hasOwnProperty","toString","un","defined","ceil","floor","isNaN","max","px","random","asc","TYPE","IS_MAP","IS_FILTER","IS_SOME","IS_EVERY","IS_FIND_INDEX","NO_HOLES","$this","val","res","f","SPECIES","original","arg","store","uid","Symbol","SHARED","IS_INCLUDES","redefine","$fails","shared","setToStringTag","wks","keyOf","$names","enumKeys","_create","$Symbol","$JSON","JSON","_stringify","stringify","setter","HIDDEN","SymbolRegistry","AllSymbols","useNative","setSymbolDesc","D","protoDesc","wrap","tag","sym","_k","set","isSymbol","$defineProperty","$defineProperties","l","$create","$propertyIsEnumerable","E","$getOwnPropertyDescriptor","$getOwnPropertyNames","$getOwnPropertySymbols","$stringify","replacer","$replacer","$$","buggyJSON","symbolStatics","for","keyFor","useSetter","useSimple","def","TAG","stat","windowNames","getWindowNames","symbols","assign","A","K","k","T","$$len","j","x","setPrototypeOf","check","proto","test","buggy","__proto__","$freeze","freeze","KEY","exp","$seal","seal","$preventExtensions","preventExtensions","$isFrozen","isFrozen","$isSealed","isSealed","$isExtensible","isExtensible","$getPrototypeOf","$keys","HAS_INSTANCE","FunctionProto","EPSILON","pow","_isFinite","isInteger","number","isSafeInteger","MAX_SAFE_INTEGER","MIN_SAFE_INTEGER","parseFloat","parseInt","log1p","sqrt","$acosh","acosh","Number","MAX_VALUE","log","LN2","asinh","atanh","sign","cbrt","clz32","LOG2E","cosh","expm1","EPSILON32","MAX32","MIN32","roundTiesToEven","fround","$abs","$sign","Infinity","hypot","value1","value2","div","sum","larg","$imul","imul","UINT16","xn","yn","xl","yl","log10","LN10","log2","sinh","tanh","trunc","fromCharCode","String","$fromCodePoint","fromCodePoint","code","raw","callSite","tpl","$trim","trim","spaces","space","non","ltrim","RegExp","rtrim","exporter","string","replace","$at","codePointAt","pos","TO_STRING","charCodeAt","context","ENDS_WITH","$endsWith","endsWith","searchString","endPosition","search","isRegExp","NAME","MATCH","re","INCLUDES","includes","repeat","count","str","STARTS_WITH","$startsWith","startsWith","iterated","_t","_i","point","done","LIBRARY","hide","Iterators","$iterCreate","ITERATOR","BUGGY","FF_ITERATOR","KEYS","VALUES","returnThis","Base","Constructor","next","DEFAULT","IS_SET","FORCED","methods","getMethod","kind","values","entries","DEF_VALUES","VALUES_BUG","$native","$default","IteratorPrototype","descriptor","isArrayIter","getIterFn","iter","from","arrayLike","step","iterator","mapfn","mapping","iterFn","ret","classof","getIteratorMethod","ARG","callee","SAFE_CLOSING","riter","skipClosing","safe","arr","of","addToUnscopables","Arguments","copyWithin","to","inc","fill","endPos","$find","forced","find","findIndex","Wrapper","strictNew","forOf","setProto","same","speciesConstructor","asap","PROMISE","process","isNode","empty","testResolve","sub","promise","resolve","USE_NATIVE","P2","works","then","thenableThenGotten","sameConstructor","getConstructor","isThenable","PromiseCapability","reject","$$resolve","$$reject","perform","error","notify","record","isReject","chain","v","ok","run","reaction","handler","fail","h","setTimeout","console","isUnhandled","emit","onunhandledrejection","reason","_d","$reject","r","$resolve","wrapper","Promise","executor","err","onFulfilled","onRejected","catch","capability","all","iterable","abrupt","remaining","results","alreadyCalled","race","head","last","macrotask","Observer","MutationObserver","WebKitMutationObserver","flush","parent","domain","exit","enter","nextTick","toggle","node","createTextNode","observe","characterData","data","task","defer","channel","port","setTask","setImmediate","clearTask","clearImmediate","MessageChannel","counter","queue","ONREADYSTATECHANGE","listner","event","port2","port1","onmessage","postMessage","addEventListener","importScripts","removeChild","clear","strong","Map","entry","getEntry","redefineAll","$iterDefine","ID","$has","setSpecies","SIZE","fastKey","_f","ADDER","_l","delete","prev","setStrong","common","IS_WEAK","_c","IS_ADDER","Set","add","weak","frozenStore","WEAK","tmp","$WeakMap","WeakMap","method","arrayFind","arrayFindIndex","FrozenStore","findFrozen","splice","WeakSet","_apply","thisArgument","argumentsList","Reflect","Target","newTarget","$args","instance","propertyKey","attributes","deleteProperty","desc","Enumerate","enumerate","receiver","ownKeys","V","existingDescriptor","ownDesc","$includes","at","$pad","padLeft","maxLength","fillString","left","stringLength","fillStr","intMaxLength","fillLen","stringFiller","padRight","trimLeft","trimRight","$re","escape","regExp","part","getOwnPropertyDescriptors","$values","isEntries","$entries","toJSON","$task","NodeList","HTMLCollection","partial","navigator","MSIE","userAgent","time","setInterval","path","pargs","_","holder","Dict","dict","isIterable","init","findKey","isDict","createDictMethod","createDictIter","DictIterator","_a","mapPairs","getIterator","delay","define","mixin","make","&","<",">","\"","'","escapeHTML","&","<",">",""","'","unescapeHTML","enabled","$console","enable","disable","$ctx","$Array","statics","setStatics","amd"],"mappings":";;;;;;CAMC,SAASA,EAAKC,EAAKC,GACpB,cACS,SAAUC,GAKT,QAASC,qBAAoBC,GAG5B,GAAGC,EAAiBD,GACnB,MAAOC,GAAiBD,GAAUE,OAGnC,IAAIC,GAASF,EAAiBD,IAC7BE,WACAE,GAAIJ,EACJK,QAAQ,EAUT,OANAP,GAAQE,GAAUM,KAAKH,EAAOD,QAASC,EAAQA,EAAOD,QAASH,qBAG/DI,EAAOE,QAAS,EAGTF,EAAOD,QAvBf,GAAID,KAqCJ,OATAF,qBAAoBQ,EAAIT,EAGxBC,oBAAoBS,EAAIP,EAGxBF,oBAAoBU,EAAI,GAGjBV,oBAAoB,KAK/B,SAASI,EAAQD,EAASH,GAE/BA,EAAoB,GACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBI,EAAOD,QAAUH,EAAoB,MAKhC,SAASI,EAAQD,EAASH,GAG/B,GA8BIW,GA9BAC,EAAoBZ,EAAoB,GACxCa,EAAoBb,EAAoB,GACxCc,EAAoBd,EAAoB,GACxCe,EAAoBf,EAAoB,IACxCgB,EAAoBhB,EAAoB,IACxCiB,EAAoBjB,EAAoB,IACxCkB,EAAoBlB,EAAoB,IACxCmB,EAAoBnB,EAAoB,IACxCoB,EAAoBpB,EAAoB,IACxCqB,EAAoBrB,EAAoB,GACxCsB,EAAoBtB,EAAoB,IACxCuB,EAAoBvB,EAAoB,GACxCwB,EAAoBxB,EAAoB,IACxCyB,EAAoBzB,EAAoB,IACxC0B,EAAoB1B,EAAoB,IACxC2B,EAAoB3B,EAAoB,IACxC4B,EAAoB5B,EAAoB,IACxC6B,EAAoB7B,EAAoB,IACxC8B,EAAoB9B,EAAoB,IACxC+B,EAAoB/B,EAAoB,IAAI,aAC5CgC,EAAoBhC,EAAoB,IACxCiC,EAAoBjC,EAAoB,KAAI,GAC5CkC,EAAoBC,OAAOC,UAC3BC,EAAoBC,MAAMF,UAC1BG,EAAoBF,EAAWG,MAC/BC,EAAoBJ,EAAWK,KAC/BC,EAAoB/B,EAAEgC,QACtBC,EAAoBjC,EAAEkC,QACtBC,EAAoBnC,EAAEoC,SACtBC,IAGAnC,KACFH,GAAkBU,EAAM,WACtB,MAA4E,IAArEsB,EAAe1B,EAAI,OAAQ,KAAMiC,IAAK,WAAY,MAAO,MAAOC,IAEzEvC,EAAEgC,QAAU,SAASQ,EAAGC,EAAGC,GACzB,GAAG3C,EAAe,IAChB,MAAOgC,GAAeS,EAAGC,EAAGC,GAC5B,MAAMC,IACR,GAAG,OAASD,IAAc,OAASA,GAAW,KAAME,WAAU,2BAE9D,OADG,SAAWF,KAAWhC,EAAS8B,GAAGC,GAAKC,EAAWG,OAC9CL,GAETxC,EAAEkC,QAAU,SAASM,EAAGC,GACtB,GAAG1C,EAAe,IAChB,MAAOkC,GAAiBO,EAAGC,GAC3B,MAAME,IACR,MAAGrC,GAAIkC,EAAGC,GAAUtC,GAAYmB,EAAYwB,qBAAqBnD,KAAK6C,EAAGC,GAAID,EAAEC,IAA/E,QAEFzC,EAAEoC,SAAWD,EAAmB,SAASK,EAAGO,GAC1CrC,EAAS8B,EAKT,KAJA,GAGIC,GAHAO,EAAShD,EAAEiD,QAAQF,GACnBG,EAASF,EAAKE,OACdC,EAAI,EAEFD,EAASC,GAAEnD,EAAEgC,QAAQQ,EAAGC,EAAIO,EAAKG,KAAMJ,EAAWN,GACxD,OAAOD,KAGXvC,EAAQA,EAAQmD,EAAInD,EAAQoD,GAAKnD,EAAa,UAE5CoD,yBAA0BtD,EAAEkC,QAE5BH,eAAgB/B,EAAEgC,QAElBG,iBAAkBA,GAIpB,IAAIoB,GAAQ,gGACmCC,MAAM,KAEjDC,EAAQF,EAAMG,OAAO,SAAU,aAC/BC,EAAWJ,EAAML,OAGjBU,EAAa,WAEf,GAGIC,GAHAC,EAASzD,EAAI,UACb8C,EAASQ,EACTI,EAAS,GAYb,KAVAD,EAAOE,MAAMC,QAAU,OACvB7D,EAAK8D,YAAYJ,GACjBA,EAAOK,IAAM,cAGbN,EAAiBC,EAAOM,cAAcC,SACtCR,EAAeS,OACfT,EAAeU,MAAM,oCAAsCR,GAC3DF,EAAeW,QACfZ,EAAaC,EAAeR,EACtBF,WAAWS,GAAWpC,UAAU+B,EAAMJ,GAC5C,OAAOS,MAELa,EAAgB,SAASC,EAAOxB,GAClC,MAAO,UAASyB,GACd,GAGIC,GAHApC,EAAS1B,EAAU6D,GACnBxB,EAAS,EACT0B,IAEJ,KAAID,IAAOpC,GAAKoC,GAAOzD,GAASb,EAAIkC,EAAGoC,IAAQC,EAAOC,KAAKF,EAE3D,MAAM1B,EAASC,GAAK7C,EAAIkC,EAAGoC,EAAMF,EAAMvB,SACpC9B,EAAawD,EAAQD,IAAQC,EAAOC,KAAKF,GAE5C,OAAOC,KAGPE,EAAQ,YACZ9E,GAAQA,EAAQmD,EAAG,UAEjB4B,eAAgBhF,EAAEiF,SAAWjF,EAAEiF,UAAY,SAASzC,GAElD,MADAA,GAAI3B,EAAS2B,GACVlC,EAAIkC,EAAGrB,GAAiBqB,EAAErB,GACF,kBAAjBqB,GAAE0C,aAA6B1C,YAAaA,GAAE0C,YAC/C1C,EAAE0C,YAAY1D,UACdgB,YAAajB,QAASD,EAAc,MAG/C6D,oBAAqBnF,EAAEoF,SAAWpF,EAAEoF,UAAYX,EAAchB,EAAOA,EAAMP,QAAQ,GAEnFmC,OAAQrF,EAAEqF,OAASrF,EAAEqF,QAAU,SAAS7C,EAAQO,GAC9C,GAAI8B,EAQJ,OAPS,QAANrC,GACDuC,EAAMvD,UAAYd,EAAS8B,GAC3BqC,EAAS,GAAIE,GACbA,EAAMvD,UAAY,KAElBqD,EAAO1D,GAAYqB,GACdqC,EAASjB,IACTb,IAAe7D,EAAY2F,EAAS1C,EAAiB0C,EAAQ9B,IAGtEC,KAAMhD,EAAEiD,QAAUjD,EAAEiD,SAAWwB,EAAclB,EAAOI,GAAU,IAGhE,IAAI2B,GAAY,SAASjC,EAAGkC,EAAKC,GAC/B,KAAKD,IAAOlD,IAAW,CACrB,IAAI,GAAIoD,MAAQtC,EAAI,EAAOoC,EAAJpC,EAASA,IAAIsC,EAAEtC,GAAK,KAAOA,EAAI,GACtDd,GAAUkD,GAAOG,SAAS,MAAO,gBAAkBD,EAAE3D,KAAK,KAAO,KAEnE,MAAOO,GAAUkD,GAAKlC,EAAGmC,GAI3BvF,GAAQA,EAAQwC,EAAG,YACjBkD,KAAM,QAASA,MAAKC,GAClB,GAAIC,GAAWlF,EAAUmF,MACrBC,EAAWpE,EAAWhC,KAAKqG,UAAW,GACtCC,EAAQ,WACV,GAAIT,GAAOO,EAASrC,OAAO/B,EAAWhC,KAAKqG,WAC3C,OAAOF,gBAAgBG,GAAQX,EAAUO,EAAIL,EAAKtC,OAAQsC,GAAQhF,EAAOqF,EAAIL,EAAMI,GAGrF,OADGhF,GAASiF,EAAGrE,aAAWyE,EAAMzE,UAAYqE,EAAGrE,WACxCyE,KAKXhG,EAAQA,EAAQwC,EAAIxC,EAAQoD,EAAI5C,EAAM,WACjCL,GAAKuB,EAAWhC,KAAKS,KACtB,SACFwB,MAAO,SAASsE,EAAOC,GACrB,GAAIZ,GAAQtE,EAAS6E,KAAK5C,QACtBkD,EAAQ7F,EAAIuF,KAEhB,IADAK,EAAMA,IAAQjH,EAAYqG,EAAMY,EACpB,SAATC,EAAiB,MAAOzE,GAAWhC,KAAKmG,KAAMI,EAAOC,EAMxD,KALA,GAAIE,GAASrF,EAAQkF,EAAOX,GACxBe,EAAStF,EAAQmF,EAAKZ,GACtBgB,EAAStF,EAASqF,EAAOD,GACzBG,EAAS9E,MAAM6E,GACfpD,EAAS,EACHoD,EAAJpD,EAAUA,IAAIqD,EAAOrD,GAAc,UAATiD,EAC5BN,KAAKW,OAAOJ,EAAQlD,GACpB2C,KAAKO,EAAQlD,EACjB,OAAOqD,MAGXvG,EAAQA,EAAQwC,EAAIxC,EAAQoD,GAAKnC,GAAWK,QAAS,SACnDO,KAAM,QAASA,MAAK4E,GAClB,MAAO7E,GAAUlC,KAAKuB,EAAQ4E,MAAOY,IAAcxH,EAAY,IAAMwH,MAKzEzG,EAAQA,EAAQmD,EAAG,SAAUuD,QAASvH,EAAoB,KAE1D,IAAIwH,GAAoB,SAASC,GAC/B,MAAO,UAASC,EAAYC,GAC1BpG,EAAUmG,EACV,IAAItE,GAAStB,EAAQ4E,MACjB5C,EAASjC,EAASuB,EAAEU,QACpB8D,EAASH,EAAU3D,EAAS,EAAI,EAChCC,EAAS0D,EAAU,GAAK,CAC5B,IAAGb,UAAU9C,OAAS,EAAE,OAAO,CAC7B,GAAG8D,IAASxE,GAAE,CACZuE,EAAOvE,EAAEwE,GACTA,GAAS7D,CACT,OAGF,GADA6D,GAAS7D,EACN0D,EAAkB,EAARG,EAAsBA,GAAV9D,EACvB,KAAMN,WAAU,+CAGpB,KAAKiE,EAAUG,GAAS,EAAI9D,EAAS8D,EAAOA,GAAS7D,EAAK6D,IAASxE,KACjEuE,EAAOD,EAAWC,EAAMvE,EAAEwE,GAAQA,EAAOlB,MAE3C,OAAOiB,KAIPE,EAAY,SAASC,GACvB,MAAO,UAASC,GACd,MAAOD,GAAIpB,KAAMqB,EAAMnB,UAAU,KAIrC/F,GAAQA,EAAQwC,EAAG,SAEjB2E,QAASpH,EAAEqH,KAAOrH,EAAEqH,MAAQJ,EAAU7F,EAAkB,IAExDkG,IAAKL,EAAU7F,EAAkB,IAEjCmG,OAAQN,EAAU7F,EAAkB,IAEpCoG,KAAMP,EAAU7F,EAAkB,IAElCqG,MAAOR,EAAU7F,EAAkB,IAEnCsG,OAAQd,GAAkB,GAE1Be,YAAaf,GAAkB,GAE/BgB,QAASX,EAAU5F,GAEnBwG,YAAa,SAASC,EAAIC,GACxB,GAAIvF,GAAS1B,EAAUgF,MACnB5C,EAASjC,EAASuB,EAAEU,QACpB8D,EAAS9D,EAAS,CAGtB,KAFG8C,UAAU9C,OAAS,IAAE8D,EAAQgB,KAAKC,IAAIjB,EAAOjG,EAAUgH,KAC/C,EAARf,IAAUA,EAAQ/F,EAASiC,EAAS8D,IAClCA,GAAS,EAAGA,IAAQ,GAAGA,IAASxE,IAAKA,EAAEwE,KAAWc,EAAG,MAAOd,EACjE,OAAO,MAKX/G,EAAQA,EAAQmD,EAAG,QAAS8E,IAAK,WAAY,OAAQ,GAAIC,QAEzD,IAAIC,GAAK,SAASC,GAChB,MAAOA,GAAM,EAAIA,EAAM,IAAMA,EAK/BpI,GAAQA,EAAQwC,EAAIxC,EAAQoD,GAAK5C,EAAM,WACrC,MAA4C,4BAArC,GAAI0H,MAAK,MAAQ,GAAGG,kBACtB7H,EAAM,WACX,GAAI0H,MAAKI,KAAKD,iBACX,QACHA,YAAa,QAASA,eACpB,IAAIE,SAAS1C,MAAM,KAAM2C,YAAW,qBACpC,IAAIC,GAAI5C,KACJ6C,EAAID,EAAEE,iBACNhJ,EAAI8I,EAAEG,qBACNC,EAAQ,EAAJH,EAAQ,IAAMA,EAAI,KAAO,IAAM,EACvC,OAAOG,IAAK,QAAUd,KAAKe,IAAIJ,IAAI/G,MAAMkH,EAAI,GAAK,IAChD,IAAMV,EAAGM,EAAEM,cAAgB,GAAK,IAAMZ,EAAGM,EAAEO,cAC3C,IAAMb,EAAGM,EAAEQ,eAAiB,IAAMd,EAAGM,EAAES,iBACvC,IAAMf,EAAGM,EAAEU,iBAAmB,KAAOxJ,EAAI,GAAKA,EAAI,IAAMwI,EAAGxI,IAAM,QAMlE,SAASJ,EAAQD,GAEtB,GAAI8J,GAAU9H,MACd/B,GAAOD,SACL8F,OAAYgE,EAAQhE,OACpBJ,SAAYoE,EAAQrE,eACpBsE,UAAexG,qBACfZ,QAAYmH,EAAQ/F,yBACpBtB,QAAYqH,EAAQtH,eACpBK,SAAYiH,EAAQlH,iBACpBc,QAAYoG,EAAQrG,KACpBoC,SAAYiE,EAAQlE,oBACpBoE,WAAYF,EAAQG,sBACpBnC,QAAeD,UAKZ,SAAS5H,EAAQD,EAASH,GAE/B,GAAIqK,GAAYrK,EAAoB,GAChCsK,EAAYtK,EAAoB,GAChCuK,EAAYvK,EAAoB,GAChCwK,EAAY,YAEZ3J,EAAU,SAAS4J,EAAMC,EAAMC,GACjC,GAQInF,GAAKoF,EAAKC,EARVC,EAAYL,EAAO5J,EAAQoD,EAC3B8G,EAAYN,EAAO5J,EAAQmK,EAC3BC,EAAYR,EAAO5J,EAAQmD,EAC3BkH,EAAYT,EAAO5J,EAAQwC,EAC3B8H,EAAYV,EAAO5J,EAAQuK,EAC3BC,EAAYZ,EAAO5J,EAAQyK,EAC3BnL,EAAY4K,EAAYT,EAAOA,EAAKI,KAAUJ,EAAKI,OACnDa,EAAYR,EAAYV,EAASY,EAAYZ,EAAOK,IAASL,EAAOK,QAAaF,EAElFO,KAAUJ,EAASD,EACtB,KAAIlF,IAAOmF,GAETC,GAAOE,GAAaS,GAAU/F,IAAO+F,GAClCX,GAAOpF,IAAOrF,KAEjB0K,EAAMD,EAAMW,EAAO/F,GAAOmF,EAAOnF,GAEjCrF,EAAQqF,GAAOuF,GAAmC,kBAAfQ,GAAO/F,GAAqBmF,EAAOnF,GAEpE2F,GAAWP,EAAML,EAAIM,EAAKR,GAE1BgB,GAAWE,EAAO/F,IAAQqF,EAAM,SAAUW,GAC1C,GAAIvH,GAAI,SAASwH,GACf,MAAO/E,gBAAgB8E,GAAI,GAAIA,GAAEC,GAASD,EAAEC,GAG9C,OADAxH,GAAEuG,GAAagB,EAAEhB,GACVvG,GAEN4G,GAAOK,GAA0B,kBAAPL,GAAoBN,EAAIjE,SAAS/F,KAAMsK,GAAOA,EACxEK,KAAU/K,EAAQqK,KAAerK,EAAQqK,QAAkBhF,GAAOqF,IAIzEhK,GAAQoD,EAAI,EACZpD,EAAQmK,EAAI,EACZnK,EAAQmD,EAAI,EACZnD,EAAQwC,EAAI,EACZxC,EAAQuK,EAAI,GACZvK,EAAQyK,EAAI,GACZlL,EAAOD,QAAUU,GAIZ,SAAST,EAAQD,GAGtB,GAAIkK,GAASjK,EAAOD,QAA2B,mBAAVuL,SAAyBA,OAAO9C,MAAQA,KACzE8C,OAAwB,mBAARC,OAAuBA,KAAK/C,MAAQA,KAAO+C,KAAOrF,SAAS,gBAC9D,iBAAPzG,KAAgBA,EAAMwK,IAI3B,SAASjK,EAAQD,GAEtB,GAAImK,GAAOlK,EAAOD,SAAWyL,QAAS,QACrB,iBAAPhM,KAAgBA,EAAM0K,IAI3B,SAASlK,EAAQD,EAASH,GAG/B,GAAIuB,GAAYvB,EAAoB,EACpCI,GAAOD,QAAU,SAASsG,EAAID,EAAM1C,GAElC,GADAvC,EAAUkF,GACPD,IAAS1G,EAAU,MAAO2G,EAC7B,QAAO3C,GACL,IAAK,GAAG,MAAO,UAASX,GACtB,MAAOsD,GAAGlG,KAAKiG,EAAMrD,GAEvB,KAAK,GAAG,MAAO,UAASA,EAAG0I,GACzB,MAAOpF,GAAGlG,KAAKiG,EAAMrD,EAAG0I,GAE1B,KAAK,GAAG,MAAO,UAAS1I,EAAG0I,EAAGpL,GAC5B,MAAOgG,GAAGlG,KAAKiG,EAAMrD,EAAG0I,EAAGpL,IAG/B,MAAO,YACL,MAAOgG,GAAGqF,MAAMtF,EAAMI,cAMrB,SAASxG,EAAQD,GAEtBC,EAAOD,QAAU,SAAS4L,GACxB,GAAgB,kBAANA,GAAiB,KAAMvI,WAAUuI,EAAK,sBAChD,OAAOA,KAKJ,SAAS3L,EAAQD,EAASH,GAG/BI,EAAOD,SAAWH,EAAoB,GAAG,WACvC,MAA2E,IAApEmC,OAAOQ,kBAAmB,KAAMO,IAAK,WAAY,MAAO,MAAOC,KAKnE,SAAS/C,EAAQD,GAEtBC,EAAOD,QAAU,SAAS6L,GACxB,IACE,QAASA,IACT,MAAMzI,GACN,OAAO,KAMN,SAASnD,EAAQD,GAEtBC,EAAOD,QAAU,SAAS8L,EAAQxI,GAChC,OACEyI,aAAyB,EAATD,GAChBE,eAAyB,EAATF,GAChBG,WAAyB,EAATH,GAChBxI,MAAcA,KAMb,SAASrD,EAAQD,EAASH,GAE/BI,EAAOD,QAAUH,EAAoB,GAAGiF,UAAYA,SAASoH,iBAIxD,SAASjM,EAAQD,EAASH,GAE/B,GAAIwB,GAAWxB,EAAoB,IAC/BiF,EAAWjF,EAAoB,GAAGiF,SAElCqH,EAAK9K,EAASyD,IAAazD,EAASyD,EAASsH,cACjDnM,GAAOD,QAAU,SAAS4L,GACxB,MAAOO,GAAKrH,EAASsH,cAAcR,QAKhC,SAAS3L,EAAQD,GAEtBC,EAAOD,QAAU,SAAS4L,GACxB,MAAqB,gBAAPA,GAAyB,OAAPA,EAA4B,kBAAPA,KAKlD,SAAS3L,EAAQD,GAEtB,GAAIqM,MAAoBA,cACxBpM,GAAOD,QAAU,SAAS4L,EAAIvG,GAC5B,MAAOgH,GAAejM,KAAKwL,EAAIvG,KAK5B,SAASpF,EAAQD,GAEtB,GAAIsM,MAAcA,QAElBrM,GAAOD,QAAU,SAAS4L,GACxB,MAAOU,GAASlM,KAAKwL,GAAIvJ,MAAM,EAAG,MAK/B,SAASpC,EAAQD,GAGtBC,EAAOD,QAAU,SAASsG,EAAIL,EAAMI,GAClC,GAAIkG,GAAKlG,IAAS1G,CAClB,QAAOsG,EAAKtC,QACV,IAAK,GAAG,MAAO4I,GAAKjG,IACAA,EAAGlG,KAAKiG,EAC5B,KAAK,GAAG,MAAOkG,GAAKjG,EAAGL,EAAK,IACRK,EAAGlG,KAAKiG,EAAMJ,EAAK,GACvC,KAAK,GAAG,MAAOsG,GAAKjG,EAAGL,EAAK,GAAIA,EAAK,IACjBK,EAAGlG,KAAKiG,EAAMJ,EAAK,GAAIA,EAAK,GAChD,KAAK,GAAG,MAAOsG,GAAKjG,EAAGL,EAAK,GAAIA,EAAK,GAAIA,EAAK,IAC1BK,EAAGlG,KAAKiG,EAAMJ,EAAK,GAAIA,EAAK,GAAIA,EAAK,GACzD,KAAK,GAAG,MAAOsG,GAAKjG,EAAGL,EAAK,GAAIA,EAAK,GAAIA,EAAK,GAAIA,EAAK,IACnCK,EAAGlG,KAAKiG,EAAMJ,EAAK,GAAIA,EAAK,GAAIA,EAAK,GAAIA,EAAK,IAClE,MAAoBK,GAAGqF,MAAMtF,EAAMJ,KAKlC,SAAShG,EAAQD,EAASH,GAE/B,GAAIwB,GAAWxB,EAAoB,GACnCI,GAAOD,QAAU,SAAS4L,GACxB,IAAIvK,EAASuK,GAAI,KAAMvI,WAAUuI,EAAK,qBACtC,OAAOA,KAKJ,SAAS3L,EAAQD,EAASH,GAG/B,GAAI2M,GAAU3M,EAAoB,GAClCI,GAAOD,QAAU,SAAS4L,GACxB,MAAO5J,QAAOwK,EAAQZ,MAKnB,SAAS3L,EAAQD,GAGtBC,EAAOD,QAAU,SAAS4L,GACxB,GAAGA,GAAMjM,EAAU,KAAM0D,WAAU,yBAA2BuI,EAC9D,OAAOA,KAKJ,SAAS3L,EAAQD,EAASH,GAG/B,GAAI8B,GAAU9B,EAAoB,IAC9B2M,EAAU3M,EAAoB,GAClCI,GAAOD,QAAU,SAAS4L,GACxB,MAAOjK,GAAQ6K,EAAQZ,MAKpB,SAAS3L,EAAQD,EAASH,GAG/B,GAAImB,GAAMnB,EAAoB,GAC9BI,GAAOD,QAAUgC,OAAO,KAAKuB,qBAAqB,GAAKvB,OAAS,SAAS4J,GACvE,MAAkB,UAAX5K,EAAI4K,GAAkBA,EAAG3H,MAAM,IAAMjC,OAAO4J,KAKhD,SAAS3L,EAAQD,GAGtB,GAAIyM,GAAQhE,KAAKgE,KACbC,EAAQjE,KAAKiE,KACjBzM,GAAOD,QAAU,SAAS4L,GACxB,MAAOe,OAAMf,GAAMA,GAAM,GAAKA,EAAK,EAAIc,EAAQD,GAAMb,KAKlD,SAAS3L,EAAQD,EAASH,GAE/B,GAAI2B,GAAY3B,EAAoB,IAChC+M,EAAYnE,KAAKmE,IACjBlE,EAAYD,KAAKC,GACrBzI,GAAOD,QAAU,SAASyH,EAAO9D,GAE/B,MADA8D,GAAQjG,EAAUiG,GACH,EAARA,EAAYmF,EAAInF,EAAQ9D,EAAQ,GAAK+E,EAAIjB,EAAO9D,KAKpD,SAAS1D,EAAQD,EAASH,GAG/B,GAAI2B,GAAY3B,EAAoB,IAChC6I,EAAYD,KAAKC,GACrBzI,GAAOD,QAAU,SAAS4L,GACxB,MAAOA,GAAK,EAAIlD,EAAIlH,EAAUoK,GAAK,kBAAoB,IAKpD,SAAS3L,EAAQD,GAEtB,GAAIE,GAAK,EACL2M,EAAKpE,KAAKqE,QACd7M,GAAOD,QAAU,SAASqF,GACxB,MAAO,UAAUlB,OAAOkB,IAAQ1F,EAAY,GAAK0F,EAAK,QAASnF,EAAK2M,GAAIP,SAAS,OAK9E,SAASrM,EAAQD,EAASH,GAS/B,GAAIuK,GAAWvK,EAAoB,GAC/B8B,EAAW9B,EAAoB,IAC/ByB,EAAWzB,EAAoB,IAC/B6B,EAAW7B,EAAoB,IAC/BkN,EAAWlN,EAAoB,GACnCI,GAAOD,QAAU,SAASgN,GACxB,GAAIC,GAAwB,GAARD,EAChBE,EAAwB,GAARF,EAChBG,EAAwB,GAARH,EAChBI,EAAwB,GAARJ,EAChBK,EAAwB,GAARL,EAChBM,EAAwB,GAARN,GAAaK,CACjC,OAAO,UAASE,EAAOhG,EAAYlB,GAQjC,IAPA,GAMImH,GAAKC,EANLxK,EAAS3B,EAASiM,GAClB/B,EAAS7J,EAAQsB,GACjByK,EAAStD,EAAI7C,EAAYlB,EAAM,GAC/B1C,EAASjC,EAAS8J,EAAK7H,QACvB8D,EAAS,EACTnC,EAAS2H,EAASF,EAAIQ,EAAO5J,GAAUuJ,EAAYH,EAAIQ,EAAO,GAAK5N,EAElEgE,EAAS8D,EAAOA,IAAQ,IAAG6F,GAAY7F,IAAS+D,MACnDgC,EAAMhC,EAAK/D,GACXgG,EAAMC,EAAEF,EAAK/F,EAAOxE,GACjB+J,GACD,GAAGC,EAAO3H,EAAOmC,GAASgG,MACrB,IAAGA,EAAI,OAAOT,GACjB,IAAK,GAAG,OAAO,CACf,KAAK,GAAG,MAAOQ,EACf,KAAK,GAAG,MAAO/F,EACf,KAAK,GAAGnC,EAAOC,KAAKiI,OACf,IAAGJ,EAAS,OAAO,CAG9B,OAAOC,GAAgB,GAAKF,GAAWC,EAAWA,EAAW9H,KAM5D,SAASrF,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,IAC/BuH,EAAWvH,EAAoB,IAC/B8N,EAAW9N,EAAoB,IAAI,UACvCI,GAAOD,QAAU,SAAS4N,EAAUjK,GAClC,GAAI0H,EASF,OARCjE,GAAQwG,KACTvC,EAAIuC,EAASjI,YAEE,kBAAL0F,IAAoBA,IAAMlJ,QAASiF,EAAQiE,EAAEpJ,aAAYoJ,EAAI1L,GACpE0B,EAASgK,KACVA,EAAIA,EAAEsC,GACG,OAANtC,IAAWA,EAAI1L,KAEb,IAAK0L,IAAM1L,EAAYwC,MAAQkJ,GAAG1H,KAKxC,SAAS1D,EAAQD,EAASH,GAG/B,GAAImB,GAAMnB,EAAoB,GAC9BI,GAAOD,QAAUmC,MAAMiF,SAAW,SAASyG,GACzC,MAAmB,SAAZ7M,EAAI6M,KAKR,SAAS5N,EAAQD,EAASH,GAE/B,GAAIiO,GAASjO,EAAoB,IAAI,OACjCkO,EAASlO,EAAoB,IAC7BmO,EAASnO,EAAoB,GAAGmO,MACpC/N,GAAOD,QAAU,SAASuK,GACxB,MAAOuD,GAAMvD,KAAUuD,EAAMvD,GAC3ByD,GAAUA,EAAOzD,KAAUyD,GAAUD,GAAK,UAAYxD,MAKrD,SAAStK,EAAQD,EAASH,GAE/B,GAAIqK,GAASrK,EAAoB,GAC7BoO,EAAS,qBACTH,EAAS5D,EAAO+D,KAAY/D,EAAO+D,MACvChO,GAAOD,QAAU,SAASqF,GACxB,MAAOyI,GAAMzI,KAASyI,EAAMzI,SAKzB,SAASpF,EAAQD,EAASH,GAI/B,GAAI0B,GAAY1B,EAAoB,IAChC6B,EAAY7B,EAAoB,IAChC4B,EAAY5B,EAAoB,GACpCI,GAAOD,QAAU,SAASkO,GACxB,MAAO,UAASX,EAAOhF,EAAIC,GACzB,GAGIlF,GAHAL,EAAS1B,EAAUgM,GACnB5J,EAASjC,EAASuB,EAAEU,QACpB8D,EAAShG,EAAQ+G,EAAW7E,EAGhC,IAAGuK,GAAe3F,GAAMA,GAAG,KAAM5E,EAAS8D,GAExC,GADAnE,EAAQL,EAAEwE,KACPnE,GAASA,EAAM,OAAO,MAEpB,MAAKK,EAAS8D,EAAOA,IAAQ,IAAGyG,GAAezG,IAASxE,KAC1DA,EAAEwE,KAAWc,EAAG,MAAO2F,IAAezG,CACzC,QAAQyG,GAAe,MAMxB,SAASjO,EAAQD,EAASH,GAI/B,GAAIY,GAAiBZ,EAAoB,GACrCqK,EAAiBrK,EAAoB,GACrCkB,EAAiBlB,EAAoB,IACrCc,EAAiBd,EAAoB,GACrCa,EAAiBb,EAAoB,GACrCsO,EAAiBtO,EAAoB,IACrCuO,EAAiBvO,EAAoB,GACrCwO,EAAiBxO,EAAoB,IACrCyO,EAAiBzO,EAAoB,IACrCkO,EAAiBlO,EAAoB,IACrC0O,EAAiB1O,EAAoB,IACrC2O,EAAiB3O,EAAoB,IACrC4O,EAAiB5O,EAAoB,IACrC6O,EAAiB7O,EAAoB,IACrCuH,EAAiBvH,EAAoB,IACrCsB,EAAiBtB,EAAoB,IACrC0B,EAAiB1B,EAAoB,IACrCe,EAAiBf,EAAoB,IACrC8C,EAAiBlC,EAAEkC,QACnBF,EAAiBhC,EAAEgC,QACnBkM,EAAiBlO,EAAEqF,OACnBD,EAAiB4I,EAAO1L,IACxB6L,EAAiB1E,EAAO8D,OACxBa,EAAiB3E,EAAO4E,KACxBC,EAAiBF,GAASA,EAAMG,UAChCC,GAAiB,EACjBC,EAAiBX,EAAI,WACrBxE,EAAiBtJ,EAAEsJ,OACnBoF,EAAiBd,EAAO,mBACxBe,EAAiBf,EAAO,WACxBgB,EAAmC,kBAAXT,GACxB7M,EAAiBC,OAAOC,UAGxBqN,EAAgB3O,GAAeyN,EAAO,WACxC,MAES,IAFFO,EAAQlM,KAAY,KACzBM,IAAK,WAAY,MAAON,GAAQ8D,KAAM,KAAMjD,MAAO,IAAIN,MACrDA,IACD,SAAS4I,EAAIvG,EAAKkK,GACrB,GAAIC,GAAY7M,EAAQZ,EAAasD,EAClCmK,UAAiBzN,GAAYsD,GAChC5C,EAAQmJ,EAAIvG,EAAKkK,GACdC,GAAa5D,IAAO7J,GAAYU,EAAQV,EAAasD,EAAKmK,IAC3D/M,EAEAgN,EAAO,SAASC,GAClB,GAAIC,GAAMP,EAAWM,GAAOf,EAAQC,EAAQ3M,UAS5C,OARA0N,GAAIC,GAAKF,EACT/O,GAAesO,GAAUK,EAAcvN,EAAa2N,GAClD1D,cAAc,EACd6D,IAAK,SAASvM,GACTvC,EAAIwF,KAAM2I,IAAWnO,EAAIwF,KAAK2I,GAASQ,KAAKnJ,KAAK2I,GAAQQ,IAAO,GACnEJ,EAAc/I,KAAMmJ,EAAK9O,EAAW,EAAG0C,OAGpCqM,GAGLG,EAAW,SAASlE,GACtB,MAAoB,gBAANA,IAGZmE,EAAkB,QAASvN,gBAAeoJ,EAAIvG,EAAKkK,GACrD,MAAGA,IAAKxO,EAAIqO,EAAY/J,IAClBkK,EAAExD,YAIDhL,EAAI6K,EAAIsD,IAAWtD,EAAGsD,GAAQ7J,KAAKuG,EAAGsD,GAAQ7J,IAAO,GACxDkK,EAAIZ,EAAQY,GAAIxD,WAAYnL,EAAW,GAAG,OAJtCG,EAAI6K,EAAIsD,IAAQzM,EAAQmJ,EAAIsD,EAAQtO,EAAW,OACnDgL,EAAGsD,GAAQ7J,IAAO,GAIXiK,EAAc1D,EAAIvG,EAAKkK,IACzB9M,EAAQmJ,EAAIvG,EAAKkK,IAExBS,EAAoB,QAASpN,kBAAiBgJ,EAAI1I,GACpD/B,EAASyK,EAKT,KAJA,GAGIvG,GAHA5B,EAAOiL,EAASxL,EAAI3B,EAAU2B,IAC9BU,EAAO,EACPqM,EAAIxM,EAAKE,OAEPsM,EAAIrM,GAAEmM,EAAgBnE,EAAIvG,EAAM5B,EAAKG,KAAMV,EAAEmC,GACnD,OAAOuG,IAELsE,EAAU,QAASpK,QAAO8F,EAAI1I,GAChC,MAAOA,KAAMvD,EAAYgP,EAAQ/C,GAAMoE,EAAkBrB,EAAQ/C,GAAK1I,IAEpEiN,EAAwB,QAAS5M,sBAAqB8B,GACxD,GAAI+K,GAAIrG,EAAO3J,KAAKmG,KAAMlB,EAC1B,OAAO+K,KAAMrP,EAAIwF,KAAMlB,KAAStE,EAAIqO,EAAY/J,IAAQtE,EAAIwF,KAAM2I,IAAW3I,KAAK2I,GAAQ7J,GACtF+K,GAAI,GAENC,EAA4B,QAAStM,0BAAyB6H,EAAIvG,GACpE,GAAIkK,GAAI5M,EAAQiJ,EAAKrK,EAAUqK,GAAKvG,EAEpC,QADGkK,IAAKxO,EAAIqO,EAAY/J,IAAUtE,EAAI6K,EAAIsD,IAAWtD,EAAGsD,GAAQ7J,KAAMkK,EAAExD,YAAa,GAC9EwD,GAELe,EAAuB,QAAS1K,qBAAoBgG,GAKtD,IAJA,GAGIvG,GAHAF,EAASU,EAAStE,EAAUqK,IAC5BtG,KACA1B,EAAS,EAEPuB,EAAMxB,OAASC,GAAM7C,EAAIqO,EAAY/J,EAAMF,EAAMvB,OAASyB,GAAO6J,GAAO5J,EAAOC,KAAKF,EAC1F,OAAOC,IAELiL,EAAyB,QAAStG,uBAAsB2B,GAK1D,IAJA,GAGIvG,GAHAF,EAASU,EAAStE,EAAUqK,IAC5BtG,KACA1B,EAAS,EAEPuB,EAAMxB,OAASC,GAAK7C,EAAIqO,EAAY/J,EAAMF,EAAMvB,OAAM0B,EAAOC,KAAK6J,EAAW/J,GACnF,OAAOC,IAELkL,EAAa,QAASxB,WAAUpD,GAClC,GAAGA,IAAOjM,IAAamQ,EAASlE,GAAhC,CAKA,IAJA,GAGI6E,GAAUC,EAHVzK,GAAQ2F,GACRhI,EAAO,EACP+M,EAAOlK,UAELkK,EAAGhN,OAASC,GAAEqC,EAAKV,KAAKoL,EAAG/M,KAQjC,OAPA6M,GAAWxK,EAAK,GACM,kBAAZwK,KAAuBC,EAAYD,IAC1CC,IAActJ,EAAQqJ,MAAUA,EAAW,SAASpL,EAAK/B,GAE1D,MADGoN,KAAUpN,EAAQoN,EAAUtQ,KAAKmG,KAAMlB,EAAK/B,IAC3CwM,EAASxM,GAAb,OAA2BA,IAE7B2C,EAAK,GAAKwK,EACH1B,EAAWpD,MAAMkD,EAAO5I,KAE7B2K,EAAYxC,EAAO,WACrB,GAAIvK,GAAI+K,GAIR,OAA0B,UAAnBG,GAAYlL,KAAyC,MAAtBkL,GAAY/L,EAAGa,KAAwC,MAAzBkL,EAAW/M,OAAO6B,KAIpFwL,KACFT,EAAU,QAASZ,UACjB,GAAG8B,EAASvJ,MAAM,KAAMlD,WAAU,8BAClC,OAAOoM,GAAK1B,EAAItH,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,KAExDwO,EAASS,EAAQ3M,UAAW,WAAY,QAASqK,YAC/C,MAAO/F,MAAKqJ,KAGdE,EAAW,SAASlE,GAClB,MAAOA,aAAcgD,IAGvBnO,EAAEqF,OAAaoK,EACfzP,EAAEsJ,OAAaoG,EACf1P,EAAEkC,QAAa0N,EACf5P,EAAEgC,QAAasN,EACftP,EAAEoC,SAAamN,EACfvP,EAAEoF,SAAa4I,EAAO1L,IAAMuN,EAC5B7P,EAAEuJ,WAAauG,EAEZ5P,IAAgBd,EAAoB,KACrCsO,EAASpM,EAAa,uBAAwBoO,GAAuB,GAIzE,IAAIU,IAEFC,MAAO,SAASzL,GACd,MAAOtE,GAAIoO,EAAgB9J,GAAO,IAC9B8J,EAAe9J,GACf8J,EAAe9J,GAAOuJ,EAAQvJ,IAGpC0L,OAAQ,QAASA,QAAO1L,GACtB,MAAOmJ,GAAMW,EAAgB9J,IAE/B2L,UAAW,WAAY/B,GAAS,GAChCgC,UAAW,WAAYhC,GAAS,GAalCxO,GAAEqH,KAAK1H,KAAK,iHAGV6D,MAAM,KAAM,SAAS2H,GACrB,GAAI+D,GAAMpB,EAAI3C,EACdiF,GAAcjF,GAAMyD,EAAYM,EAAMF,EAAKE,KAG7CV,GAAS,EAETvO,EAAQA,EAAQmK,EAAInK,EAAQyK,GAAI6C,OAAQY,IAExClO,EAAQA,EAAQmD,EAAG,SAAUgN,GAE7BnQ,EAAQA,EAAQmD,EAAInD,EAAQoD,GAAKuL,EAAW,UAE1CvJ,OAAQoK,EAER1N,eAAgBuN,EAEhBnN,iBAAkBoN,EAElBjM,yBAA0BsM,EAE1BzK,oBAAqB0K,EAErBrG,sBAAuBsG,IAIzB1B,GAASnO,EAAQA,EAAQmD,EAAInD,EAAQoD,IAAMuL,GAAauB,GAAY,QAAS5B,UAAWwB,IAGxFlC,EAAeM,EAAS,UAExBN,EAAe7F,KAAM,QAAQ,GAE7B6F,EAAepE,EAAO4E,KAAM,QAAQ,IAI/B,SAAS7O,EAAQD,EAASH,GAE/BI,EAAOD,QAAUH,EAAoB,KAIhC,SAASI,EAAQD,EAASH,GAE/B,GAAIY,GAAaZ,EAAoB,GACjCe,EAAaf,EAAoB,GACrCI,GAAOD,QAAUH,EAAoB,GAAK,SAASuF,EAAQC,EAAK/B,GAC9D,MAAO7C,GAAEgC,QAAQ2C,EAAQC,EAAKzE,EAAW,EAAG0C,KAC1C,SAAS8B,EAAQC,EAAK/B,GAExB,MADA8B,GAAOC,GAAO/B,EACP8B,IAKJ,SAASnF,EAAQD,EAASH,GAE/B,GAAIqR,GAAMrR,EAAoB,GAAG4C,QAC7B1B,EAAMlB,EAAoB,IAC1BsR,EAAMtR,EAAoB,IAAI,cAElCI,GAAOD,QAAU,SAAS4L,EAAI8D,EAAK0B,GAC9BxF,IAAO7K,EAAI6K,EAAKwF,EAAOxF,EAAKA,EAAG3J,UAAWkP,IAAKD,EAAItF,EAAIuF,GAAMnF,cAAc,EAAM1I,MAAOoM,MAKxF,SAASzP,EAAQD,EAASH,GAE/B,GAAIY,GAAYZ,EAAoB,GAChC0B,EAAY1B,EAAoB,GACpCI,GAAOD,QAAU,SAASoF,EAAQmD,GAMhC,IALA,GAIIlD,GAJApC,EAAS1B,EAAU6D,GACnB3B,EAAShD,EAAEiD,QAAQT,GACnBU,EAASF,EAAKE,OACd8D,EAAS,EAEP9D,EAAS8D,GAAM,GAAGxE,EAAEoC,EAAM5B,EAAKgE,QAAcc,EAAG,MAAOlD,KAK1D,SAASpF,EAAQD,EAASH,GAG/B,GAAI0B,GAAY1B,EAAoB,IAChCgG,EAAYhG,EAAoB,GAAGgG,SACnCyG,KAAeA,SAEf+E,EAA+B,gBAAV9F,SAAsBvJ,OAAO4D,oBAClD5D,OAAO4D,oBAAoB2F,WAE3B+F,EAAiB,SAAS1F,GAC5B,IACE,MAAO/F,GAAS+F,GAChB,MAAMxI,GACN,MAAOiO,GAAYhP,SAIvBpC,GAAOD,QAAQ+C,IAAM,QAAS6C,qBAAoBgG,GAChD,MAAGyF,IAAoC,mBAArB/E,EAASlM,KAAKwL,GAAgC0F,EAAe1F,GACxE/F,EAAStE,EAAUqK,MAKvB,SAAS3L,EAAQD,EAASH,GAG/B,GAAIY,GAAIZ,EAAoB,EAC5BI,GAAOD,QAAU,SAAS4L,GACxB,GAAInI,GAAahD,EAAEiD,QAAQkI,GACvB5B,EAAavJ,EAAEuJ,UACnB,IAAGA,EAKD,IAJA,GAGI3E,GAHAkM,EAAUvH,EAAW4B,GACrB7B,EAAUtJ,EAAEsJ,OACZnG,EAAU,EAER2N,EAAQ5N,OAASC,GAAKmG,EAAO3J,KAAKwL,EAAIvG,EAAMkM,EAAQ3N,OAAMH,EAAK8B,KAAKF,EAE5E,OAAO5B,KAKJ,SAASxD,EAAQD,GAEtBC,EAAOD,SAAU,GAIZ,SAASC,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAG,UAAW0N,OAAQ3R,EAAoB,OAIjE,SAASI,EAAQD,EAASH,GAG/B,GAAIY,GAAWZ,EAAoB,GAC/ByB,EAAWzB,EAAoB,IAC/B8B,EAAW9B,EAAoB,GAGnCI,GAAOD,QAAUH,EAAoB,GAAG,WACtC,GAAImD,GAAIhB,OAAOwP,OACXC,KACAxG,KACApH,EAAImK,SACJ0D,EAAI,sBAGR,OAFAD,GAAE5N,GAAK,EACP6N,EAAEzN,MAAM,IAAI4D,QAAQ,SAAS8J,GAAI1G,EAAE0G,GAAKA,IAClB,GAAf3O,KAAMyO,GAAG5N,IAAW7B,OAAOyB,KAAKT,KAAMiI,IAAI1I,KAAK,KAAOmP,IAC1D,QAASF,QAAOpG,EAAQZ,GAQ3B,IAPA,GAAIoH,GAAQtQ,EAAS8J,GACjBuF,EAAQlK,UACRoL,EAAQlB,EAAGhN,OACX8D,EAAQ,EACR/D,EAAajD,EAAEiD,QACfsG,EAAavJ,EAAEuJ,WACfD,EAAatJ,EAAEsJ,OACb8H,EAAQpK,GAMZ,IALA,GAIIpC,GAJAxB,EAASlC,EAAQgP,EAAGlJ,MACpBhE,EAASuG,EAAatG,EAAQG,GAAGM,OAAO6F,EAAWnG,IAAMH,EAAQG,GACjEF,EAASF,EAAKE,OACdmO,EAAS,EAEPnO,EAASmO,GAAK/H,EAAO3J,KAAKyD,EAAGwB,EAAM5B,EAAKqO,QAAMF,EAAEvM,GAAOxB,EAAEwB,GAEjE,OAAOuM,IACL5P,OAAOwP,QAIN,SAASvR,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAClCa,GAAQA,EAAQmD,EAAG,UAAWsI,GAAItM,EAAoB,OAIjD,SAASI,EAAQD,GAGtBC,EAAOD,QAAUgC,OAAOmK,IAAM,QAASA,IAAG4F,EAAG3I,GAC3C,MAAO2I,KAAM3I,EAAU,IAAN2I,GAAW,EAAIA,IAAM,EAAI3I,EAAI2I,GAAKA,GAAK3I,GAAKA,IAK1D,SAASnJ,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAClCa,GAAQA,EAAQmD,EAAG,UAAWmO,eAAgBnS,EAAoB,IAAIgQ,OAIjE,SAAS5P,EAAQD,EAASH,GAI/B,GAAI8C,GAAW9C,EAAoB,GAAG8C,QAClCtB,EAAWxB,EAAoB,IAC/BsB,EAAWtB,EAAoB,IAC/BoS,EAAQ,SAAShP,EAAGiP,GAEtB,GADA/Q,EAAS8B,IACL5B,EAAS6Q,IAAoB,OAAVA,EAAe,KAAM7O,WAAU6O,EAAQ,6BAEhEjS,GAAOD,SACL6P,IAAK7N,OAAOgQ,iBAAmB,gBAC7B,SAASG,EAAMC,EAAOvC,GACpB,IACEA,EAAMhQ,EAAoB,GAAGsG,SAAS/F,KAAMuC,EAAQX,OAAOC,UAAW,aAAa4N,IAAK,GACxFA,EAAIsC,MACJC,IAAUD,YAAgBhQ,QAC1B,MAAMiB,GAAIgP,GAAQ,EACpB,MAAO,SAASJ,gBAAe/O,EAAGiP,GAIhC,MAHAD,GAAMhP,EAAGiP,GACNE,EAAMnP,EAAEoP,UAAYH,EAClBrC,EAAI5M,EAAGiP,GACLjP,QAEL,GAAStD,GACjBsS,MAAOA,IAKJ,SAAShS,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,GAEnCA,GAAoB,IAAI,SAAU,SAASyS,GACzC,MAAO,SAASC,QAAO3G,GACrB,MAAO0G,IAAWjR,EAASuK,GAAM0G,EAAQ1G,GAAMA,MAM9C,SAAS3L,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9BsK,EAAUtK,EAAoB,GAC9BqB,EAAUrB,EAAoB,EAClCI,GAAOD,QAAU,SAASwS,EAAK3G,GAC7B,GAAIvF,IAAO6D,EAAKnI,YAAcwQ,IAAQxQ,OAAOwQ,GACzCC,IACJA,GAAID,GAAO3G,EAAKvF,GAChB5F,EAAQA,EAAQmD,EAAInD,EAAQoD,EAAI5C,EAAM,WAAYoF,EAAG,KAAQ,SAAUmM,KAKpE,SAASxS,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,GAEnCA,GAAoB,IAAI,OAAQ,SAAS6S,GACvC,MAAO,SAASC,MAAK/G,GACnB,MAAO8G,IAASrR,EAASuK,GAAM8G,EAAM9G,GAAMA,MAM1C,SAAS3L,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,GAEnCA,GAAoB,IAAI,oBAAqB,SAAS+S,GACpD,MAAO,SAASC,mBAAkBjH,GAChC,MAAOgH,IAAsBvR,EAASuK,GAAMgH,EAAmBhH,GAAMA,MAMpE,SAAS3L,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,GAEnCA,GAAoB,IAAI,WAAY,SAASiT,GAC3C,MAAO,SAASC,UAASnH,GACvB,MAAOvK,GAASuK,GAAMkH,EAAYA,EAAUlH,IAAM,GAAQ,MAMzD,SAAS3L,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,GAEnCA,GAAoB,IAAI,WAAY,SAASmT,GAC3C,MAAO,SAASC,UAASrH,GACvB,MAAOvK,GAASuK,GAAMoH,EAAYA,EAAUpH,IAAM,GAAQ,MAMzD,SAAS3L,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,GAEnCA,GAAoB,IAAI,eAAgB,SAASqT,GAC/C,MAAO,SAASC,cAAavH,GAC3B,MAAOvK,GAASuK,GAAMsH,EAAgBA,EAActH,IAAM,GAAO,MAMhE,SAAS3L,EAAQD,EAASH,GAG/B,GAAI0B,GAAY1B,EAAoB,GAEpCA,GAAoB,IAAI,2BAA4B,SAASwQ,GAC3D,MAAO,SAAStM,0BAAyB6H,EAAIvG,GAC3C,MAAOgL,GAA0B9O,EAAUqK,GAAKvG,OAM/C,SAASpF,EAAQD,EAASH,GAG/B,GAAIyB,GAAWzB,EAAoB,GAEnCA,GAAoB,IAAI,iBAAkB,SAASuT,GACjD,MAAO,SAAS3N,gBAAemG,GAC7B,MAAOwH,GAAgB9R,EAASsK,QAM/B,SAAS3L,EAAQD,EAASH,GAG/B,GAAIyB,GAAWzB,EAAoB,GAEnCA,GAAoB,IAAI,OAAQ,SAASwT,GACvC,MAAO,SAAS5P,MAAKmI,GACnB,MAAOyH,GAAM/R,EAASsK,QAMrB,SAAS3L,EAAQD,EAASH,GAG/BA,EAAoB,IAAI,sBAAuB,WAC7C,MAAOA,GAAoB,IAAIkD,OAK5B,SAAS9C,EAAQD,EAASH,GAG/B,GAAIY,GAAgBZ,EAAoB,GACpCwB,EAAgBxB,EAAoB,IACpCyT,EAAgBzT,EAAoB,IAAI,eACxC0T,EAAgBpN,SAASlE,SAExBqR,KAAgBC,IAAe9S,EAAEgC,QAAQ8Q,EAAeD,GAAehQ,MAAO,SAASL,GAC1F,GAAkB,kBAARsD,QAAuBlF,EAAS4B,GAAG,OAAO,CACpD,KAAI5B,EAASkF,KAAKtE,WAAW,MAAOgB,aAAasD,KAEjD,MAAMtD,EAAIxC,EAAEiF,SAASzC,IAAG,GAAGsD,KAAKtE,YAAcgB,EAAE,OAAO,CACvD,QAAO,MAKJ,SAAShD,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UAAW2P,QAAS/K,KAAKgL,IAAI,EAAG,QAI9C,SAASxT,EAAQD,EAASH,GAG/B,GAAIa,GAAYb,EAAoB,GAChC6T,EAAY7T,EAAoB,GAAGoJ,QAEvCvI,GAAQA,EAAQmD,EAAG,UACjBoF,SAAU,QAASA,UAAS2C,GAC1B,MAAoB,gBAANA,IAAkB8H,EAAU9H,OAMzC,SAAS3L,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UAAW8P,UAAW9T,EAAoB,OAIxD,SAASI,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,IAC/B6M,EAAWjE,KAAKiE,KACpBzM,GAAOD,QAAU,QAAS2T,WAAU/H,GAClC,OAAQvK,EAASuK,IAAO3C,SAAS2C,IAAOc,EAAMd,KAAQA,IAKnD,SAAS3L,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UACjB8I,MAAO,QAASA,OAAMiH,GACpB,MAAOA,IAAUA,MAMhB,SAAS3T,EAAQD,EAASH,GAG/B,GAAIa,GAAYb,EAAoB,GAChC8T,EAAY9T,EAAoB,IAChC2J,EAAYf,KAAKe,GAErB9I,GAAQA,EAAQmD,EAAG,UACjBgQ,cAAe,QAASA,eAAcD,GACpC,MAAOD,GAAUC,IAAWpK,EAAIoK,IAAW,qBAM1C,SAAS3T,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UAAWiQ,iBAAkB,oBAI3C,SAAS7T,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UAAWkQ,iBAAkB,qBAI3C,SAAS9T,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UAAWmQ,WAAYA,cAIrC,SAAS/T,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UAAWoQ,SAAUA,YAInC,SAAShU,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9BqU,EAAUrU,EAAoB,IAC9BsU,EAAU1L,KAAK0L,KACfC,EAAU3L,KAAK4L,KAGnB3T,GAAQA,EAAQmD,EAAInD,EAAQoD,IAAMsQ,GAAkD,KAAxC3L,KAAKiE,MAAM0H,EAAOE,OAAOC,aAAqB,QACxFF,MAAO,QAASA,OAAMtC,GACpB,OAAQA,GAAKA,GAAK,EAAI/I,IAAM+I,EAAI,kBAC5BtJ,KAAK+L,IAAIzC,GAAKtJ,KAAKgM,IACnBP,EAAMnC,EAAI,EAAIoC,EAAKpC,EAAI,GAAKoC,EAAKpC,EAAI,QAMxC,SAAS9R,EAAQD,GAGtBC,EAAOD,QAAUyI,KAAKyL,OAAS,QAASA,OAAMnC,GAC5C,OAAQA,GAAKA,GAAK,OAAa,KAAJA,EAAWA,EAAIA,EAAIA,EAAI,EAAItJ,KAAK+L,IAAI,EAAIzC,KAKhE,SAAS9R,EAAQD,EAASH,GAK/B,QAAS6U,OAAM3C,GACb,MAAQ9I,UAAS8I,GAAKA,IAAW,GAALA,EAAiB,EAAJA,GAAS2C,OAAO3C,GAAKtJ,KAAK+L,IAAIzC,EAAItJ,KAAK0L,KAAKpC,EAAIA,EAAI,IAAxDA,EAHvC,GAAIrR,GAAUb,EAAoB,EAMlCa,GAAQA,EAAQmD,EAAG,QAAS6Q,MAAOA,SAI9B,SAASzU,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QACjB8Q,MAAO,QAASA,OAAM5C,GACpB,MAAmB,KAAXA,GAAKA,GAAUA,EAAItJ,KAAK+L,KAAK,EAAIzC,IAAM,EAAIA,IAAM,MAMxD,SAAS9R,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B+U,EAAU/U,EAAoB,GAElCa,GAAQA,EAAQmD,EAAG,QACjBgR,KAAM,QAASA,MAAK9C,GAClB,MAAO6C,GAAK7C,GAAKA,GAAKtJ,KAAKgL,IAAIhL,KAAKe,IAAIuI,GAAI,EAAI,OAM/C,SAAS9R,EAAQD,GAGtBC,EAAOD,QAAUyI,KAAKmM,MAAQ,QAASA,MAAK7C,GAC1C,MAAmB,KAAXA,GAAKA,IAAWA,GAAKA,EAAIA,EAAQ,EAAJA,EAAQ,GAAK,IAK/C,SAAS9R,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QACjBiR,MAAO,QAASA,OAAM/C,GACpB,OAAQA,KAAO,GAAK,GAAKtJ,KAAKiE,MAAMjE,KAAK+L,IAAIzC,EAAI,IAAOtJ,KAAKsM,OAAS,OAMrE,SAAS9U,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B4S,EAAUhK,KAAKgK,GAEnB/R,GAAQA,EAAQmD,EAAG,QACjBmR,KAAM,QAASA,MAAKjD,GAClB,OAAQU,EAAIV,GAAKA,GAAKU,GAAKV,IAAM,MAMhC,SAAS9R,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QAASoR,MAAOpV,EAAoB,OAIlD,SAASI,EAAQD,GAGtBC,EAAOD,QAAUyI,KAAKwM,OAAS,QAASA,OAAMlD,GAC5C,MAAmB,KAAXA,GAAKA,GAAUA,EAAIA,GAAK,MAAY,KAAJA,EAAWA,EAAIA,EAAIA,EAAI,EAAItJ,KAAKgK,IAAIV,GAAK,IAK9E,SAAS9R,EAAQD,EAASH,GAG/B,GAAIa,GAAYb,EAAoB,GAChC+U,EAAY/U,EAAoB,IAChC4T,EAAYhL,KAAKgL,IACjBD,EAAYC,EAAI,EAAG,KACnByB,EAAYzB,EAAI,EAAG,KACnB0B,EAAY1B,EAAI,EAAG,MAAQ,EAAIyB,GAC/BE,EAAY3B,EAAI,EAAG,MAEnB4B,EAAkB,SAASnP,GAC7B,MAAOA,GAAI,EAAIsN,EAAU,EAAIA,EAI/B9S,GAAQA,EAAQmD,EAAG,QACjByR,OAAQ,QAASA,QAAOvD,GACtB,GAEI/O,GAAGsC,EAFHiQ,EAAQ9M,KAAKe,IAAIuI,GACjByD,EAAQZ,EAAK7C,EAEjB,OAAUqD,GAAPG,EAAoBC,EAAQH,EAAgBE,EAAOH,EAAQF,GAAaE,EAAQF,GACnFlS,GAAK,EAAIkS,EAAY1B,GAAW+B,EAChCjQ,EAAStC,GAAKA,EAAIuS,GACfjQ,EAAS6P,GAAS7P,GAAUA,EAAckQ,GAAQC,EAAAA,GAC9CD,EAAQlQ,OAMd,SAASrF,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B2J,EAAUf,KAAKe,GAEnB9I,GAAQA,EAAQmD,EAAG,QACjB6R,MAAO,QAASA,OAAMC,EAAQC,GAO5B,IANA,GAKI/H,GAAKgI,EALLC,EAAQ,EACRlS,EAAQ,EACR+M,EAAQlK,UACRoL,EAAQlB,EAAGhN,OACXoS,EAAQ,EAEFlE,EAAJjO,GACJiK,EAAMrE,EAAImH,EAAG/M,MACHiK,EAAPkI,GACDF,EAAOE,EAAOlI,EACdiI,EAAOA,EAAMD,EAAMA,EAAM,EACzBE,EAAOlI,GACCA,EAAM,GACdgI,EAAOhI,EAAMkI,EACbD,GAAOD,EAAMA,GACRC,GAAOjI,CAEhB,OAAOkI,KAASN,EAAAA,EAAWA,EAAAA,EAAWM,EAAOtN,KAAK0L,KAAK2B,OAMtD,SAAS7V,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9BmW,EAAUvN,KAAKwN,IAGnBvV,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAIjE,EAAoB,GAAG,WACrD,MAA+B,IAAxBmW,EAAM,WAAY,IAA4B,GAAhBA,EAAMrS,SACzC,QACFsS,KAAM,QAASA,MAAKlE,EAAG3I,GACrB,GAAI8M,GAAS,MACTC,GAAMpE,EACNqE,GAAMhN,EACNiN,EAAKH,EAASC,EACdG,EAAKJ,EAASE,CAClB,OAAO,GAAIC,EAAKC,IAAOJ,EAASC,IAAO,IAAMG,EAAKD,GAAMH,EAASE,IAAO,KAAO,KAAO,OAMrF,SAASnW,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QACjB0S,MAAO,QAASA,OAAMxE,GACpB,MAAOtJ,MAAK+L,IAAIzC,GAAKtJ,KAAK+N,SAMzB,SAASvW,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QAASqQ,MAAOrU,EAAoB,OAIlD,SAASI,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QACjB4S,KAAM,QAASA,MAAK1E,GAClB,MAAOtJ,MAAK+L,IAAIzC,GAAKtJ,KAAKgM,QAMzB,SAASxU,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QAAS+Q,KAAM/U,EAAoB,OAIjD,SAASI,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9BoV,EAAUpV,EAAoB,IAC9B4S,EAAUhK,KAAKgK,GAGnB/R,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAIjE,EAAoB,GAAG,WACrD,MAA6B,SAArB4I,KAAKiO,KAAK,UAChB,QACFA,KAAM,QAASA,MAAK3E,GAClB,MAAOtJ,MAAKe,IAAIuI,GAAKA,GAAK,GACrBkD,EAAMlD,GAAKkD,GAAOlD,IAAM,GACxBU,EAAIV,EAAI,GAAKU,GAAKV,EAAI,KAAOtJ,KAAK2H,EAAI,OAM1C,SAASnQ,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9BoV,EAAUpV,EAAoB,IAC9B4S,EAAUhK,KAAKgK,GAEnB/R,GAAQA,EAAQmD,EAAG,QACjB8S,KAAM,QAASA,MAAK5E,GAClB,GAAI/O,GAAIiS,EAAMlD,GAAKA,GACfrG,EAAIuJ,GAAOlD,EACf,OAAO/O,IAAKyS,EAAAA,EAAW,EAAI/J,GAAK+J,EAAAA,EAAW,IAAMzS,EAAI0I,IAAM+G,EAAIV,GAAKU,GAAKV,QAMxE,SAAS9R,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QACjB+S,MAAO,QAASA,OAAMhL,GACpB,OAAQA,EAAK,EAAInD,KAAKiE,MAAQjE,KAAKgE,MAAMb,OAMxC,SAAS3L,EAAQD,EAASH,GAE/B,GAAIa,GAAiBb,EAAoB,GACrC4B,EAAiB5B,EAAoB,IACrCgX,EAAiBC,OAAOD,aACxBE,EAAiBD,OAAOE,aAG5BtW,GAAQA,EAAQmD,EAAInD,EAAQoD,KAAOiT,GAA2C,GAAzBA,EAAepT,QAAc,UAEhFqT,cAAe,QAASA,eAAcjF,GAMpC,IALA,GAIIkF,GAJAxJ,KACAkD,EAAQlK,UACRoL,EAAQlB,EAAGhN,OACXC,EAAQ,EAENiO,EAAQjO,GAAE,CAEd,GADAqT,GAAQtG,EAAG/M,KACRnC,EAAQwV,EAAM,WAAcA,EAAK,KAAM/N,YAAW+N,EAAO,6BAC5DxJ,GAAIlI,KAAY,MAAP0R,EACLJ,EAAaI,GACbJ,IAAeI,GAAQ,QAAY,IAAM,MAAQA,EAAO,KAAQ,QAEpE,MAAOxJ,GAAIlL,KAAK,QAMjB,SAAStC,EAAQD,EAASH,GAE/B,GAAIa,GAAYb,EAAoB,GAChC0B,EAAY1B,EAAoB,IAChC6B,EAAY7B,EAAoB,GAEpCa,GAAQA,EAAQmD,EAAG,UAEjBqT,IAAK,QAASA,KAAIC,GAOhB,IANA,GAAIC,GAAQ7V,EAAU4V,EAASD,KAC3BlR,EAAQtE,EAAS0V,EAAIzT,QACrBgN,EAAQlK,UACRoL,EAAQlB,EAAGhN,OACX8J,KACA7J,EAAQ,EACNoC,EAAMpC,GACV6J,EAAIlI,KAAKuR,OAAOM,EAAIxT,OACbiO,EAAJjO,GAAU6J,EAAIlI,KAAKuR,OAAOnG,EAAG/M,IAChC,OAAO6J,GAAIlL,KAAK,QAMjB,SAAStC,EAAQD,EAASH,GAI/BA,EAAoB,IAAI,OAAQ,SAASwX,GACvC,MAAO,SAASC,QACd,MAAOD,GAAM9Q,KAAM,OAMlB,SAAStG,EAAQD,EAASH,GAE/B,GAAIa,GAAUb,EAAoB,GAC9B2M,EAAU3M,EAAoB,IAC9BqB,EAAUrB,EAAoB,GAC9B0X,EAAU,+CAEVC,EAAU,IAAMD,EAAS,IACzBE,EAAU,KACVC,EAAUC,OAAO,IAAMH,EAAQA,EAAQ,KACvCI,EAAUD,OAAOH,EAAQA,EAAQ,MAEjCK,EAAW,SAASrF,EAAK3G,GAC3B,GAAI4G,KACJA,GAAID,GAAO3G,EAAKyL,GAChB5W,EAAQA,EAAQwC,EAAIxC,EAAQoD,EAAI5C,EAAM,WACpC,QAASqW,EAAO/E,MAAUiF,EAAIjF,MAAUiF,IACtC,SAAUhF,IAMZ6E,EAAOO,EAASP,KAAO,SAASQ,EAAQ9K,GAI1C,MAHA8K,GAAShB,OAAOtK,EAAQsL,IACd,EAAP9K,IAAS8K,EAASA,EAAOC,QAAQL,EAAO,KACjC,EAAP1K,IAAS8K,EAASA,EAAOC,QAAQH,EAAO,KACpCE,EAGT7X,GAAOD,QAAU6X,GAIZ,SAAS5X,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9BmY,EAAUnY,EAAoB,KAAI,EACtCa,GAAQA,EAAQwC,EAAG,UAEjB+U,YAAa,QAASA,aAAYC,GAChC,MAAOF,GAAIzR,KAAM2R,OAMhB,SAASjY,EAAQD,EAASH,GAE/B,GAAI2B,GAAY3B,EAAoB,IAChC2M,EAAY3M,EAAoB,GAGpCI,GAAOD,QAAU,SAASmY,GACxB,MAAO,UAAS9R,EAAM6R,GACpB,GAGIlV,GAAG0I,EAHHnC,EAAIuN,OAAOtK,EAAQnG,IACnBzC,EAAIpC,EAAU0W,GACdjI,EAAI1G,EAAE5F,MAEV,OAAO,GAAJC,GAASA,GAAKqM,EAASkI,EAAY,GAAKxY,GAC3CqD,EAAIuG,EAAE6O,WAAWxU,GACN,MAAJZ,GAAcA,EAAI,OAAUY,EAAI,IAAMqM,IAAMvE,EAAInC,EAAE6O,WAAWxU,EAAI,IAAM,OAAU8H,EAAI,MACxFyM,EAAY5O,EAAErC,OAAOtD,GAAKZ,EAC1BmV,EAAY5O,EAAElH,MAAMuB,EAAGA,EAAI,IAAMZ,EAAI,OAAU,KAAO0I,EAAI,OAAU,UAMvE,SAASzL,EAAQD,EAASH,GAI/B,GAAIa,GAAYb,EAAoB,GAChC6B,EAAY7B,EAAoB,IAChCwY,EAAYxY,EAAoB,IAChCyY,EAAY,WACZC,EAAY,GAAGD,EAEnB5X,GAAQA,EAAQwC,EAAIxC,EAAQoD,EAAIjE,EAAoB,IAAIyY,GAAY,UAClEE,SAAU,QAASA,UAASC,GAC1B,GAAIpS,GAAOgS,EAAQ9R,KAAMkS,EAAcH,GACnC3H,EAAOlK,UACPiS,EAAc/H,EAAGhN,OAAS,EAAIgN,EAAG,GAAKhR,EACtCqG,EAAStE,EAAS2E,EAAK1C,QACvBiD,EAAS8R,IAAgB/Y,EAAYqG,EAAMyC,KAAKC,IAAIhH,EAASgX,GAAc1S,GAC3E2S,EAAS7B,OAAO2B,EACpB,OAAOF,GACHA,EAAUnY,KAAKiG,EAAMsS,EAAQ/R,GAC7BP,EAAKhE,MAAMuE,EAAM+R,EAAOhV,OAAQiD,KAAS+R,MAM5C,SAAS1Y,EAAQD,EAASH,GAG/B,GAAI+Y,GAAW/Y,EAAoB,IAC/B2M,EAAW3M,EAAoB,GAEnCI,GAAOD,QAAU,SAASqG,EAAMoS,EAAcI,GAC5C,GAAGD,EAASH,GAAc,KAAMpV,WAAU,UAAYwV,EAAO,yBAC7D,OAAO/B,QAAOtK,EAAQnG,MAKnB,SAASpG,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,IAC/BmB,EAAWnB,EAAoB,IAC/BiZ,EAAWjZ,EAAoB,IAAI,QACvCI,GAAOD,QAAU,SAAS4L,GACxB,GAAIgN,EACJ,OAAOvX,GAASuK,MAASgN,EAAWhN,EAAGkN,MAAYnZ,IAAciZ,EAAsB,UAAX5X,EAAI4K,MAK7E,SAAS3L,EAAQD,EAASH,GAE/B,GAAIiZ,GAAQjZ,EAAoB,IAAI,QACpCI,GAAOD,QAAU,SAASwS,GACxB,GAAIuG,GAAK,GACT,KACE,MAAMvG,GAAKuG,GACX,MAAM3V,GACN,IAEE,MADA2V,GAAGD,IAAS,GACJ,MAAMtG,GAAKuG,GACnB,MAAMrL,KACR,OAAO,IAKN,SAASzN,EAAQD,EAASH,GAI/B,GAAIa,GAAWb,EAAoB,GAC/BwY,EAAWxY,EAAoB,IAC/BmZ,EAAW,UAEftY,GAAQA,EAAQwC,EAAIxC,EAAQoD,EAAIjE,EAAoB,IAAImZ,GAAW,UACjEC,SAAU,QAASA,UAASR,GAC1B,SAAUJ,EAAQ9R,KAAMkS,EAAcO,GACnC3Q,QAAQoQ,EAAchS,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,OAM9D,SAASM,EAAQD,EAASH,GAE/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQwC,EAAG,UAEjBgW,OAAQrZ,EAAoB,QAKzB,SAASI,EAAQD,EAASH,GAG/B,GAAI2B,GAAY3B,EAAoB,IAChC2M,EAAY3M,EAAoB,GAEpCI,GAAOD,QAAU,QAASkZ,QAAOC,GAC/B,GAAIC,GAAMtC,OAAOtK,EAAQjG,OACrBkH,EAAM,GACNvH,EAAM1E,EAAU2X,EACpB,IAAO,EAAJjT,GAASA,GAAKuP,EAAAA,EAAS,KAAMvM,YAAW,0BAC3C,MAAKhD,EAAI,GAAIA,KAAO,KAAOkT,GAAOA,GAAY,EAAJlT,IAAMuH,GAAO2L,EACvD,OAAO3L,KAKJ,SAASxN,EAAQD,EAASH,GAI/B,GAAIa,GAAcb,EAAoB,GAClC6B,EAAc7B,EAAoB,IAClCwY,EAAcxY,EAAoB,IAClCwZ,EAAc,aACdC,EAAc,GAAGD,EAErB3Y,GAAQA,EAAQwC,EAAIxC,EAAQoD,EAAIjE,EAAoB,IAAIwZ,GAAc,UACpEE,WAAY,QAASA,YAAWd,GAC9B,GAAIpS,GAASgS,EAAQ9R,KAAMkS,EAAcY,GACrC1I,EAASlK,UACTgB,EAAS/F,EAAS+G,KAAKC,IAAIiI,EAAGhN,OAAS,EAAIgN,EAAG,GAAKhR,EAAW0G,EAAK1C,SACnEgV,EAAS7B,OAAO2B,EACpB,OAAOa,GACHA,EAAYlZ,KAAKiG,EAAMsS,EAAQlR,GAC/BpB,EAAKhE,MAAMoF,EAAOA,EAAQkR,EAAOhV,UAAYgV,MAMhD,SAAS1Y,EAAQD,EAASH,GAG/B,GAAImY,GAAOnY,EAAoB,KAAI,EAGnCA,GAAoB,KAAKiX,OAAQ,SAAU,SAAS0C,GAClDjT,KAAKkT,GAAK3C,OAAO0C,GACjBjT,KAAKmT,GAAK,GAET,WACD,GAEIC,GAFA1W,EAAQsD,KAAKkT,GACbhS,EAAQlB,KAAKmT,EAEjB,OAAGjS,IAASxE,EAAEU,QAAeL,MAAO3D,EAAWia,MAAM,IACrDD,EAAQ3B,EAAI/U,EAAGwE,GACflB,KAAKmT,IAAMC,EAAMhW,QACTL,MAAOqW,EAAOC,MAAM,OAKzB,SAAS3Z,EAAQD,EAASH,GAG/B,GAAIga,GAAiBha,EAAoB,IACrCa,EAAiBb,EAAoB,GACrCsO,EAAiBtO,EAAoB,IACrCia,EAAiBja,EAAoB,IACrCkB,EAAiBlB,EAAoB,IACrCka,EAAiBla,EAAoB,KACrCma,EAAiBna,EAAoB,KACrCyO,EAAiBzO,EAAoB,IACrC6F,EAAiB7F,EAAoB,GAAG6F,SACxCuU,EAAiBpa,EAAoB,IAAI,YACzCqa,OAAsBzW,MAAQ,WAAaA,QAC3C0W,EAAiB,aACjBC,EAAiB,OACjBC,EAAiB,SAEjBC,EAAa,WAAY,MAAO/T,MAEpCtG,GAAOD,QAAU,SAASua,EAAM1B,EAAM2B,EAAaC,EAAMC,EAASC,EAAQC,GACxEZ,EAAYQ,EAAa3B,EAAM4B,EAC/B,IAaII,GAASxV,EAbTyV,EAAY,SAASC,GACvB,IAAIb,GAASa,IAAQ7I,GAAM,MAAOA,GAAM6I,EACxC,QAAOA,GACL,IAAKX,GAAM,MAAO,SAAS3W,QAAQ,MAAO,IAAI+W,GAAYjU,KAAMwU,GAChE,KAAKV,GAAQ,MAAO,SAASW,UAAU,MAAO,IAAIR,GAAYjU,KAAMwU,IACpE,MAAO,SAASE,WAAW,MAAO,IAAIT,GAAYjU,KAAMwU,KAExD5J,EAAa0H,EAAO,YACpBqC,EAAaR,GAAWL,EACxBc,GAAa,EACbjJ,EAAaqI,EAAKtY,UAClBmZ,EAAalJ,EAAM+H,IAAa/H,EAAMiI,IAAgBO,GAAWxI,EAAMwI,GACvEW,EAAaD,GAAWN,EAAUJ,EAGtC,IAAGU,EAAQ,CACT,GAAIE,GAAoB5V,EAAS2V,EAASjb,KAAK,GAAIma,IAEnDjM,GAAegN,EAAmBnK,GAAK,IAEnC0I,GAAW9Y,EAAImR,EAAOiI,IAAaL,EAAKwB,EAAmBrB,EAAUK,GAEtEY,GAAcE,EAAQ7Q,OAAS8P,IAChCc,GAAa,EACbE,EAAW,QAASL,UAAU,MAAOI,GAAQhb,KAAKmG,QAUtD,GANKsT,IAAWe,IAAYV,IAASiB,GAAejJ,EAAM+H,IACxDH,EAAK5H,EAAO+H,EAAUoB,GAGxBtB,EAAUlB,GAAQwC,EAClBtB,EAAU5I,GAAQmJ,EACfI,EAMD,GALAG,GACEG,OAASE,EAAcG,EAAWP,EAAUT,GAC5C5W,KAASkX,EAAcU,EAAWP,EAAUV,GAC5Ca,QAAUC,EAAwBJ,EAAU,WAArBO,GAEtBT,EAAO,IAAIvV,IAAOwV,GACdxV,IAAO6M,IAAO/D,EAAS+D,EAAO7M,EAAKwV,EAAQxV,QAC3C3E,GAAQA,EAAQwC,EAAIxC,EAAQoD,GAAKoW,GAASiB,GAAatC,EAAMgC,EAEtE,OAAOA,KAKJ,SAAS5a,EAAQD,GAEtBC,EAAOD,YAIF,SAASC,EAAQD,EAASH,GAG/B,GAAIY,GAAiBZ,EAAoB,GACrC0b,EAAiB1b,EAAoB,IACrCyO,EAAiBzO,EAAoB,IACrCyb,IAGJzb,GAAoB,IAAIyb,EAAmBzb,EAAoB,IAAI,YAAa,WAAY,MAAO0G,QAEnGtG,EAAOD,QAAU,SAASwa,EAAa3B,EAAM4B,GAC3CD,EAAYvY,UAAYxB,EAAEqF,OAAOwV,GAAoBb,KAAMc,EAAW,EAAGd,KACzEnM,EAAekM,EAAa3B,EAAO,eAKhC,SAAS5Y,EAAQD,EAASH,GAG/B,GAAIuK,GAAcvK,EAAoB,GAClCa,EAAcb,EAAoB,GAClCyB,EAAczB,EAAoB,IAClCO,EAAcP,EAAoB,KAClC2b,EAAc3b,EAAoB,KAClC6B,EAAc7B,EAAoB,IAClC4b,EAAc5b,EAAoB,IACtCa,GAAQA,EAAQmD,EAAInD,EAAQoD,GAAKjE,EAAoB,KAAK,SAAS6b,GAAOvZ,MAAMwZ,KAAKD,KAAW,SAE9FC,KAAM,QAASA,MAAKC,GAClB,GAQIjY,GAAQ2B,EAAQuW,EAAMC,EARtB7Y,EAAU3B,EAASsa,GACnBvQ,EAAyB,kBAAR9E,MAAqBA,KAAOpE,MAC7CwO,EAAUlK,UACVoL,EAAUlB,EAAGhN,OACboY,EAAUlK,EAAQ,EAAIlB,EAAG,GAAKhR,EAC9Bqc,EAAUD,IAAUpc,EACpB8H,EAAU,EACVwU,EAAUR,EAAUxY,EAIxB,IAFG+Y,IAAQD,EAAQ3R,EAAI2R,EAAOlK,EAAQ,EAAIlB,EAAG,GAAKhR,EAAW,IAE1Dsc,GAAUtc,GAAe0L,GAAKlJ,OAASqZ,EAAYS,GAMpD,IADAtY,EAASjC,EAASuB,EAAEU,QAChB2B,EAAS,GAAI+F,GAAE1H,GAASA,EAAS8D,EAAOA,IAC1CnC,EAAOmC,GAASuU,EAAUD,EAAM9Y,EAAEwE,GAAQA,GAASxE,EAAEwE,OANvD,KAAIqU,EAAWG,EAAO7b,KAAK6C,GAAIqC,EAAS,GAAI+F,KAAKwQ,EAAOC,EAASrB,QAAQb,KAAMnS,IAC7EnC,EAAOmC,GAASuU,EAAU5b,EAAK0b,EAAUC,GAAQF,EAAKvY,MAAOmE,IAAQ,GAAQoU,EAAKvY,KAStF,OADAgC,GAAO3B,OAAS8D,EACTnC,MAON,SAASrF,EAAQD,EAASH,GAG/B,GAAIsB,GAAWtB,EAAoB,GACnCI,GAAOD,QAAU,SAAS8b,EAAUxV,EAAIhD,EAAO2X,GAC7C,IACE,MAAOA,GAAU3U,EAAGnF,EAASmC,GAAO,GAAIA,EAAM,IAAMgD,EAAGhD,GAEvD,MAAMF,GACN,GAAI8Y,GAAMJ,EAAS,SAEnB,MADGI,KAAQvc,GAAUwB,EAAS+a,EAAI9b,KAAK0b,IACjC1Y,KAML,SAASnD,EAAQD,EAASH,GAG/B,GAAIka,GAAala,EAAoB,KACjCoa,EAAapa,EAAoB,IAAI,YACrCqC,EAAaC,MAAMF,SAEvBhC,GAAOD,QAAU,SAAS4L,GACxB,MAAOA,KAAOjM,IAAcoa,EAAU5X,QAAUyJ,GAAM1J,EAAW+X,KAAcrO,KAK5E,SAAS3L,EAAQD,EAASH,GAE/B,GAAIsc,GAAYtc,EAAoB,KAChCoa,EAAYpa,EAAoB,IAAI,YACpCka,EAAYla,EAAoB,IACpCI,GAAOD,QAAUH,EAAoB,GAAGuc,kBAAoB,SAASxQ,GACnE,MAAGA,IAAMjM,EAAiBiM,EAAGqO,IACxBrO,EAAG,eACHmO,EAAUoC,EAAQvQ,IAFvB,SAOG,SAAS3L,EAAQD,EAASH,GAG/B,GAAImB,GAAMnB,EAAoB,IAC1BsR,EAAMtR,EAAoB,IAAI,eAE9Bwc,EAAgD,aAA1Crb,EAAI,WAAY,MAAOyF,cAEjCxG,GAAOD,QAAU,SAAS4L,GACxB,GAAI3I,GAAG2O,EAAG3G,CACV,OAAOW,KAAOjM,EAAY,YAAqB,OAAPiM,EAAc,OAEZ,iBAA9BgG,GAAK3O,EAAIjB,OAAO4J,IAAKuF,IAAoBS,EAEjDyK,EAAMrb,EAAIiC,GAEM,WAAfgI,EAAIjK,EAAIiC,KAAsC,kBAAZA,GAAEqZ,OAAuB,YAAcrR,IAK3E,SAAShL,EAAQD,EAASH,GAE/B,GAAIoa,GAAepa,EAAoB,IAAI,YACvC0c,GAAe,CAEnB,KACE,GAAIC,IAAS,GAAGvC,IAChBuC,GAAM,UAAY,WAAYD,GAAe,GAC7Cpa,MAAMwZ,KAAKa,EAAO,WAAY,KAAM,KACpC,MAAMpZ,IAERnD,EAAOD,QAAU,SAAS6L,EAAM4Q,GAC9B,IAAIA,IAAgBF,EAAa,OAAO,CACxC,IAAIG,IAAO,CACX,KACE,GAAIC,IAAQ,GACRjB,EAAOiB,EAAI1C,IACfyB,GAAKjB,KAAO,WAAY,OAAQb,KAAM8C,GAAO,IAC7CC,EAAI1C,GAAY,WAAY,MAAOyB,IACnC7P,EAAK8Q,GACL,MAAMvZ,IACR,MAAOsZ,KAKJ,SAASzc,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAGlCa,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAIjE,EAAoB,GAAG,WACrD,QAASiE,MACT,QAAS3B,MAAMya,GAAGxc,KAAK0D,YAAcA,MACnC,SAEF8Y,GAAI,QAASA,MAKX,IAJA,GAAInV,GAAS,EACTkJ,EAASlK,UACToL,EAASlB,EAAGhN,OACZ2B,EAAS,IAAoB,kBAARiB,MAAqBA,KAAOpE,OAAO0P,GACtDA,EAAQpK,GAAMnC,EAAOmC,GAASkJ,EAAGlJ,IAEvC,OADAnC,GAAO3B,OAASkO,EACTvM,MAMN,SAASrF,EAAQD,EAASH,GAG/B,GAAIgd,GAAmBhd,EAAoB,KACvCgc,EAAmBhc,EAAoB,KACvCka,EAAmBla,EAAoB,KACvC0B,EAAmB1B,EAAoB,GAM3CI,GAAOD,QAAUH,EAAoB,KAAKsC,MAAO,QAAS,SAASqX,EAAUuB,GAC3ExU,KAAKkT,GAAKlY,EAAUiY,GACpBjT,KAAKmT,GAAK,EACVnT,KAAKqJ,GAAKmL,GAET,WACD,GAAI9X,GAAQsD,KAAKkT,GACbsB,EAAQxU,KAAKqJ,GACbnI,EAAQlB,KAAKmT,IACjB,QAAIzW,GAAKwE,GAASxE,EAAEU,QAClB4C,KAAKkT,GAAK9Z,EACHkc,EAAK,IAEH,QAARd,EAAwBc,EAAK,EAAGpU,GACxB,UAARsT,EAAwBc,EAAK,EAAG5Y,EAAEwE,IAC9BoU,EAAK,GAAIpU,EAAOxE,EAAEwE,MACxB,UAGHsS,EAAU+C,UAAY/C,EAAU5X,MAEhC0a,EAAiB,QACjBA,EAAiB,UACjBA,EAAiB,YAIZ,SAAS5c,EAAQD,GAEtBC,EAAOD,QAAU,cAIZ,SAASC,EAAQD,GAEtBC,EAAOD,QAAU,SAAS4Z,EAAMtW,GAC9B,OAAQA,MAAOA,EAAOsW,OAAQA,KAK3B,SAAS3Z,EAAQD,EAASH,GAE/BA,EAAoB,KAAK,UAIpB,SAASI,EAAQD,EAASH,GAG/B,GAAIsK,GAActK,EAAoB,GAClCY,EAAcZ,EAAoB,GAClCc,EAAcd,EAAoB,GAClC8N,EAAc9N,EAAoB,IAAI,UAE1CI,GAAOD,QAAU,SAASwS,GACxB,GAAInH,GAAIlB,EAAKqI,EACV7R,IAAe0K,IAAMA,EAAEsC,IAASlN,EAAEgC,QAAQ4I,EAAGsC,GAC9C3B,cAAc,EACdjJ,IAAK,WAAY,MAAOwD,WAMvB,SAAStG,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQwC,EAAG,SAAU6Z,WAAYld,EAAoB,OAE7DA,EAAoB,KAAK,eAIpB,SAASI,EAAQD,EAASH,GAI/B,GAAIyB,GAAWzB,EAAoB,IAC/B4B,EAAW5B,EAAoB,IAC/B6B,EAAW7B,EAAoB,GAEnCI,GAAOD,WAAa+c,YAAc,QAASA,YAAW3R,EAAetE,GACnE,GAAI7D,GAAQ3B,EAASiF,MACjBP,EAAQtE,EAASuB,EAAEU,QACnBqZ,EAAQvb,EAAQ2J,EAAQpF,GACxB2V,EAAQla,EAAQqF,EAAOd,GACvB2K,EAAQlK,UACRG,EAAQ+J,EAAGhN,OAAS,EAAIgN,EAAG,GAAKhR,EAChCwZ,EAAQ1Q,KAAKC,KAAK9B,IAAQjH,EAAYqG,EAAMvE,EAAQmF,EAAKZ,IAAQ2V,EAAM3V,EAAMgX,GAC7EC,EAAQ,CAMZ,KALUD,EAAPrB,GAAkBA,EAAOxC,EAAZ6D,IACdC,EAAO,GACPtB,GAAQxC,EAAQ,EAChB6D,GAAQ7D,EAAQ,GAEZA,IAAU,GACXwC,IAAQ1Y,GAAEA,EAAE+Z,GAAM/Z,EAAE0Y,SACX1Y,GAAE+Z,GACdA,GAAQC,EACRtB,GAAQsB,CACR,OAAOha,KAKN,SAAShD,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQwC,EAAG,SAAUga,KAAMrd,EAAoB,OAEvDA,EAAoB,KAAK,SAIpB,SAASI,EAAQD,EAASH,GAI/B,GAAIyB,GAAWzB,EAAoB,IAC/B4B,EAAW5B,EAAoB,IAC/B6B,EAAW7B,EAAoB,GACnCI,GAAOD,WAAakd,MAAQ,QAASA,MAAK5Z,GAQxC,IAPA,GAAIL,GAAS3B,EAASiF,MAClB5C,EAASjC,EAASuB,EAAEU,QACpBgN,EAASlK,UACToL,EAASlB,EAAGhN,OACZ8D,EAAShG,EAAQoQ,EAAQ,EAAIlB,EAAG,GAAKhR,EAAWgE,GAChDiD,EAASiL,EAAQ,EAAIlB,EAAG,GAAKhR,EAC7Bwd,EAASvW,IAAQjH,EAAYgE,EAASlC,EAAQmF,EAAKjD,GACjDwZ,EAAS1V,GAAMxE,EAAEwE,KAAWnE,CAClC,OAAOL,KAKJ,SAAShD,EAAQD,EAASH,GAI/B,GAAIa,GAAUb,EAAoB,GAC9Bud,EAAUvd,EAAoB,IAAI,GAClC2S,EAAU,OACV6K,GAAU,CAEX7K,SAAUrQ,MAAM,GAAGqQ,GAAK,WAAY6K,GAAS,IAChD3c,EAAQA,EAAQwC,EAAIxC,EAAQoD,EAAIuZ,EAAQ,SACtCC,KAAM,QAASA,MAAK/V,GAClB,MAAO6V,GAAM7W,KAAMgB,EAAYd,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAGzEE,EAAoB,KAAK2S,IAIpB,SAASvS,EAAQD,EAASH,GAI/B,GAAIa,GAAUb,EAAoB,GAC9Bud,EAAUvd,EAAoB,IAAI,GAClC2S,EAAU,YACV6K,GAAU,CAEX7K,SAAUrQ,MAAM,GAAGqQ,GAAK,WAAY6K,GAAS,IAChD3c,EAAQA,EAAQwC,EAAIxC,EAAQoD,EAAIuZ,EAAQ,SACtCE,UAAW,QAASA,WAAUhW,GAC5B,MAAO6V,GAAM7W,KAAMgB,EAAYd,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAGzEE,EAAoB,KAAK2S,IAIpB,SAASvS,EAAQD,EAASH,GAG/B,GAqBI2d,GArBA/c,EAAaZ,EAAoB,GACjCga,EAAaha,EAAoB,IACjCqK,EAAarK,EAAoB,GACjCuK,EAAavK,EAAoB,GACjCsc,EAAatc,EAAoB,KACjCa,EAAab,EAAoB,GACjCwB,EAAaxB,EAAoB,IACjCsB,EAAatB,EAAoB,IACjCuB,EAAavB,EAAoB,GACjC4d,EAAa5d,EAAoB,KACjC6d,EAAa7d,EAAoB,KACjC8d,EAAa9d,EAAoB,IAAIgQ,IACrC+N,EAAa/d,EAAoB,IACjC8N,EAAa9N,EAAoB,IAAI,WACrCge,EAAqBhe,EAAoB,KACzCie,EAAaje,EAAoB,KACjCke,EAAa,UACbC,EAAa9T,EAAO8T,QACpBC,EAAiC,WAApB9B,EAAQ6B,GACrB9a,EAAagH,EAAO6T,GACpBG,EAAa,aAGbC,EAAc,SAASC,GACzB,GAAyBC,GAArBlM,EAAO,GAAIjP,GAAEgb,EAKjB,OAJGE,KAAIjM,EAAKxM,YAAc,SAASkG,GACjCA,EAAKqS,EAAOA,MAEbG,EAAUnb,EAAEob,QAAQnM,IAAO,SAAS+L,GAC9BG,IAAYlM,GAGjBoM,EAAa,WAEf,QAASC,IAAGzM,GACV,GAAIvG,GAAO,GAAItI,GAAE6O,EAEjB,OADA4L,GAASnS,EAAMgT,GAAGvc,WACXuJ,EAJT,GAAIiT,IAAQ,CAMZ,KASE,GARAA,EAAQvb,GAAKA,EAAEob,SAAWH,IAC1BR,EAASa,GAAItb,GACbsb,GAAGvc,UAAYxB,EAAEqF,OAAO5C,EAAEjB,WAAY0D,aAAcrC,MAAOkb,MAEtDA,GAAGF,QAAQ,GAAGI,KAAK,uBAAyBF,MAC/CC,GAAQ,GAGPA,GAAS5e,EAAoB,GAAG,CACjC,GAAI8e,IAAqB,CACzBzb,GAAEob,QAAQ7d,EAAEgC,WAAY,QACtBM,IAAK,WAAY4b,GAAqB,MAExCF,EAAQE,GAEV,MAAMvb,GAAIqb,GAAQ,EACpB,MAAOA,MAILG,EAAkB,SAAS5b,EAAG0I,GAEhC,MAAGmO,IAAW7W,IAAME,GAAKwI,IAAM8R,GAAe,EACvCI,EAAK5a,EAAG0I,IAEbmT,EAAiB,SAASxT,GAC5B,GAAIxH,GAAI1C,EAASkK,GAAGsC,EACpB,OAAO9J,IAAKlE,EAAYkE,EAAIwH,GAE1ByT,EAAa,SAASlT,GACxB,GAAI8S,EACJ,OAAOrd,GAASuK,IAAkC,mBAAnB8S,EAAO9S,EAAG8S,MAAsBA,GAAO,GAEpEK,EAAoB,SAAS1T,GAC/B,GAAIiT,GAASU,CACbzY,MAAK8X,QAAU,GAAIhT,GAAE,SAAS4T,EAAWC,GACvC,GAAGZ,IAAY3e,GAAaqf,IAAWrf,EAAU,KAAM0D,WAAU,0BACjEib,GAAUW,EACVD,EAAUE,IAEZ3Y,KAAK+X,QAAUld,EAAUkd,GACzB/X,KAAKyY,OAAU5d,EAAU4d,IAEvBG,EAAU,SAAStT,GACrB,IACEA,IACA,MAAMzI,GACN,OAAQgc,MAAOhc,KAGfic,EAAS,SAASC,EAAQC,GAC5B,IAAGD,EAAOpZ,EAAV,CACAoZ,EAAOpZ,GAAI,CACX,IAAIsZ,GAAQF,EAAOhf,CACnBwd,GAAK,WAuBH,IAtBA,GAAIxa,GAAQgc,EAAOG,EACfC,EAAoB,GAAZJ,EAAO/V,EACf3F,EAAQ,EACR+b,EAAM,SAASC,GACjB,GAGIta,GAAQoZ,EAHRmB,EAAUH,EAAKE,EAASF,GAAKE,EAASE,KACtCxB,EAAUsB,EAAStB,QACnBU,EAAUY,EAASZ,MAEvB,KACKa,GACGH,IAAGJ,EAAOS,GAAI,GAClBza,EAASua,KAAY,EAAOvc,EAAQuc,EAAQvc,GACzCgC,IAAWsa,EAASvB,QACrBW,EAAO3b,UAAU,yBACTqb,EAAOI,EAAWxZ,IAC1BoZ,EAAKte,KAAKkF,EAAQgZ,EAASU,GACtBV,EAAQhZ,IACV0Z,EAAO1b,GACd,MAAMF,GACN4b,EAAO5b,KAGLoc,EAAM7b,OAASC,GAAE+b,EAAIH,EAAM5b,KACjC4b,GAAM7b,OAAS,EACf2b,EAAOpZ,GAAI,EACRqZ,GAASS,WAAW,WACrB,GACIH,GAASI,EADT5B,EAAUiB,EAAO/e,CAElB2f,GAAY7B,KACVJ,EACDD,EAAQmC,KAAK,qBAAsB7c,EAAO+a,IAClCwB,EAAU3V,EAAOkW,sBACzBP,GAASxB,QAASA,EAASgC,OAAQ/c,KAC1B2c,EAAU/V,EAAO+V,UAAYA,EAAQb,OAC9Ca,EAAQb,MAAM,8BAA+B9b,IAE/Cgc,EAAOtc,EAAIrD,GACZ,OAGHugB,EAAc,SAAS7B,GACzB,GAGIuB,GAHAN,EAASjB,EAAQiC,GACjBd,EAASF,EAAOtc,GAAKsc,EAAOhf,EAC5BsD,EAAS,CAEb,IAAG0b,EAAOS,EAAE,OAAO,CACnB,MAAMP,EAAM7b,OAASC,GAEnB,GADAgc,EAAWJ,EAAM5b,KACdgc,EAASE,OAASI,EAAYN,EAASvB,SAAS,OAAO,CAC1D,QAAO,GAEPkC,EAAU,SAASjd,GACrB,GAAIgc,GAAS/Y,IACV+Y,GAAOnW,IACVmW,EAAOnW,GAAI,EACXmW,EAASA,EAAOkB,GAAKlB,EACrBA,EAAOG,EAAInc,EACXgc,EAAO/V,EAAI,EACX+V,EAAOtc,EAAIsc,EAAOhf,EAAE+B,QACpBgd,EAAOC,GAAQ,KAEbmB,EAAW,SAASnd,GACtB,GACIob,GADAY,EAAS/Y,IAEb,KAAG+Y,EAAOnW,EAAV,CACAmW,EAAOnW,GAAI,EACXmW,EAASA,EAAOkB,GAAKlB,CACrB,KACE,GAAGA,EAAO/e,IAAM+C,EAAM,KAAMD,WAAU,qCACnCqb,EAAOI,EAAWxb,IACnBwa,EAAK,WACH,GAAI4C,IAAWF,EAAGlB,EAAQnW,GAAG,EAC7B,KACEuV,EAAKte,KAAKkD,EAAO8G,EAAIqW,EAAUC,EAAS,GAAItW,EAAImW,EAASG,EAAS,IAClE,MAAMtd,GACNmd,EAAQngB,KAAKsgB,EAAStd,OAI1Bkc,EAAOG,EAAInc,EACXgc,EAAO/V,EAAI,EACX8V,EAAOC,GAAQ,IAEjB,MAAMlc,GACNmd,EAAQngB,MAAMogB,EAAGlB,EAAQnW,GAAG,GAAQ/F,KAKpCmb,KAEFrb,EAAI,QAASyd,SAAQC,GACnBxf,EAAUwf,EACV,IAAItB,GAAS/Y,KAAK+Z,IAChB/f,EAAGkd,EAAUlX,KAAMrD,EAAG6a,GACtBzd,KACA0C,EAAGrD,EACH4J,EAAG,EACHJ,GAAG,EACHsW,EAAG9f,EACHogB,GAAG,EACH7Z,GAAG,EAEL,KACE0a,EAASxW,EAAIqW,EAAUnB,EAAQ,GAAIlV,EAAImW,EAASjB,EAAQ,IACxD,MAAMuB,GACNN,EAAQngB,KAAKkf,EAAQuB,KAGzBhhB,EAAoB,KAAKqD,EAAEjB,WAEzByc,KAAM,QAASA,MAAKoC,EAAaC,GAC/B,GAAInB,GAAW,GAAIb,GAAkBlB,EAAmBtX,KAAMrD,IAC1Dmb,EAAWuB,EAASvB,QACpBiB,EAAW/Y,KAAK+Z,EAMpB,OALAV,GAASF,GAA6B,kBAAfoB,GAA4BA,GAAc,EACjElB,EAASE,KAA4B,kBAAdiB,IAA4BA,EACnDzB,EAAOhf,EAAEiF,KAAKqa,GACXN,EAAOtc,GAAEsc,EAAOtc,EAAEuC,KAAKqa,GACvBN,EAAO/V,GAAE8V,EAAOC,GAAQ,GACpBjB,GAGT2C,QAAS,SAASD,GAChB,MAAOxa,MAAKmY,KAAK/e,EAAWohB,OAKlCrgB,EAAQA,EAAQmK,EAAInK,EAAQyK,EAAIzK,EAAQoD,GAAKya,GAAaoC,QAASzd,IACnErD,EAAoB,IAAIqD,EAAG6a,GAC3Ble,EAAoB,KAAKke,GACzBP,EAAU3d,EAAoB,GAAGke,GAGjCrd,EAAQA,EAAQmD,EAAInD,EAAQoD,GAAKya,EAAYR,GAE3CiB,OAAQ,QAASA,QAAOwB,GACtB,GAAIS,GAAa,GAAIlC,GAAkBxY,MACnC2Y,EAAa+B,EAAWjC,MAE5B,OADAE,GAASsB,GACFS,EAAW5C,WAGtB3d,EAAQA,EAAQmD,EAAInD,EAAQoD,IAAMya,GAAcJ,GAAY,IAAQJ,GAElEO,QAAS,QAASA,SAAQvM,GAExB,GAAGA,YAAa7O,IAAK0b,EAAgB7M,EAAEpM,YAAaY,MAAM,MAAOwL,EACjE,IAAIkP,GAAa,GAAIlC,GAAkBxY,MACnC0Y,EAAagC,EAAW3C,OAE5B,OADAW,GAAUlN,GACHkP,EAAW5C,WAGtB3d,EAAQA,EAAQmD,EAAInD,EAAQoD,IAAMya,GAAc1e,EAAoB,KAAK,SAAS6b,GAChFxY,EAAEge,IAAIxF,GAAM,SAAS,iBAClBqC,GAEHmD,IAAK,QAASA,KAAIC,GAChB,GAAI9V,GAAawT,EAAetY,MAC5B0a,EAAa,GAAIlC,GAAkB1T,GACnCiT,EAAa2C,EAAW3C,QACxBU,EAAaiC,EAAWjC,OACxBhE,KACAoG,EAASjC,EAAQ,WACnBzB,EAAMyD,GAAU,EAAOnG,EAAOzV,KAAMyV,EACpC,IAAIqG,GAAYrG,EAAOrX,OACnB2d,EAAYnf,MAAMkf,EACnBA,GAAU5gB,EAAEqH,KAAK1H,KAAK4a,EAAQ,SAASqD,EAAS5W,GACjD,GAAI8Z,IAAgB,CACpBlW,GAAEiT,QAAQD,GAASK,KAAK,SAASpb,GAC5Bie,IACHA,GAAgB,EAChBD,EAAQ7Z,GAASnE,IACf+d,GAAa/C,EAAQgD,KACtBtC,KAEAV,EAAQgD,IAGf,OADGF,IAAOpC,EAAOoC,EAAOhC,OACjB6B,EAAW5C,SAGpBmD,KAAM,QAASA,MAAKL,GAClB,GAAI9V,GAAawT,EAAetY,MAC5B0a,EAAa,GAAIlC,GAAkB1T,GACnC2T,EAAaiC,EAAWjC,OACxBoC,EAASjC,EAAQ,WACnBzB,EAAMyD,GAAU,EAAO,SAAS9C,GAC9BhT,EAAEiT,QAAQD,GAASK,KAAKuC,EAAW3C,QAASU,MAIhD,OADGoC,IAAOpC,EAAOoC,EAAOhC,OACjB6B,EAAW5C,YAMjB,SAASpe,EAAQD,GAEtBC,EAAOD,QAAU,SAAS4L,EAAI4O,EAAajQ,GACzC,KAAKqB,YAAc4O,IAAa,KAAMnX,WAAUkH,EAAO,4BACvD,OAAOqB,KAKJ,SAAS3L,EAAQD,EAASH,GAE/B,GAAIuK,GAAcvK,EAAoB,GAClCO,EAAcP,EAAoB,KAClC2b,EAAc3b,EAAoB,KAClCsB,EAActB,EAAoB,IAClC6B,EAAc7B,EAAoB,IAClC4b,EAAc5b,EAAoB,IACtCI,GAAOD,QAAU,SAASmhB,EAAUlG,EAAS3U,EAAID,GAC/C,GAGI1C,GAAQkY,EAAMC,EAHdG,EAASR,EAAU0F,GACnBzT,EAAStD,EAAI9D,EAAID,EAAM4U,EAAU,EAAI,GACrCxT,EAAS,CAEb,IAAoB,kBAAVwU,GAAqB,KAAM5Y,WAAU8d,EAAW,oBAE1D,IAAG3F,EAAYS,GAAQ,IAAItY,EAASjC,EAASyf,EAASxd,QAASA,EAAS8D,EAAOA,IAC7EwT,EAAUvN,EAAEvM,EAAS0a,EAAOsF,EAAS1Z,IAAQ,GAAIoU,EAAK,IAAMnO,EAAEyT,EAAS1Z,QAClE,KAAIqU,EAAWG,EAAO7b,KAAK+gB,KAAatF,EAAOC,EAASrB,QAAQb,MACrExZ,EAAK0b,EAAUpO,EAAGmO,EAAKvY,MAAO2X,KAM7B,SAAShb,EAAQD,EAASH,GAG/B,GAAIsB,GAAYtB,EAAoB,IAChCuB,EAAYvB,EAAoB,GAChC8N,EAAY9N,EAAoB,IAAI,UACxCI,GAAOD,QAAU,SAASiD,EAAGsM,GAC3B,GAAiC1L,GAA7BwH,EAAIlK,EAAS8B,GAAG0C,WACpB,OAAO0F,KAAM1L,IAAckE,EAAI1C,EAASkK,GAAGsC,KAAahO,EAAY4P,EAAInO,EAAUyC,KAK/E,SAAS5D,EAAQD,EAASH,GAE/B,GAMI4hB,GAAMC,EAAMrC,EANZnV,EAAYrK,EAAoB,GAChC8hB,EAAY9hB,EAAoB,KAAKgQ,IACrC+R,EAAY1X,EAAO2X,kBAAoB3X,EAAO4X,uBAC9C9D,EAAY9T,EAAO8T,QACnB2C,EAAYzW,EAAOyW,QACnB1C,EAAgD,WAApCpe,EAAoB,IAAIme,GAGpC+D,EAAQ,WACV,GAAIC,GAAQC,EAAQ3b,CAKpB,KAJG2X,IAAW+D,EAAShE,EAAQiE,UAC7BjE,EAAQiE,OAAS,KACjBD,EAAOE,QAEHT,GACJQ,EAASR,EAAKQ,OACd3b,EAASmb,EAAKnb,GACX2b,GAAOA,EAAOE,QACjB7b,IACG2b,GAAOA,EAAOC,OACjBT,EAAOA,EAAKhH,IACZiH,GAAO/hB,EACNqiB,GAAOA,EAAOG,QAInB,IAAGlE,EACDoB,EAAS,WACPrB,EAAQoE,SAASL,QAGd,IAAGH,EAAS,CACjB,GAAIS,GAAS,EACTC,EAASxd,SAASyd,eAAe,GACrC,IAAIX,GAASG,GAAOS,QAAQF,GAAOG,eAAe,IAClDpD,EAAS,WACPiD,EAAKI,KAAOL,GAAUA,OAIxBhD,GADQsB,GAAWA,EAAQrC,QAClB,WACPqC,EAAQrC,UAAUI,KAAKqD,IAShB,WAEPJ,EAAUvhB,KAAK8J,EAAQ6X,GAI3B9hB,GAAOD,QAAU,QAAS8d,MAAKxX,GAC7B,GAAIqc,IAAQrc,GAAIA,EAAImU,KAAM9a,EAAWsiB,OAAQhE,GAAUD,EAAQiE,OAC5DP,KAAKA,EAAKjH,KAAOkI,GAChBlB,IACFA,EAAOkB,EACPtD,KACAqC,EAAOiB,IAKN,SAAS1iB,EAAQD,EAASH,GAE/B,GAYI+iB,GAAOC,EAASC,EAZhB1Y,EAAqBvK,EAAoB,GACzCoB,EAAqBpB,EAAoB,IACzCgB,EAAqBhB,EAAoB,IACzCiB,EAAqBjB,EAAoB,IACzCqK,EAAqBrK,EAAoB,GACzCme,EAAqB9T,EAAO8T,QAC5B+E,EAAqB7Y,EAAO8Y,aAC5BC,EAAqB/Y,EAAOgZ,eAC5BC,EAAqBjZ,EAAOiZ,eAC5BC,EAAqB,EACrBC,KACAC,EAAqB,qBAErB3D,EAAM;AACR,GAAIzf,IAAMqG,IACV,IAAG8c,EAAMhX,eAAenM,GAAI,CAC1B,GAAIoG,GAAK+c,EAAMnjB,SACRmjB,GAAMnjB,GACboG,MAGAid,EAAU,SAASC,GACrB7D,EAAIvf,KAAKojB,EAAMd,MAGbK,IAAYE,IACdF,EAAU,QAASC,cAAa1c,GAE9B,IADA,GAAIL,MAAWrC,EAAI,EACb6C,UAAU9C,OAASC,GAAEqC,EAAKV,KAAKkB,UAAU7C,KAK/C,OAJAyf,KAAQD,GAAW,WACjBniB,EAAoB,kBAANqF,GAAmBA,EAAKH,SAASG,GAAKL,IAEtD2c,EAAMQ,GACCA,GAETH,EAAY,QAASC,gBAAehjB,SAC3BmjB,GAAMnjB,IAGwB,WAApCL,EAAoB,IAAIme,GACzB4E,EAAQ,SAAS1iB,GACf8d,EAAQoE,SAAShY,EAAIuV,EAAKzf,EAAI,KAGxBijB,GACRN,EAAU,GAAIM,GACdL,EAAUD,EAAQY,MAClBZ,EAAQa,MAAMC,UAAYJ,EAC1BX,EAAQxY,EAAI0Y,EAAKc,YAAad,EAAM,IAG5B5Y,EAAO2Z,kBAA0C,kBAAfD,eAA8B1Z,EAAO4Z,eAC/ElB,EAAQ,SAAS1iB,GACfgK,EAAO0Z,YAAY1jB,EAAK,GAAI,MAE9BgK,EAAO2Z,iBAAiB,UAAWN,GAAS,IAG5CX,EADQU,IAAsBxiB,GAAI,UAC1B,SAASZ,GACfW,EAAK8D,YAAY7D,EAAI,WAAWwiB,GAAsB,WACpDziB,EAAKkjB,YAAYxd,MACjBoZ,EAAIvf,KAAKF,KAKL,SAASA,GACf8f,WAAW5V,EAAIuV,EAAKzf,EAAI,GAAI,KAIlCD,EAAOD,SACL6P,IAAOkT,EACPiB,MAAOf,IAKJ,SAAShjB,EAAQD,EAASH,GAE/B,GAAIsO,GAAWtO,EAAoB,GACnCI,GAAOD,QAAU,SAASoL,EAAQxG,GAChC,IAAI,GAAIS,KAAOT,GAAIuJ,EAAS/C,EAAQ/F,EAAKT,EAAIS,GAC7C,OAAO+F,KAKJ,SAASnL,EAAQD,EAASH,GAG/B,GAAIokB,GAASpkB,EAAoB,IAGjCA,GAAoB,KAAK,MAAO,SAASkD,GACvC,MAAO,SAASmhB,OAAO,MAAOnhB,GAAIwD,KAAME,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAG9EoD,IAAK,QAASA,KAAIsC,GAChB,GAAI8e,GAAQF,EAAOG,SAAS7d,KAAMlB,EAClC,OAAO8e,IAASA,EAAM1E,GAGxB5P,IAAK,QAASA,KAAIxK,EAAK/B,GACrB,MAAO2gB,GAAO/S,IAAI3K,KAAc,IAARlB,EAAY,EAAIA,EAAK/B,KAE9C2gB,GAAQ,IAIN,SAAShkB,EAAQD,EAASH,GAG/B,GAAIY,GAAeZ,EAAoB,GACnCia,EAAeja,EAAoB,IACnCwkB,EAAexkB,EAAoB,KACnCuK,EAAevK,EAAoB,GACnC4d,EAAe5d,EAAoB,KACnC2M,EAAe3M,EAAoB,IACnC6d,EAAe7d,EAAoB,KACnCykB,EAAezkB,EAAoB,KACnCgc,EAAehc,EAAoB,KACnC0kB,EAAe1kB,EAAoB,IAAI,MACvC2kB,EAAe3kB,EAAoB,IACnCwB,EAAexB,EAAoB,IACnC4kB,EAAe5kB,EAAoB,KACnCc,EAAed,EAAoB,GACnCsT,EAAenR,OAAOmR,cAAgB9R,EACtCqjB,EAAe/jB,EAAc,KAAO,OACpCT,EAAe,EAEfykB,EAAU,SAAS/Y,EAAI9F,GAEzB,IAAIzE,EAASuK,GAAI,MAAoB,gBAANA,GAAiBA,GAAmB,gBAANA,GAAiB,IAAM,KAAOA,CAC3F,KAAI4Y,EAAK5Y,EAAI2Y,GAAI,CAEf,IAAIpR,EAAavH,GAAI,MAAO,GAE5B,KAAI9F,EAAO,MAAO,GAElBgU,GAAKlO,EAAI2Y,IAAMrkB,GAEf,MAAO,IAAM0L,EAAG2Y,IAGhBH,EAAW,SAAS/d,EAAMhB,GAE5B,GAA0B8e,GAAtB1c,EAAQkd,EAAQtf,EACpB,IAAa,MAAVoC,EAAc,MAAOpB,GAAKqT,GAAGjS,EAEhC,KAAI0c,EAAQ9d,EAAKue,GAAIT,EAAOA,EAAQA,EAAMje,EACxC,GAAGie,EAAMxS,GAAKtM,EAAI,MAAO8e,GAI7BlkB,GAAOD,SACL6e,eAAgB,SAAS6B,EAAS7H,EAAM5L,EAAQ4X,GAC9C,GAAIxZ,GAAIqV,EAAQ,SAASra,EAAM8a,GAC7B1D,EAAUpX,EAAMgF,EAAGwN,GACnBxS,EAAKqT,GAAKjZ,EAAEqF,OAAO,MACnBO,EAAKue,GAAKjlB,EACV0G,EAAKye,GAAKnlB,EACV0G,EAAKqe,GAAQ,EACVvD,GAAYxhB,GAAU+d,EAAMyD,EAAUlU,EAAQ5G,EAAKwe,GAAQxe,IAqDhE,OAnDAge,GAAYhZ,EAAEpJ,WAGZ+hB,MAAO,QAASA,SACd,IAAI,GAAI3d,GAAOE,KAAMmc,EAAOrc,EAAKqT,GAAIyK,EAAQ9d,EAAKue,GAAIT,EAAOA,EAAQA,EAAMje,EACzEie,EAAM3D,GAAI,EACP2D,EAAM5jB,IAAE4jB,EAAM5jB,EAAI4jB,EAAM5jB,EAAE2F,EAAIvG,SAC1B+iB,GAAKyB,EAAMvgB,EAEpByC,GAAKue,GAAKve,EAAKye,GAAKnlB,EACpB0G,EAAKqe,GAAQ,GAIfK,SAAU,SAAS1f,GACjB,GAAIgB,GAAQE,KACR4d,EAAQC,EAAS/d,EAAMhB,EAC3B,IAAG8e,EAAM,CACP,GAAI1J,GAAO0J,EAAMje,EACb8e,EAAOb,EAAM5jB,QACV8F,GAAKqT,GAAGyK,EAAMvgB,GACrBugB,EAAM3D,GAAI,EACPwE,IAAKA,EAAK9e,EAAIuU,GACdA,IAAKA,EAAKla,EAAIykB,GACd3e,EAAKue,IAAMT,IAAM9d,EAAKue,GAAKnK,GAC3BpU,EAAKye,IAAMX,IAAM9d,EAAKye,GAAKE,GAC9B3e,EAAKqe,KACL,QAASP,GAIbtc,QAAS,QAASA,SAAQN,GAGxB,IAFA,GACI4c,GADAzW,EAAItD,EAAI7C,EAAYd,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,EAAW,GAEnEwkB,EAAQA,EAAQA,EAAMje,EAAIK,KAAKqe,IAGnC,IAFAlX,EAAEyW,EAAM1E,EAAG0E,EAAMxS,EAAGpL,MAEd4d,GAASA,EAAM3D,GAAE2D,EAAQA,EAAM5jB,GAKzCQ,IAAK,QAASA,KAAIsE,GAChB,QAAS+e,EAAS7d,KAAMlB,MAGzB1E,GAAYF,EAAEgC,QAAQ4I,EAAEpJ,UAAW,QACpCc,IAAK,WACH,MAAOyJ,GAAQjG,KAAKme,OAGjBrZ,GAET6F,IAAK,SAAS7K,EAAMhB,EAAK/B,GACvB,GACI0hB,GAAMvd,EADN0c,EAAQC,EAAS/d,EAAMhB,EAoBzB,OAjBC8e,GACDA,EAAM1E,EAAInc,GAGV+C,EAAKye,GAAKX,GACRvgB,EAAG6D,EAAQkd,EAAQtf,GAAK,GACxBsM,EAAGtM,EACHoa,EAAGnc,EACH/C,EAAGykB,EAAO3e,EAAKye,GACf5e,EAAGvG,EACH6gB,GAAG,GAEDna,EAAKue,KAAGve,EAAKue,GAAKT,GACnBa,IAAKA,EAAK9e,EAAIie,GACjB9d,EAAKqe,KAEQ,MAAVjd,IAAcpB,EAAKqT,GAAGjS,GAAS0c,IAC3B9d,GAEX+d,SAAUA,EACVa,UAAW,SAAS5Z,EAAGwN,EAAM5L,GAG3BqX,EAAYjZ,EAAGwN,EAAM,SAASW,EAAUuB,GACtCxU,KAAKkT,GAAKD,EACVjT,KAAKqJ,GAAKmL,EACVxU,KAAKue,GAAKnlB,GACT,WAKD,IAJA,GAAI0G,GAAQE,KACRwU,EAAQ1U,EAAKuJ,GACbuU,EAAQ9d,EAAKye,GAEXX,GAASA,EAAM3D,GAAE2D,EAAQA,EAAM5jB,CAErC,OAAI8F,GAAKoT,KAAQpT,EAAKye,GAAKX,EAAQA,EAAQA,EAAMje,EAAIG,EAAKoT,GAAGmL,IAMlD,QAAR7J,EAAwBc,EAAK,EAAGsI,EAAMxS,GAC9B,UAARoJ,EAAwBc,EAAK,EAAGsI,EAAM1E,GAClC5D,EAAK,GAAIsI,EAAMxS,EAAGwS,EAAM1E,KAN7BpZ,EAAKoT,GAAK9Z,EACHkc,EAAK,KAMb5O,EAAS,UAAY,UAAYA,GAAQ,GAG5CwX,EAAW5L,MAMV,SAAS5Y,EAAQD,EAASH,GAG/B,GAAIY,GAAiBZ,EAAoB,GACrCqK,EAAiBrK,EAAoB,GACrCa,EAAiBb,EAAoB,GACrCqB,EAAiBrB,EAAoB,GACrCia,EAAiBja,EAAoB,IACrCwkB,EAAiBxkB,EAAoB,KACrC6d,EAAiB7d,EAAoB,KACrC4d,EAAiB5d,EAAoB,KACrCwB,EAAiBxB,EAAoB,IACrCyO,EAAiBzO,EAAoB,IACrCc,EAAiBd,EAAoB,EAEzCI,GAAOD,QAAU,SAAS6Y,EAAM6H,EAAS7F,EAASqK,EAAQjY,EAAQkY,GAChE,GAAI5K,GAAQrQ,EAAO2O,GACfxN,EAAQkP,EACRsK,EAAQ5X,EAAS,MAAQ,MACzBiF,EAAQ7G,GAAKA,EAAEpJ,UACfgB,IAmCJ,OAlCItC,IAA2B,kBAAL0K,KAAqB8Z,GAAWjT,EAAMrK,UAAY3G,EAAM,YAChF,GAAImK,IAAI4P,UAAUR,WAMlBpP,EAAIqV,EAAQ,SAAStV,EAAQ+V,GAC3B1D,EAAUrS,EAAQC,EAAGwN,GACrBzN,EAAOga,GAAK,GAAI7K,GACb4G,GAAYxhB,GAAU+d,EAAMyD,EAAUlU,EAAQ7B,EAAOyZ,GAAQzZ,KAElE3K,EAAEqH,KAAK1H,KAAK,2DAA2D6D,MAAM,KAAK,SAASuO,GACzF,GAAI6S,GAAkB,OAAP7S,GAAuB,OAAPA,CAC5BA,KAAON,MAAWiT,GAAkB,SAAP3S,IAAgBsH,EAAKzO,EAAEpJ,UAAWuQ,EAAK,SAASxP,EAAG0I,GACjF,IAAI2Z,GAAYF,IAAY9jB,EAAS2B,GAAG,MAAc,OAAPwP,EAAe7S,GAAY,CAC1E,IAAI2F,GAASiB,KAAK6e,GAAG5S,GAAW,IAANxP,EAAU,EAAIA,EAAG0I,EAC3C,OAAO2Z,GAAW9e,KAAOjB,MAG1B,QAAU4M,IAAMzR,EAAEgC,QAAQ4I,EAAEpJ,UAAW,QACxCc,IAAK,WACH,MAAOwD,MAAK6e,GAAGpe,UAlBnBqE,EAAI6Z,EAAOrG,eAAe6B,EAAS7H,EAAM5L,EAAQ4X,GACjDR,EAAYhZ,EAAEpJ,UAAW4Y,IAsB3BvM,EAAejD,EAAGwN,GAElB5V,EAAE4V,GAAQxN,EACV3K,EAAQA,EAAQmK,EAAInK,EAAQyK,EAAIzK,EAAQoD,EAAGb,GAEvCkiB,GAAQD,EAAOD,UAAU5Z,EAAGwN,EAAM5L,GAE/B5B,IAKJ,SAASpL,EAAQD,EAASH,GAG/B,GAAIokB,GAASpkB,EAAoB,IAGjCA,GAAoB,KAAK,MAAO,SAASkD,GACvC,MAAO,SAASuiB,OAAO,MAAOviB,GAAIwD,KAAME,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAG9E4lB,IAAK,QAASA,KAAIjiB,GAChB,MAAO2gB,GAAO/S,IAAI3K,KAAMjD,EAAkB,IAAVA,EAAc,EAAIA,EAAOA,KAE1D2gB,IAIE,SAAShkB,EAAQD,EAASH,GAG/B,GAAIY,GAAeZ,EAAoB,GACnCsO,EAAetO,EAAoB,IACnC2lB,EAAe3lB,EAAoB,KACnCwB,EAAexB,EAAoB,IACnCkB,EAAelB,EAAoB,IACnC4lB,EAAeD,EAAKC,YACpBC,EAAeF,EAAKE,KACpBvS,EAAenR,OAAOmR,cAAgB9R,EACtCskB,KAGAC,EAAW/lB,EAAoB,KAAK,UAAW,SAASkD,GAC1D,MAAO,SAAS8iB,WAAW,MAAO9iB,GAAIwD,KAAME,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAGlFoD,IAAK,QAASA,KAAIsC,GAChB,GAAGhE,EAASgE,GAAK,CACf,IAAI8N,EAAa9N,GAAK,MAAOogB,GAAYlf,MAAMxD,IAAIsC,EACnD,IAAGtE,EAAIsE,EAAKqgB,GAAM,MAAOrgB,GAAIqgB,GAAMnf,KAAKmT,MAI5C7J,IAAK,QAASA,KAAIxK,EAAK/B,GACrB,MAAOkiB,GAAKtU,IAAI3K,KAAMlB,EAAK/B,KAE5BkiB,GAAM,GAAM,EAGsD,KAAlE,GAAII,IAAW/V,KAAK7N,OAAOuQ,QAAUvQ,QAAQ2jB,GAAM,GAAG5iB,IAAI4iB,IAC3DllB,EAAEqH,KAAK1H,MAAM,SAAU,MAAO,MAAO,OAAQ,SAASiF,GACpD,GAAI6M,GAAS0T,EAAS3jB,UAClB6jB,EAAS5T,EAAM7M,EACnB8I,GAAS+D,EAAO7M,EAAK,SAASrC,EAAG0I,GAE/B,GAAGrK,EAAS2B,KAAOmQ,EAAanQ,GAAG,CACjC,GAAIsC,GAASmgB,EAAYlf,MAAMlB,GAAKrC,EAAG0I,EACvC,OAAc,OAAPrG,EAAekB,KAAOjB,EAE7B,MAAOwgB,GAAO1lB,KAAKmG,KAAMvD,EAAG0I,QAO/B,SAASzL,EAAQD,EAASH,GAG/B,GAAIia,GAAoBja,EAAoB,IACxCwkB,EAAoBxkB,EAAoB,KACxCsB,EAAoBtB,EAAoB,IACxCwB,EAAoBxB,EAAoB,IACxC4d,EAAoB5d,EAAoB,KACxC6d,EAAoB7d,EAAoB,KACxCgC,EAAoBhC,EAAoB,IACxC2kB,EAAoB3kB,EAAoB,IACxC6lB,EAAoB7lB,EAAoB,IAAI,QAC5CsT,EAAoBnR,OAAOmR,cAAgB9R,EAC3C0kB,EAAoBlkB,EAAkB,GACtCmkB,EAAoBnkB,EAAkB,GACtC3B,EAAoB,EAGpBulB,EAAc,SAASpf,GACzB,MAAOA,GAAKye,KAAOze,EAAKye,GAAK,GAAImB,KAE/BA,EAAc,WAChB1f,KAAKvD,MAEHkjB,EAAa,SAASpY,EAAOzI,GAC/B,MAAO0gB,GAAUjY,EAAM9K,EAAG,SAAS4I,GACjC,MAAOA,GAAG,KAAOvG,IAGrB4gB,GAAYhkB,WACVc,IAAK,SAASsC,GACZ,GAAI8e,GAAQ+B,EAAW3f,KAAMlB,EAC7B,OAAG8e,GAAaA,EAAM,GAAtB,QAEFpjB,IAAK,SAASsE,GACZ,QAAS6gB,EAAW3f,KAAMlB,IAE5BwK,IAAK,SAASxK,EAAK/B,GACjB,GAAI6gB,GAAQ+B,EAAW3f,KAAMlB,EAC1B8e,GAAMA,EAAM,GAAK7gB,EACfiD,KAAKvD,EAAEuC,MAAMF,EAAK/B,KAEzByhB,SAAU,SAAS1f,GACjB,GAAIoC,GAAQue,EAAezf,KAAKvD,EAAG,SAAS4I,GAC1C,MAAOA,GAAG,KAAOvG,GAGnB,QADIoC,GAAMlB,KAAKvD,EAAEmjB,OAAO1e,EAAO,MACrBA,IAIdxH,EAAOD,SACL6e,eAAgB,SAAS6B,EAAS7H,EAAM5L,EAAQ4X,GAC9C,GAAIxZ,GAAIqV,EAAQ,SAASra,EAAM8a,GAC7B1D,EAAUpX,EAAMgF,EAAGwN,GACnBxS,EAAKqT,GAAKxZ,IACVmG,EAAKye,GAAKnlB,EACPwhB,GAAYxhB,GAAU+d,EAAMyD,EAAUlU,EAAQ5G,EAAKwe,GAAQxe,IAkBhE,OAhBAge,GAAYhZ,EAAEpJ,WAGZ8iB,SAAU,SAAS1f,GACjB,MAAIhE,GAASgE,GACT8N,EAAa9N,GACVmf,EAAKnf,EAAKqgB,IAASlB,EAAKnf,EAAIqgB,GAAOnf,KAAKmT,WAAcrU,GAAIqgB,GAAMnf,KAAKmT,IAD/C+L,EAAYlf,MAAM,UAAUlB,IADhC,GAM3BtE,IAAK,QAASA,KAAIsE,GAChB,MAAIhE,GAASgE,GACT8N,EAAa9N,GACVmf,EAAKnf,EAAKqgB,IAASlB,EAAKnf,EAAIqgB,GAAOnf,KAAKmT,IADlB+L,EAAYlf,MAAMxF,IAAIsE,IAD1B,KAKtBgG,GAET6F,IAAK,SAAS7K,EAAMhB,EAAK/B,GAMrB,MALE6P,GAAahS,EAASkE,KAGxBmf,EAAKnf,EAAKqgB,IAAS5L,EAAKzU,EAAKqgB,MAC7BrgB,EAAIqgB,GAAMrf,EAAKqT,IAAMpW,GAHrBmiB,EAAYpf,GAAMwJ,IAAIxK,EAAK/B,GAIpB+C,GAEXof,YAAaA,EACbC,KAAMA,IAKH,SAASzlB,EAAQD,EAASH,GAG/B,GAAI2lB,GAAO3lB,EAAoB,IAG/BA,GAAoB,KAAK,UAAW,SAASkD,GAC3C,MAAO,SAASqjB,WAAW,MAAOrjB,GAAIwD,KAAME,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAGlF4lB,IAAK,QAASA,KAAIjiB,GAChB,MAAOkiB,GAAKtU,IAAI3K,KAAMjD,GAAO,KAE9BkiB,GAAM,GAAO,IAIX,SAASvlB,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,GAC/BwmB,EAAWlgB,SAASwF,MACpBxK,EAAWtB,EAAoB,GAEnCa,GAAQA,EAAQmD,EAAG,WACjB8H,MAAO,QAASA,OAAMP,EAAQkb,EAAcC,GAC1C,MAAOF,GAAOjmB,KAAKgL,EAAQkb,EAAcnlB,EAASolB,QAMjD,SAAStmB,EAAQD,EAASH,GAG/B,GAAIY,GAAYZ,EAAoB,GAChCa,EAAYb,EAAoB,GAChCuB,EAAYvB,EAAoB,GAChCsB,EAAYtB,EAAoB,IAChCwB,EAAYxB,EAAoB,IAChCuG,EAAYD,SAASC,MAAQvG,EAAoB,GAAGsG,SAASlE,UAAUmE,IAI3E1F,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAIjE,EAAoB,GAAG,WACrD,QAASiE,MACT,QAAS0iB,QAAQzgB,UAAU,gBAAkBjC,YAAcA,MACzD,WACFiC,UAAW,QAASA,WAAU0gB,EAAQxgB,GACpC7E,EAAUqlB,GACVtlB,EAAS8E,EACT,IAAIygB,GAAYjgB,UAAU9C,OAAS,EAAI8iB,EAASrlB,EAAUqF,UAAU,GACpE,IAAGggB,GAAUC,EAAU,CAErB,OAAOzgB,EAAKtC,QACV,IAAK,GAAG,MAAO,IAAI8iB,EACnB,KAAK,GAAG,MAAO,IAAIA,GAAOxgB,EAAK,GAC/B,KAAK,GAAG,MAAO,IAAIwgB,GAAOxgB,EAAK,GAAIA,EAAK,GACxC,KAAK,GAAG,MAAO,IAAIwgB,GAAOxgB,EAAK,GAAIA,EAAK,GAAIA,EAAK,GACjD,KAAK,GAAG,MAAO,IAAIwgB,GAAOxgB,EAAK,GAAIA,EAAK,GAAIA,EAAK,GAAIA,EAAK,IAG5D,GAAI0gB,IAAS,KAEb,OADAA,GAAMphB,KAAKoG,MAAMgb,EAAO1gB,GACjB,IAAKG,EAAKuF,MAAM8a,EAAQE,IAGjC,GAAIzU,GAAWwU,EAAUzkB,UACrB2kB,EAAWnmB,EAAEqF,OAAOzE,EAAS6Q,GAASA,EAAQlQ,OAAOC,WACrDqD,EAAWa,SAASwF,MAAMvL,KAAKqmB,EAAQG,EAAU3gB,EACrD,OAAO5E,GAASiE,GAAUA,EAASshB,MAMlC,SAAS3mB,EAAQD,EAASH,GAG/B,GAAIY,GAAWZ,EAAoB,GAC/Ba,EAAWb,EAAoB,GAC/BsB,EAAWtB,EAAoB,GAGnCa,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAIjE,EAAoB,GAAG,WACrD2mB,QAAQhkB,eAAe/B,EAAEgC,WAAY,GAAIa,MAAO,IAAK,GAAIA,MAAO,MAC9D,WACFd,eAAgB,QAASA,gBAAe4I,EAAQyb,EAAaC,GAC3D3lB,EAASiK,EACT,KAEE,MADA3K,GAAEgC,QAAQ2I,EAAQyb,EAAaC,IACxB,EACP,MAAM1jB,GACN,OAAO,OAOR,SAASnD,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,GAC/B8C,EAAW9C,EAAoB,GAAG8C,QAClCxB,EAAWtB,EAAoB,GAEnCa,GAAQA,EAAQmD,EAAG,WACjBkjB,eAAgB,QAASA,gBAAe3b,EAAQyb,GAC9C,GAAIG,GAAOrkB,EAAQxB,EAASiK,GAASyb,EACrC,OAAOG,KAASA,EAAKhb,cAAe,QAAeZ,GAAOyb,OAMzD,SAAS5mB,EAAQD,EAASH,GAI/B,GAAIa,GAAWb,EAAoB,GAC/BsB,EAAWtB,EAAoB,IAC/BonB,EAAY,SAASzN,GACvBjT,KAAKkT,GAAKtY,EAASqY,GACnBjT,KAAKmT,GAAK,CACV,IACIrU,GADA5B,EAAO8C,KAAKqJ,KAEhB,KAAIvK,IAAOmU,GAAS/V,EAAK8B,KAAKF,GAEhCxF,GAAoB,KAAKonB,EAAW,SAAU,WAC5C,GAEI5hB,GAFAgB,EAAOE,KACP9C,EAAO4C,EAAKuJ,EAEhB,GACE,IAAGvJ,EAAKqT,IAAMjW,EAAKE,OAAO,OAAQL,MAAO3D,EAAWia,MAAM,YACjDvU,EAAM5B,EAAK4C,EAAKqT,QAAUrT,GAAKoT,IAC1C,QAAQnW,MAAO+B,EAAKuU,MAAM,KAG5BlZ,EAAQA,EAAQmD,EAAG,WACjBqjB,UAAW,QAASA,WAAU9b,GAC5B,MAAO,IAAI6b,GAAU7b,OAMpB,SAASnL,EAAQD,EAASH,GAS/B,QAASkD,KAAIqI,EAAQyb,GACnB,GACIG,GAAM9U,EADNiV,EAAW1gB,UAAU9C,OAAS,EAAIyH,EAAS3E,UAAU,EAEzD,OAAGtF,GAASiK,KAAY+b,EAAgB/b,EAAOyb,IAC5CG,EAAOvmB,EAAEkC,QAAQyI,EAAQyb,IAAoB9lB,EAAIimB,EAAM,SACtDA,EAAK1jB,MACL0jB,EAAKjkB,MAAQpD,EACXqnB,EAAKjkB,IAAI3C,KAAK+mB,GACdxnB,EACH0B,EAAS6Q,EAAQzR,EAAEiF,SAAS0F,IAAgBrI,IAAImP,EAAO2U,EAAaM,GAAvE,OAfF,GAAI1mB,GAAWZ,EAAoB,GAC/BkB,EAAWlB,EAAoB,IAC/Ba,EAAWb,EAAoB,GAC/BwB,EAAWxB,EAAoB,IAC/BsB,EAAWtB,EAAoB,GAcnCa,GAAQA,EAAQmD,EAAG,WAAYd,IAAKA,OAI/B,SAAS9C,EAAQD,EAASH,GAG/B,GAAIY,GAAWZ,EAAoB,GAC/Ba,EAAWb,EAAoB,GAC/BsB,EAAWtB,EAAoB,GAEnCa,GAAQA,EAAQmD,EAAG,WACjBE,yBAA0B,QAASA,0BAAyBqH,EAAQyb,GAClE,MAAOpmB,GAAEkC,QAAQxB,EAASiK,GAASyb,OAMlC,SAAS5mB,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,GAC/B6F,EAAW7F,EAAoB,GAAG6F,SAClCvE,EAAWtB,EAAoB,GAEnCa,GAAQA,EAAQmD,EAAG,WACjB4B,eAAgB,QAASA,gBAAe2F,GACtC,MAAO1F,GAASvE,EAASiK,QAMxB,SAASnL,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,WACjB9C,IAAK,QAASA,KAAIqK,EAAQyb,GACxB,MAAOA,KAAezb,OAMrB,SAASnL,EAAQD,EAASH,GAG/B,GAAIa,GAAgBb,EAAoB,GACpCsB,EAAgBtB,EAAoB,IACpCqT,EAAgBlR,OAAOmR,YAE3BzS,GAAQA,EAAQmD,EAAG,WACjBsP,aAAc,QAASA,cAAa/H,GAElC,MADAjK,GAASiK,GACF8H,EAAgBA,EAAc9H,IAAU,MAM9C,SAASnL,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,WAAYujB,QAASvnB,EAAoB,QAIvD,SAASI,EAAQD,EAASH,GAG/B,GAAIY,GAAWZ,EAAoB,GAC/BsB,EAAWtB,EAAoB,IAC/B2mB,EAAW3mB,EAAoB,GAAG2mB,OACtCvmB,GAAOD,QAAUwmB,GAAWA,EAAQY,SAAW,QAASA,SAAQxb,GAC9D,GAAInI,GAAahD,EAAEoF,SAAS1E,EAASyK,IACjC5B,EAAavJ,EAAEuJ,UACnB,OAAOA,GAAavG,EAAKU,OAAO6F,EAAW4B,IAAOnI,IAK/C,SAASxD,EAAQD,EAASH,GAG/B,GAAIa,GAAqBb,EAAoB,GACzCsB,EAAqBtB,EAAoB,IACzC+S,EAAqB5Q,OAAO6Q,iBAEhCnS,GAAQA,EAAQmD,EAAG,WACjBgP,kBAAmB,QAASA,mBAAkBzH,GAC5CjK,EAASiK,EACT,KAEE,MADGwH,IAAmBA,EAAmBxH,IAClC,EACP,MAAMhI,GACN,OAAO,OAOR,SAASnD,EAAQD,EAASH,GAU/B,QAASgQ,KAAIzE,EAAQyb,EAAaQ,GAChC,GAEIC,GAAoBpV,EAFpBiV,EAAW1gB,UAAU9C,OAAS,EAAIyH,EAAS3E,UAAU,GACrD8gB,EAAW9mB,EAAEkC,QAAQxB,EAASiK,GAASyb,EAE3C,KAAIU,EAAQ,CACV,GAAGlmB,EAAS6Q,EAAQzR,EAAEiF,SAAS0F,IAC7B,MAAOyE,KAAIqC,EAAO2U,EAAaQ,EAAGF,EAEpCI,GAAU3mB,EAAW,GAEvB,MAAGG,GAAIwmB,EAAS,SACXA,EAAQtb,YAAa,GAAU5K,EAAS8lB,IAC3CG,EAAqB7mB,EAAEkC,QAAQwkB,EAAUN,IAAgBjmB,EAAW,GACpE0mB,EAAmBhkB,MAAQ+jB,EAC3B5mB,EAAEgC,QAAQ0kB,EAAUN,EAAaS,IAC1B,IAJqD,EAMvDC,EAAQ1X,MAAQlQ,GAAY,GAAS4nB,EAAQ1X,IAAIzP,KAAK+mB,EAAUE,IAAI,GAxB7E,GAAI5mB,GAAaZ,EAAoB,GACjCkB,EAAalB,EAAoB,IACjCa,EAAab,EAAoB,GACjCe,EAAaf,EAAoB,IACjCsB,EAAatB,EAAoB,IACjCwB,EAAaxB,EAAoB,GAsBrCa,GAAQA,EAAQmD,EAAG,WAAYgM,IAAKA,OAI/B,SAAS5P,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,GAC/B8d,EAAW9d,EAAoB,GAEhC8d,IAASjd,EAAQA,EAAQmD,EAAG,WAC7BmO,eAAgB,QAASA,gBAAe5G,EAAQ8G,GAC9CyL,EAAS1L,MAAM7G,EAAQ8G,EACvB,KAEE,MADAyL,GAAS9N,IAAIzE,EAAQ8G,IACd,EACP,MAAM9O,GACN,OAAO,OAOR,SAASnD,EAAQD,EAASH,GAG/B,GAAIa,GAAYb,EAAoB,GAChC2nB,EAAY3nB,EAAoB,KAAI,EAExCa,GAAQA,EAAQwC,EAAG,SAEjB+V,SAAU,QAASA,UAAS1Q,GAC1B,MAAOif,GAAUjhB,KAAMgC,EAAI9B,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAIrEE,EAAoB,KAAK,aAIpB,SAASI,EAAQD,EAASH,GAI/B,GAAIa,GAAUb,EAAoB,GAC9BmY,EAAUnY,EAAoB,KAAI,EAEtCa,GAAQA,EAAQwC,EAAG,UACjBukB,GAAI,QAASA,IAAGvP,GACd,MAAOF,GAAIzR,KAAM2R,OAMhB,SAASjY,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B6nB,EAAU7nB,EAAoB,IAElCa,GAAQA,EAAQwC,EAAG,UACjBykB,QAAS,QAASA,SAAQC,GACxB,MAAOF,GAAKnhB,KAAMqhB,EAAWnhB,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,GAAW,OAM7E,SAASM,EAAQD,EAASH,GAG/B,GAAI6B,GAAW7B,EAAoB,IAC/BqZ,EAAWrZ,EAAoB,KAC/B2M,EAAW3M,EAAoB,GAEnCI,GAAOD,QAAU,SAASqG,EAAMuhB,EAAWC,EAAYC,GACrD,GAAIjkB,GAAeiT,OAAOtK,EAAQnG,IAC9B0hB,EAAelkB,EAAEF,OACjBqkB,EAAeH,IAAeloB,EAAY,IAAMmX,OAAO+Q,GACvDI,EAAevmB,EAASkmB,EAC5B,IAAmBG,GAAhBE,EAA6B,MAAOpkB,EACzB,KAAXmkB,IAAcA,EAAU,IAC3B,IAAIE,GAAUD,EAAeF,EACzBI,EAAejP,EAAO9Y,KAAK4nB,EAASvf,KAAKgE,KAAKyb,EAAUF,EAAQrkB,QAEpE,OADGwkB,GAAaxkB,OAASukB,IAAQC,EAAeA,EAAa9lB,MAAM,EAAG6lB,IAC/DJ,EAAOK,EAAetkB,EAAIA,EAAIskB,IAKlC,SAASloB,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B6nB,EAAU7nB,EAAoB,IAElCa,GAAQA,EAAQwC,EAAG,UACjBklB,SAAU,QAASA,UAASR,GAC1B,MAAOF,GAAKnhB,KAAMqhB,EAAWnhB,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,GAAW,OAM7E,SAASM,EAAQD,EAASH,GAI/BA,EAAoB,IAAI,WAAY,SAASwX,GAC3C,MAAO,SAASgR,YACd,MAAOhR,GAAM9Q,KAAM,OAMlB,SAAStG,EAAQD,EAASH,GAI/BA,EAAoB,IAAI,YAAa,SAASwX,GAC5C,MAAO,SAASiR,aACd,MAAOjR,GAAM9Q,KAAM,OAMlB,SAAStG,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B0oB,EAAU1oB,EAAoB,KAAK,sBAAuB,OAE9Da,GAAQA,EAAQmD,EAAG,UAAW2kB,OAAQ,QAASA,QAAO5c,GAAK,MAAO2c,GAAI3c,OAKjE,SAAS3L,EAAQD,GAEtBC,EAAOD,QAAU,SAASyoB,EAAQ1Q,GAChC,GAAItH,GAAWsH,IAAY/V,OAAO+V,GAAW,SAAS2Q,GACpD,MAAO3Q,GAAQ2Q,IACb3Q,CACJ,OAAO,UAASnM,GACd,MAAOkL,QAAOlL,GAAImM,QAAQ0Q,EAAQhY,MAMjC,SAASxQ,EAAQD,EAASH,GAG/B,GAAIY,GAAaZ,EAAoB,GACjCa,EAAab,EAAoB,GACjCunB,EAAavnB,EAAoB,KACjC0B,EAAa1B,EAAoB,IACjCe,EAAaf,EAAoB,GAErCa,GAAQA,EAAQmD,EAAG,UACjB8kB,0BAA2B,QAASA,2BAA0BvjB,GAQ5D,IAPA,GAMIC,GAAKkK,EANLtM,EAAU1B,EAAU6D,GACpB3C,EAAUhC,EAAEgC,QACZE,EAAUlC,EAAEkC,QACZc,EAAU2jB,EAAQnkB,GAClBqC,KACA1B,EAAU,EAERH,EAAKE,OAASC,GAClB2L,EAAI5M,EAAQM,EAAGoC,EAAM5B,EAAKG,MACvByB,IAAOC,GAAO7C,EAAQ6C,EAAQD,EAAKzE,EAAW,EAAG2O,IAC/CjK,EAAOD,GAAOkK,CACnB,OAAOjK,OAMR,SAASrF,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B+oB,EAAU/oB,EAAoB,MAAK,EAEvCa,GAAQA,EAAQmD,EAAG,UACjBmX,OAAQ,QAASA,QAAOpP,GACtB,MAAOgd,GAAQhd,OAMd,SAAS3L,EAAQD,EAASH,GAE/B,GAAIY,GAAYZ,EAAoB,GAChC0B,EAAY1B,EAAoB,IAChCkK,EAAYtJ,EAAEsJ,MAClB9J,GAAOD,QAAU,SAAS6oB,GACxB,MAAO,UAASjd,GAOd,IANA,GAKIvG,GALApC,EAAS1B,EAAUqK,GACnBnI,EAAShD,EAAEiD,QAAQT,GACnBU,EAASF,EAAKE,OACdC,EAAS,EACT0B,KAEE3B,EAASC,GAAKmG,EAAO3J,KAAK6C,EAAGoC,EAAM5B,EAAKG,OAC5C0B,EAAOC,KAAKsjB,GAAaxjB,EAAKpC,EAAEoC,IAAQpC,EAAEoC,GAC1C,OAAOC,MAMR,SAASrF,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,GAC/BipB,EAAWjpB,EAAoB,MAAK,EAExCa,GAAQA,EAAQmD,EAAG,UACjBoX,QAAS,QAASA,SAAQrP,GACxB,MAAOkd,GAASld,OAMf,SAAS3L,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,EAEnCa,GAAQA,EAAQwC,EAAG,OAAQ6lB,OAAQlpB,EAAoB,KAAK,UAIvD,SAASI,EAAQD,EAASH,GAG/B,GAAI6d,GAAU7d,EAAoB,KAC9Bsc,EAAUtc,EAAoB,IAClCI,GAAOD,QAAU,SAAS6Y,GACxB,MAAO,SAASkQ,UACd,GAAG5M,EAAQ5V,OAASsS,EAAK,KAAMxV,WAAUwV,EAAO,wBAChD,IAAI8D,KAEJ,OADAe,GAAMnX,MAAM,EAAOoW,EAAIpX,KAAMoX,GACtBA,KAMN,SAAS1c,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,EAEnCa,GAAQA,EAAQwC,EAAG,OAAQ6lB,OAAQlpB,EAAoB,KAAK,UAIvD,SAASI,EAAQD,EAASH,GAE/B,GAAIa,GAAUb,EAAoB,GAC9BmpB,EAAUnpB,EAAoB,IAClCa,GAAQA,EAAQmK,EAAInK,EAAQuK,GAC1B+X,aAAgBgG,EAAMnZ,IACtBqT,eAAgB8F,EAAMhF,SAKnB,SAAS/jB,EAAQD,EAASH,GAE/BA,EAAoB,IACpB,IAAIka,GAAYla,EAAoB,IACpCka,GAAUkP,SAAWlP,EAAUmP,eAAiBnP,EAAU5X,OAIrD,SAASlC,EAAQD,EAASH,GAG/B,GAAIqK,GAAarK,EAAoB,GACjCa,EAAab,EAAoB,GACjCoB,EAAapB,EAAoB,IACjCspB,EAAatpB,EAAoB,KACjCupB,EAAalf,EAAOkf,UACpBC,IAAeD,GAAa,WAAWjX,KAAKiX,EAAUE,WACtD7Z,EAAO,SAASI,GAClB,MAAOwZ,GAAO,SAAS/iB,EAAIijB,GACzB,MAAO1Z,GAAI5O,EACTkoB,KACG9mB,MAAMjC,KAAKqG,UAAW,GACZ,kBAANH,GAAmBA,EAAKH,SAASG,IACvCijB,IACD1Z,EAENnP,GAAQA,EAAQmK,EAAInK,EAAQuK,EAAIvK,EAAQoD,EAAIulB,GAC1CrJ,WAAavQ,EAAKvF,EAAO8V,YACzBwJ,YAAa/Z,EAAKvF,EAAOsf,gBAKtB,SAASvpB,EAAQD,EAASH,GAG/B,GAAI4pB,GAAY5pB,EAAoB,KAChCoB,EAAYpB,EAAoB,IAChCuB,EAAYvB,EAAoB,EACpCI,GAAOD,QAAU,WAOf,IANA,GAAIsG,GAASlF,EAAUmF,MACnB5C,EAAS8C,UAAU9C,OACnB+lB,EAASvnB,MAAMwB,GACfC,EAAS,EACT+lB,EAASF,EAAKE,EACdC,GAAS,EACPjmB,EAASC,IAAM8lB,EAAM9lB,GAAK6C,UAAU7C,QAAU+lB,IAAEC,GAAS,EAC/D,OAAO,YACL,GAGkB3jB,GAHdI,EAAQE,KACRoK,EAAQlK,UACRoL,EAAQlB,EAAGhN,OACXmO,EAAI,EAAGH,EAAI,CACf,KAAIiY,IAAW/X,EAAM,MAAO5Q,GAAOqF,EAAIojB,EAAOrjB,EAE9C,IADAJ,EAAOyjB,EAAMrnB,QACVunB,EAAO,KAAKjmB,EAASmO,EAAGA,IAAO7L,EAAK6L,KAAO6X,IAAE1jB,EAAK6L,GAAKnB,EAAGgB,KAC7D,MAAME,EAAQF,GAAE1L,EAAKV,KAAKoL,EAAGgB,KAC7B,OAAO1Q,GAAOqF,EAAIL,EAAMI,MAMvB,SAASpG,EAAQD,EAASH,GAE/BI,EAAOD,QAAUH,EAAoB,IAIhC,SAASI,EAAQD,EAASH,GAoF/B,QAASgqB,MAAK1I,GACZ,GAAI2I,GAAOrpB,EAAEqF,OAAO,KAQpB,OAPGqb,IAAYxhB,IACVoqB,EAAW5I,GACZzD,EAAMyD,GAAU,EAAM,SAAS9b,EAAK/B,GAClCwmB,EAAKzkB,GAAO/B,IAETkO,EAAOsY,EAAM3I,IAEf2I,EAIT,QAAS3hB,QAAO/C,EAAQ2W,EAAOiO,GAC7B5oB,EAAU2a,EACV,IAIIvU,GAAMnC,EAJNpC,EAAS1B,EAAU6D,GACnB3B,EAASC,EAAQT,GACjBU,EAASF,EAAKE,OACdC,EAAS,CAEb,IAAG6C,UAAU9C,OAAS,EAAE,CACtB,IAAIA,EAAO,KAAMN,WAAU,+CAC3BmE,GAAOvE,EAAEQ,EAAKG,UACT4D,GAAOxF,OAAOgoB,EACrB,MAAMrmB,EAASC,GAAK7C,EAAIkC,EAAGoC,EAAM5B,EAAKG,QACpC4D,EAAOuU,EAAMvU,EAAMvE,EAAEoC,GAAMA,EAAKD,GAElC,OAAOoC,GAGT,QAASyR,UAAS7T,EAAQmD,GACxB,OAAQA,GAAMA,EAAKiG,EAAMpJ,EAAQmD,GAAM0hB,EAAQ7kB,EAAQ,SAASwG,GAC9D,MAAOA,IAAMA,OACPjM,EAGV,QAASoD,KAAIqC,EAAQC,GACnB,MAAGtE,GAAIqE,EAAQC,GAAYD,EAAOC,GAAlC,OAEF,QAASwK,KAAIzK,EAAQC,EAAK/B,GAGxB,MAFG3C,IAAe0E,IAAOrD,QAAOvB,EAAEgC,QAAQ2C,EAAQC,EAAKzE,EAAW,EAAG0C,IAChE8B,EAAOC,GAAO/B,EACZ8B,EAGT,QAAS8kB,QAAOte,GACd,MAAOvK,GAASuK,IAAOnL,EAAEiF,SAASkG,KAAQie,KAAK5nB,UA/HjD,GAAIxB,GAAcZ,EAAoB,GAClCuK,EAAcvK,EAAoB,GAClCa,EAAcb,EAAoB,GAClCe,EAAcf,EAAoB,IAClC2R,EAAc3R,EAAoB,IAClC2O,EAAc3O,EAAoB,IAClCuB,EAAcvB,EAAoB,GAClC6d,EAAc7d,EAAoB,KAClCkqB,EAAclqB,EAAoB,KAClCma,EAAcna,EAAoB,KAClCgc,EAAchc,EAAoB,KAClCwB,EAAcxB,EAAoB,IAClC0B,EAAc1B,EAAoB,IAClCc,EAAcd,EAAoB,GAClCkB,EAAclB,EAAoB,IAClC6D,EAAcjD,EAAEiD,QAUhBymB,EAAmB,SAASnd,GAC9B,GAAIC,GAAmB,GAARD,EACXI,EAAmB,GAARJ,CACf,OAAO,UAAS5H,EAAQmC,EAAYlB,GAClC,GAIIhB,GAAKmI,EAAKC,EAJVC,EAAStD,EAAI7C,EAAYlB,EAAM,GAC/BpD,EAAS1B,EAAU6D,GACnBE,EAAS2H,GAAkB,GAARD,GAAqB,GAARA,EAC5B,IAAoB,kBAARzG,MAAqBA,KAAOsjB,MAAQlqB,CAExD,KAAI0F,IAAOpC,GAAE,GAAGlC,EAAIkC,EAAGoC,KACrBmI,EAAMvK,EAAEoC,GACRoI,EAAMC,EAAEF,EAAKnI,EAAKD,GACf4H,GACD,GAAGC,EAAO3H,EAAOD,GAAOoI,MACnB,IAAGA,EAAI,OAAOT,GACjB,IAAK,GAAG1H,EAAOD,GAAOmI,CAAK,MAC3B,KAAK,GAAG,OAAO,CACf,KAAK,GAAG,MAAOA,EACf,KAAK,GAAG,MAAOnI,EACf,KAAK,GAAGC,EAAOmI,EAAI,IAAMA,EAAI,OACxB,IAAGL,EAAS,OAAO,CAG9B,OAAe,IAARJ,GAAaI,EAAWA,EAAW9H,IAG1C2kB,EAAUE,EAAiB,GAE3BC,EAAiB,SAASrP,GAC5B,MAAO,UAASnP,GACd,MAAO,IAAIye,GAAaze,EAAImP,KAG5BsP,EAAe,SAAS7Q,EAAUuB,GACpCxU,KAAKkT,GAAKlY,EAAUiY,GACpBjT,KAAK+jB,GAAK5mB,EAAQ8V,GAClBjT,KAAKmT,GAAK,EACVnT,KAAKqJ,GAAKmL,EAEZf,GAAYqQ,EAAc,OAAQ,WAChC,GAIIhlB,GAJAgB,EAAOE,KACPtD,EAAOoD,EAAKoT,GACZhW,EAAO4C,EAAKikB,GACZvP,EAAO1U,EAAKuJ,EAEhB,GACE,IAAGvJ,EAAKqT,IAAMjW,EAAKE,OAEjB,MADA0C,GAAKoT,GAAK9Z,EACHkc,EAAK,UAEP9a,EAAIkC,EAAGoC,EAAM5B,EAAK4C,EAAKqT,OAChC,OAAW,QAARqB,EAAwBc,EAAK,EAAGxW,GACxB,UAAR0V,EAAwBc,EAAK,EAAG5Y,EAAEoC,IAC9BwW,EAAK,GAAIxW,EAAKpC,EAAEoC,OAczBwkB,KAAK5nB,UAAY,KAsCjBvB,EAAQA,EAAQmK,EAAInK,EAAQoD,GAAI+lB,KAAMA,OAEtCnpB,EAAQA,EAAQmD,EAAG,QACjBJ,KAAU2mB,EAAe,QACzBpP,OAAUoP,EAAe,UACzBnP,QAAUmP,EAAe,WACzBviB,QAAUsiB,EAAiB,GAC3BpiB,IAAUoiB,EAAiB,GAC3BniB,OAAUmiB,EAAiB,GAC3BliB,KAAUkiB,EAAiB,GAC3BjiB,MAAUiiB,EAAiB,GAC3B7M,KAAU6M,EAAiB,GAC3BF,QAAUA,EACVM,SAAUJ,EAAiB,GAC3BhiB,OAAUA,OACVqG,MAAUA,EACVyK,SAAUA,SACVlY,IAAUA,EACVgC,IAAUA,IACV8M,IAAUA,IACVqa,OAAUA,UAKP,SAASjqB,EAAQD,EAASH,GAE/B,GAAIsc,GAAYtc,EAAoB,KAChCoa,EAAYpa,EAAoB,IAAI,YACpCka,EAAYla,EAAoB,IACpCI,GAAOD,QAAUH,EAAoB,GAAGkqB,WAAa,SAASne,GAC5D,GAAI3I,GAAIjB,OAAO4J,EACf,OAAO3I,GAAEgX,KAActa,GAClB,cAAgBsD,IAChB8W,EAAU1N,eAAe8P,EAAQlZ,MAKnC,SAAShD,EAAQD,EAASH,GAE/B,GAAIsB,GAAWtB,EAAoB,IAC/BkD,EAAWlD,EAAoB,IACnCI,GAAOD,QAAUH,EAAoB,GAAG2qB,YAAc,SAAS5e,GAC7D,GAAIqQ,GAASlZ,EAAI6I,EACjB,IAAoB,kBAAVqQ,GAAqB,KAAM5Y,WAAUuI,EAAK,oBACpD,OAAOzK,GAAS8a,EAAO7b,KAAKwL,MAKzB,SAAS3L,EAAQD,EAASH,GAE/B,GAAIqK,GAAUrK,EAAoB,GAC9BsK,EAAUtK,EAAoB,GAC9Ba,EAAUb,EAAoB,GAC9BspB,EAAUtpB,EAAoB,IAElCa,GAAQA,EAAQmK,EAAInK,EAAQoD,GAC1B2mB,MAAO,QAASA,OAAMlB,GACpB,MAAO,KAAKpf,EAAKwW,SAAWzW,EAAOyW,SAAS,SAASrC,GACnD0B,WAAWmJ,EAAQ/oB,KAAKke,GAAS,GAAOiL,SAOzC,SAAStpB,EAAQD,EAASH,GAE/B,GAAI4pB,GAAU5pB,EAAoB,KAC9Ba,EAAUb,EAAoB,EAGlCA,GAAoB,GAAG8pB,EAAIF,EAAKE,EAAIF,EAAKE,MAEzCjpB,EAAQA,EAAQwC,EAAIxC,EAAQoD,EAAG,YAAa4kB,KAAM7oB,EAAoB,QAIjE,SAASI,EAAQD,EAASH,GAE/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAG,UAAWzC,SAAUxB,EAAoB,OAInE,SAASI,EAAQD,EAASH,GAE/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAG,UAAWqY,QAAStc,EAAoB,QAIlE,SAASI,EAAQD,EAASH,GAE/B,GAAIa,GAAUb,EAAoB,GAC9B6qB,EAAU7qB,EAAoB,IAElCa,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAG,UAAW4mB,OAAQA,KAI7C,SAASzqB,EAAQD,EAASH,GAE/B,GAAIY,GAAYZ,EAAoB,GAChCunB,EAAYvnB,EAAoB,KAChC0B,EAAY1B,EAAoB,GAEpCI,GAAOD,QAAU,QAAS0qB,QAAOtf,EAAQuf,GAIvC,IAHA,GAEWtlB,GAFP5B,EAAS2jB,EAAQ7lB,EAAUopB,IAC3BhnB,EAASF,EAAKE,OACdC,EAAI,EACFD,EAASC,GAAEnD,EAAEgC,QAAQ2I,EAAQ/F,EAAM5B,EAAKG,KAAMnD,EAAEkC,QAAQgoB,EAAOtlB,GACrE,OAAO+F,KAKJ,SAASnL,EAAQD,EAASH,GAE/B,GAAIa,GAAUb,EAAoB,GAC9B6qB,EAAU7qB,EAAoB,KAC9BiG,EAAUjG,EAAoB,GAAGiG,MAErCpF,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAG,UAC7B8mB,KAAM,SAAS1Y,EAAOyY,GACpB,MAAOD,GAAO5kB,EAAOoM,GAAQyY,OAM5B,SAAS1qB,EAAQD,EAASH,GAG/BA,EAAoB,KAAKyU,OAAQ,SAAU,SAASkF,GAClDjT,KAAKue,IAAMtL,EACXjT,KAAKmT,GAAK,GACT,WACD,GAAI9V,GAAO2C,KAAKmT,KACZE,IAAarT,KAAKue,GAATlhB,EACb,QAAQgW,KAAMA,EAAMtW,MAAOsW,EAAOja,EAAYiE,MAK3C,SAAS3D,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B0oB,EAAM1oB,EAAoB,KAAK,YACjCgrB,IAAK,QACLC,IAAK,OACLC,IAAK,OACLC,IAAK,SACLC,IAAK,UAGPvqB,GAAQA,EAAQwC,EAAIxC,EAAQoD,EAAG,UAAWonB,WAAY,QAASA,cAAc,MAAO3C,GAAIhiB,UAInF,SAAStG,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B0oB,EAAM1oB,EAAoB,KAAK,8BACjCsrB,QAAU,IACVC,OAAU,IACVC,OAAU,IACVC,SAAU,IACVC,SAAU,KAGZ7qB,GAAQA,EAAQwC,EAAIxC,EAAQoD,EAAG,UAAW0nB,aAAe,QAASA,gBAAgB,MAAOjD,GAAIhiB,UAIxF,SAAStG,EAAQD,EAASH,GAE/B,GAAIY,GAAUZ,EAAoB,GAC9BqK,EAAUrK,EAAoB,GAC9Ba,EAAUb,EAAoB,GAC9B2U,KACAiX,GAAU,CAEdhrB,GAAEqH,KAAK1H,KAAK,kNAIV6D,MAAM,KAAM,SAASoB,GACrBmP,EAAInP,GAAO,WACT,GAAIqmB,GAAWxhB,EAAO+V,OACtB,OAAGwL,IAAWC,GAAYA,EAASrmB,GAC1Bc,SAASwF,MAAMvL,KAAKsrB,EAASrmB,GAAMqmB,EAAUjlB,WADtD,UAKJ/F,EAAQA,EAAQmK,EAAInK,EAAQoD,GAAI0Q,IAAK3U,EAAoB,IAAI2U,EAAIA,IAAKA,GACpEmX,OAAQ,WACNF,GAAU,GAEZG,QAAS,WACPH,GAAU,QAMT,SAASxrB,EAAQD,EAASH,GAG/B,GAAIY,GAAUZ,EAAoB,GAC9Ba,EAAUb,EAAoB,GAC9BgsB,EAAUhsB,EAAoB,GAC9BisB,EAAUjsB,EAAoB,GAAGsC,OAASA,MAC1C4pB,KACAC,EAAa,SAASvoB,EAAME,GAC9BlD,EAAEqH,KAAK1H,KAAKqD,EAAKQ,MAAM,KAAM,SAASoB,GACjC1B,GAAUhE,GAAa0F,IAAOymB,GAAOC,EAAQ1mB,GAAOymB,EAAOzmB,GACtDA,SAAU0mB,EAAQ1mB,GAAOwmB,EAAK1lB,SAAS/F,QAASiF,GAAM1B,MAGlEqoB,GAAW,wCAAyC,GACpDA,EAAW,gEAAiE,GAC5EA,EAAW,6FAEXtrB,EAAQA,EAAQmD,EAAG,QAASkoB,MAKT,mBAAV9rB,SAAyBA,OAAOD,QAAQC,OAAOD,QAAUP,EAE1C,kBAAVirB,SAAwBA,OAAOuB,IAAIvB,OAAO,WAAW,MAAOjrB,KAEtEC,EAAIyK,KAAO1K,GACd,EAAG","file":"library.min.js"}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/shim.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/shim.js
new file mode 100644
index 00000000..7a24843d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/shim.js
@@ -0,0 +1,4551 @@
+/**
+ * core-js 1.2.7
+ * https://github.com/zloirock/core-js
+ * License: http://rock.mit-license.org
+ * © 2016 Denis Pushkarev
+ */
+!function(__e, __g, undefined){
+'use strict';
+/******/ (function(modules) { // webpackBootstrap
+/******/ // The module cache
+/******/ var installedModules = {};
+
+/******/ // The require function
+/******/ function __webpack_require__(moduleId) {
+
+/******/ // Check if module is in cache
+/******/ if(installedModules[moduleId])
+/******/ return installedModules[moduleId].exports;
+
+/******/ // Create a new module (and put it into the cache)
+/******/ var module = installedModules[moduleId] = {
+/******/ exports: {},
+/******/ id: moduleId,
+/******/ loaded: false
+/******/ };
+
+/******/ // Execute the module function
+/******/ modules[moduleId].call(module.exports, module, module.exports, __webpack_require__);
+
+/******/ // Flag the module as loaded
+/******/ module.loaded = true;
+
+/******/ // Return the exports of the module
+/******/ return module.exports;
+/******/ }
+
+
+/******/ // expose the modules object (__webpack_modules__)
+/******/ __webpack_require__.m = modules;
+
+/******/ // expose the module cache
+/******/ __webpack_require__.c = installedModules;
+
+/******/ // __webpack_public_path__
+/******/ __webpack_require__.p = "";
+
+/******/ // Load entry module and return exports
+/******/ return __webpack_require__(0);
+/******/ })
+/************************************************************************/
+/******/ ([
+/* 0 */
+/***/ function(module, exports, __webpack_require__) {
+
+ __webpack_require__(1);
+ __webpack_require__(34);
+ __webpack_require__(40);
+ __webpack_require__(42);
+ __webpack_require__(44);
+ __webpack_require__(46);
+ __webpack_require__(48);
+ __webpack_require__(50);
+ __webpack_require__(51);
+ __webpack_require__(52);
+ __webpack_require__(53);
+ __webpack_require__(54);
+ __webpack_require__(55);
+ __webpack_require__(56);
+ __webpack_require__(57);
+ __webpack_require__(58);
+ __webpack_require__(59);
+ __webpack_require__(60);
+ __webpack_require__(61);
+ __webpack_require__(64);
+ __webpack_require__(65);
+ __webpack_require__(66);
+ __webpack_require__(68);
+ __webpack_require__(69);
+ __webpack_require__(70);
+ __webpack_require__(71);
+ __webpack_require__(72);
+ __webpack_require__(73);
+ __webpack_require__(74);
+ __webpack_require__(76);
+ __webpack_require__(77);
+ __webpack_require__(78);
+ __webpack_require__(80);
+ __webpack_require__(81);
+ __webpack_require__(82);
+ __webpack_require__(84);
+ __webpack_require__(85);
+ __webpack_require__(86);
+ __webpack_require__(87);
+ __webpack_require__(88);
+ __webpack_require__(89);
+ __webpack_require__(90);
+ __webpack_require__(91);
+ __webpack_require__(92);
+ __webpack_require__(93);
+ __webpack_require__(94);
+ __webpack_require__(95);
+ __webpack_require__(96);
+ __webpack_require__(97);
+ __webpack_require__(99);
+ __webpack_require__(103);
+ __webpack_require__(104);
+ __webpack_require__(106);
+ __webpack_require__(107);
+ __webpack_require__(111);
+ __webpack_require__(116);
+ __webpack_require__(117);
+ __webpack_require__(120);
+ __webpack_require__(122);
+ __webpack_require__(124);
+ __webpack_require__(126);
+ __webpack_require__(127);
+ __webpack_require__(128);
+ __webpack_require__(130);
+ __webpack_require__(131);
+ __webpack_require__(133);
+ __webpack_require__(134);
+ __webpack_require__(135);
+ __webpack_require__(136);
+ __webpack_require__(143);
+ __webpack_require__(146);
+ __webpack_require__(147);
+ __webpack_require__(149);
+ __webpack_require__(150);
+ __webpack_require__(151);
+ __webpack_require__(152);
+ __webpack_require__(153);
+ __webpack_require__(154);
+ __webpack_require__(155);
+ __webpack_require__(156);
+ __webpack_require__(157);
+ __webpack_require__(158);
+ __webpack_require__(159);
+ __webpack_require__(160);
+ __webpack_require__(162);
+ __webpack_require__(163);
+ __webpack_require__(164);
+ __webpack_require__(165);
+ __webpack_require__(166);
+ __webpack_require__(167);
+ __webpack_require__(169);
+ __webpack_require__(170);
+ __webpack_require__(171);
+ __webpack_require__(172);
+ __webpack_require__(174);
+ __webpack_require__(175);
+ __webpack_require__(177);
+ __webpack_require__(178);
+ __webpack_require__(180);
+ __webpack_require__(181);
+ __webpack_require__(182);
+ __webpack_require__(183);
+ module.exports = __webpack_require__(186);
+
+
+/***/ },
+/* 1 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , $export = __webpack_require__(3)
+ , DESCRIPTORS = __webpack_require__(8)
+ , createDesc = __webpack_require__(7)
+ , html = __webpack_require__(14)
+ , cel = __webpack_require__(15)
+ , has = __webpack_require__(17)
+ , cof = __webpack_require__(18)
+ , invoke = __webpack_require__(19)
+ , fails = __webpack_require__(9)
+ , anObject = __webpack_require__(20)
+ , aFunction = __webpack_require__(13)
+ , isObject = __webpack_require__(16)
+ , toObject = __webpack_require__(21)
+ , toIObject = __webpack_require__(23)
+ , toInteger = __webpack_require__(25)
+ , toIndex = __webpack_require__(26)
+ , toLength = __webpack_require__(27)
+ , IObject = __webpack_require__(24)
+ , IE_PROTO = __webpack_require__(11)('__proto__')
+ , createArrayMethod = __webpack_require__(28)
+ , arrayIndexOf = __webpack_require__(33)(false)
+ , ObjectProto = Object.prototype
+ , ArrayProto = Array.prototype
+ , arraySlice = ArrayProto.slice
+ , arrayJoin = ArrayProto.join
+ , defineProperty = $.setDesc
+ , getOwnDescriptor = $.getDesc
+ , defineProperties = $.setDescs
+ , factories = {}
+ , IE8_DOM_DEFINE;
+
+ if(!DESCRIPTORS){
+ IE8_DOM_DEFINE = !fails(function(){
+ return defineProperty(cel('div'), 'a', {get: function(){ return 7; }}).a != 7;
+ });
+ $.setDesc = function(O, P, Attributes){
+ if(IE8_DOM_DEFINE)try {
+ return defineProperty(O, P, Attributes);
+ } catch(e){ /* empty */ }
+ if('get' in Attributes || 'set' in Attributes)throw TypeError('Accessors not supported!');
+ if('value' in Attributes)anObject(O)[P] = Attributes.value;
+ return O;
+ };
+ $.getDesc = function(O, P){
+ if(IE8_DOM_DEFINE)try {
+ return getOwnDescriptor(O, P);
+ } catch(e){ /* empty */ }
+ if(has(O, P))return createDesc(!ObjectProto.propertyIsEnumerable.call(O, P), O[P]);
+ };
+ $.setDescs = defineProperties = function(O, Properties){
+ anObject(O);
+ var keys = $.getKeys(Properties)
+ , length = keys.length
+ , i = 0
+ , P;
+ while(length > i)$.setDesc(O, P = keys[i++], Properties[P]);
+ return O;
+ };
+ }
+ $export($export.S + $export.F * !DESCRIPTORS, 'Object', {
+ // 19.1.2.6 / 15.2.3.3 Object.getOwnPropertyDescriptor(O, P)
+ getOwnPropertyDescriptor: $.getDesc,
+ // 19.1.2.4 / 15.2.3.6 Object.defineProperty(O, P, Attributes)
+ defineProperty: $.setDesc,
+ // 19.1.2.3 / 15.2.3.7 Object.defineProperties(O, Properties)
+ defineProperties: defineProperties
+ });
+
+ // IE 8- don't enum bug keys
+ var keys1 = ('constructor,hasOwnProperty,isPrototypeOf,propertyIsEnumerable,' +
+ 'toLocaleString,toString,valueOf').split(',')
+ // Additional keys for getOwnPropertyNames
+ , keys2 = keys1.concat('length', 'prototype')
+ , keysLen1 = keys1.length;
+
+ // Create object with `null` prototype: use iframe Object with cleared prototype
+ var createDict = function(){
+ // Thrash, waste and sodomy: IE GC bug
+ var iframe = cel('iframe')
+ , i = keysLen1
+ , gt = '>'
+ , iframeDocument;
+ iframe.style.display = 'none';
+ html.appendChild(iframe);
+ iframe.src = 'javascript:'; // eslint-disable-line no-script-url
+ // createDict = iframe.contentWindow.Object;
+ // html.removeChild(iframe);
+ iframeDocument = iframe.contentWindow.document;
+ iframeDocument.open();
+ iframeDocument.write(' i)if(has(O, key = names[i++])){
+ ~arrayIndexOf(result, key) || result.push(key);
+ }
+ return result;
+ };
+ };
+ var Empty = function(){};
+ $export($export.S, 'Object', {
+ // 19.1.2.9 / 15.2.3.2 Object.getPrototypeOf(O)
+ getPrototypeOf: $.getProto = $.getProto || function(O){
+ O = toObject(O);
+ if(has(O, IE_PROTO))return O[IE_PROTO];
+ if(typeof O.constructor == 'function' && O instanceof O.constructor){
+ return O.constructor.prototype;
+ } return O instanceof Object ? ObjectProto : null;
+ },
+ // 19.1.2.7 / 15.2.3.4 Object.getOwnPropertyNames(O)
+ getOwnPropertyNames: $.getNames = $.getNames || createGetKeys(keys2, keys2.length, true),
+ // 19.1.2.2 / 15.2.3.5 Object.create(O [, Properties])
+ create: $.create = $.create || function(O, /*?*/Properties){
+ var result;
+ if(O !== null){
+ Empty.prototype = anObject(O);
+ result = new Empty();
+ Empty.prototype = null;
+ // add "__proto__" for Object.getPrototypeOf shim
+ result[IE_PROTO] = O;
+ } else result = createDict();
+ return Properties === undefined ? result : defineProperties(result, Properties);
+ },
+ // 19.1.2.14 / 15.2.3.14 Object.keys(O)
+ keys: $.getKeys = $.getKeys || createGetKeys(keys1, keysLen1, false)
+ });
+
+ var construct = function(F, len, args){
+ if(!(len in factories)){
+ for(var n = [], i = 0; i < len; i++)n[i] = 'a[' + i + ']';
+ factories[len] = Function('F,a', 'return new F(' + n.join(',') + ')');
+ }
+ return factories[len](F, args);
+ };
+
+ // 19.2.3.2 / 15.3.4.5 Function.prototype.bind(thisArg, args...)
+ $export($export.P, 'Function', {
+ bind: function bind(that /*, args... */){
+ var fn = aFunction(this)
+ , partArgs = arraySlice.call(arguments, 1);
+ var bound = function(/* args... */){
+ var args = partArgs.concat(arraySlice.call(arguments));
+ return this instanceof bound ? construct(fn, args.length, args) : invoke(fn, args, that);
+ };
+ if(isObject(fn.prototype))bound.prototype = fn.prototype;
+ return bound;
+ }
+ });
+
+ // fallback for not array-like ES3 strings and DOM objects
+ $export($export.P + $export.F * fails(function(){
+ if(html)arraySlice.call(html);
+ }), 'Array', {
+ slice: function(begin, end){
+ var len = toLength(this.length)
+ , klass = cof(this);
+ end = end === undefined ? len : end;
+ if(klass == 'Array')return arraySlice.call(this, begin, end);
+ var start = toIndex(begin, len)
+ , upTo = toIndex(end, len)
+ , size = toLength(upTo - start)
+ , cloned = Array(size)
+ , i = 0;
+ for(; i < size; i++)cloned[i] = klass == 'String'
+ ? this.charAt(start + i)
+ : this[start + i];
+ return cloned;
+ }
+ });
+ $export($export.P + $export.F * (IObject != Object), 'Array', {
+ join: function join(separator){
+ return arrayJoin.call(IObject(this), separator === undefined ? ',' : separator);
+ }
+ });
+
+ // 22.1.2.2 / 15.4.3.2 Array.isArray(arg)
+ $export($export.S, 'Array', {isArray: __webpack_require__(30)});
+
+ var createArrayReduce = function(isRight){
+ return function(callbackfn, memo){
+ aFunction(callbackfn);
+ var O = IObject(this)
+ , length = toLength(O.length)
+ , index = isRight ? length - 1 : 0
+ , i = isRight ? -1 : 1;
+ if(arguments.length < 2)for(;;){
+ if(index in O){
+ memo = O[index];
+ index += i;
+ break;
+ }
+ index += i;
+ if(isRight ? index < 0 : length <= index){
+ throw TypeError('Reduce of empty array with no initial value');
+ }
+ }
+ for(;isRight ? index >= 0 : length > index; index += i)if(index in O){
+ memo = callbackfn(memo, O[index], index, this);
+ }
+ return memo;
+ };
+ };
+
+ var methodize = function($fn){
+ return function(arg1/*, arg2 = undefined */){
+ return $fn(this, arg1, arguments[1]);
+ };
+ };
+
+ $export($export.P, 'Array', {
+ // 22.1.3.10 / 15.4.4.18 Array.prototype.forEach(callbackfn [, thisArg])
+ forEach: $.each = $.each || methodize(createArrayMethod(0)),
+ // 22.1.3.15 / 15.4.4.19 Array.prototype.map(callbackfn [, thisArg])
+ map: methodize(createArrayMethod(1)),
+ // 22.1.3.7 / 15.4.4.20 Array.prototype.filter(callbackfn [, thisArg])
+ filter: methodize(createArrayMethod(2)),
+ // 22.1.3.23 / 15.4.4.17 Array.prototype.some(callbackfn [, thisArg])
+ some: methodize(createArrayMethod(3)),
+ // 22.1.3.5 / 15.4.4.16 Array.prototype.every(callbackfn [, thisArg])
+ every: methodize(createArrayMethod(4)),
+ // 22.1.3.18 / 15.4.4.21 Array.prototype.reduce(callbackfn [, initialValue])
+ reduce: createArrayReduce(false),
+ // 22.1.3.19 / 15.4.4.22 Array.prototype.reduceRight(callbackfn [, initialValue])
+ reduceRight: createArrayReduce(true),
+ // 22.1.3.11 / 15.4.4.14 Array.prototype.indexOf(searchElement [, fromIndex])
+ indexOf: methodize(arrayIndexOf),
+ // 22.1.3.14 / 15.4.4.15 Array.prototype.lastIndexOf(searchElement [, fromIndex])
+ lastIndexOf: function(el, fromIndex /* = @[*-1] */){
+ var O = toIObject(this)
+ , length = toLength(O.length)
+ , index = length - 1;
+ if(arguments.length > 1)index = Math.min(index, toInteger(fromIndex));
+ if(index < 0)index = toLength(length + index);
+ for(;index >= 0; index--)if(index in O)if(O[index] === el)return index;
+ return -1;
+ }
+ });
+
+ // 20.3.3.1 / 15.9.4.4 Date.now()
+ $export($export.S, 'Date', {now: function(){ return +new Date; }});
+
+ var lz = function(num){
+ return num > 9 ? num : '0' + num;
+ };
+
+ // 20.3.4.36 / 15.9.5.43 Date.prototype.toISOString()
+ // PhantomJS / old WebKit has a broken implementations
+ $export($export.P + $export.F * (fails(function(){
+ return new Date(-5e13 - 1).toISOString() != '0385-07-25T07:06:39.999Z';
+ }) || !fails(function(){
+ new Date(NaN).toISOString();
+ })), 'Date', {
+ toISOString: function toISOString(){
+ if(!isFinite(this))throw RangeError('Invalid time value');
+ var d = this
+ , y = d.getUTCFullYear()
+ , m = d.getUTCMilliseconds()
+ , s = y < 0 ? '-' : y > 9999 ? '+' : '';
+ return s + ('00000' + Math.abs(y)).slice(s ? -6 : -4) +
+ '-' + lz(d.getUTCMonth() + 1) + '-' + lz(d.getUTCDate()) +
+ 'T' + lz(d.getUTCHours()) + ':' + lz(d.getUTCMinutes()) +
+ ':' + lz(d.getUTCSeconds()) + '.' + (m > 99 ? m : '0' + lz(m)) + 'Z';
+ }
+ });
+
+/***/ },
+/* 2 */
+/***/ function(module, exports) {
+
+ var $Object = Object;
+ module.exports = {
+ create: $Object.create,
+ getProto: $Object.getPrototypeOf,
+ isEnum: {}.propertyIsEnumerable,
+ getDesc: $Object.getOwnPropertyDescriptor,
+ setDesc: $Object.defineProperty,
+ setDescs: $Object.defineProperties,
+ getKeys: $Object.keys,
+ getNames: $Object.getOwnPropertyNames,
+ getSymbols: $Object.getOwnPropertySymbols,
+ each: [].forEach
+ };
+
+/***/ },
+/* 3 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var global = __webpack_require__(4)
+ , core = __webpack_require__(5)
+ , hide = __webpack_require__(6)
+ , redefine = __webpack_require__(10)
+ , ctx = __webpack_require__(12)
+ , PROTOTYPE = 'prototype';
+
+ var $export = function(type, name, source){
+ var IS_FORCED = type & $export.F
+ , IS_GLOBAL = type & $export.G
+ , IS_STATIC = type & $export.S
+ , IS_PROTO = type & $export.P
+ , IS_BIND = type & $export.B
+ , target = IS_GLOBAL ? global : IS_STATIC ? global[name] || (global[name] = {}) : (global[name] || {})[PROTOTYPE]
+ , exports = IS_GLOBAL ? core : core[name] || (core[name] = {})
+ , expProto = exports[PROTOTYPE] || (exports[PROTOTYPE] = {})
+ , key, own, out, exp;
+ if(IS_GLOBAL)source = name;
+ for(key in source){
+ // contains in native
+ own = !IS_FORCED && target && key in target;
+ // export native or passed
+ out = (own ? target : source)[key];
+ // bind timers to global for call from export context
+ exp = IS_BIND && own ? ctx(out, global) : IS_PROTO && typeof out == 'function' ? ctx(Function.call, out) : out;
+ // extend global
+ if(target && !own)redefine(target, key, out);
+ // export
+ if(exports[key] != out)hide(exports, key, exp);
+ if(IS_PROTO && expProto[key] != out)expProto[key] = out;
+ }
+ };
+ global.core = core;
+ // type bitmap
+ $export.F = 1; // forced
+ $export.G = 2; // global
+ $export.S = 4; // static
+ $export.P = 8; // proto
+ $export.B = 16; // bind
+ $export.W = 32; // wrap
+ module.exports = $export;
+
+/***/ },
+/* 4 */
+/***/ function(module, exports) {
+
+ // https://github.com/zloirock/core-js/issues/86#issuecomment-115759028
+ var global = module.exports = typeof window != 'undefined' && window.Math == Math
+ ? window : typeof self != 'undefined' && self.Math == Math ? self : Function('return this')();
+ if(typeof __g == 'number')__g = global; // eslint-disable-line no-undef
+
+/***/ },
+/* 5 */
+/***/ function(module, exports) {
+
+ var core = module.exports = {version: '1.2.6'};
+ if(typeof __e == 'number')__e = core; // eslint-disable-line no-undef
+
+/***/ },
+/* 6 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $ = __webpack_require__(2)
+ , createDesc = __webpack_require__(7);
+ module.exports = __webpack_require__(8) ? function(object, key, value){
+ return $.setDesc(object, key, createDesc(1, value));
+ } : function(object, key, value){
+ object[key] = value;
+ return object;
+ };
+
+/***/ },
+/* 7 */
+/***/ function(module, exports) {
+
+ module.exports = function(bitmap, value){
+ return {
+ enumerable : !(bitmap & 1),
+ configurable: !(bitmap & 2),
+ writable : !(bitmap & 4),
+ value : value
+ };
+ };
+
+/***/ },
+/* 8 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // Thank's IE8 for his funny defineProperty
+ module.exports = !__webpack_require__(9)(function(){
+ return Object.defineProperty({}, 'a', {get: function(){ return 7; }}).a != 7;
+ });
+
+/***/ },
+/* 9 */
+/***/ function(module, exports) {
+
+ module.exports = function(exec){
+ try {
+ return !!exec();
+ } catch(e){
+ return true;
+ }
+ };
+
+/***/ },
+/* 10 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // add fake Function#toString
+ // for correct work wrapped methods / constructors with methods like LoDash isNative
+ var global = __webpack_require__(4)
+ , hide = __webpack_require__(6)
+ , SRC = __webpack_require__(11)('src')
+ , TO_STRING = 'toString'
+ , $toString = Function[TO_STRING]
+ , TPL = ('' + $toString).split(TO_STRING);
+
+ __webpack_require__(5).inspectSource = function(it){
+ return $toString.call(it);
+ };
+
+ (module.exports = function(O, key, val, safe){
+ if(typeof val == 'function'){
+ val.hasOwnProperty(SRC) || hide(val, SRC, O[key] ? '' + O[key] : TPL.join(String(key)));
+ val.hasOwnProperty('name') || hide(val, 'name', key);
+ }
+ if(O === global){
+ O[key] = val;
+ } else {
+ if(!safe)delete O[key];
+ hide(O, key, val);
+ }
+ })(Function.prototype, TO_STRING, function toString(){
+ return typeof this == 'function' && this[SRC] || $toString.call(this);
+ });
+
+/***/ },
+/* 11 */
+/***/ function(module, exports) {
+
+ var id = 0
+ , px = Math.random();
+ module.exports = function(key){
+ return 'Symbol('.concat(key === undefined ? '' : key, ')_', (++id + px).toString(36));
+ };
+
+/***/ },
+/* 12 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // optional / simple context binding
+ var aFunction = __webpack_require__(13);
+ module.exports = function(fn, that, length){
+ aFunction(fn);
+ if(that === undefined)return fn;
+ switch(length){
+ case 1: return function(a){
+ return fn.call(that, a);
+ };
+ case 2: return function(a, b){
+ return fn.call(that, a, b);
+ };
+ case 3: return function(a, b, c){
+ return fn.call(that, a, b, c);
+ };
+ }
+ return function(/* ...args */){
+ return fn.apply(that, arguments);
+ };
+ };
+
+/***/ },
+/* 13 */
+/***/ function(module, exports) {
+
+ module.exports = function(it){
+ if(typeof it != 'function')throw TypeError(it + ' is not a function!');
+ return it;
+ };
+
+/***/ },
+/* 14 */
+/***/ function(module, exports, __webpack_require__) {
+
+ module.exports = __webpack_require__(4).document && document.documentElement;
+
+/***/ },
+/* 15 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var isObject = __webpack_require__(16)
+ , document = __webpack_require__(4).document
+ // in old IE typeof document.createElement is 'object'
+ , is = isObject(document) && isObject(document.createElement);
+ module.exports = function(it){
+ return is ? document.createElement(it) : {};
+ };
+
+/***/ },
+/* 16 */
+/***/ function(module, exports) {
+
+ module.exports = function(it){
+ return typeof it === 'object' ? it !== null : typeof it === 'function';
+ };
+
+/***/ },
+/* 17 */
+/***/ function(module, exports) {
+
+ var hasOwnProperty = {}.hasOwnProperty;
+ module.exports = function(it, key){
+ return hasOwnProperty.call(it, key);
+ };
+
+/***/ },
+/* 18 */
+/***/ function(module, exports) {
+
+ var toString = {}.toString;
+
+ module.exports = function(it){
+ return toString.call(it).slice(8, -1);
+ };
+
+/***/ },
+/* 19 */
+/***/ function(module, exports) {
+
+ // fast apply, http://jsperf.lnkit.com/fast-apply/5
+ module.exports = function(fn, args, that){
+ var un = that === undefined;
+ switch(args.length){
+ case 0: return un ? fn()
+ : fn.call(that);
+ case 1: return un ? fn(args[0])
+ : fn.call(that, args[0]);
+ case 2: return un ? fn(args[0], args[1])
+ : fn.call(that, args[0], args[1]);
+ case 3: return un ? fn(args[0], args[1], args[2])
+ : fn.call(that, args[0], args[1], args[2]);
+ case 4: return un ? fn(args[0], args[1], args[2], args[3])
+ : fn.call(that, args[0], args[1], args[2], args[3]);
+ } return fn.apply(that, args);
+ };
+
+/***/ },
+/* 20 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var isObject = __webpack_require__(16);
+ module.exports = function(it){
+ if(!isObject(it))throw TypeError(it + ' is not an object!');
+ return it;
+ };
+
+/***/ },
+/* 21 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 7.1.13 ToObject(argument)
+ var defined = __webpack_require__(22);
+ module.exports = function(it){
+ return Object(defined(it));
+ };
+
+/***/ },
+/* 22 */
+/***/ function(module, exports) {
+
+ // 7.2.1 RequireObjectCoercible(argument)
+ module.exports = function(it){
+ if(it == undefined)throw TypeError("Can't call method on " + it);
+ return it;
+ };
+
+/***/ },
+/* 23 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // to indexed object, toObject with fallback for non-array-like ES3 strings
+ var IObject = __webpack_require__(24)
+ , defined = __webpack_require__(22);
+ module.exports = function(it){
+ return IObject(defined(it));
+ };
+
+/***/ },
+/* 24 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // fallback for non-array-like ES3 and non-enumerable old V8 strings
+ var cof = __webpack_require__(18);
+ module.exports = Object('z').propertyIsEnumerable(0) ? Object : function(it){
+ return cof(it) == 'String' ? it.split('') : Object(it);
+ };
+
+/***/ },
+/* 25 */
+/***/ function(module, exports) {
+
+ // 7.1.4 ToInteger
+ var ceil = Math.ceil
+ , floor = Math.floor;
+ module.exports = function(it){
+ return isNaN(it = +it) ? 0 : (it > 0 ? floor : ceil)(it);
+ };
+
+/***/ },
+/* 26 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var toInteger = __webpack_require__(25)
+ , max = Math.max
+ , min = Math.min;
+ module.exports = function(index, length){
+ index = toInteger(index);
+ return index < 0 ? max(index + length, 0) : min(index, length);
+ };
+
+/***/ },
+/* 27 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 7.1.15 ToLength
+ var toInteger = __webpack_require__(25)
+ , min = Math.min;
+ module.exports = function(it){
+ return it > 0 ? min(toInteger(it), 0x1fffffffffffff) : 0; // pow(2, 53) - 1 == 9007199254740991
+ };
+
+/***/ },
+/* 28 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 0 -> Array#forEach
+ // 1 -> Array#map
+ // 2 -> Array#filter
+ // 3 -> Array#some
+ // 4 -> Array#every
+ // 5 -> Array#find
+ // 6 -> Array#findIndex
+ var ctx = __webpack_require__(12)
+ , IObject = __webpack_require__(24)
+ , toObject = __webpack_require__(21)
+ , toLength = __webpack_require__(27)
+ , asc = __webpack_require__(29);
+ module.exports = function(TYPE){
+ var IS_MAP = TYPE == 1
+ , IS_FILTER = TYPE == 2
+ , IS_SOME = TYPE == 3
+ , IS_EVERY = TYPE == 4
+ , IS_FIND_INDEX = TYPE == 6
+ , NO_HOLES = TYPE == 5 || IS_FIND_INDEX;
+ return function($this, callbackfn, that){
+ var O = toObject($this)
+ , self = IObject(O)
+ , f = ctx(callbackfn, that, 3)
+ , length = toLength(self.length)
+ , index = 0
+ , result = IS_MAP ? asc($this, length) : IS_FILTER ? asc($this, 0) : undefined
+ , val, res;
+ for(;length > index; index++)if(NO_HOLES || index in self){
+ val = self[index];
+ res = f(val, index, O);
+ if(TYPE){
+ if(IS_MAP)result[index] = res; // map
+ else if(res)switch(TYPE){
+ case 3: return true; // some
+ case 5: return val; // find
+ case 6: return index; // findIndex
+ case 2: result.push(val); // filter
+ } else if(IS_EVERY)return false; // every
+ }
+ }
+ return IS_FIND_INDEX ? -1 : IS_SOME || IS_EVERY ? IS_EVERY : result;
+ };
+ };
+
+/***/ },
+/* 29 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 9.4.2.3 ArraySpeciesCreate(originalArray, length)
+ var isObject = __webpack_require__(16)
+ , isArray = __webpack_require__(30)
+ , SPECIES = __webpack_require__(31)('species');
+ module.exports = function(original, length){
+ var C;
+ if(isArray(original)){
+ C = original.constructor;
+ // cross-realm fallback
+ if(typeof C == 'function' && (C === Array || isArray(C.prototype)))C = undefined;
+ if(isObject(C)){
+ C = C[SPECIES];
+ if(C === null)C = undefined;
+ }
+ } return new (C === undefined ? Array : C)(length);
+ };
+
+/***/ },
+/* 30 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 7.2.2 IsArray(argument)
+ var cof = __webpack_require__(18);
+ module.exports = Array.isArray || function(arg){
+ return cof(arg) == 'Array';
+ };
+
+/***/ },
+/* 31 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var store = __webpack_require__(32)('wks')
+ , uid = __webpack_require__(11)
+ , Symbol = __webpack_require__(4).Symbol;
+ module.exports = function(name){
+ return store[name] || (store[name] =
+ Symbol && Symbol[name] || (Symbol || uid)('Symbol.' + name));
+ };
+
+/***/ },
+/* 32 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var global = __webpack_require__(4)
+ , SHARED = '__core-js_shared__'
+ , store = global[SHARED] || (global[SHARED] = {});
+ module.exports = function(key){
+ return store[key] || (store[key] = {});
+ };
+
+/***/ },
+/* 33 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // false -> Array#indexOf
+ // true -> Array#includes
+ var toIObject = __webpack_require__(23)
+ , toLength = __webpack_require__(27)
+ , toIndex = __webpack_require__(26);
+ module.exports = function(IS_INCLUDES){
+ return function($this, el, fromIndex){
+ var O = toIObject($this)
+ , length = toLength(O.length)
+ , index = toIndex(fromIndex, length)
+ , value;
+ // Array#includes uses SameValueZero equality algorithm
+ if(IS_INCLUDES && el != el)while(length > index){
+ value = O[index++];
+ if(value != value)return true;
+ // Array#toIndex ignores holes, Array#includes - not
+ } else for(;length > index; index++)if(IS_INCLUDES || index in O){
+ if(O[index] === el)return IS_INCLUDES || index;
+ } return !IS_INCLUDES && -1;
+ };
+ };
+
+/***/ },
+/* 34 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // ECMAScript 6 symbols shim
+ var $ = __webpack_require__(2)
+ , global = __webpack_require__(4)
+ , has = __webpack_require__(17)
+ , DESCRIPTORS = __webpack_require__(8)
+ , $export = __webpack_require__(3)
+ , redefine = __webpack_require__(10)
+ , $fails = __webpack_require__(9)
+ , shared = __webpack_require__(32)
+ , setToStringTag = __webpack_require__(35)
+ , uid = __webpack_require__(11)
+ , wks = __webpack_require__(31)
+ , keyOf = __webpack_require__(36)
+ , $names = __webpack_require__(37)
+ , enumKeys = __webpack_require__(38)
+ , isArray = __webpack_require__(30)
+ , anObject = __webpack_require__(20)
+ , toIObject = __webpack_require__(23)
+ , createDesc = __webpack_require__(7)
+ , getDesc = $.getDesc
+ , setDesc = $.setDesc
+ , _create = $.create
+ , getNames = $names.get
+ , $Symbol = global.Symbol
+ , $JSON = global.JSON
+ , _stringify = $JSON && $JSON.stringify
+ , setter = false
+ , HIDDEN = wks('_hidden')
+ , isEnum = $.isEnum
+ , SymbolRegistry = shared('symbol-registry')
+ , AllSymbols = shared('symbols')
+ , useNative = typeof $Symbol == 'function'
+ , ObjectProto = Object.prototype;
+
+ // fallback for old Android, https://code.google.com/p/v8/issues/detail?id=687
+ var setSymbolDesc = DESCRIPTORS && $fails(function(){
+ return _create(setDesc({}, 'a', {
+ get: function(){ return setDesc(this, 'a', {value: 7}).a; }
+ })).a != 7;
+ }) ? function(it, key, D){
+ var protoDesc = getDesc(ObjectProto, key);
+ if(protoDesc)delete ObjectProto[key];
+ setDesc(it, key, D);
+ if(protoDesc && it !== ObjectProto)setDesc(ObjectProto, key, protoDesc);
+ } : setDesc;
+
+ var wrap = function(tag){
+ var sym = AllSymbols[tag] = _create($Symbol.prototype);
+ sym._k = tag;
+ DESCRIPTORS && setter && setSymbolDesc(ObjectProto, tag, {
+ configurable: true,
+ set: function(value){
+ if(has(this, HIDDEN) && has(this[HIDDEN], tag))this[HIDDEN][tag] = false;
+ setSymbolDesc(this, tag, createDesc(1, value));
+ }
+ });
+ return sym;
+ };
+
+ var isSymbol = function(it){
+ return typeof it == 'symbol';
+ };
+
+ var $defineProperty = function defineProperty(it, key, D){
+ if(D && has(AllSymbols, key)){
+ if(!D.enumerable){
+ if(!has(it, HIDDEN))setDesc(it, HIDDEN, createDesc(1, {}));
+ it[HIDDEN][key] = true;
+ } else {
+ if(has(it, HIDDEN) && it[HIDDEN][key])it[HIDDEN][key] = false;
+ D = _create(D, {enumerable: createDesc(0, false)});
+ } return setSymbolDesc(it, key, D);
+ } return setDesc(it, key, D);
+ };
+ var $defineProperties = function defineProperties(it, P){
+ anObject(it);
+ var keys = enumKeys(P = toIObject(P))
+ , i = 0
+ , l = keys.length
+ , key;
+ while(l > i)$defineProperty(it, key = keys[i++], P[key]);
+ return it;
+ };
+ var $create = function create(it, P){
+ return P === undefined ? _create(it) : $defineProperties(_create(it), P);
+ };
+ var $propertyIsEnumerable = function propertyIsEnumerable(key){
+ var E = isEnum.call(this, key);
+ return E || !has(this, key) || !has(AllSymbols, key) || has(this, HIDDEN) && this[HIDDEN][key]
+ ? E : true;
+ };
+ var $getOwnPropertyDescriptor = function getOwnPropertyDescriptor(it, key){
+ var D = getDesc(it = toIObject(it), key);
+ if(D && has(AllSymbols, key) && !(has(it, HIDDEN) && it[HIDDEN][key]))D.enumerable = true;
+ return D;
+ };
+ var $getOwnPropertyNames = function getOwnPropertyNames(it){
+ var names = getNames(toIObject(it))
+ , result = []
+ , i = 0
+ , key;
+ while(names.length > i)if(!has(AllSymbols, key = names[i++]) && key != HIDDEN)result.push(key);
+ return result;
+ };
+ var $getOwnPropertySymbols = function getOwnPropertySymbols(it){
+ var names = getNames(toIObject(it))
+ , result = []
+ , i = 0
+ , key;
+ while(names.length > i)if(has(AllSymbols, key = names[i++]))result.push(AllSymbols[key]);
+ return result;
+ };
+ var $stringify = function stringify(it){
+ if(it === undefined || isSymbol(it))return; // IE8 returns string on undefined
+ var args = [it]
+ , i = 1
+ , $$ = arguments
+ , replacer, $replacer;
+ while($$.length > i)args.push($$[i++]);
+ replacer = args[1];
+ if(typeof replacer == 'function')$replacer = replacer;
+ if($replacer || !isArray(replacer))replacer = function(key, value){
+ if($replacer)value = $replacer.call(this, key, value);
+ if(!isSymbol(value))return value;
+ };
+ args[1] = replacer;
+ return _stringify.apply($JSON, args);
+ };
+ var buggyJSON = $fails(function(){
+ var S = $Symbol();
+ // MS Edge converts symbol values to JSON as {}
+ // WebKit converts symbol values to JSON as null
+ // V8 throws on boxed symbols
+ return _stringify([S]) != '[null]' || _stringify({a: S}) != '{}' || _stringify(Object(S)) != '{}';
+ });
+
+ // 19.4.1.1 Symbol([description])
+ if(!useNative){
+ $Symbol = function Symbol(){
+ if(isSymbol(this))throw TypeError('Symbol is not a constructor');
+ return wrap(uid(arguments.length > 0 ? arguments[0] : undefined));
+ };
+ redefine($Symbol.prototype, 'toString', function toString(){
+ return this._k;
+ });
+
+ isSymbol = function(it){
+ return it instanceof $Symbol;
+ };
+
+ $.create = $create;
+ $.isEnum = $propertyIsEnumerable;
+ $.getDesc = $getOwnPropertyDescriptor;
+ $.setDesc = $defineProperty;
+ $.setDescs = $defineProperties;
+ $.getNames = $names.get = $getOwnPropertyNames;
+ $.getSymbols = $getOwnPropertySymbols;
+
+ if(DESCRIPTORS && !__webpack_require__(39)){
+ redefine(ObjectProto, 'propertyIsEnumerable', $propertyIsEnumerable, true);
+ }
+ }
+
+ var symbolStatics = {
+ // 19.4.2.1 Symbol.for(key)
+ 'for': function(key){
+ return has(SymbolRegistry, key += '')
+ ? SymbolRegistry[key]
+ : SymbolRegistry[key] = $Symbol(key);
+ },
+ // 19.4.2.5 Symbol.keyFor(sym)
+ keyFor: function keyFor(key){
+ return keyOf(SymbolRegistry, key);
+ },
+ useSetter: function(){ setter = true; },
+ useSimple: function(){ setter = false; }
+ };
+ // 19.4.2.2 Symbol.hasInstance
+ // 19.4.2.3 Symbol.isConcatSpreadable
+ // 19.4.2.4 Symbol.iterator
+ // 19.4.2.6 Symbol.match
+ // 19.4.2.8 Symbol.replace
+ // 19.4.2.9 Symbol.search
+ // 19.4.2.10 Symbol.species
+ // 19.4.2.11 Symbol.split
+ // 19.4.2.12 Symbol.toPrimitive
+ // 19.4.2.13 Symbol.toStringTag
+ // 19.4.2.14 Symbol.unscopables
+ $.each.call((
+ 'hasInstance,isConcatSpreadable,iterator,match,replace,search,' +
+ 'species,split,toPrimitive,toStringTag,unscopables'
+ ).split(','), function(it){
+ var sym = wks(it);
+ symbolStatics[it] = useNative ? sym : wrap(sym);
+ });
+
+ setter = true;
+
+ $export($export.G + $export.W, {Symbol: $Symbol});
+
+ $export($export.S, 'Symbol', symbolStatics);
+
+ $export($export.S + $export.F * !useNative, 'Object', {
+ // 19.1.2.2 Object.create(O [, Properties])
+ create: $create,
+ // 19.1.2.4 Object.defineProperty(O, P, Attributes)
+ defineProperty: $defineProperty,
+ // 19.1.2.3 Object.defineProperties(O, Properties)
+ defineProperties: $defineProperties,
+ // 19.1.2.6 Object.getOwnPropertyDescriptor(O, P)
+ getOwnPropertyDescriptor: $getOwnPropertyDescriptor,
+ // 19.1.2.7 Object.getOwnPropertyNames(O)
+ getOwnPropertyNames: $getOwnPropertyNames,
+ // 19.1.2.8 Object.getOwnPropertySymbols(O)
+ getOwnPropertySymbols: $getOwnPropertySymbols
+ });
+
+ // 24.3.2 JSON.stringify(value [, replacer [, space]])
+ $JSON && $export($export.S + $export.F * (!useNative || buggyJSON), 'JSON', {stringify: $stringify});
+
+ // 19.4.3.5 Symbol.prototype[@@toStringTag]
+ setToStringTag($Symbol, 'Symbol');
+ // 20.2.1.9 Math[@@toStringTag]
+ setToStringTag(Math, 'Math', true);
+ // 24.3.3 JSON[@@toStringTag]
+ setToStringTag(global.JSON, 'JSON', true);
+
+/***/ },
+/* 35 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var def = __webpack_require__(2).setDesc
+ , has = __webpack_require__(17)
+ , TAG = __webpack_require__(31)('toStringTag');
+
+ module.exports = function(it, tag, stat){
+ if(it && !has(it = stat ? it : it.prototype, TAG))def(it, TAG, {configurable: true, value: tag});
+ };
+
+/***/ },
+/* 36 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $ = __webpack_require__(2)
+ , toIObject = __webpack_require__(23);
+ module.exports = function(object, el){
+ var O = toIObject(object)
+ , keys = $.getKeys(O)
+ , length = keys.length
+ , index = 0
+ , key;
+ while(length > index)if(O[key = keys[index++]] === el)return key;
+ };
+
+/***/ },
+/* 37 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // fallback for IE11 buggy Object.getOwnPropertyNames with iframe and window
+ var toIObject = __webpack_require__(23)
+ , getNames = __webpack_require__(2).getNames
+ , toString = {}.toString;
+
+ var windowNames = typeof window == 'object' && Object.getOwnPropertyNames
+ ? Object.getOwnPropertyNames(window) : [];
+
+ var getWindowNames = function(it){
+ try {
+ return getNames(it);
+ } catch(e){
+ return windowNames.slice();
+ }
+ };
+
+ module.exports.get = function getOwnPropertyNames(it){
+ if(windowNames && toString.call(it) == '[object Window]')return getWindowNames(it);
+ return getNames(toIObject(it));
+ };
+
+/***/ },
+/* 38 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // all enumerable object keys, includes symbols
+ var $ = __webpack_require__(2);
+ module.exports = function(it){
+ var keys = $.getKeys(it)
+ , getSymbols = $.getSymbols;
+ if(getSymbols){
+ var symbols = getSymbols(it)
+ , isEnum = $.isEnum
+ , i = 0
+ , key;
+ while(symbols.length > i)if(isEnum.call(it, key = symbols[i++]))keys.push(key);
+ }
+ return keys;
+ };
+
+/***/ },
+/* 39 */
+/***/ function(module, exports) {
+
+ module.exports = false;
+
+/***/ },
+/* 40 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.3.1 Object.assign(target, source)
+ var $export = __webpack_require__(3);
+
+ $export($export.S + $export.F, 'Object', {assign: __webpack_require__(41)});
+
+/***/ },
+/* 41 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.1 Object.assign(target, source, ...)
+ var $ = __webpack_require__(2)
+ , toObject = __webpack_require__(21)
+ , IObject = __webpack_require__(24);
+
+ // should work with symbols and should have deterministic property order (V8 bug)
+ module.exports = __webpack_require__(9)(function(){
+ var a = Object.assign
+ , A = {}
+ , B = {}
+ , S = Symbol()
+ , K = 'abcdefghijklmnopqrst';
+ A[S] = 7;
+ K.split('').forEach(function(k){ B[k] = k; });
+ return a({}, A)[S] != 7 || Object.keys(a({}, B)).join('') != K;
+ }) ? function assign(target, source){ // eslint-disable-line no-unused-vars
+ var T = toObject(target)
+ , $$ = arguments
+ , $$len = $$.length
+ , index = 1
+ , getKeys = $.getKeys
+ , getSymbols = $.getSymbols
+ , isEnum = $.isEnum;
+ while($$len > index){
+ var S = IObject($$[index++])
+ , keys = getSymbols ? getKeys(S).concat(getSymbols(S)) : getKeys(S)
+ , length = keys.length
+ , j = 0
+ , key;
+ while(length > j)if(isEnum.call(S, key = keys[j++]))T[key] = S[key];
+ }
+ return T;
+ } : Object.assign;
+
+/***/ },
+/* 42 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.3.10 Object.is(value1, value2)
+ var $export = __webpack_require__(3);
+ $export($export.S, 'Object', {is: __webpack_require__(43)});
+
+/***/ },
+/* 43 */
+/***/ function(module, exports) {
+
+ // 7.2.9 SameValue(x, y)
+ module.exports = Object.is || function is(x, y){
+ return x === y ? x !== 0 || 1 / x === 1 / y : x != x && y != y;
+ };
+
+/***/ },
+/* 44 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.3.19 Object.setPrototypeOf(O, proto)
+ var $export = __webpack_require__(3);
+ $export($export.S, 'Object', {setPrototypeOf: __webpack_require__(45).set});
+
+/***/ },
+/* 45 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // Works with __proto__ only. Old v8 can't work with null proto objects.
+ /* eslint-disable no-proto */
+ var getDesc = __webpack_require__(2).getDesc
+ , isObject = __webpack_require__(16)
+ , anObject = __webpack_require__(20);
+ var check = function(O, proto){
+ anObject(O);
+ if(!isObject(proto) && proto !== null)throw TypeError(proto + ": can't set as prototype!");
+ };
+ module.exports = {
+ set: Object.setPrototypeOf || ('__proto__' in {} ? // eslint-disable-line
+ function(test, buggy, set){
+ try {
+ set = __webpack_require__(12)(Function.call, getDesc(Object.prototype, '__proto__').set, 2);
+ set(test, []);
+ buggy = !(test instanceof Array);
+ } catch(e){ buggy = true; }
+ return function setPrototypeOf(O, proto){
+ check(O, proto);
+ if(buggy)O.__proto__ = proto;
+ else set(O, proto);
+ return O;
+ };
+ }({}, false) : undefined),
+ check: check
+ };
+
+/***/ },
+/* 46 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // 19.1.3.6 Object.prototype.toString()
+ var classof = __webpack_require__(47)
+ , test = {};
+ test[__webpack_require__(31)('toStringTag')] = 'z';
+ if(test + '' != '[object z]'){
+ __webpack_require__(10)(Object.prototype, 'toString', function toString(){
+ return '[object ' + classof(this) + ']';
+ }, true);
+ }
+
+/***/ },
+/* 47 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // getting tag from 19.1.3.6 Object.prototype.toString()
+ var cof = __webpack_require__(18)
+ , TAG = __webpack_require__(31)('toStringTag')
+ // ES3 wrong here
+ , ARG = cof(function(){ return arguments; }()) == 'Arguments';
+
+ module.exports = function(it){
+ var O, T, B;
+ return it === undefined ? 'Undefined' : it === null ? 'Null'
+ // @@toStringTag case
+ : typeof (T = (O = Object(it))[TAG]) == 'string' ? T
+ // builtinTag case
+ : ARG ? cof(O)
+ // ES3 arguments fallback
+ : (B = cof(O)) == 'Object' && typeof O.callee == 'function' ? 'Arguments' : B;
+ };
+
+/***/ },
+/* 48 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.5 Object.freeze(O)
+ var isObject = __webpack_require__(16);
+
+ __webpack_require__(49)('freeze', function($freeze){
+ return function freeze(it){
+ return $freeze && isObject(it) ? $freeze(it) : it;
+ };
+ });
+
+/***/ },
+/* 49 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // most Object methods by ES6 should accept primitives
+ var $export = __webpack_require__(3)
+ , core = __webpack_require__(5)
+ , fails = __webpack_require__(9);
+ module.exports = function(KEY, exec){
+ var fn = (core.Object || {})[KEY] || Object[KEY]
+ , exp = {};
+ exp[KEY] = exec(fn);
+ $export($export.S + $export.F * fails(function(){ fn(1); }), 'Object', exp);
+ };
+
+/***/ },
+/* 50 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.17 Object.seal(O)
+ var isObject = __webpack_require__(16);
+
+ __webpack_require__(49)('seal', function($seal){
+ return function seal(it){
+ return $seal && isObject(it) ? $seal(it) : it;
+ };
+ });
+
+/***/ },
+/* 51 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.15 Object.preventExtensions(O)
+ var isObject = __webpack_require__(16);
+
+ __webpack_require__(49)('preventExtensions', function($preventExtensions){
+ return function preventExtensions(it){
+ return $preventExtensions && isObject(it) ? $preventExtensions(it) : it;
+ };
+ });
+
+/***/ },
+/* 52 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.12 Object.isFrozen(O)
+ var isObject = __webpack_require__(16);
+
+ __webpack_require__(49)('isFrozen', function($isFrozen){
+ return function isFrozen(it){
+ return isObject(it) ? $isFrozen ? $isFrozen(it) : false : true;
+ };
+ });
+
+/***/ },
+/* 53 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.13 Object.isSealed(O)
+ var isObject = __webpack_require__(16);
+
+ __webpack_require__(49)('isSealed', function($isSealed){
+ return function isSealed(it){
+ return isObject(it) ? $isSealed ? $isSealed(it) : false : true;
+ };
+ });
+
+/***/ },
+/* 54 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.11 Object.isExtensible(O)
+ var isObject = __webpack_require__(16);
+
+ __webpack_require__(49)('isExtensible', function($isExtensible){
+ return function isExtensible(it){
+ return isObject(it) ? $isExtensible ? $isExtensible(it) : true : false;
+ };
+ });
+
+/***/ },
+/* 55 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.6 Object.getOwnPropertyDescriptor(O, P)
+ var toIObject = __webpack_require__(23);
+
+ __webpack_require__(49)('getOwnPropertyDescriptor', function($getOwnPropertyDescriptor){
+ return function getOwnPropertyDescriptor(it, key){
+ return $getOwnPropertyDescriptor(toIObject(it), key);
+ };
+ });
+
+/***/ },
+/* 56 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.9 Object.getPrototypeOf(O)
+ var toObject = __webpack_require__(21);
+
+ __webpack_require__(49)('getPrototypeOf', function($getPrototypeOf){
+ return function getPrototypeOf(it){
+ return $getPrototypeOf(toObject(it));
+ };
+ });
+
+/***/ },
+/* 57 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.14 Object.keys(O)
+ var toObject = __webpack_require__(21);
+
+ __webpack_require__(49)('keys', function($keys){
+ return function keys(it){
+ return $keys(toObject(it));
+ };
+ });
+
+/***/ },
+/* 58 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 19.1.2.7 Object.getOwnPropertyNames(O)
+ __webpack_require__(49)('getOwnPropertyNames', function(){
+ return __webpack_require__(37).get;
+ });
+
+/***/ },
+/* 59 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var setDesc = __webpack_require__(2).setDesc
+ , createDesc = __webpack_require__(7)
+ , has = __webpack_require__(17)
+ , FProto = Function.prototype
+ , nameRE = /^\s*function ([^ (]*)/
+ , NAME = 'name';
+ // 19.2.4.2 name
+ NAME in FProto || __webpack_require__(8) && setDesc(FProto, NAME, {
+ configurable: true,
+ get: function(){
+ var match = ('' + this).match(nameRE)
+ , name = match ? match[1] : '';
+ has(this, NAME) || setDesc(this, NAME, createDesc(5, name));
+ return name;
+ }
+ });
+
+/***/ },
+/* 60 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , isObject = __webpack_require__(16)
+ , HAS_INSTANCE = __webpack_require__(31)('hasInstance')
+ , FunctionProto = Function.prototype;
+ // 19.2.3.6 Function.prototype[@@hasInstance](V)
+ if(!(HAS_INSTANCE in FunctionProto))$.setDesc(FunctionProto, HAS_INSTANCE, {value: function(O){
+ if(typeof this != 'function' || !isObject(O))return false;
+ if(!isObject(this.prototype))return O instanceof this;
+ // for environment w/o native `@@hasInstance` logic enough `instanceof`, but add this:
+ while(O = $.getProto(O))if(this.prototype === O)return true;
+ return false;
+ }});
+
+/***/ },
+/* 61 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , global = __webpack_require__(4)
+ , has = __webpack_require__(17)
+ , cof = __webpack_require__(18)
+ , toPrimitive = __webpack_require__(62)
+ , fails = __webpack_require__(9)
+ , $trim = __webpack_require__(63).trim
+ , NUMBER = 'Number'
+ , $Number = global[NUMBER]
+ , Base = $Number
+ , proto = $Number.prototype
+ // Opera ~12 has broken Object#toString
+ , BROKEN_COF = cof($.create(proto)) == NUMBER
+ , TRIM = 'trim' in String.prototype;
+
+ // 7.1.3 ToNumber(argument)
+ var toNumber = function(argument){
+ var it = toPrimitive(argument, false);
+ if(typeof it == 'string' && it.length > 2){
+ it = TRIM ? it.trim() : $trim(it, 3);
+ var first = it.charCodeAt(0)
+ , third, radix, maxCode;
+ if(first === 43 || first === 45){
+ third = it.charCodeAt(2);
+ if(third === 88 || third === 120)return NaN; // Number('+0x1') should be NaN, old V8 fix
+ } else if(first === 48){
+ switch(it.charCodeAt(1)){
+ case 66 : case 98 : radix = 2; maxCode = 49; break; // fast equal /^0b[01]+$/i
+ case 79 : case 111 : radix = 8; maxCode = 55; break; // fast equal /^0o[0-7]+$/i
+ default : return +it;
+ }
+ for(var digits = it.slice(2), i = 0, l = digits.length, code; i < l; i++){
+ code = digits.charCodeAt(i);
+ // parseInt parses a string to a first unavailable symbol
+ // but ToNumber should return NaN if a string contains unavailable symbols
+ if(code < 48 || code > maxCode)return NaN;
+ } return parseInt(digits, radix);
+ }
+ } return +it;
+ };
+
+ if(!$Number(' 0o1') || !$Number('0b1') || $Number('+0x1')){
+ $Number = function Number(value){
+ var it = arguments.length < 1 ? 0 : value
+ , that = this;
+ return that instanceof $Number
+ // check on 1..constructor(foo) case
+ && (BROKEN_COF ? fails(function(){ proto.valueOf.call(that); }) : cof(that) != NUMBER)
+ ? new Base(toNumber(it)) : toNumber(it);
+ };
+ $.each.call(__webpack_require__(8) ? $.getNames(Base) : (
+ // ES3:
+ 'MAX_VALUE,MIN_VALUE,NaN,NEGATIVE_INFINITY,POSITIVE_INFINITY,' +
+ // ES6 (in case, if modules with ES6 Number statics required before):
+ 'EPSILON,isFinite,isInteger,isNaN,isSafeInteger,MAX_SAFE_INTEGER,' +
+ 'MIN_SAFE_INTEGER,parseFloat,parseInt,isInteger'
+ ).split(','), function(key){
+ if(has(Base, key) && !has($Number, key)){
+ $.setDesc($Number, key, $.getDesc(Base, key));
+ }
+ });
+ $Number.prototype = proto;
+ proto.constructor = $Number;
+ __webpack_require__(10)(global, NUMBER, $Number);
+ }
+
+/***/ },
+/* 62 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 7.1.1 ToPrimitive(input [, PreferredType])
+ var isObject = __webpack_require__(16);
+ // instead of the ES6 spec version, we didn't implement @@toPrimitive case
+ // and the second argument - flag - preferred type is a string
+ module.exports = function(it, S){
+ if(!isObject(it))return it;
+ var fn, val;
+ if(S && typeof (fn = it.toString) == 'function' && !isObject(val = fn.call(it)))return val;
+ if(typeof (fn = it.valueOf) == 'function' && !isObject(val = fn.call(it)))return val;
+ if(!S && typeof (fn = it.toString) == 'function' && !isObject(val = fn.call(it)))return val;
+ throw TypeError("Can't convert object to primitive value");
+ };
+
+/***/ },
+/* 63 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3)
+ , defined = __webpack_require__(22)
+ , fails = __webpack_require__(9)
+ , spaces = '\x09\x0A\x0B\x0C\x0D\x20\xA0\u1680\u180E\u2000\u2001\u2002\u2003' +
+ '\u2004\u2005\u2006\u2007\u2008\u2009\u200A\u202F\u205F\u3000\u2028\u2029\uFEFF'
+ , space = '[' + spaces + ']'
+ , non = '\u200b\u0085'
+ , ltrim = RegExp('^' + space + space + '*')
+ , rtrim = RegExp(space + space + '*$');
+
+ var exporter = function(KEY, exec){
+ var exp = {};
+ exp[KEY] = exec(trim);
+ $export($export.P + $export.F * fails(function(){
+ return !!spaces[KEY]() || non[KEY]() != non;
+ }), 'String', exp);
+ };
+
+ // 1 -> String#trimLeft
+ // 2 -> String#trimRight
+ // 3 -> String#trim
+ var trim = exporter.trim = function(string, TYPE){
+ string = String(defined(string));
+ if(TYPE & 1)string = string.replace(ltrim, '');
+ if(TYPE & 2)string = string.replace(rtrim, '');
+ return string;
+ };
+
+ module.exports = exporter;
+
+/***/ },
+/* 64 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.1 Number.EPSILON
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {EPSILON: Math.pow(2, -52)});
+
+/***/ },
+/* 65 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.2 Number.isFinite(number)
+ var $export = __webpack_require__(3)
+ , _isFinite = __webpack_require__(4).isFinite;
+
+ $export($export.S, 'Number', {
+ isFinite: function isFinite(it){
+ return typeof it == 'number' && _isFinite(it);
+ }
+ });
+
+/***/ },
+/* 66 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.3 Number.isInteger(number)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {isInteger: __webpack_require__(67)});
+
+/***/ },
+/* 67 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.3 Number.isInteger(number)
+ var isObject = __webpack_require__(16)
+ , floor = Math.floor;
+ module.exports = function isInteger(it){
+ return !isObject(it) && isFinite(it) && floor(it) === it;
+ };
+
+/***/ },
+/* 68 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.4 Number.isNaN(number)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {
+ isNaN: function isNaN(number){
+ return number != number;
+ }
+ });
+
+/***/ },
+/* 69 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.5 Number.isSafeInteger(number)
+ var $export = __webpack_require__(3)
+ , isInteger = __webpack_require__(67)
+ , abs = Math.abs;
+
+ $export($export.S, 'Number', {
+ isSafeInteger: function isSafeInteger(number){
+ return isInteger(number) && abs(number) <= 0x1fffffffffffff;
+ }
+ });
+
+/***/ },
+/* 70 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.6 Number.MAX_SAFE_INTEGER
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {MAX_SAFE_INTEGER: 0x1fffffffffffff});
+
+/***/ },
+/* 71 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.10 Number.MIN_SAFE_INTEGER
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {MIN_SAFE_INTEGER: -0x1fffffffffffff});
+
+/***/ },
+/* 72 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.12 Number.parseFloat(string)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {parseFloat: parseFloat});
+
+/***/ },
+/* 73 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.1.2.13 Number.parseInt(string, radix)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Number', {parseInt: parseInt});
+
+/***/ },
+/* 74 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.3 Math.acosh(x)
+ var $export = __webpack_require__(3)
+ , log1p = __webpack_require__(75)
+ , sqrt = Math.sqrt
+ , $acosh = Math.acosh;
+
+ // V8 bug https://code.google.com/p/v8/issues/detail?id=3509
+ $export($export.S + $export.F * !($acosh && Math.floor($acosh(Number.MAX_VALUE)) == 710), 'Math', {
+ acosh: function acosh(x){
+ return (x = +x) < 1 ? NaN : x > 94906265.62425156
+ ? Math.log(x) + Math.LN2
+ : log1p(x - 1 + sqrt(x - 1) * sqrt(x + 1));
+ }
+ });
+
+/***/ },
+/* 75 */
+/***/ function(module, exports) {
+
+ // 20.2.2.20 Math.log1p(x)
+ module.exports = Math.log1p || function log1p(x){
+ return (x = +x) > -1e-8 && x < 1e-8 ? x - x * x / 2 : Math.log(1 + x);
+ };
+
+/***/ },
+/* 76 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.5 Math.asinh(x)
+ var $export = __webpack_require__(3);
+
+ function asinh(x){
+ return !isFinite(x = +x) || x == 0 ? x : x < 0 ? -asinh(-x) : Math.log(x + Math.sqrt(x * x + 1));
+ }
+
+ $export($export.S, 'Math', {asinh: asinh});
+
+/***/ },
+/* 77 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.7 Math.atanh(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {
+ atanh: function atanh(x){
+ return (x = +x) == 0 ? x : Math.log((1 + x) / (1 - x)) / 2;
+ }
+ });
+
+/***/ },
+/* 78 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.9 Math.cbrt(x)
+ var $export = __webpack_require__(3)
+ , sign = __webpack_require__(79);
+
+ $export($export.S, 'Math', {
+ cbrt: function cbrt(x){
+ return sign(x = +x) * Math.pow(Math.abs(x), 1 / 3);
+ }
+ });
+
+/***/ },
+/* 79 */
+/***/ function(module, exports) {
+
+ // 20.2.2.28 Math.sign(x)
+ module.exports = Math.sign || function sign(x){
+ return (x = +x) == 0 || x != x ? x : x < 0 ? -1 : 1;
+ };
+
+/***/ },
+/* 80 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.11 Math.clz32(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {
+ clz32: function clz32(x){
+ return (x >>>= 0) ? 31 - Math.floor(Math.log(x + 0.5) * Math.LOG2E) : 32;
+ }
+ });
+
+/***/ },
+/* 81 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.12 Math.cosh(x)
+ var $export = __webpack_require__(3)
+ , exp = Math.exp;
+
+ $export($export.S, 'Math', {
+ cosh: function cosh(x){
+ return (exp(x = +x) + exp(-x)) / 2;
+ }
+ });
+
+/***/ },
+/* 82 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.14 Math.expm1(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {expm1: __webpack_require__(83)});
+
+/***/ },
+/* 83 */
+/***/ function(module, exports) {
+
+ // 20.2.2.14 Math.expm1(x)
+ module.exports = Math.expm1 || function expm1(x){
+ return (x = +x) == 0 ? x : x > -1e-6 && x < 1e-6 ? x + x * x / 2 : Math.exp(x) - 1;
+ };
+
+/***/ },
+/* 84 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.16 Math.fround(x)
+ var $export = __webpack_require__(3)
+ , sign = __webpack_require__(79)
+ , pow = Math.pow
+ , EPSILON = pow(2, -52)
+ , EPSILON32 = pow(2, -23)
+ , MAX32 = pow(2, 127) * (2 - EPSILON32)
+ , MIN32 = pow(2, -126);
+
+ var roundTiesToEven = function(n){
+ return n + 1 / EPSILON - 1 / EPSILON;
+ };
+
+
+ $export($export.S, 'Math', {
+ fround: function fround(x){
+ var $abs = Math.abs(x)
+ , $sign = sign(x)
+ , a, result;
+ if($abs < MIN32)return $sign * roundTiesToEven($abs / MIN32 / EPSILON32) * MIN32 * EPSILON32;
+ a = (1 + EPSILON32 / EPSILON) * $abs;
+ result = a - (a - $abs);
+ if(result > MAX32 || result != result)return $sign * Infinity;
+ return $sign * result;
+ }
+ });
+
+/***/ },
+/* 85 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.17 Math.hypot([value1[, value2[, … ]]])
+ var $export = __webpack_require__(3)
+ , abs = Math.abs;
+
+ $export($export.S, 'Math', {
+ hypot: function hypot(value1, value2){ // eslint-disable-line no-unused-vars
+ var sum = 0
+ , i = 0
+ , $$ = arguments
+ , $$len = $$.length
+ , larg = 0
+ , arg, div;
+ while(i < $$len){
+ arg = abs($$[i++]);
+ if(larg < arg){
+ div = larg / arg;
+ sum = sum * div * div + 1;
+ larg = arg;
+ } else if(arg > 0){
+ div = arg / larg;
+ sum += div * div;
+ } else sum += arg;
+ }
+ return larg === Infinity ? Infinity : larg * Math.sqrt(sum);
+ }
+ });
+
+/***/ },
+/* 86 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.18 Math.imul(x, y)
+ var $export = __webpack_require__(3)
+ , $imul = Math.imul;
+
+ // some WebKit versions fails with big numbers, some has wrong arity
+ $export($export.S + $export.F * __webpack_require__(9)(function(){
+ return $imul(0xffffffff, 5) != -5 || $imul.length != 2;
+ }), 'Math', {
+ imul: function imul(x, y){
+ var UINT16 = 0xffff
+ , xn = +x
+ , yn = +y
+ , xl = UINT16 & xn
+ , yl = UINT16 & yn;
+ return 0 | xl * yl + ((UINT16 & xn >>> 16) * yl + xl * (UINT16 & yn >>> 16) << 16 >>> 0);
+ }
+ });
+
+/***/ },
+/* 87 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.21 Math.log10(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {
+ log10: function log10(x){
+ return Math.log(x) / Math.LN10;
+ }
+ });
+
+/***/ },
+/* 88 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.20 Math.log1p(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {log1p: __webpack_require__(75)});
+
+/***/ },
+/* 89 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.22 Math.log2(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {
+ log2: function log2(x){
+ return Math.log(x) / Math.LN2;
+ }
+ });
+
+/***/ },
+/* 90 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.28 Math.sign(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {sign: __webpack_require__(79)});
+
+/***/ },
+/* 91 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.30 Math.sinh(x)
+ var $export = __webpack_require__(3)
+ , expm1 = __webpack_require__(83)
+ , exp = Math.exp;
+
+ // V8 near Chromium 38 has a problem with very small numbers
+ $export($export.S + $export.F * __webpack_require__(9)(function(){
+ return !Math.sinh(-2e-17) != -2e-17;
+ }), 'Math', {
+ sinh: function sinh(x){
+ return Math.abs(x = +x) < 1
+ ? (expm1(x) - expm1(-x)) / 2
+ : (exp(x - 1) - exp(-x - 1)) * (Math.E / 2);
+ }
+ });
+
+/***/ },
+/* 92 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.33 Math.tanh(x)
+ var $export = __webpack_require__(3)
+ , expm1 = __webpack_require__(83)
+ , exp = Math.exp;
+
+ $export($export.S, 'Math', {
+ tanh: function tanh(x){
+ var a = expm1(x = +x)
+ , b = expm1(-x);
+ return a == Infinity ? 1 : b == Infinity ? -1 : (a - b) / (exp(x) + exp(-x));
+ }
+ });
+
+/***/ },
+/* 93 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 20.2.2.34 Math.trunc(x)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Math', {
+ trunc: function trunc(it){
+ return (it > 0 ? Math.floor : Math.ceil)(it);
+ }
+ });
+
+/***/ },
+/* 94 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3)
+ , toIndex = __webpack_require__(26)
+ , fromCharCode = String.fromCharCode
+ , $fromCodePoint = String.fromCodePoint;
+
+ // length should be 1, old FF problem
+ $export($export.S + $export.F * (!!$fromCodePoint && $fromCodePoint.length != 1), 'String', {
+ // 21.1.2.2 String.fromCodePoint(...codePoints)
+ fromCodePoint: function fromCodePoint(x){ // eslint-disable-line no-unused-vars
+ var res = []
+ , $$ = arguments
+ , $$len = $$.length
+ , i = 0
+ , code;
+ while($$len > i){
+ code = +$$[i++];
+ if(toIndex(code, 0x10ffff) !== code)throw RangeError(code + ' is not a valid code point');
+ res.push(code < 0x10000
+ ? fromCharCode(code)
+ : fromCharCode(((code -= 0x10000) >> 10) + 0xd800, code % 0x400 + 0xdc00)
+ );
+ } return res.join('');
+ }
+ });
+
+/***/ },
+/* 95 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3)
+ , toIObject = __webpack_require__(23)
+ , toLength = __webpack_require__(27);
+
+ $export($export.S, 'String', {
+ // 21.1.2.4 String.raw(callSite, ...substitutions)
+ raw: function raw(callSite){
+ var tpl = toIObject(callSite.raw)
+ , len = toLength(tpl.length)
+ , $$ = arguments
+ , $$len = $$.length
+ , res = []
+ , i = 0;
+ while(len > i){
+ res.push(String(tpl[i++]));
+ if(i < $$len)res.push(String($$[i]));
+ } return res.join('');
+ }
+ });
+
+/***/ },
+/* 96 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // 21.1.3.25 String.prototype.trim()
+ __webpack_require__(63)('trim', function($trim){
+ return function trim(){
+ return $trim(this, 3);
+ };
+ });
+
+/***/ },
+/* 97 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , $at = __webpack_require__(98)(false);
+ $export($export.P, 'String', {
+ // 21.1.3.3 String.prototype.codePointAt(pos)
+ codePointAt: function codePointAt(pos){
+ return $at(this, pos);
+ }
+ });
+
+/***/ },
+/* 98 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var toInteger = __webpack_require__(25)
+ , defined = __webpack_require__(22);
+ // true -> String#at
+ // false -> String#codePointAt
+ module.exports = function(TO_STRING){
+ return function(that, pos){
+ var s = String(defined(that))
+ , i = toInteger(pos)
+ , l = s.length
+ , a, b;
+ if(i < 0 || i >= l)return TO_STRING ? '' : undefined;
+ a = s.charCodeAt(i);
+ return a < 0xd800 || a > 0xdbff || i + 1 === l || (b = s.charCodeAt(i + 1)) < 0xdc00 || b > 0xdfff
+ ? TO_STRING ? s.charAt(i) : a
+ : TO_STRING ? s.slice(i, i + 2) : (a - 0xd800 << 10) + (b - 0xdc00) + 0x10000;
+ };
+ };
+
+/***/ },
+/* 99 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 21.1.3.6 String.prototype.endsWith(searchString [, endPosition])
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , toLength = __webpack_require__(27)
+ , context = __webpack_require__(100)
+ , ENDS_WITH = 'endsWith'
+ , $endsWith = ''[ENDS_WITH];
+
+ $export($export.P + $export.F * __webpack_require__(102)(ENDS_WITH), 'String', {
+ endsWith: function endsWith(searchString /*, endPosition = @length */){
+ var that = context(this, searchString, ENDS_WITH)
+ , $$ = arguments
+ , endPosition = $$.length > 1 ? $$[1] : undefined
+ , len = toLength(that.length)
+ , end = endPosition === undefined ? len : Math.min(toLength(endPosition), len)
+ , search = String(searchString);
+ return $endsWith
+ ? $endsWith.call(that, search, end)
+ : that.slice(end - search.length, end) === search;
+ }
+ });
+
+/***/ },
+/* 100 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // helper for String#{startsWith, endsWith, includes}
+ var isRegExp = __webpack_require__(101)
+ , defined = __webpack_require__(22);
+
+ module.exports = function(that, searchString, NAME){
+ if(isRegExp(searchString))throw TypeError('String#' + NAME + " doesn't accept regex!");
+ return String(defined(that));
+ };
+
+/***/ },
+/* 101 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 7.2.8 IsRegExp(argument)
+ var isObject = __webpack_require__(16)
+ , cof = __webpack_require__(18)
+ , MATCH = __webpack_require__(31)('match');
+ module.exports = function(it){
+ var isRegExp;
+ return isObject(it) && ((isRegExp = it[MATCH]) !== undefined ? !!isRegExp : cof(it) == 'RegExp');
+ };
+
+/***/ },
+/* 102 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var MATCH = __webpack_require__(31)('match');
+ module.exports = function(KEY){
+ var re = /./;
+ try {
+ '/./'[KEY](re);
+ } catch(e){
+ try {
+ re[MATCH] = false;
+ return !'/./'[KEY](re);
+ } catch(f){ /* empty */ }
+ } return true;
+ };
+
+/***/ },
+/* 103 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 21.1.3.7 String.prototype.includes(searchString, position = 0)
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , context = __webpack_require__(100)
+ , INCLUDES = 'includes';
+
+ $export($export.P + $export.F * __webpack_require__(102)(INCLUDES), 'String', {
+ includes: function includes(searchString /*, position = 0 */){
+ return !!~context(this, searchString, INCLUDES)
+ .indexOf(searchString, arguments.length > 1 ? arguments[1] : undefined);
+ }
+ });
+
+/***/ },
+/* 104 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3);
+
+ $export($export.P, 'String', {
+ // 21.1.3.13 String.prototype.repeat(count)
+ repeat: __webpack_require__(105)
+ });
+
+/***/ },
+/* 105 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var toInteger = __webpack_require__(25)
+ , defined = __webpack_require__(22);
+
+ module.exports = function repeat(count){
+ var str = String(defined(this))
+ , res = ''
+ , n = toInteger(count);
+ if(n < 0 || n == Infinity)throw RangeError("Count can't be negative");
+ for(;n > 0; (n >>>= 1) && (str += str))if(n & 1)res += str;
+ return res;
+ };
+
+/***/ },
+/* 106 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 21.1.3.18 String.prototype.startsWith(searchString [, position ])
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , toLength = __webpack_require__(27)
+ , context = __webpack_require__(100)
+ , STARTS_WITH = 'startsWith'
+ , $startsWith = ''[STARTS_WITH];
+
+ $export($export.P + $export.F * __webpack_require__(102)(STARTS_WITH), 'String', {
+ startsWith: function startsWith(searchString /*, position = 0 */){
+ var that = context(this, searchString, STARTS_WITH)
+ , $$ = arguments
+ , index = toLength(Math.min($$.length > 1 ? $$[1] : undefined, that.length))
+ , search = String(searchString);
+ return $startsWith
+ ? $startsWith.call(that, search, index)
+ : that.slice(index, index + search.length) === search;
+ }
+ });
+
+/***/ },
+/* 107 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $at = __webpack_require__(98)(true);
+
+ // 21.1.3.27 String.prototype[@@iterator]()
+ __webpack_require__(108)(String, 'String', function(iterated){
+ this._t = String(iterated); // target
+ this._i = 0; // next index
+ // 21.1.5.2.1 %StringIteratorPrototype%.next()
+ }, function(){
+ var O = this._t
+ , index = this._i
+ , point;
+ if(index >= O.length)return {value: undefined, done: true};
+ point = $at(O, index);
+ this._i += point.length;
+ return {value: point, done: false};
+ });
+
+/***/ },
+/* 108 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var LIBRARY = __webpack_require__(39)
+ , $export = __webpack_require__(3)
+ , redefine = __webpack_require__(10)
+ , hide = __webpack_require__(6)
+ , has = __webpack_require__(17)
+ , Iterators = __webpack_require__(109)
+ , $iterCreate = __webpack_require__(110)
+ , setToStringTag = __webpack_require__(35)
+ , getProto = __webpack_require__(2).getProto
+ , ITERATOR = __webpack_require__(31)('iterator')
+ , BUGGY = !([].keys && 'next' in [].keys()) // Safari has buggy iterators w/o `next`
+ , FF_ITERATOR = '@@iterator'
+ , KEYS = 'keys'
+ , VALUES = 'values';
+
+ var returnThis = function(){ return this; };
+
+ module.exports = function(Base, NAME, Constructor, next, DEFAULT, IS_SET, FORCED){
+ $iterCreate(Constructor, NAME, next);
+ var getMethod = function(kind){
+ if(!BUGGY && kind in proto)return proto[kind];
+ switch(kind){
+ case KEYS: return function keys(){ return new Constructor(this, kind); };
+ case VALUES: return function values(){ return new Constructor(this, kind); };
+ } return function entries(){ return new Constructor(this, kind); };
+ };
+ var TAG = NAME + ' Iterator'
+ , DEF_VALUES = DEFAULT == VALUES
+ , VALUES_BUG = false
+ , proto = Base.prototype
+ , $native = proto[ITERATOR] || proto[FF_ITERATOR] || DEFAULT && proto[DEFAULT]
+ , $default = $native || getMethod(DEFAULT)
+ , methods, key;
+ // Fix native
+ if($native){
+ var IteratorPrototype = getProto($default.call(new Base));
+ // Set @@toStringTag to native iterators
+ setToStringTag(IteratorPrototype, TAG, true);
+ // FF fix
+ if(!LIBRARY && has(proto, FF_ITERATOR))hide(IteratorPrototype, ITERATOR, returnThis);
+ // fix Array#{values, @@iterator}.name in V8 / FF
+ if(DEF_VALUES && $native.name !== VALUES){
+ VALUES_BUG = true;
+ $default = function values(){ return $native.call(this); };
+ }
+ }
+ // Define iterator
+ if((!LIBRARY || FORCED) && (BUGGY || VALUES_BUG || !proto[ITERATOR])){
+ hide(proto, ITERATOR, $default);
+ }
+ // Plug for library
+ Iterators[NAME] = $default;
+ Iterators[TAG] = returnThis;
+ if(DEFAULT){
+ methods = {
+ values: DEF_VALUES ? $default : getMethod(VALUES),
+ keys: IS_SET ? $default : getMethod(KEYS),
+ entries: !DEF_VALUES ? $default : getMethod('entries')
+ };
+ if(FORCED)for(key in methods){
+ if(!(key in proto))redefine(proto, key, methods[key]);
+ } else $export($export.P + $export.F * (BUGGY || VALUES_BUG), NAME, methods);
+ }
+ return methods;
+ };
+
+/***/ },
+/* 109 */
+/***/ function(module, exports) {
+
+ module.exports = {};
+
+/***/ },
+/* 110 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , descriptor = __webpack_require__(7)
+ , setToStringTag = __webpack_require__(35)
+ , IteratorPrototype = {};
+
+ // 25.1.2.1.1 %IteratorPrototype%[@@iterator]()
+ __webpack_require__(6)(IteratorPrototype, __webpack_require__(31)('iterator'), function(){ return this; });
+
+ module.exports = function(Constructor, NAME, next){
+ Constructor.prototype = $.create(IteratorPrototype, {next: descriptor(1, next)});
+ setToStringTag(Constructor, NAME + ' Iterator');
+ };
+
+/***/ },
+/* 111 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var ctx = __webpack_require__(12)
+ , $export = __webpack_require__(3)
+ , toObject = __webpack_require__(21)
+ , call = __webpack_require__(112)
+ , isArrayIter = __webpack_require__(113)
+ , toLength = __webpack_require__(27)
+ , getIterFn = __webpack_require__(114);
+ $export($export.S + $export.F * !__webpack_require__(115)(function(iter){ Array.from(iter); }), 'Array', {
+ // 22.1.2.1 Array.from(arrayLike, mapfn = undefined, thisArg = undefined)
+ from: function from(arrayLike/*, mapfn = undefined, thisArg = undefined*/){
+ var O = toObject(arrayLike)
+ , C = typeof this == 'function' ? this : Array
+ , $$ = arguments
+ , $$len = $$.length
+ , mapfn = $$len > 1 ? $$[1] : undefined
+ , mapping = mapfn !== undefined
+ , index = 0
+ , iterFn = getIterFn(O)
+ , length, result, step, iterator;
+ if(mapping)mapfn = ctx(mapfn, $$len > 2 ? $$[2] : undefined, 2);
+ // if object isn't iterable or it's array with default iterator - use simple case
+ if(iterFn != undefined && !(C == Array && isArrayIter(iterFn))){
+ for(iterator = iterFn.call(O), result = new C; !(step = iterator.next()).done; index++){
+ result[index] = mapping ? call(iterator, mapfn, [step.value, index], true) : step.value;
+ }
+ } else {
+ length = toLength(O.length);
+ for(result = new C(length); length > index; index++){
+ result[index] = mapping ? mapfn(O[index], index) : O[index];
+ }
+ }
+ result.length = index;
+ return result;
+ }
+ });
+
+
+/***/ },
+/* 112 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // call something on iterator step with safe closing on error
+ var anObject = __webpack_require__(20);
+ module.exports = function(iterator, fn, value, entries){
+ try {
+ return entries ? fn(anObject(value)[0], value[1]) : fn(value);
+ // 7.4.6 IteratorClose(iterator, completion)
+ } catch(e){
+ var ret = iterator['return'];
+ if(ret !== undefined)anObject(ret.call(iterator));
+ throw e;
+ }
+ };
+
+/***/ },
+/* 113 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // check on default Array iterator
+ var Iterators = __webpack_require__(109)
+ , ITERATOR = __webpack_require__(31)('iterator')
+ , ArrayProto = Array.prototype;
+
+ module.exports = function(it){
+ return it !== undefined && (Iterators.Array === it || ArrayProto[ITERATOR] === it);
+ };
+
+/***/ },
+/* 114 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var classof = __webpack_require__(47)
+ , ITERATOR = __webpack_require__(31)('iterator')
+ , Iterators = __webpack_require__(109);
+ module.exports = __webpack_require__(5).getIteratorMethod = function(it){
+ if(it != undefined)return it[ITERATOR]
+ || it['@@iterator']
+ || Iterators[classof(it)];
+ };
+
+/***/ },
+/* 115 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var ITERATOR = __webpack_require__(31)('iterator')
+ , SAFE_CLOSING = false;
+
+ try {
+ var riter = [7][ITERATOR]();
+ riter['return'] = function(){ SAFE_CLOSING = true; };
+ Array.from(riter, function(){ throw 2; });
+ } catch(e){ /* empty */ }
+
+ module.exports = function(exec, skipClosing){
+ if(!skipClosing && !SAFE_CLOSING)return false;
+ var safe = false;
+ try {
+ var arr = [7]
+ , iter = arr[ITERATOR]();
+ iter.next = function(){ return {done: safe = true}; };
+ arr[ITERATOR] = function(){ return iter; };
+ exec(arr);
+ } catch(e){ /* empty */ }
+ return safe;
+ };
+
+/***/ },
+/* 116 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $export = __webpack_require__(3);
+
+ // WebKit Array.of isn't generic
+ $export($export.S + $export.F * __webpack_require__(9)(function(){
+ function F(){}
+ return !(Array.of.call(F) instanceof F);
+ }), 'Array', {
+ // 22.1.2.3 Array.of( ...items)
+ of: function of(/* ...args */){
+ var index = 0
+ , $$ = arguments
+ , $$len = $$.length
+ , result = new (typeof this == 'function' ? this : Array)($$len);
+ while($$len > index)result[index] = $$[index++];
+ result.length = $$len;
+ return result;
+ }
+ });
+
+/***/ },
+/* 117 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var addToUnscopables = __webpack_require__(118)
+ , step = __webpack_require__(119)
+ , Iterators = __webpack_require__(109)
+ , toIObject = __webpack_require__(23);
+
+ // 22.1.3.4 Array.prototype.entries()
+ // 22.1.3.13 Array.prototype.keys()
+ // 22.1.3.29 Array.prototype.values()
+ // 22.1.3.30 Array.prototype[@@iterator]()
+ module.exports = __webpack_require__(108)(Array, 'Array', function(iterated, kind){
+ this._t = toIObject(iterated); // target
+ this._i = 0; // next index
+ this._k = kind; // kind
+ // 22.1.5.2.1 %ArrayIteratorPrototype%.next()
+ }, function(){
+ var O = this._t
+ , kind = this._k
+ , index = this._i++;
+ if(!O || index >= O.length){
+ this._t = undefined;
+ return step(1);
+ }
+ if(kind == 'keys' )return step(0, index);
+ if(kind == 'values')return step(0, O[index]);
+ return step(0, [index, O[index]]);
+ }, 'values');
+
+ // argumentsList[@@iterator] is %ArrayProto_values% (9.4.4.6, 9.4.4.7)
+ Iterators.Arguments = Iterators.Array;
+
+ addToUnscopables('keys');
+ addToUnscopables('values');
+ addToUnscopables('entries');
+
+/***/ },
+/* 118 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 22.1.3.31 Array.prototype[@@unscopables]
+ var UNSCOPABLES = __webpack_require__(31)('unscopables')
+ , ArrayProto = Array.prototype;
+ if(ArrayProto[UNSCOPABLES] == undefined)__webpack_require__(6)(ArrayProto, UNSCOPABLES, {});
+ module.exports = function(key){
+ ArrayProto[UNSCOPABLES][key] = true;
+ };
+
+/***/ },
+/* 119 */
+/***/ function(module, exports) {
+
+ module.exports = function(done, value){
+ return {value: value, done: !!done};
+ };
+
+/***/ },
+/* 120 */
+/***/ function(module, exports, __webpack_require__) {
+
+ __webpack_require__(121)('Array');
+
+/***/ },
+/* 121 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var global = __webpack_require__(4)
+ , $ = __webpack_require__(2)
+ , DESCRIPTORS = __webpack_require__(8)
+ , SPECIES = __webpack_require__(31)('species');
+
+ module.exports = function(KEY){
+ var C = global[KEY];
+ if(DESCRIPTORS && C && !C[SPECIES])$.setDesc(C, SPECIES, {
+ configurable: true,
+ get: function(){ return this; }
+ });
+ };
+
+/***/ },
+/* 122 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 22.1.3.3 Array.prototype.copyWithin(target, start, end = this.length)
+ var $export = __webpack_require__(3);
+
+ $export($export.P, 'Array', {copyWithin: __webpack_require__(123)});
+
+ __webpack_require__(118)('copyWithin');
+
+/***/ },
+/* 123 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 22.1.3.3 Array.prototype.copyWithin(target, start, end = this.length)
+ 'use strict';
+ var toObject = __webpack_require__(21)
+ , toIndex = __webpack_require__(26)
+ , toLength = __webpack_require__(27);
+
+ module.exports = [].copyWithin || function copyWithin(target/*= 0*/, start/*= 0, end = @length*/){
+ var O = toObject(this)
+ , len = toLength(O.length)
+ , to = toIndex(target, len)
+ , from = toIndex(start, len)
+ , $$ = arguments
+ , end = $$.length > 2 ? $$[2] : undefined
+ , count = Math.min((end === undefined ? len : toIndex(end, len)) - from, len - to)
+ , inc = 1;
+ if(from < to && to < from + count){
+ inc = -1;
+ from += count - 1;
+ to += count - 1;
+ }
+ while(count-- > 0){
+ if(from in O)O[to] = O[from];
+ else delete O[to];
+ to += inc;
+ from += inc;
+ } return O;
+ };
+
+/***/ },
+/* 124 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 22.1.3.6 Array.prototype.fill(value, start = 0, end = this.length)
+ var $export = __webpack_require__(3);
+
+ $export($export.P, 'Array', {fill: __webpack_require__(125)});
+
+ __webpack_require__(118)('fill');
+
+/***/ },
+/* 125 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 22.1.3.6 Array.prototype.fill(value, start = 0, end = this.length)
+ 'use strict';
+ var toObject = __webpack_require__(21)
+ , toIndex = __webpack_require__(26)
+ , toLength = __webpack_require__(27);
+ module.exports = [].fill || function fill(value /*, start = 0, end = @length */){
+ var O = toObject(this)
+ , length = toLength(O.length)
+ , $$ = arguments
+ , $$len = $$.length
+ , index = toIndex($$len > 1 ? $$[1] : undefined, length)
+ , end = $$len > 2 ? $$[2] : undefined
+ , endPos = end === undefined ? length : toIndex(end, length);
+ while(endPos > index)O[index++] = value;
+ return O;
+ };
+
+/***/ },
+/* 126 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // 22.1.3.8 Array.prototype.find(predicate, thisArg = undefined)
+ var $export = __webpack_require__(3)
+ , $find = __webpack_require__(28)(5)
+ , KEY = 'find'
+ , forced = true;
+ // Shouldn't skip holes
+ if(KEY in [])Array(1)[KEY](function(){ forced = false; });
+ $export($export.P + $export.F * forced, 'Array', {
+ find: function find(callbackfn/*, that = undefined */){
+ return $find(this, callbackfn, arguments.length > 1 ? arguments[1] : undefined);
+ }
+ });
+ __webpack_require__(118)(KEY);
+
+/***/ },
+/* 127 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // 22.1.3.9 Array.prototype.findIndex(predicate, thisArg = undefined)
+ var $export = __webpack_require__(3)
+ , $find = __webpack_require__(28)(6)
+ , KEY = 'findIndex'
+ , forced = true;
+ // Shouldn't skip holes
+ if(KEY in [])Array(1)[KEY](function(){ forced = false; });
+ $export($export.P + $export.F * forced, 'Array', {
+ findIndex: function findIndex(callbackfn/*, that = undefined */){
+ return $find(this, callbackfn, arguments.length > 1 ? arguments[1] : undefined);
+ }
+ });
+ __webpack_require__(118)(KEY);
+
+/***/ },
+/* 128 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $ = __webpack_require__(2)
+ , global = __webpack_require__(4)
+ , isRegExp = __webpack_require__(101)
+ , $flags = __webpack_require__(129)
+ , $RegExp = global.RegExp
+ , Base = $RegExp
+ , proto = $RegExp.prototype
+ , re1 = /a/g
+ , re2 = /a/g
+ // "new" creates a new object, old webkit buggy here
+ , CORRECT_NEW = new $RegExp(re1) !== re1;
+
+ if(__webpack_require__(8) && (!CORRECT_NEW || __webpack_require__(9)(function(){
+ re2[__webpack_require__(31)('match')] = false;
+ // RegExp constructor can alter flags and IsRegExp works correct with @@match
+ return $RegExp(re1) != re1 || $RegExp(re2) == re2 || $RegExp(re1, 'i') != '/a/i';
+ }))){
+ $RegExp = function RegExp(p, f){
+ var piRE = isRegExp(p)
+ , fiU = f === undefined;
+ return !(this instanceof $RegExp) && piRE && p.constructor === $RegExp && fiU ? p
+ : CORRECT_NEW
+ ? new Base(piRE && !fiU ? p.source : p, f)
+ : Base((piRE = p instanceof $RegExp) ? p.source : p, piRE && fiU ? $flags.call(p) : f);
+ };
+ $.each.call($.getNames(Base), function(key){
+ key in $RegExp || $.setDesc($RegExp, key, {
+ configurable: true,
+ get: function(){ return Base[key]; },
+ set: function(it){ Base[key] = it; }
+ });
+ });
+ proto.constructor = $RegExp;
+ $RegExp.prototype = proto;
+ __webpack_require__(10)(global, 'RegExp', $RegExp);
+ }
+
+ __webpack_require__(121)('RegExp');
+
+/***/ },
+/* 129 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // 21.2.5.3 get RegExp.prototype.flags
+ var anObject = __webpack_require__(20);
+ module.exports = function(){
+ var that = anObject(this)
+ , result = '';
+ if(that.global) result += 'g';
+ if(that.ignoreCase) result += 'i';
+ if(that.multiline) result += 'm';
+ if(that.unicode) result += 'u';
+ if(that.sticky) result += 'y';
+ return result;
+ };
+
+/***/ },
+/* 130 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 21.2.5.3 get RegExp.prototype.flags()
+ var $ = __webpack_require__(2);
+ if(__webpack_require__(8) && /./g.flags != 'g')$.setDesc(RegExp.prototype, 'flags', {
+ configurable: true,
+ get: __webpack_require__(129)
+ });
+
+/***/ },
+/* 131 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // @@match logic
+ __webpack_require__(132)('match', 1, function(defined, MATCH){
+ // 21.1.3.11 String.prototype.match(regexp)
+ return function match(regexp){
+ 'use strict';
+ var O = defined(this)
+ , fn = regexp == undefined ? undefined : regexp[MATCH];
+ return fn !== undefined ? fn.call(regexp, O) : new RegExp(regexp)[MATCH](String(O));
+ };
+ });
+
+/***/ },
+/* 132 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var hide = __webpack_require__(6)
+ , redefine = __webpack_require__(10)
+ , fails = __webpack_require__(9)
+ , defined = __webpack_require__(22)
+ , wks = __webpack_require__(31);
+
+ module.exports = function(KEY, length, exec){
+ var SYMBOL = wks(KEY)
+ , original = ''[KEY];
+ if(fails(function(){
+ var O = {};
+ O[SYMBOL] = function(){ return 7; };
+ return ''[KEY](O) != 7;
+ })){
+ redefine(String.prototype, KEY, exec(defined, SYMBOL, original));
+ hide(RegExp.prototype, SYMBOL, length == 2
+ // 21.2.5.8 RegExp.prototype[@@replace](string, replaceValue)
+ // 21.2.5.11 RegExp.prototype[@@split](string, limit)
+ ? function(string, arg){ return original.call(string, this, arg); }
+ // 21.2.5.6 RegExp.prototype[@@match](string)
+ // 21.2.5.9 RegExp.prototype[@@search](string)
+ : function(string){ return original.call(string, this); }
+ );
+ }
+ };
+
+/***/ },
+/* 133 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // @@replace logic
+ __webpack_require__(132)('replace', 2, function(defined, REPLACE, $replace){
+ // 21.1.3.14 String.prototype.replace(searchValue, replaceValue)
+ return function replace(searchValue, replaceValue){
+ 'use strict';
+ var O = defined(this)
+ , fn = searchValue == undefined ? undefined : searchValue[REPLACE];
+ return fn !== undefined
+ ? fn.call(searchValue, O, replaceValue)
+ : $replace.call(String(O), searchValue, replaceValue);
+ };
+ });
+
+/***/ },
+/* 134 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // @@search logic
+ __webpack_require__(132)('search', 1, function(defined, SEARCH){
+ // 21.1.3.15 String.prototype.search(regexp)
+ return function search(regexp){
+ 'use strict';
+ var O = defined(this)
+ , fn = regexp == undefined ? undefined : regexp[SEARCH];
+ return fn !== undefined ? fn.call(regexp, O) : new RegExp(regexp)[SEARCH](String(O));
+ };
+ });
+
+/***/ },
+/* 135 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // @@split logic
+ __webpack_require__(132)('split', 2, function(defined, SPLIT, $split){
+ // 21.1.3.17 String.prototype.split(separator, limit)
+ return function split(separator, limit){
+ 'use strict';
+ var O = defined(this)
+ , fn = separator == undefined ? undefined : separator[SPLIT];
+ return fn !== undefined
+ ? fn.call(separator, O, limit)
+ : $split.call(String(O), separator, limit);
+ };
+ });
+
+/***/ },
+/* 136 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , LIBRARY = __webpack_require__(39)
+ , global = __webpack_require__(4)
+ , ctx = __webpack_require__(12)
+ , classof = __webpack_require__(47)
+ , $export = __webpack_require__(3)
+ , isObject = __webpack_require__(16)
+ , anObject = __webpack_require__(20)
+ , aFunction = __webpack_require__(13)
+ , strictNew = __webpack_require__(137)
+ , forOf = __webpack_require__(138)
+ , setProto = __webpack_require__(45).set
+ , same = __webpack_require__(43)
+ , SPECIES = __webpack_require__(31)('species')
+ , speciesConstructor = __webpack_require__(139)
+ , asap = __webpack_require__(140)
+ , PROMISE = 'Promise'
+ , process = global.process
+ , isNode = classof(process) == 'process'
+ , P = global[PROMISE]
+ , empty = function(){ /* empty */ }
+ , Wrapper;
+
+ var testResolve = function(sub){
+ var test = new P(empty), promise;
+ if(sub)test.constructor = function(exec){
+ exec(empty, empty);
+ };
+ (promise = P.resolve(test))['catch'](empty);
+ return promise === test;
+ };
+
+ var USE_NATIVE = function(){
+ var works = false;
+ function P2(x){
+ var self = new P(x);
+ setProto(self, P2.prototype);
+ return self;
+ }
+ try {
+ works = P && P.resolve && testResolve();
+ setProto(P2, P);
+ P2.prototype = $.create(P.prototype, {constructor: {value: P2}});
+ // actual Firefox has broken subclass support, test that
+ if(!(P2.resolve(5).then(function(){}) instanceof P2)){
+ works = false;
+ }
+ // actual V8 bug, https://code.google.com/p/v8/issues/detail?id=4162
+ if(works && __webpack_require__(8)){
+ var thenableThenGotten = false;
+ P.resolve($.setDesc({}, 'then', {
+ get: function(){ thenableThenGotten = true; }
+ }));
+ works = thenableThenGotten;
+ }
+ } catch(e){ works = false; }
+ return works;
+ }();
+
+ // helpers
+ var sameConstructor = function(a, b){
+ // library wrapper special case
+ if(LIBRARY && a === P && b === Wrapper)return true;
+ return same(a, b);
+ };
+ var getConstructor = function(C){
+ var S = anObject(C)[SPECIES];
+ return S != undefined ? S : C;
+ };
+ var isThenable = function(it){
+ var then;
+ return isObject(it) && typeof (then = it.then) == 'function' ? then : false;
+ };
+ var PromiseCapability = function(C){
+ var resolve, reject;
+ this.promise = new C(function($$resolve, $$reject){
+ if(resolve !== undefined || reject !== undefined)throw TypeError('Bad Promise constructor');
+ resolve = $$resolve;
+ reject = $$reject;
+ });
+ this.resolve = aFunction(resolve),
+ this.reject = aFunction(reject)
+ };
+ var perform = function(exec){
+ try {
+ exec();
+ } catch(e){
+ return {error: e};
+ }
+ };
+ var notify = function(record, isReject){
+ if(record.n)return;
+ record.n = true;
+ var chain = record.c;
+ asap(function(){
+ var value = record.v
+ , ok = record.s == 1
+ , i = 0;
+ var run = function(reaction){
+ var handler = ok ? reaction.ok : reaction.fail
+ , resolve = reaction.resolve
+ , reject = reaction.reject
+ , result, then;
+ try {
+ if(handler){
+ if(!ok)record.h = true;
+ result = handler === true ? value : handler(value);
+ if(result === reaction.promise){
+ reject(TypeError('Promise-chain cycle'));
+ } else if(then = isThenable(result)){
+ then.call(result, resolve, reject);
+ } else resolve(result);
+ } else reject(value);
+ } catch(e){
+ reject(e);
+ }
+ };
+ while(chain.length > i)run(chain[i++]); // variable length - can't use forEach
+ chain.length = 0;
+ record.n = false;
+ if(isReject)setTimeout(function(){
+ var promise = record.p
+ , handler, console;
+ if(isUnhandled(promise)){
+ if(isNode){
+ process.emit('unhandledRejection', value, promise);
+ } else if(handler = global.onunhandledrejection){
+ handler({promise: promise, reason: value});
+ } else if((console = global.console) && console.error){
+ console.error('Unhandled promise rejection', value);
+ }
+ } record.a = undefined;
+ }, 1);
+ });
+ };
+ var isUnhandled = function(promise){
+ var record = promise._d
+ , chain = record.a || record.c
+ , i = 0
+ , reaction;
+ if(record.h)return false;
+ while(chain.length > i){
+ reaction = chain[i++];
+ if(reaction.fail || !isUnhandled(reaction.promise))return false;
+ } return true;
+ };
+ var $reject = function(value){
+ var record = this;
+ if(record.d)return;
+ record.d = true;
+ record = record.r || record; // unwrap
+ record.v = value;
+ record.s = 2;
+ record.a = record.c.slice();
+ notify(record, true);
+ };
+ var $resolve = function(value){
+ var record = this
+ , then;
+ if(record.d)return;
+ record.d = true;
+ record = record.r || record; // unwrap
+ try {
+ if(record.p === value)throw TypeError("Promise can't be resolved itself");
+ if(then = isThenable(value)){
+ asap(function(){
+ var wrapper = {r: record, d: false}; // wrap
+ try {
+ then.call(value, ctx($resolve, wrapper, 1), ctx($reject, wrapper, 1));
+ } catch(e){
+ $reject.call(wrapper, e);
+ }
+ });
+ } else {
+ record.v = value;
+ record.s = 1;
+ notify(record, false);
+ }
+ } catch(e){
+ $reject.call({r: record, d: false}, e); // wrap
+ }
+ };
+
+ // constructor polyfill
+ if(!USE_NATIVE){
+ // 25.4.3.1 Promise(executor)
+ P = function Promise(executor){
+ aFunction(executor);
+ var record = this._d = {
+ p: strictNew(this, P, PROMISE), // <- promise
+ c: [], // <- awaiting reactions
+ a: undefined, // <- checked in isUnhandled reactions
+ s: 0, // <- state
+ d: false, // <- done
+ v: undefined, // <- value
+ h: false, // <- handled rejection
+ n: false // <- notify
+ };
+ try {
+ executor(ctx($resolve, record, 1), ctx($reject, record, 1));
+ } catch(err){
+ $reject.call(record, err);
+ }
+ };
+ __webpack_require__(142)(P.prototype, {
+ // 25.4.5.3 Promise.prototype.then(onFulfilled, onRejected)
+ then: function then(onFulfilled, onRejected){
+ var reaction = new PromiseCapability(speciesConstructor(this, P))
+ , promise = reaction.promise
+ , record = this._d;
+ reaction.ok = typeof onFulfilled == 'function' ? onFulfilled : true;
+ reaction.fail = typeof onRejected == 'function' && onRejected;
+ record.c.push(reaction);
+ if(record.a)record.a.push(reaction);
+ if(record.s)notify(record, false);
+ return promise;
+ },
+ // 25.4.5.1 Promise.prototype.catch(onRejected)
+ 'catch': function(onRejected){
+ return this.then(undefined, onRejected);
+ }
+ });
+ }
+
+ $export($export.G + $export.W + $export.F * !USE_NATIVE, {Promise: P});
+ __webpack_require__(35)(P, PROMISE);
+ __webpack_require__(121)(PROMISE);
+ Wrapper = __webpack_require__(5)[PROMISE];
+
+ // statics
+ $export($export.S + $export.F * !USE_NATIVE, PROMISE, {
+ // 25.4.4.5 Promise.reject(r)
+ reject: function reject(r){
+ var capability = new PromiseCapability(this)
+ , $$reject = capability.reject;
+ $$reject(r);
+ return capability.promise;
+ }
+ });
+ $export($export.S + $export.F * (!USE_NATIVE || testResolve(true)), PROMISE, {
+ // 25.4.4.6 Promise.resolve(x)
+ resolve: function resolve(x){
+ // instanceof instead of internal slot check because we should fix it without replacement native Promise core
+ if(x instanceof P && sameConstructor(x.constructor, this))return x;
+ var capability = new PromiseCapability(this)
+ , $$resolve = capability.resolve;
+ $$resolve(x);
+ return capability.promise;
+ }
+ });
+ $export($export.S + $export.F * !(USE_NATIVE && __webpack_require__(115)(function(iter){
+ P.all(iter)['catch'](function(){});
+ })), PROMISE, {
+ // 25.4.4.1 Promise.all(iterable)
+ all: function all(iterable){
+ var C = getConstructor(this)
+ , capability = new PromiseCapability(C)
+ , resolve = capability.resolve
+ , reject = capability.reject
+ , values = [];
+ var abrupt = perform(function(){
+ forOf(iterable, false, values.push, values);
+ var remaining = values.length
+ , results = Array(remaining);
+ if(remaining)$.each.call(values, function(promise, index){
+ var alreadyCalled = false;
+ C.resolve(promise).then(function(value){
+ if(alreadyCalled)return;
+ alreadyCalled = true;
+ results[index] = value;
+ --remaining || resolve(results);
+ }, reject);
+ });
+ else resolve(results);
+ });
+ if(abrupt)reject(abrupt.error);
+ return capability.promise;
+ },
+ // 25.4.4.4 Promise.race(iterable)
+ race: function race(iterable){
+ var C = getConstructor(this)
+ , capability = new PromiseCapability(C)
+ , reject = capability.reject;
+ var abrupt = perform(function(){
+ forOf(iterable, false, function(promise){
+ C.resolve(promise).then(capability.resolve, reject);
+ });
+ });
+ if(abrupt)reject(abrupt.error);
+ return capability.promise;
+ }
+ });
+
+/***/ },
+/* 137 */
+/***/ function(module, exports) {
+
+ module.exports = function(it, Constructor, name){
+ if(!(it instanceof Constructor))throw TypeError(name + ": use the 'new' operator!");
+ return it;
+ };
+
+/***/ },
+/* 138 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var ctx = __webpack_require__(12)
+ , call = __webpack_require__(112)
+ , isArrayIter = __webpack_require__(113)
+ , anObject = __webpack_require__(20)
+ , toLength = __webpack_require__(27)
+ , getIterFn = __webpack_require__(114);
+ module.exports = function(iterable, entries, fn, that){
+ var iterFn = getIterFn(iterable)
+ , f = ctx(fn, that, entries ? 2 : 1)
+ , index = 0
+ , length, step, iterator;
+ if(typeof iterFn != 'function')throw TypeError(iterable + ' is not iterable!');
+ // fast case for arrays with default iterator
+ if(isArrayIter(iterFn))for(length = toLength(iterable.length); length > index; index++){
+ entries ? f(anObject(step = iterable[index])[0], step[1]) : f(iterable[index]);
+ } else for(iterator = iterFn.call(iterable); !(step = iterator.next()).done; ){
+ call(iterator, f, step.value, entries);
+ }
+ };
+
+/***/ },
+/* 139 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 7.3.20 SpeciesConstructor(O, defaultConstructor)
+ var anObject = __webpack_require__(20)
+ , aFunction = __webpack_require__(13)
+ , SPECIES = __webpack_require__(31)('species');
+ module.exports = function(O, D){
+ var C = anObject(O).constructor, S;
+ return C === undefined || (S = anObject(C)[SPECIES]) == undefined ? D : aFunction(S);
+ };
+
+/***/ },
+/* 140 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var global = __webpack_require__(4)
+ , macrotask = __webpack_require__(141).set
+ , Observer = global.MutationObserver || global.WebKitMutationObserver
+ , process = global.process
+ , Promise = global.Promise
+ , isNode = __webpack_require__(18)(process) == 'process'
+ , head, last, notify;
+
+ var flush = function(){
+ var parent, domain, fn;
+ if(isNode && (parent = process.domain)){
+ process.domain = null;
+ parent.exit();
+ }
+ while(head){
+ domain = head.domain;
+ fn = head.fn;
+ if(domain)domain.enter();
+ fn(); // <- currently we use it only for Promise - try / catch not required
+ if(domain)domain.exit();
+ head = head.next;
+ } last = undefined;
+ if(parent)parent.enter();
+ };
+
+ // Node.js
+ if(isNode){
+ notify = function(){
+ process.nextTick(flush);
+ };
+ // browsers with MutationObserver
+ } else if(Observer){
+ var toggle = 1
+ , node = document.createTextNode('');
+ new Observer(flush).observe(node, {characterData: true}); // eslint-disable-line no-new
+ notify = function(){
+ node.data = toggle = -toggle;
+ };
+ // environments with maybe non-completely correct, but existent Promise
+ } else if(Promise && Promise.resolve){
+ notify = function(){
+ Promise.resolve().then(flush);
+ };
+ // for other environments - macrotask based on:
+ // - setImmediate
+ // - MessageChannel
+ // - window.postMessag
+ // - onreadystatechange
+ // - setTimeout
+ } else {
+ notify = function(){
+ // strange IE + webpack dev server bug - use .call(global)
+ macrotask.call(global, flush);
+ };
+ }
+
+ module.exports = function asap(fn){
+ var task = {fn: fn, next: undefined, domain: isNode && process.domain};
+ if(last)last.next = task;
+ if(!head){
+ head = task;
+ notify();
+ } last = task;
+ };
+
+/***/ },
+/* 141 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var ctx = __webpack_require__(12)
+ , invoke = __webpack_require__(19)
+ , html = __webpack_require__(14)
+ , cel = __webpack_require__(15)
+ , global = __webpack_require__(4)
+ , process = global.process
+ , setTask = global.setImmediate
+ , clearTask = global.clearImmediate
+ , MessageChannel = global.MessageChannel
+ , counter = 0
+ , queue = {}
+ , ONREADYSTATECHANGE = 'onreadystatechange'
+ , defer, channel, port;
+ var run = function(){
+ var id = +this;
+ if(queue.hasOwnProperty(id)){
+ var fn = queue[id];
+ delete queue[id];
+ fn();
+ }
+ };
+ var listner = function(event){
+ run.call(event.data);
+ };
+ // Node.js 0.9+ & IE10+ has setImmediate, otherwise:
+ if(!setTask || !clearTask){
+ setTask = function setImmediate(fn){
+ var args = [], i = 1;
+ while(arguments.length > i)args.push(arguments[i++]);
+ queue[++counter] = function(){
+ invoke(typeof fn == 'function' ? fn : Function(fn), args);
+ };
+ defer(counter);
+ return counter;
+ };
+ clearTask = function clearImmediate(id){
+ delete queue[id];
+ };
+ // Node.js 0.8-
+ if(__webpack_require__(18)(process) == 'process'){
+ defer = function(id){
+ process.nextTick(ctx(run, id, 1));
+ };
+ // Browsers with MessageChannel, includes WebWorkers
+ } else if(MessageChannel){
+ channel = new MessageChannel;
+ port = channel.port2;
+ channel.port1.onmessage = listner;
+ defer = ctx(port.postMessage, port, 1);
+ // Browsers with postMessage, skip WebWorkers
+ // IE8 has postMessage, but it's sync & typeof its postMessage is 'object'
+ } else if(global.addEventListener && typeof postMessage == 'function' && !global.importScripts){
+ defer = function(id){
+ global.postMessage(id + '', '*');
+ };
+ global.addEventListener('message', listner, false);
+ // IE8-
+ } else if(ONREADYSTATECHANGE in cel('script')){
+ defer = function(id){
+ html.appendChild(cel('script'))[ONREADYSTATECHANGE] = function(){
+ html.removeChild(this);
+ run.call(id);
+ };
+ };
+ // Rest old browsers
+ } else {
+ defer = function(id){
+ setTimeout(ctx(run, id, 1), 0);
+ };
+ }
+ }
+ module.exports = {
+ set: setTask,
+ clear: clearTask
+ };
+
+/***/ },
+/* 142 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var redefine = __webpack_require__(10);
+ module.exports = function(target, src){
+ for(var key in src)redefine(target, key, src[key]);
+ return target;
+ };
+
+/***/ },
+/* 143 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var strong = __webpack_require__(144);
+
+ // 23.1 Map Objects
+ __webpack_require__(145)('Map', function(get){
+ return function Map(){ return get(this, arguments.length > 0 ? arguments[0] : undefined); };
+ }, {
+ // 23.1.3.6 Map.prototype.get(key)
+ get: function get(key){
+ var entry = strong.getEntry(this, key);
+ return entry && entry.v;
+ },
+ // 23.1.3.9 Map.prototype.set(key, value)
+ set: function set(key, value){
+ return strong.def(this, key === 0 ? 0 : key, value);
+ }
+ }, strong, true);
+
+/***/ },
+/* 144 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , hide = __webpack_require__(6)
+ , redefineAll = __webpack_require__(142)
+ , ctx = __webpack_require__(12)
+ , strictNew = __webpack_require__(137)
+ , defined = __webpack_require__(22)
+ , forOf = __webpack_require__(138)
+ , $iterDefine = __webpack_require__(108)
+ , step = __webpack_require__(119)
+ , ID = __webpack_require__(11)('id')
+ , $has = __webpack_require__(17)
+ , isObject = __webpack_require__(16)
+ , setSpecies = __webpack_require__(121)
+ , DESCRIPTORS = __webpack_require__(8)
+ , isExtensible = Object.isExtensible || isObject
+ , SIZE = DESCRIPTORS ? '_s' : 'size'
+ , id = 0;
+
+ var fastKey = function(it, create){
+ // return primitive with prefix
+ if(!isObject(it))return typeof it == 'symbol' ? it : (typeof it == 'string' ? 'S' : 'P') + it;
+ if(!$has(it, ID)){
+ // can't set id to frozen object
+ if(!isExtensible(it))return 'F';
+ // not necessary to add id
+ if(!create)return 'E';
+ // add missing object id
+ hide(it, ID, ++id);
+ // return object id with prefix
+ } return 'O' + it[ID];
+ };
+
+ var getEntry = function(that, key){
+ // fast case
+ var index = fastKey(key), entry;
+ if(index !== 'F')return that._i[index];
+ // frozen object case
+ for(entry = that._f; entry; entry = entry.n){
+ if(entry.k == key)return entry;
+ }
+ };
+
+ module.exports = {
+ getConstructor: function(wrapper, NAME, IS_MAP, ADDER){
+ var C = wrapper(function(that, iterable){
+ strictNew(that, C, NAME);
+ that._i = $.create(null); // index
+ that._f = undefined; // first entry
+ that._l = undefined; // last entry
+ that[SIZE] = 0; // size
+ if(iterable != undefined)forOf(iterable, IS_MAP, that[ADDER], that);
+ });
+ redefineAll(C.prototype, {
+ // 23.1.3.1 Map.prototype.clear()
+ // 23.2.3.2 Set.prototype.clear()
+ clear: function clear(){
+ for(var that = this, data = that._i, entry = that._f; entry; entry = entry.n){
+ entry.r = true;
+ if(entry.p)entry.p = entry.p.n = undefined;
+ delete data[entry.i];
+ }
+ that._f = that._l = undefined;
+ that[SIZE] = 0;
+ },
+ // 23.1.3.3 Map.prototype.delete(key)
+ // 23.2.3.4 Set.prototype.delete(value)
+ 'delete': function(key){
+ var that = this
+ , entry = getEntry(that, key);
+ if(entry){
+ var next = entry.n
+ , prev = entry.p;
+ delete that._i[entry.i];
+ entry.r = true;
+ if(prev)prev.n = next;
+ if(next)next.p = prev;
+ if(that._f == entry)that._f = next;
+ if(that._l == entry)that._l = prev;
+ that[SIZE]--;
+ } return !!entry;
+ },
+ // 23.2.3.6 Set.prototype.forEach(callbackfn, thisArg = undefined)
+ // 23.1.3.5 Map.prototype.forEach(callbackfn, thisArg = undefined)
+ forEach: function forEach(callbackfn /*, that = undefined */){
+ var f = ctx(callbackfn, arguments.length > 1 ? arguments[1] : undefined, 3)
+ , entry;
+ while(entry = entry ? entry.n : this._f){
+ f(entry.v, entry.k, this);
+ // revert to the last existing entry
+ while(entry && entry.r)entry = entry.p;
+ }
+ },
+ // 23.1.3.7 Map.prototype.has(key)
+ // 23.2.3.7 Set.prototype.has(value)
+ has: function has(key){
+ return !!getEntry(this, key);
+ }
+ });
+ if(DESCRIPTORS)$.setDesc(C.prototype, 'size', {
+ get: function(){
+ return defined(this[SIZE]);
+ }
+ });
+ return C;
+ },
+ def: function(that, key, value){
+ var entry = getEntry(that, key)
+ , prev, index;
+ // change existing entry
+ if(entry){
+ entry.v = value;
+ // create new entry
+ } else {
+ that._l = entry = {
+ i: index = fastKey(key, true), // <- index
+ k: key, // <- key
+ v: value, // <- value
+ p: prev = that._l, // <- previous entry
+ n: undefined, // <- next entry
+ r: false // <- removed
+ };
+ if(!that._f)that._f = entry;
+ if(prev)prev.n = entry;
+ that[SIZE]++;
+ // add to index
+ if(index !== 'F')that._i[index] = entry;
+ } return that;
+ },
+ getEntry: getEntry,
+ setStrong: function(C, NAME, IS_MAP){
+ // add .keys, .values, .entries, [@@iterator]
+ // 23.1.3.4, 23.1.3.8, 23.1.3.11, 23.1.3.12, 23.2.3.5, 23.2.3.8, 23.2.3.10, 23.2.3.11
+ $iterDefine(C, NAME, function(iterated, kind){
+ this._t = iterated; // target
+ this._k = kind; // kind
+ this._l = undefined; // previous
+ }, function(){
+ var that = this
+ , kind = that._k
+ , entry = that._l;
+ // revert to the last existing entry
+ while(entry && entry.r)entry = entry.p;
+ // get next entry
+ if(!that._t || !(that._l = entry = entry ? entry.n : that._t._f)){
+ // or finish the iteration
+ that._t = undefined;
+ return step(1);
+ }
+ // return step by kind
+ if(kind == 'keys' )return step(0, entry.k);
+ if(kind == 'values')return step(0, entry.v);
+ return step(0, [entry.k, entry.v]);
+ }, IS_MAP ? 'entries' : 'values' , !IS_MAP, true);
+
+ // add [@@species], 23.1.2.2, 23.2.2.2
+ setSpecies(NAME);
+ }
+ };
+
+/***/ },
+/* 145 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var global = __webpack_require__(4)
+ , $export = __webpack_require__(3)
+ , redefine = __webpack_require__(10)
+ , redefineAll = __webpack_require__(142)
+ , forOf = __webpack_require__(138)
+ , strictNew = __webpack_require__(137)
+ , isObject = __webpack_require__(16)
+ , fails = __webpack_require__(9)
+ , $iterDetect = __webpack_require__(115)
+ , setToStringTag = __webpack_require__(35);
+
+ module.exports = function(NAME, wrapper, methods, common, IS_MAP, IS_WEAK){
+ var Base = global[NAME]
+ , C = Base
+ , ADDER = IS_MAP ? 'set' : 'add'
+ , proto = C && C.prototype
+ , O = {};
+ var fixMethod = function(KEY){
+ var fn = proto[KEY];
+ redefine(proto, KEY,
+ KEY == 'delete' ? function(a){
+ return IS_WEAK && !isObject(a) ? false : fn.call(this, a === 0 ? 0 : a);
+ } : KEY == 'has' ? function has(a){
+ return IS_WEAK && !isObject(a) ? false : fn.call(this, a === 0 ? 0 : a);
+ } : KEY == 'get' ? function get(a){
+ return IS_WEAK && !isObject(a) ? undefined : fn.call(this, a === 0 ? 0 : a);
+ } : KEY == 'add' ? function add(a){ fn.call(this, a === 0 ? 0 : a); return this; }
+ : function set(a, b){ fn.call(this, a === 0 ? 0 : a, b); return this; }
+ );
+ };
+ if(typeof C != 'function' || !(IS_WEAK || proto.forEach && !fails(function(){
+ new C().entries().next();
+ }))){
+ // create collection constructor
+ C = common.getConstructor(wrapper, NAME, IS_MAP, ADDER);
+ redefineAll(C.prototype, methods);
+ } else {
+ var instance = new C
+ // early implementations not supports chaining
+ , HASNT_CHAINING = instance[ADDER](IS_WEAK ? {} : -0, 1) != instance
+ // V8 ~ Chromium 40- weak-collections throws on primitives, but should return false
+ , THROWS_ON_PRIMITIVES = fails(function(){ instance.has(1); })
+ // most early implementations doesn't supports iterables, most modern - not close it correctly
+ , ACCEPT_ITERABLES = $iterDetect(function(iter){ new C(iter); }) // eslint-disable-line no-new
+ // for early implementations -0 and +0 not the same
+ , BUGGY_ZERO;
+ if(!ACCEPT_ITERABLES){
+ C = wrapper(function(target, iterable){
+ strictNew(target, C, NAME);
+ var that = new Base;
+ if(iterable != undefined)forOf(iterable, IS_MAP, that[ADDER], that);
+ return that;
+ });
+ C.prototype = proto;
+ proto.constructor = C;
+ }
+ IS_WEAK || instance.forEach(function(val, key){
+ BUGGY_ZERO = 1 / key === -Infinity;
+ });
+ if(THROWS_ON_PRIMITIVES || BUGGY_ZERO){
+ fixMethod('delete');
+ fixMethod('has');
+ IS_MAP && fixMethod('get');
+ }
+ if(BUGGY_ZERO || HASNT_CHAINING)fixMethod(ADDER);
+ // weak collections should not contains .clear method
+ if(IS_WEAK && proto.clear)delete proto.clear;
+ }
+
+ setToStringTag(C, NAME);
+
+ O[NAME] = C;
+ $export($export.G + $export.W + $export.F * (C != Base), O);
+
+ if(!IS_WEAK)common.setStrong(C, NAME, IS_MAP);
+
+ return C;
+ };
+
+/***/ },
+/* 146 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var strong = __webpack_require__(144);
+
+ // 23.2 Set Objects
+ __webpack_require__(145)('Set', function(get){
+ return function Set(){ return get(this, arguments.length > 0 ? arguments[0] : undefined); };
+ }, {
+ // 23.2.3.1 Set.prototype.add(value)
+ add: function add(value){
+ return strong.def(this, value = value === 0 ? 0 : value, value);
+ }
+ }, strong);
+
+/***/ },
+/* 147 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $ = __webpack_require__(2)
+ , redefine = __webpack_require__(10)
+ , weak = __webpack_require__(148)
+ , isObject = __webpack_require__(16)
+ , has = __webpack_require__(17)
+ , frozenStore = weak.frozenStore
+ , WEAK = weak.WEAK
+ , isExtensible = Object.isExtensible || isObject
+ , tmp = {};
+
+ // 23.3 WeakMap Objects
+ var $WeakMap = __webpack_require__(145)('WeakMap', function(get){
+ return function WeakMap(){ return get(this, arguments.length > 0 ? arguments[0] : undefined); };
+ }, {
+ // 23.3.3.3 WeakMap.prototype.get(key)
+ get: function get(key){
+ if(isObject(key)){
+ if(!isExtensible(key))return frozenStore(this).get(key);
+ if(has(key, WEAK))return key[WEAK][this._i];
+ }
+ },
+ // 23.3.3.5 WeakMap.prototype.set(key, value)
+ set: function set(key, value){
+ return weak.def(this, key, value);
+ }
+ }, weak, true, true);
+
+ // IE11 WeakMap frozen keys fix
+ if(new $WeakMap().set((Object.freeze || Object)(tmp), 7).get(tmp) != 7){
+ $.each.call(['delete', 'has', 'get', 'set'], function(key){
+ var proto = $WeakMap.prototype
+ , method = proto[key];
+ redefine(proto, key, function(a, b){
+ // store frozen objects on leaky map
+ if(isObject(a) && !isExtensible(a)){
+ var result = frozenStore(this)[key](a, b);
+ return key == 'set' ? this : result;
+ // store all the rest on native weakmap
+ } return method.call(this, a, b);
+ });
+ });
+ }
+
+/***/ },
+/* 148 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var hide = __webpack_require__(6)
+ , redefineAll = __webpack_require__(142)
+ , anObject = __webpack_require__(20)
+ , isObject = __webpack_require__(16)
+ , strictNew = __webpack_require__(137)
+ , forOf = __webpack_require__(138)
+ , createArrayMethod = __webpack_require__(28)
+ , $has = __webpack_require__(17)
+ , WEAK = __webpack_require__(11)('weak')
+ , isExtensible = Object.isExtensible || isObject
+ , arrayFind = createArrayMethod(5)
+ , arrayFindIndex = createArrayMethod(6)
+ , id = 0;
+
+ // fallback for frozen keys
+ var frozenStore = function(that){
+ return that._l || (that._l = new FrozenStore);
+ };
+ var FrozenStore = function(){
+ this.a = [];
+ };
+ var findFrozen = function(store, key){
+ return arrayFind(store.a, function(it){
+ return it[0] === key;
+ });
+ };
+ FrozenStore.prototype = {
+ get: function(key){
+ var entry = findFrozen(this, key);
+ if(entry)return entry[1];
+ },
+ has: function(key){
+ return !!findFrozen(this, key);
+ },
+ set: function(key, value){
+ var entry = findFrozen(this, key);
+ if(entry)entry[1] = value;
+ else this.a.push([key, value]);
+ },
+ 'delete': function(key){
+ var index = arrayFindIndex(this.a, function(it){
+ return it[0] === key;
+ });
+ if(~index)this.a.splice(index, 1);
+ return !!~index;
+ }
+ };
+
+ module.exports = {
+ getConstructor: function(wrapper, NAME, IS_MAP, ADDER){
+ var C = wrapper(function(that, iterable){
+ strictNew(that, C, NAME);
+ that._i = id++; // collection id
+ that._l = undefined; // leak store for frozen objects
+ if(iterable != undefined)forOf(iterable, IS_MAP, that[ADDER], that);
+ });
+ redefineAll(C.prototype, {
+ // 23.3.3.2 WeakMap.prototype.delete(key)
+ // 23.4.3.3 WeakSet.prototype.delete(value)
+ 'delete': function(key){
+ if(!isObject(key))return false;
+ if(!isExtensible(key))return frozenStore(this)['delete'](key);
+ return $has(key, WEAK) && $has(key[WEAK], this._i) && delete key[WEAK][this._i];
+ },
+ // 23.3.3.4 WeakMap.prototype.has(key)
+ // 23.4.3.4 WeakSet.prototype.has(value)
+ has: function has(key){
+ if(!isObject(key))return false;
+ if(!isExtensible(key))return frozenStore(this).has(key);
+ return $has(key, WEAK) && $has(key[WEAK], this._i);
+ }
+ });
+ return C;
+ },
+ def: function(that, key, value){
+ if(!isExtensible(anObject(key))){
+ frozenStore(that).set(key, value);
+ } else {
+ $has(key, WEAK) || hide(key, WEAK, {});
+ key[WEAK][that._i] = value;
+ } return that;
+ },
+ frozenStore: frozenStore,
+ WEAK: WEAK
+ };
+
+/***/ },
+/* 149 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var weak = __webpack_require__(148);
+
+ // 23.4 WeakSet Objects
+ __webpack_require__(145)('WeakSet', function(get){
+ return function WeakSet(){ return get(this, arguments.length > 0 ? arguments[0] : undefined); };
+ }, {
+ // 23.4.3.1 WeakSet.prototype.add(value)
+ add: function add(value){
+ return weak.def(this, value, true);
+ }
+ }, weak, false, true);
+
+/***/ },
+/* 150 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.1 Reflect.apply(target, thisArgument, argumentsList)
+ var $export = __webpack_require__(3)
+ , _apply = Function.apply
+ , anObject = __webpack_require__(20);
+
+ $export($export.S, 'Reflect', {
+ apply: function apply(target, thisArgument, argumentsList){
+ return _apply.call(target, thisArgument, anObject(argumentsList));
+ }
+ });
+
+/***/ },
+/* 151 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.2 Reflect.construct(target, argumentsList [, newTarget])
+ var $ = __webpack_require__(2)
+ , $export = __webpack_require__(3)
+ , aFunction = __webpack_require__(13)
+ , anObject = __webpack_require__(20)
+ , isObject = __webpack_require__(16)
+ , bind = Function.bind || __webpack_require__(5).Function.prototype.bind;
+
+ // MS Edge supports only 2 arguments
+ // FF Nightly sets third argument as `new.target`, but does not create `this` from it
+ $export($export.S + $export.F * __webpack_require__(9)(function(){
+ function F(){}
+ return !(Reflect.construct(function(){}, [], F) instanceof F);
+ }), 'Reflect', {
+ construct: function construct(Target, args /*, newTarget*/){
+ aFunction(Target);
+ anObject(args);
+ var newTarget = arguments.length < 3 ? Target : aFunction(arguments[2]);
+ if(Target == newTarget){
+ // w/o altered newTarget, optimization for 0-4 arguments
+ switch(args.length){
+ case 0: return new Target;
+ case 1: return new Target(args[0]);
+ case 2: return new Target(args[0], args[1]);
+ case 3: return new Target(args[0], args[1], args[2]);
+ case 4: return new Target(args[0], args[1], args[2], args[3]);
+ }
+ // w/o altered newTarget, lot of arguments case
+ var $args = [null];
+ $args.push.apply($args, args);
+ return new (bind.apply(Target, $args));
+ }
+ // with altered newTarget, not support built-in constructors
+ var proto = newTarget.prototype
+ , instance = $.create(isObject(proto) ? proto : Object.prototype)
+ , result = Function.apply.call(Target, instance, args);
+ return isObject(result) ? result : instance;
+ }
+ });
+
+/***/ },
+/* 152 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.3 Reflect.defineProperty(target, propertyKey, attributes)
+ var $ = __webpack_require__(2)
+ , $export = __webpack_require__(3)
+ , anObject = __webpack_require__(20);
+
+ // MS Edge has broken Reflect.defineProperty - throwing instead of returning false
+ $export($export.S + $export.F * __webpack_require__(9)(function(){
+ Reflect.defineProperty($.setDesc({}, 1, {value: 1}), 1, {value: 2});
+ }), 'Reflect', {
+ defineProperty: function defineProperty(target, propertyKey, attributes){
+ anObject(target);
+ try {
+ $.setDesc(target, propertyKey, attributes);
+ return true;
+ } catch(e){
+ return false;
+ }
+ }
+ });
+
+/***/ },
+/* 153 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.4 Reflect.deleteProperty(target, propertyKey)
+ var $export = __webpack_require__(3)
+ , getDesc = __webpack_require__(2).getDesc
+ , anObject = __webpack_require__(20);
+
+ $export($export.S, 'Reflect', {
+ deleteProperty: function deleteProperty(target, propertyKey){
+ var desc = getDesc(anObject(target), propertyKey);
+ return desc && !desc.configurable ? false : delete target[propertyKey];
+ }
+ });
+
+/***/ },
+/* 154 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // 26.1.5 Reflect.enumerate(target)
+ var $export = __webpack_require__(3)
+ , anObject = __webpack_require__(20);
+ var Enumerate = function(iterated){
+ this._t = anObject(iterated); // target
+ this._i = 0; // next index
+ var keys = this._k = [] // keys
+ , key;
+ for(key in iterated)keys.push(key);
+ };
+ __webpack_require__(110)(Enumerate, 'Object', function(){
+ var that = this
+ , keys = that._k
+ , key;
+ do {
+ if(that._i >= keys.length)return {value: undefined, done: true};
+ } while(!((key = keys[that._i++]) in that._t));
+ return {value: key, done: false};
+ });
+
+ $export($export.S, 'Reflect', {
+ enumerate: function enumerate(target){
+ return new Enumerate(target);
+ }
+ });
+
+/***/ },
+/* 155 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.6 Reflect.get(target, propertyKey [, receiver])
+ var $ = __webpack_require__(2)
+ , has = __webpack_require__(17)
+ , $export = __webpack_require__(3)
+ , isObject = __webpack_require__(16)
+ , anObject = __webpack_require__(20);
+
+ function get(target, propertyKey/*, receiver*/){
+ var receiver = arguments.length < 3 ? target : arguments[2]
+ , desc, proto;
+ if(anObject(target) === receiver)return target[propertyKey];
+ if(desc = $.getDesc(target, propertyKey))return has(desc, 'value')
+ ? desc.value
+ : desc.get !== undefined
+ ? desc.get.call(receiver)
+ : undefined;
+ if(isObject(proto = $.getProto(target)))return get(proto, propertyKey, receiver);
+ }
+
+ $export($export.S, 'Reflect', {get: get});
+
+/***/ },
+/* 156 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.7 Reflect.getOwnPropertyDescriptor(target, propertyKey)
+ var $ = __webpack_require__(2)
+ , $export = __webpack_require__(3)
+ , anObject = __webpack_require__(20);
+
+ $export($export.S, 'Reflect', {
+ getOwnPropertyDescriptor: function getOwnPropertyDescriptor(target, propertyKey){
+ return $.getDesc(anObject(target), propertyKey);
+ }
+ });
+
+/***/ },
+/* 157 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.8 Reflect.getPrototypeOf(target)
+ var $export = __webpack_require__(3)
+ , getProto = __webpack_require__(2).getProto
+ , anObject = __webpack_require__(20);
+
+ $export($export.S, 'Reflect', {
+ getPrototypeOf: function getPrototypeOf(target){
+ return getProto(anObject(target));
+ }
+ });
+
+/***/ },
+/* 158 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.9 Reflect.has(target, propertyKey)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Reflect', {
+ has: function has(target, propertyKey){
+ return propertyKey in target;
+ }
+ });
+
+/***/ },
+/* 159 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.10 Reflect.isExtensible(target)
+ var $export = __webpack_require__(3)
+ , anObject = __webpack_require__(20)
+ , $isExtensible = Object.isExtensible;
+
+ $export($export.S, 'Reflect', {
+ isExtensible: function isExtensible(target){
+ anObject(target);
+ return $isExtensible ? $isExtensible(target) : true;
+ }
+ });
+
+/***/ },
+/* 160 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.11 Reflect.ownKeys(target)
+ var $export = __webpack_require__(3);
+
+ $export($export.S, 'Reflect', {ownKeys: __webpack_require__(161)});
+
+/***/ },
+/* 161 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // all object keys, includes non-enumerable and symbols
+ var $ = __webpack_require__(2)
+ , anObject = __webpack_require__(20)
+ , Reflect = __webpack_require__(4).Reflect;
+ module.exports = Reflect && Reflect.ownKeys || function ownKeys(it){
+ var keys = $.getNames(anObject(it))
+ , getSymbols = $.getSymbols;
+ return getSymbols ? keys.concat(getSymbols(it)) : keys;
+ };
+
+/***/ },
+/* 162 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.12 Reflect.preventExtensions(target)
+ var $export = __webpack_require__(3)
+ , anObject = __webpack_require__(20)
+ , $preventExtensions = Object.preventExtensions;
+
+ $export($export.S, 'Reflect', {
+ preventExtensions: function preventExtensions(target){
+ anObject(target);
+ try {
+ if($preventExtensions)$preventExtensions(target);
+ return true;
+ } catch(e){
+ return false;
+ }
+ }
+ });
+
+/***/ },
+/* 163 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.13 Reflect.set(target, propertyKey, V [, receiver])
+ var $ = __webpack_require__(2)
+ , has = __webpack_require__(17)
+ , $export = __webpack_require__(3)
+ , createDesc = __webpack_require__(7)
+ , anObject = __webpack_require__(20)
+ , isObject = __webpack_require__(16);
+
+ function set(target, propertyKey, V/*, receiver*/){
+ var receiver = arguments.length < 4 ? target : arguments[3]
+ , ownDesc = $.getDesc(anObject(target), propertyKey)
+ , existingDescriptor, proto;
+ if(!ownDesc){
+ if(isObject(proto = $.getProto(target))){
+ return set(proto, propertyKey, V, receiver);
+ }
+ ownDesc = createDesc(0);
+ }
+ if(has(ownDesc, 'value')){
+ if(ownDesc.writable === false || !isObject(receiver))return false;
+ existingDescriptor = $.getDesc(receiver, propertyKey) || createDesc(0);
+ existingDescriptor.value = V;
+ $.setDesc(receiver, propertyKey, existingDescriptor);
+ return true;
+ }
+ return ownDesc.set === undefined ? false : (ownDesc.set.call(receiver, V), true);
+ }
+
+ $export($export.S, 'Reflect', {set: set});
+
+/***/ },
+/* 164 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // 26.1.14 Reflect.setPrototypeOf(target, proto)
+ var $export = __webpack_require__(3)
+ , setProto = __webpack_require__(45);
+
+ if(setProto)$export($export.S, 'Reflect', {
+ setPrototypeOf: function setPrototypeOf(target, proto){
+ setProto.check(target, proto);
+ try {
+ setProto.set(target, proto);
+ return true;
+ } catch(e){
+ return false;
+ }
+ }
+ });
+
+/***/ },
+/* 165 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , $includes = __webpack_require__(33)(true);
+
+ $export($export.P, 'Array', {
+ // https://github.com/domenic/Array.prototype.includes
+ includes: function includes(el /*, fromIndex = 0 */){
+ return $includes(this, el, arguments.length > 1 ? arguments[1] : undefined);
+ }
+ });
+
+ __webpack_require__(118)('includes');
+
+/***/ },
+/* 166 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // https://github.com/mathiasbynens/String.prototype.at
+ var $export = __webpack_require__(3)
+ , $at = __webpack_require__(98)(true);
+
+ $export($export.P, 'String', {
+ at: function at(pos){
+ return $at(this, pos);
+ }
+ });
+
+/***/ },
+/* 167 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , $pad = __webpack_require__(168);
+
+ $export($export.P, 'String', {
+ padLeft: function padLeft(maxLength /*, fillString = ' ' */){
+ return $pad(this, maxLength, arguments.length > 1 ? arguments[1] : undefined, true);
+ }
+ });
+
+/***/ },
+/* 168 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // https://github.com/ljharb/proposal-string-pad-left-right
+ var toLength = __webpack_require__(27)
+ , repeat = __webpack_require__(105)
+ , defined = __webpack_require__(22);
+
+ module.exports = function(that, maxLength, fillString, left){
+ var S = String(defined(that))
+ , stringLength = S.length
+ , fillStr = fillString === undefined ? ' ' : String(fillString)
+ , intMaxLength = toLength(maxLength);
+ if(intMaxLength <= stringLength)return S;
+ if(fillStr == '')fillStr = ' ';
+ var fillLen = intMaxLength - stringLength
+ , stringFiller = repeat.call(fillStr, Math.ceil(fillLen / fillStr.length));
+ if(stringFiller.length > fillLen)stringFiller = stringFiller.slice(0, fillLen);
+ return left ? stringFiller + S : S + stringFiller;
+ };
+
+/***/ },
+/* 169 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var $export = __webpack_require__(3)
+ , $pad = __webpack_require__(168);
+
+ $export($export.P, 'String', {
+ padRight: function padRight(maxLength /*, fillString = ' ' */){
+ return $pad(this, maxLength, arguments.length > 1 ? arguments[1] : undefined, false);
+ }
+ });
+
+/***/ },
+/* 170 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // https://github.com/sebmarkbage/ecmascript-string-left-right-trim
+ __webpack_require__(63)('trimLeft', function($trim){
+ return function trimLeft(){
+ return $trim(this, 1);
+ };
+ });
+
+/***/ },
+/* 171 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ // https://github.com/sebmarkbage/ecmascript-string-left-right-trim
+ __webpack_require__(63)('trimRight', function($trim){
+ return function trimRight(){
+ return $trim(this, 2);
+ };
+ });
+
+/***/ },
+/* 172 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // https://github.com/benjamingr/RexExp.escape
+ var $export = __webpack_require__(3)
+ , $re = __webpack_require__(173)(/[\\^$*+?.()|[\]{}]/g, '\\$&');
+
+ $export($export.S, 'RegExp', {escape: function escape(it){ return $re(it); }});
+
+
+/***/ },
+/* 173 */
+/***/ function(module, exports) {
+
+ module.exports = function(regExp, replace){
+ var replacer = replace === Object(replace) ? function(part){
+ return replace[part];
+ } : replace;
+ return function(it){
+ return String(it).replace(regExp, replacer);
+ };
+ };
+
+/***/ },
+/* 174 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // https://gist.github.com/WebReflection/9353781
+ var $ = __webpack_require__(2)
+ , $export = __webpack_require__(3)
+ , ownKeys = __webpack_require__(161)
+ , toIObject = __webpack_require__(23)
+ , createDesc = __webpack_require__(7);
+
+ $export($export.S, 'Object', {
+ getOwnPropertyDescriptors: function getOwnPropertyDescriptors(object){
+ var O = toIObject(object)
+ , setDesc = $.setDesc
+ , getDesc = $.getDesc
+ , keys = ownKeys(O)
+ , result = {}
+ , i = 0
+ , key, D;
+ while(keys.length > i){
+ D = getDesc(O, key = keys[i++]);
+ if(key in result)setDesc(result, key, createDesc(0, D));
+ else result[key] = D;
+ } return result;
+ }
+ });
+
+/***/ },
+/* 175 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // http://goo.gl/XkBrjD
+ var $export = __webpack_require__(3)
+ , $values = __webpack_require__(176)(false);
+
+ $export($export.S, 'Object', {
+ values: function values(it){
+ return $values(it);
+ }
+ });
+
+/***/ },
+/* 176 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $ = __webpack_require__(2)
+ , toIObject = __webpack_require__(23)
+ , isEnum = $.isEnum;
+ module.exports = function(isEntries){
+ return function(it){
+ var O = toIObject(it)
+ , keys = $.getKeys(O)
+ , length = keys.length
+ , i = 0
+ , result = []
+ , key;
+ while(length > i)if(isEnum.call(O, key = keys[i++])){
+ result.push(isEntries ? [key, O[key]] : O[key]);
+ } return result;
+ };
+ };
+
+/***/ },
+/* 177 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // http://goo.gl/XkBrjD
+ var $export = __webpack_require__(3)
+ , $entries = __webpack_require__(176)(true);
+
+ $export($export.S, 'Object', {
+ entries: function entries(it){
+ return $entries(it);
+ }
+ });
+
+/***/ },
+/* 178 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // https://github.com/DavidBruant/Map-Set.prototype.toJSON
+ var $export = __webpack_require__(3);
+
+ $export($export.P, 'Map', {toJSON: __webpack_require__(179)('Map')});
+
+/***/ },
+/* 179 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // https://github.com/DavidBruant/Map-Set.prototype.toJSON
+ var forOf = __webpack_require__(138)
+ , classof = __webpack_require__(47);
+ module.exports = function(NAME){
+ return function toJSON(){
+ if(classof(this) != NAME)throw TypeError(NAME + "#toJSON isn't generic");
+ var arr = [];
+ forOf(this, false, arr.push, arr);
+ return arr;
+ };
+ };
+
+/***/ },
+/* 180 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // https://github.com/DavidBruant/Map-Set.prototype.toJSON
+ var $export = __webpack_require__(3);
+
+ $export($export.P, 'Set', {toJSON: __webpack_require__(179)('Set')});
+
+/***/ },
+/* 181 */
+/***/ function(module, exports, __webpack_require__) {
+
+ var $export = __webpack_require__(3)
+ , $task = __webpack_require__(141);
+ $export($export.G + $export.B, {
+ setImmediate: $task.set,
+ clearImmediate: $task.clear
+ });
+
+/***/ },
+/* 182 */
+/***/ function(module, exports, __webpack_require__) {
+
+ __webpack_require__(117);
+ var global = __webpack_require__(4)
+ , hide = __webpack_require__(6)
+ , Iterators = __webpack_require__(109)
+ , ITERATOR = __webpack_require__(31)('iterator')
+ , NL = global.NodeList
+ , HTC = global.HTMLCollection
+ , NLProto = NL && NL.prototype
+ , HTCProto = HTC && HTC.prototype
+ , ArrayValues = Iterators.NodeList = Iterators.HTMLCollection = Iterators.Array;
+ if(NLProto && !NLProto[ITERATOR])hide(NLProto, ITERATOR, ArrayValues);
+ if(HTCProto && !HTCProto[ITERATOR])hide(HTCProto, ITERATOR, ArrayValues);
+
+/***/ },
+/* 183 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // ie9- setTimeout & setInterval additional parameters fix
+ var global = __webpack_require__(4)
+ , $export = __webpack_require__(3)
+ , invoke = __webpack_require__(19)
+ , partial = __webpack_require__(184)
+ , navigator = global.navigator
+ , MSIE = !!navigator && /MSIE .\./.test(navigator.userAgent); // <- dirty ie9- check
+ var wrap = function(set){
+ return MSIE ? function(fn, time /*, ...args */){
+ return set(invoke(
+ partial,
+ [].slice.call(arguments, 2),
+ typeof fn == 'function' ? fn : Function(fn)
+ ), time);
+ } : set;
+ };
+ $export($export.G + $export.B + $export.F * MSIE, {
+ setTimeout: wrap(global.setTimeout),
+ setInterval: wrap(global.setInterval)
+ });
+
+/***/ },
+/* 184 */
+/***/ function(module, exports, __webpack_require__) {
+
+ 'use strict';
+ var path = __webpack_require__(185)
+ , invoke = __webpack_require__(19)
+ , aFunction = __webpack_require__(13);
+ module.exports = function(/* ...pargs */){
+ var fn = aFunction(this)
+ , length = arguments.length
+ , pargs = Array(length)
+ , i = 0
+ , _ = path._
+ , holder = false;
+ while(length > i)if((pargs[i] = arguments[i++]) === _)holder = true;
+ return function(/* ...args */){
+ var that = this
+ , $$ = arguments
+ , $$len = $$.length
+ , j = 0, k = 0, args;
+ if(!holder && !$$len)return invoke(fn, pargs, that);
+ args = pargs.slice();
+ if(holder)for(;length > j; j++)if(args[j] === _)args[j] = $$[k++];
+ while($$len > k)args.push($$[k++]);
+ return invoke(fn, args, that);
+ };
+ };
+
+/***/ },
+/* 185 */
+/***/ function(module, exports, __webpack_require__) {
+
+ module.exports = __webpack_require__(4);
+
+/***/ },
+/* 186 */
+/***/ function(module, exports, __webpack_require__) {
+
+ // JavaScript 1.6 / Strawman array statics shim
+ var $ = __webpack_require__(2)
+ , $export = __webpack_require__(3)
+ , $ctx = __webpack_require__(12)
+ , $Array = __webpack_require__(5).Array || Array
+ , statics = {};
+ var setStatics = function(keys, length){
+ $.each.call(keys.split(','), function(key){
+ if(length == undefined && key in $Array)statics[key] = $Array[key];
+ else if(key in [])statics[key] = $ctx(Function.call, [][key], length);
+ });
+ };
+ setStatics('pop,reverse,shift,keys,values,entries', 1);
+ setStatics('indexOf,every,some,forEach,map,filter,find,findIndex,includes', 3);
+ setStatics('join,slice,concat,push,splice,unshift,sort,lastIndexOf,' +
+ 'reduce,reduceRight,copyWithin,fill');
+ $export($export.S, 'Array', statics);
+
+/***/ }
+/******/ ]);
+// CommonJS export
+if(typeof module != 'undefined' && module.exports)module.exports = __e;
+// RequireJS export
+else if(typeof define == 'function' && define.amd)define(function(){return __e});
+// Export to global object
+else __g.core = __e;
+}(1, 1);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/shim.min.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/shim.min.js
new file mode 100644
index 00000000..bdfd6632
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/shim.min.js
@@ -0,0 +1,9 @@
+/**
+ * core-js 1.2.7
+ * https://github.com/zloirock/core-js
+ * License: http://rock.mit-license.org
+ * © 2016 Denis Pushkarev
+ */
+!function(b,c,a){"use strict";!function(b){function __webpack_require__(c){if(a[c])return a[c].exports;var d=a[c]={exports:{},id:c,loaded:!1};return b[c].call(d.exports,d,d.exports,__webpack_require__),d.loaded=!0,d.exports}var a={};return __webpack_require__.m=b,__webpack_require__.c=a,__webpack_require__.p="",__webpack_require__(0)}([function(b,c,a){a(1),a(34),a(40),a(42),a(44),a(46),a(48),a(50),a(51),a(52),a(53),a(54),a(55),a(56),a(57),a(58),a(59),a(60),a(61),a(64),a(65),a(66),a(68),a(69),a(70),a(71),a(72),a(73),a(74),a(76),a(77),a(78),a(80),a(81),a(82),a(84),a(85),a(86),a(87),a(88),a(89),a(90),a(91),a(92),a(93),a(94),a(95),a(96),a(97),a(99),a(103),a(104),a(106),a(107),a(111),a(116),a(117),a(120),a(122),a(124),a(126),a(127),a(128),a(130),a(131),a(133),a(134),a(135),a(136),a(143),a(146),a(147),a(149),a(150),a(151),a(152),a(153),a(154),a(155),a(156),a(157),a(158),a(159),a(160),a(162),a(163),a(164),a(165),a(166),a(167),a(169),a(170),a(171),a(172),a(174),a(175),a(177),a(178),a(180),a(181),a(182),a(183),b.exports=a(186)},function(S,R,b){var r,d=b(2),c=b(3),x=b(8),O=b(7),o=b(14),E=b(15),n=b(17),N=b(18),J=b(19),j=b(9),p=b(20),v=b(13),I=b(16),Q=b(21),y=b(23),K=b(25),w=b(26),h=b(27),s=b(24),m=b(11)("__proto__"),g=b(28),A=b(33)(!1),B=Object.prototype,C=Array.prototype,k=C.slice,M=C.join,F=d.setDesc,L=d.getDesc,q=d.setDescs,u={};x||(r=!j(function(){return 7!=F(E("div"),"a",{get:function(){return 7}}).a}),d.setDesc=function(b,c,a){if(r)try{return F(b,c,a)}catch(d){}if("get"in a||"set"in a)throw TypeError("Accessors not supported!");return"value"in a&&(p(b)[c]=a.value),b},d.getDesc=function(a,b){if(r)try{return L(a,b)}catch(c){}return n(a,b)?O(!B.propertyIsEnumerable.call(a,b),a[b]):void 0},d.setDescs=q=function(a,b){p(a);for(var c,e=d.getKeys(b),g=e.length,f=0;g>f;)d.setDesc(a,c=e[f++],b[c]);return a}),c(c.S+c.F*!x,"Object",{getOwnPropertyDescriptor:d.getDesc,defineProperty:d.setDesc,defineProperties:q});var i="constructor,hasOwnProperty,isPrototypeOf,propertyIsEnumerable,toLocaleString,toString,valueOf".split(","),H=i.concat("length","prototype"),G=i.length,l=function(){var a,b=E("iframe"),c=G,d=">";for(b.style.display="none",o.appendChild(b),b.src="javascript:",a=b.contentWindow.document,a.open(),a.write("f;)n(e,c=a[f++])&&(~A(d,c)||d.push(c));return d}},t=function(){};c(c.S,"Object",{getPrototypeOf:d.getProto=d.getProto||function(a){return a=Q(a),n(a,m)?a[m]:"function"==typeof a.constructor&&a instanceof a.constructor?a.constructor.prototype:a instanceof Object?B:null},getOwnPropertyNames:d.getNames=d.getNames||D(H,H.length,!0),create:d.create=d.create||function(c,d){var b;return null!==c?(t.prototype=p(c),b=new t,t.prototype=null,b[m]=c):b=l(),d===a?b:q(b,d)},keys:d.getKeys=d.getKeys||D(i,G,!1)});var P=function(d,a,e){if(!(a in u)){for(var c=[],b=0;a>b;b++)c[b]="a["+b+"]";u[a]=Function("F,a","return new F("+c.join(",")+")")}return u[a](d,e)};c(c.P,"Function",{bind:function bind(c){var a=v(this),d=k.call(arguments,1),b=function(){var e=d.concat(k.call(arguments));return this instanceof b?P(a,e.length,e):J(a,e,c)};return I(a.prototype)&&(b.prototype=a.prototype),b}}),c(c.P+c.F*j(function(){o&&k.call(o)}),"Array",{slice:function(f,b){var d=h(this.length),g=N(this);if(b=b===a?d:b,"Array"==g)return k.call(this,f,b);for(var e=w(f,d),l=w(b,d),i=h(l-e),j=Array(i),c=0;i>c;c++)j[c]="String"==g?this.charAt(e+c):this[e+c];return j}}),c(c.P+c.F*(s!=Object),"Array",{join:function join(b){return M.call(s(this),b===a?",":b)}}),c(c.S,"Array",{isArray:b(30)});var z=function(a){return function(g,d){v(g);var c=s(this),e=h(c.length),b=a?e-1:0,f=a?-1:1;if(arguments.length<2)for(;;){if(b in c){d=c[b],b+=f;break}if(b+=f,a?0>b:b>=e)throw TypeError("Reduce of empty array with no initial value")}for(;a?b>=0:e>b;b+=f)b in c&&(d=g(d,c[b],b,this));return d}},f=function(a){return function(b){return a(this,b,arguments[1])}};c(c.P,"Array",{forEach:d.each=d.each||f(g(0)),map:f(g(1)),filter:f(g(2)),some:f(g(3)),every:f(g(4)),reduce:z(!1),reduceRight:z(!0),indexOf:f(A),lastIndexOf:function(d,e){var b=y(this),c=h(b.length),a=c-1;for(arguments.length>1&&(a=Math.min(a,K(e))),0>a&&(a=h(c+a));a>=0;a--)if(a in b&&b[a]===d)return a;return-1}}),c(c.S,"Date",{now:function(){return+new Date}});var e=function(a){return a>9?a:"0"+a};c(c.P+c.F*(j(function(){return"0385-07-25T07:06:39.999Z"!=new Date(-5e13-1).toISOString()})||!j(function(){new Date(NaN).toISOString()})),"Date",{toISOString:function toISOString(){if(!isFinite(this))throw RangeError("Invalid time value");var a=this,b=a.getUTCFullYear(),c=a.getUTCMilliseconds(),d=0>b?"-":b>9999?"+":"";return d+("00000"+Math.abs(b)).slice(d?-6:-4)+"-"+e(a.getUTCMonth()+1)+"-"+e(a.getUTCDate())+"T"+e(a.getUTCHours())+":"+e(a.getUTCMinutes())+":"+e(a.getUTCSeconds())+"."+(c>99?c:"0"+e(c))+"Z"}})},function(b,c){var a=Object;b.exports={create:a.create,getProto:a.getPrototypeOf,isEnum:{}.propertyIsEnumerable,getDesc:a.getOwnPropertyDescriptor,setDesc:a.defineProperty,setDescs:a.defineProperties,getKeys:a.keys,getNames:a.getOwnPropertyNames,getSymbols:a.getOwnPropertySymbols,each:[].forEach}},function(g,j,c){var b=c(4),d=c(5),h=c(6),i=c(10),f=c(12),e="prototype",a=function(k,j,o){var g,m,c,s,v=k&a.F,p=k&a.G,u=k&a.S,r=k&a.P,t=k&a.B,l=p?b:u?b[j]||(b[j]={}):(b[j]||{})[e],n=p?d:d[j]||(d[j]={}),q=n[e]||(n[e]={});p&&(o=j);for(g in o)m=!v&&l&&g in l,c=(m?l:o)[g],s=t&&m?f(c,b):r&&"function"==typeof c?f(Function.call,c):c,l&&!m&&i(l,g,c),n[g]!=c&&h(n,g,s),r&&q[g]!=c&&(q[g]=c)};b.core=d,a.F=1,a.G=2,a.S=4,a.P=8,a.B=16,a.W=32,g.exports=a},function(a,d){var b=a.exports="undefined"!=typeof window&&window.Math==Math?window:"undefined"!=typeof self&&self.Math==Math?self:Function("return this")();"number"==typeof c&&(c=b)},function(a,d){var c=a.exports={version:"1.2.6"};"number"==typeof b&&(b=c)},function(b,e,a){var c=a(2),d=a(7);b.exports=a(8)?function(a,b,e){return c.setDesc(a,b,d(1,e))}:function(a,b,c){return a[b]=c,a}},function(a,b){a.exports=function(a,b){return{enumerable:!(1&a),configurable:!(2&a),writable:!(4&a),value:b}}},function(a,c,b){a.exports=!b(9)(function(){return 7!=Object.defineProperty({},"a",{get:function(){return 7}}).a})},function(a,b){a.exports=function(a){try{return!!a()}catch(b){return!0}}},function(f,i,a){var g=a(4),b=a(6),c=a(11)("src"),d="toString",e=Function[d],h=(""+e).split(d);a(5).inspectSource=function(a){return e.call(a)},(f.exports=function(e,a,d,f){"function"==typeof d&&(d.hasOwnProperty(c)||b(d,c,e[a]?""+e[a]:h.join(String(a))),d.hasOwnProperty("name")||b(d,"name",a)),e===g?e[a]=d:(f||delete e[a],b(e,a,d))})(Function.prototype,d,function toString(){return"function"==typeof this&&this[c]||e.call(this)})},function(b,e){var c=0,d=Math.random();b.exports=function(b){return"Symbol(".concat(b===a?"":b,")_",(++c+d).toString(36))}},function(b,e,c){var d=c(13);b.exports=function(b,c,e){if(d(b),c===a)return b;switch(e){case 1:return function(a){return b.call(c,a)};case 2:return function(a,d){return b.call(c,a,d)};case 3:return function(a,d,e){return b.call(c,a,d,e)}}return function(){return b.apply(c,arguments)}}},function(a,b){a.exports=function(a){if("function"!=typeof a)throw TypeError(a+" is not a function!");return a}},function(a,c,b){a.exports=b(4).document&&document.documentElement},function(d,f,b){var c=b(16),a=b(4).document,e=c(a)&&c(a.createElement);d.exports=function(b){return e?a.createElement(b):{}}},function(a,b){a.exports=function(a){return"object"==typeof a?null!==a:"function"==typeof a}},function(a,c){var b={}.hasOwnProperty;a.exports=function(a,c){return b.call(a,c)}},function(a,c){var b={}.toString;a.exports=function(a){return b.call(a).slice(8,-1)}},function(b,c){b.exports=function(c,b,d){var e=d===a;switch(b.length){case 0:return e?c():c.call(d);case 1:return e?c(b[0]):c.call(d,b[0]);case 2:return e?c(b[0],b[1]):c.call(d,b[0],b[1]);case 3:return e?c(b[0],b[1],b[2]):c.call(d,b[0],b[1],b[2]);case 4:return e?c(b[0],b[1],b[2],b[3]):c.call(d,b[0],b[1],b[2],b[3])}return c.apply(d,b)}},function(a,d,b){var c=b(16);a.exports=function(a){if(!c(a))throw TypeError(a+" is not an object!");return a}},function(a,d,b){var c=b(22);a.exports=function(a){return Object(c(a))}},function(b,c){b.exports=function(b){if(b==a)throw TypeError("Can't call method on "+b);return b}},function(b,e,a){var c=a(24),d=a(22);b.exports=function(a){return c(d(a))}},function(a,d,b){var c=b(18);a.exports=Object("z").propertyIsEnumerable(0)?Object:function(a){return"String"==c(a)?a.split(""):Object(a)}},function(a,d){var b=Math.ceil,c=Math.floor;a.exports=function(a){return isNaN(a=+a)?0:(a>0?c:b)(a)}},function(a,f,b){var c=b(25),d=Math.max,e=Math.min;a.exports=function(a,b){return a=c(a),0>a?d(a+b,0):e(a,b)}},function(a,e,b){var c=b(25),d=Math.min;a.exports=function(a){return a>0?d(c(a),9007199254740991):0}},function(d,i,b){var e=b(12),f=b(24),g=b(21),h=b(27),c=b(29);d.exports=function(b){var i=1==b,k=2==b,l=3==b,d=4==b,j=6==b,m=5==b||j;return function(p,v,x){for(var o,r,u=g(p),s=f(u),w=e(v,x,3),t=h(s.length),n=0,q=i?c(p,t):k?c(p,0):a;t>n;n++)if((m||n in s)&&(o=s[n],r=w(o,n,u),b))if(i)q[n]=r;else if(r)switch(b){case 3:return!0;case 5:return o;case 6:return n;case 2:q.push(o)}else if(d)return!1;return j?-1:l||d?d:q}}},function(d,g,b){var e=b(16),c=b(30),f=b(31)("species");d.exports=function(d,g){var b;return c(d)&&(b=d.constructor,"function"!=typeof b||b!==Array&&!c(b.prototype)||(b=a),e(b)&&(b=b[f],null===b&&(b=a))),new(b===a?Array:b)(g)}},function(a,d,b){var c=b(18);a.exports=Array.isArray||function(a){return"Array"==c(a)}},function(d,f,a){var c=a(32)("wks"),e=a(11),b=a(4).Symbol;d.exports=function(a){return c[a]||(c[a]=b&&b[a]||(b||e)("Symbol."+a))}},function(d,f,e){var a=e(4),b="__core-js_shared__",c=a[b]||(a[b]={});d.exports=function(a){return c[a]||(c[a]={})}},function(b,f,a){var c=a(23),d=a(27),e=a(26);b.exports=function(a){return function(j,g,k){var h,f=c(j),i=d(f.length),b=e(k,i);if(a&&g!=g){for(;i>b;)if(h=f[b++],h!=h)return!0}else for(;i>b;b++)if((a||b in f)&&f[b]===g)return a||b;return!a&&-1}}},function(W,V,b){var e=b(2),x=b(4),d=b(17),w=b(8),f=b(3),G=b(10),H=b(9),J=b(32),s=b(35),S=b(11),A=b(31),R=b(36),C=b(37),Q=b(38),P=b(30),O=b(20),p=b(23),v=b(7),I=e.getDesc,i=e.setDesc,k=e.create,z=C.get,g=x.Symbol,l=x.JSON,m=l&&l.stringify,n=!1,c=A("_hidden"),N=e.isEnum,o=J("symbol-registry"),h=J("symbols"),q="function"==typeof g,j=Object.prototype,y=w&&H(function(){return 7!=k(i({},"a",{get:function(){return i(this,"a",{value:7}).a}})).a})?function(c,a,d){var b=I(j,a);b&&delete j[a],i(c,a,d),b&&c!==j&&i(j,a,b)}:i,L=function(a){var b=h[a]=k(g.prototype);return b._k=a,w&&n&&y(j,a,{configurable:!0,set:function(b){d(this,c)&&d(this[c],a)&&(this[c][a]=!1),y(this,a,v(1,b))}}),b},r=function(a){return"symbol"==typeof a},t=function defineProperty(a,b,e){return e&&d(h,b)?(e.enumerable?(d(a,c)&&a[c][b]&&(a[c][b]=!1),e=k(e,{enumerable:v(0,!1)})):(d(a,c)||i(a,c,v(1,{})),a[c][b]=!0),y(a,b,e)):i(a,b,e)},u=function defineProperties(a,b){O(a);for(var c,d=Q(b=p(b)),e=0,f=d.length;f>e;)t(a,c=d[e++],b[c]);return a},F=function create(b,c){return c===a?k(b):u(k(b),c)},E=function propertyIsEnumerable(a){var b=N.call(this,a);return b||!d(this,a)||!d(h,a)||d(this,c)&&this[c][a]?b:!0},D=function getOwnPropertyDescriptor(a,b){var e=I(a=p(a),b);return!e||!d(h,b)||d(a,c)&&a[c][b]||(e.enumerable=!0),e},B=function getOwnPropertyNames(g){for(var a,b=z(p(g)),e=[],f=0;b.length>f;)d(h,a=b[f++])||a==c||e.push(a);return e},M=function getOwnPropertySymbols(f){for(var a,b=z(p(f)),c=[],e=0;b.length>e;)d(h,a=b[e++])&&c.push(h[a]);return c},T=function stringify(e){if(e!==a&&!r(e)){for(var b,c,d=[e],f=1,g=arguments;g.length>f;)d.push(g[f++]);return b=d[1],"function"==typeof b&&(c=b),(c||!P(b))&&(b=function(b,a){return c&&(a=c.call(this,b,a)),r(a)?void 0:a}),d[1]=b,m.apply(l,d)}},U=H(function(){var a=g();return"[null]"!=m([a])||"{}"!=m({a:a})||"{}"!=m(Object(a))});q||(g=function Symbol(){if(r(this))throw TypeError("Symbol is not a constructor");return L(S(arguments.length>0?arguments[0]:a))},G(g.prototype,"toString",function toString(){return this._k}),r=function(a){return a instanceof g},e.create=F,e.isEnum=E,e.getDesc=D,e.setDesc=t,e.setDescs=u,e.getNames=C.get=B,e.getSymbols=M,w&&!b(39)&&G(j,"propertyIsEnumerable",E,!0));var K={"for":function(a){return d(o,a+="")?o[a]:o[a]=g(a)},keyFor:function keyFor(a){return R(o,a)},useSetter:function(){n=!0},useSimple:function(){n=!1}};e.each.call("hasInstance,isConcatSpreadable,iterator,match,replace,search,species,split,toPrimitive,toStringTag,unscopables".split(","),function(a){var b=A(a);K[a]=q?b:L(b)}),n=!0,f(f.G+f.W,{Symbol:g}),f(f.S,"Symbol",K),f(f.S+f.F*!q,"Object",{create:F,defineProperty:t,defineProperties:u,getOwnPropertyDescriptor:D,getOwnPropertyNames:B,getOwnPropertySymbols:M}),l&&f(f.S+f.F*(!q||U),"JSON",{stringify:T}),s(g,"Symbol"),s(Math,"Math",!0),s(x.JSON,"JSON",!0)},function(c,f,a){var d=a(2).setDesc,e=a(17),b=a(31)("toStringTag");c.exports=function(a,c,f){a&&!e(a=f?a:a.prototype,b)&&d(a,b,{configurable:!0,value:c})}},function(b,e,a){var c=a(2),d=a(23);b.exports=function(g,h){for(var a,b=d(g),e=c.getKeys(b),i=e.length,f=0;i>f;)if(b[a=e[f++]]===h)return a}},function(d,h,a){var e=a(23),b=a(2).getNames,f={}.toString,c="object"==typeof window&&Object.getOwnPropertyNames?Object.getOwnPropertyNames(window):[],g=function(a){try{return b(a)}catch(d){return c.slice()}};d.exports.get=function getOwnPropertyNames(a){return c&&"[object Window]"==f.call(a)?g(a):b(e(a))}},function(b,d,c){var a=c(2);b.exports=function(b){var c=a.getKeys(b),d=a.getSymbols;if(d)for(var e,f=d(b),h=a.isEnum,g=0;f.length>g;)h.call(b,e=f[g++])&&c.push(e);return c}},function(a,b){a.exports=!1},function(c,d,b){var a=b(3);a(a.S+a.F,"Object",{assign:b(41)})},function(c,f,a){var b=a(2),d=a(21),e=a(24);c.exports=a(9)(function(){var a=Object.assign,b={},c={},d=Symbol(),e="abcdefghijklmnopqrst";return b[d]=7,e.split("").forEach(function(a){c[a]=a}),7!=a({},b)[d]||Object.keys(a({},c)).join("")!=e})?function assign(n,q){for(var g=d(n),h=arguments,o=h.length,j=1,f=b.getKeys,l=b.getSymbols,m=b.isEnum;o>j;)for(var c,a=e(h[j++]),k=l?f(a).concat(l(a)):f(a),p=k.length,i=0;p>i;)m.call(a,c=k[i++])&&(g[c]=a[c]);return g}:Object.assign},function(c,d,a){var b=a(3);b(b.S,"Object",{is:a(43)})},function(a,b){a.exports=Object.is||function is(a,b){return a===b?0!==a||1/a===1/b:a!=a&&b!=b}},function(c,d,a){var b=a(3);b(b.S,"Object",{setPrototypeOf:a(45).set})},function(d,h,b){var e=b(2).getDesc,f=b(16),g=b(20),c=function(b,a){if(g(b),!f(a)&&null!==a)throw TypeError(a+": can't set as prototype!")};d.exports={set:Object.setPrototypeOf||("__proto__"in{}?function(f,a,d){try{d=b(12)(Function.call,e(Object.prototype,"__proto__").set,2),d(f,[]),a=!(f instanceof Array)}catch(g){a=!0}return function setPrototypeOf(b,e){return c(b,e),a?b.__proto__=e:d(b,e),b}}({},!1):a),check:c}},function(d,e,a){var c=a(47),b={};b[a(31)("toStringTag")]="z",b+""!="[object z]"&&a(10)(Object.prototype,"toString",function toString(){return"[object "+c(this)+"]"},!0)},function(d,g,c){var b=c(18),e=c(31)("toStringTag"),f="Arguments"==b(function(){return arguments}());d.exports=function(d){var c,g,h;return d===a?"Undefined":null===d?"Null":"string"==typeof(g=(c=Object(d))[e])?g:f?b(c):"Object"==(h=b(c))&&"function"==typeof c.callee?"Arguments":h}},function(c,d,a){var b=a(16);a(49)("freeze",function(a){return function freeze(c){return a&&b(c)?a(c):c}})},function(c,f,a){var b=a(3),d=a(5),e=a(9);c.exports=function(a,g){var c=(d.Object||{})[a]||Object[a],f={};f[a]=g(c),b(b.S+b.F*e(function(){c(1)}),"Object",f)}},function(c,d,a){var b=a(16);a(49)("seal",function(a){return function seal(c){return a&&b(c)?a(c):c}})},function(c,d,a){var b=a(16);a(49)("preventExtensions",function(a){return function preventExtensions(c){return a&&b(c)?a(c):c}})},function(c,d,a){var b=a(16);a(49)("isFrozen",function(a){return function isFrozen(c){return b(c)?a?a(c):!1:!0}})},function(c,d,a){var b=a(16);a(49)("isSealed",function(a){return function isSealed(c){return b(c)?a?a(c):!1:!0}})},function(c,d,a){var b=a(16);a(49)("isExtensible",function(a){return function isExtensible(c){return b(c)?a?a(c):!0:!1}})},function(c,d,a){var b=a(23);a(49)("getOwnPropertyDescriptor",function(a){return function getOwnPropertyDescriptor(c,d){return a(b(c),d)}})},function(c,d,a){var b=a(21);a(49)("getPrototypeOf",function(a){return function getPrototypeOf(c){return a(b(c))}})},function(c,d,a){var b=a(21);a(49)("keys",function(a){return function keys(c){return a(b(c))}})},function(b,c,a){a(49)("getOwnPropertyNames",function(){return a(37).get})},function(h,i,a){var c=a(2).setDesc,e=a(7),f=a(17),d=Function.prototype,g=/^\s*function ([^ (]*)/,b="name";b in d||a(8)&&c(d,b,{configurable:!0,get:function(){var a=(""+this).match(g),d=a?a[1]:"";return f(this,b)||c(this,b,e(5,d)),d}})},function(f,g,a){var b=a(2),c=a(16),d=a(31)("hasInstance"),e=Function.prototype;d in e||b.setDesc(e,d,{value:function(a){if("function"!=typeof this||!c(a))return!1;if(!c(this.prototype))return a instanceof this;for(;a=b.getProto(a);)if(this.prototype===a)return!0;return!1}})},function(q,p,b){var c=b(2),h=b(4),i=b(17),j=b(18),l=b(62),k=b(9),n=b(63).trim,d="Number",a=h[d],e=a,f=a.prototype,o=j(c.create(f))==d,m="trim"in String.prototype,g=function(i){var a=l(i,!1);if("string"==typeof a&&a.length>2){a=m?a.trim():n(a,3);var b,c,d,e=a.charCodeAt(0);if(43===e||45===e){if(b=a.charCodeAt(2),88===b||120===b)return NaN}else if(48===e){switch(a.charCodeAt(1)){case 66:case 98:c=2,d=49;break;case 79:case 111:c=8,d=55;break;default:return+a}for(var f,g=a.slice(2),h=0,j=g.length;j>h;h++)if(f=g.charCodeAt(h),48>f||f>d)return NaN;return parseInt(g,c)}}return+a};a(" 0o1")&&a("0b1")&&!a("+0x1")||(a=function Number(h){var c=arguments.length<1?0:h,b=this;return b instanceof a&&(o?k(function(){f.valueOf.call(b)}):j(b)!=d)?new e(g(c)):g(c)},c.each.call(b(8)?c.getNames(e):"MAX_VALUE,MIN_VALUE,NaN,NEGATIVE_INFINITY,POSITIVE_INFINITY,EPSILON,isFinite,isInteger,isNaN,isSafeInteger,MAX_SAFE_INTEGER,MIN_SAFE_INTEGER,parseFloat,parseInt,isInteger".split(","),function(b){i(e,b)&&!i(a,b)&&c.setDesc(a,b,c.getDesc(e,b))}),a.prototype=f,f.constructor=a,b(10)(h,d,a))},function(b,d,c){var a=c(16);b.exports=function(b,e){if(!a(b))return b;var c,d;if(e&&"function"==typeof(c=b.toString)&&!a(d=c.call(b)))return d;if("function"==typeof(c=b.valueOf)&&!a(d=c.call(b)))return d;if(!e&&"function"==typeof(c=b.toString)&&!a(d=c.call(b)))return d;throw TypeError("Can't convert object to primitive value")}},function(g,m,b){var c=b(3),h=b(22),i=b(9),d=" \n\f\r \u2028\u2029\ufeff",a="["+d+"]",f="
",j=RegExp("^"+a+a+"*"),k=RegExp(a+a+"*$"),e=function(a,e){var b={};b[a]=e(l),c(c.P+c.F*i(function(){return!!d[a]()||f[a]()!=f}),"String",b)},l=e.trim=function(a,b){return a=String(h(a)),1&b&&(a=a.replace(j,"")),2&b&&(a=a.replace(k,"")),a};g.exports=e},function(c,d,b){var a=b(3);a(a.S,"Number",{EPSILON:Math.pow(2,-52)})},function(d,e,a){var b=a(3),c=a(4).isFinite;b(b.S,"Number",{isFinite:function isFinite(a){return"number"==typeof a&&c(a)}})},function(c,d,a){var b=a(3);b(b.S,"Number",{isInteger:a(67)})},function(a,e,b){var c=b(16),d=Math.floor;a.exports=function isInteger(a){return!c(a)&&isFinite(a)&&d(a)===a}},function(c,d,b){var a=b(3);a(a.S,"Number",{isNaN:function isNaN(a){return a!=a}})},function(e,f,a){var b=a(3),c=a(67),d=Math.abs;b(b.S,"Number",{isSafeInteger:function isSafeInteger(a){return c(a)&&d(a)<=9007199254740991}})},function(c,d,b){var a=b(3);a(a.S,"Number",{MAX_SAFE_INTEGER:9007199254740991})},function(c,d,b){var a=b(3);a(a.S,"Number",{MIN_SAFE_INTEGER:-9007199254740991})},function(c,d,b){var a=b(3);a(a.S,"Number",{parseFloat:parseFloat})},function(c,d,b){var a=b(3);a(a.S,"Number",{parseInt:parseInt})},function(f,g,b){var a=b(3),e=b(75),c=Math.sqrt,d=Math.acosh;a(a.S+a.F*!(d&&710==Math.floor(d(Number.MAX_VALUE))),"Math",{acosh:function acosh(a){return(a=+a)<1?NaN:a>94906265.62425156?Math.log(a)+Math.LN2:e(a-1+c(a-1)*c(a+1))}})},function(a,b){a.exports=Math.log1p||function log1p(a){return(a=+a)>-1e-8&&1e-8>a?a-a*a/2:Math.log(1+a)}},function(c,d,b){function asinh(a){return isFinite(a=+a)&&0!=a?0>a?-asinh(-a):Math.log(a+Math.sqrt(a*a+1)):a}var a=b(3);a(a.S,"Math",{asinh:asinh})},function(c,d,b){var a=b(3);a(a.S,"Math",{atanh:function atanh(a){return 0==(a=+a)?a:Math.log((1+a)/(1-a))/2}})},function(d,e,a){var b=a(3),c=a(79);b(b.S,"Math",{cbrt:function cbrt(a){return c(a=+a)*Math.pow(Math.abs(a),1/3)}})},function(a,b){a.exports=Math.sign||function sign(a){return 0==(a=+a)||a!=a?a:0>a?-1:1}},function(c,d,b){var a=b(3);a(a.S,"Math",{clz32:function clz32(a){return(a>>>=0)?31-Math.floor(Math.log(a+.5)*Math.LOG2E):32}})},function(d,e,c){var a=c(3),b=Math.exp;a(a.S,"Math",{cosh:function cosh(a){return(b(a=+a)+b(-a))/2}})},function(c,d,a){var b=a(3);b(b.S,"Math",{expm1:a(83)})},function(a,b){a.exports=Math.expm1||function expm1(a){return 0==(a=+a)?a:a>-1e-6&&1e-6>a?a+a*a/2:Math.exp(a)-1}},function(k,j,e){var f=e(3),g=e(79),a=Math.pow,d=a(2,-52),b=a(2,-23),i=a(2,127)*(2-b),c=a(2,-126),h=function(a){return a+1/d-1/d};f(f.S,"Math",{fround:function fround(k){var f,a,e=Math.abs(k),j=g(k);return c>e?j*h(e/c/b)*c*b:(f=(1+b/d)*e,a=f-(f-e),a>i||a!=a?j*(1/0):j*a)}})},function(d,e,b){var a=b(3),c=Math.abs;a(a.S,"Math",{hypot:function hypot(i,j){for(var a,b,e=0,f=0,g=arguments,h=g.length,d=0;h>f;)a=c(g[f++]),a>d?(b=d/a,e=e*b*b+1,d=a):a>0?(b=a/d,e+=b*b):e+=a;return d===1/0?1/0:d*Math.sqrt(e)}})},function(d,e,b){var a=b(3),c=Math.imul;a(a.S+a.F*b(9)(function(){return-5!=c(4294967295,5)||2!=c.length}),"Math",{imul:function imul(f,g){var a=65535,b=+f,c=+g,d=a&b,e=a&c;return 0|d*e+((a&b>>>16)*e+d*(a&c>>>16)<<16>>>0)}})},function(c,d,b){var a=b(3);a(a.S,"Math",{log10:function log10(a){return Math.log(a)/Math.LN10}})},function(c,d,a){var b=a(3);b(b.S,"Math",{log1p:a(75)})},function(c,d,b){var a=b(3);a(a.S,"Math",{log2:function log2(a){return Math.log(a)/Math.LN2}})},function(c,d,a){var b=a(3);b(b.S,"Math",{sign:a(79)})},function(e,f,a){var b=a(3),c=a(83),d=Math.exp;b(b.S+b.F*a(9)(function(){return-2e-17!=!Math.sinh(-2e-17)}),"Math",{sinh:function sinh(a){return Math.abs(a=+a)<1?(c(a)-c(-a))/2:(d(a-1)-d(-a-1))*(Math.E/2)}})},function(e,f,a){var b=a(3),c=a(83),d=Math.exp;b(b.S,"Math",{tanh:function tanh(a){var b=c(a=+a),e=c(-a);return b==1/0?1:e==1/0?-1:(b-e)/(d(a)+d(-a))}})},function(c,d,b){var a=b(3);a(a.S,"Math",{trunc:function trunc(a){return(a>0?Math.floor:Math.ceil)(a)}})},function(f,g,b){var a=b(3),e=b(26),c=String.fromCharCode,d=String.fromCodePoint;a(a.S+a.F*(!!d&&1!=d.length),"String",{fromCodePoint:function fromCodePoint(h){for(var a,b=[],d=arguments,g=d.length,f=0;g>f;){if(a=+d[f++],e(a,1114111)!==a)throw RangeError(a+" is not a valid code point");b.push(65536>a?c(a):c(((a-=65536)>>10)+55296,a%1024+56320))}return b.join("")}})},function(e,f,a){var b=a(3),c=a(23),d=a(27);b(b.S,"String",{raw:function raw(g){for(var e=c(g.raw),h=d(e.length),f=arguments,i=f.length,b=[],a=0;h>a;)b.push(String(e[a++])),i>a&&b.push(String(f[a]));return b.join("")}})},function(b,c,a){a(63)("trim",function(a){return function trim(){return a(this,3)}})},function(d,e,a){var b=a(3),c=a(98)(!1);b(b.P,"String",{codePointAt:function codePointAt(a){return c(this,a)}})},function(c,f,b){var d=b(25),e=b(22);c.exports=function(b){return function(j,k){var f,h,g=String(e(j)),c=d(k),i=g.length;return 0>c||c>=i?b?"":a:(f=g.charCodeAt(c),55296>f||f>56319||c+1===i||(h=g.charCodeAt(c+1))<56320||h>57343?b?g.charAt(c):f:b?g.slice(c,c+2):(f-55296<<10)+(h-56320)+65536)}}},function(h,i,b){var c=b(3),e=b(27),g=b(100),d="endsWith",f=""[d];c(c.P+c.F*b(102)(d),"String",{endsWith:function endsWith(i){var b=g(this,i,d),j=arguments,k=j.length>1?j[1]:a,l=e(b.length),c=k===a?l:Math.min(e(k),l),h=String(i);return f?f.call(b,h,c):b.slice(c-h.length,c)===h}})},function(b,e,a){var c=a(101),d=a(22);b.exports=function(a,b,e){if(c(b))throw TypeError("String#"+e+" doesn't accept regex!");return String(d(a))}},function(c,g,b){var d=b(16),e=b(18),f=b(31)("match");c.exports=function(b){var c;return d(b)&&((c=b[f])!==a?!!c:"RegExp"==e(b))}},function(a,d,b){var c=b(31)("match");a.exports=function(b){var a=/./;try{"/./"[b](a)}catch(d){try{return a[c]=!1,!"/./"[b](a)}catch(e){}}return!0}},function(f,g,b){var c=b(3),e=b(100),d="includes";c(c.P+c.F*b(102)(d),"String",{includes:function includes(b){return!!~e(this,b,d).indexOf(b,arguments.length>1?arguments[1]:a)}})},function(c,d,a){var b=a(3);b(b.P,"String",{repeat:a(105)})},function(b,e,a){var c=a(25),d=a(22);b.exports=function repeat(f){var b=String(d(this)),e="",a=c(f);if(0>a||a==1/0)throw RangeError("Count can't be negative");for(;a>0;(a>>>=1)&&(b+=b))1&a&&(e+=b);return e}},function(h,i,b){var c=b(3),f=b(27),g=b(100),d="startsWith",e=""[d];c(c.P+c.F*b(102)(d),"String",{startsWith:function startsWith(i){var b=g(this,i,d),j=arguments,c=f(Math.min(j.length>1?j[1]:a,b.length)),h=String(i);return e?e.call(b,h,c):b.slice(c,c+h.length)===h}})},function(d,e,b){var c=b(98)(!0);b(108)(String,"String",function(a){this._t=String(a),this._i=0},function(){var b,d=this._t,e=this._i;return e>=d.length?{value:a,done:!0}:(b=c(d,e),this._i+=b.length,{value:b,done:!1})})},function(o,r,a){var i=a(39),d=a(3),n=a(10),h=a(6),m=a(17),f=a(109),q=a(110),p=a(35),l=a(2).getProto,c=a(31)("iterator"),e=!([].keys&&"next"in[].keys()),j="@@iterator",k="keys",b="values",g=function(){return this};o.exports=function(B,v,u,F,s,E,A){q(u,v,F);var r,x,w=function(c){if(!e&&c in a)return a[c];switch(c){case k:return function keys(){return new u(this,c)};case b:return function values(){return new u(this,c)}}return function entries(){return new u(this,c)}},C=v+" Iterator",y=s==b,z=!1,a=B.prototype,t=a[c]||a[j]||s&&a[s],o=t||w(s);if(t){var D=l(o.call(new B));p(D,C,!0),!i&&m(a,j)&&h(D,c,g),y&&t.name!==b&&(z=!0,o=function values(){return t.call(this)})}if(i&&!A||!e&&!z&&a[c]||h(a,c,o),f[v]=o,f[C]=g,s)if(r={values:y?o:w(b),keys:E?o:w(k),entries:y?w("entries"):o},A)for(x in r)x in a||n(a,x,r[x]);else d(d.P+d.F*(e||z),v,r);return r}},function(a,b){a.exports={}},function(c,g,a){var d=a(2),e=a(7),f=a(35),b={};a(6)(b,a(31)("iterator"),function(){return this}),c.exports=function(a,c,g){a.prototype=d.create(b,{next:e(1,g)}),f(a,c+" Iterator")}},function(j,k,b){var d=b(12),c=b(3),e=b(21),f=b(112),g=b(113),h=b(27),i=b(114);c(c.S+c.F*!b(115)(function(a){Array.from(a)}),"Array",{from:function from(t){var n,c,r,m,j=e(t),l="function"==typeof this?this:Array,p=arguments,s=p.length,k=s>1?p[1]:a,q=k!==a,b=0,o=i(j);if(q&&(k=d(k,s>2?p[2]:a,2)),o==a||l==Array&&g(o))for(n=h(j.length),c=new l(n);n>b;b++)c[b]=q?k(j[b],b):j[b];else for(m=o.call(j),c=new l;!(r=m.next()).done;b++)c[b]=q?f(m,k,[r.value,b],!0):r.value;return c.length=b,c}})},function(c,e,d){var b=d(20);c.exports=function(d,e,c,g){try{return g?e(b(c)[0],c[1]):e(c)}catch(h){var f=d["return"];throw f!==a&&b(f.call(d)),h}}},function(c,g,b){var d=b(109),e=b(31)("iterator"),f=Array.prototype;c.exports=function(b){return b!==a&&(d.Array===b||f[e]===b)}},function(c,g,b){var d=b(47),e=b(31)("iterator"),f=b(109);c.exports=b(5).getIteratorMethod=function(b){return b!=a?b[e]||b["@@iterator"]||f[d(b)]:void 0}},function(d,f,e){var a=e(31)("iterator"),b=!1;try{var c=[7][a]();c["return"]=function(){b=!0},Array.from(c,function(){throw 2})}catch(g){}d.exports=function(f,g){if(!g&&!b)return!1;var d=!1;try{var c=[7],e=c[a]();e.next=function(){return{done:d=!0}},c[a]=function(){return e},f(c)}catch(h){}return d}},function(c,d,b){var a=b(3);a(a.S+a.F*b(9)(function(){function F(){}return!(Array.of.call(F)instanceof F)}),"Array",{of:function of(){for(var a=0,d=arguments,b=d.length,c=new("function"==typeof this?this:Array)(b);b>a;)c[a]=d[a++];return c.length=b,c}})},function(f,h,b){var d=b(118),c=b(119),e=b(109),g=b(23);f.exports=b(108)(Array,"Array",function(a,b){this._t=g(a),this._i=0,this._k=b},function(){var d=this._t,e=this._k,b=this._i++;return!d||b>=d.length?(this._t=a,c(1)):"keys"==e?c(0,b):"values"==e?c(0,d[b]):c(0,[b,d[b]])},"values"),e.Arguments=e.Array,d("keys"),d("values"),d("entries")},function(e,f,d){var b=d(31)("unscopables"),c=Array.prototype;c[b]==a&&d(6)(c,b,{}),e.exports=function(a){c[b][a]=!0}},function(a,b){a.exports=function(a,b){return{value:b,done:!!a}}},function(b,c,a){a(121)("Array")},function(c,g,a){var d=a(4),e=a(2),f=a(8),b=a(31)("species");c.exports=function(c){var a=d[c];f&&a&&!a[b]&&e.setDesc(a,b,{configurable:!0,get:function(){return this}})}},function(c,d,a){var b=a(3);b(b.P,"Array",{copyWithin:a(123)}),a(118)("copyWithin")},function(d,g,b){var e=b(21),c=b(26),f=b(27);d.exports=[].copyWithin||function copyWithin(m,n){var g=e(this),h=f(g.length),b=c(m,h),d=c(n,h),k=arguments,l=k.length>2?k[2]:a,i=Math.min((l===a?h:c(l,h))-d,h-b),j=1;for(b>d&&d+i>b&&(j=-1,d+=i-1,b+=i-1);i-->0;)d in g?g[b]=g[d]:delete g[b],b+=j,d+=j;return g}},function(c,d,a){var b=a(3);b(b.P,"Array",{fill:a(125)}),a(118)("fill")},function(d,g,b){var e=b(21),c=b(26),f=b(27);d.exports=[].fill||function fill(k){for(var b=e(this),d=f(b.length),g=arguments,h=g.length,i=c(h>1?g[1]:a,d),j=h>2?g[2]:a,l=j===a?d:c(j,d);l>i;)b[i++]=k;return b}},function(g,h,b){var c=b(3),f=b(28)(5),d="find",e=!0;d in[]&&Array(1)[d](function(){e=!1}),c(c.P+c.F*e,"Array",{find:function find(b){return f(this,b,arguments.length>1?arguments[1]:a)}}),b(118)(d)},function(g,h,b){var c=b(3),f=b(28)(6),d="findIndex",e=!0;d in[]&&Array(1)[d](function(){e=!1}),c(c.P+c.F*e,"Array",{findIndex:function findIndex(b){return f(this,b,arguments.length>1?arguments[1]:a)}}),b(118)(d)},function(n,m,c){var f=c(2),i=c(4),k=c(101),l=c(129),b=i.RegExp,d=b,j=b.prototype,e=/a/g,g=/a/g,h=new b(e)!==e;!c(8)||h&&!c(9)(function(){return g[c(31)("match")]=!1,b(e)!=e||b(g)==g||"/a/i"!=b(e,"i")})||(b=function RegExp(c,f){var e=k(c),g=f===a;return this instanceof b||!e||c.constructor!==b||!g?h?new d(e&&!g?c.source:c,f):d((e=c instanceof b)?c.source:c,e&&g?l.call(c):f):c},f.each.call(f.getNames(d),function(a){a in b||f.setDesc(b,a,{configurable:!0,get:function(){return d[a]},set:function(b){d[a]=b}})}),j.constructor=b,b.prototype=j,c(10)(i,"RegExp",b)),c(121)("RegExp")},function(a,d,b){var c=b(20);a.exports=function(){var b=c(this),a="";return b.global&&(a+="g"),b.ignoreCase&&(a+="i"),b.multiline&&(a+="m"),b.unicode&&(a+="u"),b.sticky&&(a+="y"),a}},function(c,d,a){var b=a(2);a(8)&&"g"!=/./g.flags&&b.setDesc(RegExp.prototype,"flags",{configurable:!0,get:a(129)})},function(c,d,b){b(132)("match",1,function(c,b){return function match(d){var e=c(this),f=d==a?a:d[b];return f!==a?f.call(d,e):new RegExp(d)[b](String(e))}})},function(b,h,a){var c=a(6),d=a(10),e=a(9),f=a(22),g=a(31);b.exports=function(a,i,j){var b=g(a),h=""[a];e(function(){var c={};return c[b]=function(){return 7},7!=""[a](c)})&&(d(String.prototype,a,j(f,b,h)),c(RegExp.prototype,b,2==i?function(a,b){return h.call(a,this,b)}:function(a){return h.call(a,this)}))}},function(c,d,b){b(132)("replace",2,function(b,c,d){return function replace(e,f){var g=b(this),h=e==a?a:e[c];return h!==a?h.call(e,g,f):d.call(String(g),e,f)}})},function(c,d,b){b(132)("search",1,function(c,b){return function search(d){var e=c(this),f=d==a?a:d[b];return f!==a?f.call(d,e):new RegExp(d)[b](String(e))}})},function(c,d,b){b(132)("split",2,function(b,c,d){return function split(e,f){var g=b(this),h=e==a?a:e[c];return h!==a?h.call(e,g,f):d.call(String(g),e,f)}})},function(K,J,b){var s,l=b(2),F=b(39),k=b(4),j=b(12),I=b(47),d=b(3),D=b(16),E=b(20),m=b(13),G=b(137),p=b(138),q=b(45).set,A=b(43),B=b(31)("species"),z=b(139),v=b(140),e="Promise",o=k.process,H="process"==I(o),c=k[e],i=function(){},r=function(d){var b,a=new c(i);return d&&(a.constructor=function(a){a(i,i)}),(b=c.resolve(a))["catch"](i),b===a},h=function(){function P2(b){var a=new c(b);return q(a,P2.prototype),a}var a=!1;try{if(a=c&&c.resolve&&r(),q(P2,c),P2.prototype=l.create(c.prototype,{constructor:{value:P2}}),P2.resolve(5).then(function(){})instanceof P2||(a=!1),
+a&&b(8)){var d=!1;c.resolve(l.setDesc({},"then",{get:function(){d=!0}})),a=d}}catch(e){a=!1}return a}(),C=function(a,b){return F&&a===c&&b===s?!0:A(a,b)},t=function(b){var c=E(b)[B];return c!=a?c:b},u=function(a){var b;return D(a)&&"function"==typeof(b=a.then)?b:!1},g=function(d){var b,c;this.promise=new d(function(d,e){if(b!==a||c!==a)throw TypeError("Bad Promise constructor");b=d,c=e}),this.resolve=m(b),this.reject=m(c)},w=function(a){try{a()}catch(b){return{error:b}}},n=function(b,d){if(!b.n){b.n=!0;var c=b.c;v(function(){for(var e=b.v,f=1==b.s,g=0,h=function(a){var c,h,g=f?a.ok:a.fail,i=a.resolve,d=a.reject;try{g?(f||(b.h=!0),c=g===!0?e:g(e),c===a.promise?d(TypeError("Promise-chain cycle")):(h=u(c))?h.call(c,i,d):i(c)):d(e)}catch(j){d(j)}};c.length>g;)h(c[g++]);c.length=0,b.n=!1,d&&setTimeout(function(){var f,c,d=b.p;y(d)&&(H?o.emit("unhandledRejection",e,d):(f=k.onunhandledrejection)?f({promise:d,reason:e}):(c=k.console)&&c.error&&c.error("Unhandled promise rejection",e)),b.a=a},1)})}},y=function(e){var a,b=e._d,c=b.a||b.c,d=0;if(b.h)return!1;for(;c.length>d;)if(a=c[d++],a.fail||!y(a.promise))return!1;return!0},f=function(b){var a=this;a.d||(a.d=!0,a=a.r||a,a.v=b,a.s=2,a.a=a.c.slice(),n(a,!0))},x=function(b){var c,a=this;if(!a.d){a.d=!0,a=a.r||a;try{if(a.p===b)throw TypeError("Promise can't be resolved itself");(c=u(b))?v(function(){var d={r:a,d:!1};try{c.call(b,j(x,d,1),j(f,d,1))}catch(e){f.call(d,e)}}):(a.v=b,a.s=1,n(a,!1))}catch(d){f.call({r:a,d:!1},d)}}};h||(c=function Promise(d){m(d);var b=this._d={p:G(this,c,e),c:[],a:a,s:0,d:!1,v:a,h:!1,n:!1};try{d(j(x,b,1),j(f,b,1))}catch(g){f.call(b,g)}},b(142)(c.prototype,{then:function then(d,e){var a=new g(z(this,c)),f=a.promise,b=this._d;return a.ok="function"==typeof d?d:!0,a.fail="function"==typeof e&&e,b.c.push(a),b.a&&b.a.push(a),b.s&&n(b,!1),f},"catch":function(b){return this.then(a,b)}})),d(d.G+d.W+d.F*!h,{Promise:c}),b(35)(c,e),b(121)(e),s=b(5)[e],d(d.S+d.F*!h,e,{reject:function reject(b){var a=new g(this),c=a.reject;return c(b),a.promise}}),d(d.S+d.F*(!h||r(!0)),e,{resolve:function resolve(a){if(a instanceof c&&C(a.constructor,this))return a;var b=new g(this),d=b.resolve;return d(a),b.promise}}),d(d.S+d.F*!(h&&b(115)(function(a){c.all(a)["catch"](function(){})})),e,{all:function all(h){var c=t(this),b=new g(c),d=b.resolve,e=b.reject,a=[],f=w(function(){p(h,!1,a.push,a);var b=a.length,f=Array(b);b?l.each.call(a,function(g,h){var a=!1;c.resolve(g).then(function(c){a||(a=!0,f[h]=c,--b||d(f))},e)}):d(f)});return f&&e(f.error),b.promise},race:function race(e){var b=t(this),a=new g(b),c=a.reject,d=w(function(){p(e,!1,function(d){b.resolve(d).then(a.resolve,c)})});return d&&c(d.error),a.promise}})},function(a,b){a.exports=function(a,b,c){if(!(a instanceof b))throw TypeError(c+": use the 'new' operator!");return a}},function(b,i,a){var c=a(12),d=a(112),e=a(113),f=a(20),g=a(27),h=a(114);b.exports=function(a,j,o,p){var n,b,k,l=h(a),m=c(o,p,j?2:1),i=0;if("function"!=typeof l)throw TypeError(a+" is not iterable!");if(e(l))for(n=g(a.length);n>i;i++)j?m(f(b=a[i])[0],b[1]):m(a[i]);else for(k=l.call(a);!(b=k.next()).done;)d(k,m,b.value,j)}},function(d,g,b){var c=b(20),e=b(13),f=b(31)("species");d.exports=function(g,h){var b,d=c(g).constructor;return d===a||(b=c(d)[f])==a?h:e(b)}},function(n,p,h){var b,f,g,c=h(4),o=h(141).set,k=c.MutationObserver||c.WebKitMutationObserver,d=c.process,i=c.Promise,j="process"==h(18)(d),e=function(){var e,c,g;for(j&&(e=d.domain)&&(d.domain=null,e.exit());b;)c=b.domain,g=b.fn,c&&c.enter(),g(),c&&c.exit(),b=b.next;f=a,e&&e.enter()};if(j)g=function(){d.nextTick(e)};else if(k){var m=1,l=document.createTextNode("");new k(e).observe(l,{characterData:!0}),g=function(){l.data=m=-m}}else g=i&&i.resolve?function(){i.resolve().then(e)}:function(){o.call(c,e)};n.exports=function asap(e){var c={fn:e,next:a,domain:j&&d.domain};f&&(f.next=c),b||(b=c,g()),f=c}},function(s,t,b){var c,g,f,k=b(12),r=b(19),n=b(14),p=b(15),a=b(4),l=a.process,h=a.setImmediate,i=a.clearImmediate,o=a.MessageChannel,j=0,d={},q="onreadystatechange",e=function(){var a=+this;if(d.hasOwnProperty(a)){var b=d[a];delete d[a],b()}},m=function(a){e.call(a.data)};h&&i||(h=function setImmediate(a){for(var b=[],e=1;arguments.length>e;)b.push(arguments[e++]);return d[++j]=function(){r("function"==typeof a?a:Function(a),b)},c(j),j},i=function clearImmediate(a){delete d[a]},"process"==b(18)(l)?c=function(a){l.nextTick(k(e,a,1))}:o?(g=new o,f=g.port2,g.port1.onmessage=m,c=k(f.postMessage,f,1)):a.addEventListener&&"function"==typeof postMessage&&!a.importScripts?(c=function(b){a.postMessage(b+"","*")},a.addEventListener("message",m,!1)):c=q in p("script")?function(a){n.appendChild(p("script"))[q]=function(){n.removeChild(this),e.call(a)}}:function(a){setTimeout(k(e,a,1),0)}),s.exports={set:h,clear:i}},function(a,d,b){var c=b(10);a.exports=function(a,b){for(var d in b)c(a,d,b[d]);return a}},function(d,e,c){var b=c(144);c(145)("Map",function(b){return function Map(){return b(this,arguments.length>0?arguments[0]:a)}},{get:function get(c){var a=b.getEntry(this,c);return a&&a.v},set:function set(a,c){return b.def(this,0===a?0:a,c)}},b,!0)},function(v,w,b){var j=b(2),m=b(6),o=b(142),n=b(12),p=b(137),r=b(22),t=b(138),l=b(108),d=b(119),f=b(11)("id"),k=b(17),h=b(16),q=b(121),i=b(8),s=Object.isExtensible||h,c=i?"_s":"size",u=0,g=function(a,b){if(!h(a))return"symbol"==typeof a?a:("string"==typeof a?"S":"P")+a;if(!k(a,f)){if(!s(a))return"F";if(!b)return"E";m(a,f,++u)}return"O"+a[f]},e=function(b,c){var a,d=g(c);if("F"!==d)return b._i[d];for(a=b._f;a;a=a.n)if(a.k==c)return a};v.exports={getConstructor:function(d,f,g,h){var b=d(function(d,e){p(d,b,f),d._i=j.create(null),d._f=a,d._l=a,d[c]=0,e!=a&&t(e,g,d[h],d)});return o(b.prototype,{clear:function clear(){for(var d=this,e=d._i,b=d._f;b;b=b.n)b.r=!0,b.p&&(b.p=b.p.n=a),delete e[b.i];d._f=d._l=a,d[c]=0},"delete":function(g){var b=this,a=e(b,g);if(a){var d=a.n,f=a.p;delete b._i[a.i],a.r=!0,f&&(f.n=d),d&&(d.p=f),b._f==a&&(b._f=d),b._l==a&&(b._l=f),b[c]--}return!!a},forEach:function forEach(c){for(var b,d=n(c,arguments.length>1?arguments[1]:a,3);b=b?b.n:this._f;)for(d(b.v,b.k,this);b&&b.r;)b=b.p},has:function has(a){return!!e(this,a)}}),i&&j.setDesc(b.prototype,"size",{get:function(){return r(this[c])}}),b},def:function(b,f,j){var h,i,d=e(b,f);return d?d.v=j:(b._l=d={i:i=g(f,!0),k:f,v:j,p:h=b._l,n:a,r:!1},b._f||(b._f=d),h&&(h.n=d),b[c]++,"F"!==i&&(b._i[i]=d)),b},getEntry:e,setStrong:function(e,b,c){l(e,b,function(b,c){this._t=b,this._k=c,this._l=a},function(){for(var c=this,e=c._k,b=c._l;b&&b.r;)b=b.p;return c._t&&(c._l=b=b?b.n:c._t._f)?"keys"==e?d(0,b.k):"values"==e?d(0,b.v):d(0,[b.k,b.v]):(c._t=a,d(1))},c?"entries":"values",!c,!0),q(b)}}},function(l,n,b){var k=b(4),c=b(3),g=b(10),f=b(142),i=b(138),j=b(137),d=b(16),e=b(9),h=b(115),m=b(35);l.exports=function(o,v,y,x,p,l){var t=k[o],b=t,s=p?"set":"add",n=b&&b.prototype,w={},r=function(b){var c=n[b];g(n,b,"delete"==b?function(a){return l&&!d(a)?!1:c.call(this,0===a?0:a)}:"has"==b?function has(a){return l&&!d(a)?!1:c.call(this,0===a?0:a)}:"get"==b?function get(b){return l&&!d(b)?a:c.call(this,0===b?0:b)}:"add"==b?function add(a){return c.call(this,0===a?0:a),this}:function set(a,b){return c.call(this,0===a?0:a,b),this})};if("function"==typeof b&&(l||n.forEach&&!e(function(){(new b).entries().next()}))){var u,q=new b,z=q[s](l?{}:-0,1)!=q,A=e(function(){q.has(1)}),B=h(function(a){new b(a)});B||(b=v(function(e,d){j(e,b,o);var c=new t;return d!=a&&i(d,p,c[s],c),c}),b.prototype=n,n.constructor=b),l||q.forEach(function(b,a){u=1/a===-(1/0)}),(A||u)&&(r("delete"),r("has"),p&&r("get")),(u||z)&&r(s),l&&n.clear&&delete n.clear}else b=x.getConstructor(v,o,p,s),f(b.prototype,y);return m(b,o),w[o]=b,c(c.G+c.W+c.F*(b!=t),w),l||x.setStrong(b,o,p),b}},function(d,e,b){var c=b(144);b(145)("Set",function(b){return function Set(){return b(this,arguments.length>0?arguments[0]:a)}},{add:function add(a){return c.def(this,a=0===a?0:a,a)}},c)},function(n,m,b){var l=b(2),k=b(10),c=b(148),d=b(16),j=b(17),i=c.frozenStore,h=c.WEAK,f=Object.isExtensible||d,e={},g=b(145)("WeakMap",function(b){return function WeakMap(){return b(this,arguments.length>0?arguments[0]:a)}},{get:function get(a){if(d(a)){if(!f(a))return i(this).get(a);if(j(a,h))return a[h][this._i]}},set:function set(a,b){return c.def(this,a,b)}},c,!0,!0);7!=(new g).set((Object.freeze||Object)(e),7).get(e)&&l.each.call(["delete","has","get","set"],function(a){var b=g.prototype,c=b[a];k(b,a,function(b,e){if(d(b)&&!f(b)){var g=i(this)[a](b,e);return"set"==a?this:g}return c.call(this,b,e)})})},function(s,t,b){var r=b(6),q=b(142),m=b(20),h=b(16),l=b(137),k=b(138),j=b(28),d=b(17),c=b(11)("weak"),g=Object.isExtensible||h,n=j(5),o=j(6),p=0,e=function(a){return a._l||(a._l=new i)},i=function(){this.a=[]},f=function(a,b){return n(a.a,function(a){return a[0]===b})};i.prototype={get:function(b){var a=f(this,b);return a?a[1]:void 0},has:function(a){return!!f(this,a)},set:function(a,b){var c=f(this,a);c?c[1]=b:this.a.push([a,b])},"delete":function(b){var a=o(this.a,function(a){return a[0]===b});return~a&&this.a.splice(a,1),!!~a}},s.exports={getConstructor:function(f,i,j,m){var b=f(function(c,d){l(c,b,i),c._i=p++,c._l=a,d!=a&&k(d,j,c[m],c)});return q(b.prototype,{"delete":function(a){return h(a)?g(a)?d(a,c)&&d(a[c],this._i)&&delete a[c][this._i]:e(this)["delete"](a):!1},has:function has(a){return h(a)?g(a)?d(a,c)&&d(a[c],this._i):e(this).has(a):!1}}),b},def:function(b,a,f){return g(m(a))?(d(a,c)||r(a,c,{}),a[c][b._i]=f):e(b).set(a,f),b},frozenStore:e,WEAK:c}},function(d,e,b){var c=b(148);b(145)("WeakSet",function(b){return function WeakSet(){return b(this,arguments.length>0?arguments[0]:a)}},{add:function add(a){return c.def(this,a,!0)}},c,!1,!0)},function(e,f,a){var b=a(3),c=Function.apply,d=a(20);b(b.S,"Reflect",{apply:function apply(a,b,e){return c.call(a,b,d(e))}})},function(h,i,a){var e=a(2),b=a(3),c=a(13),f=a(20),d=a(16),g=Function.bind||a(5).Function.prototype.bind;b(b.S+b.F*a(9)(function(){function F(){}return!(Reflect.construct(function(){},[],F)instanceof F)}),"Reflect",{construct:function construct(b,a){c(b),f(a);var i=arguments.length<3?b:c(arguments[2]);if(b==i){switch(a.length){case 0:return new b;case 1:return new b(a[0]);case 2:return new b(a[0],a[1]);case 3:return new b(a[0],a[1],a[2]);case 4:return new b(a[0],a[1],a[2],a[3])}var h=[null];return h.push.apply(h,a),new(g.apply(b,h))}var j=i.prototype,k=e.create(d(j)?j:Object.prototype),l=Function.apply.call(b,k,a);return d(l)?l:k}})},function(e,f,a){var c=a(2),b=a(3),d=a(20);b(b.S+b.F*a(9)(function(){Reflect.defineProperty(c.setDesc({},1,{value:1}),1,{value:2})}),"Reflect",{defineProperty:function defineProperty(a,b,e){d(a);try{return c.setDesc(a,b,e),!0}catch(f){return!1}}})},function(e,f,a){var b=a(3),c=a(2).getDesc,d=a(20);b(b.S,"Reflect",{deleteProperty:function deleteProperty(a,b){var e=c(d(a),b);return e&&!e.configurable?!1:delete a[b]}})},function(f,g,b){var c=b(3),e=b(20),d=function(a){this._t=e(a),this._i=0;var b,c=this._k=[];for(b in a)c.push(b)};b(110)(d,"Object",function(){var c,b=this,d=b._k;do if(b._i>=d.length)return{value:a,done:!0};while(!((c=d[b._i++])in b._t));return{value:c,done:!1}}),c(c.S,"Reflect",{enumerate:function enumerate(a){return new d(a)}})},function(h,i,b){function get(b,h){var d,j,i=arguments.length<3?b:arguments[2];return g(b)===i?b[h]:(d=c.getDesc(b,h))?e(d,"value")?d.value:d.get!==a?d.get.call(i):a:f(j=c.getProto(b))?get(j,h,i):void 0}var c=b(2),e=b(17),d=b(3),f=b(16),g=b(20);d(d.S,"Reflect",{get:get})},function(e,f,a){var c=a(2),b=a(3),d=a(20);b(b.S,"Reflect",{getOwnPropertyDescriptor:function getOwnPropertyDescriptor(a,b){return c.getDesc(d(a),b)}})},function(e,f,a){var b=a(3),c=a(2).getProto,d=a(20);b(b.S,"Reflect",{getPrototypeOf:function getPrototypeOf(a){return c(d(a))}})},function(c,d,b){var a=b(3);a(a.S,"Reflect",{has:function has(a,b){return b in a}})},function(e,f,a){var b=a(3),d=a(20),c=Object.isExtensible;b(b.S,"Reflect",{isExtensible:function isExtensible(a){return d(a),c?c(a):!0}})},function(c,d,a){var b=a(3);b(b.S,"Reflect",{ownKeys:a(161)})},function(d,f,a){var b=a(2),e=a(20),c=a(4).Reflect;d.exports=c&&c.ownKeys||function ownKeys(a){var c=b.getNames(e(a)),d=b.getSymbols;return d?c.concat(d(a)):c}},function(e,f,a){var b=a(3),d=a(20),c=Object.preventExtensions;b(b.S,"Reflect",{preventExtensions:function preventExtensions(a){d(a);try{return c&&c(a),!0}catch(b){return!1}}})},function(i,j,b){function set(j,i,k){var l,m,d=arguments.length<4?j:arguments[3],b=c.getDesc(h(j),i);if(!b){if(f(m=c.getProto(j)))return set(m,i,k,d);b=e(0)}return g(b,"value")?b.writable!==!1&&f(d)?(l=c.getDesc(d,i)||e(0),l.value=k,c.setDesc(d,i,l),!0):!1:b.set===a?!1:(b.set.call(d,k),!0)}var c=b(2),g=b(17),d=b(3),e=b(7),h=b(20),f=b(16);d(d.S,"Reflect",{set:set})},function(d,e,b){var c=b(3),a=b(45);a&&c(c.S,"Reflect",{setPrototypeOf:function setPrototypeOf(b,c){a.check(b,c);try{return a.set(b,c),!0}catch(d){return!1}}})},function(e,f,b){var c=b(3),d=b(33)(!0);c(c.P,"Array",{includes:function includes(b){return d(this,b,arguments.length>1?arguments[1]:a)}}),b(118)("includes")},function(d,e,a){var b=a(3),c=a(98)(!0);b(b.P,"String",{at:function at(a){return c(this,a)}})},function(e,f,b){var c=b(3),d=b(168);c(c.P,"String",{padLeft:function padLeft(b){return d(this,b,arguments.length>1?arguments[1]:a,!0)}})},function(c,g,b){var d=b(27),e=b(105),f=b(22);c.exports=function(l,m,i,n){var c=String(f(l)),j=c.length,g=i===a?" ":String(i),k=d(m);if(j>=k)return c;""==g&&(g=" ");var h=k-j,b=e.call(g,Math.ceil(h/g.length));return b.length>h&&(b=b.slice(0,h)),n?b+c:c+b}},function(e,f,b){var c=b(3),d=b(168);c(c.P,"String",{padRight:function padRight(b){return d(this,b,arguments.length>1?arguments[1]:a,!1)}})},function(b,c,a){a(63)("trimLeft",function(a){return function trimLeft(){return a(this,1)}})},function(b,c,a){a(63)("trimRight",function(a){return function trimRight(){return a(this,2)}})},function(d,e,a){var b=a(3),c=a(173)(/[\\^$*+?.()|[\]{}]/g,"\\$&");b(b.S,"RegExp",{escape:function escape(a){return c(a)}})},function(a,b){a.exports=function(b,a){var c=a===Object(a)?function(b){return a[b]}:a;return function(a){return String(a).replace(b,c)}}},function(g,h,a){var b=a(2),c=a(3),d=a(161),e=a(23),f=a(7);c(c.S,"Object",{getOwnPropertyDescriptors:function getOwnPropertyDescriptors(k){for(var a,g,h=e(k),l=b.setDesc,m=b.getDesc,i=d(h),c={},j=0;i.length>j;)g=m(h,a=i[j++]),a in c?l(c,a,f(0,g)):c[a]=g;return c}})},function(d,e,a){var b=a(3),c=a(176)(!1);b(b.S,"Object",{values:function values(a){return c(a)}})},function(c,f,a){var b=a(2),d=a(23),e=b.isEnum;c.exports=function(a){return function(j){for(var c,f=d(j),g=b.getKeys(f),k=g.length,h=0,i=[];k>h;)e.call(f,c=g[h++])&&i.push(a?[c,f[c]]:f[c]);return i}}},function(d,e,a){var b=a(3),c=a(176)(!0);b(b.S,"Object",{entries:function entries(a){return c(a)}})},function(c,d,a){var b=a(3);b(b.P,"Map",{toJSON:a(179)("Map")})},function(b,e,a){var c=a(138),d=a(47);b.exports=function(a){return function toJSON(){if(d(this)!=a)throw TypeError(a+"#toJSON isn't generic");var b=[];return c(this,!1,b.push,b),b}}},function(c,d,a){var b=a(3);b(b.P,"Set",{toJSON:a(179)("Set")})},function(d,e,b){var a=b(3),c=b(141);a(a.G+a.B,{setImmediate:c.set,clearImmediate:c.clear})},function(l,k,a){a(117);var g=a(4),j=a(6),c=a(109),b=a(31)("iterator"),h=g.NodeList,i=g.HTMLCollection,e=h&&h.prototype,d=i&&i.prototype,f=c.NodeList=c.HTMLCollection=c.Array;e&&!e[b]&&j(e,b,f),d&&!d[b]&&j(d,b,f)},function(i,j,a){var c=a(4),b=a(3),g=a(19),h=a(184),d=c.navigator,e=!!d&&/MSIE .\./.test(d.userAgent),f=function(a){return e?function(b,c){return a(g(h,[].slice.call(arguments,2),"function"==typeof b?b:Function(b)),c)}:a};b(b.G+b.B+b.F*e,{setTimeout:f(c.setTimeout),setInterval:f(c.setInterval)})},function(c,f,a){var d=a(185),b=a(19),e=a(13);c.exports=function(){for(var h=e(this),a=arguments.length,c=Array(a),f=0,i=d._,g=!1;a>f;)(c[f]=arguments[f++])===i&&(g=!0);return function(){var d,k=this,f=arguments,l=f.length,e=0,j=0;if(!g&&!l)return b(h,c,k);if(d=c.slice(),g)for(;a>e;e++)d[e]===i&&(d[e]=f[j++]);for(;l>j;)d.push(f[j++]);return b(h,d,k)}}},function(a,c,b){a.exports=b(4)},function(i,j,b){var g=b(2),e=b(3),h=b(12),f=b(5).Array||Array,c={},d=function(d,b){g.each.call(d.split(","),function(d){b==a&&d in f?c[d]=f[d]:d in[]&&(c[d]=h(Function.call,[][d],b))})};d("pop,reverse,shift,keys,values,entries",1),d("indexOf,every,some,forEach,map,filter,find,findIndex,includes",3),d("join,slice,concat,push,splice,unshift,sort,lastIndexOf,reduce,reduceRight,copyWithin,fill"),e(e.S,"Array",c)}]),"undefined"!=typeof module&&module.exports?module.exports=b:"function"==typeof define&&define.amd?define(function(){return b}):c.core=b}(1,1);
+//# sourceMappingURL=shim.min.js.map
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/shim.min.js.map b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/shim.min.js.map
new file mode 100644
index 00000000..60b23fa2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/client/shim.min.js.map
@@ -0,0 +1 @@
+{"version":3,"sources":["shim.js"],"names":["__e","__g","undefined","modules","__webpack_require__","moduleId","installedModules","exports","module","id","loaded","call","m","c","p","IE8_DOM_DEFINE","$","$export","DESCRIPTORS","createDesc","html","cel","has","cof","invoke","fails","anObject","aFunction","isObject","toObject","toIObject","toInteger","toIndex","toLength","IObject","IE_PROTO","createArrayMethod","arrayIndexOf","ObjectProto","Object","prototype","ArrayProto","Array","arraySlice","slice","arrayJoin","join","defineProperty","setDesc","getOwnDescriptor","getDesc","defineProperties","setDescs","factories","get","a","O","P","Attributes","e","TypeError","value","propertyIsEnumerable","Properties","keys","getKeys","length","i","S","F","getOwnPropertyDescriptor","keys1","split","keys2","concat","keysLen1","createDict","iframeDocument","iframe","gt","style","display","appendChild","src","contentWindow","document","open","write","close","createGetKeys","names","object","key","result","push","Empty","getPrototypeOf","getProto","constructor","getOwnPropertyNames","getNames","create","construct","len","args","n","Function","bind","that","fn","this","partArgs","arguments","bound","begin","end","klass","start","upTo","size","cloned","charAt","separator","isArray","createArrayReduce","isRight","callbackfn","memo","index","methodize","$fn","arg1","forEach","each","map","filter","some","every","reduce","reduceRight","indexOf","lastIndexOf","el","fromIndex","Math","min","now","Date","lz","num","toISOString","NaN","isFinite","RangeError","d","y","getUTCFullYear","getUTCMilliseconds","s","abs","getUTCMonth","getUTCDate","getUTCHours","getUTCMinutes","getUTCSeconds","$Object","isEnum","getSymbols","getOwnPropertySymbols","global","core","hide","redefine","ctx","PROTOTYPE","type","name","source","own","out","exp","IS_FORCED","IS_GLOBAL","G","IS_STATIC","IS_PROTO","IS_BIND","B","target","expProto","W","window","self","version","bitmap","enumerable","configurable","writable","exec","SRC","TO_STRING","$toString","TPL","inspectSource","it","val","safe","hasOwnProperty","String","toString","px","random","b","apply","documentElement","is","createElement","un","defined","ceil","floor","isNaN","max","asc","TYPE","IS_MAP","IS_FILTER","IS_SOME","IS_EVERY","IS_FIND_INDEX","NO_HOLES","$this","res","f","SPECIES","original","C","arg","store","uid","Symbol","SHARED","IS_INCLUDES","$fails","shared","setToStringTag","wks","keyOf","$names","enumKeys","_create","$Symbol","$JSON","JSON","_stringify","stringify","setter","HIDDEN","SymbolRegistry","AllSymbols","useNative","setSymbolDesc","D","protoDesc","wrap","tag","sym","_k","set","isSymbol","$defineProperty","$defineProperties","l","$create","$propertyIsEnumerable","E","$getOwnPropertyDescriptor","$getOwnPropertyNames","$getOwnPropertySymbols","$stringify","replacer","$replacer","$$","buggyJSON","symbolStatics","for","keyFor","useSetter","useSimple","def","TAG","stat","windowNames","getWindowNames","symbols","assign","A","K","k","T","$$len","j","x","setPrototypeOf","check","proto","test","buggy","__proto__","classof","ARG","callee","$freeze","freeze","KEY","$seal","seal","$preventExtensions","preventExtensions","$isFrozen","isFrozen","$isSealed","isSealed","$isExtensible","isExtensible","$getPrototypeOf","$keys","FProto","nameRE","NAME","match","HAS_INSTANCE","FunctionProto","toPrimitive","$trim","trim","NUMBER","$Number","Base","BROKEN_COF","TRIM","toNumber","argument","third","radix","maxCode","first","charCodeAt","code","digits","parseInt","Number","valueOf","spaces","space","non","ltrim","RegExp","rtrim","exporter","string","replace","EPSILON","pow","_isFinite","isInteger","number","isSafeInteger","MAX_SAFE_INTEGER","MIN_SAFE_INTEGER","parseFloat","log1p","sqrt","$acosh","acosh","MAX_VALUE","log","LN2","asinh","atanh","sign","cbrt","clz32","LOG2E","cosh","expm1","EPSILON32","MAX32","MIN32","roundTiesToEven","fround","$abs","$sign","Infinity","hypot","value1","value2","div","sum","larg","$imul","imul","UINT16","xn","yn","xl","yl","log10","LN10","log2","sinh","tanh","trunc","fromCharCode","$fromCodePoint","fromCodePoint","raw","callSite","tpl","$at","codePointAt","pos","context","ENDS_WITH","$endsWith","endsWith","searchString","endPosition","search","isRegExp","MATCH","re","INCLUDES","includes","repeat","count","str","STARTS_WITH","$startsWith","startsWith","iterated","_t","_i","point","done","LIBRARY","Iterators","$iterCreate","ITERATOR","BUGGY","FF_ITERATOR","KEYS","VALUES","returnThis","Constructor","next","DEFAULT","IS_SET","FORCED","methods","getMethod","kind","values","entries","DEF_VALUES","VALUES_BUG","$native","$default","IteratorPrototype","descriptor","isArrayIter","getIterFn","iter","from","arrayLike","step","iterator","mapfn","mapping","iterFn","ret","getIteratorMethod","SAFE_CLOSING","riter","skipClosing","arr","of","addToUnscopables","Arguments","UNSCOPABLES","copyWithin","to","inc","fill","endPos","$find","forced","find","findIndex","$flags","$RegExp","re1","re2","CORRECT_NEW","piRE","fiU","ignoreCase","multiline","unicode","sticky","flags","regexp","SYMBOL","REPLACE","$replace","searchValue","replaceValue","SEARCH","SPLIT","$split","limit","Wrapper","strictNew","forOf","setProto","same","speciesConstructor","asap","PROMISE","process","isNode","empty","testResolve","sub","promise","resolve","USE_NATIVE","P2","works","then","thenableThenGotten","sameConstructor","getConstructor","isThenable","PromiseCapability","reject","$$resolve","$$reject","perform","error","notify","record","isReject","chain","v","ok","run","reaction","handler","fail","h","setTimeout","console","isUnhandled","emit","onunhandledrejection","reason","_d","$reject","r","$resolve","wrapper","Promise","executor","err","onFulfilled","onRejected","catch","capability","all","iterable","abrupt","remaining","results","alreadyCalled","race","head","last","macrotask","Observer","MutationObserver","WebKitMutationObserver","flush","parent","domain","exit","enter","nextTick","toggle","node","createTextNode","observe","characterData","data","task","defer","channel","port","setTask","setImmediate","clearTask","clearImmediate","MessageChannel","counter","queue","ONREADYSTATECHANGE","listner","event","port2","port1","onmessage","postMessage","addEventListener","importScripts","removeChild","clear","strong","Map","entry","getEntry","redefineAll","$iterDefine","ID","$has","setSpecies","SIZE","fastKey","_f","ADDER","_l","delete","prev","setStrong","$iterDetect","common","IS_WEAK","fixMethod","add","BUGGY_ZERO","instance","HASNT_CHAINING","THROWS_ON_PRIMITIVES","ACCEPT_ITERABLES","Set","weak","frozenStore","WEAK","tmp","$WeakMap","WeakMap","method","arrayFind","arrayFindIndex","FrozenStore","findFrozen","splice","WeakSet","_apply","thisArgument","argumentsList","Reflect","Target","newTarget","$args","propertyKey","attributes","deleteProperty","desc","Enumerate","enumerate","receiver","ownKeys","V","existingDescriptor","ownDesc","$includes","at","$pad","padLeft","maxLength","fillString","left","stringLength","fillStr","intMaxLength","fillLen","stringFiller","padRight","trimLeft","trimRight","$re","escape","regExp","part","getOwnPropertyDescriptors","$values","isEntries","$entries","toJSON","$task","NL","NodeList","HTC","HTMLCollection","NLProto","HTCProto","ArrayValues","partial","navigator","MSIE","userAgent","time","setInterval","path","pargs","_","holder","$ctx","$Array","statics","setStatics","define","amd"],"mappings":";;;;;;CAMC,SAASA,EAAKC,EAAKC,GACpB,cACS,SAAUC,GAKT,QAASC,qBAAoBC,GAG5B,GAAGC,EAAiBD,GACnB,MAAOC,GAAiBD,GAAUE,OAGnC,IAAIC,GAASF,EAAiBD,IAC7BE,WACAE,GAAIJ,EACJK,QAAQ,EAUT,OANAP,GAAQE,GAAUM,KAAKH,EAAOD,QAASC,EAAQA,EAAOD,QAASH,qBAG/DI,EAAOE,QAAS,EAGTF,EAAOD,QAvBf,GAAID,KAqCJ,OATAF,qBAAoBQ,EAAIT,EAGxBC,oBAAoBS,EAAIP,EAGxBF,oBAAoBU,EAAI,GAGjBV,oBAAoB,KAK/B,SAASI,EAAQD,EAASH,GAE/BA,EAAoB,GACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,IACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBA,EAAoB,KACpBI,EAAOD,QAAUH,EAAoB,MAKhC,SAASI,EAAQD,EAASH,GAG/B,GA8BIW,GA9BAC,EAAoBZ,EAAoB,GACxCa,EAAoBb,EAAoB,GACxCc,EAAoBd,EAAoB,GACxCe,EAAoBf,EAAoB,GACxCgB,EAAoBhB,EAAoB,IACxCiB,EAAoBjB,EAAoB,IACxCkB,EAAoBlB,EAAoB,IACxCmB,EAAoBnB,EAAoB,IACxCoB,EAAoBpB,EAAoB,IACxCqB,EAAoBrB,EAAoB,GACxCsB,EAAoBtB,EAAoB,IACxCuB,EAAoBvB,EAAoB,IACxCwB,EAAoBxB,EAAoB,IACxCyB,EAAoBzB,EAAoB,IACxC0B,EAAoB1B,EAAoB,IACxC2B,EAAoB3B,EAAoB,IACxC4B,EAAoB5B,EAAoB,IACxC6B,EAAoB7B,EAAoB,IACxC8B,EAAoB9B,EAAoB,IACxC+B,EAAoB/B,EAAoB,IAAI,aAC5CgC,EAAoBhC,EAAoB,IACxCiC,EAAoBjC,EAAoB,KAAI,GAC5CkC,EAAoBC,OAAOC,UAC3BC,EAAoBC,MAAMF,UAC1BG,EAAoBF,EAAWG,MAC/BC,EAAoBJ,EAAWK,KAC/BC,EAAoB/B,EAAEgC,QACtBC,EAAoBjC,EAAEkC,QACtBC,EAAoBnC,EAAEoC,SACtBC,IAGAnC,KACFH,GAAkBU,EAAM,WACtB,MAA4E,IAArEsB,EAAe1B,EAAI,OAAQ,KAAMiC,IAAK,WAAY,MAAO,MAAOC,IAEzEvC,EAAEgC,QAAU,SAASQ,EAAGC,EAAGC,GACzB,GAAG3C,EAAe,IAChB,MAAOgC,GAAeS,EAAGC,EAAGC,GAC5B,MAAMC,IACR,GAAG,OAASD,IAAc,OAASA,GAAW,KAAME,WAAU,2BAE9D,OADG,SAAWF,KAAWhC,EAAS8B,GAAGC,GAAKC,EAAWG,OAC9CL,GAETxC,EAAEkC,QAAU,SAASM,EAAGC,GACtB,GAAG1C,EAAe,IAChB,MAAOkC,GAAiBO,EAAGC,GAC3B,MAAME,IACR,MAAGrC,GAAIkC,EAAGC,GAAUtC,GAAYmB,EAAYwB,qBAAqBnD,KAAK6C,EAAGC,GAAID,EAAEC,IAA/E,QAEFzC,EAAEoC,SAAWD,EAAmB,SAASK,EAAGO,GAC1CrC,EAAS8B,EAKT,KAJA,GAGIC,GAHAO,EAAShD,EAAEiD,QAAQF,GACnBG,EAASF,EAAKE,OACdC,EAAI,EAEFD,EAASC,GAAEnD,EAAEgC,QAAQQ,EAAGC,EAAIO,EAAKG,KAAMJ,EAAWN,GACxD,OAAOD,KAGXvC,EAAQA,EAAQmD,EAAInD,EAAQoD,GAAKnD,EAAa,UAE5CoD,yBAA0BtD,EAAEkC,QAE5BH,eAAgB/B,EAAEgC,QAElBG,iBAAkBA,GAIpB,IAAIoB,GAAQ,gGACmCC,MAAM,KAEjDC,EAAQF,EAAMG,OAAO,SAAU,aAC/BC,EAAWJ,EAAML,OAGjBU,EAAa,WAEf,GAGIC,GAHAC,EAASzD,EAAI,UACb8C,EAASQ,EACTI,EAAS,GAYb,KAVAD,EAAOE,MAAMC,QAAU,OACvB7D,EAAK8D,YAAYJ,GACjBA,EAAOK,IAAM,cAGbN,EAAiBC,EAAOM,cAAcC,SACtCR,EAAeS,OACfT,EAAeU,MAAM,oCAAsCR,GAC3DF,EAAeW,QACfZ,EAAaC,EAAeR,EACtBF,WAAWS,GAAWpC,UAAU+B,EAAMJ,GAC5C,OAAOS,MAELa,EAAgB,SAASC,EAAOxB,GAClC,MAAO,UAASyB,GACd,GAGIC,GAHApC,EAAS1B,EAAU6D,GACnBxB,EAAS,EACT0B,IAEJ,KAAID,IAAOpC,GAAKoC,GAAOzD,GAASb,EAAIkC,EAAGoC,IAAQC,EAAOC,KAAKF,EAE3D,MAAM1B,EAASC,GAAK7C,EAAIkC,EAAGoC,EAAMF,EAAMvB,SACpC9B,EAAawD,EAAQD,IAAQC,EAAOC,KAAKF,GAE5C,OAAOC,KAGPE,EAAQ,YACZ9E,GAAQA,EAAQmD,EAAG,UAEjB4B,eAAgBhF,EAAEiF,SAAWjF,EAAEiF,UAAY,SAASzC,GAElD,MADAA,GAAI3B,EAAS2B,GACVlC,EAAIkC,EAAGrB,GAAiBqB,EAAErB,GACF,kBAAjBqB,GAAE0C,aAA6B1C,YAAaA,GAAE0C,YAC/C1C,EAAE0C,YAAY1D,UACdgB,YAAajB,QAASD,EAAc,MAG/C6D,oBAAqBnF,EAAEoF,SAAWpF,EAAEoF,UAAYX,EAAchB,EAAOA,EAAMP,QAAQ,GAEnFmC,OAAQrF,EAAEqF,OAASrF,EAAEqF,QAAU,SAAS7C,EAAQO,GAC9C,GAAI8B,EAQJ,OAPS,QAANrC,GACDuC,EAAMvD,UAAYd,EAAS8B,GAC3BqC,EAAS,GAAIE,GACbA,EAAMvD,UAAY,KAElBqD,EAAO1D,GAAYqB,GACdqC,EAASjB,IACTb,IAAe7D,EAAY2F,EAAS1C,EAAiB0C,EAAQ9B,IAGtEC,KAAMhD,EAAEiD,QAAUjD,EAAEiD,SAAWwB,EAAclB,EAAOI,GAAU,IAGhE,IAAI2B,GAAY,SAASjC,EAAGkC,EAAKC,GAC/B,KAAKD,IAAOlD,IAAW,CACrB,IAAI,GAAIoD,MAAQtC,EAAI,EAAOoC,EAAJpC,EAASA,IAAIsC,EAAEtC,GAAK,KAAOA,EAAI,GACtDd,GAAUkD,GAAOG,SAAS,MAAO,gBAAkBD,EAAE3D,KAAK,KAAO,KAEnE,MAAOO,GAAUkD,GAAKlC,EAAGmC,GAI3BvF,GAAQA,EAAQwC,EAAG,YACjBkD,KAAM,QAASA,MAAKC,GAClB,GAAIC,GAAWlF,EAAUmF,MACrBC,EAAWpE,EAAWhC,KAAKqG,UAAW,GACtCC,EAAQ,WACV,GAAIT,GAAOO,EAASrC,OAAO/B,EAAWhC,KAAKqG,WAC3C,OAAOF,gBAAgBG,GAAQX,EAAUO,EAAIL,EAAKtC,OAAQsC,GAAQhF,EAAOqF,EAAIL,EAAMI,GAGrF,OADGhF,GAASiF,EAAGrE,aAAWyE,EAAMzE,UAAYqE,EAAGrE,WACxCyE,KAKXhG,EAAQA,EAAQwC,EAAIxC,EAAQoD,EAAI5C,EAAM,WACjCL,GAAKuB,EAAWhC,KAAKS,KACtB,SACFwB,MAAO,SAASsE,EAAOC,GACrB,GAAIZ,GAAQtE,EAAS6E,KAAK5C,QACtBkD,EAAQ7F,EAAIuF,KAEhB,IADAK,EAAMA,IAAQjH,EAAYqG,EAAMY,EACpB,SAATC,EAAiB,MAAOzE,GAAWhC,KAAKmG,KAAMI,EAAOC,EAMxD,KALA,GAAIE,GAASrF,EAAQkF,EAAOX,GACxBe,EAAStF,EAAQmF,EAAKZ,GACtBgB,EAAStF,EAASqF,EAAOD,GACzBG,EAAS9E,MAAM6E,GACfpD,EAAS,EACHoD,EAAJpD,EAAUA,IAAIqD,EAAOrD,GAAc,UAATiD,EAC5BN,KAAKW,OAAOJ,EAAQlD,GACpB2C,KAAKO,EAAQlD,EACjB,OAAOqD,MAGXvG,EAAQA,EAAQwC,EAAIxC,EAAQoD,GAAKnC,GAAWK,QAAS,SACnDO,KAAM,QAASA,MAAK4E,GAClB,MAAO7E,GAAUlC,KAAKuB,EAAQ4E,MAAOY,IAAcxH,EAAY,IAAMwH,MAKzEzG,EAAQA,EAAQmD,EAAG,SAAUuD,QAASvH,EAAoB,KAE1D,IAAIwH,GAAoB,SAASC,GAC/B,MAAO,UAASC,EAAYC,GAC1BpG,EAAUmG,EACV,IAAItE,GAAStB,EAAQ4E,MACjB5C,EAASjC,EAASuB,EAAEU,QACpB8D,EAASH,EAAU3D,EAAS,EAAI,EAChCC,EAAS0D,EAAU,GAAK,CAC5B,IAAGb,UAAU9C,OAAS,EAAE,OAAO,CAC7B,GAAG8D,IAASxE,GAAE,CACZuE,EAAOvE,EAAEwE,GACTA,GAAS7D,CACT,OAGF,GADA6D,GAAS7D,EACN0D,EAAkB,EAARG,EAAsBA,GAAV9D,EACvB,KAAMN,WAAU,+CAGpB,KAAKiE,EAAUG,GAAS,EAAI9D,EAAS8D,EAAOA,GAAS7D,EAAK6D,IAASxE,KACjEuE,EAAOD,EAAWC,EAAMvE,EAAEwE,GAAQA,EAAOlB,MAE3C,OAAOiB,KAIPE,EAAY,SAASC,GACvB,MAAO,UAASC,GACd,MAAOD,GAAIpB,KAAMqB,EAAMnB,UAAU,KAIrC/F,GAAQA,EAAQwC,EAAG,SAEjB2E,QAASpH,EAAEqH,KAAOrH,EAAEqH,MAAQJ,EAAU7F,EAAkB,IAExDkG,IAAKL,EAAU7F,EAAkB,IAEjCmG,OAAQN,EAAU7F,EAAkB,IAEpCoG,KAAMP,EAAU7F,EAAkB,IAElCqG,MAAOR,EAAU7F,EAAkB,IAEnCsG,OAAQd,GAAkB,GAE1Be,YAAaf,GAAkB,GAE/BgB,QAASX,EAAU5F,GAEnBwG,YAAa,SAASC,EAAIC,GACxB,GAAIvF,GAAS1B,EAAUgF,MACnB5C,EAASjC,EAASuB,EAAEU,QACpB8D,EAAS9D,EAAS,CAGtB,KAFG8C,UAAU9C,OAAS,IAAE8D,EAAQgB,KAAKC,IAAIjB,EAAOjG,EAAUgH,KAC/C,EAARf,IAAUA,EAAQ/F,EAASiC,EAAS8D,IAClCA,GAAS,EAAGA,IAAQ,GAAGA,IAASxE,IAAKA,EAAEwE,KAAWc,EAAG,MAAOd,EACjE,OAAO,MAKX/G,EAAQA,EAAQmD,EAAG,QAAS8E,IAAK,WAAY,OAAQ,GAAIC,QAEzD,IAAIC,GAAK,SAASC,GAChB,MAAOA,GAAM,EAAIA,EAAM,IAAMA,EAK/BpI,GAAQA,EAAQwC,EAAIxC,EAAQoD,GAAK5C,EAAM,WACrC,MAA4C,4BAArC,GAAI0H,MAAK,MAAQ,GAAGG,kBACtB7H,EAAM,WACX,GAAI0H,MAAKI,KAAKD,iBACX,QACHA,YAAa,QAASA,eACpB,IAAIE,SAAS1C,MAAM,KAAM2C,YAAW,qBACpC,IAAIC,GAAI5C,KACJ6C,EAAID,EAAEE,iBACNhJ,EAAI8I,EAAEG,qBACNC,EAAQ,EAAJH,EAAQ,IAAMA,EAAI,KAAO,IAAM,EACvC,OAAOG,IAAK,QAAUd,KAAKe,IAAIJ,IAAI/G,MAAMkH,EAAI,GAAK,IAChD,IAAMV,EAAGM,EAAEM,cAAgB,GAAK,IAAMZ,EAAGM,EAAEO,cAC3C,IAAMb,EAAGM,EAAEQ,eAAiB,IAAMd,EAAGM,EAAES,iBACvC,IAAMf,EAAGM,EAAEU,iBAAmB,KAAOxJ,EAAI,GAAKA,EAAI,IAAMwI,EAAGxI,IAAM,QAMlE,SAASJ,EAAQD,GAEtB,GAAI8J,GAAU9H,MACd/B,GAAOD,SACL8F,OAAYgE,EAAQhE,OACpBJ,SAAYoE,EAAQrE,eACpBsE,UAAexG,qBACfZ,QAAYmH,EAAQ/F,yBACpBtB,QAAYqH,EAAQtH,eACpBK,SAAYiH,EAAQlH,iBACpBc,QAAYoG,EAAQrG,KACpBoC,SAAYiE,EAAQlE,oBACpBoE,WAAYF,EAAQG,sBACpBnC,QAAeD,UAKZ,SAAS5H,EAAQD,EAASH,GAE/B,GAAIqK,GAAYrK,EAAoB,GAChCsK,EAAYtK,EAAoB,GAChCuK,EAAYvK,EAAoB,GAChCwK,EAAYxK,EAAoB,IAChCyK,EAAYzK,EAAoB,IAChC0K,EAAY,YAEZ7J,EAAU,SAAS8J,EAAMC,EAAMC,GACjC,GAQIrF,GAAKsF,EAAKC,EAAKC,EARfC,EAAYN,EAAO9J,EAAQoD,EAC3BiH,EAAYP,EAAO9J,EAAQsK,EAC3BC,EAAYT,EAAO9J,EAAQmD,EAC3BqH,EAAYV,EAAO9J,EAAQwC,EAC3BiI,EAAYX,EAAO9J,EAAQ0K,EAC3BC,EAAYN,EAAYb,EAASe,EAAYf,EAAOO,KAAUP,EAAOO,QAAeP,EAAOO,QAAaF,GACxGvK,EAAY+K,EAAYZ,EAAOA,EAAKM,KAAUN,EAAKM,OACnDa,EAAYtL,EAAQuK,KAAevK,EAAQuK,MAE5CQ,KAAUL,EAASD,EACtB,KAAIpF,IAAOqF,GAETC,GAAOG,GAAaO,GAAUhG,IAAOgG,GAErCT,GAAOD,EAAMU,EAASX,GAAQrF,GAE9BwF,EAAMM,GAAWR,EAAML,EAAIM,EAAKV,GAAUgB,GAA0B,kBAAPN,GAAoBN,EAAInE,SAAS/F,KAAMwK,GAAOA,EAExGS,IAAWV,GAAIN,EAASgB,EAAQhG,EAAKuF,GAErC5K,EAAQqF,IAAQuF,GAAIR,EAAKpK,EAASqF,EAAKwF,GACvCK,GAAYI,EAASjG,IAAQuF,IAAIU,EAASjG,GAAOuF,GAGxDV,GAAOC,KAAOA,EAEdzJ,EAAQoD,EAAI,EACZpD,EAAQsK,EAAI,EACZtK,EAAQmD,EAAI,EACZnD,EAAQwC,EAAI,EACZxC,EAAQ0K,EAAI,GACZ1K,EAAQ6K,EAAI,GACZtL,EAAOD,QAAUU,GAIZ,SAAST,EAAQD,GAGtB,GAAIkK,GAASjK,EAAOD,QAA2B,mBAAVwL,SAAyBA,OAAO/C,MAAQA,KACzE+C,OAAwB,mBAARC,OAAuBA,KAAKhD,MAAQA,KAAOgD,KAAOtF,SAAS,gBAC9D,iBAAPzG,KAAgBA,EAAMwK,IAI3B,SAASjK,EAAQD,GAEtB,GAAImK,GAAOlK,EAAOD,SAAW0L,QAAS,QACrB,iBAAPjM,KAAgBA,EAAM0K,IAI3B,SAASlK,EAAQD,EAASH,GAE/B,GAAIY,GAAaZ,EAAoB,GACjCe,EAAaf,EAAoB,EACrCI,GAAOD,QAAUH,EAAoB,GAAK,SAASuF,EAAQC,EAAK/B,GAC9D,MAAO7C,GAAEgC,QAAQ2C,EAAQC,EAAKzE,EAAW,EAAG0C,KAC1C,SAAS8B,EAAQC,EAAK/B,GAExB,MADA8B,GAAOC,GAAO/B,EACP8B,IAKJ,SAASnF,EAAQD,GAEtBC,EAAOD,QAAU,SAAS2L,EAAQrI,GAChC,OACEsI,aAAyB,EAATD,GAChBE,eAAyB,EAATF,GAChBG,WAAyB,EAATH,GAChBrI,MAAcA,KAMb,SAASrD,EAAQD,EAASH,GAG/BI,EAAOD,SAAWH,EAAoB,GAAG,WACvC,MAA2E,IAApEmC,OAAOQ,kBAAmB,KAAMO,IAAK,WAAY,MAAO,MAAOC,KAKnE,SAAS/C,EAAQD,GAEtBC,EAAOD,QAAU,SAAS+L,GACxB,IACE,QAASA,IACT,MAAM3I,GACN,OAAO,KAMN,SAASnD,EAAQD,EAASH,GAI/B,GAAIqK,GAAYrK,EAAoB,GAChCuK,EAAYvK,EAAoB,GAChCmM,EAAYnM,EAAoB,IAAI,OACpCoM,EAAY,WACZC,EAAY/F,SAAS8F,GACrBE,GAAa,GAAKD,GAAWjI,MAAMgI,EAEvCpM,GAAoB,GAAGuM,cAAgB,SAASC,GAC9C,MAAOH,GAAU9L,KAAKiM,KAGvBpM,EAAOD,QAAU,SAASiD,EAAGoC,EAAKiH,EAAKC,GACrB,kBAAPD,KACRA,EAAIE,eAAeR,IAAQ5B,EAAKkC,EAAKN,EAAK/I,EAAEoC,GAAO,GAAKpC,EAAEoC,GAAO8G,EAAI5J,KAAKkK,OAAOpH,KACjFiH,EAAIE,eAAe,SAAWpC,EAAKkC,EAAK,OAAQjH,IAE/CpC,IAAMiH,EACPjH,EAAEoC,GAAOiH,GAELC,SAAYtJ,GAAEoC,GAClB+E,EAAKnH,EAAGoC,EAAKiH,MAEdnG,SAASlE,UAAWgK,EAAW,QAASS,YACzC,MAAsB,kBAARnG,OAAsBA,KAAKyF,IAAQE,EAAU9L,KAAKmG,SAK7D,SAAStG,EAAQD,GAEtB,GAAIE,GAAK,EACLyM,EAAKlE,KAAKmE,QACd3M,GAAOD,QAAU,SAASqF,GACxB,MAAO,UAAUlB,OAAOkB,IAAQ1F,EAAY,GAAK0F,EAAK,QAASnF,EAAKyM,GAAID,SAAS,OAK9E,SAASzM,EAAQD,EAASH,GAG/B,GAAIuB,GAAYvB,EAAoB,GACpCI,GAAOD,QAAU,SAASsG,EAAID,EAAM1C,GAElC,GADAvC,EAAUkF,GACPD,IAAS1G,EAAU,MAAO2G,EAC7B,QAAO3C,GACL,IAAK,GAAG,MAAO,UAASX,GACtB,MAAOsD,GAAGlG,KAAKiG,EAAMrD,GAEvB,KAAK,GAAG,MAAO,UAASA,EAAG6J,GACzB,MAAOvG,GAAGlG,KAAKiG,EAAMrD,EAAG6J,GAE1B,KAAK,GAAG,MAAO,UAAS7J,EAAG6J,EAAGvM,GAC5B,MAAOgG,GAAGlG,KAAKiG,EAAMrD,EAAG6J,EAAGvM,IAG/B,MAAO,YACL,MAAOgG,GAAGwG,MAAMzG,EAAMI,cAMrB,SAASxG,EAAQD,GAEtBC,EAAOD,QAAU,SAASqM,GACxB,GAAgB,kBAANA,GAAiB,KAAMhJ,WAAUgJ,EAAK,sBAChD,OAAOA,KAKJ,SAASpM,EAAQD,EAASH,GAE/BI,EAAOD,QAAUH,EAAoB,GAAGiF,UAAYA,SAASiI,iBAIxD,SAAS9M,EAAQD,EAASH,GAE/B,GAAIwB,GAAWxB,EAAoB,IAC/BiF,EAAWjF,EAAoB,GAAGiF,SAElCkI,EAAK3L,EAASyD,IAAazD,EAASyD,EAASmI,cACjDhN,GAAOD,QAAU,SAASqM,GACxB,MAAOW,GAAKlI,EAASmI,cAAcZ,QAKhC,SAASpM,EAAQD,GAEtBC,EAAOD,QAAU,SAASqM,GACxB,MAAqB,gBAAPA,GAAyB,OAAPA,EAA4B,kBAAPA,KAKlD,SAASpM,EAAQD,GAEtB,GAAIwM,MAAoBA,cACxBvM,GAAOD,QAAU,SAASqM,EAAIhH,GAC5B,MAAOmH,GAAepM,KAAKiM,EAAIhH,KAK5B,SAASpF,EAAQD,GAEtB,GAAI0M,MAAcA,QAElBzM,GAAOD,QAAU,SAASqM,GACxB,MAAOK,GAAStM,KAAKiM,GAAIhK,MAAM,EAAG,MAK/B,SAASpC,EAAQD,GAGtBC,EAAOD,QAAU,SAASsG,EAAIL,EAAMI,GAClC,GAAI6G,GAAK7G,IAAS1G,CAClB,QAAOsG,EAAKtC,QACV,IAAK,GAAG,MAAOuJ,GAAK5G,IACAA,EAAGlG,KAAKiG,EAC5B,KAAK,GAAG,MAAO6G,GAAK5G,EAAGL,EAAK,IACRK,EAAGlG,KAAKiG,EAAMJ,EAAK,GACvC,KAAK,GAAG,MAAOiH,GAAK5G,EAAGL,EAAK,GAAIA,EAAK,IACjBK,EAAGlG,KAAKiG,EAAMJ,EAAK,GAAIA,EAAK,GAChD,KAAK,GAAG,MAAOiH,GAAK5G,EAAGL,EAAK,GAAIA,EAAK,GAAIA,EAAK,IAC1BK,EAAGlG,KAAKiG,EAAMJ,EAAK,GAAIA,EAAK,GAAIA,EAAK,GACzD,KAAK,GAAG,MAAOiH,GAAK5G,EAAGL,EAAK,GAAIA,EAAK,GAAIA,EAAK,GAAIA,EAAK,IACnCK,EAAGlG,KAAKiG,EAAMJ,EAAK,GAAIA,EAAK,GAAIA,EAAK,GAAIA,EAAK,IAClE,MAAoBK,GAAGwG,MAAMzG,EAAMJ,KAKlC,SAAShG,EAAQD,EAASH,GAE/B,GAAIwB,GAAWxB,EAAoB,GACnCI,GAAOD,QAAU,SAASqM,GACxB,IAAIhL,EAASgL,GAAI,KAAMhJ,WAAUgJ,EAAK,qBACtC,OAAOA,KAKJ,SAASpM,EAAQD,EAASH,GAG/B,GAAIsN,GAAUtN,EAAoB,GAClCI,GAAOD,QAAU,SAASqM,GACxB,MAAOrK,QAAOmL,EAAQd,MAKnB,SAASpM,EAAQD,GAGtBC,EAAOD,QAAU,SAASqM,GACxB,GAAGA,GAAM1M,EAAU,KAAM0D,WAAU,yBAA2BgJ,EAC9D,OAAOA,KAKJ,SAASpM,EAAQD,EAASH,GAG/B,GAAI8B,GAAU9B,EAAoB,IAC9BsN,EAAUtN,EAAoB,GAClCI,GAAOD,QAAU,SAASqM,GACxB,MAAO1K,GAAQwL,EAAQd,MAKpB,SAASpM,EAAQD,EAASH,GAG/B,GAAImB,GAAMnB,EAAoB,GAC9BI,GAAOD,QAAUgC,OAAO,KAAKuB,qBAAqB,GAAKvB,OAAS,SAASqK,GACvE,MAAkB,UAAXrL,EAAIqL,GAAkBA,EAAGpI,MAAM,IAAMjC,OAAOqK,KAKhD,SAASpM,EAAQD,GAGtB,GAAIoN,GAAQ3E,KAAK2E,KACbC,EAAQ5E,KAAK4E,KACjBpN,GAAOD,QAAU,SAASqM,GACxB,MAAOiB,OAAMjB,GAAMA,GAAM,GAAKA,EAAK,EAAIgB,EAAQD,GAAMf,KAKlD,SAASpM,EAAQD,EAASH,GAE/B,GAAI2B,GAAY3B,EAAoB,IAChC0N,EAAY9E,KAAK8E,IACjB7E,EAAYD,KAAKC,GACrBzI,GAAOD,QAAU,SAASyH,EAAO9D,GAE/B,MADA8D,GAAQjG,EAAUiG,GACH,EAARA,EAAY8F,EAAI9F,EAAQ9D,EAAQ,GAAK+E,EAAIjB,EAAO9D,KAKpD,SAAS1D,EAAQD,EAASH,GAG/B,GAAI2B,GAAY3B,EAAoB,IAChC6I,EAAYD,KAAKC,GACrBzI,GAAOD,QAAU,SAASqM,GACxB,MAAOA,GAAK,EAAI3D,EAAIlH,EAAU6K,GAAK,kBAAoB,IAKpD,SAASpM,EAAQD,EAASH,GAS/B,GAAIyK,GAAWzK,EAAoB,IAC/B8B,EAAW9B,EAAoB,IAC/ByB,EAAWzB,EAAoB,IAC/B6B,EAAW7B,EAAoB,IAC/B2N,EAAW3N,EAAoB,GACnCI,GAAOD,QAAU,SAASyN,GACxB,GAAIC,GAAwB,GAARD,EAChBE,EAAwB,GAARF,EAChBG,EAAwB,GAARH,EAChBI,EAAwB,GAARJ,EAChBK,EAAwB,GAARL,EAChBM,EAAwB,GAARN,GAAaK,CACjC,OAAO,UAASE,EAAOzG,EAAYlB,GAQjC,IAPA,GAMIiG,GAAK2B,EANLhL,EAAS3B,EAAS0M,GAClBvC,EAAS9J,EAAQsB,GACjBiL,EAAS5D,EAAI/C,EAAYlB,EAAM,GAC/B1C,EAASjC,EAAS+J,EAAK9H,QACvB8D,EAAS,EACTnC,EAASoI,EAASF,EAAIQ,EAAOrK,GAAUgK,EAAYH,EAAIQ,EAAO,GAAKrO,EAElEgE,EAAS8D,EAAOA,IAAQ,IAAGsG,GAAYtG,IAASgE,MACnDa,EAAMb,EAAKhE,GACXwG,EAAMC,EAAE5B,EAAK7E,EAAOxE,GACjBwK,GACD,GAAGC,EAAOpI,EAAOmC,GAASwG,MACrB,IAAGA,EAAI,OAAOR,GACjB,IAAK,GAAG,OAAO,CACf,KAAK,GAAG,MAAOnB,EACf,KAAK,GAAG,MAAO7E,EACf,KAAK,GAAGnC,EAAOC,KAAK+G,OACf,IAAGuB,EAAS,OAAO,CAG9B,OAAOC,GAAgB,GAAKF,GAAWC,EAAWA,EAAWvI,KAM5D,SAASrF,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,IAC/BuH,EAAWvH,EAAoB,IAC/BsO,EAAWtO,EAAoB,IAAI,UACvCI,GAAOD,QAAU,SAASoO,EAAUzK,GAClC,GAAI0K,EASF,OARCjH,GAAQgH,KACTC,EAAID,EAASzI,YAEE,kBAAL0I,IAAoBA,IAAMlM,QAASiF,EAAQiH,EAAEpM,aAAYoM,EAAI1O,GACpE0B,EAASgN,KACVA,EAAIA,EAAEF,GACG,OAANE,IAAWA,EAAI1O,KAEb,IAAK0O,IAAM1O,EAAYwC,MAAQkM,GAAG1K,KAKxC,SAAS1D,EAAQD,EAASH,GAG/B,GAAImB,GAAMnB,EAAoB,GAC9BI,GAAOD,QAAUmC,MAAMiF,SAAW,SAASkH,GACzC,MAAmB,SAAZtN,EAAIsN,KAKR,SAASrO,EAAQD,EAASH,GAE/B,GAAI0O,GAAS1O,EAAoB,IAAI,OACjC2O,EAAS3O,EAAoB,IAC7B4O,EAAS5O,EAAoB,GAAG4O,MACpCxO,GAAOD,QAAU,SAASyK,GACxB,MAAO8D,GAAM9D,KAAU8D,EAAM9D,GAC3BgE,GAAUA,EAAOhE,KAAUgE,GAAUD,GAAK,UAAY/D,MAKrD,SAASxK,EAAQD,EAASH,GAE/B,GAAIqK,GAASrK,EAAoB,GAC7B6O,EAAS,qBACTH,EAASrE,EAAOwE,KAAYxE,EAAOwE,MACvCzO,GAAOD,QAAU,SAASqF,GACxB,MAAOkJ,GAAMlJ,KAASkJ,EAAMlJ,SAKzB,SAASpF,EAAQD,EAASH,GAI/B,GAAI0B,GAAY1B,EAAoB,IAChC6B,EAAY7B,EAAoB,IAChC4B,EAAY5B,EAAoB,GACpCI,GAAOD,QAAU,SAAS2O,GACxB,MAAO,UAASX,EAAOzF,EAAIC,GACzB,GAGIlF,GAHAL,EAAS1B,EAAUyM,GACnBrK,EAASjC,EAASuB,EAAEU,QACpB8D,EAAShG,EAAQ+G,EAAW7E,EAGhC,IAAGgL,GAAepG,GAAMA,GAAG,KAAM5E,EAAS8D,GAExC,GADAnE,EAAQL,EAAEwE,KACPnE,GAASA,EAAM,OAAO,MAEpB,MAAKK,EAAS8D,EAAOA,IAAQ,IAAGkH,GAAelH,IAASxE,KAC1DA,EAAEwE,KAAWc,EAAG,MAAOoG,IAAelH,CACzC,QAAQkH,GAAe,MAMxB,SAAS1O,EAAQD,EAASH,GAI/B,GAAIY,GAAiBZ,EAAoB,GACrCqK,EAAiBrK,EAAoB,GACrCkB,EAAiBlB,EAAoB,IACrCc,EAAiBd,EAAoB,GACrCa,EAAiBb,EAAoB,GACrCwK,EAAiBxK,EAAoB,IACrC+O,EAAiB/O,EAAoB,GACrCgP,EAAiBhP,EAAoB,IACrCiP,EAAiBjP,EAAoB,IACrC2O,EAAiB3O,EAAoB,IACrCkP,EAAiBlP,EAAoB,IACrCmP,EAAiBnP,EAAoB,IACrCoP,EAAiBpP,EAAoB,IACrCqP,EAAiBrP,EAAoB,IACrCuH,EAAiBvH,EAAoB,IACrCsB,EAAiBtB,EAAoB,IACrC0B,EAAiB1B,EAAoB,IACrCe,EAAiBf,EAAoB,GACrC8C,EAAiBlC,EAAEkC,QACnBF,EAAiBhC,EAAEgC,QACnB0M,EAAiB1O,EAAEqF,OACnBD,EAAiBoJ,EAAOlM,IACxBqM,EAAiBlF,EAAOuE,OACxBY,EAAiBnF,EAAOoF,KACxBC,EAAiBF,GAASA,EAAMG,UAChCC,GAAiB,EACjBC,EAAiBX,EAAI,WACrBhF,EAAiBtJ,EAAEsJ,OACnB4F,EAAiBd,EAAO,mBACxBe,EAAiBf,EAAO,WACxBgB,EAAmC,kBAAXT,GACxBrN,EAAiBC,OAAOC,UAGxB6N,EAAgBnP,GAAeiO,EAAO,WACxC,MAES,IAFFO,EAAQ1M,KAAY,KACzBM,IAAK,WAAY,MAAON,GAAQ8D,KAAM,KAAMjD,MAAO,IAAIN,MACrDA,IACD,SAASqJ,EAAIhH,EAAK0K,GACrB,GAAIC,GAAYrN,EAAQZ,EAAasD,EAClC2K,UAAiBjO,GAAYsD,GAChC5C,EAAQ4J,EAAIhH,EAAK0K,GACdC,GAAa3D,IAAOtK,GAAYU,EAAQV,EAAasD,EAAK2K,IAC3DvN,EAEAwN,EAAO,SAASC,GAClB,GAAIC,GAAMP,EAAWM,GAAOf,EAAQC,EAAQnN,UAS5C,OARAkO,GAAIC,GAAKF,EACTvP,GAAe8O,GAAUK,EAAc/N,EAAamO,GAClDrE,cAAc,EACdwE,IAAK,SAAS/M,GACTvC,EAAIwF,KAAMmJ,IAAW3O,EAAIwF,KAAKmJ,GAASQ,KAAK3J,KAAKmJ,GAAQQ,IAAO,GACnEJ,EAAcvJ,KAAM2J,EAAKtP,EAAW,EAAG0C,OAGpC6M,GAGLG,EAAW,SAASjE,GACtB,MAAoB,gBAANA,IAGZkE,EAAkB,QAAS/N,gBAAe6J,EAAIhH,EAAK0K,GACrD,MAAGA,IAAKhP,EAAI6O,EAAYvK,IAClB0K,EAAEnE,YAID7K,EAAIsL,EAAIqD,IAAWrD,EAAGqD,GAAQrK,KAAKgH,EAAGqD,GAAQrK,IAAO,GACxD0K,EAAIZ,EAAQY,GAAInE,WAAYhL,EAAW,GAAG,OAJtCG,EAAIsL,EAAIqD,IAAQjN,EAAQ4J,EAAIqD,EAAQ9O,EAAW,OACnDyL,EAAGqD,GAAQrK,IAAO,GAIXyK,EAAczD,EAAIhH,EAAK0K,IACzBtN,EAAQ4J,EAAIhH,EAAK0K,IAExBS,EAAoB,QAAS5N,kBAAiByJ,EAAInJ,GACpD/B,EAASkL,EAKT,KAJA,GAGIhH,GAHA5B,EAAOyL,EAAShM,EAAI3B,EAAU2B,IAC9BU,EAAO,EACP6M,EAAIhN,EAAKE,OAEP8M,EAAI7M,GAAE2M,EAAgBlE,EAAIhH,EAAM5B,EAAKG,KAAMV,EAAEmC,GACnD,OAAOgH,IAELqE,EAAU,QAAS5K,QAAOuG,EAAInJ,GAChC,MAAOA,KAAMvD,EAAYwP,EAAQ9C,GAAMmE,EAAkBrB,EAAQ9C,GAAKnJ,IAEpEyN,EAAwB,QAASpN,sBAAqB8B,GACxD,GAAIuL,GAAI7G,EAAO3J,KAAKmG,KAAMlB,EAC1B,OAAOuL,KAAM7P,EAAIwF,KAAMlB,KAAStE,EAAI6O,EAAYvK,IAAQtE,EAAIwF,KAAMmJ,IAAWnJ,KAAKmJ,GAAQrK,GACtFuL,GAAI,GAENC,EAA4B,QAAS9M,0BAAyBsI,EAAIhH,GACpE,GAAI0K,GAAIpN,EAAQ0J,EAAK9K,EAAU8K,GAAKhH,EAEpC,QADG0K,IAAKhP,EAAI6O,EAAYvK,IAAUtE,EAAIsL,EAAIqD,IAAWrD,EAAGqD,GAAQrK,KAAM0K,EAAEnE,YAAa,GAC9EmE,GAELe,EAAuB,QAASlL,qBAAoByG,GAKtD,IAJA,GAGIhH,GAHAF,EAASU,EAAStE,EAAU8K,IAC5B/G,KACA1B,EAAS,EAEPuB,EAAMxB,OAASC,GAAM7C,EAAI6O,EAAYvK,EAAMF,EAAMvB,OAASyB,GAAOqK,GAAOpK,EAAOC,KAAKF,EAC1F,OAAOC,IAELyL,EAAyB,QAAS9G,uBAAsBoC,GAK1D,IAJA,GAGIhH,GAHAF,EAASU,EAAStE,EAAU8K,IAC5B/G,KACA1B,EAAS,EAEPuB,EAAMxB,OAASC,GAAK7C,EAAI6O,EAAYvK,EAAMF,EAAMvB,OAAM0B,EAAOC,KAAKqK,EAAWvK,GACnF,OAAOC,IAEL0L,EAAa,QAASxB,WAAUnD,GAClC,GAAGA,IAAO1M,IAAa2Q,EAASjE,GAAhC,CAKA,IAJA,GAGI4E,GAAUC,EAHVjL,GAAQoG,GACRzI,EAAO,EACPuN,EAAO1K,UAEL0K,EAAGxN,OAASC,GAAEqC,EAAKV,KAAK4L,EAAGvN,KAQjC,OAPAqN,GAAWhL,EAAK,GACM,kBAAZgL,KAAuBC,EAAYD,IAC1CC,IAAc9J,EAAQ6J,MAAUA,EAAW,SAAS5L,EAAK/B,GAE1D,MADG4N,KAAU5N,EAAQ4N,EAAU9Q,KAAKmG,KAAMlB,EAAK/B,IAC3CgN,EAAShN,GAAb,OAA2BA,IAE7B2C,EAAK,GAAKgL,EACH1B,EAAWzC,MAAMuC,EAAOpJ,KAE7BmL,EAAYxC,EAAO,WACrB,GAAI/K,GAAIuL,GAIR,OAA0B,UAAnBG,GAAY1L,KAAyC,MAAtB0L,GAAYvM,EAAGa,KAAwC,MAAzB0L,EAAWvN,OAAO6B,KAIpFgM,KACFT,EAAU,QAASX,UACjB,GAAG6B,EAAS/J,MAAM,KAAMlD,WAAU,8BAClC,OAAO4M,GAAKzB,EAAI/H,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,KAExD0K,EAAS+E,EAAQnN,UAAW,WAAY,QAASyK,YAC/C,MAAOnG,MAAK6J,KAGdE,EAAW,SAASjE,GAClB,MAAOA,aAAc+C,IAGvB3O,EAAEqF,OAAa4K,EACfjQ,EAAEsJ,OAAa4G,EACflQ,EAAEkC,QAAakO,EACfpQ,EAAEgC,QAAa8N,EACf9P,EAAEoC,SAAa2N,EACf/P,EAAEoF,SAAaoJ,EAAOlM,IAAM+N,EAC5BrQ,EAAEuJ,WAAa+G,EAEZpQ,IAAgBd,EAAoB,KACrCwK,EAAStI,EAAa,uBAAwB4O,GAAuB,GAIzE,IAAIU,IAEFC,MAAO,SAASjM,GACd,MAAOtE,GAAI4O,EAAgBtK,GAAO,IAC9BsK,EAAetK,GACfsK,EAAetK,GAAO+J,EAAQ/J,IAGpCkM,OAAQ,QAASA,QAAOlM,GACtB,MAAO2J,GAAMW,EAAgBtK,IAE/BmM,UAAW,WAAY/B,GAAS,GAChCgC,UAAW,WAAYhC,GAAS,GAalChP,GAAEqH,KAAK1H,KAAK,iHAGV6D,MAAM,KAAM,SAASoI,GACrB,GAAI8D,GAAMpB,EAAI1C,EACdgF,GAAchF,GAAMwD,EAAYM,EAAMF,EAAKE,KAG7CV,GAAS,EAET/O,EAAQA,EAAQsK,EAAItK,EAAQ6K,GAAIkD,OAAQW,IAExC1O,EAAQA,EAAQmD,EAAG,SAAUwN,GAE7B3Q,EAAQA,EAAQmD,EAAInD,EAAQoD,GAAK+L,EAAW,UAE1C/J,OAAQ4K,EAERlO,eAAgB+N,EAEhB3N,iBAAkB4N,EAElBzM,yBAA0B8M,EAE1BjL,oBAAqBkL,EAErB7G,sBAAuB8G,IAIzB1B,GAAS3O,EAAQA,EAAQmD,EAAInD,EAAQoD,IAAM+L,GAAauB,GAAY,QAAS5B,UAAWwB,IAGxFlC,EAAeM,EAAS,UAExBN,EAAerG,KAAM,QAAQ,GAE7BqG,EAAe5E,EAAOoF,KAAM,QAAQ,IAI/B,SAASrP,EAAQD,EAASH,GAE/B,GAAI6R,GAAM7R,EAAoB,GAAG4C,QAC7B1B,EAAMlB,EAAoB,IAC1B8R,EAAM9R,EAAoB,IAAI,cAElCI,GAAOD,QAAU,SAASqM,EAAI6D,EAAK0B,GAC9BvF,IAAOtL,EAAIsL,EAAKuF,EAAOvF,EAAKA,EAAGpK,UAAW0P,IAAKD,EAAIrF,EAAIsF,GAAM9F,cAAc,EAAMvI,MAAO4M,MAKxF,SAASjQ,EAAQD,EAASH,GAE/B,GAAIY,GAAYZ,EAAoB,GAChC0B,EAAY1B,EAAoB,GACpCI,GAAOD,QAAU,SAASoF,EAAQmD,GAMhC,IALA,GAIIlD,GAJApC,EAAS1B,EAAU6D,GACnB3B,EAAShD,EAAEiD,QAAQT,GACnBU,EAASF,EAAKE,OACd8D,EAAS,EAEP9D,EAAS8D,GAAM,GAAGxE,EAAEoC,EAAM5B,EAAKgE,QAAcc,EAAG,MAAOlD,KAK1D,SAASpF,EAAQD,EAASH,GAG/B,GAAI0B,GAAY1B,EAAoB,IAChCgG,EAAYhG,EAAoB,GAAGgG,SACnC6G,KAAeA,SAEfmF,EAA+B,gBAAVrG,SAAsBxJ,OAAO4D,oBAClD5D,OAAO4D,oBAAoB4F,WAE3BsG,EAAiB,SAASzF,GAC5B,IACE,MAAOxG,GAASwG,GAChB,MAAMjJ,GACN,MAAOyO,GAAYxP,SAIvBpC,GAAOD,QAAQ+C,IAAM,QAAS6C,qBAAoByG,GAChD,MAAGwF,IAAoC,mBAArBnF,EAAStM,KAAKiM,GAAgCyF,EAAezF,GACxExG,EAAStE,EAAU8K,MAKvB,SAASpM,EAAQD,EAASH,GAG/B,GAAIY,GAAIZ,EAAoB,EAC5BI,GAAOD,QAAU,SAASqM,GACxB,GAAI5I,GAAahD,EAAEiD,QAAQ2I,GACvBrC,EAAavJ,EAAEuJ,UACnB,IAAGA,EAKD,IAJA,GAGI3E,GAHA0M,EAAU/H,EAAWqC,GACrBtC,EAAUtJ,EAAEsJ,OACZnG,EAAU,EAERmO,EAAQpO,OAASC,GAAKmG,EAAO3J,KAAKiM,EAAIhH,EAAM0M,EAAQnO,OAAMH,EAAK8B,KAAKF,EAE5E,OAAO5B,KAKJ,SAASxD,EAAQD,GAEtBC,EAAOD,SAAU,GAIZ,SAASC,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAG,UAAWkO,OAAQnS,EAAoB,OAIjE,SAASI,EAAQD,EAASH,GAG/B,GAAIY,GAAWZ,EAAoB,GAC/ByB,EAAWzB,EAAoB,IAC/B8B,EAAW9B,EAAoB,GAGnCI,GAAOD,QAAUH,EAAoB,GAAG,WACtC,GAAImD,GAAIhB,OAAOgQ,OACXC,KACA7G,KACAvH,EAAI4K,SACJyD,EAAI,sBAGR,OAFAD,GAAEpO,GAAK,EACPqO,EAAEjO,MAAM,IAAI4D,QAAQ,SAASsK,GAAI/G,EAAE+G,GAAKA,IAClB,GAAfnP,KAAMiP,GAAGpO,IAAW7B,OAAOyB,KAAKT,KAAMoI,IAAI7I,KAAK,KAAO2P,IAC1D,QAASF,QAAO3G,EAAQX,GAQ3B,IAPA,GAAI0H,GAAQ9Q,EAAS+J,GACjB8F,EAAQ1K,UACR4L,EAAQlB,EAAGxN,OACX8D,EAAQ,EACR/D,EAAajD,EAAEiD,QACfsG,EAAavJ,EAAEuJ,WACfD,EAAatJ,EAAEsJ,OACbsI,EAAQ5K,GAMZ,IALA,GAIIpC,GAJAxB,EAASlC,EAAQwP,EAAG1J,MACpBhE,EAASuG,EAAatG,EAAQG,GAAGM,OAAO6F,EAAWnG,IAAMH,EAAQG,GACjEF,EAASF,EAAKE,OACd2O,EAAS,EAEP3O,EAAS2O,GAAKvI,EAAO3J,KAAKyD,EAAGwB,EAAM5B,EAAK6O,QAAMF,EAAE/M,GAAOxB,EAAEwB,GAEjE,OAAO+M,IACLpQ,OAAOgQ,QAIN,SAAS/R,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAClCa,GAAQA,EAAQmD,EAAG,UAAWmJ,GAAInN,EAAoB,OAIjD,SAASI,EAAQD,GAGtBC,EAAOD,QAAUgC,OAAOgL,IAAM,QAASA,IAAGuF,EAAGnJ,GAC3C,MAAOmJ,KAAMnJ,EAAU,IAANmJ,GAAW,EAAIA,IAAM,EAAInJ,EAAImJ,GAAKA,GAAKnJ,GAAKA,IAK1D,SAASnJ,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAClCa,GAAQA,EAAQmD,EAAG,UAAW2O,eAAgB3S,EAAoB,IAAIwQ,OAIjE,SAASpQ,EAAQD,EAASH,GAI/B,GAAI8C,GAAW9C,EAAoB,GAAG8C,QAClCtB,EAAWxB,EAAoB,IAC/BsB,EAAWtB,EAAoB,IAC/B4S,EAAQ,SAASxP,EAAGyP,GAEtB,GADAvR,EAAS8B,IACL5B,EAASqR,IAAoB,OAAVA,EAAe,KAAMrP,WAAUqP,EAAQ,6BAEhEzS,GAAOD,SACLqQ,IAAKrO,OAAOwQ,iBAAmB,gBAC7B,SAASG,EAAMC,EAAOvC,GACpB,IACEA,EAAMxQ,EAAoB,IAAIsG,SAAS/F,KAAMuC,EAAQX,OAAOC,UAAW,aAAaoO,IAAK,GACzFA,EAAIsC,MACJC,IAAUD,YAAgBxQ,QAC1B,MAAMiB,GAAIwP,GAAQ,EACpB,MAAO,SAASJ,gBAAevP,EAAGyP,GAIhC,MAHAD,GAAMxP,EAAGyP,GACNE,EAAM3P,EAAE4P,UAAYH,EAClBrC,EAAIpN,EAAGyP,GACLzP,QAEL,GAAStD,GACjB8S,MAAOA,IAKJ,SAASxS,EAAQD,EAASH,GAI/B,GAAIiT,GAAUjT,EAAoB,IAC9B8S,IACJA,GAAK9S,EAAoB,IAAI,gBAAkB,IAC5C8S,EAAO,IAAM,cACd9S,EAAoB,IAAImC,OAAOC,UAAW,WAAY,QAASyK,YAC7D,MAAO,WAAaoG,EAAQvM,MAAQ,MACnC,IAKA,SAAStG,EAAQD,EAASH,GAG/B,GAAImB,GAAMnB,EAAoB,IAC1B8R,EAAM9R,EAAoB,IAAI,eAE9BkT,EAAgD,aAA1C/R,EAAI,WAAY,MAAOyF,cAEjCxG,GAAOD,QAAU,SAASqM,GACxB,GAAIpJ,GAAGmP,EAAGhH,CACV,OAAOiB,KAAO1M,EAAY,YAAqB,OAAP0M,EAAc,OAEZ,iBAA9B+F,GAAKnP,EAAIjB,OAAOqK,IAAKsF,IAAoBS,EAEjDW,EAAM/R,EAAIiC,GAEM,WAAfmI,EAAIpK,EAAIiC,KAAsC,kBAAZA,GAAE+P,OAAuB,YAAc5H,IAK3E,SAASnL,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,GAEnCA,GAAoB,IAAI,SAAU,SAASoT,GACzC,MAAO,SAASC,QAAO7G,GACrB,MAAO4G,IAAW5R,EAASgL,GAAM4G,EAAQ5G,GAAMA,MAM9C,SAASpM,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9BsK,EAAUtK,EAAoB,GAC9BqB,EAAUrB,EAAoB,EAClCI,GAAOD,QAAU,SAASmT,EAAKpH,GAC7B,GAAIzF,IAAO6D,EAAKnI,YAAcmR,IAAQnR,OAAOmR,GACzCtI,IACJA,GAAIsI,GAAOpH,EAAKzF,GAChB5F,EAAQA,EAAQmD,EAAInD,EAAQoD,EAAI5C,EAAM,WAAYoF,EAAG,KAAQ,SAAUuE,KAKpE,SAAS5K,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,GAEnCA,GAAoB,IAAI,OAAQ,SAASuT,GACvC,MAAO,SAASC,MAAKhH,GACnB,MAAO+G,IAAS/R,EAASgL,GAAM+G,EAAM/G,GAAMA,MAM1C,SAASpM,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,GAEnCA,GAAoB,IAAI,oBAAqB,SAASyT,GACpD,MAAO,SAASC,mBAAkBlH,GAChC,MAAOiH,IAAsBjS,EAASgL,GAAMiH,EAAmBjH,GAAMA,MAMpE,SAASpM,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,GAEnCA,GAAoB,IAAI,WAAY,SAAS2T,GAC3C,MAAO,SAASC,UAASpH,GACvB,MAAOhL,GAASgL,GAAMmH,EAAYA,EAAUnH,IAAM,GAAQ,MAMzD,SAASpM,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,GAEnCA,GAAoB,IAAI,WAAY,SAAS6T,GAC3C,MAAO,SAASC,UAAStH,GACvB,MAAOhL,GAASgL,GAAMqH,EAAYA,EAAUrH,IAAM,GAAQ,MAMzD,SAASpM,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,GAEnCA,GAAoB,IAAI,eAAgB,SAAS+T,GAC/C,MAAO,SAASC,cAAaxH,GAC3B,MAAOhL,GAASgL,GAAMuH,EAAgBA,EAAcvH,IAAM,GAAO,MAMhE,SAASpM,EAAQD,EAASH,GAG/B,GAAI0B,GAAY1B,EAAoB,GAEpCA,GAAoB,IAAI,2BAA4B,SAASgR,GAC3D,MAAO,SAAS9M,0BAAyBsI,EAAIhH,GAC3C,MAAOwL,GAA0BtP,EAAU8K,GAAKhH,OAM/C,SAASpF,EAAQD,EAASH,GAG/B,GAAIyB,GAAWzB,EAAoB,GAEnCA,GAAoB,IAAI,iBAAkB,SAASiU,GACjD,MAAO,SAASrO,gBAAe4G,GAC7B,MAAOyH,GAAgBxS,EAAS+K,QAM/B,SAASpM,EAAQD,EAASH,GAG/B,GAAIyB,GAAWzB,EAAoB,GAEnCA,GAAoB,IAAI,OAAQ,SAASkU,GACvC,MAAO,SAAStQ,MAAK4I,GACnB,MAAO0H,GAAMzS,EAAS+K,QAMrB,SAASpM,EAAQD,EAASH,GAG/BA,EAAoB,IAAI,sBAAuB,WAC7C,MAAOA,GAAoB,IAAIkD,OAK5B,SAAS9C,EAAQD,EAASH,GAE/B,GAAI4C,GAAa5C,EAAoB,GAAG4C,QACpC7B,EAAaf,EAAoB,GACjCkB,EAAalB,EAAoB,IACjCmU,EAAa7N,SAASlE,UACtBgS,EAAa,wBACbC,EAAa,MAEjBA,KAAQF,IAAUnU,EAAoB,IAAM4C,EAAQuR,EAAQE,GAC1DrI,cAAc,EACd9I,IAAK,WACH,GAAIoR,IAAS,GAAK5N,MAAM4N,MAAMF,GAC1BxJ,EAAQ0J,EAAQA,EAAM,GAAK,EAE/B,OADApT,GAAIwF,KAAM2N,IAASzR,EAAQ8D,KAAM2N,EAAMtT,EAAW,EAAG6J,IAC9CA,MAMN,SAASxK,EAAQD,EAASH,GAG/B,GAAIY,GAAgBZ,EAAoB,GACpCwB,EAAgBxB,EAAoB,IACpCuU,EAAgBvU,EAAoB,IAAI,eACxCwU,EAAgBlO,SAASlE,SAExBmS,KAAgBC,IAAe5T,EAAEgC,QAAQ4R,EAAeD,GAAe9Q,MAAO,SAASL,GAC1F,GAAkB,kBAARsD,QAAuBlF,EAAS4B,GAAG,OAAO,CACpD,KAAI5B,EAASkF,KAAKtE,WAAW,MAAOgB,aAAasD,KAEjD,MAAMtD,EAAIxC,EAAEiF,SAASzC,IAAG,GAAGsD,KAAKtE,YAAcgB,EAAE,OAAO,CACvD,QAAO,MAKJ,SAAShD,EAAQD,EAASH,GAG/B,GAAIY,GAAcZ,EAAoB,GAClCqK,EAAcrK,EAAoB,GAClCkB,EAAclB,EAAoB,IAClCmB,EAAcnB,EAAoB,IAClCyU,EAAczU,EAAoB,IAClCqB,EAAcrB,EAAoB,GAClC0U,EAAc1U,EAAoB,IAAI2U,KACtCC,EAAc,SACdC,EAAcxK,EAAOuK,GACrBE,EAAcD,EACdhC,EAAcgC,EAAQzS,UAEtB2S,EAAc5T,EAAIP,EAAEqF,OAAO4M,KAAW+B,EACtCI,EAAc,QAAUpI,QAAOxK,UAG/B6S,EAAW,SAASC,GACtB,GAAI1I,GAAKiI,EAAYS,GAAU,EAC/B,IAAgB,gBAAN1I,IAAkBA,EAAG1I,OAAS,EAAE,CACxC0I,EAAKwI,EAAOxI,EAAGmI,OAASD,EAAMlI,EAAI,EAClC,IACI2I,GAAOC,EAAOC,EADdC,EAAQ9I,EAAG+I,WAAW,EAE1B,IAAa,KAAVD,GAA0B,KAAVA,GAEjB,GADAH,EAAQ3I,EAAG+I,WAAW,GACT,KAAVJ,GAA0B,MAAVA,EAAc,MAAOhM,SACnC,IAAa,KAAVmM,EAAa,CACrB,OAAO9I,EAAG+I,WAAW,IACnB,IAAK,IAAK,IAAK,IAAMH,EAAQ,EAAGC,EAAU,EAAI,MAC9C,KAAK,IAAK,IAAK,KAAMD,EAAQ,EAAGC,EAAU,EAAI,MAC9C,SAAU,OAAQ7I,EAEpB,IAAI,GAAoDgJ,GAAhDC,EAASjJ,EAAGhK,MAAM,GAAIuB,EAAI,EAAG6M,EAAI6E,EAAO3R,OAAkB8M,EAAJ7M,EAAOA,IAInE,GAHAyR,EAAOC,EAAOF,WAAWxR,GAGf,GAAPyR,GAAaA,EAAOH,EAAQ,MAAOlM,IACtC,OAAOuM,UAASD,EAAQL,IAE5B,OAAQ5I,EAGRqI,GAAQ,SAAYA,EAAQ,SAAUA,EAAQ,UAChDA,EAAU,QAASc,QAAOlS,GACxB,GAAI+I,GAAK5F,UAAU9C,OAAS,EAAI,EAAIL,EAChC+C,EAAOE,IACX,OAAOF,aAAgBqO,KAEjBE,EAAa1T,EAAM,WAAYwR,EAAM+C,QAAQrV,KAAKiG,KAAYrF,EAAIqF,IAASoO,GAC3E,GAAIE,GAAKG,EAASzI,IAAOyI,EAASzI,IAE1C5L,EAAEqH,KAAK1H,KAAKP,EAAoB,GAAKY,EAAEoF,SAAS8O,GAAQ,6KAMtD1Q,MAAM,KAAM,SAASoB,GAClBtE,EAAI4T,EAAMtP,KAAStE,EAAI2T,EAASrP,IACjC5E,EAAEgC,QAAQiS,EAASrP,EAAK5E,EAAEkC,QAAQgS,EAAMtP,MAG5CqP,EAAQzS,UAAYyQ,EACpBA,EAAM/M,YAAc+O,EACpB7U,EAAoB,IAAIqK,EAAQuK,EAAQC,KAKrC,SAASzU,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,GAGnCI,GAAOD,QAAU,SAASqM,EAAIxI,GAC5B,IAAIxC,EAASgL,GAAI,MAAOA,EACxB,IAAI/F,GAAIgG,CACR,IAAGzI,GAAkC,mBAArByC,EAAK+F,EAAGK,YAA4BrL,EAASiL,EAAMhG,EAAGlG,KAAKiM,IAAK,MAAOC,EACvF,IAA+B,mBAApBhG,EAAK+F,EAAGoJ,WAA2BpU,EAASiL,EAAMhG,EAAGlG,KAAKiM,IAAK,MAAOC,EACjF,KAAIzI,GAAkC,mBAArByC,EAAK+F,EAAGK,YAA4BrL,EAASiL,EAAMhG,EAAGlG,KAAKiM,IAAK,MAAOC,EACxF,MAAMjJ,WAAU,6CAKb,SAASpD,EAAQD,EAASH,GAE/B,GAAIa,GAAUb,EAAoB,GAC9BsN,EAAUtN,EAAoB,IAC9BqB,EAAUrB,EAAoB,GAC9B6V,EAAU,+CAEVC,EAAU,IAAMD,EAAS,IACzBE,EAAU,KACVC,EAAUC,OAAO,IAAMH,EAAQA,EAAQ,KACvCI,EAAUD,OAAOH,EAAQA,EAAQ,MAEjCK,EAAW,SAAS7C,EAAKpH,GAC3B,GAAIlB,KACJA,GAAIsI,GAAOpH,EAAKyI,GAChB9T,EAAQA,EAAQwC,EAAIxC,EAAQoD,EAAI5C,EAAM,WACpC,QAASwU,EAAOvC,MAAUyC,EAAIzC,MAAUyC,IACtC,SAAU/K,IAMZ2J,EAAOwB,EAASxB,KAAO,SAASyB,EAAQxI,GAI1C,MAHAwI,GAASxJ,OAAOU,EAAQ8I,IACd,EAAPxI,IAASwI,EAASA,EAAOC,QAAQL,EAAO,KACjC,EAAPpI,IAASwI,EAASA,EAAOC,QAAQH,EAAO,KACpCE,EAGThW,GAAOD,QAAUgW,GAIZ,SAAS/V,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UAAWsS,QAAS1N,KAAK2N,IAAI,EAAG,QAI9C,SAASnW,EAAQD,EAASH,GAG/B,GAAIa,GAAYb,EAAoB,GAChCwW,EAAYxW,EAAoB,GAAGoJ,QAEvCvI,GAAQA,EAAQmD,EAAG,UACjBoF,SAAU,QAASA,UAASoD,GAC1B,MAAoB,gBAANA,IAAkBgK,EAAUhK,OAMzC,SAASpM,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UAAWyS,UAAWzW,EAAoB,OAIxD,SAASI,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,IAC/BwN,EAAW5E,KAAK4E,KACpBpN,GAAOD,QAAU,QAASsW,WAAUjK,GAClC,OAAQhL,EAASgL,IAAOpD,SAASoD,IAAOgB,EAAMhB,KAAQA,IAKnD,SAASpM,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UACjByJ,MAAO,QAASA,OAAMiJ,GACpB,MAAOA,IAAUA,MAMhB,SAAStW,EAAQD,EAASH,GAG/B,GAAIa,GAAYb,EAAoB,GAChCyW,EAAYzW,EAAoB,IAChC2J,EAAYf,KAAKe,GAErB9I,GAAQA,EAAQmD,EAAG,UACjB2S,cAAe,QAASA,eAAcD,GACpC,MAAOD,GAAUC,IAAW/M,EAAI+M,IAAW,qBAM1C,SAAStW,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UAAW4S,iBAAkB,oBAI3C,SAASxW,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UAAW6S,iBAAkB,qBAI3C,SAASzW,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UAAW8S,WAAYA,cAIrC,SAAS1W,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,UAAW0R,SAAUA,YAInC,SAAStV,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B+W,EAAU/W,EAAoB,IAC9BgX,EAAUpO,KAAKoO,KACfC,EAAUrO,KAAKsO,KAGnBrW,GAAQA,EAAQmD,EAAInD,EAAQoD,IAAMgT,GAAkD,KAAxCrO,KAAK4E,MAAMyJ,EAAOtB,OAAOwB,aAAqB,QACxFD,MAAO,QAASA,OAAMxE,GACpB,OAAQA,GAAKA,GAAK,EAAIvJ,IAAMuJ,EAAI,kBAC5B9J,KAAKwO,IAAI1E,GAAK9J,KAAKyO,IACnBN,EAAMrE,EAAI,EAAIsE,EAAKtE,EAAI,GAAKsE,EAAKtE,EAAI,QAMxC,SAAStS,EAAQD,GAGtBC,EAAOD,QAAUyI,KAAKmO,OAAS,QAASA,OAAMrE,GAC5C,OAAQA,GAAKA,GAAK,OAAa,KAAJA,EAAWA,EAAIA,EAAIA,EAAI,EAAI9J,KAAKwO,IAAI,EAAI1E,KAKhE,SAAStS,EAAQD,EAASH,GAK/B,QAASsX,OAAM5E,GACb,MAAQtJ,UAASsJ,GAAKA,IAAW,GAALA,EAAiB,EAAJA,GAAS4E,OAAO5E,GAAK9J,KAAKwO,IAAI1E,EAAI9J,KAAKoO,KAAKtE,EAAIA,EAAI,IAAxDA,EAHvC,GAAI7R,GAAUb,EAAoB,EAMlCa,GAAQA,EAAQmD,EAAG,QAASsT,MAAOA,SAI9B,SAASlX,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QACjBuT,MAAO,QAASA,OAAM7E,GACpB,MAAmB,KAAXA,GAAKA,GAAUA,EAAI9J,KAAKwO,KAAK,EAAI1E,IAAM,EAAIA,IAAM,MAMxD,SAAStS,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9BwX,EAAUxX,EAAoB,GAElCa,GAAQA,EAAQmD,EAAG,QACjByT,KAAM,QAASA,MAAK/E,GAClB,MAAO8E,GAAK9E,GAAKA,GAAK9J,KAAK2N,IAAI3N,KAAKe,IAAI+I,GAAI,EAAI,OAM/C,SAAStS,EAAQD,GAGtBC,EAAOD,QAAUyI,KAAK4O,MAAQ,QAASA,MAAK9E,GAC1C,MAAmB,KAAXA,GAAKA,IAAWA,GAAKA,EAAIA,EAAQ,EAAJA,EAAQ,GAAK,IAK/C,SAAStS,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QACjB0T,MAAO,QAASA,OAAMhF,GACpB,OAAQA,KAAO,GAAK,GAAK9J,KAAK4E,MAAM5E,KAAKwO,IAAI1E,EAAI,IAAO9J,KAAK+O,OAAS,OAMrE,SAASvX,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9BgL,EAAUpC,KAAKoC,GAEnBnK,GAAQA,EAAQmD,EAAG,QACjB4T,KAAM,QAASA,MAAKlF,GAClB,OAAQ1H,EAAI0H,GAAKA,GAAK1H,GAAK0H,IAAM,MAMhC,SAAStS,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QAAS6T,MAAO7X,EAAoB,OAIlD,SAASI,EAAQD,GAGtBC,EAAOD,QAAUyI,KAAKiP,OAAS,QAASA,OAAMnF,GAC5C,MAAmB,KAAXA,GAAKA,GAAUA,EAAIA,GAAK,MAAY,KAAJA,EAAWA,EAAIA,EAAIA,EAAI,EAAI9J,KAAKoC,IAAI0H,GAAK,IAK9E,SAAStS,EAAQD,EAASH,GAG/B,GAAIa,GAAYb,EAAoB,GAChCwX,EAAYxX,EAAoB,IAChCuW,EAAY3N,KAAK2N,IACjBD,EAAYC,EAAI,EAAG,KACnBuB,EAAYvB,EAAI,EAAG,KACnBwB,EAAYxB,EAAI,EAAG,MAAQ,EAAIuB,GAC/BE,EAAYzB,EAAI,EAAG,MAEnB0B,EAAkB,SAAS5R,GAC7B,MAAOA,GAAI,EAAIiQ,EAAU,EAAIA,EAI/BzV,GAAQA,EAAQmD,EAAG,QACjBkU,OAAQ,QAASA,QAAOxF,GACtB,GAEIvP,GAAGsC,EAFH0S,EAAQvP,KAAKe,IAAI+I,GACjB0F,EAAQZ,EAAK9E,EAEjB,OAAUsF,GAAPG,EAAoBC,EAAQH,EAAgBE,EAAOH,EAAQF,GAAaE,EAAQF,GACnF3U,GAAK,EAAI2U,EAAYxB,GAAW6B,EAChC1S,EAAStC,GAAKA,EAAIgV,GACf1S,EAASsS,GAAStS,GAAUA,EAAc2S,GAAQC,EAAAA,GAC9CD,EAAQ3S,OAMd,SAASrF,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B2J,EAAUf,KAAKe,GAEnB9I,GAAQA,EAAQmD,EAAG,QACjBsU,MAAO,QAASA,OAAMC,EAAQC,GAO5B,IANA,GAKI/J,GAAKgK,EALLC,EAAQ,EACR3U,EAAQ,EACRuN,EAAQ1K,UACR4L,EAAQlB,EAAGxN,OACX6U,EAAQ,EAEFnG,EAAJzO,GACJ0K,EAAM9E,EAAI2H,EAAGvN,MACH0K,EAAPkK,GACDF,EAAOE,EAAOlK,EACdiK,EAAOA,EAAMD,EAAMA,EAAM,EACzBE,EAAOlK,GACCA,EAAM,GACdgK,EAAOhK,EAAMkK,EACbD,GAAOD,EAAMA,GACRC,GAAOjK,CAEhB,OAAOkK,KAASN,EAAAA,EAAWA,EAAAA,EAAWM,EAAO/P,KAAKoO,KAAK0B,OAMtD,SAAStY,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B4Y,EAAUhQ,KAAKiQ,IAGnBhY,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAIjE,EAAoB,GAAG,WACrD,MAA+B,IAAxB4Y,EAAM,WAAY,IAA4B,GAAhBA,EAAM9U,SACzC,QACF+U,KAAM,QAASA,MAAKnG,EAAGnJ,GACrB,GAAIuP,GAAS,MACTC,GAAMrG,EACNsG,GAAMzP,EACN0P,EAAKH,EAASC,EACdG,EAAKJ,EAASE,CAClB,OAAO,GAAIC,EAAKC,IAAOJ,EAASC,IAAO,IAAMG,EAAKD,GAAMH,EAASE,IAAO,KAAO,KAAO,OAMrF,SAAS5Y,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QACjBmV,MAAO,QAASA,OAAMzG,GACpB,MAAO9J,MAAKwO,IAAI1E,GAAK9J,KAAKwQ,SAMzB,SAAShZ,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QAAS+S,MAAO/W,EAAoB,OAIlD,SAASI,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QACjBqV,KAAM,QAASA,MAAK3G,GAClB,MAAO9J,MAAKwO,IAAI1E,GAAK9J,KAAKyO,QAMzB,SAASjX,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QAASwT,KAAMxX,EAAoB,OAIjD,SAASI,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B6X,EAAU7X,EAAoB,IAC9BgL,EAAUpC,KAAKoC,GAGnBnK,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAIjE,EAAoB,GAAG,WACrD,MAA6B,SAArB4I,KAAK0Q,KAAK,UAChB,QACFA,KAAM,QAASA,MAAK5G,GAClB,MAAO9J,MAAKe,IAAI+I,GAAKA,GAAK,GACrBmF,EAAMnF,GAAKmF,GAAOnF,IAAM,GACxB1H,EAAI0H,EAAI,GAAK1H,GAAK0H,EAAI,KAAO9J,KAAKmI,EAAI,OAM1C,SAAS3Q,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B6X,EAAU7X,EAAoB,IAC9BgL,EAAUpC,KAAKoC,GAEnBnK,GAAQA,EAAQmD,EAAG,QACjBuV,KAAM,QAASA,MAAK7G,GAClB,GAAIvP,GAAI0U,EAAMnF,GAAKA,GACf1F,EAAI6K,GAAOnF,EACf,OAAOvP,IAAKkV,EAAAA,EAAW,EAAIrL,GAAKqL,EAAAA,EAAW,IAAMlV,EAAI6J,IAAMhC,EAAI0H,GAAK1H,GAAK0H,QAMxE,SAAStS,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,QACjBwV,MAAO,QAASA,OAAMhN,GACpB,OAAQA,EAAK,EAAI5D,KAAK4E,MAAQ5E,KAAK2E,MAAMf,OAMxC,SAASpM,EAAQD,EAASH,GAE/B,GAAIa,GAAiBb,EAAoB,GACrC4B,EAAiB5B,EAAoB,IACrCyZ,EAAiB7M,OAAO6M,aACxBC,EAAiB9M,OAAO+M,aAG5B9Y,GAAQA,EAAQmD,EAAInD,EAAQoD,KAAOyV,GAA2C,GAAzBA,EAAe5V,QAAc,UAEhF6V,cAAe,QAASA,eAAcjH,GAMpC,IALA,GAII8C,GAJApH,KACAkD,EAAQ1K,UACR4L,EAAQlB,EAAGxN,OACXC,EAAQ,EAENyO,EAAQzO,GAAE,CAEd,GADAyR,GAAQlE,EAAGvN,KACRnC,EAAQ4T,EAAM,WAAcA,EAAK,KAAMnM,YAAWmM,EAAO,6BAC5DpH,GAAI1I,KAAY,MAAP8P,EACLiE,EAAajE,GACbiE,IAAejE,GAAQ,QAAY,IAAM,MAAQA,EAAO,KAAQ,QAEpE,MAAOpH,GAAI1L,KAAK,QAMjB,SAAStC,EAAQD,EAASH,GAE/B,GAAIa,GAAYb,EAAoB,GAChC0B,EAAY1B,EAAoB,IAChC6B,EAAY7B,EAAoB,GAEpCa,GAAQA,EAAQmD,EAAG,UAEjB4V,IAAK,QAASA,KAAIC,GAOhB,IANA,GAAIC,GAAQpY,EAAUmY,EAASD,KAC3BzT,EAAQtE,EAASiY,EAAIhW,QACrBwN,EAAQ1K,UACR4L,EAAQlB,EAAGxN,OACXsK,KACArK,EAAQ,EACNoC,EAAMpC,GACVqK,EAAI1I,KAAKkH,OAAOkN,EAAI/V,OACbyO,EAAJzO,GAAUqK,EAAI1I,KAAKkH,OAAO0E,EAAGvN,IAChC,OAAOqK,GAAI1L,KAAK,QAMjB,SAAStC,EAAQD,EAASH,GAI/BA,EAAoB,IAAI,OAAQ,SAAS0U,GACvC,MAAO,SAASC,QACd,MAAOD,GAAMhO,KAAM,OAMlB,SAAStG,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B+Z,EAAU/Z,EAAoB,KAAI,EACtCa,GAAQA,EAAQwC,EAAG,UAEjB2W,YAAa,QAASA,aAAYC,GAChC,MAAOF,GAAIrT,KAAMuT,OAMhB,SAAS7Z,EAAQD,EAASH,GAE/B,GAAI2B,GAAY3B,EAAoB,IAChCsN,EAAYtN,EAAoB,GAGpCI,GAAOD,QAAU,SAASiM,GACxB,MAAO,UAAS5F,EAAMyT,GACpB,GAGI9W,GAAG6J,EAHHtD,EAAIkD,OAAOU,EAAQ9G,IACnBzC,EAAIpC,EAAUsY,GACdrJ,EAAIlH,EAAE5F,MAEV,OAAO,GAAJC,GAASA,GAAK6M,EAASxE,EAAY,GAAKtM,GAC3CqD,EAAIuG,EAAE6L,WAAWxR,GACN,MAAJZ,GAAcA,EAAI,OAAUY,EAAI,IAAM6M,IAAM5D,EAAItD,EAAE6L,WAAWxR,EAAI,IAAM,OAAUiJ,EAAI,MACxFZ,EAAY1C,EAAErC,OAAOtD,GAAKZ,EAC1BiJ,EAAY1C,EAAElH,MAAMuB,EAAGA,EAAI,IAAMZ,EAAI,OAAU,KAAO6J,EAAI,OAAU,UAMvE,SAAS5M,EAAQD,EAASH,GAI/B,GAAIa,GAAYb,EAAoB,GAChC6B,EAAY7B,EAAoB,IAChCka,EAAYla,EAAoB,KAChCma,EAAY,WACZC,EAAY,GAAGD,EAEnBtZ,GAAQA,EAAQwC,EAAIxC,EAAQoD,EAAIjE,EAAoB,KAAKma,GAAY,UACnEE,SAAU,QAASA,UAASC,GAC1B,GAAI9T,GAAO0T,EAAQxT,KAAM4T,EAAcH,GACnC7I,EAAO1K,UACP2T,EAAcjJ,EAAGxN,OAAS,EAAIwN,EAAG,GAAKxR,EACtCqG,EAAStE,EAAS2E,EAAK1C,QACvBiD,EAASwT,IAAgBza,EAAYqG,EAAMyC,KAAKC,IAAIhH,EAAS0Y,GAAcpU,GAC3EqU,EAAS5N,OAAO0N,EACpB,OAAOF,GACHA,EAAU7Z,KAAKiG,EAAMgU,EAAQzT,GAC7BP,EAAKhE,MAAMuE,EAAMyT,EAAO1W,OAAQiD,KAASyT,MAM5C,SAASpa,EAAQD,EAASH,GAG/B,GAAIya,GAAWza,EAAoB,KAC/BsN,EAAWtN,EAAoB,GAEnCI,GAAOD,QAAU,SAASqG,EAAM8T,EAAcjG,GAC5C,GAAGoG,EAASH,GAAc,KAAM9W,WAAU,UAAY6Q,EAAO,yBAC7D,OAAOzH,QAAOU,EAAQ9G,MAKnB,SAASpG,EAAQD,EAASH,GAG/B,GAAIwB,GAAWxB,EAAoB,IAC/BmB,EAAWnB,EAAoB,IAC/B0a,EAAW1a,EAAoB,IAAI,QACvCI,GAAOD,QAAU,SAASqM,GACxB,GAAIiO,EACJ,OAAOjZ,GAASgL,MAASiO,EAAWjO,EAAGkO,MAAY5a,IAAc2a,EAAsB,UAAXtZ,EAAIqL,MAK7E,SAASpM,EAAQD,EAASH,GAE/B,GAAI0a,GAAQ1a,EAAoB,IAAI,QACpCI,GAAOD,QAAU,SAASmT,GACxB,GAAIqH,GAAK,GACT,KACE,MAAMrH,GAAKqH,GACX,MAAMpX,GACN,IAEE,MADAoX,GAAGD,IAAS,GACJ,MAAMpH,GAAKqH,GACnB,MAAMtM,KACR,OAAO,IAKN,SAASjO,EAAQD,EAASH,GAI/B,GAAIa,GAAWb,EAAoB,GAC/Bka,EAAWla,EAAoB,KAC/B4a,EAAW,UAEf/Z,GAAQA,EAAQwC,EAAIxC,EAAQoD,EAAIjE,EAAoB,KAAK4a,GAAW,UAClEC,SAAU,QAASA,UAASP,GAC1B,SAAUJ,EAAQxT,KAAM4T,EAAcM,GACnCpS,QAAQ8R,EAAc1T,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,OAM9D,SAASM,EAAQD,EAASH,GAE/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQwC,EAAG,UAEjByX,OAAQ9a,EAAoB,QAKzB,SAASI,EAAQD,EAASH,GAG/B,GAAI2B,GAAY3B,EAAoB,IAChCsN,EAAYtN,EAAoB,GAEpCI,GAAOD,QAAU,QAAS2a,QAAOC,GAC/B,GAAIC,GAAMpO,OAAOU,EAAQ5G,OACrB0H,EAAM,GACN/H,EAAM1E,EAAUoZ,EACpB,IAAO,EAAJ1U,GAASA,GAAKgS,EAAAA,EAAS,KAAMhP,YAAW,0BAC3C,MAAKhD,EAAI,GAAIA,KAAO,KAAO2U,GAAOA,GAAY,EAAJ3U,IAAM+H,GAAO4M,EACvD,OAAO5M,KAKJ,SAAShO,EAAQD,EAASH,GAI/B,GAAIa,GAAcb,EAAoB,GAClC6B,EAAc7B,EAAoB,IAClCka,EAAcla,EAAoB,KAClCib,EAAc,aACdC,EAAc,GAAGD,EAErBpa,GAAQA,EAAQwC,EAAIxC,EAAQoD,EAAIjE,EAAoB,KAAKib,GAAc,UACrEE,WAAY,QAASA,YAAWb,GAC9B,GAAI9T,GAAS0T,EAAQxT,KAAM4T,EAAcW,GACrC3J,EAAS1K,UACTgB,EAAS/F,EAAS+G,KAAKC,IAAIyI,EAAGxN,OAAS,EAAIwN,EAAG,GAAKxR,EAAW0G,EAAK1C,SACnE0W,EAAS5N,OAAO0N,EACpB,OAAOY,GACHA,EAAY3a,KAAKiG,EAAMgU,EAAQ5S,GAC/BpB,EAAKhE,MAAMoF,EAAOA,EAAQ4S,EAAO1W,UAAY0W,MAMhD,SAASpa,EAAQD,EAASH,GAG/B,GAAI+Z,GAAO/Z,EAAoB,KAAI,EAGnCA,GAAoB,KAAK4M,OAAQ,SAAU,SAASwO,GAClD1U,KAAK2U,GAAKzO,OAAOwO,GACjB1U,KAAK4U,GAAK,GAET,WACD,GAEIC,GAFAnY,EAAQsD,KAAK2U,GACbzT,EAAQlB,KAAK4U,EAEjB,OAAG1T,IAASxE,EAAEU,QAAeL,MAAO3D,EAAW0b,MAAM,IACrDD,EAAQxB,EAAI3W,EAAGwE,GACflB,KAAK4U,IAAMC,EAAMzX,QACTL,MAAO8X,EAAOC,MAAM,OAKzB,SAASpb,EAAQD,EAASH,GAG/B,GAAIyb,GAAiBzb,EAAoB,IACrCa,EAAiBb,EAAoB,GACrCwK,EAAiBxK,EAAoB,IACrCuK,EAAiBvK,EAAoB,GACrCkB,EAAiBlB,EAAoB,IACrC0b,EAAiB1b,EAAoB,KACrC2b,EAAiB3b,EAAoB,KACrCiP,EAAiBjP,EAAoB,IACrC6F,EAAiB7F,EAAoB,GAAG6F,SACxC+V,EAAiB5b,EAAoB,IAAI,YACzC6b,OAAsBjY,MAAQ,WAAaA,QAC3CkY,EAAiB,aACjBC,EAAiB,OACjBC,EAAiB,SAEjBC,EAAa,WAAY,MAAOvV,MAEpCtG,GAAOD,QAAU,SAAS2U,EAAMT,EAAM6H,EAAaC,EAAMC,EAASC,EAAQC,GACxEX,EAAYO,EAAa7H,EAAM8H,EAC/B,IAaII,GAAS/W,EAbTgX,EAAY,SAASC,GACvB,IAAIZ,GAASY,IAAQ5J,GAAM,MAAOA,GAAM4J,EACxC,QAAOA,GACL,IAAKV,GAAM,MAAO,SAASnY,QAAQ,MAAO,IAAIsY,GAAYxV,KAAM+V,GAChE,KAAKT,GAAQ,MAAO,SAASU,UAAU,MAAO,IAAIR,GAAYxV,KAAM+V,IACpE,MAAO,SAASE,WAAW,MAAO,IAAIT,GAAYxV,KAAM+V,KAExD3K,EAAauC,EAAO,YACpBuI,EAAaR,GAAWJ,EACxBa,GAAa,EACbhK,EAAaiC,EAAK1S,UAClB0a,EAAajK,EAAM+I,IAAa/I,EAAMiJ,IAAgBM,GAAWvJ,EAAMuJ,GACvEW,EAAaD,GAAWN,EAAUJ,EAGtC,IAAGU,EAAQ,CACT,GAAIE,GAAoBnX,EAASkX,EAASxc,KAAK,GAAIuU,IAEnD7F,GAAe+N,EAAmBlL,GAAK,IAEnC2J,GAAWva,EAAI2R,EAAOiJ,IAAavR,EAAKyS,EAAmBpB,EAAUK,GAEtEW,GAAcE,EAAQlS,OAASoR,IAChCa,GAAa,EACbE,EAAW,QAASL,UAAU,MAAOI,GAAQvc,KAAKmG,QAUtD,GANK+U,IAAWa,IAAYT,IAASgB,GAAehK,EAAM+I,IACxDrR,EAAKsI,EAAO+I,EAAUmB,GAGxBrB,EAAUrH,GAAQ0I,EAClBrB,EAAU5J,GAAQmK,EACfG,EAMD,GALAG,GACEG,OAASE,EAAcG,EAAWP,EAAUR,GAC5CpY,KAASyY,EAAcU,EAAWP,EAAUT,GAC5CY,QAAUC,EAAwBJ,EAAU,WAArBO,GAEtBT,EAAO,IAAI9W,IAAO+W,GACd/W,IAAOqN,IAAOrI,EAASqI,EAAOrN,EAAK+W,EAAQ/W,QAC3C3E,GAAQA,EAAQwC,EAAIxC,EAAQoD,GAAK4X,GAASgB,GAAaxI,EAAMkI,EAEtE,OAAOA,KAKJ,SAASnc,EAAQD,GAEtBC,EAAOD,YAIF,SAASC,EAAQD,EAASH,GAG/B,GAAIY,GAAiBZ,EAAoB,GACrCid,EAAiBjd,EAAoB,GACrCiP,EAAiBjP,EAAoB,IACrCgd,IAGJhd,GAAoB,GAAGgd,EAAmBhd,EAAoB,IAAI,YAAa,WAAY,MAAO0G,QAElGtG,EAAOD,QAAU,SAAS+b,EAAa7H,EAAM8H,GAC3CD,EAAY9Z,UAAYxB,EAAEqF,OAAO+W,GAAoBb,KAAMc,EAAW,EAAGd,KACzElN,EAAeiN,EAAa7H,EAAO,eAKhC,SAASjU,EAAQD,EAASH,GAG/B,GAAIyK,GAAczK,EAAoB,IAClCa,EAAcb,EAAoB,GAClCyB,EAAczB,EAAoB,IAClCO,EAAcP,EAAoB,KAClCkd,EAAcld,EAAoB,KAClC6B,EAAc7B,EAAoB,IAClCmd,EAAcnd,EAAoB,IACtCa,GAAQA,EAAQmD,EAAInD,EAAQoD,GAAKjE,EAAoB,KAAK,SAASod,GAAO9a,MAAM+a,KAAKD,KAAW,SAE9FC,KAAM,QAASA,MAAKC,GAClB,GAQIxZ,GAAQ2B,EAAQ8X,EAAMC,EARtBpa,EAAU3B,EAAS6b,GACnB9O,EAAyB,kBAAR9H,MAAqBA,KAAOpE,MAC7CgP,EAAU1K,UACV4L,EAAUlB,EAAGxN,OACb2Z,EAAUjL,EAAQ,EAAIlB,EAAG,GAAKxR,EAC9B4d,EAAUD,IAAU3d,EACpB8H,EAAU,EACV+V,EAAUR,EAAU/Z,EAIxB,IAFGsa,IAAQD,EAAQhT,EAAIgT,EAAOjL,EAAQ,EAAIlB,EAAG,GAAKxR,EAAW,IAE1D6d,GAAU7d,GAAe0O,GAAKlM,OAAS4a,EAAYS,GAMpD,IADA7Z,EAASjC,EAASuB,EAAEU,QAChB2B,EAAS,GAAI+I,GAAE1K,GAASA,EAAS8D,EAAOA,IAC1CnC,EAAOmC,GAAS8V,EAAUD,EAAMra,EAAEwE,GAAQA,GAASxE,EAAEwE,OANvD,KAAI4V,EAAWG,EAAOpd,KAAK6C,GAAIqC,EAAS,GAAI+I,KAAK+O,EAAOC,EAASrB,QAAQX,KAAM5T,IAC7EnC,EAAOmC,GAAS8V,EAAUnd,EAAKid,EAAUC,GAAQF,EAAK9Z,MAAOmE,IAAQ,GAAQ2V,EAAK9Z,KAStF,OADAgC,GAAO3B,OAAS8D,EACTnC,MAON,SAASrF,EAAQD,EAASH,GAG/B,GAAIsB,GAAWtB,EAAoB,GACnCI,GAAOD,QAAU,SAASqd,EAAU/W,EAAIhD,EAAOkZ,GAC7C,IACE,MAAOA,GAAUlW,EAAGnF,EAASmC,GAAO,GAAIA,EAAM,IAAMgD,EAAGhD,GAEvD,MAAMF,GACN,GAAIqa,GAAMJ,EAAS,SAEnB,MADGI,KAAQ9d,GAAUwB,EAASsc,EAAIrd,KAAKid,IACjCja,KAML,SAASnD,EAAQD,EAASH,GAG/B,GAAI0b,GAAa1b,EAAoB,KACjC4b,EAAa5b,EAAoB,IAAI,YACrCqC,EAAaC,MAAMF,SAEvBhC,GAAOD,QAAU,SAASqM,GACxB,MAAOA,KAAO1M,IAAc4b,EAAUpZ,QAAUkK,GAAMnK,EAAWuZ,KAAcpP,KAK5E,SAASpM,EAAQD,EAASH,GAE/B,GAAIiT,GAAYjT,EAAoB,IAChC4b,EAAY5b,EAAoB,IAAI,YACpC0b,EAAY1b,EAAoB,IACpCI,GAAOD,QAAUH,EAAoB,GAAG6d,kBAAoB,SAASrR,GACnE,MAAGA,IAAM1M,EAAiB0M,EAAGoP,IACxBpP,EAAG,eACHkP,EAAUzI,EAAQzG,IAFvB,SAOG,SAASpM,EAAQD,EAASH,GAE/B,GAAI4b,GAAe5b,EAAoB,IAAI,YACvC8d,GAAe,CAEnB,KACE,GAAIC,IAAS,GAAGnC,IAChBmC,GAAM,UAAY,WAAYD,GAAe,GAC7Cxb,MAAM+a,KAAKU,EAAO,WAAY,KAAM,KACpC,MAAMxa,IAERnD,EAAOD,QAAU,SAAS+L,EAAM8R,GAC9B,IAAIA,IAAgBF,EAAa,OAAO,CACxC,IAAIpR,IAAO,CACX,KACE,GAAIuR,IAAQ,GACRb,EAAOa,EAAIrC,IACfwB,GAAKjB,KAAO,WAAY,OAAQX,KAAM9O,GAAO,IAC7CuR,EAAIrC,GAAY,WAAY,MAAOwB,IACnClR,EAAK+R,GACL,MAAM1a,IACR,MAAOmJ,KAKJ,SAAStM,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAGlCa,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAIjE,EAAoB,GAAG,WACrD,QAASiE,MACT,QAAS3B,MAAM4b,GAAG3d,KAAK0D,YAAcA,MACnC,SAEFia,GAAI,QAASA,MAKX,IAJA,GAAItW,GAAS,EACT0J,EAAS1K,UACT4L,EAASlB,EAAGxN,OACZ2B,EAAS,IAAoB,kBAARiB,MAAqBA,KAAOpE,OAAOkQ,GACtDA,EAAQ5K,GAAMnC,EAAOmC,GAAS0J,EAAG1J,IAEvC,OADAnC,GAAO3B,OAAS0O,EACT/M,MAMN,SAASrF,EAAQD,EAASH,GAG/B,GAAIme,GAAmBne,EAAoB,KACvCud,EAAmBvd,EAAoB,KACvC0b,EAAmB1b,EAAoB,KACvC0B,EAAmB1B,EAAoB,GAM3CI,GAAOD,QAAUH,EAAoB,KAAKsC,MAAO,QAAS,SAAS8Y,EAAUqB,GAC3E/V,KAAK2U,GAAK3Z,EAAU0Z,GACpB1U,KAAK4U,GAAK,EACV5U,KAAK6J,GAAKkM,GAET,WACD,GAAIrZ,GAAQsD,KAAK2U,GACboB,EAAQ/V,KAAK6J,GACb3I,EAAQlB,KAAK4U,IACjB,QAAIlY,GAAKwE,GAASxE,EAAEU,QAClB4C,KAAK2U,GAAKvb,EACHyd,EAAK,IAEH,QAARd,EAAwBc,EAAK,EAAG3V,GACxB,UAAR6U,EAAwBc,EAAK,EAAGna,EAAEwE,IAC9B2V,EAAK,GAAI3V,EAAOxE,EAAEwE,MACxB,UAGH8T,EAAU0C,UAAY1C,EAAUpZ,MAEhC6b,EAAiB,QACjBA,EAAiB,UACjBA,EAAiB,YAIZ,SAAS/d,EAAQD,EAASH,GAG/B,GAAIqe,GAAcre,EAAoB,IAAI,eACtCqC,EAAcC,MAAMF,SACrBC,GAAWgc,IAAgBve,GAAUE,EAAoB,GAAGqC,EAAYgc,MAC3Eje,EAAOD,QAAU,SAASqF,GACxBnD,EAAWgc,GAAa7Y,IAAO,IAK5B,SAASpF,EAAQD,GAEtBC,EAAOD,QAAU,SAASqb,EAAM/X,GAC9B,OAAQA,MAAOA,EAAO+X,OAAQA,KAK3B,SAASpb,EAAQD,EAASH,GAE/BA,EAAoB,KAAK,UAIpB,SAASI,EAAQD,EAASH,GAG/B,GAAIqK,GAAcrK,EAAoB,GAClCY,EAAcZ,EAAoB,GAClCc,EAAcd,EAAoB,GAClCsO,EAActO,EAAoB,IAAI,UAE1CI,GAAOD,QAAU,SAASmT,GACxB,GAAI9E,GAAInE,EAAOiJ,EACZxS,IAAe0N,IAAMA,EAAEF,IAAS1N,EAAEgC,QAAQ4L,EAAGF,GAC9CtC,cAAc,EACd9I,IAAK,WAAY,MAAOwD,WAMvB,SAAStG,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQwC,EAAG,SAAUib,WAAYte,EAAoB,OAE7DA,EAAoB,KAAK,eAIpB,SAASI,EAAQD,EAASH,GAI/B,GAAIyB,GAAWzB,EAAoB,IAC/B4B,EAAW5B,EAAoB,IAC/B6B,EAAW7B,EAAoB,GAEnCI,GAAOD,WAAame,YAAc,QAASA,YAAW9S,EAAevE,GACnE,GAAI7D,GAAQ3B,EAASiF,MACjBP,EAAQtE,EAASuB,EAAEU,QACnBya,EAAQ3c,EAAQ4J,EAAQrF,GACxBkX,EAAQzb,EAAQqF,EAAOd,GACvBmL,EAAQ1K,UACRG,EAAQuK,EAAGxN,OAAS,EAAIwN,EAAG,GAAKxR,EAChCib,EAAQnS,KAAKC,KAAK9B,IAAQjH,EAAYqG,EAAMvE,EAAQmF,EAAKZ,IAAQkX,EAAMlX,EAAMoY,GAC7EC,EAAQ,CAMZ,KALUD,EAAPlB,GAAkBA,EAAOtC,EAAZwD,IACdC,EAAO,GACPnB,GAAQtC,EAAQ,EAChBwD,GAAQxD,EAAQ,GAEZA,IAAU,GACXsC,IAAQja,GAAEA,EAAEmb,GAAMnb,EAAEia,SACXja,GAAEmb,GACdA,GAAQC,EACRnB,GAAQmB,CACR,OAAOpb,KAKN,SAAShD,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQwC,EAAG,SAAUob,KAAMze,EAAoB,OAEvDA,EAAoB,KAAK,SAIpB,SAASI,EAAQD,EAASH,GAI/B,GAAIyB,GAAWzB,EAAoB,IAC/B4B,EAAW5B,EAAoB,IAC/B6B,EAAW7B,EAAoB,GACnCI,GAAOD,WAAase,MAAQ,QAASA,MAAKhb,GAQxC,IAPA,GAAIL,GAAS3B,EAASiF,MAClB5C,EAASjC,EAASuB,EAAEU,QACpBwN,EAAS1K,UACT4L,EAASlB,EAAGxN,OACZ8D,EAAShG,EAAQ4Q,EAAQ,EAAIlB,EAAG,GAAKxR,EAAWgE,GAChDiD,EAASyL,EAAQ,EAAIlB,EAAG,GAAKxR,EAC7B4e,EAAS3X,IAAQjH,EAAYgE,EAASlC,EAAQmF,EAAKjD,GACjD4a,EAAS9W,GAAMxE,EAAEwE,KAAWnE,CAClC,OAAOL,KAKJ,SAAShD,EAAQD,EAASH,GAI/B,GAAIa,GAAUb,EAAoB,GAC9B2e,EAAU3e,EAAoB,IAAI,GAClCsT,EAAU,OACVsL,GAAU,CAEXtL,SAAUhR,MAAM,GAAGgR,GAAK,WAAYsL,GAAS,IAChD/d,EAAQA,EAAQwC,EAAIxC,EAAQoD,EAAI2a,EAAQ,SACtCC,KAAM,QAASA,MAAKnX,GAClB,MAAOiX,GAAMjY,KAAMgB,EAAYd,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAGzEE,EAAoB,KAAKsT,IAIpB,SAASlT,EAAQD,EAASH,GAI/B,GAAIa,GAAUb,EAAoB,GAC9B2e,EAAU3e,EAAoB,IAAI,GAClCsT,EAAU,YACVsL,GAAU,CAEXtL,SAAUhR,MAAM,GAAGgR,GAAK,WAAYsL,GAAS,IAChD/d,EAAQA,EAAQwC,EAAIxC,EAAQoD,EAAI2a,EAAQ,SACtCE,UAAW,QAASA,WAAUpX,GAC5B,MAAOiX,GAAMjY,KAAMgB,EAAYd,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAGzEE,EAAoB,KAAKsT,IAIpB,SAASlT,EAAQD,EAASH,GAE/B,GAAIY,GAAWZ,EAAoB,GAC/BqK,EAAWrK,EAAoB,GAC/Bya,EAAWza,EAAoB,KAC/B+e,EAAW/e,EAAoB,KAC/Bgf,EAAW3U,EAAO4L,OAClBnB,EAAWkK,EACXnM,EAAWmM,EAAQ5c,UACnB6c,EAAW,KACXC,EAAW,KAEXC,EAAc,GAAIH,GAAQC,KAASA,GAEpCjf,EAAoB,IAAQmf,IAAenf,EAAoB,GAAG,WAGnE,MAFAkf,GAAIlf,EAAoB,IAAI,WAAY,EAEjCgf,EAAQC,IAAQA,GAAOD,EAAQE,IAAQA,GAA4B,QAArBF,EAAQC,EAAK,SAElED,EAAU,QAAS/I,QAAOvV,EAAG2N,GAC3B,GAAI+Q,GAAO3E,EAAS/Z,GAChB2e,EAAOhR,IAAMvO,CACjB,OAAS4G,gBAAgBsY,KAAYI,GAAQ1e,EAAEoF,cAAgBkZ,IAAWK,EACtEF,EACE,GAAIrK,GAAKsK,IAASC,EAAM3e,EAAEmK,OAASnK,EAAG2N,GACtCyG,GAAMsK,EAAO1e,YAAase,IAAWte,EAAEmK,OAASnK,EAAG0e,GAAQC,EAAMN,EAAOxe,KAAKG,GAAK2N,GAHR3N,GAKlFE,EAAEqH,KAAK1H,KAAKK,EAAEoF,SAAS8O,GAAO,SAAStP,GACrCA,IAAOwZ,IAAWpe,EAAEgC,QAAQoc,EAASxZ,GACnCwG,cAAc,EACd9I,IAAK,WAAY,MAAO4R,GAAKtP,IAC7BgL,IAAK,SAAShE,GAAKsI,EAAKtP,GAAOgH,OAGnCqG,EAAM/M,YAAckZ,EACpBA,EAAQ5c,UAAYyQ,EACpB7S,EAAoB,IAAIqK,EAAQ,SAAU2U,IAG5Chf,EAAoB,KAAK,WAIpB,SAASI,EAAQD,EAASH,GAI/B,GAAIsB,GAAWtB,EAAoB,GACnCI,GAAOD,QAAU,WACf,GAAIqG,GAASlF,EAASoF,MAClBjB,EAAS,EAMb,OALGe,GAAK6D,SAAY5E,GAAU,KAC3Be,EAAK8Y,aAAY7Z,GAAU,KAC3Be,EAAK+Y,YAAY9Z,GAAU,KAC3Be,EAAKgZ,UAAY/Z,GAAU,KAC3Be,EAAKiZ,SAAYha,GAAU,KACvBA,IAKJ,SAASrF,EAAQD,EAASH,GAG/B,GAAIY,GAAIZ,EAAoB,EACzBA,GAAoB,IAAoB,KAAd,KAAK0f,OAAa9e,EAAEgC,QAAQqT,OAAO7T,UAAW,SACzE4J,cAAc,EACd9I,IAAKlD,EAAoB,QAKtB,SAASI,EAAQD,EAASH,GAG/BA,EAAoB,KAAK,QAAS,EAAG,SAASsN,EAASoN,GAErD,MAAO,SAASpG,OAAMqL,GAEpB,GAAIvc,GAAKkK,EAAQ5G,MACbD,EAAKkZ,GAAU7f,EAAYA,EAAY6f,EAAOjF,EAClD,OAAOjU,KAAO3G,EAAY2G,EAAGlG,KAAKof,EAAQvc,GAAK,GAAI6S,QAAO0J,GAAQjF,GAAO9N,OAAOxJ,QAM/E,SAAShD,EAAQD,EAASH,GAG/B,GAAIuK,GAAWvK,EAAoB,GAC/BwK,EAAWxK,EAAoB,IAC/BqB,EAAWrB,EAAoB,GAC/BsN,EAAWtN,EAAoB,IAC/BkP,EAAWlP,EAAoB,GAEnCI,GAAOD,QAAU,SAASmT,EAAKxP,EAAQoI,GACrC,GAAI0T,GAAW1Q,EAAIoE,GACf/E,EAAW,GAAG+E,EACfjS,GAAM,WACP,GAAI+B,KAEJ,OADAA,GAAEwc,GAAU,WAAY,MAAO,IACV,GAAd,GAAGtM,GAAKlQ,OAEfoH,EAASoC,OAAOxK,UAAWkR,EAAKpH,EAAKoB,EAASsS,EAAQrR,IACtDhE,EAAK0L,OAAO7T,UAAWwd,EAAkB,GAAV9b,EAG3B,SAASsS,EAAQ3H,GAAM,MAAOF,GAAShO,KAAK6V,EAAQ1P,KAAM+H,IAG1D,SAAS2H,GAAS,MAAO7H,GAAShO,KAAK6V,EAAQ1P,WAOlD,SAAStG,EAAQD,EAASH,GAG/BA,EAAoB,KAAK,UAAW,EAAG,SAASsN,EAASuS,EAASC,GAEhE,MAAO,SAASzJ,SAAQ0J,EAAaC,GAEnC,GAAI5c,GAAKkK,EAAQ5G,MACbD,EAAKsZ,GAAejgB,EAAYA,EAAYigB,EAAYF,EAC5D,OAAOpZ,KAAO3G,EACV2G,EAAGlG,KAAKwf,EAAa3c,EAAG4c,GACxBF,EAASvf,KAAKqM,OAAOxJ,GAAI2c,EAAaC,OAMzC,SAAS5f,EAAQD,EAASH,GAG/BA,EAAoB,KAAK,SAAU,EAAG,SAASsN,EAAS2S,GAEtD,MAAO,SAASzF,QAAOmF,GAErB,GAAIvc,GAAKkK,EAAQ5G,MACbD,EAAKkZ,GAAU7f,EAAYA,EAAY6f,EAAOM,EAClD,OAAOxZ,KAAO3G,EAAY2G,EAAGlG,KAAKof,EAAQvc,GAAK,GAAI6S,QAAO0J,GAAQM,GAAQrT,OAAOxJ,QAMhF,SAAShD,EAAQD,EAASH,GAG/BA,EAAoB,KAAK,QAAS,EAAG,SAASsN,EAAS4S,EAAOC,GAE5D,MAAO,SAAS/b,OAAMkD,EAAW8Y,GAE/B,GAAIhd,GAAKkK,EAAQ5G,MACbD,EAAKa,GAAaxH,EAAYA,EAAYwH,EAAU4Y,EACxD,OAAOzZ,KAAO3G,EACV2G,EAAGlG,KAAK+G,EAAWlE,EAAGgd,GACtBD,EAAO5f,KAAKqM,OAAOxJ,GAAIkE,EAAW8Y,OAMrC,SAAShgB,EAAQD,EAASH,GAG/B,GAqBIqgB,GArBAzf,EAAaZ,EAAoB,GACjCyb,EAAazb,EAAoB,IACjCqK,EAAarK,EAAoB,GACjCyK,EAAazK,EAAoB,IACjCiT,EAAajT,EAAoB,IACjCa,EAAab,EAAoB,GACjCwB,EAAaxB,EAAoB,IACjCsB,EAAatB,EAAoB,IACjCuB,EAAavB,EAAoB,IACjCsgB,EAAatgB,EAAoB,KACjCugB,EAAavgB,EAAoB,KACjCwgB,EAAaxgB,EAAoB,IAAIwQ,IACrCiQ,EAAazgB,EAAoB,IACjCsO,EAAatO,EAAoB,IAAI,WACrC0gB,EAAqB1gB,EAAoB,KACzC2gB,EAAa3gB,EAAoB,KACjC4gB,EAAa,UACbC,EAAaxW,EAAOwW,QACpBC,EAAiC,WAApB7N,EAAQ4N,GACrBxd,EAAagH,EAAOuW,GACpBG,EAAa,aAGbC,EAAc,SAASC,GACzB,GAAyBC,GAArBpO,EAAO,GAAIzP,GAAE0d,EAKjB,OAJGE,KAAInO,EAAKhN,YAAc,SAASoG,GACjCA,EAAK6U,EAAOA,MAEbG,EAAU7d,EAAE8d,QAAQrO,IAAO,SAASiO,GAC9BG,IAAYpO,GAGjBsO,EAAa,WAEf,QAASC,IAAG3O,GACV,GAAI9G,GAAO,GAAIvI,GAAEqP,EAEjB,OADA8N,GAAS5U,EAAMyV,GAAGjf,WACXwJ,EAJT,GAAI0V,IAAQ,CAMZ,KASE,GARAA,EAAQje,GAAKA,EAAE8d,SAAWH,IAC1BR,EAASa,GAAIhe,GACbge,GAAGjf,UAAYxB,EAAEqF,OAAO5C,EAAEjB,WAAY0D,aAAcrC,MAAO4d,MAEtDA,GAAGF,QAAQ,GAAGI,KAAK,uBAAyBF,MAC/CC,GAAQ;AAGPA,GAASthB,EAAoB,GAAG,CACjC,GAAIwhB,IAAqB,CACzBne,GAAE8d,QAAQvgB,EAAEgC,WAAY,QACtBM,IAAK,WAAYse,GAAqB,MAExCF,EAAQE,GAEV,MAAMje,GAAI+d,GAAQ,EACpB,MAAOA,MAILG,EAAkB,SAASte,EAAG6J,GAEhC,MAAGyO,IAAWtY,IAAME,GAAK2J,IAAMqT,GAAe,EACvCI,EAAKtd,EAAG6J,IAEb0U,EAAiB,SAASlT,GAC5B,GAAIxK,GAAI1C,EAASkN,GAAGF,EACpB,OAAOtK,IAAKlE,EAAYkE,EAAIwK,GAE1BmT,EAAa,SAASnV,GACxB,GAAI+U,EACJ,OAAO/f,GAASgL,IAAkC,mBAAnB+U,EAAO/U,EAAG+U,MAAsBA,GAAO,GAEpEK,EAAoB,SAASpT,GAC/B,GAAI2S,GAASU,CACbnb,MAAKwa,QAAU,GAAI1S,GAAE,SAASsT,EAAWC,GACvC,GAAGZ,IAAYrhB,GAAa+hB,IAAW/hB,EAAU,KAAM0D,WAAU,0BACjE2d,GAAUW,EACVD,EAAUE,IAEZrb,KAAKya,QAAU5f,EAAU4f,GACzBza,KAAKmb,OAAUtgB,EAAUsgB,IAEvBG,EAAU,SAAS9V,GACrB,IACEA,IACA,MAAM3I,GACN,OAAQ0e,MAAO1e,KAGf2e,EAAS,SAASC,EAAQC,GAC5B,IAAGD,EAAO9b,EAAV,CACA8b,EAAO9b,GAAI,CACX,IAAIgc,GAAQF,EAAO1hB,CACnBkgB,GAAK,WAuBH,IAtBA,GAAIld,GAAQ0e,EAAOG,EACfC,EAAoB,GAAZJ,EAAOzY,EACf3F,EAAQ,EACRye,EAAM,SAASC,GACjB,GAGIhd,GAAQ8b,EAHRmB,EAAUH,EAAKE,EAASF,GAAKE,EAASE,KACtCxB,EAAUsB,EAAStB,QACnBU,EAAUY,EAASZ,MAEvB,KACKa,GACGH,IAAGJ,EAAOS,GAAI,GAClBnd,EAASid,KAAY,EAAOjf,EAAQif,EAAQjf,GACzCgC,IAAWgd,EAASvB,QACrBW,EAAOre,UAAU,yBACT+d,EAAOI,EAAWlc,IAC1B8b,EAAKhhB,KAAKkF,EAAQ0b,EAASU,GACtBV,EAAQ1b,IACVoc,EAAOpe,GACd,MAAMF,GACNse,EAAOte,KAGL8e,EAAMve,OAASC,GAAEye,EAAIH,EAAMte,KACjCse,GAAMve,OAAS,EACfqe,EAAO9b,GAAI,EACR+b,GAASS,WAAW,WACrB,GACIH,GAASI,EADT5B,EAAUiB,EAAOzhB,CAElBqiB,GAAY7B,KACVJ,EACDD,EAAQmC,KAAK,qBAAsBvf,EAAOyd,IAClCwB,EAAUrY,EAAO4Y,sBACzBP,GAASxB,QAASA,EAASgC,OAAQzf,KAC1Bqf,EAAUzY,EAAOyY,UAAYA,EAAQb,OAC9Ca,EAAQb,MAAM,8BAA+Bxe,IAE/C0e,EAAOhf,EAAIrD,GACZ,OAGHijB,EAAc,SAAS7B,GACzB,GAGIuB,GAHAN,EAASjB,EAAQiC,GACjBd,EAASF,EAAOhf,GAAKgf,EAAO1hB,EAC5BsD,EAAS,CAEb,IAAGoe,EAAOS,EAAE,OAAO,CACnB,MAAMP,EAAMve,OAASC,GAEnB,GADA0e,EAAWJ,EAAMte,KACd0e,EAASE,OAASI,EAAYN,EAASvB,SAAS,OAAO,CAC1D,QAAO,GAEPkC,EAAU,SAAS3f,GACrB,GAAI0e,GAASzb,IACVyb,GAAO7Y,IACV6Y,EAAO7Y,GAAI,EACX6Y,EAASA,EAAOkB,GAAKlB,EACrBA,EAAOG,EAAI7e,EACX0e,EAAOzY,EAAI,EACXyY,EAAOhf,EAAIgf,EAAO1hB,EAAE+B,QACpB0f,EAAOC,GAAQ,KAEbmB,EAAW,SAAS7f,GACtB,GACI8d,GADAY,EAASzb,IAEb,KAAGyb,EAAO7Y,EAAV,CACA6Y,EAAO7Y,GAAI,EACX6Y,EAASA,EAAOkB,GAAKlB,CACrB,KACE,GAAGA,EAAOzhB,IAAM+C,EAAM,KAAMD,WAAU,qCACnC+d,EAAOI,EAAWle,IACnBkd,EAAK,WACH,GAAI4C,IAAWF,EAAGlB,EAAQ7Y,GAAG,EAC7B,KACEiY,EAAKhhB,KAAKkD,EAAOgH,EAAI6Y,EAAUC,EAAS,GAAI9Y,EAAI2Y,EAASG,EAAS,IAClE,MAAMhgB,GACN6f,EAAQ7iB,KAAKgjB,EAAShgB,OAI1B4e,EAAOG,EAAI7e,EACX0e,EAAOzY,EAAI,EACXwY,EAAOC,GAAQ,IAEjB,MAAM5e,GACN6f,EAAQ7iB,MAAM8iB,EAAGlB,EAAQ7Y,GAAG,GAAQ/F,KAKpC6d,KAEF/d,EAAI,QAASmgB,SAAQC,GACnBliB,EAAUkiB,EACV,IAAItB,GAASzb,KAAKyc,IAChBziB,EAAG4f,EAAU5Z,KAAMrD,EAAGud,GACtBngB,KACA0C,EAAGrD,EACH4J,EAAG,EACHJ,GAAG,EACHgZ,EAAGxiB,EACH8iB,GAAG,EACHvc,GAAG,EAEL,KACEod,EAAShZ,EAAI6Y,EAAUnB,EAAQ,GAAI1X,EAAI2Y,EAASjB,EAAQ,IACxD,MAAMuB,GACNN,EAAQ7iB,KAAK4hB,EAAQuB,KAGzB1jB,EAAoB,KAAKqD,EAAEjB,WAEzBmf,KAAM,QAASA,MAAKoC,EAAaC,GAC/B,GAAInB,GAAW,GAAIb,GAAkBlB,EAAmBha,KAAMrD,IAC1D6d,EAAWuB,EAASvB,QACpBiB,EAAWzb,KAAKyc,EAMpB,OALAV,GAASF,GAA6B,kBAAfoB,GAA4BA,GAAc,EACjElB,EAASE,KAA4B,kBAAdiB,IAA4BA,EACnDzB,EAAO1hB,EAAEiF,KAAK+c,GACXN,EAAOhf,GAAEgf,EAAOhf,EAAEuC,KAAK+c,GACvBN,EAAOzY,GAAEwY,EAAOC,GAAQ,GACpBjB,GAGT2C,QAAS,SAASD,GAChB,MAAOld,MAAK6a,KAAKzhB,EAAW8jB,OAKlC/iB,EAAQA,EAAQsK,EAAItK,EAAQ6K,EAAI7K,EAAQoD,GAAKmd,GAAaoC,QAASngB,IACnErD,EAAoB,IAAIqD,EAAGud,GAC3B5gB,EAAoB,KAAK4gB,GACzBP,EAAUrgB,EAAoB,GAAG4gB,GAGjC/f,EAAQA,EAAQmD,EAAInD,EAAQoD,GAAKmd,EAAYR,GAE3CiB,OAAQ,QAASA,QAAOwB,GACtB,GAAIS,GAAa,GAAIlC,GAAkBlb,MACnCqb,EAAa+B,EAAWjC,MAE5B,OADAE,GAASsB,GACFS,EAAW5C,WAGtBrgB,EAAQA,EAAQmD,EAAInD,EAAQoD,IAAMmd,GAAcJ,GAAY,IAAQJ,GAElEO,QAAS,QAASA,SAAQzO,GAExB,GAAGA,YAAarP,IAAKoe,EAAgB/O,EAAE5M,YAAaY,MAAM,MAAOgM,EACjE,IAAIoR,GAAa,GAAIlC,GAAkBlb,MACnCob,EAAagC,EAAW3C,OAE5B,OADAW,GAAUpP,GACHoR,EAAW5C,WAGtBrgB,EAAQA,EAAQmD,EAAInD,EAAQoD,IAAMmd,GAAcphB,EAAoB,KAAK,SAASod,GAChF/Z,EAAE0gB,IAAI3G,GAAM,SAAS,iBAClBwD,GAEHmD,IAAK,QAASA,KAAIC,GAChB,GAAIxV,GAAakT,EAAehb,MAC5Bod,EAAa,GAAIlC,GAAkBpT,GACnC2S,EAAa2C,EAAW3C,QACxBU,EAAaiC,EAAWjC,OACxBnF,KACAuH,EAASjC,EAAQ,WACnBzB,EAAMyD,GAAU,EAAOtH,EAAOhX,KAAMgX,EACpC,IAAIwH,GAAYxH,EAAO5Y,OACnBqgB,EAAY7hB,MAAM4hB,EACnBA,GAAUtjB,EAAEqH,KAAK1H,KAAKmc,EAAQ,SAASwE,EAAStZ,GACjD,GAAIwc,IAAgB,CACpB5V,GAAE2S,QAAQD,GAASK,KAAK,SAAS9d,GAC5B2gB,IACHA,GAAgB,EAChBD,EAAQvc,GAASnE,IACfygB,GAAa/C,EAAQgD,KACtBtC,KAEAV,EAAQgD,IAGf,OADGF,IAAOpC,EAAOoC,EAAOhC,OACjB6B,EAAW5C,SAGpBmD,KAAM,QAASA,MAAKL,GAClB,GAAIxV,GAAakT,EAAehb,MAC5Bod,EAAa,GAAIlC,GAAkBpT,GACnCqT,EAAaiC,EAAWjC,OACxBoC,EAASjC,EAAQ,WACnBzB,EAAMyD,GAAU,EAAO,SAAS9C,GAC9B1S,EAAE2S,QAAQD,GAASK,KAAKuC,EAAW3C,QAASU,MAIhD,OADGoC,IAAOpC,EAAOoC,EAAOhC,OACjB6B,EAAW5C,YAMjB,SAAS9gB,EAAQD,GAEtBC,EAAOD,QAAU,SAASqM,EAAI0P,EAAatR,GACzC,KAAK4B,YAAc0P,IAAa,KAAM1Y,WAAUoH,EAAO,4BACvD,OAAO4B,KAKJ,SAASpM,EAAQD,EAASH,GAE/B,GAAIyK,GAAczK,EAAoB,IAClCO,EAAcP,EAAoB,KAClCkd,EAAcld,EAAoB,KAClCsB,EAActB,EAAoB,IAClC6B,EAAc7B,EAAoB,IAClCmd,EAAcnd,EAAoB,IACtCI,GAAOD,QAAU,SAAS6jB,EAAUrH,EAASlW,EAAID,GAC/C,GAGI1C,GAAQyZ,EAAMC,EAHdG,EAASR,EAAU6G,GACnB3V,EAAS5D,EAAIhE,EAAID,EAAMmW,EAAU,EAAI,GACrC/U,EAAS,CAEb,IAAoB,kBAAV+V,GAAqB,KAAMna,WAAUwgB,EAAW,oBAE1D,IAAG9G,EAAYS,GAAQ,IAAI7Z,EAASjC,EAASmiB,EAASlgB,QAASA,EAAS8D,EAAOA,IAC7E+U,EAAUtO,EAAE/M,EAASic,EAAOyG,EAASpc,IAAQ,GAAI2V,EAAK,IAAMlP,EAAE2V,EAASpc,QAClE,KAAI4V,EAAWG,EAAOpd,KAAKyjB,KAAazG,EAAOC,EAASrB,QAAQX,MACrEjb,EAAKid,EAAUnP,EAAGkP,EAAK9Z,MAAOkZ,KAM7B,SAASvc,EAAQD,EAASH,GAG/B,GAAIsB,GAAYtB,EAAoB,IAChCuB,EAAYvB,EAAoB,IAChCsO,EAAYtO,EAAoB,IAAI,UACxCI,GAAOD,QAAU,SAASiD,EAAG8M,GAC3B,GAAiClM,GAA7BwK,EAAIlN,EAAS8B,GAAG0C,WACpB,OAAO0I,KAAM1O,IAAckE,EAAI1C,EAASkN,GAAGF,KAAaxO,EAAYoQ,EAAI3O,EAAUyC,KAK/E,SAAS5D,EAAQD,EAASH,GAE/B,GAMIskB,GAAMC,EAAMrC,EANZ7X,EAAYrK,EAAoB,GAChCwkB,EAAYxkB,EAAoB,KAAKwQ,IACrCiU,EAAYpa,EAAOqa,kBAAoBra,EAAOsa,uBAC9C9D,EAAYxW,EAAOwW,QACnB2C,EAAYnZ,EAAOmZ,QACnB1C,EAAgD,WAApC9gB,EAAoB,IAAI6gB,GAGpC+D,EAAQ,WACV,GAAIC,GAAQC,EAAQre,CAKpB,KAJGqa,IAAW+D,EAAShE,EAAQiE,UAC7BjE,EAAQiE,OAAS,KACjBD,EAAOE,QAEHT,GACJQ,EAASR,EAAKQ,OACdre,EAAS6d,EAAK7d,GACXqe,GAAOA,EAAOE,QACjBve,IACGqe,GAAOA,EAAOC,OACjBT,EAAOA,EAAKnI,IACZoI,GAAOzkB,EACN+kB,GAAOA,EAAOG,QAInB,IAAGlE,EACDoB,EAAS,WACPrB,EAAQoE,SAASL,QAGd,IAAGH,EAAS,CACjB,GAAIS,GAAS,EACTC,EAASlgB,SAASmgB,eAAe,GACrC,IAAIX,GAASG,GAAOS,QAAQF,GAAOG,eAAe,IAClDpD,EAAS,WACPiD,EAAKI,KAAOL,GAAUA,OAIxBhD,GADQsB,GAAWA,EAAQrC,QAClB,WACPqC,EAAQrC,UAAUI,KAAKqD,IAShB,WAEPJ,EAAUjkB,KAAK8J,EAAQua,GAI3BxkB,GAAOD,QAAU,QAASwgB,MAAKla,GAC7B,GAAI+e,IAAQ/e,GAAIA,EAAI0V,KAAMrc,EAAWglB,OAAQhE,GAAUD,EAAQiE,OAC5DP,KAAKA,EAAKpI,KAAOqJ,GAChBlB,IACFA,EAAOkB,EACPtD,KACAqC,EAAOiB,IAKN,SAASplB,EAAQD,EAASH,GAE/B,GAYIylB,GAAOC,EAASC,EAZhBlb,EAAqBzK,EAAoB,IACzCoB,EAAqBpB,EAAoB,IACzCgB,EAAqBhB,EAAoB,IACzCiB,EAAqBjB,EAAoB,IACzCqK,EAAqBrK,EAAoB,GACzC6gB,EAAqBxW,EAAOwW,QAC5B+E,EAAqBvb,EAAOwb,aAC5BC,EAAqBzb,EAAO0b,eAC5BC,EAAqB3b,EAAO2b,eAC5BC,EAAqB,EACrBC,KACAC,EAAqB,qBAErB3D,EAAM,WACR,GAAIniB,IAAMqG,IACV,IAAGwf,EAAMvZ,eAAetM,GAAI,CAC1B,GAAIoG,GAAKyf,EAAM7lB,SACR6lB,GAAM7lB,GACboG,MAGA2f,EAAU,SAASC,GACrB7D,EAAIjiB,KAAK8lB,EAAMd,MAGbK,IAAYE,IACdF,EAAU,QAASC,cAAapf,GAE9B,IADA,GAAIL,MAAWrC,EAAI,EACb6C,UAAU9C,OAASC,GAAEqC,EAAKV,KAAKkB,UAAU7C,KAK/C,OAJAmiB,KAAQD,GAAW,WACjB7kB,EAAoB,kBAANqF,GAAmBA,EAAKH,SAASG,GAAKL,IAEtDqf,EAAMQ,GACCA,GAETH,EAAY,QAASC,gBAAe1lB,SAC3B6lB,GAAM7lB,IAGwB,WAApCL,EAAoB,IAAI6gB,GACzB4E,EAAQ,SAASplB,GACfwgB,EAAQoE,SAASxa,EAAI+X,EAAKniB,EAAI,KAGxB2lB,GACRN,EAAU,GAAIM,GACdL,EAAUD,EAAQY,MAClBZ,EAAQa,MAAMC,UAAYJ,EAC1BX,EAAQhb,EAAIkb,EAAKc,YAAad,EAAM,IAG5Btb,EAAOqc,kBAA0C,kBAAfD,eAA8Bpc,EAAOsc,eAC/ElB,EAAQ,SAASplB,GACfgK,EAAOoc,YAAYpmB,EAAK,GAAI,MAE9BgK,EAAOqc,iBAAiB,UAAWN,GAAS,IAG5CX,EADQU,IAAsBllB,GAAI,UAC1B,SAASZ,GACfW,EAAK8D,YAAY7D,EAAI,WAAWklB,GAAsB,WACpDnlB,EAAK4lB,YAAYlgB,MACjB8b,EAAIjiB,KAAKF,KAKL,SAASA,GACfwiB,WAAWpY,EAAI+X,EAAKniB,EAAI,GAAI,KAIlCD,EAAOD,SACLqQ,IAAOoV,EACPiB,MAAOf,IAKJ,SAAS1lB,EAAQD,EAASH,GAE/B,GAAIwK,GAAWxK,EAAoB,GACnCI,GAAOD,QAAU,SAASqL,EAAQzG,GAChC,IAAI,GAAIS,KAAOT,GAAIyF,EAASgB,EAAQhG,EAAKT,EAAIS,GAC7C,OAAOgG,KAKJ,SAASpL,EAAQD,EAASH,GAG/B,GAAI8mB,GAAS9mB,EAAoB,IAGjCA,GAAoB,KAAK,MAAO,SAASkD,GACvC,MAAO,SAAS6jB,OAAO,MAAO7jB,GAAIwD,KAAME,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAG9EoD,IAAK,QAASA,KAAIsC,GAChB,GAAIwhB,GAAQF,EAAOG,SAASvgB,KAAMlB,EAClC,OAAOwhB,IAASA,EAAM1E,GAGxB9R,IAAK,QAASA,KAAIhL,EAAK/B,GACrB,MAAOqjB,GAAOjV,IAAInL,KAAc,IAARlB,EAAY,EAAIA,EAAK/B,KAE9CqjB,GAAQ,IAIN,SAAS1mB,EAAQD,EAASH,GAG/B,GAAIY,GAAeZ,EAAoB,GACnCuK,EAAevK,EAAoB,GACnCknB,EAAelnB,EAAoB,KACnCyK,EAAezK,EAAoB,IACnCsgB,EAAetgB,EAAoB,KACnCsN,EAAetN,EAAoB,IACnCugB,EAAevgB,EAAoB,KACnCmnB,EAAennB,EAAoB,KACnCud,EAAevd,EAAoB,KACnConB,EAAepnB,EAAoB,IAAI,MACvCqnB,EAAernB,EAAoB,IACnCwB,EAAexB,EAAoB,IACnCsnB,EAAetnB,EAAoB,KACnCc,EAAed,EAAoB,GACnCgU,EAAe7R,OAAO6R,cAAgBxS,EACtC+lB,EAAezmB,EAAc,KAAO,OACpCT,EAAe,EAEfmnB,EAAU,SAAShb,EAAIvG,GAEzB,IAAIzE,EAASgL,GAAI,MAAoB,gBAANA,GAAiBA,GAAmB,gBAANA,GAAiB,IAAM,KAAOA,CAC3F,KAAI6a,EAAK7a,EAAI4a,GAAI,CAEf,IAAIpT,EAAaxH,GAAI,MAAO,GAE5B,KAAIvG,EAAO,MAAO,GAElBsE,GAAKiC,EAAI4a,IAAM/mB,GAEf,MAAO,IAAMmM,EAAG4a,IAGhBH,EAAW,SAASzgB,EAAMhB,GAE5B,GAA0BwhB,GAAtBpf,EAAQ4f,EAAQhiB,EACpB,IAAa,MAAVoC,EAAc,MAAOpB,GAAK8U,GAAG1T,EAEhC,KAAIof,EAAQxgB,EAAKihB,GAAIT,EAAOA,EAAQA,EAAM3gB,EACxC,GAAG2gB,EAAM1U,GAAK9M,EAAI,MAAOwhB,GAI7B5mB,GAAOD,SACLuhB,eAAgB,SAAS6B,EAASlP,EAAMxG,EAAQ6Z,GAC9C,GAAIlZ,GAAI+U,EAAQ,SAAS/c,EAAMwd,GAC7B1D,EAAU9Z,EAAMgI,EAAG6F,GACnB7N,EAAK8U,GAAK1a,EAAEqF,OAAO,MACnBO,EAAKihB,GAAK3nB,EACV0G,EAAKmhB,GAAK7nB,EACV0G,EAAK+gB,GAAQ,EACVvD,GAAYlkB,GAAUygB,EAAMyD,EAAUnW,EAAQrH,EAAKkhB,GAAQlhB,IAqDhE,OAnDA0gB,GAAY1Y,EAAEpM,WAGZykB,MAAO,QAASA,SACd,IAAI,GAAIrgB,GAAOE,KAAM6e,EAAO/e,EAAK8U,GAAI0L,EAAQxgB,EAAKihB,GAAIT,EAAOA,EAAQA,EAAM3gB,EACzE2gB,EAAM3D,GAAI,EACP2D,EAAMtmB,IAAEsmB,EAAMtmB,EAAIsmB,EAAMtmB,EAAE2F,EAAIvG,SAC1BylB,GAAKyB,EAAMjjB,EAEpByC,GAAKihB,GAAKjhB,EAAKmhB,GAAK7nB,EACpB0G,EAAK+gB,GAAQ,GAIfK,SAAU,SAASpiB,GACjB,GAAIgB,GAAQE,KACRsgB,EAAQC,EAASzgB,EAAMhB,EAC3B,IAAGwhB,EAAM,CACP,GAAI7K,GAAO6K,EAAM3gB,EACbwhB,EAAOb,EAAMtmB,QACV8F,GAAK8U,GAAG0L,EAAMjjB,GACrBijB,EAAM3D,GAAI,EACPwE,IAAKA,EAAKxhB,EAAI8V,GACdA,IAAKA,EAAKzb,EAAImnB,GACdrhB,EAAKihB,IAAMT,IAAMxgB,EAAKihB,GAAKtL,GAC3B3V,EAAKmhB,IAAMX,IAAMxgB,EAAKmhB,GAAKE,GAC9BrhB,EAAK+gB,KACL,QAASP,GAIbhf,QAAS,QAASA,SAAQN,GAGxB,IAFA,GACIsf,GADA3Y,EAAI5D,EAAI/C,EAAYd,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,EAAW,GAEnEknB,EAAQA,EAAQA,EAAM3gB,EAAIK,KAAK+gB,IAGnC,IAFApZ,EAAE2Y,EAAM1E,EAAG0E,EAAM1U,EAAG5L,MAEdsgB,GAASA,EAAM3D,GAAE2D,EAAQA,EAAMtmB,GAKzCQ,IAAK,QAASA,KAAIsE,GAChB,QAASyhB,EAASvgB,KAAMlB,MAGzB1E,GAAYF,EAAEgC,QAAQ4L,EAAEpM,UAAW,QACpCc,IAAK,WACH,MAAOoK,GAAQ5G,KAAK6gB,OAGjB/Y,GAETqD,IAAK,SAASrL,EAAMhB,EAAK/B,GACvB,GACIokB,GAAMjgB,EADNof,EAAQC,EAASzgB,EAAMhB,EAoBzB,OAjBCwhB,GACDA,EAAM1E,EAAI7e,GAGV+C,EAAKmhB,GAAKX,GACRjjB,EAAG6D,EAAQ4f,EAAQhiB,GAAK,GACxB8M,EAAG9M,EACH8c,EAAG7e,EACH/C,EAAGmnB,EAAOrhB,EAAKmhB,GACfthB,EAAGvG,EACHujB,GAAG,GAED7c,EAAKihB,KAAGjhB,EAAKihB,GAAKT,GACnBa,IAAKA,EAAKxhB,EAAI2gB,GACjBxgB,EAAK+gB,KAEQ,MAAV3f,IAAcpB,EAAK8U,GAAG1T,GAASof,IAC3BxgB,GAEXygB,SAAUA,EACVa,UAAW,SAAStZ,EAAG6F,EAAMxG,GAG3BsZ,EAAY3Y,EAAG6F,EAAM,SAAS+G,EAAUqB,GACtC/V,KAAK2U,GAAKD,EACV1U,KAAK6J,GAAKkM,EACV/V,KAAKihB,GAAK7nB,GACT,WAKD,IAJA,GAAI0G,GAAQE,KACR+V,EAAQjW,EAAK+J,GACbyW,EAAQxgB,EAAKmhB,GAEXX,GAASA,EAAM3D,GAAE2D,EAAQA,EAAMtmB,CAErC,OAAI8F,GAAK6U,KAAQ7U,EAAKmhB,GAAKX,EAAQA,EAAQA,EAAM3gB,EAAIG,EAAK6U,GAAGoM,IAMlD,QAARhL,EAAwBc,EAAK,EAAGyJ,EAAM1U,GAC9B,UAARmK,EAAwBc,EAAK,EAAGyJ,EAAM1E,GAClC/E,EAAK,GAAIyJ,EAAM1U,EAAG0U,EAAM1E,KAN7B9b,EAAK6U,GAAKvb,EACHyd,EAAK,KAMb1P,EAAS,UAAY,UAAYA,GAAQ,GAG5CyZ,EAAWjT,MAMV,SAASjU,EAAQD,EAASH,GAG/B,GAAIqK,GAAiBrK,EAAoB,GACrCa,EAAiBb,EAAoB,GACrCwK,EAAiBxK,EAAoB,IACrCknB,EAAiBlnB,EAAoB,KACrCugB,EAAiBvgB,EAAoB,KACrCsgB,EAAiBtgB,EAAoB,KACrCwB,EAAiBxB,EAAoB,IACrCqB,EAAiBrB,EAAoB,GACrC+nB,EAAiB/nB,EAAoB,KACrCiP,EAAiBjP,EAAoB,GAEzCI,GAAOD,QAAU,SAASkU,EAAMkP,EAAShH,EAASyL,EAAQna,EAAQoa,GAChE,GAAInT,GAAQzK,EAAOgK,GACf7F,EAAQsG,EACR4S,EAAQ7Z,EAAS,MAAQ,MACzBgF,EAAQrE,GAAKA,EAAEpM,UACfgB,KACA8kB,EAAY,SAAS5U,GACvB,GAAI7M,GAAKoM,EAAMS,EACf9I,GAASqI,EAAOS,EACP,UAAPA,EAAkB,SAASnQ,GACzB,MAAO8kB,KAAYzmB,EAAS2B,IAAK,EAAQsD,EAAGlG,KAAKmG,KAAY,IAANvD,EAAU,EAAIA,IAC5D,OAAPmQ,EAAe,QAASpS,KAAIiC,GAC9B,MAAO8kB,KAAYzmB,EAAS2B,IAAK,EAAQsD,EAAGlG,KAAKmG,KAAY,IAANvD,EAAU,EAAIA,IAC5D,OAAPmQ,EAAe,QAASpQ,KAAIC,GAC9B,MAAO8kB,KAAYzmB,EAAS2B,GAAKrD,EAAY2G,EAAGlG,KAAKmG,KAAY,IAANvD,EAAU,EAAIA,IAChE,OAAPmQ,EAAe,QAAS6U,KAAIhlB,GAAoC,MAAhCsD,GAAGlG,KAAKmG,KAAY,IAANvD,EAAU,EAAIA,GAAWuD,MACvE,QAAS8J,KAAIrN,EAAG6J,GAAuC,MAAnCvG,GAAGlG,KAAKmG,KAAY,IAANvD,EAAU,EAAIA,EAAG6J,GAAWtG,OAGtE,IAAe,kBAAL8H,KAAqByZ,GAAWpV,EAAM7K,UAAY3G,EAAM,YAChE,GAAImN,IAAImO,UAAUR,UAKb,CACL,GAQIiM,GARAC,EAAuB,GAAI7Z,GAE3B8Z,EAAuBD,EAASX,GAAOO,MAAgB,EAAG,IAAMI,EAEhEE,EAAuBlnB,EAAM,WAAYgnB,EAASnnB,IAAI,KAEtDsnB,EAAuBT,EAAY,SAAS3K,GAAO,GAAI5O,GAAE4O,IAGzDoL,KACFha,EAAI+U,EAAQ,SAAS/X,EAAQwY,GAC3B1D,EAAU9U,EAAQgD,EAAG6F,EACrB,IAAI7N,GAAO,GAAIsO,EAEf,OADGkP,IAAYlkB,GAAUygB,EAAMyD,EAAUnW,EAAQrH,EAAKkhB,GAAQlhB,GACvDA,IAETgI,EAAEpM,UAAYyQ,EACdA,EAAM/M,YAAc0I,GAEtByZ,GAAWI,EAASrgB,QAAQ,SAASyE,EAAKjH,GACxC4iB,EAAa,EAAI5iB,MAAS6S,EAAAA,MAEzBkQ,GAAwBH,KACzBF,EAAU,UACVA,EAAU,OACVra,GAAUqa,EAAU,SAEnBE,GAAcE,IAAeJ,EAAUR,GAEvCO,GAAWpV,EAAMgU,aAAahU,GAAMgU,UAhCvCrY,GAAIwZ,EAAOtG,eAAe6B,EAASlP,EAAMxG,EAAQ6Z,GACjDR,EAAY1Y,EAAEpM,UAAWma,EAyC3B,OAPAtN,GAAeT,EAAG6F,GAElBjR,EAAEiR,GAAQ7F,EACV3N,EAAQA,EAAQsK,EAAItK,EAAQ6K,EAAI7K,EAAQoD,GAAKuK,GAAKsG,GAAO1R,GAErD6kB,GAAQD,EAAOF,UAAUtZ,EAAG6F,EAAMxG,GAE/BW,IAKJ,SAASpO,EAAQD,EAASH,GAG/B,GAAI8mB,GAAS9mB,EAAoB,IAGjCA,GAAoB,KAAK,MAAO,SAASkD,GACvC,MAAO,SAASulB,OAAO,MAAOvlB,GAAIwD,KAAME,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAG9EqoB,IAAK,QAASA,KAAI1kB,GAChB,MAAOqjB,GAAOjV,IAAInL,KAAMjD,EAAkB,IAAVA,EAAc,EAAIA,EAAOA,KAE1DqjB,IAIE,SAAS1mB,EAAQD,EAASH,GAG/B,GAAIY,GAAeZ,EAAoB,GACnCwK,EAAexK,EAAoB,IACnC0oB,EAAe1oB,EAAoB,KACnCwB,EAAexB,EAAoB,IACnCkB,EAAelB,EAAoB,IACnC2oB,EAAeD,EAAKC,YACpBC,EAAeF,EAAKE,KACpB5U,EAAe7R,OAAO6R,cAAgBxS,EACtCqnB,KAGAC,EAAW9oB,EAAoB,KAAK,UAAW,SAASkD,GAC1D,MAAO,SAAS6lB,WAAW,MAAO7lB,GAAIwD,KAAME,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAGlFoD,IAAK,QAASA,KAAIsC,GAChB,GAAGhE,EAASgE,GAAK,CACf,IAAIwO,EAAaxO,GAAK,MAAOmjB,GAAYjiB,MAAMxD,IAAIsC,EACnD,IAAGtE,EAAIsE,EAAKojB,GAAM,MAAOpjB,GAAIojB,GAAMliB,KAAK4U,MAI5C9K,IAAK,QAASA,KAAIhL,EAAK/B,GACrB,MAAOilB,GAAK7W,IAAInL,KAAMlB,EAAK/B,KAE5BilB,GAAM,GAAM,EAGsD,KAAlE,GAAII,IAAWtY,KAAKrO,OAAOkR,QAAUlR,QAAQ0mB,GAAM,GAAG3lB,IAAI2lB,IAC3DjoB,EAAEqH,KAAK1H,MAAM,SAAU,MAAO,MAAO,OAAQ,SAASiF,GACpD,GAAIqN,GAASiW,EAAS1mB,UAClB4mB,EAASnW,EAAMrN,EACnBgF,GAASqI,EAAOrN,EAAK,SAASrC,EAAG6J,GAE/B,GAAGxL,EAAS2B,KAAO6Q,EAAa7Q,GAAG,CACjC,GAAIsC,GAASkjB,EAAYjiB,MAAMlB,GAAKrC,EAAG6J,EACvC,OAAc,OAAPxH,EAAekB,KAAOjB,EAE7B,MAAOujB,GAAOzoB,KAAKmG,KAAMvD,EAAG6J,QAO/B,SAAS5M,EAAQD,EAASH,GAG/B,GAAIuK,GAAoBvK,EAAoB,GACxCknB,EAAoBlnB,EAAoB,KACxCsB,EAAoBtB,EAAoB,IACxCwB,EAAoBxB,EAAoB,IACxCsgB,EAAoBtgB,EAAoB,KACxCugB,EAAoBvgB,EAAoB,KACxCgC,EAAoBhC,EAAoB,IACxCqnB,EAAoBrnB,EAAoB,IACxC4oB,EAAoB5oB,EAAoB,IAAI,QAC5CgU,EAAoB7R,OAAO6R,cAAgBxS,EAC3CynB,EAAoBjnB,EAAkB,GACtCknB,EAAoBlnB,EAAkB,GACtC3B,EAAoB,EAGpBsoB,EAAc,SAASniB,GACzB,MAAOA,GAAKmhB,KAAOnhB,EAAKmhB,GAAK,GAAIwB,KAE/BA,EAAc,WAChBziB,KAAKvD,MAEHimB,EAAa,SAAS1a,EAAOlJ,GAC/B,MAAOyjB,GAAUva,EAAMvL,EAAG,SAASqJ,GACjC,MAAOA,GAAG,KAAOhH,IAGrB2jB,GAAY/mB,WACVc,IAAK,SAASsC,GACZ,GAAIwhB,GAAQoC,EAAW1iB,KAAMlB,EAC7B,OAAGwhB,GAAaA,EAAM,GAAtB,QAEF9lB,IAAK,SAASsE,GACZ,QAAS4jB,EAAW1iB,KAAMlB,IAE5BgL,IAAK,SAAShL,EAAK/B,GACjB,GAAIujB,GAAQoC,EAAW1iB,KAAMlB,EAC1BwhB,GAAMA,EAAM,GAAKvjB,EACfiD,KAAKvD,EAAEuC,MAAMF,EAAK/B,KAEzBmkB,SAAU,SAASpiB,GACjB,GAAIoC,GAAQshB,EAAexiB,KAAKvD,EAAG,SAASqJ,GAC1C,MAAOA,GAAG,KAAOhH,GAGnB,QADIoC,GAAMlB,KAAKvD,EAAEkmB,OAAOzhB,EAAO,MACrBA,IAIdxH,EAAOD,SACLuhB,eAAgB,SAAS6B,EAASlP,EAAMxG,EAAQ6Z,GAC9C,GAAIlZ,GAAI+U,EAAQ,SAAS/c,EAAMwd,GAC7B1D,EAAU9Z,EAAMgI,EAAG6F,GACnB7N,EAAK8U,GAAKjb,IACVmG,EAAKmhB,GAAK7nB,EACPkkB,GAAYlkB,GAAUygB,EAAMyD,EAAUnW,EAAQrH,EAAKkhB,GAAQlhB,IAkBhE,OAhBA0gB,GAAY1Y,EAAEpM,WAGZwlB,SAAU,SAASpiB,GACjB,MAAIhE,GAASgE,GACTwO,EAAaxO,GACV6hB,EAAK7hB,EAAKojB,IAASvB,EAAK7hB,EAAIojB,GAAOliB,KAAK4U,WAAc9V,GAAIojB,GAAMliB,KAAK4U,IAD/CqN,EAAYjiB,MAAM,UAAUlB,IADhC,GAM3BtE,IAAK,QAASA,KAAIsE,GAChB,MAAIhE,GAASgE,GACTwO,EAAaxO,GACV6hB,EAAK7hB,EAAKojB,IAASvB,EAAK7hB,EAAIojB,GAAOliB,KAAK4U,IADlBqN,EAAYjiB,MAAMxF,IAAIsE,IAD1B,KAKtBgJ,GAETqD,IAAK,SAASrL,EAAMhB,EAAK/B,GAMrB,MALEuQ,GAAa1S,EAASkE,KAGxB6hB,EAAK7hB,EAAKojB,IAASre,EAAK/E,EAAKojB,MAC7BpjB,EAAIojB,GAAMpiB,EAAK8U,IAAM7X,GAHrBklB,EAAYniB,GAAMgK,IAAIhL,EAAK/B,GAIpB+C,GAEXmiB,YAAaA,EACbC,KAAMA,IAKH,SAASxoB,EAAQD,EAASH,GAG/B,GAAI0oB,GAAO1oB,EAAoB,IAG/BA,GAAoB,KAAK,UAAW,SAASkD,GAC3C,MAAO,SAASomB,WAAW,MAAOpmB,GAAIwD,KAAME,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAGlFqoB,IAAK,QAASA,KAAI1kB,GAChB,MAAOilB,GAAK7W,IAAInL,KAAMjD,GAAO,KAE9BilB,GAAM,GAAO,IAIX,SAAStoB,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,GAC/BupB,EAAWjjB,SAAS2G,MACpB3L,EAAWtB,EAAoB,GAEnCa,GAAQA,EAAQmD,EAAG,WACjBiJ,MAAO,QAASA,OAAMzB,EAAQge,EAAcC,GAC1C,MAAOF,GAAOhpB,KAAKiL,EAAQge,EAAcloB,EAASmoB,QAMjD,SAASrpB,EAAQD,EAASH,GAG/B,GAAIY,GAAYZ,EAAoB,GAChCa,EAAYb,EAAoB,GAChCuB,EAAYvB,EAAoB,IAChCsB,EAAYtB,EAAoB,IAChCwB,EAAYxB,EAAoB,IAChCuG,EAAYD,SAASC,MAAQvG,EAAoB,GAAGsG,SAASlE,UAAUmE,IAI3E1F,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAIjE,EAAoB,GAAG,WACrD,QAASiE,MACT,QAASylB,QAAQxjB,UAAU,gBAAkBjC,YAAcA,MACzD,WACFiC,UAAW,QAASA,WAAUyjB,EAAQvjB,GACpC7E,EAAUooB,GACVroB,EAAS8E,EACT,IAAIwjB,GAAYhjB,UAAU9C,OAAS,EAAI6lB,EAASpoB,EAAUqF,UAAU,GACpE,IAAG+iB,GAAUC,EAAU,CAErB,OAAOxjB,EAAKtC,QACV,IAAK,GAAG,MAAO,IAAI6lB,EACnB,KAAK,GAAG,MAAO,IAAIA,GAAOvjB,EAAK,GAC/B,KAAK,GAAG,MAAO,IAAIujB,GAAOvjB,EAAK,GAAIA,EAAK,GACxC,KAAK,GAAG,MAAO,IAAIujB,GAAOvjB,EAAK,GAAIA,EAAK,GAAIA,EAAK,GACjD,KAAK,GAAG,MAAO,IAAIujB,GAAOvjB,EAAK,GAAIA,EAAK,GAAIA,EAAK,GAAIA,EAAK,IAG5D,GAAIyjB,IAAS,KAEb,OADAA,GAAMnkB,KAAKuH,MAAM4c,EAAOzjB,GACjB,IAAKG,EAAK0G,MAAM0c,EAAQE,IAGjC,GAAIhX,GAAW+W,EAAUxnB,UACrBimB,EAAWznB,EAAEqF,OAAOzE,EAASqR,GAASA,EAAQ1Q,OAAOC,WACrDqD,EAAWa,SAAS2G,MAAM1M,KAAKopB,EAAQtB,EAAUjiB,EACrD,OAAO5E,GAASiE,GAAUA,EAAS4iB,MAMlC,SAASjoB,EAAQD,EAASH,GAG/B,GAAIY,GAAWZ,EAAoB,GAC/Ba,EAAWb,EAAoB,GAC/BsB,EAAWtB,EAAoB,GAGnCa,GAAQA,EAAQmD,EAAInD,EAAQoD,EAAIjE,EAAoB,GAAG,WACrD0pB,QAAQ/mB,eAAe/B,EAAEgC,WAAY,GAAIa,MAAO,IAAK,GAAIA,MAAO,MAC9D,WACFd,eAAgB,QAASA,gBAAe6I,EAAQse,EAAaC,GAC3DzoB,EAASkK,EACT,KAEE,MADA5K,GAAEgC,QAAQ4I,EAAQse,EAAaC,IACxB,EACP,MAAMxmB,GACN,OAAO,OAOR,SAASnD,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,GAC/B8C,EAAW9C,EAAoB,GAAG8C,QAClCxB,EAAWtB,EAAoB,GAEnCa,GAAQA,EAAQmD,EAAG,WACjBgmB,eAAgB,QAASA,gBAAexe,EAAQse,GAC9C,GAAIG,GAAOnnB,EAAQxB,EAASkK,GAASse,EACrC,OAAOG,KAASA,EAAKje,cAAe,QAAeR,GAAOse,OAMzD,SAAS1pB,EAAQD,EAASH,GAI/B,GAAIa,GAAWb,EAAoB,GAC/BsB,EAAWtB,EAAoB,IAC/BkqB,EAAY,SAAS9O,GACvB1U,KAAK2U,GAAK/Z,EAAS8Z,GACnB1U,KAAK4U,GAAK,CACV,IACI9V,GADA5B,EAAO8C,KAAK6J,KAEhB,KAAI/K,IAAO4V,GAASxX,EAAK8B,KAAKF,GAEhCxF,GAAoB,KAAKkqB,EAAW,SAAU,WAC5C,GAEI1kB,GAFAgB,EAAOE,KACP9C,EAAO4C,EAAK+J,EAEhB,GACE,IAAG/J,EAAK8U,IAAM1X,EAAKE,OAAO,OAAQL,MAAO3D,EAAW0b,MAAM,YACjDhW,EAAM5B,EAAK4C,EAAK8U,QAAU9U,GAAK6U,IAC1C,QAAQ5X,MAAO+B,EAAKgW,MAAM,KAG5B3a,EAAQA,EAAQmD,EAAG,WACjBmmB,UAAW,QAASA,WAAU3e,GAC5B,MAAO,IAAI0e,GAAU1e,OAMpB,SAASpL,EAAQD,EAASH,GAS/B,QAASkD,KAAIsI,EAAQse,GACnB,GACIG,GAAMpX,EADNuX,EAAWxjB,UAAU9C,OAAS,EAAI0H,EAAS5E,UAAU,EAEzD,OAAGtF,GAASkK,KAAY4e,EAAgB5e,EAAOse,IAC5CG,EAAOrpB,EAAEkC,QAAQ0I,EAAQse,IAAoB5oB,EAAI+oB,EAAM,SACtDA,EAAKxmB,MACLwmB,EAAK/mB,MAAQpD,EACXmqB,EAAK/mB,IAAI3C,KAAK6pB,GACdtqB,EACH0B,EAASqR,EAAQjS,EAAEiF,SAAS2F,IAAgBtI,IAAI2P,EAAOiX,EAAaM,GAAvE,OAfF,GAAIxpB,GAAWZ,EAAoB,GAC/BkB,EAAWlB,EAAoB,IAC/Ba,EAAWb,EAAoB,GAC/BwB,EAAWxB,EAAoB,IAC/BsB,EAAWtB,EAAoB,GAcnCa,GAAQA,EAAQmD,EAAG,WAAYd,IAAKA,OAI/B,SAAS9C,EAAQD,EAASH,GAG/B,GAAIY,GAAWZ,EAAoB,GAC/Ba,EAAWb,EAAoB,GAC/BsB,EAAWtB,EAAoB,GAEnCa,GAAQA,EAAQmD,EAAG,WACjBE,yBAA0B,QAASA,0BAAyBsH,EAAQse,GAClE,MAAOlpB,GAAEkC,QAAQxB,EAASkK,GAASse,OAMlC,SAAS1pB,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,GAC/B6F,EAAW7F,EAAoB,GAAG6F,SAClCvE,EAAWtB,EAAoB,GAEnCa,GAAQA,EAAQmD,EAAG,WACjB4B,eAAgB,QAASA,gBAAe4F,GACtC,MAAO3F,GAASvE,EAASkK,QAMxB,SAASpL,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,WACjB9C,IAAK,QAASA,KAAIsK,EAAQse,GACxB,MAAOA,KAAete,OAMrB,SAASpL,EAAQD,EAASH,GAG/B,GAAIa,GAAgBb,EAAoB,GACpCsB,EAAgBtB,EAAoB,IACpC+T,EAAgB5R,OAAO6R,YAE3BnT,GAAQA,EAAQmD,EAAG,WACjBgQ,aAAc,QAASA,cAAaxI,GAElC,MADAlK,GAASkK,GACFuI,EAAgBA,EAAcvI,IAAU,MAM9C,SAASpL,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,EAElCa,GAAQA,EAAQmD,EAAG,WAAYqmB,QAASrqB,EAAoB,QAIvD,SAASI,EAAQD,EAASH,GAG/B,GAAIY,GAAWZ,EAAoB,GAC/BsB,EAAWtB,EAAoB,IAC/B0pB,EAAW1pB,EAAoB,GAAG0pB,OACtCtpB,GAAOD,QAAUupB,GAAWA,EAAQW,SAAW,QAASA,SAAQ7d,GAC9D,GAAI5I,GAAahD,EAAEoF,SAAS1E,EAASkL,IACjCrC,EAAavJ,EAAEuJ,UACnB,OAAOA,GAAavG,EAAKU,OAAO6F,EAAWqC,IAAO5I,IAK/C,SAASxD,EAAQD,EAASH,GAG/B,GAAIa,GAAqBb,EAAoB,GACzCsB,EAAqBtB,EAAoB,IACzCyT,EAAqBtR,OAAOuR,iBAEhC7S,GAAQA,EAAQmD,EAAG,WACjB0P,kBAAmB,QAASA,mBAAkBlI,GAC5ClK,EAASkK,EACT,KAEE,MADGiI,IAAmBA,EAAmBjI,IAClC,EACP,MAAMjI,GACN,OAAO,OAOR,SAASnD,EAAQD,EAASH,GAU/B,QAASwQ,KAAIhF,EAAQse,EAAaQ,GAChC,GAEIC,GAAoB1X,EAFpBuX,EAAWxjB,UAAU9C,OAAS,EAAI0H,EAAS5E,UAAU,GACrD4jB,EAAW5pB,EAAEkC,QAAQxB,EAASkK,GAASse,EAE3C,KAAIU,EAAQ,CACV,GAAGhpB,EAASqR,EAAQjS,EAAEiF,SAAS2F,IAC7B,MAAOgF,KAAIqC,EAAOiX,EAAaQ,EAAGF,EAEpCI,GAAUzpB,EAAW,GAEvB,MAAGG,GAAIspB,EAAS,SACXA,EAAQve,YAAa,GAAUzK,EAAS4oB,IAC3CG,EAAqB3pB,EAAEkC,QAAQsnB,EAAUN,IAAgB/oB,EAAW,GACpEwpB,EAAmB9mB,MAAQ6mB,EAC3B1pB,EAAEgC,QAAQwnB,EAAUN,EAAaS,IAC1B,IAJqD,EAMvDC,EAAQha,MAAQ1Q,GAAY,GAAS0qB,EAAQha,IAAIjQ,KAAK6pB,EAAUE,IAAI,GAxB7E,GAAI1pB,GAAaZ,EAAoB,GACjCkB,EAAalB,EAAoB,IACjCa,EAAab,EAAoB,GACjCe,EAAaf,EAAoB,GACjCsB,EAAatB,EAAoB,IACjCwB,EAAaxB,EAAoB,GAsBrCa,GAAQA,EAAQmD,EAAG,WAAYwM,IAAKA,OAI/B,SAASpQ,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,GAC/BwgB,EAAWxgB,EAAoB,GAEhCwgB,IAAS3f,EAAQA,EAAQmD,EAAG,WAC7B2O,eAAgB,QAASA,gBAAenH,EAAQqH,GAC9C2N,EAAS5N,MAAMpH,EAAQqH,EACvB,KAEE,MADA2N,GAAShQ,IAAIhF,EAAQqH,IACd,EACP,MAAMtP,GACN,OAAO,OAOR,SAASnD,EAAQD,EAASH,GAG/B,GAAIa,GAAYb,EAAoB,GAChCyqB,EAAYzqB,EAAoB,KAAI,EAExCa,GAAQA,EAAQwC,EAAG,SAEjBwX,SAAU,QAASA,UAASnS,GAC1B,MAAO+hB,GAAU/jB,KAAMgC,EAAI9B,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,MAIrEE,EAAoB,KAAK,aAIpB,SAASI,EAAQD,EAASH,GAI/B,GAAIa,GAAUb,EAAoB,GAC9B+Z,EAAU/Z,EAAoB,KAAI,EAEtCa,GAAQA,EAAQwC,EAAG,UACjBqnB,GAAI,QAASA,IAAGzQ,GACd,MAAOF,GAAIrT,KAAMuT,OAMhB,SAAS7Z,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B2qB,EAAU3qB,EAAoB,IAElCa,GAAQA,EAAQwC,EAAG,UACjBunB,QAAS,QAASA,SAAQC,GACxB,MAAOF,GAAKjkB,KAAMmkB,EAAWjkB,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,GAAW,OAM7E,SAASM,EAAQD,EAASH,GAG/B,GAAI6B,GAAW7B,EAAoB,IAC/B8a,EAAW9a,EAAoB,KAC/BsN,EAAWtN,EAAoB,GAEnCI,GAAOD,QAAU,SAASqG,EAAMqkB,EAAWC,EAAYC,GACrD,GAAI/mB,GAAe4I,OAAOU,EAAQ9G,IAC9BwkB,EAAehnB,EAAEF,OACjBmnB,EAAeH,IAAehrB,EAAY,IAAM8M,OAAOke,GACvDI,EAAerpB,EAASgpB,EAC5B,IAAmBG,GAAhBE,EAA6B,MAAOlnB,EACzB,KAAXinB,IAAcA,EAAU,IAC3B,IAAIE,GAAUD,EAAeF,EACzBI,EAAetQ,EAAOva,KAAK0qB,EAASriB,KAAK2E,KAAK4d,EAAUF,EAAQnnB,QAEpE,OADGsnB,GAAatnB,OAASqnB,IAAQC,EAAeA,EAAa5oB,MAAM,EAAG2oB,IAC/DJ,EAAOK,EAAepnB,EAAIA,EAAIonB,IAKlC,SAAShrB,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B2qB,EAAU3qB,EAAoB,IAElCa,GAAQA,EAAQwC,EAAG,UACjBgoB,SAAU,QAASA,UAASR,GAC1B,MAAOF,GAAKjkB,KAAMmkB,EAAWjkB,UAAU9C,OAAS,EAAI8C,UAAU,GAAK9G,GAAW,OAM7E,SAASM,EAAQD,EAASH,GAI/BA,EAAoB,IAAI,WAAY,SAAS0U,GAC3C,MAAO,SAAS4W,YACd,MAAO5W,GAAMhO,KAAM,OAMlB,SAAStG,EAAQD,EAASH,GAI/BA,EAAoB,IAAI,YAAa,SAAS0U,GAC5C,MAAO,SAAS6W,aACd,MAAO7W,GAAMhO,KAAM,OAMlB,SAAStG,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9BwrB,EAAUxrB,EAAoB,KAAK,sBAAuB,OAE9Da,GAAQA,EAAQmD,EAAG,UAAWynB,OAAQ,QAASA,QAAOjf,GAAK,MAAOgf,GAAIhf,OAKjE,SAASpM,EAAQD,GAEtBC,EAAOD,QAAU,SAASurB,EAAQrV,GAChC,GAAIjF,GAAWiF,IAAYlU,OAAOkU,GAAW,SAASsV,GACpD,MAAOtV,GAAQsV,IACbtV,CACJ,OAAO,UAAS7J,GACd,MAAOI,QAAOJ,GAAI6J,QAAQqV,EAAQta,MAMjC,SAAShR,EAAQD,EAASH,GAG/B,GAAIY,GAAaZ,EAAoB,GACjCa,EAAab,EAAoB,GACjCqqB,EAAarqB,EAAoB,KACjC0B,EAAa1B,EAAoB,IACjCe,EAAaf,EAAoB,EAErCa,GAAQA,EAAQmD,EAAG,UACjB4nB,0BAA2B,QAASA,2BAA0BrmB,GAQ5D,IAPA,GAMIC,GAAK0K,EANL9M,EAAU1B,EAAU6D,GACpB3C,EAAUhC,EAAEgC,QACZE,EAAUlC,EAAEkC,QACZc,EAAUymB,EAAQjnB,GAClBqC,KACA1B,EAAU,EAERH,EAAKE,OAASC,GAClBmM,EAAIpN,EAAQM,EAAGoC,EAAM5B,EAAKG,MACvByB,IAAOC,GAAO7C,EAAQ6C,EAAQD,EAAKzE,EAAW,EAAGmP,IAC/CzK,EAAOD,GAAO0K,CACnB,OAAOzK,OAMR,SAASrF,EAAQD,EAASH,GAG/B,GAAIa,GAAUb,EAAoB,GAC9B6rB,EAAU7rB,EAAoB,MAAK,EAEvCa,GAAQA,EAAQmD,EAAG,UACjB0Y,OAAQ,QAASA,QAAOlQ,GACtB,MAAOqf,GAAQrf,OAMd,SAASpM,EAAQD,EAASH,GAE/B,GAAIY,GAAYZ,EAAoB,GAChC0B,EAAY1B,EAAoB,IAChCkK,EAAYtJ,EAAEsJ,MAClB9J,GAAOD,QAAU,SAAS2rB,GACxB,MAAO,UAAStf,GAOd,IANA,GAKIhH,GALApC,EAAS1B,EAAU8K,GACnB5I,EAAShD,EAAEiD,QAAQT,GACnBU,EAASF,EAAKE,OACdC,EAAS,EACT0B,KAEE3B,EAASC,GAAKmG,EAAO3J,KAAK6C,EAAGoC,EAAM5B,EAAKG,OAC5C0B,EAAOC,KAAKomB,GAAatmB,EAAKpC,EAAEoC,IAAQpC,EAAEoC,GAC1C,OAAOC,MAMR,SAASrF,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,GAC/B+rB,EAAW/rB,EAAoB,MAAK,EAExCa,GAAQA,EAAQmD,EAAG,UACjB2Y,QAAS,QAASA,SAAQnQ,GACxB,MAAOuf,GAASvf,OAMf,SAASpM,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,EAEnCa,GAAQA,EAAQwC,EAAG,OAAQ2oB,OAAQhsB,EAAoB,KAAK,UAIvD,SAASI,EAAQD,EAASH,GAG/B,GAAIugB,GAAUvgB,EAAoB,KAC9BiT,EAAUjT,EAAoB,GAClCI,GAAOD,QAAU,SAASkU,GACxB,MAAO,SAAS2X,UACd,GAAG/Y,EAAQvM,OAAS2N,EAAK,KAAM7Q,WAAU6Q,EAAO,wBAChD,IAAI4J,KAEJ,OADAsC,GAAM7Z,MAAM,EAAOuX,EAAIvY,KAAMuY,GACtBA,KAMN,SAAS7d,EAAQD,EAASH,GAG/B,GAAIa,GAAWb,EAAoB,EAEnCa,GAAQA,EAAQwC,EAAG,OAAQ2oB,OAAQhsB,EAAoB,KAAK,UAIvD,SAASI,EAAQD,EAASH,GAE/B,GAAIa,GAAUb,EAAoB,GAC9BisB,EAAUjsB,EAAoB,IAClCa,GAAQA,EAAQsK,EAAItK,EAAQ0K,GAC1Bsa,aAAgBoG,EAAMzb,IACtBuV,eAAgBkG,EAAMpF,SAKnB,SAASzmB,EAAQD,EAASH,GAE/BA,EAAoB,IACpB,IAAIqK,GAAcrK,EAAoB,GAClCuK,EAAcvK,EAAoB,GAClC0b,EAAc1b,EAAoB,KAClC4b,EAAc5b,EAAoB,IAAI,YACtCksB,EAAc7hB,EAAO8hB,SACrBC,EAAc/hB,EAAOgiB,eACrBC,EAAcJ,GAAMA,EAAG9pB,UACvBmqB,EAAcH,GAAOA,EAAIhqB,UACzBoqB,EAAc9Q,EAAUyQ,SAAWzQ,EAAU2Q,eAAiB3Q,EAAUpZ,KACzEgqB,KAAYA,EAAQ1Q,IAAUrR,EAAK+hB,EAAS1Q,EAAU4Q,GACtDD,IAAaA,EAAS3Q,IAAUrR,EAAKgiB,EAAU3Q,EAAU4Q,IAIvD,SAASpsB,EAAQD,EAASH,GAG/B,GAAIqK,GAAarK,EAAoB,GACjCa,EAAab,EAAoB,GACjCoB,EAAapB,EAAoB,IACjCysB,EAAazsB,EAAoB,KACjC0sB,EAAariB,EAAOqiB,UACpBC,IAAeD,GAAa,WAAW5Z,KAAK4Z,EAAUE,WACtDxc,EAAO,SAASI,GAClB,MAAOmc,GAAO,SAASlmB,EAAIomB,GACzB,MAAOrc,GAAIpP,EACTqrB,KACGjqB,MAAMjC,KAAKqG,UAAW,GACZ,kBAANH,GAAmBA,EAAKH,SAASG,IACvComB,IACDrc,EAEN3P,GAAQA,EAAQsK,EAAItK,EAAQ0K,EAAI1K,EAAQoD,EAAI0oB,GAC1C9J,WAAazS,EAAK/F,EAAOwY,YACzBiK,YAAa1c,EAAK/F,EAAOyiB,gBAKtB,SAAS1sB,EAAQD,EAASH,GAG/B,GAAI+sB,GAAY/sB,EAAoB,KAChCoB,EAAYpB,EAAoB,IAChCuB,EAAYvB,EAAoB,GACpCI,GAAOD,QAAU,WAOf,IANA,GAAIsG,GAASlF,EAAUmF,MACnB5C,EAAS8C,UAAU9C,OACnBkpB,EAAS1qB,MAAMwB,GACfC,EAAS,EACTkpB,EAASF,EAAKE,EACdC,GAAS,EACPppB,EAASC,IAAMipB,EAAMjpB,GAAK6C,UAAU7C,QAAUkpB,IAAEC,GAAS,EAC/D,OAAO,YACL,GAGkB9mB,GAHdI,EAAQE,KACR4K,EAAQ1K,UACR4L,EAAQlB,EAAGxN,OACX2O,EAAI,EAAGH,EAAI,CACf,KAAI4a,IAAW1a,EAAM,MAAOpR,GAAOqF,EAAIumB,EAAOxmB,EAE9C,IADAJ,EAAO4mB,EAAMxqB,QACV0qB,EAAO,KAAKppB,EAAS2O,EAAGA,IAAOrM,EAAKqM,KAAOwa,IAAE7mB,EAAKqM,GAAKnB,EAAGgB,KAC7D,MAAME,EAAQF,GAAElM,EAAKV,KAAK4L,EAAGgB,KAC7B,OAAOlR,GAAOqF,EAAIL,EAAMI,MAMvB,SAASpG,EAAQD,EAASH,GAE/BI,EAAOD,QAAUH,EAAoB,IAIhC,SAASI,EAAQD,EAASH,GAG/B,GAAIY,GAAUZ,EAAoB,GAC9Ba,EAAUb,EAAoB,GAC9BmtB,EAAUntB,EAAoB,IAC9BotB,EAAUptB,EAAoB,GAAGsC,OAASA,MAC1C+qB,KACAC,EAAa,SAAS1pB,EAAME,GAC9BlD,EAAEqH,KAAK1H,KAAKqD,EAAKQ,MAAM,KAAM,SAASoB,GACjC1B,GAAUhE,GAAa0F,IAAO4nB,GAAOC,EAAQ7nB,GAAO4nB,EAAO5nB,GACtDA,SAAU6nB,EAAQ7nB,GAAO2nB,EAAK7mB,SAAS/F,QAASiF,GAAM1B,MAGlEwpB,GAAW,wCAAyC,GACpDA,EAAW,gEAAiE,GAC5EA,EAAW,6FAEXzsB,EAAQA,EAAQmD,EAAG,QAASqpB,MAKT,mBAAVjtB,SAAyBA,OAAOD,QAAQC,OAAOD,QAAUP,EAE1C,kBAAV2tB,SAAwBA,OAAOC,IAAID,OAAO,WAAW,MAAO3tB,KAEtEC,EAAIyK,KAAO1K,GACd,EAAG","file":"shim.min.js"}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/_.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/_.js
new file mode 100644
index 00000000..475a66cd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/_.js
@@ -0,0 +1,2 @@
+require('../modules/core.function.part');
+module.exports = require('../modules/$.core')._;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/delay.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/delay.js
new file mode 100644
index 00000000..1ff9a56d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/delay.js
@@ -0,0 +1,2 @@
+require('../modules/core.delay');
+module.exports = require('../modules/$.core').delay;
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/dict.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/dict.js
new file mode 100644
index 00000000..ed848e2c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/dict.js
@@ -0,0 +1,2 @@
+require('../modules/core.dict');
+module.exports = require('../modules/$.core').Dict;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/function.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/function.js
new file mode 100644
index 00000000..42b6dbb8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/function.js
@@ -0,0 +1,2 @@
+require('../modules/core.function.part');
+module.exports = require('../modules/$.core').Function;
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/index.js
new file mode 100644
index 00000000..3d50bdb6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/index.js
@@ -0,0 +1,15 @@
+require('../modules/core.dict');
+require('../modules/core.get-iterator-method');
+require('../modules/core.get-iterator');
+require('../modules/core.is-iterable');
+require('../modules/core.delay');
+require('../modules/core.function.part');
+require('../modules/core.object.is-object');
+require('../modules/core.object.classof');
+require('../modules/core.object.define');
+require('../modules/core.object.make');
+require('../modules/core.number.iterator');
+require('../modules/core.string.escape-html');
+require('../modules/core.string.unescape-html');
+require('../modules/core.log');
+module.exports = require('../modules/$.core');
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/log.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/log.js
new file mode 100644
index 00000000..899d6ed0
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/log.js
@@ -0,0 +1,2 @@
+require('../modules/core.log');
+module.exports = require('../modules/$.core').log;
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/number.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/number.js
new file mode 100644
index 00000000..6e3cdbf6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/number.js
@@ -0,0 +1,2 @@
+require('../modules/core.number.iterator');
+module.exports = require('../modules/$.core').Number;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/object.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/object.js
new file mode 100644
index 00000000..63325663
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/object.js
@@ -0,0 +1,5 @@
+require('../modules/core.object.is-object');
+require('../modules/core.object.classof');
+require('../modules/core.object.define');
+require('../modules/core.object.make');
+module.exports = require('../modules/$.core').Object;
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/string.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/string.js
new file mode 100644
index 00000000..829e0c61
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/core/string.js
@@ -0,0 +1,3 @@
+require('../modules/core.string.escape-html');
+require('../modules/core.string.unescape-html');
+module.exports = require('../modules/$.core').String;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es5/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es5/index.js
new file mode 100644
index 00000000..3c23364a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es5/index.js
@@ -0,0 +1,9 @@
+require('../modules/es5');
+require('../modules/es6.object.freeze');
+require('../modules/es6.object.seal');
+require('../modules/es6.object.prevent-extensions');
+require('../modules/es6.object.is-frozen');
+require('../modules/es6.object.is-sealed');
+require('../modules/es6.object.is-extensible');
+require('../modules/es6.string.trim');
+module.exports = require('../modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/array.js
new file mode 100644
index 00000000..36629ca0
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/array.js
@@ -0,0 +1,10 @@
+require('../modules/es6.string.iterator');
+require('../modules/es6.array.from');
+require('../modules/es6.array.of');
+require('../modules/es6.array.species');
+require('../modules/es6.array.iterator');
+require('../modules/es6.array.copy-within');
+require('../modules/es6.array.fill');
+require('../modules/es6.array.find');
+require('../modules/es6.array.find-index');
+module.exports = require('../modules/$.core').Array;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/function.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/function.js
new file mode 100644
index 00000000..3e61dcd4
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/function.js
@@ -0,0 +1,3 @@
+require('../modules/es6.function.name');
+require('../modules/es6.function.has-instance');
+module.exports = require('../modules/$.core').Function;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/index.js
new file mode 100644
index 00000000..b9fc80be
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/index.js
@@ -0,0 +1,87 @@
+require('../modules/es6.symbol');
+require('../modules/es6.object.assign');
+require('../modules/es6.object.is');
+require('../modules/es6.object.set-prototype-of');
+require('../modules/es6.object.to-string');
+require('../modules/es6.object.freeze');
+require('../modules/es6.object.seal');
+require('../modules/es6.object.prevent-extensions');
+require('../modules/es6.object.is-frozen');
+require('../modules/es6.object.is-sealed');
+require('../modules/es6.object.is-extensible');
+require('../modules/es6.object.get-own-property-descriptor');
+require('../modules/es6.object.get-prototype-of');
+require('../modules/es6.object.keys');
+require('../modules/es6.object.get-own-property-names');
+require('../modules/es6.function.name');
+require('../modules/es6.function.has-instance');
+require('../modules/es6.number.constructor');
+require('../modules/es6.number.epsilon');
+require('../modules/es6.number.is-finite');
+require('../modules/es6.number.is-integer');
+require('../modules/es6.number.is-nan');
+require('../modules/es6.number.is-safe-integer');
+require('../modules/es6.number.max-safe-integer');
+require('../modules/es6.number.min-safe-integer');
+require('../modules/es6.number.parse-float');
+require('../modules/es6.number.parse-int');
+require('../modules/es6.math.acosh');
+require('../modules/es6.math.asinh');
+require('../modules/es6.math.atanh');
+require('../modules/es6.math.cbrt');
+require('../modules/es6.math.clz32');
+require('../modules/es6.math.cosh');
+require('../modules/es6.math.expm1');
+require('../modules/es6.math.fround');
+require('../modules/es6.math.hypot');
+require('../modules/es6.math.imul');
+require('../modules/es6.math.log10');
+require('../modules/es6.math.log1p');
+require('../modules/es6.math.log2');
+require('../modules/es6.math.sign');
+require('../modules/es6.math.sinh');
+require('../modules/es6.math.tanh');
+require('../modules/es6.math.trunc');
+require('../modules/es6.string.from-code-point');
+require('../modules/es6.string.raw');
+require('../modules/es6.string.trim');
+require('../modules/es6.string.iterator');
+require('../modules/es6.string.code-point-at');
+require('../modules/es6.string.ends-with');
+require('../modules/es6.string.includes');
+require('../modules/es6.string.repeat');
+require('../modules/es6.string.starts-with');
+require('../modules/es6.array.from');
+require('../modules/es6.array.of');
+require('../modules/es6.array.species');
+require('../modules/es6.array.iterator');
+require('../modules/es6.array.copy-within');
+require('../modules/es6.array.fill');
+require('../modules/es6.array.find');
+require('../modules/es6.array.find-index');
+require('../modules/es6.regexp.constructor');
+require('../modules/es6.regexp.flags');
+require('../modules/es6.regexp.match');
+require('../modules/es6.regexp.replace');
+require('../modules/es6.regexp.search');
+require('../modules/es6.regexp.split');
+require('../modules/es6.promise');
+require('../modules/es6.map');
+require('../modules/es6.set');
+require('../modules/es6.weak-map');
+require('../modules/es6.weak-set');
+require('../modules/es6.reflect.apply');
+require('../modules/es6.reflect.construct');
+require('../modules/es6.reflect.define-property');
+require('../modules/es6.reflect.delete-property');
+require('../modules/es6.reflect.enumerate');
+require('../modules/es6.reflect.get');
+require('../modules/es6.reflect.get-own-property-descriptor');
+require('../modules/es6.reflect.get-prototype-of');
+require('../modules/es6.reflect.has');
+require('../modules/es6.reflect.is-extensible');
+require('../modules/es6.reflect.own-keys');
+require('../modules/es6.reflect.prevent-extensions');
+require('../modules/es6.reflect.set');
+require('../modules/es6.reflect.set-prototype-of');
+module.exports = require('../modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/map.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/map.js
new file mode 100644
index 00000000..521a192e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/map.js
@@ -0,0 +1,5 @@
+require('../modules/es6.object.to-string');
+require('../modules/es6.string.iterator');
+require('../modules/web.dom.iterable');
+require('../modules/es6.map');
+module.exports = require('../modules/$.core').Map;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/math.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/math.js
new file mode 100644
index 00000000..8b5a2e79
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/math.js
@@ -0,0 +1,18 @@
+require('../modules/es6.math.acosh');
+require('../modules/es6.math.asinh');
+require('../modules/es6.math.atanh');
+require('../modules/es6.math.cbrt');
+require('../modules/es6.math.clz32');
+require('../modules/es6.math.cosh');
+require('../modules/es6.math.expm1');
+require('../modules/es6.math.fround');
+require('../modules/es6.math.hypot');
+require('../modules/es6.math.imul');
+require('../modules/es6.math.log10');
+require('../modules/es6.math.log1p');
+require('../modules/es6.math.log2');
+require('../modules/es6.math.sign');
+require('../modules/es6.math.sinh');
+require('../modules/es6.math.tanh');
+require('../modules/es6.math.trunc');
+module.exports = require('../modules/$.core').Math;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/number.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/number.js
new file mode 100644
index 00000000..8f487703
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/number.js
@@ -0,0 +1,11 @@
+require('../modules/es6.number.constructor');
+require('../modules/es6.number.epsilon');
+require('../modules/es6.number.is-finite');
+require('../modules/es6.number.is-integer');
+require('../modules/es6.number.is-nan');
+require('../modules/es6.number.is-safe-integer');
+require('../modules/es6.number.max-safe-integer');
+require('../modules/es6.number.min-safe-integer');
+require('../modules/es6.number.parse-float');
+require('../modules/es6.number.parse-int');
+module.exports = require('../modules/$.core').Number;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/object.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/object.js
new file mode 100644
index 00000000..94af189f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/object.js
@@ -0,0 +1,17 @@
+require('../modules/es6.symbol');
+require('../modules/es6.object.assign');
+require('../modules/es6.object.is');
+require('../modules/es6.object.set-prototype-of');
+require('../modules/es6.object.to-string');
+require('../modules/es6.object.freeze');
+require('../modules/es6.object.seal');
+require('../modules/es6.object.prevent-extensions');
+require('../modules/es6.object.is-frozen');
+require('../modules/es6.object.is-sealed');
+require('../modules/es6.object.is-extensible');
+require('../modules/es6.object.get-own-property-descriptor');
+require('../modules/es6.object.get-prototype-of');
+require('../modules/es6.object.keys');
+require('../modules/es6.object.get-own-property-names');
+
+module.exports = require('../modules/$.core').Object;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/promise.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/promise.js
new file mode 100644
index 00000000..0a099613
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/promise.js
@@ -0,0 +1,5 @@
+require('../modules/es6.object.to-string');
+require('../modules/es6.string.iterator');
+require('../modules/web.dom.iterable');
+require('../modules/es6.promise');
+module.exports = require('../modules/$.core').Promise;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/reflect.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/reflect.js
new file mode 100644
index 00000000..3c2f74eb
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/reflect.js
@@ -0,0 +1,15 @@
+require('../modules/es6.reflect.apply');
+require('../modules/es6.reflect.construct');
+require('../modules/es6.reflect.define-property');
+require('../modules/es6.reflect.delete-property');
+require('../modules/es6.reflect.enumerate');
+require('../modules/es6.reflect.get');
+require('../modules/es6.reflect.get-own-property-descriptor');
+require('../modules/es6.reflect.get-prototype-of');
+require('../modules/es6.reflect.has');
+require('../modules/es6.reflect.is-extensible');
+require('../modules/es6.reflect.own-keys');
+require('../modules/es6.reflect.prevent-extensions');
+require('../modules/es6.reflect.set');
+require('../modules/es6.reflect.set-prototype-of');
+module.exports = require('../modules/$.core').Reflect;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/regexp.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/regexp.js
new file mode 100644
index 00000000..195d36d4
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/regexp.js
@@ -0,0 +1,7 @@
+require('../modules/es6.regexp.constructor');
+require('../modules/es6.regexp.flags');
+require('../modules/es6.regexp.match');
+require('../modules/es6.regexp.replace');
+require('../modules/es6.regexp.search');
+require('../modules/es6.regexp.split');
+module.exports = require('../modules/$.core').RegExp;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/set.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/set.js
new file mode 100644
index 00000000..6a84f589
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/set.js
@@ -0,0 +1,5 @@
+require('../modules/es6.object.to-string');
+require('../modules/es6.string.iterator');
+require('../modules/web.dom.iterable');
+require('../modules/es6.set');
+module.exports = require('../modules/$.core').Set;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/string.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/string.js
new file mode 100644
index 00000000..bc7a1ab1
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/string.js
@@ -0,0 +1,14 @@
+require('../modules/es6.string.from-code-point');
+require('../modules/es6.string.raw');
+require('../modules/es6.string.trim');
+require('../modules/es6.string.iterator');
+require('../modules/es6.string.code-point-at');
+require('../modules/es6.string.ends-with');
+require('../modules/es6.string.includes');
+require('../modules/es6.string.repeat');
+require('../modules/es6.string.starts-with');
+require('../modules/es6.regexp.match');
+require('../modules/es6.regexp.replace');
+require('../modules/es6.regexp.search');
+require('../modules/es6.regexp.split');
+module.exports = require('../modules/$.core').String;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/symbol.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/symbol.js
new file mode 100644
index 00000000..ae2405b5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/symbol.js
@@ -0,0 +1,3 @@
+require('../modules/es6.symbol');
+require('../modules/es6.object.to-string');
+module.exports = require('../modules/$.core').Symbol;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/weak-map.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/weak-map.js
new file mode 100644
index 00000000..f2c6db89
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/weak-map.js
@@ -0,0 +1,4 @@
+require('../modules/es6.object.to-string');
+require('../modules/es6.array.iterator');
+require('../modules/es6.weak-map');
+module.exports = require('../modules/$.core').WeakMap;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/weak-set.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/weak-set.js
new file mode 100644
index 00000000..a058c8a6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es6/weak-set.js
@@ -0,0 +1,4 @@
+require('../modules/es6.object.to-string');
+require('../modules/web.dom.iterable');
+require('../modules/es6.weak-set');
+module.exports = require('../modules/$.core').WeakSet;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/array.js
new file mode 100644
index 00000000..e58f9057
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/array.js
@@ -0,0 +1,2 @@
+require('../modules/es7.array.includes');
+module.exports = require('../modules/$.core').Array;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/index.js
new file mode 100644
index 00000000..a277b639
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/index.js
@@ -0,0 +1,13 @@
+require('../modules/es7.array.includes');
+require('../modules/es7.string.at');
+require('../modules/es7.string.pad-left');
+require('../modules/es7.string.pad-right');
+require('../modules/es7.string.trim-left');
+require('../modules/es7.string.trim-right');
+require('../modules/es7.regexp.escape');
+require('../modules/es7.object.get-own-property-descriptors');
+require('../modules/es7.object.values');
+require('../modules/es7.object.entries');
+require('../modules/es7.map.to-json');
+require('../modules/es7.set.to-json');
+module.exports = require('../modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/map.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/map.js
new file mode 100644
index 00000000..fe519992
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/map.js
@@ -0,0 +1,2 @@
+require('../modules/es7.map.to-json');
+module.exports = require('../modules/$.core').Map;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/object.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/object.js
new file mode 100644
index 00000000..b0585303
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/object.js
@@ -0,0 +1,4 @@
+require('../modules/es7.object.get-own-property-descriptors');
+require('../modules/es7.object.values');
+require('../modules/es7.object.entries');
+module.exports = require('../modules/$.core').Object;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/regexp.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/regexp.js
new file mode 100644
index 00000000..cb24f98b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/regexp.js
@@ -0,0 +1,2 @@
+require('../modules/es7.regexp.escape');
+module.exports = require('../modules/$.core').RegExp;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/set.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/set.js
new file mode 100644
index 00000000..16e94528
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/set.js
@@ -0,0 +1,2 @@
+require('../modules/es7.set.to-json');
+module.exports = require('../modules/$.core').Set;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/string.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/string.js
new file mode 100644
index 00000000..bca0886c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/es7/string.js
@@ -0,0 +1,6 @@
+require('../modules/es7.string.at');
+require('../modules/es7.string.pad-left');
+require('../modules/es7.string.pad-right');
+require('../modules/es7.string.trim-left');
+require('../modules/es7.string.trim-right');
+module.exports = require('../modules/$.core').String;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/_.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/_.js
new file mode 100644
index 00000000..475a66cd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/_.js
@@ -0,0 +1,2 @@
+require('../modules/core.function.part');
+module.exports = require('../modules/$.core')._;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/concat.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/concat.js
new file mode 100644
index 00000000..176ecffe
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/concat.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.concat;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/copy-within.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/copy-within.js
new file mode 100644
index 00000000..8a011319
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/copy-within.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.array.copy-within');
+module.exports = require('../../modules/$.core').Array.copyWithin;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/entries.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/entries.js
new file mode 100644
index 00000000..bcdbc33f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/entries.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.array.iterator');
+module.exports = require('../../modules/$.core').Array.entries;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/every.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/every.js
new file mode 100644
index 00000000..0c7d0b7e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/every.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.every;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/fill.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/fill.js
new file mode 100644
index 00000000..f5362120
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/fill.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.array.fill');
+module.exports = require('../../modules/$.core').Array.fill;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/filter.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/filter.js
new file mode 100644
index 00000000..3f5b17ff
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/filter.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.filter;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/find-index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/find-index.js
new file mode 100644
index 00000000..7ec6cf7a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/find-index.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.array.find-index');
+module.exports = require('../../modules/$.core').Array.findIndex;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/find.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/find.js
new file mode 100644
index 00000000..9c3a6b31
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/find.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.array.find');
+module.exports = require('../../modules/$.core').Array.find;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/for-each.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/for-each.js
new file mode 100644
index 00000000..b2e79f0b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/for-each.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.forEach;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/from.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/from.js
new file mode 100644
index 00000000..f0483ccf
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/from.js
@@ -0,0 +1,3 @@
+require('../../modules/es6.string.iterator');
+require('../../modules/es6.array.from');
+module.exports = require('../../modules/$.core').Array.from;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/includes.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/includes.js
new file mode 100644
index 00000000..420c8318
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/includes.js
@@ -0,0 +1,2 @@
+require('../../modules/es7.array.includes');
+module.exports = require('../../modules/$.core').Array.includes;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/index-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/index-of.js
new file mode 100644
index 00000000..9f2cd14b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/index-of.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.indexOf;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/index.js
new file mode 100644
index 00000000..2707be21
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/index.js
@@ -0,0 +1,12 @@
+require('../../modules/es6.string.iterator');
+require('../../modules/es6.array.from');
+require('../../modules/es6.array.of');
+require('../../modules/es6.array.species');
+require('../../modules/es6.array.iterator');
+require('../../modules/es6.array.copy-within');
+require('../../modules/es6.array.fill');
+require('../../modules/es6.array.find');
+require('../../modules/es6.array.find-index');
+require('../../modules/es7.array.includes');
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/iterator.js
new file mode 100644
index 00000000..662f3b5c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/iterator.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.array.iterator');
+module.exports = require('../../modules/$.core').Array.values;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/join.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/join.js
new file mode 100644
index 00000000..44363922
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/join.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.join;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/keys.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/keys.js
new file mode 100644
index 00000000..e55d356e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/keys.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.array.iterator');
+module.exports = require('../../modules/$.core').Array.keys;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/last-index-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/last-index-of.js
new file mode 100644
index 00000000..678d0072
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/last-index-of.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.lastIndexOf;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/map.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/map.js
new file mode 100644
index 00000000..a1457c7a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/map.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.map;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/of.js
new file mode 100644
index 00000000..07bb5a4a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/of.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.array.of');
+module.exports = require('../../modules/$.core').Array.of;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/pop.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/pop.js
new file mode 100644
index 00000000..bd8f8610
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/pop.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.pop;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/push.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/push.js
new file mode 100644
index 00000000..3ccf0707
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/push.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.push;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/reduce-right.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/reduce-right.js
new file mode 100644
index 00000000..c592207c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/reduce-right.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.reduceRight;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/reduce.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/reduce.js
new file mode 100644
index 00000000..b8368406
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/reduce.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.reduce;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/reverse.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/reverse.js
new file mode 100644
index 00000000..4d8d235c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/reverse.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.reverse;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/shift.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/shift.js
new file mode 100644
index 00000000..806c87cd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/shift.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.shift;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/slice.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/slice.js
new file mode 100644
index 00000000..913f7ef2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/slice.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.slice;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/some.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/some.js
new file mode 100644
index 00000000..4f7c7654
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/some.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.some;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/sort.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/sort.js
new file mode 100644
index 00000000..61beed04
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/sort.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.sort;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/splice.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/splice.js
new file mode 100644
index 00000000..5f5eab07
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/splice.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.splice;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/unshift.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/unshift.js
new file mode 100644
index 00000000..a11de528
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/unshift.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.unshift;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/values.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/values.js
new file mode 100644
index 00000000..662f3b5c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/array/values.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.array.iterator');
+module.exports = require('../../modules/$.core').Array.values;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/clear-immediate.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/clear-immediate.js
new file mode 100644
index 00000000..06a97502
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/clear-immediate.js
@@ -0,0 +1,2 @@
+require('../modules/web.immediate');
+module.exports = require('../modules/$.core').clearImmediate;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/delay.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/delay.js
new file mode 100644
index 00000000..1ff9a56d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/delay.js
@@ -0,0 +1,2 @@
+require('../modules/core.delay');
+module.exports = require('../modules/$.core').delay;
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/dict.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/dict.js
new file mode 100644
index 00000000..ed848e2c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/dict.js
@@ -0,0 +1,2 @@
+require('../modules/core.dict');
+module.exports = require('../modules/$.core').Dict;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/function/has-instance.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/function/has-instance.js
new file mode 100644
index 00000000..78c8221c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/function/has-instance.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.function.has-instance');
+module.exports = Function[require('../../modules/$.wks')('hasInstance')];
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/function/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/function/index.js
new file mode 100644
index 00000000..7422fca3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/function/index.js
@@ -0,0 +1,4 @@
+require('../../modules/es6.function.name');
+require('../../modules/es6.function.has-instance');
+require('../../modules/core.function.part');
+module.exports = require('../../modules/$.core').Function;
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/function/name.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/function/name.js
new file mode 100644
index 00000000..cb70bf15
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/function/name.js
@@ -0,0 +1 @@
+require('../../modules/es6.function.name');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/function/part.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/function/part.js
new file mode 100644
index 00000000..26271d6a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/function/part.js
@@ -0,0 +1,2 @@
+require('../../modules/core.function.part');
+module.exports = require('../../modules/$.core').Function.part;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/get-iterator-method.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/get-iterator-method.js
new file mode 100644
index 00000000..5543cbbf
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/get-iterator-method.js
@@ -0,0 +1,3 @@
+require('../modules/web.dom.iterable');
+require('../modules/es6.string.iterator');
+module.exports = require('../modules/core.get-iterator-method');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/get-iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/get-iterator.js
new file mode 100644
index 00000000..762350ff
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/get-iterator.js
@@ -0,0 +1,3 @@
+require('../modules/web.dom.iterable');
+require('../modules/es6.string.iterator');
+module.exports = require('../modules/core.get-iterator');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/html-collection/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/html-collection/index.js
new file mode 100644
index 00000000..510156b8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/html-collection/index.js
@@ -0,0 +1,2 @@
+require('../../modules/web.dom.iterable');
+module.exports = require('../../modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/html-collection/iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/html-collection/iterator.js
new file mode 100644
index 00000000..c01119b1
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/html-collection/iterator.js
@@ -0,0 +1,2 @@
+require('../../modules/web.dom.iterable');
+module.exports = require('../../modules/$.core').Array.values;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/is-iterable.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/is-iterable.js
new file mode 100644
index 00000000..4c654e87
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/is-iterable.js
@@ -0,0 +1,3 @@
+require('../modules/web.dom.iterable');
+require('../modules/es6.string.iterator');
+module.exports = require('../modules/core.is-iterable');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/json/stringify.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/json/stringify.js
new file mode 100644
index 00000000..fef24250
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/json/stringify.js
@@ -0,0 +1,4 @@
+var core = require('../../modules/$.core');
+module.exports = function stringify(it){ // eslint-disable-line no-unused-vars
+ return (core.JSON && core.JSON.stringify || JSON.stringify).apply(JSON, arguments);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/log.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/log.js
new file mode 100644
index 00000000..899d6ed0
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/log.js
@@ -0,0 +1,2 @@
+require('../modules/core.log');
+module.exports = require('../modules/$.core').log;
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/map.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/map.js
new file mode 100644
index 00000000..70998924
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/map.js
@@ -0,0 +1,6 @@
+require('../modules/es6.object.to-string');
+require('../modules/es6.string.iterator');
+require('../modules/web.dom.iterable');
+require('../modules/es6.map');
+require('../modules/es7.map.to-json');
+module.exports = require('../modules/$.core').Map;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/acosh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/acosh.js
new file mode 100644
index 00000000..d29a88cc
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/acosh.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.acosh');
+module.exports = require('../../modules/$.core').Math.acosh;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/asinh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/asinh.js
new file mode 100644
index 00000000..7eac2e83
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/asinh.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.asinh');
+module.exports = require('../../modules/$.core').Math.asinh;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/atanh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/atanh.js
new file mode 100644
index 00000000..a66a47d8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/atanh.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.atanh');
+module.exports = require('../../modules/$.core').Math.atanh;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/cbrt.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/cbrt.js
new file mode 100644
index 00000000..199f5cd8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/cbrt.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.cbrt');
+module.exports = require('../../modules/$.core').Math.cbrt;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/clz32.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/clz32.js
new file mode 100644
index 00000000..2025c6e4
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/clz32.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.clz32');
+module.exports = require('../../modules/$.core').Math.clz32;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/cosh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/cosh.js
new file mode 100644
index 00000000..17a7ddc0
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/cosh.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.cosh');
+module.exports = require('../../modules/$.core').Math.cosh;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/expm1.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/expm1.js
new file mode 100644
index 00000000..732facb3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/expm1.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.expm1');
+module.exports = require('../../modules/$.core').Math.expm1;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/fround.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/fround.js
new file mode 100644
index 00000000..37f87069
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/fround.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.fround');
+module.exports = require('../../modules/$.core').Math.fround;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/hypot.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/hypot.js
new file mode 100644
index 00000000..9676c073
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/hypot.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.hypot');
+module.exports = require('../../modules/$.core').Math.hypot;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/imul.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/imul.js
new file mode 100644
index 00000000..2ea2913e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/imul.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.imul');
+module.exports = require('../../modules/$.core').Math.imul;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/index.js
new file mode 100644
index 00000000..14628ae5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/index.js
@@ -0,0 +1,18 @@
+require('../../modules/es6.math.acosh');
+require('../../modules/es6.math.asinh');
+require('../../modules/es6.math.atanh');
+require('../../modules/es6.math.cbrt');
+require('../../modules/es6.math.clz32');
+require('../../modules/es6.math.cosh');
+require('../../modules/es6.math.expm1');
+require('../../modules/es6.math.fround');
+require('../../modules/es6.math.hypot');
+require('../../modules/es6.math.imul');
+require('../../modules/es6.math.log10');
+require('../../modules/es6.math.log1p');
+require('../../modules/es6.math.log2');
+require('../../modules/es6.math.sign');
+require('../../modules/es6.math.sinh');
+require('../../modules/es6.math.tanh');
+require('../../modules/es6.math.trunc');
+module.exports = require('../../modules/$.core').Math;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/log10.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/log10.js
new file mode 100644
index 00000000..ecf7b9b2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/log10.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.log10');
+module.exports = require('../../modules/$.core').Math.log10;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/log1p.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/log1p.js
new file mode 100644
index 00000000..6db73292
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/log1p.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.log1p');
+module.exports = require('../../modules/$.core').Math.log1p;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/log2.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/log2.js
new file mode 100644
index 00000000..63c74d7b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/log2.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.log2');
+module.exports = require('../../modules/$.core').Math.log2;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/sign.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/sign.js
new file mode 100644
index 00000000..47ab74f2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/sign.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.sign');
+module.exports = require('../../modules/$.core').Math.sign;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/sinh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/sinh.js
new file mode 100644
index 00000000..72c6e857
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/sinh.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.sinh');
+module.exports = require('../../modules/$.core').Math.sinh;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/tanh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/tanh.js
new file mode 100644
index 00000000..30ddbcc8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/tanh.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.tanh');
+module.exports = require('../../modules/$.core').Math.tanh;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/trunc.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/trunc.js
new file mode 100644
index 00000000..b084efa7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/math/trunc.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.trunc');
+module.exports = require('../../modules/$.core').Math.trunc;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/node-list/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/node-list/index.js
new file mode 100644
index 00000000..510156b8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/node-list/index.js
@@ -0,0 +1,2 @@
+require('../../modules/web.dom.iterable');
+module.exports = require('../../modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/node-list/iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/node-list/iterator.js
new file mode 100644
index 00000000..c01119b1
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/node-list/iterator.js
@@ -0,0 +1,2 @@
+require('../../modules/web.dom.iterable');
+module.exports = require('../../modules/$.core').Array.values;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/epsilon.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/epsilon.js
new file mode 100644
index 00000000..56c93521
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/epsilon.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.number.epsilon');
+module.exports = Math.pow(2, -52);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/index.js
new file mode 100644
index 00000000..d048d590
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/index.js
@@ -0,0 +1,12 @@
+require('../../modules/es6.number.constructor');
+require('../../modules/es6.number.epsilon');
+require('../../modules/es6.number.is-finite');
+require('../../modules/es6.number.is-integer');
+require('../../modules/es6.number.is-nan');
+require('../../modules/es6.number.is-safe-integer');
+require('../../modules/es6.number.max-safe-integer');
+require('../../modules/es6.number.min-safe-integer');
+require('../../modules/es6.number.parse-float');
+require('../../modules/es6.number.parse-int');
+require('../../modules/core.number.iterator');
+module.exports = require('../../modules/$.core').Number;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/is-finite.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/is-finite.js
new file mode 100644
index 00000000..ff666503
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/is-finite.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.number.is-finite');
+module.exports = require('../../modules/$.core').Number.isFinite;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/is-integer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/is-integer.js
new file mode 100644
index 00000000..682e1e34
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/is-integer.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.number.is-integer');
+module.exports = require('../../modules/$.core').Number.isInteger;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/is-nan.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/is-nan.js
new file mode 100644
index 00000000..6ad6923b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/is-nan.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.number.is-nan');
+module.exports = require('../../modules/$.core').Number.isNaN;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/is-safe-integer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/is-safe-integer.js
new file mode 100644
index 00000000..a47fd42e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/is-safe-integer.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.number.is-safe-integer');
+module.exports = require('../../modules/$.core').Number.isSafeInteger;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/iterator.js
new file mode 100644
index 00000000..57cb7906
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/iterator.js
@@ -0,0 +1,5 @@
+require('../../modules/core.number.iterator');
+var get = require('../../modules/$.iterators').Number;
+module.exports = function(it){
+ return get.call(it);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/max-safe-integer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/max-safe-integer.js
new file mode 100644
index 00000000..c9b43b04
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/max-safe-integer.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.number.max-safe-integer');
+module.exports = 0x1fffffffffffff;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/min-safe-integer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/min-safe-integer.js
new file mode 100644
index 00000000..8b5e0728
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/min-safe-integer.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.number.min-safe-integer');
+module.exports = -0x1fffffffffffff;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/parse-float.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/parse-float.js
new file mode 100644
index 00000000..62f89774
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/parse-float.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.number.parse-float');
+module.exports = parseFloat;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/parse-int.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/parse-int.js
new file mode 100644
index 00000000..c197da5b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/number/parse-int.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.number.parse-int');
+module.exports = parseInt;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/assign.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/assign.js
new file mode 100644
index 00000000..a57c54aa
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/assign.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.assign');
+module.exports = require('../../modules/$.core').Object.assign;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/classof.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/classof.js
new file mode 100644
index 00000000..3afc8268
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/classof.js
@@ -0,0 +1,2 @@
+require('../../modules/core.object.classof');
+module.exports = require('../../modules/$.core').Object.classof;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/create.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/create.js
new file mode 100644
index 00000000..8f5f3263
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/create.js
@@ -0,0 +1,4 @@
+var $ = require('../../modules/$');
+module.exports = function create(P, D){
+ return $.create(P, D);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/define-properties.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/define-properties.js
new file mode 100644
index 00000000..a857aab8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/define-properties.js
@@ -0,0 +1,4 @@
+var $ = require('../../modules/$');
+module.exports = function defineProperties(T, D){
+ return $.setDescs(T, D);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/define-property.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/define-property.js
new file mode 100644
index 00000000..7384315d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/define-property.js
@@ -0,0 +1,4 @@
+var $ = require('../../modules/$');
+module.exports = function defineProperty(it, key, desc){
+ return $.setDesc(it, key, desc);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/define.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/define.js
new file mode 100644
index 00000000..690773e2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/define.js
@@ -0,0 +1,2 @@
+require('../../modules/core.object.define');
+module.exports = require('../../modules/$.core').Object.define;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/entries.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/entries.js
new file mode 100644
index 00000000..a32fe391
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/entries.js
@@ -0,0 +1,2 @@
+require('../../modules/es7.object.entries');
+module.exports = require('../../modules/$.core').Object.entries;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/freeze.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/freeze.js
new file mode 100644
index 00000000..566eec51
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/freeze.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.freeze');
+module.exports = require('../../modules/$.core').Object.freeze;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/get-own-property-descriptor.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/get-own-property-descriptor.js
new file mode 100644
index 00000000..2e1845cf
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/get-own-property-descriptor.js
@@ -0,0 +1,5 @@
+var $ = require('../../modules/$');
+require('../../modules/es6.object.get-own-property-descriptor');
+module.exports = function getOwnPropertyDescriptor(it, key){
+ return $.getDesc(it, key);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/get-own-property-descriptors.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/get-own-property-descriptors.js
new file mode 100644
index 00000000..f26341d5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/get-own-property-descriptors.js
@@ -0,0 +1,2 @@
+require('../../modules/es7.object.get-own-property-descriptors');
+module.exports = require('../../modules/$.core').Object.getOwnPropertyDescriptors;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/get-own-property-names.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/get-own-property-names.js
new file mode 100644
index 00000000..496eb197
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/get-own-property-names.js
@@ -0,0 +1,5 @@
+var $ = require('../../modules/$');
+require('../../modules/es6.object.get-own-property-names');
+module.exports = function getOwnPropertyNames(it){
+ return $.getNames(it);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/get-own-property-symbols.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/get-own-property-symbols.js
new file mode 100644
index 00000000..f78921b5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/get-own-property-symbols.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.symbol');
+module.exports = require('../../modules/$.core').Object.getOwnPropertySymbols;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/get-prototype-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/get-prototype-of.js
new file mode 100644
index 00000000..9a495afe
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/get-prototype-of.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.get-prototype-of');
+module.exports = require('../../modules/$.core').Object.getPrototypeOf;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/index.js
new file mode 100644
index 00000000..0fb75bc5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/index.js
@@ -0,0 +1,23 @@
+require('../../modules/es6.symbol');
+require('../../modules/es6.object.assign');
+require('../../modules/es6.object.is');
+require('../../modules/es6.object.set-prototype-of');
+require('../../modules/es6.object.to-string');
+require('../../modules/es6.object.freeze');
+require('../../modules/es6.object.seal');
+require('../../modules/es6.object.prevent-extensions');
+require('../../modules/es6.object.is-frozen');
+require('../../modules/es6.object.is-sealed');
+require('../../modules/es6.object.is-extensible');
+require('../../modules/es6.object.get-own-property-descriptor');
+require('../../modules/es6.object.get-prototype-of');
+require('../../modules/es6.object.keys');
+require('../../modules/es6.object.get-own-property-names');
+require('../../modules/es7.object.get-own-property-descriptors');
+require('../../modules/es7.object.values');
+require('../../modules/es7.object.entries');
+require('../../modules/core.object.is-object');
+require('../../modules/core.object.classof');
+require('../../modules/core.object.define');
+require('../../modules/core.object.make');
+module.exports = require('../../modules/$.core').Object;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/is-extensible.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/is-extensible.js
new file mode 100644
index 00000000..8bb0cf9f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/is-extensible.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.is-extensible');
+module.exports = require('../../modules/$.core').Object.isExtensible;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/is-frozen.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/is-frozen.js
new file mode 100644
index 00000000..7bf1f127
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/is-frozen.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.is-frozen');
+module.exports = require('../../modules/$.core').Object.isFrozen;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/is-object.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/is-object.js
new file mode 100644
index 00000000..935cb6ec
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/is-object.js
@@ -0,0 +1,2 @@
+require('../../modules/core.object.is-object');
+module.exports = require('../../modules/$.core').Object.isObject;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/is-sealed.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/is-sealed.js
new file mode 100644
index 00000000..05416f37
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/is-sealed.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.is-sealed');
+module.exports = require('../../modules/$.core').Object.isSealed;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/is.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/is.js
new file mode 100644
index 00000000..d07c3ae1
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/is.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.is');
+module.exports = require('../../modules/$.core').Object.is;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/keys.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/keys.js
new file mode 100644
index 00000000..ebfb8c65
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/keys.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.keys');
+module.exports = require('../../modules/$.core').Object.keys;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/make.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/make.js
new file mode 100644
index 00000000..fbfb2f85
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/make.js
@@ -0,0 +1,2 @@
+require('../../modules/core.object.make');
+module.exports = require('../../modules/$.core').Object.make;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/prevent-extensions.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/prevent-extensions.js
new file mode 100644
index 00000000..01d82fcb
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/prevent-extensions.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.prevent-extensions');
+module.exports = require('../../modules/$.core').Object.preventExtensions;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/seal.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/seal.js
new file mode 100644
index 00000000..fdf84b82
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/seal.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.seal');
+module.exports = require('../../modules/$.core').Object.seal;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/set-prototype-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/set-prototype-of.js
new file mode 100644
index 00000000..cd94d875
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/set-prototype-of.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.set-prototype-of');
+module.exports = require('../../modules/$.core').Object.setPrototypeOf;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/values.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/values.js
new file mode 100644
index 00000000..b96071ff
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/object/values.js
@@ -0,0 +1,2 @@
+require('../../modules/es7.object.values');
+module.exports = require('../../modules/$.core').Object.values;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/promise.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/promise.js
new file mode 100644
index 00000000..0a099613
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/promise.js
@@ -0,0 +1,5 @@
+require('../modules/es6.object.to-string');
+require('../modules/es6.string.iterator');
+require('../modules/web.dom.iterable');
+require('../modules/es6.promise');
+module.exports = require('../modules/$.core').Promise;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/apply.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/apply.js
new file mode 100644
index 00000000..75e5ff5b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/apply.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.apply');
+module.exports = require('../../modules/$.core').Reflect.apply;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/construct.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/construct.js
new file mode 100644
index 00000000..adc40ea8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/construct.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.construct');
+module.exports = require('../../modules/$.core').Reflect.construct;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/define-property.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/define-property.js
new file mode 100644
index 00000000..da78d62e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/define-property.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.define-property');
+module.exports = require('../../modules/$.core').Reflect.defineProperty;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/delete-property.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/delete-property.js
new file mode 100644
index 00000000..411225f6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/delete-property.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.delete-property');
+module.exports = require('../../modules/$.core').Reflect.deleteProperty;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/enumerate.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/enumerate.js
new file mode 100644
index 00000000..c19e0b6b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/enumerate.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.enumerate');
+module.exports = require('../../modules/$.core').Reflect.enumerate;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/get-own-property-descriptor.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/get-own-property-descriptor.js
new file mode 100644
index 00000000..22e2aa66
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/get-own-property-descriptor.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.get-own-property-descriptor');
+module.exports = require('../../modules/$.core').Reflect.getOwnPropertyDescriptor;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/get-prototype-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/get-prototype-of.js
new file mode 100644
index 00000000..2ff331a4
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/get-prototype-of.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.get-prototype-of');
+module.exports = require('../../modules/$.core').Reflect.getPrototypeOf;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/get.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/get.js
new file mode 100644
index 00000000..266508c6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/get.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.get');
+module.exports = require('../../modules/$.core').Reflect.get;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/has.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/has.js
new file mode 100644
index 00000000..db14fa11
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/has.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.has');
+module.exports = require('../../modules/$.core').Reflect.has;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/index.js
new file mode 100644
index 00000000..5b216653
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/index.js
@@ -0,0 +1,15 @@
+require('../../modules/es6.reflect.apply');
+require('../../modules/es6.reflect.construct');
+require('../../modules/es6.reflect.define-property');
+require('../../modules/es6.reflect.delete-property');
+require('../../modules/es6.reflect.enumerate');
+require('../../modules/es6.reflect.get');
+require('../../modules/es6.reflect.get-own-property-descriptor');
+require('../../modules/es6.reflect.get-prototype-of');
+require('../../modules/es6.reflect.has');
+require('../../modules/es6.reflect.is-extensible');
+require('../../modules/es6.reflect.own-keys');
+require('../../modules/es6.reflect.prevent-extensions');
+require('../../modules/es6.reflect.set');
+require('../../modules/es6.reflect.set-prototype-of');
+module.exports = require('../../modules/$.core').Reflect;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/is-extensible.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/is-extensible.js
new file mode 100644
index 00000000..f0329e28
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/is-extensible.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.is-extensible');
+module.exports = require('../../modules/$.core').Reflect.isExtensible;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/own-keys.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/own-keys.js
new file mode 100644
index 00000000..6da1136d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/own-keys.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.own-keys');
+module.exports = require('../../modules/$.core').Reflect.ownKeys;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/prevent-extensions.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/prevent-extensions.js
new file mode 100644
index 00000000..48fb5d5a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/prevent-extensions.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.prevent-extensions');
+module.exports = require('../../modules/$.core').Reflect.preventExtensions;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/set-prototype-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/set-prototype-of.js
new file mode 100644
index 00000000..09cddebc
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/set-prototype-of.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.set-prototype-of');
+module.exports = require('../../modules/$.core').Reflect.setPrototypeOf;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/set.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/set.js
new file mode 100644
index 00000000..d1afec9c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/reflect/set.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.set');
+module.exports = require('../../modules/$.core').Reflect.set;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/regexp/escape.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/regexp/escape.js
new file mode 100644
index 00000000..0c8d06b4
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/regexp/escape.js
@@ -0,0 +1,2 @@
+require('../../modules/es7.regexp.escape');
+module.exports = require('../../modules/$.core').RegExp.escape;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/regexp/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/regexp/index.js
new file mode 100644
index 00000000..7d905d67
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/regexp/index.js
@@ -0,0 +1,8 @@
+require('../../modules/es6.regexp.constructor');
+require('../../modules/es6.regexp.flags');
+require('../../modules/es6.regexp.match');
+require('../../modules/es6.regexp.replace');
+require('../../modules/es6.regexp.search');
+require('../../modules/es6.regexp.split');
+require('../../modules/es7.regexp.escape');
+module.exports = require('../../modules/$.core').RegExp;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/set-immediate.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/set-immediate.js
new file mode 100644
index 00000000..2dd87df2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/set-immediate.js
@@ -0,0 +1,2 @@
+require('../modules/web.immediate');
+module.exports = require('../modules/$.core').setImmediate;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/set-interval.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/set-interval.js
new file mode 100644
index 00000000..4c7dd8e9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/set-interval.js
@@ -0,0 +1,2 @@
+require('../modules/web.timers');
+module.exports = require('../modules/$.core').setInterval;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/set-timeout.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/set-timeout.js
new file mode 100644
index 00000000..4e786194
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/set-timeout.js
@@ -0,0 +1,2 @@
+require('../modules/web.timers');
+module.exports = require('../modules/$.core').setTimeout;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/set.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/set.js
new file mode 100644
index 00000000..34615f1d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/set.js
@@ -0,0 +1,6 @@
+require('../modules/es6.object.to-string');
+require('../modules/es6.string.iterator');
+require('../modules/web.dom.iterable');
+require('../modules/es6.set');
+require('../modules/es7.set.to-json');
+module.exports = require('../modules/$.core').Set;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/at.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/at.js
new file mode 100644
index 00000000..d59d2aff
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/at.js
@@ -0,0 +1,2 @@
+require('../../modules/es7.string.at');
+module.exports = require('../../modules/$.core').String.at;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/code-point-at.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/code-point-at.js
new file mode 100644
index 00000000..74e933aa
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/code-point-at.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.string.code-point-at');
+module.exports = require('../../modules/$.core').String.codePointAt;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/ends-with.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/ends-with.js
new file mode 100644
index 00000000..7fe5cb74
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/ends-with.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.string.ends-with');
+module.exports = require('../../modules/$.core').String.endsWith;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/escape-html.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/escape-html.js
new file mode 100644
index 00000000..a6c62faf
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/escape-html.js
@@ -0,0 +1,2 @@
+require('../../modules/core.string.escape-html');
+module.exports = require('../../modules/$.core').String.escapeHTML;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/from-code-point.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/from-code-point.js
new file mode 100644
index 00000000..0b42e7ae
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/from-code-point.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.string.from-code-point');
+module.exports = require('../../modules/$.core').String.fromCodePoint;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/includes.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/includes.js
new file mode 100644
index 00000000..441bc594
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/includes.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.string.includes');
+module.exports = require('../../modules/$.core').String.includes;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/index.js
new file mode 100644
index 00000000..6be228ca
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/index.js
@@ -0,0 +1,21 @@
+require('../../modules/es6.string.from-code-point');
+require('../../modules/es6.string.raw');
+require('../../modules/es6.string.trim');
+require('../../modules/es6.string.iterator');
+require('../../modules/es6.string.code-point-at');
+require('../../modules/es6.string.ends-with');
+require('../../modules/es6.string.includes');
+require('../../modules/es6.string.repeat');
+require('../../modules/es6.string.starts-with');
+require('../../modules/es6.regexp.match');
+require('../../modules/es6.regexp.replace');
+require('../../modules/es6.regexp.search');
+require('../../modules/es6.regexp.split');
+require('../../modules/es7.string.at');
+require('../../modules/es7.string.pad-left');
+require('../../modules/es7.string.pad-right');
+require('../../modules/es7.string.trim-left');
+require('../../modules/es7.string.trim-right');
+require('../../modules/core.string.escape-html');
+require('../../modules/core.string.unescape-html');
+module.exports = require('../../modules/$.core').String;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/iterator.js
new file mode 100644
index 00000000..15733645
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/iterator.js
@@ -0,0 +1,5 @@
+require('../../modules/es6.string.iterator');
+var get = require('../../modules/$.iterators').String;
+module.exports = function(it){
+ return get.call(it);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/pad-left.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/pad-left.js
new file mode 100644
index 00000000..e89419c9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/pad-left.js
@@ -0,0 +1,2 @@
+require('../../modules/es7.string.pad-left');
+module.exports = require('../../modules/$.core').String.padLeft;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/pad-right.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/pad-right.js
new file mode 100644
index 00000000..a87a412e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/pad-right.js
@@ -0,0 +1,2 @@
+require('../../modules/es7.string.pad-right');
+module.exports = require('../../modules/$.core').String.padRight;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/raw.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/raw.js
new file mode 100644
index 00000000..0c04fd34
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/raw.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.string.raw');
+module.exports = require('../../modules/$.core').String.raw;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/repeat.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/repeat.js
new file mode 100644
index 00000000..36107095
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/repeat.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.string.repeat');
+module.exports = require('../../modules/$.core').String.repeat;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/starts-with.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/starts-with.js
new file mode 100644
index 00000000..edee8311
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/starts-with.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.string.starts-with');
+module.exports = require('../../modules/$.core').String.startsWith;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/trim-left.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/trim-left.js
new file mode 100644
index 00000000..579ad397
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/trim-left.js
@@ -0,0 +1,2 @@
+require('../../modules/es7.string.trim-left');
+module.exports = require('../../modules/$.core').String.trimLeft;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/trim-right.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/trim-right.js
new file mode 100644
index 00000000..2168d945
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/trim-right.js
@@ -0,0 +1,2 @@
+require('../../modules/es7.string.trim-right');
+module.exports = require('../../modules/$.core').String.trimRight;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/trim.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/trim.js
new file mode 100644
index 00000000..61c64701
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/trim.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.string.trim');
+module.exports = require('../../modules/$.core').String.trim;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/unescape-html.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/unescape-html.js
new file mode 100644
index 00000000..de09d98b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/string/unescape-html.js
@@ -0,0 +1,2 @@
+require('../../modules/core.string.unescape-html');
+module.exports = require('../../modules/$.core').String.unescapeHTML;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/for.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/for.js
new file mode 100644
index 00000000..1b05275d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/for.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.symbol');
+module.exports = require('../../modules/$.core').Symbol['for'];
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/has-instance.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/has-instance.js
new file mode 100644
index 00000000..b264f990
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/has-instance.js
@@ -0,0 +1 @@
+module.exports = require('../../modules/$.wks')('hasInstance');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/index.js
new file mode 100644
index 00000000..c8f81d18
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/index.js
@@ -0,0 +1,3 @@
+require('../../modules/es6.symbol');
+require('../../modules/es6.object.to-string');
+module.exports = require('../../modules/$.core').Symbol;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/is-concat-spreadable.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/is-concat-spreadable.js
new file mode 100644
index 00000000..17d5a266
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/is-concat-spreadable.js
@@ -0,0 +1 @@
+module.exports = require('../../modules/$.wks')('isConcatSpreadable');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/iterator.js
new file mode 100644
index 00000000..7e1b7985
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/iterator.js
@@ -0,0 +1,3 @@
+require('../../modules/es6.string.iterator');
+require('../../modules/web.dom.iterable');
+module.exports = require('../../modules/$.wks')('iterator');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/key-for.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/key-for.js
new file mode 100644
index 00000000..e62b1abd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/key-for.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.symbol');
+module.exports = require('../../modules/$.core').Symbol.keyFor;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/match.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/match.js
new file mode 100644
index 00000000..d25c1196
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/match.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.regexp.match');
+module.exports = require('../../modules/$.wks')('match');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/replace.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/replace.js
new file mode 100644
index 00000000..ce3154b9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/replace.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.regexp.replace');
+module.exports = require('../../modules/$.wks')('replace');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/search.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/search.js
new file mode 100644
index 00000000..ad781d40
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/search.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.regexp.search');
+module.exports = require('../../modules/$.wks')('search');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/species.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/species.js
new file mode 100644
index 00000000..de937d75
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/species.js
@@ -0,0 +1 @@
+module.exports = require('../../modules/$.wks')('species');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/split.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/split.js
new file mode 100644
index 00000000..27c51667
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/split.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.regexp.split');
+module.exports = require('../../modules/$.wks')('split');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/to-primitive.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/to-primitive.js
new file mode 100644
index 00000000..129eb8b2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/to-primitive.js
@@ -0,0 +1 @@
+module.exports = require('../../modules/$.wks')('toPrimitive');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/to-string-tag.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/to-string-tag.js
new file mode 100644
index 00000000..fc22c861
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/to-string-tag.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.to-string');
+module.exports = require('../../modules/$.wks')('toStringTag');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/unscopables.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/unscopables.js
new file mode 100644
index 00000000..39939700
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/symbol/unscopables.js
@@ -0,0 +1 @@
+module.exports = require('../../modules/$.wks')('unscopables');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/weak-map.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/weak-map.js
new file mode 100644
index 00000000..ebf46e6a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/weak-map.js
@@ -0,0 +1,4 @@
+require('../modules/es6.object.to-string');
+require('../modules/web.dom.iterable');
+require('../modules/es6.weak-map');
+module.exports = require('../modules/$.core').WeakMap;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/weak-set.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/weak-set.js
new file mode 100644
index 00000000..a058c8a6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/fn/weak-set.js
@@ -0,0 +1,4 @@
+require('../modules/es6.object.to-string');
+require('../modules/web.dom.iterable');
+require('../modules/es6.weak-set');
+module.exports = require('../modules/$.core').WeakSet;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/index.js
new file mode 100644
index 00000000..9acb1b4a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/index.js
@@ -0,0 +1,16 @@
+require('./shim');
+require('./modules/core.dict');
+require('./modules/core.get-iterator-method');
+require('./modules/core.get-iterator');
+require('./modules/core.is-iterable');
+require('./modules/core.delay');
+require('./modules/core.function.part');
+require('./modules/core.object.is-object');
+require('./modules/core.object.classof');
+require('./modules/core.object.define');
+require('./modules/core.object.make');
+require('./modules/core.number.iterator');
+require('./modules/core.string.escape-html');
+require('./modules/core.string.unescape-html');
+require('./modules/core.log');
+module.exports = require('./modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/js/array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/js/array.js
new file mode 100644
index 00000000..99a53add
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/js/array.js
@@ -0,0 +1,2 @@
+require('../modules/js.array.statics');
+module.exports = require('../modules/$.core').Array;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/js/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/js/index.js
new file mode 100644
index 00000000..47cb5ab5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/js/index.js
@@ -0,0 +1,2 @@
+require('../modules/js.array.statics');
+module.exports = require('../modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/_.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/_.js
new file mode 100644
index 00000000..475a66cd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/_.js
@@ -0,0 +1,2 @@
+require('../modules/core.function.part');
+module.exports = require('../modules/$.core')._;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/delay.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/delay.js
new file mode 100644
index 00000000..1ff9a56d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/delay.js
@@ -0,0 +1,2 @@
+require('../modules/core.delay');
+module.exports = require('../modules/$.core').delay;
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/dict.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/dict.js
new file mode 100644
index 00000000..ed848e2c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/dict.js
@@ -0,0 +1,2 @@
+require('../modules/core.dict');
+module.exports = require('../modules/$.core').Dict;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/function.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/function.js
new file mode 100644
index 00000000..42b6dbb8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/function.js
@@ -0,0 +1,2 @@
+require('../modules/core.function.part');
+module.exports = require('../modules/$.core').Function;
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/index.js
new file mode 100644
index 00000000..3d50bdb6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/index.js
@@ -0,0 +1,15 @@
+require('../modules/core.dict');
+require('../modules/core.get-iterator-method');
+require('../modules/core.get-iterator');
+require('../modules/core.is-iterable');
+require('../modules/core.delay');
+require('../modules/core.function.part');
+require('../modules/core.object.is-object');
+require('../modules/core.object.classof');
+require('../modules/core.object.define');
+require('../modules/core.object.make');
+require('../modules/core.number.iterator');
+require('../modules/core.string.escape-html');
+require('../modules/core.string.unescape-html');
+require('../modules/core.log');
+module.exports = require('../modules/$.core');
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/log.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/log.js
new file mode 100644
index 00000000..899d6ed0
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/log.js
@@ -0,0 +1,2 @@
+require('../modules/core.log');
+module.exports = require('../modules/$.core').log;
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/number.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/number.js
new file mode 100644
index 00000000..6e3cdbf6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/number.js
@@ -0,0 +1,2 @@
+require('../modules/core.number.iterator');
+module.exports = require('../modules/$.core').Number;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/object.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/object.js
new file mode 100644
index 00000000..63325663
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/object.js
@@ -0,0 +1,5 @@
+require('../modules/core.object.is-object');
+require('../modules/core.object.classof');
+require('../modules/core.object.define');
+require('../modules/core.object.make');
+module.exports = require('../modules/$.core').Object;
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/string.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/string.js
new file mode 100644
index 00000000..829e0c61
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/core/string.js
@@ -0,0 +1,3 @@
+require('../modules/core.string.escape-html');
+require('../modules/core.string.unescape-html');
+module.exports = require('../modules/$.core').String;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es5/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es5/index.js
new file mode 100644
index 00000000..3c23364a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es5/index.js
@@ -0,0 +1,9 @@
+require('../modules/es5');
+require('../modules/es6.object.freeze');
+require('../modules/es6.object.seal');
+require('../modules/es6.object.prevent-extensions');
+require('../modules/es6.object.is-frozen');
+require('../modules/es6.object.is-sealed');
+require('../modules/es6.object.is-extensible');
+require('../modules/es6.string.trim');
+module.exports = require('../modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/array.js
new file mode 100644
index 00000000..36629ca0
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/array.js
@@ -0,0 +1,10 @@
+require('../modules/es6.string.iterator');
+require('../modules/es6.array.from');
+require('../modules/es6.array.of');
+require('../modules/es6.array.species');
+require('../modules/es6.array.iterator');
+require('../modules/es6.array.copy-within');
+require('../modules/es6.array.fill');
+require('../modules/es6.array.find');
+require('../modules/es6.array.find-index');
+module.exports = require('../modules/$.core').Array;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/function.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/function.js
new file mode 100644
index 00000000..3e61dcd4
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/function.js
@@ -0,0 +1,3 @@
+require('../modules/es6.function.name');
+require('../modules/es6.function.has-instance');
+module.exports = require('../modules/$.core').Function;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/index.js
new file mode 100644
index 00000000..b9fc80be
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/index.js
@@ -0,0 +1,87 @@
+require('../modules/es6.symbol');
+require('../modules/es6.object.assign');
+require('../modules/es6.object.is');
+require('../modules/es6.object.set-prototype-of');
+require('../modules/es6.object.to-string');
+require('../modules/es6.object.freeze');
+require('../modules/es6.object.seal');
+require('../modules/es6.object.prevent-extensions');
+require('../modules/es6.object.is-frozen');
+require('../modules/es6.object.is-sealed');
+require('../modules/es6.object.is-extensible');
+require('../modules/es6.object.get-own-property-descriptor');
+require('../modules/es6.object.get-prototype-of');
+require('../modules/es6.object.keys');
+require('../modules/es6.object.get-own-property-names');
+require('../modules/es6.function.name');
+require('../modules/es6.function.has-instance');
+require('../modules/es6.number.constructor');
+require('../modules/es6.number.epsilon');
+require('../modules/es6.number.is-finite');
+require('../modules/es6.number.is-integer');
+require('../modules/es6.number.is-nan');
+require('../modules/es6.number.is-safe-integer');
+require('../modules/es6.number.max-safe-integer');
+require('../modules/es6.number.min-safe-integer');
+require('../modules/es6.number.parse-float');
+require('../modules/es6.number.parse-int');
+require('../modules/es6.math.acosh');
+require('../modules/es6.math.asinh');
+require('../modules/es6.math.atanh');
+require('../modules/es6.math.cbrt');
+require('../modules/es6.math.clz32');
+require('../modules/es6.math.cosh');
+require('../modules/es6.math.expm1');
+require('../modules/es6.math.fround');
+require('../modules/es6.math.hypot');
+require('../modules/es6.math.imul');
+require('../modules/es6.math.log10');
+require('../modules/es6.math.log1p');
+require('../modules/es6.math.log2');
+require('../modules/es6.math.sign');
+require('../modules/es6.math.sinh');
+require('../modules/es6.math.tanh');
+require('../modules/es6.math.trunc');
+require('../modules/es6.string.from-code-point');
+require('../modules/es6.string.raw');
+require('../modules/es6.string.trim');
+require('../modules/es6.string.iterator');
+require('../modules/es6.string.code-point-at');
+require('../modules/es6.string.ends-with');
+require('../modules/es6.string.includes');
+require('../modules/es6.string.repeat');
+require('../modules/es6.string.starts-with');
+require('../modules/es6.array.from');
+require('../modules/es6.array.of');
+require('../modules/es6.array.species');
+require('../modules/es6.array.iterator');
+require('../modules/es6.array.copy-within');
+require('../modules/es6.array.fill');
+require('../modules/es6.array.find');
+require('../modules/es6.array.find-index');
+require('../modules/es6.regexp.constructor');
+require('../modules/es6.regexp.flags');
+require('../modules/es6.regexp.match');
+require('../modules/es6.regexp.replace');
+require('../modules/es6.regexp.search');
+require('../modules/es6.regexp.split');
+require('../modules/es6.promise');
+require('../modules/es6.map');
+require('../modules/es6.set');
+require('../modules/es6.weak-map');
+require('../modules/es6.weak-set');
+require('../modules/es6.reflect.apply');
+require('../modules/es6.reflect.construct');
+require('../modules/es6.reflect.define-property');
+require('../modules/es6.reflect.delete-property');
+require('../modules/es6.reflect.enumerate');
+require('../modules/es6.reflect.get');
+require('../modules/es6.reflect.get-own-property-descriptor');
+require('../modules/es6.reflect.get-prototype-of');
+require('../modules/es6.reflect.has');
+require('../modules/es6.reflect.is-extensible');
+require('../modules/es6.reflect.own-keys');
+require('../modules/es6.reflect.prevent-extensions');
+require('../modules/es6.reflect.set');
+require('../modules/es6.reflect.set-prototype-of');
+module.exports = require('../modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/map.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/map.js
new file mode 100644
index 00000000..521a192e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/map.js
@@ -0,0 +1,5 @@
+require('../modules/es6.object.to-string');
+require('../modules/es6.string.iterator');
+require('../modules/web.dom.iterable');
+require('../modules/es6.map');
+module.exports = require('../modules/$.core').Map;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/math.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/math.js
new file mode 100644
index 00000000..8b5a2e79
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/math.js
@@ -0,0 +1,18 @@
+require('../modules/es6.math.acosh');
+require('../modules/es6.math.asinh');
+require('../modules/es6.math.atanh');
+require('../modules/es6.math.cbrt');
+require('../modules/es6.math.clz32');
+require('../modules/es6.math.cosh');
+require('../modules/es6.math.expm1');
+require('../modules/es6.math.fround');
+require('../modules/es6.math.hypot');
+require('../modules/es6.math.imul');
+require('../modules/es6.math.log10');
+require('../modules/es6.math.log1p');
+require('../modules/es6.math.log2');
+require('../modules/es6.math.sign');
+require('../modules/es6.math.sinh');
+require('../modules/es6.math.tanh');
+require('../modules/es6.math.trunc');
+module.exports = require('../modules/$.core').Math;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/number.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/number.js
new file mode 100644
index 00000000..8f487703
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/number.js
@@ -0,0 +1,11 @@
+require('../modules/es6.number.constructor');
+require('../modules/es6.number.epsilon');
+require('../modules/es6.number.is-finite');
+require('../modules/es6.number.is-integer');
+require('../modules/es6.number.is-nan');
+require('../modules/es6.number.is-safe-integer');
+require('../modules/es6.number.max-safe-integer');
+require('../modules/es6.number.min-safe-integer');
+require('../modules/es6.number.parse-float');
+require('../modules/es6.number.parse-int');
+module.exports = require('../modules/$.core').Number;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/object.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/object.js
new file mode 100644
index 00000000..94af189f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/object.js
@@ -0,0 +1,17 @@
+require('../modules/es6.symbol');
+require('../modules/es6.object.assign');
+require('../modules/es6.object.is');
+require('../modules/es6.object.set-prototype-of');
+require('../modules/es6.object.to-string');
+require('../modules/es6.object.freeze');
+require('../modules/es6.object.seal');
+require('../modules/es6.object.prevent-extensions');
+require('../modules/es6.object.is-frozen');
+require('../modules/es6.object.is-sealed');
+require('../modules/es6.object.is-extensible');
+require('../modules/es6.object.get-own-property-descriptor');
+require('../modules/es6.object.get-prototype-of');
+require('../modules/es6.object.keys');
+require('../modules/es6.object.get-own-property-names');
+
+module.exports = require('../modules/$.core').Object;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/promise.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/promise.js
new file mode 100644
index 00000000..0a099613
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/promise.js
@@ -0,0 +1,5 @@
+require('../modules/es6.object.to-string');
+require('../modules/es6.string.iterator');
+require('../modules/web.dom.iterable');
+require('../modules/es6.promise');
+module.exports = require('../modules/$.core').Promise;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/reflect.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/reflect.js
new file mode 100644
index 00000000..3c2f74eb
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/reflect.js
@@ -0,0 +1,15 @@
+require('../modules/es6.reflect.apply');
+require('../modules/es6.reflect.construct');
+require('../modules/es6.reflect.define-property');
+require('../modules/es6.reflect.delete-property');
+require('../modules/es6.reflect.enumerate');
+require('../modules/es6.reflect.get');
+require('../modules/es6.reflect.get-own-property-descriptor');
+require('../modules/es6.reflect.get-prototype-of');
+require('../modules/es6.reflect.has');
+require('../modules/es6.reflect.is-extensible');
+require('../modules/es6.reflect.own-keys');
+require('../modules/es6.reflect.prevent-extensions');
+require('../modules/es6.reflect.set');
+require('../modules/es6.reflect.set-prototype-of');
+module.exports = require('../modules/$.core').Reflect;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/regexp.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/regexp.js
new file mode 100644
index 00000000..195d36d4
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/regexp.js
@@ -0,0 +1,7 @@
+require('../modules/es6.regexp.constructor');
+require('../modules/es6.regexp.flags');
+require('../modules/es6.regexp.match');
+require('../modules/es6.regexp.replace');
+require('../modules/es6.regexp.search');
+require('../modules/es6.regexp.split');
+module.exports = require('../modules/$.core').RegExp;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/set.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/set.js
new file mode 100644
index 00000000..6a84f589
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/set.js
@@ -0,0 +1,5 @@
+require('../modules/es6.object.to-string');
+require('../modules/es6.string.iterator');
+require('../modules/web.dom.iterable');
+require('../modules/es6.set');
+module.exports = require('../modules/$.core').Set;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/string.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/string.js
new file mode 100644
index 00000000..bc7a1ab1
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/string.js
@@ -0,0 +1,14 @@
+require('../modules/es6.string.from-code-point');
+require('../modules/es6.string.raw');
+require('../modules/es6.string.trim');
+require('../modules/es6.string.iterator');
+require('../modules/es6.string.code-point-at');
+require('../modules/es6.string.ends-with');
+require('../modules/es6.string.includes');
+require('../modules/es6.string.repeat');
+require('../modules/es6.string.starts-with');
+require('../modules/es6.regexp.match');
+require('../modules/es6.regexp.replace');
+require('../modules/es6.regexp.search');
+require('../modules/es6.regexp.split');
+module.exports = require('../modules/$.core').String;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/symbol.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/symbol.js
new file mode 100644
index 00000000..ae2405b5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/symbol.js
@@ -0,0 +1,3 @@
+require('../modules/es6.symbol');
+require('../modules/es6.object.to-string');
+module.exports = require('../modules/$.core').Symbol;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/weak-map.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/weak-map.js
new file mode 100644
index 00000000..f2c6db89
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/weak-map.js
@@ -0,0 +1,4 @@
+require('../modules/es6.object.to-string');
+require('../modules/es6.array.iterator');
+require('../modules/es6.weak-map');
+module.exports = require('../modules/$.core').WeakMap;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/weak-set.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/weak-set.js
new file mode 100644
index 00000000..a058c8a6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es6/weak-set.js
@@ -0,0 +1,4 @@
+require('../modules/es6.object.to-string');
+require('../modules/web.dom.iterable');
+require('../modules/es6.weak-set');
+module.exports = require('../modules/$.core').WeakSet;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/array.js
new file mode 100644
index 00000000..e58f9057
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/array.js
@@ -0,0 +1,2 @@
+require('../modules/es7.array.includes');
+module.exports = require('../modules/$.core').Array;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/index.js
new file mode 100644
index 00000000..a277b639
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/index.js
@@ -0,0 +1,13 @@
+require('../modules/es7.array.includes');
+require('../modules/es7.string.at');
+require('../modules/es7.string.pad-left');
+require('../modules/es7.string.pad-right');
+require('../modules/es7.string.trim-left');
+require('../modules/es7.string.trim-right');
+require('../modules/es7.regexp.escape');
+require('../modules/es7.object.get-own-property-descriptors');
+require('../modules/es7.object.values');
+require('../modules/es7.object.entries');
+require('../modules/es7.map.to-json');
+require('../modules/es7.set.to-json');
+module.exports = require('../modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/map.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/map.js
new file mode 100644
index 00000000..fe519992
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/map.js
@@ -0,0 +1,2 @@
+require('../modules/es7.map.to-json');
+module.exports = require('../modules/$.core').Map;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/object.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/object.js
new file mode 100644
index 00000000..b0585303
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/object.js
@@ -0,0 +1,4 @@
+require('../modules/es7.object.get-own-property-descriptors');
+require('../modules/es7.object.values');
+require('../modules/es7.object.entries');
+module.exports = require('../modules/$.core').Object;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/regexp.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/regexp.js
new file mode 100644
index 00000000..cb24f98b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/regexp.js
@@ -0,0 +1,2 @@
+require('../modules/es7.regexp.escape');
+module.exports = require('../modules/$.core').RegExp;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/set.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/set.js
new file mode 100644
index 00000000..16e94528
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/set.js
@@ -0,0 +1,2 @@
+require('../modules/es7.set.to-json');
+module.exports = require('../modules/$.core').Set;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/string.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/string.js
new file mode 100644
index 00000000..bca0886c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/es7/string.js
@@ -0,0 +1,6 @@
+require('../modules/es7.string.at');
+require('../modules/es7.string.pad-left');
+require('../modules/es7.string.pad-right');
+require('../modules/es7.string.trim-left');
+require('../modules/es7.string.trim-right');
+module.exports = require('../modules/$.core').String;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/_.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/_.js
new file mode 100644
index 00000000..475a66cd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/_.js
@@ -0,0 +1,2 @@
+require('../modules/core.function.part');
+module.exports = require('../modules/$.core')._;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/concat.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/concat.js
new file mode 100644
index 00000000..176ecffe
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/concat.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.concat;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/copy-within.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/copy-within.js
new file mode 100644
index 00000000..8a011319
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/copy-within.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.array.copy-within');
+module.exports = require('../../modules/$.core').Array.copyWithin;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/entries.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/entries.js
new file mode 100644
index 00000000..bcdbc33f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/entries.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.array.iterator');
+module.exports = require('../../modules/$.core').Array.entries;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/every.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/every.js
new file mode 100644
index 00000000..0c7d0b7e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/every.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.every;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/fill.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/fill.js
new file mode 100644
index 00000000..f5362120
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/fill.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.array.fill');
+module.exports = require('../../modules/$.core').Array.fill;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/filter.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/filter.js
new file mode 100644
index 00000000..3f5b17ff
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/filter.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.filter;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/find-index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/find-index.js
new file mode 100644
index 00000000..7ec6cf7a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/find-index.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.array.find-index');
+module.exports = require('../../modules/$.core').Array.findIndex;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/find.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/find.js
new file mode 100644
index 00000000..9c3a6b31
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/find.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.array.find');
+module.exports = require('../../modules/$.core').Array.find;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/for-each.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/for-each.js
new file mode 100644
index 00000000..b2e79f0b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/for-each.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.forEach;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/from.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/from.js
new file mode 100644
index 00000000..f0483ccf
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/from.js
@@ -0,0 +1,3 @@
+require('../../modules/es6.string.iterator');
+require('../../modules/es6.array.from');
+module.exports = require('../../modules/$.core').Array.from;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/includes.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/includes.js
new file mode 100644
index 00000000..420c8318
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/includes.js
@@ -0,0 +1,2 @@
+require('../../modules/es7.array.includes');
+module.exports = require('../../modules/$.core').Array.includes;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/index-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/index-of.js
new file mode 100644
index 00000000..9f2cd14b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/index-of.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.indexOf;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/index.js
new file mode 100644
index 00000000..2707be21
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/index.js
@@ -0,0 +1,12 @@
+require('../../modules/es6.string.iterator');
+require('../../modules/es6.array.from');
+require('../../modules/es6.array.of');
+require('../../modules/es6.array.species');
+require('../../modules/es6.array.iterator');
+require('../../modules/es6.array.copy-within');
+require('../../modules/es6.array.fill');
+require('../../modules/es6.array.find');
+require('../../modules/es6.array.find-index');
+require('../../modules/es7.array.includes');
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/iterator.js
new file mode 100644
index 00000000..662f3b5c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/iterator.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.array.iterator');
+module.exports = require('../../modules/$.core').Array.values;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/join.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/join.js
new file mode 100644
index 00000000..44363922
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/join.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.join;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/keys.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/keys.js
new file mode 100644
index 00000000..e55d356e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/keys.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.array.iterator');
+module.exports = require('../../modules/$.core').Array.keys;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/last-index-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/last-index-of.js
new file mode 100644
index 00000000..678d0072
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/last-index-of.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.lastIndexOf;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/map.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/map.js
new file mode 100644
index 00000000..a1457c7a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/map.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.map;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/of.js
new file mode 100644
index 00000000..07bb5a4a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/of.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.array.of');
+module.exports = require('../../modules/$.core').Array.of;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/pop.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/pop.js
new file mode 100644
index 00000000..bd8f8610
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/pop.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.pop;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/push.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/push.js
new file mode 100644
index 00000000..3ccf0707
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/push.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.push;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/reduce-right.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/reduce-right.js
new file mode 100644
index 00000000..c592207c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/reduce-right.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.reduceRight;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/reduce.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/reduce.js
new file mode 100644
index 00000000..b8368406
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/reduce.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.reduce;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/reverse.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/reverse.js
new file mode 100644
index 00000000..4d8d235c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/reverse.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.reverse;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/shift.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/shift.js
new file mode 100644
index 00000000..806c87cd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/shift.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.shift;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/slice.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/slice.js
new file mode 100644
index 00000000..913f7ef2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/slice.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.slice;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/some.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/some.js
new file mode 100644
index 00000000..4f7c7654
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/some.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.some;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/sort.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/sort.js
new file mode 100644
index 00000000..61beed04
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/sort.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.sort;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/splice.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/splice.js
new file mode 100644
index 00000000..5f5eab07
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/splice.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.splice;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/unshift.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/unshift.js
new file mode 100644
index 00000000..a11de528
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/unshift.js
@@ -0,0 +1,2 @@
+require('../../modules/js.array.statics');
+module.exports = require('../../modules/$.core').Array.unshift;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/values.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/values.js
new file mode 100644
index 00000000..662f3b5c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/array/values.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.array.iterator');
+module.exports = require('../../modules/$.core').Array.values;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/clear-immediate.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/clear-immediate.js
new file mode 100644
index 00000000..06a97502
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/clear-immediate.js
@@ -0,0 +1,2 @@
+require('../modules/web.immediate');
+module.exports = require('../modules/$.core').clearImmediate;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/delay.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/delay.js
new file mode 100644
index 00000000..1ff9a56d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/delay.js
@@ -0,0 +1,2 @@
+require('../modules/core.delay');
+module.exports = require('../modules/$.core').delay;
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/dict.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/dict.js
new file mode 100644
index 00000000..ed848e2c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/dict.js
@@ -0,0 +1,2 @@
+require('../modules/core.dict');
+module.exports = require('../modules/$.core').Dict;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/function/has-instance.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/function/has-instance.js
new file mode 100644
index 00000000..78c8221c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/function/has-instance.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.function.has-instance');
+module.exports = Function[require('../../modules/$.wks')('hasInstance')];
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/function/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/function/index.js
new file mode 100644
index 00000000..7422fca3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/function/index.js
@@ -0,0 +1,4 @@
+require('../../modules/es6.function.name');
+require('../../modules/es6.function.has-instance');
+require('../../modules/core.function.part');
+module.exports = require('../../modules/$.core').Function;
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/function/name.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/function/name.js
new file mode 100644
index 00000000..cb70bf15
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/function/name.js
@@ -0,0 +1 @@
+require('../../modules/es6.function.name');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/function/part.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/function/part.js
new file mode 100644
index 00000000..26271d6a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/function/part.js
@@ -0,0 +1,2 @@
+require('../../modules/core.function.part');
+module.exports = require('../../modules/$.core').Function.part;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/get-iterator-method.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/get-iterator-method.js
new file mode 100644
index 00000000..5543cbbf
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/get-iterator-method.js
@@ -0,0 +1,3 @@
+require('../modules/web.dom.iterable');
+require('../modules/es6.string.iterator');
+module.exports = require('../modules/core.get-iterator-method');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/get-iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/get-iterator.js
new file mode 100644
index 00000000..762350ff
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/get-iterator.js
@@ -0,0 +1,3 @@
+require('../modules/web.dom.iterable');
+require('../modules/es6.string.iterator');
+module.exports = require('../modules/core.get-iterator');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/html-collection/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/html-collection/index.js
new file mode 100644
index 00000000..510156b8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/html-collection/index.js
@@ -0,0 +1,2 @@
+require('../../modules/web.dom.iterable');
+module.exports = require('../../modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/html-collection/iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/html-collection/iterator.js
new file mode 100644
index 00000000..c01119b1
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/html-collection/iterator.js
@@ -0,0 +1,2 @@
+require('../../modules/web.dom.iterable');
+module.exports = require('../../modules/$.core').Array.values;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/is-iterable.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/is-iterable.js
new file mode 100644
index 00000000..4c654e87
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/is-iterable.js
@@ -0,0 +1,3 @@
+require('../modules/web.dom.iterable');
+require('../modules/es6.string.iterator');
+module.exports = require('../modules/core.is-iterable');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/json/stringify.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/json/stringify.js
new file mode 100644
index 00000000..fef24250
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/json/stringify.js
@@ -0,0 +1,4 @@
+var core = require('../../modules/$.core');
+module.exports = function stringify(it){ // eslint-disable-line no-unused-vars
+ return (core.JSON && core.JSON.stringify || JSON.stringify).apply(JSON, arguments);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/log.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/log.js
new file mode 100644
index 00000000..899d6ed0
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/log.js
@@ -0,0 +1,2 @@
+require('../modules/core.log');
+module.exports = require('../modules/$.core').log;
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/map.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/map.js
new file mode 100644
index 00000000..70998924
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/map.js
@@ -0,0 +1,6 @@
+require('../modules/es6.object.to-string');
+require('../modules/es6.string.iterator');
+require('../modules/web.dom.iterable');
+require('../modules/es6.map');
+require('../modules/es7.map.to-json');
+module.exports = require('../modules/$.core').Map;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/acosh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/acosh.js
new file mode 100644
index 00000000..d29a88cc
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/acosh.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.acosh');
+module.exports = require('../../modules/$.core').Math.acosh;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/asinh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/asinh.js
new file mode 100644
index 00000000..7eac2e83
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/asinh.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.asinh');
+module.exports = require('../../modules/$.core').Math.asinh;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/atanh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/atanh.js
new file mode 100644
index 00000000..a66a47d8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/atanh.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.atanh');
+module.exports = require('../../modules/$.core').Math.atanh;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/cbrt.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/cbrt.js
new file mode 100644
index 00000000..199f5cd8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/cbrt.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.cbrt');
+module.exports = require('../../modules/$.core').Math.cbrt;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/clz32.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/clz32.js
new file mode 100644
index 00000000..2025c6e4
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/clz32.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.clz32');
+module.exports = require('../../modules/$.core').Math.clz32;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/cosh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/cosh.js
new file mode 100644
index 00000000..17a7ddc0
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/cosh.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.cosh');
+module.exports = require('../../modules/$.core').Math.cosh;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/expm1.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/expm1.js
new file mode 100644
index 00000000..732facb3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/expm1.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.expm1');
+module.exports = require('../../modules/$.core').Math.expm1;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/fround.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/fround.js
new file mode 100644
index 00000000..37f87069
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/fround.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.fround');
+module.exports = require('../../modules/$.core').Math.fround;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/hypot.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/hypot.js
new file mode 100644
index 00000000..9676c073
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/hypot.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.hypot');
+module.exports = require('../../modules/$.core').Math.hypot;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/imul.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/imul.js
new file mode 100644
index 00000000..2ea2913e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/imul.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.imul');
+module.exports = require('../../modules/$.core').Math.imul;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/index.js
new file mode 100644
index 00000000..14628ae5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/index.js
@@ -0,0 +1,18 @@
+require('../../modules/es6.math.acosh');
+require('../../modules/es6.math.asinh');
+require('../../modules/es6.math.atanh');
+require('../../modules/es6.math.cbrt');
+require('../../modules/es6.math.clz32');
+require('../../modules/es6.math.cosh');
+require('../../modules/es6.math.expm1');
+require('../../modules/es6.math.fround');
+require('../../modules/es6.math.hypot');
+require('../../modules/es6.math.imul');
+require('../../modules/es6.math.log10');
+require('../../modules/es6.math.log1p');
+require('../../modules/es6.math.log2');
+require('../../modules/es6.math.sign');
+require('../../modules/es6.math.sinh');
+require('../../modules/es6.math.tanh');
+require('../../modules/es6.math.trunc');
+module.exports = require('../../modules/$.core').Math;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/log10.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/log10.js
new file mode 100644
index 00000000..ecf7b9b2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/log10.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.log10');
+module.exports = require('../../modules/$.core').Math.log10;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/log1p.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/log1p.js
new file mode 100644
index 00000000..6db73292
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/log1p.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.log1p');
+module.exports = require('../../modules/$.core').Math.log1p;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/log2.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/log2.js
new file mode 100644
index 00000000..63c74d7b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/log2.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.log2');
+module.exports = require('../../modules/$.core').Math.log2;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/sign.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/sign.js
new file mode 100644
index 00000000..47ab74f2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/sign.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.sign');
+module.exports = require('../../modules/$.core').Math.sign;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/sinh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/sinh.js
new file mode 100644
index 00000000..72c6e857
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/sinh.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.sinh');
+module.exports = require('../../modules/$.core').Math.sinh;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/tanh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/tanh.js
new file mode 100644
index 00000000..30ddbcc8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/tanh.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.tanh');
+module.exports = require('../../modules/$.core').Math.tanh;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/trunc.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/trunc.js
new file mode 100644
index 00000000..b084efa7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/math/trunc.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.math.trunc');
+module.exports = require('../../modules/$.core').Math.trunc;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/node-list/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/node-list/index.js
new file mode 100644
index 00000000..510156b8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/node-list/index.js
@@ -0,0 +1,2 @@
+require('../../modules/web.dom.iterable');
+module.exports = require('../../modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/node-list/iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/node-list/iterator.js
new file mode 100644
index 00000000..c01119b1
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/node-list/iterator.js
@@ -0,0 +1,2 @@
+require('../../modules/web.dom.iterable');
+module.exports = require('../../modules/$.core').Array.values;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/epsilon.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/epsilon.js
new file mode 100644
index 00000000..56c93521
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/epsilon.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.number.epsilon');
+module.exports = Math.pow(2, -52);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/index.js
new file mode 100644
index 00000000..d048d590
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/index.js
@@ -0,0 +1,12 @@
+require('../../modules/es6.number.constructor');
+require('../../modules/es6.number.epsilon');
+require('../../modules/es6.number.is-finite');
+require('../../modules/es6.number.is-integer');
+require('../../modules/es6.number.is-nan');
+require('../../modules/es6.number.is-safe-integer');
+require('../../modules/es6.number.max-safe-integer');
+require('../../modules/es6.number.min-safe-integer');
+require('../../modules/es6.number.parse-float');
+require('../../modules/es6.number.parse-int');
+require('../../modules/core.number.iterator');
+module.exports = require('../../modules/$.core').Number;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/is-finite.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/is-finite.js
new file mode 100644
index 00000000..ff666503
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/is-finite.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.number.is-finite');
+module.exports = require('../../modules/$.core').Number.isFinite;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/is-integer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/is-integer.js
new file mode 100644
index 00000000..682e1e34
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/is-integer.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.number.is-integer');
+module.exports = require('../../modules/$.core').Number.isInteger;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/is-nan.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/is-nan.js
new file mode 100644
index 00000000..6ad6923b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/is-nan.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.number.is-nan');
+module.exports = require('../../modules/$.core').Number.isNaN;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/is-safe-integer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/is-safe-integer.js
new file mode 100644
index 00000000..a47fd42e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/is-safe-integer.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.number.is-safe-integer');
+module.exports = require('../../modules/$.core').Number.isSafeInteger;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/iterator.js
new file mode 100644
index 00000000..57cb7906
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/iterator.js
@@ -0,0 +1,5 @@
+require('../../modules/core.number.iterator');
+var get = require('../../modules/$.iterators').Number;
+module.exports = function(it){
+ return get.call(it);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/max-safe-integer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/max-safe-integer.js
new file mode 100644
index 00000000..c9b43b04
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/max-safe-integer.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.number.max-safe-integer');
+module.exports = 0x1fffffffffffff;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/min-safe-integer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/min-safe-integer.js
new file mode 100644
index 00000000..8b5e0728
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/min-safe-integer.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.number.min-safe-integer');
+module.exports = -0x1fffffffffffff;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/parse-float.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/parse-float.js
new file mode 100644
index 00000000..62f89774
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/parse-float.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.number.parse-float');
+module.exports = parseFloat;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/parse-int.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/parse-int.js
new file mode 100644
index 00000000..c197da5b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/number/parse-int.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.number.parse-int');
+module.exports = parseInt;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/assign.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/assign.js
new file mode 100644
index 00000000..a57c54aa
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/assign.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.assign');
+module.exports = require('../../modules/$.core').Object.assign;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/classof.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/classof.js
new file mode 100644
index 00000000..3afc8268
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/classof.js
@@ -0,0 +1,2 @@
+require('../../modules/core.object.classof');
+module.exports = require('../../modules/$.core').Object.classof;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/create.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/create.js
new file mode 100644
index 00000000..8f5f3263
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/create.js
@@ -0,0 +1,4 @@
+var $ = require('../../modules/$');
+module.exports = function create(P, D){
+ return $.create(P, D);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/define-properties.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/define-properties.js
new file mode 100644
index 00000000..a857aab8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/define-properties.js
@@ -0,0 +1,4 @@
+var $ = require('../../modules/$');
+module.exports = function defineProperties(T, D){
+ return $.setDescs(T, D);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/define-property.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/define-property.js
new file mode 100644
index 00000000..7384315d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/define-property.js
@@ -0,0 +1,4 @@
+var $ = require('../../modules/$');
+module.exports = function defineProperty(it, key, desc){
+ return $.setDesc(it, key, desc);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/define.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/define.js
new file mode 100644
index 00000000..690773e2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/define.js
@@ -0,0 +1,2 @@
+require('../../modules/core.object.define');
+module.exports = require('../../modules/$.core').Object.define;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/entries.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/entries.js
new file mode 100644
index 00000000..a32fe391
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/entries.js
@@ -0,0 +1,2 @@
+require('../../modules/es7.object.entries');
+module.exports = require('../../modules/$.core').Object.entries;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/freeze.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/freeze.js
new file mode 100644
index 00000000..566eec51
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/freeze.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.freeze');
+module.exports = require('../../modules/$.core').Object.freeze;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/get-own-property-descriptor.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/get-own-property-descriptor.js
new file mode 100644
index 00000000..2e1845cf
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/get-own-property-descriptor.js
@@ -0,0 +1,5 @@
+var $ = require('../../modules/$');
+require('../../modules/es6.object.get-own-property-descriptor');
+module.exports = function getOwnPropertyDescriptor(it, key){
+ return $.getDesc(it, key);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/get-own-property-descriptors.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/get-own-property-descriptors.js
new file mode 100644
index 00000000..f26341d5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/get-own-property-descriptors.js
@@ -0,0 +1,2 @@
+require('../../modules/es7.object.get-own-property-descriptors');
+module.exports = require('../../modules/$.core').Object.getOwnPropertyDescriptors;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/get-own-property-names.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/get-own-property-names.js
new file mode 100644
index 00000000..496eb197
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/get-own-property-names.js
@@ -0,0 +1,5 @@
+var $ = require('../../modules/$');
+require('../../modules/es6.object.get-own-property-names');
+module.exports = function getOwnPropertyNames(it){
+ return $.getNames(it);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/get-own-property-symbols.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/get-own-property-symbols.js
new file mode 100644
index 00000000..f78921b5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/get-own-property-symbols.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.symbol');
+module.exports = require('../../modules/$.core').Object.getOwnPropertySymbols;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/get-prototype-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/get-prototype-of.js
new file mode 100644
index 00000000..9a495afe
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/get-prototype-of.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.get-prototype-of');
+module.exports = require('../../modules/$.core').Object.getPrototypeOf;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/index.js
new file mode 100644
index 00000000..0fb75bc5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/index.js
@@ -0,0 +1,23 @@
+require('../../modules/es6.symbol');
+require('../../modules/es6.object.assign');
+require('../../modules/es6.object.is');
+require('../../modules/es6.object.set-prototype-of');
+require('../../modules/es6.object.to-string');
+require('../../modules/es6.object.freeze');
+require('../../modules/es6.object.seal');
+require('../../modules/es6.object.prevent-extensions');
+require('../../modules/es6.object.is-frozen');
+require('../../modules/es6.object.is-sealed');
+require('../../modules/es6.object.is-extensible');
+require('../../modules/es6.object.get-own-property-descriptor');
+require('../../modules/es6.object.get-prototype-of');
+require('../../modules/es6.object.keys');
+require('../../modules/es6.object.get-own-property-names');
+require('../../modules/es7.object.get-own-property-descriptors');
+require('../../modules/es7.object.values');
+require('../../modules/es7.object.entries');
+require('../../modules/core.object.is-object');
+require('../../modules/core.object.classof');
+require('../../modules/core.object.define');
+require('../../modules/core.object.make');
+module.exports = require('../../modules/$.core').Object;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/is-extensible.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/is-extensible.js
new file mode 100644
index 00000000..8bb0cf9f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/is-extensible.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.is-extensible');
+module.exports = require('../../modules/$.core').Object.isExtensible;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/is-frozen.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/is-frozen.js
new file mode 100644
index 00000000..7bf1f127
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/is-frozen.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.is-frozen');
+module.exports = require('../../modules/$.core').Object.isFrozen;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/is-object.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/is-object.js
new file mode 100644
index 00000000..935cb6ec
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/is-object.js
@@ -0,0 +1,2 @@
+require('../../modules/core.object.is-object');
+module.exports = require('../../modules/$.core').Object.isObject;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/is-sealed.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/is-sealed.js
new file mode 100644
index 00000000..05416f37
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/is-sealed.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.is-sealed');
+module.exports = require('../../modules/$.core').Object.isSealed;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/is.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/is.js
new file mode 100644
index 00000000..d07c3ae1
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/is.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.is');
+module.exports = require('../../modules/$.core').Object.is;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/keys.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/keys.js
new file mode 100644
index 00000000..ebfb8c65
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/keys.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.keys');
+module.exports = require('../../modules/$.core').Object.keys;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/make.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/make.js
new file mode 100644
index 00000000..fbfb2f85
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/make.js
@@ -0,0 +1,2 @@
+require('../../modules/core.object.make');
+module.exports = require('../../modules/$.core').Object.make;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/prevent-extensions.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/prevent-extensions.js
new file mode 100644
index 00000000..01d82fcb
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/prevent-extensions.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.prevent-extensions');
+module.exports = require('../../modules/$.core').Object.preventExtensions;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/seal.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/seal.js
new file mode 100644
index 00000000..fdf84b82
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/seal.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.seal');
+module.exports = require('../../modules/$.core').Object.seal;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/set-prototype-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/set-prototype-of.js
new file mode 100644
index 00000000..cd94d875
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/set-prototype-of.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.set-prototype-of');
+module.exports = require('../../modules/$.core').Object.setPrototypeOf;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/values.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/values.js
new file mode 100644
index 00000000..b96071ff
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/object/values.js
@@ -0,0 +1,2 @@
+require('../../modules/es7.object.values');
+module.exports = require('../../modules/$.core').Object.values;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/promise.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/promise.js
new file mode 100644
index 00000000..0a099613
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/promise.js
@@ -0,0 +1,5 @@
+require('../modules/es6.object.to-string');
+require('../modules/es6.string.iterator');
+require('../modules/web.dom.iterable');
+require('../modules/es6.promise');
+module.exports = require('../modules/$.core').Promise;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/apply.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/apply.js
new file mode 100644
index 00000000..75e5ff5b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/apply.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.apply');
+module.exports = require('../../modules/$.core').Reflect.apply;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/construct.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/construct.js
new file mode 100644
index 00000000..adc40ea8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/construct.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.construct');
+module.exports = require('../../modules/$.core').Reflect.construct;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/define-property.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/define-property.js
new file mode 100644
index 00000000..da78d62e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/define-property.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.define-property');
+module.exports = require('../../modules/$.core').Reflect.defineProperty;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/delete-property.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/delete-property.js
new file mode 100644
index 00000000..411225f6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/delete-property.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.delete-property');
+module.exports = require('../../modules/$.core').Reflect.deleteProperty;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/enumerate.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/enumerate.js
new file mode 100644
index 00000000..c19e0b6b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/enumerate.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.enumerate');
+module.exports = require('../../modules/$.core').Reflect.enumerate;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/get-own-property-descriptor.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/get-own-property-descriptor.js
new file mode 100644
index 00000000..22e2aa66
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/get-own-property-descriptor.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.get-own-property-descriptor');
+module.exports = require('../../modules/$.core').Reflect.getOwnPropertyDescriptor;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/get-prototype-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/get-prototype-of.js
new file mode 100644
index 00000000..2ff331a4
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/get-prototype-of.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.get-prototype-of');
+module.exports = require('../../modules/$.core').Reflect.getPrototypeOf;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/get.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/get.js
new file mode 100644
index 00000000..266508c6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/get.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.get');
+module.exports = require('../../modules/$.core').Reflect.get;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/has.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/has.js
new file mode 100644
index 00000000..db14fa11
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/has.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.has');
+module.exports = require('../../modules/$.core').Reflect.has;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/index.js
new file mode 100644
index 00000000..5b216653
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/index.js
@@ -0,0 +1,15 @@
+require('../../modules/es6.reflect.apply');
+require('../../modules/es6.reflect.construct');
+require('../../modules/es6.reflect.define-property');
+require('../../modules/es6.reflect.delete-property');
+require('../../modules/es6.reflect.enumerate');
+require('../../modules/es6.reflect.get');
+require('../../modules/es6.reflect.get-own-property-descriptor');
+require('../../modules/es6.reflect.get-prototype-of');
+require('../../modules/es6.reflect.has');
+require('../../modules/es6.reflect.is-extensible');
+require('../../modules/es6.reflect.own-keys');
+require('../../modules/es6.reflect.prevent-extensions');
+require('../../modules/es6.reflect.set');
+require('../../modules/es6.reflect.set-prototype-of');
+module.exports = require('../../modules/$.core').Reflect;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/is-extensible.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/is-extensible.js
new file mode 100644
index 00000000..f0329e28
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/is-extensible.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.is-extensible');
+module.exports = require('../../modules/$.core').Reflect.isExtensible;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/own-keys.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/own-keys.js
new file mode 100644
index 00000000..6da1136d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/own-keys.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.own-keys');
+module.exports = require('../../modules/$.core').Reflect.ownKeys;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/prevent-extensions.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/prevent-extensions.js
new file mode 100644
index 00000000..48fb5d5a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/prevent-extensions.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.prevent-extensions');
+module.exports = require('../../modules/$.core').Reflect.preventExtensions;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/set-prototype-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/set-prototype-of.js
new file mode 100644
index 00000000..09cddebc
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/set-prototype-of.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.set-prototype-of');
+module.exports = require('../../modules/$.core').Reflect.setPrototypeOf;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/set.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/set.js
new file mode 100644
index 00000000..d1afec9c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/reflect/set.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.reflect.set');
+module.exports = require('../../modules/$.core').Reflect.set;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/regexp/escape.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/regexp/escape.js
new file mode 100644
index 00000000..0c8d06b4
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/regexp/escape.js
@@ -0,0 +1,2 @@
+require('../../modules/es7.regexp.escape');
+module.exports = require('../../modules/$.core').RegExp.escape;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/regexp/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/regexp/index.js
new file mode 100644
index 00000000..7d905d67
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/regexp/index.js
@@ -0,0 +1,8 @@
+require('../../modules/es6.regexp.constructor');
+require('../../modules/es6.regexp.flags');
+require('../../modules/es6.regexp.match');
+require('../../modules/es6.regexp.replace');
+require('../../modules/es6.regexp.search');
+require('../../modules/es6.regexp.split');
+require('../../modules/es7.regexp.escape');
+module.exports = require('../../modules/$.core').RegExp;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/set-immediate.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/set-immediate.js
new file mode 100644
index 00000000..2dd87df2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/set-immediate.js
@@ -0,0 +1,2 @@
+require('../modules/web.immediate');
+module.exports = require('../modules/$.core').setImmediate;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/set-interval.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/set-interval.js
new file mode 100644
index 00000000..4c7dd8e9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/set-interval.js
@@ -0,0 +1,2 @@
+require('../modules/web.timers');
+module.exports = require('../modules/$.core').setInterval;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/set-timeout.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/set-timeout.js
new file mode 100644
index 00000000..4e786194
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/set-timeout.js
@@ -0,0 +1,2 @@
+require('../modules/web.timers');
+module.exports = require('../modules/$.core').setTimeout;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/set.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/set.js
new file mode 100644
index 00000000..34615f1d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/set.js
@@ -0,0 +1,6 @@
+require('../modules/es6.object.to-string');
+require('../modules/es6.string.iterator');
+require('../modules/web.dom.iterable');
+require('../modules/es6.set');
+require('../modules/es7.set.to-json');
+module.exports = require('../modules/$.core').Set;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/at.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/at.js
new file mode 100644
index 00000000..d59d2aff
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/at.js
@@ -0,0 +1,2 @@
+require('../../modules/es7.string.at');
+module.exports = require('../../modules/$.core').String.at;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/code-point-at.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/code-point-at.js
new file mode 100644
index 00000000..74e933aa
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/code-point-at.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.string.code-point-at');
+module.exports = require('../../modules/$.core').String.codePointAt;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/ends-with.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/ends-with.js
new file mode 100644
index 00000000..7fe5cb74
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/ends-with.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.string.ends-with');
+module.exports = require('../../modules/$.core').String.endsWith;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/escape-html.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/escape-html.js
new file mode 100644
index 00000000..a6c62faf
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/escape-html.js
@@ -0,0 +1,2 @@
+require('../../modules/core.string.escape-html');
+module.exports = require('../../modules/$.core').String.escapeHTML;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/from-code-point.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/from-code-point.js
new file mode 100644
index 00000000..0b42e7ae
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/from-code-point.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.string.from-code-point');
+module.exports = require('../../modules/$.core').String.fromCodePoint;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/includes.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/includes.js
new file mode 100644
index 00000000..441bc594
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/includes.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.string.includes');
+module.exports = require('../../modules/$.core').String.includes;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/index.js
new file mode 100644
index 00000000..6be228ca
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/index.js
@@ -0,0 +1,21 @@
+require('../../modules/es6.string.from-code-point');
+require('../../modules/es6.string.raw');
+require('../../modules/es6.string.trim');
+require('../../modules/es6.string.iterator');
+require('../../modules/es6.string.code-point-at');
+require('../../modules/es6.string.ends-with');
+require('../../modules/es6.string.includes');
+require('../../modules/es6.string.repeat');
+require('../../modules/es6.string.starts-with');
+require('../../modules/es6.regexp.match');
+require('../../modules/es6.regexp.replace');
+require('../../modules/es6.regexp.search');
+require('../../modules/es6.regexp.split');
+require('../../modules/es7.string.at');
+require('../../modules/es7.string.pad-left');
+require('../../modules/es7.string.pad-right');
+require('../../modules/es7.string.trim-left');
+require('../../modules/es7.string.trim-right');
+require('../../modules/core.string.escape-html');
+require('../../modules/core.string.unescape-html');
+module.exports = require('../../modules/$.core').String;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/iterator.js
new file mode 100644
index 00000000..15733645
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/iterator.js
@@ -0,0 +1,5 @@
+require('../../modules/es6.string.iterator');
+var get = require('../../modules/$.iterators').String;
+module.exports = function(it){
+ return get.call(it);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/pad-left.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/pad-left.js
new file mode 100644
index 00000000..e89419c9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/pad-left.js
@@ -0,0 +1,2 @@
+require('../../modules/es7.string.pad-left');
+module.exports = require('../../modules/$.core').String.padLeft;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/pad-right.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/pad-right.js
new file mode 100644
index 00000000..a87a412e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/pad-right.js
@@ -0,0 +1,2 @@
+require('../../modules/es7.string.pad-right');
+module.exports = require('../../modules/$.core').String.padRight;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/raw.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/raw.js
new file mode 100644
index 00000000..0c04fd34
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/raw.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.string.raw');
+module.exports = require('../../modules/$.core').String.raw;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/repeat.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/repeat.js
new file mode 100644
index 00000000..36107095
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/repeat.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.string.repeat');
+module.exports = require('../../modules/$.core').String.repeat;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/starts-with.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/starts-with.js
new file mode 100644
index 00000000..edee8311
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/starts-with.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.string.starts-with');
+module.exports = require('../../modules/$.core').String.startsWith;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/trim-left.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/trim-left.js
new file mode 100644
index 00000000..579ad397
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/trim-left.js
@@ -0,0 +1,2 @@
+require('../../modules/es7.string.trim-left');
+module.exports = require('../../modules/$.core').String.trimLeft;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/trim-right.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/trim-right.js
new file mode 100644
index 00000000..2168d945
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/trim-right.js
@@ -0,0 +1,2 @@
+require('../../modules/es7.string.trim-right');
+module.exports = require('../../modules/$.core').String.trimRight;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/trim.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/trim.js
new file mode 100644
index 00000000..61c64701
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/trim.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.string.trim');
+module.exports = require('../../modules/$.core').String.trim;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/unescape-html.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/unescape-html.js
new file mode 100644
index 00000000..de09d98b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/string/unescape-html.js
@@ -0,0 +1,2 @@
+require('../../modules/core.string.unescape-html');
+module.exports = require('../../modules/$.core').String.unescapeHTML;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/for.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/for.js
new file mode 100644
index 00000000..1b05275d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/for.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.symbol');
+module.exports = require('../../modules/$.core').Symbol['for'];
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/has-instance.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/has-instance.js
new file mode 100644
index 00000000..b264f990
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/has-instance.js
@@ -0,0 +1 @@
+module.exports = require('../../modules/$.wks')('hasInstance');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/index.js
new file mode 100644
index 00000000..c8f81d18
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/index.js
@@ -0,0 +1,3 @@
+require('../../modules/es6.symbol');
+require('../../modules/es6.object.to-string');
+module.exports = require('../../modules/$.core').Symbol;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/is-concat-spreadable.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/is-concat-spreadable.js
new file mode 100644
index 00000000..17d5a266
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/is-concat-spreadable.js
@@ -0,0 +1 @@
+module.exports = require('../../modules/$.wks')('isConcatSpreadable');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/iterator.js
new file mode 100644
index 00000000..7e1b7985
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/iterator.js
@@ -0,0 +1,3 @@
+require('../../modules/es6.string.iterator');
+require('../../modules/web.dom.iterable');
+module.exports = require('../../modules/$.wks')('iterator');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/key-for.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/key-for.js
new file mode 100644
index 00000000..e62b1abd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/key-for.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.symbol');
+module.exports = require('../../modules/$.core').Symbol.keyFor;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/match.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/match.js
new file mode 100644
index 00000000..d25c1196
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/match.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.regexp.match');
+module.exports = require('../../modules/$.wks')('match');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/replace.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/replace.js
new file mode 100644
index 00000000..ce3154b9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/replace.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.regexp.replace');
+module.exports = require('../../modules/$.wks')('replace');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/search.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/search.js
new file mode 100644
index 00000000..ad781d40
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/search.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.regexp.search');
+module.exports = require('../../modules/$.wks')('search');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/species.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/species.js
new file mode 100644
index 00000000..de937d75
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/species.js
@@ -0,0 +1 @@
+module.exports = require('../../modules/$.wks')('species');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/split.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/split.js
new file mode 100644
index 00000000..27c51667
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/split.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.regexp.split');
+module.exports = require('../../modules/$.wks')('split');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/to-primitive.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/to-primitive.js
new file mode 100644
index 00000000..129eb8b2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/to-primitive.js
@@ -0,0 +1 @@
+module.exports = require('../../modules/$.wks')('toPrimitive');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/to-string-tag.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/to-string-tag.js
new file mode 100644
index 00000000..fc22c861
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/to-string-tag.js
@@ -0,0 +1,2 @@
+require('../../modules/es6.object.to-string');
+module.exports = require('../../modules/$.wks')('toStringTag');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/unscopables.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/unscopables.js
new file mode 100644
index 00000000..39939700
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/symbol/unscopables.js
@@ -0,0 +1 @@
+module.exports = require('../../modules/$.wks')('unscopables');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/weak-map.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/weak-map.js
new file mode 100644
index 00000000..ebf46e6a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/weak-map.js
@@ -0,0 +1,4 @@
+require('../modules/es6.object.to-string');
+require('../modules/web.dom.iterable');
+require('../modules/es6.weak-map');
+module.exports = require('../modules/$.core').WeakMap;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/weak-set.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/weak-set.js
new file mode 100644
index 00000000..a058c8a6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/fn/weak-set.js
@@ -0,0 +1,4 @@
+require('../modules/es6.object.to-string');
+require('../modules/web.dom.iterable');
+require('../modules/es6.weak-set');
+module.exports = require('../modules/$.core').WeakSet;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/index.js
new file mode 100644
index 00000000..9acb1b4a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/index.js
@@ -0,0 +1,16 @@
+require('./shim');
+require('./modules/core.dict');
+require('./modules/core.get-iterator-method');
+require('./modules/core.get-iterator');
+require('./modules/core.is-iterable');
+require('./modules/core.delay');
+require('./modules/core.function.part');
+require('./modules/core.object.is-object');
+require('./modules/core.object.classof');
+require('./modules/core.object.define');
+require('./modules/core.object.make');
+require('./modules/core.number.iterator');
+require('./modules/core.string.escape-html');
+require('./modules/core.string.unescape-html');
+require('./modules/core.log');
+module.exports = require('./modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/js/array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/js/array.js
new file mode 100644
index 00000000..99a53add
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/js/array.js
@@ -0,0 +1,2 @@
+require('../modules/js.array.statics');
+module.exports = require('../modules/$.core').Array;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/js/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/js/index.js
new file mode 100644
index 00000000..47cb5ab5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/js/index.js
@@ -0,0 +1,2 @@
+require('../modules/js.array.statics');
+module.exports = require('../modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.a-function.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.a-function.js
new file mode 100644
index 00000000..8c35f451
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.a-function.js
@@ -0,0 +1,4 @@
+module.exports = function(it){
+ if(typeof it != 'function')throw TypeError(it + ' is not a function!');
+ return it;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.add-to-unscopables.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.add-to-unscopables.js
new file mode 100644
index 00000000..faf87af3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.add-to-unscopables.js
@@ -0,0 +1 @@
+module.exports = function(){ /* empty */ };
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.an-object.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.an-object.js
new file mode 100644
index 00000000..e5c808fd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.an-object.js
@@ -0,0 +1,5 @@
+var isObject = require('./$.is-object');
+module.exports = function(it){
+ if(!isObject(it))throw TypeError(it + ' is not an object!');
+ return it;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.array-copy-within.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.array-copy-within.js
new file mode 100644
index 00000000..58563065
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.array-copy-within.js
@@ -0,0 +1,27 @@
+// 22.1.3.3 Array.prototype.copyWithin(target, start, end = this.length)
+'use strict';
+var toObject = require('./$.to-object')
+ , toIndex = require('./$.to-index')
+ , toLength = require('./$.to-length');
+
+module.exports = [].copyWithin || function copyWithin(target/*= 0*/, start/*= 0, end = @length*/){
+ var O = toObject(this)
+ , len = toLength(O.length)
+ , to = toIndex(target, len)
+ , from = toIndex(start, len)
+ , $$ = arguments
+ , end = $$.length > 2 ? $$[2] : undefined
+ , count = Math.min((end === undefined ? len : toIndex(end, len)) - from, len - to)
+ , inc = 1;
+ if(from < to && to < from + count){
+ inc = -1;
+ from += count - 1;
+ to += count - 1;
+ }
+ while(count-- > 0){
+ if(from in O)O[to] = O[from];
+ else delete O[to];
+ to += inc;
+ from += inc;
+ } return O;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.array-fill.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.array-fill.js
new file mode 100644
index 00000000..6b699df7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.array-fill.js
@@ -0,0 +1,16 @@
+// 22.1.3.6 Array.prototype.fill(value, start = 0, end = this.length)
+'use strict';
+var toObject = require('./$.to-object')
+ , toIndex = require('./$.to-index')
+ , toLength = require('./$.to-length');
+module.exports = [].fill || function fill(value /*, start = 0, end = @length */){
+ var O = toObject(this)
+ , length = toLength(O.length)
+ , $$ = arguments
+ , $$len = $$.length
+ , index = toIndex($$len > 1 ? $$[1] : undefined, length)
+ , end = $$len > 2 ? $$[2] : undefined
+ , endPos = end === undefined ? length : toIndex(end, length);
+ while(endPos > index)O[index++] = value;
+ return O;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.array-includes.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.array-includes.js
new file mode 100644
index 00000000..9781fca7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.array-includes.js
@@ -0,0 +1,21 @@
+// false -> Array#indexOf
+// true -> Array#includes
+var toIObject = require('./$.to-iobject')
+ , toLength = require('./$.to-length')
+ , toIndex = require('./$.to-index');
+module.exports = function(IS_INCLUDES){
+ return function($this, el, fromIndex){
+ var O = toIObject($this)
+ , length = toLength(O.length)
+ , index = toIndex(fromIndex, length)
+ , value;
+ // Array#includes uses SameValueZero equality algorithm
+ if(IS_INCLUDES && el != el)while(length > index){
+ value = O[index++];
+ if(value != value)return true;
+ // Array#toIndex ignores holes, Array#includes - not
+ } else for(;length > index; index++)if(IS_INCLUDES || index in O){
+ if(O[index] === el)return IS_INCLUDES || index;
+ } return !IS_INCLUDES && -1;
+ };
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.array-methods.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.array-methods.js
new file mode 100644
index 00000000..e70b99f4
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.array-methods.js
@@ -0,0 +1,43 @@
+// 0 -> Array#forEach
+// 1 -> Array#map
+// 2 -> Array#filter
+// 3 -> Array#some
+// 4 -> Array#every
+// 5 -> Array#find
+// 6 -> Array#findIndex
+var ctx = require('./$.ctx')
+ , IObject = require('./$.iobject')
+ , toObject = require('./$.to-object')
+ , toLength = require('./$.to-length')
+ , asc = require('./$.array-species-create');
+module.exports = function(TYPE){
+ var IS_MAP = TYPE == 1
+ , IS_FILTER = TYPE == 2
+ , IS_SOME = TYPE == 3
+ , IS_EVERY = TYPE == 4
+ , IS_FIND_INDEX = TYPE == 6
+ , NO_HOLES = TYPE == 5 || IS_FIND_INDEX;
+ return function($this, callbackfn, that){
+ var O = toObject($this)
+ , self = IObject(O)
+ , f = ctx(callbackfn, that, 3)
+ , length = toLength(self.length)
+ , index = 0
+ , result = IS_MAP ? asc($this, length) : IS_FILTER ? asc($this, 0) : undefined
+ , val, res;
+ for(;length > index; index++)if(NO_HOLES || index in self){
+ val = self[index];
+ res = f(val, index, O);
+ if(TYPE){
+ if(IS_MAP)result[index] = res; // map
+ else if(res)switch(TYPE){
+ case 3: return true; // some
+ case 5: return val; // find
+ case 6: return index; // findIndex
+ case 2: result.push(val); // filter
+ } else if(IS_EVERY)return false; // every
+ }
+ }
+ return IS_FIND_INDEX ? -1 : IS_SOME || IS_EVERY ? IS_EVERY : result;
+ };
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.array-species-create.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.array-species-create.js
new file mode 100644
index 00000000..d809cae6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.array-species-create.js
@@ -0,0 +1,16 @@
+// 9.4.2.3 ArraySpeciesCreate(originalArray, length)
+var isObject = require('./$.is-object')
+ , isArray = require('./$.is-array')
+ , SPECIES = require('./$.wks')('species');
+module.exports = function(original, length){
+ var C;
+ if(isArray(original)){
+ C = original.constructor;
+ // cross-realm fallback
+ if(typeof C == 'function' && (C === Array || isArray(C.prototype)))C = undefined;
+ if(isObject(C)){
+ C = C[SPECIES];
+ if(C === null)C = undefined;
+ }
+ } return new (C === undefined ? Array : C)(length);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.buffer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.buffer.js
new file mode 100644
index 00000000..d1aae58a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.buffer.js
@@ -0,0 +1,288 @@
+'use strict';
+var $ = require('./$')
+ , global = require('./$.global')
+ , $typed = require('./$.typed')
+ , redefineAll = require('./$.redefine-all')
+ , strictNew = require('./$.strict-new')
+ , toInteger = require('./$.to-integer')
+ , toLength = require('./$.to-length')
+ , arrayFill = require('./$.array-fill')
+ , $ArrayBuffer = global.ArrayBuffer
+ , $DataView = global.DataView
+ , Math = global.Math
+ , parseInt = global.parseInt
+ , abs = Math.abs
+ , pow = Math.pow
+ , min = Math.min
+ , floor = Math.floor
+ , log = Math.log
+ , LN2 = Math.LN2
+ , BYTE_LENGTH = 'byteLength';
+
+// pack / unpack based on
+// https://github.com/inexorabletash/polyfill/blob/v0.1.11/typedarray.js#L123-L264
+// TODO: simplify
+var signed = function(value, bits){
+ var s = 32 - bits;
+ return value << s >> s;
+};
+var unsigned = function(value, bits){
+ var s = 32 - bits;
+ return value << s >>> s;
+};
+var roundToEven = function(n){
+ var w = floor(n)
+ , f = n - w;
+ return f < .5 ? w : f > .5 ? w + 1 : w % 2 ? w + 1 : w;
+};
+var packI8 = function(n){
+ return [n & 0xff];
+};
+var unpackI8 = function(bytes){
+ return signed(bytes[0], 8);
+};
+var packU8 = function(n){
+ return [n & 0xff];
+};
+var unpackU8 = function(bytes){
+ return unsigned(bytes[0], 8);
+};
+var packI16 = function(n){
+ return [n & 0xff, n >> 8 & 0xff];
+};
+var unpackI16 = function(bytes){
+ return signed(bytes[1] << 8 | bytes[0], 16);
+};
+var packU16 = function(n){
+ return [n & 0xff, n >> 8 & 0xff];
+};
+var unpackU16 = function(bytes){
+ return unsigned(bytes[1] << 8 | bytes[0], 16);
+};
+var packI32 = function(n){
+ return [n & 0xff, n >> 8 & 0xff, n >> 16 & 0xff, n >> 24 & 0xff];
+};
+var unpackI32 = function(bytes){
+ return signed(bytes[3] << 24 | bytes[2] << 16 | bytes[1] << 8 | bytes[0], 32);
+};
+var packU32 = function(n){
+ return [n & 0xff, n >> 8 & 0xff, n >> 16 & 0xff, n >> 24 & 0xff];
+};
+var unpackU32 = function(bytes){
+ return unsigned(bytes[3] << 24 | bytes[2] << 16 | bytes[1] << 8 | bytes[0], 32);
+};
+var packIEEE754 = function(v, ebits, fbits) {
+ var bias = (1 << ebits - 1) - 1
+ , s, e, f, i, bits, str, bytes;
+ // Compute sign, exponent, fraction
+ if (v !== v) {
+ // NaN
+ // http://dev.w3.org/2006/webapi/WebIDL/#es-type-mapping
+ e = (1 << ebits) - 1;
+ f = pow(2, fbits - 1);
+ s = 0;
+ } else if(v === Infinity || v === -Infinity){
+ e = (1 << ebits) - 1;
+ f = 0;
+ s = v < 0 ? 1 : 0;
+ } else if(v === 0){
+ e = 0;
+ f = 0;
+ s = 1 / v === -Infinity ? 1 : 0;
+ } else {
+ s = v < 0;
+ v = abs(v);
+ if(v >= pow(2, 1 - bias)){
+ e = min(floor(log(v) / LN2), 1023);
+ var significand = v / pow(2, e);
+ if(significand < 1){
+ e -= 1;
+ significand *= 2;
+ }
+ if(significand >= 2){
+ e += 1;
+ significand /= 2;
+ }
+ f = roundToEven(significand * pow(2, fbits));
+ if(f / pow(2, fbits) >= 2){
+ e = e + 1;
+ f = 1;
+ }
+ if(e > bias){
+ // Overflow
+ e = (1 << ebits) - 1;
+ f = 0;
+ } else {
+ // Normalized
+ e = e + bias;
+ f = f - pow(2, fbits);
+ }
+ } else {
+ // Denormalized
+ e = 0;
+ f = roundToEven(v / pow(2, 1 - bias - fbits));
+ }
+ }
+ // Pack sign, exponent, fraction
+ bits = [];
+ for(i = fbits; i; i -= 1){
+ bits.push(f % 2 ? 1 : 0);
+ f = floor(f / 2);
+ }
+ for(i = ebits; i; i -= 1){
+ bits.push(e % 2 ? 1 : 0);
+ e = floor(e / 2);
+ }
+ bits.push(s ? 1 : 0);
+ bits.reverse();
+ str = bits.join('');
+ // Bits to bytes
+ bytes = [];
+ while(str.length){
+ bytes.unshift(parseInt(str.slice(0, 8), 2));
+ str = str.slice(8);
+ }
+ return bytes;
+};
+var unpackIEEE754 = function(bytes, ebits, fbits){
+ var bits = []
+ , i, j, b, str, bias, s, e, f;
+ for(i = 0; i < bytes.length; ++i)for(b = bytes[i], j = 8; j; --j){
+ bits.push(b % 2 ? 1 : 0);
+ b = b >> 1;
+ }
+ bits.reverse();
+ str = bits.join('');
+ // Unpack sign, exponent, fraction
+ bias = (1 << ebits - 1) - 1;
+ s = parseInt(str.slice(0, 1), 2) ? -1 : 1;
+ e = parseInt(str.slice(1, 1 + ebits), 2);
+ f = parseInt(str.slice(1 + ebits), 2);
+ // Produce number
+ if(e === (1 << ebits) - 1)return f !== 0 ? NaN : s * Infinity;
+ // Normalized
+ else if(e > 0)return s * pow(2, e - bias) * (1 + f / pow(2, fbits));
+ // Denormalized
+ else if(f !== 0)return s * pow(2, -(bias - 1)) * (f / pow(2, fbits));
+ return s < 0 ? -0 : 0;
+};
+var unpackF64 = function(b){
+ return unpackIEEE754(b, 11, 52);
+};
+var packF64 = function(v){
+ return packIEEE754(v, 11, 52);
+};
+var unpackF32 = function(b){
+ return unpackIEEE754(b, 8, 23);
+};
+var packF32 = function(v){
+ return packIEEE754(v, 8, 23);
+};
+
+var addGetter = function(C, key, internal){
+ $.setDesc(C.prototype, key, {get: function(){ return this[internal]; }});
+};
+
+var get = function(view, bytes, index, conversion, isLittleEndian){
+ var numIndex = +index
+ , intIndex = toInteger(numIndex);
+ if(numIndex != intIndex || intIndex < 0 || intIndex + bytes > view._l)throw RangeError();
+ var store = view._b._b
+ , start = intIndex + view._o
+ , pack = store.slice(start, start + bytes);
+ isLittleEndian || pack.reverse();
+ return conversion(pack);
+};
+var set = function(view, bytes, index, conversion, value, isLittleEndian){
+ var numIndex = +index
+ , intIndex = toInteger(numIndex);
+ if(numIndex != intIndex || intIndex < 0 || intIndex + bytes > view._l)throw RangeError();
+ var store = view._b._b
+ , start = intIndex + view._o
+ , pack = conversion(+value);
+ isLittleEndian || pack.reverse();
+ for(var i = 0; i < bytes; i++)store[start + i] = pack[i];
+};
+
+if(!$typed.ABV){
+ $ArrayBuffer = function ArrayBuffer(length){
+ strictNew(this, $ArrayBuffer, 'ArrayBuffer');
+ var numberLength = +length
+ , byteLength = toLength(numberLength);
+ if(numberLength != byteLength)throw RangeError();
+ this._b = arrayFill.call(Array(byteLength), 0);
+ this._l = byteLength;
+ };
+ addGetter($ArrayBuffer, BYTE_LENGTH, '_l');
+
+ $DataView = function DataView(buffer, byteOffset, byteLength){
+ strictNew(this, $DataView, 'DataView');
+ if(!(buffer instanceof $ArrayBuffer))throw TypeError();
+ var bufferLength = buffer._l
+ , offset = toInteger(byteOffset);
+ if(offset < 0 || offset > bufferLength)throw RangeError();
+ byteLength = byteLength === undefined ? bufferLength - offset : toLength(byteLength);
+ if(offset + byteLength > bufferLength)throw RangeError();
+ this._b = buffer;
+ this._o = offset;
+ this._l = byteLength;
+ };
+ addGetter($DataView, 'buffer', '_b');
+ addGetter($DataView, BYTE_LENGTH, '_l');
+ addGetter($DataView, 'byteOffset', '_o');
+ redefineAll($DataView.prototype, {
+ getInt8: function getInt8(byteOffset){
+ return get(this, 1, byteOffset, unpackI8);
+ },
+ getUint8: function getUint8(byteOffset){
+ return get(this, 1, byteOffset, unpackU8);
+ },
+ getInt16: function getInt16(byteOffset /*, littleEndian */){
+ return get(this, 2, byteOffset, unpackI16, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ getUint16: function getUint16(byteOffset /*, littleEndian */){
+ return get(this, 2, byteOffset, unpackU16, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ getInt32: function getInt32(byteOffset /*, littleEndian */){
+ return get(this, 4, byteOffset, unpackI32, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ getUint32: function getUint32(byteOffset /*, littleEndian */){
+ return get(this, 4, byteOffset, unpackU32, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ getFloat32: function getFloat32(byteOffset /*, littleEndian */){
+ return get(this, 4, byteOffset, unpackF32, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ getFloat64: function getFloat64(byteOffset /*, littleEndian */){
+ return get(this, 8, byteOffset, unpackF64, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ setInt8: function setInt8(byteOffset, value){
+ return set(this, 1, byteOffset, packI8, value);
+ },
+ setUint8: function setUint8(byteOffset, value){
+ return set(this, 1, byteOffset, packU8, value);
+ },
+ setInt16: function setInt16(byteOffset, value /*, littleEndian */){
+ return set(this, 2, byteOffset, packI16, value, arguments.length > 2 ? arguments[2] : undefined);
+ },
+ setUint16: function setUint16(byteOffset, value /*, littleEndian */){
+ return set(this, 2, byteOffset, packU16, value, arguments.length > 2 ? arguments[2] : undefined);
+ },
+ setInt32: function setInt32(byteOffset, value /*, littleEndian */){
+ return set(this, 4, byteOffset, packI32, value, arguments.length > 2 ? arguments[2] : undefined);
+ },
+ setUint32: function setUint32(byteOffset, value /*, littleEndian */){
+ return set(this, 4, byteOffset, packU32, value, arguments.length > 2 ? arguments[2] : undefined);
+ },
+ setFloat32: function setFloat32(byteOffset, value /*, littleEndian */){
+ return set(this, 4, byteOffset, packF32, value, arguments.length > 2 ? arguments[2] : undefined);
+ },
+ setFloat64: function setFloat64(byteOffset, value /*, littleEndian */){
+ return set(this, 8, byteOffset, packF64, value, arguments.length > 2 ? arguments[2] : undefined);
+ }
+ });
+}
+require('./$.hide')($DataView.prototype, $typed.VIEW, true);
+module.exports = {
+ ArrayBuffer: $ArrayBuffer,
+ DataView: $DataView
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.classof.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.classof.js
new file mode 100644
index 00000000..905c61f7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.classof.js
@@ -0,0 +1,16 @@
+// getting tag from 19.1.3.6 Object.prototype.toString()
+var cof = require('./$.cof')
+ , TAG = require('./$.wks')('toStringTag')
+ // ES3 wrong here
+ , ARG = cof(function(){ return arguments; }()) == 'Arguments';
+
+module.exports = function(it){
+ var O, T, B;
+ return it === undefined ? 'Undefined' : it === null ? 'Null'
+ // @@toStringTag case
+ : typeof (T = (O = Object(it))[TAG]) == 'string' ? T
+ // builtinTag case
+ : ARG ? cof(O)
+ // ES3 arguments fallback
+ : (B = cof(O)) == 'Object' && typeof O.callee == 'function' ? 'Arguments' : B;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.cof.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.cof.js
new file mode 100644
index 00000000..1dd2779a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.cof.js
@@ -0,0 +1,5 @@
+var toString = {}.toString;
+
+module.exports = function(it){
+ return toString.call(it).slice(8, -1);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.collection-strong.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.collection-strong.js
new file mode 100644
index 00000000..54df55a6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.collection-strong.js
@@ -0,0 +1,159 @@
+'use strict';
+var $ = require('./$')
+ , hide = require('./$.hide')
+ , redefineAll = require('./$.redefine-all')
+ , ctx = require('./$.ctx')
+ , strictNew = require('./$.strict-new')
+ , defined = require('./$.defined')
+ , forOf = require('./$.for-of')
+ , $iterDefine = require('./$.iter-define')
+ , step = require('./$.iter-step')
+ , ID = require('./$.uid')('id')
+ , $has = require('./$.has')
+ , isObject = require('./$.is-object')
+ , setSpecies = require('./$.set-species')
+ , DESCRIPTORS = require('./$.descriptors')
+ , isExtensible = Object.isExtensible || isObject
+ , SIZE = DESCRIPTORS ? '_s' : 'size'
+ , id = 0;
+
+var fastKey = function(it, create){
+ // return primitive with prefix
+ if(!isObject(it))return typeof it == 'symbol' ? it : (typeof it == 'string' ? 'S' : 'P') + it;
+ if(!$has(it, ID)){
+ // can't set id to frozen object
+ if(!isExtensible(it))return 'F';
+ // not necessary to add id
+ if(!create)return 'E';
+ // add missing object id
+ hide(it, ID, ++id);
+ // return object id with prefix
+ } return 'O' + it[ID];
+};
+
+var getEntry = function(that, key){
+ // fast case
+ var index = fastKey(key), entry;
+ if(index !== 'F')return that._i[index];
+ // frozen object case
+ for(entry = that._f; entry; entry = entry.n){
+ if(entry.k == key)return entry;
+ }
+};
+
+module.exports = {
+ getConstructor: function(wrapper, NAME, IS_MAP, ADDER){
+ var C = wrapper(function(that, iterable){
+ strictNew(that, C, NAME);
+ that._i = $.create(null); // index
+ that._f = undefined; // first entry
+ that._l = undefined; // last entry
+ that[SIZE] = 0; // size
+ if(iterable != undefined)forOf(iterable, IS_MAP, that[ADDER], that);
+ });
+ redefineAll(C.prototype, {
+ // 23.1.3.1 Map.prototype.clear()
+ // 23.2.3.2 Set.prototype.clear()
+ clear: function clear(){
+ for(var that = this, data = that._i, entry = that._f; entry; entry = entry.n){
+ entry.r = true;
+ if(entry.p)entry.p = entry.p.n = undefined;
+ delete data[entry.i];
+ }
+ that._f = that._l = undefined;
+ that[SIZE] = 0;
+ },
+ // 23.1.3.3 Map.prototype.delete(key)
+ // 23.2.3.4 Set.prototype.delete(value)
+ 'delete': function(key){
+ var that = this
+ , entry = getEntry(that, key);
+ if(entry){
+ var next = entry.n
+ , prev = entry.p;
+ delete that._i[entry.i];
+ entry.r = true;
+ if(prev)prev.n = next;
+ if(next)next.p = prev;
+ if(that._f == entry)that._f = next;
+ if(that._l == entry)that._l = prev;
+ that[SIZE]--;
+ } return !!entry;
+ },
+ // 23.2.3.6 Set.prototype.forEach(callbackfn, thisArg = undefined)
+ // 23.1.3.5 Map.prototype.forEach(callbackfn, thisArg = undefined)
+ forEach: function forEach(callbackfn /*, that = undefined */){
+ var f = ctx(callbackfn, arguments.length > 1 ? arguments[1] : undefined, 3)
+ , entry;
+ while(entry = entry ? entry.n : this._f){
+ f(entry.v, entry.k, this);
+ // revert to the last existing entry
+ while(entry && entry.r)entry = entry.p;
+ }
+ },
+ // 23.1.3.7 Map.prototype.has(key)
+ // 23.2.3.7 Set.prototype.has(value)
+ has: function has(key){
+ return !!getEntry(this, key);
+ }
+ });
+ if(DESCRIPTORS)$.setDesc(C.prototype, 'size', {
+ get: function(){
+ return defined(this[SIZE]);
+ }
+ });
+ return C;
+ },
+ def: function(that, key, value){
+ var entry = getEntry(that, key)
+ , prev, index;
+ // change existing entry
+ if(entry){
+ entry.v = value;
+ // create new entry
+ } else {
+ that._l = entry = {
+ i: index = fastKey(key, true), // <- index
+ k: key, // <- key
+ v: value, // <- value
+ p: prev = that._l, // <- previous entry
+ n: undefined, // <- next entry
+ r: false // <- removed
+ };
+ if(!that._f)that._f = entry;
+ if(prev)prev.n = entry;
+ that[SIZE]++;
+ // add to index
+ if(index !== 'F')that._i[index] = entry;
+ } return that;
+ },
+ getEntry: getEntry,
+ setStrong: function(C, NAME, IS_MAP){
+ // add .keys, .values, .entries, [@@iterator]
+ // 23.1.3.4, 23.1.3.8, 23.1.3.11, 23.1.3.12, 23.2.3.5, 23.2.3.8, 23.2.3.10, 23.2.3.11
+ $iterDefine(C, NAME, function(iterated, kind){
+ this._t = iterated; // target
+ this._k = kind; // kind
+ this._l = undefined; // previous
+ }, function(){
+ var that = this
+ , kind = that._k
+ , entry = that._l;
+ // revert to the last existing entry
+ while(entry && entry.r)entry = entry.p;
+ // get next entry
+ if(!that._t || !(that._l = entry = entry ? entry.n : that._t._f)){
+ // or finish the iteration
+ that._t = undefined;
+ return step(1);
+ }
+ // return step by kind
+ if(kind == 'keys' )return step(0, entry.k);
+ if(kind == 'values')return step(0, entry.v);
+ return step(0, [entry.k, entry.v]);
+ }, IS_MAP ? 'entries' : 'values' , !IS_MAP, true);
+
+ // add [@@species], 23.1.2.2, 23.2.2.2
+ setSpecies(NAME);
+ }
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.collection-to-json.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.collection-to-json.js
new file mode 100644
index 00000000..41f2e6ec
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.collection-to-json.js
@@ -0,0 +1,11 @@
+// https://github.com/DavidBruant/Map-Set.prototype.toJSON
+var forOf = require('./$.for-of')
+ , classof = require('./$.classof');
+module.exports = function(NAME){
+ return function toJSON(){
+ if(classof(this) != NAME)throw TypeError(NAME + "#toJSON isn't generic");
+ var arr = [];
+ forOf(this, false, arr.push, arr);
+ return arr;
+ };
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.collection-weak.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.collection-weak.js
new file mode 100644
index 00000000..384fb39d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.collection-weak.js
@@ -0,0 +1,86 @@
+'use strict';
+var hide = require('./$.hide')
+ , redefineAll = require('./$.redefine-all')
+ , anObject = require('./$.an-object')
+ , isObject = require('./$.is-object')
+ , strictNew = require('./$.strict-new')
+ , forOf = require('./$.for-of')
+ , createArrayMethod = require('./$.array-methods')
+ , $has = require('./$.has')
+ , WEAK = require('./$.uid')('weak')
+ , isExtensible = Object.isExtensible || isObject
+ , arrayFind = createArrayMethod(5)
+ , arrayFindIndex = createArrayMethod(6)
+ , id = 0;
+
+// fallback for frozen keys
+var frozenStore = function(that){
+ return that._l || (that._l = new FrozenStore);
+};
+var FrozenStore = function(){
+ this.a = [];
+};
+var findFrozen = function(store, key){
+ return arrayFind(store.a, function(it){
+ return it[0] === key;
+ });
+};
+FrozenStore.prototype = {
+ get: function(key){
+ var entry = findFrozen(this, key);
+ if(entry)return entry[1];
+ },
+ has: function(key){
+ return !!findFrozen(this, key);
+ },
+ set: function(key, value){
+ var entry = findFrozen(this, key);
+ if(entry)entry[1] = value;
+ else this.a.push([key, value]);
+ },
+ 'delete': function(key){
+ var index = arrayFindIndex(this.a, function(it){
+ return it[0] === key;
+ });
+ if(~index)this.a.splice(index, 1);
+ return !!~index;
+ }
+};
+
+module.exports = {
+ getConstructor: function(wrapper, NAME, IS_MAP, ADDER){
+ var C = wrapper(function(that, iterable){
+ strictNew(that, C, NAME);
+ that._i = id++; // collection id
+ that._l = undefined; // leak store for frozen objects
+ if(iterable != undefined)forOf(iterable, IS_MAP, that[ADDER], that);
+ });
+ redefineAll(C.prototype, {
+ // 23.3.3.2 WeakMap.prototype.delete(key)
+ // 23.4.3.3 WeakSet.prototype.delete(value)
+ 'delete': function(key){
+ if(!isObject(key))return false;
+ if(!isExtensible(key))return frozenStore(this)['delete'](key);
+ return $has(key, WEAK) && $has(key[WEAK], this._i) && delete key[WEAK][this._i];
+ },
+ // 23.3.3.4 WeakMap.prototype.has(key)
+ // 23.4.3.4 WeakSet.prototype.has(value)
+ has: function has(key){
+ if(!isObject(key))return false;
+ if(!isExtensible(key))return frozenStore(this).has(key);
+ return $has(key, WEAK) && $has(key[WEAK], this._i);
+ }
+ });
+ return C;
+ },
+ def: function(that, key, value){
+ if(!isExtensible(anObject(key))){
+ frozenStore(that).set(key, value);
+ } else {
+ $has(key, WEAK) || hide(key, WEAK, {});
+ key[WEAK][that._i] = value;
+ } return that;
+ },
+ frozenStore: frozenStore,
+ WEAK: WEAK
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.collection.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.collection.js
new file mode 100644
index 00000000..9d234d13
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.collection.js
@@ -0,0 +1,55 @@
+'use strict';
+var $ = require('./$')
+ , global = require('./$.global')
+ , $export = require('./$.export')
+ , fails = require('./$.fails')
+ , hide = require('./$.hide')
+ , redefineAll = require('./$.redefine-all')
+ , forOf = require('./$.for-of')
+ , strictNew = require('./$.strict-new')
+ , isObject = require('./$.is-object')
+ , setToStringTag = require('./$.set-to-string-tag')
+ , DESCRIPTORS = require('./$.descriptors');
+
+module.exports = function(NAME, wrapper, methods, common, IS_MAP, IS_WEAK){
+ var Base = global[NAME]
+ , C = Base
+ , ADDER = IS_MAP ? 'set' : 'add'
+ , proto = C && C.prototype
+ , O = {};
+ if(!DESCRIPTORS || typeof C != 'function' || !(IS_WEAK || proto.forEach && !fails(function(){
+ new C().entries().next();
+ }))){
+ // create collection constructor
+ C = common.getConstructor(wrapper, NAME, IS_MAP, ADDER);
+ redefineAll(C.prototype, methods);
+ } else {
+ C = wrapper(function(target, iterable){
+ strictNew(target, C, NAME);
+ target._c = new Base;
+ if(iterable != undefined)forOf(iterable, IS_MAP, target[ADDER], target);
+ });
+ $.each.call('add,clear,delete,forEach,get,has,set,keys,values,entries'.split(','),function(KEY){
+ var IS_ADDER = KEY == 'add' || KEY == 'set';
+ if(KEY in proto && !(IS_WEAK && KEY == 'clear'))hide(C.prototype, KEY, function(a, b){
+ if(!IS_ADDER && IS_WEAK && !isObject(a))return KEY == 'get' ? undefined : false;
+ var result = this._c[KEY](a === 0 ? 0 : a, b);
+ return IS_ADDER ? this : result;
+ });
+ });
+ if('size' in proto)$.setDesc(C.prototype, 'size', {
+ get: function(){
+ return this._c.size;
+ }
+ });
+ }
+
+ setToStringTag(C, NAME);
+
+ O[NAME] = C;
+ $export($export.G + $export.W + $export.F, O);
+
+ if(!IS_WEAK)common.setStrong(C, NAME, IS_MAP);
+
+ return C;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.core.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.core.js
new file mode 100644
index 00000000..4e2a0b59
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.core.js
@@ -0,0 +1,2 @@
+var core = module.exports = {version: '1.2.6'};
+if(typeof __e == 'number')__e = core; // eslint-disable-line no-undef
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.ctx.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.ctx.js
new file mode 100644
index 00000000..d233574a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.ctx.js
@@ -0,0 +1,20 @@
+// optional / simple context binding
+var aFunction = require('./$.a-function');
+module.exports = function(fn, that, length){
+ aFunction(fn);
+ if(that === undefined)return fn;
+ switch(length){
+ case 1: return function(a){
+ return fn.call(that, a);
+ };
+ case 2: return function(a, b){
+ return fn.call(that, a, b);
+ };
+ case 3: return function(a, b, c){
+ return fn.call(that, a, b, c);
+ };
+ }
+ return function(/* ...args */){
+ return fn.apply(that, arguments);
+ };
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.defined.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.defined.js
new file mode 100644
index 00000000..cfa476b9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.defined.js
@@ -0,0 +1,5 @@
+// 7.2.1 RequireObjectCoercible(argument)
+module.exports = function(it){
+ if(it == undefined)throw TypeError("Can't call method on " + it);
+ return it;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.descriptors.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.descriptors.js
new file mode 100644
index 00000000..9cd47b7d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.descriptors.js
@@ -0,0 +1,4 @@
+// Thank's IE8 for his funny defineProperty
+module.exports = !require('./$.fails')(function(){
+ return Object.defineProperty({}, 'a', {get: function(){ return 7; }}).a != 7;
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.dom-create.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.dom-create.js
new file mode 100644
index 00000000..240842d2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.dom-create.js
@@ -0,0 +1,7 @@
+var isObject = require('./$.is-object')
+ , document = require('./$.global').document
+ // in old IE typeof document.createElement is 'object'
+ , is = isObject(document) && isObject(document.createElement);
+module.exports = function(it){
+ return is ? document.createElement(it) : {};
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.enum-keys.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.enum-keys.js
new file mode 100644
index 00000000..06f7de7a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.enum-keys.js
@@ -0,0 +1,14 @@
+// all enumerable object keys, includes symbols
+var $ = require('./$');
+module.exports = function(it){
+ var keys = $.getKeys(it)
+ , getSymbols = $.getSymbols;
+ if(getSymbols){
+ var symbols = getSymbols(it)
+ , isEnum = $.isEnum
+ , i = 0
+ , key;
+ while(symbols.length > i)if(isEnum.call(it, key = symbols[i++]))keys.push(key);
+ }
+ return keys;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.export.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.export.js
new file mode 100644
index 00000000..507b5a22
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.export.js
@@ -0,0 +1,46 @@
+var global = require('./$.global')
+ , core = require('./$.core')
+ , ctx = require('./$.ctx')
+ , PROTOTYPE = 'prototype';
+
+var $export = function(type, name, source){
+ var IS_FORCED = type & $export.F
+ , IS_GLOBAL = type & $export.G
+ , IS_STATIC = type & $export.S
+ , IS_PROTO = type & $export.P
+ , IS_BIND = type & $export.B
+ , IS_WRAP = type & $export.W
+ , exports = IS_GLOBAL ? core : core[name] || (core[name] = {})
+ , target = IS_GLOBAL ? global : IS_STATIC ? global[name] : (global[name] || {})[PROTOTYPE]
+ , key, own, out;
+ if(IS_GLOBAL)source = name;
+ for(key in source){
+ // contains in native
+ own = !IS_FORCED && target && key in target;
+ if(own && key in exports)continue;
+ // export native or passed
+ out = own ? target[key] : source[key];
+ // prevent global pollution for namespaces
+ exports[key] = IS_GLOBAL && typeof target[key] != 'function' ? source[key]
+ // bind timers to global for call from export context
+ : IS_BIND && own ? ctx(out, global)
+ // wrap global constructors for prevent change them in library
+ : IS_WRAP && target[key] == out ? (function(C){
+ var F = function(param){
+ return this instanceof C ? new C(param) : C(param);
+ };
+ F[PROTOTYPE] = C[PROTOTYPE];
+ return F;
+ // make static versions for prototype methods
+ })(out) : IS_PROTO && typeof out == 'function' ? ctx(Function.call, out) : out;
+ if(IS_PROTO)(exports[PROTOTYPE] || (exports[PROTOTYPE] = {}))[key] = out;
+ }
+};
+// type bitmap
+$export.F = 1; // forced
+$export.G = 2; // global
+$export.S = 4; // static
+$export.P = 8; // proto
+$export.B = 16; // bind
+$export.W = 32; // wrap
+module.exports = $export;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.fails-is-regexp.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.fails-is-regexp.js
new file mode 100644
index 00000000..c459a77a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.fails-is-regexp.js
@@ -0,0 +1,12 @@
+var MATCH = require('./$.wks')('match');
+module.exports = function(KEY){
+ var re = /./;
+ try {
+ '/./'[KEY](re);
+ } catch(e){
+ try {
+ re[MATCH] = false;
+ return !'/./'[KEY](re);
+ } catch(f){ /* empty */ }
+ } return true;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.fails.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.fails.js
new file mode 100644
index 00000000..184e5ea8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.fails.js
@@ -0,0 +1,7 @@
+module.exports = function(exec){
+ try {
+ return !!exec();
+ } catch(e){
+ return true;
+ }
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.fix-re-wks.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.fix-re-wks.js
new file mode 100644
index 00000000..3597a898
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.fix-re-wks.js
@@ -0,0 +1,26 @@
+'use strict';
+var hide = require('./$.hide')
+ , redefine = require('./$.redefine')
+ , fails = require('./$.fails')
+ , defined = require('./$.defined')
+ , wks = require('./$.wks');
+
+module.exports = function(KEY, length, exec){
+ var SYMBOL = wks(KEY)
+ , original = ''[KEY];
+ if(fails(function(){
+ var O = {};
+ O[SYMBOL] = function(){ return 7; };
+ return ''[KEY](O) != 7;
+ })){
+ redefine(String.prototype, KEY, exec(defined, SYMBOL, original));
+ hide(RegExp.prototype, SYMBOL, length == 2
+ // 21.2.5.8 RegExp.prototype[@@replace](string, replaceValue)
+ // 21.2.5.11 RegExp.prototype[@@split](string, limit)
+ ? function(string, arg){ return original.call(string, this, arg); }
+ // 21.2.5.6 RegExp.prototype[@@match](string)
+ // 21.2.5.9 RegExp.prototype[@@search](string)
+ : function(string){ return original.call(string, this); }
+ );
+ }
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.flags.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.flags.js
new file mode 100644
index 00000000..fc20e5de
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.flags.js
@@ -0,0 +1,13 @@
+'use strict';
+// 21.2.5.3 get RegExp.prototype.flags
+var anObject = require('./$.an-object');
+module.exports = function(){
+ var that = anObject(this)
+ , result = '';
+ if(that.global) result += 'g';
+ if(that.ignoreCase) result += 'i';
+ if(that.multiline) result += 'm';
+ if(that.unicode) result += 'u';
+ if(that.sticky) result += 'y';
+ return result;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.for-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.for-of.js
new file mode 100644
index 00000000..0f2d8e97
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.for-of.js
@@ -0,0 +1,19 @@
+var ctx = require('./$.ctx')
+ , call = require('./$.iter-call')
+ , isArrayIter = require('./$.is-array-iter')
+ , anObject = require('./$.an-object')
+ , toLength = require('./$.to-length')
+ , getIterFn = require('./core.get-iterator-method');
+module.exports = function(iterable, entries, fn, that){
+ var iterFn = getIterFn(iterable)
+ , f = ctx(fn, that, entries ? 2 : 1)
+ , index = 0
+ , length, step, iterator;
+ if(typeof iterFn != 'function')throw TypeError(iterable + ' is not iterable!');
+ // fast case for arrays with default iterator
+ if(isArrayIter(iterFn))for(length = toLength(iterable.length); length > index; index++){
+ entries ? f(anObject(step = iterable[index])[0], step[1]) : f(iterable[index]);
+ } else for(iterator = iterFn.call(iterable); !(step = iterator.next()).done; ){
+ call(iterator, f, step.value, entries);
+ }
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.get-names.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.get-names.js
new file mode 100644
index 00000000..28209714
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.get-names.js
@@ -0,0 +1,20 @@
+// fallback for IE11 buggy Object.getOwnPropertyNames with iframe and window
+var toIObject = require('./$.to-iobject')
+ , getNames = require('./$').getNames
+ , toString = {}.toString;
+
+var windowNames = typeof window == 'object' && Object.getOwnPropertyNames
+ ? Object.getOwnPropertyNames(window) : [];
+
+var getWindowNames = function(it){
+ try {
+ return getNames(it);
+ } catch(e){
+ return windowNames.slice();
+ }
+};
+
+module.exports.get = function getOwnPropertyNames(it){
+ if(windowNames && toString.call(it) == '[object Window]')return getWindowNames(it);
+ return getNames(toIObject(it));
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.global.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.global.js
new file mode 100644
index 00000000..df6efb47
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.global.js
@@ -0,0 +1,4 @@
+// https://github.com/zloirock/core-js/issues/86#issuecomment-115759028
+var global = module.exports = typeof window != 'undefined' && window.Math == Math
+ ? window : typeof self != 'undefined' && self.Math == Math ? self : Function('return this')();
+if(typeof __g == 'number')__g = global; // eslint-disable-line no-undef
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.has.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.has.js
new file mode 100644
index 00000000..870b40e7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.has.js
@@ -0,0 +1,4 @@
+var hasOwnProperty = {}.hasOwnProperty;
+module.exports = function(it, key){
+ return hasOwnProperty.call(it, key);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.hide.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.hide.js
new file mode 100644
index 00000000..ba025d81
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.hide.js
@@ -0,0 +1,8 @@
+var $ = require('./$')
+ , createDesc = require('./$.property-desc');
+module.exports = require('./$.descriptors') ? function(object, key, value){
+ return $.setDesc(object, key, createDesc(1, value));
+} : function(object, key, value){
+ object[key] = value;
+ return object;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.html.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.html.js
new file mode 100644
index 00000000..499bd2f3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.html.js
@@ -0,0 +1 @@
+module.exports = require('./$.global').document && document.documentElement;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.invoke.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.invoke.js
new file mode 100644
index 00000000..08e307fd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.invoke.js
@@ -0,0 +1,16 @@
+// fast apply, http://jsperf.lnkit.com/fast-apply/5
+module.exports = function(fn, args, that){
+ var un = that === undefined;
+ switch(args.length){
+ case 0: return un ? fn()
+ : fn.call(that);
+ case 1: return un ? fn(args[0])
+ : fn.call(that, args[0]);
+ case 2: return un ? fn(args[0], args[1])
+ : fn.call(that, args[0], args[1]);
+ case 3: return un ? fn(args[0], args[1], args[2])
+ : fn.call(that, args[0], args[1], args[2]);
+ case 4: return un ? fn(args[0], args[1], args[2], args[3])
+ : fn.call(that, args[0], args[1], args[2], args[3]);
+ } return fn.apply(that, args);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iobject.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iobject.js
new file mode 100644
index 00000000..cea38fab
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iobject.js
@@ -0,0 +1,5 @@
+// fallback for non-array-like ES3 and non-enumerable old V8 strings
+var cof = require('./$.cof');
+module.exports = Object('z').propertyIsEnumerable(0) ? Object : function(it){
+ return cof(it) == 'String' ? it.split('') : Object(it);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.is-array-iter.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.is-array-iter.js
new file mode 100644
index 00000000..b6ef7017
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.is-array-iter.js
@@ -0,0 +1,8 @@
+// check on default Array iterator
+var Iterators = require('./$.iterators')
+ , ITERATOR = require('./$.wks')('iterator')
+ , ArrayProto = Array.prototype;
+
+module.exports = function(it){
+ return it !== undefined && (Iterators.Array === it || ArrayProto[ITERATOR] === it);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.is-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.is-array.js
new file mode 100644
index 00000000..8168b21c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.is-array.js
@@ -0,0 +1,5 @@
+// 7.2.2 IsArray(argument)
+var cof = require('./$.cof');
+module.exports = Array.isArray || function(arg){
+ return cof(arg) == 'Array';
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.is-integer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.is-integer.js
new file mode 100644
index 00000000..b51e1317
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.is-integer.js
@@ -0,0 +1,6 @@
+// 20.1.2.3 Number.isInteger(number)
+var isObject = require('./$.is-object')
+ , floor = Math.floor;
+module.exports = function isInteger(it){
+ return !isObject(it) && isFinite(it) && floor(it) === it;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.is-object.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.is-object.js
new file mode 100644
index 00000000..ee694be2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.is-object.js
@@ -0,0 +1,3 @@
+module.exports = function(it){
+ return typeof it === 'object' ? it !== null : typeof it === 'function';
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.is-regexp.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.is-regexp.js
new file mode 100644
index 00000000..9ea2aad7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.is-regexp.js
@@ -0,0 +1,8 @@
+// 7.2.8 IsRegExp(argument)
+var isObject = require('./$.is-object')
+ , cof = require('./$.cof')
+ , MATCH = require('./$.wks')('match');
+module.exports = function(it){
+ var isRegExp;
+ return isObject(it) && ((isRegExp = it[MATCH]) !== undefined ? !!isRegExp : cof(it) == 'RegExp');
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iter-call.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iter-call.js
new file mode 100644
index 00000000..e6b9d1b1
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iter-call.js
@@ -0,0 +1,12 @@
+// call something on iterator step with safe closing on error
+var anObject = require('./$.an-object');
+module.exports = function(iterator, fn, value, entries){
+ try {
+ return entries ? fn(anObject(value)[0], value[1]) : fn(value);
+ // 7.4.6 IteratorClose(iterator, completion)
+ } catch(e){
+ var ret = iterator['return'];
+ if(ret !== undefined)anObject(ret.call(iterator));
+ throw e;
+ }
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iter-create.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iter-create.js
new file mode 100644
index 00000000..adebcf9a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iter-create.js
@@ -0,0 +1,13 @@
+'use strict';
+var $ = require('./$')
+ , descriptor = require('./$.property-desc')
+ , setToStringTag = require('./$.set-to-string-tag')
+ , IteratorPrototype = {};
+
+// 25.1.2.1.1 %IteratorPrototype%[@@iterator]()
+require('./$.hide')(IteratorPrototype, require('./$.wks')('iterator'), function(){ return this; });
+
+module.exports = function(Constructor, NAME, next){
+ Constructor.prototype = $.create(IteratorPrototype, {next: descriptor(1, next)});
+ setToStringTag(Constructor, NAME + ' Iterator');
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iter-define.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iter-define.js
new file mode 100644
index 00000000..630cdf38
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iter-define.js
@@ -0,0 +1,66 @@
+'use strict';
+var LIBRARY = require('./$.library')
+ , $export = require('./$.export')
+ , redefine = require('./$.redefine')
+ , hide = require('./$.hide')
+ , has = require('./$.has')
+ , Iterators = require('./$.iterators')
+ , $iterCreate = require('./$.iter-create')
+ , setToStringTag = require('./$.set-to-string-tag')
+ , getProto = require('./$').getProto
+ , ITERATOR = require('./$.wks')('iterator')
+ , BUGGY = !([].keys && 'next' in [].keys()) // Safari has buggy iterators w/o `next`
+ , FF_ITERATOR = '@@iterator'
+ , KEYS = 'keys'
+ , VALUES = 'values';
+
+var returnThis = function(){ return this; };
+
+module.exports = function(Base, NAME, Constructor, next, DEFAULT, IS_SET, FORCED){
+ $iterCreate(Constructor, NAME, next);
+ var getMethod = function(kind){
+ if(!BUGGY && kind in proto)return proto[kind];
+ switch(kind){
+ case KEYS: return function keys(){ return new Constructor(this, kind); };
+ case VALUES: return function values(){ return new Constructor(this, kind); };
+ } return function entries(){ return new Constructor(this, kind); };
+ };
+ var TAG = NAME + ' Iterator'
+ , DEF_VALUES = DEFAULT == VALUES
+ , VALUES_BUG = false
+ , proto = Base.prototype
+ , $native = proto[ITERATOR] || proto[FF_ITERATOR] || DEFAULT && proto[DEFAULT]
+ , $default = $native || getMethod(DEFAULT)
+ , methods, key;
+ // Fix native
+ if($native){
+ var IteratorPrototype = getProto($default.call(new Base));
+ // Set @@toStringTag to native iterators
+ setToStringTag(IteratorPrototype, TAG, true);
+ // FF fix
+ if(!LIBRARY && has(proto, FF_ITERATOR))hide(IteratorPrototype, ITERATOR, returnThis);
+ // fix Array#{values, @@iterator}.name in V8 / FF
+ if(DEF_VALUES && $native.name !== VALUES){
+ VALUES_BUG = true;
+ $default = function values(){ return $native.call(this); };
+ }
+ }
+ // Define iterator
+ if((!LIBRARY || FORCED) && (BUGGY || VALUES_BUG || !proto[ITERATOR])){
+ hide(proto, ITERATOR, $default);
+ }
+ // Plug for library
+ Iterators[NAME] = $default;
+ Iterators[TAG] = returnThis;
+ if(DEFAULT){
+ methods = {
+ values: DEF_VALUES ? $default : getMethod(VALUES),
+ keys: IS_SET ? $default : getMethod(KEYS),
+ entries: !DEF_VALUES ? $default : getMethod('entries')
+ };
+ if(FORCED)for(key in methods){
+ if(!(key in proto))redefine(proto, key, methods[key]);
+ } else $export($export.P + $export.F * (BUGGY || VALUES_BUG), NAME, methods);
+ }
+ return methods;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iter-detect.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iter-detect.js
new file mode 100644
index 00000000..bff5fffb
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iter-detect.js
@@ -0,0 +1,21 @@
+var ITERATOR = require('./$.wks')('iterator')
+ , SAFE_CLOSING = false;
+
+try {
+ var riter = [7][ITERATOR]();
+ riter['return'] = function(){ SAFE_CLOSING = true; };
+ Array.from(riter, function(){ throw 2; });
+} catch(e){ /* empty */ }
+
+module.exports = function(exec, skipClosing){
+ if(!skipClosing && !SAFE_CLOSING)return false;
+ var safe = false;
+ try {
+ var arr = [7]
+ , iter = arr[ITERATOR]();
+ iter.next = function(){ return {done: safe = true}; };
+ arr[ITERATOR] = function(){ return iter; };
+ exec(arr);
+ } catch(e){ /* empty */ }
+ return safe;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iter-step.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iter-step.js
new file mode 100644
index 00000000..6ff0dc51
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iter-step.js
@@ -0,0 +1,3 @@
+module.exports = function(done, value){
+ return {value: value, done: !!done};
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iterators.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iterators.js
new file mode 100644
index 00000000..a0995453
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.iterators.js
@@ -0,0 +1 @@
+module.exports = {};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.js
new file mode 100644
index 00000000..053bae48
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.js
@@ -0,0 +1,13 @@
+var $Object = Object;
+module.exports = {
+ create: $Object.create,
+ getProto: $Object.getPrototypeOf,
+ isEnum: {}.propertyIsEnumerable,
+ getDesc: $Object.getOwnPropertyDescriptor,
+ setDesc: $Object.defineProperty,
+ setDescs: $Object.defineProperties,
+ getKeys: $Object.keys,
+ getNames: $Object.getOwnPropertyNames,
+ getSymbols: $Object.getOwnPropertySymbols,
+ each: [].forEach
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.keyof.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.keyof.js
new file mode 100644
index 00000000..09d183a7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.keyof.js
@@ -0,0 +1,10 @@
+var $ = require('./$')
+ , toIObject = require('./$.to-iobject');
+module.exports = function(object, el){
+ var O = toIObject(object)
+ , keys = $.getKeys(O)
+ , length = keys.length
+ , index = 0
+ , key;
+ while(length > index)if(O[key = keys[index++]] === el)return key;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.library.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.library.js
new file mode 100644
index 00000000..73f737c5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.library.js
@@ -0,0 +1 @@
+module.exports = true;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.math-expm1.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.math-expm1.js
new file mode 100644
index 00000000..9d91be93
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.math-expm1.js
@@ -0,0 +1,4 @@
+// 20.2.2.14 Math.expm1(x)
+module.exports = Math.expm1 || function expm1(x){
+ return (x = +x) == 0 ? x : x > -1e-6 && x < 1e-6 ? x + x * x / 2 : Math.exp(x) - 1;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.math-log1p.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.math-log1p.js
new file mode 100644
index 00000000..a92bf463
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.math-log1p.js
@@ -0,0 +1,4 @@
+// 20.2.2.20 Math.log1p(x)
+module.exports = Math.log1p || function log1p(x){
+ return (x = +x) > -1e-8 && x < 1e-8 ? x - x * x / 2 : Math.log(1 + x);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.math-sign.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.math-sign.js
new file mode 100644
index 00000000..a4848df6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.math-sign.js
@@ -0,0 +1,4 @@
+// 20.2.2.28 Math.sign(x)
+module.exports = Math.sign || function sign(x){
+ return (x = +x) == 0 || x != x ? x : x < 0 ? -1 : 1;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.microtask.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.microtask.js
new file mode 100644
index 00000000..1f9ebeb5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.microtask.js
@@ -0,0 +1,64 @@
+var global = require('./$.global')
+ , macrotask = require('./$.task').set
+ , Observer = global.MutationObserver || global.WebKitMutationObserver
+ , process = global.process
+ , Promise = global.Promise
+ , isNode = require('./$.cof')(process) == 'process'
+ , head, last, notify;
+
+var flush = function(){
+ var parent, domain, fn;
+ if(isNode && (parent = process.domain)){
+ process.domain = null;
+ parent.exit();
+ }
+ while(head){
+ domain = head.domain;
+ fn = head.fn;
+ if(domain)domain.enter();
+ fn(); // <- currently we use it only for Promise - try / catch not required
+ if(domain)domain.exit();
+ head = head.next;
+ } last = undefined;
+ if(parent)parent.enter();
+};
+
+// Node.js
+if(isNode){
+ notify = function(){
+ process.nextTick(flush);
+ };
+// browsers with MutationObserver
+} else if(Observer){
+ var toggle = 1
+ , node = document.createTextNode('');
+ new Observer(flush).observe(node, {characterData: true}); // eslint-disable-line no-new
+ notify = function(){
+ node.data = toggle = -toggle;
+ };
+// environments with maybe non-completely correct, but existent Promise
+} else if(Promise && Promise.resolve){
+ notify = function(){
+ Promise.resolve().then(flush);
+ };
+// for other environments - macrotask based on:
+// - setImmediate
+// - MessageChannel
+// - window.postMessag
+// - onreadystatechange
+// - setTimeout
+} else {
+ notify = function(){
+ // strange IE + webpack dev server bug - use .call(global)
+ macrotask.call(global, flush);
+ };
+}
+
+module.exports = function asap(fn){
+ var task = {fn: fn, next: undefined, domain: isNode && process.domain};
+ if(last)last.next = task;
+ if(!head){
+ head = task;
+ notify();
+ } last = task;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.object-assign.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.object-assign.js
new file mode 100644
index 00000000..5ce43f78
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.object-assign.js
@@ -0,0 +1,33 @@
+// 19.1.2.1 Object.assign(target, source, ...)
+var $ = require('./$')
+ , toObject = require('./$.to-object')
+ , IObject = require('./$.iobject');
+
+// should work with symbols and should have deterministic property order (V8 bug)
+module.exports = require('./$.fails')(function(){
+ var a = Object.assign
+ , A = {}
+ , B = {}
+ , S = Symbol()
+ , K = 'abcdefghijklmnopqrst';
+ A[S] = 7;
+ K.split('').forEach(function(k){ B[k] = k; });
+ return a({}, A)[S] != 7 || Object.keys(a({}, B)).join('') != K;
+}) ? function assign(target, source){ // eslint-disable-line no-unused-vars
+ var T = toObject(target)
+ , $$ = arguments
+ , $$len = $$.length
+ , index = 1
+ , getKeys = $.getKeys
+ , getSymbols = $.getSymbols
+ , isEnum = $.isEnum;
+ while($$len > index){
+ var S = IObject($$[index++])
+ , keys = getSymbols ? getKeys(S).concat(getSymbols(S)) : getKeys(S)
+ , length = keys.length
+ , j = 0
+ , key;
+ while(length > j)if(isEnum.call(S, key = keys[j++]))T[key] = S[key];
+ }
+ return T;
+} : Object.assign;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.object-define.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.object-define.js
new file mode 100644
index 00000000..2fff248f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.object-define.js
@@ -0,0 +1,11 @@
+var $ = require('./$')
+ , ownKeys = require('./$.own-keys')
+ , toIObject = require('./$.to-iobject');
+
+module.exports = function define(target, mixin){
+ var keys = ownKeys(toIObject(mixin))
+ , length = keys.length
+ , i = 0, key;
+ while(length > i)$.setDesc(target, key = keys[i++], $.getDesc(mixin, key));
+ return target;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.object-sap.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.object-sap.js
new file mode 100644
index 00000000..5fa7288a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.object-sap.js
@@ -0,0 +1,10 @@
+// most Object methods by ES6 should accept primitives
+var $export = require('./$.export')
+ , core = require('./$.core')
+ , fails = require('./$.fails');
+module.exports = function(KEY, exec){
+ var fn = (core.Object || {})[KEY] || Object[KEY]
+ , exp = {};
+ exp[KEY] = exec(fn);
+ $export($export.S + $export.F * fails(function(){ fn(1); }), 'Object', exp);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.object-to-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.object-to-array.js
new file mode 100644
index 00000000..d46425ba
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.object-to-array.js
@@ -0,0 +1,16 @@
+var $ = require('./$')
+ , toIObject = require('./$.to-iobject')
+ , isEnum = $.isEnum;
+module.exports = function(isEntries){
+ return function(it){
+ var O = toIObject(it)
+ , keys = $.getKeys(O)
+ , length = keys.length
+ , i = 0
+ , result = []
+ , key;
+ while(length > i)if(isEnum.call(O, key = keys[i++])){
+ result.push(isEntries ? [key, O[key]] : O[key]);
+ } return result;
+ };
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.own-keys.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.own-keys.js
new file mode 100644
index 00000000..0218c4bd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.own-keys.js
@@ -0,0 +1,9 @@
+// all object keys, includes non-enumerable and symbols
+var $ = require('./$')
+ , anObject = require('./$.an-object')
+ , Reflect = require('./$.global').Reflect;
+module.exports = Reflect && Reflect.ownKeys || function ownKeys(it){
+ var keys = $.getNames(anObject(it))
+ , getSymbols = $.getSymbols;
+ return getSymbols ? keys.concat(getSymbols(it)) : keys;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.partial.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.partial.js
new file mode 100644
index 00000000..53f97aa9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.partial.js
@@ -0,0 +1,24 @@
+'use strict';
+var path = require('./$.path')
+ , invoke = require('./$.invoke')
+ , aFunction = require('./$.a-function');
+module.exports = function(/* ...pargs */){
+ var fn = aFunction(this)
+ , length = arguments.length
+ , pargs = Array(length)
+ , i = 0
+ , _ = path._
+ , holder = false;
+ while(length > i)if((pargs[i] = arguments[i++]) === _)holder = true;
+ return function(/* ...args */){
+ var that = this
+ , $$ = arguments
+ , $$len = $$.length
+ , j = 0, k = 0, args;
+ if(!holder && !$$len)return invoke(fn, pargs, that);
+ args = pargs.slice();
+ if(holder)for(;length > j; j++)if(args[j] === _)args[j] = $$[k++];
+ while($$len > k)args.push($$[k++]);
+ return invoke(fn, args, that);
+ };
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.path.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.path.js
new file mode 100644
index 00000000..27bb24b8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.path.js
@@ -0,0 +1 @@
+module.exports = require('./$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.property-desc.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.property-desc.js
new file mode 100644
index 00000000..e3f7ab2d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.property-desc.js
@@ -0,0 +1,8 @@
+module.exports = function(bitmap, value){
+ return {
+ enumerable : !(bitmap & 1),
+ configurable: !(bitmap & 2),
+ writable : !(bitmap & 4),
+ value : value
+ };
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.redefine-all.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.redefine-all.js
new file mode 100644
index 00000000..01fe55ba
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.redefine-all.js
@@ -0,0 +1,5 @@
+var redefine = require('./$.redefine');
+module.exports = function(target, src){
+ for(var key in src)redefine(target, key, src[key]);
+ return target;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.redefine.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.redefine.js
new file mode 100644
index 00000000..57453fd1
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.redefine.js
@@ -0,0 +1 @@
+module.exports = require('./$.hide');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.replacer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.replacer.js
new file mode 100644
index 00000000..5360a3d3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.replacer.js
@@ -0,0 +1,8 @@
+module.exports = function(regExp, replace){
+ var replacer = replace === Object(replace) ? function(part){
+ return replace[part];
+ } : replace;
+ return function(it){
+ return String(it).replace(regExp, replacer);
+ };
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.same-value.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.same-value.js
new file mode 100644
index 00000000..8c2b8c7f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.same-value.js
@@ -0,0 +1,4 @@
+// 7.2.9 SameValue(x, y)
+module.exports = Object.is || function is(x, y){
+ return x === y ? x !== 0 || 1 / x === 1 / y : x != x && y != y;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.set-proto.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.set-proto.js
new file mode 100644
index 00000000..b1edd681
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.set-proto.js
@@ -0,0 +1,26 @@
+// Works with __proto__ only. Old v8 can't work with null proto objects.
+/* eslint-disable no-proto */
+var getDesc = require('./$').getDesc
+ , isObject = require('./$.is-object')
+ , anObject = require('./$.an-object');
+var check = function(O, proto){
+ anObject(O);
+ if(!isObject(proto) && proto !== null)throw TypeError(proto + ": can't set as prototype!");
+};
+module.exports = {
+ set: Object.setPrototypeOf || ('__proto__' in {} ? // eslint-disable-line
+ function(test, buggy, set){
+ try {
+ set = require('./$.ctx')(Function.call, getDesc(Object.prototype, '__proto__').set, 2);
+ set(test, []);
+ buggy = !(test instanceof Array);
+ } catch(e){ buggy = true; }
+ return function setPrototypeOf(O, proto){
+ check(O, proto);
+ if(buggy)O.__proto__ = proto;
+ else set(O, proto);
+ return O;
+ };
+ }({}, false) : undefined),
+ check: check
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.set-species.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.set-species.js
new file mode 100644
index 00000000..f6720c36
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.set-species.js
@@ -0,0 +1,13 @@
+'use strict';
+var core = require('./$.core')
+ , $ = require('./$')
+ , DESCRIPTORS = require('./$.descriptors')
+ , SPECIES = require('./$.wks')('species');
+
+module.exports = function(KEY){
+ var C = core[KEY];
+ if(DESCRIPTORS && C && !C[SPECIES])$.setDesc(C, SPECIES, {
+ configurable: true,
+ get: function(){ return this; }
+ });
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.set-to-string-tag.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.set-to-string-tag.js
new file mode 100644
index 00000000..22b34244
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.set-to-string-tag.js
@@ -0,0 +1,7 @@
+var def = require('./$').setDesc
+ , has = require('./$.has')
+ , TAG = require('./$.wks')('toStringTag');
+
+module.exports = function(it, tag, stat){
+ if(it && !has(it = stat ? it : it.prototype, TAG))def(it, TAG, {configurable: true, value: tag});
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.shared.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.shared.js
new file mode 100644
index 00000000..8dea827a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.shared.js
@@ -0,0 +1,6 @@
+var global = require('./$.global')
+ , SHARED = '__core-js_shared__'
+ , store = global[SHARED] || (global[SHARED] = {});
+module.exports = function(key){
+ return store[key] || (store[key] = {});
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.species-constructor.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.species-constructor.js
new file mode 100644
index 00000000..f71168b7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.species-constructor.js
@@ -0,0 +1,8 @@
+// 7.3.20 SpeciesConstructor(O, defaultConstructor)
+var anObject = require('./$.an-object')
+ , aFunction = require('./$.a-function')
+ , SPECIES = require('./$.wks')('species');
+module.exports = function(O, D){
+ var C = anObject(O).constructor, S;
+ return C === undefined || (S = anObject(C)[SPECIES]) == undefined ? D : aFunction(S);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.strict-new.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.strict-new.js
new file mode 100644
index 00000000..8bab9ed1
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.strict-new.js
@@ -0,0 +1,4 @@
+module.exports = function(it, Constructor, name){
+ if(!(it instanceof Constructor))throw TypeError(name + ": use the 'new' operator!");
+ return it;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.string-at.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.string-at.js
new file mode 100644
index 00000000..3d344bba
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.string-at.js
@@ -0,0 +1,17 @@
+var toInteger = require('./$.to-integer')
+ , defined = require('./$.defined');
+// true -> String#at
+// false -> String#codePointAt
+module.exports = function(TO_STRING){
+ return function(that, pos){
+ var s = String(defined(that))
+ , i = toInteger(pos)
+ , l = s.length
+ , a, b;
+ if(i < 0 || i >= l)return TO_STRING ? '' : undefined;
+ a = s.charCodeAt(i);
+ return a < 0xd800 || a > 0xdbff || i + 1 === l || (b = s.charCodeAt(i + 1)) < 0xdc00 || b > 0xdfff
+ ? TO_STRING ? s.charAt(i) : a
+ : TO_STRING ? s.slice(i, i + 2) : (a - 0xd800 << 10) + (b - 0xdc00) + 0x10000;
+ };
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.string-context.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.string-context.js
new file mode 100644
index 00000000..d6485a43
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.string-context.js
@@ -0,0 +1,8 @@
+// helper for String#{startsWith, endsWith, includes}
+var isRegExp = require('./$.is-regexp')
+ , defined = require('./$.defined');
+
+module.exports = function(that, searchString, NAME){
+ if(isRegExp(searchString))throw TypeError('String#' + NAME + " doesn't accept regex!");
+ return String(defined(that));
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.string-pad.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.string-pad.js
new file mode 100644
index 00000000..f0507d93
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.string-pad.js
@@ -0,0 +1,17 @@
+// https://github.com/ljharb/proposal-string-pad-left-right
+var toLength = require('./$.to-length')
+ , repeat = require('./$.string-repeat')
+ , defined = require('./$.defined');
+
+module.exports = function(that, maxLength, fillString, left){
+ var S = String(defined(that))
+ , stringLength = S.length
+ , fillStr = fillString === undefined ? ' ' : String(fillString)
+ , intMaxLength = toLength(maxLength);
+ if(intMaxLength <= stringLength)return S;
+ if(fillStr == '')fillStr = ' ';
+ var fillLen = intMaxLength - stringLength
+ , stringFiller = repeat.call(fillStr, Math.ceil(fillLen / fillStr.length));
+ if(stringFiller.length > fillLen)stringFiller = stringFiller.slice(0, fillLen);
+ return left ? stringFiller + S : S + stringFiller;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.string-repeat.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.string-repeat.js
new file mode 100644
index 00000000..491d0853
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.string-repeat.js
@@ -0,0 +1,12 @@
+'use strict';
+var toInteger = require('./$.to-integer')
+ , defined = require('./$.defined');
+
+module.exports = function repeat(count){
+ var str = String(defined(this))
+ , res = ''
+ , n = toInteger(count);
+ if(n < 0 || n == Infinity)throw RangeError("Count can't be negative");
+ for(;n > 0; (n >>>= 1) && (str += str))if(n & 1)res += str;
+ return res;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.string-trim.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.string-trim.js
new file mode 100644
index 00000000..04423f4d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.string-trim.js
@@ -0,0 +1,29 @@
+var $export = require('./$.export')
+ , defined = require('./$.defined')
+ , fails = require('./$.fails')
+ , spaces = '\x09\x0A\x0B\x0C\x0D\x20\xA0\u1680\u180E\u2000\u2001\u2002\u2003' +
+ '\u2004\u2005\u2006\u2007\u2008\u2009\u200A\u202F\u205F\u3000\u2028\u2029\uFEFF'
+ , space = '[' + spaces + ']'
+ , non = '\u200b\u0085'
+ , ltrim = RegExp('^' + space + space + '*')
+ , rtrim = RegExp(space + space + '*$');
+
+var exporter = function(KEY, exec){
+ var exp = {};
+ exp[KEY] = exec(trim);
+ $export($export.P + $export.F * fails(function(){
+ return !!spaces[KEY]() || non[KEY]() != non;
+ }), 'String', exp);
+};
+
+// 1 -> String#trimLeft
+// 2 -> String#trimRight
+// 3 -> String#trim
+var trim = exporter.trim = function(string, TYPE){
+ string = String(defined(string));
+ if(TYPE & 1)string = string.replace(ltrim, '');
+ if(TYPE & 2)string = string.replace(rtrim, '');
+ return string;
+};
+
+module.exports = exporter;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.task.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.task.js
new file mode 100644
index 00000000..5d7759e6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.task.js
@@ -0,0 +1,75 @@
+var ctx = require('./$.ctx')
+ , invoke = require('./$.invoke')
+ , html = require('./$.html')
+ , cel = require('./$.dom-create')
+ , global = require('./$.global')
+ , process = global.process
+ , setTask = global.setImmediate
+ , clearTask = global.clearImmediate
+ , MessageChannel = global.MessageChannel
+ , counter = 0
+ , queue = {}
+ , ONREADYSTATECHANGE = 'onreadystatechange'
+ , defer, channel, port;
+var run = function(){
+ var id = +this;
+ if(queue.hasOwnProperty(id)){
+ var fn = queue[id];
+ delete queue[id];
+ fn();
+ }
+};
+var listner = function(event){
+ run.call(event.data);
+};
+// Node.js 0.9+ & IE10+ has setImmediate, otherwise:
+if(!setTask || !clearTask){
+ setTask = function setImmediate(fn){
+ var args = [], i = 1;
+ while(arguments.length > i)args.push(arguments[i++]);
+ queue[++counter] = function(){
+ invoke(typeof fn == 'function' ? fn : Function(fn), args);
+ };
+ defer(counter);
+ return counter;
+ };
+ clearTask = function clearImmediate(id){
+ delete queue[id];
+ };
+ // Node.js 0.8-
+ if(require('./$.cof')(process) == 'process'){
+ defer = function(id){
+ process.nextTick(ctx(run, id, 1));
+ };
+ // Browsers with MessageChannel, includes WebWorkers
+ } else if(MessageChannel){
+ channel = new MessageChannel;
+ port = channel.port2;
+ channel.port1.onmessage = listner;
+ defer = ctx(port.postMessage, port, 1);
+ // Browsers with postMessage, skip WebWorkers
+ // IE8 has postMessage, but it's sync & typeof its postMessage is 'object'
+ } else if(global.addEventListener && typeof postMessage == 'function' && !global.importScripts){
+ defer = function(id){
+ global.postMessage(id + '', '*');
+ };
+ global.addEventListener('message', listner, false);
+ // IE8-
+ } else if(ONREADYSTATECHANGE in cel('script')){
+ defer = function(id){
+ html.appendChild(cel('script'))[ONREADYSTATECHANGE] = function(){
+ html.removeChild(this);
+ run.call(id);
+ };
+ };
+ // Rest old browsers
+ } else {
+ defer = function(id){
+ setTimeout(ctx(run, id, 1), 0);
+ };
+ }
+}
+module.exports = {
+ set: setTask,
+ clear: clearTask
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.to-index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.to-index.js
new file mode 100644
index 00000000..9346a8ff
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.to-index.js
@@ -0,0 +1,7 @@
+var toInteger = require('./$.to-integer')
+ , max = Math.max
+ , min = Math.min;
+module.exports = function(index, length){
+ index = toInteger(index);
+ return index < 0 ? max(index + length, 0) : min(index, length);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.to-integer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.to-integer.js
new file mode 100644
index 00000000..f63baaff
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.to-integer.js
@@ -0,0 +1,6 @@
+// 7.1.4 ToInteger
+var ceil = Math.ceil
+ , floor = Math.floor;
+module.exports = function(it){
+ return isNaN(it = +it) ? 0 : (it > 0 ? floor : ceil)(it);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.to-iobject.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.to-iobject.js
new file mode 100644
index 00000000..fcf54c82
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.to-iobject.js
@@ -0,0 +1,6 @@
+// to indexed object, toObject with fallback for non-array-like ES3 strings
+var IObject = require('./$.iobject')
+ , defined = require('./$.defined');
+module.exports = function(it){
+ return IObject(defined(it));
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.to-length.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.to-length.js
new file mode 100644
index 00000000..0e15b1b8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.to-length.js
@@ -0,0 +1,6 @@
+// 7.1.15 ToLength
+var toInteger = require('./$.to-integer')
+ , min = Math.min;
+module.exports = function(it){
+ return it > 0 ? min(toInteger(it), 0x1fffffffffffff) : 0; // pow(2, 53) - 1 == 9007199254740991
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.to-object.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.to-object.js
new file mode 100644
index 00000000..2c57a29f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.to-object.js
@@ -0,0 +1,5 @@
+// 7.1.13 ToObject(argument)
+var defined = require('./$.defined');
+module.exports = function(it){
+ return Object(defined(it));
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.to-primitive.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.to-primitive.js
new file mode 100644
index 00000000..6fb4585a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.to-primitive.js
@@ -0,0 +1,12 @@
+// 7.1.1 ToPrimitive(input [, PreferredType])
+var isObject = require('./$.is-object');
+// instead of the ES6 spec version, we didn't implement @@toPrimitive case
+// and the second argument - flag - preferred type is a string
+module.exports = function(it, S){
+ if(!isObject(it))return it;
+ var fn, val;
+ if(S && typeof (fn = it.toString) == 'function' && !isObject(val = fn.call(it)))return val;
+ if(typeof (fn = it.valueOf) == 'function' && !isObject(val = fn.call(it)))return val;
+ if(!S && typeof (fn = it.toString) == 'function' && !isObject(val = fn.call(it)))return val;
+ throw TypeError("Can't convert object to primitive value");
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.typed-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.typed-array.js
new file mode 100644
index 00000000..1bd5cf0e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.typed-array.js
@@ -0,0 +1,365 @@
+'use strict';
+if(require('./$.descriptors')){
+ var LIBRARY = require('./$.library')
+ , global = require('./$.global')
+ , $ = require('./$')
+ , fails = require('./$.fails')
+ , $export = require('./$.export')
+ , $typed = require('./$.typed')
+ , $buffer = require('./$.buffer')
+ , ctx = require('./$.ctx')
+ , strictNew = require('./$.strict-new')
+ , propertyDesc = require('./$.property-desc')
+ , hide = require('./$.hide')
+ , redefineAll = require('./$.redefine-all')
+ , isInteger = require('./$.is-integer')
+ , toInteger = require('./$.to-integer')
+ , toLength = require('./$.to-length')
+ , toIndex = require('./$.to-index')
+ , toPrimitive = require('./$.to-primitive')
+ , isObject = require('./$.is-object')
+ , toObject = require('./$.to-object')
+ , isArrayIter = require('./$.is-array-iter')
+ , isIterable = require('./core.is-iterable')
+ , getIterFn = require('./core.get-iterator-method')
+ , wks = require('./$.wks')
+ , createArrayMethod = require('./$.array-methods')
+ , createArrayIncludes = require('./$.array-includes')
+ , speciesConstructor = require('./$.species-constructor')
+ , ArrayIterators = require('./es6.array.iterator')
+ , Iterators = require('./$.iterators')
+ , $iterDetect = require('./$.iter-detect')
+ , setSpecies = require('./$.set-species')
+ , arrayFill = require('./$.array-fill')
+ , arrayCopyWithin = require('./$.array-copy-within')
+ , ArrayProto = Array.prototype
+ , $ArrayBuffer = $buffer.ArrayBuffer
+ , $DataView = $buffer.DataView
+ , setDesc = $.setDesc
+ , getDesc = $.getDesc
+ , arrayForEach = createArrayMethod(0)
+ , arrayMap = createArrayMethod(1)
+ , arrayFilter = createArrayMethod(2)
+ , arraySome = createArrayMethod(3)
+ , arrayEvery = createArrayMethod(4)
+ , arrayFind = createArrayMethod(5)
+ , arrayFindIndex = createArrayMethod(6)
+ , arrayIncludes = createArrayIncludes(true)
+ , arrayIndexOf = createArrayIncludes(false)
+ , arrayValues = ArrayIterators.values
+ , arrayKeys = ArrayIterators.keys
+ , arrayEntries = ArrayIterators.entries
+ , arrayLastIndexOf = ArrayProto.lastIndexOf
+ , arrayReduce = ArrayProto.reduce
+ , arrayReduceRight = ArrayProto.reduceRight
+ , arrayJoin = ArrayProto.join
+ , arrayReverse = ArrayProto.reverse
+ , arraySort = ArrayProto.sort
+ , arraySlice = ArrayProto.slice
+ , arrayToString = ArrayProto.toString
+ , arrayToLocaleString = ArrayProto.toLocaleString
+ , ITERATOR = wks('iterator')
+ , TAG = wks('toStringTag')
+ , TYPED_CONSTRUCTOR = wks('typed_constructor')
+ , DEF_CONSTRUCTOR = wks('def_constructor')
+ , ALL_ARRAYS = $typed.ARRAYS
+ , TYPED_ARRAY = $typed.TYPED
+ , VIEW = $typed.VIEW
+ , BYTES_PER_ELEMENT = 'BYTES_PER_ELEMENT';
+
+ var LITTLE_ENDIAN = fails(function(){
+ return new Uint8Array(new Uint16Array([1]).buffer)[0] === 1;
+ });
+
+ var validate = function(it){
+ if(isObject(it) && TYPED_ARRAY in it)return it;
+ throw TypeError(it + ' is not a typed array!');
+ };
+
+ var fromList = function(O, list){
+ var index = 0
+ , length = list.length
+ , result = allocate(speciesConstructor(O, O[DEF_CONSTRUCTOR]), length);
+ while(length > index)result[index] = list[index++];
+ return result;
+ };
+
+ var allocate = function(C, length){
+ if(!(isObject(C) && TYPED_CONSTRUCTOR in C)){
+ throw TypeError('It is not a typed array constructor!');
+ } return new C(length);
+ };
+
+ var $from = function from(source /*, mapfn, thisArg */){
+ var O = toObject(source)
+ , $$ = arguments
+ , $$len = $$.length
+ , mapfn = $$len > 1 ? $$[1] : undefined
+ , mapping = mapfn !== undefined
+ , iterFn = getIterFn(O)
+ , i, length, values, result, step, iterator;
+ if(iterFn != undefined && !isArrayIter(iterFn)){
+ for(iterator = iterFn.call(O), values = [], i = 0; !(step = iterator.next()).done; i++){
+ values.push(step.value);
+ } O = values;
+ }
+ if(mapping && $$len > 2)mapfn = ctx(mapfn, $$[2], 2);
+ for(i = 0, length = toLength(O.length), result = allocate(this, length); length > i; i++){
+ result[i] = mapping ? mapfn(O[i], i) : O[i];
+ }
+ return result;
+ };
+
+ var addGetter = function(C, key, internal){
+ setDesc(C.prototype, key, {get: function(){ return this._d[internal]; }});
+ };
+
+ var $of = function of(/*...items*/){
+ var index = 0
+ , length = arguments.length
+ , result = allocate(this, length);
+ while(length > index)result[index] = arguments[index++];
+ return result;
+ };
+ var $toLocaleString = function toLocaleString(){
+ return arrayToLocaleString.apply(validate(this), arguments);
+ };
+
+ var proto = {
+ copyWithin: function copyWithin(target, start /*, end */){
+ return arrayCopyWithin.call(validate(this), target, start, arguments.length > 2 ? arguments[2] : undefined);
+ },
+ every: function every(callbackfn /*, thisArg */){
+ return arrayEvery(validate(this), callbackfn, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ fill: function fill(value /*, start, end */){ // eslint-disable-line no-unused-vars
+ return arrayFill.apply(validate(this), arguments);
+ },
+ filter: function filter(callbackfn /*, thisArg */){
+ return fromList(this, arrayFilter(validate(this), callbackfn, arguments.length > 1 ? arguments[1] : undefined));
+ },
+ find: function find(predicate /*, thisArg */){
+ return arrayFind(validate(this), predicate, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ findIndex: function findIndex(predicate /*, thisArg */){
+ return arrayFindIndex(validate(this), predicate, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ forEach: function forEach(callbackfn /*, thisArg */){
+ arrayForEach(validate(this), callbackfn, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ indexOf: function indexOf(searchElement /*, fromIndex */){
+ return arrayIndexOf(validate(this), searchElement, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ includes: function includes(searchElement /*, fromIndex */){
+ return arrayIncludes(validate(this), searchElement, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ join: function join(separator){ // eslint-disable-line no-unused-vars
+ return arrayJoin.apply(validate(this), arguments);
+ },
+ lastIndexOf: function lastIndexOf(searchElement /*, fromIndex */){ // eslint-disable-line no-unused-vars
+ return arrayLastIndexOf.apply(validate(this), arguments);
+ },
+ map: function map(mapfn /*, thisArg */){
+ return fromList(this, arrayMap(validate(this), mapfn, arguments.length > 1 ? arguments[1] : undefined)); // TODO
+ },
+ reduce: function reduce(callbackfn /*, initialValue */){ // eslint-disable-line no-unused-vars
+ return arrayReduce.apply(validate(this), arguments);
+ },
+ reduceRight: function reduceRight(callbackfn /*, initialValue */){ // eslint-disable-line no-unused-vars
+ return arrayReduceRight.apply(validate(this), arguments);
+ },
+ reverse: function reverse(){
+ return arrayReverse.call(validate(this));
+ },
+ set: function set(arrayLike /*, offset */){
+ validate(this);
+ var offset = toInteger(arguments.length > 1 ? arguments[1] : undefined);
+ if(offset < 0)throw RangeError();
+ var length = this.length;
+ var src = toObject(arrayLike);
+ var index = 0;
+ var len = toLength(src.length);
+ if(len + offset > length)throw RangeError();
+ while(index < len)this[offset + index] = src[index++];
+ },
+ slice: function slice(start, end){
+ return fromList(this, arraySlice.call(validate(this), start, end)); // TODO
+ },
+ some: function some(callbackfn /*, thisArg */){
+ return arraySome(validate(this), callbackfn, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ sort: function sort(comparefn){
+ return arraySort.call(validate(this), comparefn);
+ },
+ subarray: function subarray(begin, end){
+ var O = validate(this)
+ , length = O.length
+ , $begin = toIndex(begin, length);
+ return new (speciesConstructor(O, O[DEF_CONSTRUCTOR]))(
+ O.buffer,
+ O.byteOffset + $begin * O.BYTES_PER_ELEMENT,
+ toLength((end === undefined ? length : toIndex(end, length)) - $begin)
+ );
+ },
+ entries: function entries(){
+ return arrayEntries.call(validate(this));
+ },
+ keys: function keys(){
+ return arrayKeys.call(validate(this));
+ },
+ values: function values(){
+ return arrayValues.call(validate(this));
+ }
+ };
+
+ var isTAIndex = function(target, key){
+ return isObject(target)
+ && TYPED_ARRAY in target
+ && typeof key != 'symbol'
+ && key in target
+ && String(+key) == String(key);
+ };
+ var $getDesc = function getOwnPropertyDescriptor(target, key){
+ return isTAIndex(target, key = toPrimitive(key, true))
+ ? propertyDesc(2, target[key])
+ : getDesc(target, key);
+ };
+ var $setDesc = function defineProperty(target, key, desc){
+ if(isTAIndex(target, key = toPrimitive(key, true)) && isObject(desc)){
+ if('value' in desc)target[key] = desc.value;
+ return target;
+ } else return setDesc(target, key, desc);
+ };
+
+ if(!ALL_ARRAYS){
+ $.getDesc = $getDesc;
+ $.setDesc = $setDesc;
+ }
+
+ $export($export.S + $export.F * !ALL_ARRAYS, 'Object', {
+ getOwnPropertyDescriptor: $getDesc,
+ defineProperty: $setDesc
+ });
+
+ var $TypedArrayPrototype$ = redefineAll({}, proto);
+ redefineAll($TypedArrayPrototype$, {
+ constructor: function(){ /* noop */ },
+ toString: arrayToString,
+ toLocaleString: $toLocaleString
+ });
+ $.setDesc($TypedArrayPrototype$, TAG, {
+ get: function(){ return this[TYPED_ARRAY]; }
+ });
+
+ module.exports = function(KEY, BYTES, wrapper, CLAMPED){
+ CLAMPED = !!CLAMPED;
+ var NAME = KEY + (CLAMPED ? 'Clamped' : '') + 'Array'
+ , GETTER = 'get' + KEY
+ , SETTER = 'set' + KEY
+ , TypedArray = global[NAME]
+ , Base = TypedArray || {}
+ , FORCED = !TypedArray || !$typed.ABV
+ , $iterator = proto.values
+ , O = {};
+ var addElement = function(that, index){
+ setDesc(that, index, {
+ get: function(){
+ var data = this._d;
+ return data.v[GETTER](index * BYTES + data.o, LITTLE_ENDIAN);
+ },
+ set: function(it){
+ var data = this._d;
+ if(CLAMPED)it = (it = Math.round(it)) < 0 ? 0 : it > 0xff ? 0xff : it & 0xff;
+ data.v[SETTER](index * BYTES + data.o, it, LITTLE_ENDIAN);
+ },
+ enumerable: true
+ });
+ };
+ if(!$ArrayBuffer)return;
+ if(FORCED){
+ TypedArray = wrapper(function(that, data, $offset, $length){
+ strictNew(that, TypedArray, NAME);
+ var index = 0
+ , offset = 0
+ , buffer, byteLength, length;
+ if(!isObject(data)){
+ byteLength = toInteger(data) * BYTES;
+ buffer = new $ArrayBuffer(byteLength);
+ // TODO TA case
+ } else if(data instanceof $ArrayBuffer){
+ buffer = data;
+ offset = toInteger($offset);
+ if(offset < 0 || offset % BYTES)throw RangeError();
+ var $len = data.byteLength;
+ if($length === undefined){
+ if($len % BYTES)throw RangeError();
+ byteLength = $len - offset;
+ if(byteLength < 0)throw RangeError();
+ } else {
+ byteLength = toLength($length) * BYTES;
+ if(byteLength + offset > $len)throw RangeError();
+ }
+ } else return $from.call(TypedArray, data);
+ length = byteLength / BYTES;
+ hide(that, '_d', {
+ b: buffer,
+ o: offset,
+ l: byteLength,
+ e: length,
+ v: new $DataView(buffer)
+ });
+ while(index < length)addElement(that, index++);
+ });
+ TypedArray.prototype = $.create($TypedArrayPrototype$);
+ addGetter(TypedArray, 'buffer', 'b');
+ addGetter(TypedArray, 'byteOffset', 'o');
+ addGetter(TypedArray, 'byteLength', 'l');
+ addGetter(TypedArray, 'length', 'e');
+ hide(TypedArray, BYTES_PER_ELEMENT, BYTES);
+ hide(TypedArray.prototype, BYTES_PER_ELEMENT, BYTES);
+ hide(TypedArray.prototype, 'constructor', TypedArray);
+ } else if(!$iterDetect(function(iter){
+ new TypedArray(iter); // eslint-disable-line no-new
+ }, true)){
+ TypedArray = wrapper(function(that, data, $offset, $length){
+ strictNew(that, TypedArray, NAME);
+ if(isObject(data) && isIterable(data))return $from.call(TypedArray, data);
+ return $length === undefined ? new Base(data, $offset) : new Base(data, $offset, $length);
+ });
+ TypedArray.prototype = Base.prototype;
+ if(!LIBRARY)TypedArray.prototype.constructor = TypedArray;
+ }
+ var TypedArrayPrototype = TypedArray.prototype;
+ var $nativeIterator = TypedArrayPrototype[ITERATOR];
+ hide(TypedArray, TYPED_CONSTRUCTOR, true);
+ hide(TypedArrayPrototype, TYPED_ARRAY, NAME);
+ hide(TypedArrayPrototype, VIEW, true);
+ hide(TypedArrayPrototype, DEF_CONSTRUCTOR, TypedArray);
+ TAG in TypedArrayPrototype || $.setDesc(TypedArrayPrototype, TAG, {
+ get: function(){ return NAME; }
+ });
+
+ O[NAME] = TypedArray;
+
+ $export($export.G + $export.W + $export.F * (TypedArray != Base), O);
+
+ $export($export.S + $export.F * (TypedArray != Base), NAME, {
+ BYTES_PER_ELEMENT: BYTES,
+ from: Base.from || $from,
+ of: Base.of || $of
+ });
+
+ $export($export.P + $export.F * FORCED, NAME, proto);
+
+ $export($export.P + $export.F * (TypedArrayPrototype.toString != arrayToString), NAME, {toString: arrayToString});
+
+ $export($export.P + $export.F * fails(function(){
+ return [1, 2].toLocaleString() != new Typed([1, 2]).toLocaleString()
+ }), NAME, {toLocaleString: $toLocaleString});
+
+ Iterators[NAME] = $nativeIterator || $iterator;
+ LIBRARY || $nativeIterator || hide(TypedArrayPrototype, ITERATOR, $iterator);
+
+ setSpecies(NAME);
+ };
+} else module.exports = function(){ /* empty */};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.typed.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.typed.js
new file mode 100644
index 00000000..ced24126
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.typed.js
@@ -0,0 +1,36 @@
+var global = require('./$.global')
+ , hide = require('./$.hide')
+ , uid = require('./$.uid')
+ , TYPED = uid('typed_array')
+ , VIEW = uid('view')
+ , ABV = !!(global.ArrayBuffer && global.DataView)
+ , ARRAYS = true
+ , i = 0, l = 9;
+
+var TypedArrayConstructors = [
+ 'Int8Array',
+ 'Uint8Array',
+ 'Uint8ClampedArray',
+ 'Int16Array',
+ 'Uint16Array',
+ 'Int32Array',
+ 'Uint32Array',
+ 'Float32Array',
+ 'Float64Array'
+];
+
+while(i < l){
+ var Typed = global[TypedArrayConstructors[i++]];
+ if(Typed){
+ hide(Typed.prototype, TYPED, true);
+ hide(Typed.prototype, VIEW, true);
+ } else ARRAYS = false;
+}
+
+module.exports = {
+ ARRAYS: ARRAYS,
+ ABV: ABV,
+ CONSTR: ARRAYS && ABV,
+ TYPED: TYPED,
+ VIEW: VIEW
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.uid.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.uid.js
new file mode 100644
index 00000000..3be4196b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.uid.js
@@ -0,0 +1,5 @@
+var id = 0
+ , px = Math.random();
+module.exports = function(key){
+ return 'Symbol('.concat(key === undefined ? '' : key, ')_', (++id + px).toString(36));
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.wks.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.wks.js
new file mode 100644
index 00000000..87a3d29a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/$.wks.js
@@ -0,0 +1,7 @@
+var store = require('./$.shared')('wks')
+ , uid = require('./$.uid')
+ , Symbol = require('./$.global').Symbol;
+module.exports = function(name){
+ return store[name] || (store[name] =
+ Symbol && Symbol[name] || (Symbol || uid)('Symbol.' + name));
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.delay.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.delay.js
new file mode 100644
index 00000000..3e19ef39
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.delay.js
@@ -0,0 +1,12 @@
+var global = require('./$.global')
+ , core = require('./$.core')
+ , $export = require('./$.export')
+ , partial = require('./$.partial');
+// https://esdiscuss.org/topic/promise-returning-delay-function
+$export($export.G + $export.F, {
+ delay: function delay(time){
+ return new (core.Promise || global.Promise)(function(resolve){
+ setTimeout(partial.call(resolve, true), time);
+ });
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.dict.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.dict.js
new file mode 100644
index 00000000..df314988
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.dict.js
@@ -0,0 +1,153 @@
+'use strict';
+var $ = require('./$')
+ , ctx = require('./$.ctx')
+ , $export = require('./$.export')
+ , createDesc = require('./$.property-desc')
+ , assign = require('./$.object-assign')
+ , keyOf = require('./$.keyof')
+ , aFunction = require('./$.a-function')
+ , forOf = require('./$.for-of')
+ , isIterable = require('./core.is-iterable')
+ , $iterCreate = require('./$.iter-create')
+ , step = require('./$.iter-step')
+ , isObject = require('./$.is-object')
+ , toIObject = require('./$.to-iobject')
+ , DESCRIPTORS = require('./$.descriptors')
+ , has = require('./$.has')
+ , getKeys = $.getKeys;
+
+// 0 -> Dict.forEach
+// 1 -> Dict.map
+// 2 -> Dict.filter
+// 3 -> Dict.some
+// 4 -> Dict.every
+// 5 -> Dict.find
+// 6 -> Dict.findKey
+// 7 -> Dict.mapPairs
+var createDictMethod = function(TYPE){
+ var IS_MAP = TYPE == 1
+ , IS_EVERY = TYPE == 4;
+ return function(object, callbackfn, that /* = undefined */){
+ var f = ctx(callbackfn, that, 3)
+ , O = toIObject(object)
+ , result = IS_MAP || TYPE == 7 || TYPE == 2
+ ? new (typeof this == 'function' ? this : Dict) : undefined
+ , key, val, res;
+ for(key in O)if(has(O, key)){
+ val = O[key];
+ res = f(val, key, object);
+ if(TYPE){
+ if(IS_MAP)result[key] = res; // map
+ else if(res)switch(TYPE){
+ case 2: result[key] = val; break; // filter
+ case 3: return true; // some
+ case 5: return val; // find
+ case 6: return key; // findKey
+ case 7: result[res[0]] = res[1]; // mapPairs
+ } else if(IS_EVERY)return false; // every
+ }
+ }
+ return TYPE == 3 || IS_EVERY ? IS_EVERY : result;
+ };
+};
+var findKey = createDictMethod(6);
+
+var createDictIter = function(kind){
+ return function(it){
+ return new DictIterator(it, kind);
+ };
+};
+var DictIterator = function(iterated, kind){
+ this._t = toIObject(iterated); // target
+ this._a = getKeys(iterated); // keys
+ this._i = 0; // next index
+ this._k = kind; // kind
+};
+$iterCreate(DictIterator, 'Dict', function(){
+ var that = this
+ , O = that._t
+ , keys = that._a
+ , kind = that._k
+ , key;
+ do {
+ if(that._i >= keys.length){
+ that._t = undefined;
+ return step(1);
+ }
+ } while(!has(O, key = keys[that._i++]));
+ if(kind == 'keys' )return step(0, key);
+ if(kind == 'values')return step(0, O[key]);
+ return step(0, [key, O[key]]);
+});
+
+function Dict(iterable){
+ var dict = $.create(null);
+ if(iterable != undefined){
+ if(isIterable(iterable)){
+ forOf(iterable, true, function(key, value){
+ dict[key] = value;
+ });
+ } else assign(dict, iterable);
+ }
+ return dict;
+}
+Dict.prototype = null;
+
+function reduce(object, mapfn, init){
+ aFunction(mapfn);
+ var O = toIObject(object)
+ , keys = getKeys(O)
+ , length = keys.length
+ , i = 0
+ , memo, key;
+ if(arguments.length < 3){
+ if(!length)throw TypeError('Reduce of empty object with no initial value');
+ memo = O[keys[i++]];
+ } else memo = Object(init);
+ while(length > i)if(has(O, key = keys[i++])){
+ memo = mapfn(memo, O[key], key, object);
+ }
+ return memo;
+}
+
+function includes(object, el){
+ return (el == el ? keyOf(object, el) : findKey(object, function(it){
+ return it != it;
+ })) !== undefined;
+}
+
+function get(object, key){
+ if(has(object, key))return object[key];
+}
+function set(object, key, value){
+ if(DESCRIPTORS && key in Object)$.setDesc(object, key, createDesc(0, value));
+ else object[key] = value;
+ return object;
+}
+
+function isDict(it){
+ return isObject(it) && $.getProto(it) === Dict.prototype;
+}
+
+$export($export.G + $export.F, {Dict: Dict});
+
+$export($export.S, 'Dict', {
+ keys: createDictIter('keys'),
+ values: createDictIter('values'),
+ entries: createDictIter('entries'),
+ forEach: createDictMethod(0),
+ map: createDictMethod(1),
+ filter: createDictMethod(2),
+ some: createDictMethod(3),
+ every: createDictMethod(4),
+ find: createDictMethod(5),
+ findKey: findKey,
+ mapPairs: createDictMethod(7),
+ reduce: reduce,
+ keyOf: keyOf,
+ includes: includes,
+ has: has,
+ get: get,
+ set: set,
+ isDict: isDict
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.function.part.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.function.part.js
new file mode 100644
index 00000000..9943b307
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.function.part.js
@@ -0,0 +1,7 @@
+var path = require('./$.path')
+ , $export = require('./$.export');
+
+// Placeholder
+require('./$.core')._ = path._ = path._ || {};
+
+$export($export.P + $export.F, 'Function', {part: require('./$.partial')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.get-iterator-method.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.get-iterator-method.js
new file mode 100644
index 00000000..02db743c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.get-iterator-method.js
@@ -0,0 +1,8 @@
+var classof = require('./$.classof')
+ , ITERATOR = require('./$.wks')('iterator')
+ , Iterators = require('./$.iterators');
+module.exports = require('./$.core').getIteratorMethod = function(it){
+ if(it != undefined)return it[ITERATOR]
+ || it['@@iterator']
+ || Iterators[classof(it)];
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.get-iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.get-iterator.js
new file mode 100644
index 00000000..7290904a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.get-iterator.js
@@ -0,0 +1,7 @@
+var anObject = require('./$.an-object')
+ , get = require('./core.get-iterator-method');
+module.exports = require('./$.core').getIterator = function(it){
+ var iterFn = get(it);
+ if(typeof iterFn != 'function')throw TypeError(it + ' is not iterable!');
+ return anObject(iterFn.call(it));
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.is-iterable.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.is-iterable.js
new file mode 100644
index 00000000..c27e6588
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.is-iterable.js
@@ -0,0 +1,9 @@
+var classof = require('./$.classof')
+ , ITERATOR = require('./$.wks')('iterator')
+ , Iterators = require('./$.iterators');
+module.exports = require('./$.core').isIterable = function(it){
+ var O = Object(it);
+ return O[ITERATOR] !== undefined
+ || '@@iterator' in O
+ || Iterators.hasOwnProperty(classof(O));
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.log.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.log.js
new file mode 100644
index 00000000..4c0ea53d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.log.js
@@ -0,0 +1,26 @@
+var $ = require('./$')
+ , global = require('./$.global')
+ , $export = require('./$.export')
+ , log = {}
+ , enabled = true;
+// Methods from https://github.com/DeveloperToolsWG/console-object/blob/master/api.md
+$.each.call((
+ 'assert,clear,count,debug,dir,dirxml,error,exception,group,groupCollapsed,groupEnd,' +
+ 'info,isIndependentlyComposed,log,markTimeline,profile,profileEnd,table,' +
+ 'time,timeEnd,timeline,timelineEnd,timeStamp,trace,warn'
+).split(','), function(key){
+ log[key] = function(){
+ var $console = global.console;
+ if(enabled && $console && $console[key]){
+ return Function.apply.call($console[key], $console, arguments);
+ }
+ };
+});
+$export($export.G + $export.F, {log: require('./$.object-assign')(log.log, log, {
+ enable: function(){
+ enabled = true;
+ },
+ disable: function(){
+ enabled = false;
+ }
+})});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.number.iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.number.iterator.js
new file mode 100644
index 00000000..d9273780
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.number.iterator.js
@@ -0,0 +1,9 @@
+'use strict';
+require('./$.iter-define')(Number, 'Number', function(iterated){
+ this._l = +iterated;
+ this._i = 0;
+}, function(){
+ var i = this._i++
+ , done = !(i < this._l);
+ return {done: done, value: done ? undefined : i};
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.object.classof.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.object.classof.js
new file mode 100644
index 00000000..df682e44
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.object.classof.js
@@ -0,0 +1,3 @@
+var $export = require('./$.export');
+
+$export($export.S + $export.F, 'Object', {classof: require('./$.classof')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.object.define.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.object.define.js
new file mode 100644
index 00000000..fe4cbe9b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.object.define.js
@@ -0,0 +1,4 @@
+var $export = require('./$.export')
+ , define = require('./$.object-define');
+
+$export($export.S + $export.F, 'Object', {define: define});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.object.is-object.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.object.is-object.js
new file mode 100644
index 00000000..c60a977a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.object.is-object.js
@@ -0,0 +1,3 @@
+var $export = require('./$.export');
+
+$export($export.S + $export.F, 'Object', {isObject: require('./$.is-object')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.object.make.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.object.make.js
new file mode 100644
index 00000000..95b99961
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.object.make.js
@@ -0,0 +1,9 @@
+var $export = require('./$.export')
+ , define = require('./$.object-define')
+ , create = require('./$').create;
+
+$export($export.S + $export.F, 'Object', {
+ make: function(proto, mixin){
+ return define(create(proto), mixin);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.string.escape-html.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.string.escape-html.js
new file mode 100644
index 00000000..81737e75
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.string.escape-html.js
@@ -0,0 +1,11 @@
+'use strict';
+var $export = require('./$.export');
+var $re = require('./$.replacer')(/[&<>"']/g, {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ "'": '''
+});
+
+$export($export.P + $export.F, 'String', {escapeHTML: function escapeHTML(){ return $re(this); }});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.string.unescape-html.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.string.unescape-html.js
new file mode 100644
index 00000000..9d7a3d2c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/core.string.unescape-html.js
@@ -0,0 +1,11 @@
+'use strict';
+var $export = require('./$.export');
+var $re = require('./$.replacer')(/&(?:amp|lt|gt|quot|apos);/g, {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ ''': "'"
+});
+
+$export($export.P + $export.F, 'String', {unescapeHTML: function unescapeHTML(){ return $re(this); }});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es5.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es5.js
new file mode 100644
index 00000000..50f72b04
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es5.js
@@ -0,0 +1,276 @@
+'use strict';
+var $ = require('./$')
+ , $export = require('./$.export')
+ , DESCRIPTORS = require('./$.descriptors')
+ , createDesc = require('./$.property-desc')
+ , html = require('./$.html')
+ , cel = require('./$.dom-create')
+ , has = require('./$.has')
+ , cof = require('./$.cof')
+ , invoke = require('./$.invoke')
+ , fails = require('./$.fails')
+ , anObject = require('./$.an-object')
+ , aFunction = require('./$.a-function')
+ , isObject = require('./$.is-object')
+ , toObject = require('./$.to-object')
+ , toIObject = require('./$.to-iobject')
+ , toInteger = require('./$.to-integer')
+ , toIndex = require('./$.to-index')
+ , toLength = require('./$.to-length')
+ , IObject = require('./$.iobject')
+ , IE_PROTO = require('./$.uid')('__proto__')
+ , createArrayMethod = require('./$.array-methods')
+ , arrayIndexOf = require('./$.array-includes')(false)
+ , ObjectProto = Object.prototype
+ , ArrayProto = Array.prototype
+ , arraySlice = ArrayProto.slice
+ , arrayJoin = ArrayProto.join
+ , defineProperty = $.setDesc
+ , getOwnDescriptor = $.getDesc
+ , defineProperties = $.setDescs
+ , factories = {}
+ , IE8_DOM_DEFINE;
+
+if(!DESCRIPTORS){
+ IE8_DOM_DEFINE = !fails(function(){
+ return defineProperty(cel('div'), 'a', {get: function(){ return 7; }}).a != 7;
+ });
+ $.setDesc = function(O, P, Attributes){
+ if(IE8_DOM_DEFINE)try {
+ return defineProperty(O, P, Attributes);
+ } catch(e){ /* empty */ }
+ if('get' in Attributes || 'set' in Attributes)throw TypeError('Accessors not supported!');
+ if('value' in Attributes)anObject(O)[P] = Attributes.value;
+ return O;
+ };
+ $.getDesc = function(O, P){
+ if(IE8_DOM_DEFINE)try {
+ return getOwnDescriptor(O, P);
+ } catch(e){ /* empty */ }
+ if(has(O, P))return createDesc(!ObjectProto.propertyIsEnumerable.call(O, P), O[P]);
+ };
+ $.setDescs = defineProperties = function(O, Properties){
+ anObject(O);
+ var keys = $.getKeys(Properties)
+ , length = keys.length
+ , i = 0
+ , P;
+ while(length > i)$.setDesc(O, P = keys[i++], Properties[P]);
+ return O;
+ };
+}
+$export($export.S + $export.F * !DESCRIPTORS, 'Object', {
+ // 19.1.2.6 / 15.2.3.3 Object.getOwnPropertyDescriptor(O, P)
+ getOwnPropertyDescriptor: $.getDesc,
+ // 19.1.2.4 / 15.2.3.6 Object.defineProperty(O, P, Attributes)
+ defineProperty: $.setDesc,
+ // 19.1.2.3 / 15.2.3.7 Object.defineProperties(O, Properties)
+ defineProperties: defineProperties
+});
+
+ // IE 8- don't enum bug keys
+var keys1 = ('constructor,hasOwnProperty,isPrototypeOf,propertyIsEnumerable,' +
+ 'toLocaleString,toString,valueOf').split(',')
+ // Additional keys for getOwnPropertyNames
+ , keys2 = keys1.concat('length', 'prototype')
+ , keysLen1 = keys1.length;
+
+// Create object with `null` prototype: use iframe Object with cleared prototype
+var createDict = function(){
+ // Thrash, waste and sodomy: IE GC bug
+ var iframe = cel('iframe')
+ , i = keysLen1
+ , gt = '>'
+ , iframeDocument;
+ iframe.style.display = 'none';
+ html.appendChild(iframe);
+ iframe.src = 'javascript:'; // eslint-disable-line no-script-url
+ // createDict = iframe.contentWindow.Object;
+ // html.removeChild(iframe);
+ iframeDocument = iframe.contentWindow.document;
+ iframeDocument.open();
+ iframeDocument.write(' i)if(has(O, key = names[i++])){
+ ~arrayIndexOf(result, key) || result.push(key);
+ }
+ return result;
+ };
+};
+var Empty = function(){};
+$export($export.S, 'Object', {
+ // 19.1.2.9 / 15.2.3.2 Object.getPrototypeOf(O)
+ getPrototypeOf: $.getProto = $.getProto || function(O){
+ O = toObject(O);
+ if(has(O, IE_PROTO))return O[IE_PROTO];
+ if(typeof O.constructor == 'function' && O instanceof O.constructor){
+ return O.constructor.prototype;
+ } return O instanceof Object ? ObjectProto : null;
+ },
+ // 19.1.2.7 / 15.2.3.4 Object.getOwnPropertyNames(O)
+ getOwnPropertyNames: $.getNames = $.getNames || createGetKeys(keys2, keys2.length, true),
+ // 19.1.2.2 / 15.2.3.5 Object.create(O [, Properties])
+ create: $.create = $.create || function(O, /*?*/Properties){
+ var result;
+ if(O !== null){
+ Empty.prototype = anObject(O);
+ result = new Empty();
+ Empty.prototype = null;
+ // add "__proto__" for Object.getPrototypeOf shim
+ result[IE_PROTO] = O;
+ } else result = createDict();
+ return Properties === undefined ? result : defineProperties(result, Properties);
+ },
+ // 19.1.2.14 / 15.2.3.14 Object.keys(O)
+ keys: $.getKeys = $.getKeys || createGetKeys(keys1, keysLen1, false)
+});
+
+var construct = function(F, len, args){
+ if(!(len in factories)){
+ for(var n = [], i = 0; i < len; i++)n[i] = 'a[' + i + ']';
+ factories[len] = Function('F,a', 'return new F(' + n.join(',') + ')');
+ }
+ return factories[len](F, args);
+};
+
+// 19.2.3.2 / 15.3.4.5 Function.prototype.bind(thisArg, args...)
+$export($export.P, 'Function', {
+ bind: function bind(that /*, args... */){
+ var fn = aFunction(this)
+ , partArgs = arraySlice.call(arguments, 1);
+ var bound = function(/* args... */){
+ var args = partArgs.concat(arraySlice.call(arguments));
+ return this instanceof bound ? construct(fn, args.length, args) : invoke(fn, args, that);
+ };
+ if(isObject(fn.prototype))bound.prototype = fn.prototype;
+ return bound;
+ }
+});
+
+// fallback for not array-like ES3 strings and DOM objects
+$export($export.P + $export.F * fails(function(){
+ if(html)arraySlice.call(html);
+}), 'Array', {
+ slice: function(begin, end){
+ var len = toLength(this.length)
+ , klass = cof(this);
+ end = end === undefined ? len : end;
+ if(klass == 'Array')return arraySlice.call(this, begin, end);
+ var start = toIndex(begin, len)
+ , upTo = toIndex(end, len)
+ , size = toLength(upTo - start)
+ , cloned = Array(size)
+ , i = 0;
+ for(; i < size; i++)cloned[i] = klass == 'String'
+ ? this.charAt(start + i)
+ : this[start + i];
+ return cloned;
+ }
+});
+$export($export.P + $export.F * (IObject != Object), 'Array', {
+ join: function join(separator){
+ return arrayJoin.call(IObject(this), separator === undefined ? ',' : separator);
+ }
+});
+
+// 22.1.2.2 / 15.4.3.2 Array.isArray(arg)
+$export($export.S, 'Array', {isArray: require('./$.is-array')});
+
+var createArrayReduce = function(isRight){
+ return function(callbackfn, memo){
+ aFunction(callbackfn);
+ var O = IObject(this)
+ , length = toLength(O.length)
+ , index = isRight ? length - 1 : 0
+ , i = isRight ? -1 : 1;
+ if(arguments.length < 2)for(;;){
+ if(index in O){
+ memo = O[index];
+ index += i;
+ break;
+ }
+ index += i;
+ if(isRight ? index < 0 : length <= index){
+ throw TypeError('Reduce of empty array with no initial value');
+ }
+ }
+ for(;isRight ? index >= 0 : length > index; index += i)if(index in O){
+ memo = callbackfn(memo, O[index], index, this);
+ }
+ return memo;
+ };
+};
+
+var methodize = function($fn){
+ return function(arg1/*, arg2 = undefined */){
+ return $fn(this, arg1, arguments[1]);
+ };
+};
+
+$export($export.P, 'Array', {
+ // 22.1.3.10 / 15.4.4.18 Array.prototype.forEach(callbackfn [, thisArg])
+ forEach: $.each = $.each || methodize(createArrayMethod(0)),
+ // 22.1.3.15 / 15.4.4.19 Array.prototype.map(callbackfn [, thisArg])
+ map: methodize(createArrayMethod(1)),
+ // 22.1.3.7 / 15.4.4.20 Array.prototype.filter(callbackfn [, thisArg])
+ filter: methodize(createArrayMethod(2)),
+ // 22.1.3.23 / 15.4.4.17 Array.prototype.some(callbackfn [, thisArg])
+ some: methodize(createArrayMethod(3)),
+ // 22.1.3.5 / 15.4.4.16 Array.prototype.every(callbackfn [, thisArg])
+ every: methodize(createArrayMethod(4)),
+ // 22.1.3.18 / 15.4.4.21 Array.prototype.reduce(callbackfn [, initialValue])
+ reduce: createArrayReduce(false),
+ // 22.1.3.19 / 15.4.4.22 Array.prototype.reduceRight(callbackfn [, initialValue])
+ reduceRight: createArrayReduce(true),
+ // 22.1.3.11 / 15.4.4.14 Array.prototype.indexOf(searchElement [, fromIndex])
+ indexOf: methodize(arrayIndexOf),
+ // 22.1.3.14 / 15.4.4.15 Array.prototype.lastIndexOf(searchElement [, fromIndex])
+ lastIndexOf: function(el, fromIndex /* = @[*-1] */){
+ var O = toIObject(this)
+ , length = toLength(O.length)
+ , index = length - 1;
+ if(arguments.length > 1)index = Math.min(index, toInteger(fromIndex));
+ if(index < 0)index = toLength(length + index);
+ for(;index >= 0; index--)if(index in O)if(O[index] === el)return index;
+ return -1;
+ }
+});
+
+// 20.3.3.1 / 15.9.4.4 Date.now()
+$export($export.S, 'Date', {now: function(){ return +new Date; }});
+
+var lz = function(num){
+ return num > 9 ? num : '0' + num;
+};
+
+// 20.3.4.36 / 15.9.5.43 Date.prototype.toISOString()
+// PhantomJS / old WebKit has a broken implementations
+$export($export.P + $export.F * (fails(function(){
+ return new Date(-5e13 - 1).toISOString() != '0385-07-25T07:06:39.999Z';
+}) || !fails(function(){
+ new Date(NaN).toISOString();
+})), 'Date', {
+ toISOString: function toISOString(){
+ if(!isFinite(this))throw RangeError('Invalid time value');
+ var d = this
+ , y = d.getUTCFullYear()
+ , m = d.getUTCMilliseconds()
+ , s = y < 0 ? '-' : y > 9999 ? '+' : '';
+ return s + ('00000' + Math.abs(y)).slice(s ? -6 : -4) +
+ '-' + lz(d.getUTCMonth() + 1) + '-' + lz(d.getUTCDate()) +
+ 'T' + lz(d.getUTCHours()) + ':' + lz(d.getUTCMinutes()) +
+ ':' + lz(d.getUTCSeconds()) + '.' + (m > 99 ? m : '0' + lz(m)) + 'Z';
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.copy-within.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.copy-within.js
new file mode 100644
index 00000000..930ba78d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.copy-within.js
@@ -0,0 +1,6 @@
+// 22.1.3.3 Array.prototype.copyWithin(target, start, end = this.length)
+var $export = require('./$.export');
+
+$export($export.P, 'Array', {copyWithin: require('./$.array-copy-within')});
+
+require('./$.add-to-unscopables')('copyWithin');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.fill.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.fill.js
new file mode 100644
index 00000000..c3b3e2e5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.fill.js
@@ -0,0 +1,6 @@
+// 22.1.3.6 Array.prototype.fill(value, start = 0, end = this.length)
+var $export = require('./$.export');
+
+$export($export.P, 'Array', {fill: require('./$.array-fill')});
+
+require('./$.add-to-unscopables')('fill');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.find-index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.find-index.js
new file mode 100644
index 00000000..7224a606
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.find-index.js
@@ -0,0 +1,14 @@
+'use strict';
+// 22.1.3.9 Array.prototype.findIndex(predicate, thisArg = undefined)
+var $export = require('./$.export')
+ , $find = require('./$.array-methods')(6)
+ , KEY = 'findIndex'
+ , forced = true;
+// Shouldn't skip holes
+if(KEY in [])Array(1)[KEY](function(){ forced = false; });
+$export($export.P + $export.F * forced, 'Array', {
+ findIndex: function findIndex(callbackfn/*, that = undefined */){
+ return $find(this, callbackfn, arguments.length > 1 ? arguments[1] : undefined);
+ }
+});
+require('./$.add-to-unscopables')(KEY);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.find.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.find.js
new file mode 100644
index 00000000..199e987e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.find.js
@@ -0,0 +1,14 @@
+'use strict';
+// 22.1.3.8 Array.prototype.find(predicate, thisArg = undefined)
+var $export = require('./$.export')
+ , $find = require('./$.array-methods')(5)
+ , KEY = 'find'
+ , forced = true;
+// Shouldn't skip holes
+if(KEY in [])Array(1)[KEY](function(){ forced = false; });
+$export($export.P + $export.F * forced, 'Array', {
+ find: function find(callbackfn/*, that = undefined */){
+ return $find(this, callbackfn, arguments.length > 1 ? arguments[1] : undefined);
+ }
+});
+require('./$.add-to-unscopables')(KEY);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.from.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.from.js
new file mode 100644
index 00000000..4637d8d2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.from.js
@@ -0,0 +1,36 @@
+'use strict';
+var ctx = require('./$.ctx')
+ , $export = require('./$.export')
+ , toObject = require('./$.to-object')
+ , call = require('./$.iter-call')
+ , isArrayIter = require('./$.is-array-iter')
+ , toLength = require('./$.to-length')
+ , getIterFn = require('./core.get-iterator-method');
+$export($export.S + $export.F * !require('./$.iter-detect')(function(iter){ Array.from(iter); }), 'Array', {
+ // 22.1.2.1 Array.from(arrayLike, mapfn = undefined, thisArg = undefined)
+ from: function from(arrayLike/*, mapfn = undefined, thisArg = undefined*/){
+ var O = toObject(arrayLike)
+ , C = typeof this == 'function' ? this : Array
+ , $$ = arguments
+ , $$len = $$.length
+ , mapfn = $$len > 1 ? $$[1] : undefined
+ , mapping = mapfn !== undefined
+ , index = 0
+ , iterFn = getIterFn(O)
+ , length, result, step, iterator;
+ if(mapping)mapfn = ctx(mapfn, $$len > 2 ? $$[2] : undefined, 2);
+ // if object isn't iterable or it's array with default iterator - use simple case
+ if(iterFn != undefined && !(C == Array && isArrayIter(iterFn))){
+ for(iterator = iterFn.call(O), result = new C; !(step = iterator.next()).done; index++){
+ result[index] = mapping ? call(iterator, mapfn, [step.value, index], true) : step.value;
+ }
+ } else {
+ length = toLength(O.length);
+ for(result = new C(length); length > index; index++){
+ result[index] = mapping ? mapfn(O[index], index) : O[index];
+ }
+ }
+ result.length = index;
+ return result;
+ }
+});
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.iterator.js
new file mode 100644
index 00000000..52a546dd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.iterator.js
@@ -0,0 +1,34 @@
+'use strict';
+var addToUnscopables = require('./$.add-to-unscopables')
+ , step = require('./$.iter-step')
+ , Iterators = require('./$.iterators')
+ , toIObject = require('./$.to-iobject');
+
+// 22.1.3.4 Array.prototype.entries()
+// 22.1.3.13 Array.prototype.keys()
+// 22.1.3.29 Array.prototype.values()
+// 22.1.3.30 Array.prototype[@@iterator]()
+module.exports = require('./$.iter-define')(Array, 'Array', function(iterated, kind){
+ this._t = toIObject(iterated); // target
+ this._i = 0; // next index
+ this._k = kind; // kind
+// 22.1.5.2.1 %ArrayIteratorPrototype%.next()
+}, function(){
+ var O = this._t
+ , kind = this._k
+ , index = this._i++;
+ if(!O || index >= O.length){
+ this._t = undefined;
+ return step(1);
+ }
+ if(kind == 'keys' )return step(0, index);
+ if(kind == 'values')return step(0, O[index]);
+ return step(0, [index, O[index]]);
+}, 'values');
+
+// argumentsList[@@iterator] is %ArrayProto_values% (9.4.4.6, 9.4.4.7)
+Iterators.Arguments = Iterators.Array;
+
+addToUnscopables('keys');
+addToUnscopables('values');
+addToUnscopables('entries');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.of.js
new file mode 100644
index 00000000..f623f157
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.of.js
@@ -0,0 +1,19 @@
+'use strict';
+var $export = require('./$.export');
+
+// WebKit Array.of isn't generic
+$export($export.S + $export.F * require('./$.fails')(function(){
+ function F(){}
+ return !(Array.of.call(F) instanceof F);
+}), 'Array', {
+ // 22.1.2.3 Array.of( ...items)
+ of: function of(/* ...args */){
+ var index = 0
+ , $$ = arguments
+ , $$len = $$.length
+ , result = new (typeof this == 'function' ? this : Array)($$len);
+ while($$len > index)result[index] = $$[index++];
+ result.length = $$len;
+ return result;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.species.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.species.js
new file mode 100644
index 00000000..543bdfe9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.array.species.js
@@ -0,0 +1 @@
+require('./$.set-species')('Array');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.date.to-string.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.date.to-string.js
new file mode 100644
index 00000000..e69de29b
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.function.has-instance.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.function.has-instance.js
new file mode 100644
index 00000000..94d840f8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.function.has-instance.js
@@ -0,0 +1,13 @@
+'use strict';
+var $ = require('./$')
+ , isObject = require('./$.is-object')
+ , HAS_INSTANCE = require('./$.wks')('hasInstance')
+ , FunctionProto = Function.prototype;
+// 19.2.3.6 Function.prototype[@@hasInstance](V)
+if(!(HAS_INSTANCE in FunctionProto))$.setDesc(FunctionProto, HAS_INSTANCE, {value: function(O){
+ if(typeof this != 'function' || !isObject(O))return false;
+ if(!isObject(this.prototype))return O instanceof this;
+ // for environment w/o native `@@hasInstance` logic enough `instanceof`, but add this:
+ while(O = $.getProto(O))if(this.prototype === O)return true;
+ return false;
+}});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.function.name.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.function.name.js
new file mode 100644
index 00000000..e69de29b
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.map.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.map.js
new file mode 100644
index 00000000..54fd5c1a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.map.js
@@ -0,0 +1,17 @@
+'use strict';
+var strong = require('./$.collection-strong');
+
+// 23.1 Map Objects
+require('./$.collection')('Map', function(get){
+ return function Map(){ return get(this, arguments.length > 0 ? arguments[0] : undefined); };
+}, {
+ // 23.1.3.6 Map.prototype.get(key)
+ get: function get(key){
+ var entry = strong.getEntry(this, key);
+ return entry && entry.v;
+ },
+ // 23.1.3.9 Map.prototype.set(key, value)
+ set: function set(key, value){
+ return strong.def(this, key === 0 ? 0 : key, value);
+ }
+}, strong, true);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.acosh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.acosh.js
new file mode 100644
index 00000000..f69282a8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.acosh.js
@@ -0,0 +1,14 @@
+// 20.2.2.3 Math.acosh(x)
+var $export = require('./$.export')
+ , log1p = require('./$.math-log1p')
+ , sqrt = Math.sqrt
+ , $acosh = Math.acosh;
+
+// V8 bug https://code.google.com/p/v8/issues/detail?id=3509
+$export($export.S + $export.F * !($acosh && Math.floor($acosh(Number.MAX_VALUE)) == 710), 'Math', {
+ acosh: function acosh(x){
+ return (x = +x) < 1 ? NaN : x > 94906265.62425156
+ ? Math.log(x) + Math.LN2
+ : log1p(x - 1 + sqrt(x - 1) * sqrt(x + 1));
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.asinh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.asinh.js
new file mode 100644
index 00000000..bd34adf0
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.asinh.js
@@ -0,0 +1,8 @@
+// 20.2.2.5 Math.asinh(x)
+var $export = require('./$.export');
+
+function asinh(x){
+ return !isFinite(x = +x) || x == 0 ? x : x < 0 ? -asinh(-x) : Math.log(x + Math.sqrt(x * x + 1));
+}
+
+$export($export.S, 'Math', {asinh: asinh});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.atanh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.atanh.js
new file mode 100644
index 00000000..656ea409
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.atanh.js
@@ -0,0 +1,8 @@
+// 20.2.2.7 Math.atanh(x)
+var $export = require('./$.export');
+
+$export($export.S, 'Math', {
+ atanh: function atanh(x){
+ return (x = +x) == 0 ? x : Math.log((1 + x) / (1 - x)) / 2;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.cbrt.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.cbrt.js
new file mode 100644
index 00000000..79a1fbc8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.cbrt.js
@@ -0,0 +1,9 @@
+// 20.2.2.9 Math.cbrt(x)
+var $export = require('./$.export')
+ , sign = require('./$.math-sign');
+
+$export($export.S, 'Math', {
+ cbrt: function cbrt(x){
+ return sign(x = +x) * Math.pow(Math.abs(x), 1 / 3);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.clz32.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.clz32.js
new file mode 100644
index 00000000..edd11588
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.clz32.js
@@ -0,0 +1,8 @@
+// 20.2.2.11 Math.clz32(x)
+var $export = require('./$.export');
+
+$export($export.S, 'Math', {
+ clz32: function clz32(x){
+ return (x >>>= 0) ? 31 - Math.floor(Math.log(x + 0.5) * Math.LOG2E) : 32;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.cosh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.cosh.js
new file mode 100644
index 00000000..d1df7490
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.cosh.js
@@ -0,0 +1,9 @@
+// 20.2.2.12 Math.cosh(x)
+var $export = require('./$.export')
+ , exp = Math.exp;
+
+$export($export.S, 'Math', {
+ cosh: function cosh(x){
+ return (exp(x = +x) + exp(-x)) / 2;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.expm1.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.expm1.js
new file mode 100644
index 00000000..e27742fe
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.expm1.js
@@ -0,0 +1,4 @@
+// 20.2.2.14 Math.expm1(x)
+var $export = require('./$.export');
+
+$export($export.S, 'Math', {expm1: require('./$.math-expm1')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.fround.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.fround.js
new file mode 100644
index 00000000..43cd70c7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.fround.js
@@ -0,0 +1,26 @@
+// 20.2.2.16 Math.fround(x)
+var $export = require('./$.export')
+ , sign = require('./$.math-sign')
+ , pow = Math.pow
+ , EPSILON = pow(2, -52)
+ , EPSILON32 = pow(2, -23)
+ , MAX32 = pow(2, 127) * (2 - EPSILON32)
+ , MIN32 = pow(2, -126);
+
+var roundTiesToEven = function(n){
+ return n + 1 / EPSILON - 1 / EPSILON;
+};
+
+
+$export($export.S, 'Math', {
+ fround: function fround(x){
+ var $abs = Math.abs(x)
+ , $sign = sign(x)
+ , a, result;
+ if($abs < MIN32)return $sign * roundTiesToEven($abs / MIN32 / EPSILON32) * MIN32 * EPSILON32;
+ a = (1 + EPSILON32 / EPSILON) * $abs;
+ result = a - (a - $abs);
+ if(result > MAX32 || result != result)return $sign * Infinity;
+ return $sign * result;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.hypot.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.hypot.js
new file mode 100644
index 00000000..a8edf7cd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.hypot.js
@@ -0,0 +1,26 @@
+// 20.2.2.17 Math.hypot([value1[, value2[, … ]]])
+var $export = require('./$.export')
+ , abs = Math.abs;
+
+$export($export.S, 'Math', {
+ hypot: function hypot(value1, value2){ // eslint-disable-line no-unused-vars
+ var sum = 0
+ , i = 0
+ , $$ = arguments
+ , $$len = $$.length
+ , larg = 0
+ , arg, div;
+ while(i < $$len){
+ arg = abs($$[i++]);
+ if(larg < arg){
+ div = larg / arg;
+ sum = sum * div * div + 1;
+ larg = arg;
+ } else if(arg > 0){
+ div = arg / larg;
+ sum += div * div;
+ } else sum += arg;
+ }
+ return larg === Infinity ? Infinity : larg * Math.sqrt(sum);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.imul.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.imul.js
new file mode 100644
index 00000000..926053de
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.imul.js
@@ -0,0 +1,17 @@
+// 20.2.2.18 Math.imul(x, y)
+var $export = require('./$.export')
+ , $imul = Math.imul;
+
+// some WebKit versions fails with big numbers, some has wrong arity
+$export($export.S + $export.F * require('./$.fails')(function(){
+ return $imul(0xffffffff, 5) != -5 || $imul.length != 2;
+}), 'Math', {
+ imul: function imul(x, y){
+ var UINT16 = 0xffff
+ , xn = +x
+ , yn = +y
+ , xl = UINT16 & xn
+ , yl = UINT16 & yn;
+ return 0 | xl * yl + ((UINT16 & xn >>> 16) * yl + xl * (UINT16 & yn >>> 16) << 16 >>> 0);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.log10.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.log10.js
new file mode 100644
index 00000000..ef5ae6a9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.log10.js
@@ -0,0 +1,8 @@
+// 20.2.2.21 Math.log10(x)
+var $export = require('./$.export');
+
+$export($export.S, 'Math', {
+ log10: function log10(x){
+ return Math.log(x) / Math.LN10;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.log1p.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.log1p.js
new file mode 100644
index 00000000..31c395ec
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.log1p.js
@@ -0,0 +1,4 @@
+// 20.2.2.20 Math.log1p(x)
+var $export = require('./$.export');
+
+$export($export.S, 'Math', {log1p: require('./$.math-log1p')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.log2.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.log2.js
new file mode 100644
index 00000000..24c0124b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.log2.js
@@ -0,0 +1,8 @@
+// 20.2.2.22 Math.log2(x)
+var $export = require('./$.export');
+
+$export($export.S, 'Math', {
+ log2: function log2(x){
+ return Math.log(x) / Math.LN2;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.sign.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.sign.js
new file mode 100644
index 00000000..fd8d8bc3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.sign.js
@@ -0,0 +1,4 @@
+// 20.2.2.28 Math.sign(x)
+var $export = require('./$.export');
+
+$export($export.S, 'Math', {sign: require('./$.math-sign')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.sinh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.sinh.js
new file mode 100644
index 00000000..a01c4d37
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.sinh.js
@@ -0,0 +1,15 @@
+// 20.2.2.30 Math.sinh(x)
+var $export = require('./$.export')
+ , expm1 = require('./$.math-expm1')
+ , exp = Math.exp;
+
+// V8 near Chromium 38 has a problem with very small numbers
+$export($export.S + $export.F * require('./$.fails')(function(){
+ return !Math.sinh(-2e-17) != -2e-17;
+}), 'Math', {
+ sinh: function sinh(x){
+ return Math.abs(x = +x) < 1
+ ? (expm1(x) - expm1(-x)) / 2
+ : (exp(x - 1) - exp(-x - 1)) * (Math.E / 2);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.tanh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.tanh.js
new file mode 100644
index 00000000..0d081a5d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.tanh.js
@@ -0,0 +1,12 @@
+// 20.2.2.33 Math.tanh(x)
+var $export = require('./$.export')
+ , expm1 = require('./$.math-expm1')
+ , exp = Math.exp;
+
+$export($export.S, 'Math', {
+ tanh: function tanh(x){
+ var a = expm1(x = +x)
+ , b = expm1(-x);
+ return a == Infinity ? 1 : b == Infinity ? -1 : (a - b) / (exp(x) + exp(-x));
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.trunc.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.trunc.js
new file mode 100644
index 00000000..f3f08559
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.math.trunc.js
@@ -0,0 +1,8 @@
+// 20.2.2.34 Math.trunc(x)
+var $export = require('./$.export');
+
+$export($export.S, 'Math', {
+ trunc: function trunc(it){
+ return (it > 0 ? Math.floor : Math.ceil)(it);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.constructor.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.constructor.js
new file mode 100644
index 00000000..e69de29b
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.epsilon.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.epsilon.js
new file mode 100644
index 00000000..45c865cf
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.epsilon.js
@@ -0,0 +1,4 @@
+// 20.1.2.1 Number.EPSILON
+var $export = require('./$.export');
+
+$export($export.S, 'Number', {EPSILON: Math.pow(2, -52)});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.is-finite.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.is-finite.js
new file mode 100644
index 00000000..362a6c80
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.is-finite.js
@@ -0,0 +1,9 @@
+// 20.1.2.2 Number.isFinite(number)
+var $export = require('./$.export')
+ , _isFinite = require('./$.global').isFinite;
+
+$export($export.S, 'Number', {
+ isFinite: function isFinite(it){
+ return typeof it == 'number' && _isFinite(it);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.is-integer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.is-integer.js
new file mode 100644
index 00000000..189db9a2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.is-integer.js
@@ -0,0 +1,4 @@
+// 20.1.2.3 Number.isInteger(number)
+var $export = require('./$.export');
+
+$export($export.S, 'Number', {isInteger: require('./$.is-integer')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.is-nan.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.is-nan.js
new file mode 100644
index 00000000..151bb4b2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.is-nan.js
@@ -0,0 +1,8 @@
+// 20.1.2.4 Number.isNaN(number)
+var $export = require('./$.export');
+
+$export($export.S, 'Number', {
+ isNaN: function isNaN(number){
+ return number != number;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.is-safe-integer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.is-safe-integer.js
new file mode 100644
index 00000000..e23b4cbc
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.is-safe-integer.js
@@ -0,0 +1,10 @@
+// 20.1.2.5 Number.isSafeInteger(number)
+var $export = require('./$.export')
+ , isInteger = require('./$.is-integer')
+ , abs = Math.abs;
+
+$export($export.S, 'Number', {
+ isSafeInteger: function isSafeInteger(number){
+ return isInteger(number) && abs(number) <= 0x1fffffffffffff;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.max-safe-integer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.max-safe-integer.js
new file mode 100644
index 00000000..a1aaf741
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.max-safe-integer.js
@@ -0,0 +1,4 @@
+// 20.1.2.6 Number.MAX_SAFE_INTEGER
+var $export = require('./$.export');
+
+$export($export.S, 'Number', {MAX_SAFE_INTEGER: 0x1fffffffffffff});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.min-safe-integer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.min-safe-integer.js
new file mode 100644
index 00000000..ab97cb5d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.min-safe-integer.js
@@ -0,0 +1,4 @@
+// 20.1.2.10 Number.MIN_SAFE_INTEGER
+var $export = require('./$.export');
+
+$export($export.S, 'Number', {MIN_SAFE_INTEGER: -0x1fffffffffffff});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.parse-float.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.parse-float.js
new file mode 100644
index 00000000..1d0c9674
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.parse-float.js
@@ -0,0 +1,4 @@
+// 20.1.2.12 Number.parseFloat(string)
+var $export = require('./$.export');
+
+$export($export.S, 'Number', {parseFloat: parseFloat});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.parse-int.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.parse-int.js
new file mode 100644
index 00000000..813b5b79
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.number.parse-int.js
@@ -0,0 +1,4 @@
+// 20.1.2.13 Number.parseInt(string, radix)
+var $export = require('./$.export');
+
+$export($export.S, 'Number', {parseInt: parseInt});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.assign.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.assign.js
new file mode 100644
index 00000000..b62e7a42
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.assign.js
@@ -0,0 +1,4 @@
+// 19.1.3.1 Object.assign(target, source)
+var $export = require('./$.export');
+
+$export($export.S + $export.F, 'Object', {assign: require('./$.object-assign')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.freeze.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.freeze.js
new file mode 100644
index 00000000..fa87c951
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.freeze.js
@@ -0,0 +1,8 @@
+// 19.1.2.5 Object.freeze(O)
+var isObject = require('./$.is-object');
+
+require('./$.object-sap')('freeze', function($freeze){
+ return function freeze(it){
+ return $freeze && isObject(it) ? $freeze(it) : it;
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.get-own-property-descriptor.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.get-own-property-descriptor.js
new file mode 100644
index 00000000..9b253acd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.get-own-property-descriptor.js
@@ -0,0 +1,8 @@
+// 19.1.2.6 Object.getOwnPropertyDescriptor(O, P)
+var toIObject = require('./$.to-iobject');
+
+require('./$.object-sap')('getOwnPropertyDescriptor', function($getOwnPropertyDescriptor){
+ return function getOwnPropertyDescriptor(it, key){
+ return $getOwnPropertyDescriptor(toIObject(it), key);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.get-own-property-names.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.get-own-property-names.js
new file mode 100644
index 00000000..e87bcf60
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.get-own-property-names.js
@@ -0,0 +1,4 @@
+// 19.1.2.7 Object.getOwnPropertyNames(O)
+require('./$.object-sap')('getOwnPropertyNames', function(){
+ return require('./$.get-names').get;
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.get-prototype-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.get-prototype-of.js
new file mode 100644
index 00000000..9ec0405b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.get-prototype-of.js
@@ -0,0 +1,8 @@
+// 19.1.2.9 Object.getPrototypeOf(O)
+var toObject = require('./$.to-object');
+
+require('./$.object-sap')('getPrototypeOf', function($getPrototypeOf){
+ return function getPrototypeOf(it){
+ return $getPrototypeOf(toObject(it));
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.is-extensible.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.is-extensible.js
new file mode 100644
index 00000000..ada2b95a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.is-extensible.js
@@ -0,0 +1,8 @@
+// 19.1.2.11 Object.isExtensible(O)
+var isObject = require('./$.is-object');
+
+require('./$.object-sap')('isExtensible', function($isExtensible){
+ return function isExtensible(it){
+ return isObject(it) ? $isExtensible ? $isExtensible(it) : true : false;
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.is-frozen.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.is-frozen.js
new file mode 100644
index 00000000..b3e44d1b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.is-frozen.js
@@ -0,0 +1,8 @@
+// 19.1.2.12 Object.isFrozen(O)
+var isObject = require('./$.is-object');
+
+require('./$.object-sap')('isFrozen', function($isFrozen){
+ return function isFrozen(it){
+ return isObject(it) ? $isFrozen ? $isFrozen(it) : false : true;
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.is-sealed.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.is-sealed.js
new file mode 100644
index 00000000..423caf33
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.is-sealed.js
@@ -0,0 +1,8 @@
+// 19.1.2.13 Object.isSealed(O)
+var isObject = require('./$.is-object');
+
+require('./$.object-sap')('isSealed', function($isSealed){
+ return function isSealed(it){
+ return isObject(it) ? $isSealed ? $isSealed(it) : false : true;
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.is.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.is.js
new file mode 100644
index 00000000..3ae3b604
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.is.js
@@ -0,0 +1,3 @@
+// 19.1.3.10 Object.is(value1, value2)
+var $export = require('./$.export');
+$export($export.S, 'Object', {is: require('./$.same-value')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.keys.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.keys.js
new file mode 100644
index 00000000..e3c18c02
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.keys.js
@@ -0,0 +1,8 @@
+// 19.1.2.14 Object.keys(O)
+var toObject = require('./$.to-object');
+
+require('./$.object-sap')('keys', function($keys){
+ return function keys(it){
+ return $keys(toObject(it));
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.prevent-extensions.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.prevent-extensions.js
new file mode 100644
index 00000000..20f879e2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.prevent-extensions.js
@@ -0,0 +1,8 @@
+// 19.1.2.15 Object.preventExtensions(O)
+var isObject = require('./$.is-object');
+
+require('./$.object-sap')('preventExtensions', function($preventExtensions){
+ return function preventExtensions(it){
+ return $preventExtensions && isObject(it) ? $preventExtensions(it) : it;
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.seal.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.seal.js
new file mode 100644
index 00000000..85a7fa98
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.seal.js
@@ -0,0 +1,8 @@
+// 19.1.2.17 Object.seal(O)
+var isObject = require('./$.is-object');
+
+require('./$.object-sap')('seal', function($seal){
+ return function seal(it){
+ return $seal && isObject(it) ? $seal(it) : it;
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.set-prototype-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.set-prototype-of.js
new file mode 100644
index 00000000..79a147c3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.set-prototype-of.js
@@ -0,0 +1,3 @@
+// 19.1.3.19 Object.setPrototypeOf(O, proto)
+var $export = require('./$.export');
+$export($export.S, 'Object', {setPrototypeOf: require('./$.set-proto').set});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.to-string.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.object.to-string.js
new file mode 100644
index 00000000..e69de29b
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.promise.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.promise.js
new file mode 100644
index 00000000..fbf20177
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.promise.js
@@ -0,0 +1,293 @@
+'use strict';
+var $ = require('./$')
+ , LIBRARY = require('./$.library')
+ , global = require('./$.global')
+ , ctx = require('./$.ctx')
+ , classof = require('./$.classof')
+ , $export = require('./$.export')
+ , isObject = require('./$.is-object')
+ , anObject = require('./$.an-object')
+ , aFunction = require('./$.a-function')
+ , strictNew = require('./$.strict-new')
+ , forOf = require('./$.for-of')
+ , setProto = require('./$.set-proto').set
+ , same = require('./$.same-value')
+ , SPECIES = require('./$.wks')('species')
+ , speciesConstructor = require('./$.species-constructor')
+ , asap = require('./$.microtask')
+ , PROMISE = 'Promise'
+ , process = global.process
+ , isNode = classof(process) == 'process'
+ , P = global[PROMISE]
+ , empty = function(){ /* empty */ }
+ , Wrapper;
+
+var testResolve = function(sub){
+ var test = new P(empty), promise;
+ if(sub)test.constructor = function(exec){
+ exec(empty, empty);
+ };
+ (promise = P.resolve(test))['catch'](empty);
+ return promise === test;
+};
+
+var USE_NATIVE = function(){
+ var works = false;
+ function P2(x){
+ var self = new P(x);
+ setProto(self, P2.prototype);
+ return self;
+ }
+ try {
+ works = P && P.resolve && testResolve();
+ setProto(P2, P);
+ P2.prototype = $.create(P.prototype, {constructor: {value: P2}});
+ // actual Firefox has broken subclass support, test that
+ if(!(P2.resolve(5).then(function(){}) instanceof P2)){
+ works = false;
+ }
+ // actual V8 bug, https://code.google.com/p/v8/issues/detail?id=4162
+ if(works && require('./$.descriptors')){
+ var thenableThenGotten = false;
+ P.resolve($.setDesc({}, 'then', {
+ get: function(){ thenableThenGotten = true; }
+ }));
+ works = thenableThenGotten;
+ }
+ } catch(e){ works = false; }
+ return works;
+}();
+
+// helpers
+var sameConstructor = function(a, b){
+ // library wrapper special case
+ if(LIBRARY && a === P && b === Wrapper)return true;
+ return same(a, b);
+};
+var getConstructor = function(C){
+ var S = anObject(C)[SPECIES];
+ return S != undefined ? S : C;
+};
+var isThenable = function(it){
+ var then;
+ return isObject(it) && typeof (then = it.then) == 'function' ? then : false;
+};
+var PromiseCapability = function(C){
+ var resolve, reject;
+ this.promise = new C(function($$resolve, $$reject){
+ if(resolve !== undefined || reject !== undefined)throw TypeError('Bad Promise constructor');
+ resolve = $$resolve;
+ reject = $$reject;
+ });
+ this.resolve = aFunction(resolve),
+ this.reject = aFunction(reject)
+};
+var perform = function(exec){
+ try {
+ exec();
+ } catch(e){
+ return {error: e};
+ }
+};
+var notify = function(record, isReject){
+ if(record.n)return;
+ record.n = true;
+ var chain = record.c;
+ asap(function(){
+ var value = record.v
+ , ok = record.s == 1
+ , i = 0;
+ var run = function(reaction){
+ var handler = ok ? reaction.ok : reaction.fail
+ , resolve = reaction.resolve
+ , reject = reaction.reject
+ , result, then;
+ try {
+ if(handler){
+ if(!ok)record.h = true;
+ result = handler === true ? value : handler(value);
+ if(result === reaction.promise){
+ reject(TypeError('Promise-chain cycle'));
+ } else if(then = isThenable(result)){
+ then.call(result, resolve, reject);
+ } else resolve(result);
+ } else reject(value);
+ } catch(e){
+ reject(e);
+ }
+ };
+ while(chain.length > i)run(chain[i++]); // variable length - can't use forEach
+ chain.length = 0;
+ record.n = false;
+ if(isReject)setTimeout(function(){
+ var promise = record.p
+ , handler, console;
+ if(isUnhandled(promise)){
+ if(isNode){
+ process.emit('unhandledRejection', value, promise);
+ } else if(handler = global.onunhandledrejection){
+ handler({promise: promise, reason: value});
+ } else if((console = global.console) && console.error){
+ console.error('Unhandled promise rejection', value);
+ }
+ } record.a = undefined;
+ }, 1);
+ });
+};
+var isUnhandled = function(promise){
+ var record = promise._d
+ , chain = record.a || record.c
+ , i = 0
+ , reaction;
+ if(record.h)return false;
+ while(chain.length > i){
+ reaction = chain[i++];
+ if(reaction.fail || !isUnhandled(reaction.promise))return false;
+ } return true;
+};
+var $reject = function(value){
+ var record = this;
+ if(record.d)return;
+ record.d = true;
+ record = record.r || record; // unwrap
+ record.v = value;
+ record.s = 2;
+ record.a = record.c.slice();
+ notify(record, true);
+};
+var $resolve = function(value){
+ var record = this
+ , then;
+ if(record.d)return;
+ record.d = true;
+ record = record.r || record; // unwrap
+ try {
+ if(record.p === value)throw TypeError("Promise can't be resolved itself");
+ if(then = isThenable(value)){
+ asap(function(){
+ var wrapper = {r: record, d: false}; // wrap
+ try {
+ then.call(value, ctx($resolve, wrapper, 1), ctx($reject, wrapper, 1));
+ } catch(e){
+ $reject.call(wrapper, e);
+ }
+ });
+ } else {
+ record.v = value;
+ record.s = 1;
+ notify(record, false);
+ }
+ } catch(e){
+ $reject.call({r: record, d: false}, e); // wrap
+ }
+};
+
+// constructor polyfill
+if(!USE_NATIVE){
+ // 25.4.3.1 Promise(executor)
+ P = function Promise(executor){
+ aFunction(executor);
+ var record = this._d = {
+ p: strictNew(this, P, PROMISE), // <- promise
+ c: [], // <- awaiting reactions
+ a: undefined, // <- checked in isUnhandled reactions
+ s: 0, // <- state
+ d: false, // <- done
+ v: undefined, // <- value
+ h: false, // <- handled rejection
+ n: false // <- notify
+ };
+ try {
+ executor(ctx($resolve, record, 1), ctx($reject, record, 1));
+ } catch(err){
+ $reject.call(record, err);
+ }
+ };
+ require('./$.redefine-all')(P.prototype, {
+ // 25.4.5.3 Promise.prototype.then(onFulfilled, onRejected)
+ then: function then(onFulfilled, onRejected){
+ var reaction = new PromiseCapability(speciesConstructor(this, P))
+ , promise = reaction.promise
+ , record = this._d;
+ reaction.ok = typeof onFulfilled == 'function' ? onFulfilled : true;
+ reaction.fail = typeof onRejected == 'function' && onRejected;
+ record.c.push(reaction);
+ if(record.a)record.a.push(reaction);
+ if(record.s)notify(record, false);
+ return promise;
+ },
+ // 25.4.5.1 Promise.prototype.catch(onRejected)
+ 'catch': function(onRejected){
+ return this.then(undefined, onRejected);
+ }
+ });
+}
+
+$export($export.G + $export.W + $export.F * !USE_NATIVE, {Promise: P});
+require('./$.set-to-string-tag')(P, PROMISE);
+require('./$.set-species')(PROMISE);
+Wrapper = require('./$.core')[PROMISE];
+
+// statics
+$export($export.S + $export.F * !USE_NATIVE, PROMISE, {
+ // 25.4.4.5 Promise.reject(r)
+ reject: function reject(r){
+ var capability = new PromiseCapability(this)
+ , $$reject = capability.reject;
+ $$reject(r);
+ return capability.promise;
+ }
+});
+$export($export.S + $export.F * (!USE_NATIVE || testResolve(true)), PROMISE, {
+ // 25.4.4.6 Promise.resolve(x)
+ resolve: function resolve(x){
+ // instanceof instead of internal slot check because we should fix it without replacement native Promise core
+ if(x instanceof P && sameConstructor(x.constructor, this))return x;
+ var capability = new PromiseCapability(this)
+ , $$resolve = capability.resolve;
+ $$resolve(x);
+ return capability.promise;
+ }
+});
+$export($export.S + $export.F * !(USE_NATIVE && require('./$.iter-detect')(function(iter){
+ P.all(iter)['catch'](function(){});
+})), PROMISE, {
+ // 25.4.4.1 Promise.all(iterable)
+ all: function all(iterable){
+ var C = getConstructor(this)
+ , capability = new PromiseCapability(C)
+ , resolve = capability.resolve
+ , reject = capability.reject
+ , values = [];
+ var abrupt = perform(function(){
+ forOf(iterable, false, values.push, values);
+ var remaining = values.length
+ , results = Array(remaining);
+ if(remaining)$.each.call(values, function(promise, index){
+ var alreadyCalled = false;
+ C.resolve(promise).then(function(value){
+ if(alreadyCalled)return;
+ alreadyCalled = true;
+ results[index] = value;
+ --remaining || resolve(results);
+ }, reject);
+ });
+ else resolve(results);
+ });
+ if(abrupt)reject(abrupt.error);
+ return capability.promise;
+ },
+ // 25.4.4.4 Promise.race(iterable)
+ race: function race(iterable){
+ var C = getConstructor(this)
+ , capability = new PromiseCapability(C)
+ , reject = capability.reject;
+ var abrupt = perform(function(){
+ forOf(iterable, false, function(promise){
+ C.resolve(promise).then(capability.resolve, reject);
+ });
+ });
+ if(abrupt)reject(abrupt.error);
+ return capability.promise;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.apply.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.apply.js
new file mode 100644
index 00000000..361a2e2e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.apply.js
@@ -0,0 +1,10 @@
+// 26.1.1 Reflect.apply(target, thisArgument, argumentsList)
+var $export = require('./$.export')
+ , _apply = Function.apply
+ , anObject = require('./$.an-object');
+
+$export($export.S, 'Reflect', {
+ apply: function apply(target, thisArgument, argumentsList){
+ return _apply.call(target, thisArgument, anObject(argumentsList));
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.construct.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.construct.js
new file mode 100644
index 00000000..c3928cd9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.construct.js
@@ -0,0 +1,39 @@
+// 26.1.2 Reflect.construct(target, argumentsList [, newTarget])
+var $ = require('./$')
+ , $export = require('./$.export')
+ , aFunction = require('./$.a-function')
+ , anObject = require('./$.an-object')
+ , isObject = require('./$.is-object')
+ , bind = Function.bind || require('./$.core').Function.prototype.bind;
+
+// MS Edge supports only 2 arguments
+// FF Nightly sets third argument as `new.target`, but does not create `this` from it
+$export($export.S + $export.F * require('./$.fails')(function(){
+ function F(){}
+ return !(Reflect.construct(function(){}, [], F) instanceof F);
+}), 'Reflect', {
+ construct: function construct(Target, args /*, newTarget*/){
+ aFunction(Target);
+ anObject(args);
+ var newTarget = arguments.length < 3 ? Target : aFunction(arguments[2]);
+ if(Target == newTarget){
+ // w/o altered newTarget, optimization for 0-4 arguments
+ switch(args.length){
+ case 0: return new Target;
+ case 1: return new Target(args[0]);
+ case 2: return new Target(args[0], args[1]);
+ case 3: return new Target(args[0], args[1], args[2]);
+ case 4: return new Target(args[0], args[1], args[2], args[3]);
+ }
+ // w/o altered newTarget, lot of arguments case
+ var $args = [null];
+ $args.push.apply($args, args);
+ return new (bind.apply(Target, $args));
+ }
+ // with altered newTarget, not support built-in constructors
+ var proto = newTarget.prototype
+ , instance = $.create(isObject(proto) ? proto : Object.prototype)
+ , result = Function.apply.call(Target, instance, args);
+ return isObject(result) ? result : instance;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.define-property.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.define-property.js
new file mode 100644
index 00000000..5f7fc6a1
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.define-property.js
@@ -0,0 +1,19 @@
+// 26.1.3 Reflect.defineProperty(target, propertyKey, attributes)
+var $ = require('./$')
+ , $export = require('./$.export')
+ , anObject = require('./$.an-object');
+
+// MS Edge has broken Reflect.defineProperty - throwing instead of returning false
+$export($export.S + $export.F * require('./$.fails')(function(){
+ Reflect.defineProperty($.setDesc({}, 1, {value: 1}), 1, {value: 2});
+}), 'Reflect', {
+ defineProperty: function defineProperty(target, propertyKey, attributes){
+ anObject(target);
+ try {
+ $.setDesc(target, propertyKey, attributes);
+ return true;
+ } catch(e){
+ return false;
+ }
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.delete-property.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.delete-property.js
new file mode 100644
index 00000000..18526e5b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.delete-property.js
@@ -0,0 +1,11 @@
+// 26.1.4 Reflect.deleteProperty(target, propertyKey)
+var $export = require('./$.export')
+ , getDesc = require('./$').getDesc
+ , anObject = require('./$.an-object');
+
+$export($export.S, 'Reflect', {
+ deleteProperty: function deleteProperty(target, propertyKey){
+ var desc = getDesc(anObject(target), propertyKey);
+ return desc && !desc.configurable ? false : delete target[propertyKey];
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.enumerate.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.enumerate.js
new file mode 100644
index 00000000..73452e2f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.enumerate.js
@@ -0,0 +1,26 @@
+'use strict';
+// 26.1.5 Reflect.enumerate(target)
+var $export = require('./$.export')
+ , anObject = require('./$.an-object');
+var Enumerate = function(iterated){
+ this._t = anObject(iterated); // target
+ this._i = 0; // next index
+ var keys = this._k = [] // keys
+ , key;
+ for(key in iterated)keys.push(key);
+};
+require('./$.iter-create')(Enumerate, 'Object', function(){
+ var that = this
+ , keys = that._k
+ , key;
+ do {
+ if(that._i >= keys.length)return {value: undefined, done: true};
+ } while(!((key = keys[that._i++]) in that._t));
+ return {value: key, done: false};
+});
+
+$export($export.S, 'Reflect', {
+ enumerate: function enumerate(target){
+ return new Enumerate(target);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.get-own-property-descriptor.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.get-own-property-descriptor.js
new file mode 100644
index 00000000..a3a2e016
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.get-own-property-descriptor.js
@@ -0,0 +1,10 @@
+// 26.1.7 Reflect.getOwnPropertyDescriptor(target, propertyKey)
+var $ = require('./$')
+ , $export = require('./$.export')
+ , anObject = require('./$.an-object');
+
+$export($export.S, 'Reflect', {
+ getOwnPropertyDescriptor: function getOwnPropertyDescriptor(target, propertyKey){
+ return $.getDesc(anObject(target), propertyKey);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.get-prototype-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.get-prototype-of.js
new file mode 100644
index 00000000..c06bfa45
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.get-prototype-of.js
@@ -0,0 +1,10 @@
+// 26.1.8 Reflect.getPrototypeOf(target)
+var $export = require('./$.export')
+ , getProto = require('./$').getProto
+ , anObject = require('./$.an-object');
+
+$export($export.S, 'Reflect', {
+ getPrototypeOf: function getPrototypeOf(target){
+ return getProto(anObject(target));
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.get.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.get.js
new file mode 100644
index 00000000..cbb0caaf
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.get.js
@@ -0,0 +1,20 @@
+// 26.1.6 Reflect.get(target, propertyKey [, receiver])
+var $ = require('./$')
+ , has = require('./$.has')
+ , $export = require('./$.export')
+ , isObject = require('./$.is-object')
+ , anObject = require('./$.an-object');
+
+function get(target, propertyKey/*, receiver*/){
+ var receiver = arguments.length < 3 ? target : arguments[2]
+ , desc, proto;
+ if(anObject(target) === receiver)return target[propertyKey];
+ if(desc = $.getDesc(target, propertyKey))return has(desc, 'value')
+ ? desc.value
+ : desc.get !== undefined
+ ? desc.get.call(receiver)
+ : undefined;
+ if(isObject(proto = $.getProto(target)))return get(proto, propertyKey, receiver);
+}
+
+$export($export.S, 'Reflect', {get: get});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.has.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.has.js
new file mode 100644
index 00000000..65c9e82f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.has.js
@@ -0,0 +1,8 @@
+// 26.1.9 Reflect.has(target, propertyKey)
+var $export = require('./$.export');
+
+$export($export.S, 'Reflect', {
+ has: function has(target, propertyKey){
+ return propertyKey in target;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.is-extensible.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.is-extensible.js
new file mode 100644
index 00000000..b92c4f66
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.is-extensible.js
@@ -0,0 +1,11 @@
+// 26.1.10 Reflect.isExtensible(target)
+var $export = require('./$.export')
+ , anObject = require('./$.an-object')
+ , $isExtensible = Object.isExtensible;
+
+$export($export.S, 'Reflect', {
+ isExtensible: function isExtensible(target){
+ anObject(target);
+ return $isExtensible ? $isExtensible(target) : true;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.own-keys.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.own-keys.js
new file mode 100644
index 00000000..db79fdab
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.own-keys.js
@@ -0,0 +1,4 @@
+// 26.1.11 Reflect.ownKeys(target)
+var $export = require('./$.export');
+
+$export($export.S, 'Reflect', {ownKeys: require('./$.own-keys')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.prevent-extensions.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.prevent-extensions.js
new file mode 100644
index 00000000..f5ccfc2a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.prevent-extensions.js
@@ -0,0 +1,16 @@
+// 26.1.12 Reflect.preventExtensions(target)
+var $export = require('./$.export')
+ , anObject = require('./$.an-object')
+ , $preventExtensions = Object.preventExtensions;
+
+$export($export.S, 'Reflect', {
+ preventExtensions: function preventExtensions(target){
+ anObject(target);
+ try {
+ if($preventExtensions)$preventExtensions(target);
+ return true;
+ } catch(e){
+ return false;
+ }
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.set-prototype-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.set-prototype-of.js
new file mode 100644
index 00000000..e769436f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.set-prototype-of.js
@@ -0,0 +1,15 @@
+// 26.1.14 Reflect.setPrototypeOf(target, proto)
+var $export = require('./$.export')
+ , setProto = require('./$.set-proto');
+
+if(setProto)$export($export.S, 'Reflect', {
+ setPrototypeOf: function setPrototypeOf(target, proto){
+ setProto.check(target, proto);
+ try {
+ setProto.set(target, proto);
+ return true;
+ } catch(e){
+ return false;
+ }
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.set.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.set.js
new file mode 100644
index 00000000..0a938e78
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.reflect.set.js
@@ -0,0 +1,29 @@
+// 26.1.13 Reflect.set(target, propertyKey, V [, receiver])
+var $ = require('./$')
+ , has = require('./$.has')
+ , $export = require('./$.export')
+ , createDesc = require('./$.property-desc')
+ , anObject = require('./$.an-object')
+ , isObject = require('./$.is-object');
+
+function set(target, propertyKey, V/*, receiver*/){
+ var receiver = arguments.length < 4 ? target : arguments[3]
+ , ownDesc = $.getDesc(anObject(target), propertyKey)
+ , existingDescriptor, proto;
+ if(!ownDesc){
+ if(isObject(proto = $.getProto(target))){
+ return set(proto, propertyKey, V, receiver);
+ }
+ ownDesc = createDesc(0);
+ }
+ if(has(ownDesc, 'value')){
+ if(ownDesc.writable === false || !isObject(receiver))return false;
+ existingDescriptor = $.getDesc(receiver, propertyKey) || createDesc(0);
+ existingDescriptor.value = V;
+ $.setDesc(receiver, propertyKey, existingDescriptor);
+ return true;
+ }
+ return ownDesc.set === undefined ? false : (ownDesc.set.call(receiver, V), true);
+}
+
+$export($export.S, 'Reflect', {set: set});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.regexp.constructor.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.regexp.constructor.js
new file mode 100644
index 00000000..087d9beb
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.regexp.constructor.js
@@ -0,0 +1 @@
+require('./$.set-species')('RegExp');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.regexp.flags.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.regexp.flags.js
new file mode 100644
index 00000000..e69de29b
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.regexp.match.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.regexp.match.js
new file mode 100644
index 00000000..e69de29b
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.regexp.replace.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.regexp.replace.js
new file mode 100644
index 00000000..e69de29b
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.regexp.search.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.regexp.search.js
new file mode 100644
index 00000000..e69de29b
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.regexp.split.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.regexp.split.js
new file mode 100644
index 00000000..e69de29b
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.set.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.set.js
new file mode 100644
index 00000000..8e148c9e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.set.js
@@ -0,0 +1,12 @@
+'use strict';
+var strong = require('./$.collection-strong');
+
+// 23.2 Set Objects
+require('./$.collection')('Set', function(get){
+ return function Set(){ return get(this, arguments.length > 0 ? arguments[0] : undefined); };
+}, {
+ // 23.2.3.1 Set.prototype.add(value)
+ add: function add(value){
+ return strong.def(this, value = value === 0 ? 0 : value, value);
+ }
+}, strong);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.code-point-at.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.code-point-at.js
new file mode 100644
index 00000000..ebac5518
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.code-point-at.js
@@ -0,0 +1,9 @@
+'use strict';
+var $export = require('./$.export')
+ , $at = require('./$.string-at')(false);
+$export($export.P, 'String', {
+ // 21.1.3.3 String.prototype.codePointAt(pos)
+ codePointAt: function codePointAt(pos){
+ return $at(this, pos);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.ends-with.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.ends-with.js
new file mode 100644
index 00000000..a102da2d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.ends-with.js
@@ -0,0 +1,21 @@
+// 21.1.3.6 String.prototype.endsWith(searchString [, endPosition])
+'use strict';
+var $export = require('./$.export')
+ , toLength = require('./$.to-length')
+ , context = require('./$.string-context')
+ , ENDS_WITH = 'endsWith'
+ , $endsWith = ''[ENDS_WITH];
+
+$export($export.P + $export.F * require('./$.fails-is-regexp')(ENDS_WITH), 'String', {
+ endsWith: function endsWith(searchString /*, endPosition = @length */){
+ var that = context(this, searchString, ENDS_WITH)
+ , $$ = arguments
+ , endPosition = $$.length > 1 ? $$[1] : undefined
+ , len = toLength(that.length)
+ , end = endPosition === undefined ? len : Math.min(toLength(endPosition), len)
+ , search = String(searchString);
+ return $endsWith
+ ? $endsWith.call(that, search, end)
+ : that.slice(end - search.length, end) === search;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.from-code-point.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.from-code-point.js
new file mode 100644
index 00000000..b0bd166b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.from-code-point.js
@@ -0,0 +1,24 @@
+var $export = require('./$.export')
+ , toIndex = require('./$.to-index')
+ , fromCharCode = String.fromCharCode
+ , $fromCodePoint = String.fromCodePoint;
+
+// length should be 1, old FF problem
+$export($export.S + $export.F * (!!$fromCodePoint && $fromCodePoint.length != 1), 'String', {
+ // 21.1.2.2 String.fromCodePoint(...codePoints)
+ fromCodePoint: function fromCodePoint(x){ // eslint-disable-line no-unused-vars
+ var res = []
+ , $$ = arguments
+ , $$len = $$.length
+ , i = 0
+ , code;
+ while($$len > i){
+ code = +$$[i++];
+ if(toIndex(code, 0x10ffff) !== code)throw RangeError(code + ' is not a valid code point');
+ res.push(code < 0x10000
+ ? fromCharCode(code)
+ : fromCharCode(((code -= 0x10000) >> 10) + 0xd800, code % 0x400 + 0xdc00)
+ );
+ } return res.join('');
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.includes.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.includes.js
new file mode 100644
index 00000000..e2ab8db7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.includes.js
@@ -0,0 +1,12 @@
+// 21.1.3.7 String.prototype.includes(searchString, position = 0)
+'use strict';
+var $export = require('./$.export')
+ , context = require('./$.string-context')
+ , INCLUDES = 'includes';
+
+$export($export.P + $export.F * require('./$.fails-is-regexp')(INCLUDES), 'String', {
+ includes: function includes(searchString /*, position = 0 */){
+ return !!~context(this, searchString, INCLUDES)
+ .indexOf(searchString, arguments.length > 1 ? arguments[1] : undefined);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.iterator.js
new file mode 100644
index 00000000..2f4c772c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.iterator.js
@@ -0,0 +1,17 @@
+'use strict';
+var $at = require('./$.string-at')(true);
+
+// 21.1.3.27 String.prototype[@@iterator]()
+require('./$.iter-define')(String, 'String', function(iterated){
+ this._t = String(iterated); // target
+ this._i = 0; // next index
+// 21.1.5.2.1 %StringIteratorPrototype%.next()
+}, function(){
+ var O = this._t
+ , index = this._i
+ , point;
+ if(index >= O.length)return {value: undefined, done: true};
+ point = $at(O, index);
+ this._i += point.length;
+ return {value: point, done: false};
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.raw.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.raw.js
new file mode 100644
index 00000000..64279d23
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.raw.js
@@ -0,0 +1,19 @@
+var $export = require('./$.export')
+ , toIObject = require('./$.to-iobject')
+ , toLength = require('./$.to-length');
+
+$export($export.S, 'String', {
+ // 21.1.2.4 String.raw(callSite, ...substitutions)
+ raw: function raw(callSite){
+ var tpl = toIObject(callSite.raw)
+ , len = toLength(tpl.length)
+ , $$ = arguments
+ , $$len = $$.length
+ , res = []
+ , i = 0;
+ while(len > i){
+ res.push(String(tpl[i++]));
+ if(i < $$len)res.push(String($$[i]));
+ } return res.join('');
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.repeat.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.repeat.js
new file mode 100644
index 00000000..4ec29f66
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.repeat.js
@@ -0,0 +1,6 @@
+var $export = require('./$.export');
+
+$export($export.P, 'String', {
+ // 21.1.3.13 String.prototype.repeat(count)
+ repeat: require('./$.string-repeat')
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.starts-with.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.starts-with.js
new file mode 100644
index 00000000..21143072
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.starts-with.js
@@ -0,0 +1,19 @@
+// 21.1.3.18 String.prototype.startsWith(searchString [, position ])
+'use strict';
+var $export = require('./$.export')
+ , toLength = require('./$.to-length')
+ , context = require('./$.string-context')
+ , STARTS_WITH = 'startsWith'
+ , $startsWith = ''[STARTS_WITH];
+
+$export($export.P + $export.F * require('./$.fails-is-regexp')(STARTS_WITH), 'String', {
+ startsWith: function startsWith(searchString /*, position = 0 */){
+ var that = context(this, searchString, STARTS_WITH)
+ , $$ = arguments
+ , index = toLength(Math.min($$.length > 1 ? $$[1] : undefined, that.length))
+ , search = String(searchString);
+ return $startsWith
+ ? $startsWith.call(that, search, index)
+ : that.slice(index, index + search.length) === search;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.trim.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.trim.js
new file mode 100644
index 00000000..52b75cac
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.string.trim.js
@@ -0,0 +1,7 @@
+'use strict';
+// 21.1.3.25 String.prototype.trim()
+require('./$.string-trim')('trim', function($trim){
+ return function trim(){
+ return $trim(this, 3);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.symbol.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.symbol.js
new file mode 100644
index 00000000..42b7a3aa
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.symbol.js
@@ -0,0 +1,227 @@
+'use strict';
+// ECMAScript 6 symbols shim
+var $ = require('./$')
+ , global = require('./$.global')
+ , has = require('./$.has')
+ , DESCRIPTORS = require('./$.descriptors')
+ , $export = require('./$.export')
+ , redefine = require('./$.redefine')
+ , $fails = require('./$.fails')
+ , shared = require('./$.shared')
+ , setToStringTag = require('./$.set-to-string-tag')
+ , uid = require('./$.uid')
+ , wks = require('./$.wks')
+ , keyOf = require('./$.keyof')
+ , $names = require('./$.get-names')
+ , enumKeys = require('./$.enum-keys')
+ , isArray = require('./$.is-array')
+ , anObject = require('./$.an-object')
+ , toIObject = require('./$.to-iobject')
+ , createDesc = require('./$.property-desc')
+ , getDesc = $.getDesc
+ , setDesc = $.setDesc
+ , _create = $.create
+ , getNames = $names.get
+ , $Symbol = global.Symbol
+ , $JSON = global.JSON
+ , _stringify = $JSON && $JSON.stringify
+ , setter = false
+ , HIDDEN = wks('_hidden')
+ , isEnum = $.isEnum
+ , SymbolRegistry = shared('symbol-registry')
+ , AllSymbols = shared('symbols')
+ , useNative = typeof $Symbol == 'function'
+ , ObjectProto = Object.prototype;
+
+// fallback for old Android, https://code.google.com/p/v8/issues/detail?id=687
+var setSymbolDesc = DESCRIPTORS && $fails(function(){
+ return _create(setDesc({}, 'a', {
+ get: function(){ return setDesc(this, 'a', {value: 7}).a; }
+ })).a != 7;
+}) ? function(it, key, D){
+ var protoDesc = getDesc(ObjectProto, key);
+ if(protoDesc)delete ObjectProto[key];
+ setDesc(it, key, D);
+ if(protoDesc && it !== ObjectProto)setDesc(ObjectProto, key, protoDesc);
+} : setDesc;
+
+var wrap = function(tag){
+ var sym = AllSymbols[tag] = _create($Symbol.prototype);
+ sym._k = tag;
+ DESCRIPTORS && setter && setSymbolDesc(ObjectProto, tag, {
+ configurable: true,
+ set: function(value){
+ if(has(this, HIDDEN) && has(this[HIDDEN], tag))this[HIDDEN][tag] = false;
+ setSymbolDesc(this, tag, createDesc(1, value));
+ }
+ });
+ return sym;
+};
+
+var isSymbol = function(it){
+ return typeof it == 'symbol';
+};
+
+var $defineProperty = function defineProperty(it, key, D){
+ if(D && has(AllSymbols, key)){
+ if(!D.enumerable){
+ if(!has(it, HIDDEN))setDesc(it, HIDDEN, createDesc(1, {}));
+ it[HIDDEN][key] = true;
+ } else {
+ if(has(it, HIDDEN) && it[HIDDEN][key])it[HIDDEN][key] = false;
+ D = _create(D, {enumerable: createDesc(0, false)});
+ } return setSymbolDesc(it, key, D);
+ } return setDesc(it, key, D);
+};
+var $defineProperties = function defineProperties(it, P){
+ anObject(it);
+ var keys = enumKeys(P = toIObject(P))
+ , i = 0
+ , l = keys.length
+ , key;
+ while(l > i)$defineProperty(it, key = keys[i++], P[key]);
+ return it;
+};
+var $create = function create(it, P){
+ return P === undefined ? _create(it) : $defineProperties(_create(it), P);
+};
+var $propertyIsEnumerable = function propertyIsEnumerable(key){
+ var E = isEnum.call(this, key);
+ return E || !has(this, key) || !has(AllSymbols, key) || has(this, HIDDEN) && this[HIDDEN][key]
+ ? E : true;
+};
+var $getOwnPropertyDescriptor = function getOwnPropertyDescriptor(it, key){
+ var D = getDesc(it = toIObject(it), key);
+ if(D && has(AllSymbols, key) && !(has(it, HIDDEN) && it[HIDDEN][key]))D.enumerable = true;
+ return D;
+};
+var $getOwnPropertyNames = function getOwnPropertyNames(it){
+ var names = getNames(toIObject(it))
+ , result = []
+ , i = 0
+ , key;
+ while(names.length > i)if(!has(AllSymbols, key = names[i++]) && key != HIDDEN)result.push(key);
+ return result;
+};
+var $getOwnPropertySymbols = function getOwnPropertySymbols(it){
+ var names = getNames(toIObject(it))
+ , result = []
+ , i = 0
+ , key;
+ while(names.length > i)if(has(AllSymbols, key = names[i++]))result.push(AllSymbols[key]);
+ return result;
+};
+var $stringify = function stringify(it){
+ if(it === undefined || isSymbol(it))return; // IE8 returns string on undefined
+ var args = [it]
+ , i = 1
+ , $$ = arguments
+ , replacer, $replacer;
+ while($$.length > i)args.push($$[i++]);
+ replacer = args[1];
+ if(typeof replacer == 'function')$replacer = replacer;
+ if($replacer || !isArray(replacer))replacer = function(key, value){
+ if($replacer)value = $replacer.call(this, key, value);
+ if(!isSymbol(value))return value;
+ };
+ args[1] = replacer;
+ return _stringify.apply($JSON, args);
+};
+var buggyJSON = $fails(function(){
+ var S = $Symbol();
+ // MS Edge converts symbol values to JSON as {}
+ // WebKit converts symbol values to JSON as null
+ // V8 throws on boxed symbols
+ return _stringify([S]) != '[null]' || _stringify({a: S}) != '{}' || _stringify(Object(S)) != '{}';
+});
+
+// 19.4.1.1 Symbol([description])
+if(!useNative){
+ $Symbol = function Symbol(){
+ if(isSymbol(this))throw TypeError('Symbol is not a constructor');
+ return wrap(uid(arguments.length > 0 ? arguments[0] : undefined));
+ };
+ redefine($Symbol.prototype, 'toString', function toString(){
+ return this._k;
+ });
+
+ isSymbol = function(it){
+ return it instanceof $Symbol;
+ };
+
+ $.create = $create;
+ $.isEnum = $propertyIsEnumerable;
+ $.getDesc = $getOwnPropertyDescriptor;
+ $.setDesc = $defineProperty;
+ $.setDescs = $defineProperties;
+ $.getNames = $names.get = $getOwnPropertyNames;
+ $.getSymbols = $getOwnPropertySymbols;
+
+ if(DESCRIPTORS && !require('./$.library')){
+ redefine(ObjectProto, 'propertyIsEnumerable', $propertyIsEnumerable, true);
+ }
+}
+
+var symbolStatics = {
+ // 19.4.2.1 Symbol.for(key)
+ 'for': function(key){
+ return has(SymbolRegistry, key += '')
+ ? SymbolRegistry[key]
+ : SymbolRegistry[key] = $Symbol(key);
+ },
+ // 19.4.2.5 Symbol.keyFor(sym)
+ keyFor: function keyFor(key){
+ return keyOf(SymbolRegistry, key);
+ },
+ useSetter: function(){ setter = true; },
+ useSimple: function(){ setter = false; }
+};
+// 19.4.2.2 Symbol.hasInstance
+// 19.4.2.3 Symbol.isConcatSpreadable
+// 19.4.2.4 Symbol.iterator
+// 19.4.2.6 Symbol.match
+// 19.4.2.8 Symbol.replace
+// 19.4.2.9 Symbol.search
+// 19.4.2.10 Symbol.species
+// 19.4.2.11 Symbol.split
+// 19.4.2.12 Symbol.toPrimitive
+// 19.4.2.13 Symbol.toStringTag
+// 19.4.2.14 Symbol.unscopables
+$.each.call((
+ 'hasInstance,isConcatSpreadable,iterator,match,replace,search,' +
+ 'species,split,toPrimitive,toStringTag,unscopables'
+).split(','), function(it){
+ var sym = wks(it);
+ symbolStatics[it] = useNative ? sym : wrap(sym);
+});
+
+setter = true;
+
+$export($export.G + $export.W, {Symbol: $Symbol});
+
+$export($export.S, 'Symbol', symbolStatics);
+
+$export($export.S + $export.F * !useNative, 'Object', {
+ // 19.1.2.2 Object.create(O [, Properties])
+ create: $create,
+ // 19.1.2.4 Object.defineProperty(O, P, Attributes)
+ defineProperty: $defineProperty,
+ // 19.1.2.3 Object.defineProperties(O, Properties)
+ defineProperties: $defineProperties,
+ // 19.1.2.6 Object.getOwnPropertyDescriptor(O, P)
+ getOwnPropertyDescriptor: $getOwnPropertyDescriptor,
+ // 19.1.2.7 Object.getOwnPropertyNames(O)
+ getOwnPropertyNames: $getOwnPropertyNames,
+ // 19.1.2.8 Object.getOwnPropertySymbols(O)
+ getOwnPropertySymbols: $getOwnPropertySymbols
+});
+
+// 24.3.2 JSON.stringify(value [, replacer [, space]])
+$JSON && $export($export.S + $export.F * (!useNative || buggyJSON), 'JSON', {stringify: $stringify});
+
+// 19.4.3.5 Symbol.prototype[@@toStringTag]
+setToStringTag($Symbol, 'Symbol');
+// 20.2.1.9 Math[@@toStringTag]
+setToStringTag(Math, 'Math', true);
+// 24.3.3 JSON[@@toStringTag]
+setToStringTag(global.JSON, 'JSON', true);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.array-buffer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.array-buffer.js
new file mode 100644
index 00000000..a8209bd2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.array-buffer.js
@@ -0,0 +1,43 @@
+'use strict';
+if(require('./$.descriptors')){
+ var $export = require('./$.export')
+ , $typed = require('./$.typed')
+ , buffer = require('./$.buffer')
+ , toIndex = require('./$.to-index')
+ , toLength = require('./$.to-length')
+ , isObject = require('./$.is-object')
+ , TYPED_ARRAY = require('./$.wks')('typed_array')
+ , $ArrayBuffer = buffer.ArrayBuffer
+ , $DataView = buffer.DataView
+ , $slice = $ArrayBuffer && $ArrayBuffer.prototype.slice
+ , VIEW = $typed.VIEW
+ , ARRAY_BUFFER = 'ArrayBuffer';
+
+ $export($export.G + $export.W + $export.F * !$typed.ABV, {ArrayBuffer: $ArrayBuffer});
+
+ $export($export.S + $export.F * !$typed.CONSTR, ARRAY_BUFFER, {
+ // 24.1.3.1 ArrayBuffer.isView(arg)
+ isView: function isView(it){ // not cross-realm
+ return isObject(it) && VIEW in it;
+ }
+ });
+
+ $export($export.P + $export.F * require('./$.fails')(function(){
+ return !new $ArrayBuffer(2).slice(1, undefined).byteLength;
+ }), ARRAY_BUFFER, {
+ // 24.1.4.3 ArrayBuffer.prototype.slice(start, end)
+ slice: function slice(start, end){
+ if($slice !== undefined && end === undefined)return $slice.call(this, start); // FF fix
+ var len = this.byteLength
+ , first = toIndex(start, len)
+ , final = toIndex(end === undefined ? len : end, len)
+ , result = new $ArrayBuffer(toLength(final - first))
+ , viewS = new $DataView(this)
+ , viewT = new $DataView(result)
+ , index = 0;
+ while(first < final){
+ viewT.setUint8(index++, viewS.getUint8(first++));
+ } return result;
+ }
+ });
+}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.data-view.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.data-view.js
new file mode 100644
index 00000000..44e03532
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.data-view.js
@@ -0,0 +1,4 @@
+if(require('./$.descriptors')){
+ var $export = require('./$.export');
+ $export($export.G + $export.W + $export.F * !require('./$.typed').ABV, {DataView: require('./$.buffer').DataView});
+}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.float32-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.float32-array.js
new file mode 100644
index 00000000..95d78a6d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.float32-array.js
@@ -0,0 +1,5 @@
+require('./$.typed-array')('Float32', 4, function(init){
+ return function Float32Array(data, byteOffset, length){
+ return init(this, data, byteOffset, length);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.float64-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.float64-array.js
new file mode 100644
index 00000000..16fadec9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.float64-array.js
@@ -0,0 +1,5 @@
+require('./$.typed-array')('Float64', 8, function(init){
+ return function Float64Array(data, byteOffset, length){
+ return init(this, data, byteOffset, length);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.int16-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.int16-array.js
new file mode 100644
index 00000000..a3d04cb6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.int16-array.js
@@ -0,0 +1,5 @@
+require('./$.typed-array')('Int16', 2, function(init){
+ return function Int16Array(data, byteOffset, length){
+ return init(this, data, byteOffset, length);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.int32-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.int32-array.js
new file mode 100644
index 00000000..1923463a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.int32-array.js
@@ -0,0 +1,5 @@
+require('./$.typed-array')('Int32', 4, function(init){
+ return function Int32Array(data, byteOffset, length){
+ return init(this, data, byteOffset, length);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.int8-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.int8-array.js
new file mode 100644
index 00000000..e9182c4c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.int8-array.js
@@ -0,0 +1,5 @@
+require('./$.typed-array')('Int8', 1, function(init){
+ return function Int8Array(data, byteOffset, length){
+ return init(this, data, byteOffset, length);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.uint16-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.uint16-array.js
new file mode 100644
index 00000000..ec6e8347
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.uint16-array.js
@@ -0,0 +1,5 @@
+require('./$.typed-array')('Uint16', 2, function(init){
+ return function Uint16Array(data, byteOffset, length){
+ return init(this, data, byteOffset, length);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.uint32-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.uint32-array.js
new file mode 100644
index 00000000..ddfc22d8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.uint32-array.js
@@ -0,0 +1,5 @@
+require('./$.typed-array')('Uint32', 4, function(init){
+ return function Uint32Array(data, byteOffset, length){
+ return init(this, data, byteOffset, length);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.uint8-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.uint8-array.js
new file mode 100644
index 00000000..7ab1e4df
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.uint8-array.js
@@ -0,0 +1,5 @@
+require('./$.typed-array')('Uint8', 1, function(init){
+ return function Uint8Array(data, byteOffset, length){
+ return init(this, data, byteOffset, length);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.uint8-clamped-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.uint8-clamped-array.js
new file mode 100644
index 00000000..f85f9d5d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.typed.uint8-clamped-array.js
@@ -0,0 +1,5 @@
+require('./$.typed-array')('Uint8', 1, function(init){
+ return function Uint8ClampedArray(data, byteOffset, length){
+ return init(this, data, byteOffset, length);
+ };
+}, true);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.weak-map.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.weak-map.js
new file mode 100644
index 00000000..72a9b324
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.weak-map.js
@@ -0,0 +1,43 @@
+'use strict';
+var $ = require('./$')
+ , redefine = require('./$.redefine')
+ , weak = require('./$.collection-weak')
+ , isObject = require('./$.is-object')
+ , has = require('./$.has')
+ , frozenStore = weak.frozenStore
+ , WEAK = weak.WEAK
+ , isExtensible = Object.isExtensible || isObject
+ , tmp = {};
+
+// 23.3 WeakMap Objects
+var $WeakMap = require('./$.collection')('WeakMap', function(get){
+ return function WeakMap(){ return get(this, arguments.length > 0 ? arguments[0] : undefined); };
+}, {
+ // 23.3.3.3 WeakMap.prototype.get(key)
+ get: function get(key){
+ if(isObject(key)){
+ if(!isExtensible(key))return frozenStore(this).get(key);
+ if(has(key, WEAK))return key[WEAK][this._i];
+ }
+ },
+ // 23.3.3.5 WeakMap.prototype.set(key, value)
+ set: function set(key, value){
+ return weak.def(this, key, value);
+ }
+}, weak, true, true);
+
+// IE11 WeakMap frozen keys fix
+if(new $WeakMap().set((Object.freeze || Object)(tmp), 7).get(tmp) != 7){
+ $.each.call(['delete', 'has', 'get', 'set'], function(key){
+ var proto = $WeakMap.prototype
+ , method = proto[key];
+ redefine(proto, key, function(a, b){
+ // store frozen objects on leaky map
+ if(isObject(a) && !isExtensible(a)){
+ var result = frozenStore(this)[key](a, b);
+ return key == 'set' ? this : result;
+ // store all the rest on native weakmap
+ } return method.call(this, a, b);
+ });
+ });
+}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.weak-set.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.weak-set.js
new file mode 100644
index 00000000..efdf1d76
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es6.weak-set.js
@@ -0,0 +1,12 @@
+'use strict';
+var weak = require('./$.collection-weak');
+
+// 23.4 WeakSet Objects
+require('./$.collection')('WeakSet', function(get){
+ return function WeakSet(){ return get(this, arguments.length > 0 ? arguments[0] : undefined); };
+}, {
+ // 23.4.3.1 WeakSet.prototype.add(value)
+ add: function add(value){
+ return weak.def(this, value, true);
+ }
+}, weak, false, true);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.array.includes.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.array.includes.js
new file mode 100644
index 00000000..dcfad704
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.array.includes.js
@@ -0,0 +1,12 @@
+'use strict';
+var $export = require('./$.export')
+ , $includes = require('./$.array-includes')(true);
+
+$export($export.P, 'Array', {
+ // https://github.com/domenic/Array.prototype.includes
+ includes: function includes(el /*, fromIndex = 0 */){
+ return $includes(this, el, arguments.length > 1 ? arguments[1] : undefined);
+ }
+});
+
+require('./$.add-to-unscopables')('includes');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.map.to-json.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.map.to-json.js
new file mode 100644
index 00000000..80937056
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.map.to-json.js
@@ -0,0 +1,4 @@
+// https://github.com/DavidBruant/Map-Set.prototype.toJSON
+var $export = require('./$.export');
+
+$export($export.P, 'Map', {toJSON: require('./$.collection-to-json')('Map')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.object.entries.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.object.entries.js
new file mode 100644
index 00000000..fec1bc36
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.object.entries.js
@@ -0,0 +1,9 @@
+// http://goo.gl/XkBrjD
+var $export = require('./$.export')
+ , $entries = require('./$.object-to-array')(true);
+
+$export($export.S, 'Object', {
+ entries: function entries(it){
+ return $entries(it);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.object.get-own-property-descriptors.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.object.get-own-property-descriptors.js
new file mode 100644
index 00000000..e4d80a34
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.object.get-own-property-descriptors.js
@@ -0,0 +1,23 @@
+// https://gist.github.com/WebReflection/9353781
+var $ = require('./$')
+ , $export = require('./$.export')
+ , ownKeys = require('./$.own-keys')
+ , toIObject = require('./$.to-iobject')
+ , createDesc = require('./$.property-desc');
+
+$export($export.S, 'Object', {
+ getOwnPropertyDescriptors: function getOwnPropertyDescriptors(object){
+ var O = toIObject(object)
+ , setDesc = $.setDesc
+ , getDesc = $.getDesc
+ , keys = ownKeys(O)
+ , result = {}
+ , i = 0
+ , key, D;
+ while(keys.length > i){
+ D = getDesc(O, key = keys[i++]);
+ if(key in result)setDesc(result, key, createDesc(0, D));
+ else result[key] = D;
+ } return result;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.object.values.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.object.values.js
new file mode 100644
index 00000000..697e9354
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.object.values.js
@@ -0,0 +1,9 @@
+// http://goo.gl/XkBrjD
+var $export = require('./$.export')
+ , $values = require('./$.object-to-array')(false);
+
+$export($export.S, 'Object', {
+ values: function values(it){
+ return $values(it);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.regexp.escape.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.regexp.escape.js
new file mode 100644
index 00000000..9c4c542a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.regexp.escape.js
@@ -0,0 +1,5 @@
+// https://github.com/benjamingr/RexExp.escape
+var $export = require('./$.export')
+ , $re = require('./$.replacer')(/[\\^$*+?.()|[\]{}]/g, '\\$&');
+
+$export($export.S, 'RegExp', {escape: function escape(it){ return $re(it); }});
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.set.to-json.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.set.to-json.js
new file mode 100644
index 00000000..e632f2a3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.set.to-json.js
@@ -0,0 +1,4 @@
+// https://github.com/DavidBruant/Map-Set.prototype.toJSON
+var $export = require('./$.export');
+
+$export($export.P, 'Set', {toJSON: require('./$.collection-to-json')('Set')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.string.at.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.string.at.js
new file mode 100644
index 00000000..fee583bf
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.string.at.js
@@ -0,0 +1,10 @@
+'use strict';
+// https://github.com/mathiasbynens/String.prototype.at
+var $export = require('./$.export')
+ , $at = require('./$.string-at')(true);
+
+$export($export.P, 'String', {
+ at: function at(pos){
+ return $at(this, pos);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.string.pad-left.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.string.pad-left.js
new file mode 100644
index 00000000..643621aa
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.string.pad-left.js
@@ -0,0 +1,9 @@
+'use strict';
+var $export = require('./$.export')
+ , $pad = require('./$.string-pad');
+
+$export($export.P, 'String', {
+ padLeft: function padLeft(maxLength /*, fillString = ' ' */){
+ return $pad(this, maxLength, arguments.length > 1 ? arguments[1] : undefined, true);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.string.pad-right.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.string.pad-right.js
new file mode 100644
index 00000000..e4230960
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.string.pad-right.js
@@ -0,0 +1,9 @@
+'use strict';
+var $export = require('./$.export')
+ , $pad = require('./$.string-pad');
+
+$export($export.P, 'String', {
+ padRight: function padRight(maxLength /*, fillString = ' ' */){
+ return $pad(this, maxLength, arguments.length > 1 ? arguments[1] : undefined, false);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.string.trim-left.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.string.trim-left.js
new file mode 100644
index 00000000..dbaf6308
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.string.trim-left.js
@@ -0,0 +1,7 @@
+'use strict';
+// https://github.com/sebmarkbage/ecmascript-string-left-right-trim
+require('./$.string-trim')('trimLeft', function($trim){
+ return function trimLeft(){
+ return $trim(this, 1);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.string.trim-right.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.string.trim-right.js
new file mode 100644
index 00000000..6b02d394
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/es7.string.trim-right.js
@@ -0,0 +1,7 @@
+'use strict';
+// https://github.com/sebmarkbage/ecmascript-string-left-right-trim
+require('./$.string-trim')('trimRight', function($trim){
+ return function trimRight(){
+ return $trim(this, 2);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/js.array.statics.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/js.array.statics.js
new file mode 100644
index 00000000..9536c2e4
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/js.array.statics.js
@@ -0,0 +1,17 @@
+// JavaScript 1.6 / Strawman array statics shim
+var $ = require('./$')
+ , $export = require('./$.export')
+ , $ctx = require('./$.ctx')
+ , $Array = require('./$.core').Array || Array
+ , statics = {};
+var setStatics = function(keys, length){
+ $.each.call(keys.split(','), function(key){
+ if(length == undefined && key in $Array)statics[key] = $Array[key];
+ else if(key in [])statics[key] = $ctx(Function.call, [][key], length);
+ });
+};
+setStatics('pop,reverse,shift,keys,values,entries', 1);
+setStatics('indexOf,every,some,forEach,map,filter,find,findIndex,includes', 3);
+setStatics('join,slice,concat,push,splice,unshift,sort,lastIndexOf,' +
+ 'reduce,reduceRight,copyWithin,fill');
+$export($export.S, 'Array', statics);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/web.dom.iterable.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/web.dom.iterable.js
new file mode 100644
index 00000000..988c6da2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/web.dom.iterable.js
@@ -0,0 +1,3 @@
+require('./es6.array.iterator');
+var Iterators = require('./$.iterators');
+Iterators.NodeList = Iterators.HTMLCollection = Iterators.Array;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/web.immediate.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/web.immediate.js
new file mode 100644
index 00000000..fa64f08e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/web.immediate.js
@@ -0,0 +1,6 @@
+var $export = require('./$.export')
+ , $task = require('./$.task');
+$export($export.G + $export.B, {
+ setImmediate: $task.set,
+ clearImmediate: $task.clear
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/web.timers.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/web.timers.js
new file mode 100644
index 00000000..74b72019
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/modules/web.timers.js
@@ -0,0 +1,20 @@
+// ie9- setTimeout & setInterval additional parameters fix
+var global = require('./$.global')
+ , $export = require('./$.export')
+ , invoke = require('./$.invoke')
+ , partial = require('./$.partial')
+ , navigator = global.navigator
+ , MSIE = !!navigator && /MSIE .\./.test(navigator.userAgent); // <- dirty ie9- check
+var wrap = function(set){
+ return MSIE ? function(fn, time /*, ...args */){
+ return set(invoke(
+ partial,
+ [].slice.call(arguments, 2),
+ typeof fn == 'function' ? fn : Function(fn)
+ ), time);
+ } : set;
+};
+$export($export.G + $export.B + $export.F * MSIE, {
+ setTimeout: wrap(global.setTimeout),
+ setInterval: wrap(global.setInterval)
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/shim.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/shim.js
new file mode 100644
index 00000000..6d38d2e2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/shim.js
@@ -0,0 +1,104 @@
+require('./modules/es5');
+require('./modules/es6.symbol');
+require('./modules/es6.object.assign');
+require('./modules/es6.object.is');
+require('./modules/es6.object.set-prototype-of');
+require('./modules/es6.object.to-string');
+require('./modules/es6.object.freeze');
+require('./modules/es6.object.seal');
+require('./modules/es6.object.prevent-extensions');
+require('./modules/es6.object.is-frozen');
+require('./modules/es6.object.is-sealed');
+require('./modules/es6.object.is-extensible');
+require('./modules/es6.object.get-own-property-descriptor');
+require('./modules/es6.object.get-prototype-of');
+require('./modules/es6.object.keys');
+require('./modules/es6.object.get-own-property-names');
+require('./modules/es6.function.name');
+require('./modules/es6.function.has-instance');
+require('./modules/es6.number.constructor');
+require('./modules/es6.number.epsilon');
+require('./modules/es6.number.is-finite');
+require('./modules/es6.number.is-integer');
+require('./modules/es6.number.is-nan');
+require('./modules/es6.number.is-safe-integer');
+require('./modules/es6.number.max-safe-integer');
+require('./modules/es6.number.min-safe-integer');
+require('./modules/es6.number.parse-float');
+require('./modules/es6.number.parse-int');
+require('./modules/es6.math.acosh');
+require('./modules/es6.math.asinh');
+require('./modules/es6.math.atanh');
+require('./modules/es6.math.cbrt');
+require('./modules/es6.math.clz32');
+require('./modules/es6.math.cosh');
+require('./modules/es6.math.expm1');
+require('./modules/es6.math.fround');
+require('./modules/es6.math.hypot');
+require('./modules/es6.math.imul');
+require('./modules/es6.math.log10');
+require('./modules/es6.math.log1p');
+require('./modules/es6.math.log2');
+require('./modules/es6.math.sign');
+require('./modules/es6.math.sinh');
+require('./modules/es6.math.tanh');
+require('./modules/es6.math.trunc');
+require('./modules/es6.string.from-code-point');
+require('./modules/es6.string.raw');
+require('./modules/es6.string.trim');
+require('./modules/es6.string.iterator');
+require('./modules/es6.string.code-point-at');
+require('./modules/es6.string.ends-with');
+require('./modules/es6.string.includes');
+require('./modules/es6.string.repeat');
+require('./modules/es6.string.starts-with');
+require('./modules/es6.array.from');
+require('./modules/es6.array.of');
+require('./modules/es6.array.iterator');
+require('./modules/es6.array.species');
+require('./modules/es6.array.copy-within');
+require('./modules/es6.array.fill');
+require('./modules/es6.array.find');
+require('./modules/es6.array.find-index');
+require('./modules/es6.regexp.constructor');
+require('./modules/es6.regexp.flags');
+require('./modules/es6.regexp.match');
+require('./modules/es6.regexp.replace');
+require('./modules/es6.regexp.search');
+require('./modules/es6.regexp.split');
+require('./modules/es6.promise');
+require('./modules/es6.map');
+require('./modules/es6.set');
+require('./modules/es6.weak-map');
+require('./modules/es6.weak-set');
+require('./modules/es6.reflect.apply');
+require('./modules/es6.reflect.construct');
+require('./modules/es6.reflect.define-property');
+require('./modules/es6.reflect.delete-property');
+require('./modules/es6.reflect.enumerate');
+require('./modules/es6.reflect.get');
+require('./modules/es6.reflect.get-own-property-descriptor');
+require('./modules/es6.reflect.get-prototype-of');
+require('./modules/es6.reflect.has');
+require('./modules/es6.reflect.is-extensible');
+require('./modules/es6.reflect.own-keys');
+require('./modules/es6.reflect.prevent-extensions');
+require('./modules/es6.reflect.set');
+require('./modules/es6.reflect.set-prototype-of');
+require('./modules/es7.array.includes');
+require('./modules/es7.string.at');
+require('./modules/es7.string.pad-left');
+require('./modules/es7.string.pad-right');
+require('./modules/es7.string.trim-left');
+require('./modules/es7.string.trim-right');
+require('./modules/es7.regexp.escape');
+require('./modules/es7.object.get-own-property-descriptors');
+require('./modules/es7.object.values');
+require('./modules/es7.object.entries');
+require('./modules/es7.map.to-json');
+require('./modules/es7.set.to-json');
+require('./modules/js.array.statics');
+require('./modules/web.timers');
+require('./modules/web.immediate');
+require('./modules/web.dom.iterable');
+module.exports = require('./modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/web/dom.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/web/dom.js
new file mode 100644
index 00000000..9b448cfe
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/web/dom.js
@@ -0,0 +1,2 @@
+require('../modules/web.dom.iterable');
+module.exports = require('../modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/web/immediate.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/web/immediate.js
new file mode 100644
index 00000000..e4e5493b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/web/immediate.js
@@ -0,0 +1,2 @@
+require('../modules/web.immediate');
+module.exports = require('../modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/web/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/web/index.js
new file mode 100644
index 00000000..6c3221e2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/web/index.js
@@ -0,0 +1,4 @@
+require('../modules/web.timers');
+require('../modules/web.immediate');
+require('../modules/web.dom.iterable');
+module.exports = require('../modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/web/timers.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/web/timers.js
new file mode 100644
index 00000000..763ea44e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/library/web/timers.js
@@ -0,0 +1,2 @@
+require('../modules/web.timers');
+module.exports = require('../modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.a-function.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.a-function.js
new file mode 100644
index 00000000..8c35f451
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.a-function.js
@@ -0,0 +1,4 @@
+module.exports = function(it){
+ if(typeof it != 'function')throw TypeError(it + ' is not a function!');
+ return it;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.add-to-unscopables.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.add-to-unscopables.js
new file mode 100644
index 00000000..bffd1613
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.add-to-unscopables.js
@@ -0,0 +1,7 @@
+// 22.1.3.31 Array.prototype[@@unscopables]
+var UNSCOPABLES = require('./$.wks')('unscopables')
+ , ArrayProto = Array.prototype;
+if(ArrayProto[UNSCOPABLES] == undefined)require('./$.hide')(ArrayProto, UNSCOPABLES, {});
+module.exports = function(key){
+ ArrayProto[UNSCOPABLES][key] = true;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.an-object.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.an-object.js
new file mode 100644
index 00000000..e5c808fd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.an-object.js
@@ -0,0 +1,5 @@
+var isObject = require('./$.is-object');
+module.exports = function(it){
+ if(!isObject(it))throw TypeError(it + ' is not an object!');
+ return it;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.array-copy-within.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.array-copy-within.js
new file mode 100644
index 00000000..58563065
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.array-copy-within.js
@@ -0,0 +1,27 @@
+// 22.1.3.3 Array.prototype.copyWithin(target, start, end = this.length)
+'use strict';
+var toObject = require('./$.to-object')
+ , toIndex = require('./$.to-index')
+ , toLength = require('./$.to-length');
+
+module.exports = [].copyWithin || function copyWithin(target/*= 0*/, start/*= 0, end = @length*/){
+ var O = toObject(this)
+ , len = toLength(O.length)
+ , to = toIndex(target, len)
+ , from = toIndex(start, len)
+ , $$ = arguments
+ , end = $$.length > 2 ? $$[2] : undefined
+ , count = Math.min((end === undefined ? len : toIndex(end, len)) - from, len - to)
+ , inc = 1;
+ if(from < to && to < from + count){
+ inc = -1;
+ from += count - 1;
+ to += count - 1;
+ }
+ while(count-- > 0){
+ if(from in O)O[to] = O[from];
+ else delete O[to];
+ to += inc;
+ from += inc;
+ } return O;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.array-fill.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.array-fill.js
new file mode 100644
index 00000000..6b699df7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.array-fill.js
@@ -0,0 +1,16 @@
+// 22.1.3.6 Array.prototype.fill(value, start = 0, end = this.length)
+'use strict';
+var toObject = require('./$.to-object')
+ , toIndex = require('./$.to-index')
+ , toLength = require('./$.to-length');
+module.exports = [].fill || function fill(value /*, start = 0, end = @length */){
+ var O = toObject(this)
+ , length = toLength(O.length)
+ , $$ = arguments
+ , $$len = $$.length
+ , index = toIndex($$len > 1 ? $$[1] : undefined, length)
+ , end = $$len > 2 ? $$[2] : undefined
+ , endPos = end === undefined ? length : toIndex(end, length);
+ while(endPos > index)O[index++] = value;
+ return O;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.array-includes.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.array-includes.js
new file mode 100644
index 00000000..9781fca7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.array-includes.js
@@ -0,0 +1,21 @@
+// false -> Array#indexOf
+// true -> Array#includes
+var toIObject = require('./$.to-iobject')
+ , toLength = require('./$.to-length')
+ , toIndex = require('./$.to-index');
+module.exports = function(IS_INCLUDES){
+ return function($this, el, fromIndex){
+ var O = toIObject($this)
+ , length = toLength(O.length)
+ , index = toIndex(fromIndex, length)
+ , value;
+ // Array#includes uses SameValueZero equality algorithm
+ if(IS_INCLUDES && el != el)while(length > index){
+ value = O[index++];
+ if(value != value)return true;
+ // Array#toIndex ignores holes, Array#includes - not
+ } else for(;length > index; index++)if(IS_INCLUDES || index in O){
+ if(O[index] === el)return IS_INCLUDES || index;
+ } return !IS_INCLUDES && -1;
+ };
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.array-methods.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.array-methods.js
new file mode 100644
index 00000000..e70b99f4
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.array-methods.js
@@ -0,0 +1,43 @@
+// 0 -> Array#forEach
+// 1 -> Array#map
+// 2 -> Array#filter
+// 3 -> Array#some
+// 4 -> Array#every
+// 5 -> Array#find
+// 6 -> Array#findIndex
+var ctx = require('./$.ctx')
+ , IObject = require('./$.iobject')
+ , toObject = require('./$.to-object')
+ , toLength = require('./$.to-length')
+ , asc = require('./$.array-species-create');
+module.exports = function(TYPE){
+ var IS_MAP = TYPE == 1
+ , IS_FILTER = TYPE == 2
+ , IS_SOME = TYPE == 3
+ , IS_EVERY = TYPE == 4
+ , IS_FIND_INDEX = TYPE == 6
+ , NO_HOLES = TYPE == 5 || IS_FIND_INDEX;
+ return function($this, callbackfn, that){
+ var O = toObject($this)
+ , self = IObject(O)
+ , f = ctx(callbackfn, that, 3)
+ , length = toLength(self.length)
+ , index = 0
+ , result = IS_MAP ? asc($this, length) : IS_FILTER ? asc($this, 0) : undefined
+ , val, res;
+ for(;length > index; index++)if(NO_HOLES || index in self){
+ val = self[index];
+ res = f(val, index, O);
+ if(TYPE){
+ if(IS_MAP)result[index] = res; // map
+ else if(res)switch(TYPE){
+ case 3: return true; // some
+ case 5: return val; // find
+ case 6: return index; // findIndex
+ case 2: result.push(val); // filter
+ } else if(IS_EVERY)return false; // every
+ }
+ }
+ return IS_FIND_INDEX ? -1 : IS_SOME || IS_EVERY ? IS_EVERY : result;
+ };
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.array-species-create.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.array-species-create.js
new file mode 100644
index 00000000..d809cae6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.array-species-create.js
@@ -0,0 +1,16 @@
+// 9.4.2.3 ArraySpeciesCreate(originalArray, length)
+var isObject = require('./$.is-object')
+ , isArray = require('./$.is-array')
+ , SPECIES = require('./$.wks')('species');
+module.exports = function(original, length){
+ var C;
+ if(isArray(original)){
+ C = original.constructor;
+ // cross-realm fallback
+ if(typeof C == 'function' && (C === Array || isArray(C.prototype)))C = undefined;
+ if(isObject(C)){
+ C = C[SPECIES];
+ if(C === null)C = undefined;
+ }
+ } return new (C === undefined ? Array : C)(length);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.buffer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.buffer.js
new file mode 100644
index 00000000..d1aae58a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.buffer.js
@@ -0,0 +1,288 @@
+'use strict';
+var $ = require('./$')
+ , global = require('./$.global')
+ , $typed = require('./$.typed')
+ , redefineAll = require('./$.redefine-all')
+ , strictNew = require('./$.strict-new')
+ , toInteger = require('./$.to-integer')
+ , toLength = require('./$.to-length')
+ , arrayFill = require('./$.array-fill')
+ , $ArrayBuffer = global.ArrayBuffer
+ , $DataView = global.DataView
+ , Math = global.Math
+ , parseInt = global.parseInt
+ , abs = Math.abs
+ , pow = Math.pow
+ , min = Math.min
+ , floor = Math.floor
+ , log = Math.log
+ , LN2 = Math.LN2
+ , BYTE_LENGTH = 'byteLength';
+
+// pack / unpack based on
+// https://github.com/inexorabletash/polyfill/blob/v0.1.11/typedarray.js#L123-L264
+// TODO: simplify
+var signed = function(value, bits){
+ var s = 32 - bits;
+ return value << s >> s;
+};
+var unsigned = function(value, bits){
+ var s = 32 - bits;
+ return value << s >>> s;
+};
+var roundToEven = function(n){
+ var w = floor(n)
+ , f = n - w;
+ return f < .5 ? w : f > .5 ? w + 1 : w % 2 ? w + 1 : w;
+};
+var packI8 = function(n){
+ return [n & 0xff];
+};
+var unpackI8 = function(bytes){
+ return signed(bytes[0], 8);
+};
+var packU8 = function(n){
+ return [n & 0xff];
+};
+var unpackU8 = function(bytes){
+ return unsigned(bytes[0], 8);
+};
+var packI16 = function(n){
+ return [n & 0xff, n >> 8 & 0xff];
+};
+var unpackI16 = function(bytes){
+ return signed(bytes[1] << 8 | bytes[0], 16);
+};
+var packU16 = function(n){
+ return [n & 0xff, n >> 8 & 0xff];
+};
+var unpackU16 = function(bytes){
+ return unsigned(bytes[1] << 8 | bytes[0], 16);
+};
+var packI32 = function(n){
+ return [n & 0xff, n >> 8 & 0xff, n >> 16 & 0xff, n >> 24 & 0xff];
+};
+var unpackI32 = function(bytes){
+ return signed(bytes[3] << 24 | bytes[2] << 16 | bytes[1] << 8 | bytes[0], 32);
+};
+var packU32 = function(n){
+ return [n & 0xff, n >> 8 & 0xff, n >> 16 & 0xff, n >> 24 & 0xff];
+};
+var unpackU32 = function(bytes){
+ return unsigned(bytes[3] << 24 | bytes[2] << 16 | bytes[1] << 8 | bytes[0], 32);
+};
+var packIEEE754 = function(v, ebits, fbits) {
+ var bias = (1 << ebits - 1) - 1
+ , s, e, f, i, bits, str, bytes;
+ // Compute sign, exponent, fraction
+ if (v !== v) {
+ // NaN
+ // http://dev.w3.org/2006/webapi/WebIDL/#es-type-mapping
+ e = (1 << ebits) - 1;
+ f = pow(2, fbits - 1);
+ s = 0;
+ } else if(v === Infinity || v === -Infinity){
+ e = (1 << ebits) - 1;
+ f = 0;
+ s = v < 0 ? 1 : 0;
+ } else if(v === 0){
+ e = 0;
+ f = 0;
+ s = 1 / v === -Infinity ? 1 : 0;
+ } else {
+ s = v < 0;
+ v = abs(v);
+ if(v >= pow(2, 1 - bias)){
+ e = min(floor(log(v) / LN2), 1023);
+ var significand = v / pow(2, e);
+ if(significand < 1){
+ e -= 1;
+ significand *= 2;
+ }
+ if(significand >= 2){
+ e += 1;
+ significand /= 2;
+ }
+ f = roundToEven(significand * pow(2, fbits));
+ if(f / pow(2, fbits) >= 2){
+ e = e + 1;
+ f = 1;
+ }
+ if(e > bias){
+ // Overflow
+ e = (1 << ebits) - 1;
+ f = 0;
+ } else {
+ // Normalized
+ e = e + bias;
+ f = f - pow(2, fbits);
+ }
+ } else {
+ // Denormalized
+ e = 0;
+ f = roundToEven(v / pow(2, 1 - bias - fbits));
+ }
+ }
+ // Pack sign, exponent, fraction
+ bits = [];
+ for(i = fbits; i; i -= 1){
+ bits.push(f % 2 ? 1 : 0);
+ f = floor(f / 2);
+ }
+ for(i = ebits; i; i -= 1){
+ bits.push(e % 2 ? 1 : 0);
+ e = floor(e / 2);
+ }
+ bits.push(s ? 1 : 0);
+ bits.reverse();
+ str = bits.join('');
+ // Bits to bytes
+ bytes = [];
+ while(str.length){
+ bytes.unshift(parseInt(str.slice(0, 8), 2));
+ str = str.slice(8);
+ }
+ return bytes;
+};
+var unpackIEEE754 = function(bytes, ebits, fbits){
+ var bits = []
+ , i, j, b, str, bias, s, e, f;
+ for(i = 0; i < bytes.length; ++i)for(b = bytes[i], j = 8; j; --j){
+ bits.push(b % 2 ? 1 : 0);
+ b = b >> 1;
+ }
+ bits.reverse();
+ str = bits.join('');
+ // Unpack sign, exponent, fraction
+ bias = (1 << ebits - 1) - 1;
+ s = parseInt(str.slice(0, 1), 2) ? -1 : 1;
+ e = parseInt(str.slice(1, 1 + ebits), 2);
+ f = parseInt(str.slice(1 + ebits), 2);
+ // Produce number
+ if(e === (1 << ebits) - 1)return f !== 0 ? NaN : s * Infinity;
+ // Normalized
+ else if(e > 0)return s * pow(2, e - bias) * (1 + f / pow(2, fbits));
+ // Denormalized
+ else if(f !== 0)return s * pow(2, -(bias - 1)) * (f / pow(2, fbits));
+ return s < 0 ? -0 : 0;
+};
+var unpackF64 = function(b){
+ return unpackIEEE754(b, 11, 52);
+};
+var packF64 = function(v){
+ return packIEEE754(v, 11, 52);
+};
+var unpackF32 = function(b){
+ return unpackIEEE754(b, 8, 23);
+};
+var packF32 = function(v){
+ return packIEEE754(v, 8, 23);
+};
+
+var addGetter = function(C, key, internal){
+ $.setDesc(C.prototype, key, {get: function(){ return this[internal]; }});
+};
+
+var get = function(view, bytes, index, conversion, isLittleEndian){
+ var numIndex = +index
+ , intIndex = toInteger(numIndex);
+ if(numIndex != intIndex || intIndex < 0 || intIndex + bytes > view._l)throw RangeError();
+ var store = view._b._b
+ , start = intIndex + view._o
+ , pack = store.slice(start, start + bytes);
+ isLittleEndian || pack.reverse();
+ return conversion(pack);
+};
+var set = function(view, bytes, index, conversion, value, isLittleEndian){
+ var numIndex = +index
+ , intIndex = toInteger(numIndex);
+ if(numIndex != intIndex || intIndex < 0 || intIndex + bytes > view._l)throw RangeError();
+ var store = view._b._b
+ , start = intIndex + view._o
+ , pack = conversion(+value);
+ isLittleEndian || pack.reverse();
+ for(var i = 0; i < bytes; i++)store[start + i] = pack[i];
+};
+
+if(!$typed.ABV){
+ $ArrayBuffer = function ArrayBuffer(length){
+ strictNew(this, $ArrayBuffer, 'ArrayBuffer');
+ var numberLength = +length
+ , byteLength = toLength(numberLength);
+ if(numberLength != byteLength)throw RangeError();
+ this._b = arrayFill.call(Array(byteLength), 0);
+ this._l = byteLength;
+ };
+ addGetter($ArrayBuffer, BYTE_LENGTH, '_l');
+
+ $DataView = function DataView(buffer, byteOffset, byteLength){
+ strictNew(this, $DataView, 'DataView');
+ if(!(buffer instanceof $ArrayBuffer))throw TypeError();
+ var bufferLength = buffer._l
+ , offset = toInteger(byteOffset);
+ if(offset < 0 || offset > bufferLength)throw RangeError();
+ byteLength = byteLength === undefined ? bufferLength - offset : toLength(byteLength);
+ if(offset + byteLength > bufferLength)throw RangeError();
+ this._b = buffer;
+ this._o = offset;
+ this._l = byteLength;
+ };
+ addGetter($DataView, 'buffer', '_b');
+ addGetter($DataView, BYTE_LENGTH, '_l');
+ addGetter($DataView, 'byteOffset', '_o');
+ redefineAll($DataView.prototype, {
+ getInt8: function getInt8(byteOffset){
+ return get(this, 1, byteOffset, unpackI8);
+ },
+ getUint8: function getUint8(byteOffset){
+ return get(this, 1, byteOffset, unpackU8);
+ },
+ getInt16: function getInt16(byteOffset /*, littleEndian */){
+ return get(this, 2, byteOffset, unpackI16, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ getUint16: function getUint16(byteOffset /*, littleEndian */){
+ return get(this, 2, byteOffset, unpackU16, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ getInt32: function getInt32(byteOffset /*, littleEndian */){
+ return get(this, 4, byteOffset, unpackI32, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ getUint32: function getUint32(byteOffset /*, littleEndian */){
+ return get(this, 4, byteOffset, unpackU32, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ getFloat32: function getFloat32(byteOffset /*, littleEndian */){
+ return get(this, 4, byteOffset, unpackF32, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ getFloat64: function getFloat64(byteOffset /*, littleEndian */){
+ return get(this, 8, byteOffset, unpackF64, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ setInt8: function setInt8(byteOffset, value){
+ return set(this, 1, byteOffset, packI8, value);
+ },
+ setUint8: function setUint8(byteOffset, value){
+ return set(this, 1, byteOffset, packU8, value);
+ },
+ setInt16: function setInt16(byteOffset, value /*, littleEndian */){
+ return set(this, 2, byteOffset, packI16, value, arguments.length > 2 ? arguments[2] : undefined);
+ },
+ setUint16: function setUint16(byteOffset, value /*, littleEndian */){
+ return set(this, 2, byteOffset, packU16, value, arguments.length > 2 ? arguments[2] : undefined);
+ },
+ setInt32: function setInt32(byteOffset, value /*, littleEndian */){
+ return set(this, 4, byteOffset, packI32, value, arguments.length > 2 ? arguments[2] : undefined);
+ },
+ setUint32: function setUint32(byteOffset, value /*, littleEndian */){
+ return set(this, 4, byteOffset, packU32, value, arguments.length > 2 ? arguments[2] : undefined);
+ },
+ setFloat32: function setFloat32(byteOffset, value /*, littleEndian */){
+ return set(this, 4, byteOffset, packF32, value, arguments.length > 2 ? arguments[2] : undefined);
+ },
+ setFloat64: function setFloat64(byteOffset, value /*, littleEndian */){
+ return set(this, 8, byteOffset, packF64, value, arguments.length > 2 ? arguments[2] : undefined);
+ }
+ });
+}
+require('./$.hide')($DataView.prototype, $typed.VIEW, true);
+module.exports = {
+ ArrayBuffer: $ArrayBuffer,
+ DataView: $DataView
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.classof.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.classof.js
new file mode 100644
index 00000000..905c61f7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.classof.js
@@ -0,0 +1,16 @@
+// getting tag from 19.1.3.6 Object.prototype.toString()
+var cof = require('./$.cof')
+ , TAG = require('./$.wks')('toStringTag')
+ // ES3 wrong here
+ , ARG = cof(function(){ return arguments; }()) == 'Arguments';
+
+module.exports = function(it){
+ var O, T, B;
+ return it === undefined ? 'Undefined' : it === null ? 'Null'
+ // @@toStringTag case
+ : typeof (T = (O = Object(it))[TAG]) == 'string' ? T
+ // builtinTag case
+ : ARG ? cof(O)
+ // ES3 arguments fallback
+ : (B = cof(O)) == 'Object' && typeof O.callee == 'function' ? 'Arguments' : B;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.cof.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.cof.js
new file mode 100644
index 00000000..1dd2779a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.cof.js
@@ -0,0 +1,5 @@
+var toString = {}.toString;
+
+module.exports = function(it){
+ return toString.call(it).slice(8, -1);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.collection-strong.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.collection-strong.js
new file mode 100644
index 00000000..54df55a6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.collection-strong.js
@@ -0,0 +1,159 @@
+'use strict';
+var $ = require('./$')
+ , hide = require('./$.hide')
+ , redefineAll = require('./$.redefine-all')
+ , ctx = require('./$.ctx')
+ , strictNew = require('./$.strict-new')
+ , defined = require('./$.defined')
+ , forOf = require('./$.for-of')
+ , $iterDefine = require('./$.iter-define')
+ , step = require('./$.iter-step')
+ , ID = require('./$.uid')('id')
+ , $has = require('./$.has')
+ , isObject = require('./$.is-object')
+ , setSpecies = require('./$.set-species')
+ , DESCRIPTORS = require('./$.descriptors')
+ , isExtensible = Object.isExtensible || isObject
+ , SIZE = DESCRIPTORS ? '_s' : 'size'
+ , id = 0;
+
+var fastKey = function(it, create){
+ // return primitive with prefix
+ if(!isObject(it))return typeof it == 'symbol' ? it : (typeof it == 'string' ? 'S' : 'P') + it;
+ if(!$has(it, ID)){
+ // can't set id to frozen object
+ if(!isExtensible(it))return 'F';
+ // not necessary to add id
+ if(!create)return 'E';
+ // add missing object id
+ hide(it, ID, ++id);
+ // return object id with prefix
+ } return 'O' + it[ID];
+};
+
+var getEntry = function(that, key){
+ // fast case
+ var index = fastKey(key), entry;
+ if(index !== 'F')return that._i[index];
+ // frozen object case
+ for(entry = that._f; entry; entry = entry.n){
+ if(entry.k == key)return entry;
+ }
+};
+
+module.exports = {
+ getConstructor: function(wrapper, NAME, IS_MAP, ADDER){
+ var C = wrapper(function(that, iterable){
+ strictNew(that, C, NAME);
+ that._i = $.create(null); // index
+ that._f = undefined; // first entry
+ that._l = undefined; // last entry
+ that[SIZE] = 0; // size
+ if(iterable != undefined)forOf(iterable, IS_MAP, that[ADDER], that);
+ });
+ redefineAll(C.prototype, {
+ // 23.1.3.1 Map.prototype.clear()
+ // 23.2.3.2 Set.prototype.clear()
+ clear: function clear(){
+ for(var that = this, data = that._i, entry = that._f; entry; entry = entry.n){
+ entry.r = true;
+ if(entry.p)entry.p = entry.p.n = undefined;
+ delete data[entry.i];
+ }
+ that._f = that._l = undefined;
+ that[SIZE] = 0;
+ },
+ // 23.1.3.3 Map.prototype.delete(key)
+ // 23.2.3.4 Set.prototype.delete(value)
+ 'delete': function(key){
+ var that = this
+ , entry = getEntry(that, key);
+ if(entry){
+ var next = entry.n
+ , prev = entry.p;
+ delete that._i[entry.i];
+ entry.r = true;
+ if(prev)prev.n = next;
+ if(next)next.p = prev;
+ if(that._f == entry)that._f = next;
+ if(that._l == entry)that._l = prev;
+ that[SIZE]--;
+ } return !!entry;
+ },
+ // 23.2.3.6 Set.prototype.forEach(callbackfn, thisArg = undefined)
+ // 23.1.3.5 Map.prototype.forEach(callbackfn, thisArg = undefined)
+ forEach: function forEach(callbackfn /*, that = undefined */){
+ var f = ctx(callbackfn, arguments.length > 1 ? arguments[1] : undefined, 3)
+ , entry;
+ while(entry = entry ? entry.n : this._f){
+ f(entry.v, entry.k, this);
+ // revert to the last existing entry
+ while(entry && entry.r)entry = entry.p;
+ }
+ },
+ // 23.1.3.7 Map.prototype.has(key)
+ // 23.2.3.7 Set.prototype.has(value)
+ has: function has(key){
+ return !!getEntry(this, key);
+ }
+ });
+ if(DESCRIPTORS)$.setDesc(C.prototype, 'size', {
+ get: function(){
+ return defined(this[SIZE]);
+ }
+ });
+ return C;
+ },
+ def: function(that, key, value){
+ var entry = getEntry(that, key)
+ , prev, index;
+ // change existing entry
+ if(entry){
+ entry.v = value;
+ // create new entry
+ } else {
+ that._l = entry = {
+ i: index = fastKey(key, true), // <- index
+ k: key, // <- key
+ v: value, // <- value
+ p: prev = that._l, // <- previous entry
+ n: undefined, // <- next entry
+ r: false // <- removed
+ };
+ if(!that._f)that._f = entry;
+ if(prev)prev.n = entry;
+ that[SIZE]++;
+ // add to index
+ if(index !== 'F')that._i[index] = entry;
+ } return that;
+ },
+ getEntry: getEntry,
+ setStrong: function(C, NAME, IS_MAP){
+ // add .keys, .values, .entries, [@@iterator]
+ // 23.1.3.4, 23.1.3.8, 23.1.3.11, 23.1.3.12, 23.2.3.5, 23.2.3.8, 23.2.3.10, 23.2.3.11
+ $iterDefine(C, NAME, function(iterated, kind){
+ this._t = iterated; // target
+ this._k = kind; // kind
+ this._l = undefined; // previous
+ }, function(){
+ var that = this
+ , kind = that._k
+ , entry = that._l;
+ // revert to the last existing entry
+ while(entry && entry.r)entry = entry.p;
+ // get next entry
+ if(!that._t || !(that._l = entry = entry ? entry.n : that._t._f)){
+ // or finish the iteration
+ that._t = undefined;
+ return step(1);
+ }
+ // return step by kind
+ if(kind == 'keys' )return step(0, entry.k);
+ if(kind == 'values')return step(0, entry.v);
+ return step(0, [entry.k, entry.v]);
+ }, IS_MAP ? 'entries' : 'values' , !IS_MAP, true);
+
+ // add [@@species], 23.1.2.2, 23.2.2.2
+ setSpecies(NAME);
+ }
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.collection-to-json.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.collection-to-json.js
new file mode 100644
index 00000000..41f2e6ec
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.collection-to-json.js
@@ -0,0 +1,11 @@
+// https://github.com/DavidBruant/Map-Set.prototype.toJSON
+var forOf = require('./$.for-of')
+ , classof = require('./$.classof');
+module.exports = function(NAME){
+ return function toJSON(){
+ if(classof(this) != NAME)throw TypeError(NAME + "#toJSON isn't generic");
+ var arr = [];
+ forOf(this, false, arr.push, arr);
+ return arr;
+ };
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.collection-weak.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.collection-weak.js
new file mode 100644
index 00000000..384fb39d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.collection-weak.js
@@ -0,0 +1,86 @@
+'use strict';
+var hide = require('./$.hide')
+ , redefineAll = require('./$.redefine-all')
+ , anObject = require('./$.an-object')
+ , isObject = require('./$.is-object')
+ , strictNew = require('./$.strict-new')
+ , forOf = require('./$.for-of')
+ , createArrayMethod = require('./$.array-methods')
+ , $has = require('./$.has')
+ , WEAK = require('./$.uid')('weak')
+ , isExtensible = Object.isExtensible || isObject
+ , arrayFind = createArrayMethod(5)
+ , arrayFindIndex = createArrayMethod(6)
+ , id = 0;
+
+// fallback for frozen keys
+var frozenStore = function(that){
+ return that._l || (that._l = new FrozenStore);
+};
+var FrozenStore = function(){
+ this.a = [];
+};
+var findFrozen = function(store, key){
+ return arrayFind(store.a, function(it){
+ return it[0] === key;
+ });
+};
+FrozenStore.prototype = {
+ get: function(key){
+ var entry = findFrozen(this, key);
+ if(entry)return entry[1];
+ },
+ has: function(key){
+ return !!findFrozen(this, key);
+ },
+ set: function(key, value){
+ var entry = findFrozen(this, key);
+ if(entry)entry[1] = value;
+ else this.a.push([key, value]);
+ },
+ 'delete': function(key){
+ var index = arrayFindIndex(this.a, function(it){
+ return it[0] === key;
+ });
+ if(~index)this.a.splice(index, 1);
+ return !!~index;
+ }
+};
+
+module.exports = {
+ getConstructor: function(wrapper, NAME, IS_MAP, ADDER){
+ var C = wrapper(function(that, iterable){
+ strictNew(that, C, NAME);
+ that._i = id++; // collection id
+ that._l = undefined; // leak store for frozen objects
+ if(iterable != undefined)forOf(iterable, IS_MAP, that[ADDER], that);
+ });
+ redefineAll(C.prototype, {
+ // 23.3.3.2 WeakMap.prototype.delete(key)
+ // 23.4.3.3 WeakSet.prototype.delete(value)
+ 'delete': function(key){
+ if(!isObject(key))return false;
+ if(!isExtensible(key))return frozenStore(this)['delete'](key);
+ return $has(key, WEAK) && $has(key[WEAK], this._i) && delete key[WEAK][this._i];
+ },
+ // 23.3.3.4 WeakMap.prototype.has(key)
+ // 23.4.3.4 WeakSet.prototype.has(value)
+ has: function has(key){
+ if(!isObject(key))return false;
+ if(!isExtensible(key))return frozenStore(this).has(key);
+ return $has(key, WEAK) && $has(key[WEAK], this._i);
+ }
+ });
+ return C;
+ },
+ def: function(that, key, value){
+ if(!isExtensible(anObject(key))){
+ frozenStore(that).set(key, value);
+ } else {
+ $has(key, WEAK) || hide(key, WEAK, {});
+ key[WEAK][that._i] = value;
+ } return that;
+ },
+ frozenStore: frozenStore,
+ WEAK: WEAK
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.collection.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.collection.js
new file mode 100644
index 00000000..61ae123d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.collection.js
@@ -0,0 +1,79 @@
+'use strict';
+var global = require('./$.global')
+ , $export = require('./$.export')
+ , redefine = require('./$.redefine')
+ , redefineAll = require('./$.redefine-all')
+ , forOf = require('./$.for-of')
+ , strictNew = require('./$.strict-new')
+ , isObject = require('./$.is-object')
+ , fails = require('./$.fails')
+ , $iterDetect = require('./$.iter-detect')
+ , setToStringTag = require('./$.set-to-string-tag');
+
+module.exports = function(NAME, wrapper, methods, common, IS_MAP, IS_WEAK){
+ var Base = global[NAME]
+ , C = Base
+ , ADDER = IS_MAP ? 'set' : 'add'
+ , proto = C && C.prototype
+ , O = {};
+ var fixMethod = function(KEY){
+ var fn = proto[KEY];
+ redefine(proto, KEY,
+ KEY == 'delete' ? function(a){
+ return IS_WEAK && !isObject(a) ? false : fn.call(this, a === 0 ? 0 : a);
+ } : KEY == 'has' ? function has(a){
+ return IS_WEAK && !isObject(a) ? false : fn.call(this, a === 0 ? 0 : a);
+ } : KEY == 'get' ? function get(a){
+ return IS_WEAK && !isObject(a) ? undefined : fn.call(this, a === 0 ? 0 : a);
+ } : KEY == 'add' ? function add(a){ fn.call(this, a === 0 ? 0 : a); return this; }
+ : function set(a, b){ fn.call(this, a === 0 ? 0 : a, b); return this; }
+ );
+ };
+ if(typeof C != 'function' || !(IS_WEAK || proto.forEach && !fails(function(){
+ new C().entries().next();
+ }))){
+ // create collection constructor
+ C = common.getConstructor(wrapper, NAME, IS_MAP, ADDER);
+ redefineAll(C.prototype, methods);
+ } else {
+ var instance = new C
+ // early implementations not supports chaining
+ , HASNT_CHAINING = instance[ADDER](IS_WEAK ? {} : -0, 1) != instance
+ // V8 ~ Chromium 40- weak-collections throws on primitives, but should return false
+ , THROWS_ON_PRIMITIVES = fails(function(){ instance.has(1); })
+ // most early implementations doesn't supports iterables, most modern - not close it correctly
+ , ACCEPT_ITERABLES = $iterDetect(function(iter){ new C(iter); }) // eslint-disable-line no-new
+ // for early implementations -0 and +0 not the same
+ , BUGGY_ZERO;
+ if(!ACCEPT_ITERABLES){
+ C = wrapper(function(target, iterable){
+ strictNew(target, C, NAME);
+ var that = new Base;
+ if(iterable != undefined)forOf(iterable, IS_MAP, that[ADDER], that);
+ return that;
+ });
+ C.prototype = proto;
+ proto.constructor = C;
+ }
+ IS_WEAK || instance.forEach(function(val, key){
+ BUGGY_ZERO = 1 / key === -Infinity;
+ });
+ if(THROWS_ON_PRIMITIVES || BUGGY_ZERO){
+ fixMethod('delete');
+ fixMethod('has');
+ IS_MAP && fixMethod('get');
+ }
+ if(BUGGY_ZERO || HASNT_CHAINING)fixMethod(ADDER);
+ // weak collections should not contains .clear method
+ if(IS_WEAK && proto.clear)delete proto.clear;
+ }
+
+ setToStringTag(C, NAME);
+
+ O[NAME] = C;
+ $export($export.G + $export.W + $export.F * (C != Base), O);
+
+ if(!IS_WEAK)common.setStrong(C, NAME, IS_MAP);
+
+ return C;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.core.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.core.js
new file mode 100644
index 00000000..4e2a0b59
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.core.js
@@ -0,0 +1,2 @@
+var core = module.exports = {version: '1.2.6'};
+if(typeof __e == 'number')__e = core; // eslint-disable-line no-undef
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.ctx.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.ctx.js
new file mode 100644
index 00000000..d233574a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.ctx.js
@@ -0,0 +1,20 @@
+// optional / simple context binding
+var aFunction = require('./$.a-function');
+module.exports = function(fn, that, length){
+ aFunction(fn);
+ if(that === undefined)return fn;
+ switch(length){
+ case 1: return function(a){
+ return fn.call(that, a);
+ };
+ case 2: return function(a, b){
+ return fn.call(that, a, b);
+ };
+ case 3: return function(a, b, c){
+ return fn.call(that, a, b, c);
+ };
+ }
+ return function(/* ...args */){
+ return fn.apply(that, arguments);
+ };
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.defined.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.defined.js
new file mode 100644
index 00000000..cfa476b9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.defined.js
@@ -0,0 +1,5 @@
+// 7.2.1 RequireObjectCoercible(argument)
+module.exports = function(it){
+ if(it == undefined)throw TypeError("Can't call method on " + it);
+ return it;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.descriptors.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.descriptors.js
new file mode 100644
index 00000000..9cd47b7d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.descriptors.js
@@ -0,0 +1,4 @@
+// Thank's IE8 for his funny defineProperty
+module.exports = !require('./$.fails')(function(){
+ return Object.defineProperty({}, 'a', {get: function(){ return 7; }}).a != 7;
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.dom-create.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.dom-create.js
new file mode 100644
index 00000000..240842d2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.dom-create.js
@@ -0,0 +1,7 @@
+var isObject = require('./$.is-object')
+ , document = require('./$.global').document
+ // in old IE typeof document.createElement is 'object'
+ , is = isObject(document) && isObject(document.createElement);
+module.exports = function(it){
+ return is ? document.createElement(it) : {};
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.enum-keys.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.enum-keys.js
new file mode 100644
index 00000000..06f7de7a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.enum-keys.js
@@ -0,0 +1,14 @@
+// all enumerable object keys, includes symbols
+var $ = require('./$');
+module.exports = function(it){
+ var keys = $.getKeys(it)
+ , getSymbols = $.getSymbols;
+ if(getSymbols){
+ var symbols = getSymbols(it)
+ , isEnum = $.isEnum
+ , i = 0
+ , key;
+ while(symbols.length > i)if(isEnum.call(it, key = symbols[i++]))keys.push(key);
+ }
+ return keys;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.export.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.export.js
new file mode 100644
index 00000000..5d4fea03
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.export.js
@@ -0,0 +1,41 @@
+var global = require('./$.global')
+ , core = require('./$.core')
+ , hide = require('./$.hide')
+ , redefine = require('./$.redefine')
+ , ctx = require('./$.ctx')
+ , PROTOTYPE = 'prototype';
+
+var $export = function(type, name, source){
+ var IS_FORCED = type & $export.F
+ , IS_GLOBAL = type & $export.G
+ , IS_STATIC = type & $export.S
+ , IS_PROTO = type & $export.P
+ , IS_BIND = type & $export.B
+ , target = IS_GLOBAL ? global : IS_STATIC ? global[name] || (global[name] = {}) : (global[name] || {})[PROTOTYPE]
+ , exports = IS_GLOBAL ? core : core[name] || (core[name] = {})
+ , expProto = exports[PROTOTYPE] || (exports[PROTOTYPE] = {})
+ , key, own, out, exp;
+ if(IS_GLOBAL)source = name;
+ for(key in source){
+ // contains in native
+ own = !IS_FORCED && target && key in target;
+ // export native or passed
+ out = (own ? target : source)[key];
+ // bind timers to global for call from export context
+ exp = IS_BIND && own ? ctx(out, global) : IS_PROTO && typeof out == 'function' ? ctx(Function.call, out) : out;
+ // extend global
+ if(target && !own)redefine(target, key, out);
+ // export
+ if(exports[key] != out)hide(exports, key, exp);
+ if(IS_PROTO && expProto[key] != out)expProto[key] = out;
+ }
+};
+global.core = core;
+// type bitmap
+$export.F = 1; // forced
+$export.G = 2; // global
+$export.S = 4; // static
+$export.P = 8; // proto
+$export.B = 16; // bind
+$export.W = 32; // wrap
+module.exports = $export;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.fails-is-regexp.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.fails-is-regexp.js
new file mode 100644
index 00000000..c459a77a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.fails-is-regexp.js
@@ -0,0 +1,12 @@
+var MATCH = require('./$.wks')('match');
+module.exports = function(KEY){
+ var re = /./;
+ try {
+ '/./'[KEY](re);
+ } catch(e){
+ try {
+ re[MATCH] = false;
+ return !'/./'[KEY](re);
+ } catch(f){ /* empty */ }
+ } return true;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.fails.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.fails.js
new file mode 100644
index 00000000..184e5ea8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.fails.js
@@ -0,0 +1,7 @@
+module.exports = function(exec){
+ try {
+ return !!exec();
+ } catch(e){
+ return true;
+ }
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.fix-re-wks.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.fix-re-wks.js
new file mode 100644
index 00000000..3597a898
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.fix-re-wks.js
@@ -0,0 +1,26 @@
+'use strict';
+var hide = require('./$.hide')
+ , redefine = require('./$.redefine')
+ , fails = require('./$.fails')
+ , defined = require('./$.defined')
+ , wks = require('./$.wks');
+
+module.exports = function(KEY, length, exec){
+ var SYMBOL = wks(KEY)
+ , original = ''[KEY];
+ if(fails(function(){
+ var O = {};
+ O[SYMBOL] = function(){ return 7; };
+ return ''[KEY](O) != 7;
+ })){
+ redefine(String.prototype, KEY, exec(defined, SYMBOL, original));
+ hide(RegExp.prototype, SYMBOL, length == 2
+ // 21.2.5.8 RegExp.prototype[@@replace](string, replaceValue)
+ // 21.2.5.11 RegExp.prototype[@@split](string, limit)
+ ? function(string, arg){ return original.call(string, this, arg); }
+ // 21.2.5.6 RegExp.prototype[@@match](string)
+ // 21.2.5.9 RegExp.prototype[@@search](string)
+ : function(string){ return original.call(string, this); }
+ );
+ }
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.flags.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.flags.js
new file mode 100644
index 00000000..fc20e5de
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.flags.js
@@ -0,0 +1,13 @@
+'use strict';
+// 21.2.5.3 get RegExp.prototype.flags
+var anObject = require('./$.an-object');
+module.exports = function(){
+ var that = anObject(this)
+ , result = '';
+ if(that.global) result += 'g';
+ if(that.ignoreCase) result += 'i';
+ if(that.multiline) result += 'm';
+ if(that.unicode) result += 'u';
+ if(that.sticky) result += 'y';
+ return result;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.for-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.for-of.js
new file mode 100644
index 00000000..0f2d8e97
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.for-of.js
@@ -0,0 +1,19 @@
+var ctx = require('./$.ctx')
+ , call = require('./$.iter-call')
+ , isArrayIter = require('./$.is-array-iter')
+ , anObject = require('./$.an-object')
+ , toLength = require('./$.to-length')
+ , getIterFn = require('./core.get-iterator-method');
+module.exports = function(iterable, entries, fn, that){
+ var iterFn = getIterFn(iterable)
+ , f = ctx(fn, that, entries ? 2 : 1)
+ , index = 0
+ , length, step, iterator;
+ if(typeof iterFn != 'function')throw TypeError(iterable + ' is not iterable!');
+ // fast case for arrays with default iterator
+ if(isArrayIter(iterFn))for(length = toLength(iterable.length); length > index; index++){
+ entries ? f(anObject(step = iterable[index])[0], step[1]) : f(iterable[index]);
+ } else for(iterator = iterFn.call(iterable); !(step = iterator.next()).done; ){
+ call(iterator, f, step.value, entries);
+ }
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.get-names.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.get-names.js
new file mode 100644
index 00000000..28209714
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.get-names.js
@@ -0,0 +1,20 @@
+// fallback for IE11 buggy Object.getOwnPropertyNames with iframe and window
+var toIObject = require('./$.to-iobject')
+ , getNames = require('./$').getNames
+ , toString = {}.toString;
+
+var windowNames = typeof window == 'object' && Object.getOwnPropertyNames
+ ? Object.getOwnPropertyNames(window) : [];
+
+var getWindowNames = function(it){
+ try {
+ return getNames(it);
+ } catch(e){
+ return windowNames.slice();
+ }
+};
+
+module.exports.get = function getOwnPropertyNames(it){
+ if(windowNames && toString.call(it) == '[object Window]')return getWindowNames(it);
+ return getNames(toIObject(it));
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.global.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.global.js
new file mode 100644
index 00000000..df6efb47
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.global.js
@@ -0,0 +1,4 @@
+// https://github.com/zloirock/core-js/issues/86#issuecomment-115759028
+var global = module.exports = typeof window != 'undefined' && window.Math == Math
+ ? window : typeof self != 'undefined' && self.Math == Math ? self : Function('return this')();
+if(typeof __g == 'number')__g = global; // eslint-disable-line no-undef
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.has.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.has.js
new file mode 100644
index 00000000..870b40e7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.has.js
@@ -0,0 +1,4 @@
+var hasOwnProperty = {}.hasOwnProperty;
+module.exports = function(it, key){
+ return hasOwnProperty.call(it, key);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.hide.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.hide.js
new file mode 100644
index 00000000..ba025d81
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.hide.js
@@ -0,0 +1,8 @@
+var $ = require('./$')
+ , createDesc = require('./$.property-desc');
+module.exports = require('./$.descriptors') ? function(object, key, value){
+ return $.setDesc(object, key, createDesc(1, value));
+} : function(object, key, value){
+ object[key] = value;
+ return object;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.html.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.html.js
new file mode 100644
index 00000000..499bd2f3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.html.js
@@ -0,0 +1 @@
+module.exports = require('./$.global').document && document.documentElement;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.invoke.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.invoke.js
new file mode 100644
index 00000000..08e307fd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.invoke.js
@@ -0,0 +1,16 @@
+// fast apply, http://jsperf.lnkit.com/fast-apply/5
+module.exports = function(fn, args, that){
+ var un = that === undefined;
+ switch(args.length){
+ case 0: return un ? fn()
+ : fn.call(that);
+ case 1: return un ? fn(args[0])
+ : fn.call(that, args[0]);
+ case 2: return un ? fn(args[0], args[1])
+ : fn.call(that, args[0], args[1]);
+ case 3: return un ? fn(args[0], args[1], args[2])
+ : fn.call(that, args[0], args[1], args[2]);
+ case 4: return un ? fn(args[0], args[1], args[2], args[3])
+ : fn.call(that, args[0], args[1], args[2], args[3]);
+ } return fn.apply(that, args);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iobject.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iobject.js
new file mode 100644
index 00000000..cea38fab
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iobject.js
@@ -0,0 +1,5 @@
+// fallback for non-array-like ES3 and non-enumerable old V8 strings
+var cof = require('./$.cof');
+module.exports = Object('z').propertyIsEnumerable(0) ? Object : function(it){
+ return cof(it) == 'String' ? it.split('') : Object(it);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.is-array-iter.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.is-array-iter.js
new file mode 100644
index 00000000..b6ef7017
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.is-array-iter.js
@@ -0,0 +1,8 @@
+// check on default Array iterator
+var Iterators = require('./$.iterators')
+ , ITERATOR = require('./$.wks')('iterator')
+ , ArrayProto = Array.prototype;
+
+module.exports = function(it){
+ return it !== undefined && (Iterators.Array === it || ArrayProto[ITERATOR] === it);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.is-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.is-array.js
new file mode 100644
index 00000000..8168b21c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.is-array.js
@@ -0,0 +1,5 @@
+// 7.2.2 IsArray(argument)
+var cof = require('./$.cof');
+module.exports = Array.isArray || function(arg){
+ return cof(arg) == 'Array';
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.is-integer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.is-integer.js
new file mode 100644
index 00000000..b51e1317
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.is-integer.js
@@ -0,0 +1,6 @@
+// 20.1.2.3 Number.isInteger(number)
+var isObject = require('./$.is-object')
+ , floor = Math.floor;
+module.exports = function isInteger(it){
+ return !isObject(it) && isFinite(it) && floor(it) === it;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.is-object.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.is-object.js
new file mode 100644
index 00000000..ee694be2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.is-object.js
@@ -0,0 +1,3 @@
+module.exports = function(it){
+ return typeof it === 'object' ? it !== null : typeof it === 'function';
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.is-regexp.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.is-regexp.js
new file mode 100644
index 00000000..9ea2aad7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.is-regexp.js
@@ -0,0 +1,8 @@
+// 7.2.8 IsRegExp(argument)
+var isObject = require('./$.is-object')
+ , cof = require('./$.cof')
+ , MATCH = require('./$.wks')('match');
+module.exports = function(it){
+ var isRegExp;
+ return isObject(it) && ((isRegExp = it[MATCH]) !== undefined ? !!isRegExp : cof(it) == 'RegExp');
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iter-call.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iter-call.js
new file mode 100644
index 00000000..e6b9d1b1
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iter-call.js
@@ -0,0 +1,12 @@
+// call something on iterator step with safe closing on error
+var anObject = require('./$.an-object');
+module.exports = function(iterator, fn, value, entries){
+ try {
+ return entries ? fn(anObject(value)[0], value[1]) : fn(value);
+ // 7.4.6 IteratorClose(iterator, completion)
+ } catch(e){
+ var ret = iterator['return'];
+ if(ret !== undefined)anObject(ret.call(iterator));
+ throw e;
+ }
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iter-create.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iter-create.js
new file mode 100644
index 00000000..adebcf9a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iter-create.js
@@ -0,0 +1,13 @@
+'use strict';
+var $ = require('./$')
+ , descriptor = require('./$.property-desc')
+ , setToStringTag = require('./$.set-to-string-tag')
+ , IteratorPrototype = {};
+
+// 25.1.2.1.1 %IteratorPrototype%[@@iterator]()
+require('./$.hide')(IteratorPrototype, require('./$.wks')('iterator'), function(){ return this; });
+
+module.exports = function(Constructor, NAME, next){
+ Constructor.prototype = $.create(IteratorPrototype, {next: descriptor(1, next)});
+ setToStringTag(Constructor, NAME + ' Iterator');
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iter-define.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iter-define.js
new file mode 100644
index 00000000..630cdf38
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iter-define.js
@@ -0,0 +1,66 @@
+'use strict';
+var LIBRARY = require('./$.library')
+ , $export = require('./$.export')
+ , redefine = require('./$.redefine')
+ , hide = require('./$.hide')
+ , has = require('./$.has')
+ , Iterators = require('./$.iterators')
+ , $iterCreate = require('./$.iter-create')
+ , setToStringTag = require('./$.set-to-string-tag')
+ , getProto = require('./$').getProto
+ , ITERATOR = require('./$.wks')('iterator')
+ , BUGGY = !([].keys && 'next' in [].keys()) // Safari has buggy iterators w/o `next`
+ , FF_ITERATOR = '@@iterator'
+ , KEYS = 'keys'
+ , VALUES = 'values';
+
+var returnThis = function(){ return this; };
+
+module.exports = function(Base, NAME, Constructor, next, DEFAULT, IS_SET, FORCED){
+ $iterCreate(Constructor, NAME, next);
+ var getMethod = function(kind){
+ if(!BUGGY && kind in proto)return proto[kind];
+ switch(kind){
+ case KEYS: return function keys(){ return new Constructor(this, kind); };
+ case VALUES: return function values(){ return new Constructor(this, kind); };
+ } return function entries(){ return new Constructor(this, kind); };
+ };
+ var TAG = NAME + ' Iterator'
+ , DEF_VALUES = DEFAULT == VALUES
+ , VALUES_BUG = false
+ , proto = Base.prototype
+ , $native = proto[ITERATOR] || proto[FF_ITERATOR] || DEFAULT && proto[DEFAULT]
+ , $default = $native || getMethod(DEFAULT)
+ , methods, key;
+ // Fix native
+ if($native){
+ var IteratorPrototype = getProto($default.call(new Base));
+ // Set @@toStringTag to native iterators
+ setToStringTag(IteratorPrototype, TAG, true);
+ // FF fix
+ if(!LIBRARY && has(proto, FF_ITERATOR))hide(IteratorPrototype, ITERATOR, returnThis);
+ // fix Array#{values, @@iterator}.name in V8 / FF
+ if(DEF_VALUES && $native.name !== VALUES){
+ VALUES_BUG = true;
+ $default = function values(){ return $native.call(this); };
+ }
+ }
+ // Define iterator
+ if((!LIBRARY || FORCED) && (BUGGY || VALUES_BUG || !proto[ITERATOR])){
+ hide(proto, ITERATOR, $default);
+ }
+ // Plug for library
+ Iterators[NAME] = $default;
+ Iterators[TAG] = returnThis;
+ if(DEFAULT){
+ methods = {
+ values: DEF_VALUES ? $default : getMethod(VALUES),
+ keys: IS_SET ? $default : getMethod(KEYS),
+ entries: !DEF_VALUES ? $default : getMethod('entries')
+ };
+ if(FORCED)for(key in methods){
+ if(!(key in proto))redefine(proto, key, methods[key]);
+ } else $export($export.P + $export.F * (BUGGY || VALUES_BUG), NAME, methods);
+ }
+ return methods;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iter-detect.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iter-detect.js
new file mode 100644
index 00000000..bff5fffb
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iter-detect.js
@@ -0,0 +1,21 @@
+var ITERATOR = require('./$.wks')('iterator')
+ , SAFE_CLOSING = false;
+
+try {
+ var riter = [7][ITERATOR]();
+ riter['return'] = function(){ SAFE_CLOSING = true; };
+ Array.from(riter, function(){ throw 2; });
+} catch(e){ /* empty */ }
+
+module.exports = function(exec, skipClosing){
+ if(!skipClosing && !SAFE_CLOSING)return false;
+ var safe = false;
+ try {
+ var arr = [7]
+ , iter = arr[ITERATOR]();
+ iter.next = function(){ return {done: safe = true}; };
+ arr[ITERATOR] = function(){ return iter; };
+ exec(arr);
+ } catch(e){ /* empty */ }
+ return safe;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iter-step.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iter-step.js
new file mode 100644
index 00000000..6ff0dc51
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iter-step.js
@@ -0,0 +1,3 @@
+module.exports = function(done, value){
+ return {value: value, done: !!done};
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iterators.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iterators.js
new file mode 100644
index 00000000..a0995453
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.iterators.js
@@ -0,0 +1 @@
+module.exports = {};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.js
new file mode 100644
index 00000000..053bae48
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.js
@@ -0,0 +1,13 @@
+var $Object = Object;
+module.exports = {
+ create: $Object.create,
+ getProto: $Object.getPrototypeOf,
+ isEnum: {}.propertyIsEnumerable,
+ getDesc: $Object.getOwnPropertyDescriptor,
+ setDesc: $Object.defineProperty,
+ setDescs: $Object.defineProperties,
+ getKeys: $Object.keys,
+ getNames: $Object.getOwnPropertyNames,
+ getSymbols: $Object.getOwnPropertySymbols,
+ each: [].forEach
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.keyof.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.keyof.js
new file mode 100644
index 00000000..09d183a7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.keyof.js
@@ -0,0 +1,10 @@
+var $ = require('./$')
+ , toIObject = require('./$.to-iobject');
+module.exports = function(object, el){
+ var O = toIObject(object)
+ , keys = $.getKeys(O)
+ , length = keys.length
+ , index = 0
+ , key;
+ while(length > index)if(O[key = keys[index++]] === el)return key;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.library.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.library.js
new file mode 100644
index 00000000..82e47dd5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.library.js
@@ -0,0 +1 @@
+module.exports = false;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.math-expm1.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.math-expm1.js
new file mode 100644
index 00000000..9d91be93
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.math-expm1.js
@@ -0,0 +1,4 @@
+// 20.2.2.14 Math.expm1(x)
+module.exports = Math.expm1 || function expm1(x){
+ return (x = +x) == 0 ? x : x > -1e-6 && x < 1e-6 ? x + x * x / 2 : Math.exp(x) - 1;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.math-log1p.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.math-log1p.js
new file mode 100644
index 00000000..a92bf463
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.math-log1p.js
@@ -0,0 +1,4 @@
+// 20.2.2.20 Math.log1p(x)
+module.exports = Math.log1p || function log1p(x){
+ return (x = +x) > -1e-8 && x < 1e-8 ? x - x * x / 2 : Math.log(1 + x);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.math-sign.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.math-sign.js
new file mode 100644
index 00000000..a4848df6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.math-sign.js
@@ -0,0 +1,4 @@
+// 20.2.2.28 Math.sign(x)
+module.exports = Math.sign || function sign(x){
+ return (x = +x) == 0 || x != x ? x : x < 0 ? -1 : 1;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.microtask.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.microtask.js
new file mode 100644
index 00000000..1f9ebeb5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.microtask.js
@@ -0,0 +1,64 @@
+var global = require('./$.global')
+ , macrotask = require('./$.task').set
+ , Observer = global.MutationObserver || global.WebKitMutationObserver
+ , process = global.process
+ , Promise = global.Promise
+ , isNode = require('./$.cof')(process) == 'process'
+ , head, last, notify;
+
+var flush = function(){
+ var parent, domain, fn;
+ if(isNode && (parent = process.domain)){
+ process.domain = null;
+ parent.exit();
+ }
+ while(head){
+ domain = head.domain;
+ fn = head.fn;
+ if(domain)domain.enter();
+ fn(); // <- currently we use it only for Promise - try / catch not required
+ if(domain)domain.exit();
+ head = head.next;
+ } last = undefined;
+ if(parent)parent.enter();
+};
+
+// Node.js
+if(isNode){
+ notify = function(){
+ process.nextTick(flush);
+ };
+// browsers with MutationObserver
+} else if(Observer){
+ var toggle = 1
+ , node = document.createTextNode('');
+ new Observer(flush).observe(node, {characterData: true}); // eslint-disable-line no-new
+ notify = function(){
+ node.data = toggle = -toggle;
+ };
+// environments with maybe non-completely correct, but existent Promise
+} else if(Promise && Promise.resolve){
+ notify = function(){
+ Promise.resolve().then(flush);
+ };
+// for other environments - macrotask based on:
+// - setImmediate
+// - MessageChannel
+// - window.postMessag
+// - onreadystatechange
+// - setTimeout
+} else {
+ notify = function(){
+ // strange IE + webpack dev server bug - use .call(global)
+ macrotask.call(global, flush);
+ };
+}
+
+module.exports = function asap(fn){
+ var task = {fn: fn, next: undefined, domain: isNode && process.domain};
+ if(last)last.next = task;
+ if(!head){
+ head = task;
+ notify();
+ } last = task;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.object-assign.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.object-assign.js
new file mode 100644
index 00000000..5ce43f78
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.object-assign.js
@@ -0,0 +1,33 @@
+// 19.1.2.1 Object.assign(target, source, ...)
+var $ = require('./$')
+ , toObject = require('./$.to-object')
+ , IObject = require('./$.iobject');
+
+// should work with symbols and should have deterministic property order (V8 bug)
+module.exports = require('./$.fails')(function(){
+ var a = Object.assign
+ , A = {}
+ , B = {}
+ , S = Symbol()
+ , K = 'abcdefghijklmnopqrst';
+ A[S] = 7;
+ K.split('').forEach(function(k){ B[k] = k; });
+ return a({}, A)[S] != 7 || Object.keys(a({}, B)).join('') != K;
+}) ? function assign(target, source){ // eslint-disable-line no-unused-vars
+ var T = toObject(target)
+ , $$ = arguments
+ , $$len = $$.length
+ , index = 1
+ , getKeys = $.getKeys
+ , getSymbols = $.getSymbols
+ , isEnum = $.isEnum;
+ while($$len > index){
+ var S = IObject($$[index++])
+ , keys = getSymbols ? getKeys(S).concat(getSymbols(S)) : getKeys(S)
+ , length = keys.length
+ , j = 0
+ , key;
+ while(length > j)if(isEnum.call(S, key = keys[j++]))T[key] = S[key];
+ }
+ return T;
+} : Object.assign;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.object-define.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.object-define.js
new file mode 100644
index 00000000..2fff248f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.object-define.js
@@ -0,0 +1,11 @@
+var $ = require('./$')
+ , ownKeys = require('./$.own-keys')
+ , toIObject = require('./$.to-iobject');
+
+module.exports = function define(target, mixin){
+ var keys = ownKeys(toIObject(mixin))
+ , length = keys.length
+ , i = 0, key;
+ while(length > i)$.setDesc(target, key = keys[i++], $.getDesc(mixin, key));
+ return target;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.object-sap.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.object-sap.js
new file mode 100644
index 00000000..5fa7288a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.object-sap.js
@@ -0,0 +1,10 @@
+// most Object methods by ES6 should accept primitives
+var $export = require('./$.export')
+ , core = require('./$.core')
+ , fails = require('./$.fails');
+module.exports = function(KEY, exec){
+ var fn = (core.Object || {})[KEY] || Object[KEY]
+ , exp = {};
+ exp[KEY] = exec(fn);
+ $export($export.S + $export.F * fails(function(){ fn(1); }), 'Object', exp);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.object-to-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.object-to-array.js
new file mode 100644
index 00000000..d46425ba
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.object-to-array.js
@@ -0,0 +1,16 @@
+var $ = require('./$')
+ , toIObject = require('./$.to-iobject')
+ , isEnum = $.isEnum;
+module.exports = function(isEntries){
+ return function(it){
+ var O = toIObject(it)
+ , keys = $.getKeys(O)
+ , length = keys.length
+ , i = 0
+ , result = []
+ , key;
+ while(length > i)if(isEnum.call(O, key = keys[i++])){
+ result.push(isEntries ? [key, O[key]] : O[key]);
+ } return result;
+ };
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.own-keys.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.own-keys.js
new file mode 100644
index 00000000..0218c4bd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.own-keys.js
@@ -0,0 +1,9 @@
+// all object keys, includes non-enumerable and symbols
+var $ = require('./$')
+ , anObject = require('./$.an-object')
+ , Reflect = require('./$.global').Reflect;
+module.exports = Reflect && Reflect.ownKeys || function ownKeys(it){
+ var keys = $.getNames(anObject(it))
+ , getSymbols = $.getSymbols;
+ return getSymbols ? keys.concat(getSymbols(it)) : keys;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.partial.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.partial.js
new file mode 100644
index 00000000..53f97aa9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.partial.js
@@ -0,0 +1,24 @@
+'use strict';
+var path = require('./$.path')
+ , invoke = require('./$.invoke')
+ , aFunction = require('./$.a-function');
+module.exports = function(/* ...pargs */){
+ var fn = aFunction(this)
+ , length = arguments.length
+ , pargs = Array(length)
+ , i = 0
+ , _ = path._
+ , holder = false;
+ while(length > i)if((pargs[i] = arguments[i++]) === _)holder = true;
+ return function(/* ...args */){
+ var that = this
+ , $$ = arguments
+ , $$len = $$.length
+ , j = 0, k = 0, args;
+ if(!holder && !$$len)return invoke(fn, pargs, that);
+ args = pargs.slice();
+ if(holder)for(;length > j; j++)if(args[j] === _)args[j] = $$[k++];
+ while($$len > k)args.push($$[k++]);
+ return invoke(fn, args, that);
+ };
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.path.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.path.js
new file mode 100644
index 00000000..11ff15eb
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.path.js
@@ -0,0 +1 @@
+module.exports = require('./$.global');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.property-desc.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.property-desc.js
new file mode 100644
index 00000000..e3f7ab2d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.property-desc.js
@@ -0,0 +1,8 @@
+module.exports = function(bitmap, value){
+ return {
+ enumerable : !(bitmap & 1),
+ configurable: !(bitmap & 2),
+ writable : !(bitmap & 4),
+ value : value
+ };
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.redefine-all.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.redefine-all.js
new file mode 100644
index 00000000..01fe55ba
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.redefine-all.js
@@ -0,0 +1,5 @@
+var redefine = require('./$.redefine');
+module.exports = function(target, src){
+ for(var key in src)redefine(target, key, src[key]);
+ return target;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.redefine.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.redefine.js
new file mode 100644
index 00000000..1783e3a5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.redefine.js
@@ -0,0 +1,27 @@
+// add fake Function#toString
+// for correct work wrapped methods / constructors with methods like LoDash isNative
+var global = require('./$.global')
+ , hide = require('./$.hide')
+ , SRC = require('./$.uid')('src')
+ , TO_STRING = 'toString'
+ , $toString = Function[TO_STRING]
+ , TPL = ('' + $toString).split(TO_STRING);
+
+require('./$.core').inspectSource = function(it){
+ return $toString.call(it);
+};
+
+(module.exports = function(O, key, val, safe){
+ if(typeof val == 'function'){
+ val.hasOwnProperty(SRC) || hide(val, SRC, O[key] ? '' + O[key] : TPL.join(String(key)));
+ val.hasOwnProperty('name') || hide(val, 'name', key);
+ }
+ if(O === global){
+ O[key] = val;
+ } else {
+ if(!safe)delete O[key];
+ hide(O, key, val);
+ }
+})(Function.prototype, TO_STRING, function toString(){
+ return typeof this == 'function' && this[SRC] || $toString.call(this);
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.replacer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.replacer.js
new file mode 100644
index 00000000..5360a3d3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.replacer.js
@@ -0,0 +1,8 @@
+module.exports = function(regExp, replace){
+ var replacer = replace === Object(replace) ? function(part){
+ return replace[part];
+ } : replace;
+ return function(it){
+ return String(it).replace(regExp, replacer);
+ };
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.same-value.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.same-value.js
new file mode 100644
index 00000000..8c2b8c7f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.same-value.js
@@ -0,0 +1,4 @@
+// 7.2.9 SameValue(x, y)
+module.exports = Object.is || function is(x, y){
+ return x === y ? x !== 0 || 1 / x === 1 / y : x != x && y != y;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.set-proto.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.set-proto.js
new file mode 100644
index 00000000..b1edd681
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.set-proto.js
@@ -0,0 +1,26 @@
+// Works with __proto__ only. Old v8 can't work with null proto objects.
+/* eslint-disable no-proto */
+var getDesc = require('./$').getDesc
+ , isObject = require('./$.is-object')
+ , anObject = require('./$.an-object');
+var check = function(O, proto){
+ anObject(O);
+ if(!isObject(proto) && proto !== null)throw TypeError(proto + ": can't set as prototype!");
+};
+module.exports = {
+ set: Object.setPrototypeOf || ('__proto__' in {} ? // eslint-disable-line
+ function(test, buggy, set){
+ try {
+ set = require('./$.ctx')(Function.call, getDesc(Object.prototype, '__proto__').set, 2);
+ set(test, []);
+ buggy = !(test instanceof Array);
+ } catch(e){ buggy = true; }
+ return function setPrototypeOf(O, proto){
+ check(O, proto);
+ if(buggy)O.__proto__ = proto;
+ else set(O, proto);
+ return O;
+ };
+ }({}, false) : undefined),
+ check: check
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.set-species.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.set-species.js
new file mode 100644
index 00000000..b1d04c6b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.set-species.js
@@ -0,0 +1,13 @@
+'use strict';
+var global = require('./$.global')
+ , $ = require('./$')
+ , DESCRIPTORS = require('./$.descriptors')
+ , SPECIES = require('./$.wks')('species');
+
+module.exports = function(KEY){
+ var C = global[KEY];
+ if(DESCRIPTORS && C && !C[SPECIES])$.setDesc(C, SPECIES, {
+ configurable: true,
+ get: function(){ return this; }
+ });
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.set-to-string-tag.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.set-to-string-tag.js
new file mode 100644
index 00000000..22b34244
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.set-to-string-tag.js
@@ -0,0 +1,7 @@
+var def = require('./$').setDesc
+ , has = require('./$.has')
+ , TAG = require('./$.wks')('toStringTag');
+
+module.exports = function(it, tag, stat){
+ if(it && !has(it = stat ? it : it.prototype, TAG))def(it, TAG, {configurable: true, value: tag});
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.shared.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.shared.js
new file mode 100644
index 00000000..8dea827a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.shared.js
@@ -0,0 +1,6 @@
+var global = require('./$.global')
+ , SHARED = '__core-js_shared__'
+ , store = global[SHARED] || (global[SHARED] = {});
+module.exports = function(key){
+ return store[key] || (store[key] = {});
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.species-constructor.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.species-constructor.js
new file mode 100644
index 00000000..f71168b7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.species-constructor.js
@@ -0,0 +1,8 @@
+// 7.3.20 SpeciesConstructor(O, defaultConstructor)
+var anObject = require('./$.an-object')
+ , aFunction = require('./$.a-function')
+ , SPECIES = require('./$.wks')('species');
+module.exports = function(O, D){
+ var C = anObject(O).constructor, S;
+ return C === undefined || (S = anObject(C)[SPECIES]) == undefined ? D : aFunction(S);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.strict-new.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.strict-new.js
new file mode 100644
index 00000000..8bab9ed1
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.strict-new.js
@@ -0,0 +1,4 @@
+module.exports = function(it, Constructor, name){
+ if(!(it instanceof Constructor))throw TypeError(name + ": use the 'new' operator!");
+ return it;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.string-at.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.string-at.js
new file mode 100644
index 00000000..3d344bba
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.string-at.js
@@ -0,0 +1,17 @@
+var toInteger = require('./$.to-integer')
+ , defined = require('./$.defined');
+// true -> String#at
+// false -> String#codePointAt
+module.exports = function(TO_STRING){
+ return function(that, pos){
+ var s = String(defined(that))
+ , i = toInteger(pos)
+ , l = s.length
+ , a, b;
+ if(i < 0 || i >= l)return TO_STRING ? '' : undefined;
+ a = s.charCodeAt(i);
+ return a < 0xd800 || a > 0xdbff || i + 1 === l || (b = s.charCodeAt(i + 1)) < 0xdc00 || b > 0xdfff
+ ? TO_STRING ? s.charAt(i) : a
+ : TO_STRING ? s.slice(i, i + 2) : (a - 0xd800 << 10) + (b - 0xdc00) + 0x10000;
+ };
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.string-context.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.string-context.js
new file mode 100644
index 00000000..d6485a43
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.string-context.js
@@ -0,0 +1,8 @@
+// helper for String#{startsWith, endsWith, includes}
+var isRegExp = require('./$.is-regexp')
+ , defined = require('./$.defined');
+
+module.exports = function(that, searchString, NAME){
+ if(isRegExp(searchString))throw TypeError('String#' + NAME + " doesn't accept regex!");
+ return String(defined(that));
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.string-pad.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.string-pad.js
new file mode 100644
index 00000000..f0507d93
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.string-pad.js
@@ -0,0 +1,17 @@
+// https://github.com/ljharb/proposal-string-pad-left-right
+var toLength = require('./$.to-length')
+ , repeat = require('./$.string-repeat')
+ , defined = require('./$.defined');
+
+module.exports = function(that, maxLength, fillString, left){
+ var S = String(defined(that))
+ , stringLength = S.length
+ , fillStr = fillString === undefined ? ' ' : String(fillString)
+ , intMaxLength = toLength(maxLength);
+ if(intMaxLength <= stringLength)return S;
+ if(fillStr == '')fillStr = ' ';
+ var fillLen = intMaxLength - stringLength
+ , stringFiller = repeat.call(fillStr, Math.ceil(fillLen / fillStr.length));
+ if(stringFiller.length > fillLen)stringFiller = stringFiller.slice(0, fillLen);
+ return left ? stringFiller + S : S + stringFiller;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.string-repeat.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.string-repeat.js
new file mode 100644
index 00000000..491d0853
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.string-repeat.js
@@ -0,0 +1,12 @@
+'use strict';
+var toInteger = require('./$.to-integer')
+ , defined = require('./$.defined');
+
+module.exports = function repeat(count){
+ var str = String(defined(this))
+ , res = ''
+ , n = toInteger(count);
+ if(n < 0 || n == Infinity)throw RangeError("Count can't be negative");
+ for(;n > 0; (n >>>= 1) && (str += str))if(n & 1)res += str;
+ return res;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.string-trim.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.string-trim.js
new file mode 100644
index 00000000..04423f4d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.string-trim.js
@@ -0,0 +1,29 @@
+var $export = require('./$.export')
+ , defined = require('./$.defined')
+ , fails = require('./$.fails')
+ , spaces = '\x09\x0A\x0B\x0C\x0D\x20\xA0\u1680\u180E\u2000\u2001\u2002\u2003' +
+ '\u2004\u2005\u2006\u2007\u2008\u2009\u200A\u202F\u205F\u3000\u2028\u2029\uFEFF'
+ , space = '[' + spaces + ']'
+ , non = '\u200b\u0085'
+ , ltrim = RegExp('^' + space + space + '*')
+ , rtrim = RegExp(space + space + '*$');
+
+var exporter = function(KEY, exec){
+ var exp = {};
+ exp[KEY] = exec(trim);
+ $export($export.P + $export.F * fails(function(){
+ return !!spaces[KEY]() || non[KEY]() != non;
+ }), 'String', exp);
+};
+
+// 1 -> String#trimLeft
+// 2 -> String#trimRight
+// 3 -> String#trim
+var trim = exporter.trim = function(string, TYPE){
+ string = String(defined(string));
+ if(TYPE & 1)string = string.replace(ltrim, '');
+ if(TYPE & 2)string = string.replace(rtrim, '');
+ return string;
+};
+
+module.exports = exporter;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.task.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.task.js
new file mode 100644
index 00000000..5d7759e6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.task.js
@@ -0,0 +1,75 @@
+var ctx = require('./$.ctx')
+ , invoke = require('./$.invoke')
+ , html = require('./$.html')
+ , cel = require('./$.dom-create')
+ , global = require('./$.global')
+ , process = global.process
+ , setTask = global.setImmediate
+ , clearTask = global.clearImmediate
+ , MessageChannel = global.MessageChannel
+ , counter = 0
+ , queue = {}
+ , ONREADYSTATECHANGE = 'onreadystatechange'
+ , defer, channel, port;
+var run = function(){
+ var id = +this;
+ if(queue.hasOwnProperty(id)){
+ var fn = queue[id];
+ delete queue[id];
+ fn();
+ }
+};
+var listner = function(event){
+ run.call(event.data);
+};
+// Node.js 0.9+ & IE10+ has setImmediate, otherwise:
+if(!setTask || !clearTask){
+ setTask = function setImmediate(fn){
+ var args = [], i = 1;
+ while(arguments.length > i)args.push(arguments[i++]);
+ queue[++counter] = function(){
+ invoke(typeof fn == 'function' ? fn : Function(fn), args);
+ };
+ defer(counter);
+ return counter;
+ };
+ clearTask = function clearImmediate(id){
+ delete queue[id];
+ };
+ // Node.js 0.8-
+ if(require('./$.cof')(process) == 'process'){
+ defer = function(id){
+ process.nextTick(ctx(run, id, 1));
+ };
+ // Browsers with MessageChannel, includes WebWorkers
+ } else if(MessageChannel){
+ channel = new MessageChannel;
+ port = channel.port2;
+ channel.port1.onmessage = listner;
+ defer = ctx(port.postMessage, port, 1);
+ // Browsers with postMessage, skip WebWorkers
+ // IE8 has postMessage, but it's sync & typeof its postMessage is 'object'
+ } else if(global.addEventListener && typeof postMessage == 'function' && !global.importScripts){
+ defer = function(id){
+ global.postMessage(id + '', '*');
+ };
+ global.addEventListener('message', listner, false);
+ // IE8-
+ } else if(ONREADYSTATECHANGE in cel('script')){
+ defer = function(id){
+ html.appendChild(cel('script'))[ONREADYSTATECHANGE] = function(){
+ html.removeChild(this);
+ run.call(id);
+ };
+ };
+ // Rest old browsers
+ } else {
+ defer = function(id){
+ setTimeout(ctx(run, id, 1), 0);
+ };
+ }
+}
+module.exports = {
+ set: setTask,
+ clear: clearTask
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.to-index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.to-index.js
new file mode 100644
index 00000000..9346a8ff
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.to-index.js
@@ -0,0 +1,7 @@
+var toInteger = require('./$.to-integer')
+ , max = Math.max
+ , min = Math.min;
+module.exports = function(index, length){
+ index = toInteger(index);
+ return index < 0 ? max(index + length, 0) : min(index, length);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.to-integer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.to-integer.js
new file mode 100644
index 00000000..f63baaff
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.to-integer.js
@@ -0,0 +1,6 @@
+// 7.1.4 ToInteger
+var ceil = Math.ceil
+ , floor = Math.floor;
+module.exports = function(it){
+ return isNaN(it = +it) ? 0 : (it > 0 ? floor : ceil)(it);
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.to-iobject.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.to-iobject.js
new file mode 100644
index 00000000..fcf54c82
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.to-iobject.js
@@ -0,0 +1,6 @@
+// to indexed object, toObject with fallback for non-array-like ES3 strings
+var IObject = require('./$.iobject')
+ , defined = require('./$.defined');
+module.exports = function(it){
+ return IObject(defined(it));
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.to-length.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.to-length.js
new file mode 100644
index 00000000..0e15b1b8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.to-length.js
@@ -0,0 +1,6 @@
+// 7.1.15 ToLength
+var toInteger = require('./$.to-integer')
+ , min = Math.min;
+module.exports = function(it){
+ return it > 0 ? min(toInteger(it), 0x1fffffffffffff) : 0; // pow(2, 53) - 1 == 9007199254740991
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.to-object.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.to-object.js
new file mode 100644
index 00000000..2c57a29f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.to-object.js
@@ -0,0 +1,5 @@
+// 7.1.13 ToObject(argument)
+var defined = require('./$.defined');
+module.exports = function(it){
+ return Object(defined(it));
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.to-primitive.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.to-primitive.js
new file mode 100644
index 00000000..6fb4585a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.to-primitive.js
@@ -0,0 +1,12 @@
+// 7.1.1 ToPrimitive(input [, PreferredType])
+var isObject = require('./$.is-object');
+// instead of the ES6 spec version, we didn't implement @@toPrimitive case
+// and the second argument - flag - preferred type is a string
+module.exports = function(it, S){
+ if(!isObject(it))return it;
+ var fn, val;
+ if(S && typeof (fn = it.toString) == 'function' && !isObject(val = fn.call(it)))return val;
+ if(typeof (fn = it.valueOf) == 'function' && !isObject(val = fn.call(it)))return val;
+ if(!S && typeof (fn = it.toString) == 'function' && !isObject(val = fn.call(it)))return val;
+ throw TypeError("Can't convert object to primitive value");
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.typed-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.typed-array.js
new file mode 100644
index 00000000..1bd5cf0e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.typed-array.js
@@ -0,0 +1,365 @@
+'use strict';
+if(require('./$.descriptors')){
+ var LIBRARY = require('./$.library')
+ , global = require('./$.global')
+ , $ = require('./$')
+ , fails = require('./$.fails')
+ , $export = require('./$.export')
+ , $typed = require('./$.typed')
+ , $buffer = require('./$.buffer')
+ , ctx = require('./$.ctx')
+ , strictNew = require('./$.strict-new')
+ , propertyDesc = require('./$.property-desc')
+ , hide = require('./$.hide')
+ , redefineAll = require('./$.redefine-all')
+ , isInteger = require('./$.is-integer')
+ , toInteger = require('./$.to-integer')
+ , toLength = require('./$.to-length')
+ , toIndex = require('./$.to-index')
+ , toPrimitive = require('./$.to-primitive')
+ , isObject = require('./$.is-object')
+ , toObject = require('./$.to-object')
+ , isArrayIter = require('./$.is-array-iter')
+ , isIterable = require('./core.is-iterable')
+ , getIterFn = require('./core.get-iterator-method')
+ , wks = require('./$.wks')
+ , createArrayMethod = require('./$.array-methods')
+ , createArrayIncludes = require('./$.array-includes')
+ , speciesConstructor = require('./$.species-constructor')
+ , ArrayIterators = require('./es6.array.iterator')
+ , Iterators = require('./$.iterators')
+ , $iterDetect = require('./$.iter-detect')
+ , setSpecies = require('./$.set-species')
+ , arrayFill = require('./$.array-fill')
+ , arrayCopyWithin = require('./$.array-copy-within')
+ , ArrayProto = Array.prototype
+ , $ArrayBuffer = $buffer.ArrayBuffer
+ , $DataView = $buffer.DataView
+ , setDesc = $.setDesc
+ , getDesc = $.getDesc
+ , arrayForEach = createArrayMethod(0)
+ , arrayMap = createArrayMethod(1)
+ , arrayFilter = createArrayMethod(2)
+ , arraySome = createArrayMethod(3)
+ , arrayEvery = createArrayMethod(4)
+ , arrayFind = createArrayMethod(5)
+ , arrayFindIndex = createArrayMethod(6)
+ , arrayIncludes = createArrayIncludes(true)
+ , arrayIndexOf = createArrayIncludes(false)
+ , arrayValues = ArrayIterators.values
+ , arrayKeys = ArrayIterators.keys
+ , arrayEntries = ArrayIterators.entries
+ , arrayLastIndexOf = ArrayProto.lastIndexOf
+ , arrayReduce = ArrayProto.reduce
+ , arrayReduceRight = ArrayProto.reduceRight
+ , arrayJoin = ArrayProto.join
+ , arrayReverse = ArrayProto.reverse
+ , arraySort = ArrayProto.sort
+ , arraySlice = ArrayProto.slice
+ , arrayToString = ArrayProto.toString
+ , arrayToLocaleString = ArrayProto.toLocaleString
+ , ITERATOR = wks('iterator')
+ , TAG = wks('toStringTag')
+ , TYPED_CONSTRUCTOR = wks('typed_constructor')
+ , DEF_CONSTRUCTOR = wks('def_constructor')
+ , ALL_ARRAYS = $typed.ARRAYS
+ , TYPED_ARRAY = $typed.TYPED
+ , VIEW = $typed.VIEW
+ , BYTES_PER_ELEMENT = 'BYTES_PER_ELEMENT';
+
+ var LITTLE_ENDIAN = fails(function(){
+ return new Uint8Array(new Uint16Array([1]).buffer)[0] === 1;
+ });
+
+ var validate = function(it){
+ if(isObject(it) && TYPED_ARRAY in it)return it;
+ throw TypeError(it + ' is not a typed array!');
+ };
+
+ var fromList = function(O, list){
+ var index = 0
+ , length = list.length
+ , result = allocate(speciesConstructor(O, O[DEF_CONSTRUCTOR]), length);
+ while(length > index)result[index] = list[index++];
+ return result;
+ };
+
+ var allocate = function(C, length){
+ if(!(isObject(C) && TYPED_CONSTRUCTOR in C)){
+ throw TypeError('It is not a typed array constructor!');
+ } return new C(length);
+ };
+
+ var $from = function from(source /*, mapfn, thisArg */){
+ var O = toObject(source)
+ , $$ = arguments
+ , $$len = $$.length
+ , mapfn = $$len > 1 ? $$[1] : undefined
+ , mapping = mapfn !== undefined
+ , iterFn = getIterFn(O)
+ , i, length, values, result, step, iterator;
+ if(iterFn != undefined && !isArrayIter(iterFn)){
+ for(iterator = iterFn.call(O), values = [], i = 0; !(step = iterator.next()).done; i++){
+ values.push(step.value);
+ } O = values;
+ }
+ if(mapping && $$len > 2)mapfn = ctx(mapfn, $$[2], 2);
+ for(i = 0, length = toLength(O.length), result = allocate(this, length); length > i; i++){
+ result[i] = mapping ? mapfn(O[i], i) : O[i];
+ }
+ return result;
+ };
+
+ var addGetter = function(C, key, internal){
+ setDesc(C.prototype, key, {get: function(){ return this._d[internal]; }});
+ };
+
+ var $of = function of(/*...items*/){
+ var index = 0
+ , length = arguments.length
+ , result = allocate(this, length);
+ while(length > index)result[index] = arguments[index++];
+ return result;
+ };
+ var $toLocaleString = function toLocaleString(){
+ return arrayToLocaleString.apply(validate(this), arguments);
+ };
+
+ var proto = {
+ copyWithin: function copyWithin(target, start /*, end */){
+ return arrayCopyWithin.call(validate(this), target, start, arguments.length > 2 ? arguments[2] : undefined);
+ },
+ every: function every(callbackfn /*, thisArg */){
+ return arrayEvery(validate(this), callbackfn, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ fill: function fill(value /*, start, end */){ // eslint-disable-line no-unused-vars
+ return arrayFill.apply(validate(this), arguments);
+ },
+ filter: function filter(callbackfn /*, thisArg */){
+ return fromList(this, arrayFilter(validate(this), callbackfn, arguments.length > 1 ? arguments[1] : undefined));
+ },
+ find: function find(predicate /*, thisArg */){
+ return arrayFind(validate(this), predicate, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ findIndex: function findIndex(predicate /*, thisArg */){
+ return arrayFindIndex(validate(this), predicate, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ forEach: function forEach(callbackfn /*, thisArg */){
+ arrayForEach(validate(this), callbackfn, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ indexOf: function indexOf(searchElement /*, fromIndex */){
+ return arrayIndexOf(validate(this), searchElement, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ includes: function includes(searchElement /*, fromIndex */){
+ return arrayIncludes(validate(this), searchElement, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ join: function join(separator){ // eslint-disable-line no-unused-vars
+ return arrayJoin.apply(validate(this), arguments);
+ },
+ lastIndexOf: function lastIndexOf(searchElement /*, fromIndex */){ // eslint-disable-line no-unused-vars
+ return arrayLastIndexOf.apply(validate(this), arguments);
+ },
+ map: function map(mapfn /*, thisArg */){
+ return fromList(this, arrayMap(validate(this), mapfn, arguments.length > 1 ? arguments[1] : undefined)); // TODO
+ },
+ reduce: function reduce(callbackfn /*, initialValue */){ // eslint-disable-line no-unused-vars
+ return arrayReduce.apply(validate(this), arguments);
+ },
+ reduceRight: function reduceRight(callbackfn /*, initialValue */){ // eslint-disable-line no-unused-vars
+ return arrayReduceRight.apply(validate(this), arguments);
+ },
+ reverse: function reverse(){
+ return arrayReverse.call(validate(this));
+ },
+ set: function set(arrayLike /*, offset */){
+ validate(this);
+ var offset = toInteger(arguments.length > 1 ? arguments[1] : undefined);
+ if(offset < 0)throw RangeError();
+ var length = this.length;
+ var src = toObject(arrayLike);
+ var index = 0;
+ var len = toLength(src.length);
+ if(len + offset > length)throw RangeError();
+ while(index < len)this[offset + index] = src[index++];
+ },
+ slice: function slice(start, end){
+ return fromList(this, arraySlice.call(validate(this), start, end)); // TODO
+ },
+ some: function some(callbackfn /*, thisArg */){
+ return arraySome(validate(this), callbackfn, arguments.length > 1 ? arguments[1] : undefined);
+ },
+ sort: function sort(comparefn){
+ return arraySort.call(validate(this), comparefn);
+ },
+ subarray: function subarray(begin, end){
+ var O = validate(this)
+ , length = O.length
+ , $begin = toIndex(begin, length);
+ return new (speciesConstructor(O, O[DEF_CONSTRUCTOR]))(
+ O.buffer,
+ O.byteOffset + $begin * O.BYTES_PER_ELEMENT,
+ toLength((end === undefined ? length : toIndex(end, length)) - $begin)
+ );
+ },
+ entries: function entries(){
+ return arrayEntries.call(validate(this));
+ },
+ keys: function keys(){
+ return arrayKeys.call(validate(this));
+ },
+ values: function values(){
+ return arrayValues.call(validate(this));
+ }
+ };
+
+ var isTAIndex = function(target, key){
+ return isObject(target)
+ && TYPED_ARRAY in target
+ && typeof key != 'symbol'
+ && key in target
+ && String(+key) == String(key);
+ };
+ var $getDesc = function getOwnPropertyDescriptor(target, key){
+ return isTAIndex(target, key = toPrimitive(key, true))
+ ? propertyDesc(2, target[key])
+ : getDesc(target, key);
+ };
+ var $setDesc = function defineProperty(target, key, desc){
+ if(isTAIndex(target, key = toPrimitive(key, true)) && isObject(desc)){
+ if('value' in desc)target[key] = desc.value;
+ return target;
+ } else return setDesc(target, key, desc);
+ };
+
+ if(!ALL_ARRAYS){
+ $.getDesc = $getDesc;
+ $.setDesc = $setDesc;
+ }
+
+ $export($export.S + $export.F * !ALL_ARRAYS, 'Object', {
+ getOwnPropertyDescriptor: $getDesc,
+ defineProperty: $setDesc
+ });
+
+ var $TypedArrayPrototype$ = redefineAll({}, proto);
+ redefineAll($TypedArrayPrototype$, {
+ constructor: function(){ /* noop */ },
+ toString: arrayToString,
+ toLocaleString: $toLocaleString
+ });
+ $.setDesc($TypedArrayPrototype$, TAG, {
+ get: function(){ return this[TYPED_ARRAY]; }
+ });
+
+ module.exports = function(KEY, BYTES, wrapper, CLAMPED){
+ CLAMPED = !!CLAMPED;
+ var NAME = KEY + (CLAMPED ? 'Clamped' : '') + 'Array'
+ , GETTER = 'get' + KEY
+ , SETTER = 'set' + KEY
+ , TypedArray = global[NAME]
+ , Base = TypedArray || {}
+ , FORCED = !TypedArray || !$typed.ABV
+ , $iterator = proto.values
+ , O = {};
+ var addElement = function(that, index){
+ setDesc(that, index, {
+ get: function(){
+ var data = this._d;
+ return data.v[GETTER](index * BYTES + data.o, LITTLE_ENDIAN);
+ },
+ set: function(it){
+ var data = this._d;
+ if(CLAMPED)it = (it = Math.round(it)) < 0 ? 0 : it > 0xff ? 0xff : it & 0xff;
+ data.v[SETTER](index * BYTES + data.o, it, LITTLE_ENDIAN);
+ },
+ enumerable: true
+ });
+ };
+ if(!$ArrayBuffer)return;
+ if(FORCED){
+ TypedArray = wrapper(function(that, data, $offset, $length){
+ strictNew(that, TypedArray, NAME);
+ var index = 0
+ , offset = 0
+ , buffer, byteLength, length;
+ if(!isObject(data)){
+ byteLength = toInteger(data) * BYTES;
+ buffer = new $ArrayBuffer(byteLength);
+ // TODO TA case
+ } else if(data instanceof $ArrayBuffer){
+ buffer = data;
+ offset = toInteger($offset);
+ if(offset < 0 || offset % BYTES)throw RangeError();
+ var $len = data.byteLength;
+ if($length === undefined){
+ if($len % BYTES)throw RangeError();
+ byteLength = $len - offset;
+ if(byteLength < 0)throw RangeError();
+ } else {
+ byteLength = toLength($length) * BYTES;
+ if(byteLength + offset > $len)throw RangeError();
+ }
+ } else return $from.call(TypedArray, data);
+ length = byteLength / BYTES;
+ hide(that, '_d', {
+ b: buffer,
+ o: offset,
+ l: byteLength,
+ e: length,
+ v: new $DataView(buffer)
+ });
+ while(index < length)addElement(that, index++);
+ });
+ TypedArray.prototype = $.create($TypedArrayPrototype$);
+ addGetter(TypedArray, 'buffer', 'b');
+ addGetter(TypedArray, 'byteOffset', 'o');
+ addGetter(TypedArray, 'byteLength', 'l');
+ addGetter(TypedArray, 'length', 'e');
+ hide(TypedArray, BYTES_PER_ELEMENT, BYTES);
+ hide(TypedArray.prototype, BYTES_PER_ELEMENT, BYTES);
+ hide(TypedArray.prototype, 'constructor', TypedArray);
+ } else if(!$iterDetect(function(iter){
+ new TypedArray(iter); // eslint-disable-line no-new
+ }, true)){
+ TypedArray = wrapper(function(that, data, $offset, $length){
+ strictNew(that, TypedArray, NAME);
+ if(isObject(data) && isIterable(data))return $from.call(TypedArray, data);
+ return $length === undefined ? new Base(data, $offset) : new Base(data, $offset, $length);
+ });
+ TypedArray.prototype = Base.prototype;
+ if(!LIBRARY)TypedArray.prototype.constructor = TypedArray;
+ }
+ var TypedArrayPrototype = TypedArray.prototype;
+ var $nativeIterator = TypedArrayPrototype[ITERATOR];
+ hide(TypedArray, TYPED_CONSTRUCTOR, true);
+ hide(TypedArrayPrototype, TYPED_ARRAY, NAME);
+ hide(TypedArrayPrototype, VIEW, true);
+ hide(TypedArrayPrototype, DEF_CONSTRUCTOR, TypedArray);
+ TAG in TypedArrayPrototype || $.setDesc(TypedArrayPrototype, TAG, {
+ get: function(){ return NAME; }
+ });
+
+ O[NAME] = TypedArray;
+
+ $export($export.G + $export.W + $export.F * (TypedArray != Base), O);
+
+ $export($export.S + $export.F * (TypedArray != Base), NAME, {
+ BYTES_PER_ELEMENT: BYTES,
+ from: Base.from || $from,
+ of: Base.of || $of
+ });
+
+ $export($export.P + $export.F * FORCED, NAME, proto);
+
+ $export($export.P + $export.F * (TypedArrayPrototype.toString != arrayToString), NAME, {toString: arrayToString});
+
+ $export($export.P + $export.F * fails(function(){
+ return [1, 2].toLocaleString() != new Typed([1, 2]).toLocaleString()
+ }), NAME, {toLocaleString: $toLocaleString});
+
+ Iterators[NAME] = $nativeIterator || $iterator;
+ LIBRARY || $nativeIterator || hide(TypedArrayPrototype, ITERATOR, $iterator);
+
+ setSpecies(NAME);
+ };
+} else module.exports = function(){ /* empty */};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.typed.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.typed.js
new file mode 100644
index 00000000..ced24126
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.typed.js
@@ -0,0 +1,36 @@
+var global = require('./$.global')
+ , hide = require('./$.hide')
+ , uid = require('./$.uid')
+ , TYPED = uid('typed_array')
+ , VIEW = uid('view')
+ , ABV = !!(global.ArrayBuffer && global.DataView)
+ , ARRAYS = true
+ , i = 0, l = 9;
+
+var TypedArrayConstructors = [
+ 'Int8Array',
+ 'Uint8Array',
+ 'Uint8ClampedArray',
+ 'Int16Array',
+ 'Uint16Array',
+ 'Int32Array',
+ 'Uint32Array',
+ 'Float32Array',
+ 'Float64Array'
+];
+
+while(i < l){
+ var Typed = global[TypedArrayConstructors[i++]];
+ if(Typed){
+ hide(Typed.prototype, TYPED, true);
+ hide(Typed.prototype, VIEW, true);
+ } else ARRAYS = false;
+}
+
+module.exports = {
+ ARRAYS: ARRAYS,
+ ABV: ABV,
+ CONSTR: ARRAYS && ABV,
+ TYPED: TYPED,
+ VIEW: VIEW
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.uid.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.uid.js
new file mode 100644
index 00000000..3be4196b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.uid.js
@@ -0,0 +1,5 @@
+var id = 0
+ , px = Math.random();
+module.exports = function(key){
+ return 'Symbol('.concat(key === undefined ? '' : key, ')_', (++id + px).toString(36));
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.wks.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.wks.js
new file mode 100644
index 00000000..87a3d29a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/$.wks.js
@@ -0,0 +1,7 @@
+var store = require('./$.shared')('wks')
+ , uid = require('./$.uid')
+ , Symbol = require('./$.global').Symbol;
+module.exports = function(name){
+ return store[name] || (store[name] =
+ Symbol && Symbol[name] || (Symbol || uid)('Symbol.' + name));
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.delay.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.delay.js
new file mode 100644
index 00000000..3e19ef39
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.delay.js
@@ -0,0 +1,12 @@
+var global = require('./$.global')
+ , core = require('./$.core')
+ , $export = require('./$.export')
+ , partial = require('./$.partial');
+// https://esdiscuss.org/topic/promise-returning-delay-function
+$export($export.G + $export.F, {
+ delay: function delay(time){
+ return new (core.Promise || global.Promise)(function(resolve){
+ setTimeout(partial.call(resolve, true), time);
+ });
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.dict.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.dict.js
new file mode 100644
index 00000000..df314988
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.dict.js
@@ -0,0 +1,153 @@
+'use strict';
+var $ = require('./$')
+ , ctx = require('./$.ctx')
+ , $export = require('./$.export')
+ , createDesc = require('./$.property-desc')
+ , assign = require('./$.object-assign')
+ , keyOf = require('./$.keyof')
+ , aFunction = require('./$.a-function')
+ , forOf = require('./$.for-of')
+ , isIterable = require('./core.is-iterable')
+ , $iterCreate = require('./$.iter-create')
+ , step = require('./$.iter-step')
+ , isObject = require('./$.is-object')
+ , toIObject = require('./$.to-iobject')
+ , DESCRIPTORS = require('./$.descriptors')
+ , has = require('./$.has')
+ , getKeys = $.getKeys;
+
+// 0 -> Dict.forEach
+// 1 -> Dict.map
+// 2 -> Dict.filter
+// 3 -> Dict.some
+// 4 -> Dict.every
+// 5 -> Dict.find
+// 6 -> Dict.findKey
+// 7 -> Dict.mapPairs
+var createDictMethod = function(TYPE){
+ var IS_MAP = TYPE == 1
+ , IS_EVERY = TYPE == 4;
+ return function(object, callbackfn, that /* = undefined */){
+ var f = ctx(callbackfn, that, 3)
+ , O = toIObject(object)
+ , result = IS_MAP || TYPE == 7 || TYPE == 2
+ ? new (typeof this == 'function' ? this : Dict) : undefined
+ , key, val, res;
+ for(key in O)if(has(O, key)){
+ val = O[key];
+ res = f(val, key, object);
+ if(TYPE){
+ if(IS_MAP)result[key] = res; // map
+ else if(res)switch(TYPE){
+ case 2: result[key] = val; break; // filter
+ case 3: return true; // some
+ case 5: return val; // find
+ case 6: return key; // findKey
+ case 7: result[res[0]] = res[1]; // mapPairs
+ } else if(IS_EVERY)return false; // every
+ }
+ }
+ return TYPE == 3 || IS_EVERY ? IS_EVERY : result;
+ };
+};
+var findKey = createDictMethod(6);
+
+var createDictIter = function(kind){
+ return function(it){
+ return new DictIterator(it, kind);
+ };
+};
+var DictIterator = function(iterated, kind){
+ this._t = toIObject(iterated); // target
+ this._a = getKeys(iterated); // keys
+ this._i = 0; // next index
+ this._k = kind; // kind
+};
+$iterCreate(DictIterator, 'Dict', function(){
+ var that = this
+ , O = that._t
+ , keys = that._a
+ , kind = that._k
+ , key;
+ do {
+ if(that._i >= keys.length){
+ that._t = undefined;
+ return step(1);
+ }
+ } while(!has(O, key = keys[that._i++]));
+ if(kind == 'keys' )return step(0, key);
+ if(kind == 'values')return step(0, O[key]);
+ return step(0, [key, O[key]]);
+});
+
+function Dict(iterable){
+ var dict = $.create(null);
+ if(iterable != undefined){
+ if(isIterable(iterable)){
+ forOf(iterable, true, function(key, value){
+ dict[key] = value;
+ });
+ } else assign(dict, iterable);
+ }
+ return dict;
+}
+Dict.prototype = null;
+
+function reduce(object, mapfn, init){
+ aFunction(mapfn);
+ var O = toIObject(object)
+ , keys = getKeys(O)
+ , length = keys.length
+ , i = 0
+ , memo, key;
+ if(arguments.length < 3){
+ if(!length)throw TypeError('Reduce of empty object with no initial value');
+ memo = O[keys[i++]];
+ } else memo = Object(init);
+ while(length > i)if(has(O, key = keys[i++])){
+ memo = mapfn(memo, O[key], key, object);
+ }
+ return memo;
+}
+
+function includes(object, el){
+ return (el == el ? keyOf(object, el) : findKey(object, function(it){
+ return it != it;
+ })) !== undefined;
+}
+
+function get(object, key){
+ if(has(object, key))return object[key];
+}
+function set(object, key, value){
+ if(DESCRIPTORS && key in Object)$.setDesc(object, key, createDesc(0, value));
+ else object[key] = value;
+ return object;
+}
+
+function isDict(it){
+ return isObject(it) && $.getProto(it) === Dict.prototype;
+}
+
+$export($export.G + $export.F, {Dict: Dict});
+
+$export($export.S, 'Dict', {
+ keys: createDictIter('keys'),
+ values: createDictIter('values'),
+ entries: createDictIter('entries'),
+ forEach: createDictMethod(0),
+ map: createDictMethod(1),
+ filter: createDictMethod(2),
+ some: createDictMethod(3),
+ every: createDictMethod(4),
+ find: createDictMethod(5),
+ findKey: findKey,
+ mapPairs: createDictMethod(7),
+ reduce: reduce,
+ keyOf: keyOf,
+ includes: includes,
+ has: has,
+ get: get,
+ set: set,
+ isDict: isDict
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.function.part.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.function.part.js
new file mode 100644
index 00000000..9943b307
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.function.part.js
@@ -0,0 +1,7 @@
+var path = require('./$.path')
+ , $export = require('./$.export');
+
+// Placeholder
+require('./$.core')._ = path._ = path._ || {};
+
+$export($export.P + $export.F, 'Function', {part: require('./$.partial')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.get-iterator-method.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.get-iterator-method.js
new file mode 100644
index 00000000..02db743c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.get-iterator-method.js
@@ -0,0 +1,8 @@
+var classof = require('./$.classof')
+ , ITERATOR = require('./$.wks')('iterator')
+ , Iterators = require('./$.iterators');
+module.exports = require('./$.core').getIteratorMethod = function(it){
+ if(it != undefined)return it[ITERATOR]
+ || it['@@iterator']
+ || Iterators[classof(it)];
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.get-iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.get-iterator.js
new file mode 100644
index 00000000..7290904a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.get-iterator.js
@@ -0,0 +1,7 @@
+var anObject = require('./$.an-object')
+ , get = require('./core.get-iterator-method');
+module.exports = require('./$.core').getIterator = function(it){
+ var iterFn = get(it);
+ if(typeof iterFn != 'function')throw TypeError(it + ' is not iterable!');
+ return anObject(iterFn.call(it));
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.is-iterable.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.is-iterable.js
new file mode 100644
index 00000000..c27e6588
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.is-iterable.js
@@ -0,0 +1,9 @@
+var classof = require('./$.classof')
+ , ITERATOR = require('./$.wks')('iterator')
+ , Iterators = require('./$.iterators');
+module.exports = require('./$.core').isIterable = function(it){
+ var O = Object(it);
+ return O[ITERATOR] !== undefined
+ || '@@iterator' in O
+ || Iterators.hasOwnProperty(classof(O));
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.log.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.log.js
new file mode 100644
index 00000000..4c0ea53d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.log.js
@@ -0,0 +1,26 @@
+var $ = require('./$')
+ , global = require('./$.global')
+ , $export = require('./$.export')
+ , log = {}
+ , enabled = true;
+// Methods from https://github.com/DeveloperToolsWG/console-object/blob/master/api.md
+$.each.call((
+ 'assert,clear,count,debug,dir,dirxml,error,exception,group,groupCollapsed,groupEnd,' +
+ 'info,isIndependentlyComposed,log,markTimeline,profile,profileEnd,table,' +
+ 'time,timeEnd,timeline,timelineEnd,timeStamp,trace,warn'
+).split(','), function(key){
+ log[key] = function(){
+ var $console = global.console;
+ if(enabled && $console && $console[key]){
+ return Function.apply.call($console[key], $console, arguments);
+ }
+ };
+});
+$export($export.G + $export.F, {log: require('./$.object-assign')(log.log, log, {
+ enable: function(){
+ enabled = true;
+ },
+ disable: function(){
+ enabled = false;
+ }
+})});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.number.iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.number.iterator.js
new file mode 100644
index 00000000..d9273780
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.number.iterator.js
@@ -0,0 +1,9 @@
+'use strict';
+require('./$.iter-define')(Number, 'Number', function(iterated){
+ this._l = +iterated;
+ this._i = 0;
+}, function(){
+ var i = this._i++
+ , done = !(i < this._l);
+ return {done: done, value: done ? undefined : i};
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.object.classof.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.object.classof.js
new file mode 100644
index 00000000..df682e44
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.object.classof.js
@@ -0,0 +1,3 @@
+var $export = require('./$.export');
+
+$export($export.S + $export.F, 'Object', {classof: require('./$.classof')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.object.define.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.object.define.js
new file mode 100644
index 00000000..fe4cbe9b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.object.define.js
@@ -0,0 +1,4 @@
+var $export = require('./$.export')
+ , define = require('./$.object-define');
+
+$export($export.S + $export.F, 'Object', {define: define});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.object.is-object.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.object.is-object.js
new file mode 100644
index 00000000..c60a977a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.object.is-object.js
@@ -0,0 +1,3 @@
+var $export = require('./$.export');
+
+$export($export.S + $export.F, 'Object', {isObject: require('./$.is-object')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.object.make.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.object.make.js
new file mode 100644
index 00000000..95b99961
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.object.make.js
@@ -0,0 +1,9 @@
+var $export = require('./$.export')
+ , define = require('./$.object-define')
+ , create = require('./$').create;
+
+$export($export.S + $export.F, 'Object', {
+ make: function(proto, mixin){
+ return define(create(proto), mixin);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.string.escape-html.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.string.escape-html.js
new file mode 100644
index 00000000..81737e75
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.string.escape-html.js
@@ -0,0 +1,11 @@
+'use strict';
+var $export = require('./$.export');
+var $re = require('./$.replacer')(/[&<>"']/g, {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ "'": '''
+});
+
+$export($export.P + $export.F, 'String', {escapeHTML: function escapeHTML(){ return $re(this); }});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.string.unescape-html.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.string.unescape-html.js
new file mode 100644
index 00000000..9d7a3d2c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/core.string.unescape-html.js
@@ -0,0 +1,11 @@
+'use strict';
+var $export = require('./$.export');
+var $re = require('./$.replacer')(/&(?:amp|lt|gt|quot|apos);/g, {
+ '&': '&',
+ '<': '<',
+ '>': '>',
+ '"': '"',
+ ''': "'"
+});
+
+$export($export.P + $export.F, 'String', {unescapeHTML: function unescapeHTML(){ return $re(this); }});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es5.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es5.js
new file mode 100644
index 00000000..50f72b04
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es5.js
@@ -0,0 +1,276 @@
+'use strict';
+var $ = require('./$')
+ , $export = require('./$.export')
+ , DESCRIPTORS = require('./$.descriptors')
+ , createDesc = require('./$.property-desc')
+ , html = require('./$.html')
+ , cel = require('./$.dom-create')
+ , has = require('./$.has')
+ , cof = require('./$.cof')
+ , invoke = require('./$.invoke')
+ , fails = require('./$.fails')
+ , anObject = require('./$.an-object')
+ , aFunction = require('./$.a-function')
+ , isObject = require('./$.is-object')
+ , toObject = require('./$.to-object')
+ , toIObject = require('./$.to-iobject')
+ , toInteger = require('./$.to-integer')
+ , toIndex = require('./$.to-index')
+ , toLength = require('./$.to-length')
+ , IObject = require('./$.iobject')
+ , IE_PROTO = require('./$.uid')('__proto__')
+ , createArrayMethod = require('./$.array-methods')
+ , arrayIndexOf = require('./$.array-includes')(false)
+ , ObjectProto = Object.prototype
+ , ArrayProto = Array.prototype
+ , arraySlice = ArrayProto.slice
+ , arrayJoin = ArrayProto.join
+ , defineProperty = $.setDesc
+ , getOwnDescriptor = $.getDesc
+ , defineProperties = $.setDescs
+ , factories = {}
+ , IE8_DOM_DEFINE;
+
+if(!DESCRIPTORS){
+ IE8_DOM_DEFINE = !fails(function(){
+ return defineProperty(cel('div'), 'a', {get: function(){ return 7; }}).a != 7;
+ });
+ $.setDesc = function(O, P, Attributes){
+ if(IE8_DOM_DEFINE)try {
+ return defineProperty(O, P, Attributes);
+ } catch(e){ /* empty */ }
+ if('get' in Attributes || 'set' in Attributes)throw TypeError('Accessors not supported!');
+ if('value' in Attributes)anObject(O)[P] = Attributes.value;
+ return O;
+ };
+ $.getDesc = function(O, P){
+ if(IE8_DOM_DEFINE)try {
+ return getOwnDescriptor(O, P);
+ } catch(e){ /* empty */ }
+ if(has(O, P))return createDesc(!ObjectProto.propertyIsEnumerable.call(O, P), O[P]);
+ };
+ $.setDescs = defineProperties = function(O, Properties){
+ anObject(O);
+ var keys = $.getKeys(Properties)
+ , length = keys.length
+ , i = 0
+ , P;
+ while(length > i)$.setDesc(O, P = keys[i++], Properties[P]);
+ return O;
+ };
+}
+$export($export.S + $export.F * !DESCRIPTORS, 'Object', {
+ // 19.1.2.6 / 15.2.3.3 Object.getOwnPropertyDescriptor(O, P)
+ getOwnPropertyDescriptor: $.getDesc,
+ // 19.1.2.4 / 15.2.3.6 Object.defineProperty(O, P, Attributes)
+ defineProperty: $.setDesc,
+ // 19.1.2.3 / 15.2.3.7 Object.defineProperties(O, Properties)
+ defineProperties: defineProperties
+});
+
+ // IE 8- don't enum bug keys
+var keys1 = ('constructor,hasOwnProperty,isPrototypeOf,propertyIsEnumerable,' +
+ 'toLocaleString,toString,valueOf').split(',')
+ // Additional keys for getOwnPropertyNames
+ , keys2 = keys1.concat('length', 'prototype')
+ , keysLen1 = keys1.length;
+
+// Create object with `null` prototype: use iframe Object with cleared prototype
+var createDict = function(){
+ // Thrash, waste and sodomy: IE GC bug
+ var iframe = cel('iframe')
+ , i = keysLen1
+ , gt = '>'
+ , iframeDocument;
+ iframe.style.display = 'none';
+ html.appendChild(iframe);
+ iframe.src = 'javascript:'; // eslint-disable-line no-script-url
+ // createDict = iframe.contentWindow.Object;
+ // html.removeChild(iframe);
+ iframeDocument = iframe.contentWindow.document;
+ iframeDocument.open();
+ iframeDocument.write(' i)if(has(O, key = names[i++])){
+ ~arrayIndexOf(result, key) || result.push(key);
+ }
+ return result;
+ };
+};
+var Empty = function(){};
+$export($export.S, 'Object', {
+ // 19.1.2.9 / 15.2.3.2 Object.getPrototypeOf(O)
+ getPrototypeOf: $.getProto = $.getProto || function(O){
+ O = toObject(O);
+ if(has(O, IE_PROTO))return O[IE_PROTO];
+ if(typeof O.constructor == 'function' && O instanceof O.constructor){
+ return O.constructor.prototype;
+ } return O instanceof Object ? ObjectProto : null;
+ },
+ // 19.1.2.7 / 15.2.3.4 Object.getOwnPropertyNames(O)
+ getOwnPropertyNames: $.getNames = $.getNames || createGetKeys(keys2, keys2.length, true),
+ // 19.1.2.2 / 15.2.3.5 Object.create(O [, Properties])
+ create: $.create = $.create || function(O, /*?*/Properties){
+ var result;
+ if(O !== null){
+ Empty.prototype = anObject(O);
+ result = new Empty();
+ Empty.prototype = null;
+ // add "__proto__" for Object.getPrototypeOf shim
+ result[IE_PROTO] = O;
+ } else result = createDict();
+ return Properties === undefined ? result : defineProperties(result, Properties);
+ },
+ // 19.1.2.14 / 15.2.3.14 Object.keys(O)
+ keys: $.getKeys = $.getKeys || createGetKeys(keys1, keysLen1, false)
+});
+
+var construct = function(F, len, args){
+ if(!(len in factories)){
+ for(var n = [], i = 0; i < len; i++)n[i] = 'a[' + i + ']';
+ factories[len] = Function('F,a', 'return new F(' + n.join(',') + ')');
+ }
+ return factories[len](F, args);
+};
+
+// 19.2.3.2 / 15.3.4.5 Function.prototype.bind(thisArg, args...)
+$export($export.P, 'Function', {
+ bind: function bind(that /*, args... */){
+ var fn = aFunction(this)
+ , partArgs = arraySlice.call(arguments, 1);
+ var bound = function(/* args... */){
+ var args = partArgs.concat(arraySlice.call(arguments));
+ return this instanceof bound ? construct(fn, args.length, args) : invoke(fn, args, that);
+ };
+ if(isObject(fn.prototype))bound.prototype = fn.prototype;
+ return bound;
+ }
+});
+
+// fallback for not array-like ES3 strings and DOM objects
+$export($export.P + $export.F * fails(function(){
+ if(html)arraySlice.call(html);
+}), 'Array', {
+ slice: function(begin, end){
+ var len = toLength(this.length)
+ , klass = cof(this);
+ end = end === undefined ? len : end;
+ if(klass == 'Array')return arraySlice.call(this, begin, end);
+ var start = toIndex(begin, len)
+ , upTo = toIndex(end, len)
+ , size = toLength(upTo - start)
+ , cloned = Array(size)
+ , i = 0;
+ for(; i < size; i++)cloned[i] = klass == 'String'
+ ? this.charAt(start + i)
+ : this[start + i];
+ return cloned;
+ }
+});
+$export($export.P + $export.F * (IObject != Object), 'Array', {
+ join: function join(separator){
+ return arrayJoin.call(IObject(this), separator === undefined ? ',' : separator);
+ }
+});
+
+// 22.1.2.2 / 15.4.3.2 Array.isArray(arg)
+$export($export.S, 'Array', {isArray: require('./$.is-array')});
+
+var createArrayReduce = function(isRight){
+ return function(callbackfn, memo){
+ aFunction(callbackfn);
+ var O = IObject(this)
+ , length = toLength(O.length)
+ , index = isRight ? length - 1 : 0
+ , i = isRight ? -1 : 1;
+ if(arguments.length < 2)for(;;){
+ if(index in O){
+ memo = O[index];
+ index += i;
+ break;
+ }
+ index += i;
+ if(isRight ? index < 0 : length <= index){
+ throw TypeError('Reduce of empty array with no initial value');
+ }
+ }
+ for(;isRight ? index >= 0 : length > index; index += i)if(index in O){
+ memo = callbackfn(memo, O[index], index, this);
+ }
+ return memo;
+ };
+};
+
+var methodize = function($fn){
+ return function(arg1/*, arg2 = undefined */){
+ return $fn(this, arg1, arguments[1]);
+ };
+};
+
+$export($export.P, 'Array', {
+ // 22.1.3.10 / 15.4.4.18 Array.prototype.forEach(callbackfn [, thisArg])
+ forEach: $.each = $.each || methodize(createArrayMethod(0)),
+ // 22.1.3.15 / 15.4.4.19 Array.prototype.map(callbackfn [, thisArg])
+ map: methodize(createArrayMethod(1)),
+ // 22.1.3.7 / 15.4.4.20 Array.prototype.filter(callbackfn [, thisArg])
+ filter: methodize(createArrayMethod(2)),
+ // 22.1.3.23 / 15.4.4.17 Array.prototype.some(callbackfn [, thisArg])
+ some: methodize(createArrayMethod(3)),
+ // 22.1.3.5 / 15.4.4.16 Array.prototype.every(callbackfn [, thisArg])
+ every: methodize(createArrayMethod(4)),
+ // 22.1.3.18 / 15.4.4.21 Array.prototype.reduce(callbackfn [, initialValue])
+ reduce: createArrayReduce(false),
+ // 22.1.3.19 / 15.4.4.22 Array.prototype.reduceRight(callbackfn [, initialValue])
+ reduceRight: createArrayReduce(true),
+ // 22.1.3.11 / 15.4.4.14 Array.prototype.indexOf(searchElement [, fromIndex])
+ indexOf: methodize(arrayIndexOf),
+ // 22.1.3.14 / 15.4.4.15 Array.prototype.lastIndexOf(searchElement [, fromIndex])
+ lastIndexOf: function(el, fromIndex /* = @[*-1] */){
+ var O = toIObject(this)
+ , length = toLength(O.length)
+ , index = length - 1;
+ if(arguments.length > 1)index = Math.min(index, toInteger(fromIndex));
+ if(index < 0)index = toLength(length + index);
+ for(;index >= 0; index--)if(index in O)if(O[index] === el)return index;
+ return -1;
+ }
+});
+
+// 20.3.3.1 / 15.9.4.4 Date.now()
+$export($export.S, 'Date', {now: function(){ return +new Date; }});
+
+var lz = function(num){
+ return num > 9 ? num : '0' + num;
+};
+
+// 20.3.4.36 / 15.9.5.43 Date.prototype.toISOString()
+// PhantomJS / old WebKit has a broken implementations
+$export($export.P + $export.F * (fails(function(){
+ return new Date(-5e13 - 1).toISOString() != '0385-07-25T07:06:39.999Z';
+}) || !fails(function(){
+ new Date(NaN).toISOString();
+})), 'Date', {
+ toISOString: function toISOString(){
+ if(!isFinite(this))throw RangeError('Invalid time value');
+ var d = this
+ , y = d.getUTCFullYear()
+ , m = d.getUTCMilliseconds()
+ , s = y < 0 ? '-' : y > 9999 ? '+' : '';
+ return s + ('00000' + Math.abs(y)).slice(s ? -6 : -4) +
+ '-' + lz(d.getUTCMonth() + 1) + '-' + lz(d.getUTCDate()) +
+ 'T' + lz(d.getUTCHours()) + ':' + lz(d.getUTCMinutes()) +
+ ':' + lz(d.getUTCSeconds()) + '.' + (m > 99 ? m : '0' + lz(m)) + 'Z';
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.copy-within.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.copy-within.js
new file mode 100644
index 00000000..930ba78d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.copy-within.js
@@ -0,0 +1,6 @@
+// 22.1.3.3 Array.prototype.copyWithin(target, start, end = this.length)
+var $export = require('./$.export');
+
+$export($export.P, 'Array', {copyWithin: require('./$.array-copy-within')});
+
+require('./$.add-to-unscopables')('copyWithin');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.fill.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.fill.js
new file mode 100644
index 00000000..c3b3e2e5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.fill.js
@@ -0,0 +1,6 @@
+// 22.1.3.6 Array.prototype.fill(value, start = 0, end = this.length)
+var $export = require('./$.export');
+
+$export($export.P, 'Array', {fill: require('./$.array-fill')});
+
+require('./$.add-to-unscopables')('fill');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.find-index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.find-index.js
new file mode 100644
index 00000000..7224a606
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.find-index.js
@@ -0,0 +1,14 @@
+'use strict';
+// 22.1.3.9 Array.prototype.findIndex(predicate, thisArg = undefined)
+var $export = require('./$.export')
+ , $find = require('./$.array-methods')(6)
+ , KEY = 'findIndex'
+ , forced = true;
+// Shouldn't skip holes
+if(KEY in [])Array(1)[KEY](function(){ forced = false; });
+$export($export.P + $export.F * forced, 'Array', {
+ findIndex: function findIndex(callbackfn/*, that = undefined */){
+ return $find(this, callbackfn, arguments.length > 1 ? arguments[1] : undefined);
+ }
+});
+require('./$.add-to-unscopables')(KEY);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.find.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.find.js
new file mode 100644
index 00000000..199e987e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.find.js
@@ -0,0 +1,14 @@
+'use strict';
+// 22.1.3.8 Array.prototype.find(predicate, thisArg = undefined)
+var $export = require('./$.export')
+ , $find = require('./$.array-methods')(5)
+ , KEY = 'find'
+ , forced = true;
+// Shouldn't skip holes
+if(KEY in [])Array(1)[KEY](function(){ forced = false; });
+$export($export.P + $export.F * forced, 'Array', {
+ find: function find(callbackfn/*, that = undefined */){
+ return $find(this, callbackfn, arguments.length > 1 ? arguments[1] : undefined);
+ }
+});
+require('./$.add-to-unscopables')(KEY);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.from.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.from.js
new file mode 100644
index 00000000..4637d8d2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.from.js
@@ -0,0 +1,36 @@
+'use strict';
+var ctx = require('./$.ctx')
+ , $export = require('./$.export')
+ , toObject = require('./$.to-object')
+ , call = require('./$.iter-call')
+ , isArrayIter = require('./$.is-array-iter')
+ , toLength = require('./$.to-length')
+ , getIterFn = require('./core.get-iterator-method');
+$export($export.S + $export.F * !require('./$.iter-detect')(function(iter){ Array.from(iter); }), 'Array', {
+ // 22.1.2.1 Array.from(arrayLike, mapfn = undefined, thisArg = undefined)
+ from: function from(arrayLike/*, mapfn = undefined, thisArg = undefined*/){
+ var O = toObject(arrayLike)
+ , C = typeof this == 'function' ? this : Array
+ , $$ = arguments
+ , $$len = $$.length
+ , mapfn = $$len > 1 ? $$[1] : undefined
+ , mapping = mapfn !== undefined
+ , index = 0
+ , iterFn = getIterFn(O)
+ , length, result, step, iterator;
+ if(mapping)mapfn = ctx(mapfn, $$len > 2 ? $$[2] : undefined, 2);
+ // if object isn't iterable or it's array with default iterator - use simple case
+ if(iterFn != undefined && !(C == Array && isArrayIter(iterFn))){
+ for(iterator = iterFn.call(O), result = new C; !(step = iterator.next()).done; index++){
+ result[index] = mapping ? call(iterator, mapfn, [step.value, index], true) : step.value;
+ }
+ } else {
+ length = toLength(O.length);
+ for(result = new C(length); length > index; index++){
+ result[index] = mapping ? mapfn(O[index], index) : O[index];
+ }
+ }
+ result.length = index;
+ return result;
+ }
+});
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.iterator.js
new file mode 100644
index 00000000..52a546dd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.iterator.js
@@ -0,0 +1,34 @@
+'use strict';
+var addToUnscopables = require('./$.add-to-unscopables')
+ , step = require('./$.iter-step')
+ , Iterators = require('./$.iterators')
+ , toIObject = require('./$.to-iobject');
+
+// 22.1.3.4 Array.prototype.entries()
+// 22.1.3.13 Array.prototype.keys()
+// 22.1.3.29 Array.prototype.values()
+// 22.1.3.30 Array.prototype[@@iterator]()
+module.exports = require('./$.iter-define')(Array, 'Array', function(iterated, kind){
+ this._t = toIObject(iterated); // target
+ this._i = 0; // next index
+ this._k = kind; // kind
+// 22.1.5.2.1 %ArrayIteratorPrototype%.next()
+}, function(){
+ var O = this._t
+ , kind = this._k
+ , index = this._i++;
+ if(!O || index >= O.length){
+ this._t = undefined;
+ return step(1);
+ }
+ if(kind == 'keys' )return step(0, index);
+ if(kind == 'values')return step(0, O[index]);
+ return step(0, [index, O[index]]);
+}, 'values');
+
+// argumentsList[@@iterator] is %ArrayProto_values% (9.4.4.6, 9.4.4.7)
+Iterators.Arguments = Iterators.Array;
+
+addToUnscopables('keys');
+addToUnscopables('values');
+addToUnscopables('entries');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.of.js
new file mode 100644
index 00000000..f623f157
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.of.js
@@ -0,0 +1,19 @@
+'use strict';
+var $export = require('./$.export');
+
+// WebKit Array.of isn't generic
+$export($export.S + $export.F * require('./$.fails')(function(){
+ function F(){}
+ return !(Array.of.call(F) instanceof F);
+}), 'Array', {
+ // 22.1.2.3 Array.of( ...items)
+ of: function of(/* ...args */){
+ var index = 0
+ , $$ = arguments
+ , $$len = $$.length
+ , result = new (typeof this == 'function' ? this : Array)($$len);
+ while($$len > index)result[index] = $$[index++];
+ result.length = $$len;
+ return result;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.species.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.species.js
new file mode 100644
index 00000000..543bdfe9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.array.species.js
@@ -0,0 +1 @@
+require('./$.set-species')('Array');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.date.to-string.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.date.to-string.js
new file mode 100644
index 00000000..59bf8365
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.date.to-string.js
@@ -0,0 +1,10 @@
+var DateProto = Date.prototype
+ , INVALID_DATE = 'Invalid Date'
+ , TO_STRING = 'toString'
+ , $toString = DateProto[TO_STRING];
+if(new Date(NaN) + '' != INVALID_DATE){
+ require('./$.redefine')(DateProto, TO_STRING, function toString(){
+ var value = +this;
+ return value === value ? $toString.call(this) : INVALID_DATE;
+ });
+}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.function.has-instance.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.function.has-instance.js
new file mode 100644
index 00000000..94d840f8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.function.has-instance.js
@@ -0,0 +1,13 @@
+'use strict';
+var $ = require('./$')
+ , isObject = require('./$.is-object')
+ , HAS_INSTANCE = require('./$.wks')('hasInstance')
+ , FunctionProto = Function.prototype;
+// 19.2.3.6 Function.prototype[@@hasInstance](V)
+if(!(HAS_INSTANCE in FunctionProto))$.setDesc(FunctionProto, HAS_INSTANCE, {value: function(O){
+ if(typeof this != 'function' || !isObject(O))return false;
+ if(!isObject(this.prototype))return O instanceof this;
+ // for environment w/o native `@@hasInstance` logic enough `instanceof`, but add this:
+ while(O = $.getProto(O))if(this.prototype === O)return true;
+ return false;
+}});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.function.name.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.function.name.js
new file mode 100644
index 00000000..0f10fc11
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.function.name.js
@@ -0,0 +1,16 @@
+var setDesc = require('./$').setDesc
+ , createDesc = require('./$.property-desc')
+ , has = require('./$.has')
+ , FProto = Function.prototype
+ , nameRE = /^\s*function ([^ (]*)/
+ , NAME = 'name';
+// 19.2.4.2 name
+NAME in FProto || require('./$.descriptors') && setDesc(FProto, NAME, {
+ configurable: true,
+ get: function(){
+ var match = ('' + this).match(nameRE)
+ , name = match ? match[1] : '';
+ has(this, NAME) || setDesc(this, NAME, createDesc(5, name));
+ return name;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.map.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.map.js
new file mode 100644
index 00000000..54fd5c1a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.map.js
@@ -0,0 +1,17 @@
+'use strict';
+var strong = require('./$.collection-strong');
+
+// 23.1 Map Objects
+require('./$.collection')('Map', function(get){
+ return function Map(){ return get(this, arguments.length > 0 ? arguments[0] : undefined); };
+}, {
+ // 23.1.3.6 Map.prototype.get(key)
+ get: function get(key){
+ var entry = strong.getEntry(this, key);
+ return entry && entry.v;
+ },
+ // 23.1.3.9 Map.prototype.set(key, value)
+ set: function set(key, value){
+ return strong.def(this, key === 0 ? 0 : key, value);
+ }
+}, strong, true);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.acosh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.acosh.js
new file mode 100644
index 00000000..f69282a8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.acosh.js
@@ -0,0 +1,14 @@
+// 20.2.2.3 Math.acosh(x)
+var $export = require('./$.export')
+ , log1p = require('./$.math-log1p')
+ , sqrt = Math.sqrt
+ , $acosh = Math.acosh;
+
+// V8 bug https://code.google.com/p/v8/issues/detail?id=3509
+$export($export.S + $export.F * !($acosh && Math.floor($acosh(Number.MAX_VALUE)) == 710), 'Math', {
+ acosh: function acosh(x){
+ return (x = +x) < 1 ? NaN : x > 94906265.62425156
+ ? Math.log(x) + Math.LN2
+ : log1p(x - 1 + sqrt(x - 1) * sqrt(x + 1));
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.asinh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.asinh.js
new file mode 100644
index 00000000..bd34adf0
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.asinh.js
@@ -0,0 +1,8 @@
+// 20.2.2.5 Math.asinh(x)
+var $export = require('./$.export');
+
+function asinh(x){
+ return !isFinite(x = +x) || x == 0 ? x : x < 0 ? -asinh(-x) : Math.log(x + Math.sqrt(x * x + 1));
+}
+
+$export($export.S, 'Math', {asinh: asinh});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.atanh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.atanh.js
new file mode 100644
index 00000000..656ea409
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.atanh.js
@@ -0,0 +1,8 @@
+// 20.2.2.7 Math.atanh(x)
+var $export = require('./$.export');
+
+$export($export.S, 'Math', {
+ atanh: function atanh(x){
+ return (x = +x) == 0 ? x : Math.log((1 + x) / (1 - x)) / 2;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.cbrt.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.cbrt.js
new file mode 100644
index 00000000..79a1fbc8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.cbrt.js
@@ -0,0 +1,9 @@
+// 20.2.2.9 Math.cbrt(x)
+var $export = require('./$.export')
+ , sign = require('./$.math-sign');
+
+$export($export.S, 'Math', {
+ cbrt: function cbrt(x){
+ return sign(x = +x) * Math.pow(Math.abs(x), 1 / 3);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.clz32.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.clz32.js
new file mode 100644
index 00000000..edd11588
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.clz32.js
@@ -0,0 +1,8 @@
+// 20.2.2.11 Math.clz32(x)
+var $export = require('./$.export');
+
+$export($export.S, 'Math', {
+ clz32: function clz32(x){
+ return (x >>>= 0) ? 31 - Math.floor(Math.log(x + 0.5) * Math.LOG2E) : 32;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.cosh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.cosh.js
new file mode 100644
index 00000000..d1df7490
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.cosh.js
@@ -0,0 +1,9 @@
+// 20.2.2.12 Math.cosh(x)
+var $export = require('./$.export')
+ , exp = Math.exp;
+
+$export($export.S, 'Math', {
+ cosh: function cosh(x){
+ return (exp(x = +x) + exp(-x)) / 2;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.expm1.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.expm1.js
new file mode 100644
index 00000000..e27742fe
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.expm1.js
@@ -0,0 +1,4 @@
+// 20.2.2.14 Math.expm1(x)
+var $export = require('./$.export');
+
+$export($export.S, 'Math', {expm1: require('./$.math-expm1')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.fround.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.fround.js
new file mode 100644
index 00000000..43cd70c7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.fround.js
@@ -0,0 +1,26 @@
+// 20.2.2.16 Math.fround(x)
+var $export = require('./$.export')
+ , sign = require('./$.math-sign')
+ , pow = Math.pow
+ , EPSILON = pow(2, -52)
+ , EPSILON32 = pow(2, -23)
+ , MAX32 = pow(2, 127) * (2 - EPSILON32)
+ , MIN32 = pow(2, -126);
+
+var roundTiesToEven = function(n){
+ return n + 1 / EPSILON - 1 / EPSILON;
+};
+
+
+$export($export.S, 'Math', {
+ fround: function fround(x){
+ var $abs = Math.abs(x)
+ , $sign = sign(x)
+ , a, result;
+ if($abs < MIN32)return $sign * roundTiesToEven($abs / MIN32 / EPSILON32) * MIN32 * EPSILON32;
+ a = (1 + EPSILON32 / EPSILON) * $abs;
+ result = a - (a - $abs);
+ if(result > MAX32 || result != result)return $sign * Infinity;
+ return $sign * result;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.hypot.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.hypot.js
new file mode 100644
index 00000000..a8edf7cd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.hypot.js
@@ -0,0 +1,26 @@
+// 20.2.2.17 Math.hypot([value1[, value2[, … ]]])
+var $export = require('./$.export')
+ , abs = Math.abs;
+
+$export($export.S, 'Math', {
+ hypot: function hypot(value1, value2){ // eslint-disable-line no-unused-vars
+ var sum = 0
+ , i = 0
+ , $$ = arguments
+ , $$len = $$.length
+ , larg = 0
+ , arg, div;
+ while(i < $$len){
+ arg = abs($$[i++]);
+ if(larg < arg){
+ div = larg / arg;
+ sum = sum * div * div + 1;
+ larg = arg;
+ } else if(arg > 0){
+ div = arg / larg;
+ sum += div * div;
+ } else sum += arg;
+ }
+ return larg === Infinity ? Infinity : larg * Math.sqrt(sum);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.imul.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.imul.js
new file mode 100644
index 00000000..926053de
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.imul.js
@@ -0,0 +1,17 @@
+// 20.2.2.18 Math.imul(x, y)
+var $export = require('./$.export')
+ , $imul = Math.imul;
+
+// some WebKit versions fails with big numbers, some has wrong arity
+$export($export.S + $export.F * require('./$.fails')(function(){
+ return $imul(0xffffffff, 5) != -5 || $imul.length != 2;
+}), 'Math', {
+ imul: function imul(x, y){
+ var UINT16 = 0xffff
+ , xn = +x
+ , yn = +y
+ , xl = UINT16 & xn
+ , yl = UINT16 & yn;
+ return 0 | xl * yl + ((UINT16 & xn >>> 16) * yl + xl * (UINT16 & yn >>> 16) << 16 >>> 0);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.log10.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.log10.js
new file mode 100644
index 00000000..ef5ae6a9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.log10.js
@@ -0,0 +1,8 @@
+// 20.2.2.21 Math.log10(x)
+var $export = require('./$.export');
+
+$export($export.S, 'Math', {
+ log10: function log10(x){
+ return Math.log(x) / Math.LN10;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.log1p.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.log1p.js
new file mode 100644
index 00000000..31c395ec
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.log1p.js
@@ -0,0 +1,4 @@
+// 20.2.2.20 Math.log1p(x)
+var $export = require('./$.export');
+
+$export($export.S, 'Math', {log1p: require('./$.math-log1p')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.log2.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.log2.js
new file mode 100644
index 00000000..24c0124b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.log2.js
@@ -0,0 +1,8 @@
+// 20.2.2.22 Math.log2(x)
+var $export = require('./$.export');
+
+$export($export.S, 'Math', {
+ log2: function log2(x){
+ return Math.log(x) / Math.LN2;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.sign.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.sign.js
new file mode 100644
index 00000000..fd8d8bc3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.sign.js
@@ -0,0 +1,4 @@
+// 20.2.2.28 Math.sign(x)
+var $export = require('./$.export');
+
+$export($export.S, 'Math', {sign: require('./$.math-sign')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.sinh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.sinh.js
new file mode 100644
index 00000000..a01c4d37
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.sinh.js
@@ -0,0 +1,15 @@
+// 20.2.2.30 Math.sinh(x)
+var $export = require('./$.export')
+ , expm1 = require('./$.math-expm1')
+ , exp = Math.exp;
+
+// V8 near Chromium 38 has a problem with very small numbers
+$export($export.S + $export.F * require('./$.fails')(function(){
+ return !Math.sinh(-2e-17) != -2e-17;
+}), 'Math', {
+ sinh: function sinh(x){
+ return Math.abs(x = +x) < 1
+ ? (expm1(x) - expm1(-x)) / 2
+ : (exp(x - 1) - exp(-x - 1)) * (Math.E / 2);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.tanh.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.tanh.js
new file mode 100644
index 00000000..0d081a5d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.tanh.js
@@ -0,0 +1,12 @@
+// 20.2.2.33 Math.tanh(x)
+var $export = require('./$.export')
+ , expm1 = require('./$.math-expm1')
+ , exp = Math.exp;
+
+$export($export.S, 'Math', {
+ tanh: function tanh(x){
+ var a = expm1(x = +x)
+ , b = expm1(-x);
+ return a == Infinity ? 1 : b == Infinity ? -1 : (a - b) / (exp(x) + exp(-x));
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.trunc.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.trunc.js
new file mode 100644
index 00000000..f3f08559
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.math.trunc.js
@@ -0,0 +1,8 @@
+// 20.2.2.34 Math.trunc(x)
+var $export = require('./$.export');
+
+$export($export.S, 'Math', {
+ trunc: function trunc(it){
+ return (it > 0 ? Math.floor : Math.ceil)(it);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.constructor.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.constructor.js
new file mode 100644
index 00000000..065af1a9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.constructor.js
@@ -0,0 +1,66 @@
+'use strict';
+var $ = require('./$')
+ , global = require('./$.global')
+ , has = require('./$.has')
+ , cof = require('./$.cof')
+ , toPrimitive = require('./$.to-primitive')
+ , fails = require('./$.fails')
+ , $trim = require('./$.string-trim').trim
+ , NUMBER = 'Number'
+ , $Number = global[NUMBER]
+ , Base = $Number
+ , proto = $Number.prototype
+ // Opera ~12 has broken Object#toString
+ , BROKEN_COF = cof($.create(proto)) == NUMBER
+ , TRIM = 'trim' in String.prototype;
+
+// 7.1.3 ToNumber(argument)
+var toNumber = function(argument){
+ var it = toPrimitive(argument, false);
+ if(typeof it == 'string' && it.length > 2){
+ it = TRIM ? it.trim() : $trim(it, 3);
+ var first = it.charCodeAt(0)
+ , third, radix, maxCode;
+ if(first === 43 || first === 45){
+ third = it.charCodeAt(2);
+ if(third === 88 || third === 120)return NaN; // Number('+0x1') should be NaN, old V8 fix
+ } else if(first === 48){
+ switch(it.charCodeAt(1)){
+ case 66 : case 98 : radix = 2; maxCode = 49; break; // fast equal /^0b[01]+$/i
+ case 79 : case 111 : radix = 8; maxCode = 55; break; // fast equal /^0o[0-7]+$/i
+ default : return +it;
+ }
+ for(var digits = it.slice(2), i = 0, l = digits.length, code; i < l; i++){
+ code = digits.charCodeAt(i);
+ // parseInt parses a string to a first unavailable symbol
+ // but ToNumber should return NaN if a string contains unavailable symbols
+ if(code < 48 || code > maxCode)return NaN;
+ } return parseInt(digits, radix);
+ }
+ } return +it;
+};
+
+if(!$Number(' 0o1') || !$Number('0b1') || $Number('+0x1')){
+ $Number = function Number(value){
+ var it = arguments.length < 1 ? 0 : value
+ , that = this;
+ return that instanceof $Number
+ // check on 1..constructor(foo) case
+ && (BROKEN_COF ? fails(function(){ proto.valueOf.call(that); }) : cof(that) != NUMBER)
+ ? new Base(toNumber(it)) : toNumber(it);
+ };
+ $.each.call(require('./$.descriptors') ? $.getNames(Base) : (
+ // ES3:
+ 'MAX_VALUE,MIN_VALUE,NaN,NEGATIVE_INFINITY,POSITIVE_INFINITY,' +
+ // ES6 (in case, if modules with ES6 Number statics required before):
+ 'EPSILON,isFinite,isInteger,isNaN,isSafeInteger,MAX_SAFE_INTEGER,' +
+ 'MIN_SAFE_INTEGER,parseFloat,parseInt,isInteger'
+ ).split(','), function(key){
+ if(has(Base, key) && !has($Number, key)){
+ $.setDesc($Number, key, $.getDesc(Base, key));
+ }
+ });
+ $Number.prototype = proto;
+ proto.constructor = $Number;
+ require('./$.redefine')(global, NUMBER, $Number);
+}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.epsilon.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.epsilon.js
new file mode 100644
index 00000000..45c865cf
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.epsilon.js
@@ -0,0 +1,4 @@
+// 20.1.2.1 Number.EPSILON
+var $export = require('./$.export');
+
+$export($export.S, 'Number', {EPSILON: Math.pow(2, -52)});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.is-finite.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.is-finite.js
new file mode 100644
index 00000000..362a6c80
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.is-finite.js
@@ -0,0 +1,9 @@
+// 20.1.2.2 Number.isFinite(number)
+var $export = require('./$.export')
+ , _isFinite = require('./$.global').isFinite;
+
+$export($export.S, 'Number', {
+ isFinite: function isFinite(it){
+ return typeof it == 'number' && _isFinite(it);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.is-integer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.is-integer.js
new file mode 100644
index 00000000..189db9a2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.is-integer.js
@@ -0,0 +1,4 @@
+// 20.1.2.3 Number.isInteger(number)
+var $export = require('./$.export');
+
+$export($export.S, 'Number', {isInteger: require('./$.is-integer')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.is-nan.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.is-nan.js
new file mode 100644
index 00000000..151bb4b2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.is-nan.js
@@ -0,0 +1,8 @@
+// 20.1.2.4 Number.isNaN(number)
+var $export = require('./$.export');
+
+$export($export.S, 'Number', {
+ isNaN: function isNaN(number){
+ return number != number;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.is-safe-integer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.is-safe-integer.js
new file mode 100644
index 00000000..e23b4cbc
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.is-safe-integer.js
@@ -0,0 +1,10 @@
+// 20.1.2.5 Number.isSafeInteger(number)
+var $export = require('./$.export')
+ , isInteger = require('./$.is-integer')
+ , abs = Math.abs;
+
+$export($export.S, 'Number', {
+ isSafeInteger: function isSafeInteger(number){
+ return isInteger(number) && abs(number) <= 0x1fffffffffffff;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.max-safe-integer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.max-safe-integer.js
new file mode 100644
index 00000000..a1aaf741
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.max-safe-integer.js
@@ -0,0 +1,4 @@
+// 20.1.2.6 Number.MAX_SAFE_INTEGER
+var $export = require('./$.export');
+
+$export($export.S, 'Number', {MAX_SAFE_INTEGER: 0x1fffffffffffff});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.min-safe-integer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.min-safe-integer.js
new file mode 100644
index 00000000..ab97cb5d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.min-safe-integer.js
@@ -0,0 +1,4 @@
+// 20.1.2.10 Number.MIN_SAFE_INTEGER
+var $export = require('./$.export');
+
+$export($export.S, 'Number', {MIN_SAFE_INTEGER: -0x1fffffffffffff});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.parse-float.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.parse-float.js
new file mode 100644
index 00000000..1d0c9674
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.parse-float.js
@@ -0,0 +1,4 @@
+// 20.1.2.12 Number.parseFloat(string)
+var $export = require('./$.export');
+
+$export($export.S, 'Number', {parseFloat: parseFloat});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.parse-int.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.parse-int.js
new file mode 100644
index 00000000..813b5b79
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.number.parse-int.js
@@ -0,0 +1,4 @@
+// 20.1.2.13 Number.parseInt(string, radix)
+var $export = require('./$.export');
+
+$export($export.S, 'Number', {parseInt: parseInt});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.assign.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.assign.js
new file mode 100644
index 00000000..b62e7a42
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.assign.js
@@ -0,0 +1,4 @@
+// 19.1.3.1 Object.assign(target, source)
+var $export = require('./$.export');
+
+$export($export.S + $export.F, 'Object', {assign: require('./$.object-assign')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.freeze.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.freeze.js
new file mode 100644
index 00000000..fa87c951
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.freeze.js
@@ -0,0 +1,8 @@
+// 19.1.2.5 Object.freeze(O)
+var isObject = require('./$.is-object');
+
+require('./$.object-sap')('freeze', function($freeze){
+ return function freeze(it){
+ return $freeze && isObject(it) ? $freeze(it) : it;
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.get-own-property-descriptor.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.get-own-property-descriptor.js
new file mode 100644
index 00000000..9b253acd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.get-own-property-descriptor.js
@@ -0,0 +1,8 @@
+// 19.1.2.6 Object.getOwnPropertyDescriptor(O, P)
+var toIObject = require('./$.to-iobject');
+
+require('./$.object-sap')('getOwnPropertyDescriptor', function($getOwnPropertyDescriptor){
+ return function getOwnPropertyDescriptor(it, key){
+ return $getOwnPropertyDescriptor(toIObject(it), key);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.get-own-property-names.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.get-own-property-names.js
new file mode 100644
index 00000000..e87bcf60
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.get-own-property-names.js
@@ -0,0 +1,4 @@
+// 19.1.2.7 Object.getOwnPropertyNames(O)
+require('./$.object-sap')('getOwnPropertyNames', function(){
+ return require('./$.get-names').get;
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.get-prototype-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.get-prototype-of.js
new file mode 100644
index 00000000..9ec0405b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.get-prototype-of.js
@@ -0,0 +1,8 @@
+// 19.1.2.9 Object.getPrototypeOf(O)
+var toObject = require('./$.to-object');
+
+require('./$.object-sap')('getPrototypeOf', function($getPrototypeOf){
+ return function getPrototypeOf(it){
+ return $getPrototypeOf(toObject(it));
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.is-extensible.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.is-extensible.js
new file mode 100644
index 00000000..ada2b95a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.is-extensible.js
@@ -0,0 +1,8 @@
+// 19.1.2.11 Object.isExtensible(O)
+var isObject = require('./$.is-object');
+
+require('./$.object-sap')('isExtensible', function($isExtensible){
+ return function isExtensible(it){
+ return isObject(it) ? $isExtensible ? $isExtensible(it) : true : false;
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.is-frozen.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.is-frozen.js
new file mode 100644
index 00000000..b3e44d1b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.is-frozen.js
@@ -0,0 +1,8 @@
+// 19.1.2.12 Object.isFrozen(O)
+var isObject = require('./$.is-object');
+
+require('./$.object-sap')('isFrozen', function($isFrozen){
+ return function isFrozen(it){
+ return isObject(it) ? $isFrozen ? $isFrozen(it) : false : true;
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.is-sealed.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.is-sealed.js
new file mode 100644
index 00000000..423caf33
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.is-sealed.js
@@ -0,0 +1,8 @@
+// 19.1.2.13 Object.isSealed(O)
+var isObject = require('./$.is-object');
+
+require('./$.object-sap')('isSealed', function($isSealed){
+ return function isSealed(it){
+ return isObject(it) ? $isSealed ? $isSealed(it) : false : true;
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.is.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.is.js
new file mode 100644
index 00000000..3ae3b604
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.is.js
@@ -0,0 +1,3 @@
+// 19.1.3.10 Object.is(value1, value2)
+var $export = require('./$.export');
+$export($export.S, 'Object', {is: require('./$.same-value')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.keys.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.keys.js
new file mode 100644
index 00000000..e3c18c02
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.keys.js
@@ -0,0 +1,8 @@
+// 19.1.2.14 Object.keys(O)
+var toObject = require('./$.to-object');
+
+require('./$.object-sap')('keys', function($keys){
+ return function keys(it){
+ return $keys(toObject(it));
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.prevent-extensions.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.prevent-extensions.js
new file mode 100644
index 00000000..20f879e2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.prevent-extensions.js
@@ -0,0 +1,8 @@
+// 19.1.2.15 Object.preventExtensions(O)
+var isObject = require('./$.is-object');
+
+require('./$.object-sap')('preventExtensions', function($preventExtensions){
+ return function preventExtensions(it){
+ return $preventExtensions && isObject(it) ? $preventExtensions(it) : it;
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.seal.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.seal.js
new file mode 100644
index 00000000..85a7fa98
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.seal.js
@@ -0,0 +1,8 @@
+// 19.1.2.17 Object.seal(O)
+var isObject = require('./$.is-object');
+
+require('./$.object-sap')('seal', function($seal){
+ return function seal(it){
+ return $seal && isObject(it) ? $seal(it) : it;
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.set-prototype-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.set-prototype-of.js
new file mode 100644
index 00000000..79a147c3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.set-prototype-of.js
@@ -0,0 +1,3 @@
+// 19.1.3.19 Object.setPrototypeOf(O, proto)
+var $export = require('./$.export');
+$export($export.S, 'Object', {setPrototypeOf: require('./$.set-proto').set});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.to-string.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.to-string.js
new file mode 100644
index 00000000..409d5193
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.object.to-string.js
@@ -0,0 +1,10 @@
+'use strict';
+// 19.1.3.6 Object.prototype.toString()
+var classof = require('./$.classof')
+ , test = {};
+test[require('./$.wks')('toStringTag')] = 'z';
+if(test + '' != '[object z]'){
+ require('./$.redefine')(Object.prototype, 'toString', function toString(){
+ return '[object ' + classof(this) + ']';
+ }, true);
+}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.promise.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.promise.js
new file mode 100644
index 00000000..fbf20177
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.promise.js
@@ -0,0 +1,293 @@
+'use strict';
+var $ = require('./$')
+ , LIBRARY = require('./$.library')
+ , global = require('./$.global')
+ , ctx = require('./$.ctx')
+ , classof = require('./$.classof')
+ , $export = require('./$.export')
+ , isObject = require('./$.is-object')
+ , anObject = require('./$.an-object')
+ , aFunction = require('./$.a-function')
+ , strictNew = require('./$.strict-new')
+ , forOf = require('./$.for-of')
+ , setProto = require('./$.set-proto').set
+ , same = require('./$.same-value')
+ , SPECIES = require('./$.wks')('species')
+ , speciesConstructor = require('./$.species-constructor')
+ , asap = require('./$.microtask')
+ , PROMISE = 'Promise'
+ , process = global.process
+ , isNode = classof(process) == 'process'
+ , P = global[PROMISE]
+ , empty = function(){ /* empty */ }
+ , Wrapper;
+
+var testResolve = function(sub){
+ var test = new P(empty), promise;
+ if(sub)test.constructor = function(exec){
+ exec(empty, empty);
+ };
+ (promise = P.resolve(test))['catch'](empty);
+ return promise === test;
+};
+
+var USE_NATIVE = function(){
+ var works = false;
+ function P2(x){
+ var self = new P(x);
+ setProto(self, P2.prototype);
+ return self;
+ }
+ try {
+ works = P && P.resolve && testResolve();
+ setProto(P2, P);
+ P2.prototype = $.create(P.prototype, {constructor: {value: P2}});
+ // actual Firefox has broken subclass support, test that
+ if(!(P2.resolve(5).then(function(){}) instanceof P2)){
+ works = false;
+ }
+ // actual V8 bug, https://code.google.com/p/v8/issues/detail?id=4162
+ if(works && require('./$.descriptors')){
+ var thenableThenGotten = false;
+ P.resolve($.setDesc({}, 'then', {
+ get: function(){ thenableThenGotten = true; }
+ }));
+ works = thenableThenGotten;
+ }
+ } catch(e){ works = false; }
+ return works;
+}();
+
+// helpers
+var sameConstructor = function(a, b){
+ // library wrapper special case
+ if(LIBRARY && a === P && b === Wrapper)return true;
+ return same(a, b);
+};
+var getConstructor = function(C){
+ var S = anObject(C)[SPECIES];
+ return S != undefined ? S : C;
+};
+var isThenable = function(it){
+ var then;
+ return isObject(it) && typeof (then = it.then) == 'function' ? then : false;
+};
+var PromiseCapability = function(C){
+ var resolve, reject;
+ this.promise = new C(function($$resolve, $$reject){
+ if(resolve !== undefined || reject !== undefined)throw TypeError('Bad Promise constructor');
+ resolve = $$resolve;
+ reject = $$reject;
+ });
+ this.resolve = aFunction(resolve),
+ this.reject = aFunction(reject)
+};
+var perform = function(exec){
+ try {
+ exec();
+ } catch(e){
+ return {error: e};
+ }
+};
+var notify = function(record, isReject){
+ if(record.n)return;
+ record.n = true;
+ var chain = record.c;
+ asap(function(){
+ var value = record.v
+ , ok = record.s == 1
+ , i = 0;
+ var run = function(reaction){
+ var handler = ok ? reaction.ok : reaction.fail
+ , resolve = reaction.resolve
+ , reject = reaction.reject
+ , result, then;
+ try {
+ if(handler){
+ if(!ok)record.h = true;
+ result = handler === true ? value : handler(value);
+ if(result === reaction.promise){
+ reject(TypeError('Promise-chain cycle'));
+ } else if(then = isThenable(result)){
+ then.call(result, resolve, reject);
+ } else resolve(result);
+ } else reject(value);
+ } catch(e){
+ reject(e);
+ }
+ };
+ while(chain.length > i)run(chain[i++]); // variable length - can't use forEach
+ chain.length = 0;
+ record.n = false;
+ if(isReject)setTimeout(function(){
+ var promise = record.p
+ , handler, console;
+ if(isUnhandled(promise)){
+ if(isNode){
+ process.emit('unhandledRejection', value, promise);
+ } else if(handler = global.onunhandledrejection){
+ handler({promise: promise, reason: value});
+ } else if((console = global.console) && console.error){
+ console.error('Unhandled promise rejection', value);
+ }
+ } record.a = undefined;
+ }, 1);
+ });
+};
+var isUnhandled = function(promise){
+ var record = promise._d
+ , chain = record.a || record.c
+ , i = 0
+ , reaction;
+ if(record.h)return false;
+ while(chain.length > i){
+ reaction = chain[i++];
+ if(reaction.fail || !isUnhandled(reaction.promise))return false;
+ } return true;
+};
+var $reject = function(value){
+ var record = this;
+ if(record.d)return;
+ record.d = true;
+ record = record.r || record; // unwrap
+ record.v = value;
+ record.s = 2;
+ record.a = record.c.slice();
+ notify(record, true);
+};
+var $resolve = function(value){
+ var record = this
+ , then;
+ if(record.d)return;
+ record.d = true;
+ record = record.r || record; // unwrap
+ try {
+ if(record.p === value)throw TypeError("Promise can't be resolved itself");
+ if(then = isThenable(value)){
+ asap(function(){
+ var wrapper = {r: record, d: false}; // wrap
+ try {
+ then.call(value, ctx($resolve, wrapper, 1), ctx($reject, wrapper, 1));
+ } catch(e){
+ $reject.call(wrapper, e);
+ }
+ });
+ } else {
+ record.v = value;
+ record.s = 1;
+ notify(record, false);
+ }
+ } catch(e){
+ $reject.call({r: record, d: false}, e); // wrap
+ }
+};
+
+// constructor polyfill
+if(!USE_NATIVE){
+ // 25.4.3.1 Promise(executor)
+ P = function Promise(executor){
+ aFunction(executor);
+ var record = this._d = {
+ p: strictNew(this, P, PROMISE), // <- promise
+ c: [], // <- awaiting reactions
+ a: undefined, // <- checked in isUnhandled reactions
+ s: 0, // <- state
+ d: false, // <- done
+ v: undefined, // <- value
+ h: false, // <- handled rejection
+ n: false // <- notify
+ };
+ try {
+ executor(ctx($resolve, record, 1), ctx($reject, record, 1));
+ } catch(err){
+ $reject.call(record, err);
+ }
+ };
+ require('./$.redefine-all')(P.prototype, {
+ // 25.4.5.3 Promise.prototype.then(onFulfilled, onRejected)
+ then: function then(onFulfilled, onRejected){
+ var reaction = new PromiseCapability(speciesConstructor(this, P))
+ , promise = reaction.promise
+ , record = this._d;
+ reaction.ok = typeof onFulfilled == 'function' ? onFulfilled : true;
+ reaction.fail = typeof onRejected == 'function' && onRejected;
+ record.c.push(reaction);
+ if(record.a)record.a.push(reaction);
+ if(record.s)notify(record, false);
+ return promise;
+ },
+ // 25.4.5.1 Promise.prototype.catch(onRejected)
+ 'catch': function(onRejected){
+ return this.then(undefined, onRejected);
+ }
+ });
+}
+
+$export($export.G + $export.W + $export.F * !USE_NATIVE, {Promise: P});
+require('./$.set-to-string-tag')(P, PROMISE);
+require('./$.set-species')(PROMISE);
+Wrapper = require('./$.core')[PROMISE];
+
+// statics
+$export($export.S + $export.F * !USE_NATIVE, PROMISE, {
+ // 25.4.4.5 Promise.reject(r)
+ reject: function reject(r){
+ var capability = new PromiseCapability(this)
+ , $$reject = capability.reject;
+ $$reject(r);
+ return capability.promise;
+ }
+});
+$export($export.S + $export.F * (!USE_NATIVE || testResolve(true)), PROMISE, {
+ // 25.4.4.6 Promise.resolve(x)
+ resolve: function resolve(x){
+ // instanceof instead of internal slot check because we should fix it without replacement native Promise core
+ if(x instanceof P && sameConstructor(x.constructor, this))return x;
+ var capability = new PromiseCapability(this)
+ , $$resolve = capability.resolve;
+ $$resolve(x);
+ return capability.promise;
+ }
+});
+$export($export.S + $export.F * !(USE_NATIVE && require('./$.iter-detect')(function(iter){
+ P.all(iter)['catch'](function(){});
+})), PROMISE, {
+ // 25.4.4.1 Promise.all(iterable)
+ all: function all(iterable){
+ var C = getConstructor(this)
+ , capability = new PromiseCapability(C)
+ , resolve = capability.resolve
+ , reject = capability.reject
+ , values = [];
+ var abrupt = perform(function(){
+ forOf(iterable, false, values.push, values);
+ var remaining = values.length
+ , results = Array(remaining);
+ if(remaining)$.each.call(values, function(promise, index){
+ var alreadyCalled = false;
+ C.resolve(promise).then(function(value){
+ if(alreadyCalled)return;
+ alreadyCalled = true;
+ results[index] = value;
+ --remaining || resolve(results);
+ }, reject);
+ });
+ else resolve(results);
+ });
+ if(abrupt)reject(abrupt.error);
+ return capability.promise;
+ },
+ // 25.4.4.4 Promise.race(iterable)
+ race: function race(iterable){
+ var C = getConstructor(this)
+ , capability = new PromiseCapability(C)
+ , reject = capability.reject;
+ var abrupt = perform(function(){
+ forOf(iterable, false, function(promise){
+ C.resolve(promise).then(capability.resolve, reject);
+ });
+ });
+ if(abrupt)reject(abrupt.error);
+ return capability.promise;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.apply.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.apply.js
new file mode 100644
index 00000000..361a2e2e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.apply.js
@@ -0,0 +1,10 @@
+// 26.1.1 Reflect.apply(target, thisArgument, argumentsList)
+var $export = require('./$.export')
+ , _apply = Function.apply
+ , anObject = require('./$.an-object');
+
+$export($export.S, 'Reflect', {
+ apply: function apply(target, thisArgument, argumentsList){
+ return _apply.call(target, thisArgument, anObject(argumentsList));
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.construct.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.construct.js
new file mode 100644
index 00000000..c3928cd9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.construct.js
@@ -0,0 +1,39 @@
+// 26.1.2 Reflect.construct(target, argumentsList [, newTarget])
+var $ = require('./$')
+ , $export = require('./$.export')
+ , aFunction = require('./$.a-function')
+ , anObject = require('./$.an-object')
+ , isObject = require('./$.is-object')
+ , bind = Function.bind || require('./$.core').Function.prototype.bind;
+
+// MS Edge supports only 2 arguments
+// FF Nightly sets third argument as `new.target`, but does not create `this` from it
+$export($export.S + $export.F * require('./$.fails')(function(){
+ function F(){}
+ return !(Reflect.construct(function(){}, [], F) instanceof F);
+}), 'Reflect', {
+ construct: function construct(Target, args /*, newTarget*/){
+ aFunction(Target);
+ anObject(args);
+ var newTarget = arguments.length < 3 ? Target : aFunction(arguments[2]);
+ if(Target == newTarget){
+ // w/o altered newTarget, optimization for 0-4 arguments
+ switch(args.length){
+ case 0: return new Target;
+ case 1: return new Target(args[0]);
+ case 2: return new Target(args[0], args[1]);
+ case 3: return new Target(args[0], args[1], args[2]);
+ case 4: return new Target(args[0], args[1], args[2], args[3]);
+ }
+ // w/o altered newTarget, lot of arguments case
+ var $args = [null];
+ $args.push.apply($args, args);
+ return new (bind.apply(Target, $args));
+ }
+ // with altered newTarget, not support built-in constructors
+ var proto = newTarget.prototype
+ , instance = $.create(isObject(proto) ? proto : Object.prototype)
+ , result = Function.apply.call(Target, instance, args);
+ return isObject(result) ? result : instance;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.define-property.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.define-property.js
new file mode 100644
index 00000000..5f7fc6a1
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.define-property.js
@@ -0,0 +1,19 @@
+// 26.1.3 Reflect.defineProperty(target, propertyKey, attributes)
+var $ = require('./$')
+ , $export = require('./$.export')
+ , anObject = require('./$.an-object');
+
+// MS Edge has broken Reflect.defineProperty - throwing instead of returning false
+$export($export.S + $export.F * require('./$.fails')(function(){
+ Reflect.defineProperty($.setDesc({}, 1, {value: 1}), 1, {value: 2});
+}), 'Reflect', {
+ defineProperty: function defineProperty(target, propertyKey, attributes){
+ anObject(target);
+ try {
+ $.setDesc(target, propertyKey, attributes);
+ return true;
+ } catch(e){
+ return false;
+ }
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.delete-property.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.delete-property.js
new file mode 100644
index 00000000..18526e5b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.delete-property.js
@@ -0,0 +1,11 @@
+// 26.1.4 Reflect.deleteProperty(target, propertyKey)
+var $export = require('./$.export')
+ , getDesc = require('./$').getDesc
+ , anObject = require('./$.an-object');
+
+$export($export.S, 'Reflect', {
+ deleteProperty: function deleteProperty(target, propertyKey){
+ var desc = getDesc(anObject(target), propertyKey);
+ return desc && !desc.configurable ? false : delete target[propertyKey];
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.enumerate.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.enumerate.js
new file mode 100644
index 00000000..73452e2f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.enumerate.js
@@ -0,0 +1,26 @@
+'use strict';
+// 26.1.5 Reflect.enumerate(target)
+var $export = require('./$.export')
+ , anObject = require('./$.an-object');
+var Enumerate = function(iterated){
+ this._t = anObject(iterated); // target
+ this._i = 0; // next index
+ var keys = this._k = [] // keys
+ , key;
+ for(key in iterated)keys.push(key);
+};
+require('./$.iter-create')(Enumerate, 'Object', function(){
+ var that = this
+ , keys = that._k
+ , key;
+ do {
+ if(that._i >= keys.length)return {value: undefined, done: true};
+ } while(!((key = keys[that._i++]) in that._t));
+ return {value: key, done: false};
+});
+
+$export($export.S, 'Reflect', {
+ enumerate: function enumerate(target){
+ return new Enumerate(target);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.get-own-property-descriptor.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.get-own-property-descriptor.js
new file mode 100644
index 00000000..a3a2e016
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.get-own-property-descriptor.js
@@ -0,0 +1,10 @@
+// 26.1.7 Reflect.getOwnPropertyDescriptor(target, propertyKey)
+var $ = require('./$')
+ , $export = require('./$.export')
+ , anObject = require('./$.an-object');
+
+$export($export.S, 'Reflect', {
+ getOwnPropertyDescriptor: function getOwnPropertyDescriptor(target, propertyKey){
+ return $.getDesc(anObject(target), propertyKey);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.get-prototype-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.get-prototype-of.js
new file mode 100644
index 00000000..c06bfa45
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.get-prototype-of.js
@@ -0,0 +1,10 @@
+// 26.1.8 Reflect.getPrototypeOf(target)
+var $export = require('./$.export')
+ , getProto = require('./$').getProto
+ , anObject = require('./$.an-object');
+
+$export($export.S, 'Reflect', {
+ getPrototypeOf: function getPrototypeOf(target){
+ return getProto(anObject(target));
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.get.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.get.js
new file mode 100644
index 00000000..cbb0caaf
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.get.js
@@ -0,0 +1,20 @@
+// 26.1.6 Reflect.get(target, propertyKey [, receiver])
+var $ = require('./$')
+ , has = require('./$.has')
+ , $export = require('./$.export')
+ , isObject = require('./$.is-object')
+ , anObject = require('./$.an-object');
+
+function get(target, propertyKey/*, receiver*/){
+ var receiver = arguments.length < 3 ? target : arguments[2]
+ , desc, proto;
+ if(anObject(target) === receiver)return target[propertyKey];
+ if(desc = $.getDesc(target, propertyKey))return has(desc, 'value')
+ ? desc.value
+ : desc.get !== undefined
+ ? desc.get.call(receiver)
+ : undefined;
+ if(isObject(proto = $.getProto(target)))return get(proto, propertyKey, receiver);
+}
+
+$export($export.S, 'Reflect', {get: get});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.has.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.has.js
new file mode 100644
index 00000000..65c9e82f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.has.js
@@ -0,0 +1,8 @@
+// 26.1.9 Reflect.has(target, propertyKey)
+var $export = require('./$.export');
+
+$export($export.S, 'Reflect', {
+ has: function has(target, propertyKey){
+ return propertyKey in target;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.is-extensible.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.is-extensible.js
new file mode 100644
index 00000000..b92c4f66
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.is-extensible.js
@@ -0,0 +1,11 @@
+// 26.1.10 Reflect.isExtensible(target)
+var $export = require('./$.export')
+ , anObject = require('./$.an-object')
+ , $isExtensible = Object.isExtensible;
+
+$export($export.S, 'Reflect', {
+ isExtensible: function isExtensible(target){
+ anObject(target);
+ return $isExtensible ? $isExtensible(target) : true;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.own-keys.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.own-keys.js
new file mode 100644
index 00000000..db79fdab
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.own-keys.js
@@ -0,0 +1,4 @@
+// 26.1.11 Reflect.ownKeys(target)
+var $export = require('./$.export');
+
+$export($export.S, 'Reflect', {ownKeys: require('./$.own-keys')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.prevent-extensions.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.prevent-extensions.js
new file mode 100644
index 00000000..f5ccfc2a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.prevent-extensions.js
@@ -0,0 +1,16 @@
+// 26.1.12 Reflect.preventExtensions(target)
+var $export = require('./$.export')
+ , anObject = require('./$.an-object')
+ , $preventExtensions = Object.preventExtensions;
+
+$export($export.S, 'Reflect', {
+ preventExtensions: function preventExtensions(target){
+ anObject(target);
+ try {
+ if($preventExtensions)$preventExtensions(target);
+ return true;
+ } catch(e){
+ return false;
+ }
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.set-prototype-of.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.set-prototype-of.js
new file mode 100644
index 00000000..e769436f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.set-prototype-of.js
@@ -0,0 +1,15 @@
+// 26.1.14 Reflect.setPrototypeOf(target, proto)
+var $export = require('./$.export')
+ , setProto = require('./$.set-proto');
+
+if(setProto)$export($export.S, 'Reflect', {
+ setPrototypeOf: function setPrototypeOf(target, proto){
+ setProto.check(target, proto);
+ try {
+ setProto.set(target, proto);
+ return true;
+ } catch(e){
+ return false;
+ }
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.set.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.set.js
new file mode 100644
index 00000000..0a938e78
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.reflect.set.js
@@ -0,0 +1,29 @@
+// 26.1.13 Reflect.set(target, propertyKey, V [, receiver])
+var $ = require('./$')
+ , has = require('./$.has')
+ , $export = require('./$.export')
+ , createDesc = require('./$.property-desc')
+ , anObject = require('./$.an-object')
+ , isObject = require('./$.is-object');
+
+function set(target, propertyKey, V/*, receiver*/){
+ var receiver = arguments.length < 4 ? target : arguments[3]
+ , ownDesc = $.getDesc(anObject(target), propertyKey)
+ , existingDescriptor, proto;
+ if(!ownDesc){
+ if(isObject(proto = $.getProto(target))){
+ return set(proto, propertyKey, V, receiver);
+ }
+ ownDesc = createDesc(0);
+ }
+ if(has(ownDesc, 'value')){
+ if(ownDesc.writable === false || !isObject(receiver))return false;
+ existingDescriptor = $.getDesc(receiver, propertyKey) || createDesc(0);
+ existingDescriptor.value = V;
+ $.setDesc(receiver, propertyKey, existingDescriptor);
+ return true;
+ }
+ return ownDesc.set === undefined ? false : (ownDesc.set.call(receiver, V), true);
+}
+
+$export($export.S, 'Reflect', {set: set});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.regexp.constructor.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.regexp.constructor.js
new file mode 100644
index 00000000..c7ef61c2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.regexp.constructor.js
@@ -0,0 +1,38 @@
+var $ = require('./$')
+ , global = require('./$.global')
+ , isRegExp = require('./$.is-regexp')
+ , $flags = require('./$.flags')
+ , $RegExp = global.RegExp
+ , Base = $RegExp
+ , proto = $RegExp.prototype
+ , re1 = /a/g
+ , re2 = /a/g
+ // "new" creates a new object, old webkit buggy here
+ , CORRECT_NEW = new $RegExp(re1) !== re1;
+
+if(require('./$.descriptors') && (!CORRECT_NEW || require('./$.fails')(function(){
+ re2[require('./$.wks')('match')] = false;
+ // RegExp constructor can alter flags and IsRegExp works correct with @@match
+ return $RegExp(re1) != re1 || $RegExp(re2) == re2 || $RegExp(re1, 'i') != '/a/i';
+}))){
+ $RegExp = function RegExp(p, f){
+ var piRE = isRegExp(p)
+ , fiU = f === undefined;
+ return !(this instanceof $RegExp) && piRE && p.constructor === $RegExp && fiU ? p
+ : CORRECT_NEW
+ ? new Base(piRE && !fiU ? p.source : p, f)
+ : Base((piRE = p instanceof $RegExp) ? p.source : p, piRE && fiU ? $flags.call(p) : f);
+ };
+ $.each.call($.getNames(Base), function(key){
+ key in $RegExp || $.setDesc($RegExp, key, {
+ configurable: true,
+ get: function(){ return Base[key]; },
+ set: function(it){ Base[key] = it; }
+ });
+ });
+ proto.constructor = $RegExp;
+ $RegExp.prototype = proto;
+ require('./$.redefine')(global, 'RegExp', $RegExp);
+}
+
+require('./$.set-species')('RegExp');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.regexp.flags.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.regexp.flags.js
new file mode 100644
index 00000000..5984c214
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.regexp.flags.js
@@ -0,0 +1,6 @@
+// 21.2.5.3 get RegExp.prototype.flags()
+var $ = require('./$');
+if(require('./$.descriptors') && /./g.flags != 'g')$.setDesc(RegExp.prototype, 'flags', {
+ configurable: true,
+ get: require('./$.flags')
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.regexp.match.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.regexp.match.js
new file mode 100644
index 00000000..5cd194c2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.regexp.match.js
@@ -0,0 +1,10 @@
+// @@match logic
+require('./$.fix-re-wks')('match', 1, function(defined, MATCH){
+ // 21.1.3.11 String.prototype.match(regexp)
+ return function match(regexp){
+ 'use strict';
+ var O = defined(this)
+ , fn = regexp == undefined ? undefined : regexp[MATCH];
+ return fn !== undefined ? fn.call(regexp, O) : new RegExp(regexp)[MATCH](String(O));
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.regexp.replace.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.regexp.replace.js
new file mode 100644
index 00000000..140c5072
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.regexp.replace.js
@@ -0,0 +1,12 @@
+// @@replace logic
+require('./$.fix-re-wks')('replace', 2, function(defined, REPLACE, $replace){
+ // 21.1.3.14 String.prototype.replace(searchValue, replaceValue)
+ return function replace(searchValue, replaceValue){
+ 'use strict';
+ var O = defined(this)
+ , fn = searchValue == undefined ? undefined : searchValue[REPLACE];
+ return fn !== undefined
+ ? fn.call(searchValue, O, replaceValue)
+ : $replace.call(String(O), searchValue, replaceValue);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.regexp.search.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.regexp.search.js
new file mode 100644
index 00000000..adfd5c9c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.regexp.search.js
@@ -0,0 +1,10 @@
+// @@search logic
+require('./$.fix-re-wks')('search', 1, function(defined, SEARCH){
+ // 21.1.3.15 String.prototype.search(regexp)
+ return function search(regexp){
+ 'use strict';
+ var O = defined(this)
+ , fn = regexp == undefined ? undefined : regexp[SEARCH];
+ return fn !== undefined ? fn.call(regexp, O) : new RegExp(regexp)[SEARCH](String(O));
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.regexp.split.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.regexp.split.js
new file mode 100644
index 00000000..0607fb06
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.regexp.split.js
@@ -0,0 +1,12 @@
+// @@split logic
+require('./$.fix-re-wks')('split', 2, function(defined, SPLIT, $split){
+ // 21.1.3.17 String.prototype.split(separator, limit)
+ return function split(separator, limit){
+ 'use strict';
+ var O = defined(this)
+ , fn = separator == undefined ? undefined : separator[SPLIT];
+ return fn !== undefined
+ ? fn.call(separator, O, limit)
+ : $split.call(String(O), separator, limit);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.set.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.set.js
new file mode 100644
index 00000000..8e148c9e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.set.js
@@ -0,0 +1,12 @@
+'use strict';
+var strong = require('./$.collection-strong');
+
+// 23.2 Set Objects
+require('./$.collection')('Set', function(get){
+ return function Set(){ return get(this, arguments.length > 0 ? arguments[0] : undefined); };
+}, {
+ // 23.2.3.1 Set.prototype.add(value)
+ add: function add(value){
+ return strong.def(this, value = value === 0 ? 0 : value, value);
+ }
+}, strong);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.code-point-at.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.code-point-at.js
new file mode 100644
index 00000000..ebac5518
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.code-point-at.js
@@ -0,0 +1,9 @@
+'use strict';
+var $export = require('./$.export')
+ , $at = require('./$.string-at')(false);
+$export($export.P, 'String', {
+ // 21.1.3.3 String.prototype.codePointAt(pos)
+ codePointAt: function codePointAt(pos){
+ return $at(this, pos);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.ends-with.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.ends-with.js
new file mode 100644
index 00000000..a102da2d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.ends-with.js
@@ -0,0 +1,21 @@
+// 21.1.3.6 String.prototype.endsWith(searchString [, endPosition])
+'use strict';
+var $export = require('./$.export')
+ , toLength = require('./$.to-length')
+ , context = require('./$.string-context')
+ , ENDS_WITH = 'endsWith'
+ , $endsWith = ''[ENDS_WITH];
+
+$export($export.P + $export.F * require('./$.fails-is-regexp')(ENDS_WITH), 'String', {
+ endsWith: function endsWith(searchString /*, endPosition = @length */){
+ var that = context(this, searchString, ENDS_WITH)
+ , $$ = arguments
+ , endPosition = $$.length > 1 ? $$[1] : undefined
+ , len = toLength(that.length)
+ , end = endPosition === undefined ? len : Math.min(toLength(endPosition), len)
+ , search = String(searchString);
+ return $endsWith
+ ? $endsWith.call(that, search, end)
+ : that.slice(end - search.length, end) === search;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.from-code-point.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.from-code-point.js
new file mode 100644
index 00000000..b0bd166b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.from-code-point.js
@@ -0,0 +1,24 @@
+var $export = require('./$.export')
+ , toIndex = require('./$.to-index')
+ , fromCharCode = String.fromCharCode
+ , $fromCodePoint = String.fromCodePoint;
+
+// length should be 1, old FF problem
+$export($export.S + $export.F * (!!$fromCodePoint && $fromCodePoint.length != 1), 'String', {
+ // 21.1.2.2 String.fromCodePoint(...codePoints)
+ fromCodePoint: function fromCodePoint(x){ // eslint-disable-line no-unused-vars
+ var res = []
+ , $$ = arguments
+ , $$len = $$.length
+ , i = 0
+ , code;
+ while($$len > i){
+ code = +$$[i++];
+ if(toIndex(code, 0x10ffff) !== code)throw RangeError(code + ' is not a valid code point');
+ res.push(code < 0x10000
+ ? fromCharCode(code)
+ : fromCharCode(((code -= 0x10000) >> 10) + 0xd800, code % 0x400 + 0xdc00)
+ );
+ } return res.join('');
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.includes.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.includes.js
new file mode 100644
index 00000000..e2ab8db7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.includes.js
@@ -0,0 +1,12 @@
+// 21.1.3.7 String.prototype.includes(searchString, position = 0)
+'use strict';
+var $export = require('./$.export')
+ , context = require('./$.string-context')
+ , INCLUDES = 'includes';
+
+$export($export.P + $export.F * require('./$.fails-is-regexp')(INCLUDES), 'String', {
+ includes: function includes(searchString /*, position = 0 */){
+ return !!~context(this, searchString, INCLUDES)
+ .indexOf(searchString, arguments.length > 1 ? arguments[1] : undefined);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.iterator.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.iterator.js
new file mode 100644
index 00000000..2f4c772c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.iterator.js
@@ -0,0 +1,17 @@
+'use strict';
+var $at = require('./$.string-at')(true);
+
+// 21.1.3.27 String.prototype[@@iterator]()
+require('./$.iter-define')(String, 'String', function(iterated){
+ this._t = String(iterated); // target
+ this._i = 0; // next index
+// 21.1.5.2.1 %StringIteratorPrototype%.next()
+}, function(){
+ var O = this._t
+ , index = this._i
+ , point;
+ if(index >= O.length)return {value: undefined, done: true};
+ point = $at(O, index);
+ this._i += point.length;
+ return {value: point, done: false};
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.raw.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.raw.js
new file mode 100644
index 00000000..64279d23
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.raw.js
@@ -0,0 +1,19 @@
+var $export = require('./$.export')
+ , toIObject = require('./$.to-iobject')
+ , toLength = require('./$.to-length');
+
+$export($export.S, 'String', {
+ // 21.1.2.4 String.raw(callSite, ...substitutions)
+ raw: function raw(callSite){
+ var tpl = toIObject(callSite.raw)
+ , len = toLength(tpl.length)
+ , $$ = arguments
+ , $$len = $$.length
+ , res = []
+ , i = 0;
+ while(len > i){
+ res.push(String(tpl[i++]));
+ if(i < $$len)res.push(String($$[i]));
+ } return res.join('');
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.repeat.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.repeat.js
new file mode 100644
index 00000000..4ec29f66
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.repeat.js
@@ -0,0 +1,6 @@
+var $export = require('./$.export');
+
+$export($export.P, 'String', {
+ // 21.1.3.13 String.prototype.repeat(count)
+ repeat: require('./$.string-repeat')
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.starts-with.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.starts-with.js
new file mode 100644
index 00000000..21143072
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.starts-with.js
@@ -0,0 +1,19 @@
+// 21.1.3.18 String.prototype.startsWith(searchString [, position ])
+'use strict';
+var $export = require('./$.export')
+ , toLength = require('./$.to-length')
+ , context = require('./$.string-context')
+ , STARTS_WITH = 'startsWith'
+ , $startsWith = ''[STARTS_WITH];
+
+$export($export.P + $export.F * require('./$.fails-is-regexp')(STARTS_WITH), 'String', {
+ startsWith: function startsWith(searchString /*, position = 0 */){
+ var that = context(this, searchString, STARTS_WITH)
+ , $$ = arguments
+ , index = toLength(Math.min($$.length > 1 ? $$[1] : undefined, that.length))
+ , search = String(searchString);
+ return $startsWith
+ ? $startsWith.call(that, search, index)
+ : that.slice(index, index + search.length) === search;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.trim.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.trim.js
new file mode 100644
index 00000000..52b75cac
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.string.trim.js
@@ -0,0 +1,7 @@
+'use strict';
+// 21.1.3.25 String.prototype.trim()
+require('./$.string-trim')('trim', function($trim){
+ return function trim(){
+ return $trim(this, 3);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.symbol.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.symbol.js
new file mode 100644
index 00000000..42b7a3aa
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.symbol.js
@@ -0,0 +1,227 @@
+'use strict';
+// ECMAScript 6 symbols shim
+var $ = require('./$')
+ , global = require('./$.global')
+ , has = require('./$.has')
+ , DESCRIPTORS = require('./$.descriptors')
+ , $export = require('./$.export')
+ , redefine = require('./$.redefine')
+ , $fails = require('./$.fails')
+ , shared = require('./$.shared')
+ , setToStringTag = require('./$.set-to-string-tag')
+ , uid = require('./$.uid')
+ , wks = require('./$.wks')
+ , keyOf = require('./$.keyof')
+ , $names = require('./$.get-names')
+ , enumKeys = require('./$.enum-keys')
+ , isArray = require('./$.is-array')
+ , anObject = require('./$.an-object')
+ , toIObject = require('./$.to-iobject')
+ , createDesc = require('./$.property-desc')
+ , getDesc = $.getDesc
+ , setDesc = $.setDesc
+ , _create = $.create
+ , getNames = $names.get
+ , $Symbol = global.Symbol
+ , $JSON = global.JSON
+ , _stringify = $JSON && $JSON.stringify
+ , setter = false
+ , HIDDEN = wks('_hidden')
+ , isEnum = $.isEnum
+ , SymbolRegistry = shared('symbol-registry')
+ , AllSymbols = shared('symbols')
+ , useNative = typeof $Symbol == 'function'
+ , ObjectProto = Object.prototype;
+
+// fallback for old Android, https://code.google.com/p/v8/issues/detail?id=687
+var setSymbolDesc = DESCRIPTORS && $fails(function(){
+ return _create(setDesc({}, 'a', {
+ get: function(){ return setDesc(this, 'a', {value: 7}).a; }
+ })).a != 7;
+}) ? function(it, key, D){
+ var protoDesc = getDesc(ObjectProto, key);
+ if(protoDesc)delete ObjectProto[key];
+ setDesc(it, key, D);
+ if(protoDesc && it !== ObjectProto)setDesc(ObjectProto, key, protoDesc);
+} : setDesc;
+
+var wrap = function(tag){
+ var sym = AllSymbols[tag] = _create($Symbol.prototype);
+ sym._k = tag;
+ DESCRIPTORS && setter && setSymbolDesc(ObjectProto, tag, {
+ configurable: true,
+ set: function(value){
+ if(has(this, HIDDEN) && has(this[HIDDEN], tag))this[HIDDEN][tag] = false;
+ setSymbolDesc(this, tag, createDesc(1, value));
+ }
+ });
+ return sym;
+};
+
+var isSymbol = function(it){
+ return typeof it == 'symbol';
+};
+
+var $defineProperty = function defineProperty(it, key, D){
+ if(D && has(AllSymbols, key)){
+ if(!D.enumerable){
+ if(!has(it, HIDDEN))setDesc(it, HIDDEN, createDesc(1, {}));
+ it[HIDDEN][key] = true;
+ } else {
+ if(has(it, HIDDEN) && it[HIDDEN][key])it[HIDDEN][key] = false;
+ D = _create(D, {enumerable: createDesc(0, false)});
+ } return setSymbolDesc(it, key, D);
+ } return setDesc(it, key, D);
+};
+var $defineProperties = function defineProperties(it, P){
+ anObject(it);
+ var keys = enumKeys(P = toIObject(P))
+ , i = 0
+ , l = keys.length
+ , key;
+ while(l > i)$defineProperty(it, key = keys[i++], P[key]);
+ return it;
+};
+var $create = function create(it, P){
+ return P === undefined ? _create(it) : $defineProperties(_create(it), P);
+};
+var $propertyIsEnumerable = function propertyIsEnumerable(key){
+ var E = isEnum.call(this, key);
+ return E || !has(this, key) || !has(AllSymbols, key) || has(this, HIDDEN) && this[HIDDEN][key]
+ ? E : true;
+};
+var $getOwnPropertyDescriptor = function getOwnPropertyDescriptor(it, key){
+ var D = getDesc(it = toIObject(it), key);
+ if(D && has(AllSymbols, key) && !(has(it, HIDDEN) && it[HIDDEN][key]))D.enumerable = true;
+ return D;
+};
+var $getOwnPropertyNames = function getOwnPropertyNames(it){
+ var names = getNames(toIObject(it))
+ , result = []
+ , i = 0
+ , key;
+ while(names.length > i)if(!has(AllSymbols, key = names[i++]) && key != HIDDEN)result.push(key);
+ return result;
+};
+var $getOwnPropertySymbols = function getOwnPropertySymbols(it){
+ var names = getNames(toIObject(it))
+ , result = []
+ , i = 0
+ , key;
+ while(names.length > i)if(has(AllSymbols, key = names[i++]))result.push(AllSymbols[key]);
+ return result;
+};
+var $stringify = function stringify(it){
+ if(it === undefined || isSymbol(it))return; // IE8 returns string on undefined
+ var args = [it]
+ , i = 1
+ , $$ = arguments
+ , replacer, $replacer;
+ while($$.length > i)args.push($$[i++]);
+ replacer = args[1];
+ if(typeof replacer == 'function')$replacer = replacer;
+ if($replacer || !isArray(replacer))replacer = function(key, value){
+ if($replacer)value = $replacer.call(this, key, value);
+ if(!isSymbol(value))return value;
+ };
+ args[1] = replacer;
+ return _stringify.apply($JSON, args);
+};
+var buggyJSON = $fails(function(){
+ var S = $Symbol();
+ // MS Edge converts symbol values to JSON as {}
+ // WebKit converts symbol values to JSON as null
+ // V8 throws on boxed symbols
+ return _stringify([S]) != '[null]' || _stringify({a: S}) != '{}' || _stringify(Object(S)) != '{}';
+});
+
+// 19.4.1.1 Symbol([description])
+if(!useNative){
+ $Symbol = function Symbol(){
+ if(isSymbol(this))throw TypeError('Symbol is not a constructor');
+ return wrap(uid(arguments.length > 0 ? arguments[0] : undefined));
+ };
+ redefine($Symbol.prototype, 'toString', function toString(){
+ return this._k;
+ });
+
+ isSymbol = function(it){
+ return it instanceof $Symbol;
+ };
+
+ $.create = $create;
+ $.isEnum = $propertyIsEnumerable;
+ $.getDesc = $getOwnPropertyDescriptor;
+ $.setDesc = $defineProperty;
+ $.setDescs = $defineProperties;
+ $.getNames = $names.get = $getOwnPropertyNames;
+ $.getSymbols = $getOwnPropertySymbols;
+
+ if(DESCRIPTORS && !require('./$.library')){
+ redefine(ObjectProto, 'propertyIsEnumerable', $propertyIsEnumerable, true);
+ }
+}
+
+var symbolStatics = {
+ // 19.4.2.1 Symbol.for(key)
+ 'for': function(key){
+ return has(SymbolRegistry, key += '')
+ ? SymbolRegistry[key]
+ : SymbolRegistry[key] = $Symbol(key);
+ },
+ // 19.4.2.5 Symbol.keyFor(sym)
+ keyFor: function keyFor(key){
+ return keyOf(SymbolRegistry, key);
+ },
+ useSetter: function(){ setter = true; },
+ useSimple: function(){ setter = false; }
+};
+// 19.4.2.2 Symbol.hasInstance
+// 19.4.2.3 Symbol.isConcatSpreadable
+// 19.4.2.4 Symbol.iterator
+// 19.4.2.6 Symbol.match
+// 19.4.2.8 Symbol.replace
+// 19.4.2.9 Symbol.search
+// 19.4.2.10 Symbol.species
+// 19.4.2.11 Symbol.split
+// 19.4.2.12 Symbol.toPrimitive
+// 19.4.2.13 Symbol.toStringTag
+// 19.4.2.14 Symbol.unscopables
+$.each.call((
+ 'hasInstance,isConcatSpreadable,iterator,match,replace,search,' +
+ 'species,split,toPrimitive,toStringTag,unscopables'
+).split(','), function(it){
+ var sym = wks(it);
+ symbolStatics[it] = useNative ? sym : wrap(sym);
+});
+
+setter = true;
+
+$export($export.G + $export.W, {Symbol: $Symbol});
+
+$export($export.S, 'Symbol', symbolStatics);
+
+$export($export.S + $export.F * !useNative, 'Object', {
+ // 19.1.2.2 Object.create(O [, Properties])
+ create: $create,
+ // 19.1.2.4 Object.defineProperty(O, P, Attributes)
+ defineProperty: $defineProperty,
+ // 19.1.2.3 Object.defineProperties(O, Properties)
+ defineProperties: $defineProperties,
+ // 19.1.2.6 Object.getOwnPropertyDescriptor(O, P)
+ getOwnPropertyDescriptor: $getOwnPropertyDescriptor,
+ // 19.1.2.7 Object.getOwnPropertyNames(O)
+ getOwnPropertyNames: $getOwnPropertyNames,
+ // 19.1.2.8 Object.getOwnPropertySymbols(O)
+ getOwnPropertySymbols: $getOwnPropertySymbols
+});
+
+// 24.3.2 JSON.stringify(value [, replacer [, space]])
+$JSON && $export($export.S + $export.F * (!useNative || buggyJSON), 'JSON', {stringify: $stringify});
+
+// 19.4.3.5 Symbol.prototype[@@toStringTag]
+setToStringTag($Symbol, 'Symbol');
+// 20.2.1.9 Math[@@toStringTag]
+setToStringTag(Math, 'Math', true);
+// 24.3.3 JSON[@@toStringTag]
+setToStringTag(global.JSON, 'JSON', true);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.array-buffer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.array-buffer.js
new file mode 100644
index 00000000..a8209bd2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.array-buffer.js
@@ -0,0 +1,43 @@
+'use strict';
+if(require('./$.descriptors')){
+ var $export = require('./$.export')
+ , $typed = require('./$.typed')
+ , buffer = require('./$.buffer')
+ , toIndex = require('./$.to-index')
+ , toLength = require('./$.to-length')
+ , isObject = require('./$.is-object')
+ , TYPED_ARRAY = require('./$.wks')('typed_array')
+ , $ArrayBuffer = buffer.ArrayBuffer
+ , $DataView = buffer.DataView
+ , $slice = $ArrayBuffer && $ArrayBuffer.prototype.slice
+ , VIEW = $typed.VIEW
+ , ARRAY_BUFFER = 'ArrayBuffer';
+
+ $export($export.G + $export.W + $export.F * !$typed.ABV, {ArrayBuffer: $ArrayBuffer});
+
+ $export($export.S + $export.F * !$typed.CONSTR, ARRAY_BUFFER, {
+ // 24.1.3.1 ArrayBuffer.isView(arg)
+ isView: function isView(it){ // not cross-realm
+ return isObject(it) && VIEW in it;
+ }
+ });
+
+ $export($export.P + $export.F * require('./$.fails')(function(){
+ return !new $ArrayBuffer(2).slice(1, undefined).byteLength;
+ }), ARRAY_BUFFER, {
+ // 24.1.4.3 ArrayBuffer.prototype.slice(start, end)
+ slice: function slice(start, end){
+ if($slice !== undefined && end === undefined)return $slice.call(this, start); // FF fix
+ var len = this.byteLength
+ , first = toIndex(start, len)
+ , final = toIndex(end === undefined ? len : end, len)
+ , result = new $ArrayBuffer(toLength(final - first))
+ , viewS = new $DataView(this)
+ , viewT = new $DataView(result)
+ , index = 0;
+ while(first < final){
+ viewT.setUint8(index++, viewS.getUint8(first++));
+ } return result;
+ }
+ });
+}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.data-view.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.data-view.js
new file mode 100644
index 00000000..44e03532
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.data-view.js
@@ -0,0 +1,4 @@
+if(require('./$.descriptors')){
+ var $export = require('./$.export');
+ $export($export.G + $export.W + $export.F * !require('./$.typed').ABV, {DataView: require('./$.buffer').DataView});
+}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.float32-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.float32-array.js
new file mode 100644
index 00000000..95d78a6d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.float32-array.js
@@ -0,0 +1,5 @@
+require('./$.typed-array')('Float32', 4, function(init){
+ return function Float32Array(data, byteOffset, length){
+ return init(this, data, byteOffset, length);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.float64-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.float64-array.js
new file mode 100644
index 00000000..16fadec9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.float64-array.js
@@ -0,0 +1,5 @@
+require('./$.typed-array')('Float64', 8, function(init){
+ return function Float64Array(data, byteOffset, length){
+ return init(this, data, byteOffset, length);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.int16-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.int16-array.js
new file mode 100644
index 00000000..a3d04cb6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.int16-array.js
@@ -0,0 +1,5 @@
+require('./$.typed-array')('Int16', 2, function(init){
+ return function Int16Array(data, byteOffset, length){
+ return init(this, data, byteOffset, length);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.int32-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.int32-array.js
new file mode 100644
index 00000000..1923463a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.int32-array.js
@@ -0,0 +1,5 @@
+require('./$.typed-array')('Int32', 4, function(init){
+ return function Int32Array(data, byteOffset, length){
+ return init(this, data, byteOffset, length);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.int8-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.int8-array.js
new file mode 100644
index 00000000..e9182c4c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.int8-array.js
@@ -0,0 +1,5 @@
+require('./$.typed-array')('Int8', 1, function(init){
+ return function Int8Array(data, byteOffset, length){
+ return init(this, data, byteOffset, length);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.uint16-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.uint16-array.js
new file mode 100644
index 00000000..ec6e8347
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.uint16-array.js
@@ -0,0 +1,5 @@
+require('./$.typed-array')('Uint16', 2, function(init){
+ return function Uint16Array(data, byteOffset, length){
+ return init(this, data, byteOffset, length);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.uint32-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.uint32-array.js
new file mode 100644
index 00000000..ddfc22d8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.uint32-array.js
@@ -0,0 +1,5 @@
+require('./$.typed-array')('Uint32', 4, function(init){
+ return function Uint32Array(data, byteOffset, length){
+ return init(this, data, byteOffset, length);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.uint8-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.uint8-array.js
new file mode 100644
index 00000000..7ab1e4df
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.uint8-array.js
@@ -0,0 +1,5 @@
+require('./$.typed-array')('Uint8', 1, function(init){
+ return function Uint8Array(data, byteOffset, length){
+ return init(this, data, byteOffset, length);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.uint8-clamped-array.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.uint8-clamped-array.js
new file mode 100644
index 00000000..f85f9d5d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.typed.uint8-clamped-array.js
@@ -0,0 +1,5 @@
+require('./$.typed-array')('Uint8', 1, function(init){
+ return function Uint8ClampedArray(data, byteOffset, length){
+ return init(this, data, byteOffset, length);
+ };
+}, true);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.weak-map.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.weak-map.js
new file mode 100644
index 00000000..72a9b324
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.weak-map.js
@@ -0,0 +1,43 @@
+'use strict';
+var $ = require('./$')
+ , redefine = require('./$.redefine')
+ , weak = require('./$.collection-weak')
+ , isObject = require('./$.is-object')
+ , has = require('./$.has')
+ , frozenStore = weak.frozenStore
+ , WEAK = weak.WEAK
+ , isExtensible = Object.isExtensible || isObject
+ , tmp = {};
+
+// 23.3 WeakMap Objects
+var $WeakMap = require('./$.collection')('WeakMap', function(get){
+ return function WeakMap(){ return get(this, arguments.length > 0 ? arguments[0] : undefined); };
+}, {
+ // 23.3.3.3 WeakMap.prototype.get(key)
+ get: function get(key){
+ if(isObject(key)){
+ if(!isExtensible(key))return frozenStore(this).get(key);
+ if(has(key, WEAK))return key[WEAK][this._i];
+ }
+ },
+ // 23.3.3.5 WeakMap.prototype.set(key, value)
+ set: function set(key, value){
+ return weak.def(this, key, value);
+ }
+}, weak, true, true);
+
+// IE11 WeakMap frozen keys fix
+if(new $WeakMap().set((Object.freeze || Object)(tmp), 7).get(tmp) != 7){
+ $.each.call(['delete', 'has', 'get', 'set'], function(key){
+ var proto = $WeakMap.prototype
+ , method = proto[key];
+ redefine(proto, key, function(a, b){
+ // store frozen objects on leaky map
+ if(isObject(a) && !isExtensible(a)){
+ var result = frozenStore(this)[key](a, b);
+ return key == 'set' ? this : result;
+ // store all the rest on native weakmap
+ } return method.call(this, a, b);
+ });
+ });
+}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.weak-set.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.weak-set.js
new file mode 100644
index 00000000..efdf1d76
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es6.weak-set.js
@@ -0,0 +1,12 @@
+'use strict';
+var weak = require('./$.collection-weak');
+
+// 23.4 WeakSet Objects
+require('./$.collection')('WeakSet', function(get){
+ return function WeakSet(){ return get(this, arguments.length > 0 ? arguments[0] : undefined); };
+}, {
+ // 23.4.3.1 WeakSet.prototype.add(value)
+ add: function add(value){
+ return weak.def(this, value, true);
+ }
+}, weak, false, true);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.array.includes.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.array.includes.js
new file mode 100644
index 00000000..dcfad704
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.array.includes.js
@@ -0,0 +1,12 @@
+'use strict';
+var $export = require('./$.export')
+ , $includes = require('./$.array-includes')(true);
+
+$export($export.P, 'Array', {
+ // https://github.com/domenic/Array.prototype.includes
+ includes: function includes(el /*, fromIndex = 0 */){
+ return $includes(this, el, arguments.length > 1 ? arguments[1] : undefined);
+ }
+});
+
+require('./$.add-to-unscopables')('includes');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.map.to-json.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.map.to-json.js
new file mode 100644
index 00000000..80937056
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.map.to-json.js
@@ -0,0 +1,4 @@
+// https://github.com/DavidBruant/Map-Set.prototype.toJSON
+var $export = require('./$.export');
+
+$export($export.P, 'Map', {toJSON: require('./$.collection-to-json')('Map')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.object.entries.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.object.entries.js
new file mode 100644
index 00000000..fec1bc36
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.object.entries.js
@@ -0,0 +1,9 @@
+// http://goo.gl/XkBrjD
+var $export = require('./$.export')
+ , $entries = require('./$.object-to-array')(true);
+
+$export($export.S, 'Object', {
+ entries: function entries(it){
+ return $entries(it);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.object.get-own-property-descriptors.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.object.get-own-property-descriptors.js
new file mode 100644
index 00000000..e4d80a34
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.object.get-own-property-descriptors.js
@@ -0,0 +1,23 @@
+// https://gist.github.com/WebReflection/9353781
+var $ = require('./$')
+ , $export = require('./$.export')
+ , ownKeys = require('./$.own-keys')
+ , toIObject = require('./$.to-iobject')
+ , createDesc = require('./$.property-desc');
+
+$export($export.S, 'Object', {
+ getOwnPropertyDescriptors: function getOwnPropertyDescriptors(object){
+ var O = toIObject(object)
+ , setDesc = $.setDesc
+ , getDesc = $.getDesc
+ , keys = ownKeys(O)
+ , result = {}
+ , i = 0
+ , key, D;
+ while(keys.length > i){
+ D = getDesc(O, key = keys[i++]);
+ if(key in result)setDesc(result, key, createDesc(0, D));
+ else result[key] = D;
+ } return result;
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.object.values.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.object.values.js
new file mode 100644
index 00000000..697e9354
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.object.values.js
@@ -0,0 +1,9 @@
+// http://goo.gl/XkBrjD
+var $export = require('./$.export')
+ , $values = require('./$.object-to-array')(false);
+
+$export($export.S, 'Object', {
+ values: function values(it){
+ return $values(it);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.regexp.escape.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.regexp.escape.js
new file mode 100644
index 00000000..9c4c542a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.regexp.escape.js
@@ -0,0 +1,5 @@
+// https://github.com/benjamingr/RexExp.escape
+var $export = require('./$.export')
+ , $re = require('./$.replacer')(/[\\^$*+?.()|[\]{}]/g, '\\$&');
+
+$export($export.S, 'RegExp', {escape: function escape(it){ return $re(it); }});
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.set.to-json.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.set.to-json.js
new file mode 100644
index 00000000..e632f2a3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.set.to-json.js
@@ -0,0 +1,4 @@
+// https://github.com/DavidBruant/Map-Set.prototype.toJSON
+var $export = require('./$.export');
+
+$export($export.P, 'Set', {toJSON: require('./$.collection-to-json')('Set')});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.string.at.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.string.at.js
new file mode 100644
index 00000000..fee583bf
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.string.at.js
@@ -0,0 +1,10 @@
+'use strict';
+// https://github.com/mathiasbynens/String.prototype.at
+var $export = require('./$.export')
+ , $at = require('./$.string-at')(true);
+
+$export($export.P, 'String', {
+ at: function at(pos){
+ return $at(this, pos);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.string.pad-left.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.string.pad-left.js
new file mode 100644
index 00000000..643621aa
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.string.pad-left.js
@@ -0,0 +1,9 @@
+'use strict';
+var $export = require('./$.export')
+ , $pad = require('./$.string-pad');
+
+$export($export.P, 'String', {
+ padLeft: function padLeft(maxLength /*, fillString = ' ' */){
+ return $pad(this, maxLength, arguments.length > 1 ? arguments[1] : undefined, true);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.string.pad-right.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.string.pad-right.js
new file mode 100644
index 00000000..e4230960
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.string.pad-right.js
@@ -0,0 +1,9 @@
+'use strict';
+var $export = require('./$.export')
+ , $pad = require('./$.string-pad');
+
+$export($export.P, 'String', {
+ padRight: function padRight(maxLength /*, fillString = ' ' */){
+ return $pad(this, maxLength, arguments.length > 1 ? arguments[1] : undefined, false);
+ }
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.string.trim-left.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.string.trim-left.js
new file mode 100644
index 00000000..dbaf6308
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.string.trim-left.js
@@ -0,0 +1,7 @@
+'use strict';
+// https://github.com/sebmarkbage/ecmascript-string-left-right-trim
+require('./$.string-trim')('trimLeft', function($trim){
+ return function trimLeft(){
+ return $trim(this, 1);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.string.trim-right.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.string.trim-right.js
new file mode 100644
index 00000000..6b02d394
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/es7.string.trim-right.js
@@ -0,0 +1,7 @@
+'use strict';
+// https://github.com/sebmarkbage/ecmascript-string-left-right-trim
+require('./$.string-trim')('trimRight', function($trim){
+ return function trimRight(){
+ return $trim(this, 2);
+ };
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/js.array.statics.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/js.array.statics.js
new file mode 100644
index 00000000..9536c2e4
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/js.array.statics.js
@@ -0,0 +1,17 @@
+// JavaScript 1.6 / Strawman array statics shim
+var $ = require('./$')
+ , $export = require('./$.export')
+ , $ctx = require('./$.ctx')
+ , $Array = require('./$.core').Array || Array
+ , statics = {};
+var setStatics = function(keys, length){
+ $.each.call(keys.split(','), function(key){
+ if(length == undefined && key in $Array)statics[key] = $Array[key];
+ else if(key in [])statics[key] = $ctx(Function.call, [][key], length);
+ });
+};
+setStatics('pop,reverse,shift,keys,values,entries', 1);
+setStatics('indexOf,every,some,forEach,map,filter,find,findIndex,includes', 3);
+setStatics('join,slice,concat,push,splice,unshift,sort,lastIndexOf,' +
+ 'reduce,reduceRight,copyWithin,fill');
+$export($export.S, 'Array', statics);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.add-to-unscopables.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.add-to-unscopables.js
new file mode 100644
index 00000000..faf87af3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.add-to-unscopables.js
@@ -0,0 +1 @@
+module.exports = function(){ /* empty */ };
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.collection.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.collection.js
new file mode 100644
index 00000000..9d234d13
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.collection.js
@@ -0,0 +1,55 @@
+'use strict';
+var $ = require('./$')
+ , global = require('./$.global')
+ , $export = require('./$.export')
+ , fails = require('./$.fails')
+ , hide = require('./$.hide')
+ , redefineAll = require('./$.redefine-all')
+ , forOf = require('./$.for-of')
+ , strictNew = require('./$.strict-new')
+ , isObject = require('./$.is-object')
+ , setToStringTag = require('./$.set-to-string-tag')
+ , DESCRIPTORS = require('./$.descriptors');
+
+module.exports = function(NAME, wrapper, methods, common, IS_MAP, IS_WEAK){
+ var Base = global[NAME]
+ , C = Base
+ , ADDER = IS_MAP ? 'set' : 'add'
+ , proto = C && C.prototype
+ , O = {};
+ if(!DESCRIPTORS || typeof C != 'function' || !(IS_WEAK || proto.forEach && !fails(function(){
+ new C().entries().next();
+ }))){
+ // create collection constructor
+ C = common.getConstructor(wrapper, NAME, IS_MAP, ADDER);
+ redefineAll(C.prototype, methods);
+ } else {
+ C = wrapper(function(target, iterable){
+ strictNew(target, C, NAME);
+ target._c = new Base;
+ if(iterable != undefined)forOf(iterable, IS_MAP, target[ADDER], target);
+ });
+ $.each.call('add,clear,delete,forEach,get,has,set,keys,values,entries'.split(','),function(KEY){
+ var IS_ADDER = KEY == 'add' || KEY == 'set';
+ if(KEY in proto && !(IS_WEAK && KEY == 'clear'))hide(C.prototype, KEY, function(a, b){
+ if(!IS_ADDER && IS_WEAK && !isObject(a))return KEY == 'get' ? undefined : false;
+ var result = this._c[KEY](a === 0 ? 0 : a, b);
+ return IS_ADDER ? this : result;
+ });
+ });
+ if('size' in proto)$.setDesc(C.prototype, 'size', {
+ get: function(){
+ return this._c.size;
+ }
+ });
+ }
+
+ setToStringTag(C, NAME);
+
+ O[NAME] = C;
+ $export($export.G + $export.W + $export.F, O);
+
+ if(!IS_WEAK)common.setStrong(C, NAME, IS_MAP);
+
+ return C;
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.export.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.export.js
new file mode 100644
index 00000000..507b5a22
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.export.js
@@ -0,0 +1,46 @@
+var global = require('./$.global')
+ , core = require('./$.core')
+ , ctx = require('./$.ctx')
+ , PROTOTYPE = 'prototype';
+
+var $export = function(type, name, source){
+ var IS_FORCED = type & $export.F
+ , IS_GLOBAL = type & $export.G
+ , IS_STATIC = type & $export.S
+ , IS_PROTO = type & $export.P
+ , IS_BIND = type & $export.B
+ , IS_WRAP = type & $export.W
+ , exports = IS_GLOBAL ? core : core[name] || (core[name] = {})
+ , target = IS_GLOBAL ? global : IS_STATIC ? global[name] : (global[name] || {})[PROTOTYPE]
+ , key, own, out;
+ if(IS_GLOBAL)source = name;
+ for(key in source){
+ // contains in native
+ own = !IS_FORCED && target && key in target;
+ if(own && key in exports)continue;
+ // export native or passed
+ out = own ? target[key] : source[key];
+ // prevent global pollution for namespaces
+ exports[key] = IS_GLOBAL && typeof target[key] != 'function' ? source[key]
+ // bind timers to global for call from export context
+ : IS_BIND && own ? ctx(out, global)
+ // wrap global constructors for prevent change them in library
+ : IS_WRAP && target[key] == out ? (function(C){
+ var F = function(param){
+ return this instanceof C ? new C(param) : C(param);
+ };
+ F[PROTOTYPE] = C[PROTOTYPE];
+ return F;
+ // make static versions for prototype methods
+ })(out) : IS_PROTO && typeof out == 'function' ? ctx(Function.call, out) : out;
+ if(IS_PROTO)(exports[PROTOTYPE] || (exports[PROTOTYPE] = {}))[key] = out;
+ }
+};
+// type bitmap
+$export.F = 1; // forced
+$export.G = 2; // global
+$export.S = 4; // static
+$export.P = 8; // proto
+$export.B = 16; // bind
+$export.W = 32; // wrap
+module.exports = $export;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.library.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.library.js
new file mode 100644
index 00000000..73f737c5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.library.js
@@ -0,0 +1 @@
+module.exports = true;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.path.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.path.js
new file mode 100644
index 00000000..27bb24b8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.path.js
@@ -0,0 +1 @@
+module.exports = require('./$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.redefine.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.redefine.js
new file mode 100644
index 00000000..57453fd1
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.redefine.js
@@ -0,0 +1 @@
+module.exports = require('./$.hide');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.set-species.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.set-species.js
new file mode 100644
index 00000000..f6720c36
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/$.set-species.js
@@ -0,0 +1,13 @@
+'use strict';
+var core = require('./$.core')
+ , $ = require('./$')
+ , DESCRIPTORS = require('./$.descriptors')
+ , SPECIES = require('./$.wks')('species');
+
+module.exports = function(KEY){
+ var C = core[KEY];
+ if(DESCRIPTORS && C && !C[SPECIES])$.setDesc(C, SPECIES, {
+ configurable: true,
+ get: function(){ return this; }
+ });
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.date.to-string.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.date.to-string.js
new file mode 100644
index 00000000..e69de29b
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.function.name.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.function.name.js
new file mode 100644
index 00000000..e69de29b
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.number.constructor.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.number.constructor.js
new file mode 100644
index 00000000..e69de29b
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.object.to-string.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.object.to-string.js
new file mode 100644
index 00000000..e69de29b
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.regexp.constructor.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.regexp.constructor.js
new file mode 100644
index 00000000..087d9beb
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.regexp.constructor.js
@@ -0,0 +1 @@
+require('./$.set-species')('RegExp');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.regexp.flags.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.regexp.flags.js
new file mode 100644
index 00000000..e69de29b
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.regexp.match.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.regexp.match.js
new file mode 100644
index 00000000..e69de29b
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.regexp.replace.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.regexp.replace.js
new file mode 100644
index 00000000..e69de29b
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.regexp.search.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.regexp.search.js
new file mode 100644
index 00000000..e69de29b
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.regexp.split.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/es6.regexp.split.js
new file mode 100644
index 00000000..e69de29b
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/web.dom.iterable.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/web.dom.iterable.js
new file mode 100644
index 00000000..988c6da2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/library/web.dom.iterable.js
@@ -0,0 +1,3 @@
+require('./es6.array.iterator');
+var Iterators = require('./$.iterators');
+Iterators.NodeList = Iterators.HTMLCollection = Iterators.Array;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/web.dom.iterable.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/web.dom.iterable.js
new file mode 100644
index 00000000..94099b8f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/web.dom.iterable.js
@@ -0,0 +1,12 @@
+require('./es6.array.iterator');
+var global = require('./$.global')
+ , hide = require('./$.hide')
+ , Iterators = require('./$.iterators')
+ , ITERATOR = require('./$.wks')('iterator')
+ , NL = global.NodeList
+ , HTC = global.HTMLCollection
+ , NLProto = NL && NL.prototype
+ , HTCProto = HTC && HTC.prototype
+ , ArrayValues = Iterators.NodeList = Iterators.HTMLCollection = Iterators.Array;
+if(NLProto && !NLProto[ITERATOR])hide(NLProto, ITERATOR, ArrayValues);
+if(HTCProto && !HTCProto[ITERATOR])hide(HTCProto, ITERATOR, ArrayValues);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/web.immediate.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/web.immediate.js
new file mode 100644
index 00000000..fa64f08e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/web.immediate.js
@@ -0,0 +1,6 @@
+var $export = require('./$.export')
+ , $task = require('./$.task');
+$export($export.G + $export.B, {
+ setImmediate: $task.set,
+ clearImmediate: $task.clear
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/web.timers.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/web.timers.js
new file mode 100644
index 00000000..74b72019
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/modules/web.timers.js
@@ -0,0 +1,20 @@
+// ie9- setTimeout & setInterval additional parameters fix
+var global = require('./$.global')
+ , $export = require('./$.export')
+ , invoke = require('./$.invoke')
+ , partial = require('./$.partial')
+ , navigator = global.navigator
+ , MSIE = !!navigator && /MSIE .\./.test(navigator.userAgent); // <- dirty ie9- check
+var wrap = function(set){
+ return MSIE ? function(fn, time /*, ...args */){
+ return set(invoke(
+ partial,
+ [].slice.call(arguments, 2),
+ typeof fn == 'function' ? fn : Function(fn)
+ ), time);
+ } : set;
+};
+$export($export.G + $export.B + $export.F * MSIE, {
+ setTimeout: wrap(global.setTimeout),
+ setInterval: wrap(global.setInterval)
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/package.json b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/package.json
new file mode 100644
index 00000000..1da1372b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/package.json
@@ -0,0 +1,88 @@
+{
+ "_from": "core-js@^1.0.0",
+ "_id": "core-js@1.2.7",
+ "_inBundle": false,
+ "_integrity": "sha1-ZSKUwUZR2yj6k70tX/KYOk8IxjY=",
+ "_location": "/react-dropzone/core-js",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "core-js@^1.0.0",
+ "name": "core-js",
+ "escapedName": "core-js",
+ "rawSpec": "^1.0.0",
+ "saveSpec": null,
+ "fetchSpec": "^1.0.0"
+ },
+ "_requiredBy": [
+ "/react-dropzone/fbjs"
+ ],
+ "_resolved": "https://registry.npmjs.org/core-js/-/core-js-1.2.7.tgz",
+ "_shasum": "652294c14651db28fa93bd2d5ff2983a4f08c636",
+ "_spec": "core-js@^1.0.0",
+ "_where": "C:\\Users\\deranjer\\go\\src\\github.com\\deranjer\\goTorrent\\goTorrentWebUI\\node_modules\\react-dropzone\\node_modules\\fbjs",
+ "bugs": {
+ "url": "https://github.com/zloirock/core-js/issues"
+ },
+ "bundleDependencies": false,
+ "deprecated": false,
+ "description": "Standard library",
+ "devDependencies": {
+ "LiveScript": "1.3.x",
+ "eslint": "1.9.x",
+ "grunt": "0.4.x",
+ "grunt-cli": "0.1.x",
+ "grunt-contrib-clean": "0.6.x",
+ "grunt-contrib-copy": "0.8.x",
+ "grunt-contrib-uglify": "0.10.x",
+ "grunt-contrib-watch": "0.6.x",
+ "grunt-karma": "0.12.x",
+ "grunt-livescript": "0.5.x",
+ "karma": "0.13.x",
+ "karma-chrome-launcher": "0.2.x",
+ "karma-firefox-launcher": "0.1.x",
+ "karma-ie-launcher": "0.2.x",
+ "karma-phantomjs-launcher": "0.2.x",
+ "karma-qunit": "0.1.x",
+ "phantomjs": "1.9.x",
+ "promises-aplus-tests": "2.1.x",
+ "qunitjs": "1.23.x",
+ "webpack": "1.12.x"
+ },
+ "homepage": "https://github.com/zloirock/core-js#readme",
+ "keywords": [
+ "ES5",
+ "ECMAScript 5",
+ "ES6",
+ "ECMAScript 6",
+ "ES7",
+ "ECMAScript 7",
+ "Harmony",
+ "Strawman",
+ "Map",
+ "Set",
+ "WeakMap",
+ "WeakSet",
+ "Promise",
+ "Symbol",
+ "Array generics",
+ "setImmediate",
+ "Dict",
+ "partial application"
+ ],
+ "license": "MIT",
+ "main": "index.js",
+ "name": "core-js",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/zloirock/core-js.git"
+ },
+ "scripts": {
+ "grunt": "grunt",
+ "lint": "eslint es5 es6 es7 js web core fn modules",
+ "promises-tests": "promises-aplus-tests tests/promises-aplus/adapter",
+ "test": "npm run lint && npm run grunt livescript client karma:continuous library karma:continuous-library && npm run promises-tests && lsc tests/commonjs"
+ },
+ "version": "1.2.7"
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/shim.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/shim.js
new file mode 100644
index 00000000..6d38d2e2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/shim.js
@@ -0,0 +1,104 @@
+require('./modules/es5');
+require('./modules/es6.symbol');
+require('./modules/es6.object.assign');
+require('./modules/es6.object.is');
+require('./modules/es6.object.set-prototype-of');
+require('./modules/es6.object.to-string');
+require('./modules/es6.object.freeze');
+require('./modules/es6.object.seal');
+require('./modules/es6.object.prevent-extensions');
+require('./modules/es6.object.is-frozen');
+require('./modules/es6.object.is-sealed');
+require('./modules/es6.object.is-extensible');
+require('./modules/es6.object.get-own-property-descriptor');
+require('./modules/es6.object.get-prototype-of');
+require('./modules/es6.object.keys');
+require('./modules/es6.object.get-own-property-names');
+require('./modules/es6.function.name');
+require('./modules/es6.function.has-instance');
+require('./modules/es6.number.constructor');
+require('./modules/es6.number.epsilon');
+require('./modules/es6.number.is-finite');
+require('./modules/es6.number.is-integer');
+require('./modules/es6.number.is-nan');
+require('./modules/es6.number.is-safe-integer');
+require('./modules/es6.number.max-safe-integer');
+require('./modules/es6.number.min-safe-integer');
+require('./modules/es6.number.parse-float');
+require('./modules/es6.number.parse-int');
+require('./modules/es6.math.acosh');
+require('./modules/es6.math.asinh');
+require('./modules/es6.math.atanh');
+require('./modules/es6.math.cbrt');
+require('./modules/es6.math.clz32');
+require('./modules/es6.math.cosh');
+require('./modules/es6.math.expm1');
+require('./modules/es6.math.fround');
+require('./modules/es6.math.hypot');
+require('./modules/es6.math.imul');
+require('./modules/es6.math.log10');
+require('./modules/es6.math.log1p');
+require('./modules/es6.math.log2');
+require('./modules/es6.math.sign');
+require('./modules/es6.math.sinh');
+require('./modules/es6.math.tanh');
+require('./modules/es6.math.trunc');
+require('./modules/es6.string.from-code-point');
+require('./modules/es6.string.raw');
+require('./modules/es6.string.trim');
+require('./modules/es6.string.iterator');
+require('./modules/es6.string.code-point-at');
+require('./modules/es6.string.ends-with');
+require('./modules/es6.string.includes');
+require('./modules/es6.string.repeat');
+require('./modules/es6.string.starts-with');
+require('./modules/es6.array.from');
+require('./modules/es6.array.of');
+require('./modules/es6.array.iterator');
+require('./modules/es6.array.species');
+require('./modules/es6.array.copy-within');
+require('./modules/es6.array.fill');
+require('./modules/es6.array.find');
+require('./modules/es6.array.find-index');
+require('./modules/es6.regexp.constructor');
+require('./modules/es6.regexp.flags');
+require('./modules/es6.regexp.match');
+require('./modules/es6.regexp.replace');
+require('./modules/es6.regexp.search');
+require('./modules/es6.regexp.split');
+require('./modules/es6.promise');
+require('./modules/es6.map');
+require('./modules/es6.set');
+require('./modules/es6.weak-map');
+require('./modules/es6.weak-set');
+require('./modules/es6.reflect.apply');
+require('./modules/es6.reflect.construct');
+require('./modules/es6.reflect.define-property');
+require('./modules/es6.reflect.delete-property');
+require('./modules/es6.reflect.enumerate');
+require('./modules/es6.reflect.get');
+require('./modules/es6.reflect.get-own-property-descriptor');
+require('./modules/es6.reflect.get-prototype-of');
+require('./modules/es6.reflect.has');
+require('./modules/es6.reflect.is-extensible');
+require('./modules/es6.reflect.own-keys');
+require('./modules/es6.reflect.prevent-extensions');
+require('./modules/es6.reflect.set');
+require('./modules/es6.reflect.set-prototype-of');
+require('./modules/es7.array.includes');
+require('./modules/es7.string.at');
+require('./modules/es7.string.pad-left');
+require('./modules/es7.string.pad-right');
+require('./modules/es7.string.trim-left');
+require('./modules/es7.string.trim-right');
+require('./modules/es7.regexp.escape');
+require('./modules/es7.object.get-own-property-descriptors');
+require('./modules/es7.object.values');
+require('./modules/es7.object.entries');
+require('./modules/es7.map.to-json');
+require('./modules/es7.set.to-json');
+require('./modules/js.array.statics');
+require('./modules/web.timers');
+require('./modules/web.immediate');
+require('./modules/web.dom.iterable');
+module.exports = require('./modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/web/dom.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/web/dom.js
new file mode 100644
index 00000000..9b448cfe
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/web/dom.js
@@ -0,0 +1,2 @@
+require('../modules/web.dom.iterable');
+module.exports = require('../modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/web/immediate.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/web/immediate.js
new file mode 100644
index 00000000..e4e5493b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/web/immediate.js
@@ -0,0 +1,2 @@
+require('../modules/web.immediate');
+module.exports = require('../modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/web/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/web/index.js
new file mode 100644
index 00000000..6c3221e2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/web/index.js
@@ -0,0 +1,4 @@
+require('../modules/web.timers');
+require('../modules/web.immediate');
+require('../modules/web.dom.iterable');
+module.exports = require('../modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/web/timers.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/web/timers.js
new file mode 100644
index 00000000..763ea44e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/core-js/web/timers.js
@@ -0,0 +1,2 @@
+require('../modules/web.timers');
+module.exports = require('../modules/$.core');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/.npmignore b/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/.npmignore
new file mode 100644
index 00000000..b512c09d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/.npmignore
@@ -0,0 +1 @@
+node_modules
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/.travis.yml b/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/.travis.yml
new file mode 100644
index 00000000..abc4f48c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/.travis.yml
@@ -0,0 +1,25 @@
+language: node_js
+sudo: false
+node_js:
+ - "0.10"
+ - 0.12
+ - iojs
+ - 4
+ - 5
+env:
+ - CXX=g++-4.8
+addons:
+ apt:
+ sources:
+ - ubuntu-toolchain-r-test
+ packages:
+ - g++-4.8
+notifications:
+ email:
+ - andris@kreata.ee
+ webhooks:
+ urls:
+ - https://webhooks.gitter.im/e/0ed18fd9b3e529b3c2cc
+ on_success: change # options: [always|never|change] default: always
+ on_failure: always # options: [always|never|change] default: always
+ on_start: false # default: false
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/LICENSE b/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/LICENSE
new file mode 100644
index 00000000..33f5a9a3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/LICENSE
@@ -0,0 +1,16 @@
+Copyright (c) 2012-2014 Andris Reinman
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/README.md b/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/README.md
new file mode 100644
index 00000000..62e6bf88
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/README.md
@@ -0,0 +1,52 @@
+# Encoding
+
+**encoding** is a simple wrapper around [node-iconv](https://github.com/bnoordhuis/node-iconv) and [iconv-lite](https://github.com/ashtuchkin/iconv-lite/) to convert strings from one encoding to another. If node-iconv is not available for some reason,
+iconv-lite will be used instead of it as a fallback.
+
+[](http://travis-ci.org/andris9/Nodemailer)
+[](http://badge.fury.io/js/encoding)
+
+## Install
+
+Install through npm
+
+ npm install encoding
+
+## Usage
+
+Require the module
+
+ var encoding = require("encoding");
+
+Convert with encoding.convert()
+
+ var resultBuffer = encoding.convert(text, toCharset, fromCharset);
+
+Where
+
+ * **text** is either a Buffer or a String to be converted
+ * **toCharset** is the characterset to convert the string
+ * **fromCharset** (*optional*, defaults to UTF-8) is the source charset
+
+Output of the conversion is always a Buffer object.
+
+Example
+
+ var result = encoding.convert("ÕÄÖÜ", "Latin_1");
+ console.log(result); //
+
+## iconv support
+
+By default only iconv-lite is bundled. If you need node-iconv support, you need to add it
+as an additional dependency for your project:
+
+ ...,
+ "dependencies":{
+ "encoding": "*",
+ "iconv": "*"
+ },
+ ...
+
+## License
+
+**MIT**
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/lib/encoding.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/lib/encoding.js
new file mode 100644
index 00000000..cbea3ced
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/lib/encoding.js
@@ -0,0 +1,113 @@
+'use strict';
+
+var iconvLite = require('iconv-lite');
+// Load Iconv from an external file to be able to disable Iconv for webpack
+// Add /\/iconv-loader$/ to webpack.IgnorePlugin to ignore it
+var Iconv = require('./iconv-loader');
+
+// Expose to the world
+module.exports.convert = convert;
+
+/**
+ * Convert encoding of an UTF-8 string or a buffer
+ *
+ * @param {String|Buffer} str String to be converted
+ * @param {String} to Encoding to be converted to
+ * @param {String} [from='UTF-8'] Encoding to be converted from
+ * @param {Boolean} useLite If set to ture, force to use iconvLite
+ * @return {Buffer} Encoded string
+ */
+function convert(str, to, from, useLite) {
+ from = checkEncoding(from || 'UTF-8');
+ to = checkEncoding(to || 'UTF-8');
+ str = str || '';
+
+ var result;
+
+ if (from !== 'UTF-8' && typeof str === 'string') {
+ str = new Buffer(str, 'binary');
+ }
+
+ if (from === to) {
+ if (typeof str === 'string') {
+ result = new Buffer(str);
+ } else {
+ result = str;
+ }
+ } else if (Iconv && !useLite) {
+ try {
+ result = convertIconv(str, to, from);
+ } catch (E) {
+ console.error(E);
+ try {
+ result = convertIconvLite(str, to, from);
+ } catch (E) {
+ console.error(E);
+ result = str;
+ }
+ }
+ } else {
+ try {
+ result = convertIconvLite(str, to, from);
+ } catch (E) {
+ console.error(E);
+ result = str;
+ }
+ }
+
+
+ if (typeof result === 'string') {
+ result = new Buffer(result, 'utf-8');
+ }
+
+ return result;
+}
+
+/**
+ * Convert encoding of a string with node-iconv (if available)
+ *
+ * @param {String|Buffer} str String to be converted
+ * @param {String} to Encoding to be converted to
+ * @param {String} [from='UTF-8'] Encoding to be converted from
+ * @return {Buffer} Encoded string
+ */
+function convertIconv(str, to, from) {
+ var response, iconv;
+ iconv = new Iconv(from, to + '//TRANSLIT//IGNORE');
+ response = iconv.convert(str);
+ return response.slice(0, response.length);
+}
+
+/**
+ * Convert encoding of astring with iconv-lite
+ *
+ * @param {String|Buffer} str String to be converted
+ * @param {String} to Encoding to be converted to
+ * @param {String} [from='UTF-8'] Encoding to be converted from
+ * @return {Buffer} Encoded string
+ */
+function convertIconvLite(str, to, from) {
+ if (to === 'UTF-8') {
+ return iconvLite.decode(str, from);
+ } else if (from === 'UTF-8') {
+ return iconvLite.encode(str, to);
+ } else {
+ return iconvLite.encode(iconvLite.decode(str, from), to);
+ }
+}
+
+/**
+ * Converts charset name if needed
+ *
+ * @param {String} name Character set
+ * @return {String} Character set name
+ */
+function checkEncoding(name) {
+ return (name || '').toString().trim().
+ replace(/^latin[\-_]?(\d+)$/i, 'ISO-8859-$1').
+ replace(/^win(?:dows)?[\-_]?(\d+)$/i, 'WINDOWS-$1').
+ replace(/^utf[\-_]?(\d+)$/i, 'UTF-$1').
+ replace(/^ks_c_5601\-1987$/i, 'CP949').
+ replace(/^us[\-_]?ascii$/i, 'ASCII').
+ toUpperCase();
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/lib/iconv-loader.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/lib/iconv-loader.js
new file mode 100644
index 00000000..8e925fd8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/lib/iconv-loader.js
@@ -0,0 +1,14 @@
+'use strict';
+
+var iconv_package;
+var Iconv;
+
+try {
+ // this is to fool browserify so it doesn't try (in vain) to install iconv.
+ iconv_package = 'iconv';
+ Iconv = require(iconv_package).Iconv;
+} catch (E) {
+ // node-iconv not present
+}
+
+module.exports = Iconv;
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/package.json b/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/package.json
new file mode 100644
index 00000000..0ea82a33
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/package.json
@@ -0,0 +1,53 @@
+{
+ "_from": "encoding@^0.1.11",
+ "_id": "encoding@0.1.12",
+ "_inBundle": false,
+ "_integrity": "sha1-U4tm8+5izRq1HsMjgp0flIDHS+s=",
+ "_location": "/react-dropzone/encoding",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "encoding@^0.1.11",
+ "name": "encoding",
+ "escapedName": "encoding",
+ "rawSpec": "^0.1.11",
+ "saveSpec": null,
+ "fetchSpec": "^0.1.11"
+ },
+ "_requiredBy": [
+ "/react-dropzone/node-fetch"
+ ],
+ "_resolved": "https://registry.npmjs.org/encoding/-/encoding-0.1.12.tgz",
+ "_shasum": "538b66f3ee62cd1ab51ec323829d1f9480c74beb",
+ "_spec": "encoding@^0.1.11",
+ "_where": "C:\\Users\\deranjer\\go\\src\\github.com\\deranjer\\goTorrent\\goTorrentWebUI\\node_modules\\react-dropzone\\node_modules\\node-fetch",
+ "author": {
+ "name": "Andris Reinman"
+ },
+ "bugs": {
+ "url": "https://github.com/andris9/encoding/issues"
+ },
+ "bundleDependencies": false,
+ "dependencies": {
+ "iconv-lite": "~0.4.13"
+ },
+ "deprecated": false,
+ "description": "Convert encodings, uses iconv by default and fallbacks to iconv-lite if needed",
+ "devDependencies": {
+ "iconv": "~2.1.11",
+ "nodeunit": "~0.9.1"
+ },
+ "homepage": "https://github.com/andris9/encoding#readme",
+ "license": "MIT",
+ "main": "lib/encoding.js",
+ "name": "encoding",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/andris9/encoding.git"
+ },
+ "scripts": {
+ "test": "nodeunit test"
+ },
+ "version": "0.1.12"
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/test/test.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/test/test.js
new file mode 100644
index 00000000..0de4dcb1
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/encoding/test/test.js
@@ -0,0 +1,75 @@
+'use strict';
+
+var Iconv = require('../lib/iconv-loader');
+var encoding = require('../lib/encoding');
+
+exports['General tests'] = {
+
+ 'Iconv is available': function (test) {
+ test.ok(Iconv);
+ test.done();
+ },
+
+ 'From UTF-8 to Latin_1 with Iconv': function (test) {
+ var input = 'ÕÄÖÜ',
+ expected = new Buffer([0xd5, 0xc4, 0xd6, 0xdc]);
+ test.deepEqual(encoding.convert(input, 'latin1'), expected);
+ test.done();
+ },
+
+ 'From Latin_1 to UTF-8 with Iconv': function (test) {
+ var input = new Buffer([0xd5, 0xc4, 0xd6, 0xdc]),
+ expected = 'ÕÄÖÜ';
+ test.deepEqual(encoding.convert(input, 'utf-8', 'latin1').toString(), expected);
+ test.done();
+ },
+
+ 'From UTF-8 to UTF-8 with Iconv': function (test) {
+ var input = 'ÕÄÖÜ',
+ expected = new Buffer('ÕÄÖÜ');
+ test.deepEqual(encoding.convert(input, 'utf-8', 'utf-8'), expected);
+ test.done();
+ },
+
+ 'From Latin_13 to Latin_15 with Iconv': function (test) {
+ var input = new Buffer([0xd5, 0xc4, 0xd6, 0xdc, 0xd0]),
+ expected = new Buffer([0xd5, 0xc4, 0xd6, 0xdc, 0xA6]);
+ test.deepEqual(encoding.convert(input, 'latin_15', 'latin13'), expected);
+ test.done();
+ },
+
+ 'From ISO-2022-JP to UTF-8 with Iconv': function (test) {
+ var input = new Buffer('GyRCM1g5OzU7PVEwdzgmPSQ4IUYkMnFKczlwGyhC', 'base64'),
+ expected = new Buffer('5a2m5qCh5oqA6KGT5ZOh56CU5L+u5qSc6KiO5Lya5aCx5ZGK', 'base64');
+ test.deepEqual(encoding.convert(input, 'utf-8', 'ISO-2022-JP'), expected);
+ test.done();
+ },
+
+ 'From UTF-8 to Latin_1 with iconv-lite': function (test) {
+ var input = 'ÕÄÖÜ',
+ expected = new Buffer([0xd5, 0xc4, 0xd6, 0xdc]);
+ test.deepEqual(encoding.convert(input, 'latin1', false, true), expected);
+ test.done();
+ },
+
+ 'From Latin_1 to UTF-8 with iconv-lite': function (test) {
+ var input = new Buffer([0xd5, 0xc4, 0xd6, 0xdc]),
+ expected = 'ÕÄÖÜ';
+ test.deepEqual(encoding.convert(input, 'utf-8', 'latin1', true).toString(), expected);
+ test.done();
+ },
+
+ 'From UTF-8 to UTF-8 with iconv-lite': function (test) {
+ var input = 'ÕÄÖÜ',
+ expected = new Buffer('ÕÄÖÜ');
+ test.deepEqual(encoding.convert(input, 'utf-8', 'utf-8', true), expected);
+ test.done();
+ },
+
+ 'From Latin_13 to Latin_15 with iconv-lite': function (test) {
+ var input = new Buffer([0xd5, 0xc4, 0xd6, 0xdc, 0xd0]),
+ expected = new Buffer([0xd5, 0xc4, 0xd6, 0xdc, 0xA6]);
+ test.deepEqual(encoding.convert(input, 'latin_15', 'latin13', true), expected);
+ test.done();
+ }
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/CHANGELOG.md b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/CHANGELOG.md
new file mode 100644
index 00000000..eabaf001
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/CHANGELOG.md
@@ -0,0 +1,214 @@
+## [Unreleased]
+
+
+## [0.8.16] - 2017-09-25
+
+### Changed
+- Relicense to MIT as part of React relicense.
+
+
+## [0.8.15] - 2017-09-07
+
+### Fixed
+- `getDocumentScrollElement` now correctly returns the `` element in Chrome 61 instead of ``.
+
+
+## [0.8.14] - 2017-07-25
+
+### Removed
+- Flow annotations for `keyMirror` module. The annotation generates a syntax error after being re-printed by Babel.
+
+
+## [0.8.13] - 2017-07-25
+
+### Added
+- Flow annotations for `keyMirror` module.
+
+### Fixed
+- Fixed strict argument arity issues with `Deferred` module.
+- Corrected License header in `EventListener`.
+
+
+## [0.8.12] - 2017-03-29
+
+### Fixed
+- Fix use of `global` working inconsistently.
+
+
+## [0.8.11] - 2017-03-21
+
+### Fixed
+- Fixed a regression resulting from making DOM utilities work in nested browsing contexts.
+
+
+## [0.8.10] - 2017-03-20
+
+### Changed
+- Made DOM utilities work in nested browsing contexts.
+
+
+## [0.8.9] - 2017-01-31
+
+### Fixed
+- Updated `partitionObjectByKey` Flow annotations for Flow 0.38.
+
+
+## [0.8.8] - 2016-12-20
+
+### Changed
+- `invariant`: Moved `process.env.NODE_ENV` check to module scope, eliminating check on each call.
+
+
+## [0.8.7] - 2016-12-19
+
+### Added
+- New module: `setImmediate`.
+
+
+## [0.8.6] - 2016-11-09
+
+### Removed
+- Removed runtime dependency on immutable, reducing package size.
+
+
+## [0.8.5] - 2016-09-27
+
+### Fixed
+- Fixed all remaining issues resulting in Flow errors when `fbjs` is a dependency of a dependency.
+
+### Removed
+- Removed now extraneous `flow/lib/Promise.js`.
+
+## [0.8.4] - 2016-08-19
+
+### Changed
+- Moved `try/catch` in `warning` module to helper function to prevent deopts.
+
+
+## [0.8.3] - 2016-05-25
+
+### Added
+- `Deferred`: added `Deferred.prototype.catch` to avoid having to call this directly on the Promise.
+- `UnicodeUtilsExtra`: added several methods for escaping strings.
+
+### Changed
+- More Flow annotations: `containsNode`, `emptyFunction`, `memoizeStringOnly`
+- Added explicit `` type arguments to in anticipation of a future Flow change requiring them.
+- `Object.assign` calls now replaced with usage of `object-assign` module.
+
+### Fixed
+- Type imports in .js.flow files are now properly using relative paths.
+- `DataTransfer`: handle Firefox better
+
+
+## [0.8.2] - 2016-05-05
+
+### Removed
+- Removed extraneous production dependency
+
+
+## [0.8.1] - 2016-04-18
+
+### Added
+- We now include a `Promise` class definition in `flow/lib` to account for the changes in Flow v0.23 which removed non-spec methods. This will allow our code to continue typechecking while using these methods.
+
+
+## [0.8.0] - 2016-04-04
+
+### Added
+- Several additional modules. Notably, a collection of Unicode utilities and many new `functional` helpers.
+- `CSSCore`: added `matchesSelector` method
+
+### Changed
+- Copyright headers updated to reflect current boilerplate
+- `@providesModule` headers removed from generated source code
+- Flow files now contain relative requires, improving compatibility with Haste and CommonJS module systems
+
+### Fixed
+- `isEmpty`: Protect from breaking in environments without `Symbol` defined
+
+
+## [0.7.2] - 2016-02-05
+
+### Fixed
+- `URI`: correctly store reference to value in constructor and return it when stringifying
+
+### Removed
+- Backed out rejection tracking for React Native `Promise` implementation. That code now lives in React Native.
+
+
+## [0.7.1] - 2016-02-02
+
+### Fixed
+
+- Corrected require path issue for native `Promise` module
+
+
+## [0.7.0] - 2016-01-27
+
+### Added
+- `Promise` for React Native with rejection tracking in `__DEV__` and a `finally` method
+- `_shouldPolyfillES6Collection`: check if ES6 Collections need to be polyfilled.
+
+### Removed
+- `toArray`: removed in favor of using `Array.from` directly.
+
+### Changed
+- `ErrorUtils`: Re-uses any global instance that already exists
+- `fetch`: Switched to `isomorphic-fetch` when a global implementation is missing
+- `shallowEqual`: handles `NaN` values appropriately (as equal), now using `Object.is` semantics
+
+
+## [0.6.1] - 2016-01-06
+
+### Changed
+- `getActiveElement`: no longer throws in non-browser environment (again)
+
+
+## [0.6.0] - 2015-12-29
+
+### Changed
+- Flow: Original source files in `fbjs/flow/include` have been removed in favor of placing original files alongside compiled files in lib with a `.flow` suffix. This requires Flow version 0.19 or greater and a change to `.flowconfig` files to remove the include path.
+
+
+## [0.5.1] - 2015-12-13
+
+### Added
+- `base62` module
+
+
+## [0.5.0] - 2015-12-04
+
+### Changed
+
+- `getActiveElement`: No longer handles a non-existent `document`
+
+
+## [0.4.0] - 2015-10-16
+
+### Changed
+
+- `invariant`: Message is no longer prefixed with "Invariant Violation: ".
+
+
+## [0.3.2] - 2015-10-12
+
+### Added
+- Apply appropriate transform (`loose-envify`) when bundling with `browserify`
+
+
+## [0.3.1] - 2015-10-01
+
+### Fixed
+- Ensure the build completes correctly before packaging
+
+
+## [0.3.0] - 2015-10-01
+
+### Added
+- More modules: `memoizeStringOnly`, `joinClasses`
+- `UserAgent`: Query information about current user agent
+
+### Changed
+- `fetchWithRetries`: Reject failure with an Error, not the response
+- `getActiveElement`: no longer throws in non-browser environment
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/LICENSE b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/LICENSE
new file mode 100644
index 00000000..29e2bc21
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/LICENSE
@@ -0,0 +1,20 @@
+MIT License
+
+Copyright (c) 2013-present, Facebook, Inc.
+
+Permission is hereby granted, free of charge, to any person obtaining a copy of
+this software and associated documentation files (the "Software"), to deal in
+the Software without restriction, including without limitation the rights to
+use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of
+the Software, and to permit persons to whom the Software is furnished to do so,
+subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS
+FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR
+COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER
+IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN
+CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/README.md b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/README.md
new file mode 100644
index 00000000..50dcfaa3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/README.md
@@ -0,0 +1,46 @@
+# FBJS
+
+## Purpose
+
+To make it easier for Facebook to share and consume our own JavaScript. Primarily this will allow us to ship code without worrying too much about where it lives, keeping with the spirit of `@providesModule` but working in the broader JavaScript ecosystem.
+
+**Note:** If you are consuming the code here and you are not also a Facebook project, be prepared for a bad time. APIs may appear or disappear and we may not follow semver strictly, though we will do our best to. This library is being published with our use cases in mind and is not necessarily meant to be consumed by the broader public. In order for us to move fast and ship projects like React and Relay, we've made the decision to not support everybody. We probably won't take your feature requests unless they align with our needs. There will be overlap in functionality here and in other open source projects.
+
+## Usage
+
+Any `@providesModule` modules that are used by your project should be added to `src/`. They will be built and added to `module-map.json`. This file will contain a map from `@providesModule` name to what will be published as `fbjs`. The `module-map.json` file can then be consumed in your own project, along with the [rewrite-modules](https://github.com/facebook/fbjs/blob/master/babel-preset/plugins/rewrite-modules.js) Babel plugin (which we'll publish with this), to rewrite requires in your own project. Then, just make sure `fbjs` is a dependency in your `package.json` and your package will consume the shared code.
+
+```js
+// Before transform
+const emptyFunction = require('emptyFunction');
+// After transform
+const emptyFunction = require('fbjs/lib/emptyFunction');
+```
+
+See React for an example of this. *Coming soon!*
+
+## Building
+
+It's as easy as just running gulp. This assumes you've also done `npm install -g gulp`.
+
+```sh
+gulp
+```
+
+Alternatively `npm run build` will also work.
+
+### Layout
+
+Right now these packages represent a subset of packages that we use internally at Facebook. Mostly these are support libraries used when shipping larger libraries, like React and Relay, or products. Each of these packages is in its own directory under `src/`.
+
+### Process
+
+Since we use `@providesModule`, we need to rewrite requires to be relative. Thanks to `@providesModule` requiring global uniqueness, we can do this easily. Eventually we'll try to make this part of the process go away by making more projects use CommonJS.
+
+
+## TODO
+
+- Flow: Ideally we'd ship our original files with type annotations, however that's not doable right now. We have a couple options:
+ - Make sure our transpilation step converts inline type annotations to the comment format.
+ - Make our build process also build Flow interface files which we can ship to npm.
+- Split into multiple packages. This will be better for more concise versioning, otherwise we'll likely just be shipping lots of major versions.
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/flow/lib/dev.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/flow/lib/dev.js
new file mode 100644
index 00000000..01c75f73
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/flow/lib/dev.js
@@ -0,0 +1,8 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ */
+
+declare var __DEV__: boolean;
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/index.js
new file mode 100644
index 00000000..80626fa6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/index.js
@@ -0,0 +1,10 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ */
+
+'use strict';
+
+throw new Error('The fbjs package should not be required without a full path.');
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/CSSCore.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/CSSCore.js
new file mode 100644
index 00000000..75d10bd2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/CSSCore.js
@@ -0,0 +1,119 @@
+'use strict';
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @typechecks
+ */
+
+var invariant = require('./invariant');
+
+/**
+ * The CSSCore module specifies the API (and implements most of the methods)
+ * that should be used when dealing with the display of elements (via their
+ * CSS classes and visibility on screen. It is an API focused on mutating the
+ * display and not reading it as no logical state should be encoded in the
+ * display of elements.
+ */
+
+/* Slow implementation for browsers that don't natively support .matches() */
+function matchesSelector_SLOW(element, selector) {
+ var root = element;
+ while (root.parentNode) {
+ root = root.parentNode;
+ }
+
+ var all = root.querySelectorAll(selector);
+ return Array.prototype.indexOf.call(all, element) !== -1;
+}
+
+var CSSCore = {
+
+ /**
+ * Adds the class passed in to the element if it doesn't already have it.
+ *
+ * @param {DOMElement} element the element to set the class on
+ * @param {string} className the CSS className
+ * @return {DOMElement} the element passed in
+ */
+ addClass: function addClass(element, className) {
+ !!/\s/.test(className) ? process.env.NODE_ENV !== 'production' ? invariant(false, 'CSSCore.addClass takes only a single class name. "%s" contains ' + 'multiple classes.', className) : invariant(false) : void 0;
+
+ if (className) {
+ if (element.classList) {
+ element.classList.add(className);
+ } else if (!CSSCore.hasClass(element, className)) {
+ element.className = element.className + ' ' + className;
+ }
+ }
+ return element;
+ },
+
+ /**
+ * Removes the class passed in from the element
+ *
+ * @param {DOMElement} element the element to set the class on
+ * @param {string} className the CSS className
+ * @return {DOMElement} the element passed in
+ */
+ removeClass: function removeClass(element, className) {
+ !!/\s/.test(className) ? process.env.NODE_ENV !== 'production' ? invariant(false, 'CSSCore.removeClass takes only a single class name. "%s" contains ' + 'multiple classes.', className) : invariant(false) : void 0;
+
+ if (className) {
+ if (element.classList) {
+ element.classList.remove(className);
+ } else if (CSSCore.hasClass(element, className)) {
+ element.className = element.className.replace(new RegExp('(^|\\s)' + className + '(?:\\s|$)', 'g'), '$1').replace(/\s+/g, ' ') // multiple spaces to one
+ .replace(/^\s*|\s*$/g, ''); // trim the ends
+ }
+ }
+ return element;
+ },
+
+ /**
+ * Helper to add or remove a class from an element based on a condition.
+ *
+ * @param {DOMElement} element the element to set the class on
+ * @param {string} className the CSS className
+ * @param {*} bool condition to whether to add or remove the class
+ * @return {DOMElement} the element passed in
+ */
+ conditionClass: function conditionClass(element, className, bool) {
+ return (bool ? CSSCore.addClass : CSSCore.removeClass)(element, className);
+ },
+
+ /**
+ * Tests whether the element has the class specified.
+ *
+ * @param {DOMNode|DOMWindow} element the element to check the class on
+ * @param {string} className the CSS className
+ * @return {boolean} true if the element has the class, false if not
+ */
+ hasClass: function hasClass(element, className) {
+ !!/\s/.test(className) ? process.env.NODE_ENV !== 'production' ? invariant(false, 'CSS.hasClass takes only a single class name.') : invariant(false) : void 0;
+ if (element.classList) {
+ return !!className && element.classList.contains(className);
+ }
+ return (' ' + element.className + ' ').indexOf(' ' + className + ' ') > -1;
+ },
+
+ /**
+ * Tests whether the element matches the selector specified
+ *
+ * @param {DOMNode|DOMWindow} element the element that we are querying
+ * @param {string} selector the CSS selector
+ * @return {boolean} true if the element matches the selector, false if not
+ */
+ matchesSelector: function matchesSelector(element, selector) {
+ var matchesImpl = element.matches || element.webkitMatchesSelector || element.mozMatchesSelector || element.msMatchesSelector || function (s) {
+ return matchesSelector_SLOW(element, s);
+ };
+ return matchesImpl.call(element, selector);
+ }
+
+};
+
+module.exports = CSSCore;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/CSSCore.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/CSSCore.js.flow
new file mode 100644
index 00000000..967aa189
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/CSSCore.js.flow
@@ -0,0 +1,116 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule CSSCore
+ * @typechecks
+ */
+
+const invariant = require('./invariant');
+
+/**
+ * The CSSCore module specifies the API (and implements most of the methods)
+ * that should be used when dealing with the display of elements (via their
+ * CSS classes and visibility on screen. It is an API focused on mutating the
+ * display and not reading it as no logical state should be encoded in the
+ * display of elements.
+ */
+
+/* Slow implementation for browsers that don't natively support .matches() */
+function matchesSelector_SLOW(element, selector) {
+ let root = element;
+ while (root.parentNode) {
+ root = root.parentNode;
+ }
+
+ const all = root.querySelectorAll(selector);
+ return Array.prototype.indexOf.call(all, element) !== -1;
+}
+
+const CSSCore = {
+
+ /**
+ * Adds the class passed in to the element if it doesn't already have it.
+ *
+ * @param {DOMElement} element the element to set the class on
+ * @param {string} className the CSS className
+ * @return {DOMElement} the element passed in
+ */
+ addClass: function (element, className) {
+ invariant(!/\s/.test(className), 'CSSCore.addClass takes only a single class name. "%s" contains ' + 'multiple classes.', className);
+
+ if (className) {
+ if (element.classList) {
+ element.classList.add(className);
+ } else if (!CSSCore.hasClass(element, className)) {
+ element.className = element.className + ' ' + className;
+ }
+ }
+ return element;
+ },
+
+ /**
+ * Removes the class passed in from the element
+ *
+ * @param {DOMElement} element the element to set the class on
+ * @param {string} className the CSS className
+ * @return {DOMElement} the element passed in
+ */
+ removeClass: function (element, className) {
+ invariant(!/\s/.test(className), 'CSSCore.removeClass takes only a single class name. "%s" contains ' + 'multiple classes.', className);
+
+ if (className) {
+ if (element.classList) {
+ element.classList.remove(className);
+ } else if (CSSCore.hasClass(element, className)) {
+ element.className = element.className.replace(new RegExp('(^|\\s)' + className + '(?:\\s|$)', 'g'), '$1').replace(/\s+/g, ' ') // multiple spaces to one
+ .replace(/^\s*|\s*$/g, ''); // trim the ends
+ }
+ }
+ return element;
+ },
+
+ /**
+ * Helper to add or remove a class from an element based on a condition.
+ *
+ * @param {DOMElement} element the element to set the class on
+ * @param {string} className the CSS className
+ * @param {*} bool condition to whether to add or remove the class
+ * @return {DOMElement} the element passed in
+ */
+ conditionClass: function (element, className, bool) {
+ return (bool ? CSSCore.addClass : CSSCore.removeClass)(element, className);
+ },
+
+ /**
+ * Tests whether the element has the class specified.
+ *
+ * @param {DOMNode|DOMWindow} element the element to check the class on
+ * @param {string} className the CSS className
+ * @return {boolean} true if the element has the class, false if not
+ */
+ hasClass: function (element, className) {
+ invariant(!/\s/.test(className), 'CSS.hasClass takes only a single class name.');
+ if (element.classList) {
+ return !!className && element.classList.contains(className);
+ }
+ return (' ' + element.className + ' ').indexOf(' ' + className + ' ') > -1;
+ },
+
+ /**
+ * Tests whether the element matches the selector specified
+ *
+ * @param {DOMNode|DOMWindow} element the element that we are querying
+ * @param {string} selector the CSS selector
+ * @return {boolean} true if the element matches the selector, false if not
+ */
+ matchesSelector: function (element, selector) {
+ const matchesImpl = element.matches || element.webkitMatchesSelector || element.mozMatchesSelector || element.msMatchesSelector || (s => matchesSelector_SLOW(element, s));
+ return matchesImpl.call(element, selector);
+ }
+
+};
+
+module.exports = CSSCore;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/DataTransfer.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/DataTransfer.js
new file mode 100644
index 00000000..6875d9a0
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/DataTransfer.js
@@ -0,0 +1,219 @@
+'use strict';
+
+function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @typechecks
+ */
+
+var PhotosMimeType = require('./PhotosMimeType');
+
+var createArrayFromMixed = require('./createArrayFromMixed');
+var emptyFunction = require('./emptyFunction');
+
+var CR_LF_REGEX = new RegExp('\r\n', 'g');
+var LF_ONLY = '\n';
+
+var RICH_TEXT_TYPES = {
+ 'text/rtf': 1,
+ 'text/html': 1
+};
+
+/**
+ * If DataTransferItem is a file then return the Blob of data.
+ *
+ * @param {object} item
+ * @return {?blob}
+ */
+function getFileFromDataTransfer(item) {
+ if (item.kind == 'file') {
+ return item.getAsFile();
+ }
+}
+
+var DataTransfer = function () {
+ /**
+ * @param {object} data
+ */
+ function DataTransfer(data) {
+ _classCallCheck(this, DataTransfer);
+
+ this.data = data;
+
+ // Types could be DOMStringList or array
+ this.types = data.types ? createArrayFromMixed(data.types) : [];
+ }
+
+ /**
+ * Is this likely to be a rich text data transfer?
+ *
+ * @return {boolean}
+ */
+
+
+ DataTransfer.prototype.isRichText = function isRichText() {
+ // If HTML is available, treat this data as rich text. This way, we avoid
+ // using a pasted image if it is packaged with HTML -- this may occur with
+ // pastes from MS Word, for example. However this is only rich text if
+ // there's accompanying text.
+ if (this.getHTML() && this.getText()) {
+ return true;
+ }
+
+ // When an image is copied from a preview window, you end up with two
+ // DataTransferItems one of which is a file's metadata as text. Skip those.
+ if (this.isImage()) {
+ return false;
+ }
+
+ return this.types.some(function (type) {
+ return RICH_TEXT_TYPES[type];
+ });
+ };
+
+ /**
+ * Get raw text.
+ *
+ * @return {?string}
+ */
+
+
+ DataTransfer.prototype.getText = function getText() {
+ var text;
+ if (this.data.getData) {
+ if (!this.types.length) {
+ text = this.data.getData('Text');
+ } else if (this.types.indexOf('text/plain') != -1) {
+ text = this.data.getData('text/plain');
+ }
+ }
+ return text ? text.replace(CR_LF_REGEX, LF_ONLY) : null;
+ };
+
+ /**
+ * Get HTML paste data
+ *
+ * @return {?string}
+ */
+
+
+ DataTransfer.prototype.getHTML = function getHTML() {
+ if (this.data.getData) {
+ if (!this.types.length) {
+ return this.data.getData('Text');
+ } else if (this.types.indexOf('text/html') != -1) {
+ return this.data.getData('text/html');
+ }
+ }
+ };
+
+ /**
+ * Is this a link data transfer?
+ *
+ * @return {boolean}
+ */
+
+
+ DataTransfer.prototype.isLink = function isLink() {
+ return this.types.some(function (type) {
+ return type.indexOf('Url') != -1 || type.indexOf('text/uri-list') != -1 || type.indexOf('text/x-moz-url');
+ });
+ };
+
+ /**
+ * Get a link url.
+ *
+ * @return {?string}
+ */
+
+
+ DataTransfer.prototype.getLink = function getLink() {
+ if (this.data.getData) {
+ if (this.types.indexOf('text/x-moz-url') != -1) {
+ var url = this.data.getData('text/x-moz-url').split('\n');
+ return url[0];
+ }
+ return this.types.indexOf('text/uri-list') != -1 ? this.data.getData('text/uri-list') : this.data.getData('url');
+ }
+
+ return null;
+ };
+
+ /**
+ * Is this an image data transfer?
+ *
+ * @return {boolean}
+ */
+
+
+ DataTransfer.prototype.isImage = function isImage() {
+ var isImage = this.types.some(function (type) {
+ // Firefox will have a type of application/x-moz-file for images during
+ // dragging
+ return type.indexOf('application/x-moz-file') != -1;
+ });
+
+ if (isImage) {
+ return true;
+ }
+
+ var items = this.getFiles();
+ for (var i = 0; i < items.length; i++) {
+ var type = items[i].type;
+ if (!PhotosMimeType.isImage(type)) {
+ return false;
+ }
+ }
+
+ return true;
+ };
+
+ DataTransfer.prototype.getCount = function getCount() {
+ if (this.data.hasOwnProperty('items')) {
+ return this.data.items.length;
+ } else if (this.data.hasOwnProperty('mozItemCount')) {
+ return this.data.mozItemCount;
+ } else if (this.data.files) {
+ return this.data.files.length;
+ }
+ return null;
+ };
+
+ /**
+ * Get files.
+ *
+ * @return {array}
+ */
+
+
+ DataTransfer.prototype.getFiles = function getFiles() {
+ if (this.data.items) {
+ // createArrayFromMixed doesn't properly handle DataTransferItemLists.
+ return Array.prototype.slice.call(this.data.items).map(getFileFromDataTransfer).filter(emptyFunction.thatReturnsArgument);
+ } else if (this.data.files) {
+ return Array.prototype.slice.call(this.data.files);
+ } else {
+ return [];
+ }
+ };
+
+ /**
+ * Are there any files to fetch?
+ *
+ * @return {boolean}
+ */
+
+
+ DataTransfer.prototype.hasFiles = function hasFiles() {
+ return this.getFiles().length > 0;
+ };
+
+ return DataTransfer;
+}();
+
+module.exports = DataTransfer;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/DataTransfer.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/DataTransfer.js.flow
new file mode 100644
index 00000000..3588a193
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/DataTransfer.js.flow
@@ -0,0 +1,194 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule DataTransfer
+ * @typechecks
+ */
+
+var PhotosMimeType = require('./PhotosMimeType');
+
+var createArrayFromMixed = require('./createArrayFromMixed');
+var emptyFunction = require('./emptyFunction');
+
+var CR_LF_REGEX = new RegExp('\u000D\u000A', 'g');
+var LF_ONLY = '\u000A';
+
+var RICH_TEXT_TYPES = {
+ 'text/rtf': 1,
+ 'text/html': 1
+};
+
+/**
+ * If DataTransferItem is a file then return the Blob of data.
+ *
+ * @param {object} item
+ * @return {?blob}
+ */
+function getFileFromDataTransfer(item) {
+ if (item.kind == 'file') {
+ return item.getAsFile();
+ }
+}
+
+class DataTransfer {
+ /**
+ * @param {object} data
+ */
+ constructor(data) {
+ this.data = data;
+
+ // Types could be DOMStringList or array
+ this.types = data.types ? createArrayFromMixed(data.types) : [];
+ }
+
+ /**
+ * Is this likely to be a rich text data transfer?
+ *
+ * @return {boolean}
+ */
+ isRichText() {
+ // If HTML is available, treat this data as rich text. This way, we avoid
+ // using a pasted image if it is packaged with HTML -- this may occur with
+ // pastes from MS Word, for example. However this is only rich text if
+ // there's accompanying text.
+ if (this.getHTML() && this.getText()) {
+ return true;
+ }
+
+ // When an image is copied from a preview window, you end up with two
+ // DataTransferItems one of which is a file's metadata as text. Skip those.
+ if (this.isImage()) {
+ return false;
+ }
+
+ return this.types.some(type => RICH_TEXT_TYPES[type]);
+ }
+
+ /**
+ * Get raw text.
+ *
+ * @return {?string}
+ */
+ getText() {
+ var text;
+ if (this.data.getData) {
+ if (!this.types.length) {
+ text = this.data.getData('Text');
+ } else if (this.types.indexOf('text/plain') != -1) {
+ text = this.data.getData('text/plain');
+ }
+ }
+ return text ? text.replace(CR_LF_REGEX, LF_ONLY) : null;
+ }
+
+ /**
+ * Get HTML paste data
+ *
+ * @return {?string}
+ */
+ getHTML() {
+ if (this.data.getData) {
+ if (!this.types.length) {
+ return this.data.getData('Text');
+ } else if (this.types.indexOf('text/html') != -1) {
+ return this.data.getData('text/html');
+ }
+ }
+ }
+
+ /**
+ * Is this a link data transfer?
+ *
+ * @return {boolean}
+ */
+ isLink() {
+ return this.types.some(type => {
+ return type.indexOf('Url') != -1 || type.indexOf('text/uri-list') != -1 || type.indexOf('text/x-moz-url');
+ });
+ }
+
+ /**
+ * Get a link url.
+ *
+ * @return {?string}
+ */
+ getLink() {
+ if (this.data.getData) {
+ if (this.types.indexOf('text/x-moz-url') != -1) {
+ let url = this.data.getData('text/x-moz-url').split('\n');
+ return url[0];
+ }
+ return this.types.indexOf('text/uri-list') != -1 ? this.data.getData('text/uri-list') : this.data.getData('url');
+ }
+
+ return null;
+ }
+
+ /**
+ * Is this an image data transfer?
+ *
+ * @return {boolean}
+ */
+ isImage() {
+ var isImage = this.types.some(type => {
+ // Firefox will have a type of application/x-moz-file for images during
+ // dragging
+ return type.indexOf('application/x-moz-file') != -1;
+ });
+
+ if (isImage) {
+ return true;
+ }
+
+ var items = this.getFiles();
+ for (var i = 0; i < items.length; i++) {
+ var type = items[i].type;
+ if (!PhotosMimeType.isImage(type)) {
+ return false;
+ }
+ }
+
+ return true;
+ }
+
+ getCount() {
+ if (this.data.hasOwnProperty('items')) {
+ return this.data.items.length;
+ } else if (this.data.hasOwnProperty('mozItemCount')) {
+ return this.data.mozItemCount;
+ } else if (this.data.files) {
+ return this.data.files.length;
+ }
+ return null;
+ }
+
+ /**
+ * Get files.
+ *
+ * @return {array}
+ */
+ getFiles() {
+ if (this.data.items) {
+ // createArrayFromMixed doesn't properly handle DataTransferItemLists.
+ return Array.prototype.slice.call(this.data.items).map(getFileFromDataTransfer).filter(emptyFunction.thatReturnsArgument);
+ } else if (this.data.files) {
+ return Array.prototype.slice.call(this.data.files);
+ } else {
+ return [];
+ }
+ }
+
+ /**
+ * Are there any files to fetch?
+ *
+ * @return {boolean}
+ */
+ hasFiles() {
+ return this.getFiles().length > 0;
+ }
+}
+
+module.exports = DataTransfer;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Deferred.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Deferred.js
new file mode 100644
index 00000000..8ec65ccf
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Deferred.js
@@ -0,0 +1,79 @@
+"use strict";
+
+var Promise = require("./Promise");
+
+function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @typechecks
+ *
+ */
+
+/**
+ * Deferred provides a Promise-like API that exposes methods to resolve and
+ * reject the Promise. It is most useful when converting non-Promise code to use
+ * Promises.
+ *
+ * If you want to export the Promise without exposing access to the resolve and
+ * reject methods, you should export `getPromise` which returns a Promise with
+ * the same semantics excluding those methods.
+ */
+var Deferred = function () {
+ function Deferred() {
+ var _this = this;
+
+ _classCallCheck(this, Deferred);
+
+ this._settled = false;
+ this._promise = new Promise(function (resolve, reject) {
+ _this._resolve = resolve;
+ _this._reject = reject;
+ });
+ }
+
+ Deferred.prototype.getPromise = function getPromise() {
+ return this._promise;
+ };
+
+ Deferred.prototype.resolve = function resolve(value) {
+ this._settled = true;
+ this._resolve(value);
+ };
+
+ Deferred.prototype.reject = function reject(reason) {
+ this._settled = true;
+ this._reject(reason);
+ };
+
+ Deferred.prototype["catch"] = function _catch(onReject) {
+ return Promise.prototype["catch"].apply(this._promise, arguments);
+ };
+
+ Deferred.prototype.then = function then(onFulfill, onReject) {
+ return Promise.prototype.then.apply(this._promise, arguments);
+ };
+
+ Deferred.prototype.done = function done(onFulfill, onReject) {
+ // Embed the polyfill for the non-standard Promise.prototype.done so that
+ // users of the open source fbjs don't need a custom lib for Promise
+ var promise = arguments.length ? this._promise.then.apply(this._promise, arguments) : this._promise;
+ promise.then(undefined, function (err) {
+ setTimeout(function () {
+ throw err;
+ }, 0);
+ });
+ };
+
+ Deferred.prototype.isSettled = function isSettled() {
+ return this._settled;
+ };
+
+ return Deferred;
+}();
+
+module.exports = Deferred;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Deferred.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Deferred.js.flow
new file mode 100644
index 00000000..26bce875
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Deferred.js.flow
@@ -0,0 +1,73 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule Deferred
+ * @typechecks
+ * @flow
+ */
+
+/**
+ * Deferred provides a Promise-like API that exposes methods to resolve and
+ * reject the Promise. It is most useful when converting non-Promise code to use
+ * Promises.
+ *
+ * If you want to export the Promise without exposing access to the resolve and
+ * reject methods, you should export `getPromise` which returns a Promise with
+ * the same semantics excluding those methods.
+ */
+class Deferred {
+ _settled: boolean;
+ _promise: Promise;
+ _resolve: (value: Tvalue) => void;
+ _reject: (reason: Treason) => void;
+
+ constructor() {
+ this._settled = false;
+ this._promise = new Promise((resolve, reject) => {
+ this._resolve = (resolve: any);
+ this._reject = (reject: any);
+ });
+ }
+
+ getPromise(): Promise {
+ return this._promise;
+ }
+
+ resolve(value: Tvalue): void {
+ this._settled = true;
+ this._resolve(value);
+ }
+
+ reject(reason: Treason): void {
+ this._settled = true;
+ this._reject(reason);
+ }
+
+ catch(onReject?: ?(error: any) => mixed): Promise {
+ return Promise.prototype.catch.apply(this._promise, arguments);
+ }
+
+ then(onFulfill?: ?(value: any) => mixed, onReject?: ?(error: any) => mixed): Promise {
+ return Promise.prototype.then.apply(this._promise, arguments);
+ }
+
+ done(onFulfill?: ?(value: any) => mixed, onReject?: ?(error: any) => mixed): void {
+ // Embed the polyfill for the non-standard Promise.prototype.done so that
+ // users of the open source fbjs don't need a custom lib for Promise
+ const promise = arguments.length ? this._promise.then.apply(this._promise, arguments) : this._promise;
+ promise.then(undefined, function (err) {
+ setTimeout(function () {
+ throw err;
+ }, 0);
+ });
+ }
+
+ isSettled(): boolean {
+ return this._settled;
+ }
+}
+
+module.exports = Deferred;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/ErrorUtils.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/ErrorUtils.js
new file mode 100644
index 00000000..0ebdcbc4
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/ErrorUtils.js
@@ -0,0 +1,26 @@
+"use strict";
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ */
+
+/* jslint unused:false */
+
+if (global.ErrorUtils) {
+ module.exports = global.ErrorUtils;
+} else {
+ var ErrorUtils = {
+ applyWithGuard: function applyWithGuard(callback, context, args, onError, name) {
+ return callback.apply(context, args);
+ },
+ guard: function guard(callback, name) {
+ return callback;
+ }
+ };
+
+ module.exports = ErrorUtils;
+}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/ErrorUtils.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/ErrorUtils.js.flow
new file mode 100644
index 00000000..8a8e5cd4
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/ErrorUtils.js.flow
@@ -0,0 +1,25 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule ErrorUtils
+ */
+
+/* jslint unused:false */
+
+if (global.ErrorUtils) {
+ module.exports = global.ErrorUtils;
+} else {
+ var ErrorUtils = {
+ applyWithGuard(callback, context, args, onError, name) {
+ return callback.apply(context, args);
+ },
+ guard(callback, name) {
+ return callback;
+ }
+ };
+
+ module.exports = ErrorUtils;
+}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/EventListener.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/EventListener.js
new file mode 100644
index 00000000..61c2220c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/EventListener.js
@@ -0,0 +1,74 @@
+'use strict';
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @typechecks
+ */
+
+var emptyFunction = require('./emptyFunction');
+
+/**
+ * Upstream version of event listener. Does not take into account specific
+ * nature of platform.
+ */
+var EventListener = {
+ /**
+ * Listen to DOM events during the bubble phase.
+ *
+ * @param {DOMEventTarget} target DOM element to register listener on.
+ * @param {string} eventType Event type, e.g. 'click' or 'mouseover'.
+ * @param {function} callback Callback function.
+ * @return {object} Object with a `remove` method.
+ */
+ listen: function listen(target, eventType, callback) {
+ if (target.addEventListener) {
+ target.addEventListener(eventType, callback, false);
+ return {
+ remove: function remove() {
+ target.removeEventListener(eventType, callback, false);
+ }
+ };
+ } else if (target.attachEvent) {
+ target.attachEvent('on' + eventType, callback);
+ return {
+ remove: function remove() {
+ target.detachEvent('on' + eventType, callback);
+ }
+ };
+ }
+ },
+
+ /**
+ * Listen to DOM events during the capture phase.
+ *
+ * @param {DOMEventTarget} target DOM element to register listener on.
+ * @param {string} eventType Event type, e.g. 'click' or 'mouseover'.
+ * @param {function} callback Callback function.
+ * @return {object} Object with a `remove` method.
+ */
+ capture: function capture(target, eventType, callback) {
+ if (target.addEventListener) {
+ target.addEventListener(eventType, callback, true);
+ return {
+ remove: function remove() {
+ target.removeEventListener(eventType, callback, true);
+ }
+ };
+ } else {
+ if (process.env.NODE_ENV !== 'production') {
+ console.error('Attempted to listen to events during the capture phase on a ' + 'browser that does not support the capture phase. Your application ' + 'will not receive some events.');
+ }
+ return {
+ remove: emptyFunction
+ };
+ }
+ },
+
+ registerDefault: function registerDefault() {}
+};
+
+module.exports = EventListener;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/EventListener.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/EventListener.js.flow
new file mode 100644
index 00000000..283a3fa9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/EventListener.js.flow
@@ -0,0 +1,73 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule EventListener
+ * @typechecks
+ */
+
+var emptyFunction = require('./emptyFunction');
+
+/**
+ * Upstream version of event listener. Does not take into account specific
+ * nature of platform.
+ */
+var EventListener = {
+ /**
+ * Listen to DOM events during the bubble phase.
+ *
+ * @param {DOMEventTarget} target DOM element to register listener on.
+ * @param {string} eventType Event type, e.g. 'click' or 'mouseover'.
+ * @param {function} callback Callback function.
+ * @return {object} Object with a `remove` method.
+ */
+ listen: function (target, eventType, callback) {
+ if (target.addEventListener) {
+ target.addEventListener(eventType, callback, false);
+ return {
+ remove: function () {
+ target.removeEventListener(eventType, callback, false);
+ }
+ };
+ } else if (target.attachEvent) {
+ target.attachEvent('on' + eventType, callback);
+ return {
+ remove: function () {
+ target.detachEvent('on' + eventType, callback);
+ }
+ };
+ }
+ },
+
+ /**
+ * Listen to DOM events during the capture phase.
+ *
+ * @param {DOMEventTarget} target DOM element to register listener on.
+ * @param {string} eventType Event type, e.g. 'click' or 'mouseover'.
+ * @param {function} callback Callback function.
+ * @return {object} Object with a `remove` method.
+ */
+ capture: function (target, eventType, callback) {
+ if (target.addEventListener) {
+ target.addEventListener(eventType, callback, true);
+ return {
+ remove: function () {
+ target.removeEventListener(eventType, callback, true);
+ }
+ };
+ } else {
+ if (__DEV__) {
+ console.error('Attempted to listen to events during the capture phase on a ' + 'browser that does not support the capture phase. Your application ' + 'will not receive some events.');
+ }
+ return {
+ remove: emptyFunction
+ };
+ }
+ },
+
+ registerDefault: function () {}
+};
+
+module.exports = EventListener;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/ExecutionEnvironment.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/ExecutionEnvironment.js
new file mode 100644
index 00000000..32936fdc
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/ExecutionEnvironment.js
@@ -0,0 +1,33 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ */
+
+'use strict';
+
+var canUseDOM = !!(typeof window !== 'undefined' && window.document && window.document.createElement);
+
+/**
+ * Simple, lightweight module assisting with the detection and context of
+ * Worker. Helps avoid circular dependencies and allows code to reason about
+ * whether or not they are in a Worker, even if they never include the main
+ * `ReactWorker` dependency.
+ */
+var ExecutionEnvironment = {
+
+ canUseDOM: canUseDOM,
+
+ canUseWorkers: typeof Worker !== 'undefined',
+
+ canUseEventListeners: canUseDOM && !!(window.addEventListener || window.attachEvent),
+
+ canUseViewport: canUseDOM && !!window.screen,
+
+ isInWorker: !canUseDOM // For now, this is true - might change in the future.
+
+};
+
+module.exports = ExecutionEnvironment;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/ExecutionEnvironment.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/ExecutionEnvironment.js.flow
new file mode 100644
index 00000000..fbea7e1b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/ExecutionEnvironment.js.flow
@@ -0,0 +1,34 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule ExecutionEnvironment
+ */
+
+'use strict';
+
+const canUseDOM = !!(typeof window !== 'undefined' && window.document && window.document.createElement);
+
+/**
+ * Simple, lightweight module assisting with the detection and context of
+ * Worker. Helps avoid circular dependencies and allows code to reason about
+ * whether or not they are in a Worker, even if they never include the main
+ * `ReactWorker` dependency.
+ */
+const ExecutionEnvironment = {
+
+ canUseDOM: canUseDOM,
+
+ canUseWorkers: typeof Worker !== 'undefined',
+
+ canUseEventListeners: canUseDOM && !!(window.addEventListener || window.attachEvent),
+
+ canUseViewport: canUseDOM && !!window.screen,
+
+ isInWorker: !canUseDOM // For now, this is true - might change in the future.
+
+};
+
+module.exports = ExecutionEnvironment;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Keys.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Keys.js
new file mode 100644
index 00000000..f2f0d9ca
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Keys.js
@@ -0,0 +1,34 @@
+"use strict";
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ */
+
+module.exports = {
+ BACKSPACE: 8,
+ TAB: 9,
+ RETURN: 13,
+ ALT: 18,
+ ESC: 27,
+ SPACE: 32,
+ PAGE_UP: 33,
+ PAGE_DOWN: 34,
+ END: 35,
+ HOME: 36,
+ LEFT: 37,
+ UP: 38,
+ RIGHT: 39,
+ DOWN: 40,
+ DELETE: 46,
+ COMMA: 188,
+ PERIOD: 190,
+ A: 65,
+ Z: 90,
+ ZERO: 48,
+ NUMPAD_0: 96,
+ NUMPAD_9: 105
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Keys.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Keys.js.flow
new file mode 100644
index 00000000..22f57f38
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Keys.js.flow
@@ -0,0 +1,33 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule Keys
+ */
+
+module.exports = {
+ BACKSPACE: 8,
+ TAB: 9,
+ RETURN: 13,
+ ALT: 18,
+ ESC: 27,
+ SPACE: 32,
+ PAGE_UP: 33,
+ PAGE_DOWN: 34,
+ END: 35,
+ HOME: 36,
+ LEFT: 37,
+ UP: 38,
+ RIGHT: 39,
+ DOWN: 40,
+ DELETE: 46,
+ COMMA: 188,
+ PERIOD: 190,
+ A: 65,
+ Z: 90,
+ ZERO: 48,
+ NUMPAD_0: 96,
+ NUMPAD_9: 105
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Map.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Map.js
new file mode 100644
index 00000000..c224b891
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Map.js
@@ -0,0 +1,11 @@
+'use strict';
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ */
+
+module.exports = require('core-js/library/es6/map');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Map.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Map.js.flow
new file mode 100644
index 00000000..f0a6e2c1
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Map.js.flow
@@ -0,0 +1,10 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule Map
+ */
+
+module.exports = require('core-js/library/es6/map');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/PhotosMimeType.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/PhotosMimeType.js
new file mode 100644
index 00000000..467892a7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/PhotosMimeType.js
@@ -0,0 +1,26 @@
+'use strict';
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ */
+var PhotosMimeType = {
+ isImage: function isImage(mimeString) {
+ return getParts(mimeString)[0] === 'image';
+ },
+ isJpeg: function isJpeg(mimeString) {
+ var parts = getParts(mimeString);
+ return PhotosMimeType.isImage(mimeString) && (
+ // see http://fburl.com/10972194
+ parts[1] === 'jpeg' || parts[1] === 'pjpeg');
+ }
+};
+
+function getParts(mimeString) {
+ return mimeString.split('/');
+}
+
+module.exports = PhotosMimeType;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/PhotosMimeType.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/PhotosMimeType.js.flow
new file mode 100644
index 00000000..a6c3ae13
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/PhotosMimeType.js.flow
@@ -0,0 +1,26 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule PhotosMimeType
+ */
+const PhotosMimeType = {
+ isImage(mimeString) {
+ return getParts(mimeString)[0] === 'image';
+ },
+
+ isJpeg(mimeString) {
+ const parts = getParts(mimeString);
+ return PhotosMimeType.isImage(mimeString) && (
+ // see http://fburl.com/10972194
+ parts[1] === 'jpeg' || parts[1] === 'pjpeg');
+ }
+};
+
+function getParts(mimeString) {
+ return mimeString.split('/');
+}
+
+module.exports = PhotosMimeType;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Promise.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Promise.js
new file mode 100644
index 00000000..3ce10a0e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Promise.js
@@ -0,0 +1,11 @@
+'use strict';
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ */
+
+module.exports = require('promise');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Promise.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Promise.js.flow
new file mode 100644
index 00000000..1b421ef6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Promise.js.flow
@@ -0,0 +1,10 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule Promise
+ */
+
+module.exports = require('promise');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Promise.native.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Promise.native.js
new file mode 100644
index 00000000..27aa673f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Promise.native.js
@@ -0,0 +1,25 @@
+/**
+ *
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * This module wraps and augments the minimally ES6-compliant Promise
+ * implementation provided by the promise npm package.
+ *
+ */
+
+'use strict';
+
+var Promise = require('promise/setimmediate/es6-extensions');
+require('promise/setimmediate/done');
+
+/**
+ * Handle either fulfillment or rejection with the same callback.
+ */
+Promise.prototype['finally'] = function (onSettled) {
+ return this.then(onSettled, onSettled);
+};
+
+module.exports = Promise;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Promise.native.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Promise.native.js.flow
new file mode 100644
index 00000000..a988e5b3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Promise.native.js.flow
@@ -0,0 +1,25 @@
+/**
+ *
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * This module wraps and augments the minimally ES6-compliant Promise
+ * implementation provided by the promise npm package.
+ *
+ */
+
+'use strict';
+
+var Promise = require('promise/setimmediate/es6-extensions');
+require('promise/setimmediate/done');
+
+/**
+ * Handle either fulfillment or rejection with the same callback.
+ */
+Promise.prototype.finally = function (onSettled) {
+ return this.then(onSettled, onSettled);
+};
+
+module.exports = Promise;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/PromiseMap.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/PromiseMap.js
new file mode 100644
index 00000000..467060a8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/PromiseMap.js
@@ -0,0 +1,57 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ *
+ */
+
+'use strict';
+
+function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
+
+var Deferred = require('./Deferred');
+
+var invariant = require('./invariant');
+
+/**
+ * A map of asynchronous values that can be get or set in any order. Unlike a
+ * normal map, setting the value for a particular key more than once throws.
+ * Also unlike a normal map, a key can either be resolved or rejected.
+ */
+
+var PromiseMap = function () {
+ function PromiseMap() {
+ _classCallCheck(this, PromiseMap);
+
+ this._deferred = {};
+ }
+
+ PromiseMap.prototype.get = function get(key) {
+ return getDeferred(this._deferred, key).getPromise();
+ };
+
+ PromiseMap.prototype.resolveKey = function resolveKey(key, value) {
+ var entry = getDeferred(this._deferred, key);
+ !!entry.isSettled() ? process.env.NODE_ENV !== 'production' ? invariant(false, 'PromiseMap: Already settled `%s`.', key) : invariant(false) : void 0;
+ entry.resolve(value);
+ };
+
+ PromiseMap.prototype.rejectKey = function rejectKey(key, reason) {
+ var entry = getDeferred(this._deferred, key);
+ !!entry.isSettled() ? process.env.NODE_ENV !== 'production' ? invariant(false, 'PromiseMap: Already settled `%s`.', key) : invariant(false) : void 0;
+ entry.reject(reason);
+ };
+
+ return PromiseMap;
+}();
+
+function getDeferred(entries, key) {
+ if (!entries.hasOwnProperty(key)) {
+ entries[key] = new Deferred();
+ }
+ return entries[key];
+}
+
+module.exports = PromiseMap;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/PromiseMap.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/PromiseMap.js.flow
new file mode 100644
index 00000000..5ae135a4
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/PromiseMap.js.flow
@@ -0,0 +1,53 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule PromiseMap
+ * @flow
+ */
+
+'use strict';
+
+const Deferred = require('./Deferred');
+
+const invariant = require('./invariant');
+
+/**
+ * A map of asynchronous values that can be get or set in any order. Unlike a
+ * normal map, setting the value for a particular key more than once throws.
+ * Also unlike a normal map, a key can either be resolved or rejected.
+ */
+class PromiseMap {
+ _deferred: { [key: string]: Deferred };
+
+ constructor() {
+ this._deferred = {};
+ }
+
+ get(key: string): Promise {
+ return getDeferred(this._deferred, key).getPromise();
+ }
+
+ resolveKey(key: string, value: Tvalue): void {
+ const entry = getDeferred(this._deferred, key);
+ invariant(!entry.isSettled(), 'PromiseMap: Already settled `%s`.', key);
+ entry.resolve(value);
+ }
+
+ rejectKey(key: string, reason: Treason): void {
+ const entry = getDeferred(this._deferred, key);
+ invariant(!entry.isSettled(), 'PromiseMap: Already settled `%s`.', key);
+ entry.reject(reason);
+ }
+}
+
+function getDeferred(entries: { [key: string]: Deferred }, key: string): Deferred {
+ if (!entries.hasOwnProperty(key)) {
+ entries[key] = new Deferred();
+ }
+ return entries[key];
+}
+
+module.exports = PromiseMap;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Scroll.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Scroll.js
new file mode 100644
index 00000000..85dd4fd2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Scroll.js
@@ -0,0 +1,83 @@
+"use strict";
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ */
+
+/**
+ * @param {DOMElement} element
+ * @param {DOMDocument} doc
+ * @return {boolean}
+ */
+function _isViewportScrollElement(element, doc) {
+ return !!doc && (element === doc.documentElement || element === doc.body);
+}
+
+/**
+ * Scroll Module. This class contains 4 simple static functions
+ * to be used to access Element.scrollTop/scrollLeft properties.
+ * To solve the inconsistencies between browsers when either
+ * document.body or document.documentElement is supplied,
+ * below logic will be used to alleviate the issue:
+ *
+ * 1. If 'element' is either 'document.body' or 'document.documentElement,
+ * get whichever element's 'scroll{Top,Left}' is larger.
+ * 2. If 'element' is either 'document.body' or 'document.documentElement',
+ * set the 'scroll{Top,Left}' on both elements.
+ */
+
+var Scroll = {
+ /**
+ * @param {DOMElement} element
+ * @return {number}
+ */
+ getTop: function getTop(element) {
+ var doc = element.ownerDocument;
+ return _isViewportScrollElement(element, doc) ?
+ // In practice, they will either both have the same value,
+ // or one will be zero and the other will be the scroll position
+ // of the viewport. So we can use `X || Y` instead of `Math.max(X, Y)`
+ doc.body.scrollTop || doc.documentElement.scrollTop : element.scrollTop;
+ },
+
+ /**
+ * @param {DOMElement} element
+ * @param {number} newTop
+ */
+ setTop: function setTop(element, newTop) {
+ var doc = element.ownerDocument;
+ if (_isViewportScrollElement(element, doc)) {
+ doc.body.scrollTop = doc.documentElement.scrollTop = newTop;
+ } else {
+ element.scrollTop = newTop;
+ }
+ },
+
+ /**
+ * @param {DOMElement} element
+ * @return {number}
+ */
+ getLeft: function getLeft(element) {
+ var doc = element.ownerDocument;
+ return _isViewportScrollElement(element, doc) ? doc.body.scrollLeft || doc.documentElement.scrollLeft : element.scrollLeft;
+ },
+
+ /**
+ * @param {DOMElement} element
+ * @param {number} newLeft
+ */
+ setLeft: function setLeft(element, newLeft) {
+ var doc = element.ownerDocument;
+ if (_isViewportScrollElement(element, doc)) {
+ doc.body.scrollLeft = doc.documentElement.scrollLeft = newLeft;
+ } else {
+ element.scrollLeft = newLeft;
+ }
+ }
+};
+
+module.exports = Scroll;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Scroll.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Scroll.js.flow
new file mode 100644
index 00000000..1d65bcbd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Scroll.js.flow
@@ -0,0 +1,82 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule Scroll
+ */
+
+/**
+ * @param {DOMElement} element
+ * @param {DOMDocument} doc
+ * @return {boolean}
+ */
+function _isViewportScrollElement(element, doc) {
+ return !!doc && (element === doc.documentElement || element === doc.body);
+}
+
+/**
+ * Scroll Module. This class contains 4 simple static functions
+ * to be used to access Element.scrollTop/scrollLeft properties.
+ * To solve the inconsistencies between browsers when either
+ * document.body or document.documentElement is supplied,
+ * below logic will be used to alleviate the issue:
+ *
+ * 1. If 'element' is either 'document.body' or 'document.documentElement,
+ * get whichever element's 'scroll{Top,Left}' is larger.
+ * 2. If 'element' is either 'document.body' or 'document.documentElement',
+ * set the 'scroll{Top,Left}' on both elements.
+ */
+
+const Scroll = {
+ /**
+ * @param {DOMElement} element
+ * @return {number}
+ */
+ getTop: function (element) {
+ const doc = element.ownerDocument;
+ return _isViewportScrollElement(element, doc) ?
+ // In practice, they will either both have the same value,
+ // or one will be zero and the other will be the scroll position
+ // of the viewport. So we can use `X || Y` instead of `Math.max(X, Y)`
+ doc.body.scrollTop || doc.documentElement.scrollTop : element.scrollTop;
+ },
+
+ /**
+ * @param {DOMElement} element
+ * @param {number} newTop
+ */
+ setTop: function (element, newTop) {
+ const doc = element.ownerDocument;
+ if (_isViewportScrollElement(element, doc)) {
+ doc.body.scrollTop = doc.documentElement.scrollTop = newTop;
+ } else {
+ element.scrollTop = newTop;
+ }
+ },
+
+ /**
+ * @param {DOMElement} element
+ * @return {number}
+ */
+ getLeft: function (element) {
+ const doc = element.ownerDocument;
+ return _isViewportScrollElement(element, doc) ? doc.body.scrollLeft || doc.documentElement.scrollLeft : element.scrollLeft;
+ },
+
+ /**
+ * @param {DOMElement} element
+ * @param {number} newLeft
+ */
+ setLeft: function (element, newLeft) {
+ const doc = element.ownerDocument;
+ if (_isViewportScrollElement(element, doc)) {
+ doc.body.scrollLeft = doc.documentElement.scrollLeft = newLeft;
+ } else {
+ element.scrollLeft = newLeft;
+ }
+ }
+};
+
+module.exports = Scroll;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Set.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Set.js
new file mode 100644
index 00000000..43470f1e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Set.js
@@ -0,0 +1,11 @@
+'use strict';
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ */
+
+module.exports = require('core-js/library/es6/set');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Set.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Set.js.flow
new file mode 100644
index 00000000..d1a48d90
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Set.js.flow
@@ -0,0 +1,10 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule Set
+ */
+
+module.exports = require('core-js/library/es6/set');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Style.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Style.js
new file mode 100644
index 00000000..28071b91
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Style.js
@@ -0,0 +1,62 @@
+'use strict';
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @typechecks
+ */
+
+var getStyleProperty = require('./getStyleProperty');
+
+/**
+ * @param {DOMNode} element [description]
+ * @param {string} name Overflow style property name.
+ * @return {boolean} True if the supplied ndoe is scrollable.
+ */
+function _isNodeScrollable(element, name) {
+ var overflow = Style.get(element, name);
+ return overflow === 'auto' || overflow === 'scroll';
+}
+
+/**
+ * Utilities for querying and mutating style properties.
+ */
+var Style = {
+ /**
+ * Gets the style property for the supplied node. This will return either the
+ * computed style, if available, or the declared style.
+ *
+ * @param {DOMNode} node
+ * @param {string} name Style property name.
+ * @return {?string} Style property value.
+ */
+ get: getStyleProperty,
+
+ /**
+ * Determines the nearest ancestor of a node that is scrollable.
+ *
+ * NOTE: This can be expensive if used repeatedly or on a node nested deeply.
+ *
+ * @param {?DOMNode} node Node from which to start searching.
+ * @return {?DOMWindow|DOMElement} Scroll parent of the supplied node.
+ */
+ getScrollParent: function getScrollParent(node) {
+ if (!node) {
+ return null;
+ }
+ var ownerDocument = node.ownerDocument;
+ while (node && node !== ownerDocument.body) {
+ if (_isNodeScrollable(node, 'overflow') || _isNodeScrollable(node, 'overflowY') || _isNodeScrollable(node, 'overflowX')) {
+ return node;
+ }
+ node = node.parentNode;
+ }
+ return ownerDocument.defaultView || ownerDocument.parentWindow;
+ }
+
+};
+
+module.exports = Style;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Style.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Style.js.flow
new file mode 100644
index 00000000..d90db8c3
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/Style.js.flow
@@ -0,0 +1,61 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule Style
+ * @typechecks
+ */
+
+var getStyleProperty = require('./getStyleProperty');
+
+/**
+ * @param {DOMNode} element [description]
+ * @param {string} name Overflow style property name.
+ * @return {boolean} True if the supplied ndoe is scrollable.
+ */
+function _isNodeScrollable(element, name) {
+ var overflow = Style.get(element, name);
+ return overflow === 'auto' || overflow === 'scroll';
+}
+
+/**
+ * Utilities for querying and mutating style properties.
+ */
+var Style = {
+ /**
+ * Gets the style property for the supplied node. This will return either the
+ * computed style, if available, or the declared style.
+ *
+ * @param {DOMNode} node
+ * @param {string} name Style property name.
+ * @return {?string} Style property value.
+ */
+ get: getStyleProperty,
+
+ /**
+ * Determines the nearest ancestor of a node that is scrollable.
+ *
+ * NOTE: This can be expensive if used repeatedly or on a node nested deeply.
+ *
+ * @param {?DOMNode} node Node from which to start searching.
+ * @return {?DOMWindow|DOMElement} Scroll parent of the supplied node.
+ */
+ getScrollParent: function (node) {
+ if (!node) {
+ return null;
+ }
+ var ownerDocument = node.ownerDocument;
+ while (node && node !== ownerDocument.body) {
+ if (_isNodeScrollable(node, 'overflow') || _isNodeScrollable(node, 'overflowY') || _isNodeScrollable(node, 'overflowX')) {
+ return node;
+ }
+ node = node.parentNode;
+ }
+ return ownerDocument.defaultView || ownerDocument.parentWindow;
+ }
+
+};
+
+module.exports = Style;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/TokenizeUtil.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/TokenizeUtil.js
new file mode 100644
index 00000000..74567c87
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/TokenizeUtil.js
@@ -0,0 +1,35 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @typechecks
+ * @stub
+ *
+ */
+
+'use strict';
+
+// \u00a1-\u00b1\u00b4-\u00b8\u00ba\u00bb\u00bf
+// is latin supplement punctuation except fractions and superscript
+// numbers
+// \u2010-\u2027\u2030-\u205e
+// is punctuation from the general punctuation block:
+// weird quotes, commas, bullets, dashes, etc.
+// \u30fb\u3001\u3002\u3008-\u3011\u3014-\u301f
+// is CJK punctuation
+// \uff1a-\uff1f\uff01-\uff0f\uff3b-\uff40\uff5b-\uff65
+// is some full-width/half-width punctuation
+// \u2E2E\u061f\u066a-\u066c\u061b\u060c\u060d\uFD3e\uFD3F
+// is some Arabic punctuation marks
+// \u1801\u0964\u104a\u104b
+// is misc. other language punctuation marks
+
+var PUNCTUATION = '[.,+*?$|#{}()\'\\^\\-\\[\\]\\\\\\/!@%"~=<>_:;' + '\u30FB\u3001\u3002\u3008-\u3011\u3014-\u301F\uFF1A-\uFF1F\uFF01-\uFF0F' + '\uFF3B-\uFF40\uFF5B-\uFF65\u2E2E\u061F\u066A-\u066C\u061B\u060C\u060D' + '\uFD3E\uFD3F\u1801\u0964\u104A\u104B\u2010-\u2027\u2030-\u205E' + '\xA1-\xB1\xB4-\xB8\xBA\xBB\xBF]';
+
+module.exports = {
+ getPunctuation: function getPunctuation() {
+ return PUNCTUATION;
+ }
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/TokenizeUtil.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/TokenizeUtil.js.flow
new file mode 100644
index 00000000..642590ad
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/TokenizeUtil.js.flow
@@ -0,0 +1,34 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule TokenizeUtil
+ * @typechecks
+ * @stub
+ * @flow
+ */
+
+'use strict';
+
+// \u00a1-\u00b1\u00b4-\u00b8\u00ba\u00bb\u00bf
+// is latin supplement punctuation except fractions and superscript
+// numbers
+// \u2010-\u2027\u2030-\u205e
+// is punctuation from the general punctuation block:
+// weird quotes, commas, bullets, dashes, etc.
+// \u30fb\u3001\u3002\u3008-\u3011\u3014-\u301f
+// is CJK punctuation
+// \uff1a-\uff1f\uff01-\uff0f\uff3b-\uff40\uff5b-\uff65
+// is some full-width/half-width punctuation
+// \u2E2E\u061f\u066a-\u066c\u061b\u060c\u060d\uFD3e\uFD3F
+// is some Arabic punctuation marks
+// \u1801\u0964\u104a\u104b
+// is misc. other language punctuation marks
+
+var PUNCTUATION = '[.,+*?$|#{}()\'\\^\\-\\[\\]\\\\\\/!@%"~=<>_:;' + '\u30fb\u3001\u3002\u3008-\u3011\u3014-\u301f\uff1a-\uff1f\uff01-\uff0f' + '\uff3b-\uff40\uff5b-\uff65\u2E2E\u061f\u066a-\u066c\u061b\u060c\u060d' + '\uFD3e\uFD3F\u1801\u0964\u104a\u104b\u2010-\u2027\u2030-\u205e' + '\u00a1-\u00b1\u00b4-\u00b8\u00ba\u00bb\u00bf]';
+
+module.exports = {
+ getPunctuation: (): string => PUNCTUATION
+};
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/TouchEventUtils.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/TouchEventUtils.js
new file mode 100644
index 00000000..1125d80c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/TouchEventUtils.js
@@ -0,0 +1,32 @@
+"use strict";
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ */
+
+var TouchEventUtils = {
+ /**
+ * Utility function for common case of extracting out the primary touch from a
+ * touch event.
+ * - `touchEnd` events usually do not have the `touches` property.
+ * http://stackoverflow.com/questions/3666929/
+ * mobile-sarai-touchend-event-not-firing-when-last-touch-is-removed
+ *
+ * @param {Event} nativeEvent Native event that may or may not be a touch.
+ * @return {TouchesObject?} an object with pageX and pageY or null.
+ */
+ extractSingleTouch: function extractSingleTouch(nativeEvent) {
+ var touches = nativeEvent.touches;
+ var changedTouches = nativeEvent.changedTouches;
+ var hasTouches = touches && touches.length > 0;
+ var hasChangedTouches = changedTouches && changedTouches.length > 0;
+
+ return !hasTouches && hasChangedTouches ? changedTouches[0] : hasTouches ? touches[0] : nativeEvent;
+ }
+};
+
+module.exports = TouchEventUtils;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/TouchEventUtils.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/TouchEventUtils.js.flow
new file mode 100644
index 00000000..cff75f16
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/TouchEventUtils.js.flow
@@ -0,0 +1,31 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule TouchEventUtils
+ */
+
+const TouchEventUtils = {
+ /**
+ * Utility function for common case of extracting out the primary touch from a
+ * touch event.
+ * - `touchEnd` events usually do not have the `touches` property.
+ * http://stackoverflow.com/questions/3666929/
+ * mobile-sarai-touchend-event-not-firing-when-last-touch-is-removed
+ *
+ * @param {Event} nativeEvent Native event that may or may not be a touch.
+ * @return {TouchesObject?} an object with pageX and pageY or null.
+ */
+ extractSingleTouch: function (nativeEvent) {
+ const touches = nativeEvent.touches;
+ const changedTouches = nativeEvent.changedTouches;
+ const hasTouches = touches && touches.length > 0;
+ const hasChangedTouches = changedTouches && changedTouches.length > 0;
+
+ return !hasTouches && hasChangedTouches ? changedTouches[0] : hasTouches ? touches[0] : nativeEvent;
+ }
+};
+
+module.exports = TouchEventUtils;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/URI.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/URI.js
new file mode 100644
index 00000000..fdd0d7ba
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/URI.js
@@ -0,0 +1,28 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ *
+ */
+
+'use strict';
+
+function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
+
+var URI = function () {
+ function URI(uri) {
+ _classCallCheck(this, URI);
+
+ this._uri = uri;
+ }
+
+ URI.prototype.toString = function toString() {
+ return this._uri;
+ };
+
+ return URI;
+}();
+
+module.exports = URI;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/URI.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/URI.js.flow
new file mode 100644
index 00000000..e5988e9e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/URI.js.flow
@@ -0,0 +1,25 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule URI
+ * @flow
+ */
+
+'use strict';
+
+class URI {
+ _uri: string;
+
+ constructor(uri: string) {
+ this._uri = uri;
+ }
+
+ toString(): string {
+ return this._uri;
+ }
+}
+
+module.exports = URI;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeBidi.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeBidi.js
new file mode 100644
index 00000000..2cfbc586
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeBidi.js
@@ -0,0 +1,154 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @typechecks
+ *
+ */
+
+/**
+ * Basic (stateless) API for text direction detection
+ *
+ * Part of our implementation of Unicode Bidirectional Algorithm (UBA)
+ * Unicode Standard Annex #9 (UAX9)
+ * http://www.unicode.org/reports/tr9/
+ */
+
+'use strict';
+
+var UnicodeBidiDirection = require('./UnicodeBidiDirection');
+
+var invariant = require('./invariant');
+
+/**
+ * RegExp ranges of characters with a *Strong* Bidi_Class value.
+ *
+ * Data is based on DerivedBidiClass.txt in UCD version 7.0.0.
+ *
+ * NOTE: For performance reasons, we only support Unicode's
+ * Basic Multilingual Plane (BMP) for now.
+ */
+var RANGE_BY_BIDI_TYPE = {
+
+ L: 'A-Za-z\xAA\xB5\xBA\xC0-\xD6\xD8-\xF6\xF8-\u01BA\u01BB' + '\u01BC-\u01BF\u01C0-\u01C3\u01C4-\u0293\u0294\u0295-\u02AF\u02B0-\u02B8' + '\u02BB-\u02C1\u02D0-\u02D1\u02E0-\u02E4\u02EE\u0370-\u0373\u0376-\u0377' + '\u037A\u037B-\u037D\u037F\u0386\u0388-\u038A\u038C\u038E-\u03A1' + '\u03A3-\u03F5\u03F7-\u0481\u0482\u048A-\u052F\u0531-\u0556\u0559' + '\u055A-\u055F\u0561-\u0587\u0589\u0903\u0904-\u0939\u093B\u093D' + '\u093E-\u0940\u0949-\u094C\u094E-\u094F\u0950\u0958-\u0961\u0964-\u0965' + '\u0966-\u096F\u0970\u0971\u0972-\u0980\u0982-\u0983\u0985-\u098C' + '\u098F-\u0990\u0993-\u09A8\u09AA-\u09B0\u09B2\u09B6-\u09B9\u09BD' + '\u09BE-\u09C0\u09C7-\u09C8\u09CB-\u09CC\u09CE\u09D7\u09DC-\u09DD' + '\u09DF-\u09E1\u09E6-\u09EF\u09F0-\u09F1\u09F4-\u09F9\u09FA\u0A03' + '\u0A05-\u0A0A\u0A0F-\u0A10\u0A13-\u0A28\u0A2A-\u0A30\u0A32-\u0A33' + '\u0A35-\u0A36\u0A38-\u0A39\u0A3E-\u0A40\u0A59-\u0A5C\u0A5E\u0A66-\u0A6F' + '\u0A72-\u0A74\u0A83\u0A85-\u0A8D\u0A8F-\u0A91\u0A93-\u0AA8\u0AAA-\u0AB0' + '\u0AB2-\u0AB3\u0AB5-\u0AB9\u0ABD\u0ABE-\u0AC0\u0AC9\u0ACB-\u0ACC\u0AD0' + '\u0AE0-\u0AE1\u0AE6-\u0AEF\u0AF0\u0B02-\u0B03\u0B05-\u0B0C\u0B0F-\u0B10' + '\u0B13-\u0B28\u0B2A-\u0B30\u0B32-\u0B33\u0B35-\u0B39\u0B3D\u0B3E\u0B40' + '\u0B47-\u0B48\u0B4B-\u0B4C\u0B57\u0B5C-\u0B5D\u0B5F-\u0B61\u0B66-\u0B6F' + '\u0B70\u0B71\u0B72-\u0B77\u0B83\u0B85-\u0B8A\u0B8E-\u0B90\u0B92-\u0B95' + '\u0B99-\u0B9A\u0B9C\u0B9E-\u0B9F\u0BA3-\u0BA4\u0BA8-\u0BAA\u0BAE-\u0BB9' + '\u0BBE-\u0BBF\u0BC1-\u0BC2\u0BC6-\u0BC8\u0BCA-\u0BCC\u0BD0\u0BD7' + '\u0BE6-\u0BEF\u0BF0-\u0BF2\u0C01-\u0C03\u0C05-\u0C0C\u0C0E-\u0C10' + '\u0C12-\u0C28\u0C2A-\u0C39\u0C3D\u0C41-\u0C44\u0C58-\u0C59\u0C60-\u0C61' + '\u0C66-\u0C6F\u0C7F\u0C82-\u0C83\u0C85-\u0C8C\u0C8E-\u0C90\u0C92-\u0CA8' + '\u0CAA-\u0CB3\u0CB5-\u0CB9\u0CBD\u0CBE\u0CBF\u0CC0-\u0CC4\u0CC6' + '\u0CC7-\u0CC8\u0CCA-\u0CCB\u0CD5-\u0CD6\u0CDE\u0CE0-\u0CE1\u0CE6-\u0CEF' + '\u0CF1-\u0CF2\u0D02-\u0D03\u0D05-\u0D0C\u0D0E-\u0D10\u0D12-\u0D3A\u0D3D' + '\u0D3E-\u0D40\u0D46-\u0D48\u0D4A-\u0D4C\u0D4E\u0D57\u0D60-\u0D61' + '\u0D66-\u0D6F\u0D70-\u0D75\u0D79\u0D7A-\u0D7F\u0D82-\u0D83\u0D85-\u0D96' + '\u0D9A-\u0DB1\u0DB3-\u0DBB\u0DBD\u0DC0-\u0DC6\u0DCF-\u0DD1\u0DD8-\u0DDF' + '\u0DE6-\u0DEF\u0DF2-\u0DF3\u0DF4\u0E01-\u0E30\u0E32-\u0E33\u0E40-\u0E45' + '\u0E46\u0E4F\u0E50-\u0E59\u0E5A-\u0E5B\u0E81-\u0E82\u0E84\u0E87-\u0E88' + '\u0E8A\u0E8D\u0E94-\u0E97\u0E99-\u0E9F\u0EA1-\u0EA3\u0EA5\u0EA7' + '\u0EAA-\u0EAB\u0EAD-\u0EB0\u0EB2-\u0EB3\u0EBD\u0EC0-\u0EC4\u0EC6' + '\u0ED0-\u0ED9\u0EDC-\u0EDF\u0F00\u0F01-\u0F03\u0F04-\u0F12\u0F13\u0F14' + '\u0F15-\u0F17\u0F1A-\u0F1F\u0F20-\u0F29\u0F2A-\u0F33\u0F34\u0F36\u0F38' + '\u0F3E-\u0F3F\u0F40-\u0F47\u0F49-\u0F6C\u0F7F\u0F85\u0F88-\u0F8C' + '\u0FBE-\u0FC5\u0FC7-\u0FCC\u0FCE-\u0FCF\u0FD0-\u0FD4\u0FD5-\u0FD8' + '\u0FD9-\u0FDA\u1000-\u102A\u102B-\u102C\u1031\u1038\u103B-\u103C\u103F' + '\u1040-\u1049\u104A-\u104F\u1050-\u1055\u1056-\u1057\u105A-\u105D\u1061' + '\u1062-\u1064\u1065-\u1066\u1067-\u106D\u106E-\u1070\u1075-\u1081' + '\u1083-\u1084\u1087-\u108C\u108E\u108F\u1090-\u1099\u109A-\u109C' + '\u109E-\u109F\u10A0-\u10C5\u10C7\u10CD\u10D0-\u10FA\u10FB\u10FC' + '\u10FD-\u1248\u124A-\u124D\u1250-\u1256\u1258\u125A-\u125D\u1260-\u1288' + '\u128A-\u128D\u1290-\u12B0\u12B2-\u12B5\u12B8-\u12BE\u12C0\u12C2-\u12C5' + '\u12C8-\u12D6\u12D8-\u1310\u1312-\u1315\u1318-\u135A\u1360-\u1368' + '\u1369-\u137C\u1380-\u138F\u13A0-\u13F4\u1401-\u166C\u166D-\u166E' + '\u166F-\u167F\u1681-\u169A\u16A0-\u16EA\u16EB-\u16ED\u16EE-\u16F0' + '\u16F1-\u16F8\u1700-\u170C\u170E-\u1711\u1720-\u1731\u1735-\u1736' + '\u1740-\u1751\u1760-\u176C\u176E-\u1770\u1780-\u17B3\u17B6\u17BE-\u17C5' + '\u17C7-\u17C8\u17D4-\u17D6\u17D7\u17D8-\u17DA\u17DC\u17E0-\u17E9' + '\u1810-\u1819\u1820-\u1842\u1843\u1844-\u1877\u1880-\u18A8\u18AA' + '\u18B0-\u18F5\u1900-\u191E\u1923-\u1926\u1929-\u192B\u1930-\u1931' + '\u1933-\u1938\u1946-\u194F\u1950-\u196D\u1970-\u1974\u1980-\u19AB' + '\u19B0-\u19C0\u19C1-\u19C7\u19C8-\u19C9\u19D0-\u19D9\u19DA\u1A00-\u1A16' + '\u1A19-\u1A1A\u1A1E-\u1A1F\u1A20-\u1A54\u1A55\u1A57\u1A61\u1A63-\u1A64' + '\u1A6D-\u1A72\u1A80-\u1A89\u1A90-\u1A99\u1AA0-\u1AA6\u1AA7\u1AA8-\u1AAD' + '\u1B04\u1B05-\u1B33\u1B35\u1B3B\u1B3D-\u1B41\u1B43-\u1B44\u1B45-\u1B4B' + '\u1B50-\u1B59\u1B5A-\u1B60\u1B61-\u1B6A\u1B74-\u1B7C\u1B82\u1B83-\u1BA0' + '\u1BA1\u1BA6-\u1BA7\u1BAA\u1BAE-\u1BAF\u1BB0-\u1BB9\u1BBA-\u1BE5\u1BE7' + '\u1BEA-\u1BEC\u1BEE\u1BF2-\u1BF3\u1BFC-\u1BFF\u1C00-\u1C23\u1C24-\u1C2B' + '\u1C34-\u1C35\u1C3B-\u1C3F\u1C40-\u1C49\u1C4D-\u1C4F\u1C50-\u1C59' + '\u1C5A-\u1C77\u1C78-\u1C7D\u1C7E-\u1C7F\u1CC0-\u1CC7\u1CD3\u1CE1' + '\u1CE9-\u1CEC\u1CEE-\u1CF1\u1CF2-\u1CF3\u1CF5-\u1CF6\u1D00-\u1D2B' + '\u1D2C-\u1D6A\u1D6B-\u1D77\u1D78\u1D79-\u1D9A\u1D9B-\u1DBF\u1E00-\u1F15' + '\u1F18-\u1F1D\u1F20-\u1F45\u1F48-\u1F4D\u1F50-\u1F57\u1F59\u1F5B\u1F5D' + '\u1F5F-\u1F7D\u1F80-\u1FB4\u1FB6-\u1FBC\u1FBE\u1FC2-\u1FC4\u1FC6-\u1FCC' + '\u1FD0-\u1FD3\u1FD6-\u1FDB\u1FE0-\u1FEC\u1FF2-\u1FF4\u1FF6-\u1FFC\u200E' + '\u2071\u207F\u2090-\u209C\u2102\u2107\u210A-\u2113\u2115\u2119-\u211D' + '\u2124\u2126\u2128\u212A-\u212D\u212F-\u2134\u2135-\u2138\u2139' + '\u213C-\u213F\u2145-\u2149\u214E\u214F\u2160-\u2182\u2183-\u2184' + '\u2185-\u2188\u2336-\u237A\u2395\u249C-\u24E9\u26AC\u2800-\u28FF' + '\u2C00-\u2C2E\u2C30-\u2C5E\u2C60-\u2C7B\u2C7C-\u2C7D\u2C7E-\u2CE4' + '\u2CEB-\u2CEE\u2CF2-\u2CF3\u2D00-\u2D25\u2D27\u2D2D\u2D30-\u2D67\u2D6F' + '\u2D70\u2D80-\u2D96\u2DA0-\u2DA6\u2DA8-\u2DAE\u2DB0-\u2DB6\u2DB8-\u2DBE' + '\u2DC0-\u2DC6\u2DC8-\u2DCE\u2DD0-\u2DD6\u2DD8-\u2DDE\u3005\u3006\u3007' + '\u3021-\u3029\u302E-\u302F\u3031-\u3035\u3038-\u303A\u303B\u303C' + '\u3041-\u3096\u309D-\u309E\u309F\u30A1-\u30FA\u30FC-\u30FE\u30FF' + '\u3105-\u312D\u3131-\u318E\u3190-\u3191\u3192-\u3195\u3196-\u319F' + '\u31A0-\u31BA\u31F0-\u31FF\u3200-\u321C\u3220-\u3229\u322A-\u3247' + '\u3248-\u324F\u3260-\u327B\u327F\u3280-\u3289\u328A-\u32B0\u32C0-\u32CB' + '\u32D0-\u32FE\u3300-\u3376\u337B-\u33DD\u33E0-\u33FE\u3400-\u4DB5' + '\u4E00-\u9FCC\uA000-\uA014\uA015\uA016-\uA48C\uA4D0-\uA4F7\uA4F8-\uA4FD' + '\uA4FE-\uA4FF\uA500-\uA60B\uA60C\uA610-\uA61F\uA620-\uA629\uA62A-\uA62B' + '\uA640-\uA66D\uA66E\uA680-\uA69B\uA69C-\uA69D\uA6A0-\uA6E5\uA6E6-\uA6EF' + '\uA6F2-\uA6F7\uA722-\uA76F\uA770\uA771-\uA787\uA789-\uA78A\uA78B-\uA78E' + '\uA790-\uA7AD\uA7B0-\uA7B1\uA7F7\uA7F8-\uA7F9\uA7FA\uA7FB-\uA801' + '\uA803-\uA805\uA807-\uA80A\uA80C-\uA822\uA823-\uA824\uA827\uA830-\uA835' + '\uA836-\uA837\uA840-\uA873\uA880-\uA881\uA882-\uA8B3\uA8B4-\uA8C3' + '\uA8CE-\uA8CF\uA8D0-\uA8D9\uA8F2-\uA8F7\uA8F8-\uA8FA\uA8FB\uA900-\uA909' + '\uA90A-\uA925\uA92E-\uA92F\uA930-\uA946\uA952-\uA953\uA95F\uA960-\uA97C' + '\uA983\uA984-\uA9B2\uA9B4-\uA9B5\uA9BA-\uA9BB\uA9BD-\uA9C0\uA9C1-\uA9CD' + '\uA9CF\uA9D0-\uA9D9\uA9DE-\uA9DF\uA9E0-\uA9E4\uA9E6\uA9E7-\uA9EF' + '\uA9F0-\uA9F9\uA9FA-\uA9FE\uAA00-\uAA28\uAA2F-\uAA30\uAA33-\uAA34' + '\uAA40-\uAA42\uAA44-\uAA4B\uAA4D\uAA50-\uAA59\uAA5C-\uAA5F\uAA60-\uAA6F' + '\uAA70\uAA71-\uAA76\uAA77-\uAA79\uAA7A\uAA7B\uAA7D\uAA7E-\uAAAF\uAAB1' + '\uAAB5-\uAAB6\uAAB9-\uAABD\uAAC0\uAAC2\uAADB-\uAADC\uAADD\uAADE-\uAADF' + '\uAAE0-\uAAEA\uAAEB\uAAEE-\uAAEF\uAAF0-\uAAF1\uAAF2\uAAF3-\uAAF4\uAAF5' + '\uAB01-\uAB06\uAB09-\uAB0E\uAB11-\uAB16\uAB20-\uAB26\uAB28-\uAB2E' + '\uAB30-\uAB5A\uAB5B\uAB5C-\uAB5F\uAB64-\uAB65\uABC0-\uABE2\uABE3-\uABE4' + '\uABE6-\uABE7\uABE9-\uABEA\uABEB\uABEC\uABF0-\uABF9\uAC00-\uD7A3' + '\uD7B0-\uD7C6\uD7CB-\uD7FB\uE000-\uF8FF\uF900-\uFA6D\uFA70-\uFAD9' + '\uFB00-\uFB06\uFB13-\uFB17\uFF21-\uFF3A\uFF41-\uFF5A\uFF66-\uFF6F\uFF70' + '\uFF71-\uFF9D\uFF9E-\uFF9F\uFFA0-\uFFBE\uFFC2-\uFFC7\uFFCA-\uFFCF' + '\uFFD2-\uFFD7\uFFDA-\uFFDC',
+
+ R: '\u0590\u05BE\u05C0\u05C3\u05C6\u05C8-\u05CF\u05D0-\u05EA\u05EB-\u05EF' + '\u05F0-\u05F2\u05F3-\u05F4\u05F5-\u05FF\u07C0-\u07C9\u07CA-\u07EA' + '\u07F4-\u07F5\u07FA\u07FB-\u07FF\u0800-\u0815\u081A\u0824\u0828' + '\u082E-\u082F\u0830-\u083E\u083F\u0840-\u0858\u085C-\u085D\u085E' + '\u085F-\u089F\u200F\uFB1D\uFB1F-\uFB28\uFB2A-\uFB36\uFB37\uFB38-\uFB3C' + '\uFB3D\uFB3E\uFB3F\uFB40-\uFB41\uFB42\uFB43-\uFB44\uFB45\uFB46-\uFB4F',
+
+ AL: '\u0608\u060B\u060D\u061B\u061C\u061D\u061E-\u061F\u0620-\u063F\u0640' + '\u0641-\u064A\u066D\u066E-\u066F\u0671-\u06D3\u06D4\u06D5\u06E5-\u06E6' + '\u06EE-\u06EF\u06FA-\u06FC\u06FD-\u06FE\u06FF\u0700-\u070D\u070E\u070F' + '\u0710\u0712-\u072F\u074B-\u074C\u074D-\u07A5\u07B1\u07B2-\u07BF' + '\u08A0-\u08B2\u08B3-\u08E3\uFB50-\uFBB1\uFBB2-\uFBC1\uFBC2-\uFBD2' + '\uFBD3-\uFD3D\uFD40-\uFD4F\uFD50-\uFD8F\uFD90-\uFD91\uFD92-\uFDC7' + '\uFDC8-\uFDCF\uFDF0-\uFDFB\uFDFC\uFDFE-\uFDFF\uFE70-\uFE74\uFE75' + '\uFE76-\uFEFC\uFEFD-\uFEFE'
+
+};
+
+var REGEX_STRONG = new RegExp('[' + RANGE_BY_BIDI_TYPE.L + RANGE_BY_BIDI_TYPE.R + RANGE_BY_BIDI_TYPE.AL + ']');
+
+var REGEX_RTL = new RegExp('[' + RANGE_BY_BIDI_TYPE.R + RANGE_BY_BIDI_TYPE.AL + ']');
+
+/**
+ * Returns the first strong character (has Bidi_Class value of L, R, or AL).
+ *
+ * @param str A text block; e.g. paragraph, table cell, tag
+ * @return A character with strong bidi direction, or null if not found
+ */
+function firstStrongChar(str) {
+ var match = REGEX_STRONG.exec(str);
+ return match == null ? null : match[0];
+}
+
+/**
+ * Returns the direction of a block of text, based on the direction of its
+ * first strong character (has Bidi_Class value of L, R, or AL).
+ *
+ * @param str A text block; e.g. paragraph, table cell, tag
+ * @return The resolved direction
+ */
+function firstStrongCharDir(str) {
+ var strongChar = firstStrongChar(str);
+ if (strongChar == null) {
+ return UnicodeBidiDirection.NEUTRAL;
+ }
+ return REGEX_RTL.exec(strongChar) ? UnicodeBidiDirection.RTL : UnicodeBidiDirection.LTR;
+}
+
+/**
+ * Returns the direction of a block of text, based on the direction of its
+ * first strong character (has Bidi_Class value of L, R, or AL), or a fallback
+ * direction, if no strong character is found.
+ *
+ * This function is supposed to be used in respect to Higher-Level Protocol
+ * rule HL1. (http://www.unicode.org/reports/tr9/#HL1)
+ *
+ * @param str A text block; e.g. paragraph, table cell, tag
+ * @param fallback Fallback direction, used if no strong direction detected
+ * for the block (default = NEUTRAL)
+ * @return The resolved direction
+ */
+function resolveBlockDir(str, fallback) {
+ fallback = fallback || UnicodeBidiDirection.NEUTRAL;
+ if (!str.length) {
+ return fallback;
+ }
+ var blockDir = firstStrongCharDir(str);
+ return blockDir === UnicodeBidiDirection.NEUTRAL ? fallback : blockDir;
+}
+
+/**
+ * Returns the direction of a block of text, based on the direction of its
+ * first strong character (has Bidi_Class value of L, R, or AL), or a fallback
+ * direction, if no strong character is found.
+ *
+ * NOTE: This function is similar to resolveBlockDir(), but uses the global
+ * direction as the fallback, so it *always* returns a Strong direction,
+ * making it useful for integration in places that you need to make the final
+ * decision, like setting some CSS class.
+ *
+ * This function is supposed to be used in respect to Higher-Level Protocol
+ * rule HL1. (http://www.unicode.org/reports/tr9/#HL1)
+ *
+ * @param str A text block; e.g. paragraph, table cell
+ * @param strongFallback Fallback direction, used if no strong direction
+ * detected for the block (default = global direction)
+ * @return The resolved Strong direction
+ */
+function getDirection(str, strongFallback) {
+ if (!strongFallback) {
+ strongFallback = UnicodeBidiDirection.getGlobalDir();
+ }
+ !UnicodeBidiDirection.isStrong(strongFallback) ? process.env.NODE_ENV !== 'production' ? invariant(false, 'Fallback direction must be a strong direction') : invariant(false) : void 0;
+ return resolveBlockDir(str, strongFallback);
+}
+
+/**
+ * Returns true if getDirection(arguments...) returns LTR.
+ *
+ * @param str A text block; e.g. paragraph, table cell
+ * @param strongFallback Fallback direction, used if no strong direction
+ * detected for the block (default = global direction)
+ * @return True if the resolved direction is LTR
+ */
+function isDirectionLTR(str, strongFallback) {
+ return getDirection(str, strongFallback) === UnicodeBidiDirection.LTR;
+}
+
+/**
+ * Returns true if getDirection(arguments...) returns RTL.
+ *
+ * @param str A text block; e.g. paragraph, table cell
+ * @param strongFallback Fallback direction, used if no strong direction
+ * detected for the block (default = global direction)
+ * @return True if the resolved direction is RTL
+ */
+function isDirectionRTL(str, strongFallback) {
+ return getDirection(str, strongFallback) === UnicodeBidiDirection.RTL;
+}
+
+var UnicodeBidi = {
+ firstStrongChar: firstStrongChar,
+ firstStrongCharDir: firstStrongCharDir,
+ resolveBlockDir: resolveBlockDir,
+ getDirection: getDirection,
+ isDirectionLTR: isDirectionLTR,
+ isDirectionRTL: isDirectionRTL
+};
+
+module.exports = UnicodeBidi;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeBidi.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeBidi.js.flow
new file mode 100644
index 00000000..5e824a5d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeBidi.js.flow
@@ -0,0 +1,157 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule UnicodeBidi
+ * @typechecks
+ * @flow
+ */
+
+/**
+ * Basic (stateless) API for text direction detection
+ *
+ * Part of our implementation of Unicode Bidirectional Algorithm (UBA)
+ * Unicode Standard Annex #9 (UAX9)
+ * http://www.unicode.org/reports/tr9/
+ */
+
+'use strict';
+
+const UnicodeBidiDirection = require('./UnicodeBidiDirection');
+
+const invariant = require('./invariant');
+
+import type { BidiDirection } from './UnicodeBidiDirection';
+
+/**
+ * RegExp ranges of characters with a *Strong* Bidi_Class value.
+ *
+ * Data is based on DerivedBidiClass.txt in UCD version 7.0.0.
+ *
+ * NOTE: For performance reasons, we only support Unicode's
+ * Basic Multilingual Plane (BMP) for now.
+ */
+const RANGE_BY_BIDI_TYPE = {
+
+ L: 'A-Za-z\u00AA\u00B5\u00BA\u00C0-\u00D6\u00D8-\u00F6\u00F8-\u01BA\u01BB' + '\u01BC-\u01BF\u01C0-\u01C3\u01C4-\u0293\u0294\u0295-\u02AF\u02B0-\u02B8' + '\u02BB-\u02C1\u02D0-\u02D1\u02E0-\u02E4\u02EE\u0370-\u0373\u0376-\u0377' + '\u037A\u037B-\u037D\u037F\u0386\u0388-\u038A\u038C\u038E-\u03A1' + '\u03A3-\u03F5\u03F7-\u0481\u0482\u048A-\u052F\u0531-\u0556\u0559' + '\u055A-\u055F\u0561-\u0587\u0589\u0903\u0904-\u0939\u093B\u093D' + '\u093E-\u0940\u0949-\u094C\u094E-\u094F\u0950\u0958-\u0961\u0964-\u0965' + '\u0966-\u096F\u0970\u0971\u0972-\u0980\u0982-\u0983\u0985-\u098C' + '\u098F-\u0990\u0993-\u09A8\u09AA-\u09B0\u09B2\u09B6-\u09B9\u09BD' + '\u09BE-\u09C0\u09C7-\u09C8\u09CB-\u09CC\u09CE\u09D7\u09DC-\u09DD' + '\u09DF-\u09E1\u09E6-\u09EF\u09F0-\u09F1\u09F4-\u09F9\u09FA\u0A03' + '\u0A05-\u0A0A\u0A0F-\u0A10\u0A13-\u0A28\u0A2A-\u0A30\u0A32-\u0A33' + '\u0A35-\u0A36\u0A38-\u0A39\u0A3E-\u0A40\u0A59-\u0A5C\u0A5E\u0A66-\u0A6F' + '\u0A72-\u0A74\u0A83\u0A85-\u0A8D\u0A8F-\u0A91\u0A93-\u0AA8\u0AAA-\u0AB0' + '\u0AB2-\u0AB3\u0AB5-\u0AB9\u0ABD\u0ABE-\u0AC0\u0AC9\u0ACB-\u0ACC\u0AD0' + '\u0AE0-\u0AE1\u0AE6-\u0AEF\u0AF0\u0B02-\u0B03\u0B05-\u0B0C\u0B0F-\u0B10' + '\u0B13-\u0B28\u0B2A-\u0B30\u0B32-\u0B33\u0B35-\u0B39\u0B3D\u0B3E\u0B40' + '\u0B47-\u0B48\u0B4B-\u0B4C\u0B57\u0B5C-\u0B5D\u0B5F-\u0B61\u0B66-\u0B6F' + '\u0B70\u0B71\u0B72-\u0B77\u0B83\u0B85-\u0B8A\u0B8E-\u0B90\u0B92-\u0B95' + '\u0B99-\u0B9A\u0B9C\u0B9E-\u0B9F\u0BA3-\u0BA4\u0BA8-\u0BAA\u0BAE-\u0BB9' + '\u0BBE-\u0BBF\u0BC1-\u0BC2\u0BC6-\u0BC8\u0BCA-\u0BCC\u0BD0\u0BD7' + '\u0BE6-\u0BEF\u0BF0-\u0BF2\u0C01-\u0C03\u0C05-\u0C0C\u0C0E-\u0C10' + '\u0C12-\u0C28\u0C2A-\u0C39\u0C3D\u0C41-\u0C44\u0C58-\u0C59\u0C60-\u0C61' + '\u0C66-\u0C6F\u0C7F\u0C82-\u0C83\u0C85-\u0C8C\u0C8E-\u0C90\u0C92-\u0CA8' + '\u0CAA-\u0CB3\u0CB5-\u0CB9\u0CBD\u0CBE\u0CBF\u0CC0-\u0CC4\u0CC6' + '\u0CC7-\u0CC8\u0CCA-\u0CCB\u0CD5-\u0CD6\u0CDE\u0CE0-\u0CE1\u0CE6-\u0CEF' + '\u0CF1-\u0CF2\u0D02-\u0D03\u0D05-\u0D0C\u0D0E-\u0D10\u0D12-\u0D3A\u0D3D' + '\u0D3E-\u0D40\u0D46-\u0D48\u0D4A-\u0D4C\u0D4E\u0D57\u0D60-\u0D61' + '\u0D66-\u0D6F\u0D70-\u0D75\u0D79\u0D7A-\u0D7F\u0D82-\u0D83\u0D85-\u0D96' + '\u0D9A-\u0DB1\u0DB3-\u0DBB\u0DBD\u0DC0-\u0DC6\u0DCF-\u0DD1\u0DD8-\u0DDF' + '\u0DE6-\u0DEF\u0DF2-\u0DF3\u0DF4\u0E01-\u0E30\u0E32-\u0E33\u0E40-\u0E45' + '\u0E46\u0E4F\u0E50-\u0E59\u0E5A-\u0E5B\u0E81-\u0E82\u0E84\u0E87-\u0E88' + '\u0E8A\u0E8D\u0E94-\u0E97\u0E99-\u0E9F\u0EA1-\u0EA3\u0EA5\u0EA7' + '\u0EAA-\u0EAB\u0EAD-\u0EB0\u0EB2-\u0EB3\u0EBD\u0EC0-\u0EC4\u0EC6' + '\u0ED0-\u0ED9\u0EDC-\u0EDF\u0F00\u0F01-\u0F03\u0F04-\u0F12\u0F13\u0F14' + '\u0F15-\u0F17\u0F1A-\u0F1F\u0F20-\u0F29\u0F2A-\u0F33\u0F34\u0F36\u0F38' + '\u0F3E-\u0F3F\u0F40-\u0F47\u0F49-\u0F6C\u0F7F\u0F85\u0F88-\u0F8C' + '\u0FBE-\u0FC5\u0FC7-\u0FCC\u0FCE-\u0FCF\u0FD0-\u0FD4\u0FD5-\u0FD8' + '\u0FD9-\u0FDA\u1000-\u102A\u102B-\u102C\u1031\u1038\u103B-\u103C\u103F' + '\u1040-\u1049\u104A-\u104F\u1050-\u1055\u1056-\u1057\u105A-\u105D\u1061' + '\u1062-\u1064\u1065-\u1066\u1067-\u106D\u106E-\u1070\u1075-\u1081' + '\u1083-\u1084\u1087-\u108C\u108E\u108F\u1090-\u1099\u109A-\u109C' + '\u109E-\u109F\u10A0-\u10C5\u10C7\u10CD\u10D0-\u10FA\u10FB\u10FC' + '\u10FD-\u1248\u124A-\u124D\u1250-\u1256\u1258\u125A-\u125D\u1260-\u1288' + '\u128A-\u128D\u1290-\u12B0\u12B2-\u12B5\u12B8-\u12BE\u12C0\u12C2-\u12C5' + '\u12C8-\u12D6\u12D8-\u1310\u1312-\u1315\u1318-\u135A\u1360-\u1368' + '\u1369-\u137C\u1380-\u138F\u13A0-\u13F4\u1401-\u166C\u166D-\u166E' + '\u166F-\u167F\u1681-\u169A\u16A0-\u16EA\u16EB-\u16ED\u16EE-\u16F0' + '\u16F1-\u16F8\u1700-\u170C\u170E-\u1711\u1720-\u1731\u1735-\u1736' + '\u1740-\u1751\u1760-\u176C\u176E-\u1770\u1780-\u17B3\u17B6\u17BE-\u17C5' + '\u17C7-\u17C8\u17D4-\u17D6\u17D7\u17D8-\u17DA\u17DC\u17E0-\u17E9' + '\u1810-\u1819\u1820-\u1842\u1843\u1844-\u1877\u1880-\u18A8\u18AA' + '\u18B0-\u18F5\u1900-\u191E\u1923-\u1926\u1929-\u192B\u1930-\u1931' + '\u1933-\u1938\u1946-\u194F\u1950-\u196D\u1970-\u1974\u1980-\u19AB' + '\u19B0-\u19C0\u19C1-\u19C7\u19C8-\u19C9\u19D0-\u19D9\u19DA\u1A00-\u1A16' + '\u1A19-\u1A1A\u1A1E-\u1A1F\u1A20-\u1A54\u1A55\u1A57\u1A61\u1A63-\u1A64' + '\u1A6D-\u1A72\u1A80-\u1A89\u1A90-\u1A99\u1AA0-\u1AA6\u1AA7\u1AA8-\u1AAD' + '\u1B04\u1B05-\u1B33\u1B35\u1B3B\u1B3D-\u1B41\u1B43-\u1B44\u1B45-\u1B4B' + '\u1B50-\u1B59\u1B5A-\u1B60\u1B61-\u1B6A\u1B74-\u1B7C\u1B82\u1B83-\u1BA0' + '\u1BA1\u1BA6-\u1BA7\u1BAA\u1BAE-\u1BAF\u1BB0-\u1BB9\u1BBA-\u1BE5\u1BE7' + '\u1BEA-\u1BEC\u1BEE\u1BF2-\u1BF3\u1BFC-\u1BFF\u1C00-\u1C23\u1C24-\u1C2B' + '\u1C34-\u1C35\u1C3B-\u1C3F\u1C40-\u1C49\u1C4D-\u1C4F\u1C50-\u1C59' + '\u1C5A-\u1C77\u1C78-\u1C7D\u1C7E-\u1C7F\u1CC0-\u1CC7\u1CD3\u1CE1' + '\u1CE9-\u1CEC\u1CEE-\u1CF1\u1CF2-\u1CF3\u1CF5-\u1CF6\u1D00-\u1D2B' + '\u1D2C-\u1D6A\u1D6B-\u1D77\u1D78\u1D79-\u1D9A\u1D9B-\u1DBF\u1E00-\u1F15' + '\u1F18-\u1F1D\u1F20-\u1F45\u1F48-\u1F4D\u1F50-\u1F57\u1F59\u1F5B\u1F5D' + '\u1F5F-\u1F7D\u1F80-\u1FB4\u1FB6-\u1FBC\u1FBE\u1FC2-\u1FC4\u1FC6-\u1FCC' + '\u1FD0-\u1FD3\u1FD6-\u1FDB\u1FE0-\u1FEC\u1FF2-\u1FF4\u1FF6-\u1FFC\u200E' + '\u2071\u207F\u2090-\u209C\u2102\u2107\u210A-\u2113\u2115\u2119-\u211D' + '\u2124\u2126\u2128\u212A-\u212D\u212F-\u2134\u2135-\u2138\u2139' + '\u213C-\u213F\u2145-\u2149\u214E\u214F\u2160-\u2182\u2183-\u2184' + '\u2185-\u2188\u2336-\u237A\u2395\u249C-\u24E9\u26AC\u2800-\u28FF' + '\u2C00-\u2C2E\u2C30-\u2C5E\u2C60-\u2C7B\u2C7C-\u2C7D\u2C7E-\u2CE4' + '\u2CEB-\u2CEE\u2CF2-\u2CF3\u2D00-\u2D25\u2D27\u2D2D\u2D30-\u2D67\u2D6F' + '\u2D70\u2D80-\u2D96\u2DA0-\u2DA6\u2DA8-\u2DAE\u2DB0-\u2DB6\u2DB8-\u2DBE' + '\u2DC0-\u2DC6\u2DC8-\u2DCE\u2DD0-\u2DD6\u2DD8-\u2DDE\u3005\u3006\u3007' + '\u3021-\u3029\u302E-\u302F\u3031-\u3035\u3038-\u303A\u303B\u303C' + '\u3041-\u3096\u309D-\u309E\u309F\u30A1-\u30FA\u30FC-\u30FE\u30FF' + '\u3105-\u312D\u3131-\u318E\u3190-\u3191\u3192-\u3195\u3196-\u319F' + '\u31A0-\u31BA\u31F0-\u31FF\u3200-\u321C\u3220-\u3229\u322A-\u3247' + '\u3248-\u324F\u3260-\u327B\u327F\u3280-\u3289\u328A-\u32B0\u32C0-\u32CB' + '\u32D0-\u32FE\u3300-\u3376\u337B-\u33DD\u33E0-\u33FE\u3400-\u4DB5' + '\u4E00-\u9FCC\uA000-\uA014\uA015\uA016-\uA48C\uA4D0-\uA4F7\uA4F8-\uA4FD' + '\uA4FE-\uA4FF\uA500-\uA60B\uA60C\uA610-\uA61F\uA620-\uA629\uA62A-\uA62B' + '\uA640-\uA66D\uA66E\uA680-\uA69B\uA69C-\uA69D\uA6A0-\uA6E5\uA6E6-\uA6EF' + '\uA6F2-\uA6F7\uA722-\uA76F\uA770\uA771-\uA787\uA789-\uA78A\uA78B-\uA78E' + '\uA790-\uA7AD\uA7B0-\uA7B1\uA7F7\uA7F8-\uA7F9\uA7FA\uA7FB-\uA801' + '\uA803-\uA805\uA807-\uA80A\uA80C-\uA822\uA823-\uA824\uA827\uA830-\uA835' + '\uA836-\uA837\uA840-\uA873\uA880-\uA881\uA882-\uA8B3\uA8B4-\uA8C3' + '\uA8CE-\uA8CF\uA8D0-\uA8D9\uA8F2-\uA8F7\uA8F8-\uA8FA\uA8FB\uA900-\uA909' + '\uA90A-\uA925\uA92E-\uA92F\uA930-\uA946\uA952-\uA953\uA95F\uA960-\uA97C' + '\uA983\uA984-\uA9B2\uA9B4-\uA9B5\uA9BA-\uA9BB\uA9BD-\uA9C0\uA9C1-\uA9CD' + '\uA9CF\uA9D0-\uA9D9\uA9DE-\uA9DF\uA9E0-\uA9E4\uA9E6\uA9E7-\uA9EF' + '\uA9F0-\uA9F9\uA9FA-\uA9FE\uAA00-\uAA28\uAA2F-\uAA30\uAA33-\uAA34' + '\uAA40-\uAA42\uAA44-\uAA4B\uAA4D\uAA50-\uAA59\uAA5C-\uAA5F\uAA60-\uAA6F' + '\uAA70\uAA71-\uAA76\uAA77-\uAA79\uAA7A\uAA7B\uAA7D\uAA7E-\uAAAF\uAAB1' + '\uAAB5-\uAAB6\uAAB9-\uAABD\uAAC0\uAAC2\uAADB-\uAADC\uAADD\uAADE-\uAADF' + '\uAAE0-\uAAEA\uAAEB\uAAEE-\uAAEF\uAAF0-\uAAF1\uAAF2\uAAF3-\uAAF4\uAAF5' + '\uAB01-\uAB06\uAB09-\uAB0E\uAB11-\uAB16\uAB20-\uAB26\uAB28-\uAB2E' + '\uAB30-\uAB5A\uAB5B\uAB5C-\uAB5F\uAB64-\uAB65\uABC0-\uABE2\uABE3-\uABE4' + '\uABE6-\uABE7\uABE9-\uABEA\uABEB\uABEC\uABF0-\uABF9\uAC00-\uD7A3' + '\uD7B0-\uD7C6\uD7CB-\uD7FB\uE000-\uF8FF\uF900-\uFA6D\uFA70-\uFAD9' + '\uFB00-\uFB06\uFB13-\uFB17\uFF21-\uFF3A\uFF41-\uFF5A\uFF66-\uFF6F\uFF70' + '\uFF71-\uFF9D\uFF9E-\uFF9F\uFFA0-\uFFBE\uFFC2-\uFFC7\uFFCA-\uFFCF' + '\uFFD2-\uFFD7\uFFDA-\uFFDC',
+
+ R: '\u0590\u05BE\u05C0\u05C3\u05C6\u05C8-\u05CF\u05D0-\u05EA\u05EB-\u05EF' + '\u05F0-\u05F2\u05F3-\u05F4\u05F5-\u05FF\u07C0-\u07C9\u07CA-\u07EA' + '\u07F4-\u07F5\u07FA\u07FB-\u07FF\u0800-\u0815\u081A\u0824\u0828' + '\u082E-\u082F\u0830-\u083E\u083F\u0840-\u0858\u085C-\u085D\u085E' + '\u085F-\u089F\u200F\uFB1D\uFB1F-\uFB28\uFB2A-\uFB36\uFB37\uFB38-\uFB3C' + '\uFB3D\uFB3E\uFB3F\uFB40-\uFB41\uFB42\uFB43-\uFB44\uFB45\uFB46-\uFB4F',
+
+ AL: '\u0608\u060B\u060D\u061B\u061C\u061D\u061E-\u061F\u0620-\u063F\u0640' + '\u0641-\u064A\u066D\u066E-\u066F\u0671-\u06D3\u06D4\u06D5\u06E5-\u06E6' + '\u06EE-\u06EF\u06FA-\u06FC\u06FD-\u06FE\u06FF\u0700-\u070D\u070E\u070F' + '\u0710\u0712-\u072F\u074B-\u074C\u074D-\u07A5\u07B1\u07B2-\u07BF' + '\u08A0-\u08B2\u08B3-\u08E3\uFB50-\uFBB1\uFBB2-\uFBC1\uFBC2-\uFBD2' + '\uFBD3-\uFD3D\uFD40-\uFD4F\uFD50-\uFD8F\uFD90-\uFD91\uFD92-\uFDC7' + '\uFDC8-\uFDCF\uFDF0-\uFDFB\uFDFC\uFDFE-\uFDFF\uFE70-\uFE74\uFE75' + '\uFE76-\uFEFC\uFEFD-\uFEFE'
+
+};
+
+const REGEX_STRONG = new RegExp('[' + RANGE_BY_BIDI_TYPE.L + RANGE_BY_BIDI_TYPE.R + RANGE_BY_BIDI_TYPE.AL + ']');
+
+const REGEX_RTL = new RegExp('[' + RANGE_BY_BIDI_TYPE.R + RANGE_BY_BIDI_TYPE.AL + ']');
+
+/**
+ * Returns the first strong character (has Bidi_Class value of L, R, or AL).
+ *
+ * @param str A text block; e.g. paragraph, table cell, tag
+ * @return A character with strong bidi direction, or null if not found
+ */
+function firstStrongChar(str: string): ?string {
+ const match = REGEX_STRONG.exec(str);
+ return match == null ? null : match[0];
+}
+
+/**
+ * Returns the direction of a block of text, based on the direction of its
+ * first strong character (has Bidi_Class value of L, R, or AL).
+ *
+ * @param str A text block; e.g. paragraph, table cell, tag
+ * @return The resolved direction
+ */
+function firstStrongCharDir(str: string): BidiDirection {
+ const strongChar = firstStrongChar(str);
+ if (strongChar == null) {
+ return UnicodeBidiDirection.NEUTRAL;
+ }
+ return REGEX_RTL.exec(strongChar) ? UnicodeBidiDirection.RTL : UnicodeBidiDirection.LTR;
+}
+
+/**
+ * Returns the direction of a block of text, based on the direction of its
+ * first strong character (has Bidi_Class value of L, R, or AL), or a fallback
+ * direction, if no strong character is found.
+ *
+ * This function is supposed to be used in respect to Higher-Level Protocol
+ * rule HL1. (http://www.unicode.org/reports/tr9/#HL1)
+ *
+ * @param str A text block; e.g. paragraph, table cell, tag
+ * @param fallback Fallback direction, used if no strong direction detected
+ * for the block (default = NEUTRAL)
+ * @return The resolved direction
+ */
+function resolveBlockDir(str: string, fallback: ?BidiDirection): BidiDirection {
+ fallback = fallback || UnicodeBidiDirection.NEUTRAL;
+ if (!str.length) {
+ return fallback;
+ }
+ const blockDir = firstStrongCharDir(str);
+ return blockDir === UnicodeBidiDirection.NEUTRAL ? fallback : blockDir;
+}
+
+/**
+ * Returns the direction of a block of text, based on the direction of its
+ * first strong character (has Bidi_Class value of L, R, or AL), or a fallback
+ * direction, if no strong character is found.
+ *
+ * NOTE: This function is similar to resolveBlockDir(), but uses the global
+ * direction as the fallback, so it *always* returns a Strong direction,
+ * making it useful for integration in places that you need to make the final
+ * decision, like setting some CSS class.
+ *
+ * This function is supposed to be used in respect to Higher-Level Protocol
+ * rule HL1. (http://www.unicode.org/reports/tr9/#HL1)
+ *
+ * @param str A text block; e.g. paragraph, table cell
+ * @param strongFallback Fallback direction, used if no strong direction
+ * detected for the block (default = global direction)
+ * @return The resolved Strong direction
+ */
+function getDirection(str: string, strongFallback: ?BidiDirection): BidiDirection {
+ if (!strongFallback) {
+ strongFallback = UnicodeBidiDirection.getGlobalDir();
+ }
+ invariant(UnicodeBidiDirection.isStrong(strongFallback), 'Fallback direction must be a strong direction');
+ return resolveBlockDir(str, strongFallback);
+}
+
+/**
+ * Returns true if getDirection(arguments...) returns LTR.
+ *
+ * @param str A text block; e.g. paragraph, table cell
+ * @param strongFallback Fallback direction, used if no strong direction
+ * detected for the block (default = global direction)
+ * @return True if the resolved direction is LTR
+ */
+function isDirectionLTR(str: string, strongFallback: ?BidiDirection): boolean {
+ return getDirection(str, strongFallback) === UnicodeBidiDirection.LTR;
+}
+
+/**
+ * Returns true if getDirection(arguments...) returns RTL.
+ *
+ * @param str A text block; e.g. paragraph, table cell
+ * @param strongFallback Fallback direction, used if no strong direction
+ * detected for the block (default = global direction)
+ * @return True if the resolved direction is RTL
+ */
+function isDirectionRTL(str: string, strongFallback: ?BidiDirection): boolean {
+ return getDirection(str, strongFallback) === UnicodeBidiDirection.RTL;
+}
+
+const UnicodeBidi = {
+ firstStrongChar: firstStrongChar,
+ firstStrongCharDir: firstStrongCharDir,
+ resolveBlockDir: resolveBlockDir,
+ getDirection: getDirection,
+ isDirectionLTR: isDirectionLTR,
+ isDirectionRTL: isDirectionRTL
+};
+
+module.exports = UnicodeBidi;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeBidiDirection.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeBidiDirection.js
new file mode 100644
index 00000000..c62febea
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeBidiDirection.js
@@ -0,0 +1,106 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @typechecks
+ *
+ */
+
+/**
+ * Constants to represent text directionality
+ *
+ * Also defines a *global* direciton, to be used in bidi algorithms as a
+ * default fallback direciton, when no better direction is found or provided.
+ *
+ * NOTE: Use `setGlobalDir()`, or update `initGlobalDir()`, to set the initial
+ * global direction value based on the application.
+ *
+ * Part of the implementation of Unicode Bidirectional Algorithm (UBA)
+ * Unicode Standard Annex #9 (UAX9)
+ * http://www.unicode.org/reports/tr9/
+ */
+
+'use strict';
+
+var invariant = require('./invariant');
+
+var NEUTRAL = 'NEUTRAL'; // No strong direction
+var LTR = 'LTR'; // Left-to-Right direction
+var RTL = 'RTL'; // Right-to-Left direction
+
+var globalDir = null;
+
+// == Helpers ==
+
+/**
+ * Check if a directionality value is a Strong one
+ */
+function isStrong(dir) {
+ return dir === LTR || dir === RTL;
+}
+
+/**
+ * Get string value to be used for `dir` HTML attribute or `direction` CSS
+ * property.
+ */
+function getHTMLDir(dir) {
+ !isStrong(dir) ? process.env.NODE_ENV !== 'production' ? invariant(false, '`dir` must be a strong direction to be converted to HTML Direction') : invariant(false) : void 0;
+ return dir === LTR ? 'ltr' : 'rtl';
+}
+
+/**
+ * Get string value to be used for `dir` HTML attribute or `direction` CSS
+ * property, but returns null if `dir` has same value as `otherDir`.
+ * `null`.
+ */
+function getHTMLDirIfDifferent(dir, otherDir) {
+ !isStrong(dir) ? process.env.NODE_ENV !== 'production' ? invariant(false, '`dir` must be a strong direction to be converted to HTML Direction') : invariant(false) : void 0;
+ !isStrong(otherDir) ? process.env.NODE_ENV !== 'production' ? invariant(false, '`otherDir` must be a strong direction to be converted to HTML Direction') : invariant(false) : void 0;
+ return dir === otherDir ? null : getHTMLDir(dir);
+}
+
+// == Global Direction ==
+
+/**
+ * Set the global direction.
+ */
+function setGlobalDir(dir) {
+ globalDir = dir;
+}
+
+/**
+ * Initialize the global direction
+ */
+function initGlobalDir() {
+ setGlobalDir(LTR);
+}
+
+/**
+ * Get the global direction
+ */
+function getGlobalDir() {
+ if (!globalDir) {
+ this.initGlobalDir();
+ }
+ !globalDir ? process.env.NODE_ENV !== 'production' ? invariant(false, 'Global direction not set.') : invariant(false) : void 0;
+ return globalDir;
+}
+
+var UnicodeBidiDirection = {
+ // Values
+ NEUTRAL: NEUTRAL,
+ LTR: LTR,
+ RTL: RTL,
+ // Helpers
+ isStrong: isStrong,
+ getHTMLDir: getHTMLDir,
+ getHTMLDirIfDifferent: getHTMLDirIfDifferent,
+ // Global Direction
+ setGlobalDir: setGlobalDir,
+ initGlobalDir: initGlobalDir,
+ getGlobalDir: getGlobalDir
+};
+
+module.exports = UnicodeBidiDirection;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeBidiDirection.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeBidiDirection.js.flow
new file mode 100644
index 00000000..1301fedd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeBidiDirection.js.flow
@@ -0,0 +1,110 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule UnicodeBidiDirection
+ * @typechecks
+ * @flow
+ */
+
+/**
+ * Constants to represent text directionality
+ *
+ * Also defines a *global* direciton, to be used in bidi algorithms as a
+ * default fallback direciton, when no better direction is found or provided.
+ *
+ * NOTE: Use `setGlobalDir()`, or update `initGlobalDir()`, to set the initial
+ * global direction value based on the application.
+ *
+ * Part of the implementation of Unicode Bidirectional Algorithm (UBA)
+ * Unicode Standard Annex #9 (UAX9)
+ * http://www.unicode.org/reports/tr9/
+ */
+
+'use strict';
+
+const invariant = require('./invariant');
+
+export type BidiDirection = 'LTR' | 'RTL' | 'NEUTRAL';
+export type HTMLDir = 'ltr' | 'rtl';
+
+const NEUTRAL = 'NEUTRAL'; // No strong direction
+const LTR = 'LTR'; // Left-to-Right direction
+const RTL = 'RTL'; // Right-to-Left direction
+
+let globalDir: ?BidiDirection = null;
+
+// == Helpers ==
+
+/**
+ * Check if a directionality value is a Strong one
+ */
+function isStrong(dir: BidiDirection): boolean {
+ return dir === LTR || dir === RTL;
+}
+
+/**
+ * Get string value to be used for `dir` HTML attribute or `direction` CSS
+ * property.
+ */
+function getHTMLDir(dir: BidiDirection): HTMLDir {
+ invariant(isStrong(dir), '`dir` must be a strong direction to be converted to HTML Direction');
+ return dir === LTR ? 'ltr' : 'rtl';
+}
+
+/**
+ * Get string value to be used for `dir` HTML attribute or `direction` CSS
+ * property, but returns null if `dir` has same value as `otherDir`.
+ * `null`.
+ */
+function getHTMLDirIfDifferent(dir: BidiDirection, otherDir: BidiDirection): ?HTMLDir {
+ invariant(isStrong(dir), '`dir` must be a strong direction to be converted to HTML Direction');
+ invariant(isStrong(otherDir), '`otherDir` must be a strong direction to be converted to HTML Direction');
+ return dir === otherDir ? null : getHTMLDir(dir);
+}
+
+// == Global Direction ==
+
+/**
+ * Set the global direction.
+ */
+function setGlobalDir(dir: BidiDirection): void {
+ globalDir = dir;
+}
+
+/**
+ * Initialize the global direction
+ */
+function initGlobalDir(): void {
+ setGlobalDir(LTR);
+}
+
+/**
+ * Get the global direction
+ */
+function getGlobalDir(): BidiDirection {
+ if (!globalDir) {
+ this.initGlobalDir();
+ }
+ invariant(globalDir, 'Global direction not set.');
+ return globalDir;
+}
+
+const UnicodeBidiDirection = {
+ // Values
+ NEUTRAL,
+ LTR,
+ RTL,
+ // Helpers
+ isStrong,
+ getHTMLDir,
+ getHTMLDirIfDifferent,
+ // Global Direction
+ setGlobalDir,
+ initGlobalDir,
+ getGlobalDir
+};
+
+module.exports = UnicodeBidiDirection;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeBidiService.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeBidiService.js
new file mode 100644
index 00000000..ae9764ab
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeBidiService.js
@@ -0,0 +1,98 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @typechecks
+ *
+ */
+
+/**
+ * Stateful API for text direction detection
+ *
+ * This class can be used in applications where you need to detect the
+ * direction of a sequence of text blocks, where each direction shall be used
+ * as the fallback direction for the next one.
+ *
+ * NOTE: A default direction, if not provided, is set based on the global
+ * direction, as defined by `UnicodeBidiDirection`.
+ *
+ * == Example ==
+ * ```
+ * var UnicodeBidiService = require('UnicodeBidiService');
+ *
+ * var bidiService = new UnicodeBidiService();
+ *
+ * ...
+ *
+ * bidiService.reset();
+ * for (var para in paragraphs) {
+ * var dir = bidiService.getDirection(para);
+ * ...
+ * }
+ * ```
+ *
+ * Part of our implementation of Unicode Bidirectional Algorithm (UBA)
+ * Unicode Standard Annex #9 (UAX9)
+ * http://www.unicode.org/reports/tr9/
+ */
+
+'use strict';
+
+function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
+
+var UnicodeBidi = require('./UnicodeBidi');
+var UnicodeBidiDirection = require('./UnicodeBidiDirection');
+
+var invariant = require('./invariant');
+
+var UnicodeBidiService = function () {
+
+ /**
+ * Stateful class for paragraph direction detection
+ *
+ * @param defaultDir Default direction of the service
+ */
+ function UnicodeBidiService(defaultDir) {
+ _classCallCheck(this, UnicodeBidiService);
+
+ if (!defaultDir) {
+ defaultDir = UnicodeBidiDirection.getGlobalDir();
+ } else {
+ !UnicodeBidiDirection.isStrong(defaultDir) ? process.env.NODE_ENV !== 'production' ? invariant(false, 'Default direction must be a strong direction (LTR or RTL)') : invariant(false) : void 0;
+ }
+ this._defaultDir = defaultDir;
+ this.reset();
+ }
+
+ /**
+ * Reset the internal state
+ *
+ * Instead of creating a new instance, you can just reset() your instance
+ * everytime you start a new loop.
+ */
+
+
+ UnicodeBidiService.prototype.reset = function reset() {
+ this._lastDir = this._defaultDir;
+ };
+
+ /**
+ * Returns the direction of a block of text, and remembers it as the
+ * fall-back direction for the next paragraph.
+ *
+ * @param str A text block, e.g. paragraph, table cell, tag
+ * @return The resolved direction
+ */
+
+
+ UnicodeBidiService.prototype.getDirection = function getDirection(str) {
+ this._lastDir = UnicodeBidi.getDirection(str, this._lastDir);
+ return this._lastDir;
+ };
+
+ return UnicodeBidiService;
+}();
+
+module.exports = UnicodeBidiService;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeBidiService.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeBidiService.js.flow
new file mode 100644
index 00000000..7999a422
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeBidiService.js.flow
@@ -0,0 +1,95 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule UnicodeBidiService
+ * @typechecks
+ * @flow
+ */
+
+/**
+ * Stateful API for text direction detection
+ *
+ * This class can be used in applications where you need to detect the
+ * direction of a sequence of text blocks, where each direction shall be used
+ * as the fallback direction for the next one.
+ *
+ * NOTE: A default direction, if not provided, is set based on the global
+ * direction, as defined by `UnicodeBidiDirection`.
+ *
+ * == Example ==
+ * ```
+ * var UnicodeBidiService = require('UnicodeBidiService');
+ *
+ * var bidiService = new UnicodeBidiService();
+ *
+ * ...
+ *
+ * bidiService.reset();
+ * for (var para in paragraphs) {
+ * var dir = bidiService.getDirection(para);
+ * ...
+ * }
+ * ```
+ *
+ * Part of our implementation of Unicode Bidirectional Algorithm (UBA)
+ * Unicode Standard Annex #9 (UAX9)
+ * http://www.unicode.org/reports/tr9/
+ */
+
+'use strict';
+
+const UnicodeBidi = require('./UnicodeBidi');
+const UnicodeBidiDirection = require('./UnicodeBidiDirection');
+
+const invariant = require('./invariant');
+
+import type { BidiDirection } from './UnicodeBidiDirection';
+
+class UnicodeBidiService {
+
+ _defaultDir: BidiDirection;
+ _lastDir: BidiDirection;
+
+ /**
+ * Stateful class for paragraph direction detection
+ *
+ * @param defaultDir Default direction of the service
+ */
+ constructor(defaultDir: ?BidiDirection) {
+ if (!defaultDir) {
+ defaultDir = UnicodeBidiDirection.getGlobalDir();
+ } else {
+ invariant(UnicodeBidiDirection.isStrong(defaultDir), 'Default direction must be a strong direction (LTR or RTL)');
+ }
+ this._defaultDir = defaultDir;
+ this.reset();
+ }
+
+ /**
+ * Reset the internal state
+ *
+ * Instead of creating a new instance, you can just reset() your instance
+ * everytime you start a new loop.
+ */
+ reset(): void {
+ this._lastDir = this._defaultDir;
+ }
+
+ /**
+ * Returns the direction of a block of text, and remembers it as the
+ * fall-back direction for the next paragraph.
+ *
+ * @param str A text block, e.g. paragraph, table cell, tag
+ * @return The resolved direction
+ */
+ getDirection(str: string): BidiDirection {
+ this._lastDir = UnicodeBidi.getDirection(str, this._lastDir);
+ return this._lastDir;
+ }
+
+}
+
+module.exports = UnicodeBidiService;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeCJK.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeCJK.js
new file mode 100644
index 00000000..27d08bd8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeCJK.js
@@ -0,0 +1,172 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @typechecks
+ */
+
+/**
+ * Unicode algorithms for CJK (Chinese, Japanese, Korean) writing systems.
+ *
+ * Utilities for Hanzi/Kanji/Hanja logographs and Kanas (Katakana and Hiragana)
+ * syllables.
+ *
+ * For Korean Hangul see module `UnicodeHangulKorean`.
+ */
+
+'use strict';
+
+/**
+ * Latin
+ *
+ * NOTE: The code assumes these sets include only BMP characters.
+ */
+
+var R_LATIN_ASCII = 'a-zA-Z';
+var R_LATIN_FULLWIDTH = '\uFF21-\uFF3A\uFF41-\uFF5A';
+var R_LATIN = R_LATIN_ASCII + R_LATIN_FULLWIDTH;
+
+/**
+ * Hiragana & Katakana
+ *
+ * NOTE: Some ranges include non-BMP characters. We do not support those ranges
+ * for now.
+ */
+var R_HIRAGANA = '\u3040-\u309F';
+var R_KATAKANA = '\u30A0-\u30FF';
+var R_KATAKANA_PHONETIC = '\u31F0-\u31FF';
+var R_KATAKANA_HALFWIDTH = '\uFF65-\uFF9F';
+// var R_KANA_SUPPLEMENT = '\U0001B000-\U0001B0FF';
+var R_KATAKANA_ALL = R_KATAKANA + R_KATAKANA_PHONETIC + R_KATAKANA_HALFWIDTH;
+var R_KANA = R_HIRAGANA + R_KATAKANA_ALL;
+
+var I_HIRAGANA = [0x3040, 0x309F];
+var I_KATAKANA = [0x30A0, 0x30FF];
+var I_HIRAGANA_TO_KATAKANA = I_KATAKANA[0] - I_HIRAGANA[0];
+
+/**
+ * Hanzi/Kanji/Hanja
+ *
+ * NOTE: Some ranges include non-BMP characters. We do not support those ranges
+ * for now.
+ */
+var R_IDEO_MAIN = '\u4E00-\u9FCF';
+var R_IDEO_EXT_A = '\u3400-\u4DBF';
+// var R_IDEO_EXT_B = '\U00020000-\U0002A6DF';
+// var R_IDEO_EXT_C = '\U0002A700-\U0002B73F';
+// var R_IDEO_EXT_D = '\U0002B740-\U0002B81F';
+var R_IDEO = R_IDEO_MAIN + R_IDEO_EXT_A;
+
+/**
+ * Hangul
+ */
+// var R_HANGUL_JAMO = '\u1100-\u11FF';
+// var R_HANGUL_JAMO_EXT_A = '\uA960-\uA97F';
+// var R_HANGUL_JAMO_EXT_B = '\uD7B0-\uD7FF';
+// var R_HANGUL_COMPATIBILITY = '\u3130-\u318F';
+// var R_HANGUL_COMP_HALFWIDTH = '\uFFA0-\uFFDF';
+var R_HANGUL_SYLLABLES = '\uAC00-\uD7AF';
+
+/**
+ * Globals
+ */
+var R_IDEO_OR_SYLL = R_IDEO + R_KANA + R_HANGUL_SYLLABLES;
+
+var REGEX_IDEO = null;
+var REGEX_KANA = null;
+var REGEX_IDEO_OR_SYLL = null;
+var REGEX_IS_KANA_WITH_TRAILING_LATIN = null;
+
+/**
+ * Whether the string includes any Katakana or Hiragana characters.
+ *
+ * @param {string} str
+ * @return {boolean}
+ */
+function hasKana(str) {
+ REGEX_KANA = REGEX_KANA || new RegExp('[' + R_KANA + ']');
+ return REGEX_KANA.test(str);
+}
+
+/**
+ * Whether the string includes any CJK Ideograph characters.
+ *
+ * @param {string} str
+ * @return {boolean}
+ */
+function hasIdeograph(str) {
+ REGEX_IDEO = REGEX_IDEO || new RegExp('[' + R_IDEO + ']');
+ return REGEX_IDEO.test(str);
+}
+
+/**
+ * Whether the string includes any CJK Ideograph or Syllable characters.
+ *
+ * @param {string} str
+ * @return {boolean}
+ */
+function hasIdeoOrSyll(str) {
+ REGEX_IDEO_OR_SYLL = REGEX_IDEO_OR_SYLL || new RegExp('[' + R_IDEO_OR_SYLL + ']');
+ return REGEX_IDEO_OR_SYLL.test(str);
+}
+
+/**
+ * @param {string} chr
+ * @output {string}
+ */
+function charCodeToKatakana(chr) {
+ var charCode = chr.charCodeAt(0);
+ return String.fromCharCode(charCode < I_HIRAGANA[0] || charCode > I_HIRAGANA[1] ? charCode : charCode + I_HIRAGANA_TO_KATAKANA);
+}
+
+/**
+ * Replace any Hiragana character with the matching Katakana
+ *
+ * @param {string} str
+ * @output {string}
+ */
+function hiraganaToKatakana(str) {
+ if (!hasKana(str)) {
+ return str;
+ }
+ return str.split('').map(charCodeToKatakana).join('');
+}
+
+/**
+ * Whether the string is exactly a sequence of Kana characters followed by one
+ * Latin character.
+ *
+ * @param {string} str
+ * @output {string}
+ */
+function isKanaWithTrailingLatin(str) {
+ REGEX_IS_KANA_WITH_TRAILING_LATIN = REGEX_IS_KANA_WITH_TRAILING_LATIN || new RegExp('^' + '[' + R_KANA + ']+' + '[' + R_LATIN + ']' + '$');
+ return REGEX_IS_KANA_WITH_TRAILING_LATIN.test(str);
+}
+
+/**
+ * Drops the trailing Latin character from a string that is exactly a sequence
+ * of Kana characters followed by one Latin character.
+ *
+ * @param {string} str
+ * @output {string}
+ */
+function kanaRemoveTrailingLatin(str) {
+ if (isKanaWithTrailingLatin(str)) {
+ return str.substr(0, str.length - 1);
+ }
+ return str;
+}
+
+var UnicodeCJK = {
+ hasKana: hasKana,
+ hasIdeograph: hasIdeograph,
+ hasIdeoOrSyll: hasIdeoOrSyll,
+ hiraganaToKatakana: hiraganaToKatakana,
+ isKanaWithTrailingLatin: isKanaWithTrailingLatin,
+ kanaRemoveTrailingLatin: kanaRemoveTrailingLatin
+};
+
+module.exports = UnicodeCJK;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeCJK.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeCJK.js.flow
new file mode 100644
index 00000000..d1b47cac
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeCJK.js.flow
@@ -0,0 +1,173 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule UnicodeCJK
+ * @typechecks
+ */
+
+/**
+ * Unicode algorithms for CJK (Chinese, Japanese, Korean) writing systems.
+ *
+ * Utilities for Hanzi/Kanji/Hanja logographs and Kanas (Katakana and Hiragana)
+ * syllables.
+ *
+ * For Korean Hangul see module `UnicodeHangulKorean`.
+ */
+
+'use strict';
+
+/**
+ * Latin
+ *
+ * NOTE: The code assumes these sets include only BMP characters.
+ */
+
+const R_LATIN_ASCII = 'a-zA-Z';
+const R_LATIN_FULLWIDTH = '\uFF21-\uFF3A\uFF41-\uFF5A';
+const R_LATIN = R_LATIN_ASCII + R_LATIN_FULLWIDTH;
+
+/**
+ * Hiragana & Katakana
+ *
+ * NOTE: Some ranges include non-BMP characters. We do not support those ranges
+ * for now.
+ */
+const R_HIRAGANA = '\u3040-\u309F';
+const R_KATAKANA = '\u30A0-\u30FF';
+const R_KATAKANA_PHONETIC = '\u31F0-\u31FF';
+const R_KATAKANA_HALFWIDTH = '\uFF65-\uFF9F';
+// var R_KANA_SUPPLEMENT = '\U0001B000-\U0001B0FF';
+const R_KATAKANA_ALL = R_KATAKANA + R_KATAKANA_PHONETIC + R_KATAKANA_HALFWIDTH;
+const R_KANA = R_HIRAGANA + R_KATAKANA_ALL;
+
+const I_HIRAGANA = [0x3040, 0x309F];
+const I_KATAKANA = [0x30A0, 0x30FF];
+const I_HIRAGANA_TO_KATAKANA = I_KATAKANA[0] - I_HIRAGANA[0];
+
+/**
+ * Hanzi/Kanji/Hanja
+ *
+ * NOTE: Some ranges include non-BMP characters. We do not support those ranges
+ * for now.
+ */
+const R_IDEO_MAIN = '\u4E00-\u9FCF';
+const R_IDEO_EXT_A = '\u3400-\u4DBF';
+// var R_IDEO_EXT_B = '\U00020000-\U0002A6DF';
+// var R_IDEO_EXT_C = '\U0002A700-\U0002B73F';
+// var R_IDEO_EXT_D = '\U0002B740-\U0002B81F';
+const R_IDEO = R_IDEO_MAIN + R_IDEO_EXT_A;
+
+/**
+ * Hangul
+ */
+// var R_HANGUL_JAMO = '\u1100-\u11FF';
+// var R_HANGUL_JAMO_EXT_A = '\uA960-\uA97F';
+// var R_HANGUL_JAMO_EXT_B = '\uD7B0-\uD7FF';
+// var R_HANGUL_COMPATIBILITY = '\u3130-\u318F';
+// var R_HANGUL_COMP_HALFWIDTH = '\uFFA0-\uFFDF';
+const R_HANGUL_SYLLABLES = '\uAC00-\uD7AF';
+
+/**
+ * Globals
+ */
+const R_IDEO_OR_SYLL = R_IDEO + R_KANA + R_HANGUL_SYLLABLES;
+
+let REGEX_IDEO = null;
+let REGEX_KANA = null;
+let REGEX_IDEO_OR_SYLL = null;
+let REGEX_IS_KANA_WITH_TRAILING_LATIN = null;
+
+/**
+ * Whether the string includes any Katakana or Hiragana characters.
+ *
+ * @param {string} str
+ * @return {boolean}
+ */
+function hasKana(str) {
+ REGEX_KANA = REGEX_KANA || new RegExp('[' + R_KANA + ']');
+ return REGEX_KANA.test(str);
+}
+
+/**
+ * Whether the string includes any CJK Ideograph characters.
+ *
+ * @param {string} str
+ * @return {boolean}
+ */
+function hasIdeograph(str) {
+ REGEX_IDEO = REGEX_IDEO || new RegExp('[' + R_IDEO + ']');
+ return REGEX_IDEO.test(str);
+}
+
+/**
+ * Whether the string includes any CJK Ideograph or Syllable characters.
+ *
+ * @param {string} str
+ * @return {boolean}
+ */
+function hasIdeoOrSyll(str) {
+ REGEX_IDEO_OR_SYLL = REGEX_IDEO_OR_SYLL || new RegExp('[' + R_IDEO_OR_SYLL + ']');
+ return REGEX_IDEO_OR_SYLL.test(str);
+}
+
+/**
+ * @param {string} chr
+ * @output {string}
+ */
+function charCodeToKatakana(chr) {
+ const charCode = chr.charCodeAt(0);
+ return String.fromCharCode(charCode < I_HIRAGANA[0] || charCode > I_HIRAGANA[1] ? charCode : charCode + I_HIRAGANA_TO_KATAKANA);
+}
+
+/**
+ * Replace any Hiragana character with the matching Katakana
+ *
+ * @param {string} str
+ * @output {string}
+ */
+function hiraganaToKatakana(str) {
+ if (!hasKana(str)) {
+ return str;
+ }
+ return str.split('').map(charCodeToKatakana).join('');
+}
+
+/**
+ * Whether the string is exactly a sequence of Kana characters followed by one
+ * Latin character.
+ *
+ * @param {string} str
+ * @output {string}
+ */
+function isKanaWithTrailingLatin(str) {
+ REGEX_IS_KANA_WITH_TRAILING_LATIN = REGEX_IS_KANA_WITH_TRAILING_LATIN || new RegExp('^' + '[' + R_KANA + ']+' + '[' + R_LATIN + ']' + '$');
+ return REGEX_IS_KANA_WITH_TRAILING_LATIN.test(str);
+}
+
+/**
+ * Drops the trailing Latin character from a string that is exactly a sequence
+ * of Kana characters followed by one Latin character.
+ *
+ * @param {string} str
+ * @output {string}
+ */
+function kanaRemoveTrailingLatin(str) {
+ if (isKanaWithTrailingLatin(str)) {
+ return str.substr(0, str.length - 1);
+ }
+ return str;
+}
+
+const UnicodeCJK = {
+ hasKana: hasKana,
+ hasIdeograph: hasIdeograph,
+ hasIdeoOrSyll: hasIdeoOrSyll,
+ hiraganaToKatakana: hiraganaToKatakana,
+ isKanaWithTrailingLatin: isKanaWithTrailingLatin,
+ kanaRemoveTrailingLatin: kanaRemoveTrailingLatin
+};
+
+module.exports = UnicodeCJK;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeHangulKorean.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeHangulKorean.js
new file mode 100644
index 00000000..f67bd3ce
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeHangulKorean.js
@@ -0,0 +1,135 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @typechecks
+ */
+
+/**
+ * Unicode algorithms for Hangul script, the Korean writing system
+ *
+ * Hangul script has three encoded models in Unicode:
+ *
+ * A) Conjoining Jamo (covers modern and historic elements)
+ * * U+1100..U+11FF ; Hangul Jamo
+ * * U+A960..U+A97F ; Hangul Jamo Extended-A
+ * * U+D7B0..U+D7FF ; Hangul Jamo Extended-B
+ *
+ * B) Conjoined Syllables (only covers modern Korean language)
+ * * U+AC00..U+D7AF ; Hangul Syllables
+ *
+ * C) Compatibility Jamo (one code-point for each "shape")
+ * * U+3130..U+318F ; Hangul Compatibility Jamo
+ *
+ * This modules helps you convert characters from one model to another.
+ * Primary functionalities are:
+ *
+ * 1) Convert from any encodings to Conjoining Jamo characters (A),
+ * e.g. for prefix matching
+ *
+ * 2) Convert from any encodings to Syllable characters, when possible (B),
+ * e.g. to reach the normal Unicode form (NFC)
+ */
+
+'use strict';
+
+var HANGUL_COMPATIBILITY_OR_SYLLABLE_REGEX = /[\u3130-\u318F\uAC00-\uD7AF]/;
+
+/**
+ * Returns true if the input includes any Hangul Compatibility Jamo or
+ * Hangul Conjoined Syllable.
+ *
+ * @param {string} str
+ */
+function hasCompatibilityOrSyllable(str) {
+ return HANGUL_COMPATIBILITY_OR_SYLLABLE_REGEX.test(str);
+}
+
+/* Compatibility Jamo -> Conjoining Jamo
+ *
+ * Maps a compatibility character to the Conjoining Jamo character,
+ * positioned at (compatibilityCodePoint - 0x3131).
+ *
+ * Generated by:
+ * $ grep '^31[3-8].;' UnicodeData.txt |\
+ * awk -F';' '{print $6}' | awk '{print " 0x"$2","}'
+ */
+var CMAP = [0x1100, 0x1101, 0x11AA, 0x1102, 0x11AC, 0x11AD, 0x1103, 0x1104, 0x1105, 0x11B0, 0x11B1, 0x11B2, 0x11B3, 0x11B4, 0x11B5, 0x111A, 0x1106, 0x1107, 0x1108, 0x1121, 0x1109, 0x110A, 0x110B, 0x110C, 0x110D, 0x110E, 0x110F, 0x1110, 0x1111, 0x1112, 0x1161, 0x1162, 0x1163, 0x1164, 0x1165, 0x1166, 0x1167, 0x1168, 0x1169, 0x116A, 0x116B, 0x116C, 0x116D, 0x116E, 0x116F, 0x1170, 0x1171, 0x1172, 0x1173, 0x1174, 0x1175, 0x1160, 0x1114, 0x1115, 0x11C7, 0x11C8, 0x11CC, 0x11CE, 0x11D3, 0x11D7, 0x11D9, 0x111C, 0x11DD, 0x11DF, 0x111D, 0x111E, 0x1120, 0x1122, 0x1123, 0x1127, 0x1129, 0x112B, 0x112C, 0x112D, 0x112E, 0x112F, 0x1132, 0x1136, 0x1140, 0x1147, 0x114C, 0x11F1, 0x11F2, 0x1157, 0x1158, 0x1159, 0x1184, 0x1185, 0x1188, 0x1191, 0x1192, 0x1194, 0x119E, 0x11A1];
+
+var CBASE = 0x3131;
+var CCOUNT = CMAP.length;
+var CTOP = CBASE + CCOUNT;
+
+/**
+ * Maps one Hangul Compatibility Jamo code-point to the equivalent Hangul
+ * Conjoining Jamo characters, as defined in UnicodeData.txt.
+ *
+ * @param {number} codePoint One Unicode code-point
+ * @output {string}
+ */
+function fromCompatibility(codePoint) {
+ return String.fromCharCode(CMAP[codePoint - CBASE]);
+}
+
+/**
+ * Conjoined Syllable -> Conjoining Jamo
+ *
+ * Based on the "Hangul Syllable Decomposition" algorithm provided in
+ * 3.12 Conjoining Jamo Behavior, The Unicode Standard, Version 6.3.0.
+ *
+ */
+
+var LBASE = 0x1100;
+var VBASE = 0x1161;
+var TBASE = 0x11A7;
+var SBASE = 0xAC00;
+var LCOUNT = 19;
+var VCOUNT = 21;
+var TCOUNT = 28;
+var NCOUNT = VCOUNT * TCOUNT;
+var SCOUNT = LCOUNT * NCOUNT;
+var STOP = SBASE + SCOUNT;
+
+/**
+ * Maps one Hangul Syllable code-point to the equivalent Hangul
+ * Conjoining Jamo characters, as defined in UnicodeData.txt.
+ *
+ * @param {number} codePoint One Unicode character
+ * @output {string}
+ */
+function decomposeSyllable(codePoint) {
+ var sylSIndex = codePoint - SBASE;
+ var sylTIndex = sylSIndex % TCOUNT;
+ return String.fromCharCode(LBASE + sylSIndex / NCOUNT) + String.fromCharCode(VBASE + sylSIndex % NCOUNT / TCOUNT) + (sylTIndex > 0 ? String.fromCharCode(TBASE + sylTIndex) : '');
+}
+
+/* To Conjoining Jamo */
+
+/**
+ * Return Unicode characters as they are, except for Hangul characters, which
+ * will be converted to the Conjoining Jamo form.
+ *
+ * @param {string} string
+ * @output {string}
+ */
+function toConjoiningJamo(string) {
+ if (!hasCompatibilityOrSyllable(string)) {
+ return string;
+ }
+
+ var result = [];
+ for (var i = 0; i < string.length; i++) {
+ var charStr = string.charAt(i);
+ var codeUnit = charStr.charCodeAt(0);
+ result.push(CBASE <= codeUnit && codeUnit < CTOP ? fromCompatibility(codeUnit) : SBASE <= codeUnit && codeUnit < STOP ? decomposeSyllable(codeUnit) : charStr);
+ }
+ return result.join('');
+}
+
+var UnicodeHangulKorean = {
+ toConjoiningJamo: toConjoiningJamo
+};
+
+module.exports = UnicodeHangulKorean;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeHangulKorean.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeHangulKorean.js.flow
new file mode 100644
index 00000000..d184b580
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeHangulKorean.js.flow
@@ -0,0 +1,136 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule UnicodeHangulKorean
+ * @typechecks
+ */
+
+/**
+ * Unicode algorithms for Hangul script, the Korean writing system
+ *
+ * Hangul script has three encoded models in Unicode:
+ *
+ * A) Conjoining Jamo (covers modern and historic elements)
+ * * U+1100..U+11FF ; Hangul Jamo
+ * * U+A960..U+A97F ; Hangul Jamo Extended-A
+ * * U+D7B0..U+D7FF ; Hangul Jamo Extended-B
+ *
+ * B) Conjoined Syllables (only covers modern Korean language)
+ * * U+AC00..U+D7AF ; Hangul Syllables
+ *
+ * C) Compatibility Jamo (one code-point for each "shape")
+ * * U+3130..U+318F ; Hangul Compatibility Jamo
+ *
+ * This modules helps you convert characters from one model to another.
+ * Primary functionalities are:
+ *
+ * 1) Convert from any encodings to Conjoining Jamo characters (A),
+ * e.g. for prefix matching
+ *
+ * 2) Convert from any encodings to Syllable characters, when possible (B),
+ * e.g. to reach the normal Unicode form (NFC)
+ */
+
+'use strict';
+
+const HANGUL_COMPATIBILITY_OR_SYLLABLE_REGEX = /[\u3130-\u318F\uAC00-\uD7AF]/;
+
+/**
+ * Returns true if the input includes any Hangul Compatibility Jamo or
+ * Hangul Conjoined Syllable.
+ *
+ * @param {string} str
+ */
+function hasCompatibilityOrSyllable(str) {
+ return HANGUL_COMPATIBILITY_OR_SYLLABLE_REGEX.test(str);
+}
+
+/* Compatibility Jamo -> Conjoining Jamo
+ *
+ * Maps a compatibility character to the Conjoining Jamo character,
+ * positioned at (compatibilityCodePoint - 0x3131).
+ *
+ * Generated by:
+ * $ grep '^31[3-8].;' UnicodeData.txt |\
+ * awk -F';' '{print $6}' | awk '{print " 0x"$2","}'
+ */
+const CMAP = [0x1100, 0x1101, 0x11AA, 0x1102, 0x11AC, 0x11AD, 0x1103, 0x1104, 0x1105, 0x11B0, 0x11B1, 0x11B2, 0x11B3, 0x11B4, 0x11B5, 0x111A, 0x1106, 0x1107, 0x1108, 0x1121, 0x1109, 0x110A, 0x110B, 0x110C, 0x110D, 0x110E, 0x110F, 0x1110, 0x1111, 0x1112, 0x1161, 0x1162, 0x1163, 0x1164, 0x1165, 0x1166, 0x1167, 0x1168, 0x1169, 0x116A, 0x116B, 0x116C, 0x116D, 0x116E, 0x116F, 0x1170, 0x1171, 0x1172, 0x1173, 0x1174, 0x1175, 0x1160, 0x1114, 0x1115, 0x11C7, 0x11C8, 0x11CC, 0x11CE, 0x11D3, 0x11D7, 0x11D9, 0x111C, 0x11DD, 0x11DF, 0x111D, 0x111E, 0x1120, 0x1122, 0x1123, 0x1127, 0x1129, 0x112B, 0x112C, 0x112D, 0x112E, 0x112F, 0x1132, 0x1136, 0x1140, 0x1147, 0x114C, 0x11F1, 0x11F2, 0x1157, 0x1158, 0x1159, 0x1184, 0x1185, 0x1188, 0x1191, 0x1192, 0x1194, 0x119E, 0x11A1];
+
+const CBASE = 0x3131;
+const CCOUNT = CMAP.length;
+const CTOP = CBASE + CCOUNT;
+
+/**
+ * Maps one Hangul Compatibility Jamo code-point to the equivalent Hangul
+ * Conjoining Jamo characters, as defined in UnicodeData.txt.
+ *
+ * @param {number} codePoint One Unicode code-point
+ * @output {string}
+ */
+function fromCompatibility(codePoint) {
+ return String.fromCharCode(CMAP[codePoint - CBASE]);
+}
+
+/**
+ * Conjoined Syllable -> Conjoining Jamo
+ *
+ * Based on the "Hangul Syllable Decomposition" algorithm provided in
+ * 3.12 Conjoining Jamo Behavior, The Unicode Standard, Version 6.3.0.
+ *
+ */
+
+const LBASE = 0x1100;
+const VBASE = 0x1161;
+const TBASE = 0x11A7;
+const SBASE = 0xAC00;
+const LCOUNT = 19;
+const VCOUNT = 21;
+const TCOUNT = 28;
+const NCOUNT = VCOUNT * TCOUNT;
+const SCOUNT = LCOUNT * NCOUNT;
+const STOP = SBASE + SCOUNT;
+
+/**
+ * Maps one Hangul Syllable code-point to the equivalent Hangul
+ * Conjoining Jamo characters, as defined in UnicodeData.txt.
+ *
+ * @param {number} codePoint One Unicode character
+ * @output {string}
+ */
+function decomposeSyllable(codePoint) {
+ const sylSIndex = codePoint - SBASE;
+ const sylTIndex = sylSIndex % TCOUNT;
+ return String.fromCharCode(LBASE + sylSIndex / NCOUNT) + String.fromCharCode(VBASE + sylSIndex % NCOUNT / TCOUNT) + (sylTIndex > 0 ? String.fromCharCode(TBASE + sylTIndex) : '');
+}
+
+/* To Conjoining Jamo */
+
+/**
+ * Return Unicode characters as they are, except for Hangul characters, which
+ * will be converted to the Conjoining Jamo form.
+ *
+ * @param {string} string
+ * @output {string}
+ */
+function toConjoiningJamo(string) {
+ if (!hasCompatibilityOrSyllable(string)) {
+ return string;
+ }
+
+ const result = [];
+ for (let i = 0; i < string.length; i++) {
+ const charStr = string.charAt(i);
+ const codeUnit = charStr.charCodeAt(0);
+ result.push(CBASE <= codeUnit && codeUnit < CTOP ? fromCompatibility(codeUnit) : SBASE <= codeUnit && codeUnit < STOP ? decomposeSyllable(codeUnit) : charStr);
+ }
+ return result.join('');
+}
+
+const UnicodeHangulKorean = {
+ toConjoiningJamo: toConjoiningJamo
+};
+
+module.exports = UnicodeHangulKorean;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeUtils.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeUtils.js
new file mode 100644
index 00000000..72bb75d0
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeUtils.js
@@ -0,0 +1,212 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @typechecks
+ */
+
+/**
+ * Unicode-enabled replacesments for basic String functions.
+ *
+ * All the functions in this module assume that the input string is a valid
+ * UTF-16 encoding of a Unicode sequence. If it's not the case, the behavior
+ * will be undefined.
+ *
+ * WARNING: Since this module is typechecks-enforced, you may find new bugs
+ * when replacing normal String functions with ones provided here.
+ */
+
+'use strict';
+
+var invariant = require('./invariant');
+
+// These two ranges are consecutive so anything in [HIGH_START, LOW_END] is a
+// surrogate code unit.
+var SURROGATE_HIGH_START = 0xD800;
+var SURROGATE_HIGH_END = 0xDBFF;
+var SURROGATE_LOW_START = 0xDC00;
+var SURROGATE_LOW_END = 0xDFFF;
+var SURROGATE_UNITS_REGEX = /[\uD800-\uDFFF]/;
+
+/**
+ * @param {number} codeUnit A Unicode code-unit, in range [0, 0x10FFFF]
+ * @return {boolean} Whether code-unit is in a surrogate (hi/low) range
+ */
+function isCodeUnitInSurrogateRange(codeUnit) {
+ return SURROGATE_HIGH_START <= codeUnit && codeUnit <= SURROGATE_LOW_END;
+}
+
+/**
+ * Returns whether the two characters starting at `index` form a surrogate pair.
+ * For example, given the string s = "\uD83D\uDE0A", (s, 0) returns true and
+ * (s, 1) returns false.
+ *
+ * @param {string} str
+ * @param {number} index
+ * @return {boolean}
+ */
+function isSurrogatePair(str, index) {
+ !(0 <= index && index < str.length) ? process.env.NODE_ENV !== 'production' ? invariant(false, 'isSurrogatePair: Invalid index %s for string length %s.', index, str.length) : invariant(false) : void 0;
+ if (index + 1 === str.length) {
+ return false;
+ }
+ var first = str.charCodeAt(index);
+ var second = str.charCodeAt(index + 1);
+ return SURROGATE_HIGH_START <= first && first <= SURROGATE_HIGH_END && SURROGATE_LOW_START <= second && second <= SURROGATE_LOW_END;
+}
+
+/**
+ * @param {string} str Non-empty string
+ * @return {boolean} True if the input includes any surrogate code units
+ */
+function hasSurrogateUnit(str) {
+ return SURROGATE_UNITS_REGEX.test(str);
+}
+
+/**
+ * Return the length of the original Unicode character at given position in the
+ * String by looking into the UTF-16 code unit; that is equal to 1 for any
+ * non-surrogate characters in BMP ([U+0000..U+D7FF] and [U+E000, U+FFFF]); and
+ * returns 2 for the hi/low surrogates ([U+D800..U+DFFF]), which are in fact
+ * representing non-BMP characters ([U+10000..U+10FFFF]).
+ *
+ * Examples:
+ * - '\u0020' => 1
+ * - '\u3020' => 1
+ * - '\uD835' => 2
+ * - '\uD835\uDDEF' => 2
+ * - '\uDDEF' => 2
+ *
+ * @param {string} str Non-empty string
+ * @param {number} pos Position in the string to look for one code unit
+ * @return {number} Number 1 or 2
+ */
+function getUTF16Length(str, pos) {
+ return 1 + isCodeUnitInSurrogateRange(str.charCodeAt(pos));
+}
+
+/**
+ * Fully Unicode-enabled replacement for String#length
+ *
+ * @param {string} str Valid Unicode string
+ * @return {number} The number of Unicode characters in the string
+ */
+function strlen(str) {
+ // Call the native functions if there's no surrogate char
+ if (!hasSurrogateUnit(str)) {
+ return str.length;
+ }
+
+ var len = 0;
+ for (var pos = 0; pos < str.length; pos += getUTF16Length(str, pos)) {
+ len++;
+ }
+ return len;
+}
+
+/**
+ * Fully Unicode-enabled replacement for String#substr()
+ *
+ * @param {string} str Valid Unicode string
+ * @param {number} start Location in Unicode sequence to begin extracting
+ * @param {?number} length The number of Unicode characters to extract
+ * (default: to the end of the string)
+ * @return {string} Extracted sub-string
+ */
+function substr(str, start, length) {
+ start = start || 0;
+ length = length === undefined ? Infinity : length || 0;
+
+ // Call the native functions if there's no surrogate char
+ if (!hasSurrogateUnit(str)) {
+ return str.substr(start, length);
+ }
+
+ // Obvious cases
+ var size = str.length;
+ if (size <= 0 || start > size || length <= 0) {
+ return '';
+ }
+
+ // Find the actual starting position
+ var posA = 0;
+ if (start > 0) {
+ for (; start > 0 && posA < size; start--) {
+ posA += getUTF16Length(str, posA);
+ }
+ if (posA >= size) {
+ return '';
+ }
+ } else if (start < 0) {
+ for (posA = size; start < 0 && 0 < posA; start++) {
+ posA -= getUTF16Length(str, posA - 1);
+ }
+ if (posA < 0) {
+ posA = 0;
+ }
+ }
+
+ // Find the actual ending position
+ var posB = size;
+ if (length < size) {
+ for (posB = posA; length > 0 && posB < size; length--) {
+ posB += getUTF16Length(str, posB);
+ }
+ }
+
+ return str.substring(posA, posB);
+}
+
+/**
+ * Fully Unicode-enabled replacement for String#substring()
+ *
+ * @param {string} str Valid Unicode string
+ * @param {number} start Location in Unicode sequence to begin extracting
+ * @param {?number} end Location in Unicode sequence to end extracting
+ * (default: end of the string)
+ * @return {string} Extracted sub-string
+ */
+function substring(str, start, end) {
+ start = start || 0;
+ end = end === undefined ? Infinity : end || 0;
+
+ if (start < 0) {
+ start = 0;
+ }
+ if (end < 0) {
+ end = 0;
+ }
+
+ var length = Math.abs(end - start);
+ start = start < end ? start : end;
+ return substr(str, start, length);
+}
+
+/**
+ * Get a list of Unicode code-points from a String
+ *
+ * @param {string} str Valid Unicode string
+ * @return {array} A list of code-points in [0..0x10FFFF]
+ */
+function getCodePoints(str) {
+ var codePoints = [];
+ for (var pos = 0; pos < str.length; pos += getUTF16Length(str, pos)) {
+ codePoints.push(str.codePointAt(pos));
+ }
+ return codePoints;
+}
+
+var UnicodeUtils = {
+ getCodePoints: getCodePoints,
+ getUTF16Length: getUTF16Length,
+ hasSurrogateUnit: hasSurrogateUnit,
+ isCodeUnitInSurrogateRange: isCodeUnitInSurrogateRange,
+ isSurrogatePair: isSurrogatePair,
+ strlen: strlen,
+ substring: substring,
+ substr: substr
+};
+
+module.exports = UnicodeUtils;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeUtils.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeUtils.js.flow
new file mode 100644
index 00000000..a0eebbc8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeUtils.js.flow
@@ -0,0 +1,213 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule UnicodeUtils
+ * @typechecks
+ */
+
+/**
+ * Unicode-enabled replacesments for basic String functions.
+ *
+ * All the functions in this module assume that the input string is a valid
+ * UTF-16 encoding of a Unicode sequence. If it's not the case, the behavior
+ * will be undefined.
+ *
+ * WARNING: Since this module is typechecks-enforced, you may find new bugs
+ * when replacing normal String functions with ones provided here.
+ */
+
+'use strict';
+
+const invariant = require('./invariant');
+
+// These two ranges are consecutive so anything in [HIGH_START, LOW_END] is a
+// surrogate code unit.
+const SURROGATE_HIGH_START = 0xD800;
+const SURROGATE_HIGH_END = 0xDBFF;
+const SURROGATE_LOW_START = 0xDC00;
+const SURROGATE_LOW_END = 0xDFFF;
+const SURROGATE_UNITS_REGEX = /[\uD800-\uDFFF]/;
+
+/**
+ * @param {number} codeUnit A Unicode code-unit, in range [0, 0x10FFFF]
+ * @return {boolean} Whether code-unit is in a surrogate (hi/low) range
+ */
+function isCodeUnitInSurrogateRange(codeUnit) {
+ return SURROGATE_HIGH_START <= codeUnit && codeUnit <= SURROGATE_LOW_END;
+}
+
+/**
+ * Returns whether the two characters starting at `index` form a surrogate pair.
+ * For example, given the string s = "\uD83D\uDE0A", (s, 0) returns true and
+ * (s, 1) returns false.
+ *
+ * @param {string} str
+ * @param {number} index
+ * @return {boolean}
+ */
+function isSurrogatePair(str, index) {
+ invariant(0 <= index && index < str.length, 'isSurrogatePair: Invalid index %s for string length %s.', index, str.length);
+ if (index + 1 === str.length) {
+ return false;
+ }
+ const first = str.charCodeAt(index);
+ const second = str.charCodeAt(index + 1);
+ return SURROGATE_HIGH_START <= first && first <= SURROGATE_HIGH_END && SURROGATE_LOW_START <= second && second <= SURROGATE_LOW_END;
+}
+
+/**
+ * @param {string} str Non-empty string
+ * @return {boolean} True if the input includes any surrogate code units
+ */
+function hasSurrogateUnit(str) {
+ return SURROGATE_UNITS_REGEX.test(str);
+}
+
+/**
+ * Return the length of the original Unicode character at given position in the
+ * String by looking into the UTF-16 code unit; that is equal to 1 for any
+ * non-surrogate characters in BMP ([U+0000..U+D7FF] and [U+E000, U+FFFF]); and
+ * returns 2 for the hi/low surrogates ([U+D800..U+DFFF]), which are in fact
+ * representing non-BMP characters ([U+10000..U+10FFFF]).
+ *
+ * Examples:
+ * - '\u0020' => 1
+ * - '\u3020' => 1
+ * - '\uD835' => 2
+ * - '\uD835\uDDEF' => 2
+ * - '\uDDEF' => 2
+ *
+ * @param {string} str Non-empty string
+ * @param {number} pos Position in the string to look for one code unit
+ * @return {number} Number 1 or 2
+ */
+function getUTF16Length(str, pos) {
+ return 1 + isCodeUnitInSurrogateRange(str.charCodeAt(pos));
+}
+
+/**
+ * Fully Unicode-enabled replacement for String#length
+ *
+ * @param {string} str Valid Unicode string
+ * @return {number} The number of Unicode characters in the string
+ */
+function strlen(str) {
+ // Call the native functions if there's no surrogate char
+ if (!hasSurrogateUnit(str)) {
+ return str.length;
+ }
+
+ let len = 0;
+ for (let pos = 0; pos < str.length; pos += getUTF16Length(str, pos)) {
+ len++;
+ }
+ return len;
+}
+
+/**
+ * Fully Unicode-enabled replacement for String#substr()
+ *
+ * @param {string} str Valid Unicode string
+ * @param {number} start Location in Unicode sequence to begin extracting
+ * @param {?number} length The number of Unicode characters to extract
+ * (default: to the end of the string)
+ * @return {string} Extracted sub-string
+ */
+function substr(str, start, length) {
+ start = start || 0;
+ length = length === undefined ? Infinity : length || 0;
+
+ // Call the native functions if there's no surrogate char
+ if (!hasSurrogateUnit(str)) {
+ return str.substr(start, length);
+ }
+
+ // Obvious cases
+ const size = str.length;
+ if (size <= 0 || start > size || length <= 0) {
+ return '';
+ }
+
+ // Find the actual starting position
+ let posA = 0;
+ if (start > 0) {
+ for (; start > 0 && posA < size; start--) {
+ posA += getUTF16Length(str, posA);
+ }
+ if (posA >= size) {
+ return '';
+ }
+ } else if (start < 0) {
+ for (posA = size; start < 0 && 0 < posA; start++) {
+ posA -= getUTF16Length(str, posA - 1);
+ }
+ if (posA < 0) {
+ posA = 0;
+ }
+ }
+
+ // Find the actual ending position
+ let posB = size;
+ if (length < size) {
+ for (posB = posA; length > 0 && posB < size; length--) {
+ posB += getUTF16Length(str, posB);
+ }
+ }
+
+ return str.substring(posA, posB);
+}
+
+/**
+ * Fully Unicode-enabled replacement for String#substring()
+ *
+ * @param {string} str Valid Unicode string
+ * @param {number} start Location in Unicode sequence to begin extracting
+ * @param {?number} end Location in Unicode sequence to end extracting
+ * (default: end of the string)
+ * @return {string} Extracted sub-string
+ */
+function substring(str, start, end) {
+ start = start || 0;
+ end = end === undefined ? Infinity : end || 0;
+
+ if (start < 0) {
+ start = 0;
+ }
+ if (end < 0) {
+ end = 0;
+ }
+
+ const length = Math.abs(end - start);
+ start = start < end ? start : end;
+ return substr(str, start, length);
+}
+
+/**
+ * Get a list of Unicode code-points from a String
+ *
+ * @param {string} str Valid Unicode string
+ * @return {array} A list of code-points in [0..0x10FFFF]
+ */
+function getCodePoints(str) {
+ const codePoints = [];
+ for (let pos = 0; pos < str.length; pos += getUTF16Length(str, pos)) {
+ codePoints.push(str.codePointAt(pos));
+ }
+ return codePoints;
+}
+
+const UnicodeUtils = {
+ getCodePoints: getCodePoints,
+ getUTF16Length: getUTF16Length,
+ hasSurrogateUnit: hasSurrogateUnit,
+ isCodeUnitInSurrogateRange: isCodeUnitInSurrogateRange,
+ isSurrogatePair: isSurrogatePair,
+ strlen: strlen,
+ substring: substring,
+ substr: substr
+};
+
+module.exports = UnicodeUtils;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeUtilsExtra.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeUtilsExtra.js
new file mode 100644
index 00000000..d5fb9444
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeUtilsExtra.js
@@ -0,0 +1,227 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @typechecks
+ */
+
+/**
+ * Unicode-enabled extra utility functions not always needed.
+ */
+
+'use strict';
+
+var UnicodeUtils = require('./UnicodeUtils');
+
+/**
+ * @param {number} codePoint Valid Unicode code-point
+ * @param {number} len Zero-padded minimum width of result
+ * @return {string} A zero-padded hexadecimal string (00XXXX)
+ */
+function zeroPaddedHex(codePoint, len) {
+ var codePointHex = codePoint.toString(16).toUpperCase();
+ var numZeros = Math.max(0, len - codePointHex.length);
+ var result = '';
+ for (var i = 0; i < numZeros; i++) {
+ result += '0';
+ }
+ result += codePointHex;
+ return result;
+}
+
+/**
+ * @param {number} codePoint Valid Unicode code-point
+ * @return {string} A formatted Unicode code-point string
+ * of the format U+XXXX, U+XXXXX, or U+XXXXXX
+ */
+function formatCodePoint(codePoint) {
+ codePoint = codePoint || 0; // NaN --> 0
+ var formatted = '';
+ if (codePoint <= 0xFFFF) {
+ formatted = zeroPaddedHex(codePoint, 4);
+ } else {
+ formatted = codePoint.toString(16).toUpperCase();
+ }
+ return 'U+' + formatted;
+}
+
+/**
+ * Get a list of formatted (string) Unicode code-points from a String
+ *
+ * @param {string} str Valid Unicode string
+ * @return {array} A list of formatted code-point strings
+ */
+function getCodePointsFormatted(str) {
+ var codePoints = UnicodeUtils.getCodePoints(str);
+ return codePoints.map(formatCodePoint);
+}
+
+var specialEscape = {
+ 0x07: '\\a',
+ 0x08: '\\b',
+ 0x0C: '\\f',
+ 0x0A: '\\n',
+ 0x0D: '\\r',
+ 0x09: '\\t',
+ 0x0B: '\\v',
+ 0x22: '\\"',
+ 0x5c: '\\\\'
+};
+
+/**
+ * Returns a double-quoted PHP string with all non-printable and
+ * non-US-ASCII sequences escaped.
+ *
+ * @param {string} str Valid Unicode string
+ * @return {string} Double-quoted string with Unicode sequences escaped
+ */
+function phpEscape(s) {
+ var result = '"';
+ var _iteratorNormalCompletion = true;
+ var _didIteratorError = false;
+ var _iteratorError = undefined;
+
+ try {
+ for (var _iterator = UnicodeUtils.getCodePoints(s)[Symbol.iterator](), _step; !(_iteratorNormalCompletion = (_step = _iterator.next()).done); _iteratorNormalCompletion = true) {
+ var cp = _step.value;
+
+ var special = specialEscape[cp];
+ if (special !== undefined) {
+ result += special;
+ } else if (cp >= 0x20 && cp <= 0x7e) {
+ result += String.fromCodePoint(cp);
+ } else if (cp <= 0xFFFF) {
+ result += '\\u{' + zeroPaddedHex(cp, 4) + '}';
+ } else {
+ result += '\\u{' + zeroPaddedHex(cp, 6) + '}';
+ }
+ }
+ } catch (err) {
+ _didIteratorError = true;
+ _iteratorError = err;
+ } finally {
+ try {
+ if (!_iteratorNormalCompletion && _iterator['return']) {
+ _iterator['return']();
+ }
+ } finally {
+ if (_didIteratorError) {
+ throw _iteratorError;
+ }
+ }
+ }
+
+ result += '"';
+ return result;
+}
+
+/**
+ * Returns a double-quoted Java or JavaScript string with all
+ * non-printable and non-US-ASCII sequences escaped.
+ *
+ * @param {string} str Valid Unicode string
+ * @return {string} Double-quoted string with Unicode sequences escaped
+ */
+function jsEscape(s) {
+ var result = '"';
+ for (var i = 0; i < s.length; i++) {
+ var cp = s.charCodeAt(i);
+ var special = specialEscape[cp];
+ if (special !== undefined) {
+ result += special;
+ } else if (cp >= 0x20 && cp <= 0x7e) {
+ result += String.fromCodePoint(cp);
+ } else {
+ result += '\\u' + zeroPaddedHex(cp, 4);
+ }
+ }
+ result += '"';
+ return result;
+}
+
+function c11Escape(s) {
+ var result = '';
+ var _iteratorNormalCompletion2 = true;
+ var _didIteratorError2 = false;
+ var _iteratorError2 = undefined;
+
+ try {
+ for (var _iterator2 = UnicodeUtils.getCodePoints(s)[Symbol.iterator](), _step2; !(_iteratorNormalCompletion2 = (_step2 = _iterator2.next()).done); _iteratorNormalCompletion2 = true) {
+ var cp = _step2.value;
+
+ var special = specialEscape[cp];
+ if (special !== undefined) {
+ result += special;
+ } else if (cp >= 0x20 && cp <= 0x7e) {
+ result += String.fromCodePoint(cp);
+ } else if (cp <= 0xFFFF) {
+ result += '\\u' + zeroPaddedHex(cp, 4);
+ } else {
+ result += '\\U' + zeroPaddedHex(cp, 8);
+ }
+ }
+ } catch (err) {
+ _didIteratorError2 = true;
+ _iteratorError2 = err;
+ } finally {
+ try {
+ if (!_iteratorNormalCompletion2 && _iterator2['return']) {
+ _iterator2['return']();
+ }
+ } finally {
+ if (_didIteratorError2) {
+ throw _iteratorError2;
+ }
+ }
+ }
+
+ return result;
+}
+
+/**
+ * Returns a double-quoted C string with all non-printable and
+ * non-US-ASCII sequences escaped.
+ *
+ * @param {string} str Valid Unicode string
+ * @return {string} Double-quoted string with Unicode sequences escaped
+ */
+function cEscape(s) {
+ return 'u8"' + c11Escape(s) + '"';
+}
+
+/**
+ * Returns a double-quoted Objective-C string with all non-printable
+ * and non-US-ASCII sequences escaped.
+ *
+ * @param {string} str Valid Unicode string
+ * @return {string} Double-quoted string with Unicode sequences escaped
+ */
+function objcEscape(s) {
+ return '@"' + c11Escape(s) + '"';
+}
+
+/**
+ * Returns a double-quoted Python string with all non-printable
+ * and non-US-ASCII sequences escaped.
+ *
+ * @param {string} str Valid Unicode string
+ * @return {string} Double-quoted string with Unicode sequences escaped
+ */
+function pyEscape(s) {
+ return 'u"' + c11Escape(s) + '"';
+}
+
+var UnicodeUtilsExtra = {
+ formatCodePoint: formatCodePoint,
+ getCodePointsFormatted: getCodePointsFormatted,
+ zeroPaddedHex: zeroPaddedHex,
+ phpEscape: phpEscape,
+ jsEscape: jsEscape,
+ cEscape: cEscape,
+ objcEscape: objcEscape,
+ pyEscape: pyEscape
+};
+
+module.exports = UnicodeUtilsExtra;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeUtilsExtra.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeUtilsExtra.js.flow
new file mode 100644
index 00000000..dc3ecb70
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UnicodeUtilsExtra.js.flow
@@ -0,0 +1,184 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule UnicodeUtilsExtra
+ * @typechecks
+ */
+
+/**
+ * Unicode-enabled extra utility functions not always needed.
+ */
+
+'use strict';
+
+const UnicodeUtils = require('./UnicodeUtils');
+
+/**
+ * @param {number} codePoint Valid Unicode code-point
+ * @param {number} len Zero-padded minimum width of result
+ * @return {string} A zero-padded hexadecimal string (00XXXX)
+ */
+function zeroPaddedHex(codePoint, len) {
+ let codePointHex = codePoint.toString(16).toUpperCase();
+ let numZeros = Math.max(0, len - codePointHex.length);
+ var result = '';
+ for (var i = 0; i < numZeros; i++) {
+ result += '0';
+ }
+ result += codePointHex;
+ return result;
+}
+
+/**
+ * @param {number} codePoint Valid Unicode code-point
+ * @return {string} A formatted Unicode code-point string
+ * of the format U+XXXX, U+XXXXX, or U+XXXXXX
+ */
+function formatCodePoint(codePoint) {
+ codePoint = codePoint || 0; // NaN --> 0
+ var formatted = '';
+ if (codePoint <= 0xFFFF) {
+ formatted = zeroPaddedHex(codePoint, 4);
+ } else {
+ formatted = codePoint.toString(16).toUpperCase();
+ }
+ return 'U+' + formatted;
+}
+
+/**
+ * Get a list of formatted (string) Unicode code-points from a String
+ *
+ * @param {string} str Valid Unicode string
+ * @return {array} A list of formatted code-point strings
+ */
+function getCodePointsFormatted(str) {
+ const codePoints = UnicodeUtils.getCodePoints(str);
+ return codePoints.map(formatCodePoint);
+}
+
+const specialEscape = {
+ 0x07: '\\a',
+ 0x08: '\\b',
+ 0x0C: '\\f',
+ 0x0A: '\\n',
+ 0x0D: '\\r',
+ 0x09: '\\t',
+ 0x0B: '\\v',
+ 0x22: '\\"',
+ 0x5c: '\\\\'
+};
+
+/**
+ * Returns a double-quoted PHP string with all non-printable and
+ * non-US-ASCII sequences escaped.
+ *
+ * @param {string} str Valid Unicode string
+ * @return {string} Double-quoted string with Unicode sequences escaped
+ */
+function phpEscape(s) {
+ var result = '"';
+ for (let cp of UnicodeUtils.getCodePoints(s)) {
+ let special = specialEscape[cp];
+ if (special !== undefined) {
+ result += special;
+ } else if (cp >= 0x20 && cp <= 0x7e) {
+ result += String.fromCodePoint(cp);
+ } else if (cp <= 0xFFFF) {
+ result += '\\u{' + zeroPaddedHex(cp, 4) + '}';
+ } else {
+ result += '\\u{' + zeroPaddedHex(cp, 6) + '}';
+ }
+ }
+ result += '"';
+ return result;
+}
+
+/**
+ * Returns a double-quoted Java or JavaScript string with all
+ * non-printable and non-US-ASCII sequences escaped.
+ *
+ * @param {string} str Valid Unicode string
+ * @return {string} Double-quoted string with Unicode sequences escaped
+ */
+function jsEscape(s) {
+ var result = '"';
+ for (var i = 0; i < s.length; i++) {
+ let cp = s.charCodeAt(i);
+ let special = specialEscape[cp];
+ if (special !== undefined) {
+ result += special;
+ } else if (cp >= 0x20 && cp <= 0x7e) {
+ result += String.fromCodePoint(cp);
+ } else {
+ result += '\\u' + zeroPaddedHex(cp, 4);
+ }
+ }
+ result += '"';
+ return result;
+}
+
+function c11Escape(s) {
+ var result = '';
+ for (let cp of UnicodeUtils.getCodePoints(s)) {
+ let special = specialEscape[cp];
+ if (special !== undefined) {
+ result += special;
+ } else if (cp >= 0x20 && cp <= 0x7e) {
+ result += String.fromCodePoint(cp);
+ } else if (cp <= 0xFFFF) {
+ result += '\\u' + zeroPaddedHex(cp, 4);
+ } else {
+ result += '\\U' + zeroPaddedHex(cp, 8);
+ }
+ }
+ return result;
+}
+
+/**
+ * Returns a double-quoted C string with all non-printable and
+ * non-US-ASCII sequences escaped.
+ *
+ * @param {string} str Valid Unicode string
+ * @return {string} Double-quoted string with Unicode sequences escaped
+ */
+function cEscape(s) {
+ return 'u8"' + c11Escape(s) + '"';
+}
+
+/**
+ * Returns a double-quoted Objective-C string with all non-printable
+ * and non-US-ASCII sequences escaped.
+ *
+ * @param {string} str Valid Unicode string
+ * @return {string} Double-quoted string with Unicode sequences escaped
+ */
+function objcEscape(s) {
+ return '@"' + c11Escape(s) + '"';
+}
+
+/**
+ * Returns a double-quoted Python string with all non-printable
+ * and non-US-ASCII sequences escaped.
+ *
+ * @param {string} str Valid Unicode string
+ * @return {string} Double-quoted string with Unicode sequences escaped
+ */
+function pyEscape(s) {
+ return 'u"' + c11Escape(s) + '"';
+}
+
+const UnicodeUtilsExtra = {
+ formatCodePoint: formatCodePoint,
+ getCodePointsFormatted: getCodePointsFormatted,
+ zeroPaddedHex: zeroPaddedHex,
+ phpEscape: phpEscape,
+ jsEscape: jsEscape,
+ cEscape: cEscape,
+ objcEscape: objcEscape,
+ pyEscape: pyEscape
+};
+
+module.exports = UnicodeUtilsExtra;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UserAgent.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UserAgent.js
new file mode 100644
index 00000000..24030781
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UserAgent.js
@@ -0,0 +1,239 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ */
+
+'use strict';
+
+var UserAgentData = require('./UserAgentData');
+var VersionRange = require('./VersionRange');
+
+var mapObject = require('./mapObject');
+var memoizeStringOnly = require('./memoizeStringOnly');
+
+/**
+ * Checks to see whether `name` and `version` satisfy `query`.
+ *
+ * @param {string} name Name of the browser, device, engine or platform
+ * @param {?string} version Version of the browser, engine or platform
+ * @param {string} query Query of form "Name [range expression]"
+ * @param {?function} normalizer Optional pre-processor for range expression
+ * @return {boolean}
+ */
+function compare(name, version, query, normalizer) {
+ // check for exact match with no version
+ if (name === query) {
+ return true;
+ }
+
+ // check for non-matching names
+ if (!query.startsWith(name)) {
+ return false;
+ }
+
+ // full comparison with version
+ var range = query.slice(name.length);
+ if (version) {
+ range = normalizer ? normalizer(range) : range;
+ return VersionRange.contains(range, version);
+ }
+
+ return false;
+}
+
+/**
+ * Normalizes `version` by stripping any "NT" prefix, but only on the Windows
+ * platform.
+ *
+ * Mimics the stripping performed by the `UserAgentWindowsPlatform` PHP class.
+ *
+ * @param {string} version
+ * @return {string}
+ */
+function normalizePlatformVersion(version) {
+ if (UserAgentData.platformName === 'Windows') {
+ return version.replace(/^\s*NT/, '');
+ }
+
+ return version;
+}
+
+/**
+ * Provides client-side access to the authoritative PHP-generated User Agent
+ * information supplied by the server.
+ */
+var UserAgent = {
+ /**
+ * Check if the User Agent browser matches `query`.
+ *
+ * `query` should be a string like "Chrome" or "Chrome > 33".
+ *
+ * Valid browser names include:
+ *
+ * - ACCESS NetFront
+ * - AOL
+ * - Amazon Silk
+ * - Android
+ * - BlackBerry
+ * - BlackBerry PlayBook
+ * - Chrome
+ * - Chrome for iOS
+ * - Chrome frame
+ * - Facebook PHP SDK
+ * - Facebook for iOS
+ * - Firefox
+ * - IE
+ * - IE Mobile
+ * - Mobile Safari
+ * - Motorola Internet Browser
+ * - Nokia
+ * - Openwave Mobile Browser
+ * - Opera
+ * - Opera Mini
+ * - Opera Mobile
+ * - Safari
+ * - UIWebView
+ * - Unknown
+ * - webOS
+ * - etc...
+ *
+ * An authoritative list can be found in the PHP `BrowserDetector` class and
+ * related classes in the same file (see calls to `new UserAgentBrowser` here:
+ * https://fburl.com/50728104).
+ *
+ * @note Function results are memoized
+ *
+ * @param {string} query Query of the form "Name [range expression]"
+ * @return {boolean}
+ */
+ isBrowser: function isBrowser(query) {
+ return compare(UserAgentData.browserName, UserAgentData.browserFullVersion, query);
+ },
+
+
+ /**
+ * Check if the User Agent browser uses a 32 or 64 bit architecture.
+ *
+ * @note Function results are memoized
+ *
+ * @param {string} query Query of the form "32" or "64".
+ * @return {boolean}
+ */
+ isBrowserArchitecture: function isBrowserArchitecture(query) {
+ return compare(UserAgentData.browserArchitecture, null, query);
+ },
+
+
+ /**
+ * Check if the User Agent device matches `query`.
+ *
+ * `query` should be a string like "iPhone" or "iPad".
+ *
+ * Valid device names include:
+ *
+ * - Kindle
+ * - Kindle Fire
+ * - Unknown
+ * - iPad
+ * - iPhone
+ * - iPod
+ * - etc...
+ *
+ * An authoritative list can be found in the PHP `DeviceDetector` class and
+ * related classes in the same file (see calls to `new UserAgentDevice` here:
+ * https://fburl.com/50728332).
+ *
+ * @note Function results are memoized
+ *
+ * @param {string} query Query of the form "Name"
+ * @return {boolean}
+ */
+ isDevice: function isDevice(query) {
+ return compare(UserAgentData.deviceName, null, query);
+ },
+
+
+ /**
+ * Check if the User Agent rendering engine matches `query`.
+ *
+ * `query` should be a string like "WebKit" or "WebKit >= 537".
+ *
+ * Valid engine names include:
+ *
+ * - Gecko
+ * - Presto
+ * - Trident
+ * - WebKit
+ * - etc...
+ *
+ * An authoritative list can be found in the PHP `RenderingEngineDetector`
+ * class related classes in the same file (see calls to `new
+ * UserAgentRenderingEngine` here: https://fburl.com/50728617).
+ *
+ * @note Function results are memoized
+ *
+ * @param {string} query Query of the form "Name [range expression]"
+ * @return {boolean}
+ */
+ isEngine: function isEngine(query) {
+ return compare(UserAgentData.engineName, UserAgentData.engineVersion, query);
+ },
+
+
+ /**
+ * Check if the User Agent platform matches `query`.
+ *
+ * `query` should be a string like "Windows" or "iOS 5 - 6".
+ *
+ * Valid platform names include:
+ *
+ * - Android
+ * - BlackBerry OS
+ * - Java ME
+ * - Linux
+ * - Mac OS X
+ * - Mac OS X Calendar
+ * - Mac OS X Internet Account
+ * - Symbian
+ * - SymbianOS
+ * - Windows
+ * - Windows Mobile
+ * - Windows Phone
+ * - iOS
+ * - iOS Facebook Integration Account
+ * - iOS Facebook Social Sharing UI
+ * - webOS
+ * - Chrome OS
+ * - etc...
+ *
+ * An authoritative list can be found in the PHP `PlatformDetector` class and
+ * related classes in the same file (see calls to `new UserAgentPlatform`
+ * here: https://fburl.com/50729226).
+ *
+ * @note Function results are memoized
+ *
+ * @param {string} query Query of the form "Name [range expression]"
+ * @return {boolean}
+ */
+ isPlatform: function isPlatform(query) {
+ return compare(UserAgentData.platformName, UserAgentData.platformFullVersion, query, normalizePlatformVersion);
+ },
+
+
+ /**
+ * Check if the User Agent platform is a 32 or 64 bit architecture.
+ *
+ * @note Function results are memoized
+ *
+ * @param {string} query Query of the form "32" or "64".
+ * @return {boolean}
+ */
+ isPlatformArchitecture: function isPlatformArchitecture(query) {
+ return compare(UserAgentData.platformArchitecture, null, query);
+ }
+};
+
+module.exports = mapObject(UserAgent, memoizeStringOnly);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UserAgent.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UserAgent.js.flow
new file mode 100644
index 00000000..61ec1286
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UserAgent.js.flow
@@ -0,0 +1,236 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule UserAgent
+ */
+
+'use strict';
+
+const UserAgentData = require('./UserAgentData');
+const VersionRange = require('./VersionRange');
+
+const mapObject = require('./mapObject');
+const memoizeStringOnly = require('./memoizeStringOnly');
+
+/**
+ * Checks to see whether `name` and `version` satisfy `query`.
+ *
+ * @param {string} name Name of the browser, device, engine or platform
+ * @param {?string} version Version of the browser, engine or platform
+ * @param {string} query Query of form "Name [range expression]"
+ * @param {?function} normalizer Optional pre-processor for range expression
+ * @return {boolean}
+ */
+function compare(name, version, query, normalizer) {
+ // check for exact match with no version
+ if (name === query) {
+ return true;
+ }
+
+ // check for non-matching names
+ if (!query.startsWith(name)) {
+ return false;
+ }
+
+ // full comparison with version
+ let range = query.slice(name.length);
+ if (version) {
+ range = normalizer ? normalizer(range) : range;
+ return VersionRange.contains(range, version);
+ }
+
+ return false;
+}
+
+/**
+ * Normalizes `version` by stripping any "NT" prefix, but only on the Windows
+ * platform.
+ *
+ * Mimics the stripping performed by the `UserAgentWindowsPlatform` PHP class.
+ *
+ * @param {string} version
+ * @return {string}
+ */
+function normalizePlatformVersion(version) {
+ if (UserAgentData.platformName === 'Windows') {
+ return version.replace(/^\s*NT/, '');
+ }
+
+ return version;
+}
+
+/**
+ * Provides client-side access to the authoritative PHP-generated User Agent
+ * information supplied by the server.
+ */
+const UserAgent = {
+ /**
+ * Check if the User Agent browser matches `query`.
+ *
+ * `query` should be a string like "Chrome" or "Chrome > 33".
+ *
+ * Valid browser names include:
+ *
+ * - ACCESS NetFront
+ * - AOL
+ * - Amazon Silk
+ * - Android
+ * - BlackBerry
+ * - BlackBerry PlayBook
+ * - Chrome
+ * - Chrome for iOS
+ * - Chrome frame
+ * - Facebook PHP SDK
+ * - Facebook for iOS
+ * - Firefox
+ * - IE
+ * - IE Mobile
+ * - Mobile Safari
+ * - Motorola Internet Browser
+ * - Nokia
+ * - Openwave Mobile Browser
+ * - Opera
+ * - Opera Mini
+ * - Opera Mobile
+ * - Safari
+ * - UIWebView
+ * - Unknown
+ * - webOS
+ * - etc...
+ *
+ * An authoritative list can be found in the PHP `BrowserDetector` class and
+ * related classes in the same file (see calls to `new UserAgentBrowser` here:
+ * https://fburl.com/50728104).
+ *
+ * @note Function results are memoized
+ *
+ * @param {string} query Query of the form "Name [range expression]"
+ * @return {boolean}
+ */
+ isBrowser(query) {
+ return compare(UserAgentData.browserName, UserAgentData.browserFullVersion, query);
+ },
+
+ /**
+ * Check if the User Agent browser uses a 32 or 64 bit architecture.
+ *
+ * @note Function results are memoized
+ *
+ * @param {string} query Query of the form "32" or "64".
+ * @return {boolean}
+ */
+ isBrowserArchitecture(query) {
+ return compare(UserAgentData.browserArchitecture, null, query);
+ },
+
+ /**
+ * Check if the User Agent device matches `query`.
+ *
+ * `query` should be a string like "iPhone" or "iPad".
+ *
+ * Valid device names include:
+ *
+ * - Kindle
+ * - Kindle Fire
+ * - Unknown
+ * - iPad
+ * - iPhone
+ * - iPod
+ * - etc...
+ *
+ * An authoritative list can be found in the PHP `DeviceDetector` class and
+ * related classes in the same file (see calls to `new UserAgentDevice` here:
+ * https://fburl.com/50728332).
+ *
+ * @note Function results are memoized
+ *
+ * @param {string} query Query of the form "Name"
+ * @return {boolean}
+ */
+ isDevice(query) {
+ return compare(UserAgentData.deviceName, null, query);
+ },
+
+ /**
+ * Check if the User Agent rendering engine matches `query`.
+ *
+ * `query` should be a string like "WebKit" or "WebKit >= 537".
+ *
+ * Valid engine names include:
+ *
+ * - Gecko
+ * - Presto
+ * - Trident
+ * - WebKit
+ * - etc...
+ *
+ * An authoritative list can be found in the PHP `RenderingEngineDetector`
+ * class related classes in the same file (see calls to `new
+ * UserAgentRenderingEngine` here: https://fburl.com/50728617).
+ *
+ * @note Function results are memoized
+ *
+ * @param {string} query Query of the form "Name [range expression]"
+ * @return {boolean}
+ */
+ isEngine(query) {
+ return compare(UserAgentData.engineName, UserAgentData.engineVersion, query);
+ },
+
+ /**
+ * Check if the User Agent platform matches `query`.
+ *
+ * `query` should be a string like "Windows" or "iOS 5 - 6".
+ *
+ * Valid platform names include:
+ *
+ * - Android
+ * - BlackBerry OS
+ * - Java ME
+ * - Linux
+ * - Mac OS X
+ * - Mac OS X Calendar
+ * - Mac OS X Internet Account
+ * - Symbian
+ * - SymbianOS
+ * - Windows
+ * - Windows Mobile
+ * - Windows Phone
+ * - iOS
+ * - iOS Facebook Integration Account
+ * - iOS Facebook Social Sharing UI
+ * - webOS
+ * - Chrome OS
+ * - etc...
+ *
+ * An authoritative list can be found in the PHP `PlatformDetector` class and
+ * related classes in the same file (see calls to `new UserAgentPlatform`
+ * here: https://fburl.com/50729226).
+ *
+ * @note Function results are memoized
+ *
+ * @param {string} query Query of the form "Name [range expression]"
+ * @return {boolean}
+ */
+ isPlatform(query) {
+ return compare(UserAgentData.platformName, UserAgentData.platformFullVersion, query, normalizePlatformVersion);
+ },
+
+ /**
+ * Check if the User Agent platform is a 32 or 64 bit architecture.
+ *
+ * @note Function results are memoized
+ *
+ * @param {string} query Query of the form "32" or "64".
+ * @return {boolean}
+ */
+ isPlatformArchitecture(query) {
+ return compare(UserAgentData.platformArchitecture, null, query);
+ }
+
+};
+
+module.exports = mapObject(UserAgent, memoizeStringOnly);
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UserAgentData.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UserAgentData.js
new file mode 100644
index 00000000..928fbe3d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UserAgentData.js
@@ -0,0 +1,80 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ */
+
+/**
+ * Usage note:
+ * This module makes a best effort to export the same data we would internally.
+ * At Facebook we use a server-generated module that does the parsing and
+ * exports the data for the client to use. We can't rely on a server-side
+ * implementation in open source so instead we make use of an open source
+ * library to do the heavy lifting and then make some adjustments as necessary.
+ * It's likely there will be some differences. Some we can smooth over.
+ * Others are going to be harder.
+ */
+
+'use strict';
+
+var UAParser = require('ua-parser-js');
+
+var UNKNOWN = 'Unknown';
+
+var PLATFORM_MAP = {
+ 'Mac OS': 'Mac OS X'
+};
+
+/**
+ * Convert from UAParser platform name to what we expect.
+ */
+function convertPlatformName(name) {
+ return PLATFORM_MAP[name] || name;
+}
+
+/**
+ * Get the version number in parts. This is very naive. We actually get major
+ * version as a part of UAParser already, which is generally good enough, but
+ * let's get the minor just in case.
+ */
+function getBrowserVersion(version) {
+ if (!version) {
+ return {
+ major: '',
+ minor: ''
+ };
+ }
+ var parts = version.split('.');
+ return {
+ major: parts[0],
+ minor: parts[1]
+ };
+}
+
+/**
+ * Get the UA data fom UAParser and then convert it to the format we're
+ * expecting for our APIS.
+ */
+var parser = new UAParser();
+var results = parser.getResult();
+
+// Do some conversion first.
+var browserVersionData = getBrowserVersion(results.browser.version);
+var uaData = {
+ browserArchitecture: results.cpu.architecture || UNKNOWN,
+ browserFullVersion: results.browser.version || UNKNOWN,
+ browserMinorVersion: browserVersionData.minor || UNKNOWN,
+ browserName: results.browser.name || UNKNOWN,
+ browserVersion: results.browser.major || UNKNOWN,
+ deviceName: results.device.model || UNKNOWN,
+ engineName: results.engine.name || UNKNOWN,
+ engineVersion: results.engine.version || UNKNOWN,
+ platformArchitecture: results.cpu.architecture || UNKNOWN,
+ platformName: convertPlatformName(results.os.name) || UNKNOWN,
+ platformVersion: results.os.version || UNKNOWN,
+ platformFullVersion: results.os.version || UNKNOWN
+};
+
+module.exports = uaData;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UserAgentData.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UserAgentData.js.flow
new file mode 100644
index 00000000..bc2e027c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/UserAgentData.js.flow
@@ -0,0 +1,81 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule UserAgentData
+ */
+
+/**
+ * Usage note:
+ * This module makes a best effort to export the same data we would internally.
+ * At Facebook we use a server-generated module that does the parsing and
+ * exports the data for the client to use. We can't rely on a server-side
+ * implementation in open source so instead we make use of an open source
+ * library to do the heavy lifting and then make some adjustments as necessary.
+ * It's likely there will be some differences. Some we can smooth over.
+ * Others are going to be harder.
+ */
+
+'use strict';
+
+var UAParser = require('ua-parser-js');
+
+var UNKNOWN = 'Unknown';
+
+var PLATFORM_MAP = {
+ 'Mac OS': 'Mac OS X'
+};
+
+/**
+ * Convert from UAParser platform name to what we expect.
+ */
+function convertPlatformName(name) {
+ return PLATFORM_MAP[name] || name;
+}
+
+/**
+ * Get the version number in parts. This is very naive. We actually get major
+ * version as a part of UAParser already, which is generally good enough, but
+ * let's get the minor just in case.
+ */
+function getBrowserVersion(version) {
+ if (!version) {
+ return {
+ major: '',
+ minor: ''
+ };
+ }
+ var parts = version.split('.');
+ return {
+ major: parts[0],
+ minor: parts[1]
+ };
+}
+
+/**
+ * Get the UA data fom UAParser and then convert it to the format we're
+ * expecting for our APIS.
+ */
+var parser = new UAParser();
+var results = parser.getResult();
+
+// Do some conversion first.
+var browserVersionData = getBrowserVersion(results.browser.version);
+var uaData = {
+ browserArchitecture: results.cpu.architecture || UNKNOWN,
+ browserFullVersion: results.browser.version || UNKNOWN,
+ browserMinorVersion: browserVersionData.minor || UNKNOWN,
+ browserName: results.browser.name || UNKNOWN,
+ browserVersion: results.browser.major || UNKNOWN,
+ deviceName: results.device.model || UNKNOWN,
+ engineName: results.engine.name || UNKNOWN,
+ engineVersion: results.engine.version || UNKNOWN,
+ platformArchitecture: results.cpu.architecture || UNKNOWN,
+ platformName: convertPlatformName(results.os.name) || UNKNOWN,
+ platformVersion: results.os.version || UNKNOWN,
+ platformFullVersion: results.os.version || UNKNOWN
+};
+
+module.exports = uaData;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/VersionRange.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/VersionRange.js
new file mode 100644
index 00000000..93e3e53d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/VersionRange.js
@@ -0,0 +1,380 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ */
+
+'use strict';
+
+var invariant = require('./invariant');
+
+var componentRegex = /\./;
+var orRegex = /\|\|/;
+var rangeRegex = /\s+\-\s+/;
+var modifierRegex = /^(<=|<|=|>=|~>|~|>|)?\s*(.+)/;
+var numericRegex = /^(\d*)(.*)/;
+
+/**
+ * Splits input `range` on "||" and returns true if any subrange matches
+ * `version`.
+ *
+ * @param {string} range
+ * @param {string} version
+ * @returns {boolean}
+ */
+function checkOrExpression(range, version) {
+ var expressions = range.split(orRegex);
+
+ if (expressions.length > 1) {
+ return expressions.some(function (range) {
+ return VersionRange.contains(range, version);
+ });
+ } else {
+ range = expressions[0].trim();
+ return checkRangeExpression(range, version);
+ }
+}
+
+/**
+ * Splits input `range` on " - " (the surrounding whitespace is required) and
+ * returns true if version falls between the two operands.
+ *
+ * @param {string} range
+ * @param {string} version
+ * @returns {boolean}
+ */
+function checkRangeExpression(range, version) {
+ var expressions = range.split(rangeRegex);
+
+ !(expressions.length > 0 && expressions.length <= 2) ? process.env.NODE_ENV !== 'production' ? invariant(false, 'the "-" operator expects exactly 2 operands') : invariant(false) : void 0;
+
+ if (expressions.length === 1) {
+ return checkSimpleExpression(expressions[0], version);
+ } else {
+ var startVersion = expressions[0],
+ endVersion = expressions[1];
+
+ !(isSimpleVersion(startVersion) && isSimpleVersion(endVersion)) ? process.env.NODE_ENV !== 'production' ? invariant(false, 'operands to the "-" operator must be simple (no modifiers)') : invariant(false) : void 0;
+
+ return checkSimpleExpression('>=' + startVersion, version) && checkSimpleExpression('<=' + endVersion, version);
+ }
+}
+
+/**
+ * Checks if `range` matches `version`. `range` should be a "simple" range (ie.
+ * not a compound range using the " - " or "||" operators).
+ *
+ * @param {string} range
+ * @param {string} version
+ * @returns {boolean}
+ */
+function checkSimpleExpression(range, version) {
+ range = range.trim();
+ if (range === '') {
+ return true;
+ }
+
+ var versionComponents = version.split(componentRegex);
+
+ var _getModifierAndCompon = getModifierAndComponents(range),
+ modifier = _getModifierAndCompon.modifier,
+ rangeComponents = _getModifierAndCompon.rangeComponents;
+
+ switch (modifier) {
+ case '<':
+ return checkLessThan(versionComponents, rangeComponents);
+ case '<=':
+ return checkLessThanOrEqual(versionComponents, rangeComponents);
+ case '>=':
+ return checkGreaterThanOrEqual(versionComponents, rangeComponents);
+ case '>':
+ return checkGreaterThan(versionComponents, rangeComponents);
+ case '~':
+ case '~>':
+ return checkApproximateVersion(versionComponents, rangeComponents);
+ default:
+ return checkEqual(versionComponents, rangeComponents);
+ }
+}
+
+/**
+ * Checks whether `a` is less than `b`.
+ *
+ * @param {array} a
+ * @param {array} b
+ * @returns {boolean}
+ */
+function checkLessThan(a, b) {
+ return compareComponents(a, b) === -1;
+}
+
+/**
+ * Checks whether `a` is less than or equal to `b`.
+ *
+ * @param {array} a
+ * @param {array} b
+ * @returns {boolean}
+ */
+function checkLessThanOrEqual(a, b) {
+ var result = compareComponents(a, b);
+ return result === -1 || result === 0;
+}
+
+/**
+ * Checks whether `a` is equal to `b`.
+ *
+ * @param {array} a
+ * @param {array} b
+ * @returns {boolean}
+ */
+function checkEqual(a, b) {
+ return compareComponents(a, b) === 0;
+}
+
+/**
+ * Checks whether `a` is greater than or equal to `b`.
+ *
+ * @param {array} a
+ * @param {array} b
+ * @returns {boolean}
+ */
+function checkGreaterThanOrEqual(a, b) {
+ var result = compareComponents(a, b);
+ return result === 1 || result === 0;
+}
+
+/**
+ * Checks whether `a` is greater than `b`.
+ *
+ * @param {array} a
+ * @param {array} b
+ * @returns {boolean}
+ */
+function checkGreaterThan(a, b) {
+ return compareComponents(a, b) === 1;
+}
+
+/**
+ * Checks whether `a` is "reasonably close" to `b` (as described in
+ * https://www.npmjs.org/doc/misc/semver.html). For example, if `b` is "1.3.1"
+ * then "reasonably close" is defined as ">= 1.3.1 and < 1.4".
+ *
+ * @param {array} a
+ * @param {array} b
+ * @returns {boolean}
+ */
+function checkApproximateVersion(a, b) {
+ var lowerBound = b.slice();
+ var upperBound = b.slice();
+
+ if (upperBound.length > 1) {
+ upperBound.pop();
+ }
+ var lastIndex = upperBound.length - 1;
+ var numeric = parseInt(upperBound[lastIndex], 10);
+ if (isNumber(numeric)) {
+ upperBound[lastIndex] = numeric + 1 + '';
+ }
+
+ return checkGreaterThanOrEqual(a, lowerBound) && checkLessThan(a, upperBound);
+}
+
+/**
+ * Extracts the optional modifier (<, <=, =, >=, >, ~, ~>) and version
+ * components from `range`.
+ *
+ * For example, given `range` ">= 1.2.3" returns an object with a `modifier` of
+ * `">="` and `components` of `[1, 2, 3]`.
+ *
+ * @param {string} range
+ * @returns {object}
+ */
+function getModifierAndComponents(range) {
+ var rangeComponents = range.split(componentRegex);
+ var matches = rangeComponents[0].match(modifierRegex);
+ !matches ? process.env.NODE_ENV !== 'production' ? invariant(false, 'expected regex to match but it did not') : invariant(false) : void 0;
+
+ return {
+ modifier: matches[1],
+ rangeComponents: [matches[2]].concat(rangeComponents.slice(1))
+ };
+}
+
+/**
+ * Determines if `number` is a number.
+ *
+ * @param {mixed} number
+ * @returns {boolean}
+ */
+function isNumber(number) {
+ return !isNaN(number) && isFinite(number);
+}
+
+/**
+ * Tests whether `range` is a "simple" version number without any modifiers
+ * (">", "~" etc).
+ *
+ * @param {string} range
+ * @returns {boolean}
+ */
+function isSimpleVersion(range) {
+ return !getModifierAndComponents(range).modifier;
+}
+
+/**
+ * Zero-pads array `array` until it is at least `length` long.
+ *
+ * @param {array} array
+ * @param {number} length
+ */
+function zeroPad(array, length) {
+ for (var i = array.length; i < length; i++) {
+ array[i] = '0';
+ }
+}
+
+/**
+ * Normalizes `a` and `b` in preparation for comparison by doing the following:
+ *
+ * - zero-pads `a` and `b`
+ * - marks any "x", "X" or "*" component in `b` as equivalent by zero-ing it out
+ * in both `a` and `b`
+ * - marks any final "*" component in `b` as a greedy wildcard by zero-ing it
+ * and all of its successors in `a`
+ *
+ * @param {array} a
+ * @param {array} b
+ * @returns {array>}
+ */
+function normalizeVersions(a, b) {
+ a = a.slice();
+ b = b.slice();
+
+ zeroPad(a, b.length);
+
+ // mark "x" and "*" components as equal
+ for (var i = 0; i < b.length; i++) {
+ var matches = b[i].match(/^[x*]$/i);
+ if (matches) {
+ b[i] = a[i] = '0';
+
+ // final "*" greedily zeros all remaining components
+ if (matches[0] === '*' && i === b.length - 1) {
+ for (var j = i; j < a.length; j++) {
+ a[j] = '0';
+ }
+ }
+ }
+ }
+
+ zeroPad(b, a.length);
+
+ return [a, b];
+}
+
+/**
+ * Returns the numerical -- not the lexicographical -- ordering of `a` and `b`.
+ *
+ * For example, `10-alpha` is greater than `2-beta`.
+ *
+ * @param {string} a
+ * @param {string} b
+ * @returns {number} -1, 0 or 1 to indicate whether `a` is less than, equal to,
+ * or greater than `b`, respectively
+ */
+function compareNumeric(a, b) {
+ var aPrefix = a.match(numericRegex)[1];
+ var bPrefix = b.match(numericRegex)[1];
+ var aNumeric = parseInt(aPrefix, 10);
+ var bNumeric = parseInt(bPrefix, 10);
+
+ if (isNumber(aNumeric) && isNumber(bNumeric) && aNumeric !== bNumeric) {
+ return compare(aNumeric, bNumeric);
+ } else {
+ return compare(a, b);
+ }
+}
+
+/**
+ * Returns the ordering of `a` and `b`.
+ *
+ * @param {string|number} a
+ * @param {string|number} b
+ * @returns {number} -1, 0 or 1 to indicate whether `a` is less than, equal to,
+ * or greater than `b`, respectively
+ */
+function compare(a, b) {
+ !(typeof a === typeof b) ? process.env.NODE_ENV !== 'production' ? invariant(false, '"a" and "b" must be of the same type') : invariant(false) : void 0;
+
+ if (a > b) {
+ return 1;
+ } else if (a < b) {
+ return -1;
+ } else {
+ return 0;
+ }
+}
+
+/**
+ * Compares arrays of version components.
+ *
+ * @param {array} a
+ * @param {array} b
+ * @returns {number} -1, 0 or 1 to indicate whether `a` is less than, equal to,
+ * or greater than `b`, respectively
+ */
+function compareComponents(a, b) {
+ var _normalizeVersions = normalizeVersions(a, b),
+ aNormalized = _normalizeVersions[0],
+ bNormalized = _normalizeVersions[1];
+
+ for (var i = 0; i < bNormalized.length; i++) {
+ var result = compareNumeric(aNormalized[i], bNormalized[i]);
+ if (result) {
+ return result;
+ }
+ }
+
+ return 0;
+}
+
+var VersionRange = {
+ /**
+ * Checks whether `version` satisfies the `range` specification.
+ *
+ * We support a subset of the expressions defined in
+ * https://www.npmjs.org/doc/misc/semver.html:
+ *
+ * version Must match version exactly
+ * =version Same as just version
+ * >version Must be greater than version
+ * >=version Must be greater than or equal to version
+ * = 1.2.3 and < 1.3"
+ * ~>version Equivalent to ~version
+ * 1.2.x Must match "1.2.x", where "x" is a wildcard that matches
+ * anything
+ * 1.2.* Similar to "1.2.x", but "*" in the trailing position is a
+ * "greedy" wildcard, so will match any number of additional
+ * components:
+ * "1.2.*" will match "1.2.1", "1.2.1.1", "1.2.1.1.1" etc
+ * * Any version
+ * "" (Empty string) Same as *
+ * v1 - v2 Equivalent to ">= v1 and <= v2"
+ * r1 || r2 Passes if either r1 or r2 are satisfied
+ *
+ * @param {string} range
+ * @param {string} version
+ * @returns {boolean}
+ */
+ contains: function contains(range, version) {
+ return checkOrExpression(range.trim(), version.trim());
+ }
+};
+
+module.exports = VersionRange;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/VersionRange.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/VersionRange.js.flow
new file mode 100644
index 00000000..ad64790f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/VersionRange.js.flow
@@ -0,0 +1,371 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule VersionRange
+ */
+
+'use strict';
+
+const invariant = require('./invariant');
+
+const componentRegex = /\./;
+const orRegex = /\|\|/;
+const rangeRegex = /\s+\-\s+/;
+const modifierRegex = /^(<=|<|=|>=|~>|~|>|)?\s*(.+)/;
+const numericRegex = /^(\d*)(.*)/;
+
+/**
+ * Splits input `range` on "||" and returns true if any subrange matches
+ * `version`.
+ *
+ * @param {string} range
+ * @param {string} version
+ * @returns {boolean}
+ */
+function checkOrExpression(range, version) {
+ const expressions = range.split(orRegex);
+
+ if (expressions.length > 1) {
+ return expressions.some(range => VersionRange.contains(range, version));
+ } else {
+ range = expressions[0].trim();
+ return checkRangeExpression(range, version);
+ }
+}
+
+/**
+ * Splits input `range` on " - " (the surrounding whitespace is required) and
+ * returns true if version falls between the two operands.
+ *
+ * @param {string} range
+ * @param {string} version
+ * @returns {boolean}
+ */
+function checkRangeExpression(range, version) {
+ const expressions = range.split(rangeRegex);
+
+ invariant(expressions.length > 0 && expressions.length <= 2, 'the "-" operator expects exactly 2 operands');
+
+ if (expressions.length === 1) {
+ return checkSimpleExpression(expressions[0], version);
+ } else {
+ const [startVersion, endVersion] = expressions;
+ invariant(isSimpleVersion(startVersion) && isSimpleVersion(endVersion), 'operands to the "-" operator must be simple (no modifiers)');
+
+ return checkSimpleExpression('>=' + startVersion, version) && checkSimpleExpression('<=' + endVersion, version);
+ }
+}
+
+/**
+ * Checks if `range` matches `version`. `range` should be a "simple" range (ie.
+ * not a compound range using the " - " or "||" operators).
+ *
+ * @param {string} range
+ * @param {string} version
+ * @returns {boolean}
+ */
+function checkSimpleExpression(range, version) {
+ range = range.trim();
+ if (range === '') {
+ return true;
+ }
+
+ const versionComponents = version.split(componentRegex);
+ const { modifier, rangeComponents } = getModifierAndComponents(range);
+ switch (modifier) {
+ case '<':
+ return checkLessThan(versionComponents, rangeComponents);
+ case '<=':
+ return checkLessThanOrEqual(versionComponents, rangeComponents);
+ case '>=':
+ return checkGreaterThanOrEqual(versionComponents, rangeComponents);
+ case '>':
+ return checkGreaterThan(versionComponents, rangeComponents);
+ case '~':
+ case '~>':
+ return checkApproximateVersion(versionComponents, rangeComponents);
+ default:
+ return checkEqual(versionComponents, rangeComponents);
+ }
+}
+
+/**
+ * Checks whether `a` is less than `b`.
+ *
+ * @param {array} a
+ * @param {array} b
+ * @returns {boolean}
+ */
+function checkLessThan(a, b) {
+ return compareComponents(a, b) === -1;
+}
+
+/**
+ * Checks whether `a` is less than or equal to `b`.
+ *
+ * @param {array} a
+ * @param {array} b
+ * @returns {boolean}
+ */
+function checkLessThanOrEqual(a, b) {
+ const result = compareComponents(a, b);
+ return result === -1 || result === 0;
+}
+
+/**
+ * Checks whether `a` is equal to `b`.
+ *
+ * @param {array} a
+ * @param {array} b
+ * @returns {boolean}
+ */
+function checkEqual(a, b) {
+ return compareComponents(a, b) === 0;
+}
+
+/**
+ * Checks whether `a` is greater than or equal to `b`.
+ *
+ * @param {array} a
+ * @param {array} b
+ * @returns {boolean}
+ */
+function checkGreaterThanOrEqual(a, b) {
+ const result = compareComponents(a, b);
+ return result === 1 || result === 0;
+}
+
+/**
+ * Checks whether `a` is greater than `b`.
+ *
+ * @param {array} a
+ * @param {array} b
+ * @returns {boolean}
+ */
+function checkGreaterThan(a, b) {
+ return compareComponents(a, b) === 1;
+}
+
+/**
+ * Checks whether `a` is "reasonably close" to `b` (as described in
+ * https://www.npmjs.org/doc/misc/semver.html). For example, if `b` is "1.3.1"
+ * then "reasonably close" is defined as ">= 1.3.1 and < 1.4".
+ *
+ * @param {array} a
+ * @param {array} b
+ * @returns {boolean}
+ */
+function checkApproximateVersion(a, b) {
+ const lowerBound = b.slice();
+ const upperBound = b.slice();
+
+ if (upperBound.length > 1) {
+ upperBound.pop();
+ }
+ const lastIndex = upperBound.length - 1;
+ const numeric = parseInt(upperBound[lastIndex], 10);
+ if (isNumber(numeric)) {
+ upperBound[lastIndex] = numeric + 1 + '';
+ }
+
+ return checkGreaterThanOrEqual(a, lowerBound) && checkLessThan(a, upperBound);
+}
+
+/**
+ * Extracts the optional modifier (<, <=, =, >=, >, ~, ~>) and version
+ * components from `range`.
+ *
+ * For example, given `range` ">= 1.2.3" returns an object with a `modifier` of
+ * `">="` and `components` of `[1, 2, 3]`.
+ *
+ * @param {string} range
+ * @returns {object}
+ */
+function getModifierAndComponents(range) {
+ const rangeComponents = range.split(componentRegex);
+ const matches = rangeComponents[0].match(modifierRegex);
+ invariant(matches, 'expected regex to match but it did not');
+
+ return {
+ modifier: matches[1],
+ rangeComponents: [matches[2]].concat(rangeComponents.slice(1))
+ };
+}
+
+/**
+ * Determines if `number` is a number.
+ *
+ * @param {mixed} number
+ * @returns {boolean}
+ */
+function isNumber(number) {
+ return !isNaN(number) && isFinite(number);
+}
+
+/**
+ * Tests whether `range` is a "simple" version number without any modifiers
+ * (">", "~" etc).
+ *
+ * @param {string} range
+ * @returns {boolean}
+ */
+function isSimpleVersion(range) {
+ return !getModifierAndComponents(range).modifier;
+}
+
+/**
+ * Zero-pads array `array` until it is at least `length` long.
+ *
+ * @param {array} array
+ * @param {number} length
+ */
+function zeroPad(array, length) {
+ for (let i = array.length; i < length; i++) {
+ array[i] = '0';
+ }
+}
+
+/**
+ * Normalizes `a` and `b` in preparation for comparison by doing the following:
+ *
+ * - zero-pads `a` and `b`
+ * - marks any "x", "X" or "*" component in `b` as equivalent by zero-ing it out
+ * in both `a` and `b`
+ * - marks any final "*" component in `b` as a greedy wildcard by zero-ing it
+ * and all of its successors in `a`
+ *
+ * @param {array} a
+ * @param {array} b
+ * @returns {array>}
+ */
+function normalizeVersions(a, b) {
+ a = a.slice();
+ b = b.slice();
+
+ zeroPad(a, b.length);
+
+ // mark "x" and "*" components as equal
+ for (let i = 0; i < b.length; i++) {
+ const matches = b[i].match(/^[x*]$/i);
+ if (matches) {
+ b[i] = a[i] = '0';
+
+ // final "*" greedily zeros all remaining components
+ if (matches[0] === '*' && i === b.length - 1) {
+ for (let j = i; j < a.length; j++) {
+ a[j] = '0';
+ }
+ }
+ }
+ }
+
+ zeroPad(b, a.length);
+
+ return [a, b];
+}
+
+/**
+ * Returns the numerical -- not the lexicographical -- ordering of `a` and `b`.
+ *
+ * For example, `10-alpha` is greater than `2-beta`.
+ *
+ * @param {string} a
+ * @param {string} b
+ * @returns {number} -1, 0 or 1 to indicate whether `a` is less than, equal to,
+ * or greater than `b`, respectively
+ */
+function compareNumeric(a, b) {
+ const aPrefix = a.match(numericRegex)[1];
+ const bPrefix = b.match(numericRegex)[1];
+ const aNumeric = parseInt(aPrefix, 10);
+ const bNumeric = parseInt(bPrefix, 10);
+
+ if (isNumber(aNumeric) && isNumber(bNumeric) && aNumeric !== bNumeric) {
+ return compare(aNumeric, bNumeric);
+ } else {
+ return compare(a, b);
+ }
+}
+
+/**
+ * Returns the ordering of `a` and `b`.
+ *
+ * @param {string|number} a
+ * @param {string|number} b
+ * @returns {number} -1, 0 or 1 to indicate whether `a` is less than, equal to,
+ * or greater than `b`, respectively
+ */
+function compare(a, b) {
+ invariant(typeof a === typeof b, '"a" and "b" must be of the same type');
+
+ if (a > b) {
+ return 1;
+ } else if (a < b) {
+ return -1;
+ } else {
+ return 0;
+ }
+}
+
+/**
+ * Compares arrays of version components.
+ *
+ * @param {array} a
+ * @param {array} b
+ * @returns {number} -1, 0 or 1 to indicate whether `a` is less than, equal to,
+ * or greater than `b`, respectively
+ */
+function compareComponents(a, b) {
+ const [aNormalized, bNormalized] = normalizeVersions(a, b);
+
+ for (let i = 0; i < bNormalized.length; i++) {
+ const result = compareNumeric(aNormalized[i], bNormalized[i]);
+ if (result) {
+ return result;
+ }
+ }
+
+ return 0;
+}
+
+var VersionRange = {
+ /**
+ * Checks whether `version` satisfies the `range` specification.
+ *
+ * We support a subset of the expressions defined in
+ * https://www.npmjs.org/doc/misc/semver.html:
+ *
+ * version Must match version exactly
+ * =version Same as just version
+ * >version Must be greater than version
+ * >=version Must be greater than or equal to version
+ * = 1.2.3 and < 1.3"
+ * ~>version Equivalent to ~version
+ * 1.2.x Must match "1.2.x", where "x" is a wildcard that matches
+ * anything
+ * 1.2.* Similar to "1.2.x", but "*" in the trailing position is a
+ * "greedy" wildcard, so will match any number of additional
+ * components:
+ * "1.2.*" will match "1.2.1", "1.2.1.1", "1.2.1.1.1" etc
+ * * Any version
+ * "" (Empty string) Same as *
+ * v1 - v2 Equivalent to ">= v1 and <= v2"
+ * r1 || r2 Passes if either r1 or r2 are satisfied
+ *
+ * @param {string} range
+ * @param {string} version
+ * @returns {boolean}
+ */
+ contains(range, version) {
+ return checkOrExpression(range.trim(), version.trim());
+ }
+};
+
+module.exports = VersionRange;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/__mocks__/ErrorUtils.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/__mocks__/ErrorUtils.js
new file mode 100644
index 00000000..5140ac44
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/__mocks__/ErrorUtils.js
@@ -0,0 +1,20 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ */
+
+'use strict';
+
+var ErrorUtils = jest.genMockFromModule('../ErrorUtils');
+
+ErrorUtils.applyWithGuard.mockImplementation(function (callback, context, args) {
+ return callback.apply(context, args);
+});
+
+ErrorUtils.guard.mockImplementation(function (callback) {
+ return callback;
+});
+
+module.exports = ErrorUtils;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/__mocks__/base62.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/__mocks__/base62.js
new file mode 100644
index 00000000..24cc91c7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/__mocks__/base62.js
@@ -0,0 +1,10 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ */
+
+'use strict';
+
+module.exports = require.requireActual('../base62');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/__mocks__/crc32.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/__mocks__/crc32.js
new file mode 100644
index 00000000..259e4c73
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/__mocks__/crc32.js
@@ -0,0 +1,10 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ */
+
+'use strict';
+
+module.exports = require.requireActual('../crc32');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/__mocks__/fetch.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/__mocks__/fetch.js
new file mode 100644
index 00000000..2ae49d6a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/__mocks__/fetch.js
@@ -0,0 +1,26 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @noflow
+ */
+
+'use strict';
+
+var Deferred = require.requireActual('../Deferred');
+
+function fetch(uri, options) {
+ var deferred = new Deferred();
+ fetch.mock.calls.push([uri, options]);
+ fetch.mock.deferreds.push(deferred);
+ return deferred.getPromise();
+}
+
+fetch.mock = {
+ calls: [],
+ deferreds: []
+};
+
+module.exports = fetch;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/__mocks__/fetchWithRetries.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/__mocks__/fetchWithRetries.js
new file mode 100644
index 00000000..f87746b2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/__mocks__/fetchWithRetries.js
@@ -0,0 +1,31 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @noflow
+ */
+
+'use strict';
+
+var Deferred = require.requireActual('../Deferred');
+
+function fetchWithRetries() {
+ var deferred = new Deferred();
+
+ for (var _len = arguments.length, args = Array(_len), _key = 0; _key < _len; _key++) {
+ args[_key] = arguments[_key];
+ }
+
+ fetchWithRetries.mock.calls.push(args);
+ fetchWithRetries.mock.deferreds.push(deferred);
+ return deferred.getPromise();
+}
+
+fetchWithRetries.mock = {
+ calls: [],
+ deferreds: []
+};
+
+module.exports = fetchWithRetries;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/__mocks__/nullthrows.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/__mocks__/nullthrows.js
new file mode 100644
index 00000000..43422839
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/__mocks__/nullthrows.js
@@ -0,0 +1,12 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ */
+
+'use strict';
+
+jest.dontMock('../nullthrows');
+
+module.exports = require('../nullthrows');
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/_shouldPolyfillES6Collection.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/_shouldPolyfillES6Collection.js
new file mode 100644
index 00000000..86b177f4
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/_shouldPolyfillES6Collection.js
@@ -0,0 +1,39 @@
+'use strict';
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @preventMunge
+ *
+ */
+
+/**
+ * Checks whether a collection name (e.g. "Map" or "Set") has a native polyfill
+ * that is safe to be used.
+ */
+function shouldPolyfillES6Collection(collectionName) {
+ var Collection = global[collectionName];
+ if (Collection == null) {
+ return true;
+ }
+
+ // The iterator protocol depends on `Symbol.iterator`. If a collection is
+ // implemented, but `Symbol` is not, it's going to break iteration because
+ // we'll be using custom "@@iterator" instead, which is not implemented on
+ // native collections.
+ if (typeof global.Symbol !== 'function') {
+ return true;
+ }
+
+ var proto = Collection.prototype;
+
+ // These checks are adapted from es6-shim: https://fburl.com/34437854
+ // NOTE: `isCallableWithoutNew` and `!supportsSubclassing` are not checked
+ // because they make debugging with "break on exceptions" difficult.
+ return Collection == null || typeof Collection !== 'function' || typeof proto.clear !== 'function' || new Collection().size !== 0 || typeof proto.keys !== 'function' || typeof proto.forEach !== 'function';
+}
+
+module.exports = shouldPolyfillES6Collection;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/_shouldPolyfillES6Collection.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/_shouldPolyfillES6Collection.js.flow
new file mode 100644
index 00000000..672b5f1f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/_shouldPolyfillES6Collection.js.flow
@@ -0,0 +1,38 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule _shouldPolyfillES6Collection
+ * @preventMunge
+ * @flow
+ */
+
+/**
+ * Checks whether a collection name (e.g. "Map" or "Set") has a native polyfill
+ * that is safe to be used.
+ */
+function shouldPolyfillES6Collection(collectionName: string): boolean {
+ const Collection = global[collectionName];
+ if (Collection == null) {
+ return true;
+ }
+
+ // The iterator protocol depends on `Symbol.iterator`. If a collection is
+ // implemented, but `Symbol` is not, it's going to break iteration because
+ // we'll be using custom "@@iterator" instead, which is not implemented on
+ // native collections.
+ if (typeof global.Symbol !== 'function') {
+ return true;
+ }
+
+ const proto = Collection.prototype;
+
+ // These checks are adapted from es6-shim: https://fburl.com/34437854
+ // NOTE: `isCallableWithoutNew` and `!supportsSubclassing` are not checked
+ // because they make debugging with "break on exceptions" difficult.
+ return Collection == null || typeof Collection !== 'function' || typeof proto.clear !== 'function' || new Collection().size !== 0 || typeof proto.keys !== 'function' || typeof proto.forEach !== 'function';
+}
+
+module.exports = shouldPolyfillES6Collection;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/areEqual.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/areEqual.js
new file mode 100644
index 00000000..e6a7da3b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/areEqual.js
@@ -0,0 +1,106 @@
+'use strict';
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ *
+ */
+
+var aStackPool = [];
+var bStackPool = [];
+
+/**
+ * Checks if two values are equal. Values may be primitives, arrays, or objects.
+ * Returns true if both arguments have the same keys and values.
+ *
+ * @see http://underscorejs.org
+ * @copyright 2009-2013 Jeremy Ashkenas, DocumentCloud Inc.
+ * @license MIT
+ */
+function areEqual(a, b) {
+ var aStack = aStackPool.length ? aStackPool.pop() : [];
+ var bStack = bStackPool.length ? bStackPool.pop() : [];
+ var result = eq(a, b, aStack, bStack);
+ aStack.length = 0;
+ bStack.length = 0;
+ aStackPool.push(aStack);
+ bStackPool.push(bStack);
+ return result;
+}
+
+function eq(a, b, aStack, bStack) {
+ if (a === b) {
+ // Identical objects are equal. `0 === -0`, but they aren't identical.
+ return a !== 0 || 1 / a == 1 / b;
+ }
+ if (a == null || b == null) {
+ // a or b can be `null` or `undefined`
+ return false;
+ }
+ if (typeof a != 'object' || typeof b != 'object') {
+ return false;
+ }
+ var objToStr = Object.prototype.toString;
+ var className = objToStr.call(a);
+ if (className != objToStr.call(b)) {
+ return false;
+ }
+ switch (className) {
+ case '[object String]':
+ return a == String(b);
+ case '[object Number]':
+ return isNaN(a) || isNaN(b) ? false : a == Number(b);
+ case '[object Date]':
+ case '[object Boolean]':
+ return +a == +b;
+ case '[object RegExp]':
+ return a.source == b.source && a.global == b.global && a.multiline == b.multiline && a.ignoreCase == b.ignoreCase;
+ }
+ // Assume equality for cyclic structures.
+ var length = aStack.length;
+ while (length--) {
+ if (aStack[length] == a) {
+ return bStack[length] == b;
+ }
+ }
+ aStack.push(a);
+ bStack.push(b);
+ var size = 0;
+ // Recursively compare objects and arrays.
+ if (className === '[object Array]') {
+ size = a.length;
+ if (size !== b.length) {
+ return false;
+ }
+ // Deep compare the contents, ignoring non-numeric properties.
+ while (size--) {
+ if (!eq(a[size], b[size], aStack, bStack)) {
+ return false;
+ }
+ }
+ } else {
+ if (a.constructor !== b.constructor) {
+ return false;
+ }
+ if (a.hasOwnProperty('valueOf') && b.hasOwnProperty('valueOf')) {
+ return a.valueOf() == b.valueOf();
+ }
+ var keys = Object.keys(a);
+ if (keys.length != Object.keys(b).length) {
+ return false;
+ }
+ for (var i = 0; i < keys.length; i++) {
+ if (!eq(a[keys[i]], b[keys[i]], aStack, bStack)) {
+ return false;
+ }
+ }
+ }
+ aStack.pop();
+ bStack.pop();
+ return true;
+}
+
+module.exports = areEqual;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/areEqual.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/areEqual.js.flow
new file mode 100644
index 00000000..06e53ec6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/areEqual.js.flow
@@ -0,0 +1,105 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule areEqual
+ * @flow
+ */
+
+const aStackPool = [];
+const bStackPool = [];
+
+/**
+ * Checks if two values are equal. Values may be primitives, arrays, or objects.
+ * Returns true if both arguments have the same keys and values.
+ *
+ * @see http://underscorejs.org
+ * @copyright 2009-2013 Jeremy Ashkenas, DocumentCloud Inc.
+ * @license MIT
+ */
+function areEqual(a: any, b: any): boolean {
+ const aStack = aStackPool.length ? aStackPool.pop() : [];
+ const bStack = bStackPool.length ? bStackPool.pop() : [];
+ const result = eq(a, b, aStack, bStack);
+ aStack.length = 0;
+ bStack.length = 0;
+ aStackPool.push(aStack);
+ bStackPool.push(bStack);
+ return result;
+}
+
+function eq(a: any, b: any, aStack: Array, bStack: Array): boolean {
+ if (a === b) {
+ // Identical objects are equal. `0 === -0`, but they aren't identical.
+ return a !== 0 || 1 / a == 1 / b;
+ }
+ if (a == null || b == null) {
+ // a or b can be `null` or `undefined`
+ return false;
+ }
+ if (typeof a != 'object' || typeof b != 'object') {
+ return false;
+ }
+ const objToStr = Object.prototype.toString;
+ const className = objToStr.call(a);
+ if (className != objToStr.call(b)) {
+ return false;
+ }
+ switch (className) {
+ case '[object String]':
+ return a == String(b);
+ case '[object Number]':
+ return isNaN(a) || isNaN(b) ? false : a == Number(b);
+ case '[object Date]':
+ case '[object Boolean]':
+ return +a == +b;
+ case '[object RegExp]':
+ return a.source == b.source && a.global == b.global && a.multiline == b.multiline && a.ignoreCase == b.ignoreCase;
+ }
+ // Assume equality for cyclic structures.
+ let length = aStack.length;
+ while (length--) {
+ if (aStack[length] == a) {
+ return bStack[length] == b;
+ }
+ }
+ aStack.push(a);
+ bStack.push(b);
+ let size = 0;
+ // Recursively compare objects and arrays.
+ if (className === '[object Array]') {
+ size = a.length;
+ if (size !== b.length) {
+ return false;
+ }
+ // Deep compare the contents, ignoring non-numeric properties.
+ while (size--) {
+ if (!eq(a[size], b[size], aStack, bStack)) {
+ return false;
+ }
+ }
+ } else {
+ if (a.constructor !== b.constructor) {
+ return false;
+ }
+ if (a.hasOwnProperty('valueOf') && b.hasOwnProperty('valueOf')) {
+ return a.valueOf() == b.valueOf();
+ }
+ const keys = Object.keys(a);
+ if (keys.length != Object.keys(b).length) {
+ return false;
+ }
+ for (let i = 0; i < keys.length; i++) {
+ if (!eq(a[keys[i]], b[keys[i]], aStack, bStack)) {
+ return false;
+ }
+ }
+ }
+ aStack.pop();
+ bStack.pop();
+ return true;
+}
+
+module.exports = areEqual;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/base62.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/base62.js
new file mode 100644
index 00000000..e2e4d82b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/base62.js
@@ -0,0 +1,26 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ *
+ */
+
+'use strict';
+
+var BASE62 = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ';
+
+function base62(number) {
+ if (!number) {
+ return '0';
+ }
+ var string = '';
+ while (number > 0) {
+ string = BASE62[number % 62] + string;
+ number = Math.floor(number / 62);
+ }
+ return string;
+}
+
+module.exports = base62;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/base62.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/base62.js.flow
new file mode 100644
index 00000000..f8151201
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/base62.js.flow
@@ -0,0 +1,27 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule base62
+ * @flow
+ */
+
+'use strict';
+
+const BASE62 = '0123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ';
+
+function base62(number: number): string {
+ if (!number) {
+ return '0';
+ }
+ let string = '';
+ while (number > 0) {
+ string = BASE62[number % 62] + string;
+ number = Math.floor(number / 62);
+ }
+ return string;
+}
+
+module.exports = base62;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/camelize.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/camelize.js
new file mode 100644
index 00000000..ca010a29
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/camelize.js
@@ -0,0 +1,29 @@
+"use strict";
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @typechecks
+ */
+
+var _hyphenPattern = /-(.)/g;
+
+/**
+ * Camelcases a hyphenated string, for example:
+ *
+ * > camelize('background-color')
+ * < "backgroundColor"
+ *
+ * @param {string} string
+ * @return {string}
+ */
+function camelize(string) {
+ return string.replace(_hyphenPattern, function (_, character) {
+ return character.toUpperCase();
+ });
+}
+
+module.exports = camelize;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/camelize.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/camelize.js.flow
new file mode 100644
index 00000000..9b0b4234
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/camelize.js.flow
@@ -0,0 +1,28 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule camelize
+ * @typechecks
+ */
+
+const _hyphenPattern = /-(.)/g;
+
+/**
+ * Camelcases a hyphenated string, for example:
+ *
+ * > camelize('background-color')
+ * < "backgroundColor"
+ *
+ * @param {string} string
+ * @return {string}
+ */
+function camelize(string) {
+ return string.replace(_hyphenPattern, function (_, character) {
+ return character.toUpperCase();
+ });
+}
+
+module.exports = camelize;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/camelizeStyleName.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/camelizeStyleName.js
new file mode 100644
index 00000000..6b076a37
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/camelizeStyleName.js
@@ -0,0 +1,37 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @typechecks
+ */
+
+'use strict';
+
+var camelize = require('./camelize');
+
+var msPattern = /^-ms-/;
+
+/**
+ * Camelcases a hyphenated CSS property name, for example:
+ *
+ * > camelizeStyleName('background-color')
+ * < "backgroundColor"
+ * > camelizeStyleName('-moz-transition')
+ * < "MozTransition"
+ * > camelizeStyleName('-ms-transition')
+ * < "msTransition"
+ *
+ * As Andi Smith suggests
+ * (http://www.andismith.com/blog/2012/02/modernizr-prefixed/), an `-ms` prefix
+ * is converted to lowercase `ms`.
+ *
+ * @param {string} string
+ * @return {string}
+ */
+function camelizeStyleName(string) {
+ return camelize(string.replace(msPattern, 'ms-'));
+}
+
+module.exports = camelizeStyleName;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/camelizeStyleName.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/camelizeStyleName.js.flow
new file mode 100644
index 00000000..8883feac
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/camelizeStyleName.js.flow
@@ -0,0 +1,38 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule camelizeStyleName
+ * @typechecks
+ */
+
+'use strict';
+
+const camelize = require('./camelize');
+
+const msPattern = /^-ms-/;
+
+/**
+ * Camelcases a hyphenated CSS property name, for example:
+ *
+ * > camelizeStyleName('background-color')
+ * < "backgroundColor"
+ * > camelizeStyleName('-moz-transition')
+ * < "MozTransition"
+ * > camelizeStyleName('-ms-transition')
+ * < "msTransition"
+ *
+ * As Andi Smith suggests
+ * (http://www.andismith.com/blog/2012/02/modernizr-prefixed/), an `-ms` prefix
+ * is converted to lowercase `ms`.
+ *
+ * @param {string} string
+ * @return {string}
+ */
+function camelizeStyleName(string) {
+ return camelize(string.replace(msPattern, 'ms-'));
+}
+
+module.exports = camelizeStyleName;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/compactArray.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/compactArray.js
new file mode 100644
index 00000000..5db77540
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/compactArray.js
@@ -0,0 +1,27 @@
+/**
+ * Copyright 2015-present Facebook. All Rights Reserved.
+ *
+ * @typechecks
+ *
+ */
+
+'use strict';
+
+/**
+ * Returns a new Array containing all the element of the source array except
+ * `null` and `undefined` ones. This brings the benefit of strong typing over
+ * `Array.prototype.filter`.
+ */
+
+function compactArray(array) {
+ var result = [];
+ for (var i = 0; i < array.length; ++i) {
+ var elem = array[i];
+ if (elem != null) {
+ result.push(elem);
+ }
+ }
+ return result;
+}
+
+module.exports = compactArray;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/compactArray.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/compactArray.js.flow
new file mode 100644
index 00000000..5b8ccbec
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/compactArray.js.flow
@@ -0,0 +1,28 @@
+/**
+ * Copyright 2015-present Facebook. All Rights Reserved.
+ *
+ * @providesModule compactArray
+ * @typechecks
+ * @flow
+ */
+
+'use strict';
+
+/**
+ * Returns a new Array containing all the element of the source array except
+ * `null` and `undefined` ones. This brings the benefit of strong typing over
+ * `Array.prototype.filter`.
+ */
+
+function compactArray(array: Array): Array {
+ var result = [];
+ for (var i = 0; i < array.length; ++i) {
+ var elem = array[i];
+ if (elem != null) {
+ result.push(elem);
+ }
+ }
+ return result;
+}
+
+module.exports = compactArray;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/concatAllArray.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/concatAllArray.js
new file mode 100644
index 00000000..a9649824
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/concatAllArray.js
@@ -0,0 +1,33 @@
+'use strict';
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @typechecks
+ */
+
+var push = Array.prototype.push;
+
+/**
+ * Concats an array of arrays into a single flat array.
+ *
+ * @param {array} array
+ * @return {array}
+ */
+function concatAllArray(array) {
+ var ret = [];
+ for (var ii = 0; ii < array.length; ii++) {
+ var value = array[ii];
+ if (Array.isArray(value)) {
+ push.apply(ret, value);
+ } else if (value != null) {
+ throw new TypeError('concatAllArray: All items in the array must be an array or null, ' + 'got "' + value + '" at index "' + ii + '" instead');
+ }
+ }
+ return ret;
+}
+
+module.exports = concatAllArray;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/concatAllArray.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/concatAllArray.js.flow
new file mode 100644
index 00000000..41103ff2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/concatAllArray.js.flow
@@ -0,0 +1,32 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule concatAllArray
+ * @typechecks
+ */
+
+var push = Array.prototype.push;
+
+/**
+ * Concats an array of arrays into a single flat array.
+ *
+ * @param {array} array
+ * @return {array}
+ */
+function concatAllArray(array) {
+ var ret = [];
+ for (var ii = 0; ii < array.length; ii++) {
+ var value = array[ii];
+ if (Array.isArray(value)) {
+ push.apply(ret, value);
+ } else if (value != null) {
+ throw new TypeError('concatAllArray: All items in the array must be an array or null, ' + 'got "' + value + '" at index "' + ii + '" instead');
+ }
+ }
+ return ret;
+}
+
+module.exports = concatAllArray;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/containsNode.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/containsNode.js
new file mode 100644
index 00000000..bee5085e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/containsNode.js
@@ -0,0 +1,37 @@
+'use strict';
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ *
+ */
+
+var isTextNode = require('./isTextNode');
+
+/*eslint-disable no-bitwise */
+
+/**
+ * Checks if a given DOM node contains or is another DOM node.
+ */
+function containsNode(outerNode, innerNode) {
+ if (!outerNode || !innerNode) {
+ return false;
+ } else if (outerNode === innerNode) {
+ return true;
+ } else if (isTextNode(outerNode)) {
+ return false;
+ } else if (isTextNode(innerNode)) {
+ return containsNode(outerNode, innerNode.parentNode);
+ } else if ('contains' in outerNode) {
+ return outerNode.contains(innerNode);
+ } else if (outerNode.compareDocumentPosition) {
+ return !!(outerNode.compareDocumentPosition(innerNode) & 16);
+ } else {
+ return false;
+ }
+}
+
+module.exports = containsNode;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/containsNode.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/containsNode.js.flow
new file mode 100644
index 00000000..b2117f31
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/containsNode.js.flow
@@ -0,0 +1,36 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule containsNode
+ * @flow
+ */
+
+const isTextNode = require('./isTextNode');
+
+/*eslint-disable no-bitwise */
+
+/**
+ * Checks if a given DOM node contains or is another DOM node.
+ */
+function containsNode(outerNode: ?Node, innerNode: ?Node): boolean {
+ if (!outerNode || !innerNode) {
+ return false;
+ } else if (outerNode === innerNode) {
+ return true;
+ } else if (isTextNode(outerNode)) {
+ return false;
+ } else if (isTextNode(innerNode)) {
+ return containsNode(outerNode, innerNode.parentNode);
+ } else if ('contains' in outerNode) {
+ return outerNode.contains(innerNode);
+ } else if (outerNode.compareDocumentPosition) {
+ return !!(outerNode.compareDocumentPosition(innerNode) & 16);
+ } else {
+ return false;
+ }
+}
+
+module.exports = containsNode;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/countDistinct.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/countDistinct.js
new file mode 100644
index 00000000..c8aa925f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/countDistinct.js
@@ -0,0 +1,51 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ *
+ */
+
+'use strict';
+
+var Set = require('./Set');
+
+var emptyFunction = require('./emptyFunction');
+
+/**
+ * Returns the count of distinct elements selected from an array.
+ */
+function countDistinct(iter, selector) {
+ selector = selector || emptyFunction.thatReturnsArgument;
+
+ var set = new Set();
+ var _iteratorNormalCompletion = true;
+ var _didIteratorError = false;
+ var _iteratorError = undefined;
+
+ try {
+ for (var _iterator = iter[Symbol.iterator](), _step; !(_iteratorNormalCompletion = (_step = _iterator.next()).done); _iteratorNormalCompletion = true) {
+ var val = _step.value;
+
+ set.add(selector(val));
+ }
+ } catch (err) {
+ _didIteratorError = true;
+ _iteratorError = err;
+ } finally {
+ try {
+ if (!_iteratorNormalCompletion && _iterator['return']) {
+ _iterator['return']();
+ }
+ } finally {
+ if (_didIteratorError) {
+ throw _iteratorError;
+ }
+ }
+ }
+
+ return set.size;
+}
+
+module.exports = countDistinct;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/countDistinct.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/countDistinct.js.flow
new file mode 100644
index 00000000..25676aa8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/countDistinct.js.flow
@@ -0,0 +1,31 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule countDistinct
+ * @flow
+ */
+
+'use strict';
+
+var Set = require('./Set');
+
+var emptyFunction = require('./emptyFunction');
+
+/**
+ * Returns the count of distinct elements selected from an array.
+ */
+function countDistinct(iter: Iterable, selector: (item: T1) => T2): number {
+ selector = selector || emptyFunction.thatReturnsArgument;
+
+ var set = new Set();
+ for (var val of iter) {
+ set.add(selector(val));
+ }
+
+ return set.size;
+}
+
+module.exports = countDistinct;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/crc32.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/crc32.js
new file mode 100644
index 00000000..806694ca
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/crc32.js
@@ -0,0 +1,27 @@
+"use strict";
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ *
+ */
+
+function crc32(str) {
+ /* jslint bitwise: true */
+ var crc = -1;
+ for (var i = 0, len = str.length; i < len; i++) {
+ crc = crc >>> 8 ^ table[(crc ^ str.charCodeAt(i)) & 0xFF];
+ }
+ return ~crc;
+}
+
+var table = [0x00000000, 0x77073096, 0xEE0E612C, 0x990951BA, 0x076DC419, 0x706AF48F, 0xE963A535, 0x9E6495A3, 0x0EDB8832, 0x79DCB8A4, 0xE0D5E91E, 0x97D2D988, 0x09B64C2B, 0x7EB17CBD, 0xE7B82D07, 0x90BF1D91, 0x1DB71064, 0x6AB020F2, 0xF3B97148, 0x84BE41DE, 0x1ADAD47D, 0x6DDDE4EB, 0xF4D4B551, 0x83D385C7, 0x136C9856, 0x646BA8C0, 0xFD62F97A, 0x8A65C9EC, 0x14015C4F, 0x63066CD9, 0xFA0F3D63, 0x8D080DF5, 0x3B6E20C8, 0x4C69105E, 0xD56041E4, 0xA2677172, 0x3C03E4D1, 0x4B04D447, 0xD20D85FD, 0xA50AB56B, 0x35B5A8FA, 0x42B2986C, 0xDBBBC9D6, 0xACBCF940, 0x32D86CE3, 0x45DF5C75, 0xDCD60DCF, 0xABD13D59, 0x26D930AC, 0x51DE003A, 0xC8D75180, 0xBFD06116, 0x21B4F4B5, 0x56B3C423, 0xCFBA9599, 0xB8BDA50F, 0x2802B89E, 0x5F058808, 0xC60CD9B2, 0xB10BE924, 0x2F6F7C87, 0x58684C11, 0xC1611DAB, 0xB6662D3D, 0x76DC4190, 0x01DB7106, 0x98D220BC, 0xEFD5102A, 0x71B18589, 0x06B6B51F, 0x9FBFE4A5, 0xE8B8D433, 0x7807C9A2, 0x0F00F934, 0x9609A88E, 0xE10E9818, 0x7F6A0DBB, 0x086D3D2D, 0x91646C97, 0xE6635C01, 0x6B6B51F4, 0x1C6C6162, 0x856530D8, 0xF262004E, 0x6C0695ED, 0x1B01A57B, 0x8208F4C1, 0xF50FC457, 0x65B0D9C6, 0x12B7E950, 0x8BBEB8EA, 0xFCB9887C, 0x62DD1DDF, 0x15DA2D49, 0x8CD37CF3, 0xFBD44C65, 0x4DB26158, 0x3AB551CE, 0xA3BC0074, 0xD4BB30E2, 0x4ADFA541, 0x3DD895D7, 0xA4D1C46D, 0xD3D6F4FB, 0x4369E96A, 0x346ED9FC, 0xAD678846, 0xDA60B8D0, 0x44042D73, 0x33031DE5, 0xAA0A4C5F, 0xDD0D7CC9, 0x5005713C, 0x270241AA, 0xBE0B1010, 0xC90C2086, 0x5768B525, 0x206F85B3, 0xB966D409, 0xCE61E49F, 0x5EDEF90E, 0x29D9C998, 0xB0D09822, 0xC7D7A8B4, 0x59B33D17, 0x2EB40D81, 0xB7BD5C3B, 0xC0BA6CAD, 0xEDB88320, 0x9ABFB3B6, 0x03B6E20C, 0x74B1D29A, 0xEAD54739, 0x9DD277AF, 0x04DB2615, 0x73DC1683, 0xE3630B12, 0x94643B84, 0x0D6D6A3E, 0x7A6A5AA8, 0xE40ECF0B, 0x9309FF9D, 0x0A00AE27, 0x7D079EB1, 0xF00F9344, 0x8708A3D2, 0x1E01F268, 0x6906C2FE, 0xF762575D, 0x806567CB, 0x196C3671, 0x6E6B06E7, 0xFED41B76, 0x89D32BE0, 0x10DA7A5A, 0x67DD4ACC, 0xF9B9DF6F, 0x8EBEEFF9, 0x17B7BE43, 0x60B08ED5, 0xD6D6A3E8, 0xA1D1937E, 0x38D8C2C4, 0x4FDFF252, 0xD1BB67F1, 0xA6BC5767, 0x3FB506DD, 0x48B2364B, 0xD80D2BDA, 0xAF0A1B4C, 0x36034AF6, 0x41047A60, 0xDF60EFC3, 0xA867DF55, 0x316E8EEF, 0x4669BE79, 0xCB61B38C, 0xBC66831A, 0x256FD2A0, 0x5268E236, 0xCC0C7795, 0xBB0B4703, 0x220216B9, 0x5505262F, 0xC5BA3BBE, 0xB2BD0B28, 0x2BB45A92, 0x5CB36A04, 0xC2D7FFA7, 0xB5D0CF31, 0x2CD99E8B, 0x5BDEAE1D, 0x9B64C2B0, 0xEC63F226, 0x756AA39C, 0x026D930A, 0x9C0906A9, 0xEB0E363F, 0x72076785, 0x05005713, 0x95BF4A82, 0xE2B87A14, 0x7BB12BAE, 0x0CB61B38, 0x92D28E9B, 0xE5D5BE0D, 0x7CDCEFB7, 0x0BDBDF21, 0x86D3D2D4, 0xF1D4E242, 0x68DDB3F8, 0x1FDA836E, 0x81BE16CD, 0xF6B9265B, 0x6FB077E1, 0x18B74777, 0x88085AE6, 0xFF0F6A70, 0x66063BCA, 0x11010B5C, 0x8F659EFF, 0xF862AE69, 0x616BFFD3, 0x166CCF45, 0xA00AE278, 0xD70DD2EE, 0x4E048354, 0x3903B3C2, 0xA7672661, 0xD06016F7, 0x4969474D, 0x3E6E77DB, 0xAED16A4A, 0xD9D65ADC, 0x40DF0B66, 0x37D83BF0, 0xA9BCAE53, 0xDEBB9EC5, 0x47B2CF7F, 0x30B5FFE9, 0xBDBDF21C, 0xCABAC28A, 0x53B39330, 0x24B4A3A6, 0xBAD03605, 0xCDD70693, 0x54DE5729, 0x23D967BF, 0xB3667A2E, 0xC4614AB8, 0x5D681B02, 0x2A6F2B94, 0xB40BBE37, 0xC30C8EA1, 0x5A05DF1B, 0x2D02EF8D];
+
+if (global.Int32Array !== undefined) {
+ table = new Int32Array(table);
+}
+
+module.exports = crc32;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/crc32.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/crc32.js.flow
new file mode 100644
index 00000000..00cab448
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/crc32.js.flow
@@ -0,0 +1,26 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule crc32
+ * @flow
+ */
+
+function crc32(str: string): number {
+ /* jslint bitwise: true */
+ var crc = -1;
+ for (var i = 0, len = str.length; i < len; i++) {
+ crc = crc >>> 8 ^ table[(crc ^ str.charCodeAt(i)) & 0xFF];
+ }
+ return ~crc;
+}
+
+var table = [0x00000000, 0x77073096, 0xEE0E612C, 0x990951BA, 0x076DC419, 0x706AF48F, 0xE963A535, 0x9E6495A3, 0x0EDB8832, 0x79DCB8A4, 0xE0D5E91E, 0x97D2D988, 0x09B64C2B, 0x7EB17CBD, 0xE7B82D07, 0x90BF1D91, 0x1DB71064, 0x6AB020F2, 0xF3B97148, 0x84BE41DE, 0x1ADAD47D, 0x6DDDE4EB, 0xF4D4B551, 0x83D385C7, 0x136C9856, 0x646BA8C0, 0xFD62F97A, 0x8A65C9EC, 0x14015C4F, 0x63066CD9, 0xFA0F3D63, 0x8D080DF5, 0x3B6E20C8, 0x4C69105E, 0xD56041E4, 0xA2677172, 0x3C03E4D1, 0x4B04D447, 0xD20D85FD, 0xA50AB56B, 0x35B5A8FA, 0x42B2986C, 0xDBBBC9D6, 0xACBCF940, 0x32D86CE3, 0x45DF5C75, 0xDCD60DCF, 0xABD13D59, 0x26D930AC, 0x51DE003A, 0xC8D75180, 0xBFD06116, 0x21B4F4B5, 0x56B3C423, 0xCFBA9599, 0xB8BDA50F, 0x2802B89E, 0x5F058808, 0xC60CD9B2, 0xB10BE924, 0x2F6F7C87, 0x58684C11, 0xC1611DAB, 0xB6662D3D, 0x76DC4190, 0x01DB7106, 0x98D220BC, 0xEFD5102A, 0x71B18589, 0x06B6B51F, 0x9FBFE4A5, 0xE8B8D433, 0x7807C9A2, 0x0F00F934, 0x9609A88E, 0xE10E9818, 0x7F6A0DBB, 0x086D3D2D, 0x91646C97, 0xE6635C01, 0x6B6B51F4, 0x1C6C6162, 0x856530D8, 0xF262004E, 0x6C0695ED, 0x1B01A57B, 0x8208F4C1, 0xF50FC457, 0x65B0D9C6, 0x12B7E950, 0x8BBEB8EA, 0xFCB9887C, 0x62DD1DDF, 0x15DA2D49, 0x8CD37CF3, 0xFBD44C65, 0x4DB26158, 0x3AB551CE, 0xA3BC0074, 0xD4BB30E2, 0x4ADFA541, 0x3DD895D7, 0xA4D1C46D, 0xD3D6F4FB, 0x4369E96A, 0x346ED9FC, 0xAD678846, 0xDA60B8D0, 0x44042D73, 0x33031DE5, 0xAA0A4C5F, 0xDD0D7CC9, 0x5005713C, 0x270241AA, 0xBE0B1010, 0xC90C2086, 0x5768B525, 0x206F85B3, 0xB966D409, 0xCE61E49F, 0x5EDEF90E, 0x29D9C998, 0xB0D09822, 0xC7D7A8B4, 0x59B33D17, 0x2EB40D81, 0xB7BD5C3B, 0xC0BA6CAD, 0xEDB88320, 0x9ABFB3B6, 0x03B6E20C, 0x74B1D29A, 0xEAD54739, 0x9DD277AF, 0x04DB2615, 0x73DC1683, 0xE3630B12, 0x94643B84, 0x0D6D6A3E, 0x7A6A5AA8, 0xE40ECF0B, 0x9309FF9D, 0x0A00AE27, 0x7D079EB1, 0xF00F9344, 0x8708A3D2, 0x1E01F268, 0x6906C2FE, 0xF762575D, 0x806567CB, 0x196C3671, 0x6E6B06E7, 0xFED41B76, 0x89D32BE0, 0x10DA7A5A, 0x67DD4ACC, 0xF9B9DF6F, 0x8EBEEFF9, 0x17B7BE43, 0x60B08ED5, 0xD6D6A3E8, 0xA1D1937E, 0x38D8C2C4, 0x4FDFF252, 0xD1BB67F1, 0xA6BC5767, 0x3FB506DD, 0x48B2364B, 0xD80D2BDA, 0xAF0A1B4C, 0x36034AF6, 0x41047A60, 0xDF60EFC3, 0xA867DF55, 0x316E8EEF, 0x4669BE79, 0xCB61B38C, 0xBC66831A, 0x256FD2A0, 0x5268E236, 0xCC0C7795, 0xBB0B4703, 0x220216B9, 0x5505262F, 0xC5BA3BBE, 0xB2BD0B28, 0x2BB45A92, 0x5CB36A04, 0xC2D7FFA7, 0xB5D0CF31, 0x2CD99E8B, 0x5BDEAE1D, 0x9B64C2B0, 0xEC63F226, 0x756AA39C, 0x026D930A, 0x9C0906A9, 0xEB0E363F, 0x72076785, 0x05005713, 0x95BF4A82, 0xE2B87A14, 0x7BB12BAE, 0x0CB61B38, 0x92D28E9B, 0xE5D5BE0D, 0x7CDCEFB7, 0x0BDBDF21, 0x86D3D2D4, 0xF1D4E242, 0x68DDB3F8, 0x1FDA836E, 0x81BE16CD, 0xF6B9265B, 0x6FB077E1, 0x18B74777, 0x88085AE6, 0xFF0F6A70, 0x66063BCA, 0x11010B5C, 0x8F659EFF, 0xF862AE69, 0x616BFFD3, 0x166CCF45, 0xA00AE278, 0xD70DD2EE, 0x4E048354, 0x3903B3C2, 0xA7672661, 0xD06016F7, 0x4969474D, 0x3E6E77DB, 0xAED16A4A, 0xD9D65ADC, 0x40DF0B66, 0x37D83BF0, 0xA9BCAE53, 0xDEBB9EC5, 0x47B2CF7F, 0x30B5FFE9, 0xBDBDF21C, 0xCABAC28A, 0x53B39330, 0x24B4A3A6, 0xBAD03605, 0xCDD70693, 0x54DE5729, 0x23D967BF, 0xB3667A2E, 0xC4614AB8, 0x5D681B02, 0x2A6F2B94, 0xB40BBE37, 0xC30C8EA1, 0x5A05DF1B, 0x2D02EF8D];
+
+if (global.Int32Array !== undefined) {
+ table = new Int32Array(table);
+}
+
+module.exports = crc32;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/createArrayFromMixed.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/createArrayFromMixed.js
new file mode 100644
index 00000000..879141ae
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/createArrayFromMixed.js
@@ -0,0 +1,124 @@
+'use strict';
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @typechecks
+ */
+
+var invariant = require('./invariant');
+
+/**
+ * Convert array-like objects to arrays.
+ *
+ * This API assumes the caller knows the contents of the data type. For less
+ * well defined inputs use createArrayFromMixed.
+ *
+ * @param {object|function|filelist} obj
+ * @return {array}
+ */
+function toArray(obj) {
+ var length = obj.length;
+
+ // Some browsers builtin objects can report typeof 'function' (e.g. NodeList
+ // in old versions of Safari).
+ !(!Array.isArray(obj) && (typeof obj === 'object' || typeof obj === 'function')) ? process.env.NODE_ENV !== 'production' ? invariant(false, 'toArray: Array-like object expected') : invariant(false) : void 0;
+
+ !(typeof length === 'number') ? process.env.NODE_ENV !== 'production' ? invariant(false, 'toArray: Object needs a length property') : invariant(false) : void 0;
+
+ !(length === 0 || length - 1 in obj) ? process.env.NODE_ENV !== 'production' ? invariant(false, 'toArray: Object should have keys for indices') : invariant(false) : void 0;
+
+ !(typeof obj.callee !== 'function') ? process.env.NODE_ENV !== 'production' ? invariant(false, 'toArray: Object can\'t be `arguments`. Use rest params ' + '(function(...args) {}) or Array.from() instead.') : invariant(false) : void 0;
+
+ // Old IE doesn't give collections access to hasOwnProperty. Assume inputs
+ // without method will throw during the slice call and skip straight to the
+ // fallback.
+ if (obj.hasOwnProperty) {
+ try {
+ return Array.prototype.slice.call(obj);
+ } catch (e) {
+ // IE < 9 does not support Array#slice on collections objects
+ }
+ }
+
+ // Fall back to copying key by key. This assumes all keys have a value,
+ // so will not preserve sparsely populated inputs.
+ var ret = Array(length);
+ for (var ii = 0; ii < length; ii++) {
+ ret[ii] = obj[ii];
+ }
+ return ret;
+}
+
+/**
+ * Perform a heuristic test to determine if an object is "array-like".
+ *
+ * A monk asked Joshu, a Zen master, "Has a dog Buddha nature?"
+ * Joshu replied: "Mu."
+ *
+ * This function determines if its argument has "array nature": it returns
+ * true if the argument is an actual array, an `arguments' object, or an
+ * HTMLCollection (e.g. node.childNodes or node.getElementsByTagName()).
+ *
+ * It will return false for other array-like objects like Filelist.
+ *
+ * @param {*} obj
+ * @return {boolean}
+ */
+function hasArrayNature(obj) {
+ return (
+ // not null/false
+ !!obj && (
+ // arrays are objects, NodeLists are functions in Safari
+ typeof obj == 'object' || typeof obj == 'function') &&
+ // quacks like an array
+ 'length' in obj &&
+ // not window
+ !('setInterval' in obj) &&
+ // no DOM node should be considered an array-like
+ // a 'select' element has 'length' and 'item' properties on IE8
+ typeof obj.nodeType != 'number' && (
+ // a real array
+ Array.isArray(obj) ||
+ // arguments
+ 'callee' in obj ||
+ // HTMLCollection/NodeList
+ 'item' in obj)
+ );
+}
+
+/**
+ * Ensure that the argument is an array by wrapping it in an array if it is not.
+ * Creates a copy of the argument if it is already an array.
+ *
+ * This is mostly useful idiomatically:
+ *
+ * var createArrayFromMixed = require('createArrayFromMixed');
+ *
+ * function takesOneOrMoreThings(things) {
+ * things = createArrayFromMixed(things);
+ * ...
+ * }
+ *
+ * This allows you to treat `things' as an array, but accept scalars in the API.
+ *
+ * If you need to convert an array-like object, like `arguments`, into an array
+ * use toArray instead.
+ *
+ * @param {*} obj
+ * @return {array}
+ */
+function createArrayFromMixed(obj) {
+ if (!hasArrayNature(obj)) {
+ return [obj];
+ } else if (Array.isArray(obj)) {
+ return obj.slice();
+ } else {
+ return toArray(obj);
+ }
+}
+
+module.exports = createArrayFromMixed;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/createArrayFromMixed.js.flow b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/createArrayFromMixed.js.flow
new file mode 100644
index 00000000..1448db10
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/createArrayFromMixed.js.flow
@@ -0,0 +1,123 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @providesModule createArrayFromMixed
+ * @typechecks
+ */
+
+const invariant = require('./invariant');
+
+/**
+ * Convert array-like objects to arrays.
+ *
+ * This API assumes the caller knows the contents of the data type. For less
+ * well defined inputs use createArrayFromMixed.
+ *
+ * @param {object|function|filelist} obj
+ * @return {array}
+ */
+function toArray(obj) {
+ const length = obj.length;
+
+ // Some browsers builtin objects can report typeof 'function' (e.g. NodeList
+ // in old versions of Safari).
+ invariant(!Array.isArray(obj) && (typeof obj === 'object' || typeof obj === 'function'), 'toArray: Array-like object expected');
+
+ invariant(typeof length === 'number', 'toArray: Object needs a length property');
+
+ invariant(length === 0 || length - 1 in obj, 'toArray: Object should have keys for indices');
+
+ invariant(typeof obj.callee !== 'function', 'toArray: Object can\'t be `arguments`. Use rest params ' + '(function(...args) {}) or Array.from() instead.');
+
+ // Old IE doesn't give collections access to hasOwnProperty. Assume inputs
+ // without method will throw during the slice call and skip straight to the
+ // fallback.
+ if (obj.hasOwnProperty) {
+ try {
+ return Array.prototype.slice.call(obj);
+ } catch (e) {
+ // IE < 9 does not support Array#slice on collections objects
+ }
+ }
+
+ // Fall back to copying key by key. This assumes all keys have a value,
+ // so will not preserve sparsely populated inputs.
+ const ret = Array(length);
+ for (let ii = 0; ii < length; ii++) {
+ ret[ii] = obj[ii];
+ }
+ return ret;
+}
+
+/**
+ * Perform a heuristic test to determine if an object is "array-like".
+ *
+ * A monk asked Joshu, a Zen master, "Has a dog Buddha nature?"
+ * Joshu replied: "Mu."
+ *
+ * This function determines if its argument has "array nature": it returns
+ * true if the argument is an actual array, an `arguments' object, or an
+ * HTMLCollection (e.g. node.childNodes or node.getElementsByTagName()).
+ *
+ * It will return false for other array-like objects like Filelist.
+ *
+ * @param {*} obj
+ * @return {boolean}
+ */
+function hasArrayNature(obj) {
+ return (
+ // not null/false
+ !!obj && (
+ // arrays are objects, NodeLists are functions in Safari
+ typeof obj == 'object' || typeof obj == 'function') &&
+ // quacks like an array
+ 'length' in obj &&
+ // not window
+ !('setInterval' in obj) &&
+ // no DOM node should be considered an array-like
+ // a 'select' element has 'length' and 'item' properties on IE8
+ typeof obj.nodeType != 'number' && (
+ // a real array
+ Array.isArray(obj) ||
+ // arguments
+ 'callee' in obj ||
+ // HTMLCollection/NodeList
+ 'item' in obj)
+ );
+}
+
+/**
+ * Ensure that the argument is an array by wrapping it in an array if it is not.
+ * Creates a copy of the argument if it is already an array.
+ *
+ * This is mostly useful idiomatically:
+ *
+ * var createArrayFromMixed = require('createArrayFromMixed');
+ *
+ * function takesOneOrMoreThings(things) {
+ * things = createArrayFromMixed(things);
+ * ...
+ * }
+ *
+ * This allows you to treat `things' as an array, but accept scalars in the API.
+ *
+ * If you need to convert an array-like object, like `arguments`, into an array
+ * use toArray instead.
+ *
+ * @param {*} obj
+ * @return {array}
+ */
+function createArrayFromMixed(obj) {
+ if (!hasArrayNature(obj)) {
+ return [obj];
+ } else if (Array.isArray(obj)) {
+ return obj.slice();
+ } else {
+ return toArray(obj);
+ }
+}
+
+module.exports = createArrayFromMixed;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/createNodesFromMarkup.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/createNodesFromMarkup.js
new file mode 100644
index 00000000..a0c21611
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/fbjs/lib/createNodesFromMarkup.js
@@ -0,0 +1,81 @@
+'use strict';
+
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ *
+ * @typechecks
+ */
+
+/*eslint-disable fb-www/unsafe-html*/
+
+var ExecutionEnvironment = require('./ExecutionEnvironment');
+
+var createArrayFromMixed = require('./createArrayFromMixed');
+var getMarkupWrap = require('./getMarkupWrap');
+var invariant = require('./invariant');
+
+/**
+ * Dummy container used to render all markup.
+ */
+var dummyNode = ExecutionEnvironment.canUseDOM ? document.createElement('div') : null;
+
+/**
+ * Pattern used by `getNodeName`.
+ */
+var nodeNamePattern = /^\s*<(\w+)/;
+
+/**
+ * Extracts the `nodeName` of the first element in a string of markup.
+ *
+ * @param {string} markup String of markup.
+ * @return {?string} Node name of the supplied markup.
+ */
+function getNodeName(markup) {
+ var nodeNameMatch = markup.match(nodeNamePattern);
+ return nodeNameMatch && nodeNameMatch[1].toLowerCase();
+}
+
+/**
+ * Creates an array containing the nodes rendered from the supplied markup. The
+ * optionally supplied `handleScript` function will be invoked once for each
+ *
+```
+
+Note that the [es5-shim](https://github.com/es-shims/es5-shim) must be loaded before this library to support browsers pre IE9.
+
+```html
+
+```
+
+## Usage
+
+The example below shows how you can load the promise library (in a way that works on both client and server using node or browserify). It then demonstrates creating a promise from scratch. You simply call `new Promise(fn)`. There is a complete specification for what is returned by this method in [Promises/A+](http://promises-aplus.github.com/promises-spec/).
+
+```javascript
+var Promise = require('promise');
+
+var promise = new Promise(function (resolve, reject) {
+ get('http://www.google.com', function (err, res) {
+ if (err) reject(err);
+ else resolve(res);
+ });
+});
+```
+
+If you need [domains](https://iojs.org/api/domain.html) support, you should instead use:
+
+```js
+var Promise = require('promise/domains');
+```
+
+If you are in an environment that implements `setImmediate` and don't want the optimisations provided by asap, you can use:
+
+```js
+var Promise = require('promise/setimmediate');
+```
+
+If you only want part of the features, e.g. just a pure ES6 polyfill:
+
+```js
+var Promise = require('promise/lib/es6-extensions');
+// or require('promise/domains/es6-extensions');
+// or require('promise/setimmediate/es6-extensions');
+```
+
+## Unhandled Rejections
+
+By default, promises silence any unhandled rejections.
+
+You can enable logging of unhandled ReferenceErrors and TypeErrors via:
+
+```js
+require('promise/lib/rejection-tracking').enable();
+```
+
+Due to the performance cost, you should only do this during development.
+
+You can enable logging of all unhandled rejections if you need to debug an exception you think is being swallowed by promises:
+
+```js
+require('promise/lib/rejection-tracking').enable(
+ {allRejections: true}
+);
+```
+
+Due to the high probability of false positives, I only recommend using this when debugging specific issues that you think may be being swallowed. For the preferred debugging method, see `Promise#done(onFulfilled, onRejected)`.
+
+`rejection-tracking.enable(options)` takes the following options:
+
+ - allRejections (`boolean`) - track all exceptions, not just reference errors and type errors. Note that this has a high probability of resulting in false positives if your code loads data optimisticly
+ - whitelist (`Array`) - this defaults to `[ReferenceError, TypeError]` but you can override it with your own list of error constructors to track.
+ - `onUnhandled(id, error)` and `onHandled(id, error)` - you can use these to provide your own customised display for errors. Note that if possible you should indicate that the error was a false positive if `onHandled` is called. `onHandled` is only called if `onUnhandled` has already been called.
+
+To reduce the chance of false-positives there is a delay of up to 2 seconds before errors are logged. This means that if you attach an error handler within 2 seconds, it won't be logged as a false positive. ReferenceErrors and TypeErrors are only subject to a 100ms delay due to the higher likelihood that the error is due to programmer error.
+
+## API
+
+Before all examples, you will need:
+
+```js
+var Promise = require('promise');
+```
+
+### new Promise(resolver)
+
+This creates and returns a new promise. `resolver` must be a function. The `resolver` function is passed two arguments:
+
+ 1. `resolve` should be called with a single argument. If it is called with a non-promise value then the promise is fulfilled with that value. If it is called with a promise (A) then the returned promise takes on the state of that new promise (A).
+ 2. `reject` should be called with a single argument. The returned promise will be rejected with that argument.
+
+### Static Functions
+
+ These methods are invoked by calling `Promise.methodName`.
+
+#### Promise.resolve(value)
+
+(deprecated aliases: `Promise.from(value)`, `Promise.cast(value)`)
+
+Converts values and foreign promises into Promises/A+ promises. If you pass it a value then it returns a Promise for that value. If you pass it something that is close to a promise (such as a jQuery attempt at a promise) it returns a Promise that takes on the state of `value` (rejected or fulfilled).
+
+#### Promise.reject(value)
+
+Returns a rejected promise with the given value.
+
+#### Promise.all(array)
+
+Returns a promise for an array. If it is called with a single argument that `Array.isArray` then this returns a promise for a copy of that array with any promises replaced by their fulfilled values. e.g.
+
+```js
+Promise.all([Promise.resolve('a'), 'b', Promise.resolve('c')])
+ .then(function (res) {
+ assert(res[0] === 'a')
+ assert(res[1] === 'b')
+ assert(res[2] === 'c')
+ })
+```
+
+#### Promise.denodeify(fn)
+
+_Non Standard_
+
+Takes a function which accepts a node style callback and returns a new function that returns a promise instead.
+
+e.g.
+
+```javascript
+var fs = require('fs')
+
+var read = Promise.denodeify(fs.readFile)
+var write = Promise.denodeify(fs.writeFile)
+
+var p = read('foo.json', 'utf8')
+ .then(function (str) {
+ return write('foo.json', JSON.stringify(JSON.parse(str), null, ' '), 'utf8')
+ })
+```
+
+#### Promise.nodeify(fn)
+
+_Non Standard_
+
+The twin to `denodeify` is useful when you want to export an API that can be used by people who haven't learnt about the brilliance of promises yet.
+
+```javascript
+module.exports = Promise.nodeify(awesomeAPI)
+function awesomeAPI(a, b) {
+ return download(a, b)
+}
+```
+
+If the last argument passed to `module.exports` is a function, then it will be treated like a node.js callback and not parsed on to the child function, otherwise the API will just return a promise.
+
+### Prototype Methods
+
+These methods are invoked on a promise instance by calling `myPromise.methodName`
+
+### Promise#then(onFulfilled, onRejected)
+
+This method follows the [Promises/A+ spec](http://promises-aplus.github.io/promises-spec/). It explains things very clearly so I recommend you read it.
+
+Either `onFulfilled` or `onRejected` will be called and they will not be called more than once. They will be passed a single argument and will always be called asynchronously (in the next turn of the event loop).
+
+If the promise is fulfilled then `onFulfilled` is called. If the promise is rejected then `onRejected` is called.
+
+The call to `.then` also returns a promise. If the handler that is called returns a promise, the promise returned by `.then` takes on the state of that returned promise. If the handler that is called returns a value that is not a promise, the promise returned by `.then` will be fulfilled with that value. If the handler that is called throws an exception then the promise returned by `.then` is rejected with that exception.
+
+#### Promise#catch(onRejected)
+
+Sugar for `Promise#then(null, onRejected)`, to mirror `catch` in synchronous code.
+
+#### Promise#done(onFulfilled, onRejected)
+
+_Non Standard_
+
+The same semantics as `.then` except that it does not return a promise and any exceptions are re-thrown so that they can be logged (crashing the application in non-browser environments)
+
+#### Promise#nodeify(callback)
+
+_Non Standard_
+
+If `callback` is `null` or `undefined` it just returns `this`. If `callback` is a function it is called with rejection reason as the first argument and result as the second argument (as per the node.js convention).
+
+This lets you write API functions that look like:
+
+```javascript
+function awesomeAPI(foo, bar, callback) {
+ return internalAPI(foo, bar)
+ .then(parseResult)
+ .then(null, retryErrors)
+ .nodeify(callback)
+}
+```
+
+People who use typical node.js style callbacks will be able to just pass a callback and get the expected behavior. The enlightened people can not pass a callback and will get awesome promises.
+
+## License
+
+ MIT
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/build.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/build.js
new file mode 100644
index 00000000..1e028e9a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/build.js
@@ -0,0 +1,69 @@
+'use strict';
+
+var fs = require('fs');
+var rimraf = require('rimraf');
+var acorn = require('acorn');
+var walk = require('acorn/dist/walk');
+
+var ids = [];
+var names = {};
+
+function getIdFor(name) {
+ if (name in names) return names[name];
+ var id;
+ do {
+ id = '_' + Math.floor(Math.random() * 100);
+ } while (ids.indexOf(id) !== -1)
+ ids.push(id);
+ names[name] = id;
+ return id;
+}
+
+function fixup(src) {
+ var ast = acorn.parse(src);
+ src = src.split('');
+ walk.simple(ast, {
+ MemberExpression: function (node) {
+ if (node.computed) return;
+ if (node.property.type !== 'Identifier') return;
+ if (node.property.name[0] !== '_') return;
+ replace(node.property, getIdFor(node.property.name));
+ }
+ });
+ function source(node) {
+ return src.slice(node.start, node.end).join('');
+ }
+ function replace(node, str) {
+ for (var i = node.start; i < node.end; i++) {
+ src[i] = '';
+ }
+ src[node.start] = str;
+ }
+ return src.join('');
+}
+rimraf.sync(__dirname + '/lib/');
+fs.mkdirSync(__dirname + '/lib/');
+fs.readdirSync(__dirname + '/src').forEach(function (filename) {
+ var src = fs.readFileSync(__dirname + '/src/' + filename, 'utf8');
+ var out = fixup(src);
+ fs.writeFileSync(__dirname + '/lib/' + filename, out);
+});
+
+rimraf.sync(__dirname + '/domains/');
+fs.mkdirSync(__dirname + '/domains/');
+fs.readdirSync(__dirname + '/src').forEach(function (filename) {
+ var src = fs.readFileSync(__dirname + '/src/' + filename, 'utf8');
+ var out = fixup(src);
+ out = out.replace(/require\(\'asap\/raw\'\)/g, "require('asap')");
+ fs.writeFileSync(__dirname + '/domains/' + filename, out);
+});
+
+rimraf.sync(__dirname + '/setimmediate/');
+fs.mkdirSync(__dirname + '/setimmediate/');
+fs.readdirSync(__dirname + '/src').forEach(function (filename) {
+ var src = fs.readFileSync(__dirname + '/src/' + filename, 'utf8');
+ var out = fixup(src);
+ out = out.replace(/var asap \= require\(\'([a-z\/]+)\'\);/g, '');
+ out = out.replace(/asap/g, "setImmediate");
+ fs.writeFileSync(__dirname + '/setimmediate/' + filename, out);
+});
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/core.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/core.js
new file mode 100644
index 00000000..5f332a20
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/core.js
@@ -0,0 +1,5 @@
+'use strict';
+
+module.exports = require('./lib/core.js');
+
+console.error('require("promise/core") is deprecated, use require("promise/lib/core") instead.');
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/core.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/core.js
new file mode 100644
index 00000000..aea44148
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/core.js
@@ -0,0 +1,213 @@
+'use strict';
+
+var asap = require('asap');
+
+function noop() {}
+
+// States:
+//
+// 0 - pending
+// 1 - fulfilled with _value
+// 2 - rejected with _value
+// 3 - adopted the state of another promise, _value
+//
+// once the state is no longer pending (0) it is immutable
+
+// All `_` prefixed properties will be reduced to `_{random number}`
+// at build time to obfuscate them and discourage their use.
+// We don't use symbols or Object.defineProperty to fully hide them
+// because the performance isn't good enough.
+
+
+// to avoid using try/catch inside critical functions, we
+// extract them to here.
+var LAST_ERROR = null;
+var IS_ERROR = {};
+function getThen(obj) {
+ try {
+ return obj.then;
+ } catch (ex) {
+ LAST_ERROR = ex;
+ return IS_ERROR;
+ }
+}
+
+function tryCallOne(fn, a) {
+ try {
+ return fn(a);
+ } catch (ex) {
+ LAST_ERROR = ex;
+ return IS_ERROR;
+ }
+}
+function tryCallTwo(fn, a, b) {
+ try {
+ fn(a, b);
+ } catch (ex) {
+ LAST_ERROR = ex;
+ return IS_ERROR;
+ }
+}
+
+module.exports = Promise;
+
+function Promise(fn) {
+ if (typeof this !== 'object') {
+ throw new TypeError('Promises must be constructed via new');
+ }
+ if (typeof fn !== 'function') {
+ throw new TypeError('Promise constructor\'s argument is not a function');
+ }
+ this._40 = 0;
+ this._65 = 0;
+ this._55 = null;
+ this._72 = null;
+ if (fn === noop) return;
+ doResolve(fn, this);
+}
+Promise._37 = null;
+Promise._87 = null;
+Promise._61 = noop;
+
+Promise.prototype.then = function(onFulfilled, onRejected) {
+ if (this.constructor !== Promise) {
+ return safeThen(this, onFulfilled, onRejected);
+ }
+ var res = new Promise(noop);
+ handle(this, new Handler(onFulfilled, onRejected, res));
+ return res;
+};
+
+function safeThen(self, onFulfilled, onRejected) {
+ return new self.constructor(function (resolve, reject) {
+ var res = new Promise(noop);
+ res.then(resolve, reject);
+ handle(self, new Handler(onFulfilled, onRejected, res));
+ });
+}
+function handle(self, deferred) {
+ while (self._65 === 3) {
+ self = self._55;
+ }
+ if (Promise._37) {
+ Promise._37(self);
+ }
+ if (self._65 === 0) {
+ if (self._40 === 0) {
+ self._40 = 1;
+ self._72 = deferred;
+ return;
+ }
+ if (self._40 === 1) {
+ self._40 = 2;
+ self._72 = [self._72, deferred];
+ return;
+ }
+ self._72.push(deferred);
+ return;
+ }
+ handleResolved(self, deferred);
+}
+
+function handleResolved(self, deferred) {
+ asap(function() {
+ var cb = self._65 === 1 ? deferred.onFulfilled : deferred.onRejected;
+ if (cb === null) {
+ if (self._65 === 1) {
+ resolve(deferred.promise, self._55);
+ } else {
+ reject(deferred.promise, self._55);
+ }
+ return;
+ }
+ var ret = tryCallOne(cb, self._55);
+ if (ret === IS_ERROR) {
+ reject(deferred.promise, LAST_ERROR);
+ } else {
+ resolve(deferred.promise, ret);
+ }
+ });
+}
+function resolve(self, newValue) {
+ // Promise Resolution Procedure: https://github.com/promises-aplus/promises-spec#the-promise-resolution-procedure
+ if (newValue === self) {
+ return reject(
+ self,
+ new TypeError('A promise cannot be resolved with itself.')
+ );
+ }
+ if (
+ newValue &&
+ (typeof newValue === 'object' || typeof newValue === 'function')
+ ) {
+ var then = getThen(newValue);
+ if (then === IS_ERROR) {
+ return reject(self, LAST_ERROR);
+ }
+ if (
+ then === self.then &&
+ newValue instanceof Promise
+ ) {
+ self._65 = 3;
+ self._55 = newValue;
+ finale(self);
+ return;
+ } else if (typeof then === 'function') {
+ doResolve(then.bind(newValue), self);
+ return;
+ }
+ }
+ self._65 = 1;
+ self._55 = newValue;
+ finale(self);
+}
+
+function reject(self, newValue) {
+ self._65 = 2;
+ self._55 = newValue;
+ if (Promise._87) {
+ Promise._87(self, newValue);
+ }
+ finale(self);
+}
+function finale(self) {
+ if (self._40 === 1) {
+ handle(self, self._72);
+ self._72 = null;
+ }
+ if (self._40 === 2) {
+ for (var i = 0; i < self._72.length; i++) {
+ handle(self, self._72[i]);
+ }
+ self._72 = null;
+ }
+}
+
+function Handler(onFulfilled, onRejected, promise){
+ this.onFulfilled = typeof onFulfilled === 'function' ? onFulfilled : null;
+ this.onRejected = typeof onRejected === 'function' ? onRejected : null;
+ this.promise = promise;
+}
+
+/**
+ * Take a potentially misbehaving resolver function and make sure
+ * onFulfilled and onRejected are only called once.
+ *
+ * Makes no guarantees about asynchrony.
+ */
+function doResolve(fn, promise) {
+ var done = false;
+ var res = tryCallTwo(fn, function (value) {
+ if (done) return;
+ done = true;
+ resolve(promise, value);
+ }, function (reason) {
+ if (done) return;
+ done = true;
+ reject(promise, reason);
+ });
+ if (!done && res === IS_ERROR) {
+ done = true;
+ reject(promise, LAST_ERROR);
+ }
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/done.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/done.js
new file mode 100644
index 00000000..f879317d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/done.js
@@ -0,0 +1,13 @@
+'use strict';
+
+var Promise = require('./core.js');
+
+module.exports = Promise;
+Promise.prototype.done = function (onFulfilled, onRejected) {
+ var self = arguments.length ? this.then.apply(this, arguments) : this;
+ self.then(null, function (err) {
+ setTimeout(function () {
+ throw err;
+ }, 0);
+ });
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/es6-extensions.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/es6-extensions.js
new file mode 100644
index 00000000..8ab26669
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/es6-extensions.js
@@ -0,0 +1,107 @@
+'use strict';
+
+//This file contains the ES6 extensions to the core Promises/A+ API
+
+var Promise = require('./core.js');
+
+module.exports = Promise;
+
+/* Static Functions */
+
+var TRUE = valuePromise(true);
+var FALSE = valuePromise(false);
+var NULL = valuePromise(null);
+var UNDEFINED = valuePromise(undefined);
+var ZERO = valuePromise(0);
+var EMPTYSTRING = valuePromise('');
+
+function valuePromise(value) {
+ var p = new Promise(Promise._61);
+ p._65 = 1;
+ p._55 = value;
+ return p;
+}
+Promise.resolve = function (value) {
+ if (value instanceof Promise) return value;
+
+ if (value === null) return NULL;
+ if (value === undefined) return UNDEFINED;
+ if (value === true) return TRUE;
+ if (value === false) return FALSE;
+ if (value === 0) return ZERO;
+ if (value === '') return EMPTYSTRING;
+
+ if (typeof value === 'object' || typeof value === 'function') {
+ try {
+ var then = value.then;
+ if (typeof then === 'function') {
+ return new Promise(then.bind(value));
+ }
+ } catch (ex) {
+ return new Promise(function (resolve, reject) {
+ reject(ex);
+ });
+ }
+ }
+ return valuePromise(value);
+};
+
+Promise.all = function (arr) {
+ var args = Array.prototype.slice.call(arr);
+
+ return new Promise(function (resolve, reject) {
+ if (args.length === 0) return resolve([]);
+ var remaining = args.length;
+ function res(i, val) {
+ if (val && (typeof val === 'object' || typeof val === 'function')) {
+ if (val instanceof Promise && val.then === Promise.prototype.then) {
+ while (val._65 === 3) {
+ val = val._55;
+ }
+ if (val._65 === 1) return res(i, val._55);
+ if (val._65 === 2) reject(val._55);
+ val.then(function (val) {
+ res(i, val);
+ }, reject);
+ return;
+ } else {
+ var then = val.then;
+ if (typeof then === 'function') {
+ var p = new Promise(then.bind(val));
+ p.then(function (val) {
+ res(i, val);
+ }, reject);
+ return;
+ }
+ }
+ }
+ args[i] = val;
+ if (--remaining === 0) {
+ resolve(args);
+ }
+ }
+ for (var i = 0; i < args.length; i++) {
+ res(i, args[i]);
+ }
+ });
+};
+
+Promise.reject = function (value) {
+ return new Promise(function (resolve, reject) {
+ reject(value);
+ });
+};
+
+Promise.race = function (values) {
+ return new Promise(function (resolve, reject) {
+ values.forEach(function(value){
+ Promise.resolve(value).then(resolve, reject);
+ });
+ });
+};
+
+/* Prototype Methods */
+
+Promise.prototype['catch'] = function (onRejected) {
+ return this.then(null, onRejected);
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/finally.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/finally.js
new file mode 100644
index 00000000..f5ee0b98
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/finally.js
@@ -0,0 +1,16 @@
+'use strict';
+
+var Promise = require('./core.js');
+
+module.exports = Promise;
+Promise.prototype['finally'] = function (f) {
+ return this.then(function (value) {
+ return Promise.resolve(f()).then(function () {
+ return value;
+ });
+ }, function (err) {
+ return Promise.resolve(f()).then(function () {
+ throw err;
+ });
+ });
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/index.js
new file mode 100644
index 00000000..6e674f38
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/index.js
@@ -0,0 +1,8 @@
+'use strict';
+
+module.exports = require('./core.js');
+require('./done.js');
+require('./finally.js');
+require('./es6-extensions.js');
+require('./node-extensions.js');
+require('./synchronous.js');
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/node-extensions.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/node-extensions.js
new file mode 100644
index 00000000..157cddc2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/node-extensions.js
@@ -0,0 +1,130 @@
+'use strict';
+
+// This file contains then/promise specific extensions that are only useful
+// for node.js interop
+
+var Promise = require('./core.js');
+var asap = require('asap');
+
+module.exports = Promise;
+
+/* Static Functions */
+
+Promise.denodeify = function (fn, argumentCount) {
+ if (
+ typeof argumentCount === 'number' && argumentCount !== Infinity
+ ) {
+ return denodeifyWithCount(fn, argumentCount);
+ } else {
+ return denodeifyWithoutCount(fn);
+ }
+};
+
+var callbackFn = (
+ 'function (err, res) {' +
+ 'if (err) { rj(err); } else { rs(res); }' +
+ '}'
+);
+function denodeifyWithCount(fn, argumentCount) {
+ var args = [];
+ for (var i = 0; i < argumentCount; i++) {
+ args.push('a' + i);
+ }
+ var body = [
+ 'return function (' + args.join(',') + ') {',
+ 'var self = this;',
+ 'return new Promise(function (rs, rj) {',
+ 'var res = fn.call(',
+ ['self'].concat(args).concat([callbackFn]).join(','),
+ ');',
+ 'if (res &&',
+ '(typeof res === "object" || typeof res === "function") &&',
+ 'typeof res.then === "function"',
+ ') {rs(res);}',
+ '});',
+ '};'
+ ].join('');
+ return Function(['Promise', 'fn'], body)(Promise, fn);
+}
+function denodeifyWithoutCount(fn) {
+ var fnLength = Math.max(fn.length - 1, 3);
+ var args = [];
+ for (var i = 0; i < fnLength; i++) {
+ args.push('a' + i);
+ }
+ var body = [
+ 'return function (' + args.join(',') + ') {',
+ 'var self = this;',
+ 'var args;',
+ 'var argLength = arguments.length;',
+ 'if (arguments.length > ' + fnLength + ') {',
+ 'args = new Array(arguments.length + 1);',
+ 'for (var i = 0; i < arguments.length; i++) {',
+ 'args[i] = arguments[i];',
+ '}',
+ '}',
+ 'return new Promise(function (rs, rj) {',
+ 'var cb = ' + callbackFn + ';',
+ 'var res;',
+ 'switch (argLength) {',
+ args.concat(['extra']).map(function (_, index) {
+ return (
+ 'case ' + (index) + ':' +
+ 'res = fn.call(' + ['self'].concat(args.slice(0, index)).concat('cb').join(',') + ');' +
+ 'break;'
+ );
+ }).join(''),
+ 'default:',
+ 'args[argLength] = cb;',
+ 'res = fn.apply(self, args);',
+ '}',
+
+ 'if (res &&',
+ '(typeof res === "object" || typeof res === "function") &&',
+ 'typeof res.then === "function"',
+ ') {rs(res);}',
+ '});',
+ '};'
+ ].join('');
+
+ return Function(
+ ['Promise', 'fn'],
+ body
+ )(Promise, fn);
+}
+
+Promise.nodeify = function (fn) {
+ return function () {
+ var args = Array.prototype.slice.call(arguments);
+ var callback =
+ typeof args[args.length - 1] === 'function' ? args.pop() : null;
+ var ctx = this;
+ try {
+ return fn.apply(this, arguments).nodeify(callback, ctx);
+ } catch (ex) {
+ if (callback === null || typeof callback == 'undefined') {
+ return new Promise(function (resolve, reject) {
+ reject(ex);
+ });
+ } else {
+ asap(function () {
+ callback.call(ctx, ex);
+ })
+ }
+ }
+ }
+};
+
+Promise.prototype.nodeify = function (callback, ctx) {
+ if (typeof callback != 'function') return this;
+
+ this.then(function (value) {
+ asap(function () {
+ callback.call(ctx, null, value);
+ });
+ }, function (err) {
+ asap(function () {
+ callback.call(ctx, err);
+ });
+ });
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/rejection-tracking.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/rejection-tracking.js
new file mode 100644
index 00000000..10ccce30
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/rejection-tracking.js
@@ -0,0 +1,113 @@
+'use strict';
+
+var Promise = require('./core');
+
+var DEFAULT_WHITELIST = [
+ ReferenceError,
+ TypeError,
+ RangeError
+];
+
+var enabled = false;
+exports.disable = disable;
+function disable() {
+ enabled = false;
+ Promise._37 = null;
+ Promise._87 = null;
+}
+
+exports.enable = enable;
+function enable(options) {
+ options = options || {};
+ if (enabled) disable();
+ enabled = true;
+ var id = 0;
+ var displayId = 0;
+ var rejections = {};
+ Promise._37 = function (promise) {
+ if (
+ promise._65 === 2 && // IS REJECTED
+ rejections[promise._51]
+ ) {
+ if (rejections[promise._51].logged) {
+ onHandled(promise._51);
+ } else {
+ clearTimeout(rejections[promise._51].timeout);
+ }
+ delete rejections[promise._51];
+ }
+ };
+ Promise._87 = function (promise, err) {
+ if (promise._40 === 0) { // not yet handled
+ promise._51 = id++;
+ rejections[promise._51] = {
+ displayId: null,
+ error: err,
+ timeout: setTimeout(
+ onUnhandled.bind(null, promise._51),
+ // For reference errors and type errors, this almost always
+ // means the programmer made a mistake, so log them after just
+ // 100ms
+ // otherwise, wait 2 seconds to see if they get handled
+ matchWhitelist(err, DEFAULT_WHITELIST)
+ ? 100
+ : 2000
+ ),
+ logged: false
+ };
+ }
+ };
+ function onUnhandled(id) {
+ if (
+ options.allRejections ||
+ matchWhitelist(
+ rejections[id].error,
+ options.whitelist || DEFAULT_WHITELIST
+ )
+ ) {
+ rejections[id].displayId = displayId++;
+ if (options.onUnhandled) {
+ rejections[id].logged = true;
+ options.onUnhandled(
+ rejections[id].displayId,
+ rejections[id].error
+ );
+ } else {
+ rejections[id].logged = true;
+ logError(
+ rejections[id].displayId,
+ rejections[id].error
+ );
+ }
+ }
+ }
+ function onHandled(id) {
+ if (rejections[id].logged) {
+ if (options.onHandled) {
+ options.onHandled(rejections[id].displayId, rejections[id].error);
+ } else if (!rejections[id].onUnhandled) {
+ console.warn(
+ 'Promise Rejection Handled (id: ' + rejections[id].displayId + '):'
+ );
+ console.warn(
+ ' This means you can ignore any previous messages of the form "Possible Unhandled Promise Rejection" with id ' +
+ rejections[id].displayId + '.'
+ );
+ }
+ }
+ }
+}
+
+function logError(id, error) {
+ console.warn('Possible Unhandled Promise Rejection (id: ' + id + '):');
+ var errStr = (error && (error.stack || error)) + '';
+ errStr.split('\n').forEach(function (line) {
+ console.warn(' ' + line);
+ });
+}
+
+function matchWhitelist(error, list) {
+ return list.some(function (cls) {
+ return error instanceof cls;
+ });
+}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/synchronous.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/synchronous.js
new file mode 100644
index 00000000..49399644
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/domains/synchronous.js
@@ -0,0 +1,62 @@
+'use strict';
+
+var Promise = require('./core.js');
+
+module.exports = Promise;
+Promise.enableSynchronous = function () {
+ Promise.prototype.isPending = function() {
+ return this.getState() == 0;
+ };
+
+ Promise.prototype.isFulfilled = function() {
+ return this.getState() == 1;
+ };
+
+ Promise.prototype.isRejected = function() {
+ return this.getState() == 2;
+ };
+
+ Promise.prototype.getValue = function () {
+ if (this._65 === 3) {
+ return this._55.getValue();
+ }
+
+ if (!this.isFulfilled()) {
+ throw new Error('Cannot get a value of an unfulfilled promise.');
+ }
+
+ return this._55;
+ };
+
+ Promise.prototype.getReason = function () {
+ if (this._65 === 3) {
+ return this._55.getReason();
+ }
+
+ if (!this.isRejected()) {
+ throw new Error('Cannot get a rejection reason of a non-rejected promise.');
+ }
+
+ return this._55;
+ };
+
+ Promise.prototype.getState = function () {
+ if (this._65 === 3) {
+ return this._55.getState();
+ }
+ if (this._65 === -1 || this._65 === -2) {
+ return 0;
+ }
+
+ return this._65;
+ };
+};
+
+Promise.disableSynchronous = function() {
+ Promise.prototype.isPending = undefined;
+ Promise.prototype.isFulfilled = undefined;
+ Promise.prototype.isRejected = undefined;
+ Promise.prototype.getValue = undefined;
+ Promise.prototype.getReason = undefined;
+ Promise.prototype.getState = undefined;
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/index.d.ts b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/index.d.ts
new file mode 100644
index 00000000..a199cbc9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/index.d.ts
@@ -0,0 +1,256 @@
+interface Thenable {
+ /**
+ * Attaches callbacks for the resolution and/or rejection of the ThenPromise.
+ * @param onfulfilled The callback to execute when the ThenPromise is resolved.
+ * @param onrejected The callback to execute when the ThenPromise is rejected.
+ * @returns A ThenPromise for the completion of which ever callback is executed.
+ */
+ then(onfulfilled?: ((value: T) => TResult1 | Thenable) | undefined | null, onrejected?: ((reason: any) => TResult2 | Thenable) | undefined | null): Thenable;
+}
+
+/**
+ * Represents the completion of an asynchronous operation
+ */
+interface ThenPromise {
+ /**
+ * Attaches callbacks for the resolution and/or rejection of the ThenPromise.
+ * @param onfulfilled The callback to execute when the ThenPromise is resolved.
+ * @param onrejected The callback to execute when the ThenPromise is rejected.
+ * @returns A ThenPromise for the completion of which ever callback is executed.
+ */
+ then(onfulfilled?: ((value: T) => TResult1 | Thenable) | undefined | null, onrejected?: ((reason: any) => TResult2 | Thenable) | undefined | null): ThenPromise;
+
+ /**
+ * Attaches a callback for only the rejection of the ThenPromise.
+ * @param onrejected The callback to execute when the ThenPromise is rejected.
+ * @returns A ThenPromise for the completion of the callback.
+ */
+ catch(onrejected?: ((reason: any) => TResult | Thenable) | undefined | null): ThenPromise;
+
+ // Extensions specific to then/promise
+
+ /**
+ * Attaches callbacks for the resolution and/or rejection of the ThenPromise, without returning a new promise.
+ * @param onfulfilled The callback to execute when the ThenPromise is resolved.
+ * @param onrejected The callback to execute when the ThenPromise is rejected.
+ */
+ done(onfulfilled?: ((value: T) => any) | undefined | null, onrejected?: ((reason: any) => any) | undefined | null): void;
+
+
+ /**
+ * Calls a node.js style callback. If none is provided, the promise is returned.
+ */
+ nodeify(callback: void | null): ThenPromise;
+ nodeify(callback: (err: Error, value: T) => void): void;
+}
+
+interface ThenPromiseConstructor {
+ /**
+ * A reference to the prototype.
+ */
+ readonly prototype: ThenPromise;
+
+ /**
+ * Creates a new ThenPromise.
+ * @param executor A callback used to initialize the promise. This callback is passed two arguments:
+ * a resolve callback used resolve the promise with a value or the result of another promise,
+ * and a reject callback used to reject the promise with a provided reason or error.
+ */
+ new (executor: (resolve: (value?: T | Thenable) => void, reject: (reason?: any) => void) => any): ThenPromise;
+
+ /**
+ * Creates a ThenPromise that is resolved with an array of results when all of the provided Promises
+ * resolve, or rejected when any ThenPromise is rejected.
+ * @param values An array of Promises.
+ * @returns A new ThenPromise.
+ */
+ all(values: [T1 | Thenable, T2 | Thenable, T3 | Thenable, T4 | Thenable , T5 | Thenable, T6 | Thenable, T7 | Thenable, T8 | Thenable, T9 | Thenable, T10 | Thenable]): ThenPromise<[T1, T2, T3, T4, T5, T6, T7, T8, T9, T10]>;
+
+ /**
+ * Creates a ThenPromise that is resolved with an array of results when all of the provided Promises
+ * resolve, or rejected when any ThenPromise is rejected.
+ * @param values An array of Promises.
+ * @returns A new ThenPromise.
+ */
+ all(values: [T1 | Thenable, T2 | Thenable, T3 | Thenable, T4 | Thenable , T5 | Thenable, T6 | Thenable, T7 | Thenable, T8 | Thenable, T9 | Thenable]): ThenPromise<[T1, T2, T3, T4, T5, T6, T7, T8, T9]>;
+
+ /**
+ * Creates a ThenPromise that is resolved with an array of results when all of the provided Promises
+ * resolve, or rejected when any ThenPromise is rejected.
+ * @param values An array of Promises.
+ * @returns A new ThenPromise.
+ */
+ all(values: [T1 | Thenable, T2 | Thenable, T3 | Thenable, T4 | Thenable , T5 | Thenable, T6 | Thenable, T7 | Thenable, T8 | Thenable]): ThenPromise<[T1, T2, T3, T4, T5, T6, T7, T8]>;
+
+ /**
+ * Creates a ThenPromise that is resolved with an array of results when all of the provided Promises
+ * resolve, or rejected when any ThenPromise is rejected.
+ * @param values An array of Promises.
+ * @returns A new ThenPromise.
+ */
+ all(values: [T1 | Thenable, T2 | Thenable, T3 | Thenable, T4 | Thenable , T5 | Thenable, T6 | Thenable, T7 | Thenable]): ThenPromise<[T1, T2, T3, T4, T5, T6, T7]>;
+
+ /**
+ * Creates a ThenPromise that is resolved with an array of results when all of the provided Promises
+ * resolve, or rejected when any ThenPromise is rejected.
+ * @param values An array of Promises.
+ * @returns A new ThenPromise.
+ */
+ all(values: [T1 | Thenable, T2 | Thenable, T3 | Thenable, T4 | Thenable , T5 | Thenable, T6 | Thenable]): ThenPromise<[T1, T2, T3, T4, T5, T6]>;
+
+ /**
+ * Creates a ThenPromise that is resolved with an array of results when all of the provided Promises
+ * resolve, or rejected when any ThenPromise is rejected.
+ * @param values An array of Promises.
+ * @returns A new ThenPromise.
+ */
+ all(values: [T1 | Thenable, T2 | Thenable, T3 | Thenable, T4 | Thenable , T5 | Thenable]): ThenPromise<[T1, T2, T3, T4, T5]>;
+
+ /**
+ * Creates a ThenPromise that is resolved with an array of results when all of the provided Promises
+ * resolve, or rejected when any ThenPromise is rejected.
+ * @param values An array of Promises.
+ * @returns A new ThenPromise.
+ */
+ all(values: [T1 | Thenable, T2 | Thenable, T3 | Thenable, T4 | Thenable ]): ThenPromise<[T1, T2, T3, T4]>;
+
+ /**
+ * Creates a ThenPromise that is resolved with an array of results when all of the provided Promises
+ * resolve, or rejected when any ThenPromise is rejected.
+ * @param values An array of Promises.
+ * @returns A new ThenPromise.
+ */
+ all(values: [T1 | Thenable, T2 | Thenable, T3 | Thenable]): ThenPromise<[T1, T2, T3]>;
+
+ /**
+ * Creates a ThenPromise that is resolved with an array of results when all of the provided Promises
+ * resolve, or rejected when any ThenPromise is rejected.
+ * @param values An array of Promises.
+ * @returns A new ThenPromise.
+ */
+ all(values: [T1 | Thenable, T2 | Thenable]): ThenPromise<[T1, T2]>;
+
+ /**
+ * Creates a ThenPromise that is resolved with an array of results when all of the provided Promises
+ * resolve, or rejected when any ThenPromise is rejected.
+ * @param values An array of Promises.
+ * @returns A new ThenPromise.
+ */
+ all(values: (T | Thenable)[]): ThenPromise;
+
+ /**
+ * Creates a ThenPromise that is resolved or rejected when any of the provided Promises are resolved
+ * or rejected.
+ * @param values An array of Promises.
+ * @returns A new ThenPromise.
+ */
+ race(values: [T1 | Thenable, T2 | Thenable, T3 | Thenable, T4 | Thenable, T5 | Thenable, T6 | Thenable, T7 | Thenable, T8 | Thenable, T9 | Thenable, T10 | Thenable]): ThenPromise;
+
+ /**
+ * Creates a ThenPromise that is resolved or rejected when any of the provided Promises are resolved
+ * or rejected.
+ * @param values An array of Promises.
+ * @returns A new ThenPromise.
+ */
+ race(values: [T1 | Thenable, T2 | Thenable, T3 | Thenable, T4 | Thenable, T5 | Thenable, T6 | Thenable, T7 | Thenable, T8 | Thenable, T9 | Thenable]): ThenPromise;
+
+ /**
+ * Creates a ThenPromise that is resolved or rejected when any of the provided Promises are resolved
+ * or rejected.
+ * @param values An array of Promises.
+ * @returns A new ThenPromise.
+ */
+ race(values: [T1 | Thenable, T2 | Thenable, T3 | Thenable, T4 | Thenable, T5 | Thenable, T6 | Thenable, T7 | Thenable, T8 | Thenable]): ThenPromise;
+
+ /**
+ * Creates a ThenPromise that is resolved or rejected when any of the provided Promises are resolved
+ * or rejected.
+ * @param values An array of Promises.
+ * @returns A new ThenPromise.
+ */
+ race(values: [T1 | Thenable, T2 | Thenable, T3 | Thenable, T4 | Thenable, T5 | Thenable, T6 | Thenable, T7 | Thenable]): ThenPromise;
+
+ /**
+ * Creates a ThenPromise that is resolved or rejected when any of the provided Promises are resolved
+ * or rejected.
+ * @param values An array of Promises.
+ * @returns A new ThenPromise.
+ */
+ race(values: [T1 | Thenable, T2 | Thenable, T3 | Thenable, T4 | Thenable, T5 | Thenable, T6 | Thenable]): ThenPromise;
+
+ /**
+ * Creates a ThenPromise that is resolved or rejected when any of the provided Promises are resolved
+ * or rejected.
+ * @param values An array of Promises.
+ * @returns A new ThenPromise.
+ */
+ race(values: [T1 | Thenable, T2 | Thenable, T3 | Thenable, T4 | Thenable, T5 | Thenable]): ThenPromise;
+
+ /**
+ * Creates a ThenPromise that is resolved or rejected when any of the provided Promises are resolved
+ * or rejected.
+ * @param values An array of Promises.
+ * @returns A new ThenPromise.
+ */
+ race(values: [T1 | Thenable, T2 | Thenable, T3 | Thenable, T4 | Thenable]): ThenPromise;
+
+ /**
+ * Creates a ThenPromise that is resolved or rejected when any of the provided Promises are resolved
+ * or rejected.
+ * @param values An array of Promises.
+ * @returns A new ThenPromise.
+ */
+ race(values: [T1 | Thenable, T2 | Thenable, T3 | Thenable]): ThenPromise;
+
+ /**
+ * Creates a ThenPromise that is resolved or rejected when any of the provided Promises are resolved
+ * or rejected.
+ * @param values An array of Promises.
+ * @returns A new ThenPromise.
+ */
+ race(values: [T1 | Thenable, T2 | Thenable]): ThenPromise;
+
+ /**
+ * Creates a ThenPromise that is resolved or rejected when any of the provided Promises are resolved
+ * or rejected.
+ * @param values An array of Promises.
+ * @returns A new ThenPromise.
+ */
+ race(values: (T | Thenable)[]): ThenPromise;
+
+ /**
+ * Creates a new rejected promise for the provided reason.
+ * @param reason The reason the promise was rejected.
+ * @returns A new rejected ThenPromise.
+ */
+ reject(reason: any): ThenPromise;
+
+ /**
+ * Creates a new rejected promise for the provided reason.
+ * @param reason The reason the promise was rejected.
+ * @returns A new rejected ThenPromise.
+ */
+ reject(reason: any): ThenPromise;
+
+ /**
+ * Creates a new resolved promise for the provided value.
+ * @param value A promise.
+ * @returns A promise whose internal state matches the provided promise.
+ */
+ resolve(value: T | Thenable): ThenPromise;
+
+ /**
+ * Creates a new resolved promise .
+ * @returns A resolved promise.
+ */
+ resolve(): ThenPromise;
+
+ // Extensions specific to then/promise
+
+ denodeify: (fn: Function) => (...args: any[]) => ThenPromise;
+ nodeify: (fn: Function) => Function;
+}
+
+declare var ThenPromise: ThenPromiseConstructor;
+
+export = ThenPromise;
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/index.js
new file mode 100644
index 00000000..1c38e467
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/index.js
@@ -0,0 +1,3 @@
+'use strict';
+
+module.exports = require('./lib')
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/core.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/core.js
new file mode 100644
index 00000000..aefc987b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/core.js
@@ -0,0 +1,213 @@
+'use strict';
+
+var asap = require('asap/raw');
+
+function noop() {}
+
+// States:
+//
+// 0 - pending
+// 1 - fulfilled with _value
+// 2 - rejected with _value
+// 3 - adopted the state of another promise, _value
+//
+// once the state is no longer pending (0) it is immutable
+
+// All `_` prefixed properties will be reduced to `_{random number}`
+// at build time to obfuscate them and discourage their use.
+// We don't use symbols or Object.defineProperty to fully hide them
+// because the performance isn't good enough.
+
+
+// to avoid using try/catch inside critical functions, we
+// extract them to here.
+var LAST_ERROR = null;
+var IS_ERROR = {};
+function getThen(obj) {
+ try {
+ return obj.then;
+ } catch (ex) {
+ LAST_ERROR = ex;
+ return IS_ERROR;
+ }
+}
+
+function tryCallOne(fn, a) {
+ try {
+ return fn(a);
+ } catch (ex) {
+ LAST_ERROR = ex;
+ return IS_ERROR;
+ }
+}
+function tryCallTwo(fn, a, b) {
+ try {
+ fn(a, b);
+ } catch (ex) {
+ LAST_ERROR = ex;
+ return IS_ERROR;
+ }
+}
+
+module.exports = Promise;
+
+function Promise(fn) {
+ if (typeof this !== 'object') {
+ throw new TypeError('Promises must be constructed via new');
+ }
+ if (typeof fn !== 'function') {
+ throw new TypeError('Promise constructor\'s argument is not a function');
+ }
+ this._40 = 0;
+ this._65 = 0;
+ this._55 = null;
+ this._72 = null;
+ if (fn === noop) return;
+ doResolve(fn, this);
+}
+Promise._37 = null;
+Promise._87 = null;
+Promise._61 = noop;
+
+Promise.prototype.then = function(onFulfilled, onRejected) {
+ if (this.constructor !== Promise) {
+ return safeThen(this, onFulfilled, onRejected);
+ }
+ var res = new Promise(noop);
+ handle(this, new Handler(onFulfilled, onRejected, res));
+ return res;
+};
+
+function safeThen(self, onFulfilled, onRejected) {
+ return new self.constructor(function (resolve, reject) {
+ var res = new Promise(noop);
+ res.then(resolve, reject);
+ handle(self, new Handler(onFulfilled, onRejected, res));
+ });
+}
+function handle(self, deferred) {
+ while (self._65 === 3) {
+ self = self._55;
+ }
+ if (Promise._37) {
+ Promise._37(self);
+ }
+ if (self._65 === 0) {
+ if (self._40 === 0) {
+ self._40 = 1;
+ self._72 = deferred;
+ return;
+ }
+ if (self._40 === 1) {
+ self._40 = 2;
+ self._72 = [self._72, deferred];
+ return;
+ }
+ self._72.push(deferred);
+ return;
+ }
+ handleResolved(self, deferred);
+}
+
+function handleResolved(self, deferred) {
+ asap(function() {
+ var cb = self._65 === 1 ? deferred.onFulfilled : deferred.onRejected;
+ if (cb === null) {
+ if (self._65 === 1) {
+ resolve(deferred.promise, self._55);
+ } else {
+ reject(deferred.promise, self._55);
+ }
+ return;
+ }
+ var ret = tryCallOne(cb, self._55);
+ if (ret === IS_ERROR) {
+ reject(deferred.promise, LAST_ERROR);
+ } else {
+ resolve(deferred.promise, ret);
+ }
+ });
+}
+function resolve(self, newValue) {
+ // Promise Resolution Procedure: https://github.com/promises-aplus/promises-spec#the-promise-resolution-procedure
+ if (newValue === self) {
+ return reject(
+ self,
+ new TypeError('A promise cannot be resolved with itself.')
+ );
+ }
+ if (
+ newValue &&
+ (typeof newValue === 'object' || typeof newValue === 'function')
+ ) {
+ var then = getThen(newValue);
+ if (then === IS_ERROR) {
+ return reject(self, LAST_ERROR);
+ }
+ if (
+ then === self.then &&
+ newValue instanceof Promise
+ ) {
+ self._65 = 3;
+ self._55 = newValue;
+ finale(self);
+ return;
+ } else if (typeof then === 'function') {
+ doResolve(then.bind(newValue), self);
+ return;
+ }
+ }
+ self._65 = 1;
+ self._55 = newValue;
+ finale(self);
+}
+
+function reject(self, newValue) {
+ self._65 = 2;
+ self._55 = newValue;
+ if (Promise._87) {
+ Promise._87(self, newValue);
+ }
+ finale(self);
+}
+function finale(self) {
+ if (self._40 === 1) {
+ handle(self, self._72);
+ self._72 = null;
+ }
+ if (self._40 === 2) {
+ for (var i = 0; i < self._72.length; i++) {
+ handle(self, self._72[i]);
+ }
+ self._72 = null;
+ }
+}
+
+function Handler(onFulfilled, onRejected, promise){
+ this.onFulfilled = typeof onFulfilled === 'function' ? onFulfilled : null;
+ this.onRejected = typeof onRejected === 'function' ? onRejected : null;
+ this.promise = promise;
+}
+
+/**
+ * Take a potentially misbehaving resolver function and make sure
+ * onFulfilled and onRejected are only called once.
+ *
+ * Makes no guarantees about asynchrony.
+ */
+function doResolve(fn, promise) {
+ var done = false;
+ var res = tryCallTwo(fn, function (value) {
+ if (done) return;
+ done = true;
+ resolve(promise, value);
+ }, function (reason) {
+ if (done) return;
+ done = true;
+ reject(promise, reason);
+ });
+ if (!done && res === IS_ERROR) {
+ done = true;
+ reject(promise, LAST_ERROR);
+ }
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/done.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/done.js
new file mode 100644
index 00000000..f879317d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/done.js
@@ -0,0 +1,13 @@
+'use strict';
+
+var Promise = require('./core.js');
+
+module.exports = Promise;
+Promise.prototype.done = function (onFulfilled, onRejected) {
+ var self = arguments.length ? this.then.apply(this, arguments) : this;
+ self.then(null, function (err) {
+ setTimeout(function () {
+ throw err;
+ }, 0);
+ });
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/es6-extensions.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/es6-extensions.js
new file mode 100644
index 00000000..8ab26669
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/es6-extensions.js
@@ -0,0 +1,107 @@
+'use strict';
+
+//This file contains the ES6 extensions to the core Promises/A+ API
+
+var Promise = require('./core.js');
+
+module.exports = Promise;
+
+/* Static Functions */
+
+var TRUE = valuePromise(true);
+var FALSE = valuePromise(false);
+var NULL = valuePromise(null);
+var UNDEFINED = valuePromise(undefined);
+var ZERO = valuePromise(0);
+var EMPTYSTRING = valuePromise('');
+
+function valuePromise(value) {
+ var p = new Promise(Promise._61);
+ p._65 = 1;
+ p._55 = value;
+ return p;
+}
+Promise.resolve = function (value) {
+ if (value instanceof Promise) return value;
+
+ if (value === null) return NULL;
+ if (value === undefined) return UNDEFINED;
+ if (value === true) return TRUE;
+ if (value === false) return FALSE;
+ if (value === 0) return ZERO;
+ if (value === '') return EMPTYSTRING;
+
+ if (typeof value === 'object' || typeof value === 'function') {
+ try {
+ var then = value.then;
+ if (typeof then === 'function') {
+ return new Promise(then.bind(value));
+ }
+ } catch (ex) {
+ return new Promise(function (resolve, reject) {
+ reject(ex);
+ });
+ }
+ }
+ return valuePromise(value);
+};
+
+Promise.all = function (arr) {
+ var args = Array.prototype.slice.call(arr);
+
+ return new Promise(function (resolve, reject) {
+ if (args.length === 0) return resolve([]);
+ var remaining = args.length;
+ function res(i, val) {
+ if (val && (typeof val === 'object' || typeof val === 'function')) {
+ if (val instanceof Promise && val.then === Promise.prototype.then) {
+ while (val._65 === 3) {
+ val = val._55;
+ }
+ if (val._65 === 1) return res(i, val._55);
+ if (val._65 === 2) reject(val._55);
+ val.then(function (val) {
+ res(i, val);
+ }, reject);
+ return;
+ } else {
+ var then = val.then;
+ if (typeof then === 'function') {
+ var p = new Promise(then.bind(val));
+ p.then(function (val) {
+ res(i, val);
+ }, reject);
+ return;
+ }
+ }
+ }
+ args[i] = val;
+ if (--remaining === 0) {
+ resolve(args);
+ }
+ }
+ for (var i = 0; i < args.length; i++) {
+ res(i, args[i]);
+ }
+ });
+};
+
+Promise.reject = function (value) {
+ return new Promise(function (resolve, reject) {
+ reject(value);
+ });
+};
+
+Promise.race = function (values) {
+ return new Promise(function (resolve, reject) {
+ values.forEach(function(value){
+ Promise.resolve(value).then(resolve, reject);
+ });
+ });
+};
+
+/* Prototype Methods */
+
+Promise.prototype['catch'] = function (onRejected) {
+ return this.then(null, onRejected);
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/finally.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/finally.js
new file mode 100644
index 00000000..f5ee0b98
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/finally.js
@@ -0,0 +1,16 @@
+'use strict';
+
+var Promise = require('./core.js');
+
+module.exports = Promise;
+Promise.prototype['finally'] = function (f) {
+ return this.then(function (value) {
+ return Promise.resolve(f()).then(function () {
+ return value;
+ });
+ }, function (err) {
+ return Promise.resolve(f()).then(function () {
+ throw err;
+ });
+ });
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/index.js
new file mode 100644
index 00000000..6e674f38
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/index.js
@@ -0,0 +1,8 @@
+'use strict';
+
+module.exports = require('./core.js');
+require('./done.js');
+require('./finally.js');
+require('./es6-extensions.js');
+require('./node-extensions.js');
+require('./synchronous.js');
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/node-extensions.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/node-extensions.js
new file mode 100644
index 00000000..157cddc2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/node-extensions.js
@@ -0,0 +1,130 @@
+'use strict';
+
+// This file contains then/promise specific extensions that are only useful
+// for node.js interop
+
+var Promise = require('./core.js');
+var asap = require('asap');
+
+module.exports = Promise;
+
+/* Static Functions */
+
+Promise.denodeify = function (fn, argumentCount) {
+ if (
+ typeof argumentCount === 'number' && argumentCount !== Infinity
+ ) {
+ return denodeifyWithCount(fn, argumentCount);
+ } else {
+ return denodeifyWithoutCount(fn);
+ }
+};
+
+var callbackFn = (
+ 'function (err, res) {' +
+ 'if (err) { rj(err); } else { rs(res); }' +
+ '}'
+);
+function denodeifyWithCount(fn, argumentCount) {
+ var args = [];
+ for (var i = 0; i < argumentCount; i++) {
+ args.push('a' + i);
+ }
+ var body = [
+ 'return function (' + args.join(',') + ') {',
+ 'var self = this;',
+ 'return new Promise(function (rs, rj) {',
+ 'var res = fn.call(',
+ ['self'].concat(args).concat([callbackFn]).join(','),
+ ');',
+ 'if (res &&',
+ '(typeof res === "object" || typeof res === "function") &&',
+ 'typeof res.then === "function"',
+ ') {rs(res);}',
+ '});',
+ '};'
+ ].join('');
+ return Function(['Promise', 'fn'], body)(Promise, fn);
+}
+function denodeifyWithoutCount(fn) {
+ var fnLength = Math.max(fn.length - 1, 3);
+ var args = [];
+ for (var i = 0; i < fnLength; i++) {
+ args.push('a' + i);
+ }
+ var body = [
+ 'return function (' + args.join(',') + ') {',
+ 'var self = this;',
+ 'var args;',
+ 'var argLength = arguments.length;',
+ 'if (arguments.length > ' + fnLength + ') {',
+ 'args = new Array(arguments.length + 1);',
+ 'for (var i = 0; i < arguments.length; i++) {',
+ 'args[i] = arguments[i];',
+ '}',
+ '}',
+ 'return new Promise(function (rs, rj) {',
+ 'var cb = ' + callbackFn + ';',
+ 'var res;',
+ 'switch (argLength) {',
+ args.concat(['extra']).map(function (_, index) {
+ return (
+ 'case ' + (index) + ':' +
+ 'res = fn.call(' + ['self'].concat(args.slice(0, index)).concat('cb').join(',') + ');' +
+ 'break;'
+ );
+ }).join(''),
+ 'default:',
+ 'args[argLength] = cb;',
+ 'res = fn.apply(self, args);',
+ '}',
+
+ 'if (res &&',
+ '(typeof res === "object" || typeof res === "function") &&',
+ 'typeof res.then === "function"',
+ ') {rs(res);}',
+ '});',
+ '};'
+ ].join('');
+
+ return Function(
+ ['Promise', 'fn'],
+ body
+ )(Promise, fn);
+}
+
+Promise.nodeify = function (fn) {
+ return function () {
+ var args = Array.prototype.slice.call(arguments);
+ var callback =
+ typeof args[args.length - 1] === 'function' ? args.pop() : null;
+ var ctx = this;
+ try {
+ return fn.apply(this, arguments).nodeify(callback, ctx);
+ } catch (ex) {
+ if (callback === null || typeof callback == 'undefined') {
+ return new Promise(function (resolve, reject) {
+ reject(ex);
+ });
+ } else {
+ asap(function () {
+ callback.call(ctx, ex);
+ })
+ }
+ }
+ }
+};
+
+Promise.prototype.nodeify = function (callback, ctx) {
+ if (typeof callback != 'function') return this;
+
+ this.then(function (value) {
+ asap(function () {
+ callback.call(ctx, null, value);
+ });
+ }, function (err) {
+ asap(function () {
+ callback.call(ctx, err);
+ });
+ });
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/rejection-tracking.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/rejection-tracking.js
new file mode 100644
index 00000000..10ccce30
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/rejection-tracking.js
@@ -0,0 +1,113 @@
+'use strict';
+
+var Promise = require('./core');
+
+var DEFAULT_WHITELIST = [
+ ReferenceError,
+ TypeError,
+ RangeError
+];
+
+var enabled = false;
+exports.disable = disable;
+function disable() {
+ enabled = false;
+ Promise._37 = null;
+ Promise._87 = null;
+}
+
+exports.enable = enable;
+function enable(options) {
+ options = options || {};
+ if (enabled) disable();
+ enabled = true;
+ var id = 0;
+ var displayId = 0;
+ var rejections = {};
+ Promise._37 = function (promise) {
+ if (
+ promise._65 === 2 && // IS REJECTED
+ rejections[promise._51]
+ ) {
+ if (rejections[promise._51].logged) {
+ onHandled(promise._51);
+ } else {
+ clearTimeout(rejections[promise._51].timeout);
+ }
+ delete rejections[promise._51];
+ }
+ };
+ Promise._87 = function (promise, err) {
+ if (promise._40 === 0) { // not yet handled
+ promise._51 = id++;
+ rejections[promise._51] = {
+ displayId: null,
+ error: err,
+ timeout: setTimeout(
+ onUnhandled.bind(null, promise._51),
+ // For reference errors and type errors, this almost always
+ // means the programmer made a mistake, so log them after just
+ // 100ms
+ // otherwise, wait 2 seconds to see if they get handled
+ matchWhitelist(err, DEFAULT_WHITELIST)
+ ? 100
+ : 2000
+ ),
+ logged: false
+ };
+ }
+ };
+ function onUnhandled(id) {
+ if (
+ options.allRejections ||
+ matchWhitelist(
+ rejections[id].error,
+ options.whitelist || DEFAULT_WHITELIST
+ )
+ ) {
+ rejections[id].displayId = displayId++;
+ if (options.onUnhandled) {
+ rejections[id].logged = true;
+ options.onUnhandled(
+ rejections[id].displayId,
+ rejections[id].error
+ );
+ } else {
+ rejections[id].logged = true;
+ logError(
+ rejections[id].displayId,
+ rejections[id].error
+ );
+ }
+ }
+ }
+ function onHandled(id) {
+ if (rejections[id].logged) {
+ if (options.onHandled) {
+ options.onHandled(rejections[id].displayId, rejections[id].error);
+ } else if (!rejections[id].onUnhandled) {
+ console.warn(
+ 'Promise Rejection Handled (id: ' + rejections[id].displayId + '):'
+ );
+ console.warn(
+ ' This means you can ignore any previous messages of the form "Possible Unhandled Promise Rejection" with id ' +
+ rejections[id].displayId + '.'
+ );
+ }
+ }
+ }
+}
+
+function logError(id, error) {
+ console.warn('Possible Unhandled Promise Rejection (id: ' + id + '):');
+ var errStr = (error && (error.stack || error)) + '';
+ errStr.split('\n').forEach(function (line) {
+ console.warn(' ' + line);
+ });
+}
+
+function matchWhitelist(error, list) {
+ return list.some(function (cls) {
+ return error instanceof cls;
+ });
+}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/synchronous.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/synchronous.js
new file mode 100644
index 00000000..49399644
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/lib/synchronous.js
@@ -0,0 +1,62 @@
+'use strict';
+
+var Promise = require('./core.js');
+
+module.exports = Promise;
+Promise.enableSynchronous = function () {
+ Promise.prototype.isPending = function() {
+ return this.getState() == 0;
+ };
+
+ Promise.prototype.isFulfilled = function() {
+ return this.getState() == 1;
+ };
+
+ Promise.prototype.isRejected = function() {
+ return this.getState() == 2;
+ };
+
+ Promise.prototype.getValue = function () {
+ if (this._65 === 3) {
+ return this._55.getValue();
+ }
+
+ if (!this.isFulfilled()) {
+ throw new Error('Cannot get a value of an unfulfilled promise.');
+ }
+
+ return this._55;
+ };
+
+ Promise.prototype.getReason = function () {
+ if (this._65 === 3) {
+ return this._55.getReason();
+ }
+
+ if (!this.isRejected()) {
+ throw new Error('Cannot get a rejection reason of a non-rejected promise.');
+ }
+
+ return this._55;
+ };
+
+ Promise.prototype.getState = function () {
+ if (this._65 === 3) {
+ return this._55.getState();
+ }
+ if (this._65 === -1 || this._65 === -2) {
+ return 0;
+ }
+
+ return this._65;
+ };
+};
+
+Promise.disableSynchronous = function() {
+ Promise.prototype.isPending = undefined;
+ Promise.prototype.isFulfilled = undefined;
+ Promise.prototype.isRejected = undefined;
+ Promise.prototype.getValue = undefined;
+ Promise.prototype.getReason = undefined;
+ Promise.prototype.getState = undefined;
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/package.json b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/package.json
new file mode 100644
index 00000000..c4f8a8ab
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/package.json
@@ -0,0 +1,66 @@
+{
+ "_from": "promise@^7.1.1",
+ "_id": "promise@7.3.1",
+ "_inBundle": false,
+ "_integrity": "sha512-nolQXZ/4L+bP/UGlkfaIujX9BKxGwmQ9OT4mOt5yvy8iK1h3wqTEJCijzGANTCCl9nWjY41juyAn2K3Q1hLLTg==",
+ "_location": "/react-dropzone/promise",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "promise@^7.1.1",
+ "name": "promise",
+ "escapedName": "promise",
+ "rawSpec": "^7.1.1",
+ "saveSpec": null,
+ "fetchSpec": "^7.1.1"
+ },
+ "_requiredBy": [
+ "/react-dropzone/fbjs"
+ ],
+ "_resolved": "https://registry.npmjs.org/promise/-/promise-7.3.1.tgz",
+ "_shasum": "064b72602b18f90f29192b8b1bc418ffd1ebd3bf",
+ "_spec": "promise@^7.1.1",
+ "_where": "C:\\Users\\deranjer\\go\\src\\github.com\\deranjer\\goTorrent\\goTorrentWebUI\\node_modules\\react-dropzone\\node_modules\\fbjs",
+ "author": {
+ "name": "ForbesLindesay"
+ },
+ "bugs": {
+ "url": "https://github.com/then/promise/issues"
+ },
+ "bundleDependencies": false,
+ "dependencies": {
+ "asap": "~2.0.3"
+ },
+ "deprecated": false,
+ "description": "Bare bones Promises/A+ implementation",
+ "devDependencies": {
+ "acorn": "^1.0.1",
+ "better-assert": "*",
+ "istanbul": "^0.3.13",
+ "mocha": "*",
+ "promises-aplus-tests": "*",
+ "rimraf": "^2.3.2"
+ },
+ "homepage": "https://github.com/then/promise#readme",
+ "license": "MIT",
+ "main": "index.js",
+ "name": "promise",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/then/promise.git"
+ },
+ "scripts": {
+ "coverage": "istanbul cover node_modules/mocha/bin/_mocha -- --bail --timeout 200 --slow 99999 -R dot",
+ "prepublish": "node build",
+ "pretest": "node build",
+ "pretest-extensions": "node build",
+ "pretest-memory-leak": "node build",
+ "pretest-resolve": "node build",
+ "test": "mocha --bail --timeout 200 --slow 99999 -R dot && npm run test-memory-leak",
+ "test-extensions": "mocha test/extensions-tests.js --timeout 200 --slow 999999",
+ "test-memory-leak": "node --expose-gc test/memory-leak.js",
+ "test-resolve": "mocha test/resolver-tests.js --timeout 200 --slow 999999"
+ },
+ "version": "7.3.1"
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/polyfill-done.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/polyfill-done.js
new file mode 100644
index 00000000..e50b4c0e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/polyfill-done.js
@@ -0,0 +1,12 @@
+// should work in any browser without browserify
+
+if (typeof Promise.prototype.done !== 'function') {
+ Promise.prototype.done = function (onFulfilled, onRejected) {
+ var self = arguments.length ? this.then.apply(this, arguments) : this
+ self.then(null, function (err) {
+ setTimeout(function () {
+ throw err
+ }, 0)
+ })
+ }
+}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/polyfill.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/polyfill.js
new file mode 100644
index 00000000..db099f8e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/polyfill.js
@@ -0,0 +1,10 @@
+// not "use strict" so we can declare global "Promise"
+
+var asap = require('asap');
+
+if (typeof Promise === 'undefined') {
+ Promise = require('./lib/core.js')
+ require('./lib/es6-extensions.js')
+}
+
+require('./polyfill-done.js');
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/core.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/core.js
new file mode 100644
index 00000000..a84fb3da
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/core.js
@@ -0,0 +1,213 @@
+'use strict';
+
+
+
+function noop() {}
+
+// States:
+//
+// 0 - pending
+// 1 - fulfilled with _value
+// 2 - rejected with _value
+// 3 - adopted the state of another promise, _value
+//
+// once the state is no longer pending (0) it is immutable
+
+// All `_` prefixed properties will be reduced to `_{random number}`
+// at build time to obfuscate them and discourage their use.
+// We don't use symbols or Object.defineProperty to fully hide them
+// because the performance isn't good enough.
+
+
+// to avoid using try/catch inside critical functions, we
+// extract them to here.
+var LAST_ERROR = null;
+var IS_ERROR = {};
+function getThen(obj) {
+ try {
+ return obj.then;
+ } catch (ex) {
+ LAST_ERROR = ex;
+ return IS_ERROR;
+ }
+}
+
+function tryCallOne(fn, a) {
+ try {
+ return fn(a);
+ } catch (ex) {
+ LAST_ERROR = ex;
+ return IS_ERROR;
+ }
+}
+function tryCallTwo(fn, a, b) {
+ try {
+ fn(a, b);
+ } catch (ex) {
+ LAST_ERROR = ex;
+ return IS_ERROR;
+ }
+}
+
+module.exports = Promise;
+
+function Promise(fn) {
+ if (typeof this !== 'object') {
+ throw new TypeError('Promises must be constructed via new');
+ }
+ if (typeof fn !== 'function') {
+ throw new TypeError('Promise constructor\'s argument is not a function');
+ }
+ this._40 = 0;
+ this._65 = 0;
+ this._55 = null;
+ this._72 = null;
+ if (fn === noop) return;
+ doResolve(fn, this);
+}
+Promise._37 = null;
+Promise._87 = null;
+Promise._61 = noop;
+
+Promise.prototype.then = function(onFulfilled, onRejected) {
+ if (this.constructor !== Promise) {
+ return safeThen(this, onFulfilled, onRejected);
+ }
+ var res = new Promise(noop);
+ handle(this, new Handler(onFulfilled, onRejected, res));
+ return res;
+};
+
+function safeThen(self, onFulfilled, onRejected) {
+ return new self.constructor(function (resolve, reject) {
+ var res = new Promise(noop);
+ res.then(resolve, reject);
+ handle(self, new Handler(onFulfilled, onRejected, res));
+ });
+}
+function handle(self, deferred) {
+ while (self._65 === 3) {
+ self = self._55;
+ }
+ if (Promise._37) {
+ Promise._37(self);
+ }
+ if (self._65 === 0) {
+ if (self._40 === 0) {
+ self._40 = 1;
+ self._72 = deferred;
+ return;
+ }
+ if (self._40 === 1) {
+ self._40 = 2;
+ self._72 = [self._72, deferred];
+ return;
+ }
+ self._72.push(deferred);
+ return;
+ }
+ handleResolved(self, deferred);
+}
+
+function handleResolved(self, deferred) {
+ setImmediate(function() {
+ var cb = self._65 === 1 ? deferred.onFulfilled : deferred.onRejected;
+ if (cb === null) {
+ if (self._65 === 1) {
+ resolve(deferred.promise, self._55);
+ } else {
+ reject(deferred.promise, self._55);
+ }
+ return;
+ }
+ var ret = tryCallOne(cb, self._55);
+ if (ret === IS_ERROR) {
+ reject(deferred.promise, LAST_ERROR);
+ } else {
+ resolve(deferred.promise, ret);
+ }
+ });
+}
+function resolve(self, newValue) {
+ // Promise Resolution Procedure: https://github.com/promises-aplus/promises-spec#the-promise-resolution-procedure
+ if (newValue === self) {
+ return reject(
+ self,
+ new TypeError('A promise cannot be resolved with itself.')
+ );
+ }
+ if (
+ newValue &&
+ (typeof newValue === 'object' || typeof newValue === 'function')
+ ) {
+ var then = getThen(newValue);
+ if (then === IS_ERROR) {
+ return reject(self, LAST_ERROR);
+ }
+ if (
+ then === self.then &&
+ newValue instanceof Promise
+ ) {
+ self._65 = 3;
+ self._55 = newValue;
+ finale(self);
+ return;
+ } else if (typeof then === 'function') {
+ doResolve(then.bind(newValue), self);
+ return;
+ }
+ }
+ self._65 = 1;
+ self._55 = newValue;
+ finale(self);
+}
+
+function reject(self, newValue) {
+ self._65 = 2;
+ self._55 = newValue;
+ if (Promise._87) {
+ Promise._87(self, newValue);
+ }
+ finale(self);
+}
+function finale(self) {
+ if (self._40 === 1) {
+ handle(self, self._72);
+ self._72 = null;
+ }
+ if (self._40 === 2) {
+ for (var i = 0; i < self._72.length; i++) {
+ handle(self, self._72[i]);
+ }
+ self._72 = null;
+ }
+}
+
+function Handler(onFulfilled, onRejected, promise){
+ this.onFulfilled = typeof onFulfilled === 'function' ? onFulfilled : null;
+ this.onRejected = typeof onRejected === 'function' ? onRejected : null;
+ this.promise = promise;
+}
+
+/**
+ * Take a potentially misbehaving resolver function and make sure
+ * onFulfilled and onRejected are only called once.
+ *
+ * Makes no guarantees about asynchrony.
+ */
+function doResolve(fn, promise) {
+ var done = false;
+ var res = tryCallTwo(fn, function (value) {
+ if (done) return;
+ done = true;
+ resolve(promise, value);
+ }, function (reason) {
+ if (done) return;
+ done = true;
+ reject(promise, reason);
+ });
+ if (!done && res === IS_ERROR) {
+ done = true;
+ reject(promise, LAST_ERROR);
+ }
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/done.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/done.js
new file mode 100644
index 00000000..f879317d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/done.js
@@ -0,0 +1,13 @@
+'use strict';
+
+var Promise = require('./core.js');
+
+module.exports = Promise;
+Promise.prototype.done = function (onFulfilled, onRejected) {
+ var self = arguments.length ? this.then.apply(this, arguments) : this;
+ self.then(null, function (err) {
+ setTimeout(function () {
+ throw err;
+ }, 0);
+ });
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/es6-extensions.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/es6-extensions.js
new file mode 100644
index 00000000..8ab26669
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/es6-extensions.js
@@ -0,0 +1,107 @@
+'use strict';
+
+//This file contains the ES6 extensions to the core Promises/A+ API
+
+var Promise = require('./core.js');
+
+module.exports = Promise;
+
+/* Static Functions */
+
+var TRUE = valuePromise(true);
+var FALSE = valuePromise(false);
+var NULL = valuePromise(null);
+var UNDEFINED = valuePromise(undefined);
+var ZERO = valuePromise(0);
+var EMPTYSTRING = valuePromise('');
+
+function valuePromise(value) {
+ var p = new Promise(Promise._61);
+ p._65 = 1;
+ p._55 = value;
+ return p;
+}
+Promise.resolve = function (value) {
+ if (value instanceof Promise) return value;
+
+ if (value === null) return NULL;
+ if (value === undefined) return UNDEFINED;
+ if (value === true) return TRUE;
+ if (value === false) return FALSE;
+ if (value === 0) return ZERO;
+ if (value === '') return EMPTYSTRING;
+
+ if (typeof value === 'object' || typeof value === 'function') {
+ try {
+ var then = value.then;
+ if (typeof then === 'function') {
+ return new Promise(then.bind(value));
+ }
+ } catch (ex) {
+ return new Promise(function (resolve, reject) {
+ reject(ex);
+ });
+ }
+ }
+ return valuePromise(value);
+};
+
+Promise.all = function (arr) {
+ var args = Array.prototype.slice.call(arr);
+
+ return new Promise(function (resolve, reject) {
+ if (args.length === 0) return resolve([]);
+ var remaining = args.length;
+ function res(i, val) {
+ if (val && (typeof val === 'object' || typeof val === 'function')) {
+ if (val instanceof Promise && val.then === Promise.prototype.then) {
+ while (val._65 === 3) {
+ val = val._55;
+ }
+ if (val._65 === 1) return res(i, val._55);
+ if (val._65 === 2) reject(val._55);
+ val.then(function (val) {
+ res(i, val);
+ }, reject);
+ return;
+ } else {
+ var then = val.then;
+ if (typeof then === 'function') {
+ var p = new Promise(then.bind(val));
+ p.then(function (val) {
+ res(i, val);
+ }, reject);
+ return;
+ }
+ }
+ }
+ args[i] = val;
+ if (--remaining === 0) {
+ resolve(args);
+ }
+ }
+ for (var i = 0; i < args.length; i++) {
+ res(i, args[i]);
+ }
+ });
+};
+
+Promise.reject = function (value) {
+ return new Promise(function (resolve, reject) {
+ reject(value);
+ });
+};
+
+Promise.race = function (values) {
+ return new Promise(function (resolve, reject) {
+ values.forEach(function(value){
+ Promise.resolve(value).then(resolve, reject);
+ });
+ });
+};
+
+/* Prototype Methods */
+
+Promise.prototype['catch'] = function (onRejected) {
+ return this.then(null, onRejected);
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/finally.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/finally.js
new file mode 100644
index 00000000..f5ee0b98
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/finally.js
@@ -0,0 +1,16 @@
+'use strict';
+
+var Promise = require('./core.js');
+
+module.exports = Promise;
+Promise.prototype['finally'] = function (f) {
+ return this.then(function (value) {
+ return Promise.resolve(f()).then(function () {
+ return value;
+ });
+ }, function (err) {
+ return Promise.resolve(f()).then(function () {
+ throw err;
+ });
+ });
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/index.js
new file mode 100644
index 00000000..6e674f38
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/index.js
@@ -0,0 +1,8 @@
+'use strict';
+
+module.exports = require('./core.js');
+require('./done.js');
+require('./finally.js');
+require('./es6-extensions.js');
+require('./node-extensions.js');
+require('./synchronous.js');
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/node-extensions.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/node-extensions.js
new file mode 100644
index 00000000..f03e861d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/node-extensions.js
@@ -0,0 +1,130 @@
+'use strict';
+
+// This file contains then/promise specific extensions that are only useful
+// for node.js interop
+
+var Promise = require('./core.js');
+
+
+module.exports = Promise;
+
+/* Static Functions */
+
+Promise.denodeify = function (fn, argumentCount) {
+ if (
+ typeof argumentCount === 'number' && argumentCount !== Infinity
+ ) {
+ return denodeifyWithCount(fn, argumentCount);
+ } else {
+ return denodeifyWithoutCount(fn);
+ }
+};
+
+var callbackFn = (
+ 'function (err, res) {' +
+ 'if (err) { rj(err); } else { rs(res); }' +
+ '}'
+);
+function denodeifyWithCount(fn, argumentCount) {
+ var args = [];
+ for (var i = 0; i < argumentCount; i++) {
+ args.push('a' + i);
+ }
+ var body = [
+ 'return function (' + args.join(',') + ') {',
+ 'var self = this;',
+ 'return new Promise(function (rs, rj) {',
+ 'var res = fn.call(',
+ ['self'].concat(args).concat([callbackFn]).join(','),
+ ');',
+ 'if (res &&',
+ '(typeof res === "object" || typeof res === "function") &&',
+ 'typeof res.then === "function"',
+ ') {rs(res);}',
+ '});',
+ '};'
+ ].join('');
+ return Function(['Promise', 'fn'], body)(Promise, fn);
+}
+function denodeifyWithoutCount(fn) {
+ var fnLength = Math.max(fn.length - 1, 3);
+ var args = [];
+ for (var i = 0; i < fnLength; i++) {
+ args.push('a' + i);
+ }
+ var body = [
+ 'return function (' + args.join(',') + ') {',
+ 'var self = this;',
+ 'var args;',
+ 'var argLength = arguments.length;',
+ 'if (arguments.length > ' + fnLength + ') {',
+ 'args = new Array(arguments.length + 1);',
+ 'for (var i = 0; i < arguments.length; i++) {',
+ 'args[i] = arguments[i];',
+ '}',
+ '}',
+ 'return new Promise(function (rs, rj) {',
+ 'var cb = ' + callbackFn + ';',
+ 'var res;',
+ 'switch (argLength) {',
+ args.concat(['extra']).map(function (_, index) {
+ return (
+ 'case ' + (index) + ':' +
+ 'res = fn.call(' + ['self'].concat(args.slice(0, index)).concat('cb').join(',') + ');' +
+ 'break;'
+ );
+ }).join(''),
+ 'default:',
+ 'args[argLength] = cb;',
+ 'res = fn.apply(self, args);',
+ '}',
+
+ 'if (res &&',
+ '(typeof res === "object" || typeof res === "function") &&',
+ 'typeof res.then === "function"',
+ ') {rs(res);}',
+ '});',
+ '};'
+ ].join('');
+
+ return Function(
+ ['Promise', 'fn'],
+ body
+ )(Promise, fn);
+}
+
+Promise.nodeify = function (fn) {
+ return function () {
+ var args = Array.prototype.slice.call(arguments);
+ var callback =
+ typeof args[args.length - 1] === 'function' ? args.pop() : null;
+ var ctx = this;
+ try {
+ return fn.apply(this, arguments).nodeify(callback, ctx);
+ } catch (ex) {
+ if (callback === null || typeof callback == 'undefined') {
+ return new Promise(function (resolve, reject) {
+ reject(ex);
+ });
+ } else {
+ setImmediate(function () {
+ callback.call(ctx, ex);
+ })
+ }
+ }
+ }
+};
+
+Promise.prototype.nodeify = function (callback, ctx) {
+ if (typeof callback != 'function') return this;
+
+ this.then(function (value) {
+ setImmediate(function () {
+ callback.call(ctx, null, value);
+ });
+ }, function (err) {
+ setImmediate(function () {
+ callback.call(ctx, err);
+ });
+ });
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/rejection-tracking.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/rejection-tracking.js
new file mode 100644
index 00000000..10ccce30
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/rejection-tracking.js
@@ -0,0 +1,113 @@
+'use strict';
+
+var Promise = require('./core');
+
+var DEFAULT_WHITELIST = [
+ ReferenceError,
+ TypeError,
+ RangeError
+];
+
+var enabled = false;
+exports.disable = disable;
+function disable() {
+ enabled = false;
+ Promise._37 = null;
+ Promise._87 = null;
+}
+
+exports.enable = enable;
+function enable(options) {
+ options = options || {};
+ if (enabled) disable();
+ enabled = true;
+ var id = 0;
+ var displayId = 0;
+ var rejections = {};
+ Promise._37 = function (promise) {
+ if (
+ promise._65 === 2 && // IS REJECTED
+ rejections[promise._51]
+ ) {
+ if (rejections[promise._51].logged) {
+ onHandled(promise._51);
+ } else {
+ clearTimeout(rejections[promise._51].timeout);
+ }
+ delete rejections[promise._51];
+ }
+ };
+ Promise._87 = function (promise, err) {
+ if (promise._40 === 0) { // not yet handled
+ promise._51 = id++;
+ rejections[promise._51] = {
+ displayId: null,
+ error: err,
+ timeout: setTimeout(
+ onUnhandled.bind(null, promise._51),
+ // For reference errors and type errors, this almost always
+ // means the programmer made a mistake, so log them after just
+ // 100ms
+ // otherwise, wait 2 seconds to see if they get handled
+ matchWhitelist(err, DEFAULT_WHITELIST)
+ ? 100
+ : 2000
+ ),
+ logged: false
+ };
+ }
+ };
+ function onUnhandled(id) {
+ if (
+ options.allRejections ||
+ matchWhitelist(
+ rejections[id].error,
+ options.whitelist || DEFAULT_WHITELIST
+ )
+ ) {
+ rejections[id].displayId = displayId++;
+ if (options.onUnhandled) {
+ rejections[id].logged = true;
+ options.onUnhandled(
+ rejections[id].displayId,
+ rejections[id].error
+ );
+ } else {
+ rejections[id].logged = true;
+ logError(
+ rejections[id].displayId,
+ rejections[id].error
+ );
+ }
+ }
+ }
+ function onHandled(id) {
+ if (rejections[id].logged) {
+ if (options.onHandled) {
+ options.onHandled(rejections[id].displayId, rejections[id].error);
+ } else if (!rejections[id].onUnhandled) {
+ console.warn(
+ 'Promise Rejection Handled (id: ' + rejections[id].displayId + '):'
+ );
+ console.warn(
+ ' This means you can ignore any previous messages of the form "Possible Unhandled Promise Rejection" with id ' +
+ rejections[id].displayId + '.'
+ );
+ }
+ }
+ }
+}
+
+function logError(id, error) {
+ console.warn('Possible Unhandled Promise Rejection (id: ' + id + '):');
+ var errStr = (error && (error.stack || error)) + '';
+ errStr.split('\n').forEach(function (line) {
+ console.warn(' ' + line);
+ });
+}
+
+function matchWhitelist(error, list) {
+ return list.some(function (cls) {
+ return error instanceof cls;
+ });
+}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/synchronous.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/synchronous.js
new file mode 100644
index 00000000..49399644
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/setimmediate/synchronous.js
@@ -0,0 +1,62 @@
+'use strict';
+
+var Promise = require('./core.js');
+
+module.exports = Promise;
+Promise.enableSynchronous = function () {
+ Promise.prototype.isPending = function() {
+ return this.getState() == 0;
+ };
+
+ Promise.prototype.isFulfilled = function() {
+ return this.getState() == 1;
+ };
+
+ Promise.prototype.isRejected = function() {
+ return this.getState() == 2;
+ };
+
+ Promise.prototype.getValue = function () {
+ if (this._65 === 3) {
+ return this._55.getValue();
+ }
+
+ if (!this.isFulfilled()) {
+ throw new Error('Cannot get a value of an unfulfilled promise.');
+ }
+
+ return this._55;
+ };
+
+ Promise.prototype.getReason = function () {
+ if (this._65 === 3) {
+ return this._55.getReason();
+ }
+
+ if (!this.isRejected()) {
+ throw new Error('Cannot get a rejection reason of a non-rejected promise.');
+ }
+
+ return this._55;
+ };
+
+ Promise.prototype.getState = function () {
+ if (this._65 === 3) {
+ return this._55.getState();
+ }
+ if (this._65 === -1 || this._65 === -2) {
+ return 0;
+ }
+
+ return this._65;
+ };
+};
+
+Promise.disableSynchronous = function() {
+ Promise.prototype.isPending = undefined;
+ Promise.prototype.isFulfilled = undefined;
+ Promise.prototype.isRejected = undefined;
+ Promise.prototype.getValue = undefined;
+ Promise.prototype.getReason = undefined;
+ Promise.prototype.getState = undefined;
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/core.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/core.js
new file mode 100644
index 00000000..312010d9
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/core.js
@@ -0,0 +1,213 @@
+'use strict';
+
+var asap = require('asap/raw');
+
+function noop() {}
+
+// States:
+//
+// 0 - pending
+// 1 - fulfilled with _value
+// 2 - rejected with _value
+// 3 - adopted the state of another promise, _value
+//
+// once the state is no longer pending (0) it is immutable
+
+// All `_` prefixed properties will be reduced to `_{random number}`
+// at build time to obfuscate them and discourage their use.
+// We don't use symbols or Object.defineProperty to fully hide them
+// because the performance isn't good enough.
+
+
+// to avoid using try/catch inside critical functions, we
+// extract them to here.
+var LAST_ERROR = null;
+var IS_ERROR = {};
+function getThen(obj) {
+ try {
+ return obj.then;
+ } catch (ex) {
+ LAST_ERROR = ex;
+ return IS_ERROR;
+ }
+}
+
+function tryCallOne(fn, a) {
+ try {
+ return fn(a);
+ } catch (ex) {
+ LAST_ERROR = ex;
+ return IS_ERROR;
+ }
+}
+function tryCallTwo(fn, a, b) {
+ try {
+ fn(a, b);
+ } catch (ex) {
+ LAST_ERROR = ex;
+ return IS_ERROR;
+ }
+}
+
+module.exports = Promise;
+
+function Promise(fn) {
+ if (typeof this !== 'object') {
+ throw new TypeError('Promises must be constructed via new');
+ }
+ if (typeof fn !== 'function') {
+ throw new TypeError('Promise constructor\'s argument is not a function');
+ }
+ this._deferredState = 0;
+ this._state = 0;
+ this._value = null;
+ this._deferreds = null;
+ if (fn === noop) return;
+ doResolve(fn, this);
+}
+Promise._onHandle = null;
+Promise._onReject = null;
+Promise._noop = noop;
+
+Promise.prototype.then = function(onFulfilled, onRejected) {
+ if (this.constructor !== Promise) {
+ return safeThen(this, onFulfilled, onRejected);
+ }
+ var res = new Promise(noop);
+ handle(this, new Handler(onFulfilled, onRejected, res));
+ return res;
+};
+
+function safeThen(self, onFulfilled, onRejected) {
+ return new self.constructor(function (resolve, reject) {
+ var res = new Promise(noop);
+ res.then(resolve, reject);
+ handle(self, new Handler(onFulfilled, onRejected, res));
+ });
+}
+function handle(self, deferred) {
+ while (self._state === 3) {
+ self = self._value;
+ }
+ if (Promise._onHandle) {
+ Promise._onHandle(self);
+ }
+ if (self._state === 0) {
+ if (self._deferredState === 0) {
+ self._deferredState = 1;
+ self._deferreds = deferred;
+ return;
+ }
+ if (self._deferredState === 1) {
+ self._deferredState = 2;
+ self._deferreds = [self._deferreds, deferred];
+ return;
+ }
+ self._deferreds.push(deferred);
+ return;
+ }
+ handleResolved(self, deferred);
+}
+
+function handleResolved(self, deferred) {
+ asap(function() {
+ var cb = self._state === 1 ? deferred.onFulfilled : deferred.onRejected;
+ if (cb === null) {
+ if (self._state === 1) {
+ resolve(deferred.promise, self._value);
+ } else {
+ reject(deferred.promise, self._value);
+ }
+ return;
+ }
+ var ret = tryCallOne(cb, self._value);
+ if (ret === IS_ERROR) {
+ reject(deferred.promise, LAST_ERROR);
+ } else {
+ resolve(deferred.promise, ret);
+ }
+ });
+}
+function resolve(self, newValue) {
+ // Promise Resolution Procedure: https://github.com/promises-aplus/promises-spec#the-promise-resolution-procedure
+ if (newValue === self) {
+ return reject(
+ self,
+ new TypeError('A promise cannot be resolved with itself.')
+ );
+ }
+ if (
+ newValue &&
+ (typeof newValue === 'object' || typeof newValue === 'function')
+ ) {
+ var then = getThen(newValue);
+ if (then === IS_ERROR) {
+ return reject(self, LAST_ERROR);
+ }
+ if (
+ then === self.then &&
+ newValue instanceof Promise
+ ) {
+ self._state = 3;
+ self._value = newValue;
+ finale(self);
+ return;
+ } else if (typeof then === 'function') {
+ doResolve(then.bind(newValue), self);
+ return;
+ }
+ }
+ self._state = 1;
+ self._value = newValue;
+ finale(self);
+}
+
+function reject(self, newValue) {
+ self._state = 2;
+ self._value = newValue;
+ if (Promise._onReject) {
+ Promise._onReject(self, newValue);
+ }
+ finale(self);
+}
+function finale(self) {
+ if (self._deferredState === 1) {
+ handle(self, self._deferreds);
+ self._deferreds = null;
+ }
+ if (self._deferredState === 2) {
+ for (var i = 0; i < self._deferreds.length; i++) {
+ handle(self, self._deferreds[i]);
+ }
+ self._deferreds = null;
+ }
+}
+
+function Handler(onFulfilled, onRejected, promise){
+ this.onFulfilled = typeof onFulfilled === 'function' ? onFulfilled : null;
+ this.onRejected = typeof onRejected === 'function' ? onRejected : null;
+ this.promise = promise;
+}
+
+/**
+ * Take a potentially misbehaving resolver function and make sure
+ * onFulfilled and onRejected are only called once.
+ *
+ * Makes no guarantees about asynchrony.
+ */
+function doResolve(fn, promise) {
+ var done = false;
+ var res = tryCallTwo(fn, function (value) {
+ if (done) return;
+ done = true;
+ resolve(promise, value);
+ }, function (reason) {
+ if (done) return;
+ done = true;
+ reject(promise, reason);
+ });
+ if (!done && res === IS_ERROR) {
+ done = true;
+ reject(promise, LAST_ERROR);
+ }
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/done.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/done.js
new file mode 100644
index 00000000..f879317d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/done.js
@@ -0,0 +1,13 @@
+'use strict';
+
+var Promise = require('./core.js');
+
+module.exports = Promise;
+Promise.prototype.done = function (onFulfilled, onRejected) {
+ var self = arguments.length ? this.then.apply(this, arguments) : this;
+ self.then(null, function (err) {
+ setTimeout(function () {
+ throw err;
+ }, 0);
+ });
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/es6-extensions.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/es6-extensions.js
new file mode 100644
index 00000000..04358131
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/es6-extensions.js
@@ -0,0 +1,107 @@
+'use strict';
+
+//This file contains the ES6 extensions to the core Promises/A+ API
+
+var Promise = require('./core.js');
+
+module.exports = Promise;
+
+/* Static Functions */
+
+var TRUE = valuePromise(true);
+var FALSE = valuePromise(false);
+var NULL = valuePromise(null);
+var UNDEFINED = valuePromise(undefined);
+var ZERO = valuePromise(0);
+var EMPTYSTRING = valuePromise('');
+
+function valuePromise(value) {
+ var p = new Promise(Promise._noop);
+ p._state = 1;
+ p._value = value;
+ return p;
+}
+Promise.resolve = function (value) {
+ if (value instanceof Promise) return value;
+
+ if (value === null) return NULL;
+ if (value === undefined) return UNDEFINED;
+ if (value === true) return TRUE;
+ if (value === false) return FALSE;
+ if (value === 0) return ZERO;
+ if (value === '') return EMPTYSTRING;
+
+ if (typeof value === 'object' || typeof value === 'function') {
+ try {
+ var then = value.then;
+ if (typeof then === 'function') {
+ return new Promise(then.bind(value));
+ }
+ } catch (ex) {
+ return new Promise(function (resolve, reject) {
+ reject(ex);
+ });
+ }
+ }
+ return valuePromise(value);
+};
+
+Promise.all = function (arr) {
+ var args = Array.prototype.slice.call(arr);
+
+ return new Promise(function (resolve, reject) {
+ if (args.length === 0) return resolve([]);
+ var remaining = args.length;
+ function res(i, val) {
+ if (val && (typeof val === 'object' || typeof val === 'function')) {
+ if (val instanceof Promise && val.then === Promise.prototype.then) {
+ while (val._state === 3) {
+ val = val._value;
+ }
+ if (val._state === 1) return res(i, val._value);
+ if (val._state === 2) reject(val._value);
+ val.then(function (val) {
+ res(i, val);
+ }, reject);
+ return;
+ } else {
+ var then = val.then;
+ if (typeof then === 'function') {
+ var p = new Promise(then.bind(val));
+ p.then(function (val) {
+ res(i, val);
+ }, reject);
+ return;
+ }
+ }
+ }
+ args[i] = val;
+ if (--remaining === 0) {
+ resolve(args);
+ }
+ }
+ for (var i = 0; i < args.length; i++) {
+ res(i, args[i]);
+ }
+ });
+};
+
+Promise.reject = function (value) {
+ return new Promise(function (resolve, reject) {
+ reject(value);
+ });
+};
+
+Promise.race = function (values) {
+ return new Promise(function (resolve, reject) {
+ values.forEach(function(value){
+ Promise.resolve(value).then(resolve, reject);
+ });
+ });
+};
+
+/* Prototype Methods */
+
+Promise.prototype['catch'] = function (onRejected) {
+ return this.then(null, onRejected);
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/finally.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/finally.js
new file mode 100644
index 00000000..f5ee0b98
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/finally.js
@@ -0,0 +1,16 @@
+'use strict';
+
+var Promise = require('./core.js');
+
+module.exports = Promise;
+Promise.prototype['finally'] = function (f) {
+ return this.then(function (value) {
+ return Promise.resolve(f()).then(function () {
+ return value;
+ });
+ }, function (err) {
+ return Promise.resolve(f()).then(function () {
+ throw err;
+ });
+ });
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/index.js
new file mode 100644
index 00000000..6e674f38
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/index.js
@@ -0,0 +1,8 @@
+'use strict';
+
+module.exports = require('./core.js');
+require('./done.js');
+require('./finally.js');
+require('./es6-extensions.js');
+require('./node-extensions.js');
+require('./synchronous.js');
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/node-extensions.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/node-extensions.js
new file mode 100644
index 00000000..157cddc2
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/node-extensions.js
@@ -0,0 +1,130 @@
+'use strict';
+
+// This file contains then/promise specific extensions that are only useful
+// for node.js interop
+
+var Promise = require('./core.js');
+var asap = require('asap');
+
+module.exports = Promise;
+
+/* Static Functions */
+
+Promise.denodeify = function (fn, argumentCount) {
+ if (
+ typeof argumentCount === 'number' && argumentCount !== Infinity
+ ) {
+ return denodeifyWithCount(fn, argumentCount);
+ } else {
+ return denodeifyWithoutCount(fn);
+ }
+};
+
+var callbackFn = (
+ 'function (err, res) {' +
+ 'if (err) { rj(err); } else { rs(res); }' +
+ '}'
+);
+function denodeifyWithCount(fn, argumentCount) {
+ var args = [];
+ for (var i = 0; i < argumentCount; i++) {
+ args.push('a' + i);
+ }
+ var body = [
+ 'return function (' + args.join(',') + ') {',
+ 'var self = this;',
+ 'return new Promise(function (rs, rj) {',
+ 'var res = fn.call(',
+ ['self'].concat(args).concat([callbackFn]).join(','),
+ ');',
+ 'if (res &&',
+ '(typeof res === "object" || typeof res === "function") &&',
+ 'typeof res.then === "function"',
+ ') {rs(res);}',
+ '});',
+ '};'
+ ].join('');
+ return Function(['Promise', 'fn'], body)(Promise, fn);
+}
+function denodeifyWithoutCount(fn) {
+ var fnLength = Math.max(fn.length - 1, 3);
+ var args = [];
+ for (var i = 0; i < fnLength; i++) {
+ args.push('a' + i);
+ }
+ var body = [
+ 'return function (' + args.join(',') + ') {',
+ 'var self = this;',
+ 'var args;',
+ 'var argLength = arguments.length;',
+ 'if (arguments.length > ' + fnLength + ') {',
+ 'args = new Array(arguments.length + 1);',
+ 'for (var i = 0; i < arguments.length; i++) {',
+ 'args[i] = arguments[i];',
+ '}',
+ '}',
+ 'return new Promise(function (rs, rj) {',
+ 'var cb = ' + callbackFn + ';',
+ 'var res;',
+ 'switch (argLength) {',
+ args.concat(['extra']).map(function (_, index) {
+ return (
+ 'case ' + (index) + ':' +
+ 'res = fn.call(' + ['self'].concat(args.slice(0, index)).concat('cb').join(',') + ');' +
+ 'break;'
+ );
+ }).join(''),
+ 'default:',
+ 'args[argLength] = cb;',
+ 'res = fn.apply(self, args);',
+ '}',
+
+ 'if (res &&',
+ '(typeof res === "object" || typeof res === "function") &&',
+ 'typeof res.then === "function"',
+ ') {rs(res);}',
+ '});',
+ '};'
+ ].join('');
+
+ return Function(
+ ['Promise', 'fn'],
+ body
+ )(Promise, fn);
+}
+
+Promise.nodeify = function (fn) {
+ return function () {
+ var args = Array.prototype.slice.call(arguments);
+ var callback =
+ typeof args[args.length - 1] === 'function' ? args.pop() : null;
+ var ctx = this;
+ try {
+ return fn.apply(this, arguments).nodeify(callback, ctx);
+ } catch (ex) {
+ if (callback === null || typeof callback == 'undefined') {
+ return new Promise(function (resolve, reject) {
+ reject(ex);
+ });
+ } else {
+ asap(function () {
+ callback.call(ctx, ex);
+ })
+ }
+ }
+ }
+};
+
+Promise.prototype.nodeify = function (callback, ctx) {
+ if (typeof callback != 'function') return this;
+
+ this.then(function (value) {
+ asap(function () {
+ callback.call(ctx, null, value);
+ });
+ }, function (err) {
+ asap(function () {
+ callback.call(ctx, err);
+ });
+ });
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/rejection-tracking.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/rejection-tracking.js
new file mode 100644
index 00000000..33a59a19
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/rejection-tracking.js
@@ -0,0 +1,113 @@
+'use strict';
+
+var Promise = require('./core');
+
+var DEFAULT_WHITELIST = [
+ ReferenceError,
+ TypeError,
+ RangeError
+];
+
+var enabled = false;
+exports.disable = disable;
+function disable() {
+ enabled = false;
+ Promise._onHandle = null;
+ Promise._onReject = null;
+}
+
+exports.enable = enable;
+function enable(options) {
+ options = options || {};
+ if (enabled) disable();
+ enabled = true;
+ var id = 0;
+ var displayId = 0;
+ var rejections = {};
+ Promise._onHandle = function (promise) {
+ if (
+ promise._state === 2 && // IS REJECTED
+ rejections[promise._rejectionId]
+ ) {
+ if (rejections[promise._rejectionId].logged) {
+ onHandled(promise._rejectionId);
+ } else {
+ clearTimeout(rejections[promise._rejectionId].timeout);
+ }
+ delete rejections[promise._rejectionId];
+ }
+ };
+ Promise._onReject = function (promise, err) {
+ if (promise._deferredState === 0) { // not yet handled
+ promise._rejectionId = id++;
+ rejections[promise._rejectionId] = {
+ displayId: null,
+ error: err,
+ timeout: setTimeout(
+ onUnhandled.bind(null, promise._rejectionId),
+ // For reference errors and type errors, this almost always
+ // means the programmer made a mistake, so log them after just
+ // 100ms
+ // otherwise, wait 2 seconds to see if they get handled
+ matchWhitelist(err, DEFAULT_WHITELIST)
+ ? 100
+ : 2000
+ ),
+ logged: false
+ };
+ }
+ };
+ function onUnhandled(id) {
+ if (
+ options.allRejections ||
+ matchWhitelist(
+ rejections[id].error,
+ options.whitelist || DEFAULT_WHITELIST
+ )
+ ) {
+ rejections[id].displayId = displayId++;
+ if (options.onUnhandled) {
+ rejections[id].logged = true;
+ options.onUnhandled(
+ rejections[id].displayId,
+ rejections[id].error
+ );
+ } else {
+ rejections[id].logged = true;
+ logError(
+ rejections[id].displayId,
+ rejections[id].error
+ );
+ }
+ }
+ }
+ function onHandled(id) {
+ if (rejections[id].logged) {
+ if (options.onHandled) {
+ options.onHandled(rejections[id].displayId, rejections[id].error);
+ } else if (!rejections[id].onUnhandled) {
+ console.warn(
+ 'Promise Rejection Handled (id: ' + rejections[id].displayId + '):'
+ );
+ console.warn(
+ ' This means you can ignore any previous messages of the form "Possible Unhandled Promise Rejection" with id ' +
+ rejections[id].displayId + '.'
+ );
+ }
+ }
+ }
+}
+
+function logError(id, error) {
+ console.warn('Possible Unhandled Promise Rejection (id: ' + id + '):');
+ var errStr = (error && (error.stack || error)) + '';
+ errStr.split('\n').forEach(function (line) {
+ console.warn(' ' + line);
+ });
+}
+
+function matchWhitelist(error, list) {
+ return list.some(function (cls) {
+ return error instanceof cls;
+ });
+}
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/synchronous.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/synchronous.js
new file mode 100644
index 00000000..38b228f5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/promise/src/synchronous.js
@@ -0,0 +1,62 @@
+'use strict';
+
+var Promise = require('./core.js');
+
+module.exports = Promise;
+Promise.enableSynchronous = function () {
+ Promise.prototype.isPending = function() {
+ return this.getState() == 0;
+ };
+
+ Promise.prototype.isFulfilled = function() {
+ return this.getState() == 1;
+ };
+
+ Promise.prototype.isRejected = function() {
+ return this.getState() == 2;
+ };
+
+ Promise.prototype.getValue = function () {
+ if (this._state === 3) {
+ return this._value.getValue();
+ }
+
+ if (!this.isFulfilled()) {
+ throw new Error('Cannot get a value of an unfulfilled promise.');
+ }
+
+ return this._value;
+ };
+
+ Promise.prototype.getReason = function () {
+ if (this._state === 3) {
+ return this._value.getReason();
+ }
+
+ if (!this.isRejected()) {
+ throw new Error('Cannot get a rejection reason of a non-rejected promise.');
+ }
+
+ return this._value;
+ };
+
+ Promise.prototype.getState = function () {
+ if (this._state === 3) {
+ return this._value.getState();
+ }
+ if (this._state === -1 || this._state === -2) {
+ return 0;
+ }
+
+ return this._state;
+ };
+};
+
+Promise.disableSynchronous = function() {
+ Promise.prototype.isPending = undefined;
+ Promise.prototype.isFulfilled = undefined;
+ Promise.prototype.isRejected = undefined;
+ Promise.prototype.getValue = undefined;
+ Promise.prototype.getReason = undefined;
+ Promise.prototype.getState = undefined;
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/CHANGELOG.md b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/CHANGELOG.md
new file mode 100644
index 00000000..f921c8b6
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/CHANGELOG.md
@@ -0,0 +1,70 @@
+## 15.6.0
+
+* Switch from BSD + Patents to MIT license
+* Add PropTypes.exact, like PropTypes.shape but warns on extra object keys. ([@thejameskyle](https://github.com/thejameskyle) and [@aweary](https://github.com/aweary) in [#41](https://github.com/reactjs/prop-types/pull/41) and [#87](https://github.com/reactjs/prop-types/pull/87))
+
+## 15.5.10
+
+* Fix a false positive warning when using a production UMD build of a third-party library with a DEV version of React. ([@gaearon](https://github.com/gaearon) in [#50](https://github.com/reactjs/prop-types/pull/50))
+
+## 15.5.9
+
+* Add `loose-envify` Browserify transform for users who don't envify globally. ([@mridgway](https://github.com/mridgway) in [#45](https://github.com/reactjs/prop-types/pull/45))
+
+## 15.5.8
+
+* Limit the manual PropTypes call warning count because it has false positives with React versions earlier than 15.2.0 in the 15.x branch and 0.14.9 in the 0.14.x branch. ([@gaearon](https://github.com/gaearon) in [#26](https://github.com/reactjs/prop-types/pull/26))
+
+## 15.5.7
+
+* **Critical Bugfix:** Fix an accidental breaking change that caused errors in production when used through `React.PropTypes`. ([@gaearon](https://github.com/gaearon) in [#20](https://github.com/reactjs/prop-types/pull/20))
+* Improve the size of production UMD build. ([@aweary](https://github.com/aweary) in [38ba18](https://github.com/reactjs/prop-types/commit/38ba18a4a8f705f4b2b33c88204573ddd604f2d6) and [7882a7](https://github.com/reactjs/prop-types/commit/7882a7285293db5f284bcf559b869fd2cd4c44d4))
+
+## 15.5.6
+
+**Note: this release has a critical issue and was deprecated. Please update to 15.5.7 or higher.**
+
+* Fix a markdown issue in README. ([@bvaughn](https://github.com/bvaughn) in [174f77](https://github.com/reactjs/prop-types/commit/174f77a50484fa628593e84b871fb40eed78b69a))
+
+## 15.5.5
+
+**Note: this release has a critical issue and was deprecated. Please update to 15.5.7 or higher.**
+
+* Add missing documentation and license files. ([@bvaughn](https://github.com/bvaughn) in [0a53d3](https://github.com/reactjs/prop-types/commit/0a53d3a34283ae1e2d3aa396632b6dc2a2061e6a))
+
+## 15.5.4
+
+**Note: this release has a critical issue and was deprecated. Please update to 15.5.7 or higher.**
+
+* Reduce the size of the UMD Build. ([@acdlite](https://github.com/acdlite) in [31e9344](https://github.com/reactjs/prop-types/commit/31e9344ca3233159928da66295da17dad82db1a8))
+* Remove bad package url. ([@ljharb](https://github.com/ljharb) in [158198f](https://github.com/reactjs/prop-types/commit/158198fd6c468a3f6f742e0e355e622b3914048a))
+* Remove the accidentally included typechecking code from the production build.
+
+## 15.5.3
+
+**Note: this release has a critical issue and was deprecated. Please update to 15.5.7 or higher.**
+
+* Remove the accidentally included React package code from the UMD bundle. ([@acdlite](https://github.com/acdlite) in [df318bb](https://github.com/reactjs/prop-types/commit/df318bba8a89bc5aadbb0292822cf4ed71d27ace))
+
+## 15.5.2
+
+**Note: this release has a critical issue and was deprecated. Please update to 15.5.7 or higher.**
+
+* Remove dependency on React for CommonJS entry point. ([@acdlite](https://github.com/acdlite) in [cae72bb](https://github.com/reactjs/prop-types/commit/cae72bb281a3766c765e3624f6088c3713567e6d))
+
+
+## 15.5.1
+
+**Note: this release has a critical issue and was deprecated. Please update to 15.5.7 or higher.**
+
+* Remove accidental uncompiled ES6 syntax in the published package. ([@acdlite](https://github.com/acdlite) in [e191963](https://github.com/facebook/react/commit/e1919638b39dd65eedd250a8bb649773ca61b6f1))
+
+## 15.5.0
+
+**Note: this release has a critical issue and was deprecated. Please update to 15.5.7 or higher.**
+
+* Initial release.
+
+## Before 15.5.0
+
+PropTypes was previously included in React, but is now a separate package. For earlier history of PropTypes [see the React change log.](https://github.com/facebook/react/blob/master/CHANGELOG.md)
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/LICENSE b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/LICENSE
new file mode 100644
index 00000000..188fb2b0
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/LICENSE
@@ -0,0 +1,21 @@
+MIT License
+
+Copyright (c) 2013-present, Facebook, Inc.
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is
+furnished to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
+FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
+AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
+LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
+OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
+SOFTWARE.
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/README.md b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/README.md
new file mode 100644
index 00000000..8ac77c3c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/README.md
@@ -0,0 +1,262 @@
+# prop-types
+
+Runtime type checking for React props and similar objects.
+
+You can use prop-types to document the intended types of properties passed to
+components. React (and potentially other libraries—see the checkPropTypes()
+reference below) will check props passed to your components against those
+definitions, and warn in development if they don’t match.
+
+## Installation
+
+```shell
+npm install --save prop-types
+```
+
+## Importing
+
+```js
+import PropTypes from 'prop-types'; // ES6
+var PropTypes = require('prop-types'); // ES5 with npm
+```
+
+If you prefer a `
+
+
+
+```
+
+## Usage
+
+PropTypes was originally exposed as part of the React core module, and is
+commonly used with React components.
+Here is an example of using PropTypes with a React component, which also
+documents the different validators provided:
+
+```js
+import React from 'react';
+import PropTypes from 'prop-types';
+
+class MyComponent extends React.Component {
+ render() {
+ // ... do things with the props
+ }
+}
+
+MyComponent.propTypes = {
+ // You can declare that a prop is a specific JS primitive. By default, these
+ // are all optional.
+ optionalArray: PropTypes.array,
+ optionalBool: PropTypes.bool,
+ optionalFunc: PropTypes.func,
+ optionalNumber: PropTypes.number,
+ optionalObject: PropTypes.object,
+ optionalString: PropTypes.string,
+ optionalSymbol: PropTypes.symbol,
+
+ // Anything that can be rendered: numbers, strings, elements or an array
+ // (or fragment) containing these types.
+ optionalNode: PropTypes.node,
+
+ // A React element.
+ optionalElement: PropTypes.element,
+
+ // You can also declare that a prop is an instance of a class. This uses
+ // JS's instanceof operator.
+ optionalMessage: PropTypes.instanceOf(Message),
+
+ // You can ensure that your prop is limited to specific values by treating
+ // it as an enum.
+ optionalEnum: PropTypes.oneOf(['News', 'Photos']),
+
+ // An object that could be one of many types
+ optionalUnion: PropTypes.oneOfType([
+ PropTypes.string,
+ PropTypes.number,
+ PropTypes.instanceOf(Message)
+ ]),
+
+ // An array of a certain type
+ optionalArrayOf: PropTypes.arrayOf(PropTypes.number),
+
+ // An object with property values of a certain type
+ optionalObjectOf: PropTypes.objectOf(PropTypes.number),
+
+ // An object taking on a particular shape
+ optionalObjectWithShape: PropTypes.shape({
+ color: PropTypes.string,
+ fontSize: PropTypes.number
+ }),
+
+ // You can chain any of the above with `isRequired` to make sure a warning
+ // is shown if the prop isn't provided.
+ requiredFunc: PropTypes.func.isRequired,
+
+ // A value of any data type
+ requiredAny: PropTypes.any.isRequired,
+
+ // You can also specify a custom validator. It should return an Error
+ // object if the validation fails. Don't `console.warn` or throw, as this
+ // won't work inside `oneOfType`.
+ customProp: function(props, propName, componentName) {
+ if (!/matchme/.test(props[propName])) {
+ return new Error(
+ 'Invalid prop `' + propName + '` supplied to' +
+ ' `' + componentName + '`. Validation failed.'
+ );
+ }
+ },
+
+ // You can also supply a custom validator to `arrayOf` and `objectOf`.
+ // It should return an Error object if the validation fails. The validator
+ // will be called for each key in the array or object. The first two
+ // arguments of the validator are the array or object itself, and the
+ // current item's key.
+ customArrayProp: PropTypes.arrayOf(function(propValue, key, componentName, location, propFullName) {
+ if (!/matchme/.test(propValue[key])) {
+ return new Error(
+ 'Invalid prop `' + propFullName + '` supplied to' +
+ ' `' + componentName + '`. Validation failed.'
+ );
+ }
+ })
+};
+```
+
+Refer to the [React documentation](https://facebook.github.io/react/docs/typechecking-with-proptypes.html) for more information.
+
+## Migrating from React.PropTypes
+
+Check out [Migrating from React.PropTypes](https://facebook.github.io/react/blog/2017/04/07/react-v15.5.0.html#migrating-from-react.proptypes) for details on how to migrate to `prop-types` from `React.PropTypes`.
+
+Note that this blog posts **mentions a codemod script that performs the conversion automatically**.
+
+There are also important notes below.
+
+## How to Depend on This Package?
+
+For apps, we recommend putting it in `dependencies` with a caret range.
+For example:
+
+```js
+ "dependencies": {
+ "prop-types": "^15.5.7"
+ }
+```
+
+For libraries, we *also* recommend leaving it in `dependencies`:
+
+```js
+ "dependencies": {
+ "prop-types": "^15.5.7"
+ },
+ "peerDependencies": {
+ "react": "^15.5.0"
+ }
+```
+
+**Note:** there are known issues in versions before 15.5.7 so we recommend using it as the minimal version.
+
+Make sure that the version range uses a caret (`^`) and thus is broad enough for npm to efficiently deduplicate packages.
+
+For UMD bundles of your components, make sure you **don’t** include `PropTypes` in the build. Usually this is done by marking it as an external (the specifics depend on your bundler), just like you do with React.
+
+## Compatibility
+
+### React 0.14
+
+This package is compatible with **React 0.14.9**. Compared to 0.14.8 (which was released a year ago), there are no other changes in 0.14.9, so it should be a painless upgrade.
+
+```shell
+# ATTENTION: Only run this if you still use React 0.14!
+npm install --save react@^0.14.9 react-dom@^0.14.9
+```
+
+### React 15+
+
+This package is compatible with **React 15.3.0** and higher.
+
+```
+npm install --save react@^15.3.0 react-dom@^15.3.0
+```
+
+### What happens on other React versions?
+
+It outputs warnings with the message below even though the developer doesn’t do anything wrong. Unfortunately there is no solution for this other than updating React to either 15.3.0 or higher, or 0.14.9 if you’re using React 0.14.
+
+## Difference from `React.PropTypes`: Don’t Call Validator Functions
+
+First of all, **which version of React are you using**? You might be seeing this message because a component library has updated to use `prop-types` package, but your version of React is incompatible with it. See the [above section](#compatibility) for more details.
+
+Are you using either React 0.14.9 or a version higher than React 15.3.0? Read on.
+
+When you migrate components to use the standalone `prop-types`, **all validator functions will start throwing an error if you call them directly**. This makes sure that nobody relies on them in production code, and it is safe to strip their implementations to optimize the bundle size.
+
+Code like this is still fine:
+
+```js
+MyComponent.propTypes = {
+ myProp: PropTypes.bool
+};
+```
+
+However, code like this will not work with the `prop-types` package:
+
+```js
+// Will not work with `prop-types` package!
+var errorOrNull = PropTypes.bool(42, 'myProp', 'MyComponent', 'prop');
+```
+
+It will throw an error:
+
+```
+Calling PropTypes validators directly is not supported by the `prop-types` package.
+Use PropTypes.checkPropTypes() to call them.
+```
+
+(If you see **a warning** rather than an error with this message, please check the [above section about compatibility](#compatibility).)
+
+This is new behavior, and you will only encounter it when you migrate from `React.PropTypes` to the `prop-types` package. For the vast majority of components, this doesn’t matter, and if you didn’t see [this warning](https://facebook.github.io/react/warnings/dont-call-proptypes.html) in your components, your code is safe to migrate. This is not a breaking change in React because you are only opting into this change for a component by explicitly changing your imports to use `prop-types`. If you temporarily need the old behavior, you can keep using `React.PropTypes` until React 16.
+
+**If you absolutely need to trigger the validation manually**, call `PropTypes.checkPropTypes()`. Unlike the validators themselves, this function is safe to call in production, as it will be replaced by an empty function:
+
+```js
+// Works with standalone PropTypes
+PropTypes.checkPropTypes(MyComponent.propTypes, props, 'prop', 'MyComponent');
+```
+See below for more info.
+
+**You might also see this error** if you’re calling a `PropTypes` validator from your own custom `PropTypes` validator. In this case, the fix is to make sure that you are passing *all* of the arguments to the inner function. There is a more in-depth explanation of how to fix it [on this page](https://facebook.github.io/react/warnings/dont-call-proptypes.html#fixing-the-false-positive-in-third-party-proptypes). Alternatively, you can temporarily keep using `React.PropTypes` until React 16, as it would still only warn in this case.
+
+If you use a bundler like Browserify or Webpack, don’t forget to [follow these instructions](https://facebook.github.io/react/docs/installation.html#development-and-production-versions) to correctly bundle your application in development or production mode. Otherwise you’ll ship unnecessary code to your users.
+
+## PropTypes.checkPropTypes
+
+React will automatically check the propTypes you set on the component, but if
+you are using PropTypes without React then you may want to manually call
+`PropTypes.checkPropTypes`, like so:
+
+```js
+const myPropTypes = {
+ name: PropTypes.string,
+ age: PropTypes.number,
+ // ... define your prop validations
+};
+
+const props = {
+ name: 'hello', // is valid
+ age: 'world', // not valid
+};
+
+// Let's say your component is called 'MyComponent'
+
+// Works with standalone PropTypes
+PropTypes.checkPropTypes(myPropTypes, props, 'prop', 'MyComponent');
+// This will warn as follows:
+// Warning: Failed prop type: Invalid prop `age` of type `string` supplied to
+// `MyComponent`, expected `number`.
+```
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/checkPropTypes.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/checkPropTypes.js
new file mode 100644
index 00000000..0802c360
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/checkPropTypes.js
@@ -0,0 +1,59 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ */
+
+'use strict';
+
+if (process.env.NODE_ENV !== 'production') {
+ var invariant = require('fbjs/lib/invariant');
+ var warning = require('fbjs/lib/warning');
+ var ReactPropTypesSecret = require('./lib/ReactPropTypesSecret');
+ var loggedTypeFailures = {};
+}
+
+/**
+ * Assert that the values match with the type specs.
+ * Error messages are memorized and will only be shown once.
+ *
+ * @param {object} typeSpecs Map of name to a ReactPropType
+ * @param {object} values Runtime values that need to be type-checked
+ * @param {string} location e.g. "prop", "context", "child context"
+ * @param {string} componentName Name of the component for error messages.
+ * @param {?Function} getStack Returns the component stack.
+ * @private
+ */
+function checkPropTypes(typeSpecs, values, location, componentName, getStack) {
+ if (process.env.NODE_ENV !== 'production') {
+ for (var typeSpecName in typeSpecs) {
+ if (typeSpecs.hasOwnProperty(typeSpecName)) {
+ var error;
+ // Prop type validation may throw. In case they do, we don't want to
+ // fail the render phase where it didn't fail before. So we log it.
+ // After these have been cleaned up, we'll let them throw.
+ try {
+ // This is intentionally an invariant that gets caught. It's the same
+ // behavior as without this statement except with a better message.
+ invariant(typeof typeSpecs[typeSpecName] === 'function', '%s: %s type `%s` is invalid; it must be a function, usually from ' + 'the `prop-types` package, but received `%s`.', componentName || 'React class', location, typeSpecName, typeof typeSpecs[typeSpecName]);
+ error = typeSpecs[typeSpecName](values, typeSpecName, componentName, location, null, ReactPropTypesSecret);
+ } catch (ex) {
+ error = ex;
+ }
+ warning(!error || error instanceof Error, '%s: type specification of %s `%s` is invalid; the type checker ' + 'function must return `null` or an `Error` but returned a %s. ' + 'You may have forgotten to pass an argument to the type checker ' + 'creator (arrayOf, instanceOf, objectOf, oneOf, oneOfType, and ' + 'shape all require an argument).', componentName || 'React class', location, typeSpecName, typeof error);
+ if (error instanceof Error && !(error.message in loggedTypeFailures)) {
+ // Only monitor this failure once because there tends to be a lot of the
+ // same error.
+ loggedTypeFailures[error.message] = true;
+
+ var stack = getStack ? getStack() : '';
+
+ warning(false, 'Failed %s type: %s%s', location, error.message, stack != null ? stack : '');
+ }
+ }
+ }
+ }
+}
+
+module.exports = checkPropTypes;
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/factory.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/factory.js
new file mode 100644
index 00000000..abdf8e6d
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/factory.js
@@ -0,0 +1,19 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ */
+
+'use strict';
+
+// React 15.5 references this module, and assumes PropTypes are still callable in production.
+// Therefore we re-export development-only version with all the PropTypes checks here.
+// However if one is migrating to the `prop-types` npm library, they will go through the
+// `index.js` entry point, and it will branch depending on the environment.
+var factory = require('./factoryWithTypeCheckers');
+module.exports = function(isValidElement) {
+ // It is still allowed in 15.5.
+ var throwOnDirectAccess = false;
+ return factory(isValidElement, throwOnDirectAccess);
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/factoryWithThrowingShims.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/factoryWithThrowingShims.js
new file mode 100644
index 00000000..2b3c9242
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/factoryWithThrowingShims.js
@@ -0,0 +1,58 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ */
+
+'use strict';
+
+var emptyFunction = require('fbjs/lib/emptyFunction');
+var invariant = require('fbjs/lib/invariant');
+var ReactPropTypesSecret = require('./lib/ReactPropTypesSecret');
+
+module.exports = function() {
+ function shim(props, propName, componentName, location, propFullName, secret) {
+ if (secret === ReactPropTypesSecret) {
+ // It is still safe when called from React.
+ return;
+ }
+ invariant(
+ false,
+ 'Calling PropTypes validators directly is not supported by the `prop-types` package. ' +
+ 'Use PropTypes.checkPropTypes() to call them. ' +
+ 'Read more at http://fb.me/use-check-prop-types'
+ );
+ };
+ shim.isRequired = shim;
+ function getShim() {
+ return shim;
+ };
+ // Important!
+ // Keep this list in sync with production version in `./factoryWithTypeCheckers.js`.
+ var ReactPropTypes = {
+ array: shim,
+ bool: shim,
+ func: shim,
+ number: shim,
+ object: shim,
+ string: shim,
+ symbol: shim,
+
+ any: shim,
+ arrayOf: getShim,
+ element: shim,
+ instanceOf: getShim,
+ node: shim,
+ objectOf: getShim,
+ oneOf: getShim,
+ oneOfType: getShim,
+ shape: getShim,
+ exact: getShim
+ };
+
+ ReactPropTypes.checkPropTypes = emptyFunction;
+ ReactPropTypes.PropTypes = ReactPropTypes;
+
+ return ReactPropTypes;
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/factoryWithTypeCheckers.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/factoryWithTypeCheckers.js
new file mode 100644
index 00000000..7c962b16
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/factoryWithTypeCheckers.js
@@ -0,0 +1,542 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ */
+
+'use strict';
+
+var emptyFunction = require('fbjs/lib/emptyFunction');
+var invariant = require('fbjs/lib/invariant');
+var warning = require('fbjs/lib/warning');
+var assign = require('object-assign');
+
+var ReactPropTypesSecret = require('./lib/ReactPropTypesSecret');
+var checkPropTypes = require('./checkPropTypes');
+
+module.exports = function(isValidElement, throwOnDirectAccess) {
+ /* global Symbol */
+ var ITERATOR_SYMBOL = typeof Symbol === 'function' && Symbol.iterator;
+ var FAUX_ITERATOR_SYMBOL = '@@iterator'; // Before Symbol spec.
+
+ /**
+ * Returns the iterator method function contained on the iterable object.
+ *
+ * Be sure to invoke the function with the iterable as context:
+ *
+ * var iteratorFn = getIteratorFn(myIterable);
+ * if (iteratorFn) {
+ * var iterator = iteratorFn.call(myIterable);
+ * ...
+ * }
+ *
+ * @param {?object} maybeIterable
+ * @return {?function}
+ */
+ function getIteratorFn(maybeIterable) {
+ var iteratorFn = maybeIterable && (ITERATOR_SYMBOL && maybeIterable[ITERATOR_SYMBOL] || maybeIterable[FAUX_ITERATOR_SYMBOL]);
+ if (typeof iteratorFn === 'function') {
+ return iteratorFn;
+ }
+ }
+
+ /**
+ * Collection of methods that allow declaration and validation of props that are
+ * supplied to React components. Example usage:
+ *
+ * var Props = require('ReactPropTypes');
+ * var MyArticle = React.createClass({
+ * propTypes: {
+ * // An optional string prop named "description".
+ * description: Props.string,
+ *
+ * // A required enum prop named "category".
+ * category: Props.oneOf(['News','Photos']).isRequired,
+ *
+ * // A prop named "dialog" that requires an instance of Dialog.
+ * dialog: Props.instanceOf(Dialog).isRequired
+ * },
+ * render: function() { ... }
+ * });
+ *
+ * A more formal specification of how these methods are used:
+ *
+ * type := array|bool|func|object|number|string|oneOf([...])|instanceOf(...)
+ * decl := ReactPropTypes.{type}(.isRequired)?
+ *
+ * Each and every declaration produces a function with the same signature. This
+ * allows the creation of custom validation functions. For example:
+ *
+ * var MyLink = React.createClass({
+ * propTypes: {
+ * // An optional string or URI prop named "href".
+ * href: function(props, propName, componentName) {
+ * var propValue = props[propName];
+ * if (propValue != null && typeof propValue !== 'string' &&
+ * !(propValue instanceof URI)) {
+ * return new Error(
+ * 'Expected a string or an URI for ' + propName + ' in ' +
+ * componentName
+ * );
+ * }
+ * }
+ * },
+ * render: function() {...}
+ * });
+ *
+ * @internal
+ */
+
+ var ANONYMOUS = '<>';
+
+ // Important!
+ // Keep this list in sync with production version in `./factoryWithThrowingShims.js`.
+ var ReactPropTypes = {
+ array: createPrimitiveTypeChecker('array'),
+ bool: createPrimitiveTypeChecker('boolean'),
+ func: createPrimitiveTypeChecker('function'),
+ number: createPrimitiveTypeChecker('number'),
+ object: createPrimitiveTypeChecker('object'),
+ string: createPrimitiveTypeChecker('string'),
+ symbol: createPrimitiveTypeChecker('symbol'),
+
+ any: createAnyTypeChecker(),
+ arrayOf: createArrayOfTypeChecker,
+ element: createElementTypeChecker(),
+ instanceOf: createInstanceTypeChecker,
+ node: createNodeChecker(),
+ objectOf: createObjectOfTypeChecker,
+ oneOf: createEnumTypeChecker,
+ oneOfType: createUnionTypeChecker,
+ shape: createShapeTypeChecker,
+ exact: createStrictShapeTypeChecker,
+ };
+
+ /**
+ * inlined Object.is polyfill to avoid requiring consumers ship their own
+ * https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/is
+ */
+ /*eslint-disable no-self-compare*/
+ function is(x, y) {
+ // SameValue algorithm
+ if (x === y) {
+ // Steps 1-5, 7-10
+ // Steps 6.b-6.e: +0 != -0
+ return x !== 0 || 1 / x === 1 / y;
+ } else {
+ // Step 6.a: NaN == NaN
+ return x !== x && y !== y;
+ }
+ }
+ /*eslint-enable no-self-compare*/
+
+ /**
+ * We use an Error-like object for backward compatibility as people may call
+ * PropTypes directly and inspect their output. However, we don't use real
+ * Errors anymore. We don't inspect their stack anyway, and creating them
+ * is prohibitively expensive if they are created too often, such as what
+ * happens in oneOfType() for any type before the one that matched.
+ */
+ function PropTypeError(message) {
+ this.message = message;
+ this.stack = '';
+ }
+ // Make `instanceof Error` still work for returned errors.
+ PropTypeError.prototype = Error.prototype;
+
+ function createChainableTypeChecker(validate) {
+ if (process.env.NODE_ENV !== 'production') {
+ var manualPropTypeCallCache = {};
+ var manualPropTypeWarningCount = 0;
+ }
+ function checkType(isRequired, props, propName, componentName, location, propFullName, secret) {
+ componentName = componentName || ANONYMOUS;
+ propFullName = propFullName || propName;
+
+ if (secret !== ReactPropTypesSecret) {
+ if (throwOnDirectAccess) {
+ // New behavior only for users of `prop-types` package
+ invariant(
+ false,
+ 'Calling PropTypes validators directly is not supported by the `prop-types` package. ' +
+ 'Use `PropTypes.checkPropTypes()` to call them. ' +
+ 'Read more at http://fb.me/use-check-prop-types'
+ );
+ } else if (process.env.NODE_ENV !== 'production' && typeof console !== 'undefined') {
+ // Old behavior for people using React.PropTypes
+ var cacheKey = componentName + ':' + propName;
+ if (
+ !manualPropTypeCallCache[cacheKey] &&
+ // Avoid spamming the console because they are often not actionable except for lib authors
+ manualPropTypeWarningCount < 3
+ ) {
+ warning(
+ false,
+ 'You are manually calling a React.PropTypes validation ' +
+ 'function for the `%s` prop on `%s`. This is deprecated ' +
+ 'and will throw in the standalone `prop-types` package. ' +
+ 'You may be seeing this warning due to a third-party PropTypes ' +
+ 'library. See https://fb.me/react-warning-dont-call-proptypes ' + 'for details.',
+ propFullName,
+ componentName
+ );
+ manualPropTypeCallCache[cacheKey] = true;
+ manualPropTypeWarningCount++;
+ }
+ }
+ }
+ if (props[propName] == null) {
+ if (isRequired) {
+ if (props[propName] === null) {
+ return new PropTypeError('The ' + location + ' `' + propFullName + '` is marked as required ' + ('in `' + componentName + '`, but its value is `null`.'));
+ }
+ return new PropTypeError('The ' + location + ' `' + propFullName + '` is marked as required in ' + ('`' + componentName + '`, but its value is `undefined`.'));
+ }
+ return null;
+ } else {
+ return validate(props, propName, componentName, location, propFullName);
+ }
+ }
+
+ var chainedCheckType = checkType.bind(null, false);
+ chainedCheckType.isRequired = checkType.bind(null, true);
+
+ return chainedCheckType;
+ }
+
+ function createPrimitiveTypeChecker(expectedType) {
+ function validate(props, propName, componentName, location, propFullName, secret) {
+ var propValue = props[propName];
+ var propType = getPropType(propValue);
+ if (propType !== expectedType) {
+ // `propValue` being instance of, say, date/regexp, pass the 'object'
+ // check, but we can offer a more precise error message here rather than
+ // 'of type `object`'.
+ var preciseType = getPreciseType(propValue);
+
+ return new PropTypeError('Invalid ' + location + ' `' + propFullName + '` of type ' + ('`' + preciseType + '` supplied to `' + componentName + '`, expected ') + ('`' + expectedType + '`.'));
+ }
+ return null;
+ }
+ return createChainableTypeChecker(validate);
+ }
+
+ function createAnyTypeChecker() {
+ return createChainableTypeChecker(emptyFunction.thatReturnsNull);
+ }
+
+ function createArrayOfTypeChecker(typeChecker) {
+ function validate(props, propName, componentName, location, propFullName) {
+ if (typeof typeChecker !== 'function') {
+ return new PropTypeError('Property `' + propFullName + '` of component `' + componentName + '` has invalid PropType notation inside arrayOf.');
+ }
+ var propValue = props[propName];
+ if (!Array.isArray(propValue)) {
+ var propType = getPropType(propValue);
+ return new PropTypeError('Invalid ' + location + ' `' + propFullName + '` of type ' + ('`' + propType + '` supplied to `' + componentName + '`, expected an array.'));
+ }
+ for (var i = 0; i < propValue.length; i++) {
+ var error = typeChecker(propValue, i, componentName, location, propFullName + '[' + i + ']', ReactPropTypesSecret);
+ if (error instanceof Error) {
+ return error;
+ }
+ }
+ return null;
+ }
+ return createChainableTypeChecker(validate);
+ }
+
+ function createElementTypeChecker() {
+ function validate(props, propName, componentName, location, propFullName) {
+ var propValue = props[propName];
+ if (!isValidElement(propValue)) {
+ var propType = getPropType(propValue);
+ return new PropTypeError('Invalid ' + location + ' `' + propFullName + '` of type ' + ('`' + propType + '` supplied to `' + componentName + '`, expected a single ReactElement.'));
+ }
+ return null;
+ }
+ return createChainableTypeChecker(validate);
+ }
+
+ function createInstanceTypeChecker(expectedClass) {
+ function validate(props, propName, componentName, location, propFullName) {
+ if (!(props[propName] instanceof expectedClass)) {
+ var expectedClassName = expectedClass.name || ANONYMOUS;
+ var actualClassName = getClassName(props[propName]);
+ return new PropTypeError('Invalid ' + location + ' `' + propFullName + '` of type ' + ('`' + actualClassName + '` supplied to `' + componentName + '`, expected ') + ('instance of `' + expectedClassName + '`.'));
+ }
+ return null;
+ }
+ return createChainableTypeChecker(validate);
+ }
+
+ function createEnumTypeChecker(expectedValues) {
+ if (!Array.isArray(expectedValues)) {
+ process.env.NODE_ENV !== 'production' ? warning(false, 'Invalid argument supplied to oneOf, expected an instance of array.') : void 0;
+ return emptyFunction.thatReturnsNull;
+ }
+
+ function validate(props, propName, componentName, location, propFullName) {
+ var propValue = props[propName];
+ for (var i = 0; i < expectedValues.length; i++) {
+ if (is(propValue, expectedValues[i])) {
+ return null;
+ }
+ }
+
+ var valuesString = JSON.stringify(expectedValues);
+ return new PropTypeError('Invalid ' + location + ' `' + propFullName + '` of value `' + propValue + '` ' + ('supplied to `' + componentName + '`, expected one of ' + valuesString + '.'));
+ }
+ return createChainableTypeChecker(validate);
+ }
+
+ function createObjectOfTypeChecker(typeChecker) {
+ function validate(props, propName, componentName, location, propFullName) {
+ if (typeof typeChecker !== 'function') {
+ return new PropTypeError('Property `' + propFullName + '` of component `' + componentName + '` has invalid PropType notation inside objectOf.');
+ }
+ var propValue = props[propName];
+ var propType = getPropType(propValue);
+ if (propType !== 'object') {
+ return new PropTypeError('Invalid ' + location + ' `' + propFullName + '` of type ' + ('`' + propType + '` supplied to `' + componentName + '`, expected an object.'));
+ }
+ for (var key in propValue) {
+ if (propValue.hasOwnProperty(key)) {
+ var error = typeChecker(propValue, key, componentName, location, propFullName + '.' + key, ReactPropTypesSecret);
+ if (error instanceof Error) {
+ return error;
+ }
+ }
+ }
+ return null;
+ }
+ return createChainableTypeChecker(validate);
+ }
+
+ function createUnionTypeChecker(arrayOfTypeCheckers) {
+ if (!Array.isArray(arrayOfTypeCheckers)) {
+ process.env.NODE_ENV !== 'production' ? warning(false, 'Invalid argument supplied to oneOfType, expected an instance of array.') : void 0;
+ return emptyFunction.thatReturnsNull;
+ }
+
+ for (var i = 0; i < arrayOfTypeCheckers.length; i++) {
+ var checker = arrayOfTypeCheckers[i];
+ if (typeof checker !== 'function') {
+ warning(
+ false,
+ 'Invalid argument supplied to oneOfType. Expected an array of check functions, but ' +
+ 'received %s at index %s.',
+ getPostfixForTypeWarning(checker),
+ i
+ );
+ return emptyFunction.thatReturnsNull;
+ }
+ }
+
+ function validate(props, propName, componentName, location, propFullName) {
+ for (var i = 0; i < arrayOfTypeCheckers.length; i++) {
+ var checker = arrayOfTypeCheckers[i];
+ if (checker(props, propName, componentName, location, propFullName, ReactPropTypesSecret) == null) {
+ return null;
+ }
+ }
+
+ return new PropTypeError('Invalid ' + location + ' `' + propFullName + '` supplied to ' + ('`' + componentName + '`.'));
+ }
+ return createChainableTypeChecker(validate);
+ }
+
+ function createNodeChecker() {
+ function validate(props, propName, componentName, location, propFullName) {
+ if (!isNode(props[propName])) {
+ return new PropTypeError('Invalid ' + location + ' `' + propFullName + '` supplied to ' + ('`' + componentName + '`, expected a ReactNode.'));
+ }
+ return null;
+ }
+ return createChainableTypeChecker(validate);
+ }
+
+ function createShapeTypeChecker(shapeTypes) {
+ function validate(props, propName, componentName, location, propFullName) {
+ var propValue = props[propName];
+ var propType = getPropType(propValue);
+ if (propType !== 'object') {
+ return new PropTypeError('Invalid ' + location + ' `' + propFullName + '` of type `' + propType + '` ' + ('supplied to `' + componentName + '`, expected `object`.'));
+ }
+ for (var key in shapeTypes) {
+ var checker = shapeTypes[key];
+ if (!checker) {
+ continue;
+ }
+ var error = checker(propValue, key, componentName, location, propFullName + '.' + key, ReactPropTypesSecret);
+ if (error) {
+ return error;
+ }
+ }
+ return null;
+ }
+ return createChainableTypeChecker(validate);
+ }
+
+ function createStrictShapeTypeChecker(shapeTypes) {
+ function validate(props, propName, componentName, location, propFullName) {
+ var propValue = props[propName];
+ var propType = getPropType(propValue);
+ if (propType !== 'object') {
+ return new PropTypeError('Invalid ' + location + ' `' + propFullName + '` of type `' + propType + '` ' + ('supplied to `' + componentName + '`, expected `object`.'));
+ }
+ // We need to check all keys in case some are required but missing from
+ // props.
+ var allKeys = assign({}, props[propName], shapeTypes);
+ for (var key in allKeys) {
+ var checker = shapeTypes[key];
+ if (!checker) {
+ return new PropTypeError(
+ 'Invalid ' + location + ' `' + propFullName + '` key `' + key + '` supplied to `' + componentName + '`.' +
+ '\nBad object: ' + JSON.stringify(props[propName], null, ' ') +
+ '\nValid keys: ' + JSON.stringify(Object.keys(shapeTypes), null, ' ')
+ );
+ }
+ var error = checker(propValue, key, componentName, location, propFullName + '.' + key, ReactPropTypesSecret);
+ if (error) {
+ return error;
+ }
+ }
+ return null;
+ }
+
+ return createChainableTypeChecker(validate);
+ }
+
+ function isNode(propValue) {
+ switch (typeof propValue) {
+ case 'number':
+ case 'string':
+ case 'undefined':
+ return true;
+ case 'boolean':
+ return !propValue;
+ case 'object':
+ if (Array.isArray(propValue)) {
+ return propValue.every(isNode);
+ }
+ if (propValue === null || isValidElement(propValue)) {
+ return true;
+ }
+
+ var iteratorFn = getIteratorFn(propValue);
+ if (iteratorFn) {
+ var iterator = iteratorFn.call(propValue);
+ var step;
+ if (iteratorFn !== propValue.entries) {
+ while (!(step = iterator.next()).done) {
+ if (!isNode(step.value)) {
+ return false;
+ }
+ }
+ } else {
+ // Iterator will provide entry [k,v] tuples rather than values.
+ while (!(step = iterator.next()).done) {
+ var entry = step.value;
+ if (entry) {
+ if (!isNode(entry[1])) {
+ return false;
+ }
+ }
+ }
+ }
+ } else {
+ return false;
+ }
+
+ return true;
+ default:
+ return false;
+ }
+ }
+
+ function isSymbol(propType, propValue) {
+ // Native Symbol.
+ if (propType === 'symbol') {
+ return true;
+ }
+
+ // 19.4.3.5 Symbol.prototype[@@toStringTag] === 'Symbol'
+ if (propValue['@@toStringTag'] === 'Symbol') {
+ return true;
+ }
+
+ // Fallback for non-spec compliant Symbols which are polyfilled.
+ if (typeof Symbol === 'function' && propValue instanceof Symbol) {
+ return true;
+ }
+
+ return false;
+ }
+
+ // Equivalent of `typeof` but with special handling for array and regexp.
+ function getPropType(propValue) {
+ var propType = typeof propValue;
+ if (Array.isArray(propValue)) {
+ return 'array';
+ }
+ if (propValue instanceof RegExp) {
+ // Old webkits (at least until Android 4.0) return 'function' rather than
+ // 'object' for typeof a RegExp. We'll normalize this here so that /bla/
+ // passes PropTypes.object.
+ return 'object';
+ }
+ if (isSymbol(propType, propValue)) {
+ return 'symbol';
+ }
+ return propType;
+ }
+
+ // This handles more types than `getPropType`. Only used for error messages.
+ // See `createPrimitiveTypeChecker`.
+ function getPreciseType(propValue) {
+ if (typeof propValue === 'undefined' || propValue === null) {
+ return '' + propValue;
+ }
+ var propType = getPropType(propValue);
+ if (propType === 'object') {
+ if (propValue instanceof Date) {
+ return 'date';
+ } else if (propValue instanceof RegExp) {
+ return 'regexp';
+ }
+ }
+ return propType;
+ }
+
+ // Returns a string that is postfixed to a warning about an invalid type.
+ // For example, "undefined" or "of type array"
+ function getPostfixForTypeWarning(value) {
+ var type = getPreciseType(value);
+ switch (type) {
+ case 'array':
+ case 'object':
+ return 'an ' + type;
+ case 'boolean':
+ case 'date':
+ case 'regexp':
+ return 'a ' + type;
+ default:
+ return type;
+ }
+ }
+
+ // Returns class name of the object, if any.
+ function getClassName(propValue) {
+ if (!propValue.constructor || !propValue.constructor.name) {
+ return ANONYMOUS;
+ }
+ return propValue.constructor.name;
+ }
+
+ ReactPropTypes.checkPropTypes = checkPropTypes;
+ ReactPropTypes.PropTypes = ReactPropTypes;
+
+ return ReactPropTypes;
+};
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/index.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/index.js
new file mode 100644
index 00000000..11bfceab
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/index.js
@@ -0,0 +1,28 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ */
+
+if (process.env.NODE_ENV !== 'production') {
+ var REACT_ELEMENT_TYPE = (typeof Symbol === 'function' &&
+ Symbol.for &&
+ Symbol.for('react.element')) ||
+ 0xeac7;
+
+ var isValidElement = function(object) {
+ return typeof object === 'object' &&
+ object !== null &&
+ object.$$typeof === REACT_ELEMENT_TYPE;
+ };
+
+ // By explicitly using `prop-types` you are opting into new development behavior.
+ // http://fb.me/prop-types-in-prod
+ var throwOnDirectAccess = true;
+ module.exports = require('./factoryWithTypeCheckers')(isValidElement, throwOnDirectAccess);
+} else {
+ // By explicitly using `prop-types` you are opting into new production behavior.
+ // http://fb.me/prop-types-in-prod
+ module.exports = require('./factoryWithThrowingShims')();
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/lib/ReactPropTypesSecret.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/lib/ReactPropTypesSecret.js
new file mode 100644
index 00000000..f54525e7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/lib/ReactPropTypesSecret.js
@@ -0,0 +1,12 @@
+/**
+ * Copyright (c) 2013-present, Facebook, Inc.
+ *
+ * This source code is licensed under the MIT license found in the
+ * LICENSE file in the root directory of this source tree.
+ */
+
+'use strict';
+
+var ReactPropTypesSecret = 'SECRET_DO_NOT_PASS_THIS_OR_YOU_WILL_BE_FIRED';
+
+module.exports = ReactPropTypesSecret;
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/package.json b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/package.json
new file mode 100644
index 00000000..044e36d0
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/package.json
@@ -0,0 +1,83 @@
+{
+ "_from": "prop-types@^15.5.7",
+ "_id": "prop-types@15.6.0",
+ "_inBundle": false,
+ "_integrity": "sha1-zq8IMCL8RrSjX2nhPvda7Q1jmFY=",
+ "_location": "/react-dropzone/prop-types",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "prop-types@^15.5.7",
+ "name": "prop-types",
+ "escapedName": "prop-types",
+ "rawSpec": "^15.5.7",
+ "saveSpec": null,
+ "fetchSpec": "^15.5.7"
+ },
+ "_requiredBy": [
+ "/react-dropzone"
+ ],
+ "_resolved": "https://registry.npmjs.org/prop-types/-/prop-types-15.6.0.tgz",
+ "_shasum": "ceaf083022fc46b4a35f69e13ef75aed0d639856",
+ "_spec": "prop-types@^15.5.7",
+ "_where": "C:\\Users\\deranjer\\go\\src\\github.com\\deranjer\\goTorrent\\goTorrentWebUI\\node_modules\\react-dropzone",
+ "browserify": {
+ "transform": [
+ "loose-envify"
+ ]
+ },
+ "bugs": {
+ "url": "https://github.com/reactjs/prop-types/issues"
+ },
+ "bundleDependencies": false,
+ "dependencies": {
+ "fbjs": "^0.8.16",
+ "loose-envify": "^1.3.1",
+ "object-assign": "^4.1.1"
+ },
+ "deprecated": false,
+ "description": "Runtime type checking for React props and similar objects.",
+ "devDependencies": {
+ "babel-jest": "^19.0.0",
+ "babel-preset-react": "^6.24.1",
+ "browserify": "^14.3.0",
+ "bundle-collapser": "^1.2.1",
+ "envify": "^4.0.0",
+ "jest": "^19.0.2",
+ "react": "^15.5.1",
+ "uglifyify": "^3.0.4",
+ "uglifyjs": "^2.4.10"
+ },
+ "files": [
+ "LICENSE",
+ "README.md",
+ "checkPropTypes.js",
+ "factory.js",
+ "factoryWithThrowingShims.js",
+ "factoryWithTypeCheckers.js",
+ "index.js",
+ "prop-types.js",
+ "prop-types.min.js",
+ "lib"
+ ],
+ "homepage": "https://facebook.github.io/react/",
+ "keywords": [
+ "react"
+ ],
+ "license": "MIT",
+ "main": "index.js",
+ "name": "prop-types",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/reactjs/prop-types.git"
+ },
+ "scripts": {
+ "build": "yarn umd && yarn umd-min",
+ "prepublish": "yarn build",
+ "test": "jest",
+ "umd": "NODE_ENV=development browserify index.js -t envify --standalone PropTypes -o prop-types.js",
+ "umd-min": "NODE_ENV=production browserify index.js -t envify -t uglifyify --standalone PropTypes -p bundle-collapser/plugin -o | uglifyjs --compress unused,dead_code -o prop-types.min.js"
+ },
+ "version": "15.6.0"
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/prop-types.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/prop-types.js
new file mode 100644
index 00000000..ba55170c
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/prop-types.js
@@ -0,0 +1,965 @@
+(function(f){if(typeof exports==="object"&&typeof module!=="undefined"){module.exports=f()}else if(typeof define==="function"&&define.amd){define([],f)}else{var g;if(typeof window!=="undefined"){g=window}else if(typeof global!=="undefined"){g=global}else if(typeof self!=="undefined"){g=self}else{g=this}g.PropTypes = f()}})(function(){var define,module,exports;return (function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a=typeof require=="function"&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}var i=typeof require=="function"&&require;for(var o=0;o 1 ? _len - 1 : 0), _key = 1; _key < _len; _key++) {
+ args[_key - 1] = arguments[_key];
+ }
+
+ var argIndex = 0;
+ var message = 'Warning: ' + format.replace(/%s/g, function () {
+ return args[argIndex++];
+ });
+ if (typeof console !== 'undefined') {
+ console.error(message);
+ }
+ try {
+ // --- Welcome to debugging React ---
+ // This error was thrown as a convenience so that you can use this stack
+ // to find the callsite that caused this warning to fire.
+ throw new Error(message);
+ } catch (x) {}
+ };
+
+ warning = function warning(condition, format) {
+ if (format === undefined) {
+ throw new Error('`warning(condition, format, ...args)` requires a warning ' + 'message argument');
+ }
+
+ if (format.indexOf('Failed Composite propType: ') === 0) {
+ return; // Ignore CompositeComponent proptype check.
+ }
+
+ if (!condition) {
+ for (var _len2 = arguments.length, args = Array(_len2 > 2 ? _len2 - 2 : 0), _key2 = 2; _key2 < _len2; _key2++) {
+ args[_key2 - 2] = arguments[_key2];
+ }
+
+ printWarning.apply(undefined, [format].concat(args));
+ }
+ };
+ })();
+}
+
+module.exports = warning;
+},{"./emptyFunction":6}],9:[function(require,module,exports){
+/*
+object-assign
+(c) Sindre Sorhus
+@license MIT
+*/
+
+'use strict';
+/* eslint-disable no-unused-vars */
+var getOwnPropertySymbols = Object.getOwnPropertySymbols;
+var hasOwnProperty = Object.prototype.hasOwnProperty;
+var propIsEnumerable = Object.prototype.propertyIsEnumerable;
+
+function toObject(val) {
+ if (val === null || val === undefined) {
+ throw new TypeError('Object.assign cannot be called with null or undefined');
+ }
+
+ return Object(val);
+}
+
+function shouldUseNative() {
+ try {
+ if (!Object.assign) {
+ return false;
+ }
+
+ // Detect buggy property enumeration order in older V8 versions.
+
+ // https://bugs.chromium.org/p/v8/issues/detail?id=4118
+ var test1 = new String('abc'); // eslint-disable-line no-new-wrappers
+ test1[5] = 'de';
+ if (Object.getOwnPropertyNames(test1)[0] === '5') {
+ return false;
+ }
+
+ // https://bugs.chromium.org/p/v8/issues/detail?id=3056
+ var test2 = {};
+ for (var i = 0; i < 10; i++) {
+ test2['_' + String.fromCharCode(i)] = i;
+ }
+ var order2 = Object.getOwnPropertyNames(test2).map(function (n) {
+ return test2[n];
+ });
+ if (order2.join('') !== '0123456789') {
+ return false;
+ }
+
+ // https://bugs.chromium.org/p/v8/issues/detail?id=3056
+ var test3 = {};
+ 'abcdefghijklmnopqrst'.split('').forEach(function (letter) {
+ test3[letter] = letter;
+ });
+ if (Object.keys(Object.assign({}, test3)).join('') !==
+ 'abcdefghijklmnopqrst') {
+ return false;
+ }
+
+ return true;
+ } catch (err) {
+ // We don't expect any of the above to throw, but better to be safe.
+ return false;
+ }
+}
+
+module.exports = shouldUseNative() ? Object.assign : function (target, source) {
+ var from;
+ var to = toObject(target);
+ var symbols;
+
+ for (var s = 1; s < arguments.length; s++) {
+ from = Object(arguments[s]);
+
+ for (var key in from) {
+ if (hasOwnProperty.call(from, key)) {
+ to[key] = from[key];
+ }
+ }
+
+ if (getOwnPropertySymbols) {
+ symbols = getOwnPropertySymbols(from);
+ for (var i = 0; i < symbols.length; i++) {
+ if (propIsEnumerable.call(from, symbols[i])) {
+ to[symbols[i]] = from[symbols[i]];
+ }
+ }
+ }
+ }
+
+ return to;
+};
+
+},{}]},{},[4])(4)
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/prop-types.min.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/prop-types.min.js
new file mode 100644
index 00000000..71af27b7
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/prop-types/prop-types.min.js
@@ -0,0 +1 @@
+!function(f){if("object"==typeof exports&&"undefined"!=typeof module)module.exports=f();else if("function"==typeof define&&define.amd)define([],f);else{var g;g="undefined"!=typeof window?window:"undefined"!=typeof global?global:"undefined"!=typeof self?self:this,g.PropTypes=f()}}(function(){return function e(t,n,r){function s(o,u){if(!n[o]){if(!t[o]){var a="function"==typeof require&&require;if(!u&&a)return a(o,!0);if(i)return i(o,!0);var f=new Error("Cannot find module '"+o+"'");throw f.code="MODULE_NOT_FOUND",f}var l=n[o]={exports:{}};t[o][0].call(l.exports,function(e){var n=t[o][1][e];return s(n?n:e)},l,l.exports,e,t,n,r)}return n[o].exports}for(var i="function"==typeof require&&require,o=0;o element; its readystatechange event will be fired asynchronously once it is inserted
+ // into the document. Do so, thus queuing up the task. Remember to clean up once it's been called.
+ var script = doc.createElement("script");
+ script.onreadystatechange = function () {
+ runIfPresent(handle);
+ script.onreadystatechange = null;
+ html.removeChild(script);
+ script = null;
+ };
+ html.appendChild(script);
+ };
+ }
+
+ function installSetTimeoutImplementation() {
+ registerImmediate = function(handle) {
+ setTimeout(runIfPresent, 0, handle);
+ };
+ }
+
+ // If supported, we should attach to the prototype of global, since that is where setTimeout et al. live.
+ var attachTo = Object.getPrototypeOf && Object.getPrototypeOf(global);
+ attachTo = attachTo && attachTo.setTimeout ? attachTo : global;
+
+ // Don't get fooled by e.g. browserify environments.
+ if ({}.toString.call(global.process) === "[object process]") {
+ // For Node.js before 0.9
+ installNextTickImplementation();
+
+ } else if (canUsePostMessage()) {
+ // For non-IE10 modern browsers
+ installPostMessageImplementation();
+
+ } else if (global.MessageChannel) {
+ // For web workers, where supported
+ installMessageChannelImplementation();
+
+ } else if (doc && "onreadystatechange" in doc.createElement("script")) {
+ // For IE 6–8
+ installReadyStateChangeImplementation();
+
+ } else {
+ // For older browsers
+ installSetTimeoutImplementation();
+ }
+
+ attachTo.setImmediate = setImmediate;
+ attachTo.clearImmediate = clearImmediate;
+}(typeof self === "undefined" ? typeof global === "undefined" ? this : global : self));
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/.npmignore b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/.npmignore
new file mode 100644
index 00000000..154d1f06
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/.npmignore
@@ -0,0 +1,24 @@
+node_modules/
+npm-debug.log
+### vim ###
+.*.s[a-w][a-z]
+*.un~
+Session.vim
+.netrwhist
+*~
+.versions
+
+### OSX ###
+.DS_Store
+.AppleDouble
+.LSOverride
+Icon
+
+
+# Thumbnails
+._*
+
+# Files that might appear on external disk
+.Spotlight-V100
+.Trashes
+.idea
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/.travis.yml b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/.travis.yml
new file mode 100644
index 00000000..4d23aa06
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/.travis.yml
@@ -0,0 +1,13 @@
+language: node_js
+node_js:
+ - stable
+ - "0.10"
+
+notifications:
+ email: false
+
+cache:
+ directories:
+ - node_modules
+
+sudo: false
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/bower.json b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/bower.json
new file mode 100644
index 00000000..69e9aaa8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/bower.json
@@ -0,0 +1,17 @@
+{
+ "name": "ua-parser-js",
+ "version": "0.7.17",
+ "authors": [
+ "Faisal Salman "
+ ],
+ "private": false,
+ "main": "src/ua-parser.js",
+ "ignore": [
+ "build",
+ "node_modules",
+ "bower_components",
+ "test",
+ "tests"
+ ],
+ "dependencies": {}
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/dist/ua-parser.html b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/dist/ua-parser.html
new file mode 100644
index 00000000..1e530bb1
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/dist/ua-parser.html
@@ -0,0 +1 @@
+
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/dist/ua-parser.min.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/dist/ua-parser.min.js
new file mode 100644
index 00000000..49d763e1
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/dist/ua-parser.min.js
@@ -0,0 +1,9 @@
+/**
+ * UAParser.js v0.7.17
+ * Lightweight JavaScript-based User-Agent string parser
+ * https://github.com/faisalman/ua-parser-js
+ *
+ * Copyright © 2012-2016 Faisal Salman
+ * Dual licensed under GPLv2 & MIT
+ */
+(function(window,undefined){"use strict";var LIBVERSION="0.7.17",EMPTY="",UNKNOWN="?",FUNC_TYPE="function",UNDEF_TYPE="undefined",OBJ_TYPE="object",STR_TYPE="string",MAJOR="major",MODEL="model",NAME="name",TYPE="type",VENDOR="vendor",VERSION="version",ARCHITECTURE="architecture",CONSOLE="console",MOBILE="mobile",TABLET="tablet",SMARTTV="smarttv",WEARABLE="wearable",EMBEDDED="embedded";var util={extend:function(regexes,extensions){var margedRegexes={};for(var i in regexes){if(extensions[i]&&extensions[i].length%2===0){margedRegexes[i]=extensions[i].concat(regexes[i])}else{margedRegexes[i]=regexes[i]}}return margedRegexes},has:function(str1,str2){if(typeof str1==="string"){return str2.toLowerCase().indexOf(str1.toLowerCase())!==-1}else{return false}},lowerize:function(str){return str.toLowerCase()},major:function(version){return typeof version===STR_TYPE?version.replace(/[^\d\.]/g,"").split(".")[0]:undefined},trim:function(str){return str.replace(/^[\s\uFEFF\xA0]+|[\s\uFEFF\xA0]+$/g,"")}};var mapper={rgx:function(ua,arrays){var i=0,j,k,p,q,matches,match;while(i0){if(q.length==2){if(typeof q[1]==FUNC_TYPE){this[q[0]]=q[1].call(this,match)}else{this[q[0]]=q[1]}}else if(q.length==3){if(typeof q[1]===FUNC_TYPE&&!(q[1].exec&&q[1].test)){this[q[0]]=match?q[1].call(this,match,q[2]):undefined}else{this[q[0]]=match?match.replace(q[1],q[2]):undefined}}else if(q.length==4){this[q[0]]=match?q[3].call(this,match.replace(q[1],q[2])):undefined}}else{this[q]=match?match:undefined}}}}i+=2}},str:function(str,map){for(var i in map){if(typeof map[i]===OBJ_TYPE&&map[i].length>0){for(var j=0;j
+ * Dual licensed under GPLv2 & MIT
+ */
+!function(i,s){"use strict";var e="0.7.17",o="",r="?",n="function",a="undefined",d="object",t="string",l="major",w="model",u="name",c="type",m="vendor",b="version",p="architecture",g="console",f="mobile",h="tablet",v="smarttv",x="wearable",k="embedded",y={extend:function(i,s){var e={};for(var o in i)s[o]&&s[o].length%2===0?e[o]=s[o].concat(i[o]):e[o]=i[o];return e},has:function(i,s){return"string"==typeof i&&s.toLowerCase().indexOf(i.toLowerCase())!==-1},lowerize:function(i){return i.toLowerCase()},major:function(i){return typeof i===t?i.replace(/[^\d\.]/g,"").split(".")[0]:s},trim:function(i){return i.replace(/^[\s\uFEFF\xA0]+|[\s\uFEFF\xA0]+$/g,"")}},T={rgx:function(i,e){for(var o,r,a,t,l,w,u=0;u0?2==t.length?typeof t[1]==n?this[t[0]]=t[1].call(this,w):this[t[0]]=t[1]:3==t.length?typeof t[1]!==n||t[1].exec&&t[1].test?this[t[0]]=w?w.replace(t[1],t[2]):s:this[t[0]]=w?t[1].call(this,w,t[2]):s:4==t.length&&(this[t[0]]=w?t[3].call(this,w.replace(t[1],t[2])):s):this[t]=w?w:s;u+=2}},str:function(i,e){for(var o in e)if(typeof e[o]===d&&e[o].length>0){for(var n=0;n A JavaScript-based User-Agent string parser. Can be used either in browser (client-side) or in node.js (server-side) environment. Also available as jQuery/Zepto plugin, Bower/Meteor package, & RequireJS/AMD module. This library aims to identify detailed type of web browser, layout engine, operating system, cpu architecture, and device type/model, entirely from user-agent string with a relatively small footprint (~11KB when minified / ~4KB gzipped). Written in vanilla JavaScript, which means it doesn't require any other library and can be used independently. However, it's not recommended to use this library as browser detection since the result may not accurate than using feature detection.
+
+[](https://travis-ci.org/faisalman/ua-parser-js)
+[](https://www.npmjs.com/package/ua-parser-js)
+[](https://www.npmjs.com/package/ua-parser-js)
+[](https://bower.io/)
+[](https://cdnjs.com/libraries/UAParser.js)
+[](https://gratipay.com/UAParser.js)
+[](http://flattr.com/thing/3867907/faisalmanua-parser-js-on-GitHub)
+
+* Author : Faisal Salman <>
+* Demo : http://faisalman.github.io/ua-parser-js
+* Source : https://github.com/faisalman/ua-parser-js
+
+# Constructor
+
+* `new UAParser([uastring][,extensions])`
+ * returns new instance
+
+* `UAParser([uastring][,extensions])`
+ * returns result object `{ ua: '', browser: {}, cpu: {}, device: {}, engine: {}, os: {} }`
+
+# Methods
+
+* `getBrowser()`
+ * returns `{ name: '', version: '' }`
+
+```
+# Possible 'browser.name':
+Amaya, Android Browser, Arora, Avant, Baidu, Blazer, Bolt, Bowser, Camino, Chimera,
+Chrome [WebView], Chromium, Comodo Dragon, Conkeror, Dillo, Dolphin, Doris, Edge,
+Epiphany, Fennec, Firebird, Firefox, Flock, GoBrowser, iCab, ICE Browser, IceApe,
+IceCat, IceDragon, Iceweasel, IE[Mobile], Iron, Jasmine, K-Meleon, Konqueror, Kindle,
+Links, Lunascape, Lynx, Maemo, Maxthon, Midori, Minimo, MIUI Browser, [Mobile] Safari,
+Mosaic, Mozilla, Netfront, Netscape, NetSurf, Nokia, OmniWeb, Opera [Mini/Mobi/Tablet],
+PhantomJS, Phoenix, Polaris, QQBrowser, RockMelt, Silk, Skyfire, SeaMonkey, Sleipnir,
+SlimBrowser, Swiftfox, Tizen, UCBrowser, Vivaldi, w3m, WeChat, Yandex
+
+# 'browser.version' determined dynamically
+```
+
+* `getDevice()`
+ * returns `{ model: '', type: '', vendor: '' }`
+
+```
+# Possible 'device.type':
+console, mobile, tablet, smarttv, wearable, embedded
+
+# Possible 'device.vendor':
+Acer, Alcatel, Amazon, Apple, Archos, Asus, BenQ, BlackBerry, Dell, GeeksPhone,
+Google, HP, HTC, Huawei, Jolla, Lenovo, LG, Meizu, Microsoft, Motorola, Nexian,
+Nintendo, Nokia, Nvidia, OnePlus, Ouya, Palm, Panasonic, Pebble, Polytron, RIM,
+Samsung, Sharp, Siemens, Sony[Ericsson], Sprint, Xbox, Xiaomi, ZTE
+
+# 'device.model' determined dynamically
+```
+
+* `getEngine()`
+ * returns `{ name: '', version: '' }`
+
+```
+# Possible 'engine.name'
+Amaya, EdgeHTML, Gecko, iCab, KHTML, Links, Lynx, NetFront, NetSurf, Presto,
+Tasman, Trident, w3m, WebKit
+
+# 'engine.version' determined dynamically
+```
+
+* `getOS()`
+ * returns `{ name: '', version: '' }`
+
+```
+# Possible 'os.name'
+AIX, Amiga OS, Android, Arch, Bada, BeOS, BlackBerry, CentOS, Chromium OS, Contiki,
+Fedora, Firefox OS, FreeBSD, Debian, DragonFly, Gentoo, GNU, Haiku, Hurd, iOS,
+Joli, Linpus, Linux, Mac OS, Mageia, Mandriva, MeeGo, Minix, Mint, Morph OS, NetBSD,
+Nintendo, OpenBSD, OpenVMS, OS/2, Palm, PC-BSD, PCLinuxOS, Plan9, Playstation, QNX, RedHat,
+RIM Tablet OS, RISC OS, Sailfish, Series40, Slackware, Solaris, SUSE, Symbian, Tizen,
+Ubuntu, UNIX, VectorLinux, WebOS, Windows [Phone/Mobile], Zenwalk
+
+# 'os.version' determined dynamically
+```
+
+* `getCPU()`
+ * returns `{ architecture: '' }`
+
+```
+# Possible 'cpu.architecture'
+68k, amd64, arm[64], avr, ia[32/64], irix[64], mips[64], pa-risc, ppc, sparc[64]
+```
+
+* `getResult()`
+ * returns `{ ua: '', browser: {}, cpu: {}, device: {}, engine: {}, os: {} }`
+
+* `getUA()`
+ * returns UA string of current instance
+
+* `setUA(uastring)`
+ * set UA string to parse
+ * returns current instance
+
+
+# Example
+
+```html
+
+
+
+
+
+
+
+
+
+```
+
+## Using node.js
+
+```sh
+$ npm install ua-parser-js
+```
+
+```js
+var http = require('http');
+var parser = require('ua-parser-js');
+
+http.createServer(function (req, res) {
+ // get user-agent header
+ var ua = parser(req.headers['user-agent']);
+ // write the result as response
+ res.end(JSON.stringify(ua, null, ' '));
+})
+.listen(1337, '127.0.0.1');
+
+console.log('Server running at http://127.0.0.1:1337/');
+```
+
+## Using requirejs
+
+```js
+requirejs.config({
+ baseUrl : 'js/lib', // path to your script directory
+ paths : {
+ 'ua-parser-js' : 'ua-parser.min'
+ }
+});
+
+requirejs(['ua-parser-js'], function(UAParser) {
+ var parser = new UAParser();
+ console.log(parser.getResult());
+});
+```
+
+## Using CDN
+
+```html
+
+```
+
+## Using bower
+
+```sh
+$ bower install ua-parser-js
+```
+
+## Using meteor
+
+```sh
+$ meteor add faisalman:ua-parser-js
+```
+
+## Using CLI
+
+```sh
+$ node ua-parser.min.js "Mozilla/4.0 (compatible; MSIE 4.01; Windows 98)"
+# multiple args
+$ node ua-parser.min.js "Opera/1.2" "Opera/3.4"
+# piped args
+$ echo "Opera/1.2" | node ua-parser.min.js
+# log file
+$ cat ua.log | node ua-parser.min.js
+```
+
+## Using jQuery/Zepto ($.ua)
+
+Although written in vanilla js (which means it doesn't depends on jQuery), this library will automatically detect if jQuery/Zepto is present and create `$.ua` object based on browser's user-agent (although in case you need, `window.UAParser` constructor is still present). To get/set user-agent you can use: `$.ua.get()` / `$.ua.set(uastring)`.
+
+```js
+// In browser with default user-agent: 'Mozilla/5.0 (Linux; U; Android 2.3.4; en-us; Sprint APA7373KT Build/GRJ22) AppleWebKit/533.1 (KHTML, like Gecko) Version/4.0':
+
+// Do some tests
+console.log($.ua.device); // {vendor: "HTC", model: "Evo Shift 4G", type: "mobile"}
+console.log($.ua.os); // {name: "Android", version: "2.3.4"}
+console.log($.ua.os.name); // "Android"
+console.log($.ua.get()); // "Mozilla/5.0 (Linux; U; Android 2.3.4; en-us; Sprint APA7373KT Build/GRJ22) AppleWebKit/533.1 (KHTML, like Gecko) Version/4.0"
+
+// reset to custom user-agent
+$.ua.set('Mozilla/5.0 (Linux; U; Android 3.0.1; en-us; Xoom Build/HWI69) AppleWebKit/534.13 (KHTML, like Gecko) Version/4.0 Safari/534.13');
+
+// Test again
+console.log($.ua.browser.name); // "Safari"
+console.log($.ua.engine.name); // "Webkit"
+console.log($.ua.device); // {vendor: "Motorola", model: "Xoom", type: "tablet"}
+console.log(parseInt($.ua.browser.version.split('.')[0], 10)); // 4
+
+// Add class to tag
+//
+$('body').addClass('ua-browser-' + $.ua.browser.name + ' ua-devicetype-' + $.ua.device.type);
+```
+
+## Extending regex patterns
+
+* `UAParser([uastring,] extensions)`
+
+Pass your own regexes to extend the limited matching rules.
+
+```js
+// Example:
+var myOwnRegex = [[/(myownbrowser)\/([\w\.]+)/i], [UAParser.BROWSER.NAME, UAParser.BROWSER.VERSION]];
+var myParser = new UAParser({ browser: myOwnRegex });
+var uaString = 'Mozilla/5.0 MyOwnBrowser/1.3';
+console.log(myParser.setUA(uaString).getBrowser()); // {name: "MyOwnBrowser", version: "1.3"}
+```
+
+
+# Development
+
+## Contribute
+
+* Fork and clone this repository
+* Make some changes as required
+* Write a unit test to showcase your feature
+* Run the test suites to make sure the changes you made didn't break anything `$ npm run test`
+* Commit and push to your own repository
+* Submit a pull request to this repository under `develop` branch
+* Profit? $$$
+
+## Build
+
+Build a minified & packed script
+
+```sh
+$ npm run build
+```
+
+
+# Donate
+
+Do you use & like UAParser.js but you don’t find a way to show some love? If yes, please consider donating to support this project. Otherwise, no worries, regardless of whether there is support or not, I will keep maintaining this project. Still, if you buy me a cup of coffee I would be more than happy though :)
+
+[](https://pledgie.com/campaigns/34252)
+
+
+# License
+
+Dual licensed under GPLv2 & MIT
+
+Copyright © 2012-2016 Faisal Salman <>
+
+Permission is hereby granted, free of charge, to any person obtaining a copy of
+this software and associated documentation files (the "Software"), to deal in
+the Software without restriction, including without limitation the rights to use,
+copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the
+Software, and to permit persons to whom the Software is furnished to do so,
+subject to the following conditions:
+
+The above copyright notice and this permission notice shall be included in all
+copies or substantial portions of the Software.
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/src/ua-parser.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/src/ua-parser.js
new file mode 100644
index 00000000..64e64540
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/src/ua-parser.js
@@ -0,0 +1,1072 @@
+/**
+ * UAParser.js v0.7.17
+ * Lightweight JavaScript-based User-Agent string parser
+ * https://github.com/faisalman/ua-parser-js
+ *
+ * Copyright © 2012-2016 Faisal Salman
+ * Dual licensed under GPLv2 & MIT
+ */
+
+(function (window, undefined) {
+
+ 'use strict';
+
+ //////////////
+ // Constants
+ /////////////
+
+
+ var LIBVERSION = '0.7.17',
+ EMPTY = '',
+ UNKNOWN = '?',
+ FUNC_TYPE = 'function',
+ UNDEF_TYPE = 'undefined',
+ OBJ_TYPE = 'object',
+ STR_TYPE = 'string',
+ MAJOR = 'major', // deprecated
+ MODEL = 'model',
+ NAME = 'name',
+ TYPE = 'type',
+ VENDOR = 'vendor',
+ VERSION = 'version',
+ ARCHITECTURE= 'architecture',
+ CONSOLE = 'console',
+ MOBILE = 'mobile',
+ TABLET = 'tablet',
+ SMARTTV = 'smarttv',
+ WEARABLE = 'wearable',
+ EMBEDDED = 'embedded';
+
+
+ ///////////
+ // Helper
+ //////////
+
+
+ var util = {
+ extend : function (regexes, extensions) {
+ var margedRegexes = {};
+ for (var i in regexes) {
+ if (extensions[i] && extensions[i].length % 2 === 0) {
+ margedRegexes[i] = extensions[i].concat(regexes[i]);
+ } else {
+ margedRegexes[i] = regexes[i];
+ }
+ }
+ return margedRegexes;
+ },
+ has : function (str1, str2) {
+ if (typeof str1 === "string") {
+ return str2.toLowerCase().indexOf(str1.toLowerCase()) !== -1;
+ } else {
+ return false;
+ }
+ },
+ lowerize : function (str) {
+ return str.toLowerCase();
+ },
+ major : function (version) {
+ return typeof(version) === STR_TYPE ? version.replace(/[^\d\.]/g,'').split(".")[0] : undefined;
+ },
+ trim : function (str) {
+ return str.replace(/^[\s\uFEFF\xA0]+|[\s\uFEFF\xA0]+$/g, '');
+ }
+ };
+
+
+ ///////////////
+ // Map helper
+ //////////////
+
+
+ var mapper = {
+
+ rgx : function (ua, arrays) {
+
+ //var result = {},
+ var i = 0, j, k, p, q, matches, match;//, args = arguments;
+
+ /*// construct object barebones
+ for (p = 0; p < args[1].length; p++) {
+ q = args[1][p];
+ result[typeof q === OBJ_TYPE ? q[0] : q] = undefined;
+ }*/
+
+ // loop through all regexes maps
+ while (i < arrays.length && !matches) {
+
+ var regex = arrays[i], // even sequence (0,2,4,..)
+ props = arrays[i + 1]; // odd sequence (1,3,5,..)
+ j = k = 0;
+
+ // try matching uastring with regexes
+ while (j < regex.length && !matches) {
+
+ matches = regex[j++].exec(ua);
+
+ if (!!matches) {
+ for (p = 0; p < props.length; p++) {
+ match = matches[++k];
+ q = props[p];
+ // check if given property is actually array
+ if (typeof q === OBJ_TYPE && q.length > 0) {
+ if (q.length == 2) {
+ if (typeof q[1] == FUNC_TYPE) {
+ // assign modified match
+ this[q[0]] = q[1].call(this, match);
+ } else {
+ // assign given value, ignore regex match
+ this[q[0]] = q[1];
+ }
+ } else if (q.length == 3) {
+ // check whether function or regex
+ if (typeof q[1] === FUNC_TYPE && !(q[1].exec && q[1].test)) {
+ // call function (usually string mapper)
+ this[q[0]] = match ? q[1].call(this, match, q[2]) : undefined;
+ } else {
+ // sanitize match using given regex
+ this[q[0]] = match ? match.replace(q[1], q[2]) : undefined;
+ }
+ } else if (q.length == 4) {
+ this[q[0]] = match ? q[3].call(this, match.replace(q[1], q[2])) : undefined;
+ }
+ } else {
+ this[q] = match ? match : undefined;
+ }
+ }
+ }
+ }
+ i += 2;
+ }
+ // console.log(this);
+ //return this;
+ },
+
+ str : function (str, map) {
+
+ for (var i in map) {
+ // check if array
+ if (typeof map[i] === OBJ_TYPE && map[i].length > 0) {
+ for (var j = 0; j < map[i].length; j++) {
+ if (util.has(map[i][j], str)) {
+ return (i === UNKNOWN) ? undefined : i;
+ }
+ }
+ } else if (util.has(map[i], str)) {
+ return (i === UNKNOWN) ? undefined : i;
+ }
+ }
+ return str;
+ }
+ };
+
+
+ ///////////////
+ // String map
+ //////////////
+
+
+ var maps = {
+
+ browser : {
+ oldsafari : {
+ version : {
+ '1.0' : '/8',
+ '1.2' : '/1',
+ '1.3' : '/3',
+ '2.0' : '/412',
+ '2.0.2' : '/416',
+ '2.0.3' : '/417',
+ '2.0.4' : '/419',
+ '?' : '/'
+ }
+ }
+ },
+
+ device : {
+ amazon : {
+ model : {
+ 'Fire Phone' : ['SD', 'KF']
+ }
+ },
+ sprint : {
+ model : {
+ 'Evo Shift 4G' : '7373KT'
+ },
+ vendor : {
+ 'HTC' : 'APA',
+ 'Sprint' : 'Sprint'
+ }
+ }
+ },
+
+ os : {
+ windows : {
+ version : {
+ 'ME' : '4.90',
+ 'NT 3.11' : 'NT3.51',
+ 'NT 4.0' : 'NT4.0',
+ '2000' : 'NT 5.0',
+ 'XP' : ['NT 5.1', 'NT 5.2'],
+ 'Vista' : 'NT 6.0',
+ '7' : 'NT 6.1',
+ '8' : 'NT 6.2',
+ '8.1' : 'NT 6.3',
+ '10' : ['NT 6.4', 'NT 10.0'],
+ 'RT' : 'ARM'
+ }
+ }
+ }
+ };
+
+
+ //////////////
+ // Regex map
+ /////////////
+
+
+ var regexes = {
+
+ browser : [[
+
+ // Presto based
+ /(opera\smini)\/([\w\.-]+)/i, // Opera Mini
+ /(opera\s[mobiletab]+).+version\/([\w\.-]+)/i, // Opera Mobi/Tablet
+ /(opera).+version\/([\w\.]+)/i, // Opera > 9.80
+ /(opera)[\/\s]+([\w\.]+)/i // Opera < 9.80
+ ], [NAME, VERSION], [
+
+ /(opios)[\/\s]+([\w\.]+)/i // Opera mini on iphone >= 8.0
+ ], [[NAME, 'Opera Mini'], VERSION], [
+
+ /\s(opr)\/([\w\.]+)/i // Opera Webkit
+ ], [[NAME, 'Opera'], VERSION], [
+
+ // Mixed
+ /(kindle)\/([\w\.]+)/i, // Kindle
+ /(lunascape|maxthon|netfront|jasmine|blazer)[\/\s]?([\w\.]+)*/i,
+ // Lunascape/Maxthon/Netfront/Jasmine/Blazer
+
+ // Trident based
+ /(avant\s|iemobile|slim|baidu)(?:browser)?[\/\s]?([\w\.]*)/i,
+ // Avant/IEMobile/SlimBrowser/Baidu
+ /(?:ms|\()(ie)\s([\w\.]+)/i, // Internet Explorer
+
+ // Webkit/KHTML based
+ /(rekonq)\/([\w\.]+)*/i, // Rekonq
+ /(chromium|flock|rockmelt|midori|epiphany|silk|skyfire|ovibrowser|bolt|iron|vivaldi|iridium|phantomjs|bowser)\/([\w\.-]+)/i
+ // Chromium/Flock/RockMelt/Midori/Epiphany/Silk/Skyfire/Bolt/Iron/Iridium/PhantomJS/Bowser
+ ], [NAME, VERSION], [
+
+ /(trident).+rv[:\s]([\w\.]+).+like\sgecko/i // IE11
+ ], [[NAME, 'IE'], VERSION], [
+
+ /(edge)\/((\d+)?[\w\.]+)/i // Microsoft Edge
+ ], [NAME, VERSION], [
+
+ /(yabrowser)\/([\w\.]+)/i // Yandex
+ ], [[NAME, 'Yandex'], VERSION], [
+
+ /(puffin)\/([\w\.]+)/i // Puffin
+ ], [[NAME, 'Puffin'], VERSION], [
+
+ /((?:[\s\/])uc?\s?browser|(?:juc.+)ucweb)[\/\s]?([\w\.]+)/i
+ // UCBrowser
+ ], [[NAME, 'UCBrowser'], VERSION], [
+
+ /(comodo_dragon)\/([\w\.]+)/i // Comodo Dragon
+ ], [[NAME, /_/g, ' '], VERSION], [
+
+ /(micromessenger)\/([\w\.]+)/i // WeChat
+ ], [[NAME, 'WeChat'], VERSION], [
+
+ /(QQ)\/([\d\.]+)/i // QQ, aka ShouQ
+ ], [NAME, VERSION], [
+
+ /m?(qqbrowser)[\/\s]?([\w\.]+)/i // QQBrowser
+ ], [NAME, VERSION], [
+
+ /xiaomi\/miuibrowser\/([\w\.]+)/i // MIUI Browser
+ ], [VERSION, [NAME, 'MIUI Browser']], [
+
+ /;fbav\/([\w\.]+);/i // Facebook App for iOS & Android
+ ], [VERSION, [NAME, 'Facebook']], [
+
+ /headlesschrome(?:\/([\w\.]+)|\s)/i // Chrome Headless
+ ], [VERSION, [NAME, 'Chrome Headless']], [
+
+ /\swv\).+(chrome)\/([\w\.]+)/i // Chrome WebView
+ ], [[NAME, /(.+)/, '$1 WebView'], VERSION], [
+
+ /((?:oculus|samsung)browser)\/([\w\.]+)/i
+ ], [[NAME, /(.+(?:g|us))(.+)/, '$1 $2'], VERSION], [ // Oculus / Samsung Browser
+
+ /android.+version\/([\w\.]+)\s+(?:mobile\s?safari|safari)*/i // Android Browser
+ ], [VERSION, [NAME, 'Android Browser']], [
+
+ /(chrome|omniweb|arora|[tizenoka]{5}\s?browser)\/v?([\w\.]+)/i
+ // Chrome/OmniWeb/Arora/Tizen/Nokia
+ ], [NAME, VERSION], [
+
+ /(dolfin)\/([\w\.]+)/i // Dolphin
+ ], [[NAME, 'Dolphin'], VERSION], [
+
+ /((?:android.+)crmo|crios)\/([\w\.]+)/i // Chrome for Android/iOS
+ ], [[NAME, 'Chrome'], VERSION], [
+
+ /(coast)\/([\w\.]+)/i // Opera Coast
+ ], [[NAME, 'Opera Coast'], VERSION], [
+
+ /fxios\/([\w\.-]+)/i // Firefox for iOS
+ ], [VERSION, [NAME, 'Firefox']], [
+
+ /version\/([\w\.]+).+?mobile\/\w+\s(safari)/i // Mobile Safari
+ ], [VERSION, [NAME, 'Mobile Safari']], [
+
+ /version\/([\w\.]+).+?(mobile\s?safari|safari)/i // Safari & Safari Mobile
+ ], [VERSION, NAME], [
+
+ /webkit.+?(gsa)\/([\w\.]+).+?(mobile\s?safari|safari)(\/[\w\.]+)/i // Google Search Appliance on iOS
+ ], [[NAME, 'GSA'], VERSION], [
+
+ /webkit.+?(mobile\s?safari|safari)(\/[\w\.]+)/i // Safari < 3.0
+ ], [NAME, [VERSION, mapper.str, maps.browser.oldsafari.version]], [
+
+ /(konqueror)\/([\w\.]+)/i, // Konqueror
+ /(webkit|khtml)\/([\w\.]+)/i
+ ], [NAME, VERSION], [
+
+ // Gecko based
+ /(navigator|netscape)\/([\w\.-]+)/i // Netscape
+ ], [[NAME, 'Netscape'], VERSION], [
+ /(swiftfox)/i, // Swiftfox
+ /(icedragon|iceweasel|camino|chimera|fennec|maemo\sbrowser|minimo|conkeror)[\/\s]?([\w\.\+]+)/i,
+ // IceDragon/Iceweasel/Camino/Chimera/Fennec/Maemo/Minimo/Conkeror
+ /(firefox|seamonkey|k-meleon|icecat|iceape|firebird|phoenix)\/([\w\.-]+)/i,
+ // Firefox/SeaMonkey/K-Meleon/IceCat/IceApe/Firebird/Phoenix
+ /(mozilla)\/([\w\.]+).+rv\:.+gecko\/\d+/i, // Mozilla
+
+ // Other
+ /(polaris|lynx|dillo|icab|doris|amaya|w3m|netsurf|sleipnir)[\/\s]?([\w\.]+)/i,
+ // Polaris/Lynx/Dillo/iCab/Doris/Amaya/w3m/NetSurf/Sleipnir
+ /(links)\s\(([\w\.]+)/i, // Links
+ /(gobrowser)\/?([\w\.]+)*/i, // GoBrowser
+ /(ice\s?browser)\/v?([\w\._]+)/i, // ICE Browser
+ /(mosaic)[\/\s]([\w\.]+)/i // Mosaic
+ ], [NAME, VERSION]
+
+ /* /////////////////////
+ // Media players BEGIN
+ ////////////////////////
+
+ , [
+
+ /(apple(?:coremedia|))\/((\d+)[\w\._]+)/i, // Generic Apple CoreMedia
+ /(coremedia) v((\d+)[\w\._]+)/i
+ ], [NAME, VERSION], [
+
+ /(aqualung|lyssna|bsplayer)\/((\d+)?[\w\.-]+)/i // Aqualung/Lyssna/BSPlayer
+ ], [NAME, VERSION], [
+
+ /(ares|ossproxy)\s((\d+)[\w\.-]+)/i // Ares/OSSProxy
+ ], [NAME, VERSION], [
+
+ /(audacious|audimusicstream|amarok|bass|core|dalvik|gnomemplayer|music on console|nsplayer|psp-internetradioplayer|videos)\/((\d+)[\w\.-]+)/i,
+ // Audacious/AudiMusicStream/Amarok/BASS/OpenCORE/Dalvik/GnomeMplayer/MoC
+ // NSPlayer/PSP-InternetRadioPlayer/Videos
+ /(clementine|music player daemon)\s((\d+)[\w\.-]+)/i, // Clementine/MPD
+ /(lg player|nexplayer)\s((\d+)[\d\.]+)/i,
+ /player\/(nexplayer|lg player)\s((\d+)[\w\.-]+)/i // NexPlayer/LG Player
+ ], [NAME, VERSION], [
+ /(nexplayer)\s((\d+)[\w\.-]+)/i // Nexplayer
+ ], [NAME, VERSION], [
+
+ /(flrp)\/((\d+)[\w\.-]+)/i // Flip Player
+ ], [[NAME, 'Flip Player'], VERSION], [
+
+ /(fstream|nativehost|queryseekspider|ia-archiver|facebookexternalhit)/i
+ // FStream/NativeHost/QuerySeekSpider/IA Archiver/facebookexternalhit
+ ], [NAME], [
+
+ /(gstreamer) souphttpsrc (?:\([^\)]+\)){0,1} libsoup\/((\d+)[\w\.-]+)/i
+ // Gstreamer
+ ], [NAME, VERSION], [
+
+ /(htc streaming player)\s[\w_]+\s\/\s((\d+)[\d\.]+)/i, // HTC Streaming Player
+ /(java|python-urllib|python-requests|wget|libcurl)\/((\d+)[\w\.-_]+)/i,
+ // Java/urllib/requests/wget/cURL
+ /(lavf)((\d+)[\d\.]+)/i // Lavf (FFMPEG)
+ ], [NAME, VERSION], [
+
+ /(htc_one_s)\/((\d+)[\d\.]+)/i // HTC One S
+ ], [[NAME, /_/g, ' '], VERSION], [
+
+ /(mplayer)(?:\s|\/)(?:(?:sherpya-){0,1}svn)(?:-|\s)(r\d+(?:-\d+[\w\.-]+){0,1})/i
+ // MPlayer SVN
+ ], [NAME, VERSION], [
+
+ /(mplayer)(?:\s|\/|[unkow-]+)((\d+)[\w\.-]+)/i // MPlayer
+ ], [NAME, VERSION], [
+
+ /(mplayer)/i, // MPlayer (no other info)
+ /(yourmuze)/i, // YourMuze
+ /(media player classic|nero showtime)/i // Media Player Classic/Nero ShowTime
+ ], [NAME], [
+
+ /(nero (?:home|scout))\/((\d+)[\w\.-]+)/i // Nero Home/Nero Scout
+ ], [NAME, VERSION], [
+
+ /(nokia\d+)\/((\d+)[\w\.-]+)/i // Nokia
+ ], [NAME, VERSION], [
+
+ /\s(songbird)\/((\d+)[\w\.-]+)/i // Songbird/Philips-Songbird
+ ], [NAME, VERSION], [
+
+ /(winamp)3 version ((\d+)[\w\.-]+)/i, // Winamp
+ /(winamp)\s((\d+)[\w\.-]+)/i,
+ /(winamp)mpeg\/((\d+)[\w\.-]+)/i
+ ], [NAME, VERSION], [
+
+ /(ocms-bot|tapinradio|tunein radio|unknown|winamp|inlight radio)/i // OCMS-bot/tap in radio/tunein/unknown/winamp (no other info)
+ // inlight radio
+ ], [NAME], [
+
+ /(quicktime|rma|radioapp|radioclientapplication|soundtap|totem|stagefright|streamium)\/((\d+)[\w\.-]+)/i
+ // QuickTime/RealMedia/RadioApp/RadioClientApplication/
+ // SoundTap/Totem/Stagefright/Streamium
+ ], [NAME, VERSION], [
+
+ /(smp)((\d+)[\d\.]+)/i // SMP
+ ], [NAME, VERSION], [
+
+ /(vlc) media player - version ((\d+)[\w\.]+)/i, // VLC Videolan
+ /(vlc)\/((\d+)[\w\.-]+)/i,
+ /(xbmc|gvfs|xine|xmms|irapp)\/((\d+)[\w\.-]+)/i, // XBMC/gvfs/Xine/XMMS/irapp
+ /(foobar2000)\/((\d+)[\d\.]+)/i, // Foobar2000
+ /(itunes)\/((\d+)[\d\.]+)/i // iTunes
+ ], [NAME, VERSION], [
+
+ /(wmplayer)\/((\d+)[\w\.-]+)/i, // Windows Media Player
+ /(windows-media-player)\/((\d+)[\w\.-]+)/i
+ ], [[NAME, /-/g, ' '], VERSION], [
+
+ /windows\/((\d+)[\w\.-]+) upnp\/[\d\.]+ dlnadoc\/[\d\.]+ (home media server)/i
+ // Windows Media Server
+ ], [VERSION, [NAME, 'Windows']], [
+
+ /(com\.riseupradioalarm)\/((\d+)[\d\.]*)/i // RiseUP Radio Alarm
+ ], [NAME, VERSION], [
+
+ /(rad.io)\s((\d+)[\d\.]+)/i, // Rad.io
+ /(radio.(?:de|at|fr))\s((\d+)[\d\.]+)/i
+ ], [[NAME, 'rad.io'], VERSION]
+
+ //////////////////////
+ // Media players END
+ ////////////////////*/
+
+ ],
+
+ cpu : [[
+
+ /(?:(amd|x(?:(?:86|64)[_-])?|wow|win)64)[;\)]/i // AMD64
+ ], [[ARCHITECTURE, 'amd64']], [
+
+ /(ia32(?=;))/i // IA32 (quicktime)
+ ], [[ARCHITECTURE, util.lowerize]], [
+
+ /((?:i[346]|x)86)[;\)]/i // IA32
+ ], [[ARCHITECTURE, 'ia32']], [
+
+ // PocketPC mistakenly identified as PowerPC
+ /windows\s(ce|mobile);\sppc;/i
+ ], [[ARCHITECTURE, 'arm']], [
+
+ /((?:ppc|powerpc)(?:64)?)(?:\smac|;|\))/i // PowerPC
+ ], [[ARCHITECTURE, /ower/, '', util.lowerize]], [
+
+ /(sun4\w)[;\)]/i // SPARC
+ ], [[ARCHITECTURE, 'sparc']], [
+
+ /((?:avr32|ia64(?=;))|68k(?=\))|arm(?:64|(?=v\d+;))|(?=atmel\s)avr|(?:irix|mips|sparc)(?:64)?(?=;)|pa-risc)/i
+ // IA64, 68K, ARM/64, AVR/32, IRIX/64, MIPS/64, SPARC/64, PA-RISC
+ ], [[ARCHITECTURE, util.lowerize]]
+ ],
+
+ device : [[
+
+ /\((ipad|playbook);[\w\s\);-]+(rim|apple)/i // iPad/PlayBook
+ ], [MODEL, VENDOR, [TYPE, TABLET]], [
+
+ /applecoremedia\/[\w\.]+ \((ipad)/ // iPad
+ ], [MODEL, [VENDOR, 'Apple'], [TYPE, TABLET]], [
+
+ /(apple\s{0,1}tv)/i // Apple TV
+ ], [[MODEL, 'Apple TV'], [VENDOR, 'Apple']], [
+
+ /(archos)\s(gamepad2?)/i, // Archos
+ /(hp).+(touchpad)/i, // HP TouchPad
+ /(hp).+(tablet)/i, // HP Tablet
+ /(kindle)\/([\w\.]+)/i, // Kindle
+ /\s(nook)[\w\s]+build\/(\w+)/i, // Nook
+ /(dell)\s(strea[kpr\s\d]*[\dko])/i // Dell Streak
+ ], [VENDOR, MODEL, [TYPE, TABLET]], [
+
+ /(kf[A-z]+)\sbuild\/[\w\.]+.*silk\//i // Kindle Fire HD
+ ], [MODEL, [VENDOR, 'Amazon'], [TYPE, TABLET]], [
+ /(sd|kf)[0349hijorstuw]+\sbuild\/[\w\.]+.*silk\//i // Fire Phone
+ ], [[MODEL, mapper.str, maps.device.amazon.model], [VENDOR, 'Amazon'], [TYPE, MOBILE]], [
+
+ /\((ip[honed|\s\w*]+);.+(apple)/i // iPod/iPhone
+ ], [MODEL, VENDOR, [TYPE, MOBILE]], [
+ /\((ip[honed|\s\w*]+);/i // iPod/iPhone
+ ], [MODEL, [VENDOR, 'Apple'], [TYPE, MOBILE]], [
+
+ /(blackberry)[\s-]?(\w+)/i, // BlackBerry
+ /(blackberry|benq|palm(?=\-)|sonyericsson|acer|asus|dell|meizu|motorola|polytron)[\s_-]?([\w-]+)*/i,
+ // BenQ/Palm/Sony-Ericsson/Acer/Asus/Dell/Meizu/Motorola/Polytron
+ /(hp)\s([\w\s]+\w)/i, // HP iPAQ
+ /(asus)-?(\w+)/i // Asus
+ ], [VENDOR, MODEL, [TYPE, MOBILE]], [
+ /\(bb10;\s(\w+)/i // BlackBerry 10
+ ], [MODEL, [VENDOR, 'BlackBerry'], [TYPE, MOBILE]], [
+ // Asus Tablets
+ /android.+(transfo[prime\s]{4,10}\s\w+|eeepc|slider\s\w+|nexus 7|padfone)/i
+ ], [MODEL, [VENDOR, 'Asus'], [TYPE, TABLET]], [
+
+ /(sony)\s(tablet\s[ps])\sbuild\//i, // Sony
+ /(sony)?(?:sgp.+)\sbuild\//i
+ ], [[VENDOR, 'Sony'], [MODEL, 'Xperia Tablet'], [TYPE, TABLET]], [
+ /android.+\s([c-g]\d{4}|so[-l]\w+)\sbuild\//i
+ ], [MODEL, [VENDOR, 'Sony'], [TYPE, MOBILE]], [
+
+ /\s(ouya)\s/i, // Ouya
+ /(nintendo)\s([wids3u]+)/i // Nintendo
+ ], [VENDOR, MODEL, [TYPE, CONSOLE]], [
+
+ /android.+;\s(shield)\sbuild/i // Nvidia
+ ], [MODEL, [VENDOR, 'Nvidia'], [TYPE, CONSOLE]], [
+
+ /(playstation\s[34portablevi]+)/i // Playstation
+ ], [MODEL, [VENDOR, 'Sony'], [TYPE, CONSOLE]], [
+
+ /(sprint\s(\w+))/i // Sprint Phones
+ ], [[VENDOR, mapper.str, maps.device.sprint.vendor], [MODEL, mapper.str, maps.device.sprint.model], [TYPE, MOBILE]], [
+
+ /(lenovo)\s?(S(?:5000|6000)+(?:[-][\w+]))/i // Lenovo tablets
+ ], [VENDOR, MODEL, [TYPE, TABLET]], [
+
+ /(htc)[;_\s-]+([\w\s]+(?=\))|\w+)*/i, // HTC
+ /(zte)-(\w+)*/i, // ZTE
+ /(alcatel|geeksphone|lenovo|nexian|panasonic|(?=;\s)sony)[_\s-]?([\w-]+)*/i
+ // Alcatel/GeeksPhone/Lenovo/Nexian/Panasonic/Sony
+ ], [VENDOR, [MODEL, /_/g, ' '], [TYPE, MOBILE]], [
+
+ /(nexus\s9)/i // HTC Nexus 9
+ ], [MODEL, [VENDOR, 'HTC'], [TYPE, TABLET]], [
+
+ /d\/huawei([\w\s-]+)[;\)]/i,
+ /(nexus\s6p)/i // Huawei
+ ], [MODEL, [VENDOR, 'Huawei'], [TYPE, MOBILE]], [
+
+ /(microsoft);\s(lumia[\s\w]+)/i // Microsoft Lumia
+ ], [VENDOR, MODEL, [TYPE, MOBILE]], [
+
+ /[\s\(;](xbox(?:\sone)?)[\s\);]/i // Microsoft Xbox
+ ], [MODEL, [VENDOR, 'Microsoft'], [TYPE, CONSOLE]], [
+ /(kin\.[onetw]{3})/i // Microsoft Kin
+ ], [[MODEL, /\./g, ' '], [VENDOR, 'Microsoft'], [TYPE, MOBILE]], [
+
+ // Motorola
+ /\s(milestone|droid(?:[2-4x]|\s(?:bionic|x2|pro|razr))?(:?\s4g)?)[\w\s]+build\//i,
+ /mot[\s-]?(\w+)*/i,
+ /(XT\d{3,4}) build\//i,
+ /(nexus\s6)/i
+ ], [MODEL, [VENDOR, 'Motorola'], [TYPE, MOBILE]], [
+ /android.+\s(mz60\d|xoom[\s2]{0,2})\sbuild\//i
+ ], [MODEL, [VENDOR, 'Motorola'], [TYPE, TABLET]], [
+
+ /hbbtv\/\d+\.\d+\.\d+\s+\([\w\s]*;\s*(\w[^;]*);([^;]*)/i // HbbTV devices
+ ], [[VENDOR, util.trim], [MODEL, util.trim], [TYPE, SMARTTV]], [
+
+ /hbbtv.+maple;(\d+)/i
+ ], [[MODEL, /^/, 'SmartTV'], [VENDOR, 'Samsung'], [TYPE, SMARTTV]], [
+
+ /\(dtv[\);].+(aquos)/i // Sharp
+ ], [MODEL, [VENDOR, 'Sharp'], [TYPE, SMARTTV]], [
+
+ /android.+((sch-i[89]0\d|shw-m380s|gt-p\d{4}|gt-n\d+|sgh-t8[56]9|nexus 10))/i,
+ /((SM-T\w+))/i
+ ], [[VENDOR, 'Samsung'], MODEL, [TYPE, TABLET]], [ // Samsung
+ /smart-tv.+(samsung)/i
+ ], [VENDOR, [TYPE, SMARTTV], MODEL], [
+ /((s[cgp]h-\w+|gt-\w+|galaxy\snexus|sm-\w[\w\d]+))/i,
+ /(sam[sung]*)[\s-]*(\w+-?[\w-]*)*/i,
+ /sec-((sgh\w+))/i
+ ], [[VENDOR, 'Samsung'], MODEL, [TYPE, MOBILE]], [
+
+ /sie-(\w+)*/i // Siemens
+ ], [MODEL, [VENDOR, 'Siemens'], [TYPE, MOBILE]], [
+
+ /(maemo|nokia).*(n900|lumia\s\d+)/i, // Nokia
+ /(nokia)[\s_-]?([\w-]+)*/i
+ ], [[VENDOR, 'Nokia'], MODEL, [TYPE, MOBILE]], [
+
+ /android\s3\.[\s\w;-]{10}(a\d{3})/i // Acer
+ ], [MODEL, [VENDOR, 'Acer'], [TYPE, TABLET]], [
+
+ /android.+([vl]k\-?\d{3})\s+build/i // LG Tablet
+ ], [MODEL, [VENDOR, 'LG'], [TYPE, TABLET]], [
+ /android\s3\.[\s\w;-]{10}(lg?)-([06cv9]{3,4})/i // LG Tablet
+ ], [[VENDOR, 'LG'], MODEL, [TYPE, TABLET]], [
+ /(lg) netcast\.tv/i // LG SmartTV
+ ], [VENDOR, MODEL, [TYPE, SMARTTV]], [
+ /(nexus\s[45])/i, // LG
+ /lg[e;\s\/-]+(\w+)*/i,
+ /android.+lg(\-?[\d\w]+)\s+build/i
+ ], [MODEL, [VENDOR, 'LG'], [TYPE, MOBILE]], [
+
+ /android.+(ideatab[a-z0-9\-\s]+)/i // Lenovo
+ ], [MODEL, [VENDOR, 'Lenovo'], [TYPE, TABLET]], [
+
+ /linux;.+((jolla));/i // Jolla
+ ], [VENDOR, MODEL, [TYPE, MOBILE]], [
+
+ /((pebble))app\/[\d\.]+\s/i // Pebble
+ ], [VENDOR, MODEL, [TYPE, WEARABLE]], [
+
+ /android.+;\s(oppo)\s?([\w\s]+)\sbuild/i // OPPO
+ ], [VENDOR, MODEL, [TYPE, MOBILE]], [
+
+ /crkey/i // Google Chromecast
+ ], [[MODEL, 'Chromecast'], [VENDOR, 'Google']], [
+
+ /android.+;\s(glass)\s\d/i // Google Glass
+ ], [MODEL, [VENDOR, 'Google'], [TYPE, WEARABLE]], [
+
+ /android.+;\s(pixel c)\s/i // Google Pixel C
+ ], [MODEL, [VENDOR, 'Google'], [TYPE, TABLET]], [
+
+ /android.+;\s(pixel xl|pixel)\s/i // Google Pixel
+ ], [MODEL, [VENDOR, 'Google'], [TYPE, MOBILE]], [
+
+ /android.+(\w+)\s+build\/hm\1/i, // Xiaomi Hongmi 'numeric' models
+ /android.+(hm[\s\-_]*note?[\s_]*(?:\d\w)?)\s+build/i, // Xiaomi Hongmi
+ /android.+(mi[\s\-_]*(?:one|one[\s_]plus|note lte)?[\s_]*(?:\d\w)?)\s+build/i, // Xiaomi Mi
+ /android.+(redmi[\s\-_]*(?:note)?(?:[\s_]*[\w\s]+)?)\s+build/i // Redmi Phones
+ ], [[MODEL, /_/g, ' '], [VENDOR, 'Xiaomi'], [TYPE, MOBILE]], [
+ /android.+(mi[\s\-_]*(?:pad)?(?:[\s_]*[\w\s]+)?)\s+build/i // Mi Pad tablets
+ ],[[MODEL, /_/g, ' '], [VENDOR, 'Xiaomi'], [TYPE, TABLET]], [
+ /android.+;\s(m[1-5]\snote)\sbuild/i // Meizu Tablet
+ ], [MODEL, [VENDOR, 'Meizu'], [TYPE, TABLET]], [
+
+ /android.+a000(1)\s+build/i // OnePlus
+ ], [MODEL, [VENDOR, 'OnePlus'], [TYPE, MOBILE]], [
+
+ /android.+[;\/]\s*(RCT[\d\w]+)\s+build/i // RCA Tablets
+ ], [MODEL, [VENDOR, 'RCA'], [TYPE, TABLET]], [
+
+ /android.+[;\/]\s*(Venue[\d\s]*)\s+build/i // Dell Venue Tablets
+ ], [MODEL, [VENDOR, 'Dell'], [TYPE, TABLET]], [
+
+ /android.+[;\/]\s*(Q[T|M][\d\w]+)\s+build/i // Verizon Tablet
+ ], [MODEL, [VENDOR, 'Verizon'], [TYPE, TABLET]], [
+
+ /android.+[;\/]\s+(Barnes[&\s]+Noble\s+|BN[RT])(V?.*)\s+build/i // Barnes & Noble Tablet
+ ], [[VENDOR, 'Barnes & Noble'], MODEL, [TYPE, TABLET]], [
+
+ /android.+[;\/]\s+(TM\d{3}.*\b)\s+build/i // Barnes & Noble Tablet
+ ], [MODEL, [VENDOR, 'NuVision'], [TYPE, TABLET]], [
+
+ /android.+[;\/]\s*(zte)?.+(k\d{2})\s+build/i // ZTE K Series Tablet
+ ], [[VENDOR, 'ZTE'], MODEL, [TYPE, TABLET]], [
+
+ /android.+[;\/]\s*(gen\d{3})\s+build.*49h/i // Swiss GEN Mobile
+ ], [MODEL, [VENDOR, 'Swiss'], [TYPE, MOBILE]], [
+
+ /android.+[;\/]\s*(zur\d{3})\s+build/i // Swiss ZUR Tablet
+ ], [MODEL, [VENDOR, 'Swiss'], [TYPE, TABLET]], [
+
+ /android.+[;\/]\s*((Zeki)?TB.*\b)\s+build/i // Zeki Tablets
+ ], [MODEL, [VENDOR, 'Zeki'], [TYPE, TABLET]], [
+
+ /(android).+[;\/]\s+([YR]\d{2}x?.*)\s+build/i,
+ /android.+[;\/]\s+(Dragon[\-\s]+Touch\s+|DT)(.+)\s+build/i // Dragon Touch Tablet
+ ], [[VENDOR, 'Dragon Touch'], MODEL, [TYPE, TABLET]], [
+
+ /android.+[;\/]\s*(NS-?.+)\s+build/i // Insignia Tablets
+ ], [MODEL, [VENDOR, 'Insignia'], [TYPE, TABLET]], [
+
+ /android.+[;\/]\s*((NX|Next)-?.+)\s+build/i // NextBook Tablets
+ ], [MODEL, [VENDOR, 'NextBook'], [TYPE, TABLET]], [
+
+ /android.+[;\/]\s*(Xtreme\_?)?(V(1[045]|2[015]|30|40|60|7[05]|90))\s+build/i
+ ], [[VENDOR, 'Voice'], MODEL, [TYPE, MOBILE]], [ // Voice Xtreme Phones
+
+ /android.+[;\/]\s*(LVTEL\-?)?(V1[12])\s+build/i // LvTel Phones
+ ], [[VENDOR, 'LvTel'], MODEL, [TYPE, MOBILE]], [
+
+ /android.+[;\/]\s*(V(100MD|700NA|7011|917G).*\b)\s+build/i // Envizen Tablets
+ ], [MODEL, [VENDOR, 'Envizen'], [TYPE, TABLET]], [
+
+ /android.+[;\/]\s*(Le[\s\-]+Pan)[\s\-]+(.*\b)\s+build/i // Le Pan Tablets
+ ], [VENDOR, MODEL, [TYPE, TABLET]], [
+
+ /android.+[;\/]\s*(Trio[\s\-]*.*)\s+build/i // MachSpeed Tablets
+ ], [MODEL, [VENDOR, 'MachSpeed'], [TYPE, TABLET]], [
+
+ /android.+[;\/]\s*(Trinity)[\-\s]*(T\d{3})\s+build/i // Trinity Tablets
+ ], [VENDOR, MODEL, [TYPE, TABLET]], [
+
+ /android.+[;\/]\s*TU_(1491)\s+build/i // Rotor Tablets
+ ], [MODEL, [VENDOR, 'Rotor'], [TYPE, TABLET]], [
+
+ /android.+(KS(.+))\s+build/i // Amazon Kindle Tablets
+ ], [MODEL, [VENDOR, 'Amazon'], [TYPE, TABLET]], [
+
+ /android.+(Gigaset)[\s\-]+(Q.+)\s+build/i // Gigaset Tablets
+ ], [VENDOR, MODEL, [TYPE, TABLET]], [
+
+ /\s(tablet|tab)[;\/]/i, // Unidentifiable Tablet
+ /\s(mobile)(?:[;\/]|\ssafari)/i // Unidentifiable Mobile
+ ], [[TYPE, util.lowerize], VENDOR, MODEL], [
+
+ /(android.+)[;\/].+build/i // Generic Android Device
+ ], [MODEL, [VENDOR, 'Generic']]
+
+
+ /*//////////////////////////
+ // TODO: move to string map
+ ////////////////////////////
+
+ /(C6603)/i // Sony Xperia Z C6603
+ ], [[MODEL, 'Xperia Z C6603'], [VENDOR, 'Sony'], [TYPE, MOBILE]], [
+ /(C6903)/i // Sony Xperia Z 1
+ ], [[MODEL, 'Xperia Z 1'], [VENDOR, 'Sony'], [TYPE, MOBILE]], [
+
+ /(SM-G900[F|H])/i // Samsung Galaxy S5
+ ], [[MODEL, 'Galaxy S5'], [VENDOR, 'Samsung'], [TYPE, MOBILE]], [
+ /(SM-G7102)/i // Samsung Galaxy Grand 2
+ ], [[MODEL, 'Galaxy Grand 2'], [VENDOR, 'Samsung'], [TYPE, MOBILE]], [
+ /(SM-G530H)/i // Samsung Galaxy Grand Prime
+ ], [[MODEL, 'Galaxy Grand Prime'], [VENDOR, 'Samsung'], [TYPE, MOBILE]], [
+ /(SM-G313HZ)/i // Samsung Galaxy V
+ ], [[MODEL, 'Galaxy V'], [VENDOR, 'Samsung'], [TYPE, MOBILE]], [
+ /(SM-T805)/i // Samsung Galaxy Tab S 10.5
+ ], [[MODEL, 'Galaxy Tab S 10.5'], [VENDOR, 'Samsung'], [TYPE, TABLET]], [
+ /(SM-G800F)/i // Samsung Galaxy S5 Mini
+ ], [[MODEL, 'Galaxy S5 Mini'], [VENDOR, 'Samsung'], [TYPE, MOBILE]], [
+ /(SM-T311)/i // Samsung Galaxy Tab 3 8.0
+ ], [[MODEL, 'Galaxy Tab 3 8.0'], [VENDOR, 'Samsung'], [TYPE, TABLET]], [
+
+ /(T3C)/i // Advan Vandroid T3C
+ ], [MODEL, [VENDOR, 'Advan'], [TYPE, TABLET]], [
+ /(ADVAN T1J\+)/i // Advan Vandroid T1J+
+ ], [[MODEL, 'Vandroid T1J+'], [VENDOR, 'Advan'], [TYPE, TABLET]], [
+ /(ADVAN S4A)/i // Advan Vandroid S4A
+ ], [[MODEL, 'Vandroid S4A'], [VENDOR, 'Advan'], [TYPE, MOBILE]], [
+
+ /(V972M)/i // ZTE V972M
+ ], [MODEL, [VENDOR, 'ZTE'], [TYPE, MOBILE]], [
+
+ /(i-mobile)\s(IQ\s[\d\.]+)/i // i-mobile IQ
+ ], [VENDOR, MODEL, [TYPE, MOBILE]], [
+ /(IQ6.3)/i // i-mobile IQ IQ 6.3
+ ], [[MODEL, 'IQ 6.3'], [VENDOR, 'i-mobile'], [TYPE, MOBILE]], [
+ /(i-mobile)\s(i-style\s[\d\.]+)/i // i-mobile i-STYLE
+ ], [VENDOR, MODEL, [TYPE, MOBILE]], [
+ /(i-STYLE2.1)/i // i-mobile i-STYLE 2.1
+ ], [[MODEL, 'i-STYLE 2.1'], [VENDOR, 'i-mobile'], [TYPE, MOBILE]], [
+
+ /(mobiistar touch LAI 512)/i // mobiistar touch LAI 512
+ ], [[MODEL, 'Touch LAI 512'], [VENDOR, 'mobiistar'], [TYPE, MOBILE]], [
+
+ /////////////
+ // END TODO
+ ///////////*/
+
+ ],
+
+ engine : [[
+
+ /windows.+\sedge\/([\w\.]+)/i // EdgeHTML
+ ], [VERSION, [NAME, 'EdgeHTML']], [
+
+ /(presto)\/([\w\.]+)/i, // Presto
+ /(webkit|trident|netfront|netsurf|amaya|lynx|w3m)\/([\w\.]+)/i, // WebKit/Trident/NetFront/NetSurf/Amaya/Lynx/w3m
+ /(khtml|tasman|links)[\/\s]\(?([\w\.]+)/i, // KHTML/Tasman/Links
+ /(icab)[\/\s]([23]\.[\d\.]+)/i // iCab
+ ], [NAME, VERSION], [
+
+ /rv\:([\w\.]+).*(gecko)/i // Gecko
+ ], [VERSION, NAME]
+ ],
+
+ os : [[
+
+ // Windows based
+ /microsoft\s(windows)\s(vista|xp)/i // Windows (iTunes)
+ ], [NAME, VERSION], [
+ /(windows)\snt\s6\.2;\s(arm)/i, // Windows RT
+ /(windows\sphone(?:\sos)*)[\s\/]?([\d\.\s]+\w)*/i, // Windows Phone
+ /(windows\smobile|windows)[\s\/]?([ntce\d\.\s]+\w)/i
+ ], [NAME, [VERSION, mapper.str, maps.os.windows.version]], [
+ /(win(?=3|9|n)|win\s9x\s)([nt\d\.]+)/i
+ ], [[NAME, 'Windows'], [VERSION, mapper.str, maps.os.windows.version]], [
+
+ // Mobile/Embedded OS
+ /\((bb)(10);/i // BlackBerry 10
+ ], [[NAME, 'BlackBerry'], VERSION], [
+ /(blackberry)\w*\/?([\w\.]+)*/i, // Blackberry
+ /(tizen)[\/\s]([\w\.]+)/i, // Tizen
+ /(android|webos|palm\sos|qnx|bada|rim\stablet\sos|meego|contiki)[\/\s-]?([\w\.]+)*/i,
+ // Android/WebOS/Palm/QNX/Bada/RIM/MeeGo/Contiki
+ /linux;.+(sailfish);/i // Sailfish OS
+ ], [NAME, VERSION], [
+ /(symbian\s?os|symbos|s60(?=;))[\/\s-]?([\w\.]+)*/i // Symbian
+ ], [[NAME, 'Symbian'], VERSION], [
+ /\((series40);/i // Series 40
+ ], [NAME], [
+ /mozilla.+\(mobile;.+gecko.+firefox/i // Firefox OS
+ ], [[NAME, 'Firefox OS'], VERSION], [
+
+ // Console
+ /(nintendo|playstation)\s([wids34portablevu]+)/i, // Nintendo/Playstation
+
+ // GNU/Linux based
+ /(mint)[\/\s\(]?(\w+)*/i, // Mint
+ /(mageia|vectorlinux)[;\s]/i, // Mageia/VectorLinux
+ /(joli|[kxln]?ubuntu|debian|[open]*suse|gentoo|(?=\s)arch|slackware|fedora|mandriva|centos|pclinuxos|redhat|zenwalk|linpus)[\/\s-]?(?!chrom)([\w\.-]+)*/i,
+ // Joli/Ubuntu/Debian/SUSE/Gentoo/Arch/Slackware
+ // Fedora/Mandriva/CentOS/PCLinuxOS/RedHat/Zenwalk/Linpus
+ /(hurd|linux)\s?([\w\.]+)*/i, // Hurd/Linux
+ /(gnu)\s?([\w\.]+)*/i // GNU
+ ], [NAME, VERSION], [
+
+ /(cros)\s[\w]+\s([\w\.]+\w)/i // Chromium OS
+ ], [[NAME, 'Chromium OS'], VERSION],[
+
+ // Solaris
+ /(sunos)\s?([\w\.]+\d)*/i // Solaris
+ ], [[NAME, 'Solaris'], VERSION], [
+
+ // BSD based
+ /\s([frentopc-]{0,4}bsd|dragonfly)\s?([\w\.]+)*/i // FreeBSD/NetBSD/OpenBSD/PC-BSD/DragonFly
+ ], [NAME, VERSION],[
+
+ /(haiku)\s(\w+)/i // Haiku
+ ], [NAME, VERSION],[
+
+ /cfnetwork\/.+darwin/i,
+ /ip[honead]+(?:.*os\s([\w]+)\slike\smac|;\sopera)/i // iOS
+ ], [[VERSION, /_/g, '.'], [NAME, 'iOS']], [
+
+ /(mac\sos\sx)\s?([\w\s\.]+\w)*/i,
+ /(macintosh|mac(?=_powerpc)\s)/i // Mac OS
+ ], [[NAME, 'Mac OS'], [VERSION, /_/g, '.']], [
+
+ // Other
+ /((?:open)?solaris)[\/\s-]?([\w\.]+)*/i, // Solaris
+ /(aix)\s((\d)(?=\.|\)|\s)[\w\.]*)*/i, // AIX
+ /(plan\s9|minix|beos|os\/2|amigaos|morphos|risc\sos|openvms)/i,
+ // Plan9/Minix/BeOS/OS2/AmigaOS/MorphOS/RISCOS/OpenVMS
+ /(unix)\s?([\w\.]+)*/i // UNIX
+ ], [NAME, VERSION]
+ ]
+ };
+
+
+ /////////////////
+ // Constructor
+ ////////////////
+ /*
+ var Browser = function (name, version) {
+ this[NAME] = name;
+ this[VERSION] = version;
+ };
+ var CPU = function (arch) {
+ this[ARCHITECTURE] = arch;
+ };
+ var Device = function (vendor, model, type) {
+ this[VENDOR] = vendor;
+ this[MODEL] = model;
+ this[TYPE] = type;
+ };
+ var Engine = Browser;
+ var OS = Browser;
+ */
+ var UAParser = function (uastring, extensions) {
+
+ if (typeof uastring === 'object') {
+ extensions = uastring;
+ uastring = undefined;
+ }
+
+ if (!(this instanceof UAParser)) {
+ return new UAParser(uastring, extensions).getResult();
+ }
+
+ var ua = uastring || ((window && window.navigator && window.navigator.userAgent) ? window.navigator.userAgent : EMPTY);
+ var rgxmap = extensions ? util.extend(regexes, extensions) : regexes;
+ //var browser = new Browser();
+ //var cpu = new CPU();
+ //var device = new Device();
+ //var engine = new Engine();
+ //var os = new OS();
+
+ this.getBrowser = function () {
+ var browser = { name: undefined, version: undefined };
+ mapper.rgx.call(browser, ua, rgxmap.browser);
+ browser.major = util.major(browser.version); // deprecated
+ return browser;
+ };
+ this.getCPU = function () {
+ var cpu = { architecture: undefined };
+ mapper.rgx.call(cpu, ua, rgxmap.cpu);
+ return cpu;
+ };
+ this.getDevice = function () {
+ var device = { vendor: undefined, model: undefined, type: undefined };
+ mapper.rgx.call(device, ua, rgxmap.device);
+ return device;
+ };
+ this.getEngine = function () {
+ var engine = { name: undefined, version: undefined };
+ mapper.rgx.call(engine, ua, rgxmap.engine);
+ return engine;
+ };
+ this.getOS = function () {
+ var os = { name: undefined, version: undefined };
+ mapper.rgx.call(os, ua, rgxmap.os);
+ return os;
+ };
+ this.getResult = function () {
+ return {
+ ua : this.getUA(),
+ browser : this.getBrowser(),
+ engine : this.getEngine(),
+ os : this.getOS(),
+ device : this.getDevice(),
+ cpu : this.getCPU()
+ };
+ };
+ this.getUA = function () {
+ return ua;
+ };
+ this.setUA = function (uastring) {
+ ua = uastring;
+ //browser = new Browser();
+ //cpu = new CPU();
+ //device = new Device();
+ //engine = new Engine();
+ //os = new OS();
+ return this;
+ };
+ return this;
+ };
+
+ UAParser.VERSION = LIBVERSION;
+ UAParser.BROWSER = {
+ NAME : NAME,
+ MAJOR : MAJOR, // deprecated
+ VERSION : VERSION
+ };
+ UAParser.CPU = {
+ ARCHITECTURE : ARCHITECTURE
+ };
+ UAParser.DEVICE = {
+ MODEL : MODEL,
+ VENDOR : VENDOR,
+ TYPE : TYPE,
+ CONSOLE : CONSOLE,
+ MOBILE : MOBILE,
+ SMARTTV : SMARTTV,
+ TABLET : TABLET,
+ WEARABLE: WEARABLE,
+ EMBEDDED: EMBEDDED
+ };
+ UAParser.ENGINE = {
+ NAME : NAME,
+ VERSION : VERSION
+ };
+ UAParser.OS = {
+ NAME : NAME,
+ VERSION : VERSION
+ };
+ //UAParser.Utils = util;
+
+ ///////////
+ // Export
+ //////////
+
+
+ // check js environment
+ if (typeof(exports) !== UNDEF_TYPE) {
+ // nodejs env
+ if (typeof module !== UNDEF_TYPE && module.exports) {
+ exports = module.exports = UAParser;
+ }
+ // TODO: test!!!!!!!!
+ /*
+ if (require && require.main === module && process) {
+ // cli
+ var jsonize = function (arr) {
+ var res = [];
+ for (var i in arr) {
+ res.push(new UAParser(arr[i]).getResult());
+ }
+ process.stdout.write(JSON.stringify(res, null, 2) + '\n');
+ };
+ if (process.stdin.isTTY) {
+ // via args
+ jsonize(process.argv.slice(2));
+ } else {
+ // via pipe
+ var str = '';
+ process.stdin.on('readable', function() {
+ var read = process.stdin.read();
+ if (read !== null) {
+ str += read;
+ }
+ });
+ process.stdin.on('end', function () {
+ jsonize(str.replace(/\n$/, '').split('\n'));
+ });
+ }
+ }
+ */
+ exports.UAParser = UAParser;
+ } else {
+ // requirejs env (optional)
+ if (typeof(define) === FUNC_TYPE && define.amd) {
+ define(function () {
+ return UAParser;
+ });
+ } else if (window) {
+ // browser env
+ window.UAParser = UAParser;
+ }
+ }
+
+ // jQuery/Zepto specific (optional)
+ // Note:
+ // In AMD env the global scope should be kept clean, but jQuery is an exception.
+ // jQuery always exports to global scope, unless jQuery.noConflict(true) is used,
+ // and we should catch that.
+ var $ = window && (window.jQuery || window.Zepto);
+ if (typeof $ !== UNDEF_TYPE) {
+ var parser = new UAParser();
+ $.ua = parser.getResult();
+ $.ua.get = function () {
+ return parser.getUA();
+ };
+ $.ua.set = function (uastring) {
+ parser.setUA(uastring);
+ var result = parser.getResult();
+ for (var prop in result) {
+ $.ua[prop] = result[prop];
+ }
+ };
+ }
+
+})(typeof window === 'object' ? window : this);
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/browser-test.json b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/browser-test.json
new file mode 100644
index 00000000..3efba3ff
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/browser-test.json
@@ -0,0 +1,982 @@
+[
+ {
+ "desc" : "Android Browser on Galaxy Nexus",
+ "ua" : "Mozilla/5.0 (Linux; U; Android 4.0.2; en-us; Galaxy Nexus Build/ICL53F) AppleWebKit/534.30 (KHTML, like Gecko) Version/4.0 Mobile Safari/534.30",
+ "expect" :
+ {
+ "name" : "Android Browser",
+ "version" : "4.0",
+ "major" : "4"
+ }
+ },
+ {
+ "desc" : "Android Browser on Galaxy S3",
+ "ua" : "Mozilla/5.0 (Linux; Android 4.4.4; en-us; SAMSUNG GT-I9300I Build/KTU84P) AppleWebKit/537.36 (KHTML, like Gecko) Version/1.5 Chrome/28.0.1500.94 Mobile Safari/537.36",
+ "expect" :
+ {
+ "name" : "Android Browser",
+ "version" : "1.5",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "Android Browser on HTC Flyer (P510E)",
+ "ua" : "Mozilla/5.0 (Linux; U; Android 3.2.1; ru-ru; HTC Flyer P510e Build/HTK75C) AppleWebKit/534.13 (KHTML, like Gecko) Version/4.0 Safari/534.13",
+ "expect" :
+ {
+ "name" : "Android Browser",
+ "version" : "4.0",
+ "major" : "4"
+ }
+ },
+ {
+ "desc" : "Android Browser on Huawei Honor Glory II (U9508)",
+ "ua" : "Mozilla/5.0 (Linux; U; Android 4.0.4; ru-by; HUAWEI U9508 Build/HuaweiU9508) AppleWebKit/534.30 (KHTML, like Gecko) Version/4.0 Mobile Safari/534.30 ACHEETAHI/2100050044",
+ "expect" :
+ {
+ "name" : "Android Browser",
+ "version" : "4.0",
+ "major" : "4"
+ }
+ },
+ {
+ "desc" : "Android Browser on Huawei P8 (H891L)",
+ "ua" : "Mozilla/5.0 (Linux; Android 4.4.4; HUAWEI H891L Build/HuaweiH891L) AppleWebKit/537.36 (KHTML, like Gecko) Version/4.0 Chrome/33.0.0.0 Mobile Safari/537.36",
+ "expect" :
+ {
+ "name" : "Android Browser",
+ "version" : "4.0",
+ "major" : "4"
+ }
+ },
+ {
+ "desc" : "Android Browser on Samsung S6 (SM-G925F)",
+ "ua" : "Mozilla/5.0 (Linux; Android 5.0.2; SAMSUNG SM-G925F Build/LRX22G) AppleWebKit/537.36 (KHTML, like Gecko) SamsungBrowser/3.0 Chrome/38.0.2125.102 Mobile Safari/537.36",
+ "expect" :
+ {
+ "name" : "Samsung Browser",
+ "version" : "3.0",
+ "major" : "3"
+ }
+ },
+ {
+ "desc" : "Arora",
+ "ua" : "Mozilla/5.0 (Windows; U; Windows NT 5.1; de-CH) AppleWebKit/523.15 (KHTML, like Gecko, Safari/419.3) Arora/0.2",
+ "expect" :
+ {
+ "name" : "Arora",
+ "version" : "0.2",
+ "major" : "0"
+ }
+ },
+ {
+ "desc" : "Avant",
+ "ua" : "Mozilla/4.0 (compatible; MSIE 8.0; Windows NT 5.1; Trident/4.0; GTB5; Avant Browser; .NET CLR 1.1.4322; .NET CLR 2.0.50727)",
+ "expect" :
+ {
+ "name" : "Avant ",
+ "version" : "undefined",
+ "major" : "undefined"
+ }
+ },
+ {
+ "desc" : "Baidu",
+ "ua" : "Mozilla/4.0 (compatible; MSIE 6.0; Windows NT 5.1; SV1; baidubrowser 1.x)",
+ "expect" :
+ {
+ "name" : "baidu",
+ "version" : "1.x",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "Bolt",
+ "ua" : "Mozilla/5.0 (X11; 78; CentOS; US-en) AppleWebKit/527+ (KHTML, like Gecko) Bolt/0.862 Version/3.0 Safari/523.15",
+ "expect" :
+ {
+ "name" : "Bolt",
+ "version" : "0.862",
+ "major" : "0"
+ }
+ },
+ {
+ "desc" : "Bowser",
+ "ua" : "Mozilla/5.0 (iOS; like Mac OS X) AppleWebKit/536.36 (KHTML, like Gecko) not Chrome/27.0.1500.95 Mobile/10B141 Safari/537.36 Bowser/0.2.1",
+ "expect" :
+ {
+ "name" : "Bowser",
+ "version" : "0.2.1",
+ "major" : "0"
+ }
+ },
+ {
+ "desc" : "Camino",
+ "ua" : "Mozilla/5.0 (Macintosh; U; PPC Mac OS X 10.4; en; rv:1.9.0.19) Gecko/2011091218 Camino/2.0.9 (like Firefox/3.0.19)",
+ "expect" :
+ {
+ "name" : "Camino",
+ "version" : "2.0.9",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "Chimera",
+ "ua" : "Mozilla/5.0 (Macintosh; U; PPC Mac OS X; pl-PL; rv:1.0.1) Gecko/20021111 Chimera/0.6",
+ "expect" :
+ {
+ "name" : "Chimera",
+ "version" : "0.6",
+ "major" : "0"
+ }
+ },
+ {
+ "desc" : "Chrome",
+ "ua" : "Mozilla/5.0 (Windows NT 6.2) AppleWebKit/536.6 (KHTML, like Gecko) Chrome/20.0.1090.0 Safari/536.6",
+ "expect" :
+ {
+ "name" : "Chrome",
+ "version" : "20.0.1090.0",
+ "major" : "20"
+ }
+ },
+ {
+ "desc" : "Chrome Headless",
+ "ua" : "Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) HeadlessChrome Safari/537.36",
+ "expect" :
+ {
+ "name" : "Chrome Headless",
+ "version" : "undefined",
+ "major" : "undefined"
+ }
+ },
+ {
+ "desc" : "Chrome Headless",
+ "ua" : "Mozilla/5.0 (Macintosh; Intel Mac OS X 10_12_6) AppleWebKit/537.36 (KHTML, like Gecko) HeadlessChrome/60.0.3112.113 Safari/537.36",
+ "expect" :
+ {
+ "name" : "Chrome Headless",
+ "version" : "60.0.3112.113",
+ "major" : "60"
+ }
+ },
+ {
+ "desc" : "Chrome WebView",
+ "ua" : "Mozilla/5.0 (Linux; Android 5.1.1; Nexus 5 Build/LMY48B; wv) AppleWebKit/537.36 (KHTML, like Gecko) Version/4.0 Chrome/43.0.2357.65 Mobile Safari/537.36",
+ "expect" :
+ {
+ "name" : "Chrome WebView",
+ "version" : "43.0.2357.65",
+ "major" : "43"
+ }
+ },
+ {
+ "desc" : "Chrome on iOS",
+ "ua" : "Mozilla/5.0 (iPhone; U; CPU iPhone OS 5_1_1 like Mac OS X; en) AppleWebKit/534.46.0 (KHTML, like Gecko) CriOS/19.0.1084.60 Mobile/9B206 Safari/7534.48.3",
+ "expect" :
+ {
+ "name" : "Chrome",
+ "version" : "19.0.1084.60",
+ "major" : "19"
+ }
+ },
+ {
+ "desc" : "Chromium",
+ "ua" : "Mozilla/5.0 (X11; Linux i686) AppleWebKit/535.7 (KHTML, like Gecko) Ubuntu/11.10 Chromium/16.0.912.21 Chrome/16.0.912.21 Safari/535.7",
+ "expect" :
+ {
+ "name" : "Chromium",
+ "version" : "16.0.912.21",
+ "major" : "16"
+ }
+ },
+ {
+ "desc" : "Chrome on Android",
+ "ua" : "Mozilla/5.0 (Linux; U; Android-4.0.3; en-us; Galaxy Nexus Build/IML74K) AppleWebKit/535.7 (KHTML, like Gecko) CrMo/16.0.912.75 Mobile Safari/535.7",
+ "expect" :
+ {
+ "name" : "Chrome",
+ "version" : "16.0.912.75",
+ "major" : "16"
+ }
+ },
+ {
+ "desc" : "Dillo",
+ "ua" : "Dillo/2.2",
+ "expect" :
+ {
+ "name" : "Dillo",
+ "version" : "2.2",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "Dolphin",
+ "ua" : "Mozilla/5.0 (SCH-F859/F859DG12;U;NUCLEUS/2.1;Profile/MIDP-2.1 Configuration/CLDC-1.1;480*800;CTC/2.0) Dolfin/2.0",
+ "expect" :
+ {
+ "name" : "Dolphin",
+ "version" : "2.0",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "Doris",
+ "ua" : "Doris/1.15 [en] (Symbian)",
+ "expect" :
+ {
+ "name" : "Doris",
+ "version" : "1.15",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "Epiphany",
+ "ua" : "Mozilla/5.0 (X11; U; FreeBSD i386; en-US; rv:1.7) Gecko/20040628 Epiphany/1.2.6",
+ "expect" :
+ {
+ "name" : "Epiphany",
+ "version" : "1.2.6",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "Facebook in-App Browser for Android",
+ "ua" : "Mozilla/5.0 (Linux; Android 5.0; SM-G900P Build/LRX21T; wv) AppleWebKit/537.36 (KHTML, like Gecko) Version/4.0 Chrome/43.0.2357.121 Mobile Safari/537.36 [FB_IAB/FB4A;FBAV/35.0.0.48.273;]",
+ "expect" :
+ {
+ "name" : "Facebook",
+ "version" : "35.0.0.48.273",
+ "major" : "35"
+ }
+ },
+ {
+ "desc" : "Facebook in-App Browser for iOS",
+ "ua" : "Mozilla/5.0 (iPhone; CPU iPhone OS 10_3_1 like Mac OS X) AppleWebKit/603.1.30 (KHTML, like Gecko) Mobile/14E304 [FBAN/FBIOS;FBAV/91.0.0.41.73;FBBV/57050710;FBDV/iPhone8,1;FBMD/iPhone;FBSN/iOS;FBSV/10.3.1;FBSS/2;FBCR/Telekom.de;FBID/phone;FBLC/de_DE;FBOP/5;FBRV/0])",
+ "expect" :
+ {
+ "name" : "Facebook",
+ "version" : "91.0.0.41.73",
+ "major" : "91"
+ }
+ },
+ {
+ "desc" : "Firebird",
+ "ua" : "Mozilla/5.0 (Windows; U; Win98; en-US; rv:1.5) Gecko/20031007 Firebird/0.7",
+ "expect" :
+ {
+ "name" : "Firebird",
+ "version" : "0.7",
+ "major" : "0"
+ }
+ },
+ {
+ "desc" : "Firefox",
+ "ua" : "Mozilla/5.0 (Windows NT 6.1; rv:15.0) Gecko/20120716 Firefox/15.0a2",
+ "expect" :
+ {
+ "name" : "Firefox",
+ "version" : "15.0a2",
+ "major" : "15"
+ }
+ },
+ {
+ "desc" : "Fennec",
+ "ua" : "Mozilla/5.0 (X11; U; Linux armv61; en-US; rv:1.9.1b2pre) Gecko/20081015 Fennec/1.0a1",
+ "expect" :
+ {
+ "name" : "Fennec",
+ "version" : "1.0a1",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "Flock",
+ "ua" : "Mozilla/5.0 (X11; U; Linux i686; en-US; rv:1.9.0.3) Gecko/2008100716 Firefox/3.0.3 Flock/2.0",
+ "expect" :
+ {
+ "name" : "Flock",
+ "version" : "2.0",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "GoBrowser",
+ "ua" : "Nokia5700XpressMusic/GoBrowser/1.6.91",
+ "expect" :
+ {
+ "name" : "GoBrowser",
+ "version" : "1.6.91",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "IceApe",
+ "ua" : "Mozilla/5.0 (X11; U; Linux i686; en-US; rv:1.9.1.19) Gecko/20110817 Iceape/2.0.14",
+ "expect" :
+ {
+ "name" : "Iceape",
+ "version" : "2.0.14",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "IceCat",
+ "ua" : "Mozilla/5.0 (X11; U; Linux i686; en-US; rv:1.9.0.3) Gecko/2008092921 IceCat/3.0.3-g1",
+ "expect" :
+ {
+ "name" : "IceCat",
+ "version" : "3.0.3-g1",
+ "major" : "3"
+ }
+ },
+ {
+ "desc" : "Iceweasel",
+ "ua" : "Mozilla/5.0 (X11; U; Linux i686; de; rv:1.9.0.16) Gecko/2009121610 Iceweasel/3.0.6 (Debian-3.0.6-3)",
+ "expect" :
+ {
+ "name" : "Iceweasel",
+ "version" : "3.0.6",
+ "major" : "3"
+ }
+ },
+ {
+ "desc" : "iCab",
+ "ua" : "iCab/2.9.5 (Macintosh; U; PPC; Mac OS X)",
+ "expect" :
+ {
+ "name" : "iCab",
+ "version" : "2.9.5",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "IEMobile",
+ "ua" : "Mozilla/4.0 (compatible; MSIE 6.0; Windows CE; IEMobile 7.11) 320x240; VZW; Motorola-Q9c; Windows Mobile 6.1 Standard",
+ "expect" :
+ {
+ "name" : "IEMobile",
+ "version" : "7.11",
+ "major" : "7"
+ }
+ },
+ {
+ "desc" : "IE 11 with IE token",
+ "ua" : "Mozilla/5.0 (IE 11.0; Windows NT 6.3; WOW64; Trident/7.0; rv:11.0) like Gecko",
+ "expect" :
+ {
+ "name" : "IE",
+ "version" : "11.0",
+ "major" : "11"
+ }
+ },
+ {
+ "desc" : "IE 11 without IE token",
+ "ua" : "Mozilla/5.0 (Windows NT 6.3; Trident/7.0; rv 11.0) like Gecko",
+ "expect" :
+ {
+ "name" : "IE",
+ "version" : "11.0",
+ "major" : "11"
+ }
+ },
+ {
+ "desc" : "K-Meleon",
+ "ua" : "Mozilla/5.0 (Windows; U; Win98; en-US; rv:1.5) Gecko/20031016 K-Meleon/0.8.2",
+ "expect" :
+ {
+ "name" : "K-Meleon",
+ "version" : "0.8.2",
+ "major" : "0"
+ }
+ },
+ {
+ "desc" : "Kindle Browser",
+ "ua" : "Mozilla/4.0 (compatible; Linux 2.6.22) NetFront/3.4 Kindle/2.5 (screen 600x800; rotate)",
+ "expect" :
+ {
+ "name" : "Kindle",
+ "version" : "2.5",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "Konqueror",
+ "ua" : "Mozilla/5.0 (compatible; Konqueror/3.5; Linux; X11; x86_64) KHTML/3.5.6 (like Gecko) (Kubuntu)",
+ "expect" :
+ {
+ "name" : "Konqueror",
+ "version" : "3.5",
+ "major" : "3"
+ }
+ },
+ {
+ "desc" : "Lunascape",
+ "ua" : "Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.9.1.2) Gecko/20090804 Firefox/3.5.2 Lunascape/5.1.4.5",
+ "expect" :
+ {
+ "name" : "Lunascape",
+ "version" : "5.1.4.5",
+ "major" : "5"
+ }
+ },
+ {
+ "desc" : "Lynx",
+ "ua" : "Lynx/2.8.5dev.16 libwww-FM/2.14 SSL-MM/1.4.1 OpenSSL/0.9.6b",
+ "expect" :
+ {
+ "name" : "Lynx",
+ "version" : "2.8.5dev.16",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "Maemo Browser",
+ "ua" : "Mozilla/5.0 (X11; U; Linux armv7l; ru-RU; rv:1.9.2.3pre) Gecko/20100723 Firefox/3.5 Maemo Browser 1.7.4.8 RX-51 N900",
+ "expect" :
+ {
+ "name" : "Maemo Browser",
+ "version" : "1.7.4.8",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "Maxthon",
+ "ua" : "Mozilla/4.0 (compatible; MSIE 7.0; Windows NT 5.1; SV1; Maxthon; .NET CLR 1.1.4322)",
+ "expect" :
+ {
+ "name" : "Maxthon",
+ "version" : "undefined",
+ "major" : "undefined"
+ }
+ },
+ {
+ "desc" : "Midori",
+ "ua" : "Midori/0.2.2 (X11; Linux i686; U; en-us) WebKit/531.2+",
+ "expect" :
+ {
+ "name" : "Midori",
+ "version" : "0.2.2",
+ "major" : "0"
+ }
+ },
+ {
+ "desc" : "Minimo",
+ "ua" : "Mozilla/5.0 (X11; U; Linux armv6l; rv 1.8.1.5pre) Gecko/20070619 Minimo/0.020",
+ "expect" :
+ {
+ "name" : "Minimo",
+ "version" : "0.020",
+ "major" : "0"
+ }
+ },
+ {
+ "desc" : "MIUI Browser on Xiaomi Hongmi WCDMA (HM2013023)",
+ "ua" : "Mozilla/5.0 (Linux; U; Android 4.2.2; ru-ru; 2013023 Build/HM2013023) AppleWebKit/534.30 (KHTML, like Gecko) Version/4.0 Mobile Safari/534.30 XiaoMi/MiuiBrowser/1.0",
+ "expect" :
+ {
+ "name" : "MIUI Browser",
+ "version" : "1.0",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "Mobile Safari",
+ "ua" : "Mozilla/5.0 (iPhone; U; CPU iPhone OS 4_0 like Mac OS X; en-us) AppleWebKit/532.9 (KHTML, like Gecko) Version/4.0.5 Mobile/8A293 Safari/6531.22.7",
+ "expect" :
+ {
+ "name" : "Mobile Safari",
+ "version" : "4.0.5",
+ "major" : "4"
+ }
+ },
+ {
+ "desc" : "Mosaic",
+ "ua" : "NCSA_Mosaic/2.6 (X11; SunOS 4.1.3 sun4m)",
+ "expect" :
+ {
+ "name" : "Mosaic",
+ "version" : "2.6",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "Mozilla",
+ "ua" : "Mozilla/5.0 (X11; U; SunOS sun4u; en-US; rv:1.7) Gecko/20070606",
+ "expect" :
+ {
+ "name" : "Mozilla",
+ "version" : "5.0",
+ "major" : "5"
+ }
+ },
+ {
+ "desc" : "MSIE",
+ "ua" : "Mozilla/4.0 (compatible; MSIE 5.0b1; Mac_PowerPC)",
+ "expect" :
+ {
+ "name" : "IE",
+ "version" : "5.0b1",
+ "major" : "5"
+ }
+ },
+ {
+ "desc" : "NetFront",
+ "ua" : "Mozilla/4.0 (PDA; Windows CE/1.0.1) NetFront/3.0",
+ "expect" :
+ {
+ "name" : "NetFront",
+ "version" : "3.0",
+ "major" : "3"
+ }
+ },
+ {
+ "desc" : "Netscape on Windows ME",
+ "ua" : "Mozilla/5.0 (Windows; U; Win 9x 4.90; en-US; rv:1.8.1.8pre) Gecko/20071015 Firefox/2.0.0.7 Navigator/9.0",
+ "expect" :
+ {
+ "name" : "Netscape",
+ "version" : "9.0",
+ "major" : "9"
+ }
+ },
+ {
+ "desc" : "Netscape on Windows 2000",
+ "ua" : "Mozilla/5.0 (Windows; U; Windows NT 5.0; en-US; rv:1.7.5) Gecko/20050519 Netscape/8.0.1",
+ "expect" :
+ {
+ "name" : "Netscape",
+ "version" : "8.0.1",
+ "major" : "8"
+ }
+ },
+ {
+ "desc" : "Nokia Browser",
+ "ua" : "Mozilla/5.0 (Symbian/3; Series60/5.2 NokiaN8-00/025.007; Profile/MIDP-2.1 Configuration/CLDC-1.1 ) AppleWebKit/533.4 (KHTML, like Gecko) NokiaBrowser/7.3.1.37 Mobile Safari/533.4 3gpp-gba",
+ "expect" :
+ {
+ "name" : "NokiaBrowser",
+ "version" : "7.3.1.37",
+ "major" : "7"
+ }
+ },
+ {
+ "desc" : "Oculus Browser",
+ "ua" : "Mozilla/5.0 (Linux; Android 7.0; SM-G920I Build/NRD90M) AppleWebKit/537.36 (KHTML, like Gecko) OculusBrowser/3.4.9 SamsungBrowser/4.0 Chrome/57.0.2987.146 Mobile VR Safari/537.36",
+ "expect" :
+ {
+ "name" : "Oculus Browser",
+ "version" : "3.4.9",
+ "major" : "3"
+ }
+ },
+ {
+ "desc" : "OmniWeb",
+ "ua" : "Mozilla/5.0 (Macintosh; U; PPC Mac OS X; en-US) AppleWebKit/85 (KHTML, like Gecko) OmniWeb/v558.48",
+ "expect" :
+ {
+ "name" : "OmniWeb",
+ "version" : "558.48",
+ "major" : "558"
+ }
+ },
+ {
+ "desc" : "Opera > 9.80",
+ "ua" : "Opera/9.80 (X11; Linux x86_64; U; Linux Mint; en) Presto/2.2.15 Version/10.10",
+ "expect" :
+ {
+ "name" : "Opera",
+ "version" : "10.10",
+ "major" : "10"
+ }
+ },
+ {
+ "desc" : "Opera < 9.80 on Windows",
+ "ua" : "Mozilla/4.0 (compatible; MSIE 5.0; Windows 95) Opera 6.01 [en]",
+ "expect" :
+ {
+ "name" : "Opera",
+ "version" : "6.01",
+ "major" : "6"
+ }
+ },
+ {
+ "desc" : "Opera < 9.80 on OSX",
+ "ua" : "Opera/8.5 (Macintosh; PPC Mac OS X; U; en)",
+ "expect" :
+ {
+ "name" : "Opera",
+ "version" : "8.5",
+ "major" : "8"
+ }
+ },
+ {
+ "desc" : "Opera Mobile",
+ "ua" : "Opera/9.80 (Android 2.3.5; Linux; Opera Mobi/ADR-1111101157; U; de) Presto/2.9.201 Version/11.50",
+ "expect" :
+ {
+ "name" : "Opera Mobi",
+ "version" : "11.50",
+ "major" : "11"
+ }
+ },
+ {
+ "desc" : "Opera Webkit",
+ "ua" : "Mozilla/5.0 AppleWebKit/537.22 (KHTML, like Gecko) Chrome/25.0.1364.123 Mobile Safari/537.22 OPR/14.0.1025.52315",
+ "expect" :
+ {
+ "name" : "Opera",
+ "version" : "14.0.1025.52315",
+ "major" : "14"
+ }
+ },
+ {
+ "desc" : "Opera Mini",
+ "ua" : "Opera/9.80 (J2ME/MIDP; Opera Mini/5.1.21214/19.916; U; en) Presto/2.5.25",
+ "expect" :
+ {
+ "name" : "Opera Mini",
+ "version" : "5.1.21214",
+ "major" : "5"
+ }
+ },
+ {
+ "desc" : "Opera Mini 8 above on iPhone",
+ "ua" : "Mozilla/5.0 (iPhone; CPU iPhone OS 9_2 like Mac OS X) AppleWebKit/601.1.46 (KHTML, like Gecko) OPiOS/12.1.1.98980 Mobile/13C75 Safari/9537.53",
+ "expect" :
+ {
+ "name" : "Opera Mini",
+ "version" : "12.1.1.98980",
+ "major" : "12"
+ }
+ },
+ {
+ "desc" : "Opera Tablet",
+ "ua" : "Opera/9.80 (Windows NT 6.1; Opera Tablet/15165; U; en) Presto/2.8.149 Version/11.1",
+ "expect" :
+ {
+ "name" : "Opera Tablet",
+ "version" : "11.1",
+ "major" : "11"
+ }
+ },
+ {
+ "desc" : "Opera Coast",
+ "ua" : "Mozilla/5.0 (iPhone; CPU iPhone OS 9_3_2 like Mac OS X; en) AppleWebKit/601.1.46 (KHTML, like Gecko) Coast/5.04.110603 Mobile/13F69 Safari/7534.48.3",
+ "expect" :
+ {
+ "name" : "Opera Coast",
+ "version" : "5.04.110603",
+ "major" : "5"
+ }
+ },
+ {
+ "desc" : "PhantomJS",
+ "ua" : "Mozilla/5.0 (Windows NT 6.1; WOW64) AppleWebKit/534.34 (KHTML, like Gecko) PhantomJS/1.9.2 Safari/534.34",
+ "expect" :
+ {
+ "name" : "PhantomJS",
+ "version" : "1.9.2",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "Phoenix",
+ "ua" : "Mozilla/5.0 (X11; U; Linux i686; en-US; rv:1.2b) Gecko/20021029 Phoenix/0.4",
+ "expect" :
+ {
+ "name" : "Phoenix",
+ "version" : "0.4",
+ "major" : "0"
+ }
+ },
+ {
+ "desc" : "Polaris",
+ "ua" : "LG-LX600 Polaris/6.0 MMP/2.0 Profile/MIDP-2.1 Configuration/CLDC-1.1",
+ "expect" :
+ {
+ "name" : "Polaris",
+ "version" : "6.0",
+ "major" : "6"
+ }
+ },
+ {
+ "desc" : "QQ",
+ "ua" : "Mozilla/5.0 (Linux; U; Android 4.4.4; zh-cn; OPPO R7s Build/KTU84P) AppleWebKit/537.36 (KHTML, like Gecko)Version/4.0 Chrome/37.0.0.0 MQQBrowser/7.1 Mobile Safari/537.36",
+ "expect" :
+ {
+ "name" : "QQBrowser",
+ "version" : "7.1",
+ "major" : "7"
+ }
+ },
+ {
+ "desc" : "RockMelt",
+ "ua" : "Mozilla/5.0 (Windows; U; Windows NT 6.1; en-US) AppleWebKit/534.7 (KHTML, like Gecko) RockMelt/0.8.36.78 Chrome/7.0.517.44 Safari/534.7",
+ "expect" :
+ {
+ "name" : "RockMelt",
+ "version" : "0.8.36.78",
+ "major" : "0"
+ }
+ },
+ {
+ "desc" : "Safari",
+ "ua" : "Mozilla/5.0 (Windows; U; Windows NT 5.2; en-US) AppleWebKit/533.17.8 (KHTML, like Gecko) Version/5.0.1 Safari/533.17.8",
+ "expect" :
+ {
+ "name" : "Safari",
+ "version" : "5.0.1",
+ "major" : "5"
+ }
+ },
+ {
+ "desc" : "Safari < 3.0",
+ "ua" : "Mozilla/5.0 (Macintosh; U; PPC Mac OS X; sv-se) AppleWebKit/419 (KHTML, like Gecko) Safari/419.3",
+ "expect" :
+ {
+ "name" : "Safari",
+ "version" : "2.0.4",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "Samsung Browser",
+ "ua" : "Mozilla/5.0 (Linux; Android 6.0.1; SAMSUNG-SM-G925A Build/MMB29K) AppleWebKit/537.36 (KHTML, like Gecko) SamsungBrowser/4.0 Chrome/44.0.2403.133 Mobile Safari/537.36",
+ "expect" :
+ {
+ "name" : "Samsung Browser",
+ "version" : "4.0",
+ "major" : "4"
+ }
+ },
+ {
+ "desc" : "SeaMonkey",
+ "ua" : "Mozilla/5.0 (X11; U; Linux i686; en-US; rv:1.9.1b4pre) Gecko/20090405 SeaMonkey/2.0b1pre",
+ "expect" :
+ {
+ "name" : "SeaMonkey",
+ "version" : "2.0b1pre",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "Silk Browser",
+ "ua" : "Mozilla/5.0 (Macintosh; U; Intel Mac OS X 10_6_3; en-us; Silk/1.1.0-84)",
+ "expect" :
+ {
+ "name" : "Silk",
+ "version" : "1.1.0-84",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "Skyfire",
+ "ua" : "Mozilla/5.0 (Macintosh; U; Intel Mac OS X 10_5_7; en-us) AppleWebKit/530.17 (KHTML, like Gecko) Version/4.0 Safari/530.17 Skyfire/2.0",
+ "expect" :
+ {
+ "name" : "Skyfire",
+ "version" : "2.0",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "SlimBrowser",
+ "ua" : "Mozilla/4.0 (compatible; MSIE 7.0; Windows NT 5.1; Trident/4.0; SlimBrowser)",
+ "expect" :
+ {
+ "name" : "Slim",
+ "version" : "undefined",
+ "major" : "undefined"
+ }
+ },
+ {
+ "desc" : "Swiftfox",
+ "ua" : "Mozilla/5.0 (X11; U; Linux i686; en-US; rv:1.8.1) Gecko/20061024 Firefox/2.0 (Swiftfox)",
+ "expect" :
+ {
+ "name" : "Swiftfox",
+ "version" : "undefined",
+ "major" : "undefined"
+ }
+ },
+ {
+ "desc" : "Tizen Browser",
+ "ua" : "Mozilla/5.0 (Linux; U; Tizen/1.0 like Android; en-us; AppleWebKit/534.46 (KHTML, like Gecko) Tizen Browser/1.0 Mobile",
+ "expect" :
+ {
+ "name" : "Tizen Browser",
+ "version" : "1.0",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "UC Browser",
+ "ua" : "Mozilla/5.0 (Windows NT 6.1; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/54.0.2840.99 UBrowser/5.6.12860.7 Safari/537.36",
+ "expect" :
+ {
+ "name" : "UCBrowser",
+ "version" : "5.6.12860.7",
+ "major" : "5"
+ }
+ },
+ {
+ "desc" : "UC Browser",
+ "ua" : "Mozilla/5.0 (Linux; U; Android 6.0.1; en-US; Lenovo P2a42 Build/MMB29M) AppleWebKit/534.30 (KHTML, like Gecko) Version/4.0 UCBrowser/11.2.0.915 U3/0.8.0 Mobile Safari/534.30",
+ "expect" :
+ {
+ "name" : "UCBrowser",
+ "version" : "11.2.0.915",
+ "major" : "11"
+ }
+ },
+ {
+ "desc" : "UC Browser on Samsung",
+ "ua" : "Mozilla/5.0 (Java; U; Pt-br; samsung-gt-s5620) UCBrowser8.2.1.144/69/352/UCWEB Mobile UNTRUSTED/1.0",
+ "expect" :
+ {
+ "name" : "UCBrowser",
+ "version" : "8.2.1.144",
+ "major" : "8"
+ }
+ },
+ {
+ "desc" : "UC Browser on Nokia",
+ "ua" : "Mozilla/5.0 (S60V3; U; en-in; NokiaN73)/UC Browser8.4.0.159/28/351/UCWEB Mobile",
+ "expect" :
+ {
+ "name" : "UCBrowser",
+ "version" : "8.4.0.159",
+ "major" : "8"
+ }
+ },
+ {
+ "desc" : "UC Browser J2ME",
+ "ua" : "UCWEB/2.0 (MIDP-2.0; U; zh-CN; HTC EVO 3D X515m) U2/1.0.0 UCBrowser/10.4.0.558 U2/1.0.0 Mobile",
+ "expect" :
+ {
+ "name" : "UCBrowser",
+ "version" : "10.4.0.558",
+ "major" : "10"
+ }
+ },
+ {
+ "desc" : "UC Browser J2ME 2",
+ "ua" : "JUC (Linux; U; 2.3.5; zh-cn; GT-I9100; 480*800) UCWEB7.9.0.94/139/800",
+ "expect" :
+ {
+ "name" : "UCBrowser",
+ "version" : "7.9.0.94",
+ "major" : "7"
+ }
+ },
+ {
+ "desc": "WeChat on iOS",
+ "ua": "Mozilla/5.0 (iPhone; CPU iPhone OS 8_4_1 like Mac OS X) AppleWebKit/600.1.4 (KHTML, like Gecko) Mobile/12H321 MicroMessenger/6.3.6 NetType/WIFI Language/zh_CN",
+ "expect":
+ {
+ "name": "WeChat",
+ "version": "6.3.6",
+ "major": "6"
+ }
+ },
+ {
+ "desc": "WeChat on Android",
+ "ua": "Mozilla/5.0 (Linux; U; Android 5.1; zh-cn; Lenovo K50-t5 Build/LMY47D) AppleWebKit/533.1 (KHTML, like Gecko)Version/4.0 MQQBrowser/5.4 TBS/025478 Mobile Safari/533.1 MicroMessenger/6.3.5.50_r1573191.640 NetType/WIFI Language/zh_CN",
+ "expect":
+ {
+ "name": "WeChat",
+ "version": "6.3.5.50_r1573191.640",
+ "major": "6"
+ }
+ },
+ {
+ "desc" : "Vivaldi",
+ "ua" : "Mozilla/5.0 (Windows NT 6.0) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/40.0.2214.89 Vivaldi/1.0.83.38 Safari/537.36",
+ "expect" :
+ {
+ "name" : "Vivaldi",
+ "version" : "1.0.83.38",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "Yandex",
+ "ua" : "Mozilla/5.0 (Macintosh; Intel Mac OS X 10_8_2) AppleWebKit/536.5 (KHTML, like Gecko) YaBrowser/1.0.1084.5402 Chrome/19.0.1084.5402 Safari/536.5",
+ "expect" :
+ {
+ "name" : "Yandex",
+ "version" : "1.0.1084.5402",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "Puffin",
+ "ua" : "Mozilla/5.0 (Linux; Android 6.0.1; Lenovo P2a42 Build/MMB29M; en-us) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/42.0.2311.135 Mobile Safari/537.36 Puffin/6.0.8.15804AP",
+ "expect" :
+ {
+ "name" : "Puffin",
+ "version" : "6.0.8.15804AP",
+ "major" : "6"
+ }
+ },
+ {
+ "desc" : "Microsoft Edge",
+ "ua" : "Mozilla/5.0 (Windows NT 10.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/39.0.2171.71 Safari/537.36 Edge/12.0",
+ "expect" :
+ {
+ "name" : "Edge",
+ "version" : "12.0",
+ "major" : "12"
+ }
+ },
+ {
+ "desc" : "Iridium",
+ "ua" : "Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) Iridium/43.8 Safari/537.36 Chrome/43.0.2357.132",
+ "expect" :
+ {
+ "name" : "Iridium",
+ "version" : "43.8",
+ "major" : "43"
+ }
+ },
+ {
+ "desc" : "Firefox iOS",
+ "ua" : "Mozilla/5.0 (iPhone; CPU iPhone OS 9_1 like Mac OS X) AppleWebKit/601.1.46 (KHTML, like Gecko) FxiOS/1.1 Mobile/13B143 Safari/601.1.46",
+ "expect" :
+ {
+ "name" : "Firefox",
+ "version" : "1.1",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "QQ on iOS",
+ "ua" : "Mozilla/5.0 (iPhone; CPU iPhone OS 10_0_2 like Mac OS X) AppleWebKit/602.1.50 (KHTML, like Gecko) Mobile/14A456 QQ/6.5.3.410 V1_IPH_SQ_6.5.3_1_APP_A Pixel/1080 Core/UIWebView NetType/WIFI Mem/26",
+ "expect" :
+ {
+ "name" : "QQ",
+ "version" : "6.5.3.410",
+ "major" : "6"
+ }
+ },
+ {
+ "desc" : "QQ on Android",
+ "ua" : "Mozilla/5.0 (Linux; Android 6.0; PRO 6 Build/MRA58K) AppleWebKit/537.36 (KHTML, like Gecko) Version/4.0 Chrome/37.0.0.0 Mobile MQQBrowser/6.8 TBS/036824 Safari/537.36 V1_AND_SQ_6.5.8_422_YYB_D PA QQ/6.5.8.2910 NetType/WIFI WebP/0.3.0 Pixel/1080",
+ "expect" :
+ {
+ "name" : "QQ",
+ "version" : "6.5.8.2910",
+ "major" : "6"
+ }
+ },
+ {
+ "desc" : "GSA on iOS",
+ "ua" : "Mozilla/5.0 (iPhone; CPU iPhone OS 10_3_2 like Mac OS X) AppleWebKit/602.1.50 (KHTML, like Gecko) GSA/30.1.161623614 Mobile/14F89 Safari/602.1",
+ "expect" :
+ {
+ "name" : "GSA",
+ "version" : "30.1.161623614",
+ "major" : "30"
+ }
+ }
+]
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/cpu-test.json b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/cpu-test.json
new file mode 100644
index 00000000..8e9befcc
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/cpu-test.json
@@ -0,0 +1,106 @@
+[
+ {
+ "desc" : "i686",
+ "ua" : "Mozilla/5.0 (X11; Ubuntu; Linux i686; rv:19.0) Gecko/20100101 Firefox/19.0",
+ "expect" :
+ {
+ "architecture" : "ia32"
+ }
+ },
+ {
+ "desc" : "i386",
+ "ua" : "Mozilla/5.0 (X11; U; FreeBSD i386; en-US; rv:1.7) Gecko/20040628 Epiphany/1.2.6",
+ "expect" :
+ {
+ "architecture" : "ia32"
+ }
+ },
+ {
+ "desc" : "x86-64",
+ "ua" : "Opera/9.80 (X11; Linux x86_64; U; Linux Mint; en) Presto/2.2.15 Version/10.10",
+ "expect" :
+ {
+ "architecture" : "amd64"
+ }
+ },
+ {
+ "desc" : "win64",
+ "ua" : "Mozilla/4.0 (compatible; MSIE 7.0; Windows NT 6.2; Win64; x64; Trident/6.0; .NET4.0E; .NET4.0C)",
+ "expect" :
+ {
+ "architecture" : "amd64"
+ }
+ },
+ {
+ "desc" : "WOW64",
+ "ua" : "Mozilla/5.0 (compatible; MSIE 10.0; Windows NT 6.1; WOW64; Trident/6.0)",
+ "expect" :
+ {
+ "architecture" : "amd64"
+ }
+ },
+ {
+ "desc" : "ARMv6",
+ "ua" : "Mozilla/5.0 (X11; U; Linux armv61; en-US; rv:1.9.1b2pre) Gecko/20081015 Fennec/1.0a1",
+ "expect" :
+ {
+ "architecture" : "arm"
+ }
+ },
+ {
+ "desc" : "Pocket PC",
+ "ua" : "Opera/9.7 (Windows Mobile; PPC; Opera Mobi/35166; U; en) Presto/2.2.1",
+ "expect" :
+ {
+ "architecture" : "arm"
+ }
+ },
+ {
+ "desc" : "Mac PowerPC",
+ "ua" : "Mozilla/4.0 (compatible; MSIE 4.5; Mac_PowerPC)",
+ "expect" :
+ {
+ "architecture" : "ppc"
+ }
+ },
+ {
+ "desc" : "Mac PowerPC",
+ "ua" : "Mozilla/4.0 (compatible; MSIE 5.17; Mac_PowerPC Mac OS; en)",
+ "expect" :
+ {
+ "architecture" : "ppc"
+ }
+ },
+ {
+ "desc" : "Mac PowerPC",
+ "ua" : "iCab/2.9.5 (Macintosh; U; PPC; Mac OS X)",
+ "expect" :
+ {
+ "architecture" : "ppc"
+ }
+ },
+ {
+ "desc" : "UltraSPARC",
+ "ua" : "Mozilla/5.0 (X11; U; SunOS sun4u; en-US; rv:1.9b5) Gecko/2008032620 Firefox/3.0b5",
+ "expect" :
+ {
+ "architecture" : "sparc"
+ }
+ },
+ {
+ "desc" : "QuickTime",
+ "ua" : "QuickTime/7.5.6 (qtver=7.5.6;cpu=IA32;os=Mac 10.5.8)",
+ "expect" :
+ {
+ "architecture" : "ia32"
+ }
+ },
+ {
+ "desc" : "XBMC",
+ "ua" : "XBMC/12.0 Git:20130127-fb595f2 (Windows NT 6.1;WOW64;Win64;x64; http://www.xbmc.org)",
+ "expect" :
+ {
+ "architecture" : "amd64"
+ }
+ }
+]
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/device-test.json b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/device-test.json
new file mode 100644
index 00000000..c1a3075a
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/device-test.json
@@ -0,0 +1,819 @@
+[{
+ "desc": "Asus Nexus 7",
+ "ua": "Mozilla/5.0 (Linux; Android 4.4.2; Nexus 7 Build/KOT49H) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/32.0.1700.99 Safari/537.36",
+ "expect": {
+ "vendor": "Asus",
+ "model": "Nexus 7",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Asus Padfone",
+ "ua": "Mozilla/5.0 (Linux; Android 4.1.1; PadFone 2 Build/JRO03L) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/37.0.2062.117 Safari/537.36",
+ "expect": {
+ "vendor": "Asus",
+ "model": "PadFone",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Desktop (IE11 with Tablet string)",
+ "ua": "Mozilla/5.0 (Windows NT 6.3; WOW64; Trident/7.0; .NET4.0E; .NET4.0C; .NET CLR 3.5.30729; .NET CLR 2.0.50727; .NET CLR 3.0.30729; Tablet PC 2.0; GWX:MANAGED; rv:11.0) like Gecko",
+ "expect": {
+ "vendor": "undefined",
+ "model": "undefined",
+ "type": "undefined"
+ }
+ },
+ {
+ "desc": "HTC Evo Shift 4G",
+ "ua": "Mozilla/5.0 (Linux; U; Android 2.3.4; en-us; Sprint APA7373KT Build/GRJ22) AppleWebKit/533.1 (KHTML, like Gecko) Version/4.0",
+ "expect": {
+ "vendor": "HTC",
+ "model": "Evo Shift 4G",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "HTC Nexus 9",
+ "ua": "Mozilla/5.0 (Linux; Android 5.0; Nexus 9 Build/LRX21R) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/36.0.1985.143 Mobile Crosswalk/7.36.154.13 Safari/537.36",
+ "expect": {
+ "vendor": "HTC",
+ "model": "Nexus 9",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Huawei Honor",
+ "ua": "Mozilla/5.0 (Linux; U; Android 2.3; xx-xx; U8860 Build/HuaweiU8860) AppleWebKit/533.1 (KHTML, like Gecko) Version/4.0 Mobile Safari/533.1",
+ "expect": {
+ "vendor": "Huawei",
+ "model": "U8860",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Huawei Nexus 6P",
+ "ua": "Mozilla/5.0 (Linux; Android 6.0.1; Nexus 6P Build/MTC19V) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/51.0.2704.81 Mobile Safari/537",
+ "expect": {
+ "vendor": "Huawei",
+ "model": "Nexus 6P",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Huawei P10",
+ "ua": "Mozilla/5.0 (Linux; Android 7.0; VTR-L09 Build/HUAWEIVTR-L09; xx-xx) AppleWebKit/537.36 (KHTML, like Gecko) Version/4.0 Chrome/56.0.2924.87 Mobile Safari/537.36",
+ "expect": {
+ "vendor": "Huawei",
+ "model": "VTR-L09",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Huawei Y3II",
+ "ua": "Mozilla/5.0 (Linux; U; Android 5.1; xx-xx; HUAWEI LUA-L03 Build/HUAWEILUA-L03) AppleWebKit/537.36 (KHTML, like Gecko) Version/4.0 Chrome/39.0.0.0 Mobile Safari/537.36",
+ "expect": {
+ "vendor": "Huawei",
+ "model": "LUA-L03",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "iPod",
+ "ua": "Mozilla/5.0 (iPod touch; CPU iPhone OS 7_0_4 like Mac OS X) AppleWebKit/537.51.1 (KHTML, like Gecko) Version/7.0 Mobile/11B554a Safari/9537.53",
+ "expect": {
+ "vendor": "Apple",
+ "model": "iPod touch",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "LG Nexus 4",
+ "ua": "Mozilla/5.0 (Linux; Android 4.2.1; Nexus 4 Build/JOP40D) AppleWebKit/535.19 (KHTML, like Gecko) Chrome/18.0.1025.166 Mobile Safari/535.19",
+ "expect": {
+ "vendor": "LG",
+ "model": "Nexus 4",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "LG Nexus 5",
+ "ua": "Mozilla/5.0 (Linux; Android 4.2.1; en-us; Nexus 5 Build/JOP40D) AppleWebKit/535.19 (KHTML, like Gecko) Chrome/18.0.1025.166 Mobile Safari/535.19",
+ "expect": {
+ "vendor": "LG",
+ "model": "Nexus 5",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Meizu M5 Note",
+ "ua": "Mozilla/5.0 (Linux; Android 6.0; M5 Note Build/MRA58K; wv) AppleWebKit/537.36 (KHTML, like Gecko) Version/4.0 Chrome/53.0.2785.49 Mobile MQQBrowser/6.2 TBS/043024 Safari/537.36 MicroMessenger/6.5.7.1040 NetType/WIFI Language/zh_CN",
+ "expect": {
+ "vendor": "Meizu",
+ "model": "M5 Note",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Microsoft Lumia 950",
+ "ua": "Mozilla/5.0 (Windows Phone 10.0; Android 4.2.1; Microsoft; Lumia 950) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/46.0.2486.0 Mobile Safari/537.36 Edge/13.10586",
+ "expect": {
+ "vendor": "Microsoft",
+ "model": "Lumia 950",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Motorola Moto X",
+ "ua": "Mozilla/5.0 (Linux; Android 4.4.4; XT1097 Build/KXE21.187-38) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/40.0.2214.109 Mobile Safari/537.36",
+ "expect": {
+ "vendor": "Motorola",
+ "model": "XT1097",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Motorola Nexus 6",
+ "ua": "Mozilla/5.0 (Linux; Android 5.1.1; Nexus 6 Build/LYZ28E) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/44.0.2403.20 Mobile Safari/537.36",
+ "expect": {
+ "vendor": "Motorola",
+ "model": "Nexus 6",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Motorola Droid RAZR 4G",
+ "ua": "Mozilla/5.0 (Linux; U; Android 2.3; xx-xx; DROID RAZR 4G Build/6.5.1-73_DHD-11_M1-29) AppleWebKit/533.1 (KHTML, like Gecko) Version/4.0 Mobile Safari/533.1",
+ "expect": {
+ "vendor": "Motorola",
+ "model": "DROID RAZR 4G",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "iPhone",
+ "ua": "Mozilla/5.0 (iPhone; CPU iPhone OS 7_0 like Mac OS X) AppleWebKit/537.51.1 (KHTML, like Gecko) Version/7.0 Mobile/11A465 Safari/9537.53",
+ "expect": {
+ "vendor": "Apple",
+ "model": "iPhone",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Motorola Droid RAZR 4G",
+ "ua": "Mozilla/5.0 (iPod touch; CPU iPhone OS 7_0_2 like Mac OS X) AppleWebKit/537.51.1 (KHTML, like Gecko) Version/7.0 Mobile/11A501 Safari/9537.53",
+ "expect": {
+ "vendor": "Apple",
+ "model": "iPod touch",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Moto X",
+ "ua": "Mozilla/5.0 (Linux; U; Android 4.2; xx-xx; XT1058 Build/13.9.0Q2.X-70-GHOST-ATT_LE-2) AppleWebKit/534.30 (KHTML, like Gecko) Version/4.0 Mobile Safari/534.30",
+ "expect": {
+ "vendor": "Motorola",
+ "model": "XT1058",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Nokia3xx",
+ "ua": "Nokia303/14.87 CLDC-1.1",
+ "expect": {
+ "vendor": "Nokia",
+ "model": "303",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "OnePlus One",
+ "ua": "Mozilla/5.0 (Linux; Android 4.4.4; A0001 Build/KTU84Q) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/39.0.2171.59 Mobile Safari/537.36",
+ "expect": {
+ "vendor": "OnePlus",
+ "model": "1",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "OPPO R7s",
+ "ua": "Mozilla/5.0 (Linux; U; Android 4.4.4; zh-cn; OPPO R7s Build/KTU84P) AppleWebKit/537.36 (KHTML, like Gecko)Version/4.0 Chrome/37.0.0.0 MQQBrowser/7.1 Mobile Safari/537.36",
+ "expect": {
+ "vendor": "OPPO",
+ "model": "R7s",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Philips SmartTV",
+ "ua": "Opera/9.80 HbbTV/1.1.1 (; Philips; ; ; ; ) NETTV/4.0.2; en) Version/11.60",
+ "expect": {
+ "vendor": "Philips",
+ "model": "",
+ "type": "smarttv"
+ }
+ },
+ {
+ "desc": "Kindle Fire HD",
+ "ua": "Mozilla/5.0 (Linux; U; Android 4.0.3; en-us; KFTT Build/IML74K) AppleWebKit/535.19 (KHTML, like Gecko) Silk/3.4 Mobile Safari/535.19 Silk-Accelerated=true",
+ "expect": {
+ "vendor": "Amazon",
+ "model": "KFTT",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Samsung Galaxy Note 8",
+ "ua": "Mozilla/5.0 (Linux; Android 4.2.2; GT-N5100 Build/JDQ39) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/35.0.1916.141 Safari/537.36",
+ "expect": {
+ "vendor": "Samsung",
+ "model": "GT-N5100",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Samsung SM-C5000",
+ "ua": "Mozilla/5.0 (Linux; Android 6.0.1; SM-C5000 Build/MMB29M; wv) AppleWebKit/537.36 (KHTML, like Gecko) Version/4.0 Chrome/51.0.2704.81 Mobile Safari/537.36 wkbrowser 4.1.35 3065",
+ "expect": {
+ "vendor": "Samsung",
+ "model": "SM-C5000",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Samsung SM-T231",
+ "ua": "Mozilla/5.0 (Linux; Android 4.4.2; SM-T231 Build/KOT49H) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/36.0.1985.135 Safari/537.36",
+ "expect": {
+ "vendor": "Samsung",
+ "model": "SM-T231",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Samsung SM-T700",
+ "ua": "Mozilla/5.0 (Linux; Android 4.4.2; SM-T700 Build/KOT49H) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/36.0.1985.135 Safari/537.36",
+ "expect": {
+ "vendor": "Samsung",
+ "model": "SM-T700",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Samsung SM-T520",
+ "ua": "Mozilla/5.0 (Linux; Android 4.4.2; SM-T520 Build/KOT49H) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/36.0.1985.135 Safari/537.36",
+ "expect": {
+ "vendor": "Samsung",
+ "model": "SM-T520",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Samsung SmartTV2011",
+ "ua": "HbbTV/1.1.1 (;;;;;) Maple;2011",
+ "expect": {
+ "vendor": "Samsung",
+ "model": "SmartTV2011",
+ "type": "smarttv"
+ }
+ },
+ {
+ "desc": "Samsung SmartTV2012",
+ "ua": "HbbTV/1.1.1 (;Samsung;SmartTV2012;;;) WebKit",
+ "expect": {
+ "vendor": "Samsung",
+ "model": "SmartTV2012",
+ "type": "smarttv"
+ }
+ },
+ {
+ "desc": "Samsung SmartTV2014",
+ "ua": "HbbTV/1.1.1 (;Samsung;SmartTV2014;T-NT14UDEUC-1060.4;;) WebKit",
+ "expect": {
+ "vendor": "Samsung",
+ "model": "SmartTV2014",
+ "type": "smarttv"
+ }
+ },
+ {
+ "desc": "Samsung SmartTV",
+ "ua": "Mozilla/5.0 (SMART-TV; Linux; Tizen 2.3) AppleWebkit/538.1 (KHTML, like Gecko) SamsungBrowser/1.0 TV Safari/538.1",
+ "expect": {
+ "vendor": "Samsung",
+ "model": "undefined",
+ "type": "smarttv"
+ }
+ },
+ {
+ "desc": "Sony C5303 (Xperia SP)",
+ "ua": "Mozilla/5.0 (Linux; Android 4.3; C5303 Build/12.1.A.1.205) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/39.0.2171.93 Mobile Safari/537.36",
+ "expect": {
+ "vendor": "Sony",
+ "model": "C5303",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Sony SO-02F (Xperia Z1 F)",
+ "ua": "Mozilla/5.0 (Linux; Android 4.2.2; SO-02F Build/14.1.H.2.119) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/34.0.1847.114 Mobile Safari/537.36",
+ "expect": {
+ "vendor": "Sony",
+ "model": "SO-02F",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Sony D6653 (Xperia Z3)",
+ "ua": "Mozilla/5.0 (Linux; Android 4.4; D6653 Build/23.0.A.0.376) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/35.0.1916.141 Mobile Safari/537.36",
+ "expect": {
+ "vendor": "Sony",
+ "model": "D6653",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Sony Xperia SOL25 (ZL2)",
+ "ua": "Mozilla/5.0 (Linux; U; Android 4.4; SOL25 Build/17.1.1.C.1.64) AppleWebKit/534.30 (KHTML, like Gecko) Version/4.0 Mobile Safari/534.30",
+ "expect": {
+ "vendor": "Sony",
+ "model": "SOL25",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Sony Xperia SP",
+ "ua": "Mozilla/5.0 (Linux; Android 4.3; C5302 Build/12.1.A.1.201) AppleWebkit/537.36 (KHTML, like Gecko) Chrome/34.0.1847.114 Mobile Safari/537.36",
+ "expect": {
+ "vendor": "Sony",
+ "model": "C5302",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Sony SGP521 (Xperia Z2 Tablet)",
+ "ua": "Mozilla/5.0 (Linux; Android 4.4; SGP521 Build/17.1.A.0.432) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/32.0.1700.99 Safari/537.36",
+ "expect": {
+ "vendor": "Sony",
+ "model": "Xperia Tablet",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Sony Tablet S",
+ "ua": "Mozilla/5.0 (Linux; U; Android 3.1; Sony Tablet S Build/THMAS10000) AppleWebKit/534.13 (KHTML, like Gecko) Version/4.0 Safari/534.13",
+ "expect": {
+ "vendor": "Sony",
+ "model": "Xperia Tablet",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Sony Tablet Z LTE",
+ "ua": "Mozilla/5.0 (Linux; U; Android 4.1; SonySGP321 Build/10.2.C.0.143) AppleWebKit/534.30 (KHTML, like Gecko) Version/4.0 Safari/534.30",
+ "expect": {
+ "vendor": "Sony",
+ "model": "Xperia Tablet",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Xiaomi 2013023",
+ "ua": "Mozilla/5.0 (Linux; U; Android 4.2.2; en-US; 2013023 Build/HM2013023) AppleWebKit/533.1 (KHTML, like Gecko) Version/4.0 UCBrowser/10.0.1.512 U3/0.8.0 Mobile Safari/533.1",
+ "expect": {
+ "vendor": "Xiaomi",
+ "model": "2013023",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Xiaomi Hongmi Note 1W",
+ "ua": "Mozilla/5.0 (Linux; U; Android 4.2.2; zh-CN; HM NOTE 1W Build/JDQ39) AppleWebKit/533.1 (KHTML, like Gecko) Version/4.0 UCBrowser/9.7.9.439 U3/0.8.0 Mobile Safari/533.1",
+ "expect": {
+ "vendor": "Xiaomi",
+ "model": "HM NOTE 1W",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Xiaomi Mi 3C",
+ "ua": "Mozilla/5.0 (Linux; U; Android 4.3; zh-CN; MI 3C Build/JLS36C) AppleWebKit/533.1 (KHTML, like Gecko) Version/4.0 UCBrowser/9.7.9.439 U3/0.8.0 Mobile Safari/533.1",
+ "expect": {
+ "vendor": "Xiaomi",
+ "model": "MI 3C",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Xiaomi Mi Note",
+ "ua": "Mozilla/5.0 (Linux; Android 4.4.4; MI NOTE LTE Build/KTU84P) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/46.0.2490.76 Mobile Safari/537.36",
+ "expect": {
+ "vendor": "Xiaomi",
+ "model": "MI NOTE LTE",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Xiaomi Mi One Plus",
+ "ua": "Mozilla/5.0 (Linux; U; Android 4.0.4; en-us; MI-ONE Plus Build/IMM76D) AppleWebKit/534.30 (KHTML, like Gecko) Version/4.0 Mobile Safari/534.30",
+ "expect": {
+ "vendor": "Xiaomi",
+ "model": "MI-ONE Plus",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "PlayStation 4",
+ "ua": "Mozilla/5.0 (PlayStation 4 3.00) AppleWebKit/537.73 (KHTML, like Gecko)",
+ "expect": {
+ "vendor": "Sony",
+ "model": "PlayStation 4",
+ "type": "console"
+ }
+ },
+ {
+ "desc": "Galaxy Nexus",
+ "ua": "Mozilla/5.0 (Linux; Android 4.0.4; Galaxy Nexus Build/IMM76B) AppleWebKit/535.19 (KHTML, like Gecko) Chrome/18.0.1025.133 Mobile Safari/535.19",
+ "expect": {
+ "vendor": "Samsung",
+ "model": "Galaxy Nexus",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Samsung Galaxy S5",
+ "ua": "Mozilla/5.0 (Linux; Android 5.0; SM-G900F Build/LRX21T) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/43.0.2357.78 Mobile Safari/537.36",
+ "expect": {
+ "vendor": "Samsung",
+ "model": "SM-G900F",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Samsung Galaxy S6",
+ "ua": "Mozilla/5.0 (Linux; Android 4.4.2; SM-G920I Build/KOT49H) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/36.0.1985.135 Safari/537.36",
+ "expect": {
+ "vendor": "Samsung",
+ "model": "SM-G920I",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Samsung Galaxy S6 Edge",
+ "ua": "Mozilla/5.0 (Linux; Android 4.4.2; SM-G925I Build/KOT49H) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/36.0.1985.135 Safari/537.36",
+ "expect": {
+ "vendor": "Samsung",
+ "model": "SM-G925I",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Samsung Galaxy Note 5 Chrome",
+ "ua": "Mozilla/5.0 (Linux; Android 5.1.1; SM-N920C Build/LMY47X) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/49.0.2623.91 Mobile Safari/537.36",
+ "expect": {
+ "vendor": "Samsung",
+ "model": "SM-N920C",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Samsung Galaxy Note 5 Samsung Browser",
+ "ua": "Mozilla/5.0 (Linux; Android 5.1.1; SAMSUNG SM-N920C Build/LMY47X) AppleWebKit/537.36 (KHTML, like Gecko) SamsungBrowser/4.0 Chrome/44.0.2403.133 Mobile Safari/537.36",
+ "expect": {
+ "vendor": "Samsung",
+ "model": "SM-N920C",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Google Chromecast",
+ "ua": "Mozilla/5.0 (X11; Linux armv7l) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/52.0.2743.84 Safari/537.36 CrKey/1.22.79313",
+ "expect": {
+ "vendor": "Google",
+ "model": "Chromecast"
+ }
+ },
+ {
+ "desc": "Google Pixel C",
+ "ua": "Mozilla/5.0 (Linux; Android 7.0; Pixel C Build/NRD90M; wv) AppleWebKit/537.36 (KHTML, like Gecko) Version/4.0 Chrome/52.0.2743.98 Safari/537.36",
+ "expect": {
+ "vendor": "Google",
+ "model": "Pixel C",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Google Pixel",
+ "ua": "Mozilla/5.0 (Linux; Android 7.1; Pixel Build/NDE63V) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/54.0.2840.85 Mobile Safari/537.36",
+ "expect": {
+ "vendor": "Google",
+ "model": "Pixel",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Google Pixel",
+ "ua": "Mozilla/5.0 (Linux; Android 7.1; Pixel XL Build/NDE63X) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/54.0.2840.85 Mobile Safari/537.36",
+ "expect": {
+ "vendor": "Google",
+ "model": "Pixel XL",
+ "type": "mobile"
+ }
+ },
+
+ {
+ "desc": "Generic Android Device",
+ "ua": "Mozilla/5.0 (Linux; U; Android 6.0.1; i980 Build/MRA58K) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/56.0.2924.87 Safari/537.36",
+ "expect": {
+ "vendor": "Generic",
+ "model": "Android 6.0.1"
+ }
+ },
+ {
+ "desc": "LG VK Series Tablet",
+ "ua": "Mozilla/5.0 (Linux; Android 5.0.2; VK700 Build/LRX22G) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/55.0.2883.84 Safari/537.36",
+ "expect": {
+ "vendor": "LG",
+ "model": "VK700",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "LG LK Series Tablet",
+ "ua": "Mozilla/5.0 (Linux; Android 5.0.1; LGLK430 Build/LRX21Y) AppleWebKit/537.36 (KHTML, like Gecko) Version/4.0 Chrome/38.0.2125.102 Safari/537.36",
+ "expect": {
+ "vendor": "LG",
+ "model": "LK430",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "RCA Voyager III Tablet",
+ "ua": "Mozilla/5.0 (Linux; Android 6.0.1; RCT6973W43 Build/MMB29M) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/56.0.2924.87 Safari/537.36",
+ "expect": {
+ "vendor": "RCA",
+ "model": "RCT6973W43",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "RCA Voyager II Tablet",
+ "ua": "Mozilla/5.0 (Linux; Android 5.0; RCT6773W22B Build/LRX21M) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/56.0.2924.87 Safari/537.36",
+ "expect": {
+ "vendor": "RCA",
+ "model": "RCT6773W22B",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Verizon Quanta Tablet",
+ "ua": "Mozilla/5.0 (Linux; Android 4.4.2; QMV7B Build/KOT49H) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/56.0.2924.87 Safari/537.36",
+ "expect": {
+ "vendor": "Verizon",
+ "model": "QMV7B",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Verizon Ellipsis 8 Tablet",
+ "ua": "Mozilla/5.0 (Linux; Android 5.1.1; QTAQZ3 Build/LMY47V) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/56.0.2924.87 Safari/537.36",
+ "expect": {
+ "vendor": "Verizon",
+ "model": "QTAQZ3",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Verizon Ellipsis 8HD Tablet",
+ "ua": "Mozilla/5.0 (Linux; Android 6.0.1; QTASUN1 Build/MMB29M) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/51.0.2704.81 Safari/537.36",
+ "expect": {
+ "vendor": "Verizon",
+ "model": "QTASUN1",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Dell Venue 8 Tablet",
+ "ua": "Mozilla/5.0 (Linux; Android 4.4.2; Venue 8 3830 Build/KOT49H) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/56.0.2924.87 Safari/537.36",
+ "expect": {
+ "vendor": "Dell",
+ "model": "Venue 8 3830",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Dell Venue 7 Tablet",
+ "ua": "Mozilla/5.0 (Linux; Android 4.4.2; Venue 7 3730 Build/KOT49H) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/56.0.2924.87 Safari/537.36",
+ "expect": {
+ "vendor": "Dell",
+ "model": "Venue 7 3730",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Barnes & Noble Nook HD+ Tablet",
+ "ua": "Mozilla/5.0 (Linux; U; Android 4.1.2; en-us; Barnes & Noble Nook HD+ Build/JZO54K; CyanogenMod-10) AppleWebKit/534.30 (KHTML, like Gecko) Version/4.0 Mobile Safari/534.30",
+ "expect": {
+ "vendor": "Barnes & Noble",
+ "model": "Nook HD+",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Barnes & Noble V400 Tablet",
+ "ua": "Mozilla/5.0 (Linux; Android 4.0.4; BNTV400 Build/IMM76L) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/42.0.2311.111 Safari/537.36",
+ "expect": {
+ "vendor": "Barnes & Noble",
+ "model": "V400",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "NuVision TM101A540N Tablet",
+ "ua": "Mozilla/5.0 (Linux; Android 5.1; TM101A540N Build/LMY47I; wv) AppleWebKit/537.36 (KHTML, like Gecko) Version/4.0 Chrome/50.0.2661.86 Safari/537.36",
+ "expect": {
+ "vendor": "NuVision",
+ "model": "TM101A540N",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "ZTE K Series Tablet",
+ "ua": "Mozilla/5.0 (Linux; Android 6.0.1; K88 Build/MMB29M) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/56.0.2924.87 Safari/537.36",
+ "expect": {
+ "vendor": "ZTE",
+ "model": "K88",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Swizz GEN610",
+ "ua": "Mozilla/5.0 (Linux; Android 4.4.2; GEN610 Build/KOT49H) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/47.0.2526.83 Mobile Safari/537.36",
+ "expect": {
+ "vendor": "Swiss",
+ "model": "GEN610",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Swizz ZUR700",
+ "ua": "Mozilla/5.0 (Linux; Android 4.4.2; ZUR700 Build/KVT49L) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/41.0.2272.96 Safari/537.36",
+ "expect": {
+ "vendor": "Swiss",
+ "model": "ZUR700",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Zeki TB782b Tablet",
+ "ua": "Mozilla/5.0 (Linux; U; Android 4.0.4; en-US; TB782B Build/IMM76D) AppleWebKit/534.31 (KHTML, like Gecko) UCBrowser/9.0.2.299 U3/0.8.0 Mobile Safari/534.31",
+ "expect": {
+ "vendor": "Zeki",
+ "model": "TB782B",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Dragon Touch Tablet",
+ "ua": "Mozilla/5.0 (Linux; Android 4.0.4; DT9138B Build/IMM76D) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/29.0.1547.72 Mobile Safari/537.36",
+ "expect": {
+ "vendor": "Dragon Touch",
+ "model": "9138B",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Insignia Tablet",
+ "ua": "Mozilla/5.0 (Linux; U; Android 6.0.1; NS-P08A7100 Build/MMB29M; wv) AppleWebKit/537.36 (KHTML, like Gecko) Version/4.0 Chrome/56.0.2924.87 Safari/537.36",
+ "expect": {
+ "vendor": "Insignia",
+ "model": "NS-P08A7100",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Voice Xtreme V75",
+ "ua": "Mozilla/5.0 (Linux; U; Android 4.2.1; en-us; V75 Build/JOP40D) AppleWebKit/534.30 (KHTML, like Gecko) Version/4.0 Mobile Safari/534.30",
+ "expect": {
+ "vendor": "Voice",
+ "model": "V75",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "LvTel V11",
+ "ua": "Mozilla/5.0 (Linux; Android 5.1.1; V11 Build/LMY47V) AppleWebKit/537.36 (KHTML, like Gecko) Version/4.0 Chrome/39.0.0.0 Safari/537.36",
+ "expect": {
+ "vendor": "LvTel",
+ "model": "V11",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "Envizen Tablet V100MD",
+ "ua": "Mozilla/5.0 (Linux; U; Android 4.1.1; en-us; V100MD Build/V100MD.20130816) AppleWebKit/534.30 (KHTML, like Gecko) Version/4.0 Safari/534.30",
+ "expect": {
+ "vendor": "Envizen",
+ "model": "V100MD",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Rotor Tablet",
+ "ua": "mozilla/5.0 (linux; android 5.0.1; tu_1491 build/lrx22c) applewebkit/537.36 (khtml, like gecko) chrome/43.0.2357.93 safari/537.36",
+ "expect": {
+ "vendor": "Rotor",
+ "model": "1491",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "MachSpeed Tablets",
+ "ua": "Mozilla/5.0 (Linux; Android 4.4.2; Trio 7.85 vQ Build/Trio_7.85_vQ) AppleWebKit/537.36 (KHTML, like Gecko) Version/4.0 Chrome/30.0.0.0 Safari/537.36",
+ "expect": {
+ "vendor": "MachSpeed",
+ "model": "Trio 7.85 vQ",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Trinity Tablets",
+ "ua": "Mozilla/5.0 (Linux; Android 5.0.1; Trinity T101 Build/LRX22C) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/47.0.2526.83 Safari/537.36",
+ "expect": {
+ "vendor": "Trinity",
+ "model": "T101",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "NextBook Next7",
+ "ua": "Mozilla/5.0 (Linux; U; Android 4.0.4; en-us; Next7P12 Build/IMM76I) AppleWebKit/534.30 (KHTML, like Gecko) Version/4.0 Safari/534.30",
+ "expect": {
+ "vendor": "NextBook",
+ "model": "Next7P12",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "NextBook Tablets",
+ "ua": "Mozilla/5.0 (Linux; Android 5.0; NXA8QC116 Build/LRX21V) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/56.0.2924.87 Safari/537.36",
+ "expect": {
+ "vendor": "NextBook",
+ "model": "NXA8QC116",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Le Pan Tablets",
+ "ua": "Mozilla/5.0 (Linux; Android 4.2.2; Le Pan TC802A Build/JDQ39) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/56.0.2924.87 Safari/537.36",
+ "expect": {
+ "vendor": "Le Pan",
+ "model": "TC802A",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Le Pan Tablets",
+ "ua": "Mozilla/5.0 (Linux; Android 4.2.2; Le Pan TC802A Build/JDQ39) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/56.0.2924.87 Safari/537.36",
+ "expect": {
+ "vendor": "Le Pan",
+ "model": "TC802A",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Amazon Kindle Fire Tablet",
+ "ua": "Mozilla/5.0 (Linux; U; Android 4.4.3; en-us; KFSAWI Build/KTU84M) AppleWebKit/537.36 (KHTML, like Gecko) Silk/3.66 like Chrome/39.0.2171.93 Safari/537.36",
+ "expect": {
+ "vendor": "Amazon",
+ "model": "KFSAWI",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Gigaset Tablet",
+ "ua": "Mozilla/5.0 (Linux; Android 4.2.2; Gigaset QV830 Build/JDQ39) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/56.0.2924.87 Safari/537.36",
+ "expect": {
+ "vendor": "Gigaset",
+ "model": "QV830",
+ "type": "tablet"
+ }
+ },
+ {
+ "desc": "Redmi Note 3",
+ "ua": "Mozilla/5.0 (Linux; Android 6.0.1; Redmi Note 3 Build/MMB29M) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/60.0.3112.116 Mobile Safari/537.36",
+ "expect": {
+ "vendor": "Xiaomi",
+ "model": "Redmi Note 3",
+ "type": "mobile"
+ }
+ },
+ {
+ "desc": "MI PAD 2",
+ "ua": "Mozilla/5.0 (Linux; Android 5.1; MI PAD 2 Build/LMY47I; wv) AppleWebKit/537.36 (KHTML, like Gecko) Version/4.0 Chrome/60.0.3112.107 Safari/537.36 [FB_IAB/FB4A;FBAV/137.0.0.24.91;]",
+ "expect": {
+ "vendor": "Xiaomi",
+ "model": "MI PAD 2",
+ "type": "tablet"
+ }
+ }
+]
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/engine-test.json b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/engine-test.json
new file mode 100644
index 00000000..875057da
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/engine-test.json
@@ -0,0 +1,74 @@
+[
+ {
+ "desc" : "EdgeHTML",
+ "ua" : "Mozilla/5.0 (Windows NT 6.4; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/36.0.1985.143 Safari/537.36 Edge/12.0",
+ "expect" :
+ {
+ "name" : "EdgeHTML",
+ "version" : "12.0"
+ }
+ },
+ {
+ "desc" : "Gecko",
+ "ua" : "Mozilla/5.0 (X11; Linux x86_64; rv:2.0b9pre) Gecko/20110111 Firefox/4.0b9pre",
+ "expect" :
+ {
+ "name" : "Gecko",
+ "version" : "2.0b9pre"
+ }
+ },
+ {
+ "desc" : "KHTML",
+ "ua" : "Mozilla/5.0 (compatible; Konqueror/4.5; FreeBSD) KHTML/4.5.4 (like Gecko)",
+ "expect" :
+ {
+ "name" : "KHTML",
+ "version" : "4.5.4"
+ }
+ },
+ {
+ "desc" : "NetFront",
+ "ua" : "Mozilla/4.0 (PDA; Windows CE/1.0.1) NetFront/3.0",
+ "expect" :
+ {
+ "name" : "NetFront",
+ "version" : "3.0"
+ }
+ },
+ {
+ "desc" : "Presto",
+ "ua" : "Opera/9.80 (Windows NT 6.1; Opera Tablet/15165; U; en) Presto/2.8.149 Version/11.1",
+ "expect" :
+ {
+ "name" : "Presto",
+ "version" : "2.8.149"
+ }
+ },
+ {
+ "desc" : "Tasman",
+ "ua" : "Mozilla/4.0 (compatible; MSIE 6.0; PPC Mac OS X 10.4.7; Tasman 1.0)",
+ "expect" :
+ {
+ "name" : "Tasman",
+ "version" : "1.0"
+ }
+ },
+ {
+ "desc" : "Trident",
+ "ua" : "Mozilla/5.0 (compatible; MSIE 10.0; Windows NT 6.2; Win64; x64; Trident/6.0)",
+ "expect" :
+ {
+ "name" : "Trident",
+ "version" : "6.0"
+ }
+ },
+ {
+ "desc" : "WebKit",
+ "ua" : "Mozilla/5.0 (Windows; U; Windows NT 6.1; sv-SE) AppleWebKit/533.19.4 (KHTML, like Gecko) Version/5.0.3 Safari/533.19.4",
+ "expect" :
+ {
+ "name" : "WebKit",
+ "version" : "533.19.4"
+ }
+ }
+]
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/mediaplayer-test.json b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/mediaplayer-test.json
new file mode 100644
index 00000000..d40ba04f
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/mediaplayer-test.json
@@ -0,0 +1,582 @@
+[
+ {
+ "desc" : "Coremedia",
+ "ua" : "Apple Mac OS X v10.6.4 CoreMedia v1.0.0.10F2108",
+ "expect" :
+ {
+ "name" : "CoreMedia",
+ "version" : "1.0.0.10F2108",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "AppleCoreMedia",
+ "ua" : "AppleCoreMedia/1.0.0.10A403 (iPad; U; CPU OS 6_0 like Mac OS X; it_it)",
+ "expect" :
+ {
+ "name" : "AppleCoreMedia",
+ "version" : "1.0.0.10A403",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "AppleTv",
+ "ua" : "AppleTV/3.0.2 (Macintosh; Intel Mac OS X 10.4.7) AppleWebKit/528.18",
+ "expect" :
+ {
+ "name" : "WebKit",
+ "version" : "528.18",
+ "major" : "528"
+ }
+ },
+ {
+ "desc" : "Aqualung",
+ "ua" : "Aqualung/R-1114",
+ "expect" :
+ {
+ "name" : "Aqualung",
+ "version" : "R-1114",
+ "major" : "undefined"
+ }
+ },
+ {
+ "desc" : "Ares",
+ "ua" : "Ares 2.2.4.3048",
+ "expect" :
+ {
+ "name" : "Ares",
+ "version" : "2.2.4.3048",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "Audacious",
+ "ua" : "Audacious/3.2.2 neon/0.29.3",
+ "expect" :
+ {
+ "name" : "Audacious",
+ "version" : "3.2.2",
+ "major" : "3"
+ }
+ },
+ {
+ "desc" : "AudiMusicStream",
+ "ua" : "AudiMusicStream/3020.130826151911",
+ "expect" :
+ {
+ "name" : "AudiMusicStream",
+ "version" : "3020.130826151911",
+ "major" : "3020"
+ }
+ },
+ {
+ "desc" : "BASS",
+ "ua" : "BASS/2.4",
+ "expect" :
+ {
+ "name" : "BASS",
+ "version" : "2.4",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "BSPlayer",
+ "ua" : "BSPlayer/2",
+ "expect" :
+ {
+ "name" : "BSPlayer",
+ "version" : "2",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "Core",
+ "ua" : "CORE/6.506.4.1",
+ "expect" :
+ {
+ "name" : "CORE",
+ "version" : "6.506.4.1",
+ "major" : "6"
+ }
+ },
+ {
+ "desc" : "Clementine",
+ "ua" : "Clementine 1.1",
+ "expect" :
+ {
+ "name" : "Clementine",
+ "version" : "1.1",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "Dalvik",
+ "ua" : "Dalvik/1.2.0 (Linux; U; Android 2.2.1; GT-S5830L Build/FROYO)",
+ "expect" :
+ {
+ "name" : "Dalvik",
+ "version" : "1.2.0",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "NexPlayer",
+ "ua" : "E97510d/ Player/NexPlayer 4.0",
+ "expect" :
+ {
+ "name" : "NexPlayer",
+ "version" : "4.0",
+ "major" : "4"
+ }
+ },
+ {
+ "desc" : "FLRP",
+ "ua" : "FLRP/2.5 CFNetwork/609.1.4 Darwin/13.0.0",
+ "expect" :
+ {
+ "name" : "Flip Player",
+ "version" : "2.5",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "FStream",
+ "ua" : "FStream",
+ "expect" :
+ {
+ "name" : "FStream",
+ "version" : "undefined",
+ "major" : "undefined"
+ }
+ },
+ {
+ "desc" : "GStreamer",
+ "ua" : "GStreamer souphttpsrc (compatible; LG NetCast.TV-2012) libsoup/2.34.2",
+ "expect" :
+ {
+ "name" : "GStreamer",
+ "version" : "2.34.2",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "GnomeMplayer",
+ "ua" : "GnomeMplayer/1.0.2",
+ "expect" :
+ {
+ "name" : "GnomeMplayer",
+ "version" : "1.0.2",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "HTC Streaming Player",
+ "ua" : "HTC Streaming Player htc_asia_wwe / 1.0 / endeavoru / 4.1.1",
+ "expect" :
+ {
+ "name" : "HTC Streaming Player",
+ "version" : "1.0",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "HTC One S",
+ "ua" : "HTC_One_S/3.16.111.10",
+ "expect" :
+ {
+ "name" : "HTC One S",
+ "version" : "3.16.111.10",
+ "major" : "3"
+ }
+ },
+ {
+ "desc" : "Java",
+ "ua" : "Java/1.4.1_04",
+ "expect" :
+ {
+ "name" : "Java",
+ "version" : "1.4.1_04",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "LG Player",
+ "ua" : "LG Player 1.0; Android",
+ "expect" :
+ {
+ "name" : "LG Player",
+ "version" : "1.0",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "NexPlayer",
+ "ua" : "LG-P700/V10k-DEC-12-2012 Player/NexPlayer 4.0 for Android",
+ "expect" :
+ {
+ "name" : "NexPlayer",
+ "version" : "4.0",
+ "major" : "4"
+ }
+ },
+ {
+ "desc" : "LG Player",
+ "ua" : "LGE400/V10b Player/LG Player 1.0",
+ "expect" :
+ {
+ "name" : "LG Player",
+ "version" : "1.0",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "Lavf",
+ "ua" : "Lavf52.111.0",
+ "expect" :
+ {
+ "name" : "Lavf",
+ "version" : "52.111.0",
+ "major" : "52"
+ }
+ },
+ {
+ "desc" : "Lyssna",
+ "ua" : "Lyssna/46 CFNetwork/609.1.4 Darwin/13.0.0",
+ "expect" :
+ {
+ "name" : "Lyssna",
+ "version" : "46",
+ "major" : "46"
+ }
+ },
+ {
+ "desc" : "MPlayer",
+ "ua" : "MPlayer 1.1-4.2.1",
+ "expect" :
+ {
+ "name" : "MPlayer",
+ "version" : "1.1-4.2.1",
+ "major" : ""
+ }
+ },
+ {
+ "desc" : "MPlayer SVN",
+ "ua" : "MPlayer SVN-r33713-4.6.1",
+ "expect" :
+ {
+ "name" : "MPlayer",
+ "version" : "r33713-4.6.1",
+ "major" : "undefined"
+ }
+ },
+ {
+ "desc" : "MPlayer ubuntu",
+ "ua" : "MPlayer svn r34540 (Ubuntu), built with gcc-4.6",
+ "expect" :
+ {
+ "name" : "MPlayer",
+ "version" : "r34540",
+ "major" : "undefined"
+ }
+ },
+ {
+ "desc" : "MoC",
+ "ua" : "Music On Console/2.5.0-beta1",
+ "expect" :
+ {
+ "name" : "Music On Console",
+ "version" : "2.5.0-beta1",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "MPD",
+ "ua" : "Music Player Daemon 0.17.4",
+ "expect" :
+ {
+ "name" : "Music Player Daemon",
+ "version" : "0.17.4",
+ "major" : "0"
+ }
+ },
+ {
+ "desc" : "NSPlayer",
+ "ua" : "NSPlayer/11.0.5358.4827 WMFSDK/11.0",
+ "expect" :
+ {
+ "name" : "NSPlayer",
+ "version" : "11.0.5358.4827",
+ "major" : "11"
+ }
+ },
+ {
+ "desc" : "Nero Home",
+ "ua" : "Nero Home/1.5.3.0 (compatible; Nero AG; Nero Home 1.5.3.0)",
+ "expect" :
+ {
+ "name" : "Nero Home",
+ "version" : "1.5.3.0",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "NexPlayer",
+ "ua" : "NexPlayer 4.0 for Android( stagefright alternative )",
+ "expect" :
+ {
+ "name" : "NexPlayer",
+ "version" : "4.0",
+ "major" : "4"
+ }
+ },
+ {
+ "desc" : "Nokia3xx",
+ "ua" : "Nokia303/14.87 CLDC-1.1",
+ "expect" :
+ {
+ "name" : "Nokia303",
+ "version" : "14.87",
+ "major" : "14"
+ }
+ },
+ {
+ "desc" : "MPlayer SVN Sherpya",
+ "ua" : "MPlayer Sherpya-SVN-r33124-4.2.5",
+ "expect" :
+ {
+ "name" : "MPlayer",
+ "version" : "r33124-4.2.5",
+ "major" : "undefined"
+ }
+ },
+ {
+ "desc" : "Philips Songbird",
+ "ua" : "NotMoz/5.0 (Windows; U; Windows NT 5.1; it; rv:1.9.2.3) Gecko/20101207 Philips-Songbird/5.4.1980 Songbird/1.9.4 (20110302030555)",
+ "expect" :
+ {
+ "name" : "Songbird",
+ "version" : "1.9.4",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "Ossproxy",
+ "ua" : "OSSProxy 1.3.336.320 (Build 336.320 Win32 en-us)(Aug 16 2013 17:38:43)",
+ "expect" :
+ {
+ "name" : "OSSProxy",
+ "version" : "1.3.336.320",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "Winamp3",
+ "ua" : "Nullsoft Winamp3 version 3.0 (compatible)",
+ "expect" :
+ {
+ "name" : "Winamp",
+ "version" : "3.0",
+ "major" : "3"
+ }
+ },
+ {
+ "desc" : "PSP",
+ "ua" : "PSP-InternetRadioPlayer/1.00",
+ "expect" :
+ {
+ "name" : "PSP-InternetRadioPlayer",
+ "version" : "1.00",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "urllib",
+ "ua" : "Python-urllib/2.7",
+ "expect" :
+ {
+ "name" : "Python-urllib",
+ "version" : "2.7",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "QuickTime",
+ "ua" : "QuickTime/7.5.6 (qtver=7.5.6;cpu=IA32;os=Mac 10.5.8)",
+ "expect" :
+ {
+ "name" : "QuickTime",
+ "version" : "7.5.6",
+ "major" : "7"
+ }
+ },
+ {
+ "desc" : "RMA",
+ "ua" : "RMA/1.0 (compatible; RealMedia)",
+ "expect" :
+ {
+ "name" : "RMA",
+ "version" : "1.0",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "RadioApp",
+ "ua" : "RadioApp/1.0 CFNetwork/609.1.4 Darwin/11.4.2",
+ "expect" :
+ {
+ "name" : "RadioApp",
+ "version" : "1.0",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "RadioClientApplication",
+ "ua" : "RadioClientApplication/20 CFNetwork/609.1.4 Darwin/13.0.0",
+ "expect" :
+ {
+ "name" : "RadioClientApplication",
+ "version" : "20",
+ "major" : "undefined"
+ }
+ },
+ {
+ "desc" : "stagefright",
+ "ua" : "Samsung GT-I9070 stagefright/1.1 (Linux;Android 2.3.6)",
+ "expect" :
+ {
+ "name" : "stagefright",
+ "version" : "1.1",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "Soundtap",
+ "ua" : "Soundtap/1.2.4 CFNetwork/672.0.2 Darwin/14.0.0",
+ "expect" :
+ {
+ "name" : "Soundtap",
+ "version" : "1.2.4",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "Streamium",
+ "ua" : "Streamium/1.0",
+ "expect" :
+ {
+ "name" : "Streamium",
+ "version" : "1.0",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "Totem",
+ "ua" : "Totem/3.0.1",
+ "expect" :
+ {
+ "name" : "Totem",
+ "version" : "3.0.1",
+ "major" : "3"
+ }
+ },
+ {
+ "desc" : "VLC",
+ "ua" : "VLC media player - version 0.8.6c Janus - (c) 1996-2007 the VideoLAN team",
+ "expect" :
+ {
+ "name" : "VLC",
+ "version" : "0.8.6c",
+ "major" : "0"
+ }
+ },
+ {
+ "desc" : "VLC",
+ "ua" : "VLC/2.0.0 LibVLC/2.0.0",
+ "expect" :
+ {
+ "name" : "VLC",
+ "version" : "2.0.0",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "Videos",
+ "ua" : "Videos/3.8.2",
+ "expect" :
+ {
+ "name" : "Video",
+ "version" : "3.8.2",
+ "major" : "3"
+ }
+ },
+ {
+ "desc" : "Wget",
+ "ua" : "Wget/1.12 (darwin10.7.0)",
+ "expect" :
+ {
+ "name" : "Wget",
+ "version" : "1.12",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "Winamp",
+ "ua" : "Winamp 2.81",
+ "expect" :
+ {
+ "name" : "Winamp",
+ "version" : "2.81",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "Winamp",
+ "ua" : "WinampMPEG/2.00",
+ "expect" :
+ {
+ "name" : "Winamp",
+ "version" : "2.00",
+ "major" : "2"
+ }
+ },
+ {
+ "desc" : "Windows Media Player",
+ "ua" : "Windows-Media-Player/10.00.00.4019",
+ "expect" :
+ {
+ "name" : "Windows Media Player",
+ "version" : "10.00.00.4019",
+ "major" : "10"
+ }
+ },
+ {
+ "desc" : "XBMC",
+ "ua" : "XBMC/12.0 Git:20130127-fb595f2 (Windows NT 6.1;WOW64;Win64;x64; http://www.xbmc.org)",
+ "expect" :
+ {
+ "name" : "XBMC",
+ "version" : "12.0",
+ "major" : "12"
+ }
+ },
+ {
+ "desc" : "rad.io",
+ "ua" : "rad.io 1.18.1 rv:593 (iPhone 4S; iPhone OS 7.0.4; it_IT)",
+ "expect" :
+ {
+ "name" : "rad.io",
+ "version" : "1.18.1",
+ "major" : "1"
+ }
+ },
+ {
+ "desc" : "BE-Test",
+ "ua" : "APP-BE Test/1.0 (iPad; Apple; CPU iPhone OS 7_0_2 like Mac OS X)",
+ "expect" :
+ {
+ "name" : "BE Test",
+ "version" : "1.0",
+ "major" : "1"
+ }
+ }
+]
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/os-test.json b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/os-test.json
new file mode 100644
index 00000000..86aa837b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/os-test.json
@@ -0,0 +1,641 @@
+[
+ {
+ "desc" : "Windows 95",
+ "ua" : "Mozilla/1.22 (compatible; MSIE 2.0; Windows 95)",
+ "expect" :
+ {
+ "name" : "Windows",
+ "version" : "95"
+ }
+ },
+ {
+ "desc" : "Windows 98",
+ "ua" : "Mozilla/4.0 (compatible; MSIE 4.01; Windows 98)",
+ "expect" :
+ {
+ "name" : "Windows",
+ "version" : "98"
+ }
+ },
+ {
+ "desc" : "Windows ME",
+ "ua" : "Mozilla/5.0 (Windows; U; Win 9x 4.90) Gecko/20020502 CS 2000 7.0/7.0",
+ "expect" :
+ {
+ "name" : "Windows",
+ "version" : "ME"
+ }
+ },
+ {
+ "desc" : "Windows 2000",
+ "ua" : "Mozilla/3.0 (compatible; MSIE 3.0; Windows NT 5.0)",
+ "expect" :
+ {
+ "name" : "Windows",
+ "version" : "2000"
+ }
+ },
+ {
+ "desc" : "Windows XP",
+ "ua" : "Mozilla/5.0 (Windows; U; MSIE 7.0; Windows NT 5.2)",
+ "expect" :
+ {
+ "name" : "Windows",
+ "version" : "XP"
+ }
+ },
+ {
+ "desc" : "Windows Vista",
+ "ua" : "Mozilla/5.0 (compatible; MSIE 7.0; Windows NT 6.0; fr-FR)",
+ "expect" :
+ {
+ "name" : "Windows",
+ "version" : "Vista"
+ }
+ },
+ {
+ "desc" : "Windows 7",
+ "ua" : "Mozilla/5.0 (compatible; MSIE 10.0; Windows NT 6.1; Trident/6.0)",
+ "expect" :
+ {
+ "name" : "Windows",
+ "version" : "7"
+ }
+ },
+ {
+ "desc" : "Windows 8",
+ "ua" : "Mozilla/4.0 (compatible; MSIE 7.0; Windows NT 6.2; Win64; x64; Trident/6.0; .NET4.0E; .NET4.0C)",
+ "expect" :
+ {
+ "name" : "Windows",
+ "version" : "8"
+ }
+ },
+ {
+ "desc" : "Windows 10",
+ "ua" : "Mozilla/5.0 (Windows NT 10.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/39.0.2171.71 Safari/537.36 Edge/12.0",
+ "expect" :
+ {
+ "name" : "Windows",
+ "version" : "10"
+ }
+ },
+ {
+ "desc" : "Windows RT",
+ "ua" : "Mozilla/5.0 (compatible; MSIE 10.0; Windows NT 6.2; ARM; Trident/6.0)",
+ "expect" :
+ {
+ "name" : "Windows",
+ "version" : "RT"
+ }
+ },
+ {
+ "desc" : "Windows CE",
+ "ua" : "Mozilla/4.0 (compatible; MSIE 6.0; Windows CE; IEMobile 7.11)",
+ "expect" :
+ {
+ "name" : "Windows",
+ "version" : "CE"
+ }
+ },
+ {
+ "desc" : "Windows Mobile",
+ "ua" : "Mozilla/5.0 (ZTE-E_N72/N72V1.0.0B02;U;Windows Mobile/6.1;Profile/MIDP-2.0 Configuration/CLDC-1.1;320*240;CTC/2.0) IE/6.0 (compatible; MSIE 4.01; Windows CE; PPC)/UC Browser7.7.1.88",
+ "expect" :
+ {
+ "name" : "Windows Mobile",
+ "version" : "6.1"
+ }
+ },
+ {
+ "desc" : "Windows Phone",
+ "ua" : "Opera/9.80 (Windows Phone; Opera Mini/7.6.8/35.7518; U; ru) Presto/2.8.119 Version/11.10",
+ "expect" :
+ {
+ "name" : "Windows Phone",
+ "version" : "undefined"
+ }
+ },
+ {
+ "desc" : "Windows Phone OS",
+ "ua" : "Mozilla/4.0 (compatible; MSIE 7.0; Windows Phone OS 7.0; Trident/3.1; IEMobile/7.0; DELL; Venue Pro)",
+ "expect" :
+ {
+ "name" : "Windows Phone OS",
+ "version" : "7.0"
+ }
+ },
+ {
+ "desc" : "Windows Phone 8",
+ "ua" : "Mozilla/5.0 (compatible; MSIE 10.0; Windows Phone 8.0; Trident/6.0; IEMobile/10.0; ARM; Touch; HTC; Windows Phone 8X by HTC)",
+ "expect" :
+ {
+ "name" : "Windows Phone",
+ "version" : "8.0"
+ }
+ },
+ {
+ "desc" : "BlackBerry",
+ "ua" : "BlackBerry9300/5.0.0.912 Profile/MIDP-2.1 Configuration/CLDC-1.1 VendorID/378",
+ "expect" :
+ {
+ "name" : "BlackBerry",
+ "version" : "5.0.0.912"
+ }
+ },
+ {
+ "desc" : "BlackBerry 10",
+ "ua" : "Mozilla/5.0 (BB10; Touch) AppleWebKit/537.3+ (KHTML, like Gecko) Version/10.0.9.386 Mobile Safari/537.3+",
+ "expect" :
+ {
+ "name" : "BlackBerry",
+ "version" : "10"
+ }
+ },
+ {
+ "desc" : "Tizen",
+ "ua" : "Mozilla/5.0 (Linux; Tizen 2.3; SAMSUNG SM-Z130H) AppleWebKit/537.3 (KHTML, like Gecko) Version/2.3 Mobile Safari/537.3",
+ "expect" :
+ {
+ "name" : "Tizen",
+ "version" : "2.3"
+ }
+ },
+ {
+ "desc" : "Android",
+ "ua" : "Mozilla/5.0 (Linux; U; Android 2.2.2; en-us; VM670 Build/FRG83G) AppleWebKit/533.1 (KHTML, like Gecko)",
+ "expect" :
+ {
+ "name" : "Android",
+ "version" : "2.2.2"
+ }
+ },
+ {
+ "desc" : "WebOS",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "Palm OS",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "QNX",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "Bada",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "RIM Tablet OS",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "MeeGo",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "Symbian",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "Firefox OS",
+ "ua" : "Mozilla/5.0 (Mobile; rv:14.0) Gecko/14.0 Firefox/14.0",
+ "expect" :
+ {
+ "name" : "Firefox OS",
+ "version" : "undefined"
+ }
+ },
+ {
+ "desc" : "Nintendo",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "PlayStation",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "PlayStation 4",
+ "ua" : "Mozilla/5.0 (PlayStation 4 3.00) AppleWebKit/537.73 (KHTML, like Gecko)",
+ "expect" :
+ {
+ "name" : "PlayStation",
+ "version" : "4"
+ }
+ },
+ {
+ "desc" : "Mint",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "Joli",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "Ubuntu",
+ "ua" : "Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/535.22+ (KHTML, like Gecko) Chromium/17.0.963.56 Chrome/17.0.963.56 Safari/535.22+ Ubuntu/12.04 (3.4.1-0ubuntu1) Epiphany/3.4.1",
+ "expect" :
+ {
+ "name" : "Ubuntu",
+ "version" : "12.04"
+ }
+ },
+ {
+ "desc" : "Ubuntu",
+ "ua" : "Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) Ubuntu Chromium/31.0.1650.63 Chrome/31.0.1650.63 Safari/537.36",
+ "expect" :
+ {
+ "name" : "Ubuntu",
+ "version" : "undefined"
+ }
+ },
+ {
+ "desc" : "Debian",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "OpenSUSE",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "Gentoo",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "Arch",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "Slackware",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "Fedora",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "Mandriva",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "CentOS",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "PCLinuxOS",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "RedHat",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "Zenwalk",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "Hurd",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "Linux",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "GNU",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "Chromium OS",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "Solaris",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "FreeBSD",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "OpenBSD",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "NetBSD",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "DragonFly",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "iOS in App",
+ "ua" : "AppName/version CFNetwork/version Darwin/version",
+ "expect" :
+ {
+ "name" : "iOS",
+ "version" : "undefined"
+ }
+ },
+ {
+ "desc" : "iOS with Chrome",
+ "ua" : "Mozilla/5.0 (iPhone; U; CPU iPhone OS 5_1_1 like Mac OS X; en) AppleWebKit/534.46.0 (KHTML, like Gecko) CriOS/19.0.1084.60 Mobile/9B206 Safari/7534.48.3",
+ "expect" :
+ {
+ "name" : "iOS",
+ "version" : "5.1.1"
+ }
+ },
+ {
+ "desc" : "iOS with Opera Mini",
+ "ua" : "Opera/9.80 (iPhone; Opera Mini/7.1.32694/27.1407; U; en) Presto/2.8.119 Version/11.10",
+ "expect" :
+ {
+ "name" : "iOS",
+ "version" : "undefined"
+ }
+ },
+ {
+ "desc" : "Mac OS",
+ "ua" : "Mozilla/5.0 (Macintosh; Intel Mac OS X 10_6_8) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/28.0.1500.95 Safari/537.36",
+ "expect" :
+ {
+ "name" : "Mac OS",
+ "version" : "10.6.8"
+ }
+ },
+ {
+ "desc" : "Haiku",
+ "ua" : "Mozilla/5.0 (Macintosh; Intel Haiku R1 x86) AppleWebKit/602.1.1 (KHTML, like Gecko) WebPositive/1.2 Version/8.0 Safari/602.1.1",
+ "expect" :
+ {
+ "name" : "Haiku",
+ "version" : "R1"
+ }
+ },
+ {
+ "desc" : "AIX",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "Plan9",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "Minix",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "BeOS",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "OS/2",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "AmigaOS",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "MorphOS",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "UNIX",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "iTunes Windows Vista",
+ "ua" : "iTunes/10.7 (Windows; Microsoft Windows Vista Home Premium Edition Service Pack 1 (Build 6001)) AppleWebKit/536.26.9",
+ "expect" :
+ {
+ "name" : "Windows",
+ "version" : "Vista"
+ }
+ },
+ {
+ "desc" : "",
+ "ua" : "",
+ "expect" :
+ {
+ "name" : "",
+ "version" : ""
+ }
+ },
+ {
+ "desc" : "iOS BE App",
+ "ua" : "APP-BE Test/1.0 (iPad; Apple; CPU iPhone OS 7_0_2 like Mac OS X)",
+ "expect" :
+ {
+ "name" : "iOS",
+ "version" : "7.0.2"
+ }
+ },
+ {
+ "desc" : "KTB-Nexus 5",
+ "ua" : "APP-My App/1.0 (Linux; Android 4.2.1; Nexus 5 Build/JOP40D)",
+ "expect" :
+ {
+ "name" : "Android",
+ "version" : "4.2.1"
+ }
+ }
+]
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/test.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/test.js
new file mode 100644
index 00000000..99e53ffb
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/ua-parser-js/test/test.js
@@ -0,0 +1,113 @@
+var assert = require('assert');
+var requirejs = require('requirejs');
+var UAParser = require('./../src/ua-parser');
+var browsers = require('./browser-test.json');
+var cpus = require('./cpu-test.json');
+var devices = require('./device-test.json');
+var engines = require('./engine-test.json');
+var os = require('./os-test.json');
+var parser = new UAParser();
+var methods = [
+ {
+ title : 'getBrowser',
+ label : 'browser',
+ list : browsers,
+ properties : ['name', 'major', 'version']
+ },
+ {
+ title : 'getCPU',
+ label : 'cpu',
+ list : cpus,
+ properties : ['architecture']
+ },
+ {
+ title : 'getDevice',
+ label : 'device',
+ list : devices,
+ properties : ['model', 'type', 'vendor']
+ },
+ {
+ title : 'getEngine',
+ label : 'engine',
+ list : engines,
+ properties : ['name', 'version']
+ },
+ {
+ title : 'getOS',
+ label : 'os',
+ list : os,
+ properties : ['name', 'version']
+}];
+
+describe('UAParser()', function () {
+ var ua = 'Mozilla/5.0 (Windows NT 6.2) AppleWebKit/536.6 (KHTML, like Gecko) Chrome/20.0.1090.0 Safari/536.6';
+ assert.deepEqual(UAParser(ua), new UAParser().setUA(ua).getResult());
+});
+
+for (var i in methods) {
+ describe(methods[i]['title'], function () {
+ for (var j in methods[i]['list']) {
+ if (!!methods[i]['list'][j].ua) {
+ describe('[' + methods[i]['list'][j].desc + ']', function () {
+ describe('"' + methods[i]['list'][j].ua + '"', function () {
+ var expect = methods[i]['list'][j].expect;
+ var result = parser.setUA(methods[i]['list'][j].ua).getResult()[methods[i]['label']];
+
+ methods[i]['properties'].forEach(function(m) {
+ it('should return ' + methods[i]['label'] + ' ' + m + ': ' + expect[m], function () {
+ assert.equal(result[m], expect[m] != 'undefined' ? expect[m] : undefined);
+ });
+ });
+ });
+ });
+ }
+ }
+ });
+}
+
+describe('Returns', function () {
+ it('getResult() should returns JSON', function(done) {
+ assert.deepEqual(new UAParser('').getResult(),
+ {
+ ua : '',
+ browser: { name: undefined, version: undefined, major: undefined },
+ cpu: { architecture: undefined },
+ device: { vendor: undefined, model: undefined, type: undefined },
+ engine: { name: undefined, version: undefined},
+ os: { name: undefined, version: undefined }
+ });
+ done();
+ });
+});
+
+describe('Extending Regex', function () {
+ var uaString = 'Mozilla/5.0 MyOwnBrowser/1.3';
+ var myOwnBrowser = [[/(myownbrowser)\/((\d+)?[\w\.]+)/i], [UAParser.BROWSER.NAME, UAParser.BROWSER.VERSION, UAParser.BROWSER.MAJOR]];
+
+ var parser1 = new UAParser(uaString, {browser: myOwnBrowser});
+ assert.equal(parser1.getBrowser().name, 'MyOwnBrowser');
+ assert.equal(parser1.getBrowser().version, '1.3');
+ assert.equal(parser1.getBrowser().major, '1');
+
+ var parser2 = new UAParser({browser: myOwnBrowser});
+ assert.equal(parser2.getBrowser().name, undefined);
+ parser2.setUA(uaString);
+ assert.equal(parser2.getBrowser().name, 'MyOwnBrowser');
+ assert.equal(parser1.getBrowser().version, '1.3');
+});
+
+describe('Using Require.js', function () {
+ it('should loaded automatically', function(done) {
+ requirejs.config({
+ baseUrl : 'dist',
+ paths : {
+ 'ua-parser-js' : 'ua-parser.min'
+ }
+ });
+ requirejs(['ua-parser-js'], function(ua) {
+ var parser = new ua('Dillo/1.0');
+ assert.deepEqual(parser.getBrowser().name, 'Dillo');
+ done();
+ });
+ });
+});
\ No newline at end of file
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/whatwg-fetch/LICENSE b/goTorrentWebUI/node_modules/react-dropzone/node_modules/whatwg-fetch/LICENSE
new file mode 100644
index 00000000..0e319d55
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/whatwg-fetch/LICENSE
@@ -0,0 +1,20 @@
+Copyright (c) 2014-2016 GitHub, Inc.
+
+Permission is hereby granted, free of charge, to any person obtaining
+a copy of this software and associated documentation files (the
+"Software"), to deal in the Software without restriction, including
+without limitation the rights to use, copy, modify, merge, publish,
+distribute, sublicense, and/or sell copies of the Software, and to
+permit persons to whom the Software is furnished to do so, subject to
+the following conditions:
+
+The above copyright notice and this permission notice shall be
+included in all copies or substantial portions of the Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
+EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
+MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
+NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
+LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
+OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
+WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/whatwg-fetch/README.md b/goTorrentWebUI/node_modules/react-dropzone/node_modules/whatwg-fetch/README.md
new file mode 100644
index 00000000..328cb490
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/whatwg-fetch/README.md
@@ -0,0 +1,282 @@
+# window.fetch polyfill
+
+The `fetch()` function is a Promise-based mechanism for programmatically making
+web requests in the browser. This project is a polyfill that implements a subset
+of the standard [Fetch specification][], enough to make `fetch` a viable
+replacement for most uses of XMLHttpRequest in traditional web applications.
+
+This project adheres to the [Open Code of Conduct][]. By participating, you are
+expected to uphold this code.
+
+## Table of Contents
+
+* [Read this first](#read-this-first)
+* [Installation](#installation)
+* [Usage](#usage)
+ * [HTML](#html)
+ * [JSON](#json)
+ * [Response metadata](#response-metadata)
+ * [Post form](#post-form)
+ * [Post JSON](#post-json)
+ * [File upload](#file-upload)
+ * [Caveats](#caveats)
+ * [Handling HTTP error statuses](#handling-http-error-statuses)
+ * [Sending cookies](#sending-cookies)
+ * [Receiving cookies](#receiving-cookies)
+ * [Obtaining the Response URL](#obtaining-the-response-url)
+* [Browser Support](#browser-support)
+
+## Read this first
+
+* If you believe you found a bug with how `fetch` behaves in Chrome or Firefox,
+ please **avoid opening an issue in this repository**. This project is a
+ _polyfill_, and since Chrome and Firefox both implement the `window.fetch`
+ function natively, no code from this project actually takes any effect in
+ these browsers. See [Browser support](#browser-support) for detailed
+ information.
+
+* If you have trouble **making a request to another domain** (a different
+ subdomain or port number also constitutes as another domain), please
+ familiarize yourself with all the intricacies and limitations of [CORS][]
+ requests. Because CORS requires participation of the server by implementing
+ specific HTTP response headers, it is often nontrivial to set up or debug.
+ CORS is exclusively handled by the browser's internal mechanisms which this
+ polyfill cannot influence.
+
+* If you have trouble **maintaining the user's session** or [CSRF][] protection
+ through `fetch` requests, please ensure that you've read and understood the
+ [Sending cookies](#sending-cookies) section.
+
+* If this polyfill **doesn't work under Node.js environments**, that is expected,
+ because this project is meant for web browsers only. You should ensure that your
+ application doesn't try to package and run this on the server.
+
+* If you have an idea for a new feature of `fetch`, please understand that we
+ are only ever going to add features and APIs that are a part of the
+ [Fetch specification][]. You should **submit your feature requests** to the
+ [repository of the specification](https://github.com/whatwg/fetch/issues)
+ itself, rather than this repository.
+
+## Installation
+
+* `npm install whatwg-fetch --save`; or
+
+* `bower install fetch`.
+
+You will also need a Promise polyfill for [older browsers](http://caniuse.com/#feat=promises).
+We recommend [taylorhakes/promise-polyfill](https://github.com/taylorhakes/promise-polyfill)
+for its small size and Promises/A+ compatibility.
+
+For use with webpack, add this package in the `entry` configuration option
+before your application entry point:
+
+```javascript
+entry: ['whatwg-fetch', ...]
+```
+
+For Babel and ES2015+, make sure to import the file:
+
+```javascript
+import 'whatwg-fetch'
+```
+
+## Usage
+
+For a more comprehensive API reference that this polyfill supports, refer to
+https://github.github.io/fetch/.
+
+### HTML
+
+```javascript
+fetch('/users.html')
+ .then(function(response) {
+ return response.text()
+ }).then(function(body) {
+ document.body.innerHTML = body
+ })
+```
+
+### JSON
+
+```javascript
+fetch('/users.json')
+ .then(function(response) {
+ return response.json()
+ }).then(function(json) {
+ console.log('parsed json', json)
+ }).catch(function(ex) {
+ console.log('parsing failed', ex)
+ })
+```
+
+### Response metadata
+
+```javascript
+fetch('/users.json').then(function(response) {
+ console.log(response.headers.get('Content-Type'))
+ console.log(response.headers.get('Date'))
+ console.log(response.status)
+ console.log(response.statusText)
+})
+```
+
+### Post form
+
+```javascript
+var form = document.querySelector('form')
+
+fetch('/users', {
+ method: 'POST',
+ body: new FormData(form)
+})
+```
+
+### Post JSON
+
+```javascript
+fetch('/users', {
+ method: 'POST',
+ headers: {
+ 'Content-Type': 'application/json'
+ },
+ body: JSON.stringify({
+ name: 'Hubot',
+ login: 'hubot',
+ })
+})
+```
+
+### File upload
+
+```javascript
+var input = document.querySelector('input[type="file"]')
+
+var data = new FormData()
+data.append('file', input.files[0])
+data.append('user', 'hubot')
+
+fetch('/avatars', {
+ method: 'POST',
+ body: data
+})
+```
+
+### Caveats
+
+The `fetch` specification differs from `jQuery.ajax()` in mainly two ways that
+bear keeping in mind:
+
+* The Promise returned from `fetch()` **won't reject on HTTP error status**
+ even if the response is an HTTP 404 or 500. Instead, it will resolve normally,
+ and it will only reject on network failure or if anything prevented the
+ request from completing.
+
+* By default, `fetch` **won't send or receive any cookies** from the server,
+ resulting in unauthenticated requests if the site relies on maintaining a user
+ session. See [Sending cookies](#sending-cookies) for how to opt into cookie
+ handling.
+
+#### Handling HTTP error statuses
+
+To have `fetch` Promise reject on HTTP error statuses, i.e. on any non-2xx
+status, define a custom response handler:
+
+```javascript
+function checkStatus(response) {
+ if (response.status >= 200 && response.status < 300) {
+ return response
+ } else {
+ var error = new Error(response.statusText)
+ error.response = response
+ throw error
+ }
+}
+
+function parseJSON(response) {
+ return response.json()
+}
+
+fetch('/users')
+ .then(checkStatus)
+ .then(parseJSON)
+ .then(function(data) {
+ console.log('request succeeded with JSON response', data)
+ }).catch(function(error) {
+ console.log('request failed', error)
+ })
+```
+
+#### Sending cookies
+
+To automatically send cookies for the current domain, the `credentials` option
+must be provided:
+
+```javascript
+fetch('/users', {
+ credentials: 'same-origin'
+})
+```
+
+The "same-origin" value makes `fetch` behave similarly to XMLHttpRequest with
+regards to cookies. Otherwise, cookies won't get sent, resulting in these
+requests not preserving the authentication session.
+
+For [CORS][] requests, use the "include" value to allow sending credentials to
+other domains:
+
+```javascript
+fetch('https://example.com:1234/users', {
+ credentials: 'include'
+})
+```
+
+#### Receiving cookies
+
+As with XMLHttpRequest, the `Set-Cookie` response header returned from the
+server is a [forbidden header name][] and therefore can't be programmatically
+read with `response.headers.get()`. Instead, it's the browser's responsibility
+to handle new cookies being set (if applicable to the current URL). Unless they
+are HTTP-only, new cookies will be available through `document.cookie`.
+
+Bear in mind that the default behavior of `fetch` is to ignore the `Set-Cookie`
+header completely. To opt into accepting cookies from the server, you must use
+the `credentials` option.
+
+#### Obtaining the Response URL
+
+Due to limitations of XMLHttpRequest, the `response.url` value might not be
+reliable after HTTP redirects on older browsers.
+
+The solution is to configure the server to set the response HTTP header
+`X-Request-URL` to the current URL after any redirect that might have happened.
+It should be safe to set it unconditionally.
+
+``` ruby
+# Ruby on Rails controller example
+response.headers['X-Request-URL'] = request.url
+```
+
+This server workaround is necessary if you need reliable `response.url` in
+Firefox < 32, Chrome < 37, Safari, or IE.
+
+## Browser Support
+
+- Chrome
+- Firefox
+- Safari 6.1+
+- Internet Explorer 10+
+
+Note: modern browsers such as Chrome, Firefox, and Microsoft Edge contain native
+implementations of `window.fetch`, therefore the code from this polyfill doesn't
+have any effect on those browsers. If you believe you've encountered an error
+with how `window.fetch` is implemented in any of these browsers, you should file
+an issue with that browser vendor instead of this project.
+
+
+ [fetch specification]: https://fetch.spec.whatwg.org
+ [open code of conduct]: http://todogroup.org/opencodeofconduct/#fetch/opensource@github.com
+ [cors]: https://developer.mozilla.org/en-US/docs/Web/HTTP/Access_control_CORS
+ "Cross-origin resource sharing"
+ [csrf]: https://www.owasp.org/index.php/Cross-Site_Request_Forgery_(CSRF)_Prevention_Cheat_Sheet
+ "Cross-site request forgery"
+ [forbidden header name]: https://developer.mozilla.org/en-US/docs/Glossary/Forbidden_header_name
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/whatwg-fetch/fetch.js b/goTorrentWebUI/node_modules/react-dropzone/node_modules/whatwg-fetch/fetch.js
new file mode 100644
index 00000000..6bac6b39
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/whatwg-fetch/fetch.js
@@ -0,0 +1,461 @@
+(function(self) {
+ 'use strict';
+
+ if (self.fetch) {
+ return
+ }
+
+ var support = {
+ searchParams: 'URLSearchParams' in self,
+ iterable: 'Symbol' in self && 'iterator' in Symbol,
+ blob: 'FileReader' in self && 'Blob' in self && (function() {
+ try {
+ new Blob()
+ return true
+ } catch(e) {
+ return false
+ }
+ })(),
+ formData: 'FormData' in self,
+ arrayBuffer: 'ArrayBuffer' in self
+ }
+
+ if (support.arrayBuffer) {
+ var viewClasses = [
+ '[object Int8Array]',
+ '[object Uint8Array]',
+ '[object Uint8ClampedArray]',
+ '[object Int16Array]',
+ '[object Uint16Array]',
+ '[object Int32Array]',
+ '[object Uint32Array]',
+ '[object Float32Array]',
+ '[object Float64Array]'
+ ]
+
+ var isDataView = function(obj) {
+ return obj && DataView.prototype.isPrototypeOf(obj)
+ }
+
+ var isArrayBufferView = ArrayBuffer.isView || function(obj) {
+ return obj && viewClasses.indexOf(Object.prototype.toString.call(obj)) > -1
+ }
+ }
+
+ function normalizeName(name) {
+ if (typeof name !== 'string') {
+ name = String(name)
+ }
+ if (/[^a-z0-9\-#$%&'*+.\^_`|~]/i.test(name)) {
+ throw new TypeError('Invalid character in header field name')
+ }
+ return name.toLowerCase()
+ }
+
+ function normalizeValue(value) {
+ if (typeof value !== 'string') {
+ value = String(value)
+ }
+ return value
+ }
+
+ // Build a destructive iterator for the value list
+ function iteratorFor(items) {
+ var iterator = {
+ next: function() {
+ var value = items.shift()
+ return {done: value === undefined, value: value}
+ }
+ }
+
+ if (support.iterable) {
+ iterator[Symbol.iterator] = function() {
+ return iterator
+ }
+ }
+
+ return iterator
+ }
+
+ function Headers(headers) {
+ this.map = {}
+
+ if (headers instanceof Headers) {
+ headers.forEach(function(value, name) {
+ this.append(name, value)
+ }, this)
+ } else if (Array.isArray(headers)) {
+ headers.forEach(function(header) {
+ this.append(header[0], header[1])
+ }, this)
+ } else if (headers) {
+ Object.getOwnPropertyNames(headers).forEach(function(name) {
+ this.append(name, headers[name])
+ }, this)
+ }
+ }
+
+ Headers.prototype.append = function(name, value) {
+ name = normalizeName(name)
+ value = normalizeValue(value)
+ var oldValue = this.map[name]
+ this.map[name] = oldValue ? oldValue+','+value : value
+ }
+
+ Headers.prototype['delete'] = function(name) {
+ delete this.map[normalizeName(name)]
+ }
+
+ Headers.prototype.get = function(name) {
+ name = normalizeName(name)
+ return this.has(name) ? this.map[name] : null
+ }
+
+ Headers.prototype.has = function(name) {
+ return this.map.hasOwnProperty(normalizeName(name))
+ }
+
+ Headers.prototype.set = function(name, value) {
+ this.map[normalizeName(name)] = normalizeValue(value)
+ }
+
+ Headers.prototype.forEach = function(callback, thisArg) {
+ for (var name in this.map) {
+ if (this.map.hasOwnProperty(name)) {
+ callback.call(thisArg, this.map[name], name, this)
+ }
+ }
+ }
+
+ Headers.prototype.keys = function() {
+ var items = []
+ this.forEach(function(value, name) { items.push(name) })
+ return iteratorFor(items)
+ }
+
+ Headers.prototype.values = function() {
+ var items = []
+ this.forEach(function(value) { items.push(value) })
+ return iteratorFor(items)
+ }
+
+ Headers.prototype.entries = function() {
+ var items = []
+ this.forEach(function(value, name) { items.push([name, value]) })
+ return iteratorFor(items)
+ }
+
+ if (support.iterable) {
+ Headers.prototype[Symbol.iterator] = Headers.prototype.entries
+ }
+
+ function consumed(body) {
+ if (body.bodyUsed) {
+ return Promise.reject(new TypeError('Already read'))
+ }
+ body.bodyUsed = true
+ }
+
+ function fileReaderReady(reader) {
+ return new Promise(function(resolve, reject) {
+ reader.onload = function() {
+ resolve(reader.result)
+ }
+ reader.onerror = function() {
+ reject(reader.error)
+ }
+ })
+ }
+
+ function readBlobAsArrayBuffer(blob) {
+ var reader = new FileReader()
+ var promise = fileReaderReady(reader)
+ reader.readAsArrayBuffer(blob)
+ return promise
+ }
+
+ function readBlobAsText(blob) {
+ var reader = new FileReader()
+ var promise = fileReaderReady(reader)
+ reader.readAsText(blob)
+ return promise
+ }
+
+ function readArrayBufferAsText(buf) {
+ var view = new Uint8Array(buf)
+ var chars = new Array(view.length)
+
+ for (var i = 0; i < view.length; i++) {
+ chars[i] = String.fromCharCode(view[i])
+ }
+ return chars.join('')
+ }
+
+ function bufferClone(buf) {
+ if (buf.slice) {
+ return buf.slice(0)
+ } else {
+ var view = new Uint8Array(buf.byteLength)
+ view.set(new Uint8Array(buf))
+ return view.buffer
+ }
+ }
+
+ function Body() {
+ this.bodyUsed = false
+
+ this._initBody = function(body) {
+ this._bodyInit = body
+ if (!body) {
+ this._bodyText = ''
+ } else if (typeof body === 'string') {
+ this._bodyText = body
+ } else if (support.blob && Blob.prototype.isPrototypeOf(body)) {
+ this._bodyBlob = body
+ } else if (support.formData && FormData.prototype.isPrototypeOf(body)) {
+ this._bodyFormData = body
+ } else if (support.searchParams && URLSearchParams.prototype.isPrototypeOf(body)) {
+ this._bodyText = body.toString()
+ } else if (support.arrayBuffer && support.blob && isDataView(body)) {
+ this._bodyArrayBuffer = bufferClone(body.buffer)
+ // IE 10-11 can't handle a DataView body.
+ this._bodyInit = new Blob([this._bodyArrayBuffer])
+ } else if (support.arrayBuffer && (ArrayBuffer.prototype.isPrototypeOf(body) || isArrayBufferView(body))) {
+ this._bodyArrayBuffer = bufferClone(body)
+ } else {
+ throw new Error('unsupported BodyInit type')
+ }
+
+ if (!this.headers.get('content-type')) {
+ if (typeof body === 'string') {
+ this.headers.set('content-type', 'text/plain;charset=UTF-8')
+ } else if (this._bodyBlob && this._bodyBlob.type) {
+ this.headers.set('content-type', this._bodyBlob.type)
+ } else if (support.searchParams && URLSearchParams.prototype.isPrototypeOf(body)) {
+ this.headers.set('content-type', 'application/x-www-form-urlencoded;charset=UTF-8')
+ }
+ }
+ }
+
+ if (support.blob) {
+ this.blob = function() {
+ var rejected = consumed(this)
+ if (rejected) {
+ return rejected
+ }
+
+ if (this._bodyBlob) {
+ return Promise.resolve(this._bodyBlob)
+ } else if (this._bodyArrayBuffer) {
+ return Promise.resolve(new Blob([this._bodyArrayBuffer]))
+ } else if (this._bodyFormData) {
+ throw new Error('could not read FormData body as blob')
+ } else {
+ return Promise.resolve(new Blob([this._bodyText]))
+ }
+ }
+
+ this.arrayBuffer = function() {
+ if (this._bodyArrayBuffer) {
+ return consumed(this) || Promise.resolve(this._bodyArrayBuffer)
+ } else {
+ return this.blob().then(readBlobAsArrayBuffer)
+ }
+ }
+ }
+
+ this.text = function() {
+ var rejected = consumed(this)
+ if (rejected) {
+ return rejected
+ }
+
+ if (this._bodyBlob) {
+ return readBlobAsText(this._bodyBlob)
+ } else if (this._bodyArrayBuffer) {
+ return Promise.resolve(readArrayBufferAsText(this._bodyArrayBuffer))
+ } else if (this._bodyFormData) {
+ throw new Error('could not read FormData body as text')
+ } else {
+ return Promise.resolve(this._bodyText)
+ }
+ }
+
+ if (support.formData) {
+ this.formData = function() {
+ return this.text().then(decode)
+ }
+ }
+
+ this.json = function() {
+ return this.text().then(JSON.parse)
+ }
+
+ return this
+ }
+
+ // HTTP methods whose capitalization should be normalized
+ var methods = ['DELETE', 'GET', 'HEAD', 'OPTIONS', 'POST', 'PUT']
+
+ function normalizeMethod(method) {
+ var upcased = method.toUpperCase()
+ return (methods.indexOf(upcased) > -1) ? upcased : method
+ }
+
+ function Request(input, options) {
+ options = options || {}
+ var body = options.body
+
+ if (input instanceof Request) {
+ if (input.bodyUsed) {
+ throw new TypeError('Already read')
+ }
+ this.url = input.url
+ this.credentials = input.credentials
+ if (!options.headers) {
+ this.headers = new Headers(input.headers)
+ }
+ this.method = input.method
+ this.mode = input.mode
+ if (!body && input._bodyInit != null) {
+ body = input._bodyInit
+ input.bodyUsed = true
+ }
+ } else {
+ this.url = String(input)
+ }
+
+ this.credentials = options.credentials || this.credentials || 'omit'
+ if (options.headers || !this.headers) {
+ this.headers = new Headers(options.headers)
+ }
+ this.method = normalizeMethod(options.method || this.method || 'GET')
+ this.mode = options.mode || this.mode || null
+ this.referrer = null
+
+ if ((this.method === 'GET' || this.method === 'HEAD') && body) {
+ throw new TypeError('Body not allowed for GET or HEAD requests')
+ }
+ this._initBody(body)
+ }
+
+ Request.prototype.clone = function() {
+ return new Request(this, { body: this._bodyInit })
+ }
+
+ function decode(body) {
+ var form = new FormData()
+ body.trim().split('&').forEach(function(bytes) {
+ if (bytes) {
+ var split = bytes.split('=')
+ var name = split.shift().replace(/\+/g, ' ')
+ var value = split.join('=').replace(/\+/g, ' ')
+ form.append(decodeURIComponent(name), decodeURIComponent(value))
+ }
+ })
+ return form
+ }
+
+ function parseHeaders(rawHeaders) {
+ var headers = new Headers()
+ rawHeaders.split(/\r?\n/).forEach(function(line) {
+ var parts = line.split(':')
+ var key = parts.shift().trim()
+ if (key) {
+ var value = parts.join(':').trim()
+ headers.append(key, value)
+ }
+ })
+ return headers
+ }
+
+ Body.call(Request.prototype)
+
+ function Response(bodyInit, options) {
+ if (!options) {
+ options = {}
+ }
+
+ this.type = 'default'
+ this.status = 'status' in options ? options.status : 200
+ this.ok = this.status >= 200 && this.status < 300
+ this.statusText = 'statusText' in options ? options.statusText : 'OK'
+ this.headers = new Headers(options.headers)
+ this.url = options.url || ''
+ this._initBody(bodyInit)
+ }
+
+ Body.call(Response.prototype)
+
+ Response.prototype.clone = function() {
+ return new Response(this._bodyInit, {
+ status: this.status,
+ statusText: this.statusText,
+ headers: new Headers(this.headers),
+ url: this.url
+ })
+ }
+
+ Response.error = function() {
+ var response = new Response(null, {status: 0, statusText: ''})
+ response.type = 'error'
+ return response
+ }
+
+ var redirectStatuses = [301, 302, 303, 307, 308]
+
+ Response.redirect = function(url, status) {
+ if (redirectStatuses.indexOf(status) === -1) {
+ throw new RangeError('Invalid status code')
+ }
+
+ return new Response(null, {status: status, headers: {location: url}})
+ }
+
+ self.Headers = Headers
+ self.Request = Request
+ self.Response = Response
+
+ self.fetch = function(input, init) {
+ return new Promise(function(resolve, reject) {
+ var request = new Request(input, init)
+ var xhr = new XMLHttpRequest()
+
+ xhr.onload = function() {
+ var options = {
+ status: xhr.status,
+ statusText: xhr.statusText,
+ headers: parseHeaders(xhr.getAllResponseHeaders() || '')
+ }
+ options.url = 'responseURL' in xhr ? xhr.responseURL : options.headers.get('X-Request-URL')
+ var body = 'response' in xhr ? xhr.response : xhr.responseText
+ resolve(new Response(body, options))
+ }
+
+ xhr.onerror = function() {
+ reject(new TypeError('Network request failed'))
+ }
+
+ xhr.ontimeout = function() {
+ reject(new TypeError('Network request failed'))
+ }
+
+ xhr.open(request.method, request.url, true)
+
+ if (request.credentials === 'include') {
+ xhr.withCredentials = true
+ }
+
+ if ('responseType' in xhr && support.blob) {
+ xhr.responseType = 'blob'
+ }
+
+ request.headers.forEach(function(value, name) {
+ xhr.setRequestHeader(name, value)
+ })
+
+ xhr.send(typeof request._bodyInit === 'undefined' ? null : request._bodyInit)
+ })
+ }
+ self.fetch.polyfill = true
+})(typeof self !== 'undefined' ? self : this);
diff --git a/goTorrentWebUI/node_modules/react-dropzone/node_modules/whatwg-fetch/package.json b/goTorrentWebUI/node_modules/react-dropzone/node_modules/whatwg-fetch/package.json
new file mode 100644
index 00000000..2447f2b8
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/node_modules/whatwg-fetch/package.json
@@ -0,0 +1,55 @@
+{
+ "_from": "whatwg-fetch@>=0.10.0",
+ "_id": "whatwg-fetch@2.0.3",
+ "_inBundle": false,
+ "_integrity": "sha1-nITsLc9oGH/wC8ZOEnS0QhduHIQ=",
+ "_location": "/react-dropzone/whatwg-fetch",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "range",
+ "registry": true,
+ "raw": "whatwg-fetch@>=0.10.0",
+ "name": "whatwg-fetch",
+ "escapedName": "whatwg-fetch",
+ "rawSpec": ">=0.10.0",
+ "saveSpec": null,
+ "fetchSpec": ">=0.10.0"
+ },
+ "_requiredBy": [
+ "/react-dropzone/isomorphic-fetch"
+ ],
+ "_resolved": "https://registry.npmjs.org/whatwg-fetch/-/whatwg-fetch-2.0.3.tgz",
+ "_shasum": "9c84ec2dcf68187ff00bc64e1274b442176e1c84",
+ "_spec": "whatwg-fetch@>=0.10.0",
+ "_where": "C:\\Users\\deranjer\\go\\src\\github.com\\deranjer\\goTorrent\\goTorrentWebUI\\node_modules\\react-dropzone\\node_modules\\isomorphic-fetch",
+ "bugs": {
+ "url": "https://github.com/github/fetch/issues"
+ },
+ "bundleDependencies": false,
+ "deprecated": false,
+ "description": "A window.fetch polyfill.",
+ "devDependencies": {
+ "chai": "1.10.0",
+ "jshint": "2.8.0",
+ "mocha": "2.1.0",
+ "mocha-phantomjs-core": "2.0.1",
+ "promise-polyfill": "6.0.2",
+ "url-search-params": "0.6.1"
+ },
+ "files": [
+ "LICENSE",
+ "fetch.js"
+ ],
+ "homepage": "https://github.com/github/fetch#readme",
+ "license": "MIT",
+ "main": "fetch.js",
+ "name": "whatwg-fetch",
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/github/fetch.git"
+ },
+ "scripts": {
+ "test": "make"
+ },
+ "version": "2.0.3"
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/package.json b/goTorrentWebUI/node_modules/react-dropzone/package.json
new file mode 100644
index 00000000..d10632cd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/package.json
@@ -0,0 +1,167 @@
+{
+ "_from": "react-dropzone",
+ "_id": "react-dropzone@4.2.5",
+ "_inBundle": false,
+ "_integrity": "sha512-bZjPu7MwgyGiuDjlVS4MQeri3VI/wB59mfT5IoKug/WPw4DxFvrtsBagPkNVxfsMmu4fhrb1LY3s/6X1viDWHg==",
+ "_location": "/react-dropzone",
+ "_phantomChildren": {},
+ "_requested": {
+ "type": "tag",
+ "registry": true,
+ "raw": "react-dropzone",
+ "name": "react-dropzone",
+ "escapedName": "react-dropzone",
+ "rawSpec": "",
+ "saveSpec": null,
+ "fetchSpec": "latest"
+ },
+ "_requiredBy": [
+ "#USER"
+ ],
+ "_resolved": "https://registry.npmjs.org/react-dropzone/-/react-dropzone-4.2.5.tgz",
+ "_shasum": "61f4ea914ac8b0a8c7f39795c74c4718d03b13f1",
+ "_spec": "react-dropzone",
+ "_where": "C:\\Users\\deranjer\\go\\src\\github.com\\deranjer\\goTorrent\\goTorrentWebUI",
+ "author": {
+ "name": "Param Aggarwal"
+ },
+ "bugs": {
+ "url": "https://github.com/react-dropzone/react-dropzone/issues"
+ },
+ "bundleDependencies": false,
+ "config": {
+ "commitizen": {
+ "path": "@commitlint/prompt"
+ }
+ },
+ "contributors": [
+ {
+ "name": "Andrey Okonetchnikov",
+ "email": "andrey@okonet.ru",
+ "url": "http://okonet.ru"
+ },
+ {
+ "name": "Mike Olson",
+ "email": "me@mwolson.org"
+ },
+ {
+ "name": "Param Aggarwal"
+ },
+ {
+ "name": "Tyler Waters",
+ "email": "tyler.waters@gmail.com"
+ }
+ ],
+ "dependencies": {
+ "attr-accept": "^1.0.3",
+ "prop-types": "^15.5.7"
+ },
+ "deprecated": false,
+ "description": "Simple HTML5 drag-drop zone with React.js",
+ "devDependencies": {
+ "@commitlint/cli": "^3.2.0",
+ "@commitlint/config-angular": "^3.0.3",
+ "@commitlint/prompt": "^3.2.0",
+ "@commitlint/prompt-cli": "^3.2.0",
+ "babel-cli": "^6.9.0",
+ "babel-core": "^6.9.1",
+ "babel-eslint": "^7.1.1",
+ "babel-jest": "^21.0.0",
+ "babel-loader": "^7.1.2",
+ "babel-plugin-add-module-exports": "^0.2.1",
+ "babel-preset-env": "^1.6.0",
+ "babel-preset-react": "^6.3.13",
+ "babel-preset-stage-1": "^6.24.1",
+ "babel-register": "^6.9.0",
+ "commitizen": "^2.9.6",
+ "cross-env": "^5.1.3",
+ "css-loader": "^0.28.7",
+ "enzyme": "^2.6.0",
+ "eslint": "^4.6.1",
+ "eslint-config-okonet": "^5.0.1",
+ "eslint-config-prettier": "^2.4.0",
+ "eslint-plugin-prettier": "^2.2.0",
+ "husky": "^0.14.3",
+ "imagemin-cli": "^3.0.0",
+ "imagemin-pngquant": "^5.0.0",
+ "jest": "^21.0.1",
+ "jest-enzyme": "^3.8.2",
+ "lint-staged": "^4.1.0",
+ "markdownlint-cli": "^0.3.1",
+ "prettier": "^1.6.1",
+ "react": "^15.4.1",
+ "react-dom": "^15.4.1",
+ "react-styleguidist": "^6.0.23",
+ "react-test-renderer": "^15.6.1",
+ "rimraf": "^2.5.2",
+ "semantic-release": "^7.0.2",
+ "sinon": "^3.2.1",
+ "size-limit": "^0.11.0",
+ "style-loader": "^0.18.2",
+ "webpack": "^3.5.5"
+ },
+ "homepage": "https://github.com/react-dropzone/react-dropzone",
+ "jest": {
+ "setupTestFrameworkScriptFile": "/testSetup.js"
+ },
+ "keywords": [
+ "react-component",
+ "react",
+ "drag",
+ "drop",
+ "upload"
+ ],
+ "license": "MIT",
+ "lint-staged": {
+ "*.js": [
+ "eslint --fix",
+ "git add"
+ ],
+ "*.{svg,png}": [
+ "imagemin",
+ "git add"
+ ]
+ },
+ "main": "dist/index.js",
+ "module": "dist/es/index.js",
+ "name": "react-dropzone",
+ "peerDependencies": {
+ "react": ">=0.14.0"
+ },
+ "repository": {
+ "type": "git",
+ "url": "git+https://github.com/react-dropzone/react-dropzone.git"
+ },
+ "scripts": {
+ "build": "npm run clean && npm run build:umd && npm run build:es",
+ "build:es": "cross-env BABEL_ENV=es babel ./src --out-dir ./dist/es --ignore spec.js",
+ "build:umd": "cross-env NODE_ENV=production webpack",
+ "ci": "git-cz",
+ "clean": "rimraf ./dist",
+ "commitmsg": "commitlint -e",
+ "eslint:src": "eslint ./src ./examples ./*.js",
+ "imagemin": "imagemin --out-dir=logo --plugin=pngquant --plugin=svgo",
+ "logo": "cd logo && sketchtool export artboards logo.sketch",
+ "precommit": "lint-staged",
+ "prepublish": "npm run build && npm run test:umd && npm run test:es",
+ "semantic-release": "semantic-release pre && npm publish && semantic-release post",
+ "size": "size-limit",
+ "size:why": "size-limit --why",
+ "start": "styleguidist server",
+ "styleguide": "styleguidist build",
+ "test": "npm run eslint:src && jest --coverage && npm run size",
+ "test:es": "cross-env JEST_TARGET=../dist/es/index jest --coverage",
+ "test:umd": "cross-env JEST_TARGET=../dist/index jest --coverage"
+ },
+ "size-limit": [
+ {
+ "path": "dist/index.js",
+ "limit": "6 KB"
+ },
+ {
+ "path": "dist/es/index.js",
+ "limit": "3 KB"
+ }
+ ],
+ "version": "4.2.5"
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/src/__snapshots__/index.spec.js.snap b/goTorrentWebUI/node_modules/react-dropzone/src/__snapshots__/index.spec.js.snap
new file mode 100644
index 00000000..0fcec9da
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/src/__snapshots__/index.spec.js.snap
@@ -0,0 +1,7 @@
+// Jest Snapshot v1, https://goo.gl/fbAQLP
+
+exports[`Dropzone basics should render children 1`] = `""`;
+
+exports[`Dropzone document drop protection does not prevent stray drops when preventDropOnDocument is false 1`] = `"
"`;
+
+exports[`Dropzone document drop protection installs hooks to prevent stray drops from taking over the browser window 1`] = `""`;
diff --git a/goTorrentWebUI/node_modules/react-dropzone/src/index.js b/goTorrentWebUI/node_modules/react-dropzone/src/index.js
new file mode 100644
index 00000000..a31f83bd
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/src/index.js
@@ -0,0 +1,585 @@
+/* eslint prefer-template: 0 */
+
+import React from 'react'
+import PropTypes from 'prop-types'
+import {
+ supportMultiple,
+ fileAccepted,
+ allFilesAccepted,
+ fileMatchSize,
+ onDocumentDragOver,
+ getDataTransferItems
+} from './utils'
+import styles from './utils/styles'
+
+class Dropzone extends React.Component {
+ constructor(props, context) {
+ super(props, context)
+ this.composeHandlers = this.composeHandlers.bind(this)
+ this.onClick = this.onClick.bind(this)
+ this.onDocumentDrop = this.onDocumentDrop.bind(this)
+ this.onDragEnter = this.onDragEnter.bind(this)
+ this.onDragLeave = this.onDragLeave.bind(this)
+ this.onDragOver = this.onDragOver.bind(this)
+ this.onDragStart = this.onDragStart.bind(this)
+ this.onDrop = this.onDrop.bind(this)
+ this.onFileDialogCancel = this.onFileDialogCancel.bind(this)
+ this.onInputElementClick = this.onInputElementClick.bind(this)
+
+ this.setRef = this.setRef.bind(this)
+ this.setRefs = this.setRefs.bind(this)
+
+ this.isFileDialogActive = false
+
+ this.state = {
+ draggedFiles: [],
+ acceptedFiles: [],
+ rejectedFiles: []
+ }
+ }
+
+ componentDidMount() {
+ const { preventDropOnDocument } = this.props
+ this.dragTargets = []
+
+ if (preventDropOnDocument) {
+ document.addEventListener('dragover', onDocumentDragOver, false)
+ document.addEventListener('drop', this.onDocumentDrop, false)
+ }
+ this.fileInputEl.addEventListener('click', this.onInputElementClick, false)
+ // Tried implementing addEventListener, but didn't work out
+ document.body.onfocus = this.onFileDialogCancel
+ }
+
+ componentWillUnmount() {
+ const { preventDropOnDocument } = this.props
+ if (preventDropOnDocument) {
+ document.removeEventListener('dragover', onDocumentDragOver)
+ document.removeEventListener('drop', this.onDocumentDrop)
+ }
+ if (this.fileInputEl != null) {
+ this.fileInputEl.removeEventListener('click', this.onInputElementClick, false)
+ }
+ // Can be replaced with removeEventListener, if addEventListener works
+ if (document != null) {
+ document.body.onfocus = null
+ }
+ }
+
+ composeHandlers(handler) {
+ if (this.props.disabled) {
+ return null
+ }
+
+ return handler
+ }
+
+ onDocumentDrop(evt) {
+ if (this.node && this.node.contains(evt.target)) {
+ // if we intercepted an event for our instance, let it propagate down to the instance's onDrop handler
+ return
+ }
+ evt.preventDefault()
+ this.dragTargets = []
+ }
+
+ onDragStart(evt) {
+ if (this.props.onDragStart) {
+ this.props.onDragStart.call(this, evt)
+ }
+ }
+
+ onDragEnter(evt) {
+ evt.preventDefault()
+
+ // Count the dropzone and any children that are entered.
+ if (this.dragTargets.indexOf(evt.target) === -1) {
+ this.dragTargets.push(evt.target)
+ }
+
+ this.setState({
+ isDragActive: true, // Do not rely on files for the drag state. It doesn't work in Safari.
+ draggedFiles: getDataTransferItems(evt)
+ })
+
+ if (this.props.onDragEnter) {
+ this.props.onDragEnter.call(this, evt)
+ }
+ }
+
+ onDragOver(evt) {
+ // eslint-disable-line class-methods-use-this
+ evt.preventDefault()
+ evt.stopPropagation()
+ try {
+ // The file dialog on Chrome allows users to drag files from the dialog onto
+ // the dropzone, causing the browser the crash when the file dialog is closed.
+ // A drop effect of 'none' prevents the file from being dropped
+ evt.dataTransfer.dropEffect = this.isFileDialogActive ? 'none' : 'copy' // eslint-disable-line no-param-reassign
+ } catch (err) {
+ // continue regardless of error
+ }
+
+ if (this.props.onDragOver) {
+ this.props.onDragOver.call(this, evt)
+ }
+ return false
+ }
+
+ onDragLeave(evt) {
+ evt.preventDefault()
+
+ // Only deactivate once the dropzone and all children have been left.
+ this.dragTargets = this.dragTargets.filter(el => el !== evt.target && this.node.contains(el))
+ if (this.dragTargets.length > 0) {
+ return
+ }
+
+ // Clear dragging files state
+ this.setState({
+ isDragActive: false,
+ draggedFiles: []
+ })
+
+ if (this.props.onDragLeave) {
+ this.props.onDragLeave.call(this, evt)
+ }
+ }
+
+ onDrop(evt) {
+ const { onDrop, onDropAccepted, onDropRejected, multiple, disablePreview, accept } = this.props
+ const fileList = getDataTransferItems(evt)
+ const acceptedFiles = []
+ const rejectedFiles = []
+
+ // Stop default browser behavior
+ evt.preventDefault()
+
+ // Reset the counter along with the drag on a drop.
+ this.dragTargets = []
+ this.isFileDialogActive = false
+
+ fileList.forEach(file => {
+ if (!disablePreview) {
+ try {
+ file.preview = window.URL.createObjectURL(file) // eslint-disable-line no-param-reassign
+ } catch (err) {
+ if (process.env.NODE_ENV !== 'production') {
+ console.error('Failed to generate preview for file', file, err) // eslint-disable-line no-console
+ }
+ }
+ }
+
+ if (
+ fileAccepted(file, accept) &&
+ fileMatchSize(file, this.props.maxSize, this.props.minSize)
+ ) {
+ acceptedFiles.push(file)
+ } else {
+ rejectedFiles.push(file)
+ }
+ })
+
+ if (!multiple) {
+ // if not in multi mode add any extra accepted files to rejected.
+ // This will allow end users to easily ignore a multi file drop in "single" mode.
+ rejectedFiles.push(...acceptedFiles.splice(1))
+ }
+
+ if (onDrop) {
+ onDrop.call(this, acceptedFiles, rejectedFiles, evt)
+ }
+
+ if (rejectedFiles.length > 0 && onDropRejected) {
+ onDropRejected.call(this, rejectedFiles, evt)
+ }
+
+ if (acceptedFiles.length > 0 && onDropAccepted) {
+ onDropAccepted.call(this, acceptedFiles, evt)
+ }
+
+ // Clear files value
+ this.draggedFiles = null
+
+ // Reset drag state
+ this.setState({
+ isDragActive: false,
+ draggedFiles: [],
+ acceptedFiles,
+ rejectedFiles
+ })
+ }
+
+ onClick(evt) {
+ const { onClick, disableClick } = this.props
+ if (!disableClick) {
+ evt.stopPropagation()
+
+ if (onClick) {
+ onClick.call(this, evt)
+ }
+
+ // in IE11/Edge the file-browser dialog is blocking, ensure this is behind setTimeout
+ // this is so react can handle state changes in the onClick prop above above
+ // see: https://github.com/react-dropzone/react-dropzone/issues/450
+ setTimeout(this.open.bind(this), 0)
+ }
+ }
+
+ onInputElementClick(evt) {
+ evt.stopPropagation()
+ if (this.props.inputProps && this.props.inputProps.onClick) {
+ this.props.inputProps.onClick()
+ }
+ }
+
+ onFileDialogCancel() {
+ // timeout will not recognize context of this method
+ const { onFileDialogCancel } = this.props
+ const { fileInputEl } = this
+ let { isFileDialogActive } = this
+ // execute the timeout only if the onFileDialogCancel is defined and FileDialog
+ // is opened in the browser
+ if (onFileDialogCancel && isFileDialogActive) {
+ setTimeout(() => {
+ // Returns an object as FileList
+ const FileList = fileInputEl.files
+ if (!FileList.length) {
+ isFileDialogActive = false
+ onFileDialogCancel()
+ }
+ }, 300)
+ }
+ }
+
+ setRef(ref) {
+ this.node = ref
+ }
+
+ setRefs(ref) {
+ this.fileInputEl = ref
+ }
+ /**
+ * Open system file upload dialog.
+ *
+ * @public
+ */
+ open() {
+ this.isFileDialogActive = true
+ this.fileInputEl.value = null
+ this.fileInputEl.click()
+ }
+
+ renderChildren = (children, isDragActive, isDragAccept, isDragReject) => {
+ if (typeof children === 'function') {
+ return children({
+ ...this.state,
+ isDragActive,
+ isDragAccept,
+ isDragReject
+ })
+ }
+ return children
+ }
+
+ render() {
+ const {
+ accept,
+ acceptClassName,
+ activeClassName,
+ children,
+ disabled,
+ disabledClassName,
+ inputProps,
+ multiple,
+ name,
+ rejectClassName,
+ ...rest
+ } = this.props
+
+ let {
+ acceptStyle,
+ activeStyle,
+ className = '',
+ disabledStyle,
+ rejectStyle,
+ style,
+ ...props // eslint-disable-line prefer-const
+ } = rest
+
+ const { isDragActive, draggedFiles } = this.state
+ const filesCount = draggedFiles.length
+ const isMultipleAllowed = multiple || filesCount <= 1
+ const isDragAccept = filesCount > 0 && allFilesAccepted(draggedFiles, this.props.accept)
+ const isDragReject = filesCount > 0 && (!isDragAccept || !isMultipleAllowed)
+ const noStyles =
+ !className && !style && !activeStyle && !acceptStyle && !rejectStyle && !disabledStyle
+
+ if (isDragActive && activeClassName) {
+ className += ' ' + activeClassName
+ }
+ if (isDragAccept && acceptClassName) {
+ className += ' ' + acceptClassName
+ }
+ if (isDragReject && rejectClassName) {
+ className += ' ' + rejectClassName
+ }
+ if (disabled && disabledClassName) {
+ className += ' ' + disabledClassName
+ }
+
+ if (noStyles) {
+ style = styles.default
+ activeStyle = styles.active
+ acceptStyle = style.active
+ rejectStyle = styles.rejected
+ disabledStyle = styles.disabled
+ }
+
+ let appliedStyle = { ...style }
+ if (activeStyle && isDragActive) {
+ appliedStyle = {
+ ...style,
+ ...activeStyle
+ }
+ }
+ if (acceptStyle && isDragAccept) {
+ appliedStyle = {
+ ...appliedStyle,
+ ...acceptStyle
+ }
+ }
+ if (rejectStyle && isDragReject) {
+ appliedStyle = {
+ ...appliedStyle,
+ ...rejectStyle
+ }
+ }
+ if (disabledStyle && disabled) {
+ appliedStyle = {
+ ...style,
+ ...disabledStyle
+ }
+ }
+
+ const inputAttributes = {
+ accept,
+ disabled,
+ type: 'file',
+ style: { display: 'none' },
+ multiple: supportMultiple && multiple,
+ ref: this.setRefs,
+ onChange: this.onDrop,
+ autoComplete: 'off'
+ }
+
+ if (name && name.length) {
+ inputAttributes.name = name
+ }
+
+ // Destructure custom props away from props used for the div element
+ const {
+ acceptedFiles,
+ preventDropOnDocument,
+ disablePreview,
+ disableClick,
+ onDropAccepted,
+ onDropRejected,
+ onFileDialogCancel,
+ maxSize,
+ minSize,
+ ...divProps
+ } = props
+
+ return (
+
+ {this.renderChildren(children, isDragActive, isDragAccept, isDragReject)}
+
+
+ )
+ }
+}
+
+export default Dropzone
+
+Dropzone.propTypes = {
+ /**
+ * Allow specific types of files. See https://github.com/okonet/attr-accept for more information.
+ * Keep in mind that mime type determination is not reliable across platforms. CSV files,
+ * for example, are reported as text/plain under macOS but as application/vnd.ms-excel under
+ * Windows. In some cases there might not be a mime type set at all.
+ * See: https://github.com/react-dropzone/react-dropzone/issues/276
+ */
+ accept: PropTypes.string,
+
+ /**
+ * Contents of the dropzone
+ */
+ children: PropTypes.oneOfType([PropTypes.node, PropTypes.func]),
+
+ /**
+ * Disallow clicking on the dropzone container to open file dialog
+ */
+ disableClick: PropTypes.bool,
+
+ /**
+ * Enable/disable the dropzone entirely
+ */
+ disabled: PropTypes.bool,
+
+ /**
+ * Enable/disable preview generation
+ */
+ disablePreview: PropTypes.bool,
+
+ /**
+ * If false, allow dropped items to take over the current browser window
+ */
+ preventDropOnDocument: PropTypes.bool,
+
+ /**
+ * Pass additional attributes to the ` ` tag
+ */
+ inputProps: PropTypes.object,
+
+ /**
+ * Allow dropping multiple files
+ */
+ multiple: PropTypes.bool,
+
+ /**
+ * `name` attribute for the input tag
+ */
+ name: PropTypes.string,
+
+ /**
+ * Maximum file size
+ */
+ maxSize: PropTypes.number,
+
+ /**
+ * Minimum file size
+ */
+ minSize: PropTypes.number,
+
+ /**
+ * className
+ */
+ className: PropTypes.string,
+
+ /**
+ * className for active state
+ */
+ activeClassName: PropTypes.string,
+
+ /**
+ * className for accepted state
+ */
+ acceptClassName: PropTypes.string,
+
+ /**
+ * className for rejected state
+ */
+ rejectClassName: PropTypes.string,
+
+ /**
+ * className for disabled state
+ */
+ disabledClassName: PropTypes.string,
+
+ /**
+ * CSS styles to apply
+ */
+ style: PropTypes.object,
+
+ /**
+ * CSS styles to apply when drag is active
+ */
+ activeStyle: PropTypes.object,
+
+ /**
+ * CSS styles to apply when drop will be accepted
+ */
+ acceptStyle: PropTypes.object,
+
+ /**
+ * CSS styles to apply when drop will be rejected
+ */
+ rejectStyle: PropTypes.object,
+
+ /**
+ * CSS styles to apply when dropzone is disabled
+ */
+ disabledStyle: PropTypes.object,
+
+ /**
+ * onClick callback
+ * @param {Event} event
+ */
+ onClick: PropTypes.func,
+
+ /**
+ * onDrop callback
+ */
+ onDrop: PropTypes.func,
+
+ /**
+ * onDropAccepted callback
+ */
+ onDropAccepted: PropTypes.func,
+
+ /**
+ * onDropRejected callback
+ */
+ onDropRejected: PropTypes.func,
+
+ /**
+ * onDragStart callback
+ */
+ onDragStart: PropTypes.func,
+
+ /**
+ * onDragEnter callback
+ */
+ onDragEnter: PropTypes.func,
+
+ /**
+ * onDragOver callback
+ */
+ onDragOver: PropTypes.func,
+
+ /**
+ * onDragLeave callback
+ */
+ onDragLeave: PropTypes.func,
+
+ /**
+ * Provide a callback on clicking the cancel button of the file dialog
+ */
+ onFileDialogCancel: PropTypes.func
+}
+
+Dropzone.defaultProps = {
+ preventDropOnDocument: true,
+ disabled: false,
+ disablePreview: false,
+ disableClick: false,
+ multiple: true,
+ maxSize: Infinity,
+ minSize: 0
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/src/index.spec.js b/goTorrentWebUI/node_modules/react-dropzone/src/index.spec.js
new file mode 100644
index 00000000..edea701b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/src/index.spec.js
@@ -0,0 +1,1108 @@
+import React from 'react'
+import { mount, render } from 'enzyme'
+import { spy, stub } from 'sinon'
+import { onDocumentDragOver } from './utils'
+
+const Dropzone = require(process.env.JEST_TARGET ? process.env.JEST_TARGET : './index') // eslint-disable-line import/no-dynamic-require
+const DummyChildComponent = () => null
+
+let files
+let images
+
+const rejectColor = 'red'
+const acceptColor = 'green'
+
+const rejectStyle = {
+ color: rejectColor,
+ borderColor: 'black'
+}
+
+const acceptStyle = {
+ color: acceptColor,
+ borderWidth: '5px'
+}
+
+describe('Dropzone', () => {
+ beforeEach(() => {
+ files = [
+ {
+ name: 'file1.pdf',
+ size: 1111,
+ type: 'application/pdf'
+ }
+ ]
+
+ images = [
+ {
+ name: 'cats.gif',
+ size: 1234,
+ type: 'image/gif'
+ },
+ {
+ name: 'dogs.jpg',
+ size: 2345,
+ type: 'image/jpeg'
+ }
+ ]
+ })
+
+ describe('basics', () => {
+ it('should render children', () => {
+ const dropzone = mount(
+
+ some content
+
+ )
+ expect(dropzone.html()).toMatchSnapshot()
+ })
+
+ it('should render an input HTML element', () => {
+ const dropzone = mount(
+
+ some content
+
+ )
+ expect(dropzone.find('input').length).toEqual(1)
+ })
+
+ it('sets ref properly', () => {
+ const dropzone = mount( )
+ expect(dropzone.instance().fileInputEl).not.toBeUndefined()
+ expect(dropzone.instance().fileInputEl.tagName).toEqual('INPUT')
+ })
+
+ it('renders dynamic props on the root element', () => {
+ const component = mount( )
+ expect(component.html()).toContain('aria-hidden="true"')
+ expect(component.html()).toContain('hidden=""')
+ expect(component.html()).toContain('title="Dropzone"')
+ })
+
+ it('renders dynamic props on the input element', () => {
+ const component = mount( )
+ expect(component.find('input').html()).toContain('id="hiddenFileInput"')
+ })
+
+ it('applies the accept prop to the child input', () => {
+ const component = render( )
+ expect(component.find('.my-dropzone').attr()).not.toContain('accept')
+ expect(Object.keys(component.find('input').attr())).toContain('accept')
+ expect(component.find('input').attr('accept')).toEqual('image/jpeg')
+ })
+
+ it('applies the name prop to the child input', () => {
+ const component = render( )
+ expect(component.find('.my-dropzone').attr()).not.toContain('name')
+ expect(Object.keys(component.find('input').attr())).toContain('name')
+ expect(component.find('input').attr('name')).toEqual('test-file-input')
+ })
+
+ it('should render children function', () => {
+ const content = some content
+ const dropzone = mount({content} )
+ const dropzoneWithFunction = mount({() => content} )
+ expect(dropzoneWithFunction.html()).toEqual(dropzone.html())
+ })
+ })
+
+ describe('document drop protection', () => {
+ let dropzone
+ let addEventCalls
+ let savedAddEventListener
+ let savedRemoveEventListener
+
+ beforeEach(() => {
+ savedAddEventListener = document.addEventListener
+ savedRemoveEventListener = document.removeEventListener
+ document.addEventListener = spy()
+ document.removeEventListener = spy()
+ })
+
+ afterEach(() => {
+ document.addEventListener = savedAddEventListener
+ document.removeEventListener = savedRemoveEventListener
+ })
+
+ // Collect the list of addEventListener/removeEventListener spy calls into an object keyed by event name.
+ function collectEventListenerCalls(calls) {
+ return calls.reduce((acc, [eventName, ...rest]) => {
+ acc[eventName] = rest // eslint-disable-line no-param-reassign
+ return acc
+ }, {})
+ }
+
+ it('installs hooks to prevent stray drops from taking over the browser window', () => {
+ dropzone = mount(
+
+ Content
+
+ )
+ expect(dropzone.html()).toMatchSnapshot()
+ expect(document.addEventListener.callCount).toEqual(2)
+ addEventCalls = collectEventListenerCalls(document.addEventListener.args)
+ Object.keys(addEventCalls).forEach(eventName => {
+ expect(addEventCalls[eventName][0]).toBeDefined()
+ expect(addEventCalls[eventName][1]).toBe(false)
+ })
+ })
+
+ it('terminates drags and drops on elements outside our dropzone', () => {
+ const event = { preventDefault: spy() }
+ onDocumentDragOver(event)
+ expect(event.preventDefault.callCount).toEqual(1)
+ event.preventDefault.reset()
+
+ dropzone.getNode().onDocumentDrop(event)
+ expect(event.preventDefault.callCount).toEqual(1)
+ })
+
+ it('permits drags and drops on elements inside our dropzone', () => {
+ const instanceEvent = {
+ preventDefault: spy(),
+ target: dropzone.getDOMNode()
+ }
+ dropzone.getNode().onDocumentDrop(instanceEvent)
+ expect(instanceEvent.preventDefault.callCount).toEqual(0)
+ })
+
+ it('removes document hooks when unmounted', () => {
+ dropzone.unmount()
+ expect(document.removeEventListener.callCount).toEqual(2)
+ const removeEventCalls = collectEventListenerCalls(document.removeEventListener.args)
+ Object.keys(addEventCalls).forEach(eventName => {
+ expect(removeEventCalls[eventName][0]).toEqual(addEventCalls[eventName][0])
+ })
+ })
+
+ it('does not prevent stray drops when preventDropOnDocument is false', () => {
+ dropzone = mount( )
+ expect(dropzone.html()).toMatchSnapshot()
+ expect(document.addEventListener.callCount).toEqual(0)
+
+ dropzone.unmount()
+ expect(document.removeEventListener.callCount).toEqual(0)
+ })
+ })
+
+ describe('onClick', () => {
+ it('should call `open` method', done => {
+ const dropzone = mount( )
+ spy(dropzone.instance(), 'open')
+ dropzone.simulate('click')
+ setTimeout(() => {
+ expect(dropzone.instance().open.callCount).toEqual(1)
+ done()
+ }, 0)
+ })
+
+ it('should not call `open` if disableClick prop is true', () => {
+ const dropzone = mount( )
+ spy(dropzone.instance(), 'open')
+ dropzone.simulate('click')
+ expect(dropzone.instance().open.callCount).toEqual(0)
+ })
+
+ it('should call `onClick` callback if provided', done => {
+ const clickSpy = spy()
+ const dropzone = mount( )
+ spy(dropzone.instance(), 'open')
+ dropzone.simulate('click')
+ setTimeout(() => {
+ expect(dropzone.instance().open.callCount).toEqual(1)
+ expect(clickSpy.callCount).toEqual(1)
+ done()
+ }, 0)
+ })
+
+ it('should reset the value of input', () => {
+ const dropzone = mount( )
+ expect(
+ dropzone
+ .render()
+ .find('input')
+ .attr('value')
+ ).toBeUndefined()
+ expect(
+ dropzone
+ .render()
+ .find('input')
+ .attr('value', 10)
+ ).not.toBeUndefined()
+ dropzone.simulate('click')
+ expect(
+ dropzone
+ .render()
+ .find('input')
+ .attr('value')
+ ).toBeUndefined()
+ })
+
+ it('should trigger click even on the input', done => {
+ const dropzone = mount( )
+ const clickSpy = spy(dropzone.instance().fileInputEl, 'click')
+ dropzone.simulate('click')
+ dropzone.simulate('click')
+ setTimeout(() => {
+ expect(clickSpy.callCount).toEqual(2)
+ done()
+ }, 0)
+ })
+
+ it('should not invoke onClick on the wrapper', () => {
+ const onClickOuterSpy = spy()
+ const onClickInnerSpy = spy()
+ const component = mount(
+
+
+
+ )
+
+ component.simulate('click')
+ expect(onClickOuterSpy.callCount).toEqual(1)
+ expect(onClickInnerSpy.callCount).toEqual(0)
+
+ onClickOuterSpy.reset()
+ onClickInnerSpy.reset()
+
+ component.find('Dropzone').simulate('click')
+ expect(onClickOuterSpy.callCount).toEqual(0)
+ expect(onClickInnerSpy.callCount).toEqual(1)
+ })
+
+ it('should invoke onClick on the wrapper if disableClick is set', () => {
+ const onClickOuterSpy = spy()
+ const component = mount(
+
+
+
+ )
+
+ component.find('Dropzone').simulate('click')
+ expect(onClickOuterSpy.callCount).toEqual(1)
+ })
+
+ it('should invoke inputProps onClick if provided', done => {
+ const inputPropsClickSpy = spy()
+ const component = mount( )
+
+ component.find('Dropzone').simulate('click')
+ setTimeout(() => {
+ expect(inputPropsClickSpy.callCount).toEqual(1)
+ done()
+ }, 0)
+ })
+ })
+
+ describe('drag-n-drop', () => {
+ it('should override onDrag* methods', () => {
+ const dragStartSpy = spy()
+ const dragEnterSpy = spy()
+ const dragOverSpy = spy()
+ const dragLeaveSpy = spy()
+ const component = mount(
+
+ )
+ component.simulate('dragStart')
+ component.simulate('dragEnter', { dataTransfer: { items: files } })
+ component.simulate('dragOver', { dataTransfer: { items: files } })
+ component.simulate('dragLeave', { dataTransfer: { items: files } })
+ expect(dragStartSpy.callCount).toEqual(1)
+ expect(dragEnterSpy.callCount).toEqual(1)
+ expect(dragOverSpy.callCount).toEqual(1)
+ expect(dragLeaveSpy.callCount).toEqual(1)
+ })
+
+ it('should guard dropEffect in onDragOver for IE', () => {
+ const dragStartSpy = spy()
+ const dragEnterSpy = spy()
+ const dragLeaveSpy = spy()
+ const component = mount(
+
+ )
+
+ // Using Proxy we'll emulate IE throwing when setting dataTransfer.dropEffect
+ const eventProxy = new Proxy(
+ {},
+ {
+ get: (target, prop) => {
+ switch (prop) {
+ case 'dataTransfer':
+ throw new Error('IE does not support rrror')
+ default:
+ return function noop() {}
+ }
+ }
+ }
+ )
+
+ // And using then we'll call the onDragOver with the proxy instead of event
+ const dragOverSpy = stub(component.instance(), 'onDragOver').callsFake(
+ component.instance().onDragOver(eventProxy)
+ )
+
+ component.simulate('dragStart', { dataTransfer: { items: files } })
+ component.simulate('dragEnter', { dataTransfer: { items: files } })
+ component.simulate('dragOver', { dataTransfer: { items: files } })
+ component.simulate('dragLeave', { dataTransfer: { items: files } })
+ expect(dragStartSpy.callCount).toEqual(1)
+ expect(dragEnterSpy.callCount).toEqual(1)
+ expect(dragLeaveSpy.callCount).toEqual(1)
+ // It should not throw the error
+ expect(dragOverSpy).not.toThrow()
+ dragOverSpy.restore()
+ })
+
+ it('should set proper dragActive state on dragEnter', () => {
+ const dropzone = mount({props => } )
+ const child = dropzone.find(DummyChildComponent)
+ dropzone.simulate('dragEnter', { dataTransfer: { files } })
+ expect(child).toHaveProp('isDragActive', true)
+ expect(child).toHaveProp('isDragAccept', true)
+ expect(child).toHaveProp('isDragReject', false)
+ })
+
+ it('should set proper dragReject state on dragEnter', () => {
+ const dropzone = mount(
+ {props => }
+ )
+ const child = dropzone.find(DummyChildComponent)
+ dropzone.simulate('dragEnter', {
+ dataTransfer: { files: files.concat(images) }
+ })
+ expect(child).toHaveProp('isDragActive', true)
+ expect(child).toHaveProp('isDragAccept', false)
+ expect(child).toHaveProp('isDragReject', true)
+ })
+
+ it('should set proper dragAccept state if multiple is false', () => {
+ const dropzone = mount(
+
+ {props => }
+
+ )
+ const child = dropzone.find(DummyChildComponent)
+ dropzone.simulate('dragEnter', { dataTransfer: { files } })
+ expect(child).toHaveProp('isDragActive', true)
+ expect(child).toHaveProp('isDragAccept', false)
+ expect(child).toHaveProp('isDragReject', true)
+ })
+
+ it('should set proper dragAccept state if multiple is false', () => {
+ const dropzone = mount(
+
+ {props => }
+
+ )
+ const child = dropzone.find(DummyChildComponent)
+ dropzone.simulate('dragEnter', { dataTransfer: { files: images } })
+ expect(child).toHaveProp('isDragActive', true)
+ expect(child).toHaveProp('isDragAccept', true)
+ expect(child).toHaveProp('isDragReject', true)
+ })
+
+ it('should set activeClassName properly', () => {
+ const dropzone = mount(
+
+ {props => }
+
+ )
+ const child = dropzone.find(DummyChildComponent)
+ dropzone.simulate('dragEnter', { dataTransfer: { files: images } })
+ expect(child).toHaveProp('isDragActive', true)
+ expect(dropzone.hasClass('👏')).toBe(true)
+ })
+
+ it('should set rejectClassName properly', () => {
+ const dropzone = mount(
+
+ {props => }
+
+ )
+ const child = dropzone.find(DummyChildComponent)
+ dropzone.simulate('dragEnter', { dataTransfer: { files: images } })
+ expect(child).toHaveProp('isDragReject', true)
+ expect(dropzone.hasClass('👎')).toBe(true)
+ })
+
+ it('should set acceptClassName properly', () => {
+ const dropzone = mount(
+
+ {props => }
+
+ )
+ const child = dropzone.find(DummyChildComponent)
+ dropzone.simulate('dragEnter', { dataTransfer: { files: images } })
+ expect(child).toHaveProp('isDragAccept', true)
+ expect(dropzone.hasClass('👍')).toBe(true)
+ })
+
+ it('should set disabledClassName properly', () => {
+ const dropzone = mount(
+
+ {props => }
+
+ )
+ expect(dropzone.hasClass('🤐')).toBe(true)
+ })
+
+ it('should keep dragging active when leaving from arbitrary node', () => {
+ const arbitraryOverlay = mount(
)
+ const dropzone = mount({props => } )
+ dropzone.simulate('dragEnter', { dataTransfer: { files: images } })
+ dropzone.simulate('dragLeave', { target: arbitraryOverlay })
+ expect(dropzone.state('isDragActive')).toBe(true)
+ expect(dropzone.state('draggedFiles').length > 0).toBe(true)
+ })
+
+ it('should apply acceptStyle if multiple is false and single file', () => {
+ const dropzone = mount(
+
+ {props => }
+
+ )
+ dropzone.simulate('dragEnter', { dataTransfer: { files: [images[0]] } })
+ const mainDiv = dropzone.find('div').at(0)
+ expect(mainDiv).toHaveProp('style', acceptStyle)
+ })
+
+ it('should apply rejectStyle if multiple is false and single bad file type', () => {
+ const dropzone = mount(
+
+ {props => }
+
+ )
+ dropzone.simulate('dragEnter', { dataTransfer: { files: [files[0]] } })
+ const mainDiv = dropzone.find('div').at(0)
+ expect(mainDiv).toHaveProp('style', rejectStyle)
+ })
+
+ it('should apply acceptStyle + rejectStyle if multiple is false and multiple good file types', () => {
+ const dropzone = mount(
+
+ {props => }
+
+ )
+ dropzone.simulate('dragEnter', { dataTransfer: { files: images } })
+ const mainDiv = dropzone.find('div').at(0)
+ const expectedStyle = {
+ ...acceptStyle,
+ ...rejectStyle
+ }
+ expect(mainDiv).toHaveProp('style', expectedStyle)
+ })
+
+ it('should set proper dragActive state if accept prop changes mid-drag', () => {
+ const dropzone = mount(
+ {props => }
+ )
+ const child = dropzone.find(DummyChildComponent)
+ dropzone.simulate('dragEnter', { dataTransfer: { files: images } })
+ expect(child).toHaveProp('isDragActive', true)
+ expect(child).toHaveProp('isDragAccept', true)
+ expect(child).toHaveProp('isDragReject', false)
+
+ dropzone.setProps({ accept: 'text/*' })
+ expect(child).toHaveProp('isDragActive', true)
+ expect(child).toHaveProp('isDragAccept', false)
+ expect(child).toHaveProp('isDragReject', true)
+ })
+
+ it('should expose state to children', () => {
+ const dropzone = mount(
+
+ {({ isDragActive, isDragAccept, isDragReject }) => {
+ if (isDragReject) {
+ return `${isDragActive && 'Active but'} Reject`
+ }
+ if (isDragAccept) {
+ return `${isDragActive && 'Active and'} Accept`
+ }
+ return 'Empty'
+ }}
+
+ )
+ expect(dropzone.text()).toEqual('Empty')
+ dropzone.simulate('dragEnter', { dataTransfer: { files: images } })
+ expect(dropzone.text()).toEqual('Active and Accept')
+ dropzone.simulate('dragEnter', { dataTransfer: { files } })
+ expect(dropzone.text()).toEqual('Active but Reject')
+ })
+
+ it('should reset the dragAccept/dragReject state when leaving after a child goes away', () => {
+ const DragActiveComponent = () => Accept
+ const ChildComponent = () => Child component content
+ const dropzone = mount(
+
+ {({ isDragAccept, isDragReject }) => {
+ if (isDragReject) {
+ return 'Rejected'
+ }
+ if (isDragAccept) {
+ return
+ }
+ return
+ }}
+
+ )
+ const child = dropzone.find(ChildComponent)
+ child.simulate('dragEnter', { dataTransfer: { files } })
+ dropzone.simulate('dragEnter', { dataTransfer: { files } })
+ // make sure we handle any duplicate dragEnter events that the browser may send us
+ dropzone.simulate('dragEnter', { dataTransfer: { files } })
+ const dragActiveChild = dropzone.find(DragActiveComponent)
+ expect(dragActiveChild).toBePresent()
+ expect(dragActiveChild).toHaveProp('isDragAccept', true)
+ expect(dragActiveChild).toHaveProp('isDragReject', false)
+
+ dropzone.simulate('dragLeave', { dataTransfer: { files } })
+ expect(dropzone.find(DragActiveComponent)).toBeEmpty()
+ expect(child).toHaveProp('isDragAccept', false)
+ expect(child).toHaveProp('isDragReject', false)
+ })
+ })
+
+ describe('onDrop', () => {
+ let dropSpy
+ let dropAcceptedSpy
+ let dropRejectedSpy
+
+ beforeEach(() => {
+ dropSpy = spy()
+ dropAcceptedSpy = spy()
+ dropRejectedSpy = spy()
+ })
+
+ afterEach(() => {
+ dropSpy.reset()
+ dropAcceptedSpy.reset()
+ dropRejectedSpy.reset()
+ })
+
+ it('should reset the dragActive/dragReject state', () => {
+ const dropzone = mount(
+
+ {props => }
+
+ )
+ const child = dropzone.find(DummyChildComponent)
+ dropzone.simulate('dragEnter', { dataTransfer: { files } })
+ expect(child).toHaveProp('isDragActive', true)
+ expect(child).toHaveProp('isDragReject', false)
+ dropzone.simulate('drop', { dataTransfer: { files } })
+ expect(child).toHaveProp('isDragActive', false)
+ expect(child).toHaveProp('isDragReject', false)
+ })
+
+ it('should add valid files to rejected files on a multple drop when multiple false', () => {
+ const dropzone = mount( )
+ dropzone.simulate('drop', { dataTransfer: { files: images } })
+ const rejected = dropSpy.firstCall.args[0]
+ expect(rejected.length).toEqual(1)
+ })
+
+ it('should add invalid files to rejected when multiple is false', () => {
+ const dropzone = mount( )
+ dropzone.simulate('drop', {
+ dataTransfer: { files: images.concat(files) }
+ })
+ const rejected = dropSpy.firstCall.args[1]
+ expect(rejected.length).toEqual(2)
+ })
+
+ it('should allow single files to be dropped if multiple is false', () => {
+ const dropzone = mount( )
+
+ dropzone.simulate('drop', { dataTransfer: { files: [images[0]] } })
+ const [accepted, rejected] = dropSpy.firstCall.args
+ expect(accepted.length).toEqual(1)
+ expect(rejected.length).toEqual(0)
+ })
+
+ it('should take all dropped files if multiple is true', () => {
+ const dropzone = mount( )
+ dropzone.simulate('drop', { dataTransfer: { files: images } })
+ expect(dropSpy.firstCall.args[0]).toHaveLength(2)
+ expect(dropSpy.firstCall.args[0][0].name).toEqual(images[0].name)
+ expect(dropSpy.firstCall.args[0][1].name).toEqual(images[1].name)
+ })
+
+ it('should set this.isFileDialogActive to false', () => {
+ const dropzone = mount( )
+ dropzone.instance().isFileDialogActive = true
+ dropzone.simulate('drop', { dataTransfer: { files } })
+ expect(dropzone.instance().isFileDialogActive).toEqual(false)
+ })
+
+ it('should always call onDrop callback with accepted and rejected arguments', () => {
+ const dropzone = mount(
+
+ )
+ dropzone.simulate('drop', { dataTransfer: { files } })
+ expect(dropSpy.callCount).toEqual(1)
+ expect(dropSpy.firstCall.args[0]).toEqual([], [...files])
+ dropzone.simulate('drop', { dataTransfer: { files: images } })
+ expect(dropSpy.callCount).toEqual(2)
+ expect(dropSpy.lastCall.args[0]).toEqual([...images], [])
+ dropzone.simulate('drop', {
+ dataTransfer: { files: files.concat(images) }
+ })
+ expect(dropSpy.callCount).toEqual(3)
+ expect(dropSpy.lastCall.args[0]).toEqual([...images], [...files])
+ })
+
+ it('should call onDropAccepted callback if some files were accepted', () => {
+ const dropzone = mount(
+
+ )
+ dropzone.simulate('drop', { dataTransfer: { files } })
+ expect(dropAcceptedSpy.callCount).toEqual(0)
+ dropzone.simulate('drop', { dataTransfer: { files: images } })
+ expect(dropAcceptedSpy.callCount).toEqual(1)
+ expect(dropAcceptedSpy.lastCall.args[0]).toEqual([...images])
+ dropzone.simulate('drop', {
+ dataTransfer: { files: files.concat(images) }
+ })
+ expect(dropAcceptedSpy.callCount).toEqual(2)
+ expect(dropAcceptedSpy.lastCall.args[0]).toEqual([...images])
+ })
+
+ it('should call onDropRejected callback if some files were rejected', () => {
+ const dropzone = mount(
+
+ )
+ dropzone.simulate('drop', { dataTransfer: { files } })
+ expect(dropRejectedSpy.callCount).toEqual(1)
+ expect(dropRejectedSpy.lastCall.args[0]).toEqual([...files])
+ dropzone.simulate('drop', { dataTransfer: { files: images } })
+ expect(dropRejectedSpy.callCount).toEqual(1)
+ dropzone.simulate('drop', {
+ dataTransfer: { files: files.concat(images) }
+ })
+ expect(dropRejectedSpy.callCount).toEqual(2)
+ expect(dropRejectedSpy.lastCall.args[0]).toEqual([...files])
+ })
+
+ it('applies the accept prop to the dropped files', () => {
+ const dropzone = mount(
+
+ )
+ dropzone.simulate('drop', { dataTransfer: { files } })
+ expect(dropSpy.callCount).toEqual(1)
+ expect(dropSpy.firstCall.args[0]).toHaveLength(0)
+ expect(dropSpy.firstCall.args[1]).toHaveLength(1)
+ expect(dropAcceptedSpy.callCount).toEqual(0)
+ expect(dropRejectedSpy.callCount).toEqual(1)
+ expect(dropRejectedSpy.firstCall.args[0]).toHaveLength(1)
+ })
+
+ it('applies the accept prop to the dropped images', () => {
+ const dropzone = mount(
+
+ )
+
+ dropzone.simulate('drop', { dataTransfer: { files: images } })
+ expect(dropSpy.callCount).toEqual(1)
+ expect(dropSpy.firstCall.args[0]).toHaveLength(2)
+ expect(dropSpy.firstCall.args[1]).toHaveLength(0)
+ expect(dropAcceptedSpy.callCount).toEqual(1)
+ expect(dropAcceptedSpy.firstCall.args[0]).toHaveLength(2)
+ expect(dropRejectedSpy.callCount).toEqual(0)
+ })
+
+ it('accepts a dropped image when Firefox provides a bogus file type', () => {
+ const dropzone = mount(
+
+ )
+ const bogusImages = [
+ {
+ name: 'bogus.gif',
+ size: 1234,
+ type: 'application/x-moz-file'
+ }
+ ]
+
+ dropzone.simulate('drop', { dataTransfer: { files: bogusImages } })
+ expect(dropSpy.callCount).toEqual(1)
+ expect(dropSpy.firstCall.args[0]).toHaveLength(1)
+ expect(dropSpy.firstCall.args[1]).toHaveLength(0)
+ expect(dropAcceptedSpy.callCount).toEqual(1)
+ expect(dropAcceptedSpy.firstCall.args[0]).toHaveLength(1)
+ expect(dropRejectedSpy.callCount).toEqual(0)
+ })
+
+ it('accepts all dropped files and images when no accept prop is specified', () => {
+ const dropzone = mount(
+
+ )
+ dropzone.simulate('drop', {
+ dataTransfer: { files: files.concat(images) }
+ })
+ expect(dropSpy.callCount).toEqual(1)
+ expect(dropSpy.firstCall.args[0]).toHaveLength(3)
+ expect(dropSpy.firstCall.args[1]).toHaveLength(0)
+ expect(dropAcceptedSpy.callCount).toEqual(1)
+ expect(dropAcceptedSpy.firstCall.args[0]).toHaveLength(3)
+ expect(dropRejectedSpy.callCount).toEqual(0)
+ })
+
+ it('applies the maxSize prop to the dropped files', () => {
+ const dropzone = mount(
+
+ )
+
+ dropzone.simulate('drop', { dataTransfer: { files } })
+ expect(dropSpy.callCount).toEqual(1)
+ expect(dropSpy.firstCall.args[0]).toHaveLength(1)
+ expect(dropSpy.firstCall.args[1]).toHaveLength(0)
+ expect(dropAcceptedSpy.callCount).toEqual(1)
+ expect(dropAcceptedSpy.firstCall.args[0]).toHaveLength(1)
+ expect(dropRejectedSpy.callCount).toEqual(0)
+ })
+
+ it('applies the maxSize prop to the dropped images', () => {
+ const dropzone = mount(
+
+ )
+ dropzone.simulate('drop', { dataTransfer: { files: images } })
+ expect(dropSpy.callCount).toEqual(1)
+ expect(dropSpy.firstCall.args[0]).toHaveLength(0)
+ expect(dropSpy.firstCall.args[1]).toHaveLength(2)
+ expect(dropAcceptedSpy.callCount).toEqual(0)
+ expect(dropRejectedSpy.callCount).toEqual(1)
+ expect(dropRejectedSpy.firstCall.args[0]).toHaveLength(2)
+ })
+
+ it('applies the minSize prop to the dropped files', () => {
+ const dropzone = mount(
+
+ )
+ dropzone.simulate('drop', { dataTransfer: { files } })
+ expect(dropSpy.callCount).toEqual(1)
+ expect(dropSpy.firstCall.args[0]).toHaveLength(0)
+ expect(dropSpy.firstCall.args[1]).toHaveLength(1)
+ expect(dropAcceptedSpy.callCount).toEqual(0)
+ expect(dropRejectedSpy.callCount).toEqual(1)
+ expect(dropRejectedSpy.firstCall.args[0]).toHaveLength(1)
+ })
+
+ it('applies the minSize prop to the dropped images', () => {
+ const dropzone = mount(
+
+ )
+ dropzone.simulate('drop', { dataTransfer: { files: images } })
+ expect(dropSpy.callCount).toEqual(1)
+ expect(dropSpy.firstCall.args[0]).toHaveLength(2)
+ expect(dropSpy.firstCall.args[1]).toHaveLength(0)
+ expect(dropAcceptedSpy.callCount).toEqual(1)
+ expect(dropAcceptedSpy.firstCall.args[0]).toHaveLength(2)
+ expect(dropRejectedSpy.callCount).toEqual(0)
+ })
+
+ it('accepts all dropped files and images when no size prop is specified', () => {
+ const dropzone = mount(
+
+ )
+ dropzone.simulate('drop', {
+ dataTransfer: { files: files.concat(images) }
+ })
+ expect(dropSpy.callCount).toEqual(1)
+ expect(dropSpy.firstCall.args[0]).toHaveLength(3)
+ expect(dropSpy.firstCall.args[1]).toHaveLength(0)
+ expect(dropAcceptedSpy.callCount).toEqual(1)
+ expect(dropAcceptedSpy.firstCall.args[0]).toHaveLength(3)
+ expect(dropRejectedSpy.callCount).toEqual(0)
+ })
+ })
+
+ describe('preview', () => {
+ it('should generate previews for non-images', () => {
+ const dropSpy = spy()
+ const dropzone = mount( )
+ dropzone.simulate('drop', { dataTransfer: { files } })
+ expect(Object.keys(dropSpy.firstCall.args[0][0])).toContain('preview')
+ expect(dropSpy.firstCall.args[0][0].preview).toContain('data://file1.pdf')
+ })
+
+ it('should generate previews for images', () => {
+ const dropSpy = spy()
+ const dropzone = mount( )
+ dropzone.simulate('drop', { dataTransfer: { files: images } })
+ expect(Object.keys(dropSpy.firstCall.args[0][0])).toContain('preview')
+ expect(dropSpy.firstCall.args[0][0].preview).toContain('data://cats.gif')
+ })
+
+ it('should not throw error when preview cannot be created', () => {
+ const dropSpy = spy()
+ const dropzone = mount( )
+
+ dropzone.simulate('drop', { dataTransfer: { files: ['bad_val'] } })
+
+ expect(Object.keys(dropSpy.firstCall.args[1][0])).not.toContain('preview')
+ })
+
+ it('should not generate previews if disablePreview is true', () => {
+ const dropSpy = spy()
+ const dropzone = mount( )
+ dropzone.simulate('drop', { dataTransfer: { files: images } })
+ dropzone.simulate('drop', { dataTransfer: { files } })
+ expect(dropSpy.callCount).toEqual(2)
+ expect(Object.keys(dropSpy.firstCall.args[0][0])).not.toContain('preview')
+ expect(Object.keys(dropSpy.lastCall.args[0][0])).not.toContain('preview')
+ })
+ })
+
+ describe('onClick', () => {})
+
+ describe('onCancel', () => {
+ it('should not invoke onFileDialogCancel everytime window receives focus', done => {
+ const onCancelSpy = spy()
+ mount( )
+ // Simulated DOM event - onfocus
+ document.body.addEventListener('focus', () => {})
+ const evt = document.createEvent('HTMLEvents')
+ evt.initEvent('focus', false, true)
+ document.body.dispatchEvent(evt)
+ // setTimeout to match the event callback from actual Component
+ setTimeout(() => {
+ expect(onCancelSpy.callCount).toEqual(0)
+ done()
+ }, 300)
+ })
+
+ it('should invoke onFileDialogCancel when window receives focus via cancel button', done => {
+ const onCancelSpy = spy()
+ const component = mount(
+
+ )
+
+ // Test / invoke the click event
+ spy(component.instance(), 'open')
+ component.simulate('click')
+
+ setTimeout(() => {
+ expect(component.instance().open.callCount).toEqual(1)
+
+ // Simulated DOM event - onfocus
+ document.body.addEventListener('focus', () => {})
+ const evt = document.createEvent('HTMLEvents')
+ evt.initEvent('focus', false, true)
+ document.body.dispatchEvent(evt)
+
+ // setTimeout to match the event callback from actual Component
+ setTimeout(() => {
+ expect(onCancelSpy.callCount).toEqual(1)
+ done()
+ }, 300)
+ }, 0)
+ })
+ })
+
+ describe('nested Dropzone component behavior', () => {
+ let outerDropzone
+ let innerDropzone
+ let outerDropSpy
+ let outerDropAcceptedSpy
+ let outerDropRejectedSpy
+ let innerDropSpy
+ let innerDropAcceptedSpy
+ let innerDropRejectedSpy
+
+ const InnerDragAccepted = () => Accepted
+ const InnerDragRejected = () => Rejected
+ const InnerDropzone = () => (
+
+ {({ isDragActive, isDragReject }) => {
+ if (isDragReject) return
+ if (isDragActive) return
+ return No drag
+ }}
+
+ )
+
+ describe('dropping on the inner dropzone', () => {
+ it('mounts both dropzones', () => {
+ outerDropSpy = spy()
+ outerDropAcceptedSpy = spy()
+ outerDropRejectedSpy = spy()
+ innerDropSpy = spy()
+ innerDropAcceptedSpy = spy()
+ innerDropRejectedSpy = spy()
+ outerDropzone = mount(
+
+ {props => }
+
+ )
+ innerDropzone = outerDropzone.find(InnerDropzone)
+ })
+
+ it('does dragEnter on both dropzones', () => {
+ innerDropzone.simulate('dragEnter', {
+ dataTransfer: { files: images }
+ })
+ expect(innerDropzone).toHaveProp('isDragActive', true)
+ expect(innerDropzone).toHaveProp('isDragReject', false)
+ expect(innerDropzone.find(InnerDragAccepted).exists()).toEqual(true)
+ expect(innerDropzone.find(InnerDragRejected).exists()).toEqual(false)
+ })
+
+ it('drops on the child dropzone', () => {
+ innerDropzone.simulate('drop', {
+ dataTransfer: { files: files.concat(images) }
+ })
+ })
+
+ it('accepts the drop on the inner dropzone', () => {
+ expect(innerDropSpy.callCount).toEqual(1)
+ expect(innerDropSpy.firstCall.args[0]).toHaveLength(2)
+ expect(innerDropSpy.firstCall.args[1]).toHaveLength(1)
+ expect(innerDropAcceptedSpy.callCount).toEqual(1)
+ expect(innerDropAcceptedSpy.firstCall.args[0]).toHaveLength(2)
+ expect(innerDropRejectedSpy.callCount).toEqual(1)
+ expect(innerDropRejectedSpy.firstCall.args[0]).toHaveLength(1)
+ expect(innerDropzone.find(InnerDragAccepted).exists()).toEqual(false)
+ expect(innerDropzone.find(InnerDragRejected).exists()).toEqual(false)
+ })
+
+ it('also accepts the drop on the outer dropzone', () => {
+ expect(outerDropSpy.callCount).toEqual(1)
+ expect(outerDropSpy.firstCall.args[0]).toHaveLength(2)
+ expect(outerDropSpy.firstCall.args[1]).toHaveLength(1)
+ expect(outerDropAcceptedSpy.callCount).toEqual(1)
+ expect(outerDropAcceptedSpy.firstCall.args[0]).toHaveLength(2)
+ expect(outerDropRejectedSpy.callCount).toEqual(1)
+ expect(outerDropRejectedSpy.firstCall.args[0]).toHaveLength(1)
+ expect(innerDropzone).toHaveProp('isDragActive', false)
+ expect(innerDropzone).toHaveProp('isDragReject', false)
+ })
+ })
+ })
+
+ describe('behavior', () => {
+ it('does not throw an error when html is dropped instead of files and multiple is false', () => {
+ const dropzone = mount( )
+
+ const fn = () => dropzone.simulate('drop', { dataTransfer: { files: [] } })
+ expect(fn).not.toThrow()
+ })
+
+ it('does not allow actions when disabled props is true', done => {
+ const dropzone = mount( )
+
+ spy(dropzone.instance(), 'open')
+ dropzone.simulate('click')
+ setTimeout(() => {
+ expect(dropzone.instance().open.callCount).toEqual(0)
+ done()
+ }, 0)
+ })
+
+ it('when toggle disabled props, Dropzone works as expected', done => {
+ const dropzone = mount( )
+ spy(dropzone.instance(), 'open')
+
+ dropzone.setProps({ disabled: false })
+
+ dropzone.simulate('click')
+ setTimeout(() => {
+ expect(dropzone.instance().open.callCount).toEqual(1)
+ done()
+ }, 0)
+ })
+ })
+})
diff --git a/goTorrentWebUI/node_modules/react-dropzone/src/utils/index.js b/goTorrentWebUI/node_modules/react-dropzone/src/utils/index.js
new file mode 100644
index 00000000..eb265bae
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/src/utils/index.js
@@ -0,0 +1,43 @@
+import accepts from 'attr-accept'
+
+export const supportMultiple =
+ typeof document !== 'undefined' && document && document.createElement
+ ? 'multiple' in document.createElement('input')
+ : true
+
+export function getDataTransferItems(event) {
+ let dataTransferItemsList = []
+ if (event.dataTransfer) {
+ const dt = event.dataTransfer
+ if (dt.files && dt.files.length) {
+ dataTransferItemsList = dt.files
+ } else if (dt.items && dt.items.length) {
+ // During the drag even the dataTransfer.files is null
+ // but Chrome implements some drag store, which is accesible via dataTransfer.items
+ dataTransferItemsList = dt.items
+ }
+ } else if (event.target && event.target.files) {
+ dataTransferItemsList = event.target.files
+ }
+ // Convert from DataTransferItemsList to the native Array
+ return Array.prototype.slice.call(dataTransferItemsList)
+}
+
+// Firefox versions prior to 53 return a bogus MIME type for every file drag, so dragovers with
+// that MIME type will always be accepted
+export function fileAccepted(file, accept) {
+ return file.type === 'application/x-moz-file' || accepts(file, accept)
+}
+
+export function fileMatchSize(file, maxSize, minSize) {
+ return file.size <= maxSize && file.size >= minSize
+}
+
+export function allFilesAccepted(files, accept) {
+ return files.every(file => fileAccepted(file, accept))
+}
+
+// allow the entire document to be a drag target
+export function onDocumentDragOver(evt) {
+ evt.preventDefault()
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/src/utils/index.spec.js b/goTorrentWebUI/node_modules/react-dropzone/src/utils/index.spec.js
new file mode 100644
index 00000000..5220828e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/src/utils/index.spec.js
@@ -0,0 +1,89 @@
+import { getDataTransferItems } from './'
+
+const files = [
+ {
+ name: 'file1.pdf',
+ size: 1111,
+ type: 'application/pdf'
+ },
+ {
+ name: 'cats.gif',
+ size: 1234,
+ type: 'image/gif'
+ },
+ {
+ name: 'dogs.jpg',
+ size: 2345,
+ type: 'image/jpeg'
+ }
+]
+
+describe('getDataTransferItems', () => {
+ it('should return an array', () => {
+ const res = getDataTransferItems({})
+ expect(res).toBeInstanceOf(Array)
+ expect(res).toHaveLength(0)
+ })
+
+ it('should get dataTransfer before using target', () => {
+ const event = {
+ target: {
+ files
+ },
+ dataTransfer: {
+ files
+ }
+ }
+ const res = getDataTransferItems(event)
+ expect(res).toBeInstanceOf(Array)
+ expect(res).toHaveLength(3)
+ })
+
+ it('should use dataTransfer.items if files is not defined', () => {
+ const event = {
+ target: {
+ files: [{}]
+ },
+ dataTransfer: {
+ items: files
+ }
+ }
+ const res = getDataTransferItems(event)
+ expect(res).toBeInstanceOf(Array)
+ expect(res).toHaveLength(3)
+ })
+
+ it('should use event.target if dataTransfer is not defined', () => {
+ const event = {
+ target: {
+ files
+ }
+ }
+ const res = getDataTransferItems(event)
+ expect(res).toBeInstanceOf(Array)
+ expect(res).toHaveLength(3)
+ })
+
+ it('should prioritize dataTransfer.files over .files', () => {
+ const event = {
+ dataTransfer: {
+ files: [{}, {}],
+ items: [{}, {}, {}]
+ }
+ }
+ const res = getDataTransferItems(event)
+ expect(res).toBeInstanceOf(Array)
+ expect(res).toHaveLength(2)
+ })
+
+ it('should not mutate data', () => {
+ const event = {
+ dataTransfer: {
+ files
+ }
+ }
+ expect(Object.keys(files[2])).toHaveLength(3)
+ getDataTransferItems(event, true)
+ expect(Object.keys(files[2])).toHaveLength(3)
+ })
+})
diff --git a/goTorrentWebUI/node_modules/react-dropzone/src/utils/styles.js b/goTorrentWebUI/node_modules/react-dropzone/src/utils/styles.js
new file mode 100644
index 00000000..0b81fb4b
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/src/utils/styles.js
@@ -0,0 +1,23 @@
+export default {
+ rejected: {
+ borderStyle: 'solid',
+ borderColor: '#c66',
+ backgroundColor: '#eee'
+ },
+ disabled: {
+ opacity: 0.5
+ },
+ active: {
+ borderStyle: 'solid',
+ borderColor: '#6c6',
+ backgroundColor: '#eee'
+ },
+ default: {
+ width: 200,
+ height: 200,
+ borderWidth: 2,
+ borderColor: '#666',
+ borderStyle: 'dashed',
+ borderRadius: 5
+ }
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/styleguide.config.js b/goTorrentWebUI/node_modules/react-dropzone/styleguide.config.js
new file mode 100644
index 00000000..1d1bbee5
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/styleguide.config.js
@@ -0,0 +1,47 @@
+const path = require('path')
+
+module.exports = {
+ title: 'react-dropzone',
+ styleguideDir: path.join(__dirname, 'styleguide'),
+ showCode: true,
+ showUsage: true,
+ showSidebar: false,
+ serverPort: 8080,
+ sections: [
+ {
+ content: 'README.md'
+ },
+ {
+ name: 'PropTypes',
+ components: './src/index.js'
+ },
+ {
+ name: 'Examples',
+ context: {
+ Dropzone: './src/index'
+ },
+ sections: [
+ {
+ name: 'Basic example',
+ content: 'examples/Basic/Readme.md'
+ },
+ {
+ name: 'Styling Dropzone',
+ content: 'examples/Styling/Readme.md'
+ },
+ {
+ name: 'Accepting specific file types',
+ content: 'examples/Accept/Readme.md'
+ },
+ {
+ name: 'Opening File Dialog Programmatically',
+ content: 'examples/File Dialog/Readme.md'
+ },
+ {
+ name: 'Full Screen Dropzone',
+ content: 'examples/Fullscreen/Readme.md'
+ }
+ ]
+ }
+ ]
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/testSetup.js b/goTorrentWebUI/node_modules/react-dropzone/testSetup.js
new file mode 100644
index 00000000..dcd9022e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/testSetup.js
@@ -0,0 +1,8 @@
+/* eslint prefer-template: 0 */
+require('jest-enzyme')
+
+global.window.URL = {
+ createObjectURL: function createObjectURL(arg) {
+ return 'data://' + arg.name
+ }
+}
diff --git a/goTorrentWebUI/node_modules/react-dropzone/wallaby.config.js b/goTorrentWebUI/node_modules/react-dropzone/wallaby.config.js
new file mode 100644
index 00000000..a2e96548
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/wallaby.config.js
@@ -0,0 +1,17 @@
+/* eslint global-require: 0 */
+/* eslint import/no-extraneous-dependencies: 0 */
+module.exports = wallaby => ({
+ files: ['package.json', 'src/**/*.js', '!src/**/*.spec.js'],
+ tests: ['src/**/*.spec.js'],
+ compilers: {
+ 'src/**/*.js': wallaby.compilers.babel()
+ },
+ env: {
+ type: 'node',
+ runner: 'node'
+ },
+ testFramework: 'jest',
+ setup: () => {
+ wallaby.testFramework.configure(require('./package.json').jest)
+ }
+})
diff --git a/goTorrentWebUI/node_modules/react-dropzone/webpack.config.js b/goTorrentWebUI/node_modules/react-dropzone/webpack.config.js
new file mode 100644
index 00000000..2eaddb4e
--- /dev/null
+++ b/goTorrentWebUI/node_modules/react-dropzone/webpack.config.js
@@ -0,0 +1,30 @@
+const path = require('path')
+
+module.exports = {
+ entry: './src/index.js',
+ devtool: 'source-map',
+ output: {
+ path: path.resolve(__dirname, 'dist'),
+ filename: 'index.js',
+ libraryTarget: 'umd',
+ library: 'Dropzone'
+ },
+ module: {
+ loaders: [
+ {
+ include: [path.resolve(__dirname, 'src'), path.resolve(__dirname, 'examples')],
+ test: /\.js$/,
+ loader: 'babel-loader'
+ }
+ ]
+ },
+ resolve: {
+ // Can require('file') instead of require('file.js') etc.
+ extensions: ['.js', '.json']
+ },
+ externals: {
+ react: 'react',
+ 'prop-types': 'prop-types'
+ },
+ plugins: []
+}
diff --git a/goTorrentWebUI/src/TopMenu/Modals/addTorrentFileModal.js b/goTorrentWebUI/src/TopMenu/Modals/addTorrentFileModal.js
index e4438ba9..597c01a2 100644
--- a/goTorrentWebUI/src/TopMenu/Modals/addTorrentFileModal.js
+++ b/goTorrentWebUI/src/TopMenu/Modals/addTorrentFileModal.js
@@ -16,7 +16,7 @@ import ReactTooltip from 'react-tooltip'
import AddIcon from 'material-ui-icons/AddBox';
import IconButton from 'material-ui/IconButton';
-import Upload from 'material-ui-upload/Upload';
+import Dropzone from 'react-dropzone';
const button = {
fontSize: '60px',
@@ -43,8 +43,9 @@ export default class addTorrentFilePopup extends React.Component {
state = {
open: false,
torrentFileName: "",
- torrentFileValue: new File([""], "tempName"),
+ torrentFileValue: [],
storageValue: "",
+ showDrop: true,
};
handleClickOpen = () => {
@@ -58,26 +59,31 @@ export default class addTorrentFilePopup extends React.Component {
handleSubmit = () => {
this.setState({ open: false });
//let magnetLinkSubmit = this.state.textValue;
- this.setState({torrentFileValue: event.target.value});
+ console.log("File", this.state.torrentFileValue)
const reader = new FileReader()
- let torrentFileBlob = Blob(this.state.torrentFileValue)
- let base64file = reader.readAsDataURL(torrentFileBlob)
+ let torrentFileBlob = new Blob(this.state.torrentFileValue)
+ console.log("Blob", torrentFileBlob)
+ reader.readAsDataURL(torrentFileBlob)
+ reader.onloadend = () => {
+ let base64data = reader.result;
+ console.log("Base64", base64data)
- let torrentFileMessage = {
- messageType: "torrentFileSubmit",
- messageDetail: this.state.torrentFileName,
- messageDetailTwo: this.state.storageValue,
- Payload: [base64file],
- }
- console.log("Sending magnet link: ", torrentFileMessage);
- ws.send(JSON.stringify(torrentFileMessage));
+ let torrentFileMessage = {
+ messageType: "torrentFileSubmit",
+ messageDetail: this.state.torrentFileName,
+ messageDetailTwo: this.state.storageValue,
+ Payload: [base64data],
+ }
+ console.log("Sending magnet link: ", torrentFileMessage);
+ ws.send(JSON.stringify(torrentFileMessage));
+ }
}
- onFileLoad = (event, file) => {
- this.setState({torrentFileName: file.name})
- this.setState({torrentFileValue: File(file, file.name)})
-
- console.log(event.target.result, file.name);
+ onFileLoad = (file) => {
+ this.setState({torrentFileName: file[0].name})
+ this.setState({showDrop: false})
+ this.setState({torrentFileValue: file})
+ console.log("File Name", file[0].name)
}
@@ -98,9 +104,16 @@ export default class addTorrentFilePopup extends React.Component {
Add Torrent File
- Upload Torrent Here and Add Storage Path
+
-
+ {this.state.showDrop &&
+
+ Upload Torrent Here and Add Storage Path
+
+ }
+ {this.state.torrentFileName != "" &&
+ this.state.torrentFileName
+ }
diff --git a/main.go b/main.go
index 47bcb351..d17f6006 100644
--- a/main.go
+++ b/main.go
@@ -5,11 +5,11 @@ import (
"encoding/json"
"fmt"
"html/template"
- "io"
"io/ioutil"
"net/http"
"os"
"path/filepath"
+ "strings"
"github.com/anacrolix/torrent"
"github.com/asdine/storm"
@@ -57,6 +57,7 @@ func main() {
Storage.Logger = Logger
Config := Engine.FullClientSettingsNew() //grabbing from settings.go
if Config.LoggingOutput == "file" {
+ os.Remove("logs/server.log") //cleanup the old log on every restart
file, err := os.OpenFile("logs/server.log", os.O_CREATE|os.O_WRONLY, 0666) //creating the log file
if err != nil {
fmt.Println("Unable to create file for logging.... please check permissions.. forcing output to stdout")
@@ -107,36 +108,6 @@ func main() {
router.HandleFunc("/", serveHome) //Serving the main page for our SPA
http.Handle("/static/", http.FileServer(http.Dir("public")))
http.Handle("/", router)
- http.HandleFunc("/uploadTorrent", func(w http.ResponseWriter, r *http.Request) { //grabbing the uploaded Torrent File and adding it to the client TODO figure out websocket
- defer http.Redirect(w, r, "/", 301) //forcing redirect to home page after file is processed
- err := r.ParseMultipartForm(32)
- if err != nil {
- fmt.Println("Error parsing forme", err)
- }
-
- storageFilePath := r.Form["storagePath"]
- fmt.Println("Storage Path", storageFilePath)
- file, header, err := r.FormFile("fileTorrent")
- if err != nil {
- Logger.WithFields(logrus.Fields{"error": err, "file": file}).Error("Error with fetching file or request issue")
- }
-
- defer file.Close() //defer closing the file until we are done manipulating it
- var filePath = filepath.Join(Config.TFileUploadFolder, header.Filename) //creating a full filepath to store the .torrent files
- fileName, err := os.OpenFile(filePath, os.O_WRONLY|os.O_CREATE, 0666) //generating the fileName
- if err != nil {
- panic(err)
- }
- io.Copy(fileName, file) //Dumping our recieved file into the filename
- clientTorrent, err := tclient.AddTorrentFromFile(fileName.Name()) //Adding the torrent into the client
- if err != nil {
- Logger.WithFields(logrus.Fields{"error": err, "file Name": fileName.Name()}).Error("Error adding Torrent from file")
- } else {
- Logger.WithFields(logrus.Fields{"file Name": fileName.Name()}).Info("Adding Torrent via file")
- Engine.StartTorrent(clientTorrent, torrentLocalStorage, db, Config.TorrentConfig.DataDir, "file", fileName.Name(), storageFilePath[0]) // the starttorrent can take a LONG time on startup
- }
-
- })
http.HandleFunc("/api", func(w http.ResponseWriter, r *http.Request) { //exposing the data to the
TorrentLocalArray = Storage.FetchAllStoredTorrents(db)
RunningTorrentArray = Engine.CreateRunningTorrentArray(tclient, TorrentLocalArray, PreviousTorrentArray, Config, db) //Updates the RunningTorrentArray with the current client data as well
@@ -174,6 +145,7 @@ func main() {
TorrentLocalArray = Storage.FetchAllStoredTorrents(db) //Required to re-read th database since we write to the DB and this will pull the changes from it
RunningTorrentArray = Engine.CreateRunningTorrentArray(tclient, TorrentLocalArray, PreviousTorrentArray, Config, db) //Updates the RunningTorrentArray with the current client data as well
PreviousTorrentArray = RunningTorrentArray
+ //Logger.WithFields(logrus.Fields{"message": msg}).Infof("%+v\n", RunningTorrentArray)
var torrentlistArray = new(Engine.TorrentList)
torrentlistArray.MessageType = "torrentList"
torrentlistArray.ClientDBstruct = RunningTorrentArray
@@ -267,20 +239,28 @@ func main() {
}
case "torrentFileSubmit":
- file, err := base64.StdEncoding.DecodeString(msg.Payload[0]) //decoding the base64 encoded file sent from client
+ base64file := strings.TrimPrefix(msg.Payload[0], "data:;base64,") //trimming off the unneeded bits from the front of the json //TODO maybe do this in client?
+ file, err := base64.StdEncoding.DecodeString(base64file) //decoding the base64 encoded file sent from client
if err != nil {
- Logger.WithFields(logrus.Fields{"Error": err}).Info("Unable to decode base64 string to file")
+ Logger.WithFields(logrus.Fields{"Error": err, "file": file}).Info("Unable to decode base64 string to file")
}
- desiredFileName := msg.MessageDetail
+ FileName := msg.MessageDetail
storageValue := msg.MessageDetailTwo
- filePath := filepath.Join(Config.TFileUploadFolder, desiredFileName) //creating a full filepath to store the .torrent files
+ if storageValue == "" {
+ storageValue = Config.DefaultMoveFolder
+ }
+ filePath := filepath.Join(Config.TFileUploadFolder, FileName) //creating a full filepath to store the .torrent files
err = ioutil.WriteFile(filePath, file, 0644) //Dumping our recieved file into the filename
if err != nil {
Logger.WithFields(logrus.Fields{"filepath": filePath, "Error": err}).Error("Unable to write torrent data to file")
}
- clientTorrent, err := tclient.AddTorrentFromFile(filePath)
+ clientTorrent, err := tclient.AddTorrentFromFile(filePath)
+ if err != nil {
+ Logger.WithFields(logrus.Fields{"filepath": filePath, "Error": err}).Error("Unable to add Torrent to torrent server")
+ }
+ Logger.WithFields(logrus.Fields{"clienttorrent": clientTorrent.Name(), "filename": filePath}).Info("Added torrent")
Engine.StartTorrent(clientTorrent, torrentLocalStorage, db, Config.TorrentConfig.DataDir, "file", filePath, storageValue)
case "stopTorrents":
@@ -307,10 +287,11 @@ func main() {
for _, singleTorrent := range runningTorrents {
for _, singleSelection := range msg.Payload {
if singleTorrent.InfoHash().String() == singleSelection {
+ singleTorrent.Drop()
Logger.WithFields(logrus.Fields{"selection": singleSelection}).Info("Matched for deleting torrents")
if withData == "true" {
Logger.WithFields(logrus.Fields{"selection": singleSelection}).Info("Deleting torrent and data")
- Storage.DelTorrentLocalStorageAndFiles(db, singleTorrent.InfoHash().String())
+ Storage.DelTorrentLocalStorageAndFiles(db, singleTorrent.InfoHash().String(), Config.TorrentConfig.DataDir)
} else {
Logger.WithFields(logrus.Fields{"selection": singleSelection}).Info("Deleting just the torrent")
Storage.DelTorrentLocalStorage(db, singleTorrent.InfoHash().String())
diff --git a/public/static/js/bundle.js b/public/static/js/bundle.js
index a069a5d3..356d9e80 100644
--- a/public/static/js/bundle.js
+++ b/public/static/js/bundle.js
@@ -60,7 +60,7 @@
/******/ __webpack_require__.p = "";
/******/
/******/ // Load entry module and return exports
-/******/ return __webpack_require__(__webpack_require__.s = 453);
+/******/ return __webpack_require__(__webpack_require__.s = 315);
/******/ })
/************************************************************************/
/******/ ([
@@ -89,11 +89,11 @@ if (process.env.NODE_ENV !== 'production') {
// By explicitly using `prop-types` you are opting into new development behavior.
// http://fb.me/prop-types-in-prod
var throwOnDirectAccess = true;
- module.exports = __webpack_require__(582)(isValidElement, throwOnDirectAccess);
+ module.exports = __webpack_require__(444)(isValidElement, throwOnDirectAccess);
} else {
// By explicitly using `prop-types` you are opting into new production behavior.
// http://fb.me/prop-types-in-prod
- module.exports = __webpack_require__(585)();
+ module.exports = __webpack_require__(447)();
}
/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
@@ -106,9 +106,9 @@ if (process.env.NODE_ENV !== 'production') {
/* WEBPACK VAR INJECTION */(function(process) {
if (process.env.NODE_ENV === 'production') {
- module.exports = __webpack_require__(454);
+ module.exports = __webpack_require__(316);
} else {
- module.exports = __webpack_require__(455);
+ module.exports = __webpack_require__(317);
}
/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
@@ -312,7 +312,7 @@ process.umask = function() { return 0; };
exports.__esModule = true;
-var _assign = __webpack_require__(296);
+var _assign = __webpack_require__(205);
var _assign2 = _interopRequireDefault(_assign);
@@ -365,7 +365,7 @@ Object.defineProperty(exports, "__esModule", {
});
exports.sheetsManager = undefined;
-var _keys = __webpack_require__(40);
+var _keys = __webpack_require__(31);
var _keys2 = _interopRequireDefault(_keys);
@@ -373,23 +373,23 @@ var _extends2 = __webpack_require__(3);
var _extends3 = _interopRequireDefault(_extends2);
-var _getPrototypeOf = __webpack_require__(10);
+var _getPrototypeOf = __webpack_require__(8);
var _getPrototypeOf2 = _interopRequireDefault(_getPrototypeOf);
-var _classCallCheck2 = __webpack_require__(11);
+var _classCallCheck2 = __webpack_require__(9);
var _classCallCheck3 = _interopRequireDefault(_classCallCheck2);
-var _createClass2 = __webpack_require__(12);
+var _createClass2 = __webpack_require__(10);
var _createClass3 = _interopRequireDefault(_createClass2);
-var _possibleConstructorReturn2 = __webpack_require__(13);
+var _possibleConstructorReturn2 = __webpack_require__(11);
var _possibleConstructorReturn3 = _interopRequireDefault(_possibleConstructorReturn2);
-var _inherits2 = __webpack_require__(14);
+var _inherits2 = __webpack_require__(12);
var _inherits3 = _interopRequireDefault(_inherits2);
@@ -397,11 +397,11 @@ var _objectWithoutProperties2 = __webpack_require__(4);
var _objectWithoutProperties3 = _interopRequireDefault(_objectWithoutProperties2);
-var _map = __webpack_require__(590);
+var _map = __webpack_require__(452);
var _map2 = _interopRequireDefault(_map);
-var _minSafeInteger = __webpack_require__(606);
+var _minSafeInteger = __webpack_require__(468);
var _minSafeInteger2 = _interopRequireDefault(_minSafeInteger);
@@ -413,49 +413,49 @@ var _propTypes = __webpack_require__(0);
var _propTypes2 = _interopRequireDefault(_propTypes);
-var _warning = __webpack_require__(17);
+var _warning = __webpack_require__(15);
var _warning2 = _interopRequireDefault(_warning);
-var _hoistNonReactStatics = __webpack_require__(609);
+var _hoistNonReactStatics = __webpack_require__(471);
var _hoistNonReactStatics2 = _interopRequireDefault(_hoistNonReactStatics);
-var _wrapDisplayName = __webpack_require__(88);
+var _wrapDisplayName = __webpack_require__(58);
var _wrapDisplayName2 = _interopRequireDefault(_wrapDisplayName);
-var _getDisplayName = __webpack_require__(318);
+var _getDisplayName = __webpack_require__(227);
var _getDisplayName2 = _interopRequireDefault(_getDisplayName);
-var _contextTypes = __webpack_require__(610);
+var _contextTypes = __webpack_require__(472);
var _contextTypes2 = _interopRequireDefault(_contextTypes);
-var _jss = __webpack_require__(186);
+var _jss = __webpack_require__(124);
-var _jssPresetDefault = __webpack_require__(331);
+var _jssPresetDefault = __webpack_require__(240);
var _jssPresetDefault2 = _interopRequireDefault(_jssPresetDefault);
-var _ns = __webpack_require__(332);
+var _ns = __webpack_require__(241);
var ns = _interopRequireWildcard(_ns);
-var _createMuiTheme = __webpack_require__(192);
+var _createMuiTheme = __webpack_require__(130);
var _createMuiTheme2 = _interopRequireDefault(_createMuiTheme);
-var _themeListener = __webpack_require__(185);
+var _themeListener = __webpack_require__(123);
var _themeListener2 = _interopRequireDefault(_themeListener);
-var _createGenerateClassName = __webpack_require__(643);
+var _createGenerateClassName = __webpack_require__(505);
var _createGenerateClassName2 = _interopRequireDefault(_createGenerateClassName);
-var _getStylesCreator = __webpack_require__(644);
+var _getStylesCreator = __webpack_require__(506);
var _getStylesCreator2 = _interopRequireDefault(_getStylesCreator);
@@ -463,7 +463,7 @@ function _interopRequireWildcard(obj) { if (obj && obj.__esModule) { return obj;
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
-var babelPluginFlowReactPropTypes_proptype_HigherOrderComponent = __webpack_require__(133).babelPluginFlowReactPropTypes_proptype_HigherOrderComponent || __webpack_require__(0).any; // weak
+var babelPluginFlowReactPropTypes_proptype_HigherOrderComponent = __webpack_require__(84).babelPluginFlowReactPropTypes_proptype_HigherOrderComponent || __webpack_require__(0).any; // weak
// New JSS instance.
var jss = (0, _jss.create)((0, _jssPresetDefault2.default)());
@@ -792,7 +792,7 @@ exports.default = withStyles;
exports.__esModule = true;
-var _defineProperty = __webpack_require__(175);
+var _defineProperty = __webpack_require__(113);
var _defineProperty2 = _interopRequireDefault(_defineProperty);
@@ -872,141 +872,12 @@ var __WEBPACK_AMD_DEFINE_ARRAY__, __WEBPACK_AMD_DEFINE_RESULT__;/*!
/* 8 */
/***/ (function(module, exports, __webpack_require__) {
-"use strict";
-/* WEBPACK VAR INJECTION */(function(process) {/**
- * Copyright (c) 2013-present, Facebook, Inc.
- *
- * This source code is licensed under the MIT license found in the
- * LICENSE file in the root directory of this source tree.
- *
- */
-
-
-
-/**
- * Use invariant() to assert state which your program assumes to be true.
- *
- * Provide sprintf-style format (only %s is supported) and arguments
- * to provide information about what broke and what you were
- * expecting.
- *
- * The invariant message will be stripped in production, but the invariant
- * will remain to ensure logic does not differ in production.
- */
-
-var validateFormat = function validateFormat(format) {};
-
-if (process.env.NODE_ENV !== 'production') {
- validateFormat = function validateFormat(format) {
- if (format === undefined) {
- throw new Error('invariant requires an error message argument');
- }
- };
-}
-
-function invariant(condition, format, a, b, c, d, e, f) {
- validateFormat(format);
-
- if (!condition) {
- var error;
- if (format === undefined) {
- error = new Error('Minified exception occurred; use the non-minified dev environment ' + 'for the full error message and additional helpful warnings.');
- } else {
- var args = [a, b, c, d, e, f];
- var argIndex = 0;
- error = new Error(format.replace(/%s/g, function () {
- return args[argIndex++];
- }));
- error.name = 'Invariant Violation';
- }
-
- error.framesToPop = 1; // we don't care about invariant's own frame
- throw error;
- }
-}
-
-module.exports = invariant;
-/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
+module.exports = { "default": __webpack_require__(420), __esModule: true };
/***/ }),
/* 9 */
/***/ (function(module, exports, __webpack_require__) {
-"use strict";
-/* WEBPACK VAR INJECTION */(function(process) {/**
- * Copyright (c) 2014-present, Facebook, Inc.
- *
- * This source code is licensed under the MIT license found in the
- * LICENSE file in the root directory of this source tree.
- *
- */
-
-
-
-var emptyFunction = __webpack_require__(36);
-
-/**
- * Similar to invariant but only logs a warning if the condition is not met.
- * This can be used to log issues in development environments in critical
- * paths. Removing the logging code for production environments will keep the
- * same logic and follow the same code paths.
- */
-
-var warning = emptyFunction;
-
-if (process.env.NODE_ENV !== 'production') {
- var printWarning = function printWarning(format) {
- for (var _len = arguments.length, args = Array(_len > 1 ? _len - 1 : 0), _key = 1; _key < _len; _key++) {
- args[_key - 1] = arguments[_key];
- }
-
- var argIndex = 0;
- var message = 'Warning: ' + format.replace(/%s/g, function () {
- return args[argIndex++];
- });
- if (typeof console !== 'undefined') {
- console.error(message);
- }
- try {
- // --- Welcome to debugging React ---
- // This error was thrown as a convenience so that you can use this stack
- // to find the callsite that caused this warning to fire.
- throw new Error(message);
- } catch (x) {}
- };
-
- warning = function warning(condition, format) {
- if (format === undefined) {
- throw new Error('`warning(condition, format, ...args)` requires a warning ' + 'message argument');
- }
-
- if (format.indexOf('Failed Composite propType: ') === 0) {
- return; // Ignore CompositeComponent proptype check.
- }
-
- if (!condition) {
- for (var _len2 = arguments.length, args = Array(_len2 > 2 ? _len2 - 2 : 0), _key2 = 2; _key2 < _len2; _key2++) {
- args[_key2 - 2] = arguments[_key2];
- }
-
- printWarning.apply(undefined, [format].concat(args));
- }
- };
-}
-
-module.exports = warning;
-/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
-
-/***/ }),
-/* 10 */
-/***/ (function(module, exports, __webpack_require__) {
-
-module.exports = { "default": __webpack_require__(558), __esModule: true };
-
-/***/ }),
-/* 11 */
-/***/ (function(module, exports, __webpack_require__) {
-
"use strict";
@@ -1019,7 +890,7 @@ exports.default = function (instance, Constructor) {
};
/***/ }),
-/* 12 */
+/* 10 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -1027,7 +898,7 @@ exports.default = function (instance, Constructor) {
exports.__esModule = true;
-var _defineProperty = __webpack_require__(175);
+var _defineProperty = __webpack_require__(113);
var _defineProperty2 = _interopRequireDefault(_defineProperty);
@@ -1052,7 +923,7 @@ exports.default = function () {
}();
/***/ }),
-/* 13 */
+/* 11 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -1060,7 +931,7 @@ exports.default = function () {
exports.__esModule = true;
-var _typeof2 = __webpack_require__(125);
+var _typeof2 = __webpack_require__(76);
var _typeof3 = _interopRequireDefault(_typeof2);
@@ -1075,7 +946,7 @@ exports.default = function (self, call) {
};
/***/ }),
-/* 14 */
+/* 12 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -1083,15 +954,15 @@ exports.default = function (self, call) {
exports.__esModule = true;
-var _setPrototypeOf = __webpack_require__(575);
+var _setPrototypeOf = __webpack_require__(437);
var _setPrototypeOf2 = _interopRequireDefault(_setPrototypeOf);
-var _create = __webpack_require__(579);
+var _create = __webpack_require__(441);
var _create2 = _interopRequireDefault(_create);
-var _typeof2 = __webpack_require__(125);
+var _typeof2 = __webpack_require__(76);
var _typeof3 = _interopRequireDefault(_typeof2);
@@ -1114,7 +985,7 @@ exports.default = function (subClass, superClass) {
};
/***/ }),
-/* 15 */
+/* 13 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -1152,15 +1023,15 @@ if (process.env.NODE_ENV === 'production') {
// DCE check should happen before ReactDOM bundle executes so that
// DevTools can report bad minification during injection.
checkDCE();
- module.exports = __webpack_require__(458);
+ module.exports = __webpack_require__(320);
} else {
- module.exports = __webpack_require__(461);
+ module.exports = __webpack_require__(323);
}
/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
/***/ }),
-/* 16 */
+/* 14 */
/***/ (function(module, exports, __webpack_require__) {
/* WEBPACK VAR INJECTION */(function(process) {/**
@@ -1185,17 +1056,17 @@ if (process.env.NODE_ENV !== 'production') {
// By explicitly using `prop-types` you are opting into new development behavior.
// http://fb.me/prop-types-in-prod
var throwOnDirectAccess = true;
- module.exports = __webpack_require__(1024)(isValidElement, throwOnDirectAccess);
+ module.exports = __webpack_require__(726)(isValidElement, throwOnDirectAccess);
} else {
// By explicitly using `prop-types` you are opting into new production behavior.
// http://fb.me/prop-types-in-prod
- module.exports = __webpack_require__(1027)();
+ module.exports = __webpack_require__(729)();
}
/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
/***/ }),
-/* 17 */
+/* 15 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -1263,7 +1134,7 @@ module.exports = warning;
/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
/***/ }),
-/* 18 */
+/* 16 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -1273,7 +1144,7 @@ Object.defineProperty(exports, "__esModule", {
value: true
});
-var _SvgIcon = __webpack_require__(661);
+var _SvgIcon = __webpack_require__(523);
Object.defineProperty(exports, 'default', {
enumerable: true,
@@ -1285,49 +1156,7 @@ Object.defineProperty(exports, 'default', {
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
/***/ }),
-/* 19 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-/**
- * Copyright (c) 2013-present, Facebook, Inc.
- *
- * This source code is licensed under the MIT license found in the
- * LICENSE file in the root directory of this source tree.
- *
- *
- */
-
-
-/**
- * WARNING: DO NOT manually require this module.
- * This is a replacement for `invariant(...)` used by the error code system
- * and will _only_ be required by the corresponding babel pass.
- * It always throws.
- */
-
-function reactProdInvariant(code) {
- var argCount = arguments.length - 1;
-
- var message = 'Minified React error #' + code + '; visit ' + 'http://facebook.github.io/react/docs/error-decoder.html?invariant=' + code;
-
- for (var argIdx = 0; argIdx < argCount; argIdx++) {
- message += '&args[]=' + encodeURIComponent(arguments[argIdx + 1]);
- }
-
- message += ' for the full message or use the non-minified dev environment' + ' for full errors and additional helpful warnings.';
-
- var error = new Error(message);
- error.name = 'Invariant Violation';
- error.framesToPop = 1; // we don't care about reactProdInvariant's own frame
-
- throw error;
-}
-
-module.exports = reactProdInvariant;
-
-/***/ }),
-/* 20 */
+/* 17 */
/***/ (function(module, exports) {
var g;
@@ -1354,104 +1183,7 @@ module.exports = g;
/***/ }),
-/* 21 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-/*
-object-assign
-(c) Sindre Sorhus
-@license MIT
-*/
-
-
-/* eslint-disable no-unused-vars */
-var getOwnPropertySymbols = Object.getOwnPropertySymbols;
-var hasOwnProperty = Object.prototype.hasOwnProperty;
-var propIsEnumerable = Object.prototype.propertyIsEnumerable;
-
-function toObject(val) {
- if (val === null || val === undefined) {
- throw new TypeError('Object.assign cannot be called with null or undefined');
- }
-
- return Object(val);
-}
-
-function shouldUseNative() {
- try {
- if (!Object.assign) {
- return false;
- }
-
- // Detect buggy property enumeration order in older V8 versions.
-
- // https://bugs.chromium.org/p/v8/issues/detail?id=4118
- var test1 = new String('abc'); // eslint-disable-line no-new-wrappers
- test1[5] = 'de';
- if (Object.getOwnPropertyNames(test1)[0] === '5') {
- return false;
- }
-
- // https://bugs.chromium.org/p/v8/issues/detail?id=3056
- var test2 = {};
- for (var i = 0; i < 10; i++) {
- test2['_' + String.fromCharCode(i)] = i;
- }
- var order2 = Object.getOwnPropertyNames(test2).map(function (n) {
- return test2[n];
- });
- if (order2.join('') !== '0123456789') {
- return false;
- }
-
- // https://bugs.chromium.org/p/v8/issues/detail?id=3056
- var test3 = {};
- 'abcdefghijklmnopqrst'.split('').forEach(function (letter) {
- test3[letter] = letter;
- });
- if (Object.keys(Object.assign({}, test3)).join('') !==
- 'abcdefghijklmnopqrst') {
- return false;
- }
-
- return true;
- } catch (err) {
- // We don't expect any of the above to throw, but better to be safe.
- return false;
- }
-}
-
-module.exports = shouldUseNative() ? Object.assign : function (target, source) {
- var from;
- var to = toObject(target);
- var symbols;
-
- for (var s = 1; s < arguments.length; s++) {
- from = Object(arguments[s]);
-
- for (var key in from) {
- if (hasOwnProperty.call(from, key)) {
- to[key] = from[key];
- }
- }
-
- if (getOwnPropertySymbols) {
- symbols = getOwnPropertySymbols(from);
- for (var i = 0; i < symbols.length; i++) {
- if (propIsEnumerable.call(from, symbols[i])) {
- to[symbols[i]] = from[symbols[i]];
- }
- }
- }
- }
-
- return to;
-};
-
-
-/***/ }),
-/* 22 */
+/* 18 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -1557,206 +1289,7 @@ var _temp = function () {
;
/***/ }),
-/* 23 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-/* WEBPACK VAR INJECTION */(function(process) {/**
- * Copyright (c) 2013-present, Facebook, Inc.
- *
- * This source code is licensed under the MIT license found in the
- * LICENSE file in the root directory of this source tree.
- *
- */
-
-
-
-var _prodInvariant = __webpack_require__(19);
-
-var DOMProperty = __webpack_require__(63);
-var ReactDOMComponentFlags = __webpack_require__(367);
-
-var invariant = __webpack_require__(8);
-
-var ATTR_NAME = DOMProperty.ID_ATTRIBUTE_NAME;
-var Flags = ReactDOMComponentFlags;
-
-var internalInstanceKey = '__reactInternalInstance$' + Math.random().toString(36).slice(2);
-
-/**
- * Check if a given node should be cached.
- */
-function shouldPrecacheNode(node, nodeID) {
- return node.nodeType === 1 && node.getAttribute(ATTR_NAME) === String(nodeID) || node.nodeType === 8 && node.nodeValue === ' react-text: ' + nodeID + ' ' || node.nodeType === 8 && node.nodeValue === ' react-empty: ' + nodeID + ' ';
-}
-
-/**
- * Drill down (through composites and empty components) until we get a host or
- * host text component.
- *
- * This is pretty polymorphic but unavoidable with the current structure we have
- * for `_renderedChildren`.
- */
-function getRenderedHostOrTextFromComponent(component) {
- var rendered;
- while (rendered = component._renderedComponent) {
- component = rendered;
- }
- return component;
-}
-
-/**
- * Populate `_hostNode` on the rendered host/text component with the given
- * DOM node. The passed `inst` can be a composite.
- */
-function precacheNode(inst, node) {
- var hostInst = getRenderedHostOrTextFromComponent(inst);
- hostInst._hostNode = node;
- node[internalInstanceKey] = hostInst;
-}
-
-function uncacheNode(inst) {
- var node = inst._hostNode;
- if (node) {
- delete node[internalInstanceKey];
- inst._hostNode = null;
- }
-}
-
-/**
- * Populate `_hostNode` on each child of `inst`, assuming that the children
- * match up with the DOM (element) children of `node`.
- *
- * We cache entire levels at once to avoid an n^2 problem where we access the
- * children of a node sequentially and have to walk from the start to our target
- * node every time.
- *
- * Since we update `_renderedChildren` and the actual DOM at (slightly)
- * different times, we could race here and see a newer `_renderedChildren` than
- * the DOM nodes we see. To avoid this, ReactMultiChild calls
- * `prepareToManageChildren` before we change `_renderedChildren`, at which
- * time the container's child nodes are always cached (until it unmounts).
- */
-function precacheChildNodes(inst, node) {
- if (inst._flags & Flags.hasCachedChildNodes) {
- return;
- }
- var children = inst._renderedChildren;
- var childNode = node.firstChild;
- outer: for (var name in children) {
- if (!children.hasOwnProperty(name)) {
- continue;
- }
- var childInst = children[name];
- var childID = getRenderedHostOrTextFromComponent(childInst)._domID;
- if (childID === 0) {
- // We're currently unmounting this child in ReactMultiChild; skip it.
- continue;
- }
- // We assume the child nodes are in the same order as the child instances.
- for (; childNode !== null; childNode = childNode.nextSibling) {
- if (shouldPrecacheNode(childNode, childID)) {
- precacheNode(childInst, childNode);
- continue outer;
- }
- }
- // We reached the end of the DOM children without finding an ID match.
- true ? process.env.NODE_ENV !== 'production' ? invariant(false, 'Unable to find element with ID %s.', childID) : _prodInvariant('32', childID) : void 0;
- }
- inst._flags |= Flags.hasCachedChildNodes;
-}
-
-/**
- * Given a DOM node, return the closest ReactDOMComponent or
- * ReactDOMTextComponent instance ancestor.
- */
-function getClosestInstanceFromNode(node) {
- if (node[internalInstanceKey]) {
- return node[internalInstanceKey];
- }
-
- // Walk up the tree until we find an ancestor whose instance we have cached.
- var parents = [];
- while (!node[internalInstanceKey]) {
- parents.push(node);
- if (node.parentNode) {
- node = node.parentNode;
- } else {
- // Top of the tree. This node must not be part of a React tree (or is
- // unmounted, potentially).
- return null;
- }
- }
-
- var closest;
- var inst;
- for (; node && (inst = node[internalInstanceKey]); node = parents.pop()) {
- closest = inst;
- if (parents.length) {
- precacheChildNodes(inst, node);
- }
- }
-
- return closest;
-}
-
-/**
- * Given a DOM node, return the ReactDOMComponent or ReactDOMTextComponent
- * instance, or null if the node was not rendered by this React.
- */
-function getInstanceFromNode(node) {
- var inst = getClosestInstanceFromNode(node);
- if (inst != null && inst._hostNode === node) {
- return inst;
- } else {
- return null;
- }
-}
-
-/**
- * Given a ReactDOMComponent or ReactDOMTextComponent, return the corresponding
- * DOM node.
- */
-function getNodeFromInstance(inst) {
- // Without this first invariant, passing a non-DOM-component triggers the next
- // invariant for a missing parent, which is super confusing.
- !(inst._hostNode !== undefined) ? process.env.NODE_ENV !== 'production' ? invariant(false, 'getNodeFromInstance: Invalid argument.') : _prodInvariant('33') : void 0;
-
- if (inst._hostNode) {
- return inst._hostNode;
- }
-
- // Walk up the tree until we find an ancestor whose DOM node we have cached.
- var parents = [];
- while (!inst._hostNode) {
- parents.push(inst);
- !inst._hostParent ? process.env.NODE_ENV !== 'production' ? invariant(false, 'React DOM tree root should always have a node reference.') : _prodInvariant('34') : void 0;
- inst = inst._hostParent;
- }
-
- // Now parents contains each ancestor that does *not* have a cached native
- // node, and `inst` is the deepest ancestor that does.
- for (; parents.length; inst = parents.pop()) {
- precacheChildNodes(inst, inst._hostNode);
- }
-
- return inst._hostNode;
-}
-
-var ReactDOMComponentTree = {
- getClosestInstanceFromNode: getClosestInstanceFromNode,
- getInstanceFromNode: getInstanceFromNode,
- getNodeFromInstance: getNodeFromInstance,
- precacheChildNodes: precacheChildNodes,
- precacheNode: precacheNode,
- uncacheNode: uncacheNode
-};
-
-module.exports = ReactDOMComponentTree;
-/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
-
-/***/ }),
-/* 24 */
+/* 19 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -1764,19 +1297,19 @@ module.exports = ReactDOMComponentTree;
exports.__esModule = true;
-var _shouldUpdate = __webpack_require__(710);
+var _shouldUpdate = __webpack_require__(572);
var _shouldUpdate2 = _interopRequireDefault(_shouldUpdate);
-var _shallowEqual = __webpack_require__(713);
+var _shallowEqual = __webpack_require__(575);
var _shallowEqual2 = _interopRequireDefault(_shallowEqual);
-var _setDisplayName = __webpack_require__(355);
+var _setDisplayName = __webpack_require__(264);
var _setDisplayName2 = _interopRequireDefault(_setDisplayName);
-var _wrapDisplayName = __webpack_require__(356);
+var _wrapDisplayName = __webpack_require__(265);
var _wrapDisplayName2 = _interopRequireDefault(_wrapDisplayName);
@@ -1798,46 +1331,7 @@ exports.default = pure;
/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
/***/ }),
-/* 25 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-/**
- * Copyright (c) 2013-present, Facebook, Inc.
- *
- * This source code is licensed under the MIT license found in the
- * LICENSE file in the root directory of this source tree.
- *
- */
-
-
-
-var canUseDOM = !!(typeof window !== 'undefined' && window.document && window.document.createElement);
-
-/**
- * Simple, lightweight module assisting with the detection and context of
- * Worker. Helps avoid circular dependencies and allows code to reason about
- * whether or not they are in a Worker, even if they never include the main
- * `ReactWorker` dependency.
- */
-var ExecutionEnvironment = {
-
- canUseDOM: canUseDOM,
-
- canUseWorkers: typeof Worker !== 'undefined',
-
- canUseEventListeners: canUseDOM && !!(window.addEventListener || window.attachEvent),
-
- canUseViewport: canUseDOM && !!window.screen,
-
- isInWorker: !canUseDOM // For now, this is true - might change in the future.
-
-};
-
-module.exports = ExecutionEnvironment;
-
-/***/ }),
-/* 26 */
+/* 20 */
/***/ (function(module, exports) {
var core = module.exports = { version: '2.5.1' };
@@ -1845,7 +1339,7 @@ if (typeof __e == 'number') __e = core; // eslint-disable-line no-undef
/***/ }),
-/* 27 */
+/* 21 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -1855,11 +1349,11 @@ Object.defineProperty(exports, "__esModule", {
value: true
});
-var _typeof2 = __webpack_require__(125);
+var _typeof2 = __webpack_require__(76);
var _typeof3 = _interopRequireDefault(_typeof2);
-var _keys = __webpack_require__(40);
+var _keys = __webpack_require__(31);
var _keys2 = _interopRequireDefault(_keys);
@@ -1869,7 +1363,7 @@ exports.findIndex = findIndex;
exports.find = find;
exports.createChainedFunction = createChainedFunction;
-var _warning = __webpack_require__(17);
+var _warning = __webpack_require__(15);
var _warning2 = _interopRequireDefault(_warning);
@@ -1940,7 +1434,7 @@ function createChainedFunction() {
/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
/***/ }),
-/* 28 */
+/* 22 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -1963,14 +1457,14 @@ var babelPluginFlowReactPropTypes_proptype_TransitionClasses = {
};
/***/ }),
-/* 29 */
+/* 23 */
/***/ (function(module, __webpack_exports__, __webpack_require__) {
"use strict";
Object.defineProperty(__webpack_exports__, "__esModule", { value: true });
-/* harmony import */ var __WEBPACK_IMPORTED_MODULE_0__components_Provider__ = __webpack_require__(499);
-/* harmony import */ var __WEBPACK_IMPORTED_MODULE_1__components_connectAdvanced__ = __webpack_require__(292);
-/* harmony import */ var __WEBPACK_IMPORTED_MODULE_2__connect_connect__ = __webpack_require__(507);
+/* harmony import */ var __WEBPACK_IMPORTED_MODULE_0__components_Provider__ = __webpack_require__(361);
+/* harmony import */ var __WEBPACK_IMPORTED_MODULE_1__components_connectAdvanced__ = __webpack_require__(201);
+/* harmony import */ var __WEBPACK_IMPORTED_MODULE_2__connect_connect__ = __webpack_require__(369);
/* harmony reexport (binding) */ __webpack_require__.d(__webpack_exports__, "Provider", function() { return __WEBPACK_IMPORTED_MODULE_0__components_Provider__["b"]; });
/* harmony reexport (binding) */ __webpack_require__.d(__webpack_exports__, "createProvider", function() { return __WEBPACK_IMPORTED_MODULE_0__components_Provider__["a"]; });
/* harmony reexport (binding) */ __webpack_require__.d(__webpack_exports__, "connectAdvanced", function() { return __WEBPACK_IMPORTED_MODULE_1__components_connectAdvanced__["a"]; });
@@ -1982,7 +1476,7 @@ Object.defineProperty(__webpack_exports__, "__esModule", { value: true });
/***/ }),
-/* 30 */
+/* 24 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -2006,13 +1500,13 @@ var RSS_MODAL_OPEN_STATE = exports.RSS_MODAL_OPEN_STATE = 'RSS_MODAL_OPEN_STATE'
var RSS_TORRENT_LIST = exports.RSS_TORRENT_LIST = 'RSS_TORRENT_LIST';
/***/ }),
-/* 31 */
+/* 25 */
/***/ (function(module, exports, __webpack_require__) {
-var global = __webpack_require__(45);
-var core = __webpack_require__(26);
-var ctx = __webpack_require__(73);
-var hide = __webpack_require__(59);
+var global = __webpack_require__(34);
+var core = __webpack_require__(20);
+var ctx = __webpack_require__(47);
+var hide = __webpack_require__(43);
var PROTOTYPE = 'prototype';
var $export = function (type, name, source) {
@@ -2073,7 +1567,7 @@ module.exports = $export;
/***/ }),
-/* 32 */
+/* 26 */
/***/ (function(module, exports, __webpack_require__) {
var __WEBPACK_AMD_DEFINE_ARRAY__, __WEBPACK_AMD_DEFINE_RESULT__;/*!
@@ -2128,7 +1622,7 @@ var __WEBPACK_AMD_DEFINE_ARRAY__, __WEBPACK_AMD_DEFINE_RESULT__;/*!
/***/ }),
-/* 33 */
+/* 27 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -2138,7 +1632,7 @@ Object.defineProperty(exports, "__esModule", {
value: true
});
-var _MuiThemeProvider = __webpack_require__(550);
+var _MuiThemeProvider = __webpack_require__(412);
Object.defineProperty(exports, 'MuiThemeProvider', {
enumerable: true,
@@ -2156,7 +1650,7 @@ Object.defineProperty(exports, 'withStyles', {
}
});
-var _withTheme = __webpack_require__(108);
+var _withTheme = __webpack_require__(67);
Object.defineProperty(exports, 'withTheme', {
enumerable: true,
@@ -2165,7 +1659,7 @@ Object.defineProperty(exports, 'withTheme', {
}
});
-var _createMuiTheme = __webpack_require__(192);
+var _createMuiTheme = __webpack_require__(130);
Object.defineProperty(exports, 'createMuiTheme', {
enumerable: true,
@@ -2177,395 +1671,12 @@ Object.defineProperty(exports, 'createMuiTheme', {
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
/***/ }),
-/* 34 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-/* WEBPACK VAR INJECTION */(function(process) {/**
- * Copyright (c) 2016-present, Facebook, Inc.
- *
- * This source code is licensed under the MIT license found in the
- * LICENSE file in the root directory of this source tree.
- *
- *
- */
-
-
-
-var _prodInvariant = __webpack_require__(94);
-
-var ReactCurrentOwner = __webpack_require__(50);
-
-var invariant = __webpack_require__(8);
-var warning = __webpack_require__(9);
-
-function isNative(fn) {
- // Based on isNative() from Lodash
- var funcToString = Function.prototype.toString;
- var hasOwnProperty = Object.prototype.hasOwnProperty;
- var reIsNative = RegExp('^' + funcToString
- // Take an example native function source for comparison
- .call(hasOwnProperty
- // Strip regex characters so we can use it for regex
- ).replace(/[\\^$.*+?()[\]{}|]/g, '\\$&'
- // Remove hasOwnProperty from the template to make it generic
- ).replace(/hasOwnProperty|(function).*?(?=\\\()| for .+?(?=\\\])/g, '$1.*?') + '$');
- try {
- var source = funcToString.call(fn);
- return reIsNative.test(source);
- } catch (err) {
- return false;
- }
-}
-
-var canUseCollections =
-// Array.from
-typeof Array.from === 'function' &&
-// Map
-typeof Map === 'function' && isNative(Map) &&
-// Map.prototype.keys
-Map.prototype != null && typeof Map.prototype.keys === 'function' && isNative(Map.prototype.keys) &&
-// Set
-typeof Set === 'function' && isNative(Set) &&
-// Set.prototype.keys
-Set.prototype != null && typeof Set.prototype.keys === 'function' && isNative(Set.prototype.keys);
-
-var setItem;
-var getItem;
-var removeItem;
-var getItemIDs;
-var addRoot;
-var removeRoot;
-var getRootIDs;
-
-if (canUseCollections) {
- var itemMap = new Map();
- var rootIDSet = new Set();
-
- setItem = function (id, item) {
- itemMap.set(id, item);
- };
- getItem = function (id) {
- return itemMap.get(id);
- };
- removeItem = function (id) {
- itemMap['delete'](id);
- };
- getItemIDs = function () {
- return Array.from(itemMap.keys());
- };
-
- addRoot = function (id) {
- rootIDSet.add(id);
- };
- removeRoot = function (id) {
- rootIDSet['delete'](id);
- };
- getRootIDs = function () {
- return Array.from(rootIDSet.keys());
- };
-} else {
- var itemByKey = {};
- var rootByKey = {};
-
- // Use non-numeric keys to prevent V8 performance issues:
- // https://github.com/facebook/react/pull/7232
- var getKeyFromID = function (id) {
- return '.' + id;
- };
- var getIDFromKey = function (key) {
- return parseInt(key.substr(1), 10);
- };
-
- setItem = function (id, item) {
- var key = getKeyFromID(id);
- itemByKey[key] = item;
- };
- getItem = function (id) {
- var key = getKeyFromID(id);
- return itemByKey[key];
- };
- removeItem = function (id) {
- var key = getKeyFromID(id);
- delete itemByKey[key];
- };
- getItemIDs = function () {
- return Object.keys(itemByKey).map(getIDFromKey);
- };
-
- addRoot = function (id) {
- var key = getKeyFromID(id);
- rootByKey[key] = true;
- };
- removeRoot = function (id) {
- var key = getKeyFromID(id);
- delete rootByKey[key];
- };
- getRootIDs = function () {
- return Object.keys(rootByKey).map(getIDFromKey);
- };
-}
-
-var unmountedIDs = [];
-
-function purgeDeep(id) {
- var item = getItem(id);
- if (item) {
- var childIDs = item.childIDs;
-
- removeItem(id);
- childIDs.forEach(purgeDeep);
- }
-}
-
-function describeComponentFrame(name, source, ownerName) {
- return '\n in ' + (name || 'Unknown') + (source ? ' (at ' + source.fileName.replace(/^.*[\\\/]/, '') + ':' + source.lineNumber + ')' : ownerName ? ' (created by ' + ownerName + ')' : '');
-}
-
-function getDisplayName(element) {
- if (element == null) {
- return '#empty';
- } else if (typeof element === 'string' || typeof element === 'number') {
- return '#text';
- } else if (typeof element.type === 'string') {
- return element.type;
- } else {
- return element.type.displayName || element.type.name || 'Unknown';
- }
-}
-
-function describeID(id) {
- var name = ReactComponentTreeHook.getDisplayName(id);
- var element = ReactComponentTreeHook.getElement(id);
- var ownerID = ReactComponentTreeHook.getOwnerID(id);
- var ownerName;
- if (ownerID) {
- ownerName = ReactComponentTreeHook.getDisplayName(ownerID);
- }
- process.env.NODE_ENV !== 'production' ? warning(element, 'ReactComponentTreeHook: Missing React element for debugID %s when ' + 'building stack', id) : void 0;
- return describeComponentFrame(name, element && element._source, ownerName);
-}
-
-var ReactComponentTreeHook = {
- onSetChildren: function (id, nextChildIDs) {
- var item = getItem(id);
- !item ? process.env.NODE_ENV !== 'production' ? invariant(false, 'Item must have been set') : _prodInvariant('144') : void 0;
- item.childIDs = nextChildIDs;
-
- for (var i = 0; i < nextChildIDs.length; i++) {
- var nextChildID = nextChildIDs[i];
- var nextChild = getItem(nextChildID);
- !nextChild ? process.env.NODE_ENV !== 'production' ? invariant(false, 'Expected hook events to fire for the child before its parent includes it in onSetChildren().') : _prodInvariant('140') : void 0;
- !(nextChild.childIDs != null || typeof nextChild.element !== 'object' || nextChild.element == null) ? process.env.NODE_ENV !== 'production' ? invariant(false, 'Expected onSetChildren() to fire for a container child before its parent includes it in onSetChildren().') : _prodInvariant('141') : void 0;
- !nextChild.isMounted ? process.env.NODE_ENV !== 'production' ? invariant(false, 'Expected onMountComponent() to fire for the child before its parent includes it in onSetChildren().') : _prodInvariant('71') : void 0;
- if (nextChild.parentID == null) {
- nextChild.parentID = id;
- // TODO: This shouldn't be necessary but mounting a new root during in
- // componentWillMount currently causes not-yet-mounted components to
- // be purged from our tree data so their parent id is missing.
- }
- !(nextChild.parentID === id) ? process.env.NODE_ENV !== 'production' ? invariant(false, 'Expected onBeforeMountComponent() parent and onSetChildren() to be consistent (%s has parents %s and %s).', nextChildID, nextChild.parentID, id) : _prodInvariant('142', nextChildID, nextChild.parentID, id) : void 0;
- }
- },
- onBeforeMountComponent: function (id, element, parentID) {
- var item = {
- element: element,
- parentID: parentID,
- text: null,
- childIDs: [],
- isMounted: false,
- updateCount: 0
- };
- setItem(id, item);
- },
- onBeforeUpdateComponent: function (id, element) {
- var item = getItem(id);
- if (!item || !item.isMounted) {
- // We may end up here as a result of setState() in componentWillUnmount().
- // In this case, ignore the element.
- return;
- }
- item.element = element;
- },
- onMountComponent: function (id) {
- var item = getItem(id);
- !item ? process.env.NODE_ENV !== 'production' ? invariant(false, 'Item must have been set') : _prodInvariant('144') : void 0;
- item.isMounted = true;
- var isRoot = item.parentID === 0;
- if (isRoot) {
- addRoot(id);
- }
- },
- onUpdateComponent: function (id) {
- var item = getItem(id);
- if (!item || !item.isMounted) {
- // We may end up here as a result of setState() in componentWillUnmount().
- // In this case, ignore the element.
- return;
- }
- item.updateCount++;
- },
- onUnmountComponent: function (id) {
- var item = getItem(id);
- if (item) {
- // We need to check if it exists.
- // `item` might not exist if it is inside an error boundary, and a sibling
- // error boundary child threw while mounting. Then this instance never
- // got a chance to mount, but it still gets an unmounting event during
- // the error boundary cleanup.
- item.isMounted = false;
- var isRoot = item.parentID === 0;
- if (isRoot) {
- removeRoot(id);
- }
- }
- unmountedIDs.push(id);
- },
- purgeUnmountedComponents: function () {
- if (ReactComponentTreeHook._preventPurging) {
- // Should only be used for testing.
- return;
- }
-
- for (var i = 0; i < unmountedIDs.length; i++) {
- var id = unmountedIDs[i];
- purgeDeep(id);
- }
- unmountedIDs.length = 0;
- },
- isMounted: function (id) {
- var item = getItem(id);
- return item ? item.isMounted : false;
- },
- getCurrentStackAddendum: function (topElement) {
- var info = '';
- if (topElement) {
- var name = getDisplayName(topElement);
- var owner = topElement._owner;
- info += describeComponentFrame(name, topElement._source, owner && owner.getName());
- }
-
- var currentOwner = ReactCurrentOwner.current;
- var id = currentOwner && currentOwner._debugID;
-
- info += ReactComponentTreeHook.getStackAddendumByID(id);
- return info;
- },
- getStackAddendumByID: function (id) {
- var info = '';
- while (id) {
- info += describeID(id);
- id = ReactComponentTreeHook.getParentID(id);
- }
- return info;
- },
- getChildIDs: function (id) {
- var item = getItem(id);
- return item ? item.childIDs : [];
- },
- getDisplayName: function (id) {
- var element = ReactComponentTreeHook.getElement(id);
- if (!element) {
- return null;
- }
- return getDisplayName(element);
- },
- getElement: function (id) {
- var item = getItem(id);
- return item ? item.element : null;
- },
- getOwnerID: function (id) {
- var element = ReactComponentTreeHook.getElement(id);
- if (!element || !element._owner) {
- return null;
- }
- return element._owner._debugID;
- },
- getParentID: function (id) {
- var item = getItem(id);
- return item ? item.parentID : null;
- },
- getSource: function (id) {
- var item = getItem(id);
- var element = item ? item.element : null;
- var source = element != null ? element._source : null;
- return source;
- },
- getText: function (id) {
- var element = ReactComponentTreeHook.getElement(id);
- if (typeof element === 'string') {
- return element;
- } else if (typeof element === 'number') {
- return '' + element;
- } else {
- return null;
- }
- },
- getUpdateCount: function (id) {
- var item = getItem(id);
- return item ? item.updateCount : 0;
- },
-
-
- getRootIDs: getRootIDs,
- getRegisteredIDs: getItemIDs,
-
- pushNonStandardWarningStack: function (isCreatingElement, currentSource) {
- if (typeof console.reactStack !== 'function') {
- return;
- }
-
- var stack = [];
- var currentOwner = ReactCurrentOwner.current;
- var id = currentOwner && currentOwner._debugID;
-
- try {
- if (isCreatingElement) {
- stack.push({
- name: id ? ReactComponentTreeHook.getDisplayName(id) : null,
- fileName: currentSource ? currentSource.fileName : null,
- lineNumber: currentSource ? currentSource.lineNumber : null
- });
- }
-
- while (id) {
- var element = ReactComponentTreeHook.getElement(id);
- var parentID = ReactComponentTreeHook.getParentID(id);
- var ownerID = ReactComponentTreeHook.getOwnerID(id);
- var ownerName = ownerID ? ReactComponentTreeHook.getDisplayName(ownerID) : null;
- var source = element && element._source;
- stack.push({
- name: ownerName,
- fileName: source ? source.fileName : null,
- lineNumber: source ? source.lineNumber : null
- });
- id = parentID;
- }
- } catch (err) {
- // Internal state is messed up.
- // Stop building the stack (it's just a nice to have).
- }
-
- console.reactStack(stack);
- },
- popNonStandardWarningStack: function () {
- if (typeof console.reactStackEnd !== 'function') {
- return;
- }
- console.reactStackEnd();
- }
-};
-
-module.exports = ReactComponentTreeHook;
-/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
-
-/***/ }),
-/* 35 */
+/* 28 */
/***/ (function(module, exports, __webpack_require__) {
-var store = __webpack_require__(172)('wks');
-var uid = __webpack_require__(123);
-var Symbol = __webpack_require__(45).Symbol;
+var store = __webpack_require__(110)('wks');
+var uid = __webpack_require__(74);
+var Symbol = __webpack_require__(34).Symbol;
var USE_SYMBOL = typeof Symbol == 'function';
var $exports = module.exports = function (name) {
@@ -2577,77 +1688,7 @@ $exports.store = store;
/***/ }),
-/* 36 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-
-
-/**
- * Copyright (c) 2013-present, Facebook, Inc.
- *
- * This source code is licensed under the MIT license found in the
- * LICENSE file in the root directory of this source tree.
- *
- *
- */
-
-function makeEmptyFunction(arg) {
- return function () {
- return arg;
- };
-}
-
-/**
- * This function accepts and discards inputs; it has no side effects. This is
- * primarily useful idiomatically for overridable function endpoints which
- * always need to be callable, since JS lacks a null-call idiom ala Cocoa.
- */
-var emptyFunction = function emptyFunction() {};
-
-emptyFunction.thatReturns = makeEmptyFunction;
-emptyFunction.thatReturnsFalse = makeEmptyFunction(false);
-emptyFunction.thatReturnsTrue = makeEmptyFunction(true);
-emptyFunction.thatReturnsNull = makeEmptyFunction(null);
-emptyFunction.thatReturnsThis = function () {
- return this;
-};
-emptyFunction.thatReturnsArgument = function (arg) {
- return arg;
-};
-
-module.exports = emptyFunction;
-
-/***/ }),
-/* 37 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-/* WEBPACK VAR INJECTION */(function(process) {/**
- * Copyright (c) 2016-present, Facebook, Inc.
- *
- * This source code is licensed under the MIT license found in the
- * LICENSE file in the root directory of this source tree.
- *
- *
- */
-
-
-
-// Trust the developer to only use ReactInstrumentation with a __DEV__ check
-
-var debugTool = null;
-
-if (process.env.NODE_ENV !== 'production') {
- var ReactDebugTool = __webpack_require__(759);
- debugTool = ReactDebugTool;
-}
-
-module.exports = { debugTool: debugTool };
-/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
-
-/***/ }),
-/* 38 */
+/* 29 */
/***/ (function(module, exports, __webpack_require__) {
/* WEBPACK VAR INJECTION */(function(process) {/**
@@ -2672,25 +1713,25 @@ if (process.env.NODE_ENV !== 'production') {
// By explicitly using `prop-types` you are opting into new development behavior.
// http://fb.me/prop-types-in-prod
var throwOnDirectAccess = true;
- module.exports = __webpack_require__(481)(isValidElement, throwOnDirectAccess);
+ module.exports = __webpack_require__(343)(isValidElement, throwOnDirectAccess);
} else {
// By explicitly using `prop-types` you are opting into new production behavior.
// http://fb.me/prop-types-in-prod
- module.exports = __webpack_require__(484)();
+ module.exports = __webpack_require__(346)();
}
/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
/***/ }),
-/* 39 */
+/* 30 */
/***/ (function(module, exports, __webpack_require__) {
-var anObject = __webpack_require__(74);
-var IE8_DOM_DEFINE = __webpack_require__(298);
-var toPrimitive = __webpack_require__(166);
+var anObject = __webpack_require__(48);
+var IE8_DOM_DEFINE = __webpack_require__(207);
+var toPrimitive = __webpack_require__(104);
var dP = Object.defineProperty;
-exports.f = __webpack_require__(46) ? Object.defineProperty : function defineProperty(O, P, Attributes) {
+exports.f = __webpack_require__(35) ? Object.defineProperty : function defineProperty(O, P, Attributes) {
anObject(O);
P = toPrimitive(P, true);
anObject(Attributes);
@@ -2704,13 +1745,13 @@ exports.f = __webpack_require__(46) ? Object.defineProperty : function definePro
/***/ }),
-/* 40 */
+/* 31 */
/***/ (function(module, exports, __webpack_require__) {
-module.exports = { "default": __webpack_require__(588), __esModule: true };
+module.exports = { "default": __webpack_require__(450), __esModule: true };
/***/ }),
-/* 41 */
+/* 32 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -2720,7 +1761,7 @@ Object.defineProperty(exports, "__esModule", {
value: true
});
-var _IconButton = __webpack_require__(646);
+var _IconButton = __webpack_require__(508);
Object.defineProperty(exports, 'default', {
enumerable: true,
@@ -2732,25 +1773,7 @@ Object.defineProperty(exports, 'default', {
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
/***/ }),
-/* 42 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-
-
-module.exports = __webpack_require__(93);
-
-
-/***/ }),
-/* 43 */
-/***/ (function(module, exports) {
-
-var core = module.exports = { version: '2.5.3' };
-if (typeof __e == 'number') __e = core; // eslint-disable-line no-undef
-
-
-/***/ }),
-/* 44 */
+/* 33 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -2764,11 +1787,11 @@ var _react = __webpack_require__(1);
var _react2 = _interopRequireDefault(_react);
-var _Const = __webpack_require__(22);
+var _Const = __webpack_require__(18);
var _Const2 = _interopRequireDefault(_Const);
-var _classnames = __webpack_require__(32);
+var _classnames = __webpack_require__(26);
var _classnames2 = _interopRequireDefault(_classnames);
@@ -2909,7 +1932,7 @@ var _temp = function () {
;
/***/ }),
-/* 45 */
+/* 34 */
/***/ (function(module, exports) {
// https://github.com/zloirock/core-js/issues/86#issuecomment-115759028
@@ -2921,17 +1944,17 @@ if (typeof __g == 'number') __g = global; // eslint-disable-line no-undef
/***/ }),
-/* 46 */
+/* 35 */
/***/ (function(module, exports, __webpack_require__) {
// Thank's IE8 for his funny defineProperty
-module.exports = !__webpack_require__(75)(function () {
+module.exports = !__webpack_require__(49)(function () {
return Object.defineProperty({}, 'a', { get: function () { return 7; } }).a != 7;
});
/***/ }),
-/* 47 */
+/* 36 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -2941,7 +1964,7 @@ Object.defineProperty(exports, "__esModule", {
value: true
});
-var _Icon = __webpack_require__(645);
+var _Icon = __webpack_require__(507);
Object.defineProperty(exports, 'default', {
enumerable: true,
@@ -2953,7 +1976,7 @@ Object.defineProperty(exports, 'default', {
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
/***/ }),
-/* 48 */
+/* 37 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -2963,7 +1986,7 @@ Object.defineProperty(exports, "__esModule", {
value: true
});
-var _Button = __webpack_require__(663);
+var _Button = __webpack_require__(525);
Object.defineProperty(exports, 'default', {
enumerable: true,
@@ -2975,7 +1998,7 @@ Object.defineProperty(exports, 'default', {
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
/***/ }),
-/* 49 */
+/* 38 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -2983,19 +2006,19 @@ function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { de
exports.__esModule = true;
-var _shouldUpdate = __webpack_require__(701);
+var _shouldUpdate = __webpack_require__(563);
var _shouldUpdate2 = _interopRequireDefault(_shouldUpdate);
-var _shallowEqual = __webpack_require__(703);
+var _shallowEqual = __webpack_require__(565);
var _shallowEqual2 = _interopRequireDefault(_shallowEqual);
-var _setDisplayName = __webpack_require__(354);
+var _setDisplayName = __webpack_require__(263);
var _setDisplayName2 = _interopRequireDefault(_setDisplayName);
-var _wrapDisplayName = __webpack_require__(88);
+var _wrapDisplayName = __webpack_require__(58);
var _wrapDisplayName2 = _interopRequireDefault(_wrapDisplayName);
@@ -3017,346 +2040,7 @@ exports.default = pure;
/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
/***/ }),
-/* 50 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-/**
- * Copyright (c) 2013-present, Facebook, Inc.
- *
- * This source code is licensed under the MIT license found in the
- * LICENSE file in the root directory of this source tree.
- *
- *
- */
-
-
-
-/**
- * Keeps track of the current owner.
- *
- * The current owner is the component who should own any components that are
- * currently being constructed.
- */
-var ReactCurrentOwner = {
- /**
- * @internal
- * @type {ReactComponent}
- */
- current: null
-};
-
-module.exports = ReactCurrentOwner;
-
-/***/ }),
-/* 51 */
-/***/ (function(module, exports, __webpack_require__) {
-
-/* WEBPACK VAR INJECTION */(function(process) {/**
- * Copyright (c) 2013-present, Facebook, Inc.
- *
- * This source code is licensed under the MIT license found in the
- * LICENSE file in the root directory of this source tree.
- */
-
-if (process.env.NODE_ENV !== 'production') {
- var REACT_ELEMENT_TYPE = (typeof Symbol === 'function' &&
- Symbol.for &&
- Symbol.for('react.element')) ||
- 0xeac7;
-
- var isValidElement = function(object) {
- return typeof object === 'object' &&
- object !== null &&
- object.$$typeof === REACT_ELEMENT_TYPE;
- };
-
- // By explicitly using `prop-types` you are opting into new development behavior.
- // http://fb.me/prop-types-in-prod
- var throwOnDirectAccess = true;
- module.exports = __webpack_require__(366)(isValidElement, throwOnDirectAccess);
-} else {
- // By explicitly using `prop-types` you are opting into new production behavior.
- // http://fb.me/prop-types-in-prod
- module.exports = __webpack_require__(748)();
-}
-
-/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
-
-/***/ }),
-/* 52 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-/* WEBPACK VAR INJECTION */(function(process) {/**
- * Copyright (c) 2013-present, Facebook, Inc.
- *
- * This source code is licensed under the MIT license found in the
- * LICENSE file in the root directory of this source tree.
- *
- */
-
-
-
-var _prodInvariant = __webpack_require__(19),
- _assign = __webpack_require__(21);
-
-var CallbackQueue = __webpack_require__(371);
-var PooledClass = __webpack_require__(82);
-var ReactFeatureFlags = __webpack_require__(372);
-var ReactReconciler = __webpack_require__(95);
-var Transaction = __webpack_require__(140);
-
-var invariant = __webpack_require__(8);
-
-var dirtyComponents = [];
-var updateBatchNumber = 0;
-var asapCallbackQueue = CallbackQueue.getPooled();
-var asapEnqueued = false;
-
-var batchingStrategy = null;
-
-function ensureInjected() {
- !(ReactUpdates.ReactReconcileTransaction && batchingStrategy) ? process.env.NODE_ENV !== 'production' ? invariant(false, 'ReactUpdates: must inject a reconcile transaction class and batching strategy') : _prodInvariant('123') : void 0;
-}
-
-var NESTED_UPDATES = {
- initialize: function () {
- this.dirtyComponentsLength = dirtyComponents.length;
- },
- close: function () {
- if (this.dirtyComponentsLength !== dirtyComponents.length) {
- // Additional updates were enqueued by componentDidUpdate handlers or
- // similar; before our own UPDATE_QUEUEING wrapper closes, we want to run
- // these new updates so that if A's componentDidUpdate calls setState on
- // B, B will update before the callback A's updater provided when calling
- // setState.
- dirtyComponents.splice(0, this.dirtyComponentsLength);
- flushBatchedUpdates();
- } else {
- dirtyComponents.length = 0;
- }
- }
-};
-
-var UPDATE_QUEUEING = {
- initialize: function () {
- this.callbackQueue.reset();
- },
- close: function () {
- this.callbackQueue.notifyAll();
- }
-};
-
-var TRANSACTION_WRAPPERS = [NESTED_UPDATES, UPDATE_QUEUEING];
-
-function ReactUpdatesFlushTransaction() {
- this.reinitializeTransaction();
- this.dirtyComponentsLength = null;
- this.callbackQueue = CallbackQueue.getPooled();
- this.reconcileTransaction = ReactUpdates.ReactReconcileTransaction.getPooled(
- /* useCreateElement */true);
-}
-
-_assign(ReactUpdatesFlushTransaction.prototype, Transaction, {
- getTransactionWrappers: function () {
- return TRANSACTION_WRAPPERS;
- },
-
- destructor: function () {
- this.dirtyComponentsLength = null;
- CallbackQueue.release(this.callbackQueue);
- this.callbackQueue = null;
- ReactUpdates.ReactReconcileTransaction.release(this.reconcileTransaction);
- this.reconcileTransaction = null;
- },
-
- perform: function (method, scope, a) {
- // Essentially calls `this.reconcileTransaction.perform(method, scope, a)`
- // with this transaction's wrappers around it.
- return Transaction.perform.call(this, this.reconcileTransaction.perform, this.reconcileTransaction, method, scope, a);
- }
-});
-
-PooledClass.addPoolingTo(ReactUpdatesFlushTransaction);
-
-function batchedUpdates(callback, a, b, c, d, e) {
- ensureInjected();
- return batchingStrategy.batchedUpdates(callback, a, b, c, d, e);
-}
-
-/**
- * Array comparator for ReactComponents by mount ordering.
- *
- * @param {ReactComponent} c1 first component you're comparing
- * @param {ReactComponent} c2 second component you're comparing
- * @return {number} Return value usable by Array.prototype.sort().
- */
-function mountOrderComparator(c1, c2) {
- return c1._mountOrder - c2._mountOrder;
-}
-
-function runBatchedUpdates(transaction) {
- var len = transaction.dirtyComponentsLength;
- !(len === dirtyComponents.length) ? process.env.NODE_ENV !== 'production' ? invariant(false, 'Expected flush transaction\'s stored dirty-components length (%s) to match dirty-components array length (%s).', len, dirtyComponents.length) : _prodInvariant('124', len, dirtyComponents.length) : void 0;
-
- // Since reconciling a component higher in the owner hierarchy usually (not
- // always -- see shouldComponentUpdate()) will reconcile children, reconcile
- // them before their children by sorting the array.
- dirtyComponents.sort(mountOrderComparator);
-
- // Any updates enqueued while reconciling must be performed after this entire
- // batch. Otherwise, if dirtyComponents is [A, B] where A has children B and
- // C, B could update twice in a single batch if C's render enqueues an update
- // to B (since B would have already updated, we should skip it, and the only
- // way we can know to do so is by checking the batch counter).
- updateBatchNumber++;
-
- for (var i = 0; i < len; i++) {
- // If a component is unmounted before pending changes apply, it will still
- // be here, but we assume that it has cleared its _pendingCallbacks and
- // that performUpdateIfNecessary is a noop.
- var component = dirtyComponents[i];
-
- // If performUpdateIfNecessary happens to enqueue any new updates, we
- // shouldn't execute the callbacks until the next render happens, so
- // stash the callbacks first
- var callbacks = component._pendingCallbacks;
- component._pendingCallbacks = null;
-
- var markerName;
- if (ReactFeatureFlags.logTopLevelRenders) {
- var namedComponent = component;
- // Duck type TopLevelWrapper. This is probably always true.
- if (component._currentElement.type.isReactTopLevelWrapper) {
- namedComponent = component._renderedComponent;
- }
- markerName = 'React update: ' + namedComponent.getName();
- console.time(markerName);
- }
-
- ReactReconciler.performUpdateIfNecessary(component, transaction.reconcileTransaction, updateBatchNumber);
-
- if (markerName) {
- console.timeEnd(markerName);
- }
-
- if (callbacks) {
- for (var j = 0; j < callbacks.length; j++) {
- transaction.callbackQueue.enqueue(callbacks[j], component.getPublicInstance());
- }
- }
- }
-}
-
-var flushBatchedUpdates = function () {
- // ReactUpdatesFlushTransaction's wrappers will clear the dirtyComponents
- // array and perform any updates enqueued by mount-ready handlers (i.e.,
- // componentDidUpdate) but we need to check here too in order to catch
- // updates enqueued by setState callbacks and asap calls.
- while (dirtyComponents.length || asapEnqueued) {
- if (dirtyComponents.length) {
- var transaction = ReactUpdatesFlushTransaction.getPooled();
- transaction.perform(runBatchedUpdates, null, transaction);
- ReactUpdatesFlushTransaction.release(transaction);
- }
-
- if (asapEnqueued) {
- asapEnqueued = false;
- var queue = asapCallbackQueue;
- asapCallbackQueue = CallbackQueue.getPooled();
- queue.notifyAll();
- CallbackQueue.release(queue);
- }
- }
-};
-
-/**
- * Mark a component as needing a rerender, adding an optional callback to a
- * list of functions which will be executed once the rerender occurs.
- */
-function enqueueUpdate(component) {
- ensureInjected();
-
- // Various parts of our code (such as ReactCompositeComponent's
- // _renderValidatedComponent) assume that calls to render aren't nested;
- // verify that that's the case. (This is called by each top-level update
- // function, like setState, forceUpdate, etc.; creation and
- // destruction of top-level components is guarded in ReactMount.)
-
- if (!batchingStrategy.isBatchingUpdates) {
- batchingStrategy.batchedUpdates(enqueueUpdate, component);
- return;
- }
-
- dirtyComponents.push(component);
- if (component._updateBatchNumber == null) {
- component._updateBatchNumber = updateBatchNumber + 1;
- }
-}
-
-/**
- * Enqueue a callback to be run at the end of the current batching cycle. Throws
- * if no updates are currently being performed.
- */
-function asap(callback, context) {
- invariant(batchingStrategy.isBatchingUpdates, "ReactUpdates.asap: Can't enqueue an asap callback in a context where" + 'updates are not being batched.');
- asapCallbackQueue.enqueue(callback, context);
- asapEnqueued = true;
-}
-
-var ReactUpdatesInjection = {
- injectReconcileTransaction: function (ReconcileTransaction) {
- !ReconcileTransaction ? process.env.NODE_ENV !== 'production' ? invariant(false, 'ReactUpdates: must provide a reconcile transaction class') : _prodInvariant('126') : void 0;
- ReactUpdates.ReactReconcileTransaction = ReconcileTransaction;
- },
-
- injectBatchingStrategy: function (_batchingStrategy) {
- !_batchingStrategy ? process.env.NODE_ENV !== 'production' ? invariant(false, 'ReactUpdates: must provide a batching strategy') : _prodInvariant('127') : void 0;
- !(typeof _batchingStrategy.batchedUpdates === 'function') ? process.env.NODE_ENV !== 'production' ? invariant(false, 'ReactUpdates: must provide a batchedUpdates() function') : _prodInvariant('128') : void 0;
- !(typeof _batchingStrategy.isBatchingUpdates === 'boolean') ? process.env.NODE_ENV !== 'production' ? invariant(false, 'ReactUpdates: must provide an isBatchingUpdates boolean attribute') : _prodInvariant('129') : void 0;
- batchingStrategy = _batchingStrategy;
- }
-};
-
-var ReactUpdates = {
- /**
- * React references `ReactReconcileTransaction` using this property in order
- * to allow dependency injection.
- *
- * @internal
- */
- ReactReconcileTransaction: null,
-
- batchedUpdates: batchedUpdates,
- enqueueUpdate: enqueueUpdate,
- flushBatchedUpdates: flushBatchedUpdates,
- injection: ReactUpdatesInjection,
- asap: asap
-};
-
-module.exports = ReactUpdates;
-/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
-
-/***/ }),
-/* 53 */
-/***/ (function(module, exports, __webpack_require__) {
-
-var store = __webpack_require__(228)('wks');
-var uid = __webpack_require__(147);
-var Symbol = __webpack_require__(65).Symbol;
-var USE_SYMBOL = typeof Symbol == 'function';
-
-var $exports = module.exports = function (name) {
- return store[name] || (store[name] =
- USE_SYMBOL && Symbol[name] || (USE_SYMBOL ? Symbol : uid)('Symbol.' + name));
-};
-
-$exports.store = store;
-
-
-/***/ }),
-/* 54 */
+/* 39 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -3448,7 +2132,7 @@ function createBreakpoints(breakpoints) {
}
/***/ }),
-/* 55 */
+/* 40 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -3458,31 +2142,31 @@ Object.defineProperty(exports, "__esModule", {
value: true
});
-var _getPrototypeOf = __webpack_require__(10);
+var _getPrototypeOf = __webpack_require__(8);
var _getPrototypeOf2 = _interopRequireDefault(_getPrototypeOf);
-var _classCallCheck2 = __webpack_require__(11);
+var _classCallCheck2 = __webpack_require__(9);
var _classCallCheck3 = _interopRequireDefault(_classCallCheck2);
-var _createClass2 = __webpack_require__(12);
+var _createClass2 = __webpack_require__(10);
var _createClass3 = _interopRequireDefault(_createClass2);
-var _possibleConstructorReturn2 = __webpack_require__(13);
+var _possibleConstructorReturn2 = __webpack_require__(11);
var _possibleConstructorReturn3 = _interopRequireDefault(_possibleConstructorReturn2);
-var _inherits2 = __webpack_require__(14);
+var _inherits2 = __webpack_require__(12);
var _inherits3 = _interopRequireDefault(_inherits2);
-var _typeof2 = __webpack_require__(125);
+var _typeof2 = __webpack_require__(76);
var _typeof3 = _interopRequireDefault(_typeof2);
-var _keys = __webpack_require__(40);
+var _keys = __webpack_require__(31);
var _keys2 = _interopRequireDefault(_keys);
@@ -3490,7 +2174,7 @@ var _objectWithoutProperties2 = __webpack_require__(4);
var _objectWithoutProperties3 = _interopRequireDefault(_objectWithoutProperties2);
-var _assign = __webpack_require__(296);
+var _assign = __webpack_require__(205);
var _assign2 = _interopRequireDefault(_assign);
@@ -3502,15 +2186,15 @@ var _propTypes = __webpack_require__(0);
var _propTypes2 = _interopRequireDefault(_propTypes);
-var _shallowEqual = __webpack_require__(342);
+var _shallowEqual = __webpack_require__(251);
var _shallowEqual2 = _interopRequireDefault(_shallowEqual);
-var _warning = __webpack_require__(17);
+var _warning = __webpack_require__(15);
var _warning2 = _interopRequireDefault(_warning);
-var _supports = __webpack_require__(674);
+var _supports = __webpack_require__(536);
var supports = _interopRequireWildcard(_supports);
@@ -3677,7 +2361,7 @@ exports.default = EventListener;
/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
/***/ }),
-/* 56 */
+/* 41 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -3687,7 +2371,7 @@ Object.defineProperty(exports, "__esModule", {
value: true
});
-var _Typography = __webpack_require__(677);
+var _Typography = __webpack_require__(539);
Object.defineProperty(exports, 'default', {
enumerable: true,
@@ -3699,7 +2383,7 @@ Object.defineProperty(exports, 'default', {
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
/***/ }),
-/* 57 */
+/* 42 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -3709,7 +2393,7 @@ Object.defineProperty(exports, "__esModule", {
value: true
});
-var _Paper = __webpack_require__(693);
+var _Paper = __webpack_require__(555);
Object.defineProperty(exports, 'default', {
enumerable: true,
@@ -3721,339 +2405,64 @@ Object.defineProperty(exports, 'default', {
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
/***/ }),
-/* 58 */
+/* 43 */
/***/ (function(module, exports, __webpack_require__) {
-"use strict";
-/* WEBPACK VAR INJECTION */(function(process) {/**
- * Copyright (c) 2013-present, Facebook, Inc.
- *
- * This source code is licensed under the MIT license found in the
- * LICENSE file in the root directory of this source tree.
- *
- */
-
+var dP = __webpack_require__(30);
+var createDesc = __webpack_require__(64);
+module.exports = __webpack_require__(35) ? function (object, key, value) {
+ return dP.f(object, key, createDesc(1, value));
+} : function (object, key, value) {
+ object[key] = value;
+ return object;
+};
-var _assign = __webpack_require__(21);
+/***/ }),
+/* 44 */
+/***/ (function(module, exports) {
-var PooledClass = __webpack_require__(82);
+module.exports = function (it) {
+ return typeof it === 'object' ? it !== null : typeof it === 'function';
+};
-var emptyFunction = __webpack_require__(36);
-var warning = __webpack_require__(9);
-var didWarnForAddedNewProperty = false;
-var isProxySupported = typeof Proxy === 'function';
+/***/ }),
+/* 45 */
+/***/ (function(module, exports, __webpack_require__) {
-var shouldBeReleasedProperties = ['dispatchConfig', '_targetInst', 'nativeEvent', 'isDefaultPrevented', 'isPropagationStopped', '_dispatchListeners', '_dispatchInstances'];
+"use strict";
-/**
- * @interface Event
- * @see http://www.w3.org/TR/DOM-Level-3-Events/
- */
-var EventInterface = {
- type: null,
- target: null,
- // currentTarget is set when dispatching; no use in copying it here
- currentTarget: emptyFunction.thatReturnsNull,
- eventPhase: null,
- bubbles: null,
- cancelable: null,
- timeStamp: function (event) {
- return event.timeStamp || Date.now();
- },
- defaultPrevented: null,
- isTrusted: null
-};
-/**
- * Synthetic events are dispatched by event plugins, typically in response to a
- * top-level event delegation handler.
- *
- * These systems should generally use pooling to reduce the frequency of garbage
- * collection. The system should check `isPersistent` to determine whether the
- * event should be released into the pool after being dispatched. Users that
- * need a persisted event should invoke `persist`.
- *
- * Synthetic events (and subclasses) implement the DOM Level 3 Events API by
- * normalizing browser quirks. Subclasses do not necessarily have to implement a
- * DOM interface; custom application-specific events can also subclass this.
- *
- * @param {object} dispatchConfig Configuration used to dispatch this event.
- * @param {*} targetInst Marker identifying the event target.
- * @param {object} nativeEvent Native browser event.
- * @param {DOMEventTarget} nativeEventTarget Target node.
- */
-function SyntheticEvent(dispatchConfig, targetInst, nativeEvent, nativeEventTarget) {
- if (process.env.NODE_ENV !== 'production') {
- // these have a getter/setter for warnings
- delete this.nativeEvent;
- delete this.preventDefault;
- delete this.stopPropagation;
- }
+Object.defineProperty(exports, "__esModule", {
+ value: true
+});
- this.dispatchConfig = dispatchConfig;
- this._targetInst = targetInst;
- this.nativeEvent = nativeEvent;
+var _ButtonBase = __webpack_require__(509);
- var Interface = this.constructor.Interface;
- for (var propName in Interface) {
- if (!Interface.hasOwnProperty(propName)) {
- continue;
- }
- if (process.env.NODE_ENV !== 'production') {
- delete this[propName]; // this has a getter/setter for warnings
- }
- var normalize = Interface[propName];
- if (normalize) {
- this[propName] = normalize(nativeEvent);
- } else {
- if (propName === 'target') {
- this.target = nativeEventTarget;
- } else {
- this[propName] = nativeEvent[propName];
- }
- }
+Object.defineProperty(exports, 'default', {
+ enumerable: true,
+ get: function get() {
+ return _interopRequireDefault(_ButtonBase).default;
}
+});
- var defaultPrevented = nativeEvent.defaultPrevented != null ? nativeEvent.defaultPrevented : nativeEvent.returnValue === false;
- if (defaultPrevented) {
- this.isDefaultPrevented = emptyFunction.thatReturnsTrue;
- } else {
- this.isDefaultPrevented = emptyFunction.thatReturnsFalse;
- }
- this.isPropagationStopped = emptyFunction.thatReturnsFalse;
- return this;
-}
+function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
-_assign(SyntheticEvent.prototype, {
- preventDefault: function () {
- this.defaultPrevented = true;
- var event = this.nativeEvent;
- if (!event) {
- return;
- }
+/***/ }),
+/* 46 */
+/***/ (function(module, exports, __webpack_require__) {
- if (event.preventDefault) {
- event.preventDefault();
- // eslint-disable-next-line valid-typeof
- } else if (typeof event.returnValue !== 'unknown') {
- event.returnValue = false;
- }
- this.isDefaultPrevented = emptyFunction.thatReturnsTrue;
- },
+"use strict";
- stopPropagation: function () {
- var event = this.nativeEvent;
- if (!event) {
- return;
- }
- if (event.stopPropagation) {
- event.stopPropagation();
- // eslint-disable-next-line valid-typeof
- } else if (typeof event.cancelBubble !== 'unknown') {
- // The ChangeEventPlugin registers a "propertychange" event for
- // IE. This event does not support bubbling or cancelling, and
- // any references to cancelBubble throw "Member not found". A
- // typeof check of "unknown" circumvents this issue (and is also
- // IE specific).
- event.cancelBubble = true;
- }
+var _extends = Object.assign || function (target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i]; for (var key in source) { if (Object.prototype.hasOwnProperty.call(source, key)) { target[key] = source[key]; } } } return target; };
- this.isPropagationStopped = emptyFunction.thatReturnsTrue;
- },
+var _createClass = function () { function defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } return function (Constructor, protoProps, staticProps) { if (protoProps) defineProperties(Constructor.prototype, protoProps); if (staticProps) defineProperties(Constructor, staticProps); return Constructor; }; }();
- /**
- * We release all dispatched `SyntheticEvent`s after each event loop, adding
- * them back into the pool. This allows a way to hold onto a reference that
- * won't be added back into the pool.
- */
- persist: function () {
- this.isPersistent = emptyFunction.thatReturnsTrue;
- },
+var _class, _class2, _temp;
- /**
- * Checks if this event should be released back into the pool.
- *
- * @return {boolean} True if this should not be released, false otherwise.
- */
- isPersistent: emptyFunction.thatReturnsFalse,
-
- /**
- * `PooledClass` looks for `destructor` on each instance it releases.
- */
- destructor: function () {
- var Interface = this.constructor.Interface;
- for (var propName in Interface) {
- if (process.env.NODE_ENV !== 'production') {
- Object.defineProperty(this, propName, getPooledWarningPropertyDefinition(propName, Interface[propName]));
- } else {
- this[propName] = null;
- }
- }
- for (var i = 0; i < shouldBeReleasedProperties.length; i++) {
- this[shouldBeReleasedProperties[i]] = null;
- }
- if (process.env.NODE_ENV !== 'production') {
- Object.defineProperty(this, 'nativeEvent', getPooledWarningPropertyDefinition('nativeEvent', null));
- Object.defineProperty(this, 'preventDefault', getPooledWarningPropertyDefinition('preventDefault', emptyFunction));
- Object.defineProperty(this, 'stopPropagation', getPooledWarningPropertyDefinition('stopPropagation', emptyFunction));
- }
- }
-});
-
-SyntheticEvent.Interface = EventInterface;
-
-/**
- * Helper to reduce boilerplate when creating subclasses.
- *
- * @param {function} Class
- * @param {?object} Interface
- */
-SyntheticEvent.augmentClass = function (Class, Interface) {
- var Super = this;
-
- var E = function () {};
- E.prototype = Super.prototype;
- var prototype = new E();
-
- _assign(prototype, Class.prototype);
- Class.prototype = prototype;
- Class.prototype.constructor = Class;
-
- Class.Interface = _assign({}, Super.Interface, Interface);
- Class.augmentClass = Super.augmentClass;
-
- PooledClass.addPoolingTo(Class, PooledClass.fourArgumentPooler);
-};
-
-/** Proxying after everything set on SyntheticEvent
- * to resolve Proxy issue on some WebKit browsers
- * in which some Event properties are set to undefined (GH#10010)
- */
-if (process.env.NODE_ENV !== 'production') {
- if (isProxySupported) {
- /*eslint-disable no-func-assign */
- SyntheticEvent = new Proxy(SyntheticEvent, {
- construct: function (target, args) {
- return this.apply(target, Object.create(target.prototype), args);
- },
- apply: function (constructor, that, args) {
- return new Proxy(constructor.apply(that, args), {
- set: function (target, prop, value) {
- if (prop !== 'isPersistent' && !target.constructor.Interface.hasOwnProperty(prop) && shouldBeReleasedProperties.indexOf(prop) === -1) {
- process.env.NODE_ENV !== 'production' ? warning(didWarnForAddedNewProperty || target.isPersistent(), "This synthetic event is reused for performance reasons. If you're " + "seeing this, you're adding a new property in the synthetic event object. " + 'The property is never released. See ' + 'https://fb.me/react-event-pooling for more information.') : void 0;
- didWarnForAddedNewProperty = true;
- }
- target[prop] = value;
- return true;
- }
- });
- }
- });
- /*eslint-enable no-func-assign */
- }
-}
-
-PooledClass.addPoolingTo(SyntheticEvent, PooledClass.fourArgumentPooler);
-
-module.exports = SyntheticEvent;
-
-/**
- * Helper to nullify syntheticEvent instance properties when destructing
- *
- * @param {object} SyntheticEvent
- * @param {String} propName
- * @return {object} defineProperty object
- */
-function getPooledWarningPropertyDefinition(propName, getVal) {
- var isFunction = typeof getVal === 'function';
- return {
- configurable: true,
- set: set,
- get: get
- };
-
- function set(val) {
- var action = isFunction ? 'setting the method' : 'setting the property';
- warn(action, 'This is effectively a no-op');
- return val;
- }
-
- function get() {
- var action = isFunction ? 'accessing the method' : 'accessing the property';
- var result = isFunction ? 'This is a no-op function' : 'This is set to null';
- warn(action, result);
- return getVal;
- }
-
- function warn(action, result) {
- var warningCondition = false;
- process.env.NODE_ENV !== 'production' ? warning(warningCondition, "This synthetic event is reused for performance reasons. If you're seeing this, " + "you're %s `%s` on a released/nullified synthetic event. %s. " + 'If you must keep the original synthetic event around, use event.persist(). ' + 'See https://fb.me/react-event-pooling for more information.', action, propName, result) : void 0;
- }
-}
-/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
-
-/***/ }),
-/* 59 */
-/***/ (function(module, exports, __webpack_require__) {
-
-var dP = __webpack_require__(39);
-var createDesc = __webpack_require__(105);
-module.exports = __webpack_require__(46) ? function (object, key, value) {
- return dP.f(object, key, createDesc(1, value));
-} : function (object, key, value) {
- object[key] = value;
- return object;
-};
-
-
-/***/ }),
-/* 60 */
-/***/ (function(module, exports) {
-
-module.exports = function (it) {
- return typeof it === 'object' ? it !== null : typeof it === 'function';
-};
-
-
-/***/ }),
-/* 61 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-
-
-Object.defineProperty(exports, "__esModule", {
- value: true
-});
-
-var _ButtonBase = __webpack_require__(647);
-
-Object.defineProperty(exports, 'default', {
- enumerable: true,
- get: function get() {
- return _interopRequireDefault(_ButtonBase).default;
- }
-});
-
-function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
-
-/***/ }),
-/* 62 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-
-
-var _extends = Object.assign || function (target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i]; for (var key in source) { if (Object.prototype.hasOwnProperty.call(source, key)) { target[key] = source[key]; } } } return target; };
-
-var _createClass = function () { function defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } return function (Constructor, protoProps, staticProps) { if (protoProps) defineProperties(Constructor.prototype, protoProps); if (staticProps) defineProperties(Constructor, staticProps); return Constructor; }; }();
-
-var _class, _class2, _temp;
-
-/* Decoraters */
+/* Decoraters */
/* Utils */
@@ -4066,57 +2475,57 @@ var _react = __webpack_require__(1);
var _react2 = _interopRequireDefault(_react);
-var _propTypes = __webpack_require__(715);
+var _propTypes = __webpack_require__(577);
var _propTypes2 = _interopRequireDefault(_propTypes);
-var _reactDom = __webpack_require__(15);
+var _reactDom = __webpack_require__(13);
var _reactDom2 = _interopRequireDefault(_reactDom);
-var _classnames = __webpack_require__(720);
+var _classnames = __webpack_require__(582);
var _classnames2 = _interopRequireDefault(_classnames);
-var _staticMethods = __webpack_require__(721);
+var _staticMethods = __webpack_require__(583);
var _staticMethods2 = _interopRequireDefault(_staticMethods);
-var _windowListener = __webpack_require__(722);
+var _windowListener = __webpack_require__(584);
var _windowListener2 = _interopRequireDefault(_windowListener);
-var _customEvent = __webpack_require__(723);
+var _customEvent = __webpack_require__(585);
var _customEvent2 = _interopRequireDefault(_customEvent);
-var _isCapture = __webpack_require__(724);
+var _isCapture = __webpack_require__(586);
var _isCapture2 = _interopRequireDefault(_isCapture);
-var _getEffect = __webpack_require__(725);
+var _getEffect = __webpack_require__(587);
var _getEffect2 = _interopRequireDefault(_getEffect);
-var _trackRemoval = __webpack_require__(726);
+var _trackRemoval = __webpack_require__(588);
var _trackRemoval2 = _interopRequireDefault(_trackRemoval);
-var _getPosition = __webpack_require__(727);
+var _getPosition = __webpack_require__(589);
var _getPosition2 = _interopRequireDefault(_getPosition);
-var _getTipContent = __webpack_require__(728);
+var _getTipContent = __webpack_require__(590);
var _getTipContent2 = _interopRequireDefault(_getTipContent);
-var _aria = __webpack_require__(729);
+var _aria = __webpack_require__(591);
-var _nodeListToArray = __webpack_require__(730);
+var _nodeListToArray = __webpack_require__(592);
var _nodeListToArray2 = _interopRequireDefault(_nodeListToArray);
-var _style = __webpack_require__(731);
+var _style = __webpack_require__(593);
var _style2 = _interopRequireDefault(_style);
@@ -4641,458 +3050,11 @@ var ReactTooltip = (0, _staticMethods2.default)(_class = (0, _windowListener2.de
module.exports = ReactTooltip;
/***/ }),
-/* 63 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-/* WEBPACK VAR INJECTION */(function(process) {/**
- * Copyright (c) 2013-present, Facebook, Inc.
- *
- * This source code is licensed under the MIT license found in the
- * LICENSE file in the root directory of this source tree.
- *
- */
-
-
-
-var _prodInvariant = __webpack_require__(19);
-
-var invariant = __webpack_require__(8);
-
-function checkMask(value, bitmask) {
- return (value & bitmask) === bitmask;
-}
-
-var DOMPropertyInjection = {
- /**
- * Mapping from normalized, camelcased property names to a configuration that
- * specifies how the associated DOM property should be accessed or rendered.
- */
- MUST_USE_PROPERTY: 0x1,
- HAS_BOOLEAN_VALUE: 0x4,
- HAS_NUMERIC_VALUE: 0x8,
- HAS_POSITIVE_NUMERIC_VALUE: 0x10 | 0x8,
- HAS_OVERLOADED_BOOLEAN_VALUE: 0x20,
-
- /**
- * Inject some specialized knowledge about the DOM. This takes a config object
- * with the following properties:
- *
- * isCustomAttribute: function that given an attribute name will return true
- * if it can be inserted into the DOM verbatim. Useful for data-* or aria-*
- * attributes where it's impossible to enumerate all of the possible
- * attribute names,
- *
- * Properties: object mapping DOM property name to one of the
- * DOMPropertyInjection constants or null. If your attribute isn't in here,
- * it won't get written to the DOM.
- *
- * DOMAttributeNames: object mapping React attribute name to the DOM
- * attribute name. Attribute names not specified use the **lowercase**
- * normalized name.
- *
- * DOMAttributeNamespaces: object mapping React attribute name to the DOM
- * attribute namespace URL. (Attribute names not specified use no namespace.)
- *
- * DOMPropertyNames: similar to DOMAttributeNames but for DOM properties.
- * Property names not specified use the normalized name.
- *
- * DOMMutationMethods: Properties that require special mutation methods. If
- * `value` is undefined, the mutation method should unset the property.
- *
- * @param {object} domPropertyConfig the config as described above.
- */
- injectDOMPropertyConfig: function (domPropertyConfig) {
- var Injection = DOMPropertyInjection;
- var Properties = domPropertyConfig.Properties || {};
- var DOMAttributeNamespaces = domPropertyConfig.DOMAttributeNamespaces || {};
- var DOMAttributeNames = domPropertyConfig.DOMAttributeNames || {};
- var DOMPropertyNames = domPropertyConfig.DOMPropertyNames || {};
- var DOMMutationMethods = domPropertyConfig.DOMMutationMethods || {};
-
- if (domPropertyConfig.isCustomAttribute) {
- DOMProperty._isCustomAttributeFunctions.push(domPropertyConfig.isCustomAttribute);
- }
-
- for (var propName in Properties) {
- !!DOMProperty.properties.hasOwnProperty(propName) ? process.env.NODE_ENV !== 'production' ? invariant(false, 'injectDOMPropertyConfig(...): You\'re trying to inject DOM property \'%s\' which has already been injected. You may be accidentally injecting the same DOM property config twice, or you may be injecting two configs that have conflicting property names.', propName) : _prodInvariant('48', propName) : void 0;
-
- var lowerCased = propName.toLowerCase();
- var propConfig = Properties[propName];
-
- var propertyInfo = {
- attributeName: lowerCased,
- attributeNamespace: null,
- propertyName: propName,
- mutationMethod: null,
-
- mustUseProperty: checkMask(propConfig, Injection.MUST_USE_PROPERTY),
- hasBooleanValue: checkMask(propConfig, Injection.HAS_BOOLEAN_VALUE),
- hasNumericValue: checkMask(propConfig, Injection.HAS_NUMERIC_VALUE),
- hasPositiveNumericValue: checkMask(propConfig, Injection.HAS_POSITIVE_NUMERIC_VALUE),
- hasOverloadedBooleanValue: checkMask(propConfig, Injection.HAS_OVERLOADED_BOOLEAN_VALUE)
- };
- !(propertyInfo.hasBooleanValue + propertyInfo.hasNumericValue + propertyInfo.hasOverloadedBooleanValue <= 1) ? process.env.NODE_ENV !== 'production' ? invariant(false, 'DOMProperty: Value can be one of boolean, overloaded boolean, or numeric value, but not a combination: %s', propName) : _prodInvariant('50', propName) : void 0;
-
- if (process.env.NODE_ENV !== 'production') {
- DOMProperty.getPossibleStandardName[lowerCased] = propName;
- }
-
- if (DOMAttributeNames.hasOwnProperty(propName)) {
- var attributeName = DOMAttributeNames[propName];
- propertyInfo.attributeName = attributeName;
- if (process.env.NODE_ENV !== 'production') {
- DOMProperty.getPossibleStandardName[attributeName] = propName;
- }
- }
-
- if (DOMAttributeNamespaces.hasOwnProperty(propName)) {
- propertyInfo.attributeNamespace = DOMAttributeNamespaces[propName];
- }
-
- if (DOMPropertyNames.hasOwnProperty(propName)) {
- propertyInfo.propertyName = DOMPropertyNames[propName];
- }
-
- if (DOMMutationMethods.hasOwnProperty(propName)) {
- propertyInfo.mutationMethod = DOMMutationMethods[propName];
- }
-
- DOMProperty.properties[propName] = propertyInfo;
- }
- }
-};
-
-/* eslint-disable max-len */
-var ATTRIBUTE_NAME_START_CHAR = ':A-Z_a-z\\u00C0-\\u00D6\\u00D8-\\u00F6\\u00F8-\\u02FF\\u0370-\\u037D\\u037F-\\u1FFF\\u200C-\\u200D\\u2070-\\u218F\\u2C00-\\u2FEF\\u3001-\\uD7FF\\uF900-\\uFDCF\\uFDF0-\\uFFFD';
-/* eslint-enable max-len */
-
-/**
- * DOMProperty exports lookup objects that can be used like functions:
- *
- * > DOMProperty.isValid['id']
- * true
- * > DOMProperty.isValid['foobar']
- * undefined
- *
- * Although this may be confusing, it performs better in general.
- *
- * @see http://jsperf.com/key-exists
- * @see http://jsperf.com/key-missing
- */
-var DOMProperty = {
- ID_ATTRIBUTE_NAME: 'data-reactid',
- ROOT_ATTRIBUTE_NAME: 'data-reactroot',
-
- ATTRIBUTE_NAME_START_CHAR: ATTRIBUTE_NAME_START_CHAR,
- ATTRIBUTE_NAME_CHAR: ATTRIBUTE_NAME_START_CHAR + '\\-.0-9\\u00B7\\u0300-\\u036F\\u203F-\\u2040',
-
- /**
- * Map from property "standard name" to an object with info about how to set
- * the property in the DOM. Each object contains:
- *
- * attributeName:
- * Used when rendering markup or with `*Attribute()`.
- * attributeNamespace
- * propertyName:
- * Used on DOM node instances. (This includes properties that mutate due to
- * external factors.)
- * mutationMethod:
- * If non-null, used instead of the property or `setAttribute()` after
- * initial render.
- * mustUseProperty:
- * Whether the property must be accessed and mutated as an object property.
- * hasBooleanValue:
- * Whether the property should be removed when set to a falsey value.
- * hasNumericValue:
- * Whether the property must be numeric or parse as a numeric and should be
- * removed when set to a falsey value.
- * hasPositiveNumericValue:
- * Whether the property must be positive numeric or parse as a positive
- * numeric and should be removed when set to a falsey value.
- * hasOverloadedBooleanValue:
- * Whether the property can be used as a flag as well as with a value.
- * Removed when strictly equal to false; present without a value when
- * strictly equal to true; present with a value otherwise.
- */
- properties: {},
-
- /**
- * Mapping from lowercase property names to the properly cased version, used
- * to warn in the case of missing properties. Available only in __DEV__.
- *
- * autofocus is predefined, because adding it to the property whitelist
- * causes unintended side effects.
- *
- * @type {Object}
- */
- getPossibleStandardName: process.env.NODE_ENV !== 'production' ? { autofocus: 'autoFocus' } : null,
-
- /**
- * All of the isCustomAttribute() functions that have been injected.
- */
- _isCustomAttributeFunctions: [],
-
- /**
- * Checks whether a property name is a custom attribute.
- * @method
- */
- isCustomAttribute: function (attributeName) {
- for (var i = 0; i < DOMProperty._isCustomAttributeFunctions.length; i++) {
- var isCustomAttributeFn = DOMProperty._isCustomAttributeFunctions[i];
- if (isCustomAttributeFn(attributeName)) {
- return true;
- }
- }
- return false;
- },
-
- injection: DOMPropertyInjection
-};
-
-module.exports = DOMProperty;
-/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
-
-/***/ }),
-/* 64 */
-/***/ (function(module, exports, __webpack_require__) {
-
-var global = __webpack_require__(65);
-var core = __webpack_require__(43);
-var ctx = __webpack_require__(222);
-var hide = __webpack_require__(97);
-var PROTOTYPE = 'prototype';
-
-var $export = function (type, name, source) {
- var IS_FORCED = type & $export.F;
- var IS_GLOBAL = type & $export.G;
- var IS_STATIC = type & $export.S;
- var IS_PROTO = type & $export.P;
- var IS_BIND = type & $export.B;
- var IS_WRAP = type & $export.W;
- var exports = IS_GLOBAL ? core : core[name] || (core[name] = {});
- var expProto = exports[PROTOTYPE];
- var target = IS_GLOBAL ? global : IS_STATIC ? global[name] : (global[name] || {})[PROTOTYPE];
- var key, own, out;
- if (IS_GLOBAL) source = name;
- for (key in source) {
- // contains in native
- own = !IS_FORCED && target && target[key] !== undefined;
- if (own && key in exports) continue;
- // export native or passed
- out = own ? target[key] : source[key];
- // prevent global pollution for namespaces
- exports[key] = IS_GLOBAL && typeof target[key] != 'function' ? source[key]
- // bind timers to global for call from export context
- : IS_BIND && own ? ctx(out, global)
- // wrap global constructors for prevent change them in library
- : IS_WRAP && target[key] == out ? (function (C) {
- var F = function (a, b, c) {
- if (this instanceof C) {
- switch (arguments.length) {
- case 0: return new C();
- case 1: return new C(a);
- case 2: return new C(a, b);
- } return new C(a, b, c);
- } return C.apply(this, arguments);
- };
- F[PROTOTYPE] = C[PROTOTYPE];
- return F;
- // make static versions for prototype methods
- })(out) : IS_PROTO && typeof out == 'function' ? ctx(Function.call, out) : out;
- // export proto methods to core.%CONSTRUCTOR%.methods.%NAME%
- if (IS_PROTO) {
- (exports.virtual || (exports.virtual = {}))[key] = out;
- // export proto methods to core.%CONSTRUCTOR%.prototype.%NAME%
- if (type & $export.R && expProto && !expProto[key]) hide(expProto, key, out);
- }
- }
-};
-// type bitmap
-$export.F = 1; // forced
-$export.G = 2; // global
-$export.S = 4; // static
-$export.P = 8; // proto
-$export.B = 16; // bind
-$export.W = 32; // wrap
-$export.U = 64; // safe
-$export.R = 128; // real proto method for `library`
-module.exports = $export;
-
-
-/***/ }),
-/* 65 */
-/***/ (function(module, exports) {
-
-// https://github.com/zloirock/core-js/issues/86#issuecomment-115759028
-var global = module.exports = typeof window != 'undefined' && window.Math == Math
- ? window : typeof self != 'undefined' && self.Math == Math ? self
- // eslint-disable-next-line no-new-func
- : Function('return this')();
-if (typeof __g == 'number') __g = global; // eslint-disable-line no-undef
-
-
-/***/ }),
-/* 66 */
-/***/ (function(module, exports, __webpack_require__) {
-
-var anObject = __webpack_require__(98);
-var IE8_DOM_DEFINE = __webpack_require__(392);
-var toPrimitive = __webpack_require__(223);
-var dP = Object.defineProperty;
-
-exports.f = __webpack_require__(83) ? Object.defineProperty : function defineProperty(O, P, Attributes) {
- anObject(O);
- P = toPrimitive(P, true);
- anObject(Attributes);
- if (IE8_DOM_DEFINE) try {
- return dP(O, P, Attributes);
- } catch (e) { /* empty */ }
- if ('get' in Attributes || 'set' in Attributes) throw TypeError('Accessors not supported!');
- if ('value' in Attributes) O[P] = Attributes.value;
- return O;
-};
-
-
-/***/ }),
-/* 67 */
-/***/ (function(module, exports, __webpack_require__) {
-
-module.exports = { "default": __webpack_require__(843), __esModule: true };
-
-/***/ }),
-/* 68 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-
-
-exports.__esModule = true;
-
-exports.default = function (instance, Constructor) {
- if (!(instance instanceof Constructor)) {
- throw new TypeError("Cannot call a class as a function");
- }
-};
-
-/***/ }),
-/* 69 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-
-
-exports.__esModule = true;
-
-var _defineProperty = __webpack_require__(846);
-
-var _defineProperty2 = _interopRequireDefault(_defineProperty);
-
-function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
-
-exports.default = function () {
- function defineProperties(target, props) {
- for (var i = 0; i < props.length; i++) {
- var descriptor = props[i];
- descriptor.enumerable = descriptor.enumerable || false;
- descriptor.configurable = true;
- if ("value" in descriptor) descriptor.writable = true;
- (0, _defineProperty2.default)(target, descriptor.key, descriptor);
- }
- }
-
- return function (Constructor, protoProps, staticProps) {
- if (protoProps) defineProperties(Constructor.prototype, protoProps);
- if (staticProps) defineProperties(Constructor, staticProps);
- return Constructor;
- };
-}();
-
-/***/ }),
-/* 70 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-
-
-exports.__esModule = true;
-
-var _typeof2 = __webpack_require__(398);
-
-var _typeof3 = _interopRequireDefault(_typeof2);
-
-function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
-
-exports.default = function (self, call) {
- if (!self) {
- throw new ReferenceError("this hasn't been initialised - super() hasn't been called");
- }
-
- return call && ((typeof call === "undefined" ? "undefined" : (0, _typeof3.default)(call)) === "object" || typeof call === "function") ? call : self;
-};
-
-/***/ }),
-/* 71 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-
-
-exports.__esModule = true;
-
-var _setPrototypeOf = __webpack_require__(869);
-
-var _setPrototypeOf2 = _interopRequireDefault(_setPrototypeOf);
-
-var _create = __webpack_require__(873);
-
-var _create2 = _interopRequireDefault(_create);
-
-var _typeof2 = __webpack_require__(398);
-
-var _typeof3 = _interopRequireDefault(_typeof2);
-
-function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
-
-exports.default = function (subClass, superClass) {
- if (typeof superClass !== "function" && superClass !== null) {
- throw new TypeError("Super expression must either be null or a function, not " + (typeof superClass === "undefined" ? "undefined" : (0, _typeof3.default)(superClass)));
- }
-
- subClass.prototype = (0, _create2.default)(superClass && superClass.prototype, {
- constructor: {
- value: subClass,
- enumerable: false,
- writable: true,
- configurable: true
- }
- });
- if (superClass) _setPrototypeOf2.default ? (0, _setPrototypeOf2.default)(subClass, superClass) : subClass.__proto__ = superClass;
-};
-
-/***/ }),
-/* 72 */
-/***/ (function(module, exports) {
-
-module.exports = function (target) {
- for (var i = 1; i < arguments.length; i++) {
- var source = arguments[i];
- for (var key in source) {
- if (Object.prototype.hasOwnProperty.call(source, key)) {
- target[key] = source[key];
- }
- }
- }
- return target;
-};
-
-
-/***/ }),
-/* 73 */
+/* 47 */
/***/ (function(module, exports, __webpack_require__) {
// optional / simple context binding
-var aFunction = __webpack_require__(297);
+var aFunction = __webpack_require__(206);
module.exports = function (fn, that, length) {
aFunction(fn);
if (that === undefined) return fn;
@@ -5114,10 +3076,10 @@ module.exports = function (fn, that, length) {
/***/ }),
-/* 74 */
+/* 48 */
/***/ (function(module, exports, __webpack_require__) {
-var isObject = __webpack_require__(60);
+var isObject = __webpack_require__(44);
module.exports = function (it) {
if (!isObject(it)) throw TypeError(it + ' is not an object!');
return it;
@@ -5125,7 +3087,7 @@ module.exports = function (it) {
/***/ }),
-/* 75 */
+/* 49 */
/***/ (function(module, exports) {
module.exports = function (exec) {
@@ -5138,7 +3100,7 @@ module.exports = function (exec) {
/***/ }),
-/* 76 */
+/* 50 */
/***/ (function(module, exports) {
var hasOwnProperty = {}.hasOwnProperty;
@@ -5148,7 +3110,7 @@ module.exports = function (it, key) {
/***/ }),
-/* 77 */
+/* 51 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -5161,7 +3123,7 @@ exports.default = !!(typeof window !== 'undefined' && window.document && window.
module.exports = exports['default'];
/***/ }),
-/* 78 */
+/* 52 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -5171,7 +3133,7 @@ Object.defineProperty(exports, "__esModule", {
value: true
});
-var _TextField = __webpack_require__(664);
+var _TextField = __webpack_require__(526);
Object.defineProperty(exports, 'default', {
enumerable: true,
@@ -5183,12 +3145,12 @@ Object.defineProperty(exports, 'default', {
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
/***/ }),
-/* 79 */
+/* 53 */
/***/ (function(module, exports, __webpack_require__) {
-var isObject = __webpack_require__(197),
- now = __webpack_require__(666),
- toNumber = __webpack_require__(668);
+var isObject = __webpack_require__(135),
+ now = __webpack_require__(528),
+ toNumber = __webpack_require__(530);
/** Error message constants. */
var FUNC_ERROR_TEXT = 'Expected a function';
@@ -5377,7 +3339,7 @@ module.exports = debounce;
/***/ }),
-/* 80 */
+/* 54 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -5387,7 +3349,7 @@ Object.defineProperty(exports, "__esModule", {
value: true
});
-var _Dialog = __webpack_require__(704);
+var _Dialog = __webpack_require__(566);
Object.defineProperty(exports, 'default', {
enumerable: true,
@@ -5396,7 +3358,7 @@ Object.defineProperty(exports, 'default', {
}
});
-var _DialogActions = __webpack_require__(705);
+var _DialogActions = __webpack_require__(567);
Object.defineProperty(exports, 'DialogActions', {
enumerable: true,
@@ -5405,7 +3367,7 @@ Object.defineProperty(exports, 'DialogActions', {
}
});
-var _DialogTitle = __webpack_require__(706);
+var _DialogTitle = __webpack_require__(568);
Object.defineProperty(exports, 'DialogTitle', {
enumerable: true,
@@ -5414,7 +3376,7 @@ Object.defineProperty(exports, 'DialogTitle', {
}
});
-var _DialogContent = __webpack_require__(707);
+var _DialogContent = __webpack_require__(569);
Object.defineProperty(exports, 'DialogContent', {
enumerable: true,
@@ -5423,7 +3385,7 @@ Object.defineProperty(exports, 'DialogContent', {
}
});
-var _DialogContentText = __webpack_require__(708);
+var _DialogContentText = __webpack_require__(570);
Object.defineProperty(exports, 'DialogContentText', {
enumerable: true,
@@ -5432,7 +3394,7 @@ Object.defineProperty(exports, 'DialogContentText', {
}
});
-var _withMobileDialog = __webpack_require__(709);
+var _withMobileDialog = __webpack_require__(571);
Object.defineProperty(exports, 'withMobileDialog', {
enumerable: true,
@@ -5444,488 +3406,7 @@ Object.defineProperty(exports, 'withMobileDialog', {
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
/***/ }),
-/* 81 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-/* WEBPACK VAR INJECTION */(function(process) {/**
- * Copyright (c) 2014-present, Facebook, Inc.
- *
- * This source code is licensed under the MIT license found in the
- * LICENSE file in the root directory of this source tree.
- *
- */
-
-
-
-var _assign = __webpack_require__(21);
-
-var ReactCurrentOwner = __webpack_require__(50);
-
-var warning = __webpack_require__(9);
-var canDefineProperty = __webpack_require__(137);
-var hasOwnProperty = Object.prototype.hasOwnProperty;
-
-var REACT_ELEMENT_TYPE = __webpack_require__(362);
-
-var RESERVED_PROPS = {
- key: true,
- ref: true,
- __self: true,
- __source: true
-};
-
-var specialPropKeyWarningShown, specialPropRefWarningShown;
-
-function hasValidRef(config) {
- if (process.env.NODE_ENV !== 'production') {
- if (hasOwnProperty.call(config, 'ref')) {
- var getter = Object.getOwnPropertyDescriptor(config, 'ref').get;
- if (getter && getter.isReactWarning) {
- return false;
- }
- }
- }
- return config.ref !== undefined;
-}
-
-function hasValidKey(config) {
- if (process.env.NODE_ENV !== 'production') {
- if (hasOwnProperty.call(config, 'key')) {
- var getter = Object.getOwnPropertyDescriptor(config, 'key').get;
- if (getter && getter.isReactWarning) {
- return false;
- }
- }
- }
- return config.key !== undefined;
-}
-
-function defineKeyPropWarningGetter(props, displayName) {
- var warnAboutAccessingKey = function () {
- if (!specialPropKeyWarningShown) {
- specialPropKeyWarningShown = true;
- process.env.NODE_ENV !== 'production' ? warning(false, '%s: `key` is not a prop. Trying to access it will result ' + 'in `undefined` being returned. If you need to access the same ' + 'value within the child component, you should pass it as a different ' + 'prop. (https://fb.me/react-special-props)', displayName) : void 0;
- }
- };
- warnAboutAccessingKey.isReactWarning = true;
- Object.defineProperty(props, 'key', {
- get: warnAboutAccessingKey,
- configurable: true
- });
-}
-
-function defineRefPropWarningGetter(props, displayName) {
- var warnAboutAccessingRef = function () {
- if (!specialPropRefWarningShown) {
- specialPropRefWarningShown = true;
- process.env.NODE_ENV !== 'production' ? warning(false, '%s: `ref` is not a prop. Trying to access it will result ' + 'in `undefined` being returned. If you need to access the same ' + 'value within the child component, you should pass it as a different ' + 'prop. (https://fb.me/react-special-props)', displayName) : void 0;
- }
- };
- warnAboutAccessingRef.isReactWarning = true;
- Object.defineProperty(props, 'ref', {
- get: warnAboutAccessingRef,
- configurable: true
- });
-}
-
-/**
- * Factory method to create a new React element. This no longer adheres to
- * the class pattern, so do not use new to call it. Also, no instanceof check
- * will work. Instead test $$typeof field against Symbol.for('react.element') to check
- * if something is a React Element.
- *
- * @param {*} type
- * @param {*} key
- * @param {string|object} ref
- * @param {*} self A *temporary* helper to detect places where `this` is
- * different from the `owner` when React.createElement is called, so that we
- * can warn. We want to get rid of owner and replace string `ref`s with arrow
- * functions, and as long as `this` and owner are the same, there will be no
- * change in behavior.
- * @param {*} source An annotation object (added by a transpiler or otherwise)
- * indicating filename, line number, and/or other information.
- * @param {*} owner
- * @param {*} props
- * @internal
- */
-var ReactElement = function (type, key, ref, self, source, owner, props) {
- var element = {
- // This tag allow us to uniquely identify this as a React Element
- $$typeof: REACT_ELEMENT_TYPE,
-
- // Built-in properties that belong on the element
- type: type,
- key: key,
- ref: ref,
- props: props,
-
- // Record the component responsible for creating this element.
- _owner: owner
- };
-
- if (process.env.NODE_ENV !== 'production') {
- // The validation flag is currently mutative. We put it on
- // an external backing store so that we can freeze the whole object.
- // This can be replaced with a WeakMap once they are implemented in
- // commonly used development environments.
- element._store = {};
-
- // To make comparing ReactElements easier for testing purposes, we make
- // the validation flag non-enumerable (where possible, which should
- // include every environment we run tests in), so the test framework
- // ignores it.
- if (canDefineProperty) {
- Object.defineProperty(element._store, 'validated', {
- configurable: false,
- enumerable: false,
- writable: true,
- value: false
- });
- // self and source are DEV only properties.
- Object.defineProperty(element, '_self', {
- configurable: false,
- enumerable: false,
- writable: false,
- value: self
- });
- // Two elements created in two different places should be considered
- // equal for testing purposes and therefore we hide it from enumeration.
- Object.defineProperty(element, '_source', {
- configurable: false,
- enumerable: false,
- writable: false,
- value: source
- });
- } else {
- element._store.validated = false;
- element._self = self;
- element._source = source;
- }
- if (Object.freeze) {
- Object.freeze(element.props);
- Object.freeze(element);
- }
- }
-
- return element;
-};
-
-/**
- * Create and return a new ReactElement of the given type.
- * See https://facebook.github.io/react/docs/top-level-api.html#react.createelement
- */
-ReactElement.createElement = function (type, config, children) {
- var propName;
-
- // Reserved names are extracted
- var props = {};
-
- var key = null;
- var ref = null;
- var self = null;
- var source = null;
-
- if (config != null) {
- if (hasValidRef(config)) {
- ref = config.ref;
- }
- if (hasValidKey(config)) {
- key = '' + config.key;
- }
-
- self = config.__self === undefined ? null : config.__self;
- source = config.__source === undefined ? null : config.__source;
- // Remaining properties are added to a new props object
- for (propName in config) {
- if (hasOwnProperty.call(config, propName) && !RESERVED_PROPS.hasOwnProperty(propName)) {
- props[propName] = config[propName];
- }
- }
- }
-
- // Children can be more than one argument, and those are transferred onto
- // the newly allocated props object.
- var childrenLength = arguments.length - 2;
- if (childrenLength === 1) {
- props.children = children;
- } else if (childrenLength > 1) {
- var childArray = Array(childrenLength);
- for (var i = 0; i < childrenLength; i++) {
- childArray[i] = arguments[i + 2];
- }
- if (process.env.NODE_ENV !== 'production') {
- if (Object.freeze) {
- Object.freeze(childArray);
- }
- }
- props.children = childArray;
- }
-
- // Resolve default props
- if (type && type.defaultProps) {
- var defaultProps = type.defaultProps;
- for (propName in defaultProps) {
- if (props[propName] === undefined) {
- props[propName] = defaultProps[propName];
- }
- }
- }
- if (process.env.NODE_ENV !== 'production') {
- if (key || ref) {
- if (typeof props.$$typeof === 'undefined' || props.$$typeof !== REACT_ELEMENT_TYPE) {
- var displayName = typeof type === 'function' ? type.displayName || type.name || 'Unknown' : type;
- if (key) {
- defineKeyPropWarningGetter(props, displayName);
- }
- if (ref) {
- defineRefPropWarningGetter(props, displayName);
- }
- }
- }
- }
- return ReactElement(type, key, ref, self, source, ReactCurrentOwner.current, props);
-};
-
-/**
- * Return a function that produces ReactElements of a given type.
- * See https://facebook.github.io/react/docs/top-level-api.html#react.createfactory
- */
-ReactElement.createFactory = function (type) {
- var factory = ReactElement.createElement.bind(null, type);
- // Expose the type on the factory and the prototype so that it can be
- // easily accessed on elements. E.g. ` .type === Foo`.
- // This should not be named `constructor` since this may not be the function
- // that created the element, and it may not even be a constructor.
- // Legacy hook TODO: Warn if this is accessed
- factory.type = type;
- return factory;
-};
-
-ReactElement.cloneAndReplaceKey = function (oldElement, newKey) {
- var newElement = ReactElement(oldElement.type, newKey, oldElement.ref, oldElement._self, oldElement._source, oldElement._owner, oldElement.props);
-
- return newElement;
-};
-
-/**
- * Clone and return a new ReactElement using element as the starting point.
- * See https://facebook.github.io/react/docs/top-level-api.html#react.cloneelement
- */
-ReactElement.cloneElement = function (element, config, children) {
- var propName;
-
- // Original props are copied
- var props = _assign({}, element.props);
-
- // Reserved names are extracted
- var key = element.key;
- var ref = element.ref;
- // Self is preserved since the owner is preserved.
- var self = element._self;
- // Source is preserved since cloneElement is unlikely to be targeted by a
- // transpiler, and the original source is probably a better indicator of the
- // true owner.
- var source = element._source;
-
- // Owner will be preserved, unless ref is overridden
- var owner = element._owner;
-
- if (config != null) {
- if (hasValidRef(config)) {
- // Silently steal the ref from the parent.
- ref = config.ref;
- owner = ReactCurrentOwner.current;
- }
- if (hasValidKey(config)) {
- key = '' + config.key;
- }
-
- // Remaining properties override existing props
- var defaultProps;
- if (element.type && element.type.defaultProps) {
- defaultProps = element.type.defaultProps;
- }
- for (propName in config) {
- if (hasOwnProperty.call(config, propName) && !RESERVED_PROPS.hasOwnProperty(propName)) {
- if (config[propName] === undefined && defaultProps !== undefined) {
- // Resolve default props
- props[propName] = defaultProps[propName];
- } else {
- props[propName] = config[propName];
- }
- }
- }
- }
-
- // Children can be more than one argument, and those are transferred onto
- // the newly allocated props object.
- var childrenLength = arguments.length - 2;
- if (childrenLength === 1) {
- props.children = children;
- } else if (childrenLength > 1) {
- var childArray = Array(childrenLength);
- for (var i = 0; i < childrenLength; i++) {
- childArray[i] = arguments[i + 2];
- }
- props.children = childArray;
- }
-
- return ReactElement(element.type, key, ref, self, source, owner, props);
-};
-
-/**
- * Verifies the object is a ReactElement.
- * See https://facebook.github.io/react/docs/top-level-api.html#react.isvalidelement
- * @param {?object} object
- * @return {boolean} True if `object` is a valid component.
- * @final
- */
-ReactElement.isValidElement = function (object) {
- return typeof object === 'object' && object !== null && object.$$typeof === REACT_ELEMENT_TYPE;
-};
-
-module.exports = ReactElement;
-/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
-
-/***/ }),
-/* 82 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-/* WEBPACK VAR INJECTION */(function(process) {/**
- * Copyright (c) 2013-present, Facebook, Inc.
- *
- * This source code is licensed under the MIT license found in the
- * LICENSE file in the root directory of this source tree.
- *
- *
- */
-
-
-
-var _prodInvariant = __webpack_require__(19);
-
-var invariant = __webpack_require__(8);
-
-/**
- * Static poolers. Several custom versions for each potential number of
- * arguments. A completely generic pooler is easy to implement, but would
- * require accessing the `arguments` object. In each of these, `this` refers to
- * the Class itself, not an instance. If any others are needed, simply add them
- * here, or in their own files.
- */
-var oneArgumentPooler = function (copyFieldsFrom) {
- var Klass = this;
- if (Klass.instancePool.length) {
- var instance = Klass.instancePool.pop();
- Klass.call(instance, copyFieldsFrom);
- return instance;
- } else {
- return new Klass(copyFieldsFrom);
- }
-};
-
-var twoArgumentPooler = function (a1, a2) {
- var Klass = this;
- if (Klass.instancePool.length) {
- var instance = Klass.instancePool.pop();
- Klass.call(instance, a1, a2);
- return instance;
- } else {
- return new Klass(a1, a2);
- }
-};
-
-var threeArgumentPooler = function (a1, a2, a3) {
- var Klass = this;
- if (Klass.instancePool.length) {
- var instance = Klass.instancePool.pop();
- Klass.call(instance, a1, a2, a3);
- return instance;
- } else {
- return new Klass(a1, a2, a3);
- }
-};
-
-var fourArgumentPooler = function (a1, a2, a3, a4) {
- var Klass = this;
- if (Klass.instancePool.length) {
- var instance = Klass.instancePool.pop();
- Klass.call(instance, a1, a2, a3, a4);
- return instance;
- } else {
- return new Klass(a1, a2, a3, a4);
- }
-};
-
-var standardReleaser = function (instance) {
- var Klass = this;
- !(instance instanceof Klass) ? process.env.NODE_ENV !== 'production' ? invariant(false, 'Trying to release an instance into a pool of a different type.') : _prodInvariant('25') : void 0;
- instance.destructor();
- if (Klass.instancePool.length < Klass.poolSize) {
- Klass.instancePool.push(instance);
- }
-};
-
-var DEFAULT_POOL_SIZE = 10;
-var DEFAULT_POOLER = oneArgumentPooler;
-
-/**
- * Augments `CopyConstructor` to be a poolable class, augmenting only the class
- * itself (statically) not adding any prototypical fields. Any CopyConstructor
- * you give this may have a `poolSize` property, and will look for a
- * prototypical `destructor` on instances.
- *
- * @param {Function} CopyConstructor Constructor that can be used to reset.
- * @param {Function} pooler Customizable pooler.
- */
-var addPoolingTo = function (CopyConstructor, pooler) {
- // Casting as any so that flow ignores the actual implementation and trusts
- // it to match the type we declared
- var NewKlass = CopyConstructor;
- NewKlass.instancePool = [];
- NewKlass.getPooled = pooler || DEFAULT_POOLER;
- if (!NewKlass.poolSize) {
- NewKlass.poolSize = DEFAULT_POOL_SIZE;
- }
- NewKlass.release = standardReleaser;
- return NewKlass;
-};
-
-var PooledClass = {
- addPoolingTo: addPoolingTo,
- oneArgumentPooler: oneArgumentPooler,
- twoArgumentPooler: twoArgumentPooler,
- threeArgumentPooler: threeArgumentPooler,
- fourArgumentPooler: fourArgumentPooler
-};
-
-module.exports = PooledClass;
-/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
-
-/***/ }),
-/* 83 */
-/***/ (function(module, exports, __webpack_require__) {
-
-// Thank's IE8 for his funny defineProperty
-module.exports = !__webpack_require__(100)(function () {
- return Object.defineProperty({}, 'a', { get: function () { return 7; } }).a != 7;
-});
-
-
-/***/ }),
-/* 84 */
-/***/ (function(module, exports) {
-
-var hasOwnProperty = {}.hasOwnProperty;
-module.exports = function (it, key) {
- return hasOwnProperty.call(it, key);
-};
-
-
-/***/ }),
-/* 85 */
+/* 55 */
/***/ (function(module, exports, __webpack_require__) {
/* WEBPACK VAR INJECTION */(function(process) {/**
@@ -5950,40 +3431,40 @@ if (process.env.NODE_ENV !== 'production') {
// By explicitly using `prop-types` you are opting into new development behavior.
// http://fb.me/prop-types-in-prod
var throwOnDirectAccess = true;
- module.exports = __webpack_require__(471)(isValidElement, throwOnDirectAccess);
+ module.exports = __webpack_require__(333)(isValidElement, throwOnDirectAccess);
} else {
// By explicitly using `prop-types` you are opting into new production behavior.
// http://fb.me/prop-types-in-prod
- module.exports = __webpack_require__(474)();
+ module.exports = __webpack_require__(336)();
}
/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
/***/ }),
-/* 86 */
+/* 56 */
/***/ (function(module, exports, __webpack_require__) {
// to indexed object, toObject with fallback for non-array-like ES3 strings
-var IObject = __webpack_require__(167);
-var defined = __webpack_require__(169);
+var IObject = __webpack_require__(105);
+var defined = __webpack_require__(107);
module.exports = function (it) {
return IObject(defined(it));
};
/***/ }),
-/* 87 */
+/* 57 */
/***/ (function(module, exports, __webpack_require__) {
// 7.1.13 ToObject(argument)
-var defined = __webpack_require__(169);
+var defined = __webpack_require__(107);
module.exports = function (it) {
return Object(defined(it));
};
/***/ }),
-/* 88 */
+/* 58 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -5991,7 +3472,7 @@ module.exports = function (it) {
exports.__esModule = true;
-var _getDisplayName = __webpack_require__(318);
+var _getDisplayName = __webpack_require__(227);
var _getDisplayName2 = _interopRequireDefault(_getDisplayName);
@@ -6004,7 +3485,7 @@ var wrapDisplayName = function wrapDisplayName(BaseComponent, hocName) {
exports.default = wrapDisplayName;
/***/ }),
-/* 89 */
+/* 59 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -6015,7 +3496,7 @@ Object.defineProperty(exports, "__esModule", {
});
exports.isNumber = exports.isString = exports.formatMs = exports.duration = exports.easing = undefined;
-var _keys = __webpack_require__(40);
+var _keys = __webpack_require__(31);
var _keys2 = _interopRequireDefault(_keys);
@@ -6023,11 +3504,11 @@ var _objectWithoutProperties2 = __webpack_require__(4);
var _objectWithoutProperties3 = _interopRequireDefault(_objectWithoutProperties2);
-var _isNan = __webpack_require__(336);
+var _isNan = __webpack_require__(245);
var _isNan2 = _interopRequireDefault(_isNan);
-var _warning = __webpack_require__(17);
+var _warning = __webpack_require__(15);
var _warning2 = _interopRequireDefault(_warning);
@@ -6123,7 +3604,7 @@ exports.default = {
/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
/***/ }),
-/* 90 */
+/* 60 */
/***/ (function(module, exports) {
// Source: http://jsfiddle.net/vWx8V/
@@ -6275,7 +3756,7 @@ for (var alias in aliases) {
/***/ }),
-/* 91 */
+/* 61 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -6310,7 +3791,7 @@ function isMuiComponent(element, muiNames) {
}
/***/ }),
-/* 92 */
+/* 62 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
@@ -6320,7 +3801,7 @@ Object.defineProperty(exports, "__esModule", {
value: true
});
-var _List = __webpack_require__(694);
+var _List = __webpack_require__(556);
Object.defineProperty(exports, 'default', {
enumerable: true,
@@ -6329,7 +3810,7 @@ Object.defineProperty(exports, 'default', {
}
});
-var _ListItem = __webpack_require__(353);
+var _ListItem = __webpack_require__(262);
Object.defineProperty(exports, 'ListItem', {
enumerable: true,
@@ -6338,7 +3819,7 @@ Object.defineProperty(exports, 'ListItem', {
}
});
-var _ListItemAvatar = __webpack_require__(695);
+var _ListItemAvatar = __webpack_require__(557);
Object.defineProperty(exports, 'ListItemAvatar', {
enumerable: true,
@@ -6347,7 +3828,7 @@ Object.defineProperty(exports, 'ListItemAvatar', {
}
});
-var _ListItemText = __webpack_require__(696);
+var _ListItemText = __webpack_require__(558);
Object.defineProperty(exports, 'ListItemText', {
enumerable: true,
@@ -6356,7 +3837,7 @@ Object.defineProperty(exports, 'ListItemText', {
}
});
-var _ListItemIcon = __webpack_require__(697);
+var _ListItemIcon = __webpack_require__(559);
Object.defineProperty(exports, 'ListItemIcon', {
enumerable: true,
@@ -6365,7 +3846,7 @@ Object.defineProperty(exports, 'ListItemIcon', {
}
});
-var _ListItemSecondaryAction = __webpack_require__(698);
+var _ListItemSecondaryAction = __webpack_require__(560);
Object.defineProperty(exports, 'ListItemSecondaryAction', {
enumerable: true,
@@ -6374,7 +3855,7 @@ Object.defineProperty(exports, 'ListItemSecondaryAction', {
}
});
-var _ListSubheader = __webpack_require__(699);
+var _ListSubheader = __webpack_require__(561);
Object.defineProperty(exports, 'ListSubheader', {
enumerable: true,
@@ -6386,1069 +3867,87 @@ Object.defineProperty(exports, 'ListSubheader', {
function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
/***/ }),
-/* 93 */
+/* 63 */
/***/ (function(module, exports, __webpack_require__) {
"use strict";
-/* WEBPACK VAR INJECTION */(function(process) {/**
- * Copyright (c) 2013-present, Facebook, Inc.
- *
- * This source code is licensed under the MIT license found in the
- * LICENSE file in the root directory of this source tree.
- *
- */
+/* WEBPACK VAR INJECTION */(function(process) {
+exports.__esModule = true;
+var _extends = Object.assign || function (target) { for (var i = 1; i < arguments.length; i++) { var source = arguments[i]; for (var key in source) { if (Object.prototype.hasOwnProperty.call(source, key)) { target[key] = source[key]; } } } return target; };
-var _assign = __webpack_require__(21);
+exports.bottom = bottom;
+exports.cloneLayout = cloneLayout;
+exports.cloneLayoutItem = cloneLayoutItem;
+exports.childrenEqual = childrenEqual;
+exports.collides = collides;
+exports.compact = compact;
+exports.compactItem = compactItem;
+exports.correctBounds = correctBounds;
+exports.getLayoutItem = getLayoutItem;
+exports.getFirstCollision = getFirstCollision;
+exports.getAllCollisions = getAllCollisions;
+exports.getStatics = getStatics;
+exports.moveElement = moveElement;
+exports.moveElementAwayFromCollision = moveElementAwayFromCollision;
+exports.perc = perc;
+exports.setTransform = setTransform;
+exports.setTopLeft = setTopLeft;
+exports.sortLayoutItems = sortLayoutItems;
+exports.sortLayoutItemsByRowCol = sortLayoutItemsByRowCol;
+exports.sortLayoutItemsByColRow = sortLayoutItemsByColRow;
+exports.synchronizeLayoutWithChildren = synchronizeLayoutWithChildren;
+exports.validateLayout = validateLayout;
+exports.autoBindHandlers = autoBindHandlers;
-var ReactBaseClasses = __webpack_require__(360);
-var ReactChildren = __webpack_require__(734);
-var ReactDOMFactories = __webpack_require__(738);
-var ReactElement = __webpack_require__(81);
-var ReactPropTypes = __webpack_require__(742);
-var ReactVersion = __webpack_require__(744);
+var _lodash = __webpack_require__(94);
-var createReactClass = __webpack_require__(745);
-var onlyChild = __webpack_require__(747);
+var _lodash2 = _interopRequireDefault(_lodash);
-var createElement = ReactElement.createElement;
-var createFactory = ReactElement.createFactory;
-var cloneElement = ReactElement.cloneElement;
+var _react = __webpack_require__(1);
-if (process.env.NODE_ENV !== 'production') {
- var lowPriorityWarning = __webpack_require__(205);
- var canDefineProperty = __webpack_require__(137);
- var ReactElementValidator = __webpack_require__(364);
- var didWarnPropTypesDeprecated = false;
- createElement = ReactElementValidator.createElement;
- createFactory = ReactElementValidator.createFactory;
- cloneElement = ReactElementValidator.cloneElement;
-}
+var _react2 = _interopRequireDefault(_react);
-var __spread = _assign;
-var createMixin = function (mixin) {
- return mixin;
-};
+function _interopRequireDefault(obj) { return obj && obj.__esModule ? obj : { default: obj }; }
-if (process.env.NODE_ENV !== 'production') {
- var warnedForSpread = false;
- var warnedForCreateMixin = false;
- __spread = function () {
- lowPriorityWarning(warnedForSpread, 'React.__spread is deprecated and should not be used. Use ' + 'Object.assign directly or another helper function with similar ' + 'semantics. You may be seeing this warning due to your compiler. ' + 'See https://fb.me/react-spread-deprecation for more details.');
- warnedForSpread = true;
- return _assign.apply(null, arguments);
- };
+// All callbacks are of the signature (layout, oldItem, newItem, placeholder, e).
+var isProduction = process.env.NODE_ENV === 'production';
- createMixin = function (mixin) {
- lowPriorityWarning(warnedForCreateMixin, 'React.createMixin is deprecated and should not be used. ' + 'In React v16.0, it will be removed. ' + 'You can use this mixin directly instead. ' + 'See https://fb.me/createmixin-was-never-implemented for more info.');
- warnedForCreateMixin = true;
- return mixin;
- };
+/**
+ * Return the bottom coordinate of the layout.
+ *
+ * @param {Array} layout Layout array.
+ * @return {Number} Bottom coordinate.
+ */
+function bottom(layout) {
+ var max = 0,
+ bottomY = void 0;
+ for (var _i = 0, len = layout.length; _i < len; _i++) {
+ bottomY = layout[_i].y + layout[_i].h;
+ if (bottomY > max) max = bottomY;
+ }
+ return max;
}
-var React = {
- // Modern
-
- Children: {
- map: ReactChildren.map,
- forEach: ReactChildren.forEach,
- count: ReactChildren.count,
- toArray: ReactChildren.toArray,
- only: onlyChild
- },
-
- Component: ReactBaseClasses.Component,
- PureComponent: ReactBaseClasses.PureComponent,
-
- createElement: createElement,
- cloneElement: cloneElement,
- isValidElement: ReactElement.isValidElement,
-
- // Classic
-
- PropTypes: ReactPropTypes,
- createClass: createReactClass,
- createFactory: createFactory,
- createMixin: createMixin,
-
- // This looks DOM specific but these are actually isomorphic helpers
- // since they are just generating DOM strings.
- DOM: ReactDOMFactories,
-
- version: ReactVersion,
-
- // Deprecated hook for JSX spread, don't use this for anything.
- __spread: __spread
-};
-
-if (process.env.NODE_ENV !== 'production') {
- var warnedForCreateClass = false;
- if (canDefineProperty) {
- Object.defineProperty(React, 'PropTypes', {
- get: function () {
- lowPriorityWarning(didWarnPropTypesDeprecated, 'Accessing PropTypes via the main React package is deprecated,' + ' and will be removed in React v16.0.' + ' Use the latest available v15.* prop-types package from npm instead.' + ' For info on usage, compatibility, migration and more, see ' + 'https://fb.me/prop-types-docs');
- didWarnPropTypesDeprecated = true;
- return ReactPropTypes;
- }
- });
-
- Object.defineProperty(React, 'createClass', {
- get: function () {
- lowPriorityWarning(warnedForCreateClass, 'Accessing createClass via the main React package is deprecated,' + ' and will be removed in React v16.0.' + " Use a plain JavaScript class instead. If you're not yet " + 'ready to migrate, create-react-class v15.* is available ' + 'on npm as a temporary, drop-in replacement. ' + 'For more info see https://fb.me/react-create-class');
- warnedForCreateClass = true;
- return createReactClass;
- }
- });
+function cloneLayout(layout) {
+ var newLayout = Array(layout.length);
+ for (var _i2 = 0, len = layout.length; _i2 < len; _i2++) {
+ newLayout[_i2] = cloneLayoutItem(layout[_i2]);
}
-
- // React.DOM factories are deprecated. Wrap these methods so that
- // invocations of the React.DOM namespace and alert users to switch
- // to the `react-dom-factories` package.
- React.DOM = {};
- var warnedForFactories = false;
- Object.keys(ReactDOMFactories).forEach(function (factory) {
- React.DOM[factory] = function () {
- if (!warnedForFactories) {
- lowPriorityWarning(false, 'Accessing factories like React.DOM.%s has been deprecated ' + 'and will be removed in v16.0+. Use the ' + 'react-dom-factories package instead. ' + ' Version 1.0 provides a drop-in replacement.' + ' For more info, see https://fb.me/react-dom-factories', factory);
- warnedForFactories = true;
- }
- return ReactDOMFactories[factory].apply(ReactDOMFactories, arguments);
- };
- });
+ return newLayout;
}
-module.exports = React;
-/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
-
-/***/ }),
-/* 94 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-/**
- * Copyright (c) 2013-present, Facebook, Inc.
- *
- * This source code is licensed under the MIT license found in the
- * LICENSE file in the root directory of this source tree.
- *
- *
- */
-
-
-/**
- * WARNING: DO NOT manually require this module.
- * This is a replacement for `invariant(...)` used by the error code system
- * and will _only_ be required by the corresponding babel pass.
- * It always throws.
- */
-
-function reactProdInvariant(code) {
- var argCount = arguments.length - 1;
-
- var message = 'Minified React error #' + code + '; visit ' + 'http://facebook.github.io/react/docs/error-decoder.html?invariant=' + code;
-
- for (var argIdx = 0; argIdx < argCount; argIdx++) {
- message += '&args[]=' + encodeURIComponent(arguments[argIdx + 1]);
- }
-
- message += ' for the full message or use the non-minified dev environment' + ' for full errors and additional helpful warnings.';
-
- var error = new Error(message);
- error.name = 'Invariant Violation';
- error.framesToPop = 1; // we don't care about reactProdInvariant's own frame
-
- throw error;
-}
-
-module.exports = reactProdInvariant;
-
-/***/ }),
-/* 95 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-/* WEBPACK VAR INJECTION */(function(process) {/**
- * Copyright (c) 2013-present, Facebook, Inc.
- *
- * This source code is licensed under the MIT license found in the
- * LICENSE file in the root directory of this source tree.
- *
- */
-
-
-
-var ReactRef = __webpack_require__(757);
-var ReactInstrumentation = __webpack_require__(37);
-
-var warning = __webpack_require__(9);
-
-/**
- * Helper to call ReactRef.attachRefs with this composite component, split out
- * to avoid allocations in the transaction mount-ready queue.
- */
-function attachRefs() {
- ReactRef.attachRefs(this, this._currentElement);
-}
-
-var ReactReconciler = {
- /**
- * Initializes the component, renders markup, and registers event listeners.
- *
- * @param {ReactComponent} internalInstance
- * @param {ReactReconcileTransaction|ReactServerRenderingTransaction} transaction
- * @param {?object} the containing host component instance
- * @param {?object} info about the host container
- * @return {?string} Rendered markup to be inserted into the DOM.
- * @final
- * @internal
- */
- mountComponent: function (internalInstance, transaction, hostParent, hostContainerInfo, context, parentDebugID) // 0 in production and for roots
- {
- if (process.env.NODE_ENV !== 'production') {
- if (internalInstance._debugID !== 0) {
- ReactInstrumentation.debugTool.onBeforeMountComponent(internalInstance._debugID, internalInstance._currentElement, parentDebugID);
- }
- }
- var markup = internalInstance.mountComponent(transaction, hostParent, hostContainerInfo, context, parentDebugID);
- if (internalInstance._currentElement && internalInstance._currentElement.ref != null) {
- transaction.getReactMountReady().enqueue(attachRefs, internalInstance);
- }
- if (process.env.NODE_ENV !== 'production') {
- if (internalInstance._debugID !== 0) {
- ReactInstrumentation.debugTool.onMountComponent(internalInstance._debugID);
- }
- }
- return markup;
- },
-
- /**
- * Returns a value that can be passed to
- * ReactComponentEnvironment.replaceNodeWithMarkup.
- */
- getHostNode: function (internalInstance) {
- return internalInstance.getHostNode();
- },
-
- /**
- * Releases any resources allocated by `mountComponent`.
- *
- * @final
- * @internal
- */
- unmountComponent: function (internalInstance, safely) {
- if (process.env.NODE_ENV !== 'production') {
- if (internalInstance._debugID !== 0) {
- ReactInstrumentation.debugTool.onBeforeUnmountComponent(internalInstance._debugID);
- }
- }
- ReactRef.detachRefs(internalInstance, internalInstance._currentElement);
- internalInstance.unmountComponent(safely);
- if (process.env.NODE_ENV !== 'production') {
- if (internalInstance._debugID !== 0) {
- ReactInstrumentation.debugTool.onUnmountComponent(internalInstance._debugID);
- }
- }
- },
-
- /**
- * Update a component using a new element.
- *
- * @param {ReactComponent} internalInstance
- * @param {ReactElement} nextElement
- * @param {ReactReconcileTransaction} transaction
- * @param {object} context
- * @internal
- */
- receiveComponent: function (internalInstance, nextElement, transaction, context) {
- var prevElement = internalInstance._currentElement;
-
- if (nextElement === prevElement && context === internalInstance._context) {
- // Since elements are immutable after the owner is rendered,
- // we can do a cheap identity compare here to determine if this is a
- // superfluous reconcile. It's possible for state to be mutable but such
- // change should trigger an update of the owner which would recreate
- // the element. We explicitly check for the existence of an owner since
- // it's possible for an element created outside a composite to be
- // deeply mutated and reused.
-
- // TODO: Bailing out early is just a perf optimization right?
- // TODO: Removing the return statement should affect correctness?
- return;
- }
-
- if (process.env.NODE_ENV !== 'production') {
- if (internalInstance._debugID !== 0) {
- ReactInstrumentation.debugTool.onBeforeUpdateComponent(internalInstance._debugID, nextElement);
- }
- }
-
- var refsChanged = ReactRef.shouldUpdateRefs(prevElement, nextElement);
-
- if (refsChanged) {
- ReactRef.detachRefs(internalInstance, prevElement);
- }
-
- internalInstance.receiveComponent(nextElement, transaction, context);
-
- if (refsChanged && internalInstance._currentElement && internalInstance._currentElement.ref != null) {
- transaction.getReactMountReady().enqueue(attachRefs, internalInstance);
- }
-
- if (process.env.NODE_ENV !== 'production') {
- if (internalInstance._debugID !== 0) {
- ReactInstrumentation.debugTool.onUpdateComponent(internalInstance._debugID);
- }
- }
- },
-
- /**
- * Flush any dirty changes in a component.
- *
- * @param {ReactComponent} internalInstance
- * @param {ReactReconcileTransaction} transaction
- * @internal
- */
- performUpdateIfNecessary: function (internalInstance, transaction, updateBatchNumber) {
- if (internalInstance._updateBatchNumber !== updateBatchNumber) {
- // The component's enqueued batch number should always be the current
- // batch or the following one.
- process.env.NODE_ENV !== 'production' ? warning(internalInstance._updateBatchNumber == null || internalInstance._updateBatchNumber === updateBatchNumber + 1, 'performUpdateIfNecessary: Unexpected batch number (current %s, ' + 'pending %s)', updateBatchNumber, internalInstance._updateBatchNumber) : void 0;
- return;
- }
- if (process.env.NODE_ENV !== 'production') {
- if (internalInstance._debugID !== 0) {
- ReactInstrumentation.debugTool.onBeforeUpdateComponent(internalInstance._debugID, internalInstance._currentElement);
- }
- }
- internalInstance.performUpdateIfNecessary(transaction);
- if (process.env.NODE_ENV !== 'production') {
- if (internalInstance._debugID !== 0) {
- ReactInstrumentation.debugTool.onUpdateComponent(internalInstance._debugID);
- }
- }
- }
-};
-
-module.exports = ReactReconciler;
-/* WEBPACK VAR INJECTION */}.call(exports, __webpack_require__(2)))
-
-/***/ }),
-/* 96 */
-/***/ (function(module, exports, __webpack_require__) {
-
-"use strict";
-/**
- * Copyright (c) 2015-present, Facebook, Inc.
- *
- * This source code is licensed under the MIT license found in the
- * LICENSE file in the root directory of this source tree.
- *
- */
-
-
-
-var DOMNamespaces = __webpack_require__(213);
-var setInnerHTML = __webpack_require__(142);
-
-var createMicrosoftUnsafeLocalFunction = __webpack_require__(214);
-var setTextContent = __webpack_require__(376);
-
-var ELEMENT_NODE_TYPE = 1;
-var DOCUMENT_FRAGMENT_NODE_TYPE = 11;
-
-/**
- * In IE (8-11) and Edge, appending nodes with no children is dramatically
- * faster than appending a full subtree, so we essentially queue up the
- * .appendChild calls here and apply them so each node is added to its parent
- * before any children are added.
- *
- * In other browsers, doing so is slower or neutral compared to the other order
- * (in Firefox, twice as slow) so we only do this inversion in IE.
- *
- * See https://github.com/spicyj/innerhtml-vs-createelement-vs-clonenode.
- */
-var enableLazy = typeof document !== 'undefined' && typeof document.documentMode === 'number' || typeof navigator !== 'undefined' && typeof navigator.userAgent === 'string' && /\bEdge\/\d/.test(navigator.userAgent);
-
-function insertTreeChildren(tree) {
- if (!enableLazy) {
- return;
- }
- var node = tree.node;
- var children = tree.children;
- if (children.length) {
- for (var i = 0; i < children.length; i++) {
- insertTreeBefore(node, children[i], null);
- }
- } else if (tree.html != null) {
- setInnerHTML(node, tree.html);
- } else if (tree.text != null) {
- setTextContent(node, tree.text);
- }
-}
-
-var insertTreeBefore = createMicrosoftUnsafeLocalFunction(function (parentNode, tree, referenceNode) {
- // DocumentFragments aren't actually part of the DOM after insertion so
- // appending children won't update the DOM. We need to ensure the fragment
- // is properly populated first, breaking out of our lazy approach for just
- // this level. Also, some plugins (like Flash Player) will read
- // nodes immediately upon insertion into the DOM, so
- // must also be populated prior to insertion into the DOM.
- if (tree.node.nodeType === DOCUMENT_FRAGMENT_NODE_TYPE || tree.node.nodeType === ELEMENT_NODE_TYPE && tree.node.nodeName.toLowerCase() === 'object' && (tree.node.namespaceURI == null || tree.node.namespaceURI === DOMNamespaces.html)) {
- insertTreeChildren(tree);
- parentNode.insertBefore(tree.node, referenceNode);
- } else {
- parentNode.insertBefore(tree.node, referenceNode);
- insertTreeChildren(tree);
- }
-});
-
-function replaceChildWithTree(oldNode, newTree) {
- oldNode.parentNode.replaceChild(newTree.node, oldNode);
- insertTreeChildren(newTree);
-}
-
-function queueChild(parentTree, childTree) {
- if (enableLazy) {
- parentTree.children.push(childTree);
- } else {
- parentTree.node.appendChild(childTree.node);
- }
-}
-
-function queueHTML(tree, html) {
- if (enableLazy) {
- tree.html = html;
- } else {
- setInnerHTML(tree.node, html);
- }
-}
-
-function queueText(tree, text) {
- if (enableLazy) {
- tree.text = text;
- } else {
- setTextContent(tree.node, text);
- }
-}
-
-function toString() {
- return this.node.nodeName;
-}
-
-function DOMLazyTree(node) {
- return {
- node: node,
- children: [],
- html: null,
- text: null,
- toString: toString
- };
-}
-
-DOMLazyTree.insertTreeBefore = insertTreeBefore;
-DOMLazyTree.replaceChildWithTree = replaceChildWithTree;
-DOMLazyTree.queueChild = queueChild;
-DOMLazyTree.queueHTML = queueHTML;
-DOMLazyTree.queueText = queueText;
-
-module.exports = DOMLazyTree;
-
-/***/ }),
-/* 97 */
-/***/ (function(module, exports, __webpack_require__) {
-
-var dP = __webpack_require__(66);
-var createDesc = __webpack_require__(116);
-module.exports = __webpack_require__(83) ? function (object, key, value) {
- return dP.f(object, key, createDesc(1, value));
-} : function (object, key, value) {
- object[key] = value;
- return object;
-};
-
-
-/***/ }),
-/* 98 */
-/***/ (function(module, exports, __webpack_require__) {
-
-var isObject = __webpack_require__(99);
-module.exports = function (it) {
- if (!isObject(it)) throw TypeError(it + ' is not an object!');
- return it;
-};
-
-
-/***/ }),
-/* 99 */
-/***/ (function(module, exports) {
-
-module.exports = function (it) {
- return typeof it === 'object' ? it !== null : typeof it === 'function';
-};
-
-
-/***/ }),
-/* 100 */
-/***/ (function(module, exports) {
-
-module.exports = function (exec) {
- try {
- return !!exec();
- } catch (e) {
- return true;
- }
-};
-
-
-/***/ }),
-/* 101 */
-/***/ (function(module, exports, __webpack_require__) {
-
-// to indexed object, toObject with fallback for non-array-like ES3 strings
-var IObject = __webpack_require__(395);
-var defined = __webpack_require__(225);
-module.exports = function (it) {
- return IObject(defined(it));
-};
-
-
-/***/ }),
-/* 102 */
-/***/ (function(module, exports) {
-
-/*
- MIT License http://www.opensource.org/licenses/mit-license.php
- Author Tobias Koppers @sokra
-*/
-// css base code, injected by the css-loader
-module.exports = function(useSourceMap) {
- var list = [];
-
- // return the list of modules as css string
- list.toString = function toString() {
- return this.map(function (item) {
- var content = cssWithMappingToString(item, useSourceMap);
- if(item[2]) {
- return "@media " + item[2] + "{" + content + "}";
- } else {
- return content;
- }
- }).join("");
- };
-
- // import a list of modules into the list
- list.i = function(modules, mediaQuery) {
- if(typeof modules === "string")
- modules = [[null, modules, ""]];
- var alreadyImportedModules = {};
- for(var i = 0; i < this.length; i++) {
- var id = this[i][0];
- if(typeof id === "number")
- alreadyImportedModules[id] = true;
- }
- for(i = 0; i < modules.length; i++) {
- var item = modules[i];
- // skip already imported module
- // this implementation is not 100% perfect for weird media query combinations
- // when a module is imported multiple times with different media queries.
- // I hope this will never occur (Hey this way we have smaller bundles)
- if(typeof item[0] !== "number" || !alreadyImportedModules[item[0]]) {
- if(mediaQuery && !item[2]) {
- item[2] = mediaQuery;
- } else if(mediaQuery) {
- item[2] = "(" + item[2] + ") and (" + mediaQuery + ")";
- }
- list.push(item);
- }
- }
- };
- return list;
-};
-
-function cssWithMappingToString(item, useSourceMap) {
- var content = item[1] || '';
- var cssMapping = item[3];
- if (!cssMapping) {
- return content;
- }
-
- if (useSourceMap && typeof btoa === 'function') {
- var sourceMapping = toComment(cssMapping);
- var sourceURLs = cssMapping.sources.map(function (source) {
- return '/*# sourceURL=' + cssMapping.sourceRoot + source + ' */'
- });
-
- return [content].concat(sourceURLs).concat([sourceMapping]).join('\n');
- }
-
- return [content].join('\n');
-}
-
-// Adapted from convert-source-map (MIT)
-function toComment(sourceMap) {
- // eslint-disable-next-line no-undef
- var base64 = btoa(unescape(encodeURIComponent(JSON.stringify(sourceMap))));
- var data = 'sourceMappingURL=data:application/json;charset=utf-8;base64,' + base64;
-
- return '/*# ' + data + ' */';
-}
-
-
-/***/ }),
-/* 103 */
-/***/ (function(module, exports, __webpack_require__) {
-
-/*
- MIT License http://www.opensource.org/licenses/mit-license.php
- Author Tobias Koppers @sokra
-*/
-
-var stylesInDom = {};
-
-var memoize = function (fn) {
- var memo;
-
- return function () {
- if (typeof memo === "undefined") memo = fn.apply(this, arguments);
- return memo;
- };
-};
-
-var isOldIE = memoize(function () {
- // Test for IE <= 9 as proposed by Browserhacks
- // @see http://browserhacks.com/#hack-e71d8692f65334173fee715c222cb805
- // Tests for existence of standard globals is to allow style-loader
- // to operate correctly into non-standard environments
- // @see https://github.com/webpack-contrib/style-loader/issues/177
- return window && document && document.all && !window.atob;
-});
-
-var getElement = (function (fn) {
- var memo = {};
-
- return function(selector) {
- if (typeof memo[selector] === "undefined") {
- var styleTarget = fn.call(this, selector);
- // Special case to return head of iframe instead of iframe itself
- if (styleTarget instanceof window.HTMLIFrameElement) {
- try {
- // This will throw an exception if access to iframe is blocked
- // due to cross-origin restrictions
- styleTarget = styleTarget.contentDocument.head;
- } catch(e) {
- styleTarget = null;
- }
- }
- memo[selector] = styleTarget;
- }
- return memo[selector]
- };
-})(function (target) {
- return document.querySelector(target)
-});
-
-var singleton = null;
-var singletonCounter = 0;
-var stylesInsertedAtTop = [];
-
-var fixUrls = __webpack_require__(469);
-
-module.exports = function(list, options) {
- if (typeof DEBUG !== "undefined" && DEBUG) {
- if (typeof document !== "object") throw new Error("The style-loader cannot be used in a non-browser environment");
- }
-
- options = options || {};
-
- options.attrs = typeof options.attrs === "object" ? options.attrs : {};
-
- // Force single-tag solution on IE6-9, which has a hard limit on the # of